Python Aliasing Copyright Software Carpentry 2010 This work
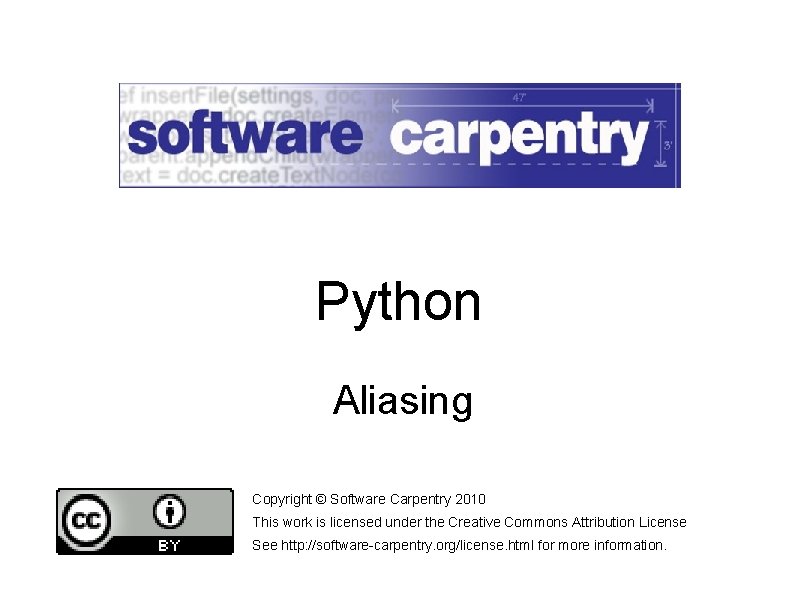
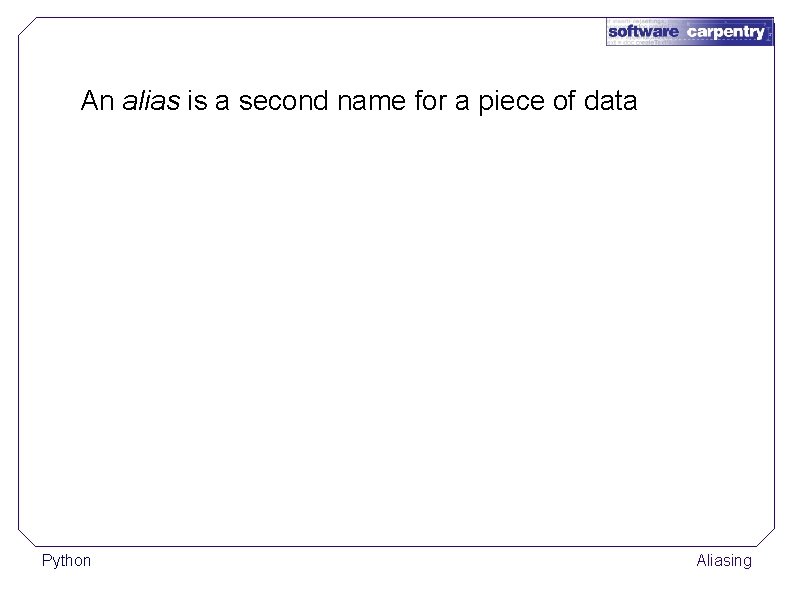
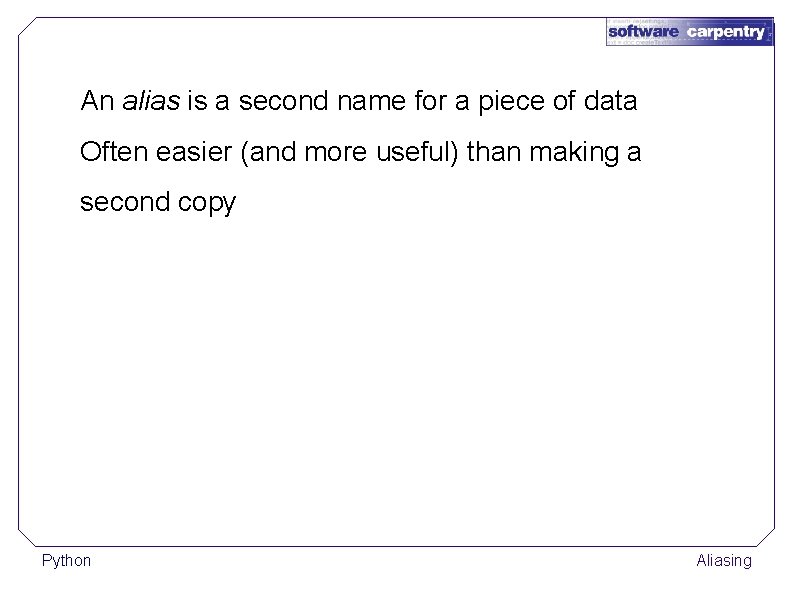
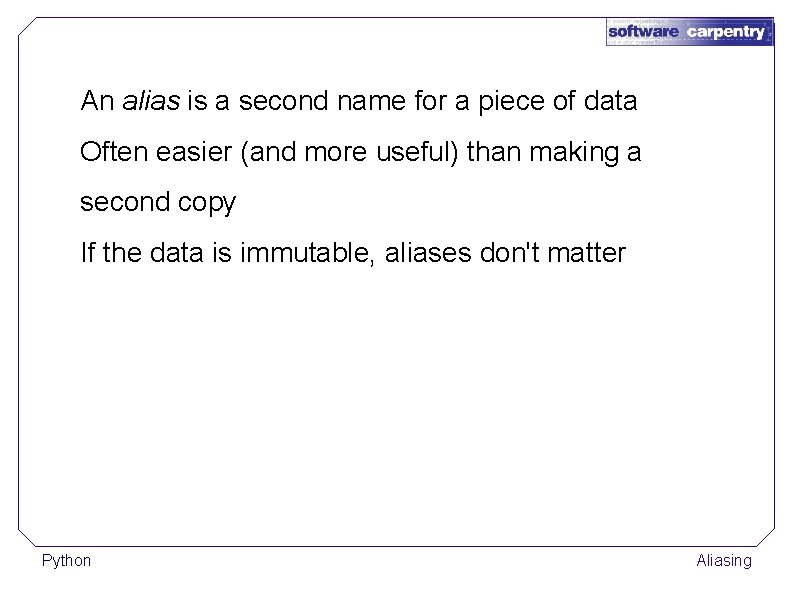
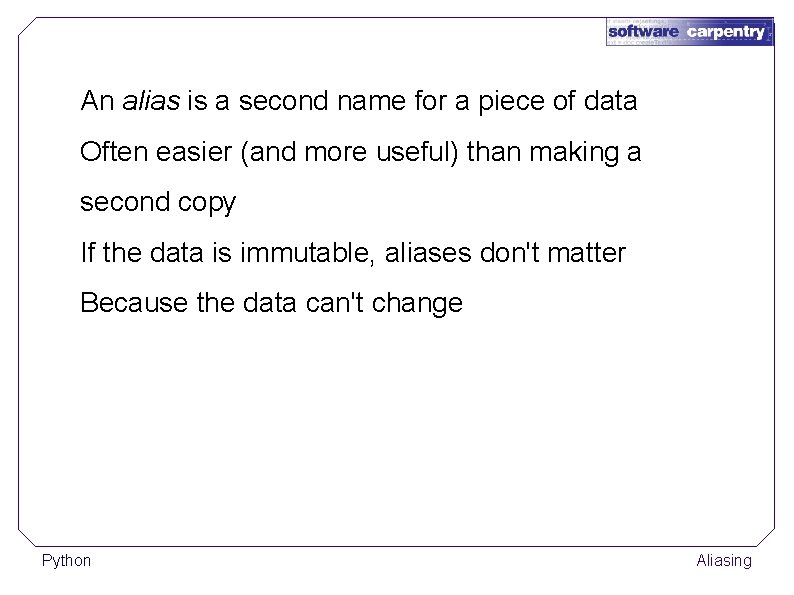
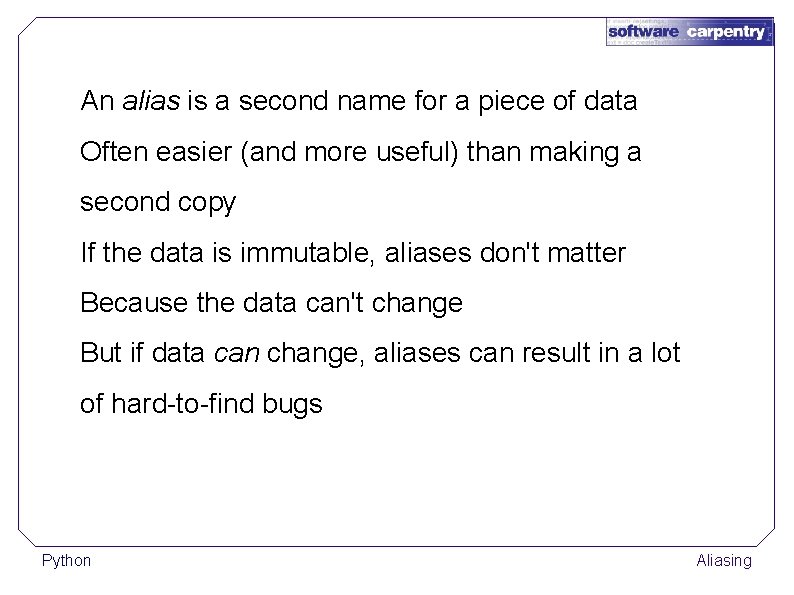
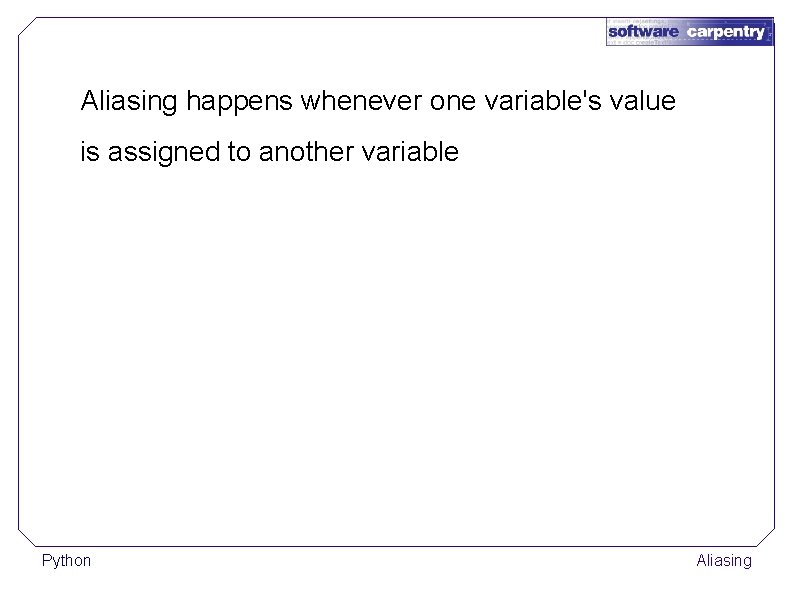
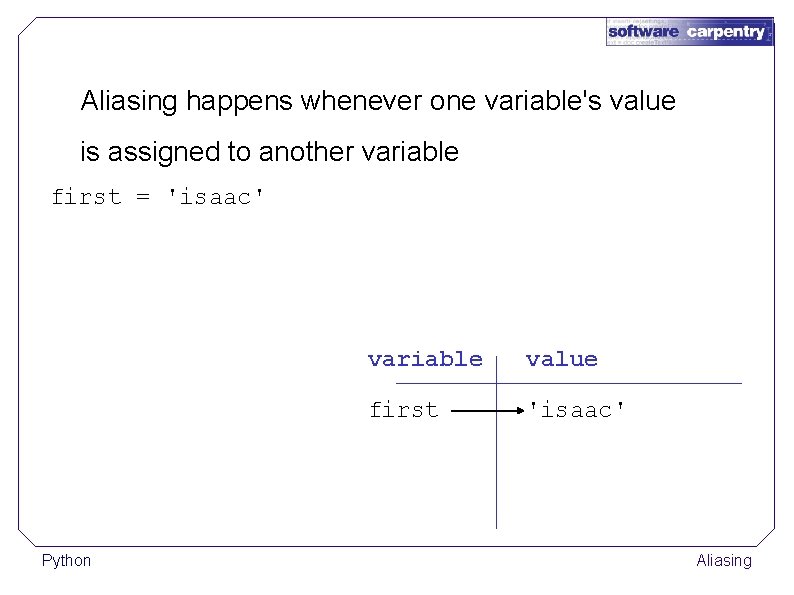
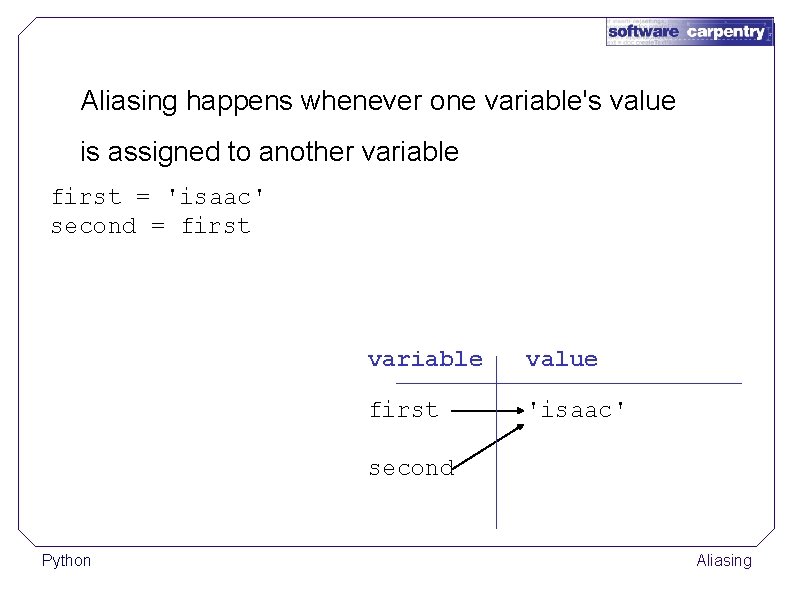
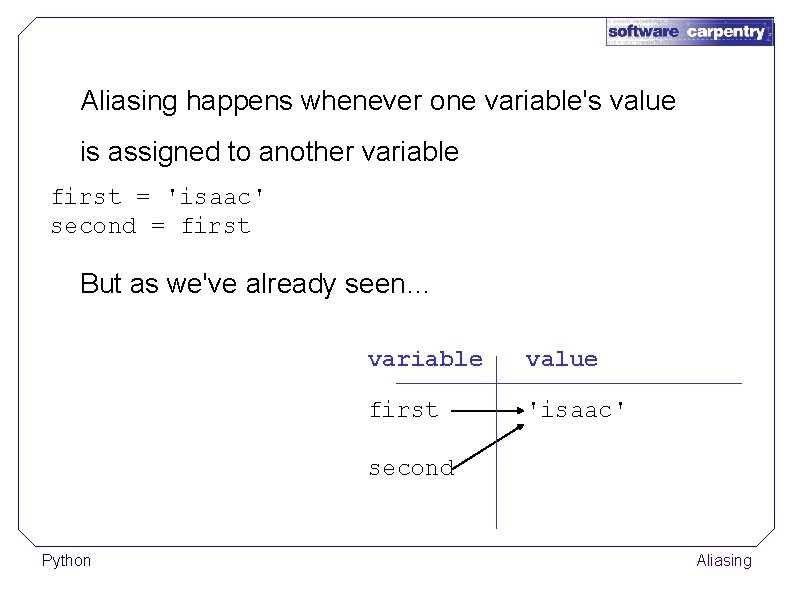
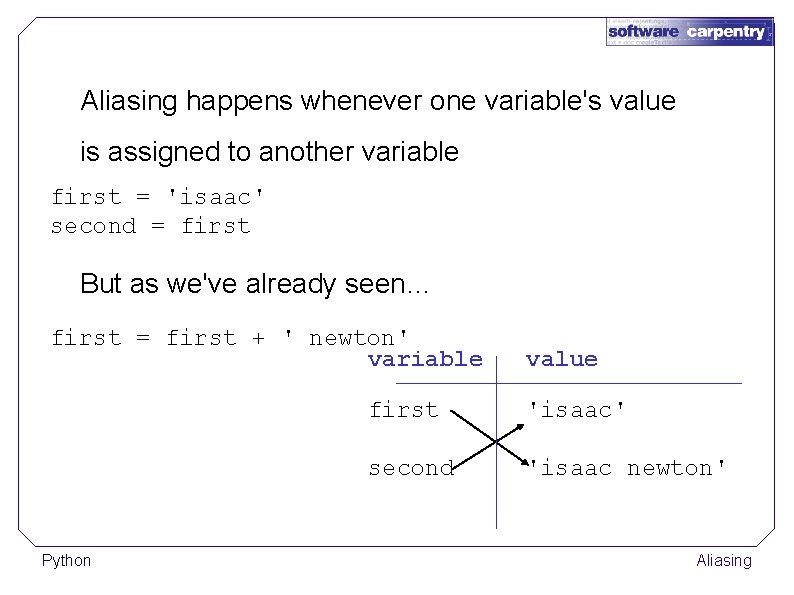
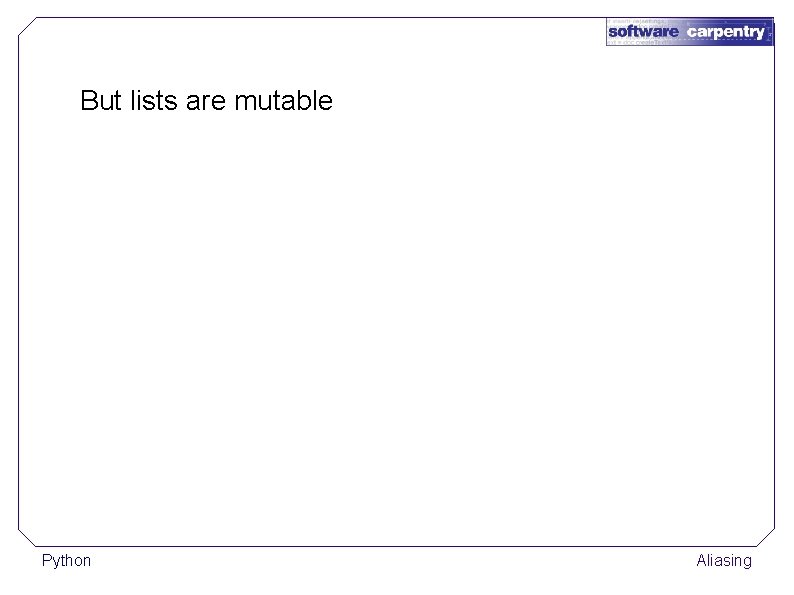
![But lists are mutable first = ['isaac'] variable value first 'isaac' Python Aliasing But lists are mutable first = ['isaac'] variable value first 'isaac' Python Aliasing](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-13.jpg)
![But lists are mutable first = ['isaac'] second = first variable value first second But lists are mutable first = ['isaac'] second = first variable value first second](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-14.jpg)
![But lists are mutable first = ['isaac'] second = first. append('newton') print first ['isaac', But lists are mutable first = ['isaac'] second = first. append('newton') print first ['isaac',](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-15.jpg)
![But lists are mutable first = ['isaac'] second = first. append('newton') print first ['isaac', But lists are mutable first = ['isaac'] second = first. append('newton') print first ['isaac',](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-16.jpg)
![But lists are mutable first = ['isaac'] second = first. append('newton') print first ['isaac', But lists are mutable first = ['isaac'] second = first. append('newton') print first ['isaac',](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-17.jpg)
