Lesson 27 Classes and Functions Python MiniCourse University
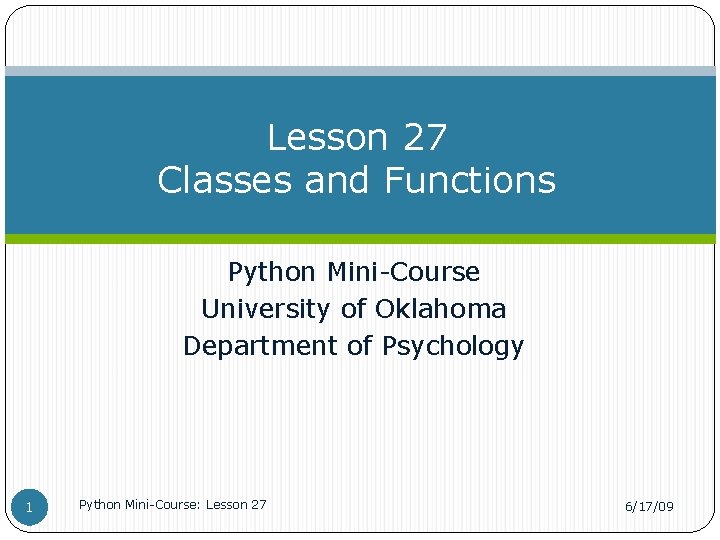
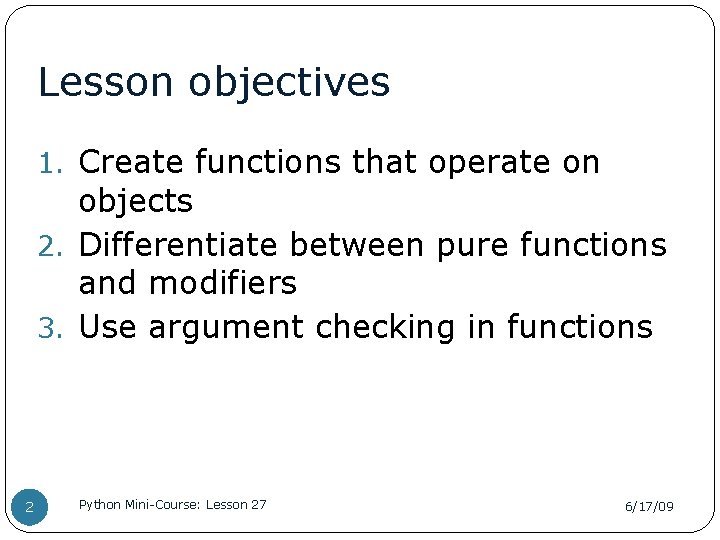
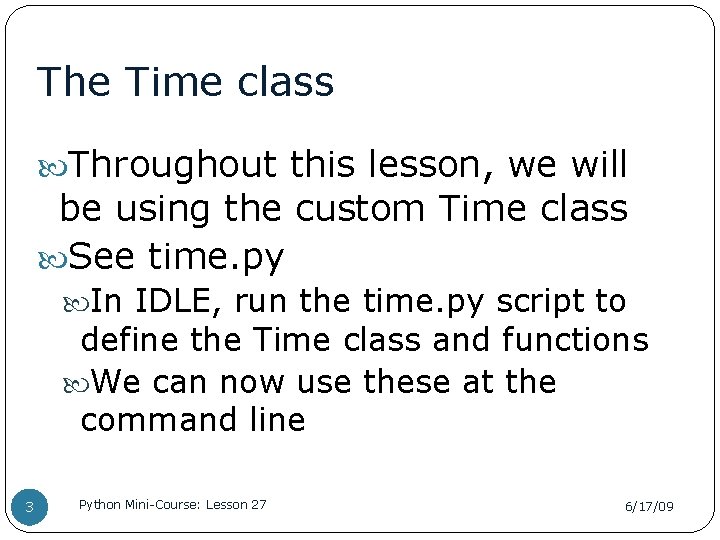
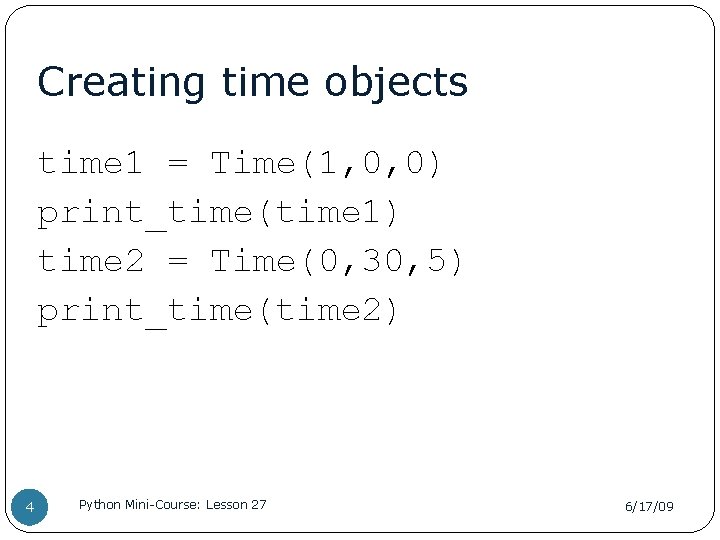
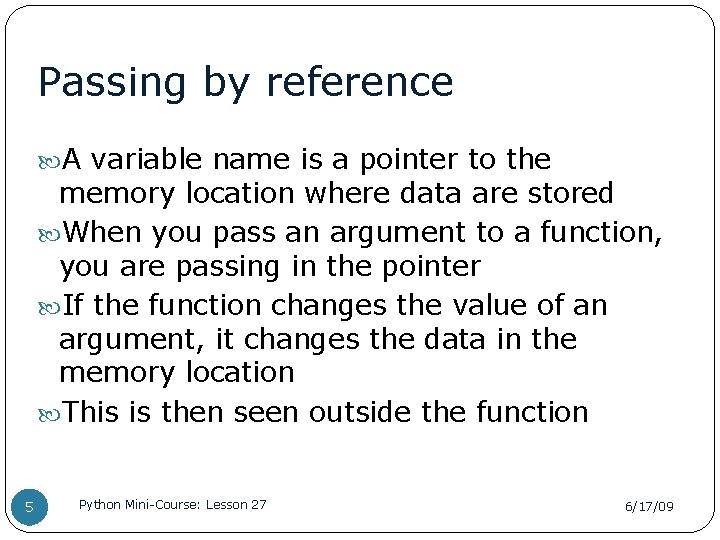
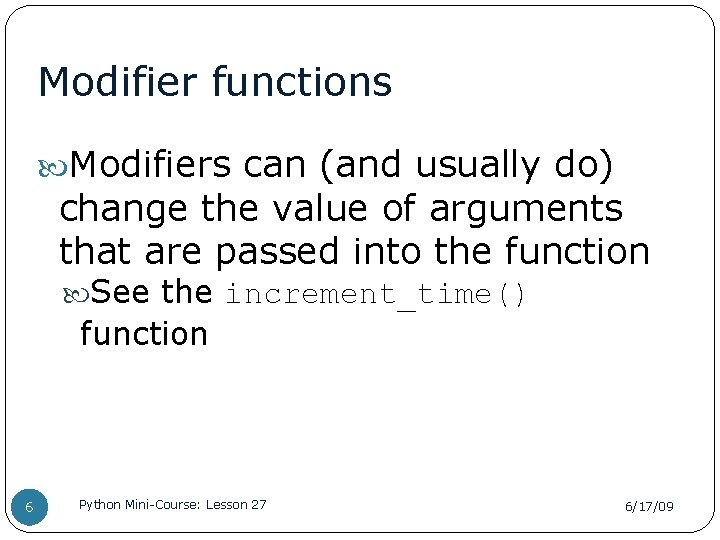
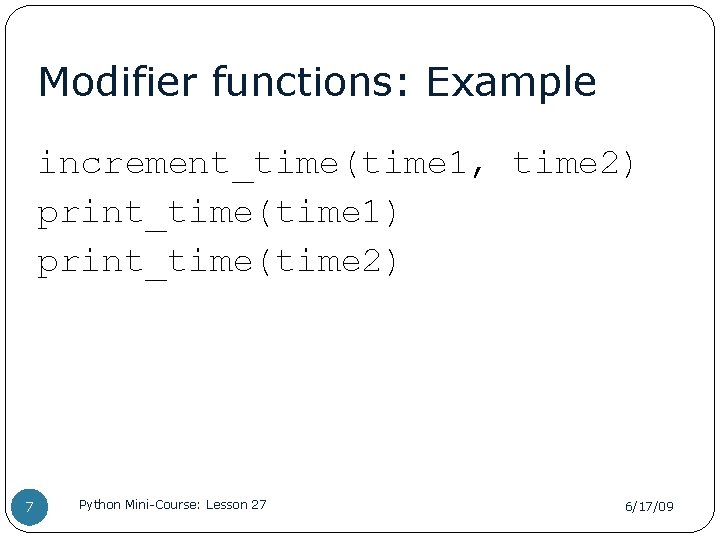
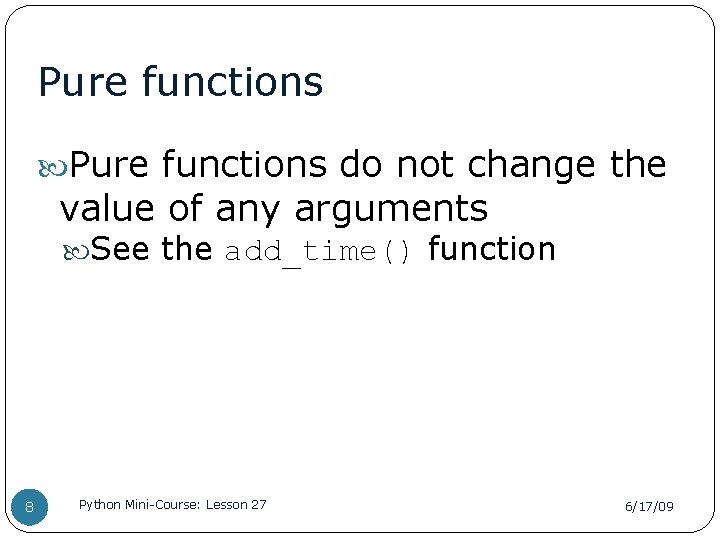
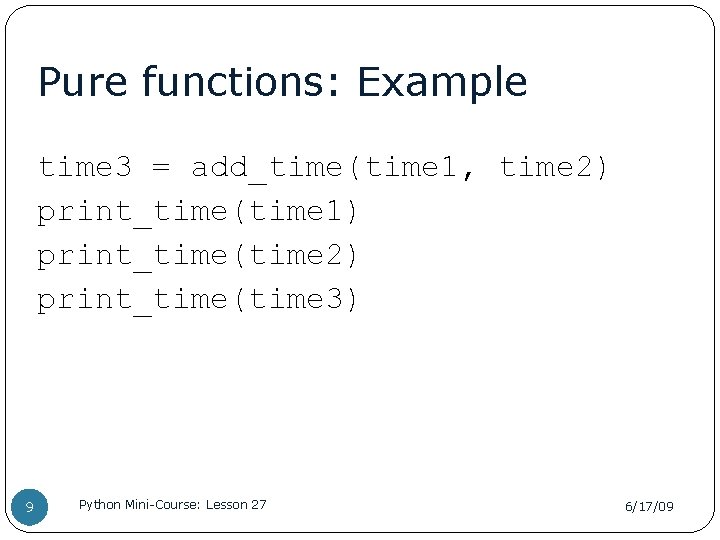
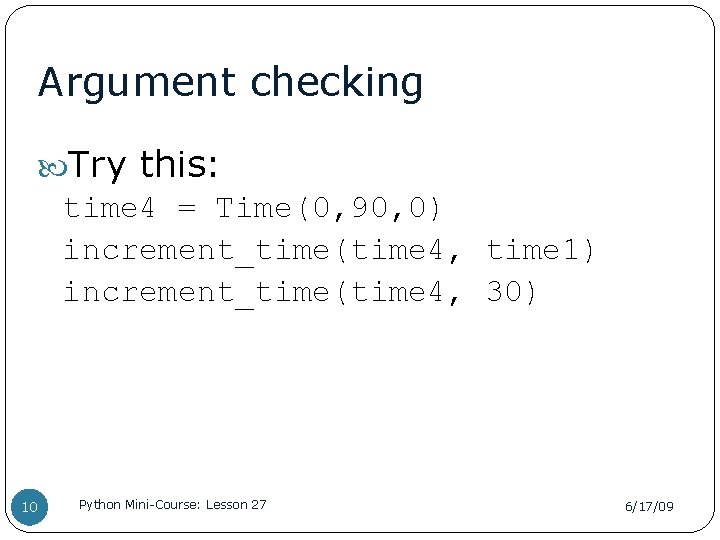
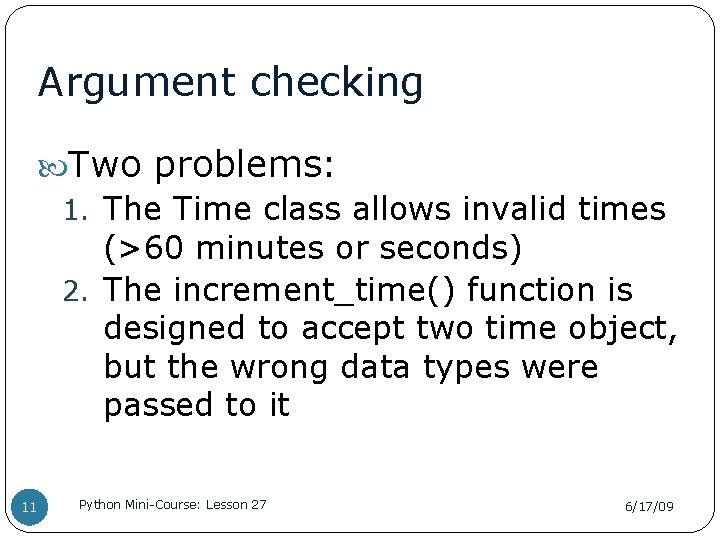
