Python Functions Copyright Software Carpentry 2010 This work
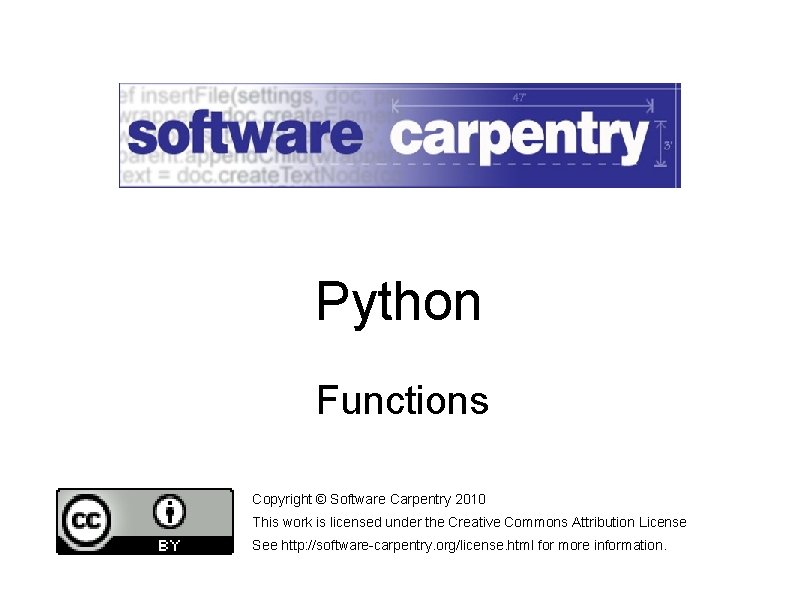
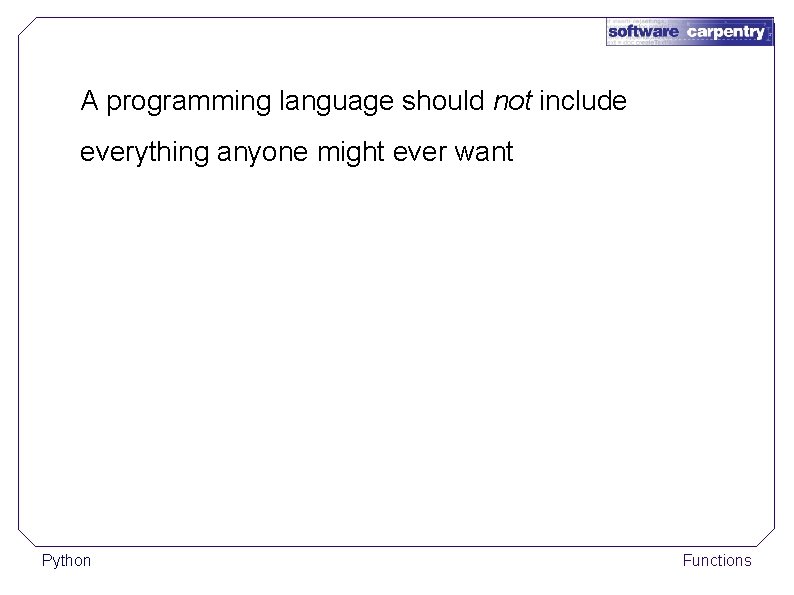
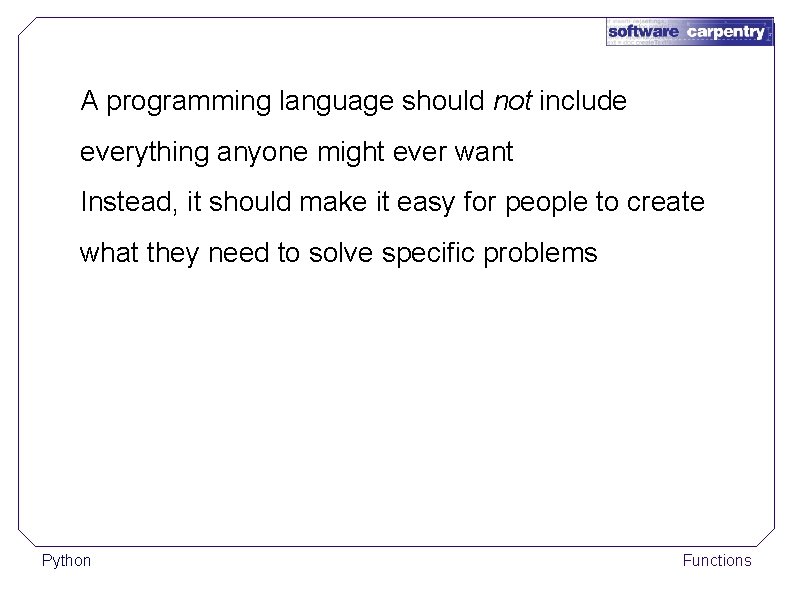
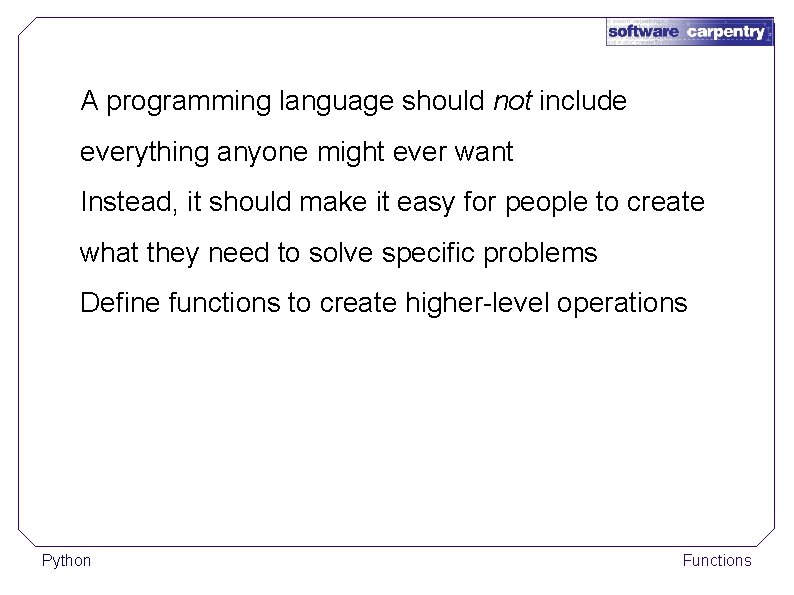
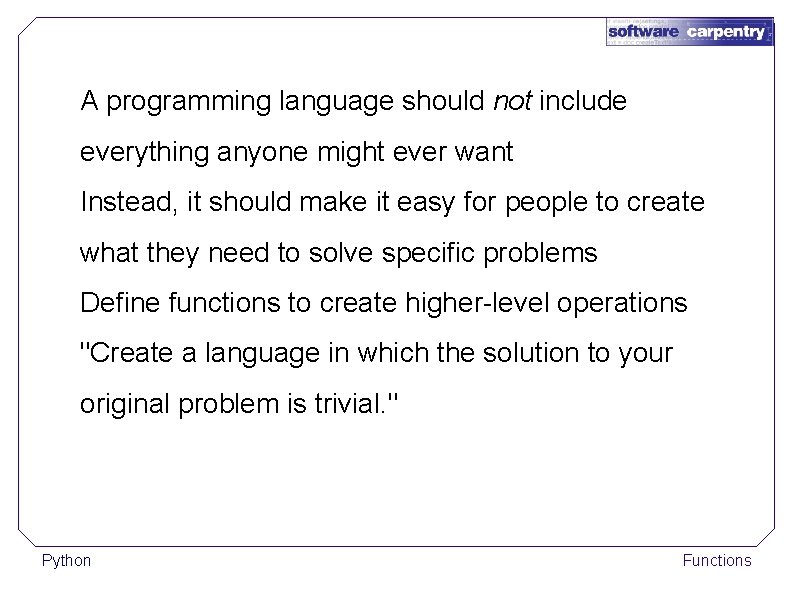
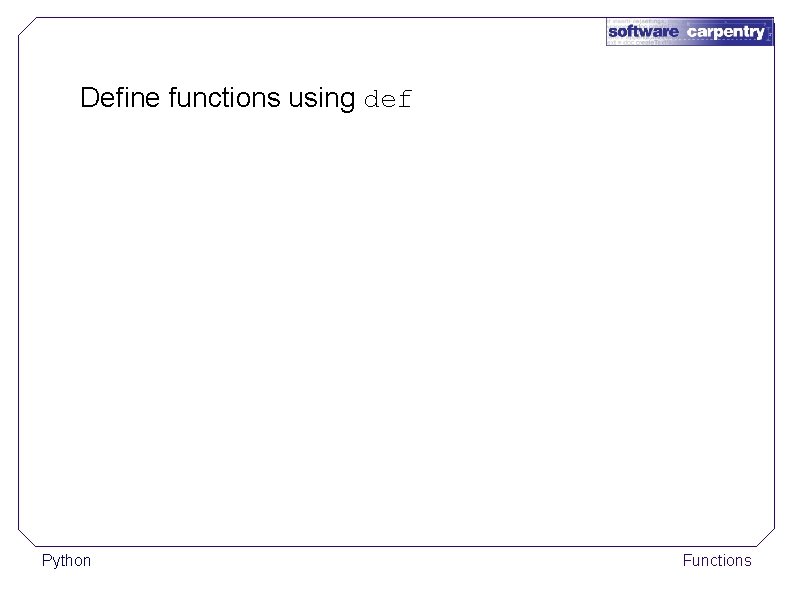
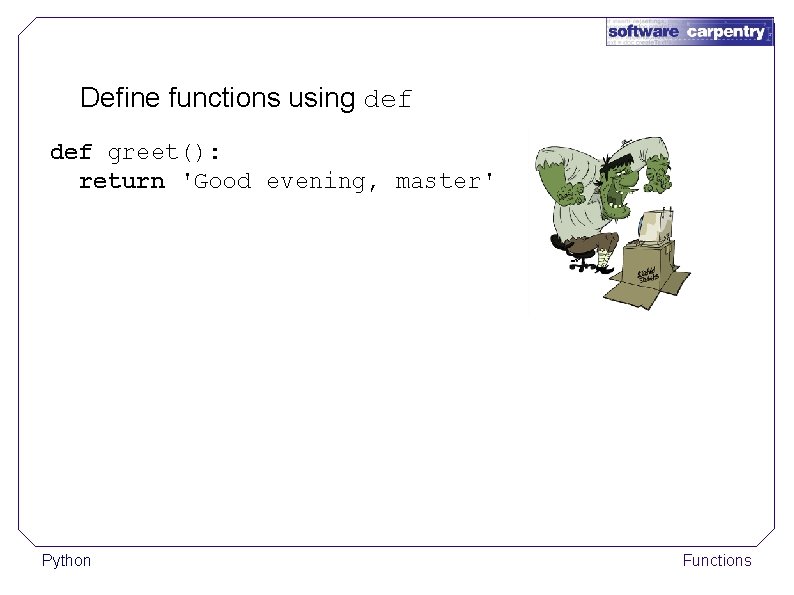
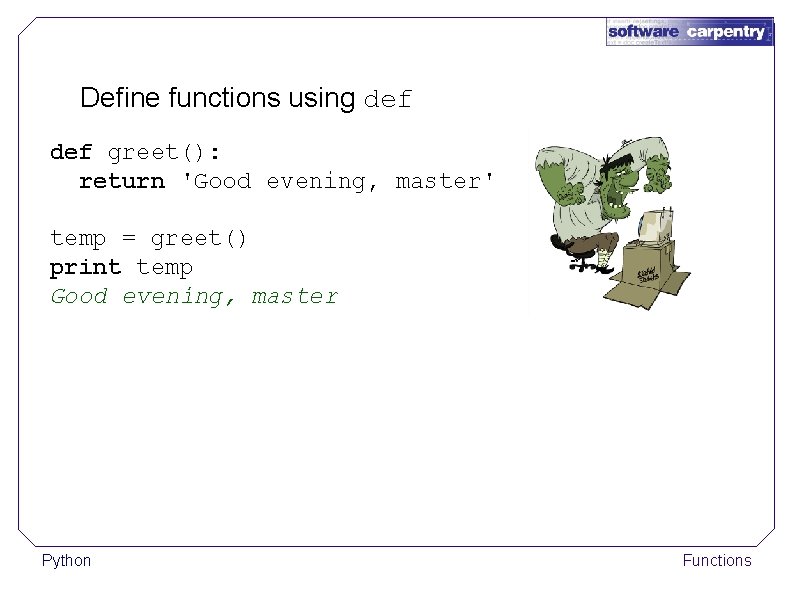
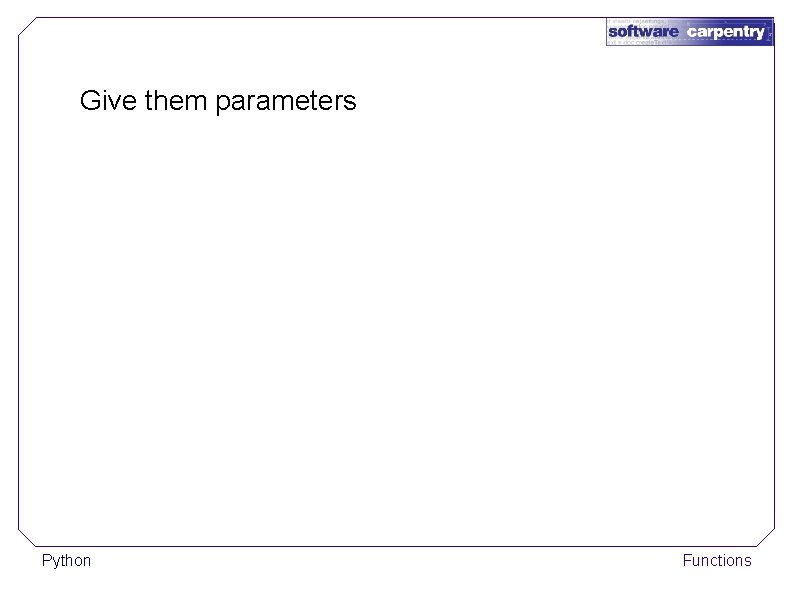
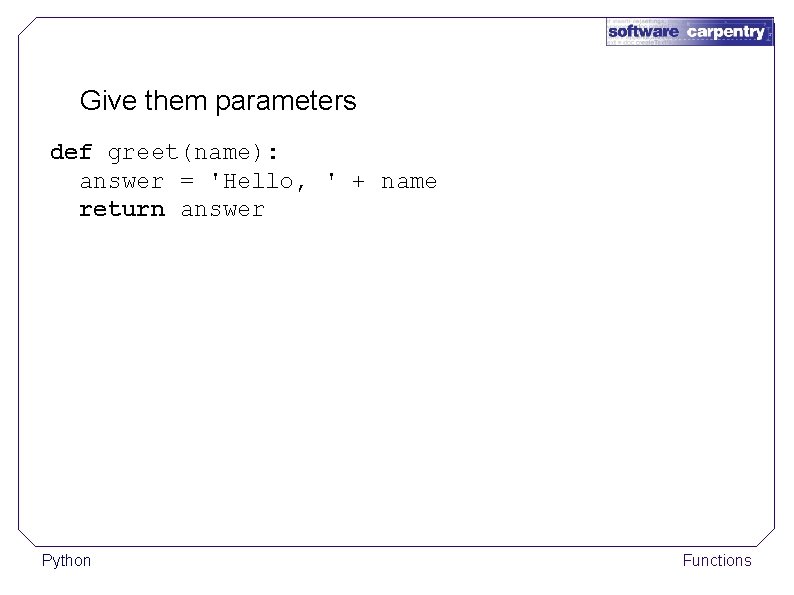
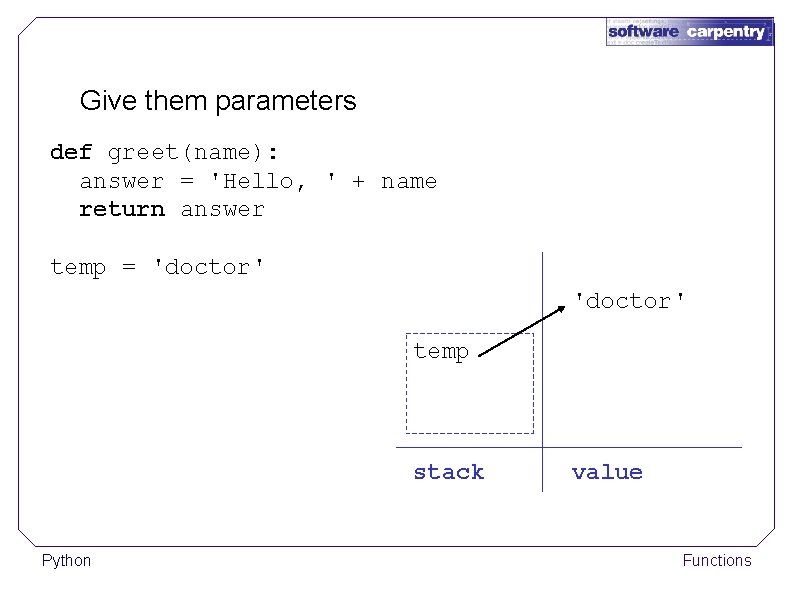
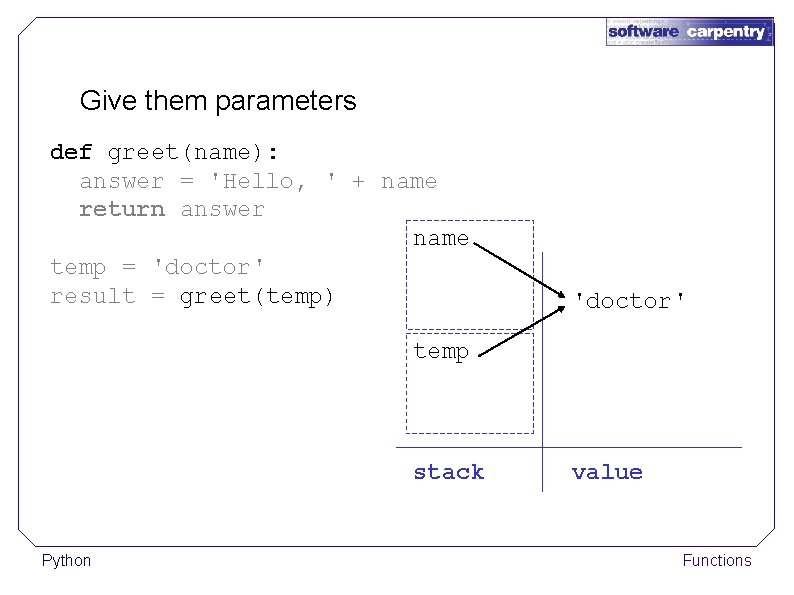
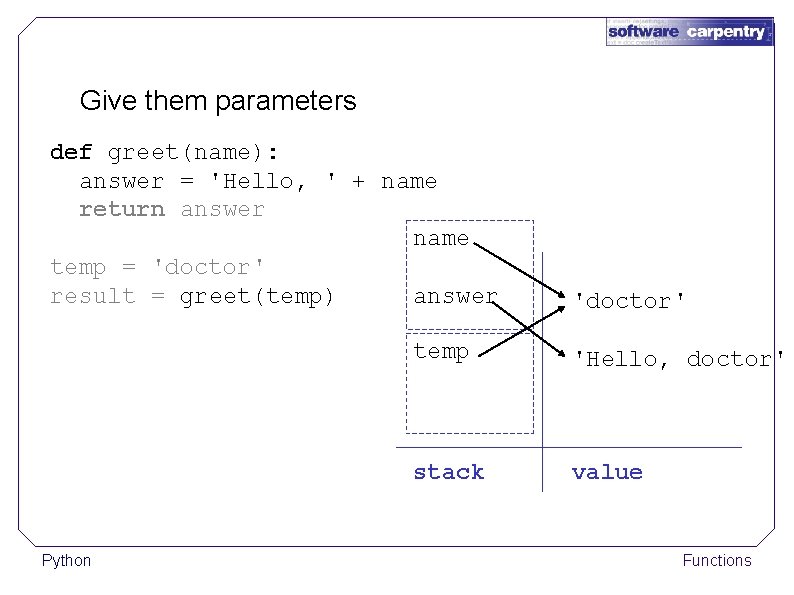
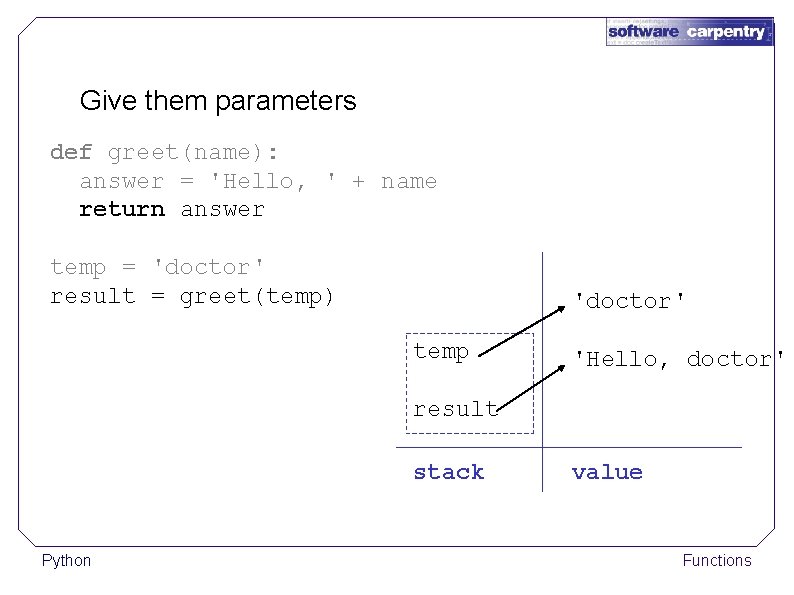
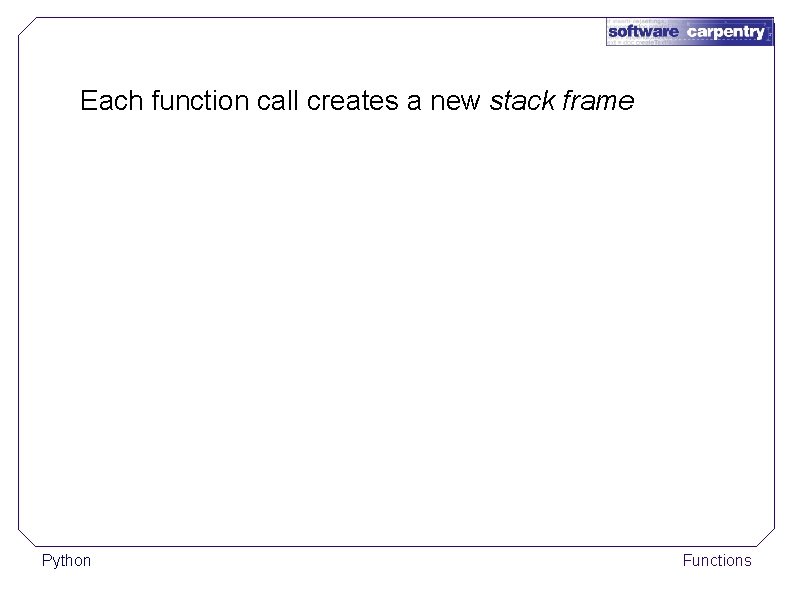
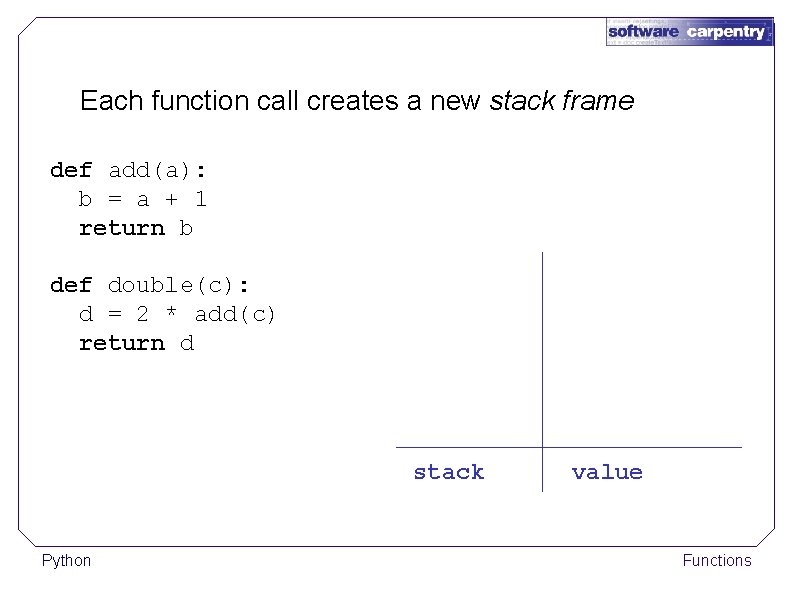
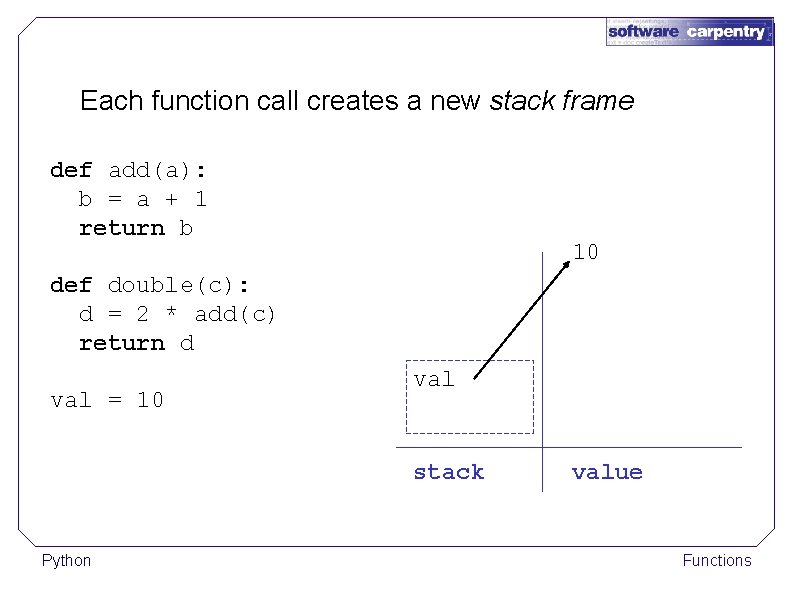
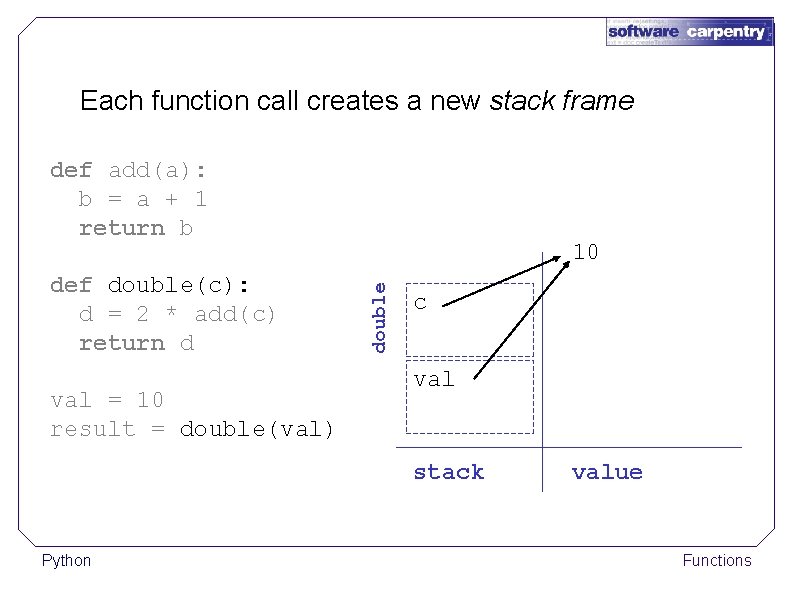
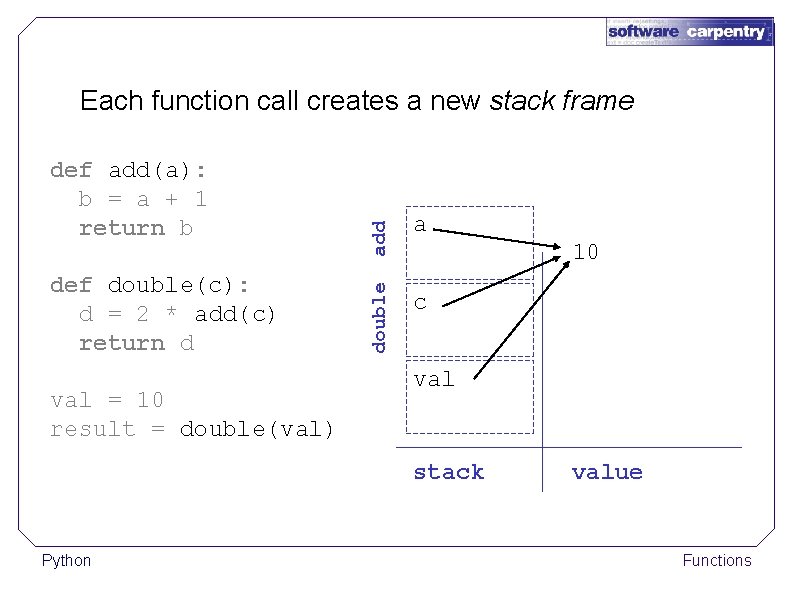
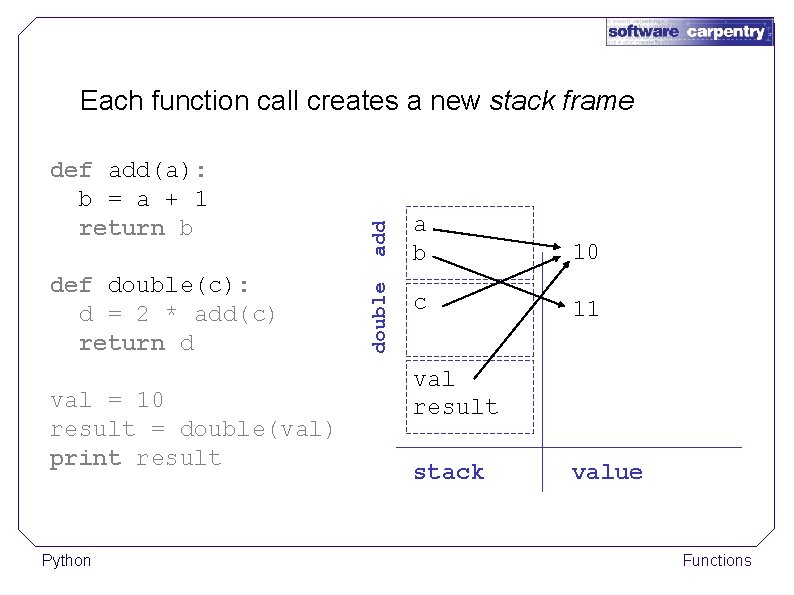
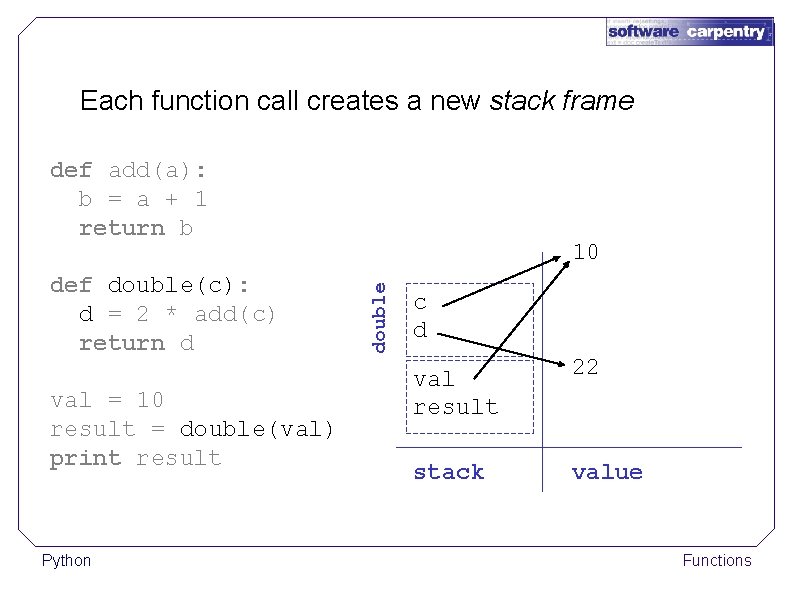
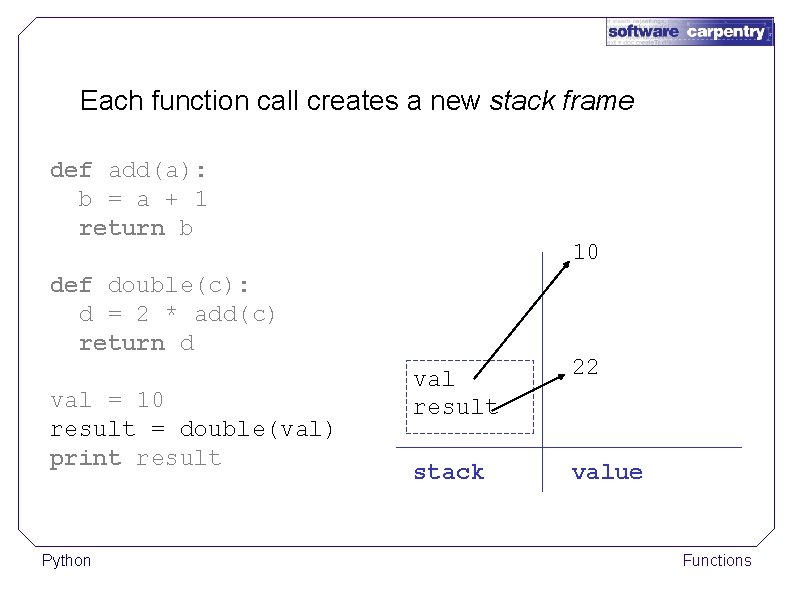
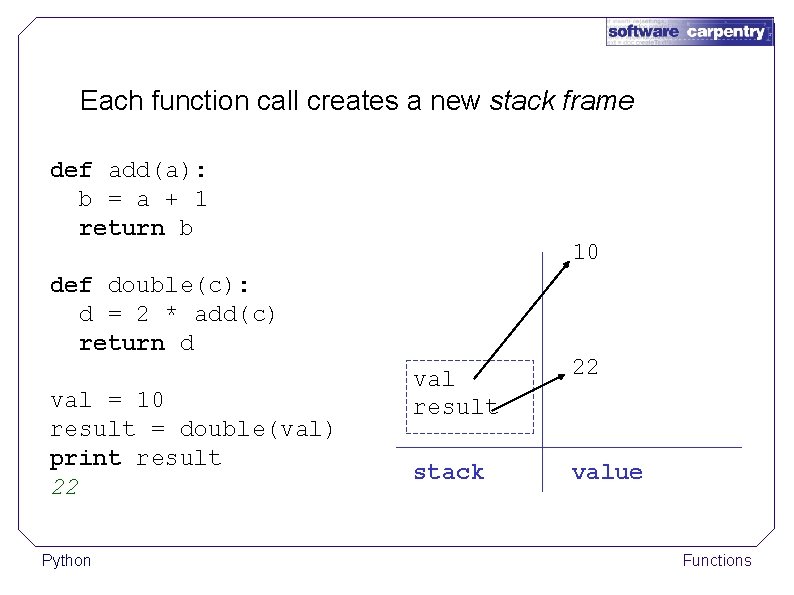
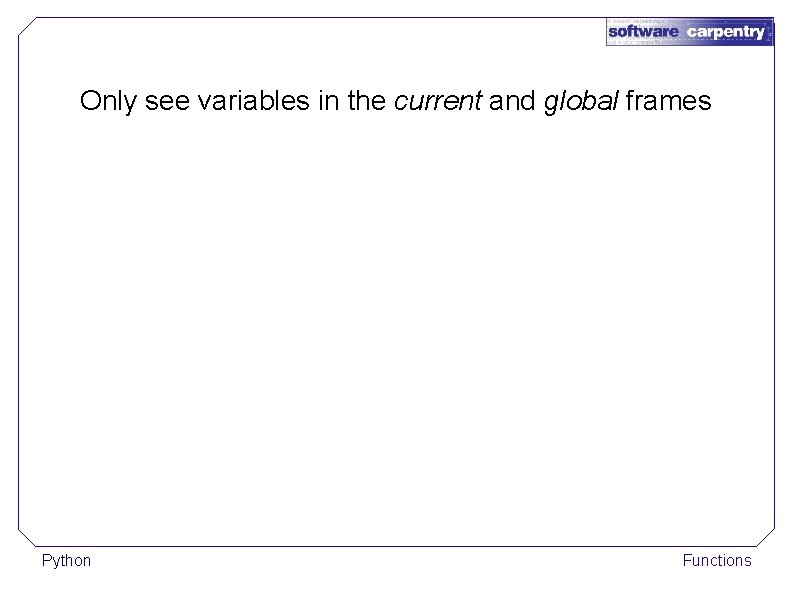
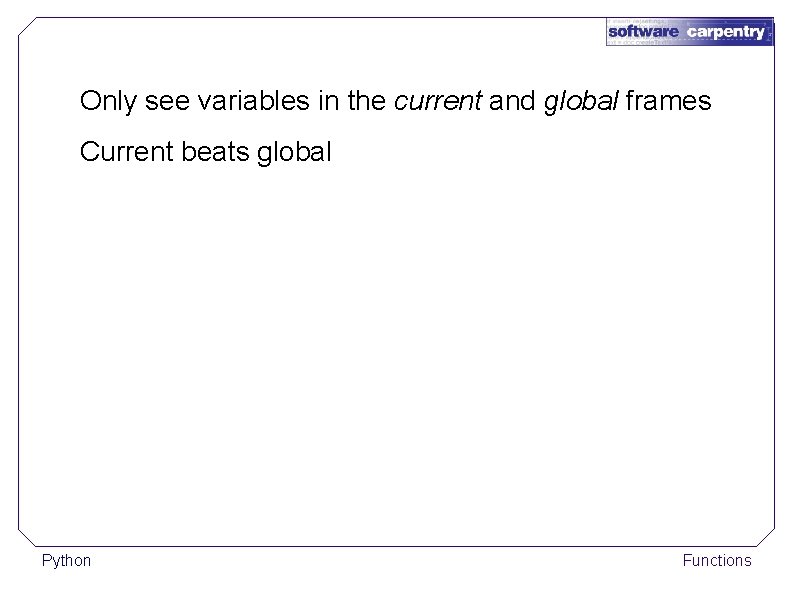