![But lists are mutable first = ['isaac'] second = first. append('newton') print first ['isaac', But lists are mutable first = ['isaac'] second = first. append('newton') print first ['isaac',](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-18.jpg)
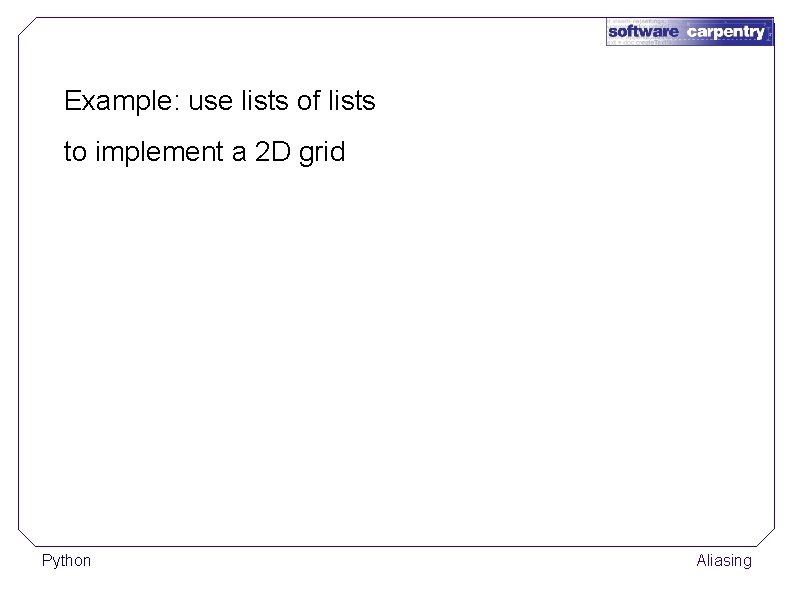
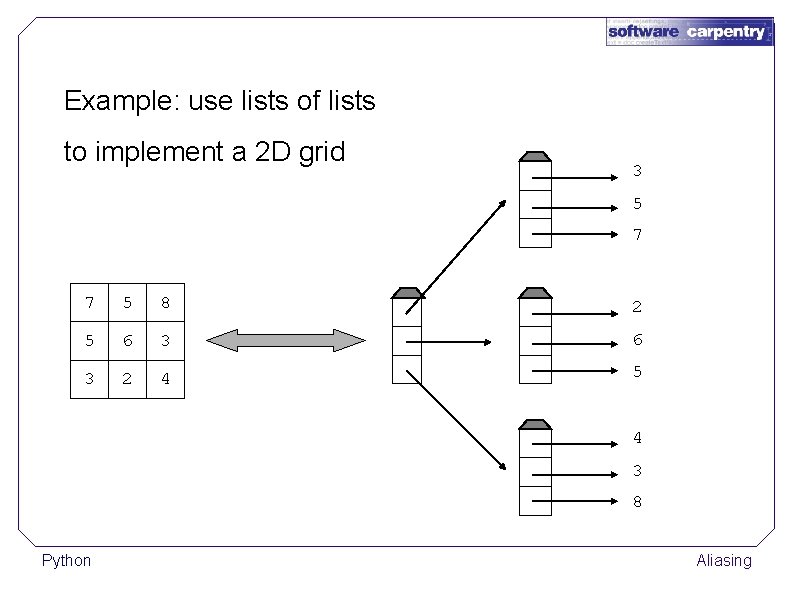
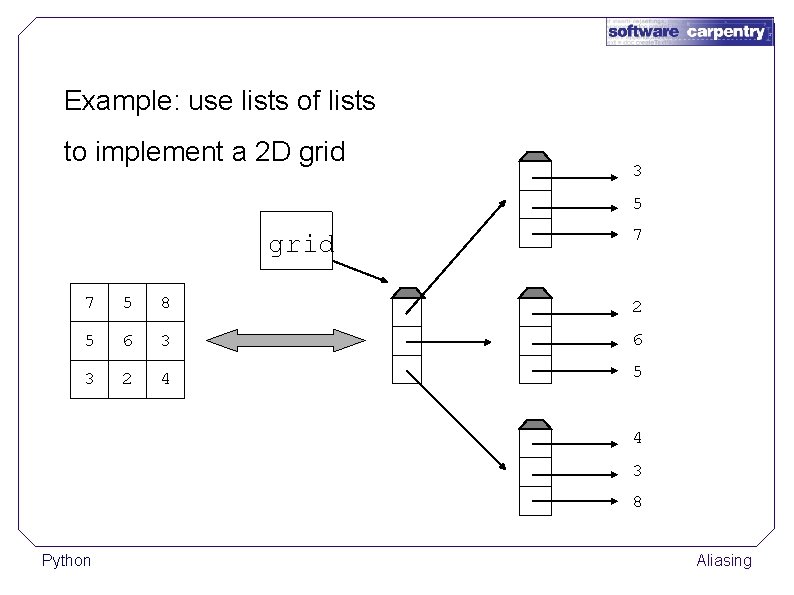
![Example: use lists of lists to implement a 2 D grid 3 5 grid[0] Example: use lists of lists to implement a 2 D grid 3 5 grid[0]](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-22.jpg)
![Example: use lists of lists to implement a 2 D grid 3 5 grid[0][1] Example: use lists of lists to implement a 2 D grid 3 5 grid[0][1]](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-23.jpg)
![# Correct code grid = [] for x in range(N): temp = [] for # Correct code grid = [] for x in range(N): temp = [] for](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-24.jpg)
![# Correct code grid = [] Outer "spine" of structure for x in range(N): # Correct code grid = [] Outer "spine" of structure for x in range(N):](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-25.jpg)
![# Correct code grid = [] for x in range(N): temp = [] for # Correct code grid = [] for x in range(N): temp = [] for](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-26.jpg)
![# Correct code grid = [] for x in range(N): temp = [] for # Correct code grid = [] for x in range(N): temp = [] for](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-27.jpg)
![# Equivalent code grid = [] for x in range(N): grid. append([]) for y # Equivalent code grid = [] for x in range(N): grid. append([]) for y](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-28.jpg)
![# Equivalent code grid = [] for x in range(N): grid. append([]) for y # Equivalent code grid = [] for x in range(N): grid. append([]) for y](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-29.jpg)