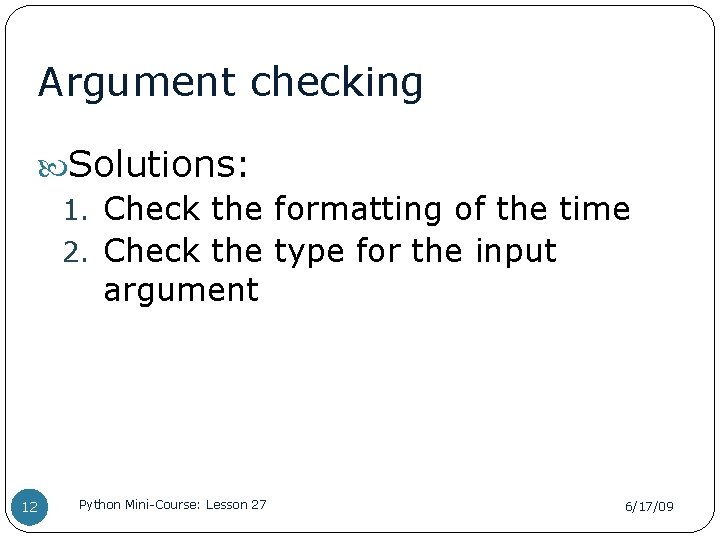
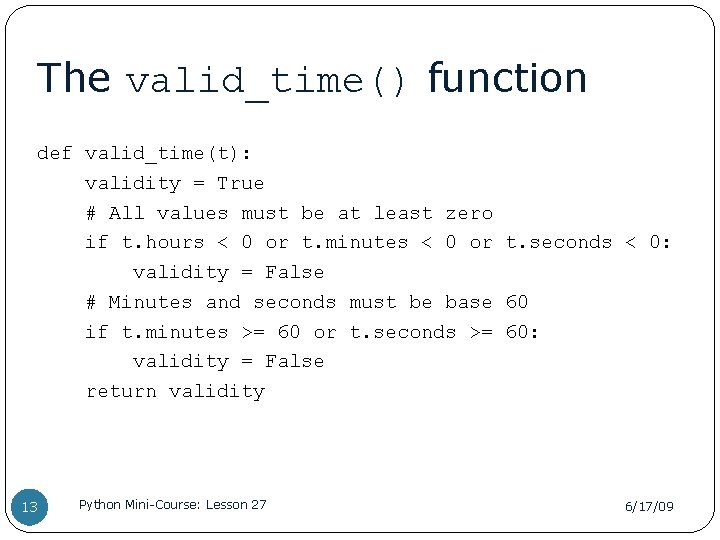
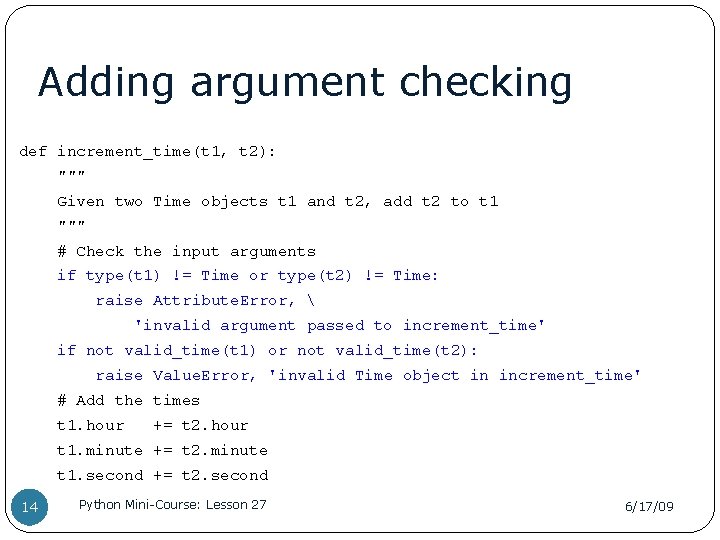
- Slides: 14
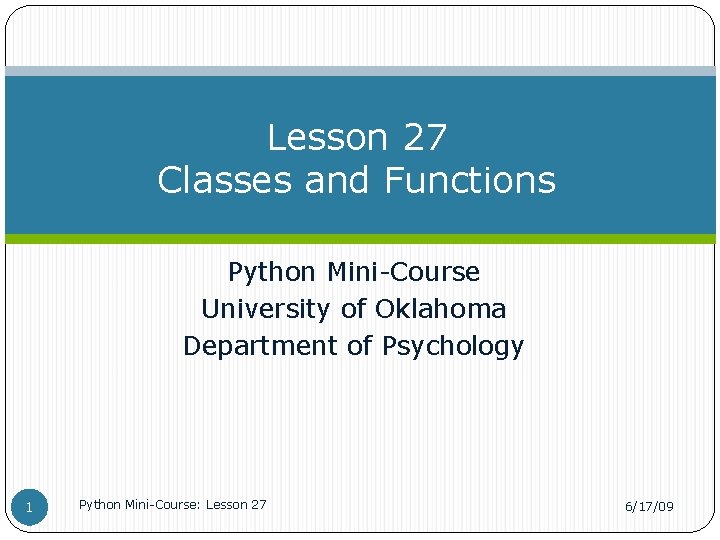
Lesson 27 Classes and Functions Python Mini-Course University of Oklahoma Department of Psychology 1 Python Mini-Course: Lesson 27 6/17/09
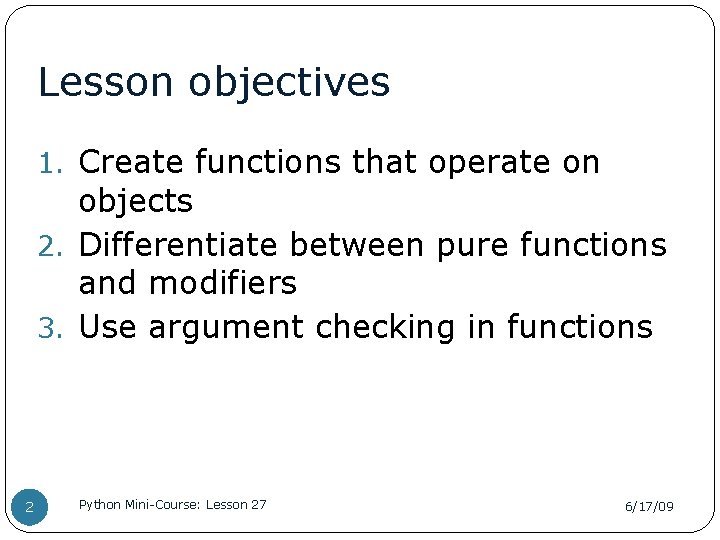
Lesson objectives 1. Create functions that operate on objects 2. Differentiate between pure functions and modifiers 3. Use argument checking in functions 2 Python Mini-Course: Lesson 27 6/17/09