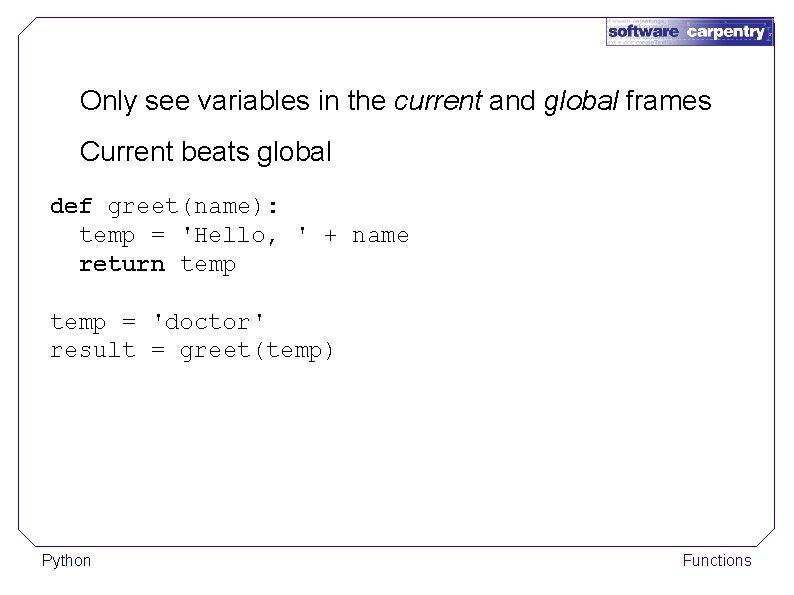
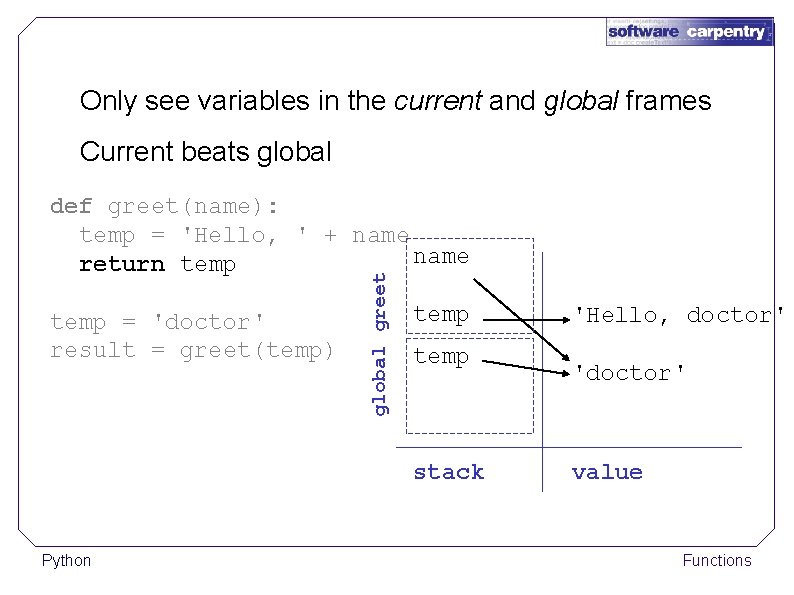
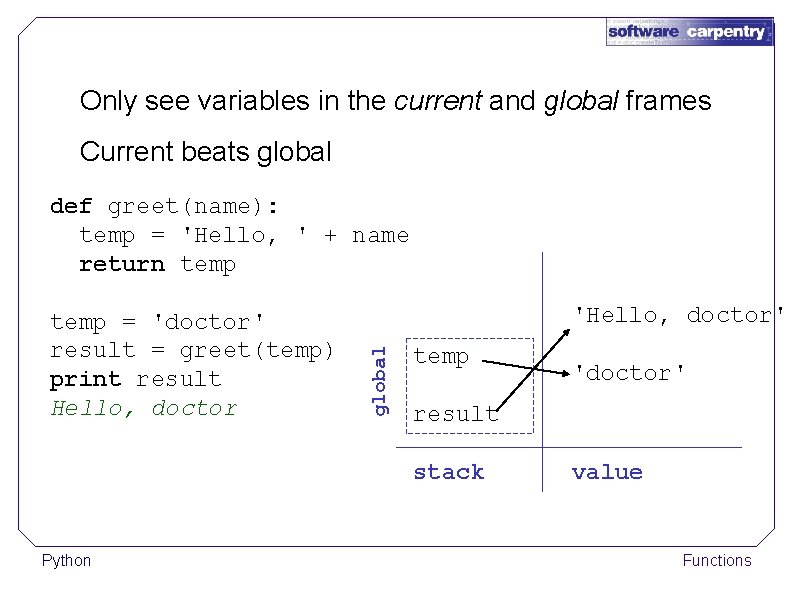
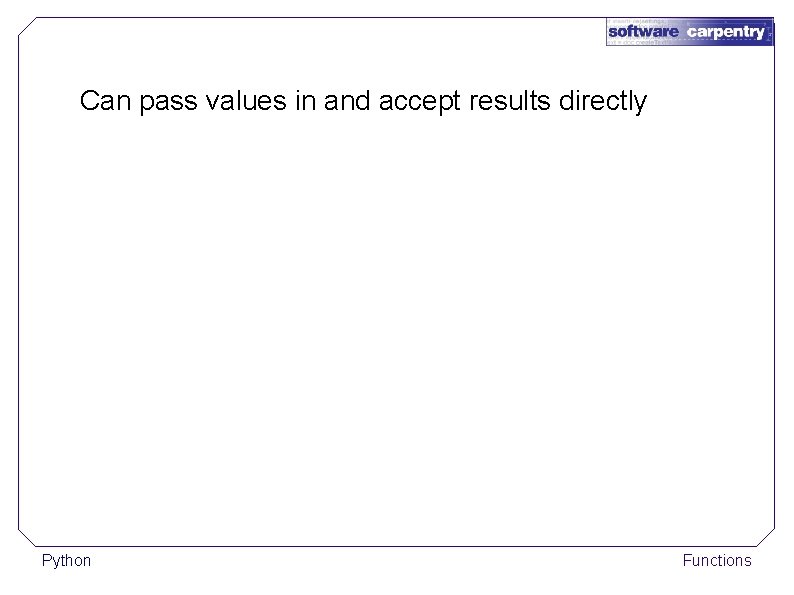
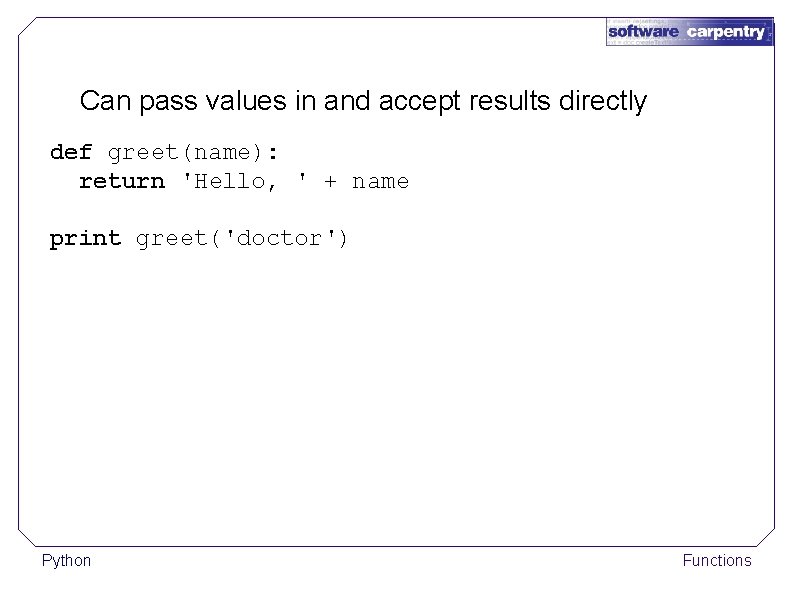
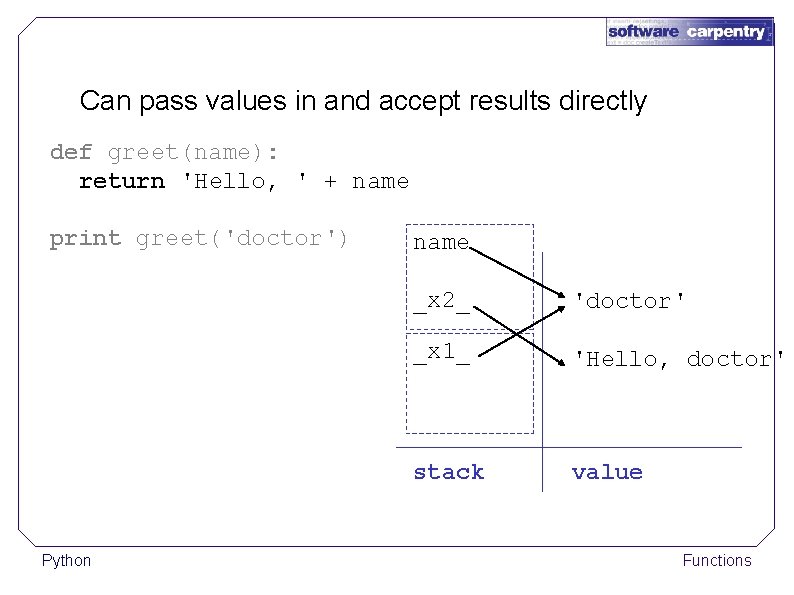
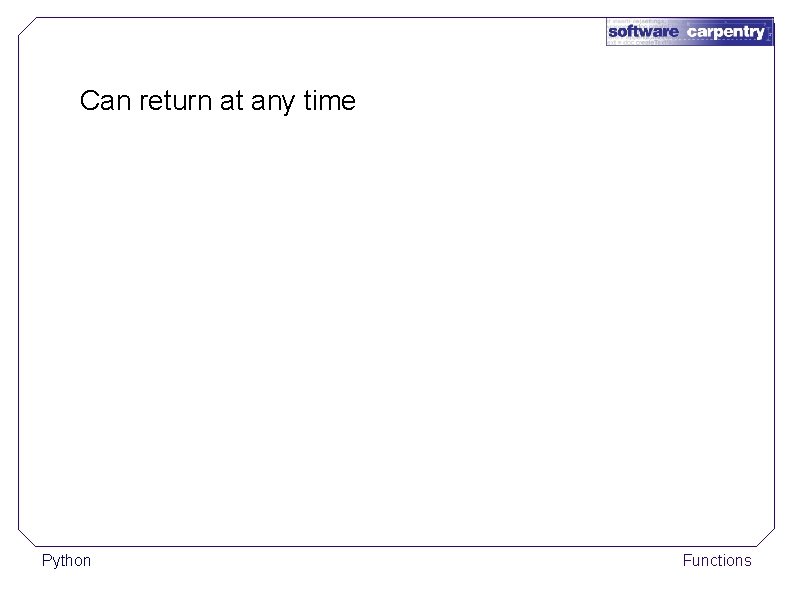
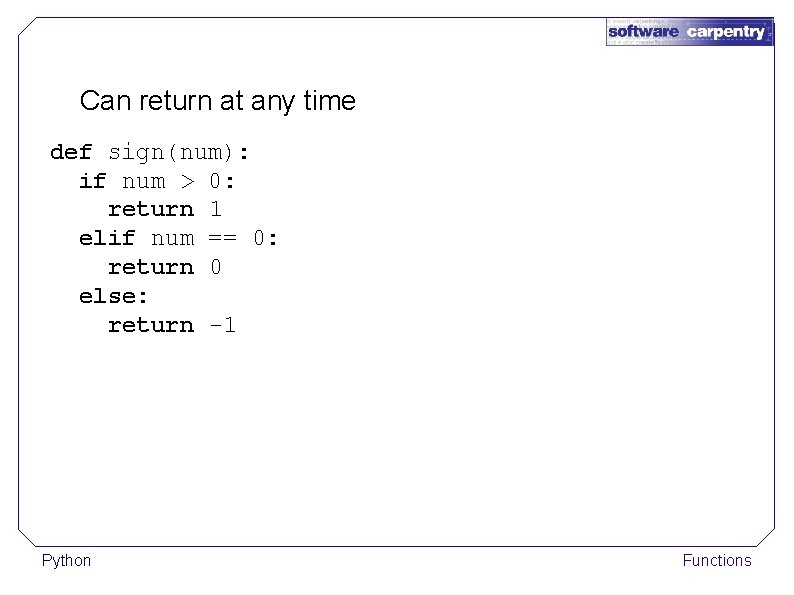
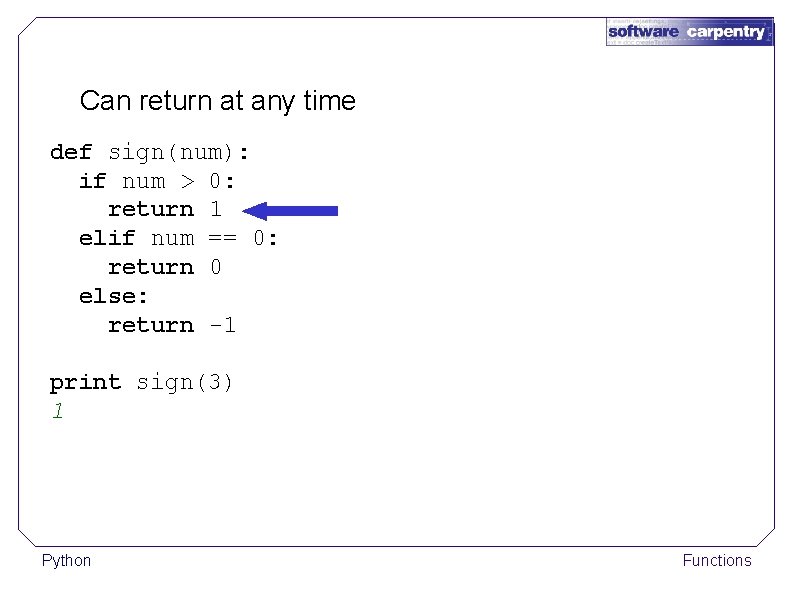
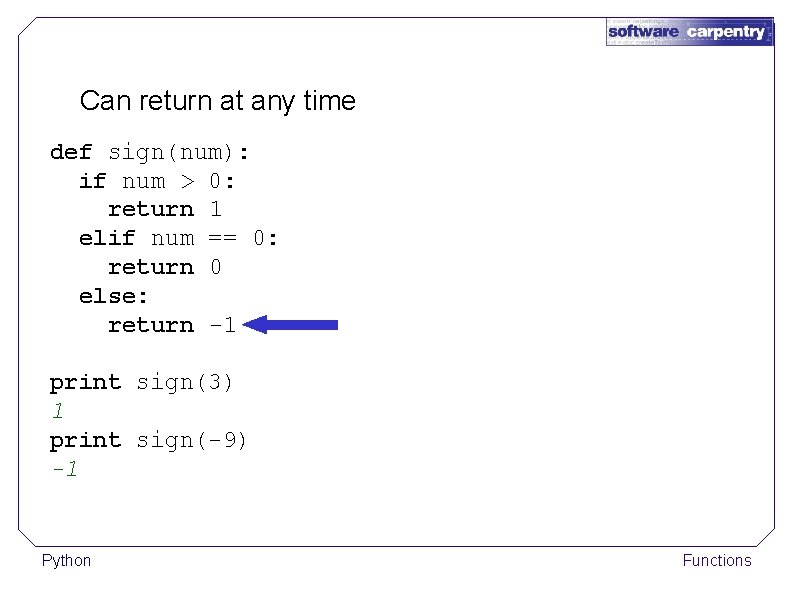
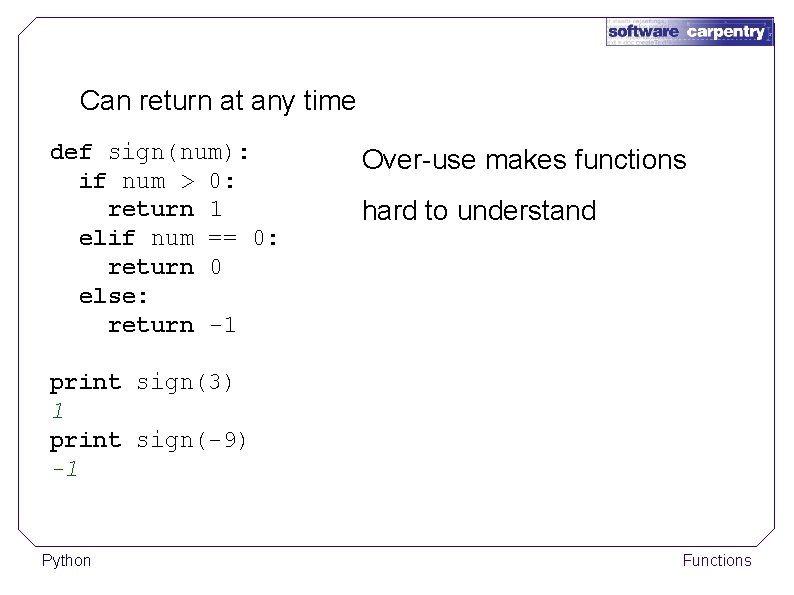
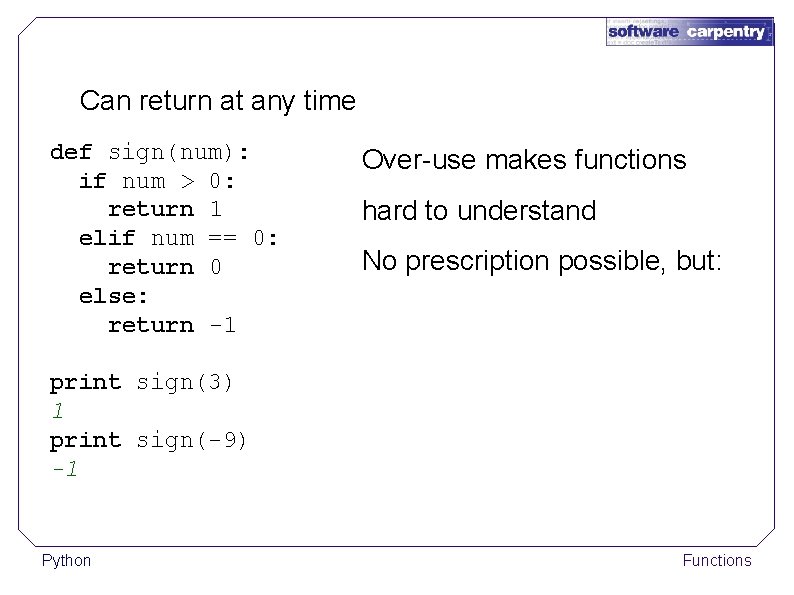
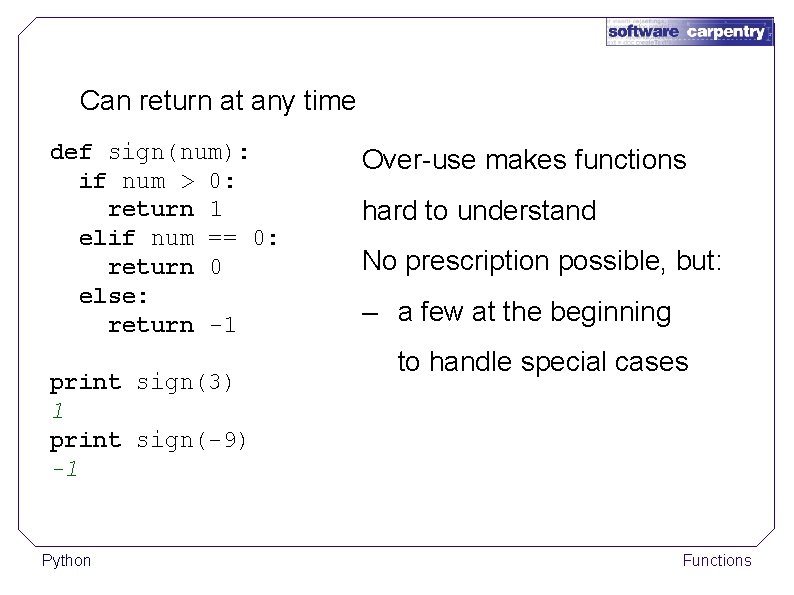
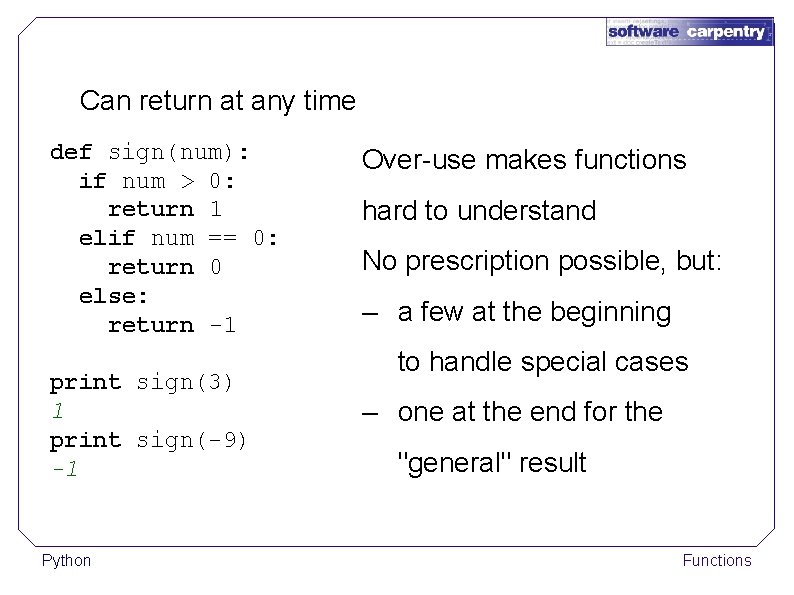
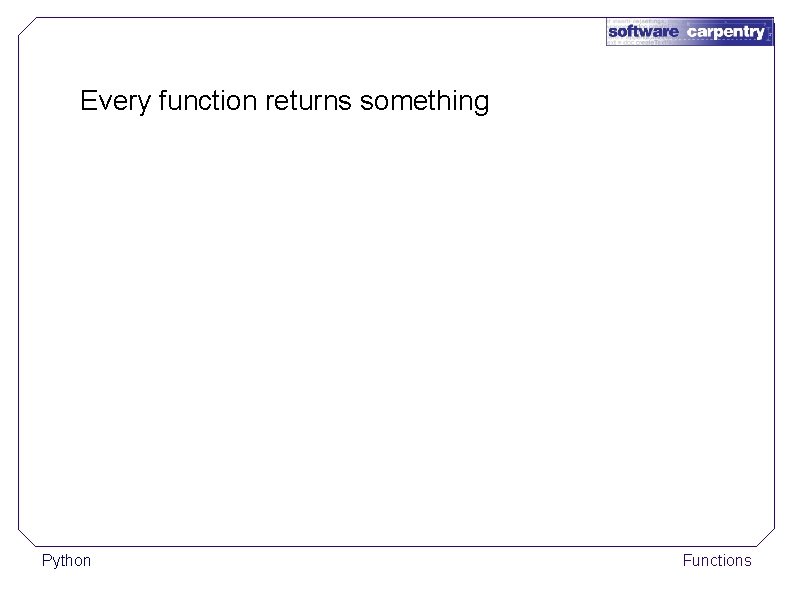
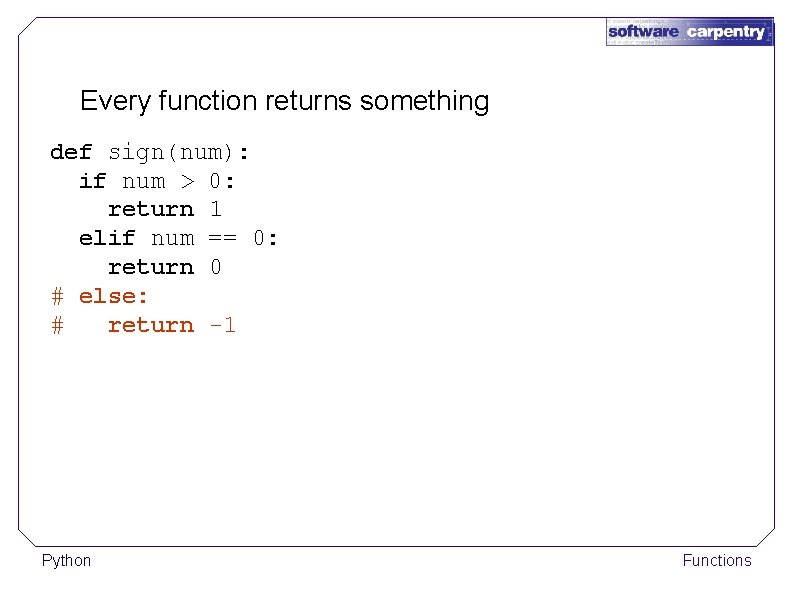
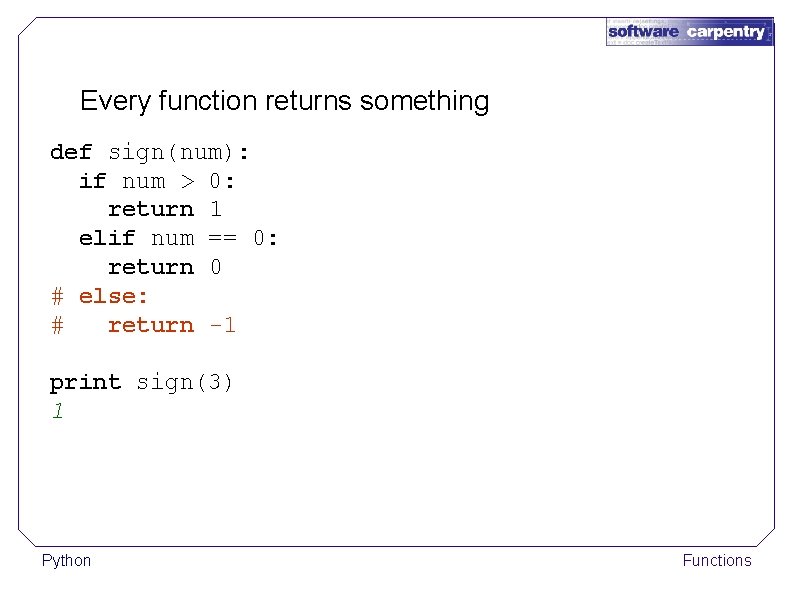
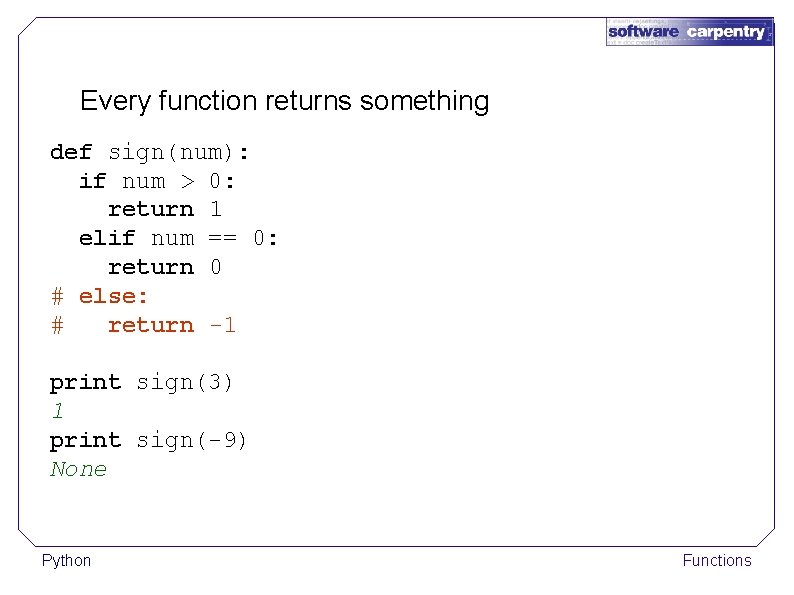
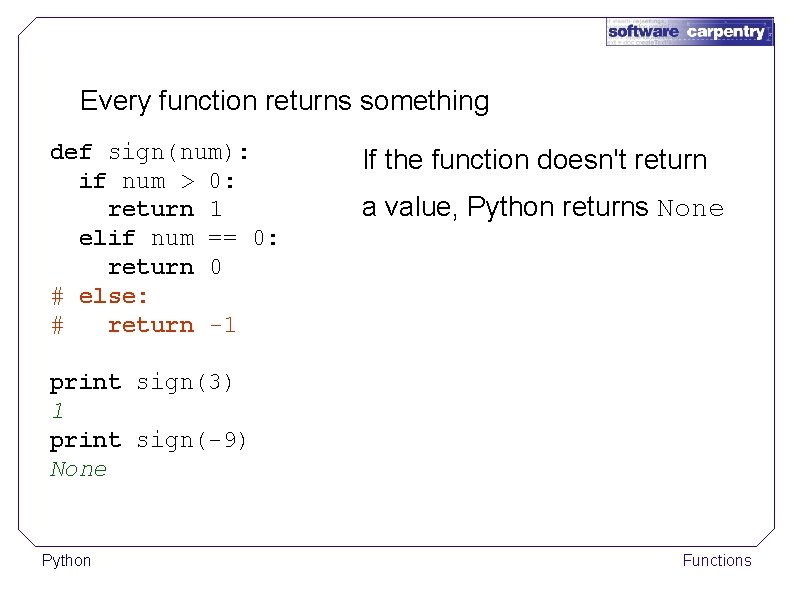
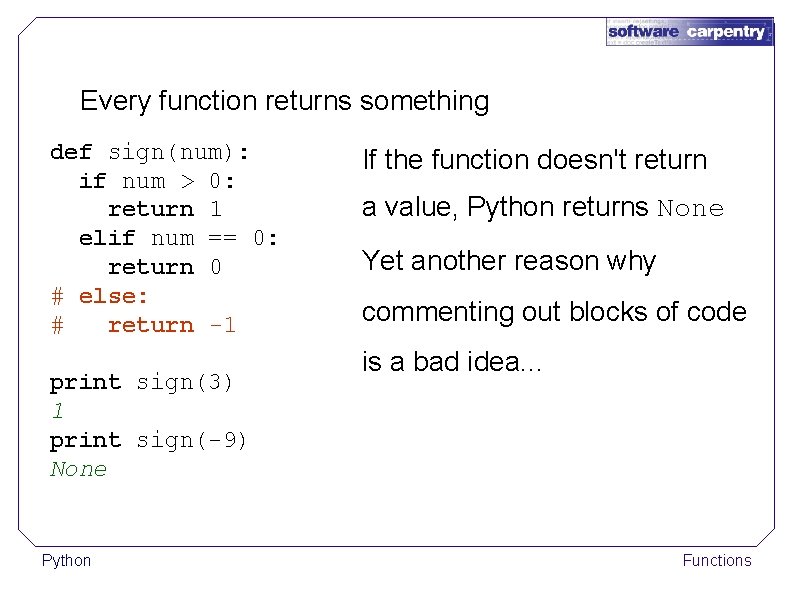
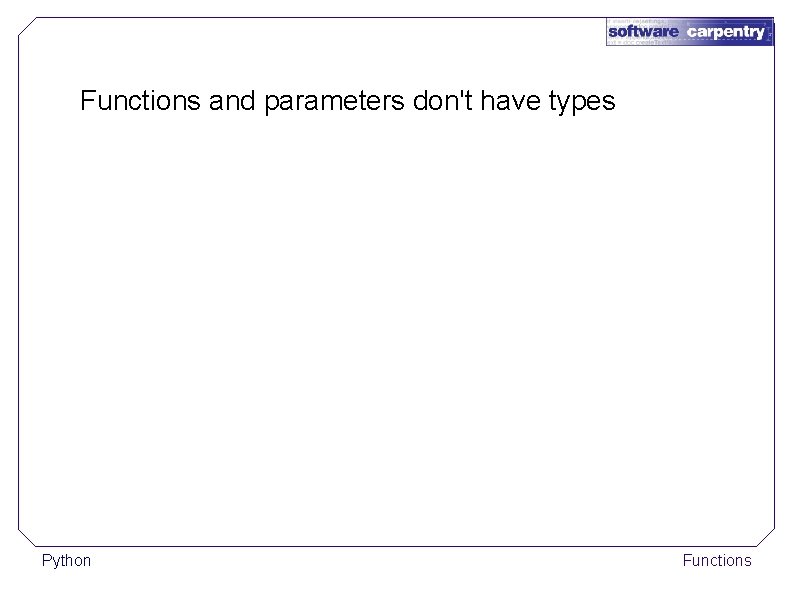
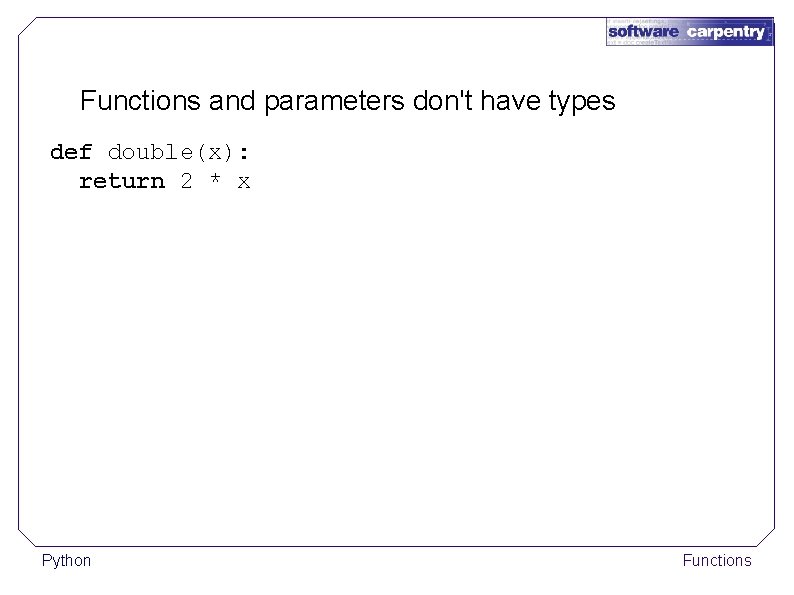
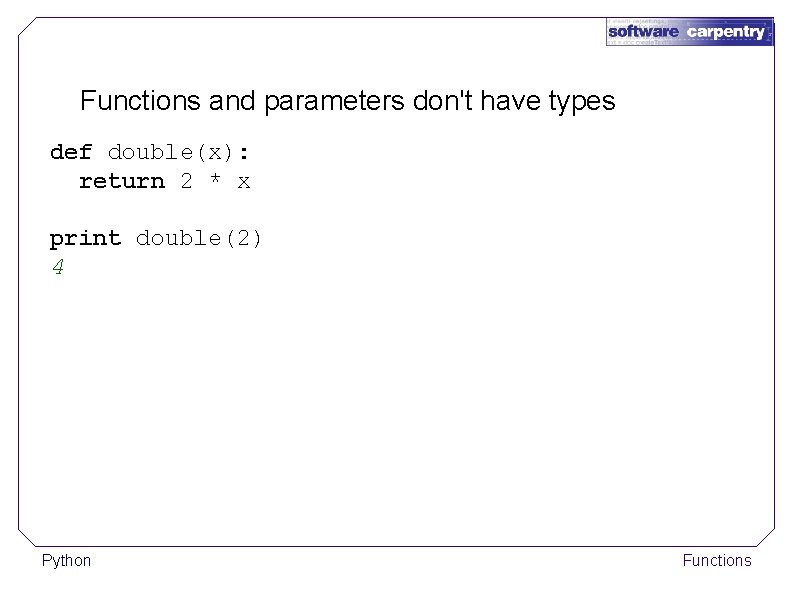
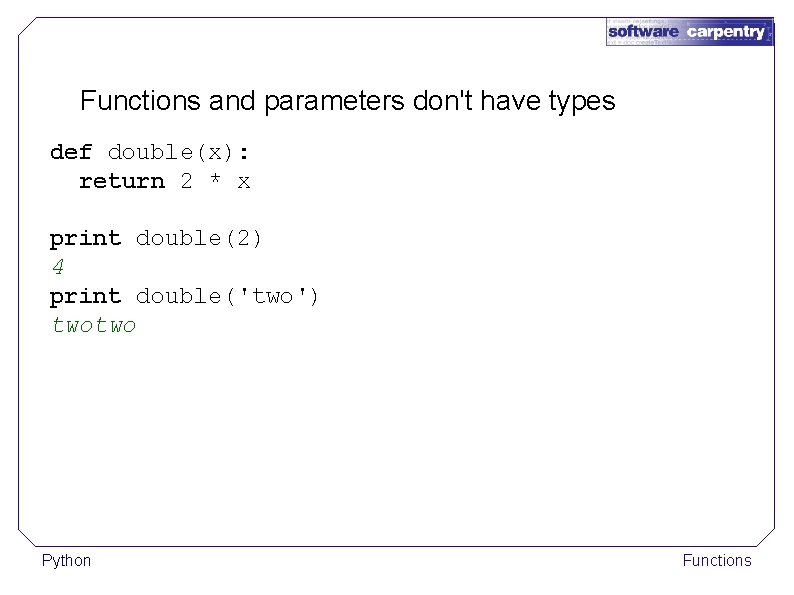
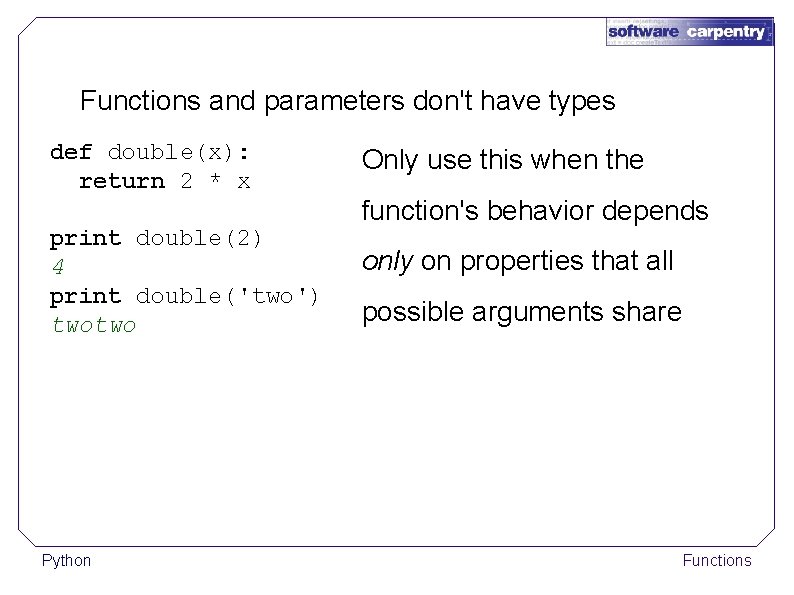
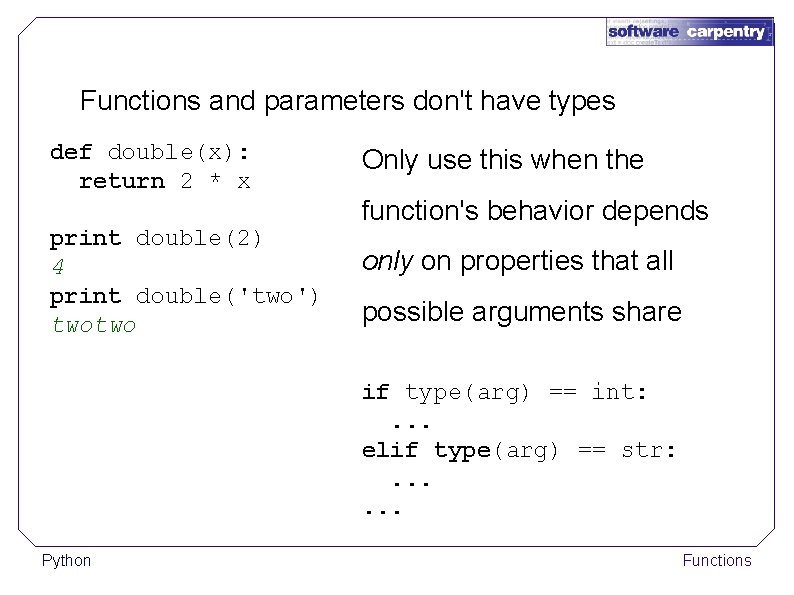
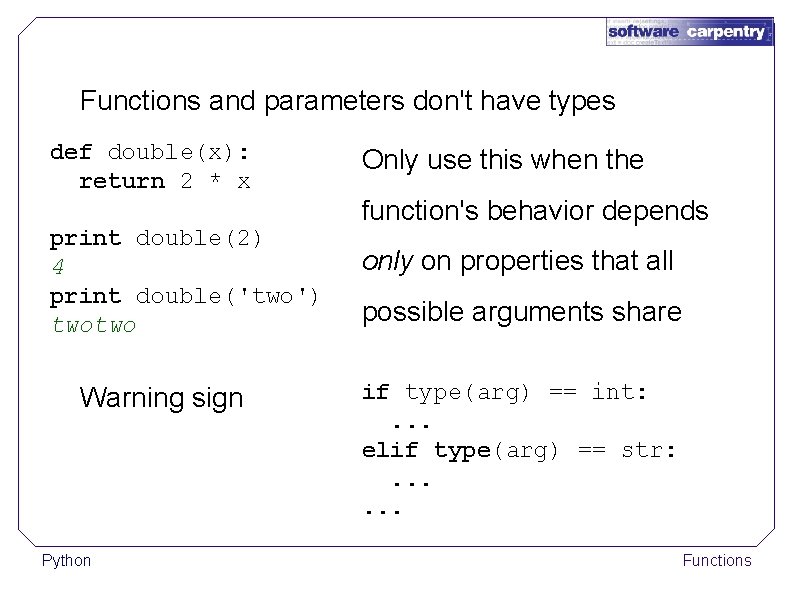