![# Incorrect code grid = [] EMPTY = [] for x in range(N): grid. # Incorrect code grid = [] EMPTY = [] for x in range(N): grid.](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-30.jpg)
![# Incorrect code grid = [] EMPTY = [] for x in range(N): grid. # Incorrect code grid = [] EMPTY = [] for x in range(N): grid.](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-31.jpg)
![# Incorrect code grid = [] EMPTY = [] for x in range(N): grid. # Incorrect code grid = [] EMPTY = [] for x in range(N): grid.](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-32.jpg)
![variable x grid value 0 grid = [] EMPTY = [] for x in variable x grid value 0 grid = [] EMPTY = [] for x in](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-33.jpg)
![variable x grid value 0 grid = [] EMPTY = [] for x in variable x grid value 0 grid = [] EMPTY = [] for x in](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-34.jpg)
![variable value x 0 y 0 grid = [] EMPTY = [] for x variable value x 0 y 0 grid = [] EMPTY = [] for x](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-35.jpg)
![variable value x 0 y 0 grid = [] EMPTY = [] for x variable value x 0 y 0 grid = [] EMPTY = [] for x](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-36.jpg)
![variable value x 0 y 2 grid = [] EMPTY = [] for x variable value x 0 y 2 grid = [] EMPTY = [] for x](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-37.jpg)
![variable value x 1 y 2 grid = [] EMPTY = [] for x variable value x 1 y 2 grid = [] EMPTY = [] for x](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-38.jpg)
![variable value x 1 y 2 grid = [] EMPTY = [] for x variable value x 1 y 2 grid = [] EMPTY = [] for x](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-39.jpg)