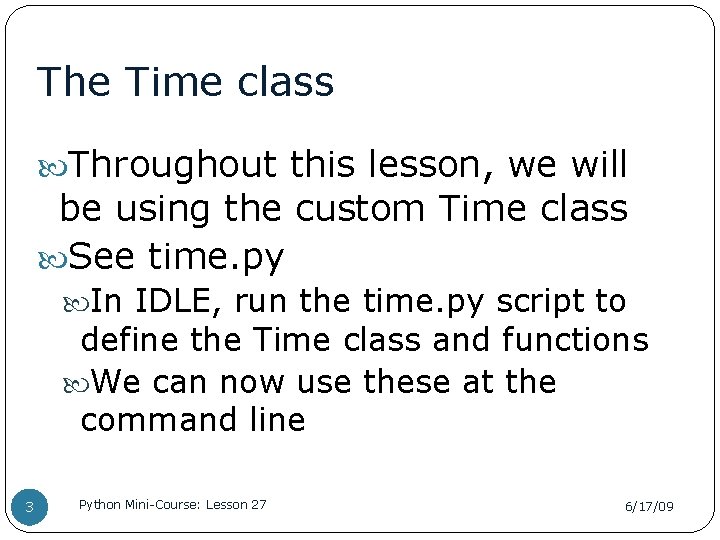
The Time class Throughout this lesson, we will be using the custom Time class See time. py In IDLE, run the time. py script to define the Time class and functions We can now use these at the command line 3 Python Mini-Course: Lesson 27 6/17/09
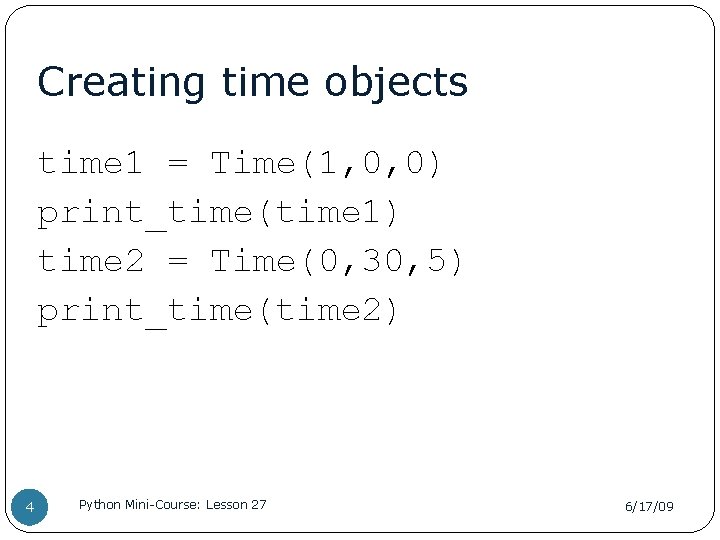
Creating time objects time 1 = Time(1, 0, 0) print_time(time 1) time 2 = Time(0, 30, 5) print_time(time 2) 4 Python Mini-Course: Lesson 27 6/17/09
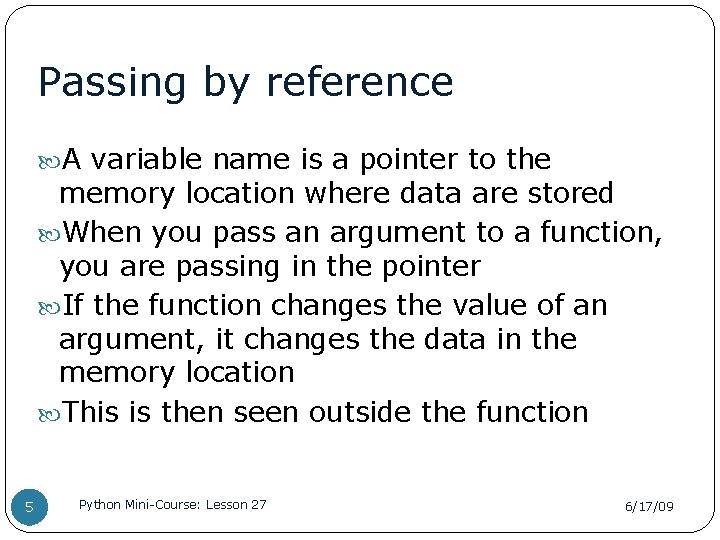