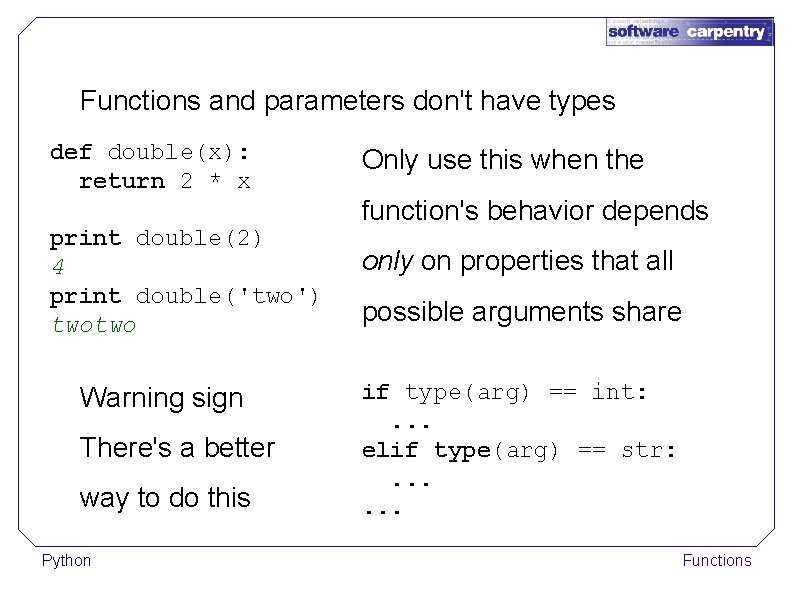
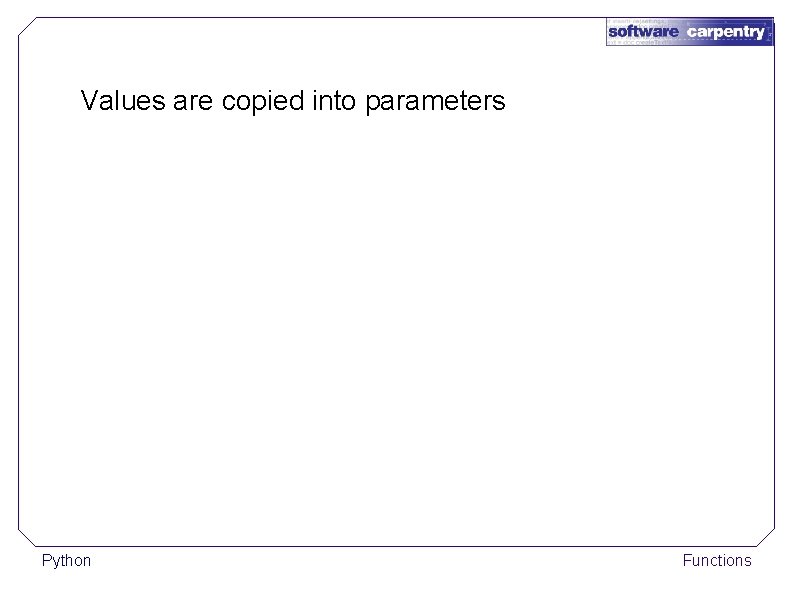
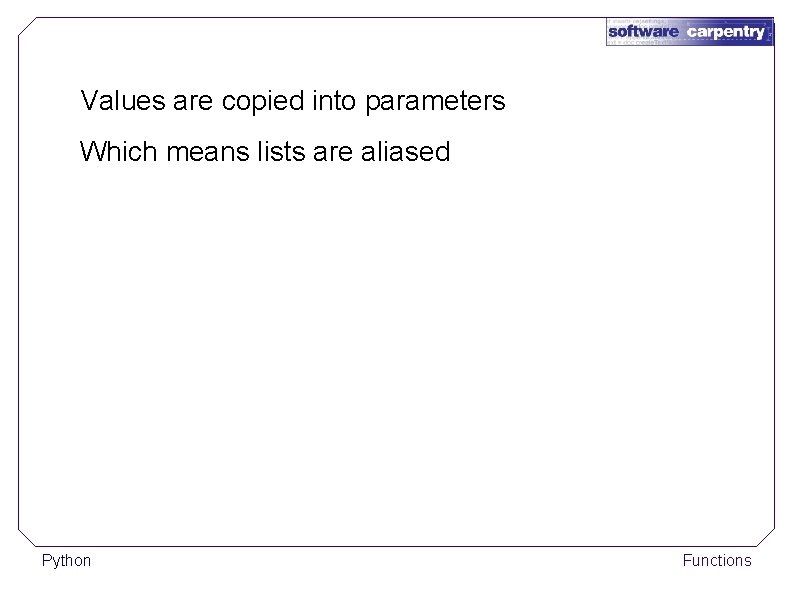
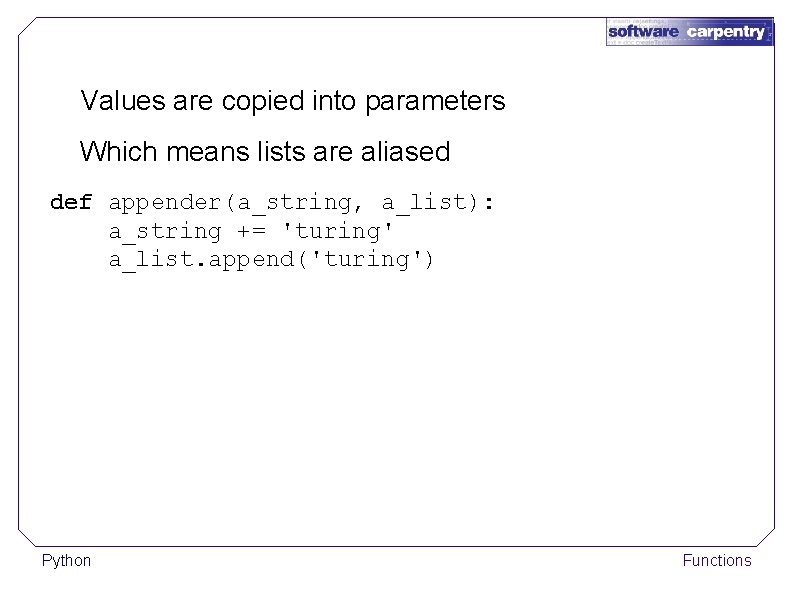
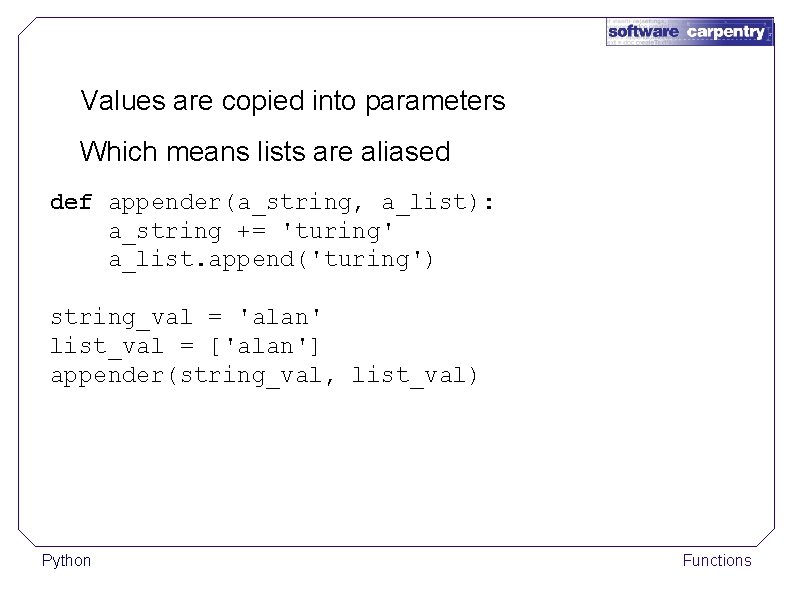
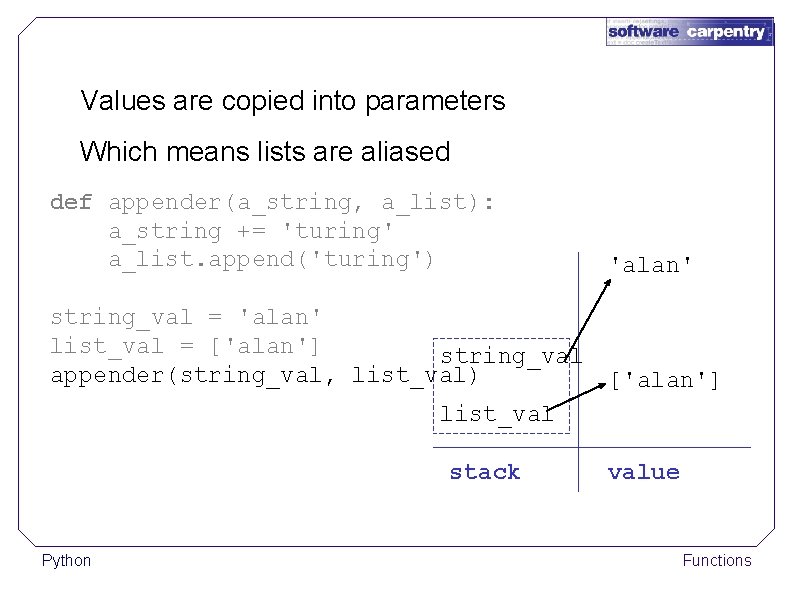
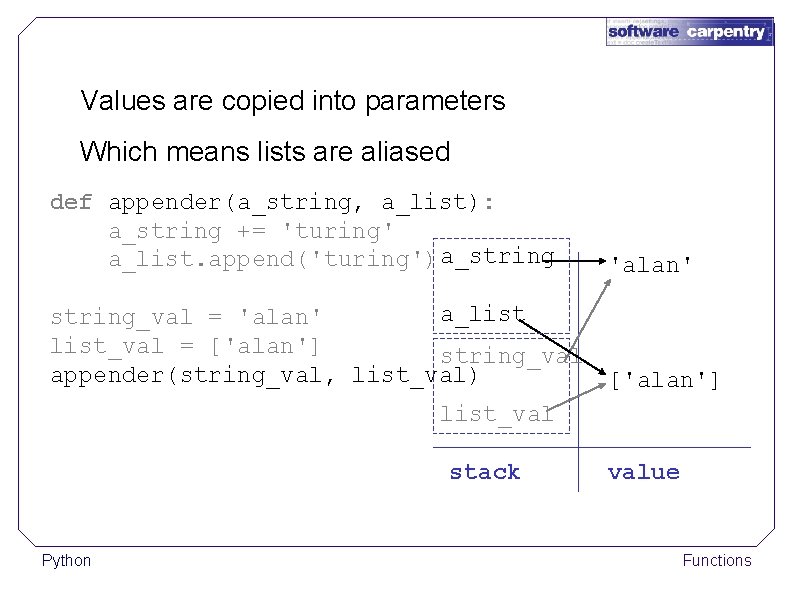
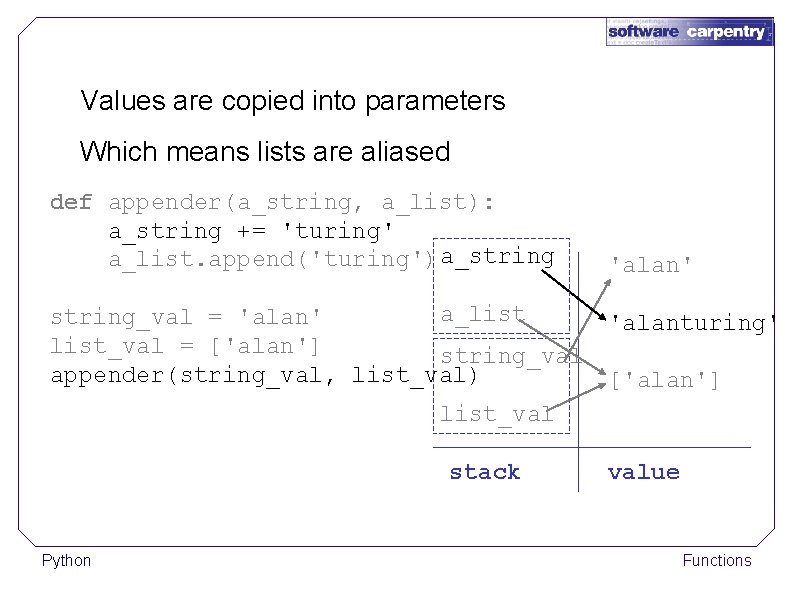
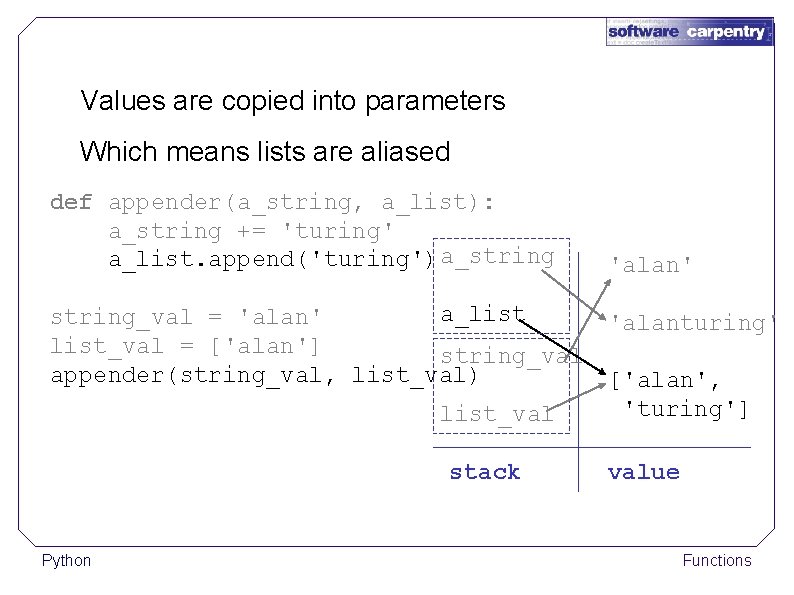
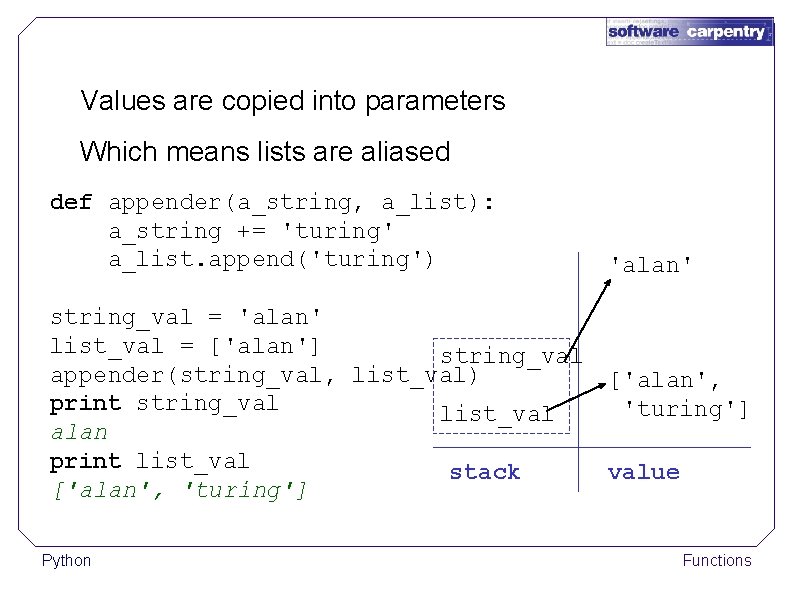
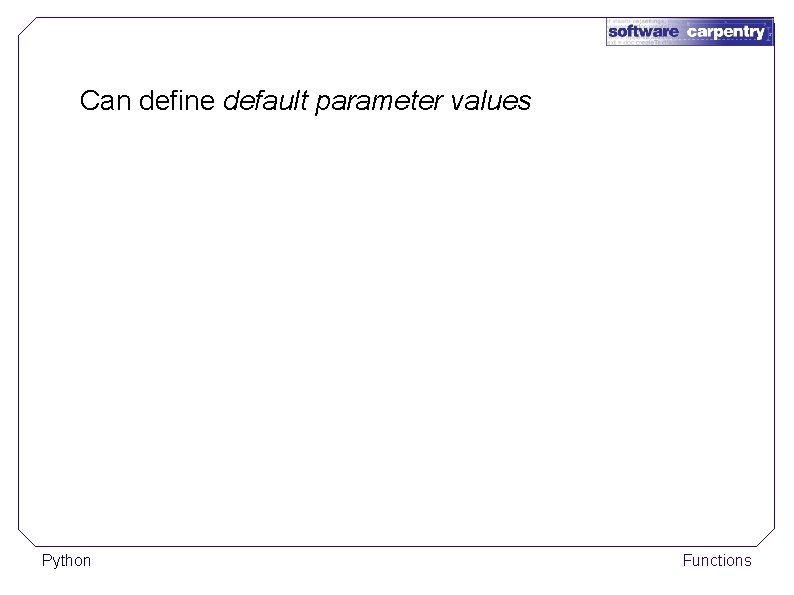
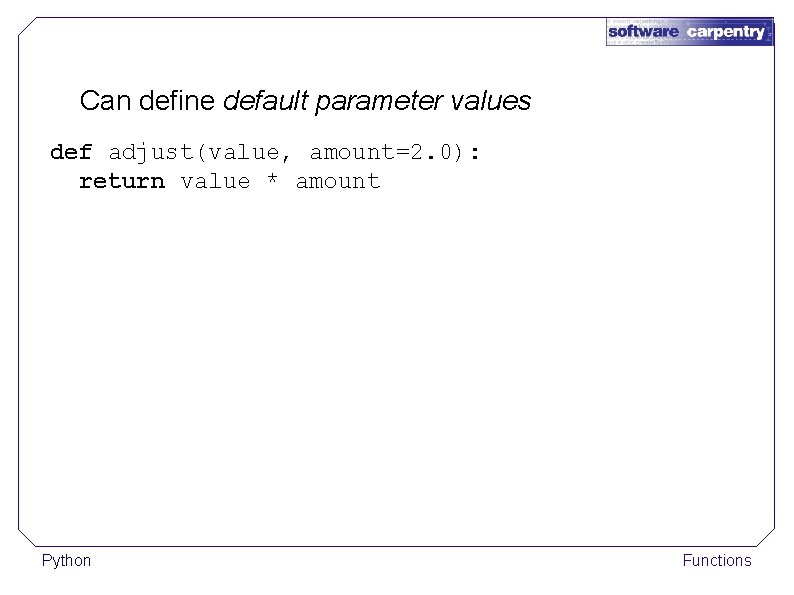
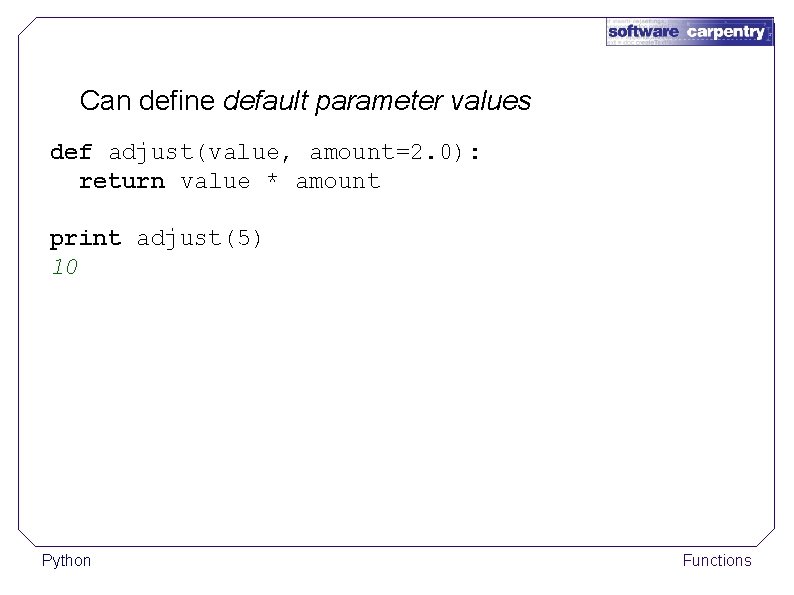
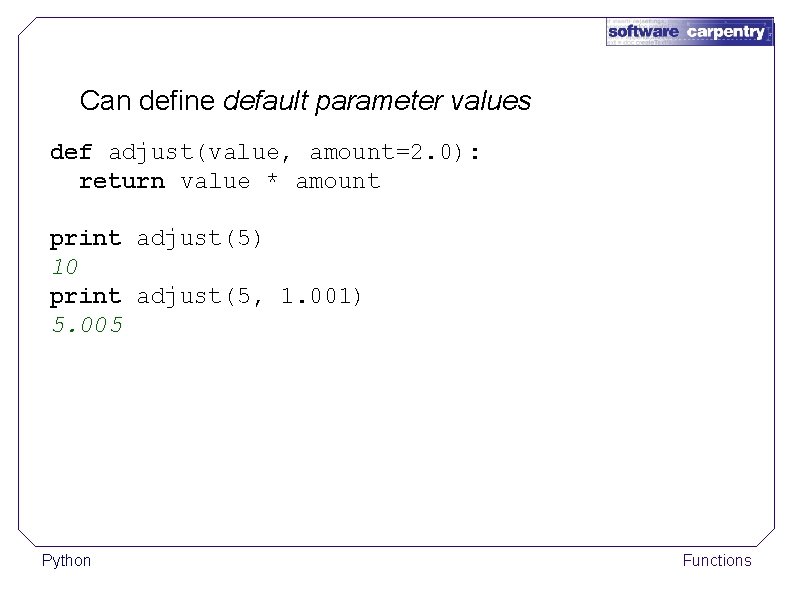
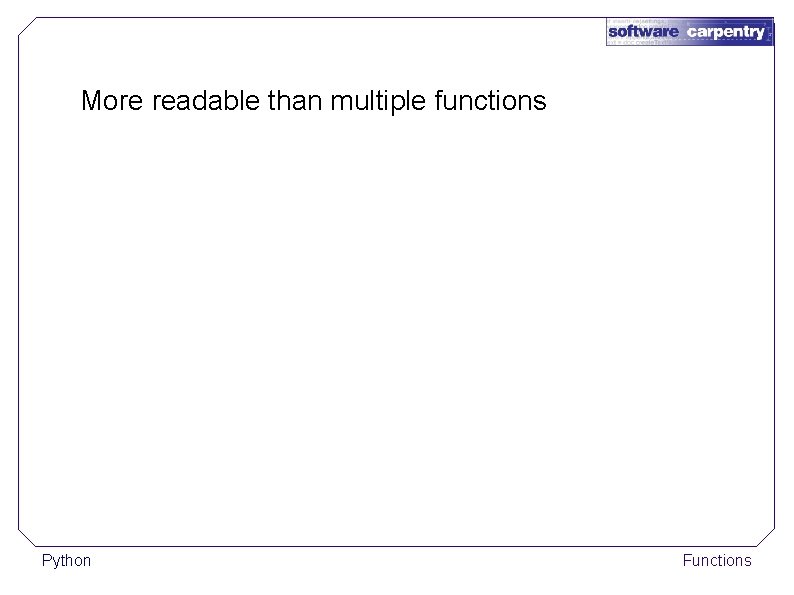
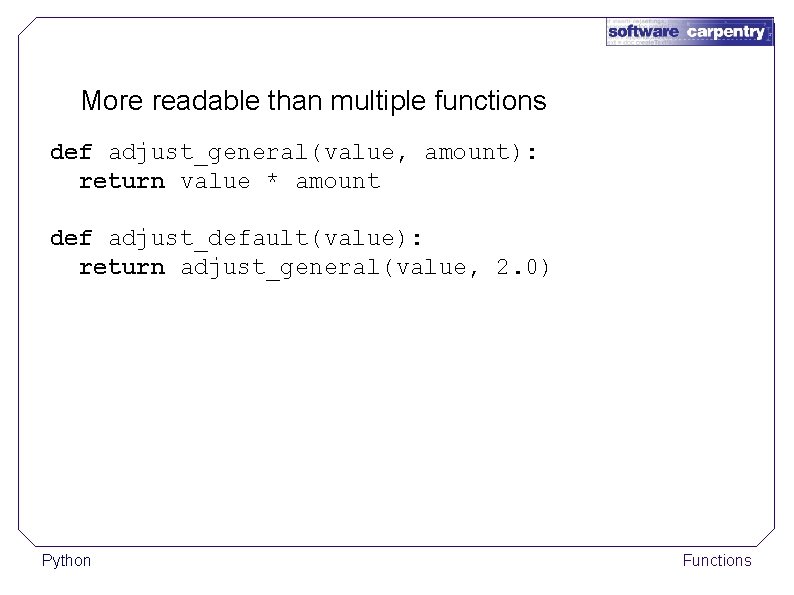
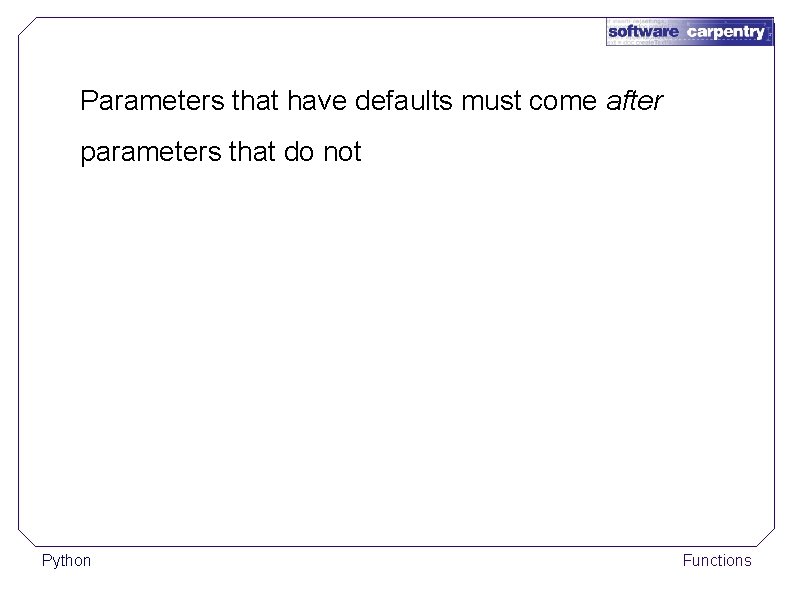
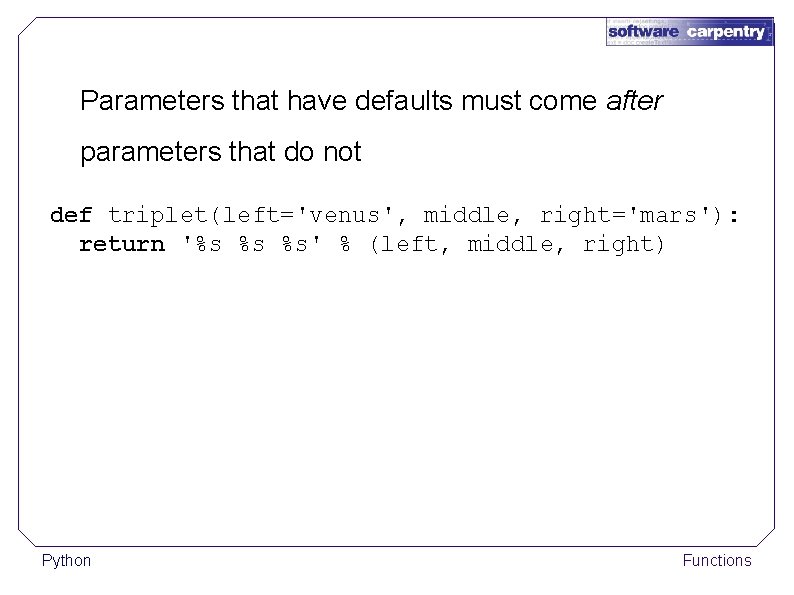
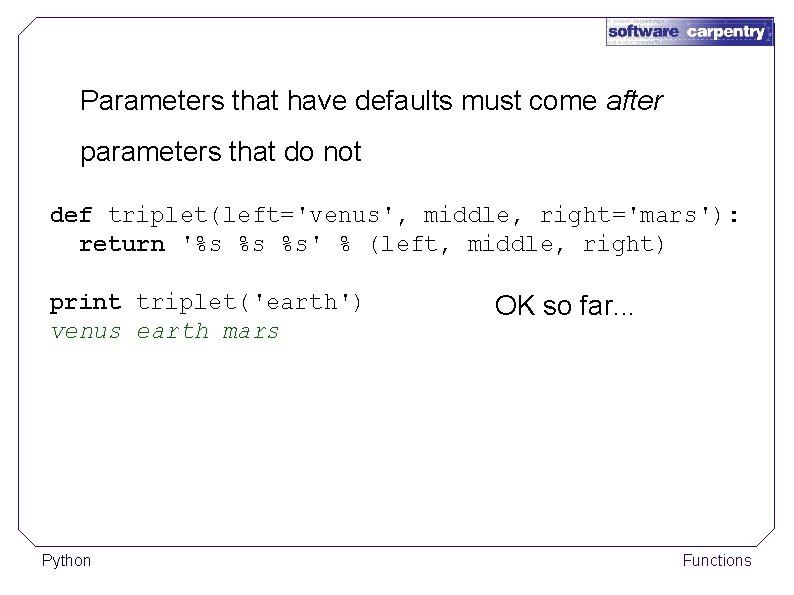
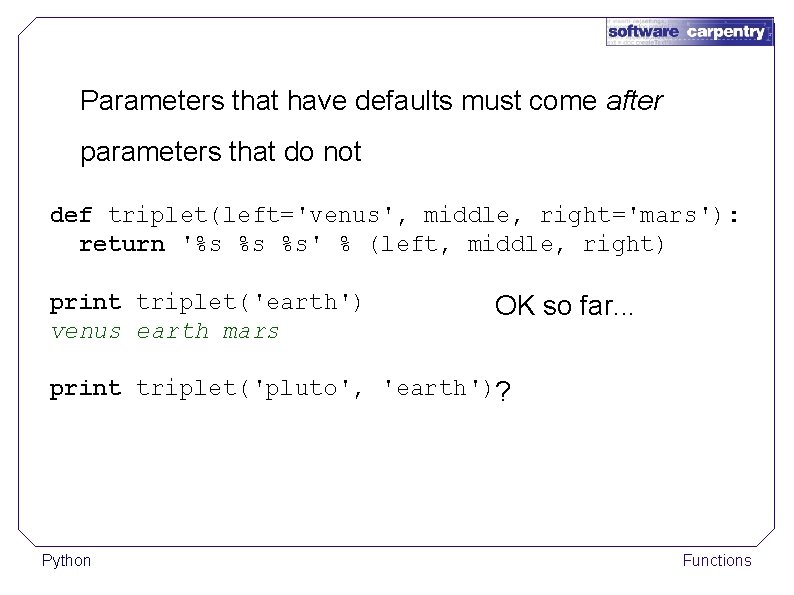
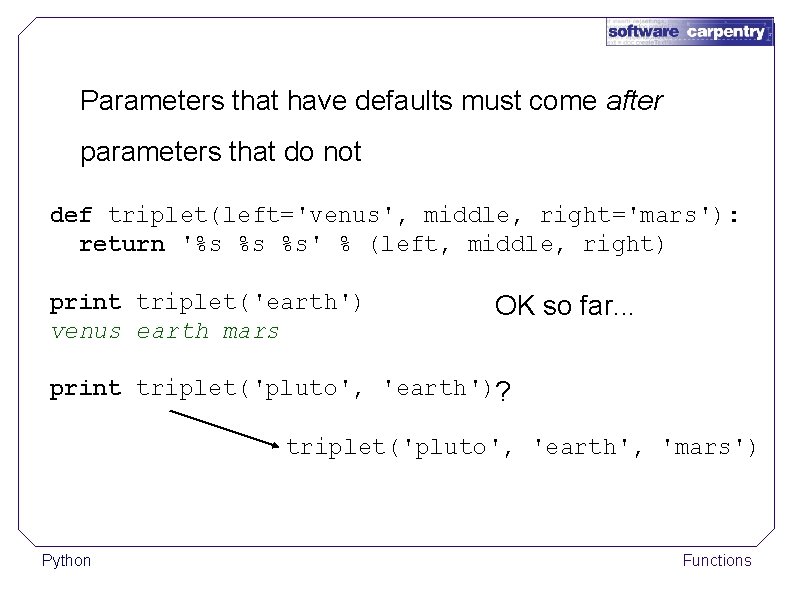
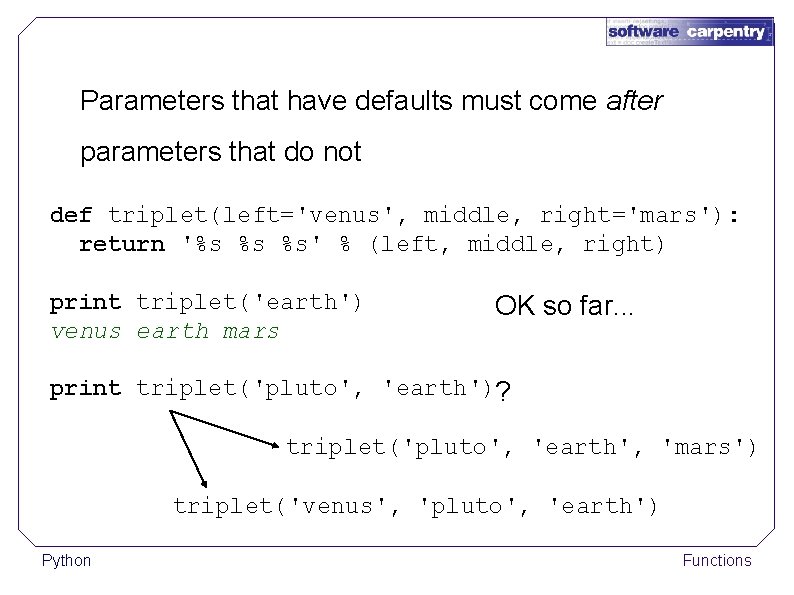
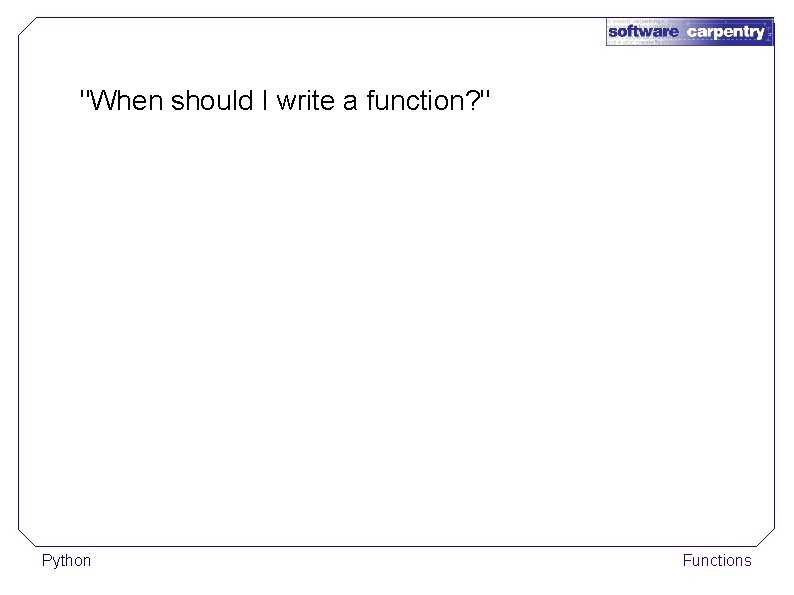
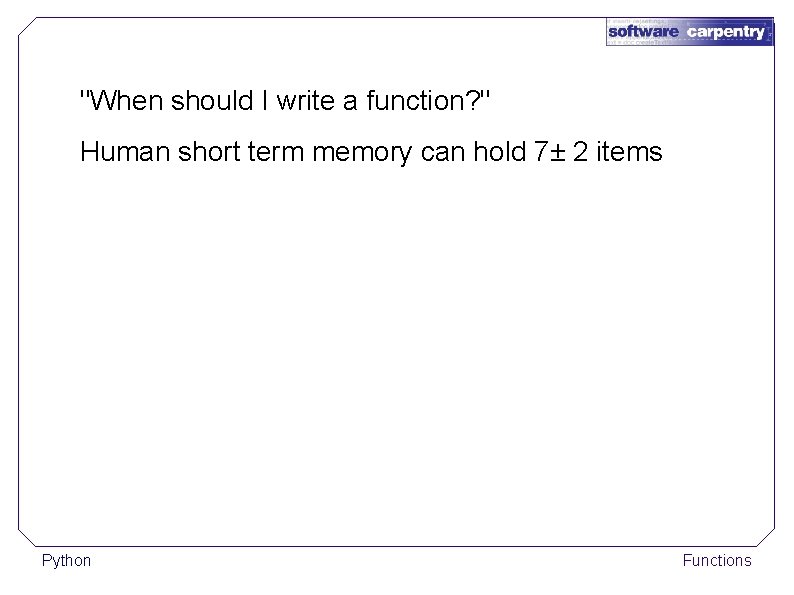
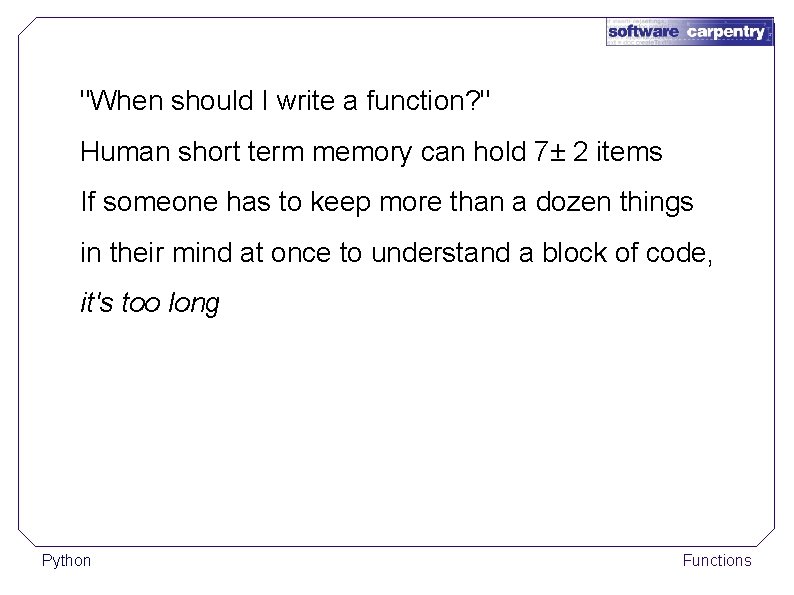
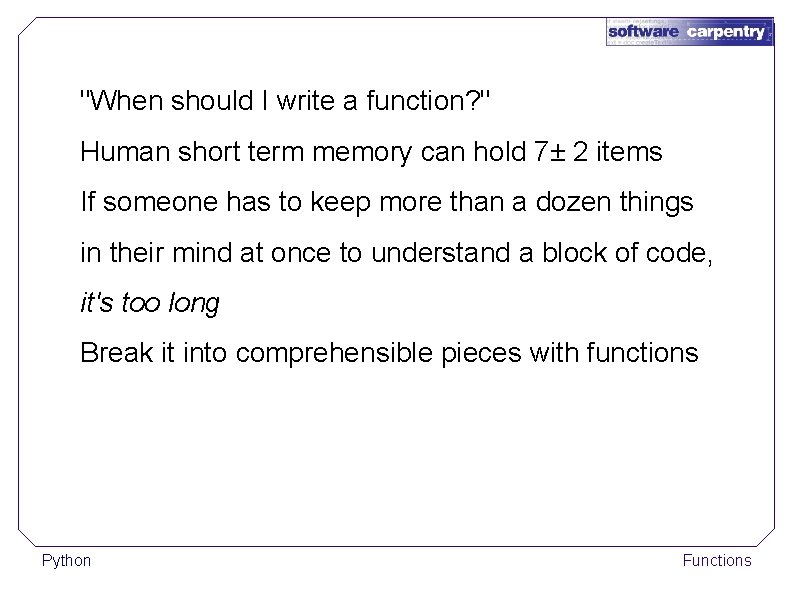
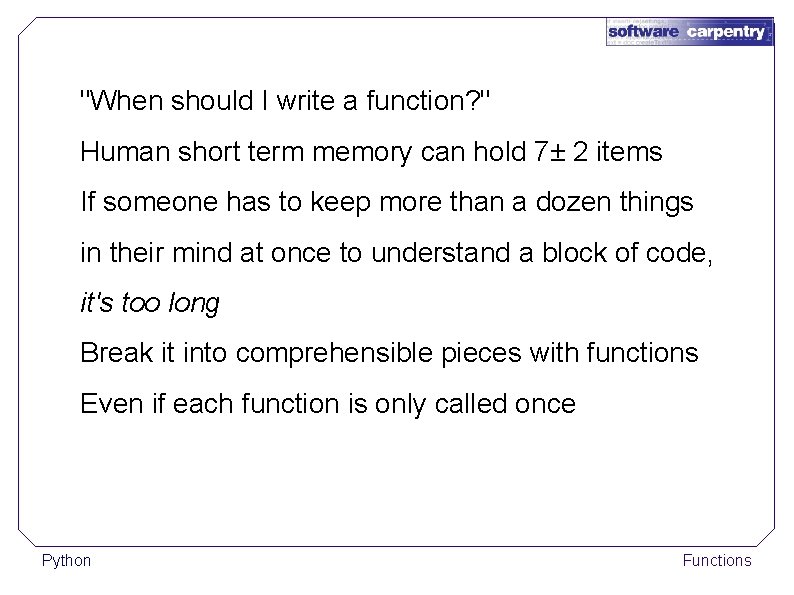