![No Aliasing first = [] second = [] Python Aliasing No Aliasing first = [] second = [] Python Aliasing](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-40.jpg)
![Python No Aliasing first = [] second = first Aliasing Python No Aliasing first = [] second = first Aliasing](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-41.jpg)
![variable x grid Python value 0 grid = [] for x in range(N): grid. variable x grid Python value 0 grid = [] for x in range(N): grid.](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-42.jpg)
![variable x grid Python value 0 grid = [] for x in range(N): grid. variable x grid Python value 0 grid = [] for x in range(N): grid.](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-43.jpg)
![variable value x 0 y 2 grid = [] for x in range(N): grid. variable value x 0 y 2 grid = [] for x in range(N): grid.](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-44.jpg)
![variable value x 1 y 2 grid = [] for x in range(N): grid. variable value x 1 y 2 grid = [] for x in range(N): grid.](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-45.jpg)
![variable value x 1 y 0 grid = [] for x in range(N): grid. variable value x 1 y 0 grid = [] for x in range(N): grid.](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-46.jpg)
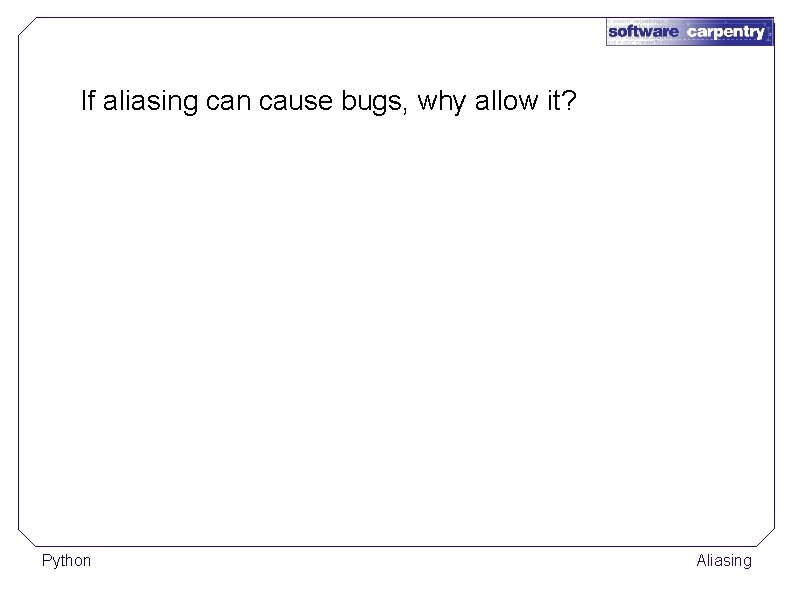
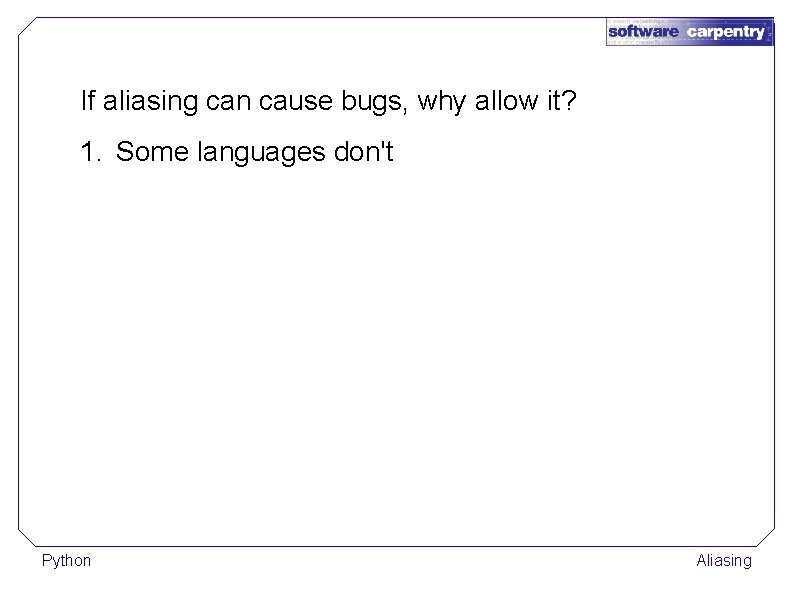
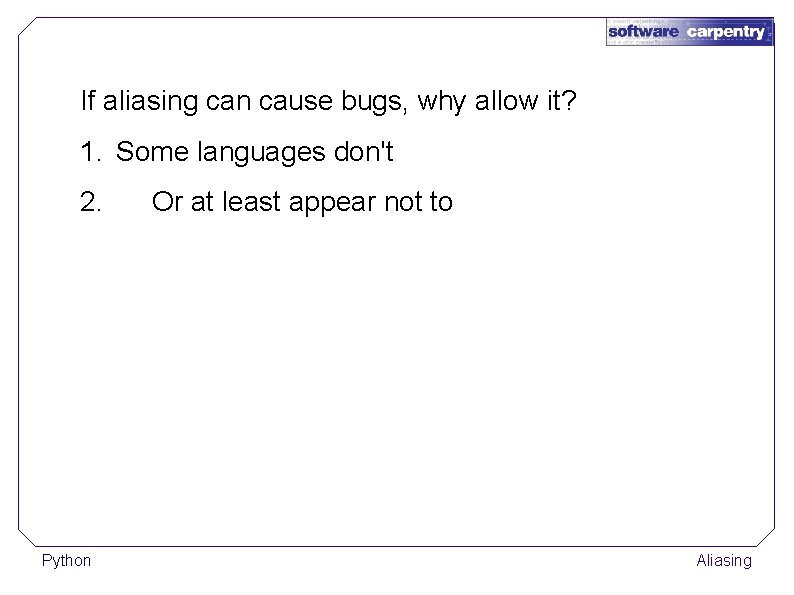
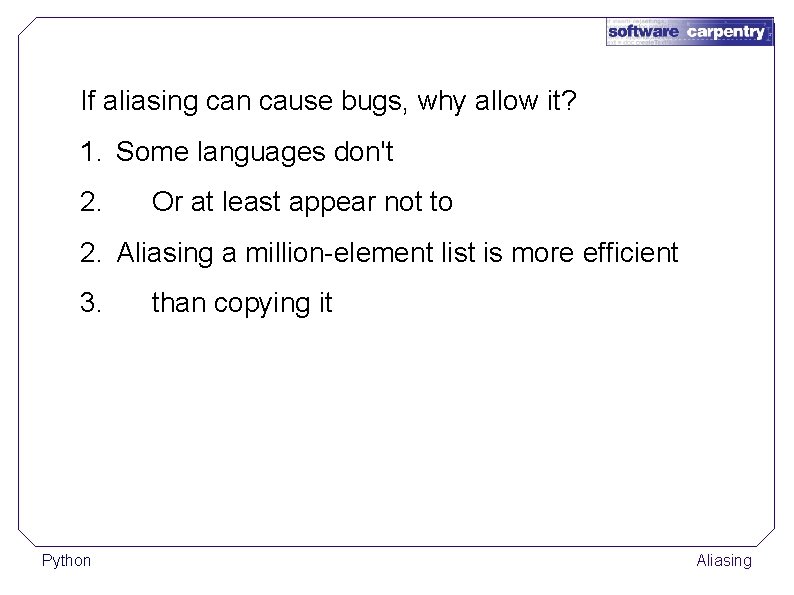
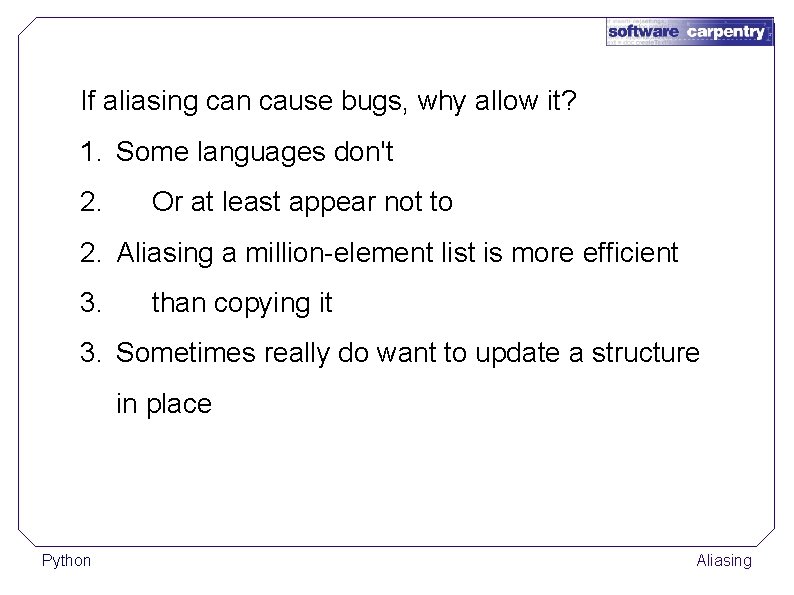
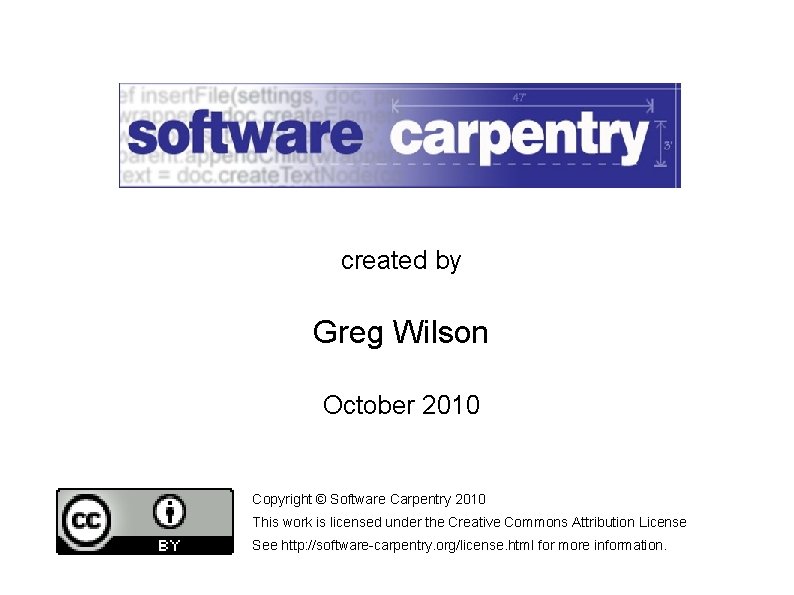
- Slides: 52
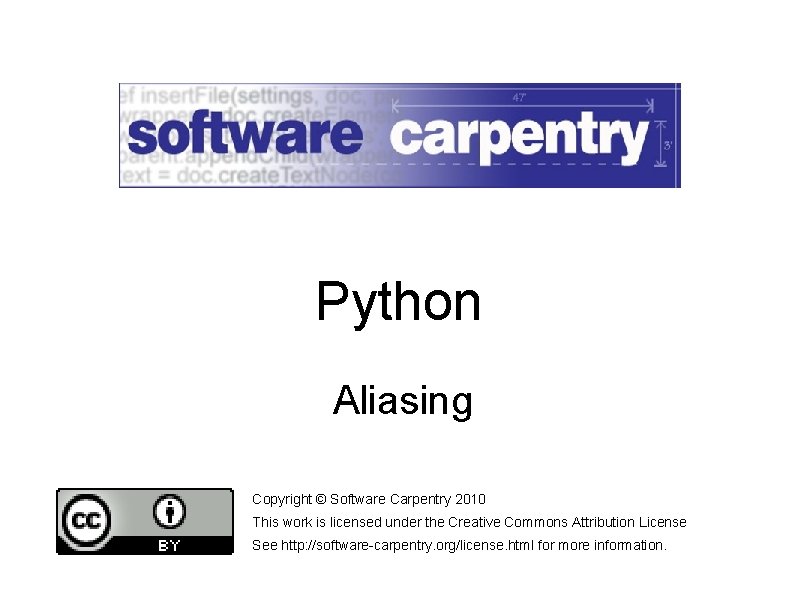
Python Aliasing Copyright © Software Carpentry 2010 This work is licensed under the Creative Commons Attribution License See http: //software-carpentry. org/license. html for more information.
