Programming Language Concepts Its Applications Mahmoud Ali Ahmed
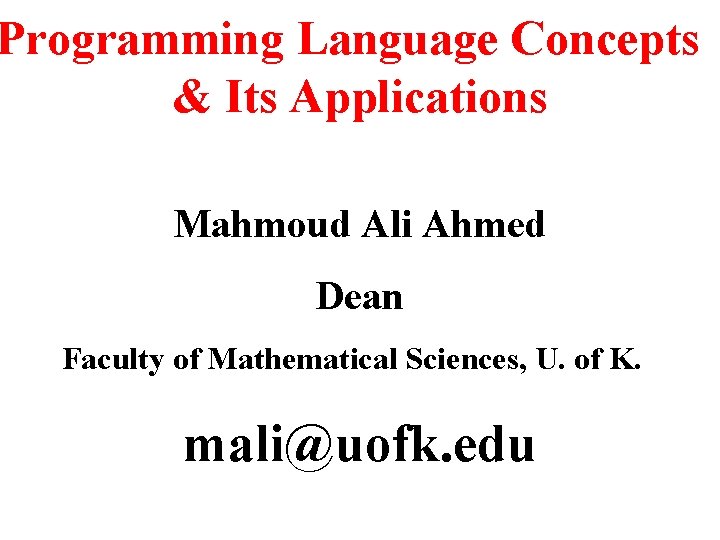
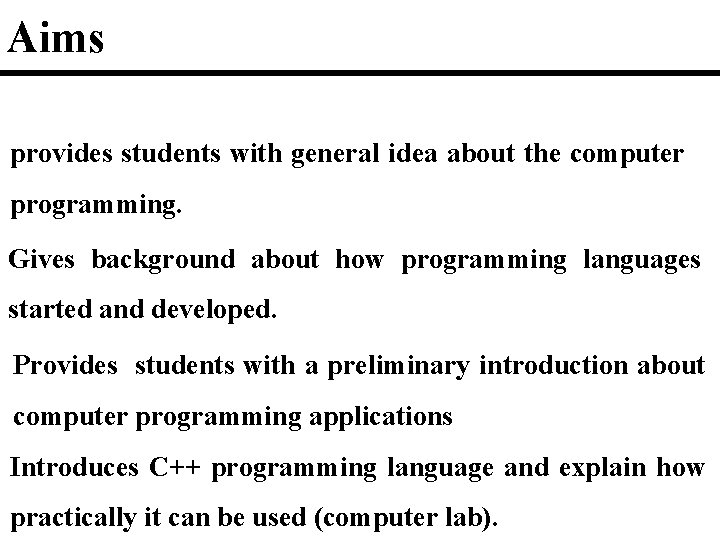