![Example for x in range(1, GRID_WIDTH-1): for y in range(1, GRID_HEIGHT-1): if (density[x-1][y] > Example for x in range(1, GRID_WIDTH-1): for y in range(1, GRID_HEIGHT-1): if (density[x-1][y] >](https://slidetodoc.com/presentation_image/448cd4ea5e49150d34b736a7ce64fbec/image-80.jpg)
![Refactoring #1: grid interior for x in grid_interior(GRID_WIDTH): for y in grid_interior(GRID_HEIGHT): if (density[x-1][y] Refactoring #1: grid interior for x in grid_interior(GRID_WIDTH): for y in grid_interior(GRID_HEIGHT): if (density[x-1][y]](https://slidetodoc.com/presentation_image/448cd4ea5e49150d34b736a7ce64fbec/image-81.jpg)
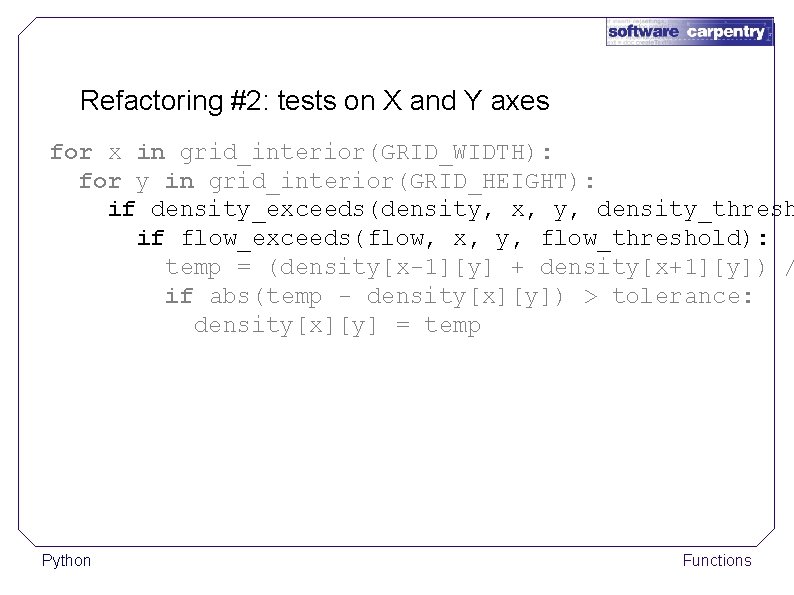
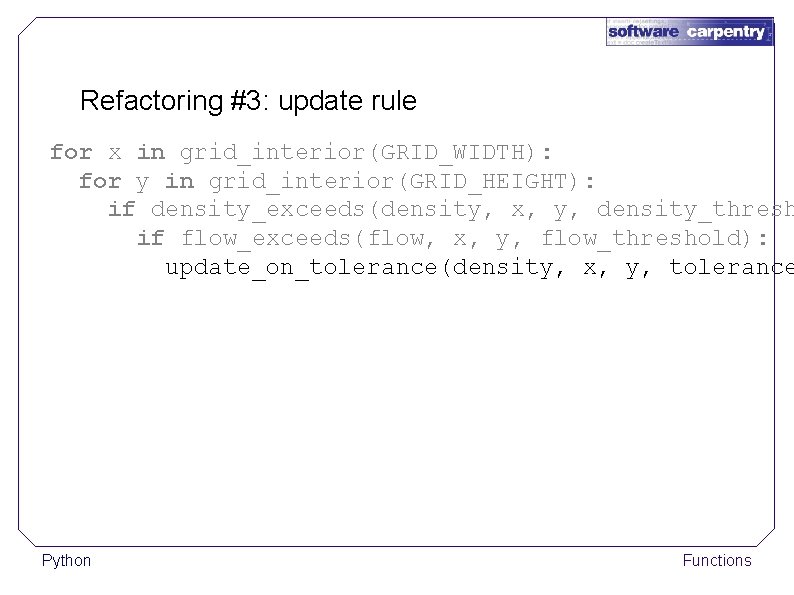
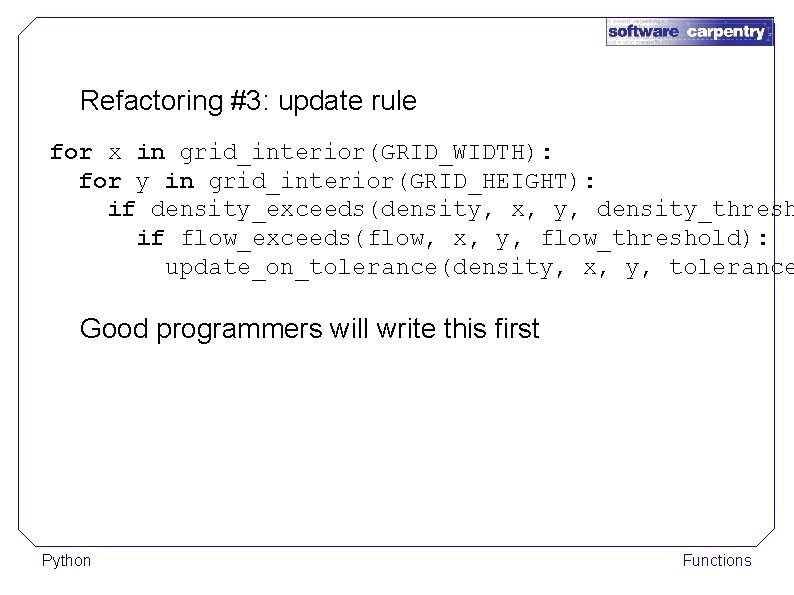
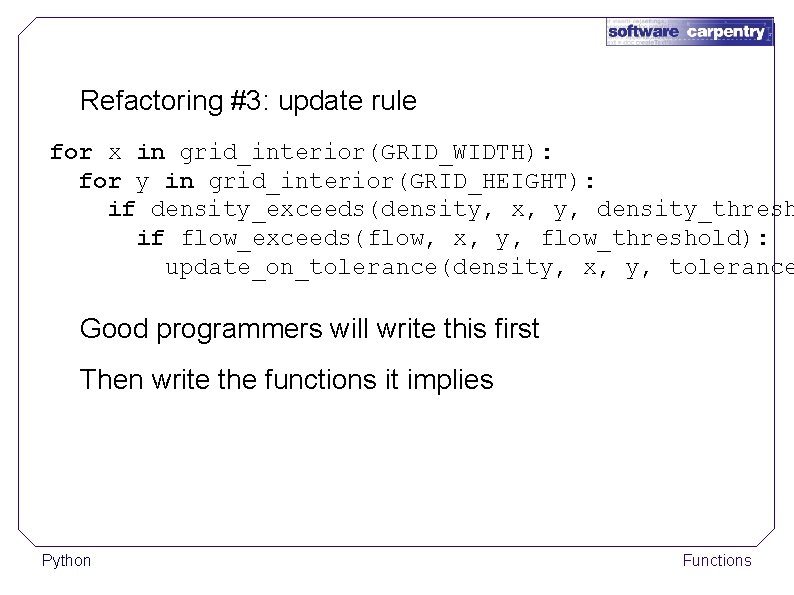
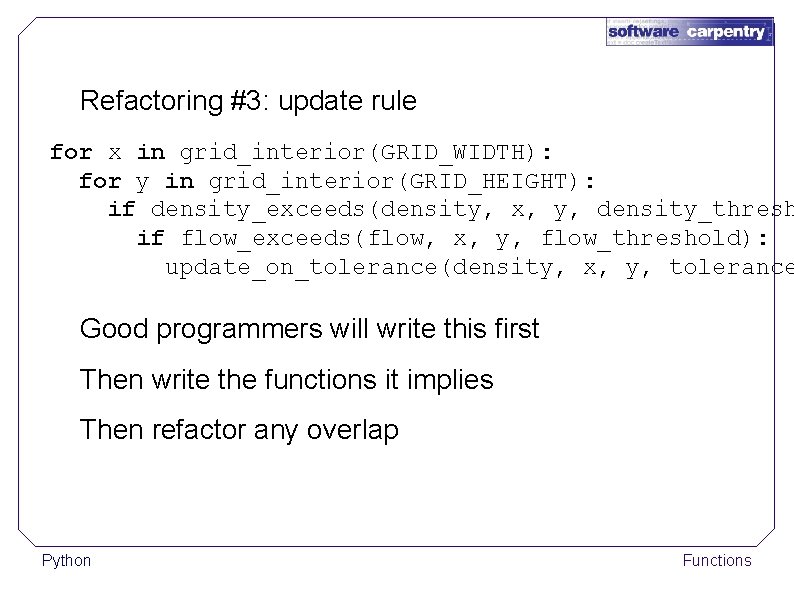
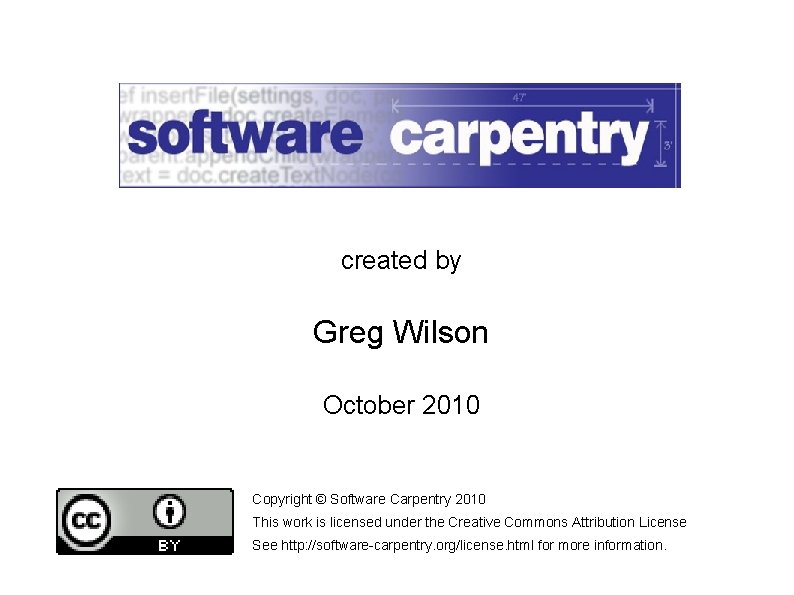
- Slides: 87
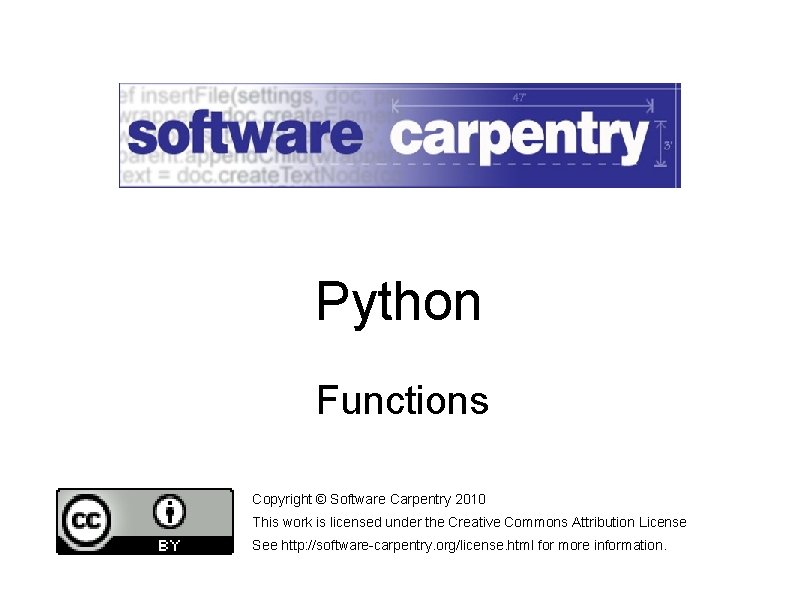
Python Functions Copyright © Software Carpentry 2010 This work is licensed under the Creative Commons Attribution License See http: //software-carpentry. org/license. html for more information.