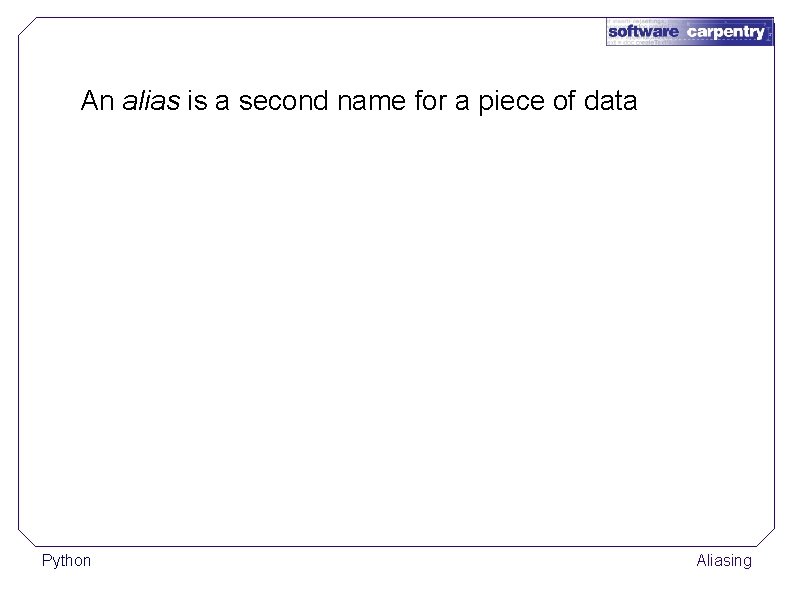
An alias is a second name for a piece of data Python Aliasing
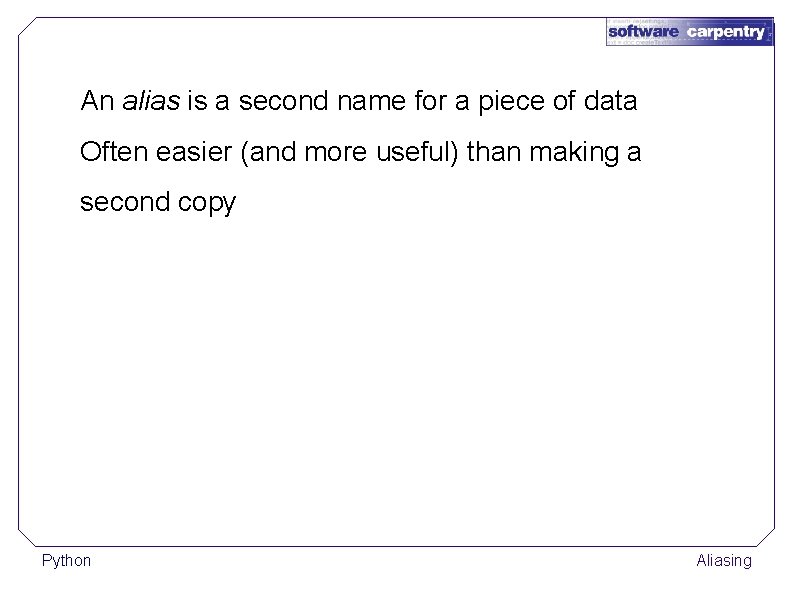
An alias is a second name for a piece of data Often easier (and more useful) than making a second copy Python Aliasing
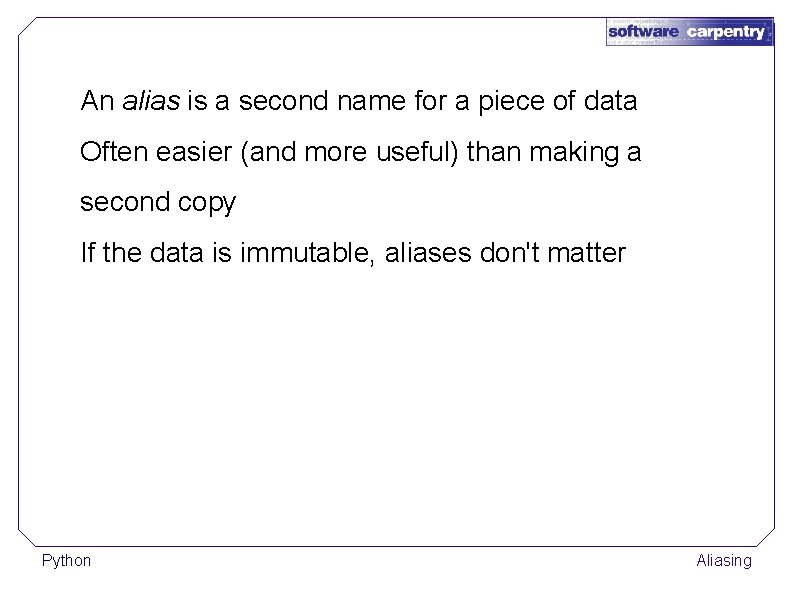
An alias is a second name for a piece of data Often easier (and more useful) than making a second copy If the data is immutable, aliases don't matter Python Aliasing
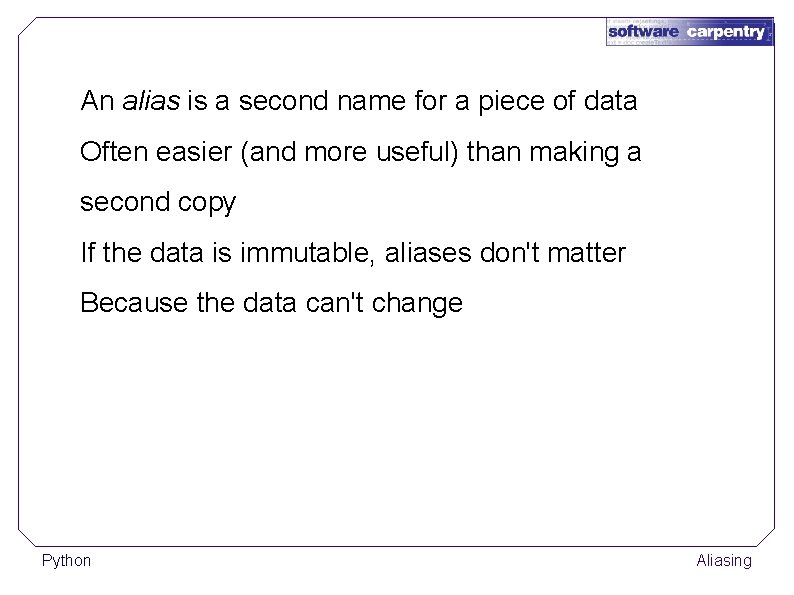
An alias is a second name for a piece of data Often easier (and more useful) than making a second copy If the data is immutable, aliases don't matter Because the data can't change Python Aliasing
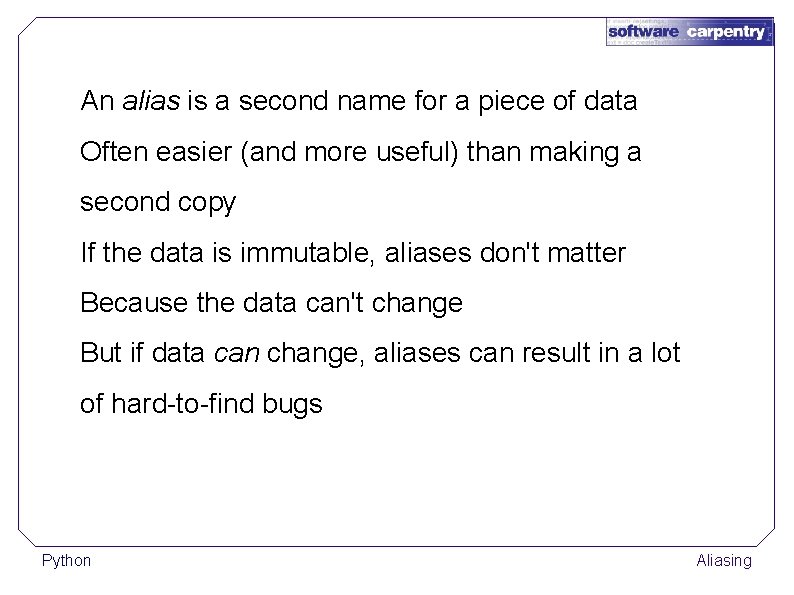
An alias is a second name for a piece of data Often easier (and more useful) than making a second copy If the data is immutable, aliases don't matter Because the data can't change But if data can change, aliases can result in a lot of hard-to-find bugs Python Aliasing
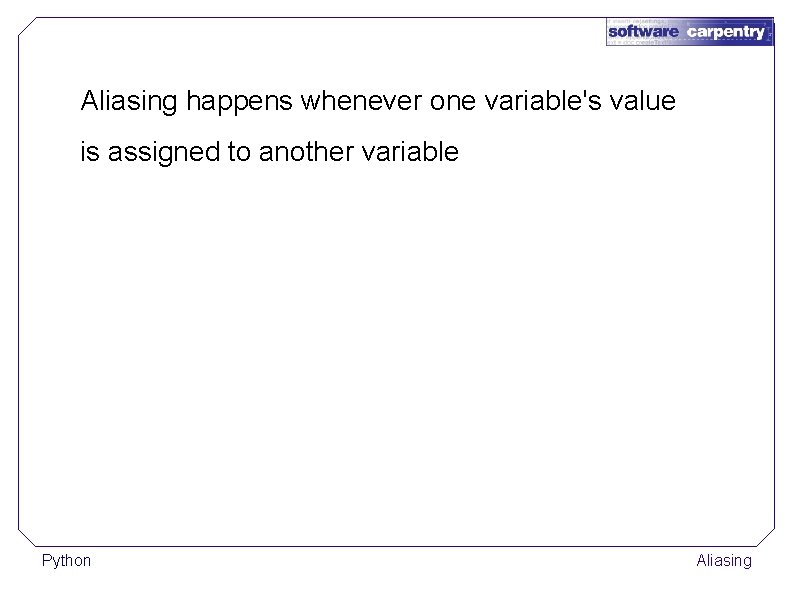
Aliasing happens whenever one variable's value is assigned to another variable Python Aliasing
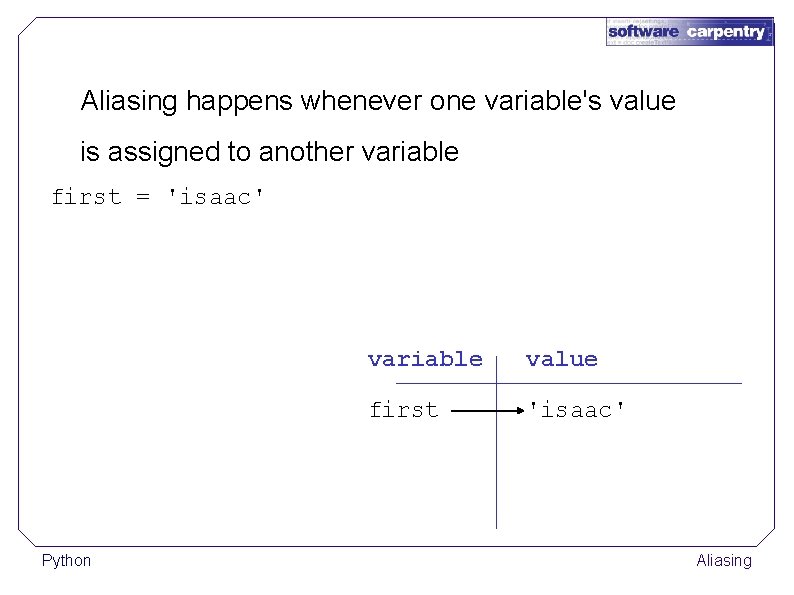
Aliasing happens whenever one variable's value is assigned to another variable first = 'isaac' Python variable value first 'isaac' Aliasing
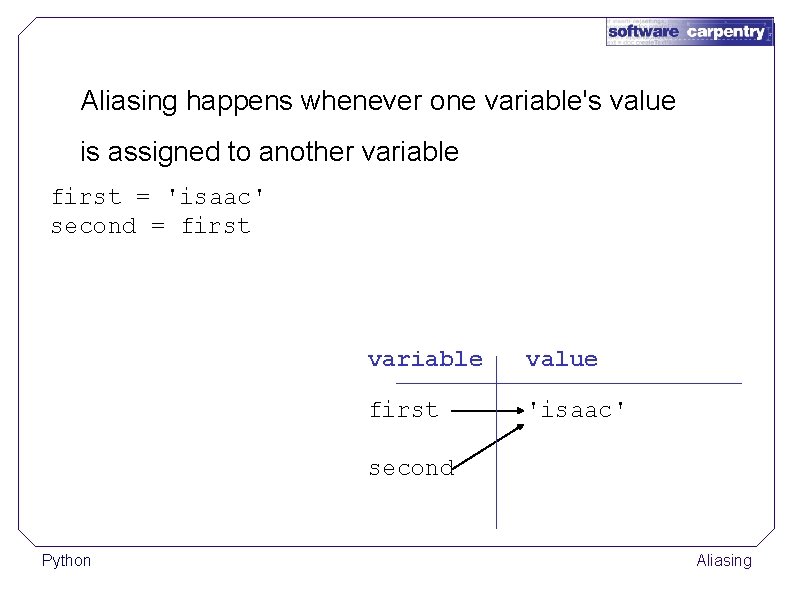
Aliasing happens whenever one variable's value is assigned to another variable first = 'isaac' second = first variable value first 'isaac' second Python Aliasing
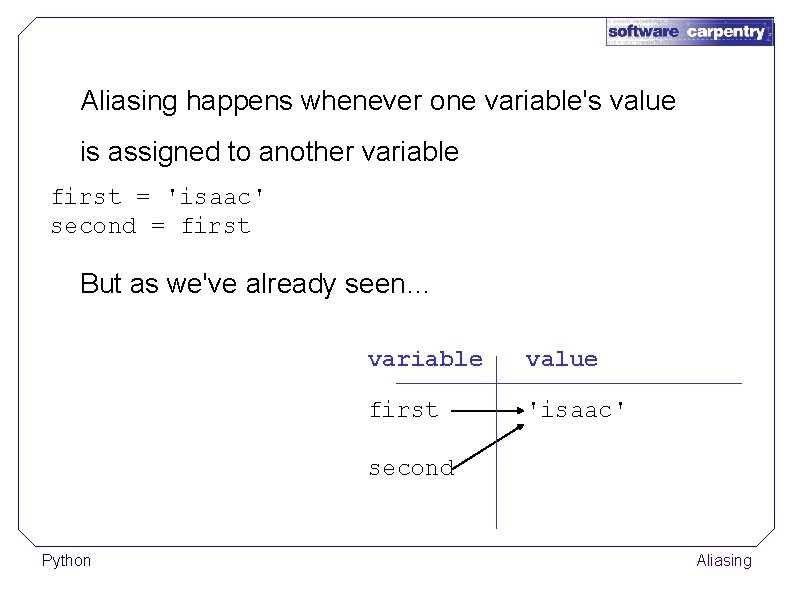
Aliasing happens whenever one variable's value is assigned to another variable first = 'isaac' second = first But as we've already seen… variable value first 'isaac' second Python Aliasing
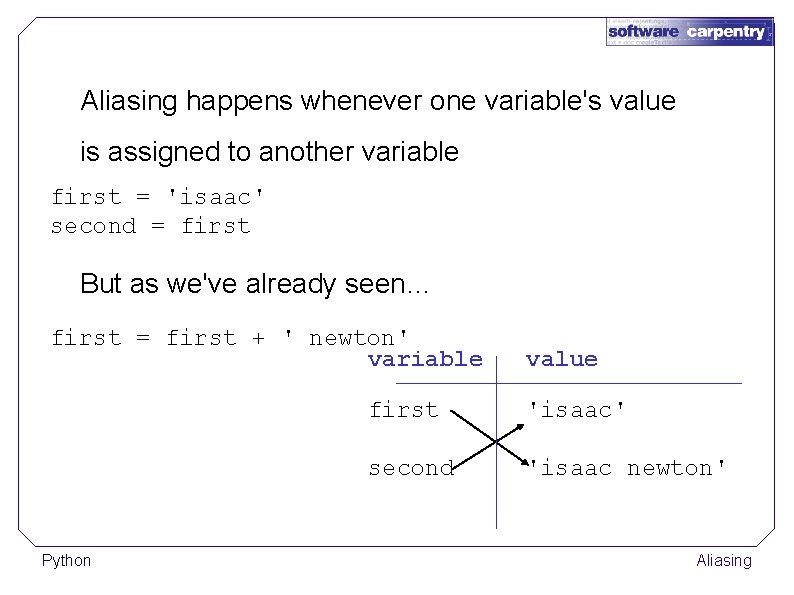
Aliasing happens whenever one variable's value is assigned to another variable first = 'isaac' second = first But as we've already seen… first = first + ' newton' variable Python value first 'isaac' second 'isaac newton' Aliasing
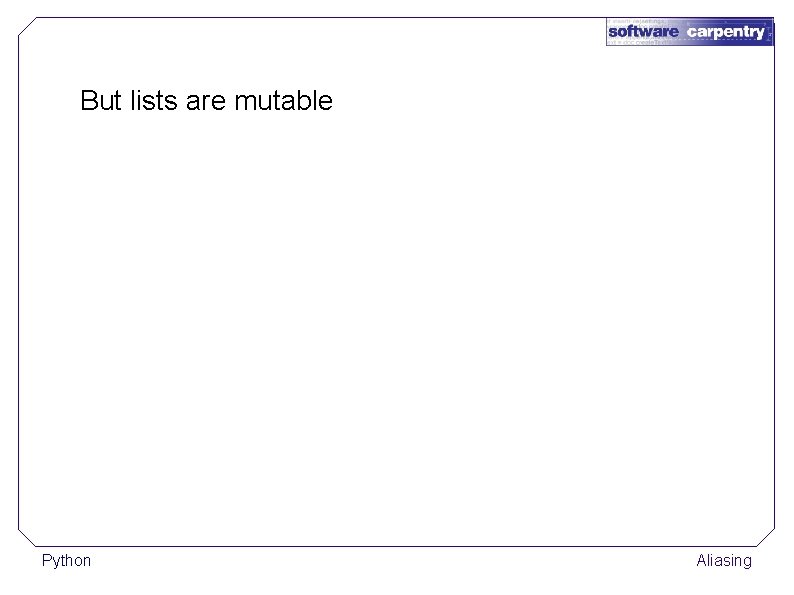
But lists are mutable Python Aliasing
![But lists are mutable first isaac variable value first isaac Python Aliasing But lists are mutable first = ['isaac'] variable value first 'isaac' Python Aliasing](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-13.jpg)
But lists are mutable first = ['isaac'] variable value first 'isaac' Python Aliasing
![But lists are mutable first isaac second first variable value first second But lists are mutable first = ['isaac'] second = first variable value first second](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-14.jpg)
But lists are mutable first = ['isaac'] second = first variable value first second 'isaac' Python Aliasing
![But lists are mutable first isaac second first appendnewton print first isaac But lists are mutable first = ['isaac'] second = first. append('newton') print first ['isaac',](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-15.jpg)
But lists are mutable first = ['isaac'] second = first. append('newton') print first ['isaac', 'newton'] variable value first second 'isaac' Python 'newton' Aliasing
![But lists are mutable first isaac second first appendnewton print first isaac But lists are mutable first = ['isaac'] second = first. append('newton') print first ['isaac',](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-16.jpg)
But lists are mutable first = ['isaac'] second = first. append('newton') print first ['isaac', 'newton'] print second variable ['isaac', 'newton'] value first second 'isaac' Python 'newton' Aliasing
![But lists are mutable first isaac second first appendnewton print first isaac But lists are mutable first = ['isaac'] second = first. append('newton') print first ['isaac',](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-17.jpg)