Passing by reference A variable name is a pointer to the memory location where data are stored When you pass an argument to a function, you are passing in the pointer If the function changes the value of an argument, it changes the data in the memory location This is then seen outside the function 5 Python Mini-Course: Lesson 27 6/17/09
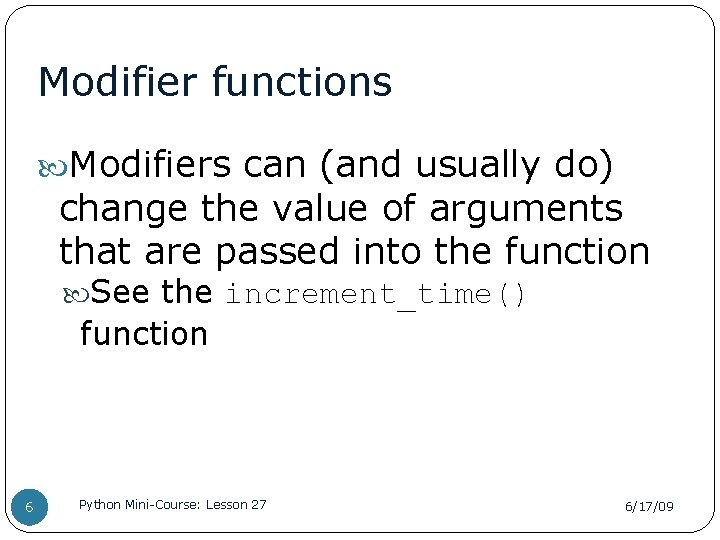
Modifier functions Modifiers can (and usually do) change the value of arguments that are passed into the function See the increment_time() function 6 Python Mini-Course: Lesson 27 6/17/09