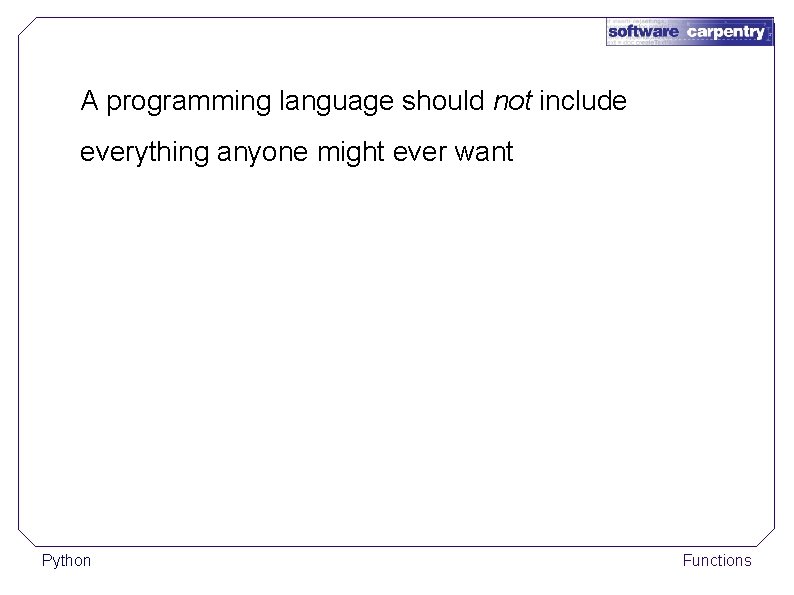
A programming language should not include everything anyone might ever want Python Functions
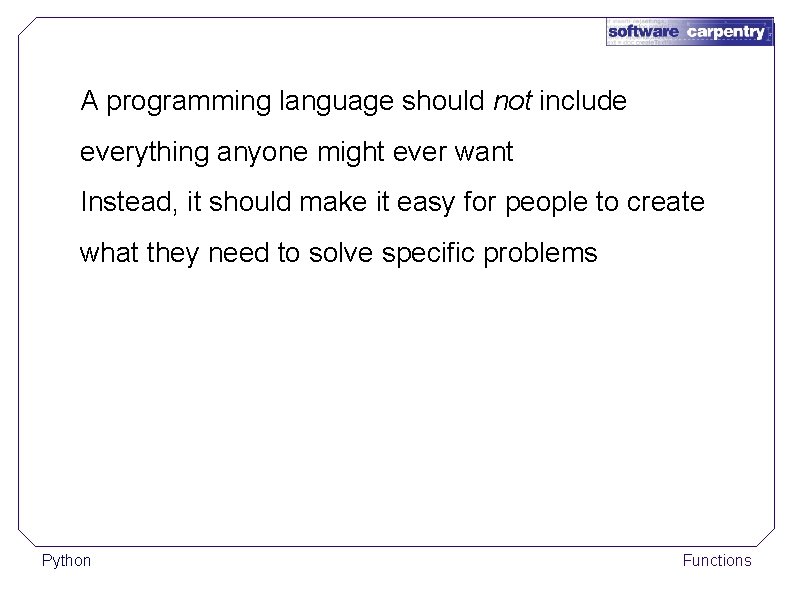
A programming language should not include everything anyone might ever want Instead, it should make it easy for people to create what they need to solve specific problems Python Functions
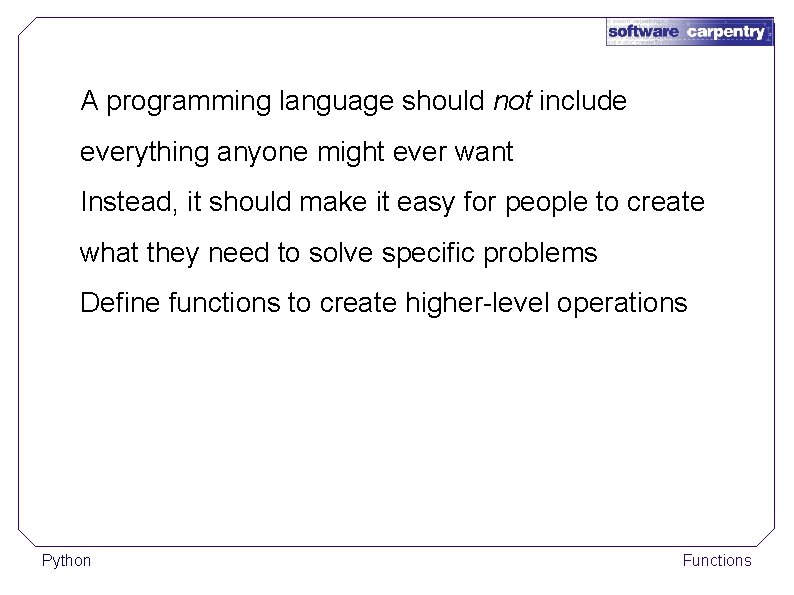
A programming language should not include everything anyone might ever want Instead, it should make it easy for people to create what they need to solve specific problems Define functions to create higher-level operations Python Functions
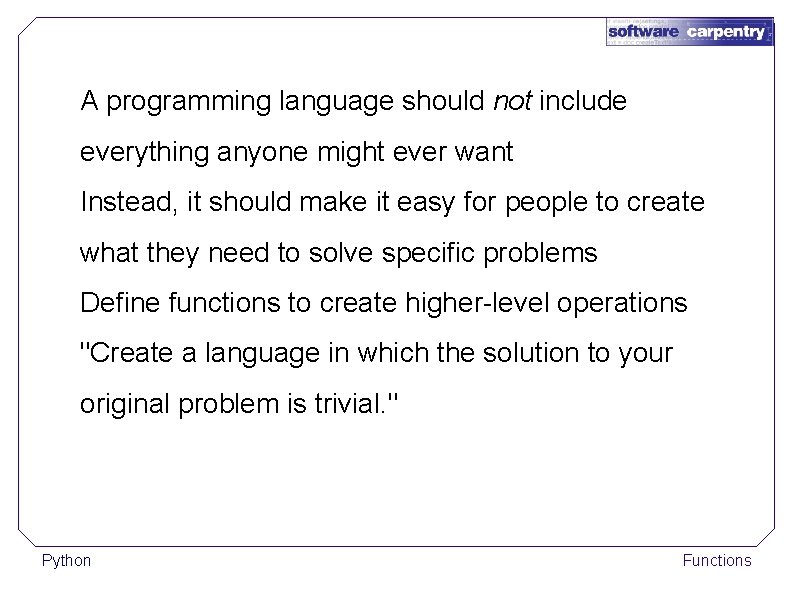
A programming language should not include everything anyone might ever want Instead, it should make it easy for people to create what they need to solve specific problems Define functions to create higher-level operations "Create a language in which the solution to your original problem is trivial. " Python Functions
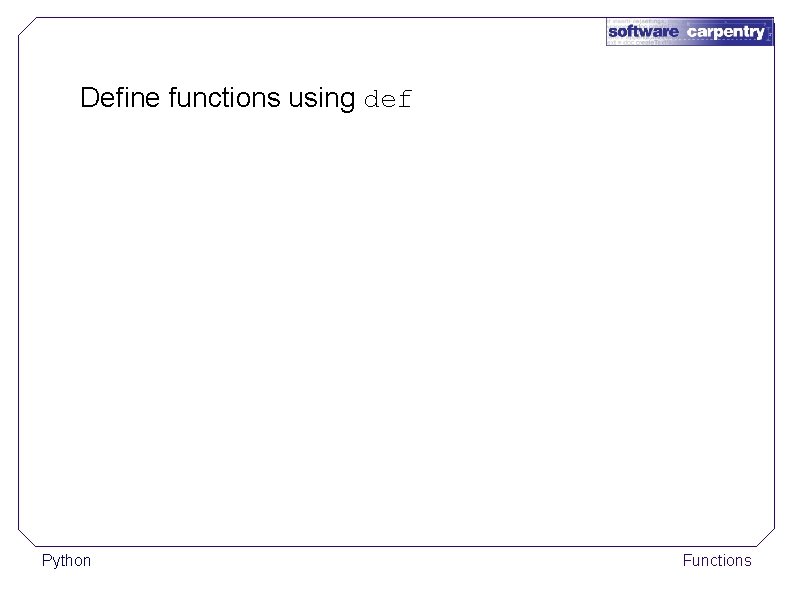
Define functions using def Python Functions
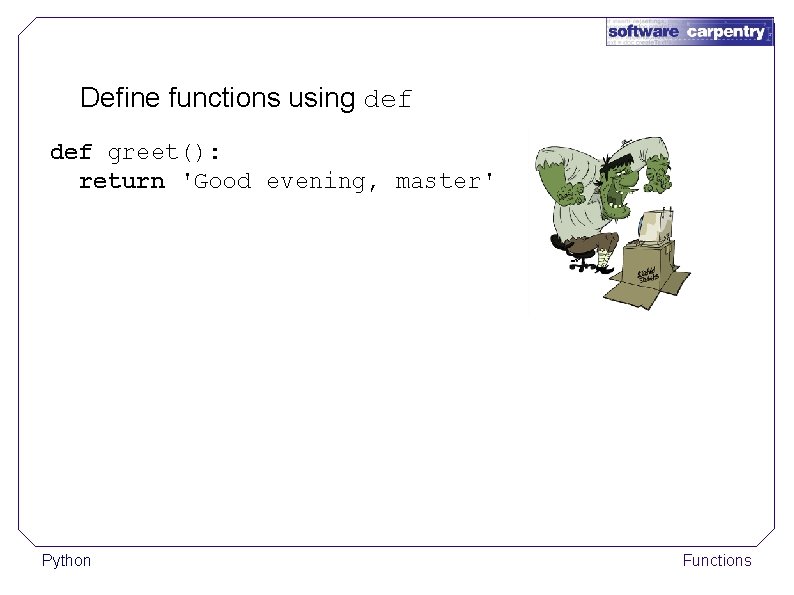
Define functions using def greet(): return 'Good evening, master' Python Functions
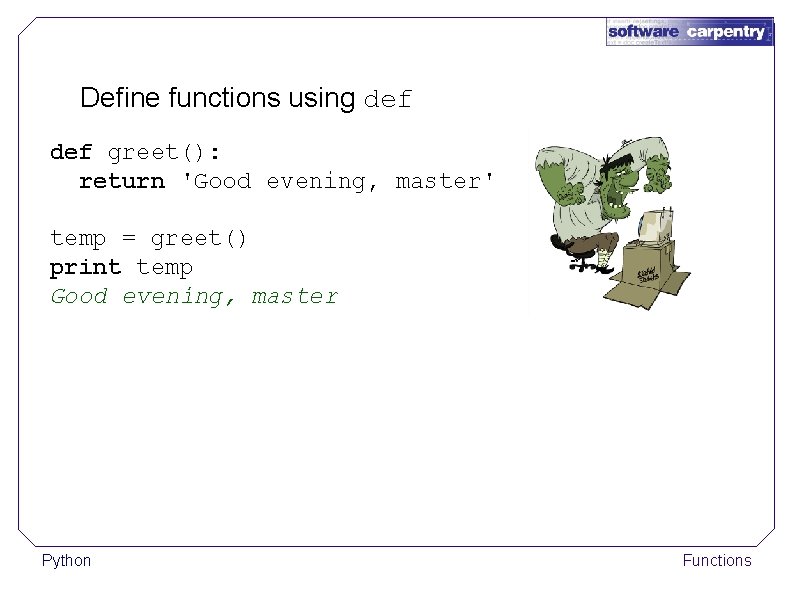
Define functions using def greet(): return 'Good evening, master' temp = greet() print temp Good evening, master Python Functions
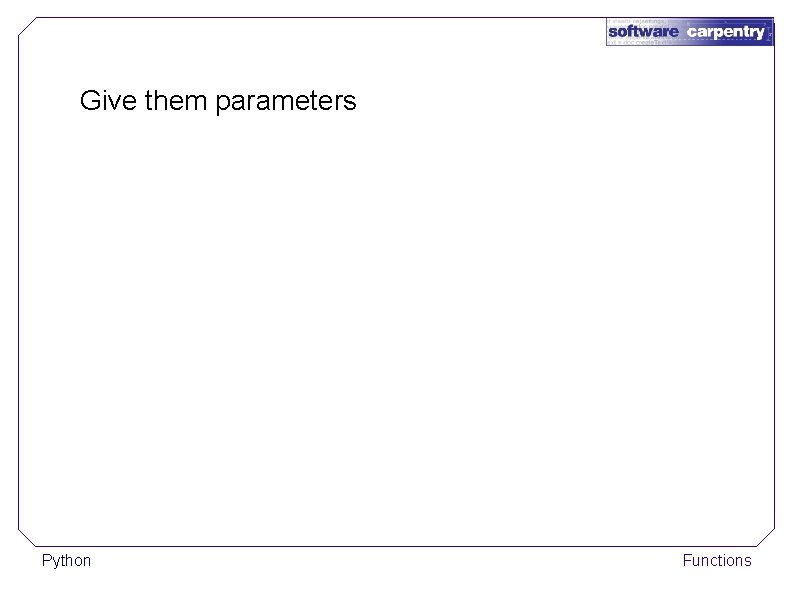
Give them parameters Python Functions
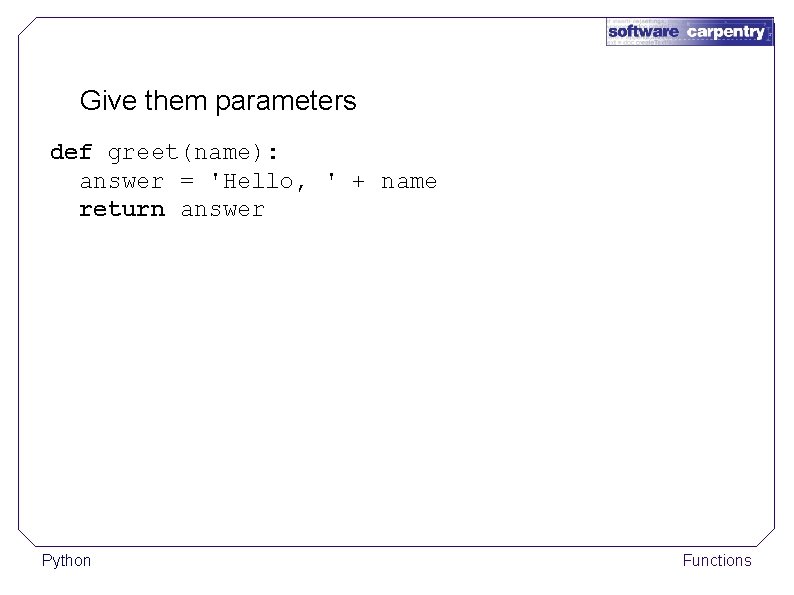
Give them parameters def greet(name): answer = 'Hello, ' + name return answer Python Functions
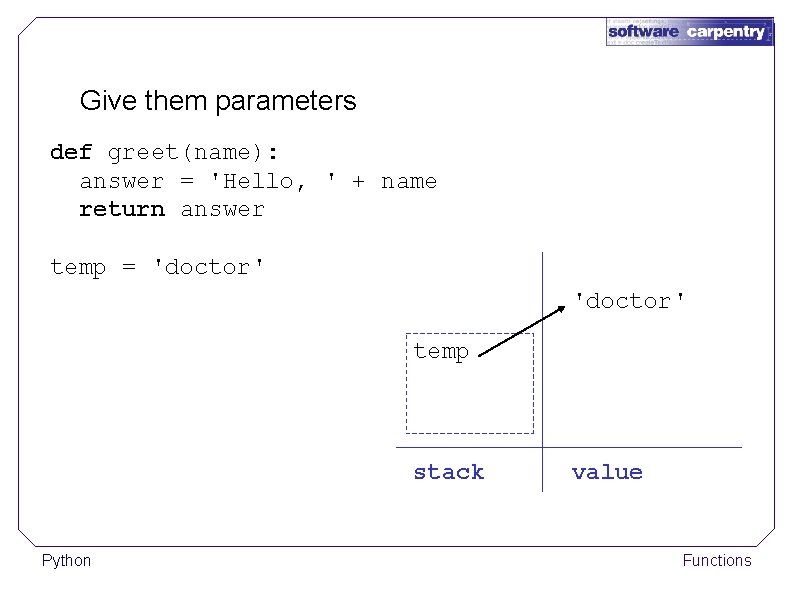
Give them parameters def greet(name): answer = 'Hello, ' + name return answer temp = 'doctor' temp stack Python value Functions
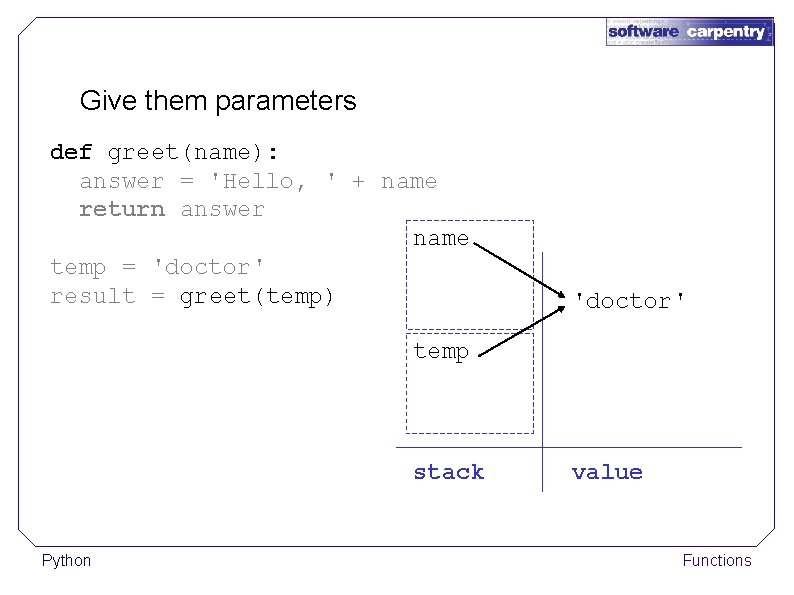
Give them parameters def greet(name): answer = 'Hello, ' + name return answer name temp = 'doctor' result = greet(temp) 'doctor' temp stack Python value Functions
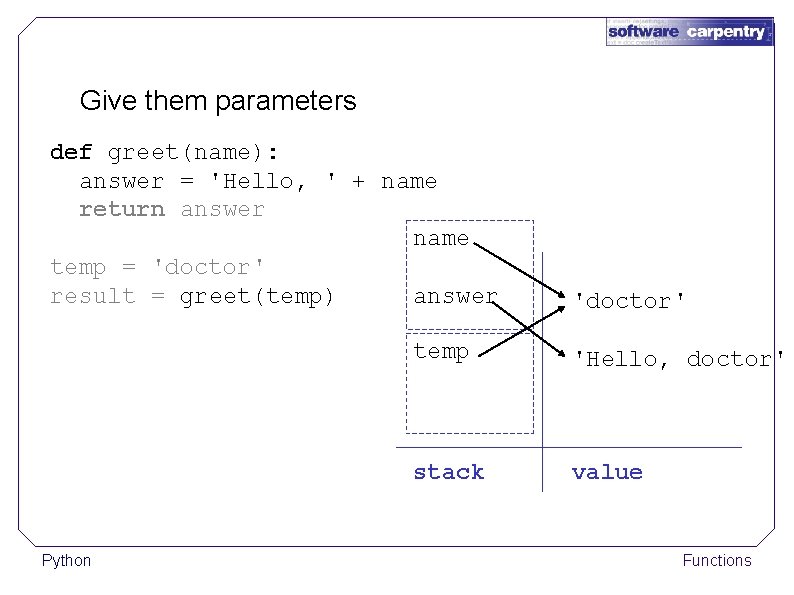
Give them parameters def greet(name): answer = 'Hello, ' + name return answer name temp = 'doctor' answer result = greet(temp) Python 'doctor' temp 'Hello, doctor' stack value Functions
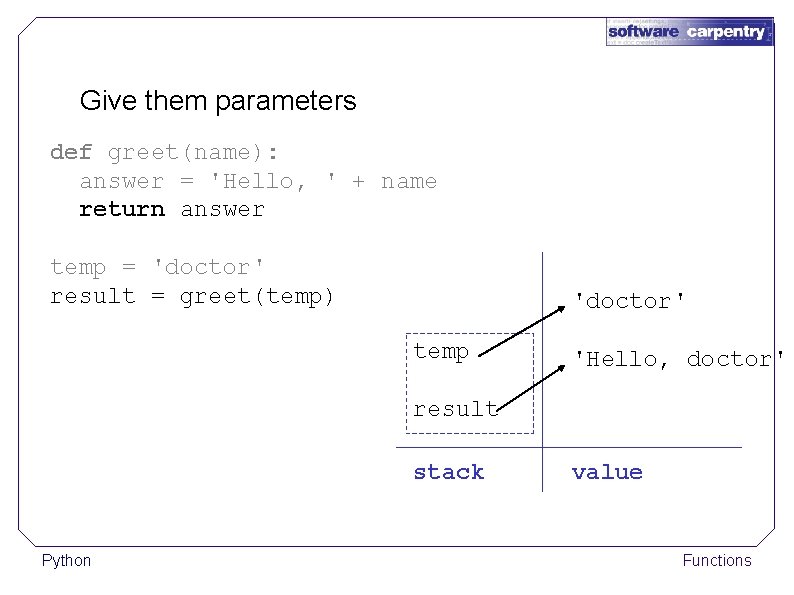
Give them parameters def greet(name): answer = 'Hello, ' + name return answer temp = 'doctor' result = greet(temp) 'doctor' temp 'Hello, doctor' result stack Python value Functions
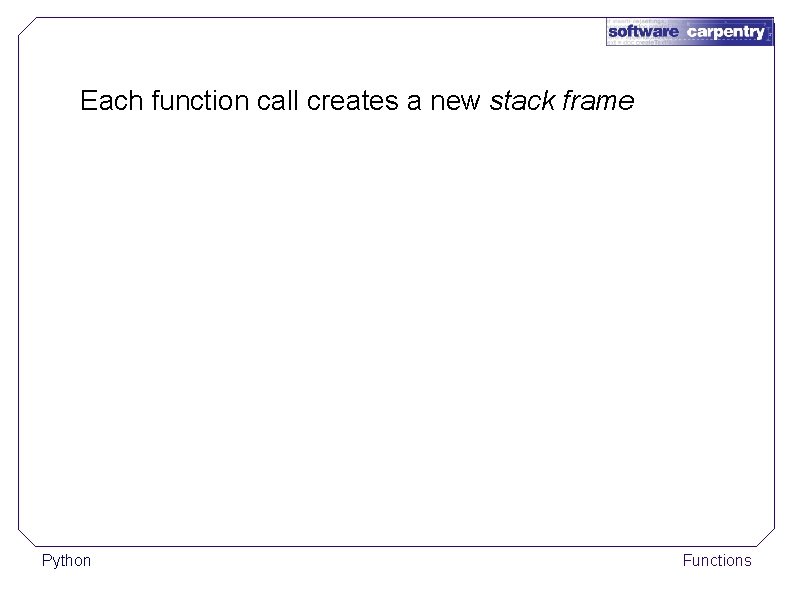
Each function call creates a new stack frame Python Functions
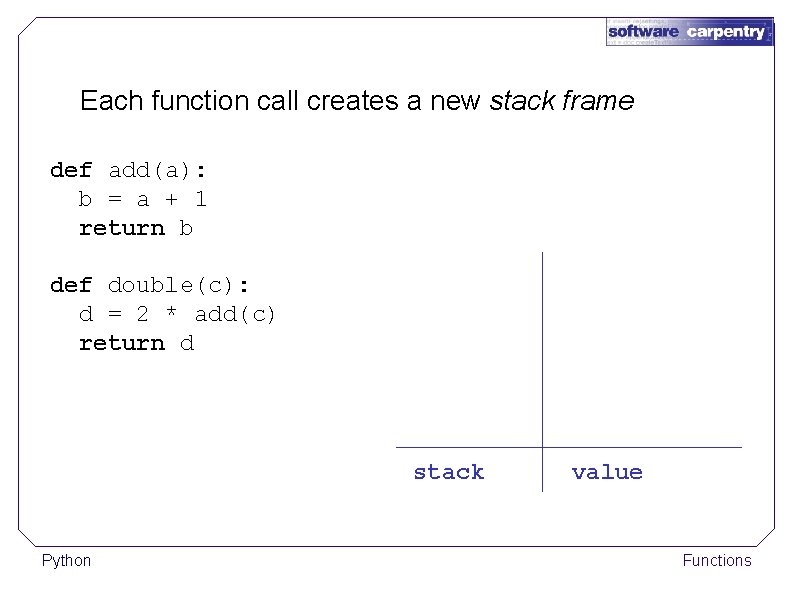
Each function call creates a new stack frame def add(a): b = a + 1 return b def double(c): d = 2 * add(c) return d stack Python value Functions
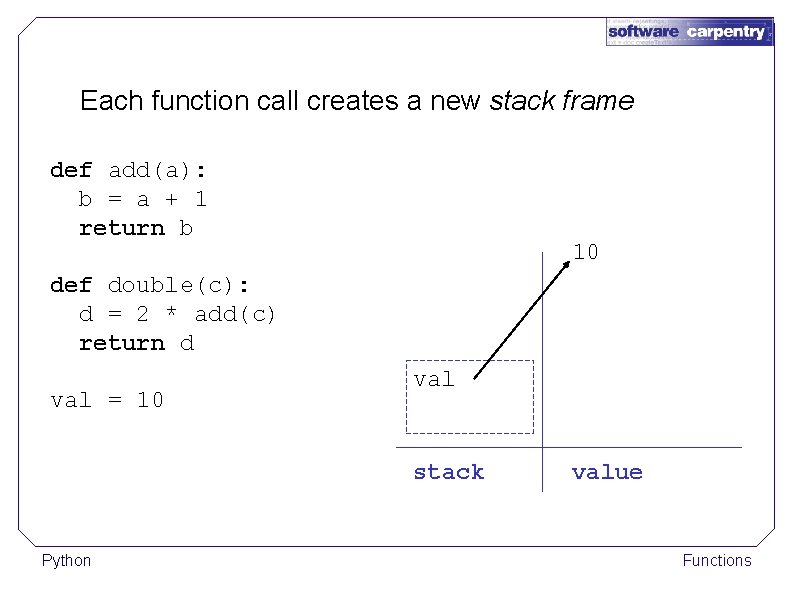
Each function call creates a new stack frame def add(a): b = a + 1 return b 10 def double(c): d = 2 * add(c) return d val = 10 val stack Python value Functions
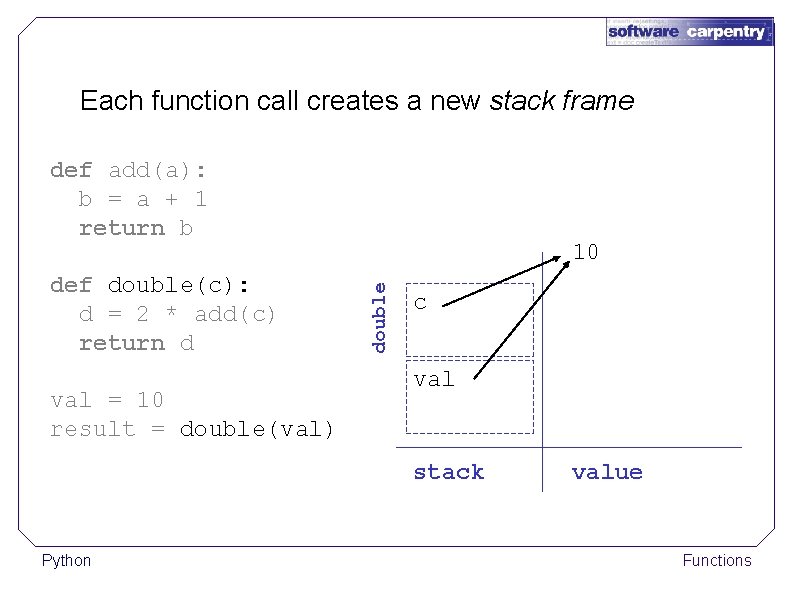
Each function call creates a new stack frame def add(a): b = a + 1 return b val = 10 result = double(val) double def double(c): d = 2 * add(c) return d 10 c val stack Python value Functions
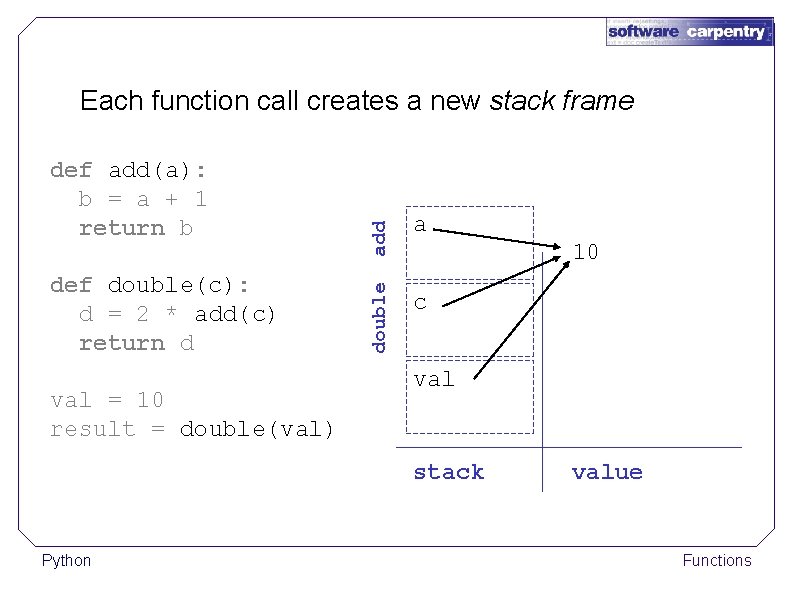
def double(c): d = 2 * add(c) return d val = 10 result = double(val) add def add(a): b = a + 1 return b a double Each function call creates a new stack frame c 10 val stack Python value Functions
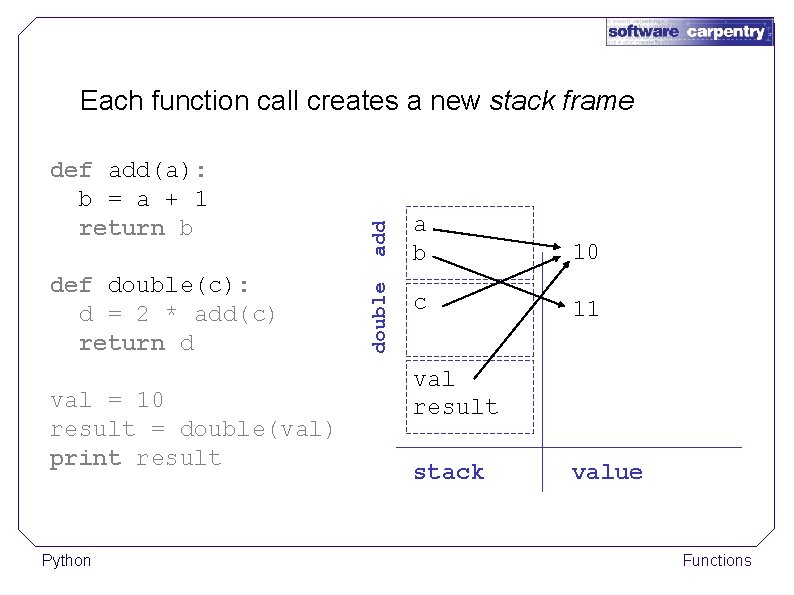
def double(c): d = 2 * add(c) return d val = 10 result = double(val) print result Python add def add(a): b = a + 1 return b a b 10 double Each function call creates a new stack frame c 11 val result stack value Functions
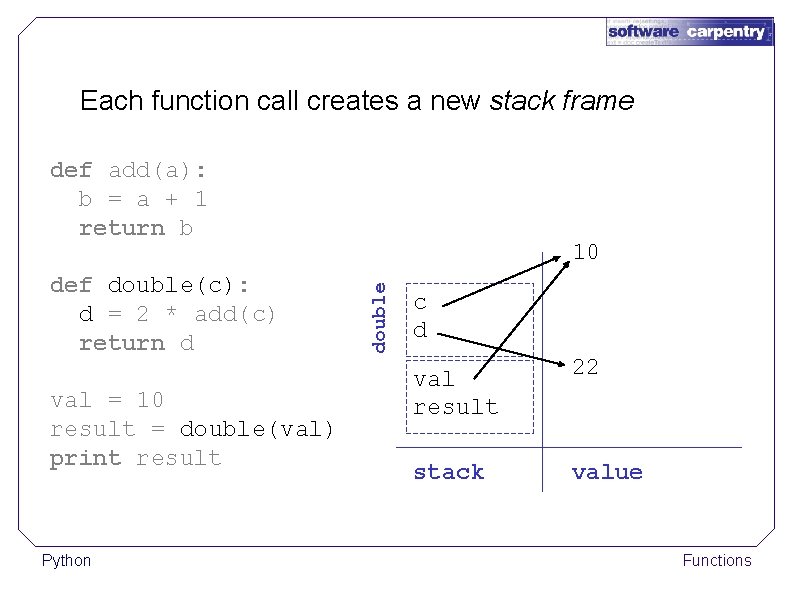
Each function call creates a new stack frame def add(a): b = a + 1 return b val = 10 result = double(val) print result Python double def double(c): d = 2 * add(c) return d 10 c d val result 22 stack value Functions
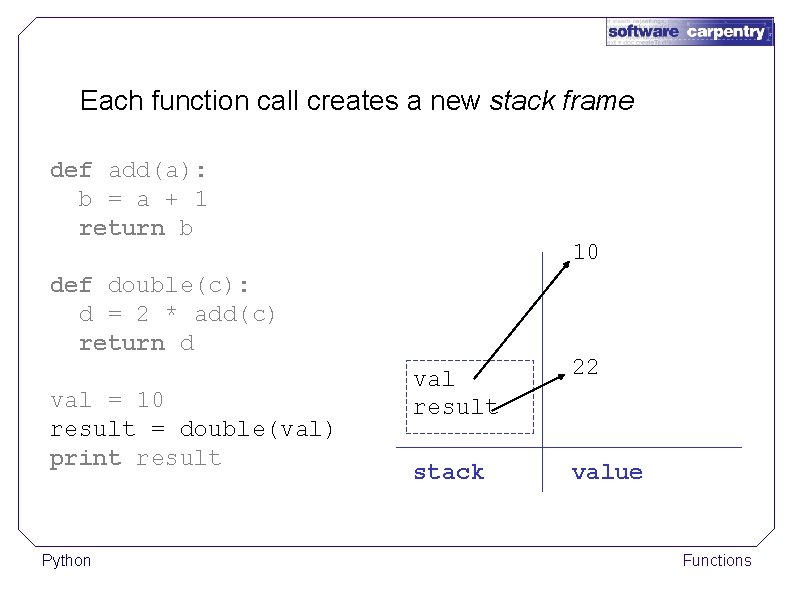
Each function call creates a new stack frame def add(a): b = a + 1 return b 10 def double(c): d = 2 * add(c) return d val = 10 result = double(val) print result Python val result 22 stack value Functions
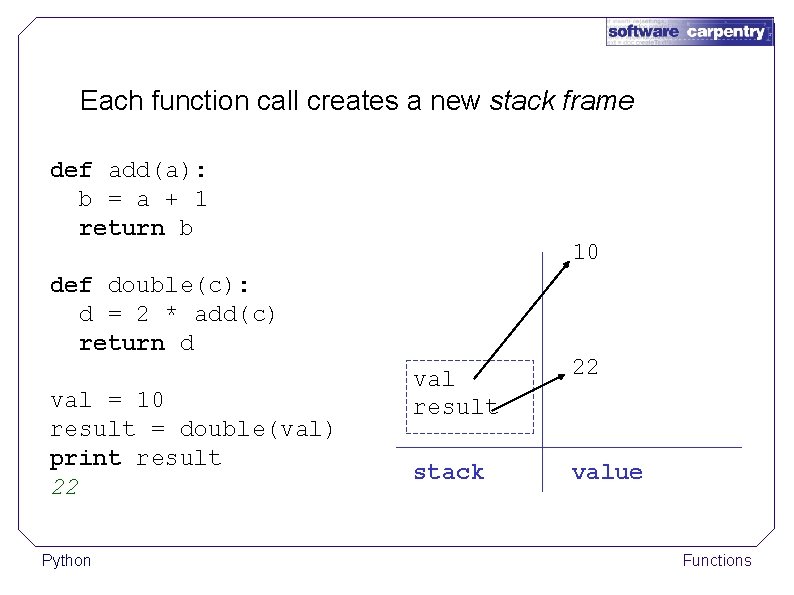
Each function call creates a new stack frame def add(a): b = a + 1 return b 10 def double(c): d = 2 * add(c) return d val = 10 result = double(val) print result 22 Python val result 22 stack value Functions
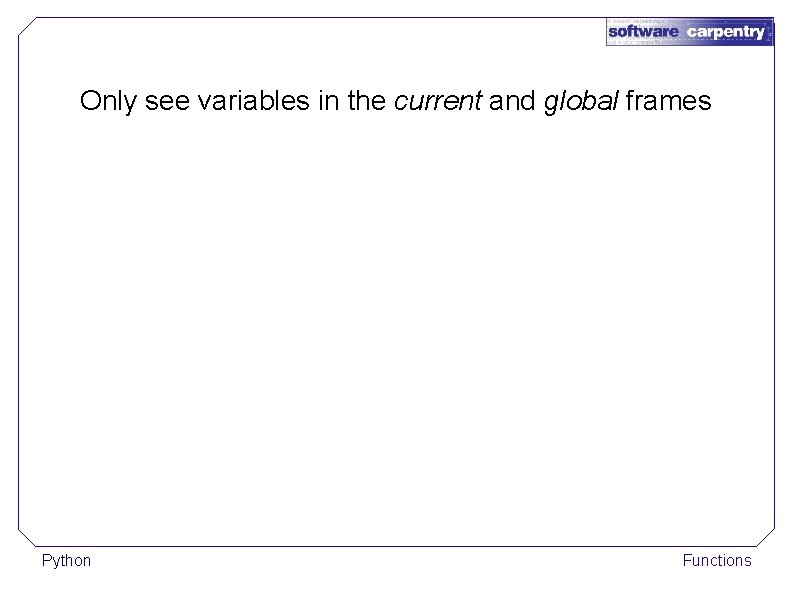
Only see variables in the current and global frames Python Functions
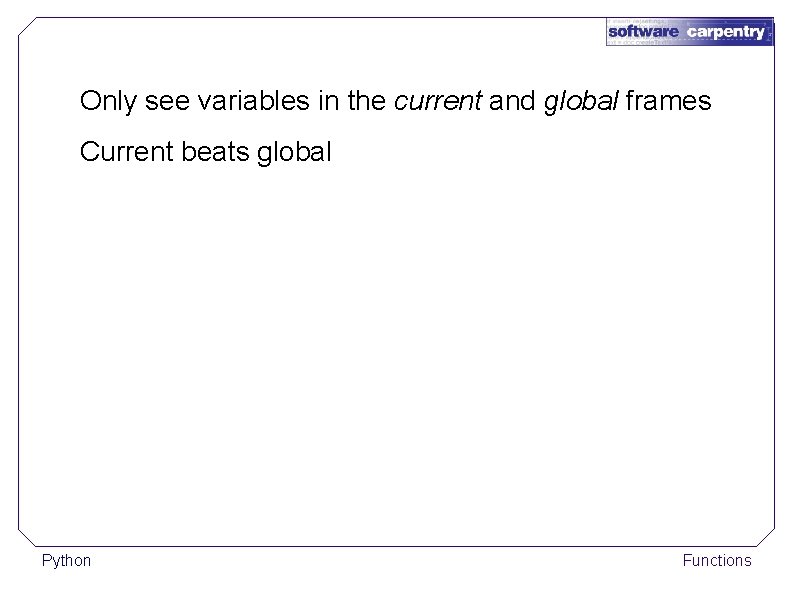
Only see variables in the current and global frames Current beats global Python Functions
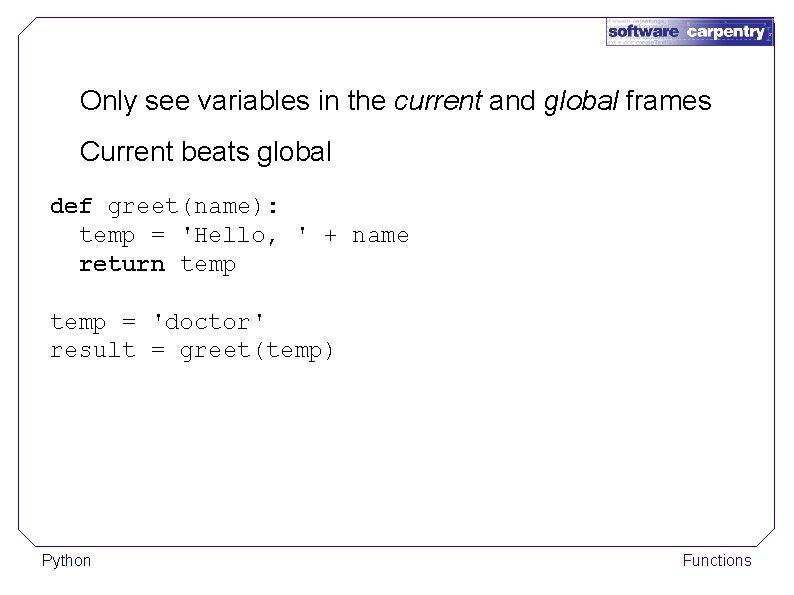
Only see variables in the current and global frames Current beats global def greet(name): temp = 'Hello, ' + name return temp = 'doctor' result = greet(temp) Python Functions
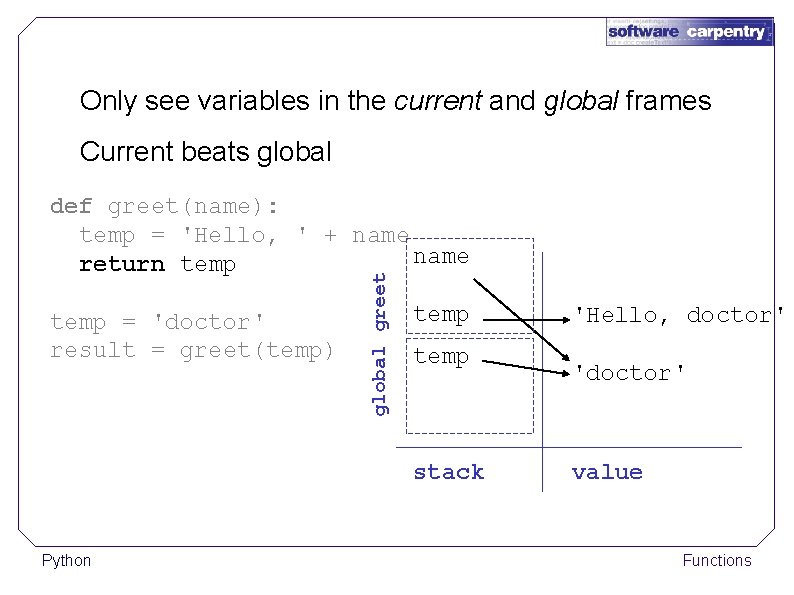
Only see variables in the current and global frames Current beats global temp = 'doctor' result = greet(temp) global greet def greet(name): temp = 'Hello, ' + name return temp stack Python 'Hello, doctor' 'doctor' value Functions
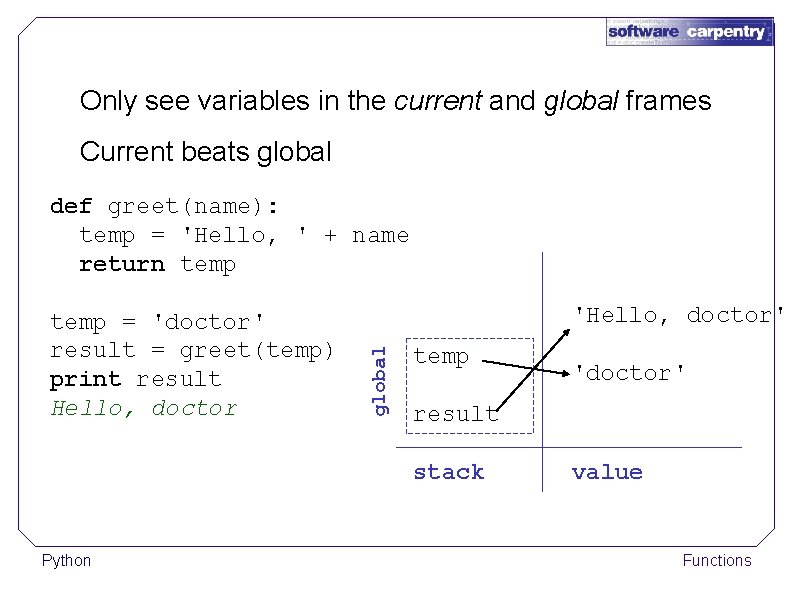
Only see variables in the current and global frames Current beats global def greet(name): temp = 'Hello, ' + name return temp 'Hello, doctor' global temp = 'doctor' result = greet(temp) print result Hello, doctor temp result stack Python 'doctor' value Functions
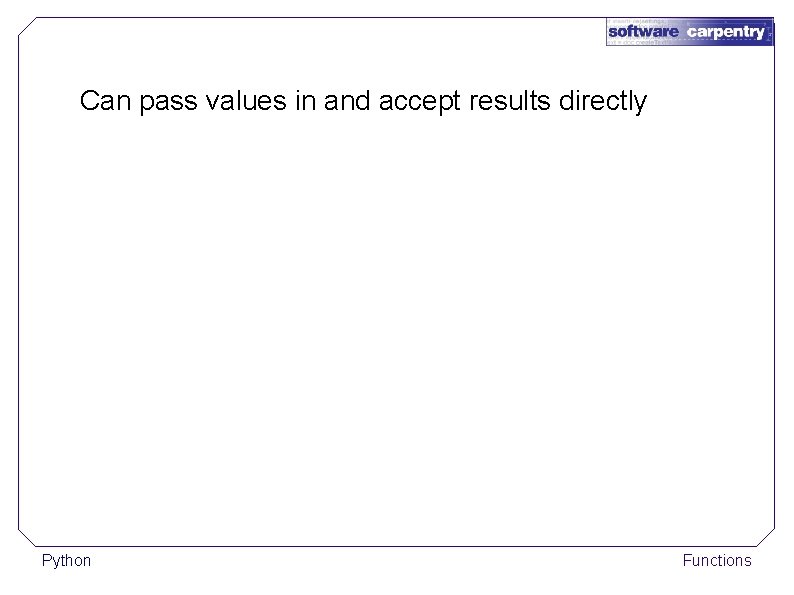
Can pass values in and accept results directly Python Functions
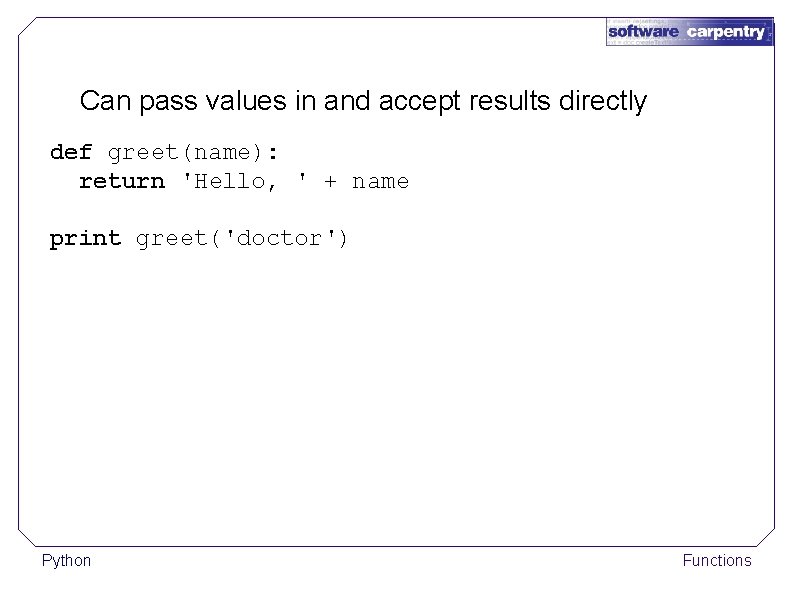
Can pass values in and accept results directly def greet(name): return 'Hello, ' + name print greet('doctor') Python Functions
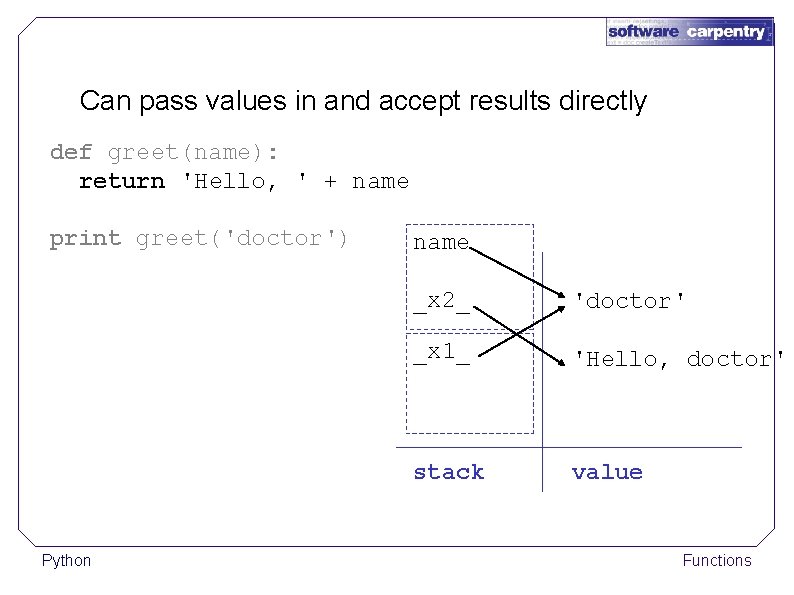