But lists are mutable first = ['isaac'] second = first. append('newton') print first ['isaac', 'newton'] print second variable ['isaac', 'newton'] value first Didn't explicitly modify second Python second 'isaac' 'newton' Aliasing
![But lists are mutable first isaac second first appendnewton print first isaac But lists are mutable first = ['isaac'] second = first. append('newton') print first ['isaac',](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-18.jpg)
But lists are mutable first = ['isaac'] second = first. append('newton') print first ['isaac', 'newton'] print second variable ['isaac', 'newton'] value first Didn't explicitly modify second 'isaac' 'newton' A side effect Python Aliasing
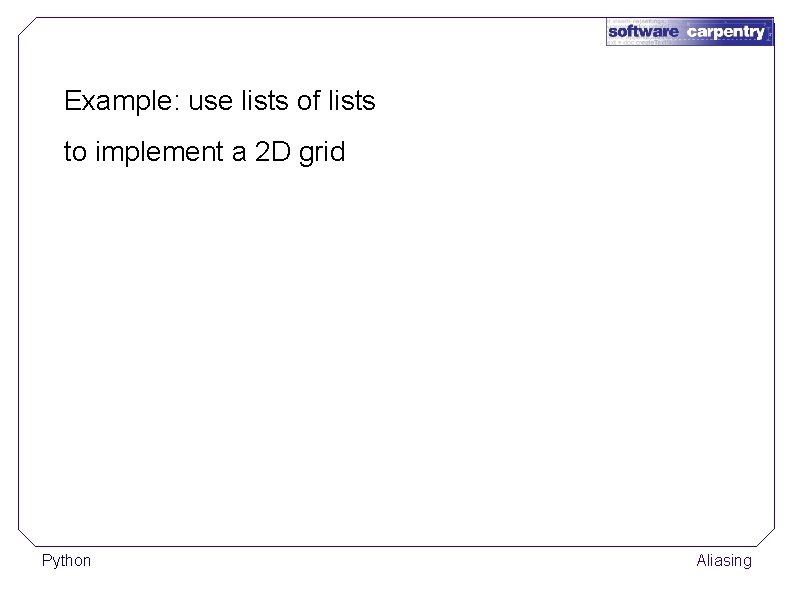
Example: use lists of lists to implement a 2 D grid Python Aliasing
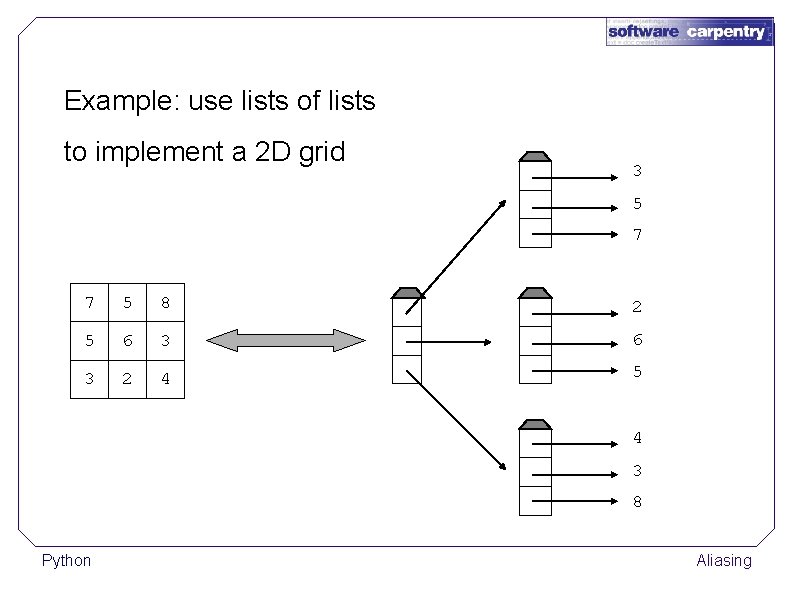
Example: use lists of lists to implement a 2 D grid 3 5 7 7 5 8 2 5 6 3 2 4 5 4 3 8 Python Aliasing
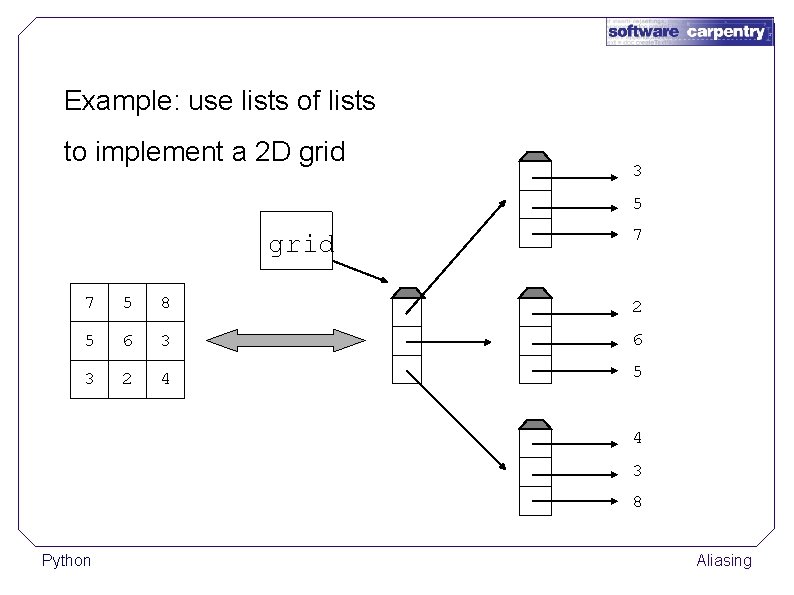
Example: use lists of lists to implement a 2 D grid 3 5 grid 7 7 5 8 2 5 6 3 2 4 5 4 3 8 Python Aliasing
![Example use lists of lists to implement a 2 D grid 3 5 grid0 Example: use lists of lists to implement a 2 D grid 3 5 grid[0]](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-22.jpg)
Example: use lists of lists to implement a 2 D grid 3 5 grid[0] 7 7 5 8 2 5 6 3 2 4 5 4 3 8 Python Aliasing
![Example use lists of lists to implement a 2 D grid 3 5 grid01 Example: use lists of lists to implement a 2 D grid 3 5 grid[0][1]](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-23.jpg)
Example: use lists of lists to implement a 2 D grid 3 5 grid[0][1] 7 7 5 8 2 5 6 3 2 4 5 4 3 8 Python Aliasing
![Correct code grid for x in rangeN temp for # Correct code grid = [] for x in range(N): temp = [] for](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-24.jpg)
# Correct code grid = [] for x in range(N): temp = [] for y in range(N): temp. append(1) grid. append(temp) Python Aliasing
![Correct code grid Outer spine of structure for x in rangeN # Correct code grid = [] Outer "spine" of structure for x in range(N):](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-25.jpg)
# Correct code grid = [] Outer "spine" of structure for x in range(N): temp = [] for y in range(N): temp. append(1) grid. append(temp) Python Aliasing
![Correct code grid for x in rangeN temp for # Correct code grid = [] for x in range(N): temp = [] for](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-26.jpg)
# Correct code grid = [] for x in range(N): temp = [] for y in range(N): temp. append(1) grid. append(temp) Python Add N sub-lists to outer list Aliasing
![Correct code grid for x in rangeN temp for # Correct code grid = [] for x in range(N): temp = [] for](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-27.jpg)
# Correct code grid = [] for x in range(N): temp = [] for y in range(N): temp. append(1) grid. append(temp) Python Create a sublist of N 1's Aliasing
![Equivalent code grid for x in rangeN grid append for y # Equivalent code grid = [] for x in range(N): grid. append([]) for y](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-28.jpg)
# Equivalent code grid = [] for x in range(N): grid. append([]) for y in range(N): grid[-1]. append(1) Python Aliasing
![Equivalent code grid for x in rangeN grid append for y # Equivalent code grid = [] for x in range(N): grid. append([]) for y](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-29.jpg)
# Equivalent code grid = [] for x in range(N): grid. append([]) for y in range(N): grid[-1]. append(1) Last element of outer list is the sublist currently being filled in Python Aliasing
![Incorrect code grid EMPTY for x in rangeN grid # Incorrect code grid = [] EMPTY = [] for x in range(N): grid.](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-30.jpg)