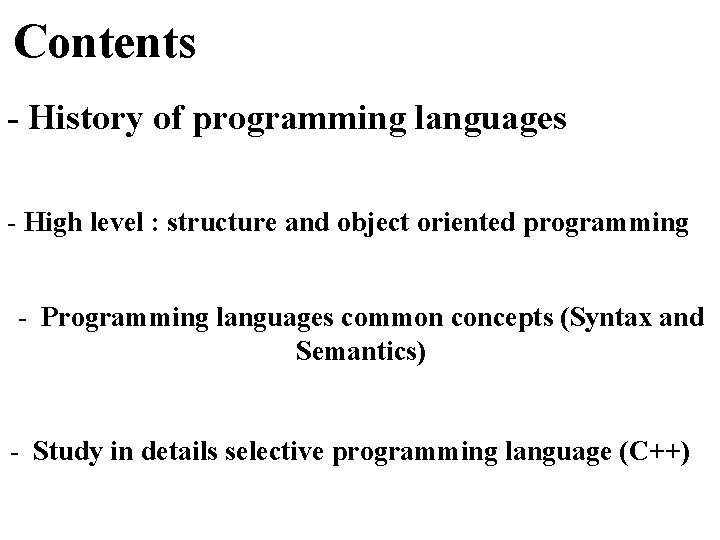
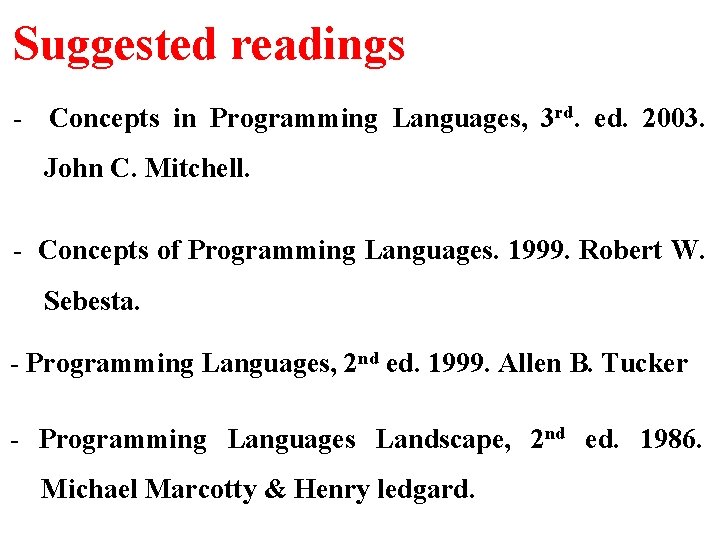
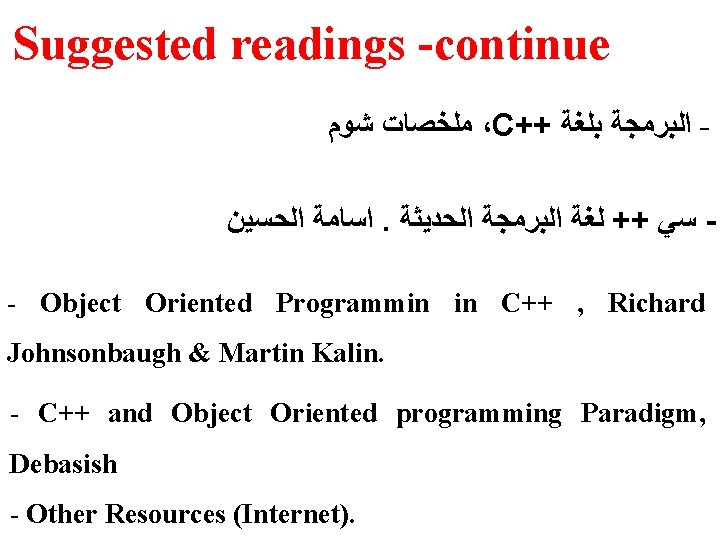