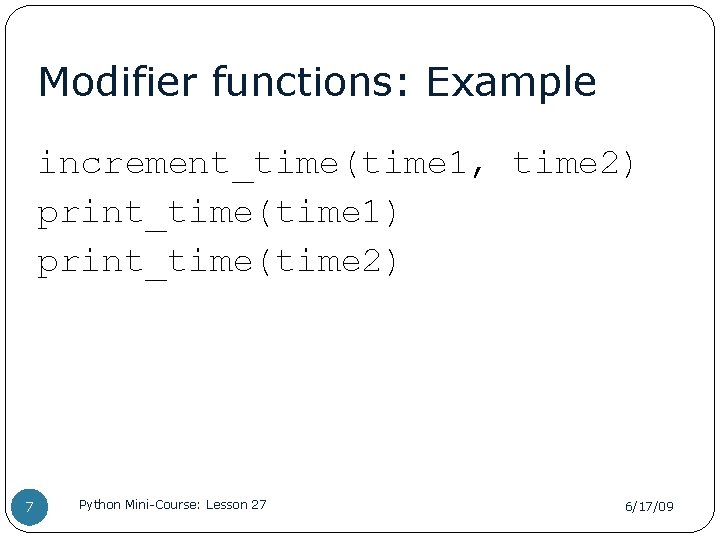
Modifier functions: Example increment_time(time 1, time 2) print_time(time 1) print_time(time 2) 7 Python Mini-Course: Lesson 27 6/17/09
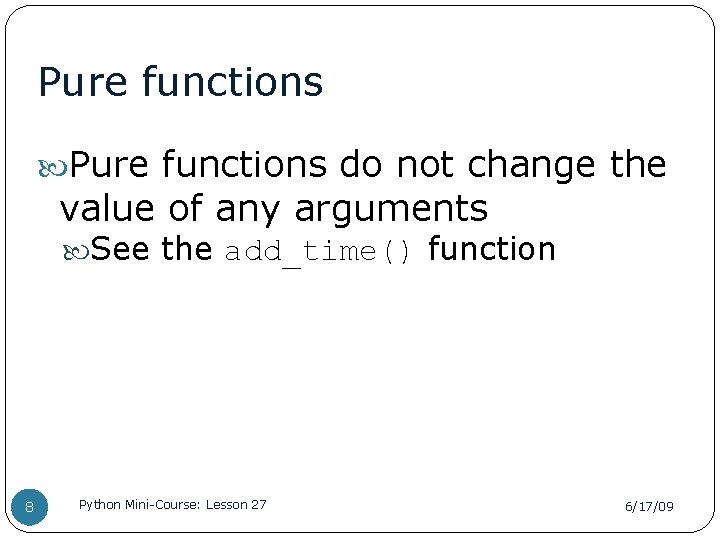
Pure functions do not change the value of any arguments See the add_time() function 8 Python Mini-Course: Lesson 27 6/17/09
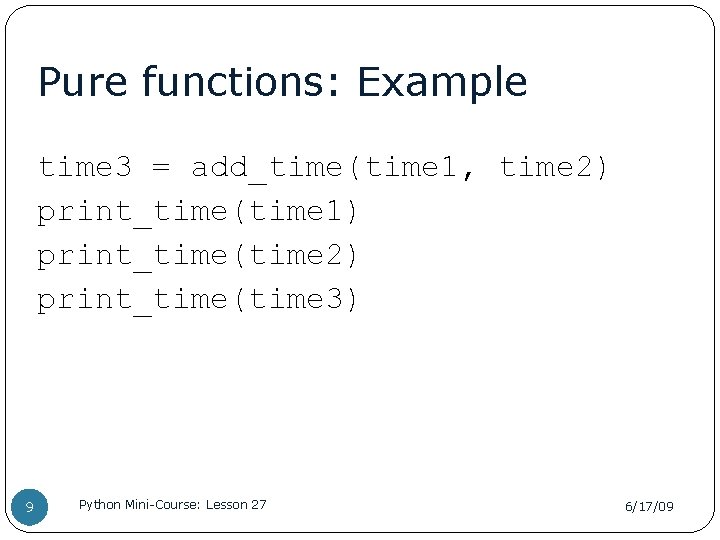
Pure functions: Example time 3 = add_time(time 1, time 2) print_time(time 1) print_time(time 2) print_time(time 3) 9 Python Mini-Course: Lesson 27 6/17/09