# Incorrect code grid = [] EMPTY = [] for x in range(N): grid. append(EMPTY) for y in range(N): grid[-1]. append(1) Python Aliasing
![Incorrect code grid EMPTY for x in rangeN grid # Incorrect code grid = [] EMPTY = [] for x in range(N): grid.](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-31.jpg)
# Incorrect code grid = [] EMPTY = [] for x in range(N): grid. append(EMPTY) for y in range(N): grid[-1]. append(1) Python # Equivalent code grid = [] for x in range(N): grid. append([]) for y in range(N): grid[-1]. append(1) Aliasing
![Incorrect code grid EMPTY for x in rangeN grid # Incorrect code grid = [] EMPTY = [] for x in range(N): grid.](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-32.jpg)
# Incorrect code grid = [] EMPTY = [] for x in range(N): grid. append(EMPTY) for y in range(N): grid[-1]. append(1) Python Aren't meaningful variable names supposed to be a good thing? Aliasing
![variable x grid value 0 grid EMPTY for x in variable x grid value 0 grid = [] EMPTY = [] for x in](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-33.jpg)
variable x grid value 0 grid = [] EMPTY = [] for x in range(N): grid. append(EMPTY) for y in range(N): grid[-1]. append(1) EMPTY Python Aliasing
![variable x grid value 0 grid EMPTY for x in variable x grid value 0 grid = [] EMPTY = [] for x in](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-34.jpg)
variable x grid value 0 grid = [] EMPTY = [] for x in range(N): grid. append(EMPTY) for y in range(N): grid[-1]. append(1) EMPTY Python Aliasing
![variable value x 0 y 0 grid EMPTY for x variable value x 0 y 0 grid = [] EMPTY = [] for x](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-35.jpg)
variable value x 0 y 0 grid = [] EMPTY = [] for x in range(N): grid. append(EMPTY) for y in range(N): grid[-1]. append(1) EMPTY Python Aliasing
![variable value x 0 y 0 grid EMPTY for x variable value x 0 y 0 grid = [] EMPTY = [] for x](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-36.jpg)
variable value x 0 y 0 grid = [] EMPTY = [] for x in range(N): grid. append(EMPTY) for y in range(N): grid[-1]. append(1) grid EMPTY 1 Python Aliasing
![variable value x 0 y 2 grid EMPTY for x variable value x 0 y 2 grid = [] EMPTY = [] for x](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-37.jpg)
variable value x 0 y 2 grid = [] EMPTY = [] for x in range(N): grid. append(EMPTY) for y in range(N): grid[-1]. append(1) grid EMPTY 1 1 1 Python Aliasing
![variable value x 1 y 2 grid EMPTY for x variable value x 1 y 2 grid = [] EMPTY = [] for x](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-38.jpg)
variable value x 1 y 2 grid = [] EMPTY = [] for x in range(N): grid. append(EMPTY) for y in range(N): grid[-1]. append(1) grid EMPTY 1 1 1 Python Aliasing
![variable value x 1 y 2 grid EMPTY for x variable value x 1 y 2 grid = [] EMPTY = [] for x](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-39.jpg)
variable value x 1 y 2 grid = [] EMPTY = [] for x in range(N): grid. append(EMPTY) for y in range(N): grid[-1]. append(1) grid EMPTY 1 1 1 You see the problem. . . Python Aliasing
![No Aliasing first second Python Aliasing No Aliasing first = [] second = [] Python Aliasing](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-40.jpg)
No Aliasing first = [] second = [] Python Aliasing
![Python No Aliasing first second first Aliasing Python No Aliasing first = [] second = first Aliasing](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-41.jpg)
Python No Aliasing first = [] second = first Aliasing
![variable x grid Python value 0 grid for x in rangeN grid variable x grid Python value 0 grid = [] for x in range(N): grid.](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-42.jpg)