Can pass values in and accept results directly def greet(name): return 'Hello, ' + name print greet('doctor') Python name _x 2_ 'doctor' _x 1_ 'Hello, doctor' stack value Functions
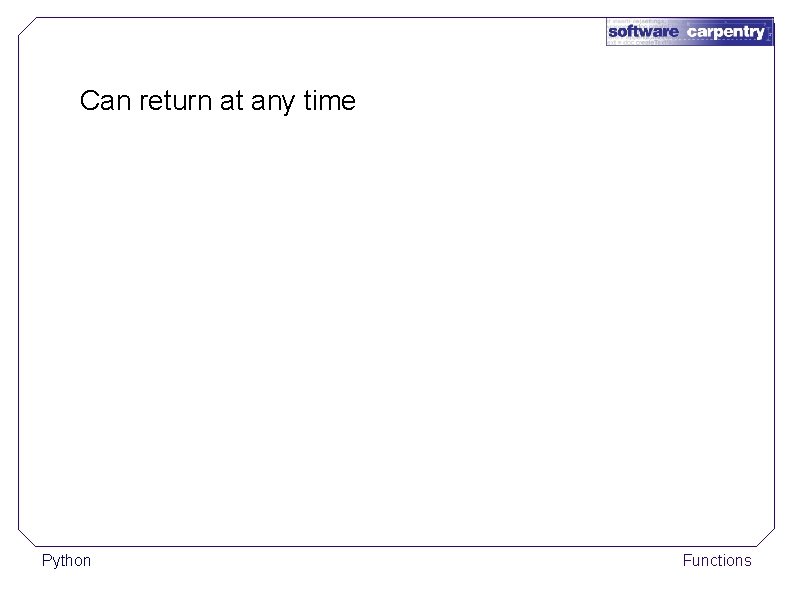
Can return at any time Python Functions
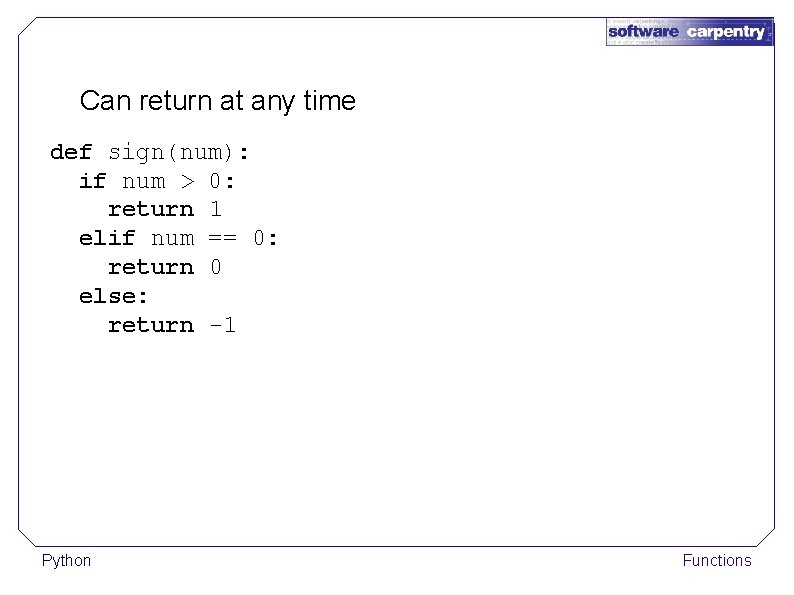
Can return at any time def sign(num): if num > 0: return 1 elif num == 0: return 0 else: return -1 Python Functions
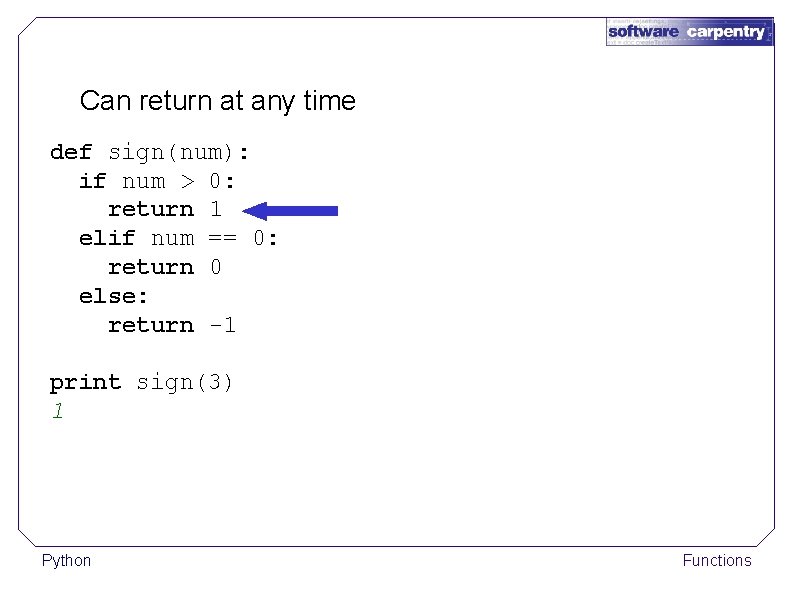
Can return at any time def sign(num): if num > 0: return 1 elif num == 0: return 0 else: return -1 print sign(3) 1 Python Functions
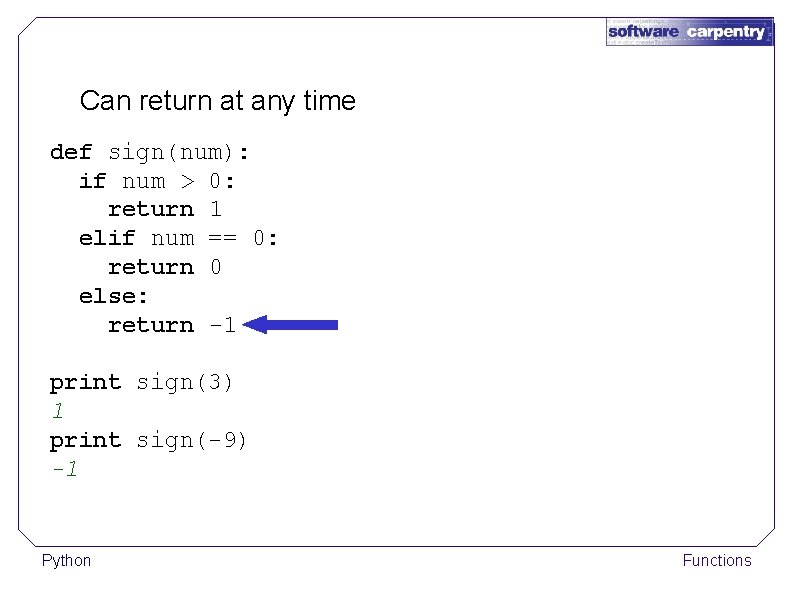
Can return at any time def sign(num): if num > 0: return 1 elif num == 0: return 0 else: return -1 print sign(3) 1 print sign(-9) -1 Python Functions
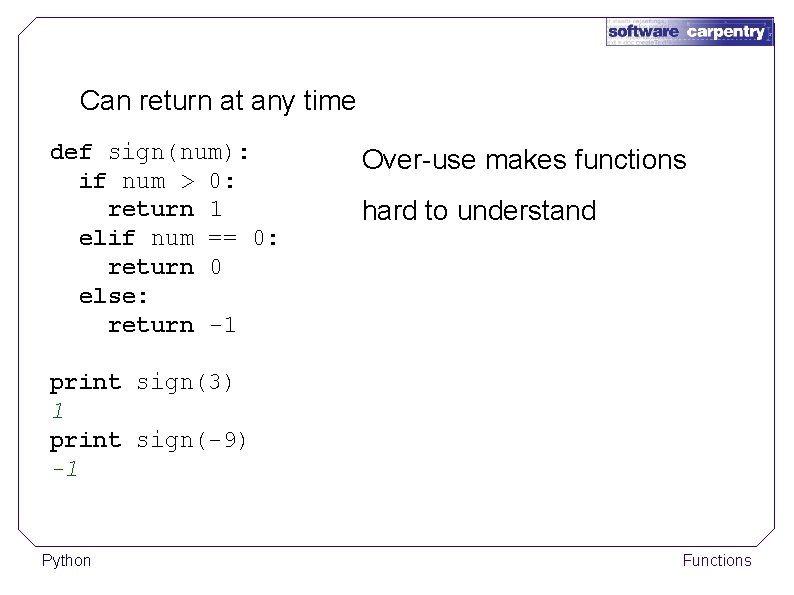
Can return at any time def sign(num): if num > 0: return 1 elif num == 0: return 0 else: return -1 Over-use makes functions hard to understand print sign(3) 1 print sign(-9) -1 Python Functions
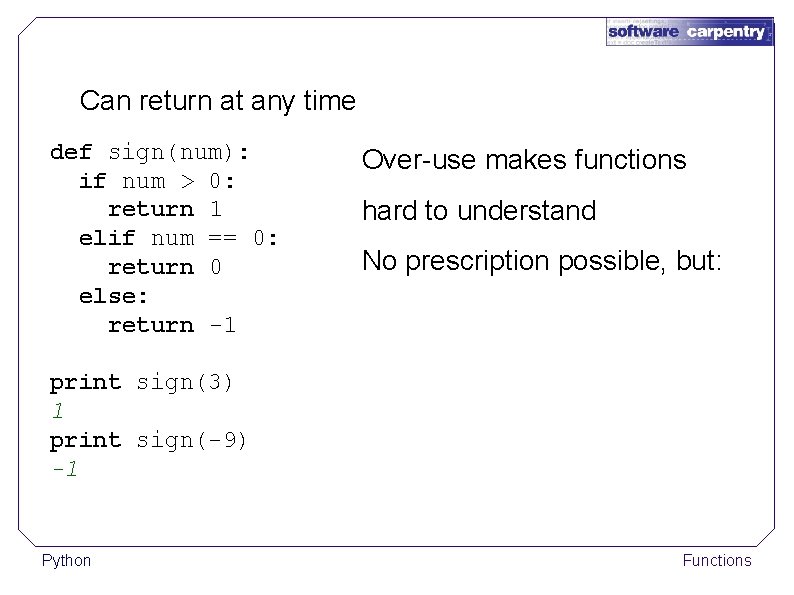
Can return at any time def sign(num): if num > 0: return 1 elif num == 0: return 0 else: return -1 Over-use makes functions hard to understand No prescription possible, but: print sign(3) 1 print sign(-9) -1 Python Functions
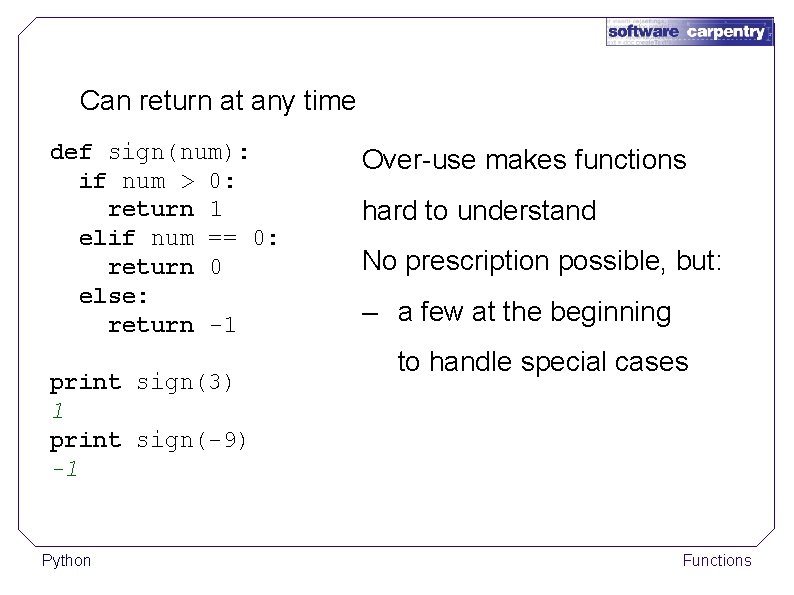
Can return at any time def sign(num): if num > 0: return 1 elif num == 0: return 0 else: return -1 print sign(3) 1 print sign(-9) -1 Python Over-use makes functions hard to understand No prescription possible, but: – a few at the beginning to handle special cases Functions
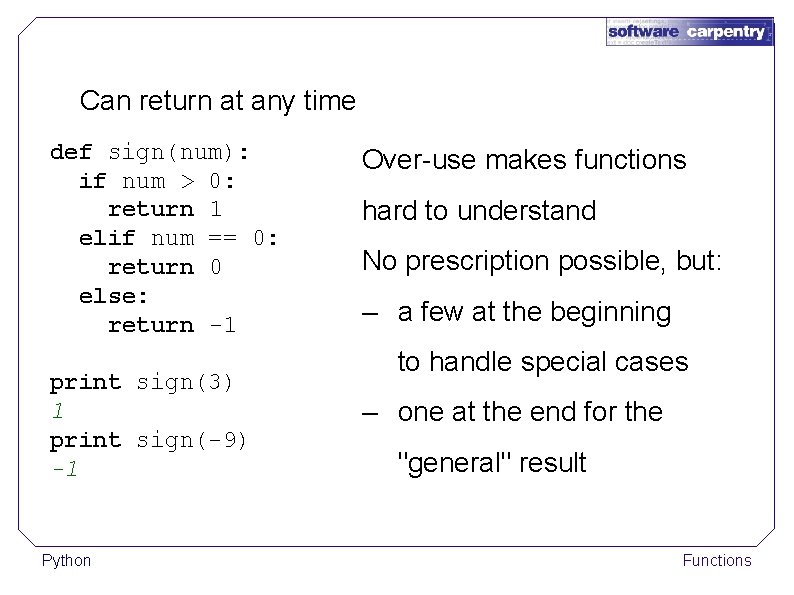
Can return at any time def sign(num): if num > 0: return 1 elif num == 0: return 0 else: return -1 print sign(3) 1 print sign(-9) -1 Python Over-use makes functions hard to understand No prescription possible, but: – a few at the beginning to handle special cases – one at the end for the "general" result Functions
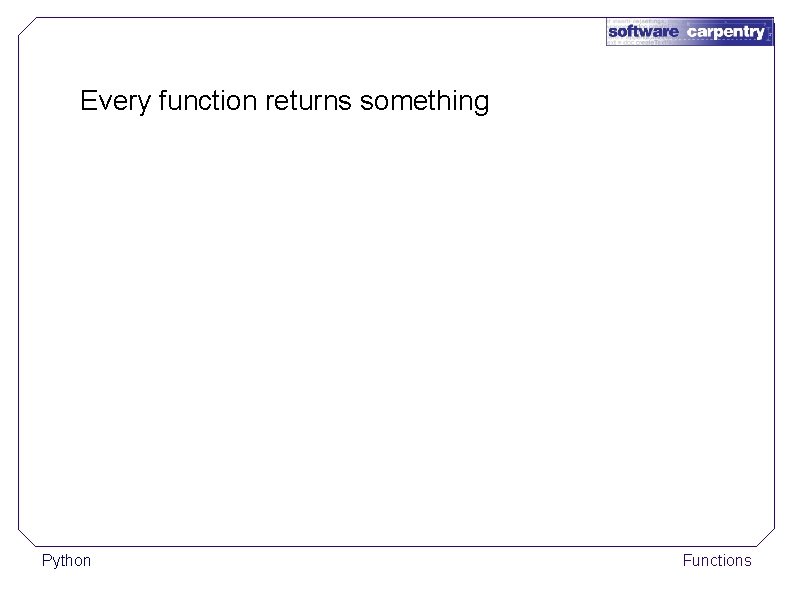
Every function returns something Python Functions
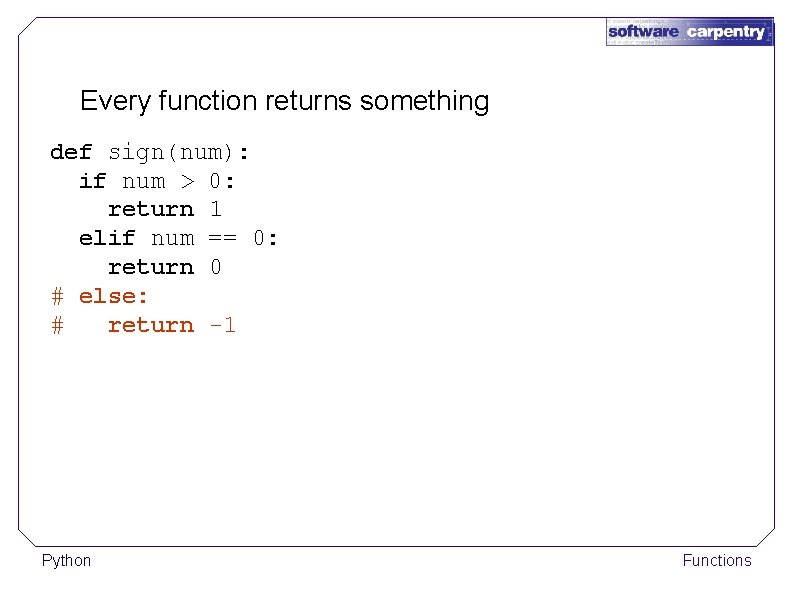
Every function returns something def sign(num): if num > 0: return 1 elif num == 0: return 0 # else: # return -1 Python Functions
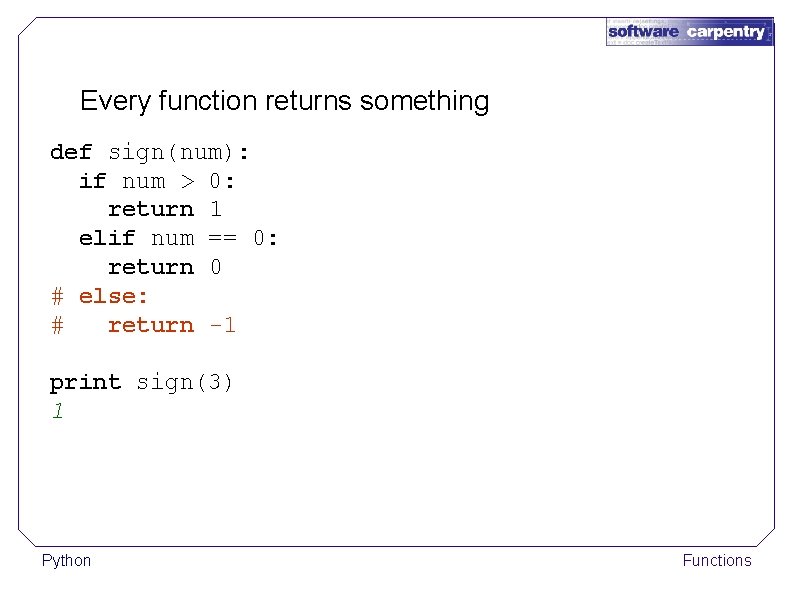
Every function returns something def sign(num): if num > 0: return 1 elif num == 0: return 0 # else: # return -1 print sign(3) 1 Python Functions
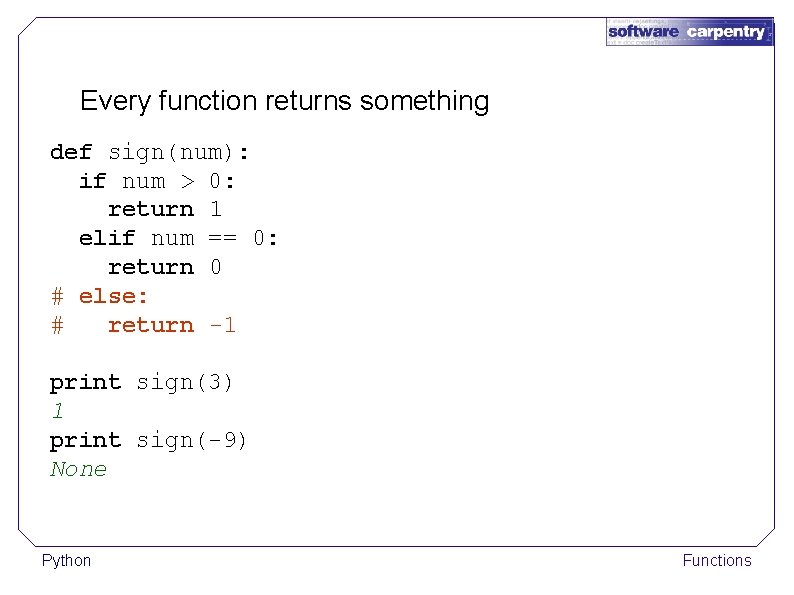
Every function returns something def sign(num): if num > 0: return 1 elif num == 0: return 0 # else: # return -1 print sign(3) 1 print sign(-9) None Python Functions
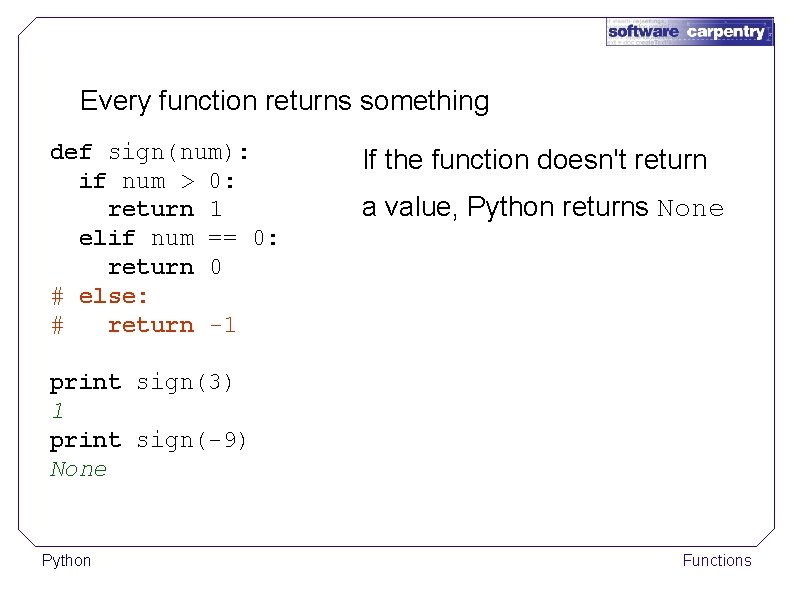
Every function returns something def sign(num): if num > 0: return 1 elif num == 0: return 0 # else: # return -1 If the function doesn't return a value, Python returns None print sign(3) 1 print sign(-9) None Python Functions
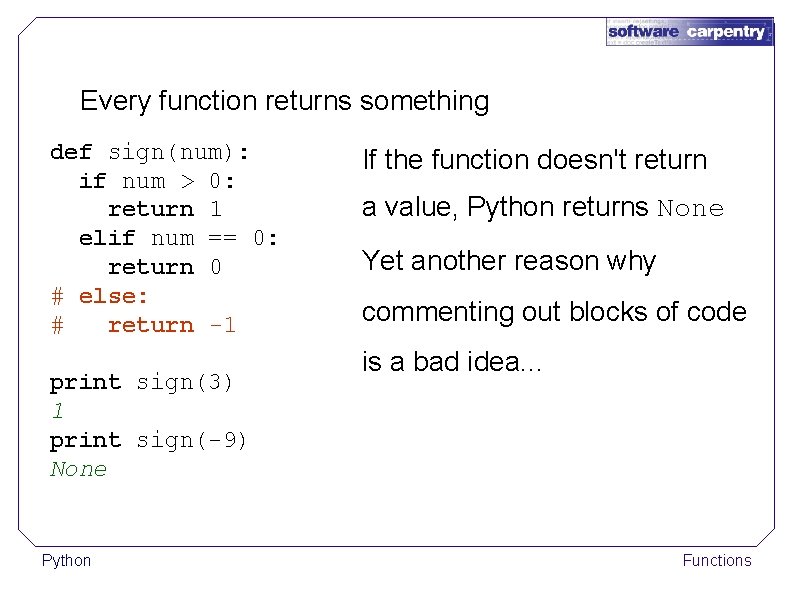
Every function returns something def sign(num): if num > 0: return 1 elif num == 0: return 0 # else: # return -1 print sign(3) 1 print sign(-9) None Python If the function doesn't return a value, Python returns None Yet another reason why commenting out blocks of code is a bad idea. . . Functions
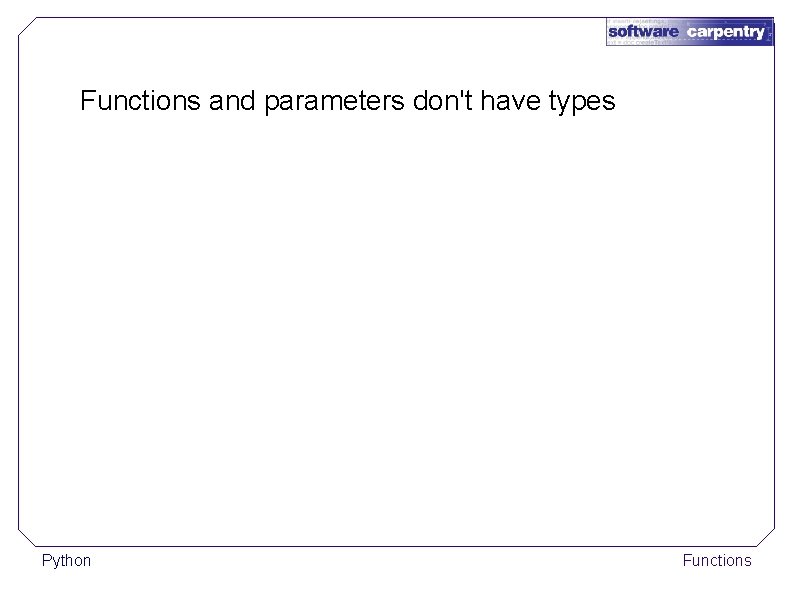
Functions and parameters don't have types Python Functions
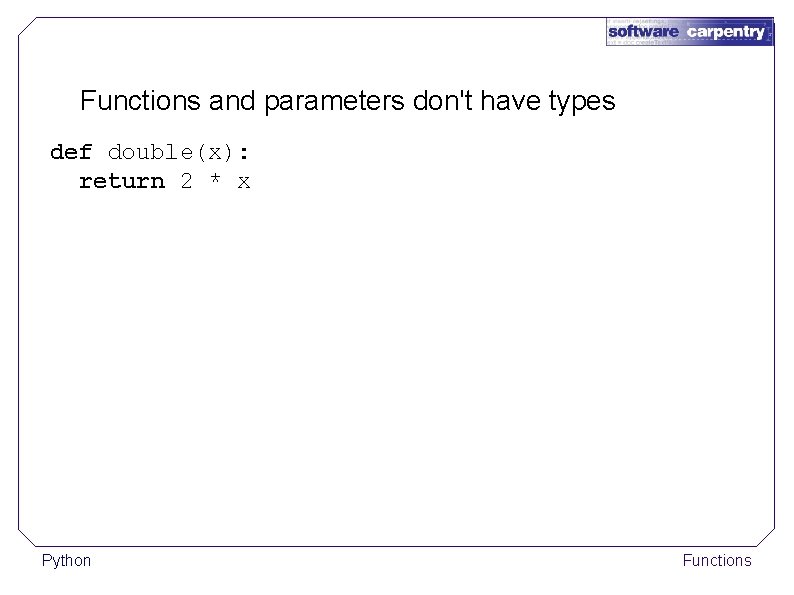
Functions and parameters don't have types def double(x): return 2 * x Python Functions
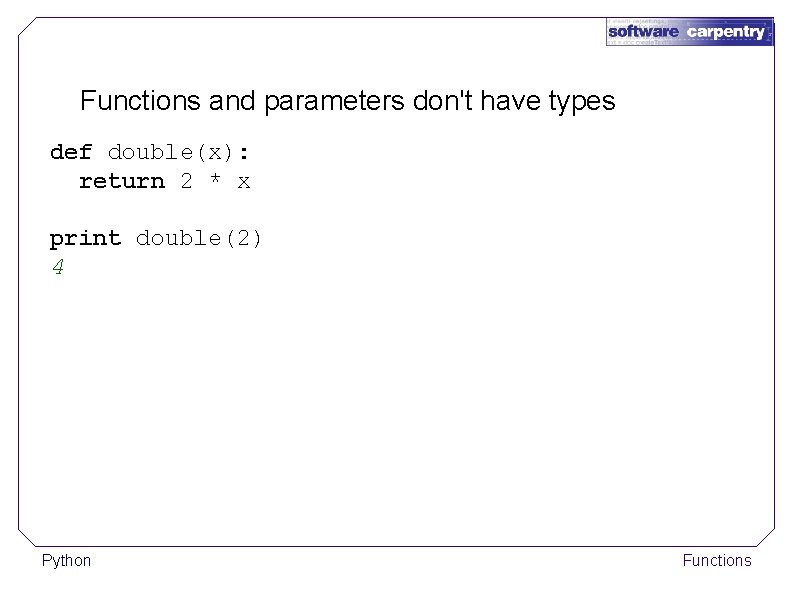
Functions and parameters don't have types def double(x): return 2 * x print double(2) 4 Python Functions
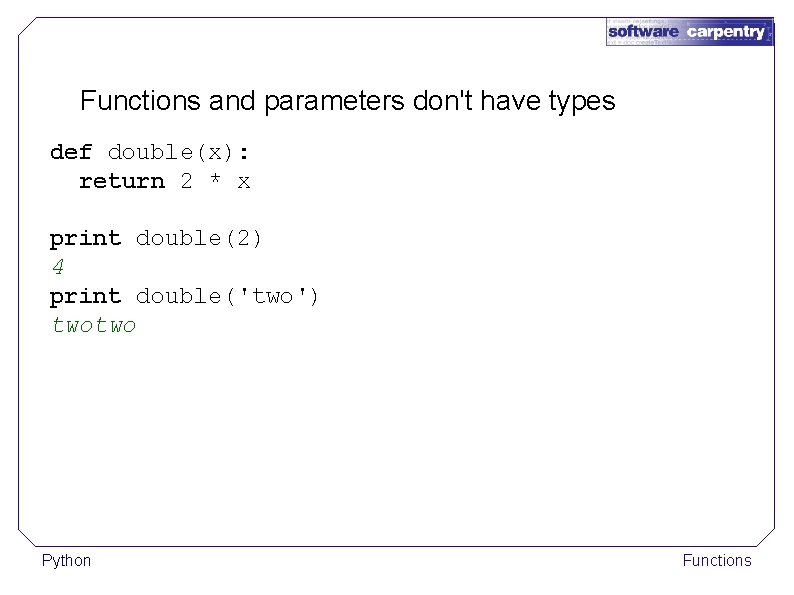
Functions and parameters don't have types def double(x): return 2 * x print double(2) 4 print double('two') twotwo Python Functions
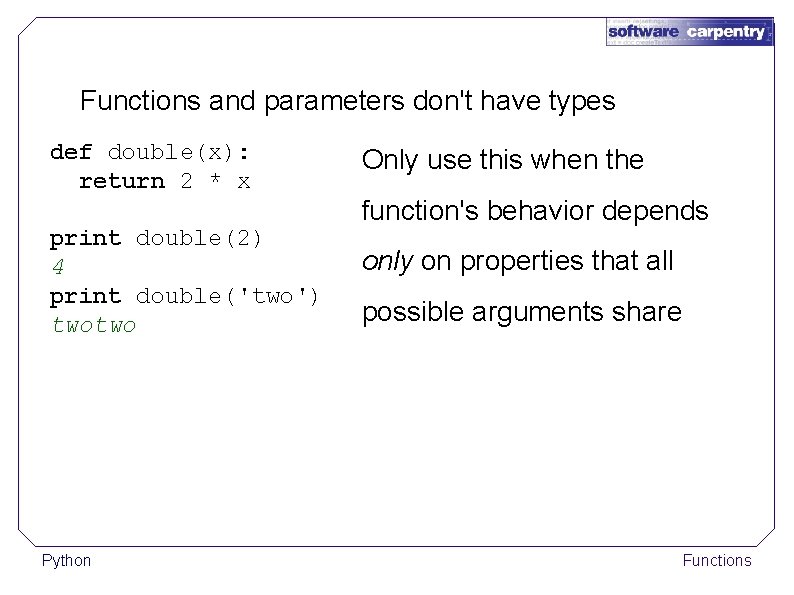
Functions and parameters don't have types def double(x): return 2 * x print double(2) 4 print double('two') twotwo Python Only use this when the function's behavior depends only on properties that all possible arguments share Functions
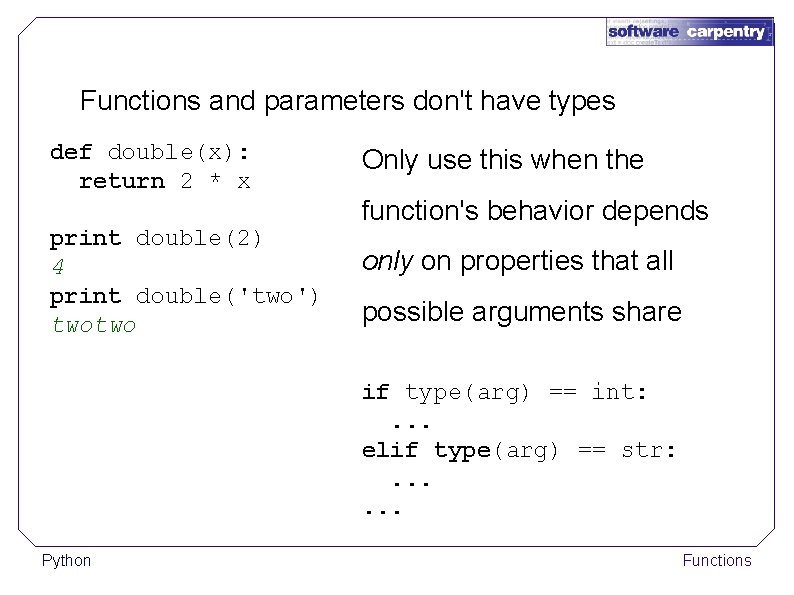
Functions and parameters don't have types def double(x): return 2 * x print double(2) 4 print double('two') twotwo Only use this when the function's behavior depends only on properties that all possible arguments share if type(arg) == int: . . . elif type(arg) == str: . . . Python Functions
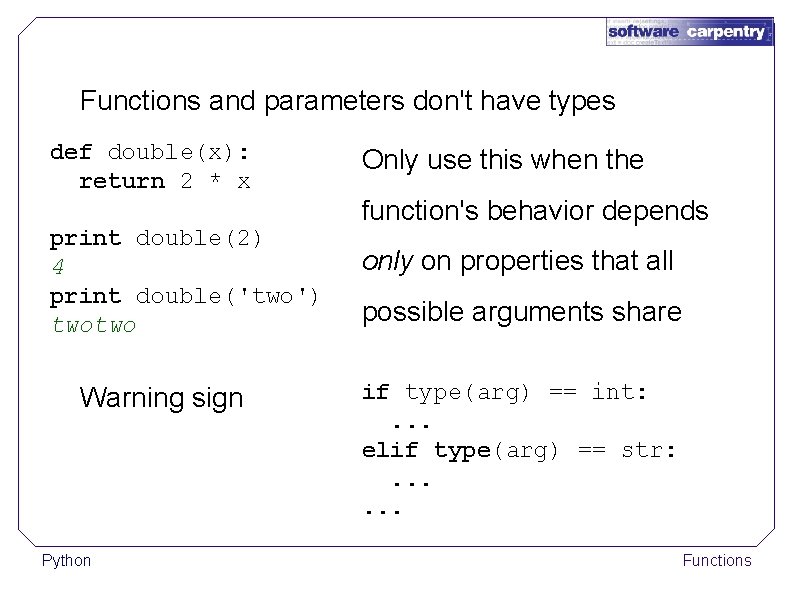
Functions and parameters don't have types def double(x): return 2 * x print double(2) 4 print double('two') twotwo Warning sign Python Only use this when the function's behavior depends only on properties that all possible arguments share if type(arg) == int: . . . elif type(arg) == str: . . . Functions
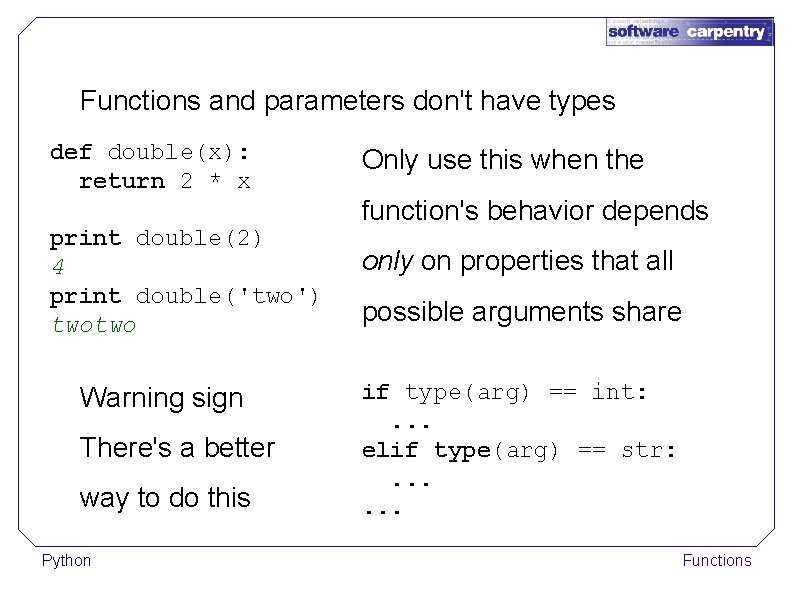
Functions and parameters don't have types def double(x): return 2 * x print double(2) 4 print double('two') twotwo Warning sign There's a better way to do this Python Only use this when the function's behavior depends only on properties that all possible arguments share if type(arg) == int: . . . elif type(arg) == str: . . . Functions
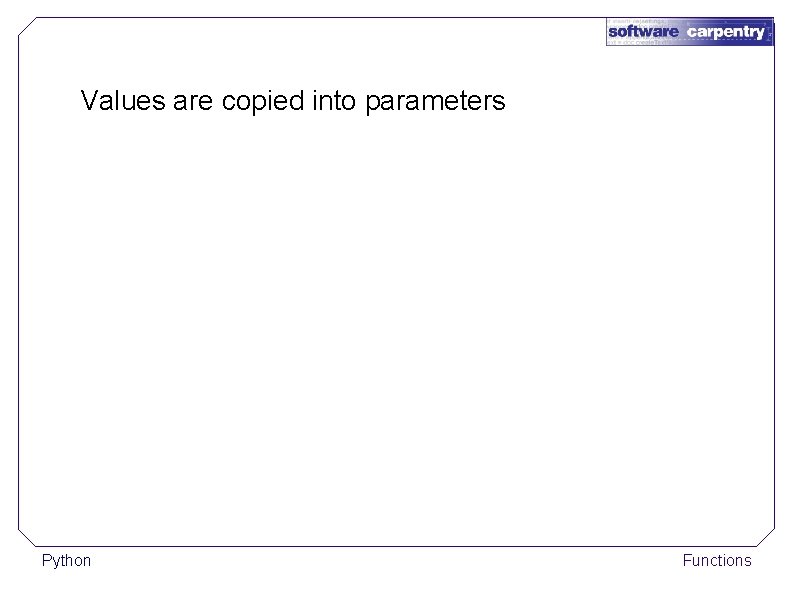
Values are copied into parameters Python Functions
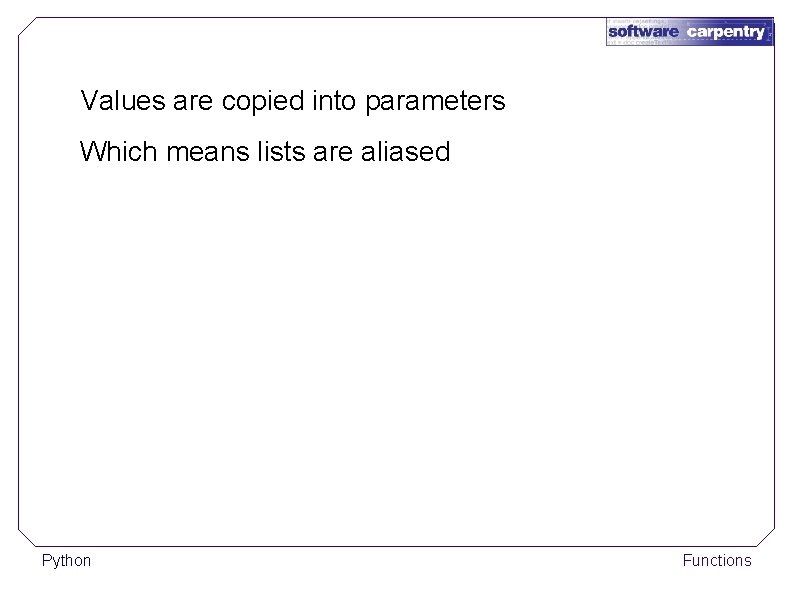
Values are copied into parameters Which means lists are aliased Python Functions
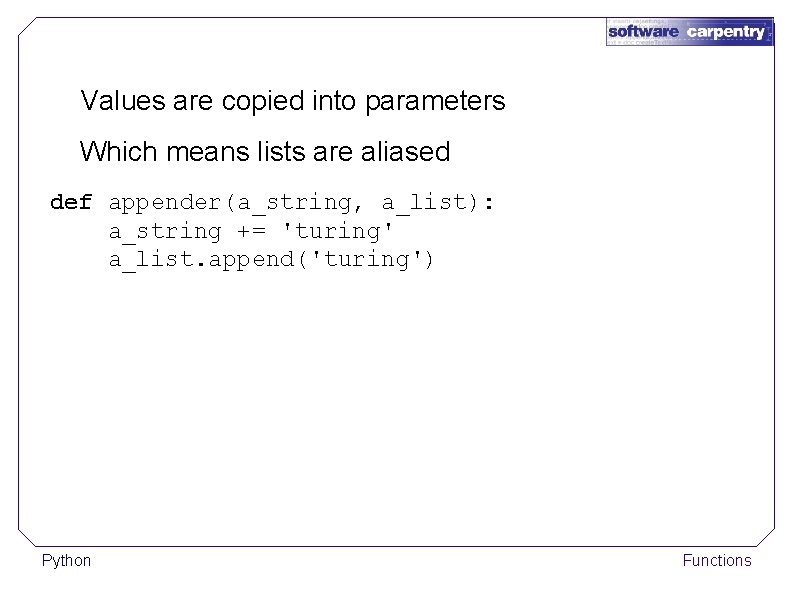
Values are copied into parameters Which means lists are aliased def appender(a_string, a_list): a_string += 'turing' a_list. append('turing') Python Functions
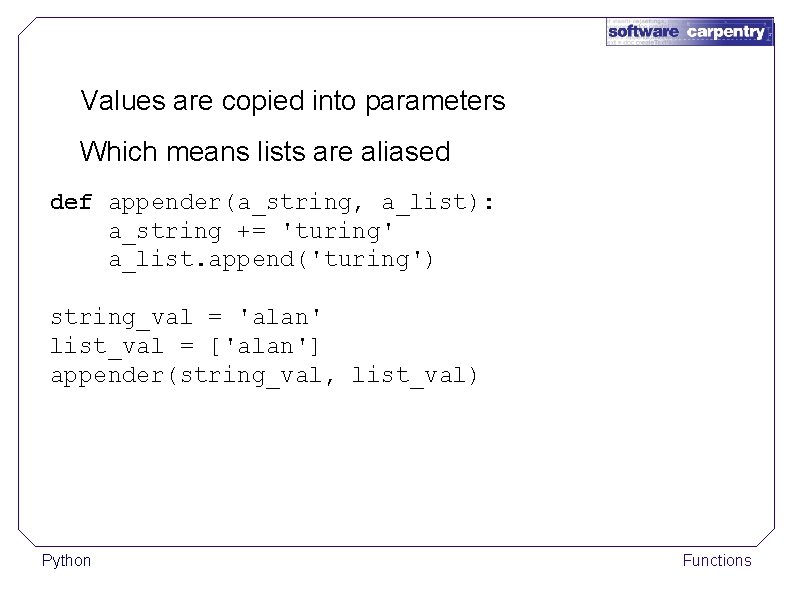
Values are copied into parameters Which means lists are aliased def appender(a_string, a_list): a_string += 'turing' a_list. append('turing') string_val = 'alan' list_val = ['alan'] appender(string_val, list_val) Python Functions