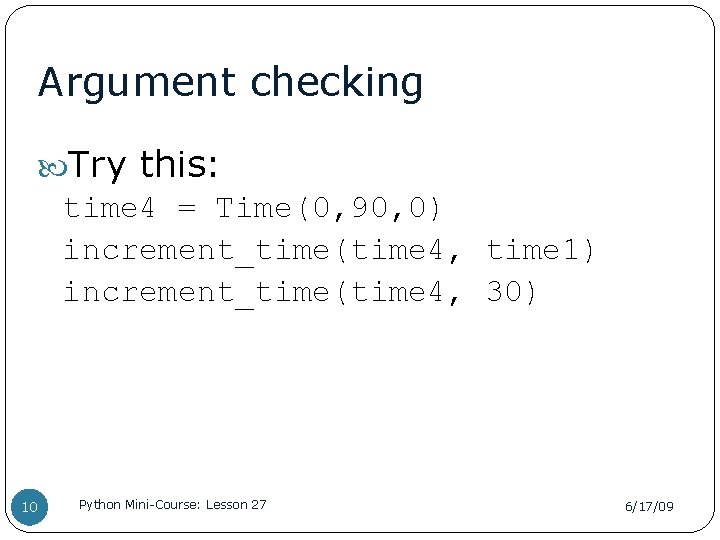
Argument checking Try this: time 4 = Time(0, 90, 0) increment_time(time 4, time 1) increment_time(time 4, 30) 10 Python Mini-Course: Lesson 27 6/17/09
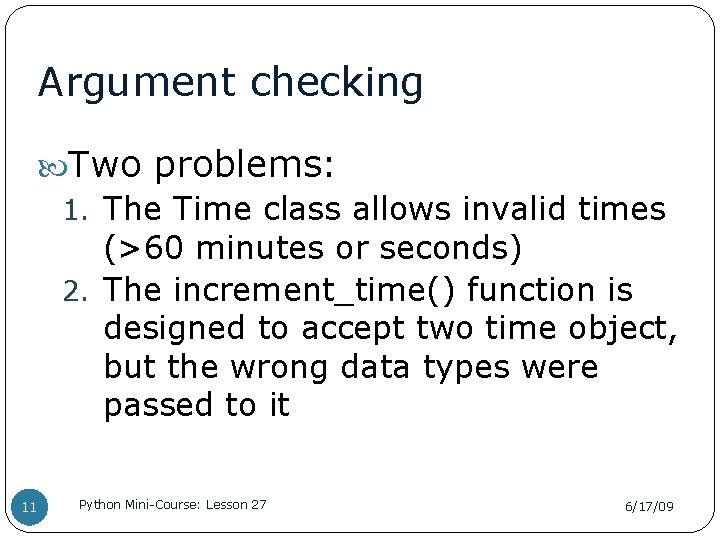
Argument checking Two problems: 1. The Time class allows invalid times (>60 minutes or seconds) 2. The increment_time() function is designed to accept two time object, but the wrong data types were passed to it 11 Python Mini-Course: Lesson 27 6/17/09