variable x grid Python value 0 grid = [] for x in range(N): grid. append([]) for y in range(N): grid[-1]. append(1) Aliasing
![variable x grid Python value 0 grid for x in rangeN grid variable x grid Python value 0 grid = [] for x in range(N): grid.](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-43.jpg)
variable x grid Python value 0 grid = [] for x in range(N): grid. append([]) for y in range(N): grid[-1]. append(1) Aliasing
![variable value x 0 y 2 grid for x in rangeN grid variable value x 0 y 2 grid = [] for x in range(N): grid.](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-44.jpg)
variable value x 0 y 2 grid = [] for x in range(N): grid. append([]) for y in range(N): grid[-1]. append(1) grid 1 1 1 Python Aliasing
![variable value x 1 y 2 grid for x in rangeN grid variable value x 1 y 2 grid = [] for x in range(N): grid.](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-45.jpg)
variable value x 1 y 2 grid = [] for x in range(N): grid. append([]) for y in range(N): grid[-1]. append(1) 1 1 1 Python Aliasing
![variable value x 1 y 0 grid for x in rangeN grid variable value x 1 y 0 grid = [] for x in range(N): grid.](https://slidetodoc.com/presentation_image_h2/aa79d6c9098527f788b4621968e3b0ac/image-46.jpg)
variable value x 1 y 0 grid = [] for x in range(N): grid. append([]) for y in range(N): grid[-1]. append(1) grid 1 1 Python Aliasing
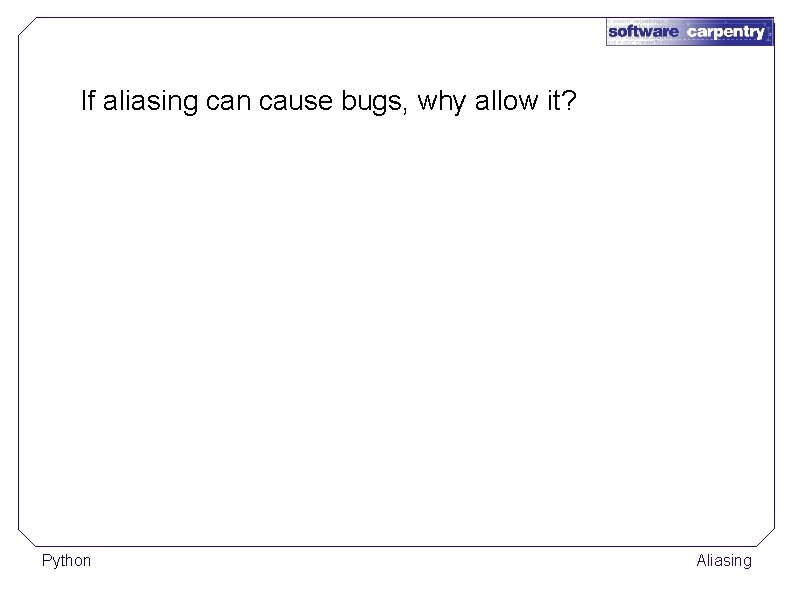
If aliasing can cause bugs, why allow it? Python Aliasing
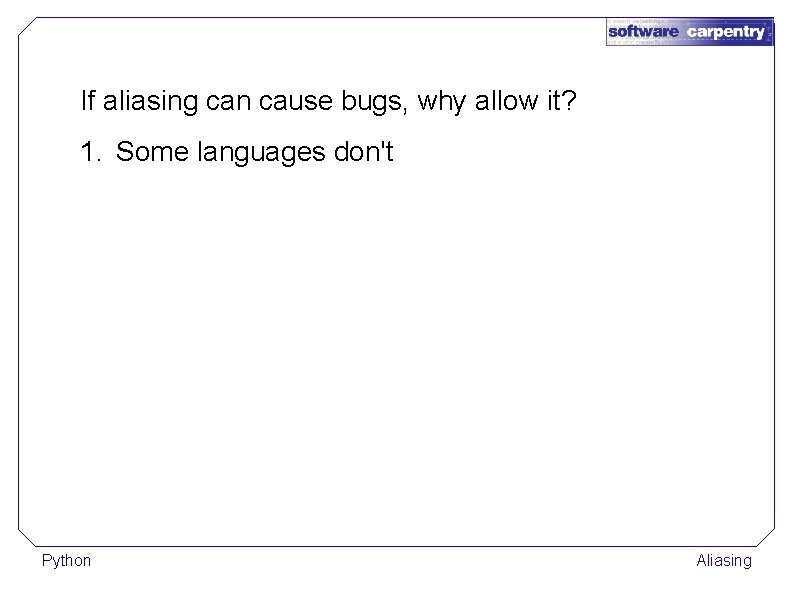
If aliasing can cause bugs, why allow it? 1. Some languages don't Python Aliasing
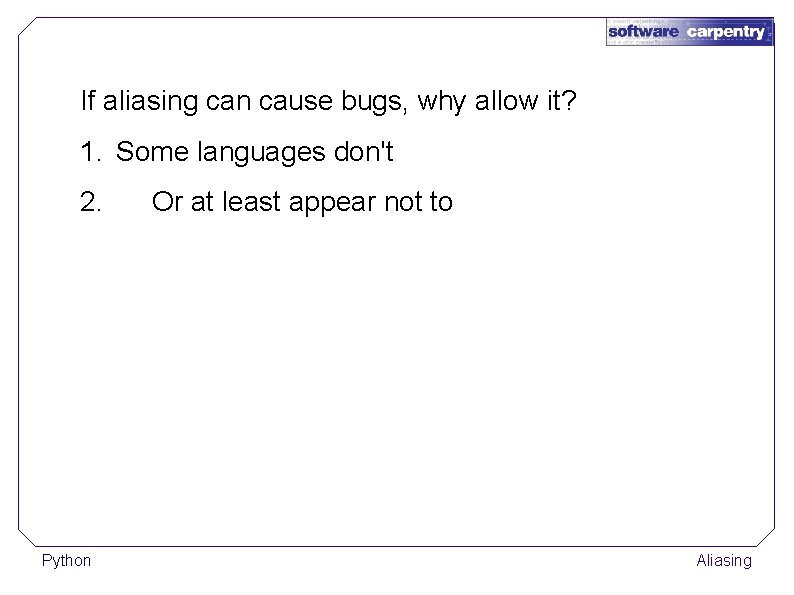
If aliasing can cause bugs, why allow it? 1. Some languages don't 2. Python Or at least appear not to Aliasing
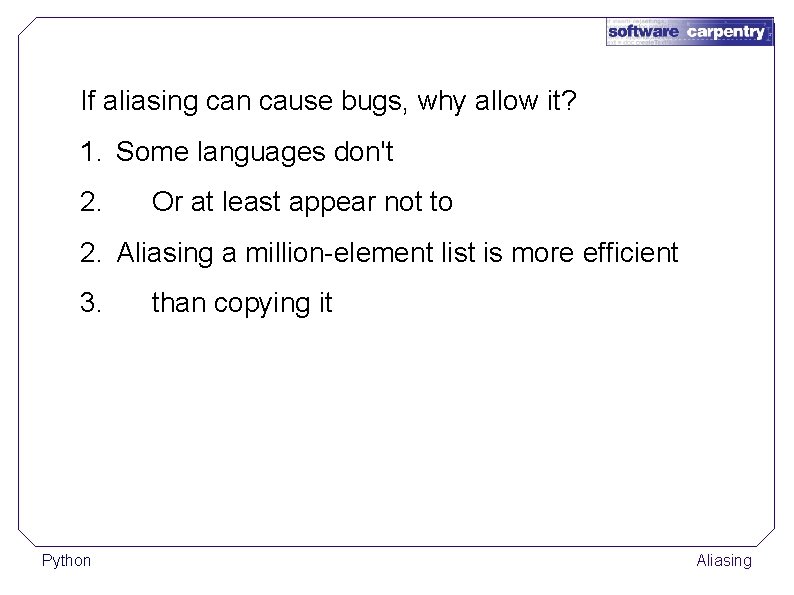
If aliasing can cause bugs, why allow it? 1. Some languages don't 2. Or at least appear not to 2. Aliasing a million-element list is more efficient 3. Python than copying it Aliasing
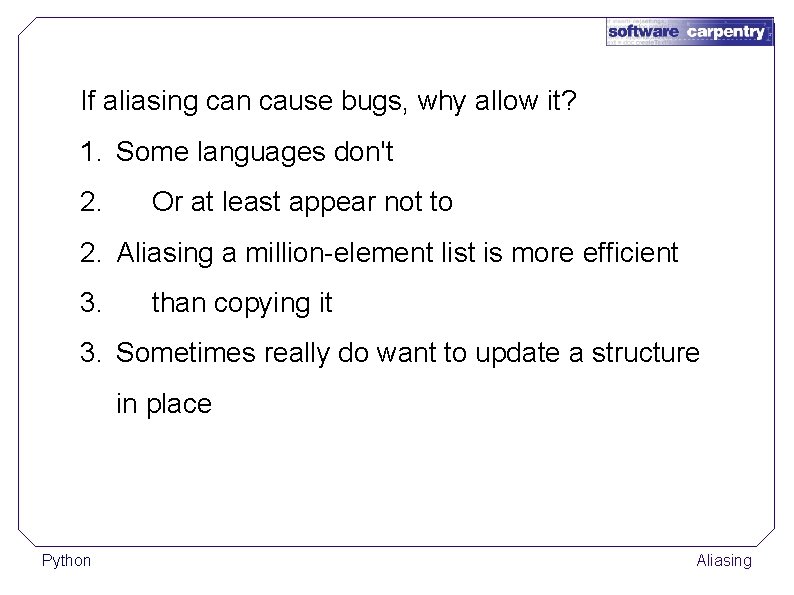
If aliasing can cause bugs, why allow it? 1. Some languages don't 2. Or at least appear not to 2. Aliasing a million-element list is more efficient 3. than copying it 3. Sometimes really do want to update a structure in place Python Aliasing
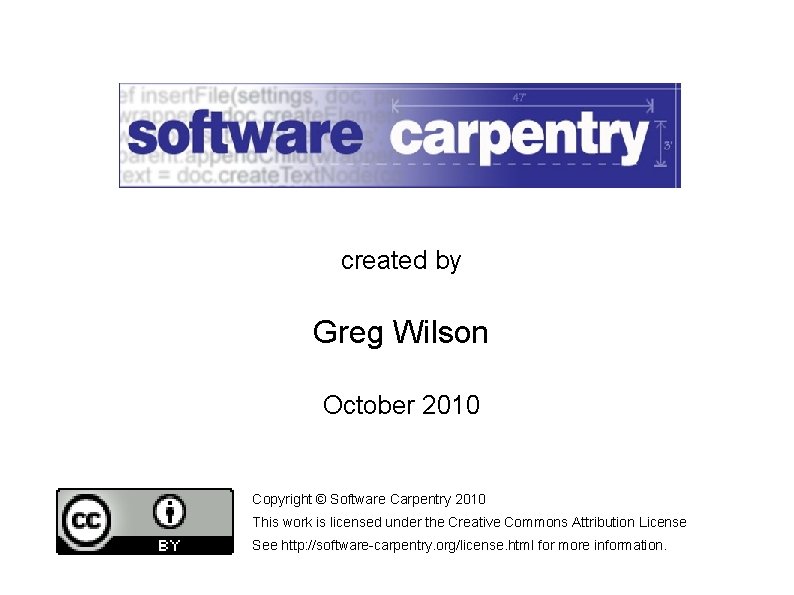
created by Greg Wilson October 2010 Copyright © Software Carpentry 2010 This work is licensed under the Creative Commons Attribution License See http: //software-carpentry. org/license. html for more information.
Copyright 2010 pearson education inc
Copyright 2010
Copyright 2010
Copyright 2010 pearson education inc
Copyright 2010 pearson education inc
Copyright 2010
Nwoz
Copyright 2010 pearson education inc
Copyright 2010 pearson education inc
Copyright 2010 pearson education inc
Copyright 2010 pearson education inc
Copyright 2010 pearson education inc
Copyright 2010 pearson education inc
Pearson education inc all rights reserved
What is aliasing in sampling
Aliasing frequency formula
Sampling dan aliasing
Aliasing frequency formula
Aliasing in digital image processing
Aliasing
Aliasing
Aliasing
Filterpro ti
Aliasing
Ergodicity
Memory aliasing optimization blocker
Aliasing
7l128
Aliasing doppler
Suseptibilite artefaktı
Nyquist aliasing
Dlaa anti aliasing
Aliasing ecografia
Phénomène d'aliasing doppler
Aliasing and antialiasing in computer graphics
Columbus custom carpentry
10 classification of hand tools
Joint carpentry
A carpentry hammer with a slightly rounded
Big carpentry
Driving tools in carpentry
Types of joint wood
Python event manager
Objectives of work immersion portfolio
Teamwork in work immersion
Smart work and hard work
Present simple work
Social goals model
Casework components
Team vs working group
Work energy theorem
Chapter 4 work and energy section 1 work and machines
I work all day i work all night