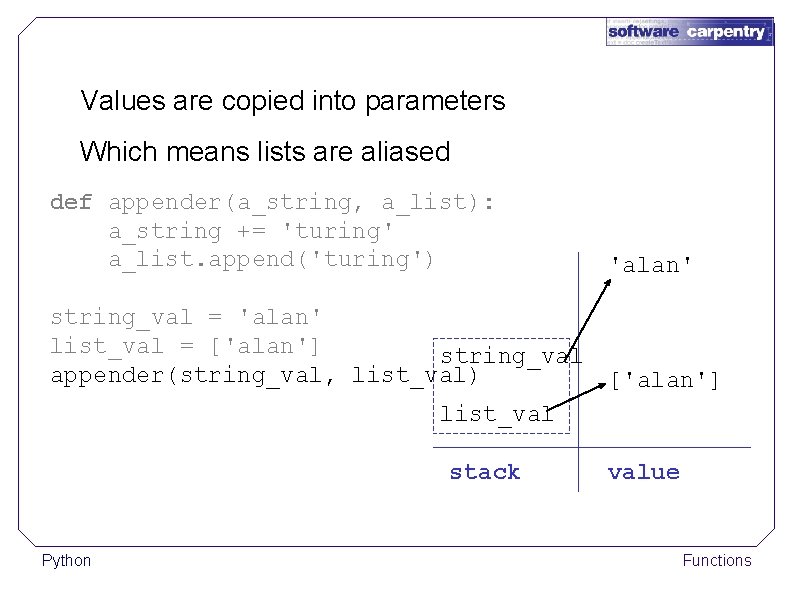
Values are copied into parameters Which means lists are aliased def appender(a_string, a_list): a_string += 'turing' a_list. append('turing') 'alan' string_val = 'alan' list_val = ['alan'] string_val appender(string_val, list_val) ['alan'] list_val stack Python value Functions
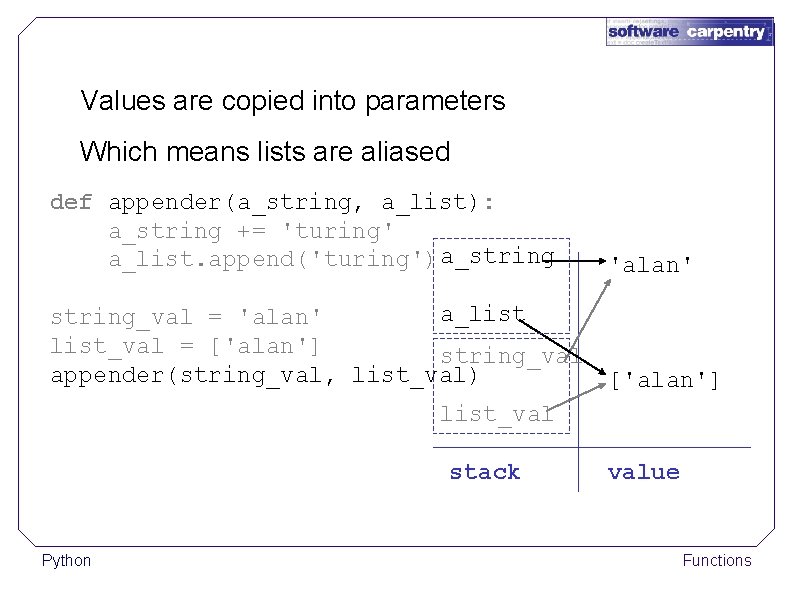
Values are copied into parameters Which means lists are aliased def appender(a_string, a_list): a_string += 'turing' a_list. append('turing') a_string 'alan' a_list string_val = 'alan' list_val = ['alan'] string_val appender(string_val, list_val) ['alan'] list_val stack Python value Functions
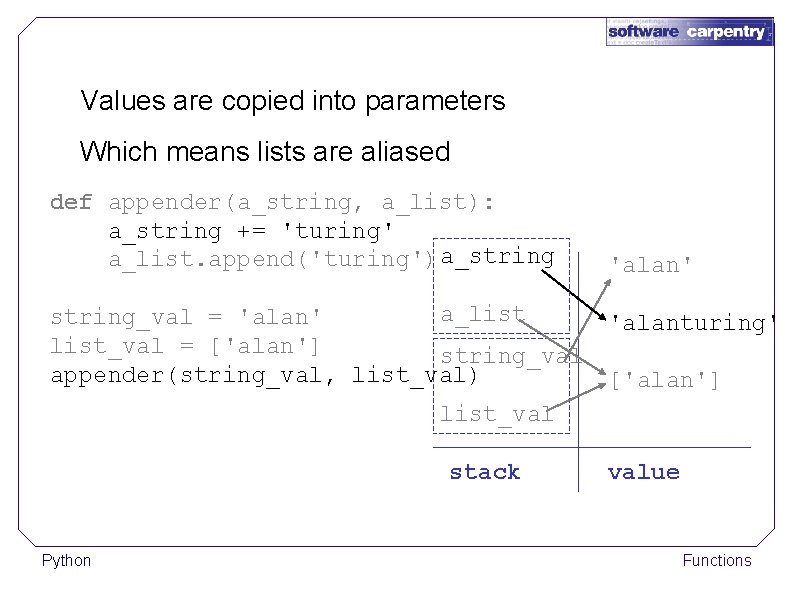
Values are copied into parameters Which means lists are aliased def appender(a_string, a_list): a_string += 'turing' a_list. append('turing') a_string 'alan' a_list string_val = 'alan' 'alanturing' list_val = ['alan'] string_val appender(string_val, list_val) ['alan'] list_val stack Python value Functions
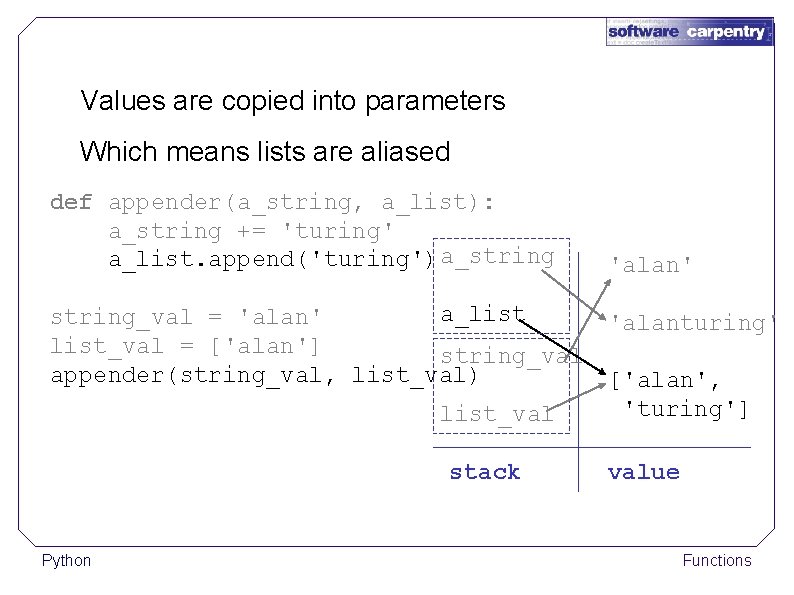
Values are copied into parameters Which means lists are aliased def appender(a_string, a_list): a_string += 'turing' a_list. append('turing') a_string 'alan' a_list string_val = 'alan' 'alanturing' list_val = ['alan'] string_val appender(string_val, list_val) ['alan', 'turing'] list_val stack Python value Functions
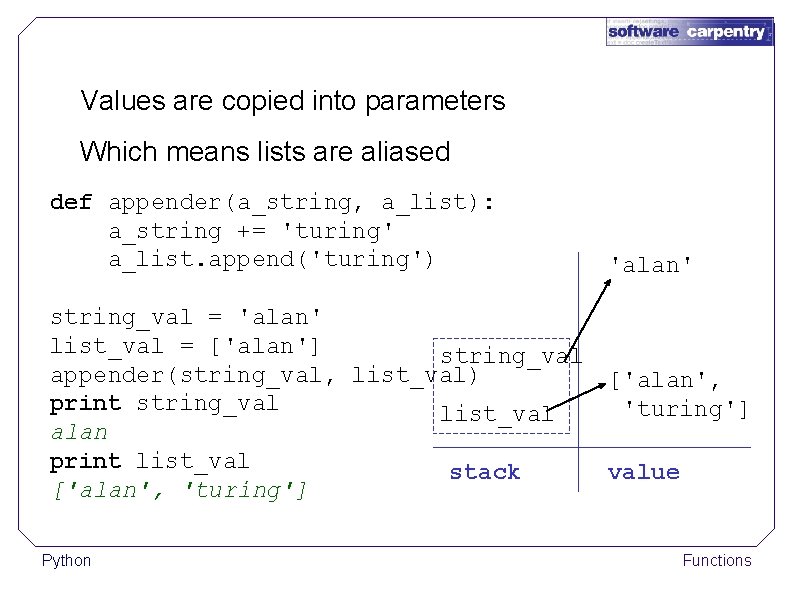
Values are copied into parameters Which means lists are aliased def appender(a_string, a_list): a_string += 'turing' a_list. append('turing') 'alan' string_val = 'alan' list_val = ['alan'] string_val appender(string_val, list_val) ['alan', print string_val 'turing'] list_val alan print list_val stack value ['alan', 'turing'] Python Functions
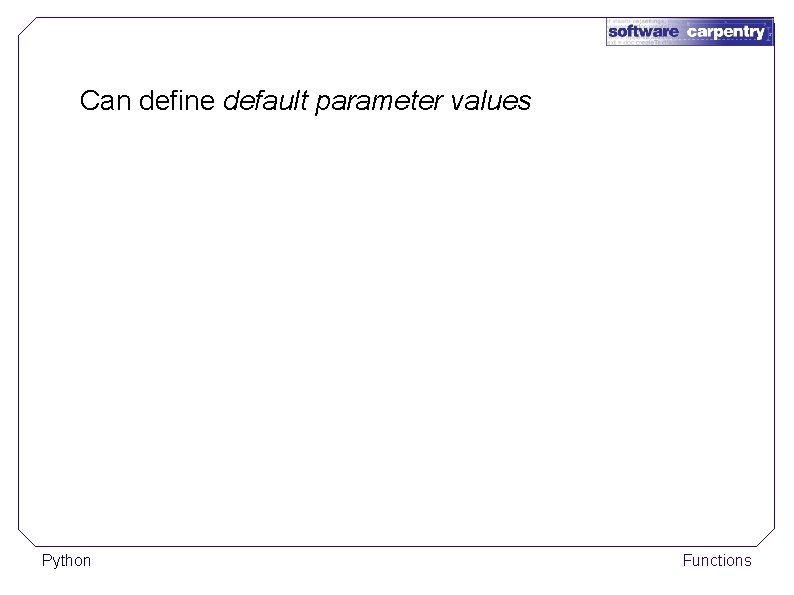
Can define default parameter values Python Functions
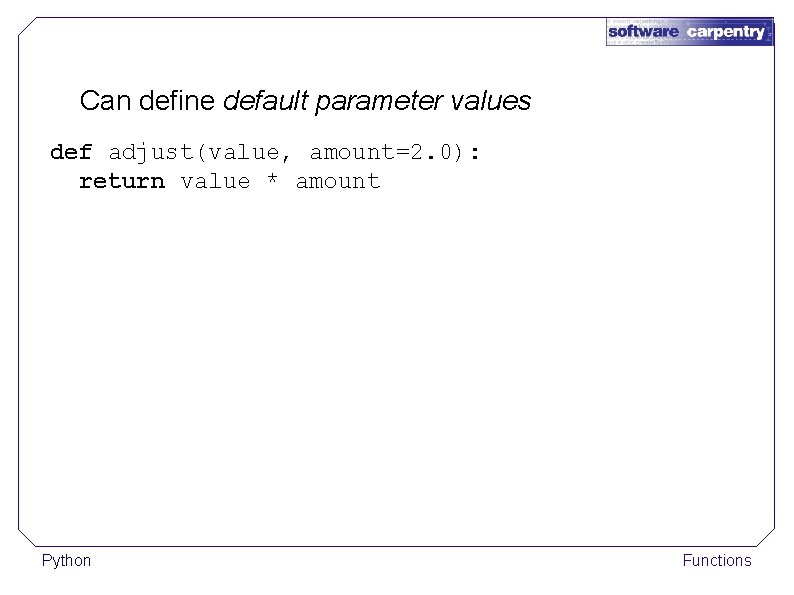
Can define default parameter values def adjust(value, amount=2. 0): return value * amount Python Functions
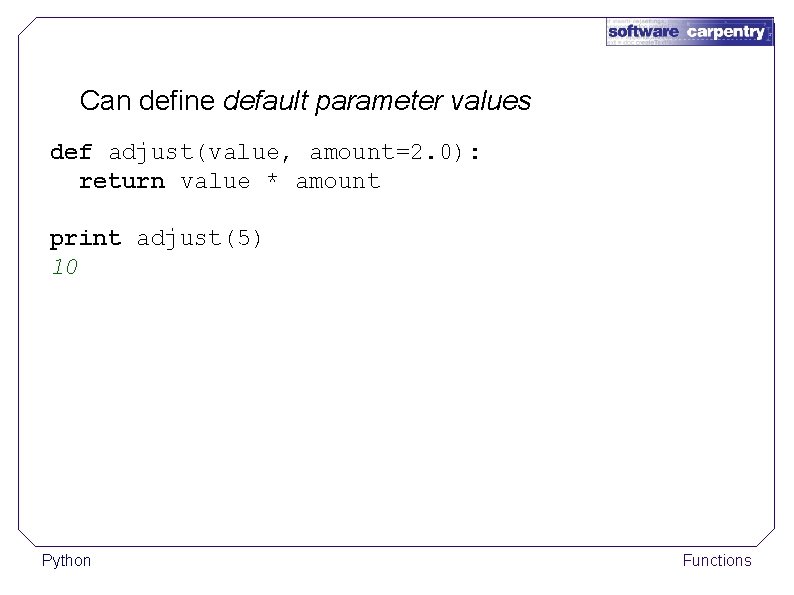
Can define default parameter values def adjust(value, amount=2. 0): return value * amount print adjust(5) 10 Python Functions
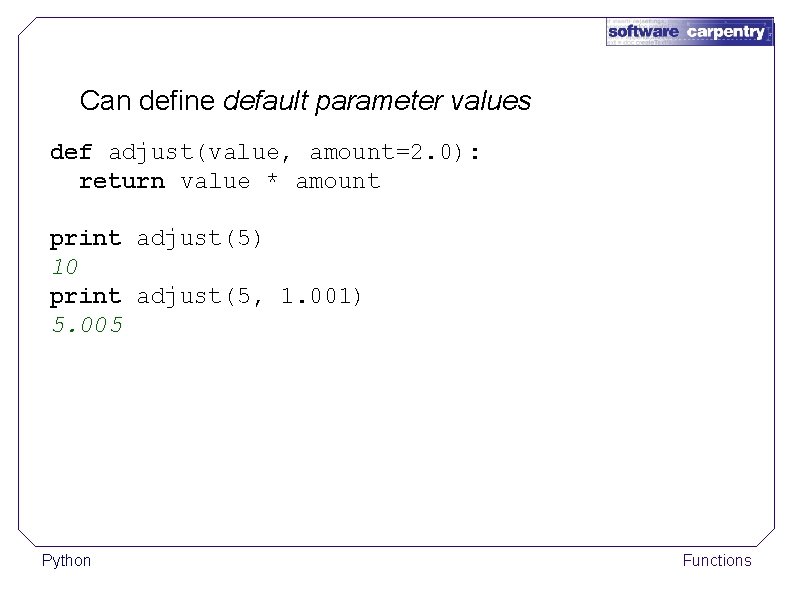
Can define default parameter values def adjust(value, amount=2. 0): return value * amount print adjust(5) 10 print adjust(5, 1. 001) 5. 005 Python Functions
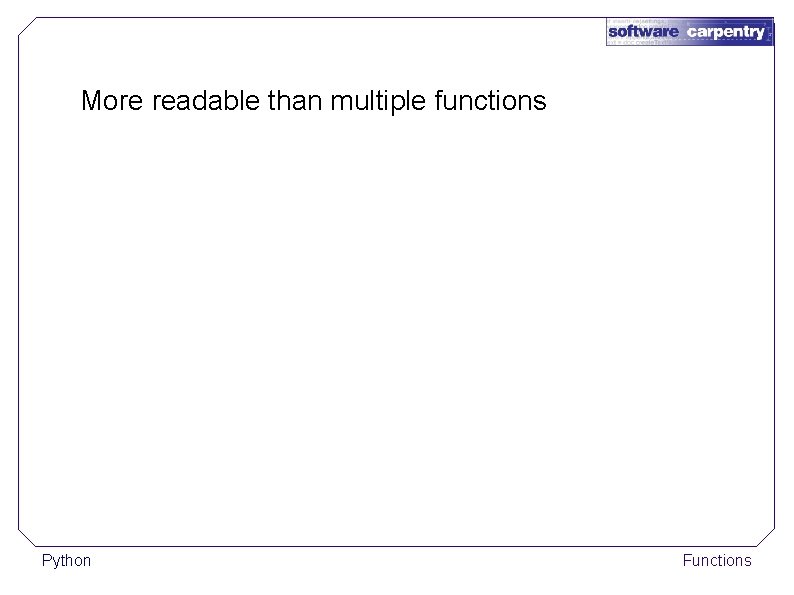
More readable than multiple functions Python Functions
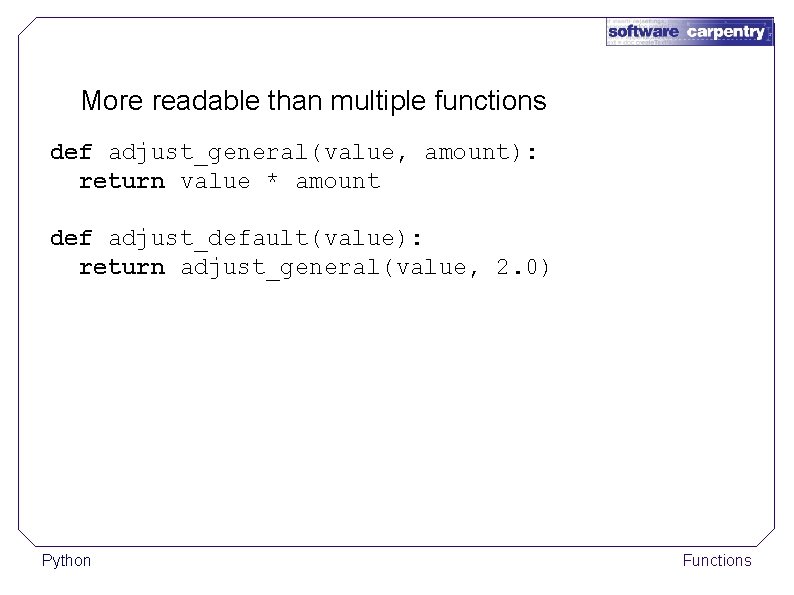
More readable than multiple functions def adjust_general(value, amount): return value * amount def adjust_default(value): return adjust_general(value, 2. 0) Python Functions
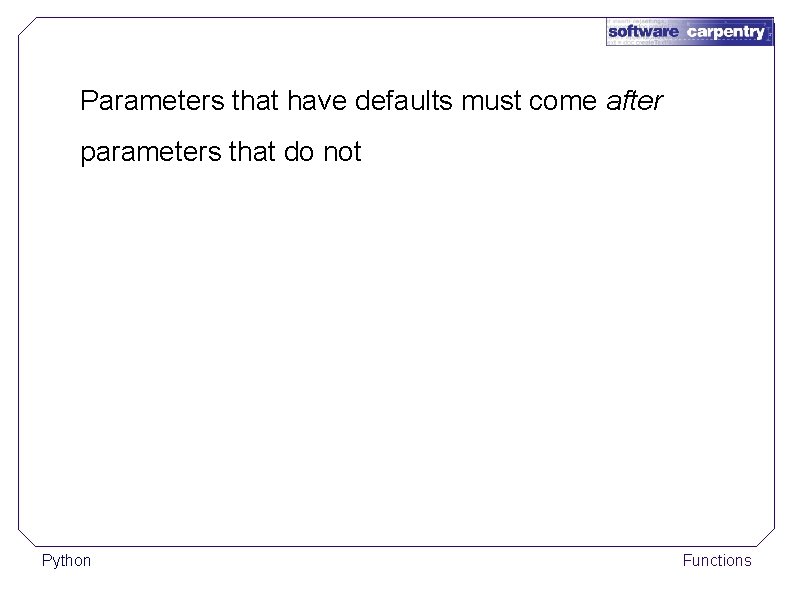
Parameters that have defaults must come after parameters that do not Python Functions
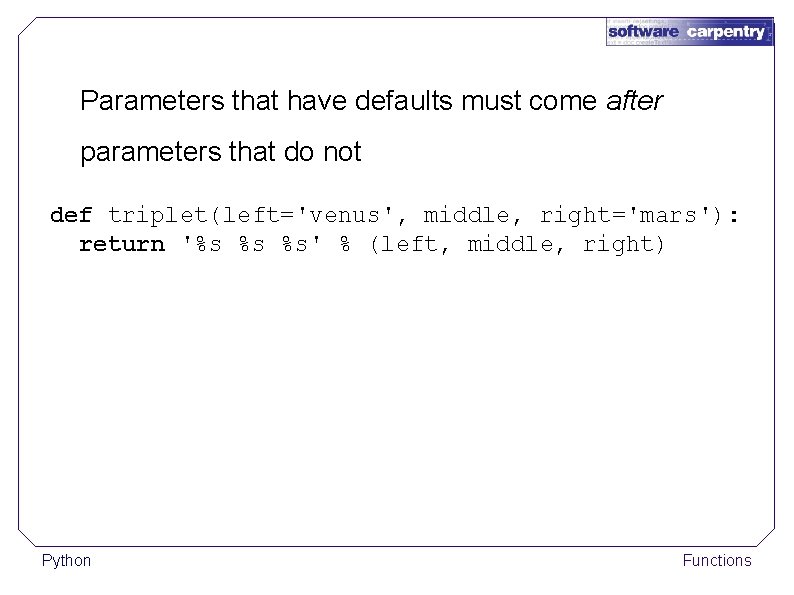
Parameters that have defaults must come after parameters that do not def triplet(left='venus', middle, right='mars'): return '%s %s %s' % (left, middle, right) Python Functions
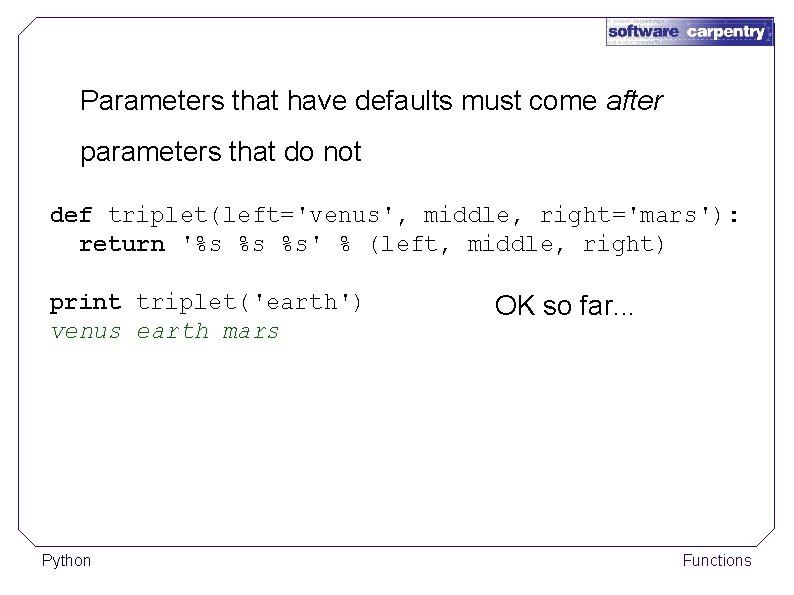
Parameters that have defaults must come after parameters that do not def triplet(left='venus', middle, right='mars'): return '%s %s %s' % (left, middle, right) print triplet('earth') venus earth mars Python OK so far. . . Functions
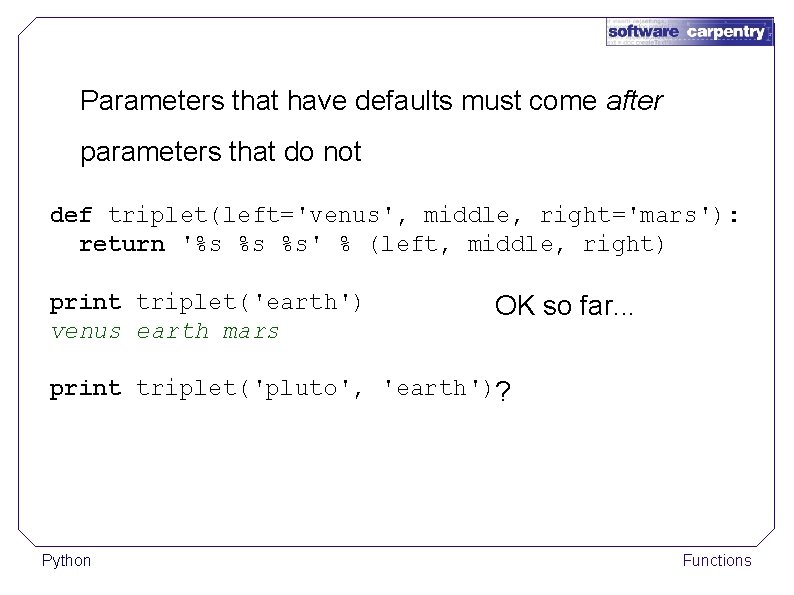
Parameters that have defaults must come after parameters that do not def triplet(left='venus', middle, right='mars'): return '%s %s %s' % (left, middle, right) print triplet('earth') venus earth mars OK so far. . . print triplet('pluto', 'earth')? Python Functions
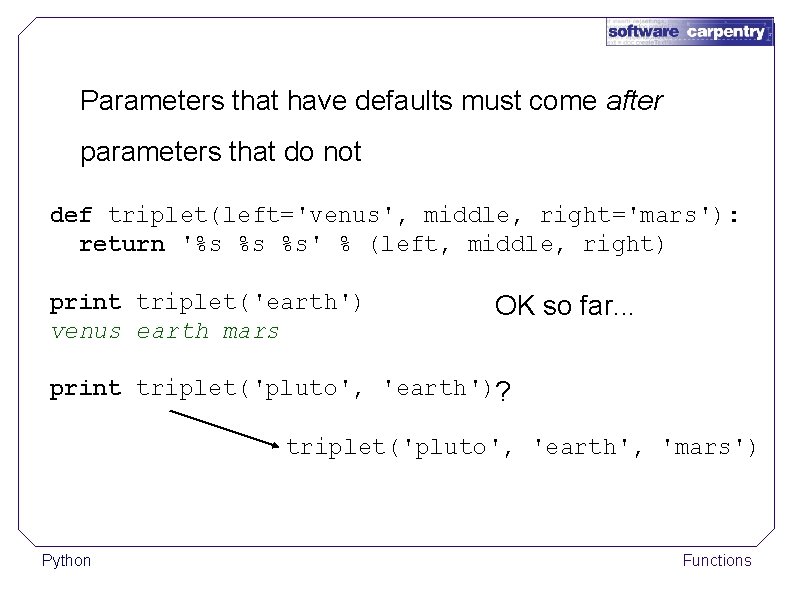
Parameters that have defaults must come after parameters that do not def triplet(left='venus', middle, right='mars'): return '%s %s %s' % (left, middle, right) print triplet('earth') venus earth mars OK so far. . . print triplet('pluto', 'earth')? triplet('pluto', 'earth', 'mars') Python Functions
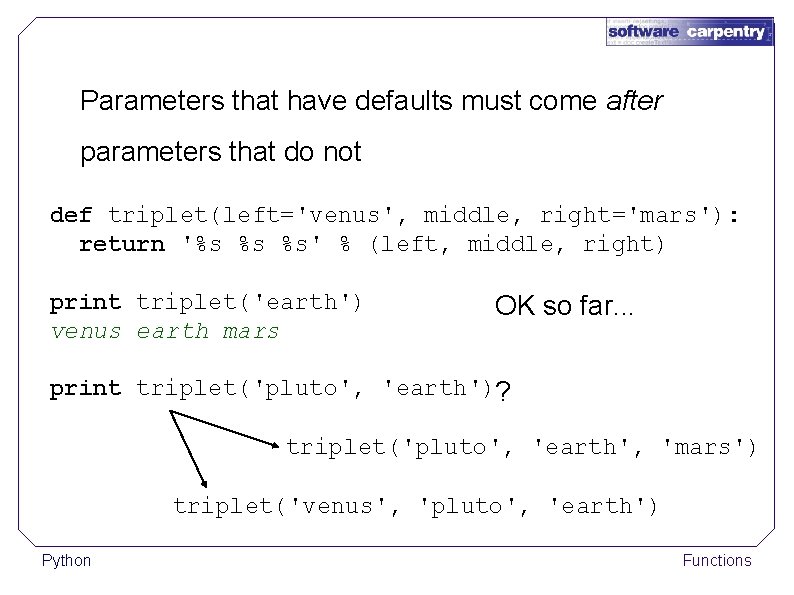
Parameters that have defaults must come after parameters that do not def triplet(left='venus', middle, right='mars'): return '%s %s %s' % (left, middle, right) print triplet('earth') venus earth mars OK so far. . . print triplet('pluto', 'earth')? triplet('pluto', 'earth', 'mars') triplet('venus', 'pluto', 'earth') Python Functions
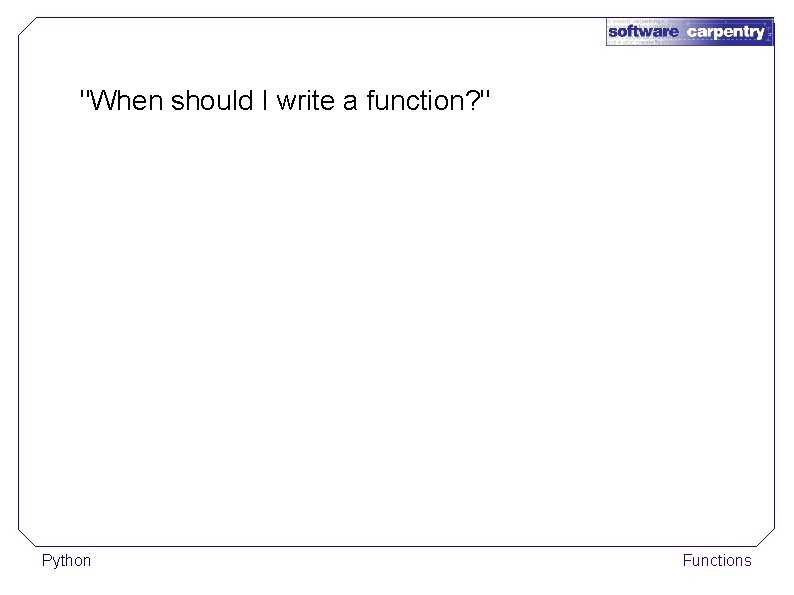
"When should I write a function? " Python Functions
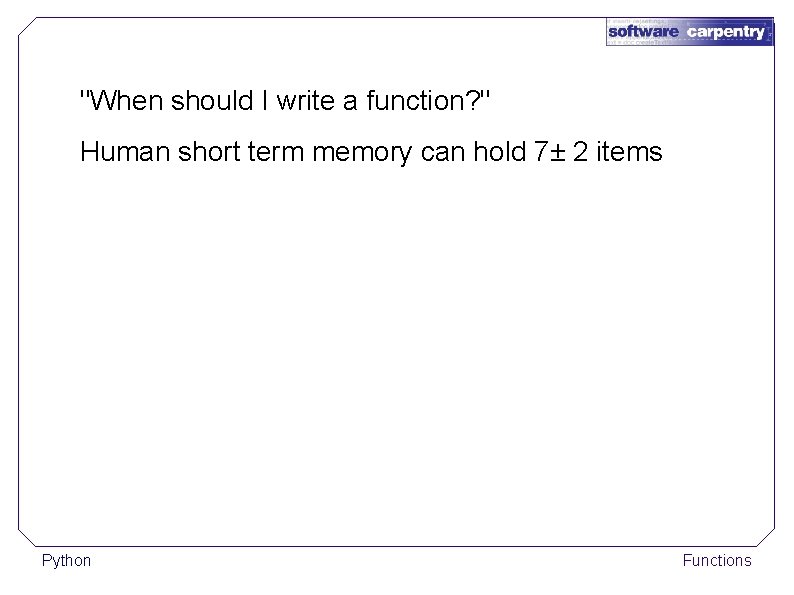
"When should I write a function? " Human short term memory can hold 7± 2 items Python Functions
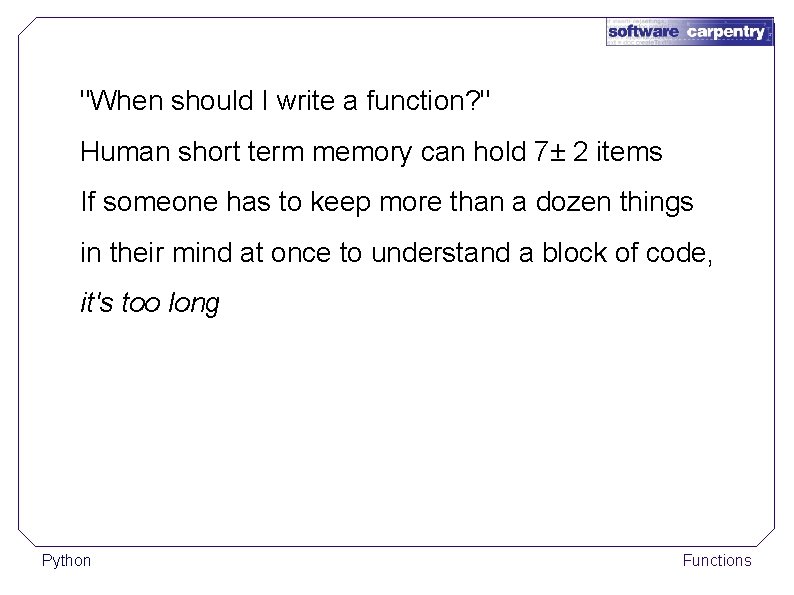
"When should I write a function? " Human short term memory can hold 7± 2 items If someone has to keep more than a dozen things in their mind at once to understand a block of code, it's too long Python Functions
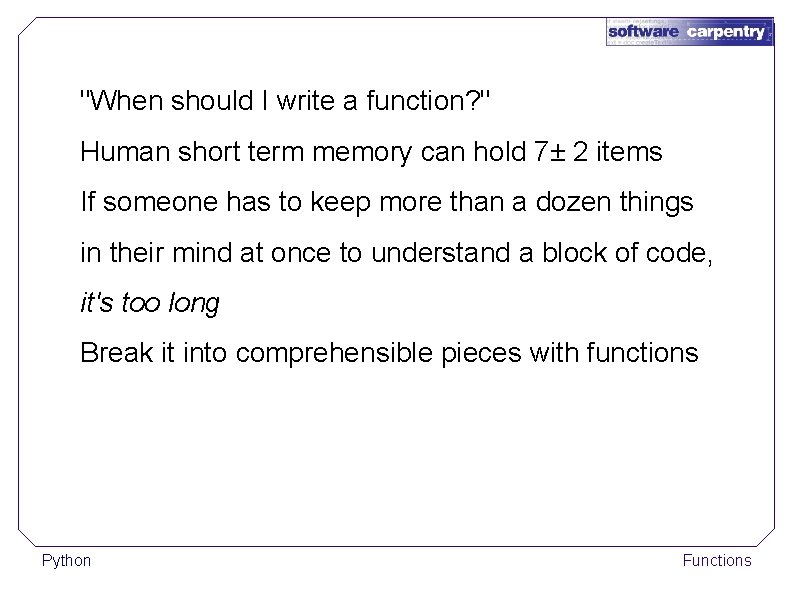
"When should I write a function? " Human short term memory can hold 7± 2 items If someone has to keep more than a dozen things in their mind at once to understand a block of code, it's too long Break it into comprehensible pieces with functions Python Functions
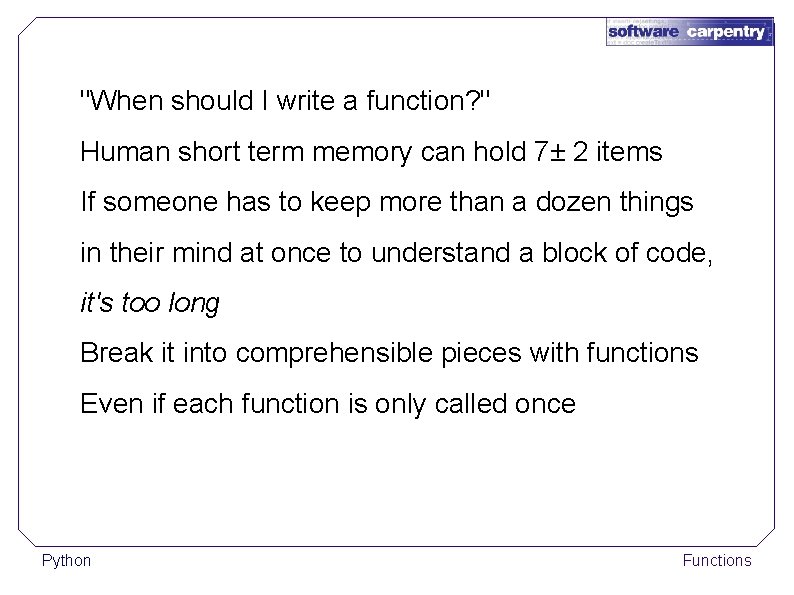
"When should I write a function? " Human short term memory can hold 7± 2 items If someone has to keep more than a dozen things in their mind at once to understand a block of code, it's too long Break it into comprehensible pieces with functions Even if each function is only called once Python Functions
![Example for x in range1 GRIDWIDTH1 for y in range1 GRIDHEIGHT1 if densityx1y Example for x in range(1, GRID_WIDTH-1): for y in range(1, GRID_HEIGHT-1): if (density[x-1][y] >](https://slidetodoc.com/presentation_image/448cd4ea5e49150d34b736a7ce64fbec/image-80.jpg)