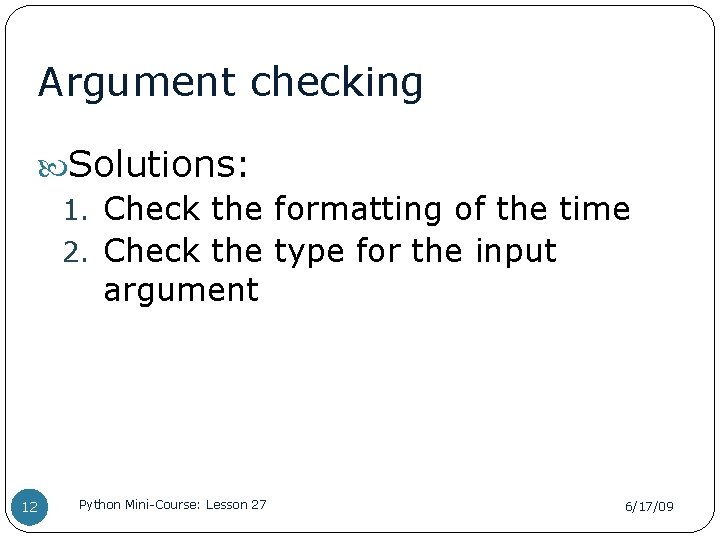
Argument checking Solutions: 1. Check the formatting of the time 2. Check the type for the input argument 12 Python Mini-Course: Lesson 27 6/17/09
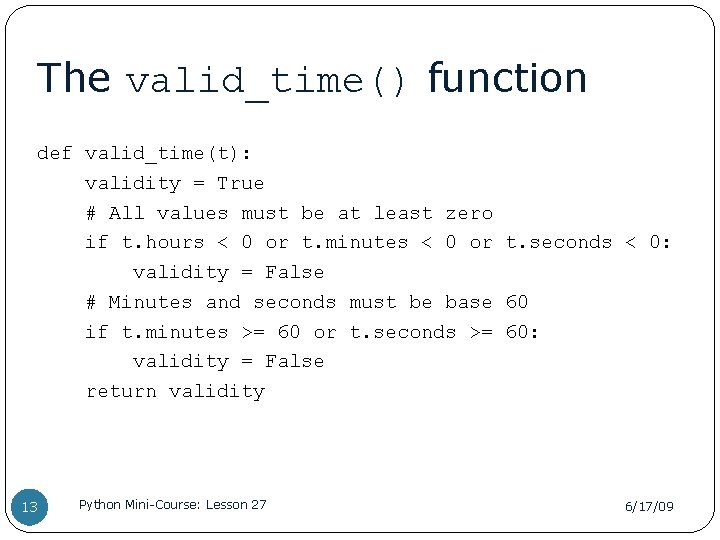
The valid_time() function def valid_time(t): validity = True # All values must be at least zero if t. hours < 0 or t. minutes < 0 or t. seconds < 0: validity = False # Minutes and seconds must be base 60 if t. minutes >= 60 or t. seconds >= 60: validity = False return validity 13 Python Mini-Course: Lesson 27 6/17/09