Example for x in range(1, GRID_WIDTH-1): for y in range(1, GRID_HEIGHT-1): if (density[x-1][y] > density_threshold) or (density[x+1][y] > density_threshold): if (flow[x][y-1] < flow_threshold) or (flow[x][y+1] < flow_threshold): temp = (density[x-1][y] + density[x+1][y]) / if abs(temp - density[x][y]) > update_thresh density[x][y] = temp Python Functions
![Refactoring 1 grid interior for x in gridinteriorGRIDWIDTH for y in gridinteriorGRIDHEIGHT if densityx1y Refactoring #1: grid interior for x in grid_interior(GRID_WIDTH): for y in grid_interior(GRID_HEIGHT): if (density[x-1][y]](https://slidetodoc.com/presentation_image/448cd4ea5e49150d34b736a7ce64fbec/image-81.jpg)
Refactoring #1: grid interior for x in grid_interior(GRID_WIDTH): for y in grid_interior(GRID_HEIGHT): if (density[x-1][y] > density_threshold) or (density[x+1][y] > density_threshold): if (flow[x][y-1] < flow_threshold) or (flow[x][y+1] < flow_threshold): temp = (density[x-1][y] + density[x+1][y]) / if abs(temp - density[x][y]) > update_thresh density[x][y] = temp Python Functions
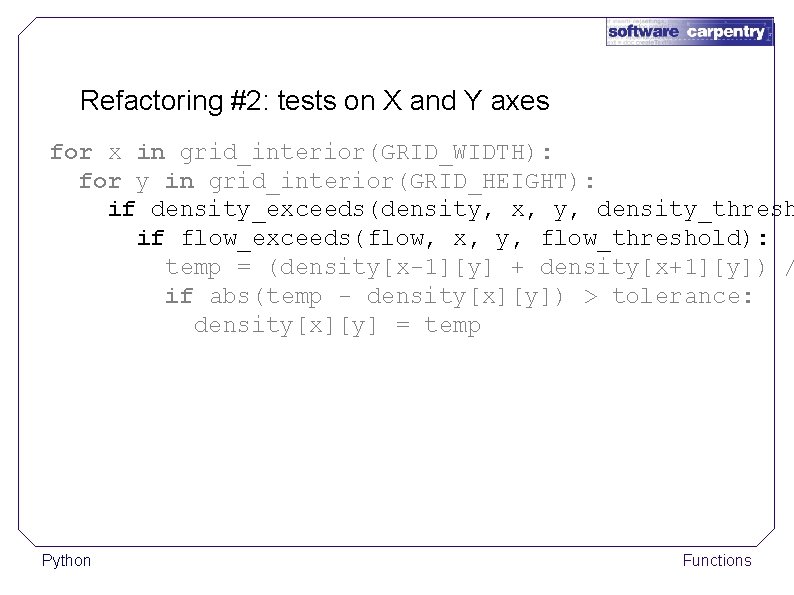
Refactoring #2: tests on X and Y axes for x in grid_interior(GRID_WIDTH): for y in grid_interior(GRID_HEIGHT): if density_exceeds(density, x, y, density_thresh if flow_exceeds(flow, x, y, flow_threshold): temp = (density[x-1][y] + density[x+1][y]) / if abs(temp - density[x][y]) > tolerance: density[x][y] = temp Python Functions
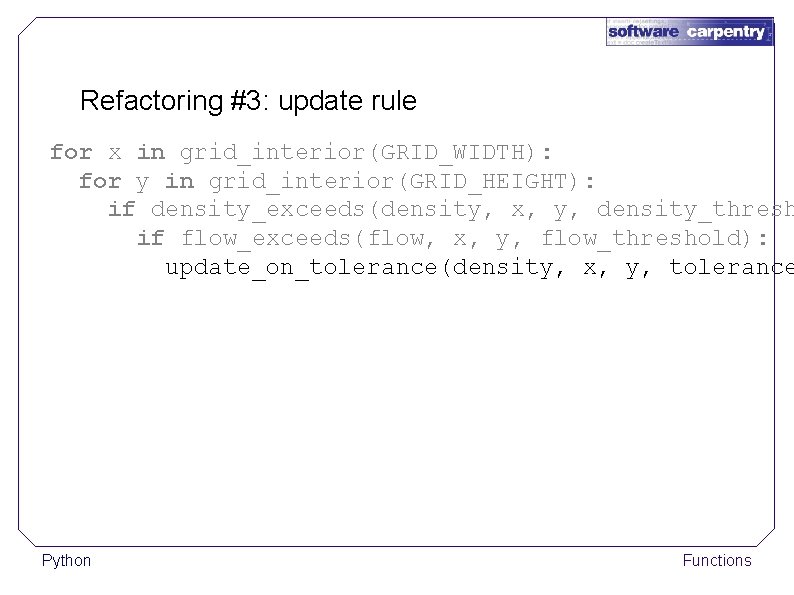
Refactoring #3: update rule for x in grid_interior(GRID_WIDTH): for y in grid_interior(GRID_HEIGHT): if density_exceeds(density, x, y, density_thresh if flow_exceeds(flow, x, y, flow_threshold): update_on_tolerance(density, x, y, tolerance Python Functions
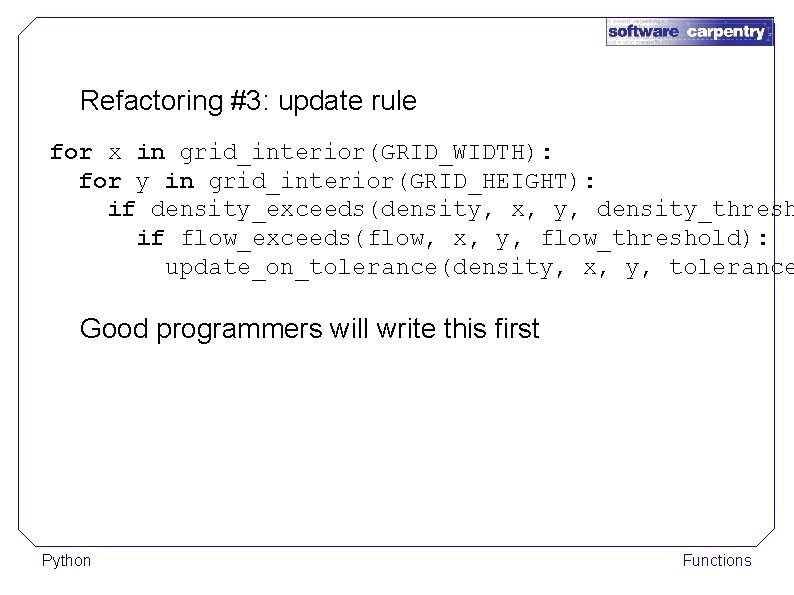
Refactoring #3: update rule for x in grid_interior(GRID_WIDTH): for y in grid_interior(GRID_HEIGHT): if density_exceeds(density, x, y, density_thresh if flow_exceeds(flow, x, y, flow_threshold): update_on_tolerance(density, x, y, tolerance Good programmers will write this first Python Functions
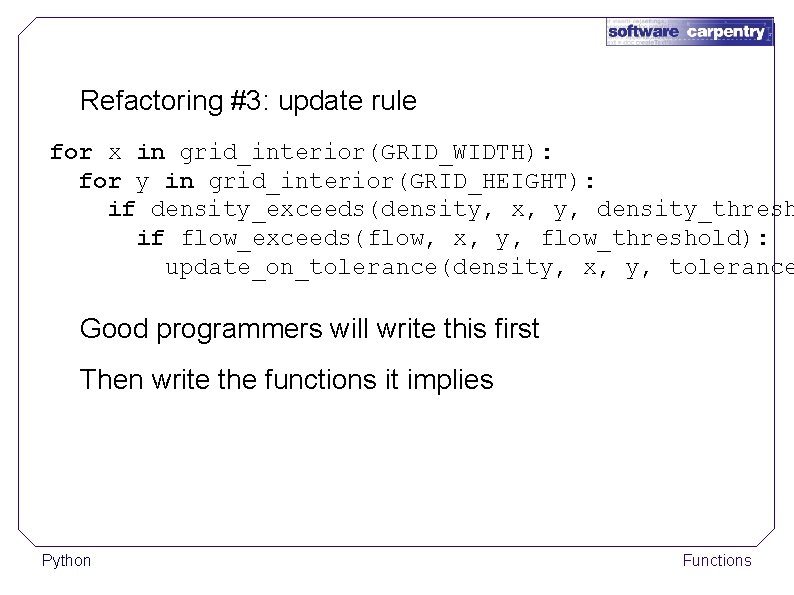
Refactoring #3: update rule for x in grid_interior(GRID_WIDTH): for y in grid_interior(GRID_HEIGHT): if density_exceeds(density, x, y, density_thresh if flow_exceeds(flow, x, y, flow_threshold): update_on_tolerance(density, x, y, tolerance Good programmers will write this first Then write the functions it implies Python Functions
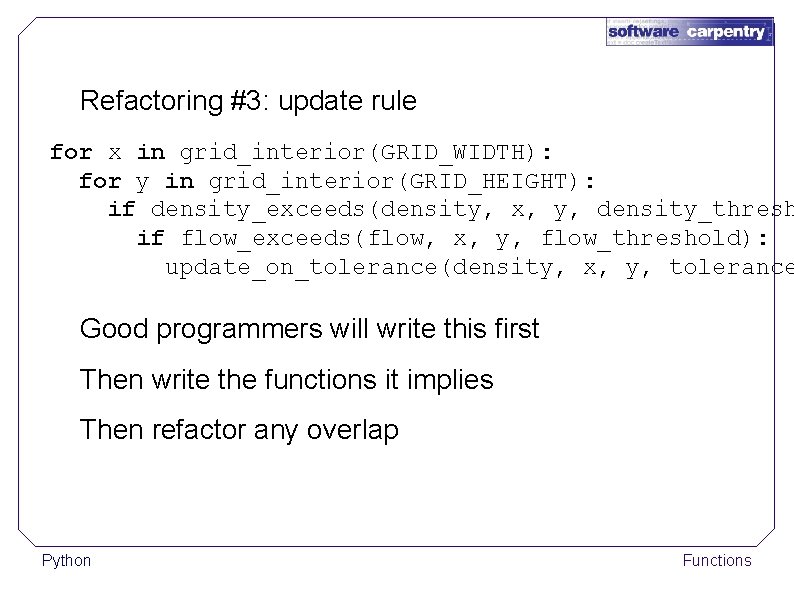
Refactoring #3: update rule for x in grid_interior(GRID_WIDTH): for y in grid_interior(GRID_HEIGHT): if density_exceeds(density, x, y, density_thresh if flow_exceeds(flow, x, y, flow_threshold): update_on_tolerance(density, x, y, tolerance Good programmers will write this first Then write the functions it implies Then refactor any overlap Python Functions
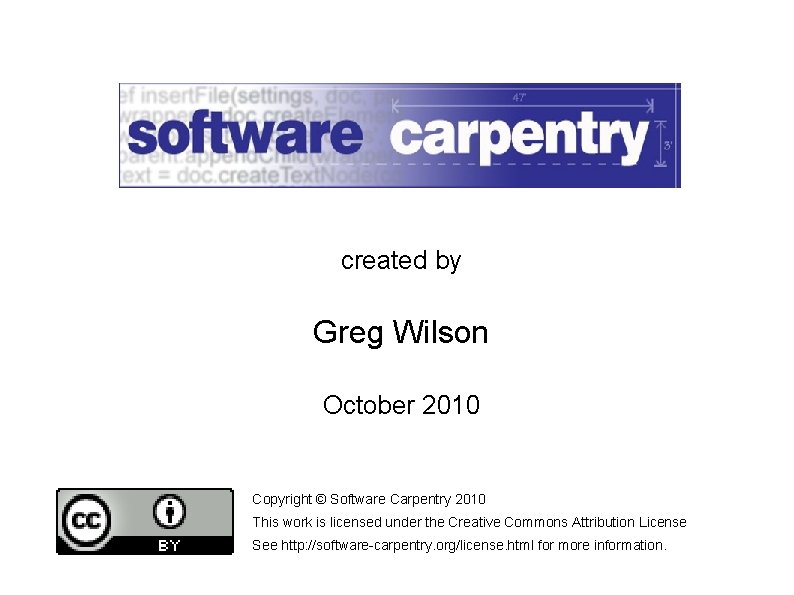
created by Greg Wilson October 2010 Copyright © Software Carpentry 2010 This work is licensed under the Creative Commons Attribution License See http: //software-carpentry. org/license. html for more information.
Copyright 2010 pearson education inc
C-929-a
Copyright 2010
Copyright 2010 pearson education inc
Copyright 2010 pearson education inc
Copyright 2010
Nwoz
Copyright 2010 pearson education inc
Copyright 2010 pearson education inc
Copyright 2010 pearson education inc
Copyright 2010 pearson education inc
Copyright 2010 pearson education inc
Copyright 2010 pearson education inc
Pearson education inc all rights reserved
Method vs function python
Methods python list
Pure functions and modifiers in python
Pure functions and modifiers in python
Python intrinsic functions
Saadbani
Fruitful functions in python
Columbus custom carpentry
Marking gauge classification
Types of lengthening joints
A carpentry hammer with a slightly rounded
Big carpentry
Holding tools classification
Carpentry joints
Software defined networking python
Piecewise function absolute value
How to solve evaluating functions
Evaluating functions and operations on functions
Work immersion portfolio weekly diary
Important points in work immersion
Smart work vs hard work
Work in present simple
Reciprocal model of social group work
What is social case
Team vs working group
Physics 03-02 potential energy and conservative forces
Chapter 4 section 1 work and machines answer key
I work all night
Smart work vs hard work group discussion
Work immersion checklist
Basic compiler functions
System software functions
Types of utility software
Iwp meaning in construction
Work rest hours software
Software maintenance in software engineering ppt
Frank maurer
Improving software economics
Software engineering
Metrics computer science
Application software and system software difference
Generic product in software engineering
Difference between student software and industrial software
Example of software crisis
Software measurement and metrics in software engineering
Is an os system software or application software
Eic software software review
Real time software design in software engineering
Design principles in software engineering
Graphics & multimedia software
Electronic copyright office
Tmg copyright
Copyright houghton mifflin company
Hunger games copyright
Copyright is debit or credit in trial balance
Copyright 2015 all rights reserved
Population growth factors
Copyright 2019 macnica
Copyright 2008
Difference between copyright and patent
Alex pagliaro
Geographic distribution of species evolution
Encyclopedia britannica copyright
Illium
A right handed operator generally begins seated at
1mbti
Copyright disclaimer
Copyright quiz for students
Copyright notice on powerpoint slides
Video copyright attorneys
The sonny bono copyright extension act
Copyright chaos
Copyright 2008
Copyright 2003