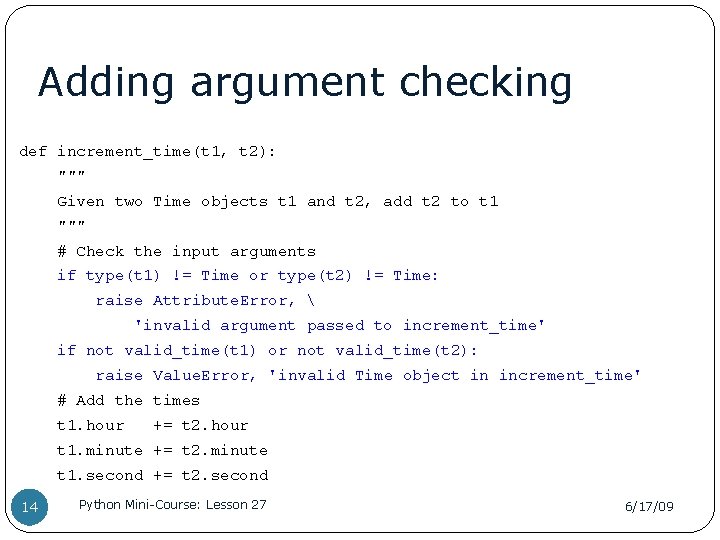
Adding argument checking def increment_time(t 1, t 2): """ Given two Time objects t 1 and t 2, add t 2 to t 1 """ # Check the input arguments if type(t 1) != Time or type(t 2) != Time: raise Attribute. Error, 'invalid argument passed to increment_time' if not valid_time(t 1) or not valid_time(t 2): raise Value. Error, 'invalid Time object in increment_time' # Add the times t 1. hour += t 2. hour t 1. minute += t 2. minute t 1. second += t 2. second 14 Python Mini-Course: Lesson 27 6/17/09