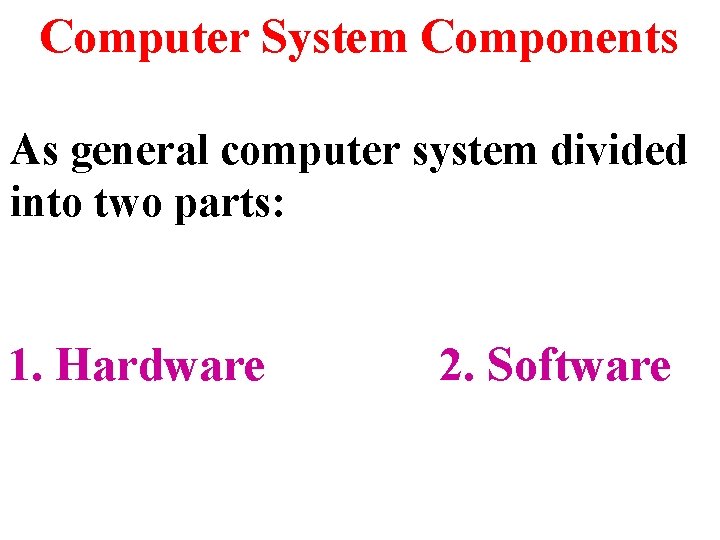
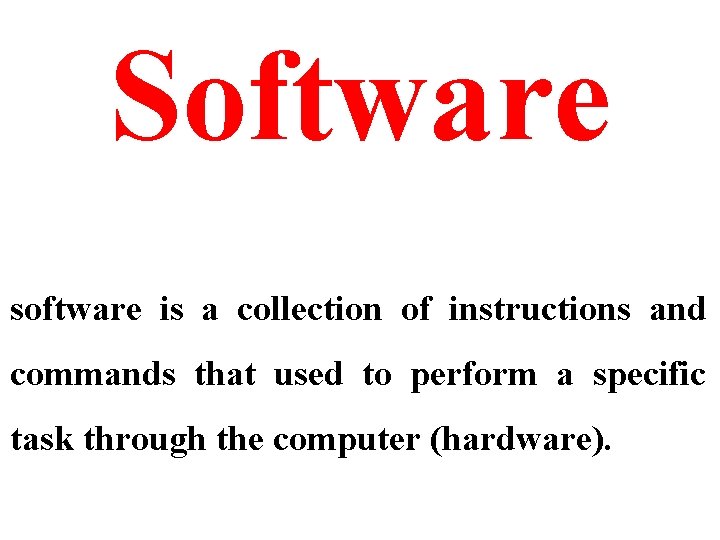
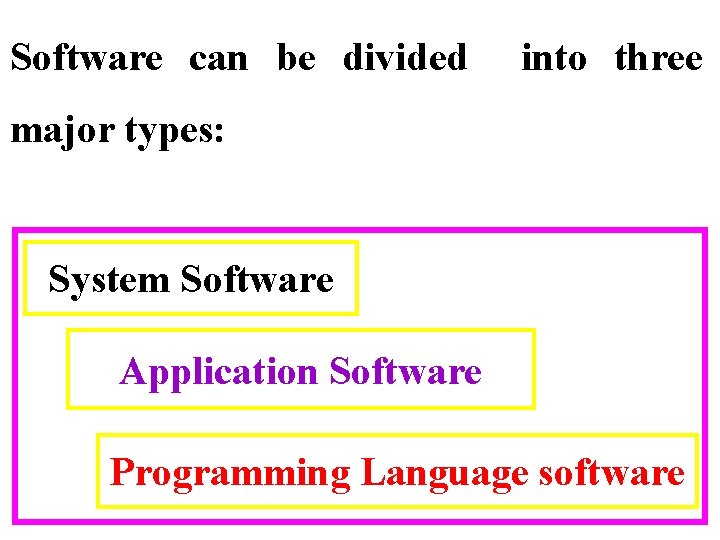
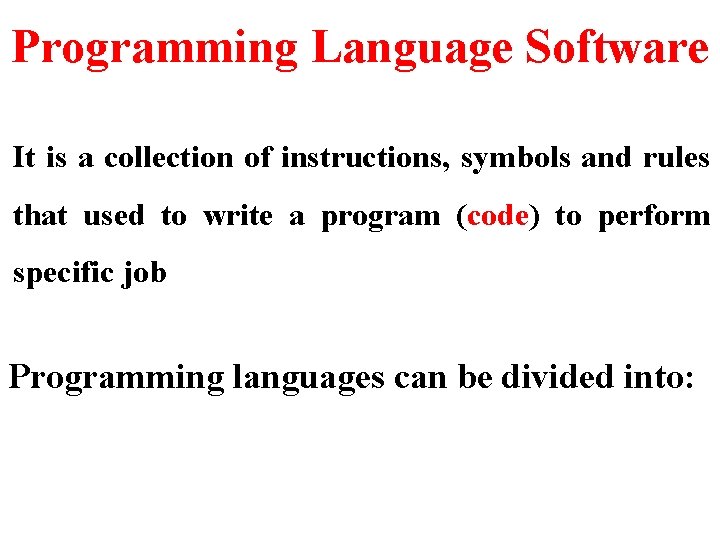
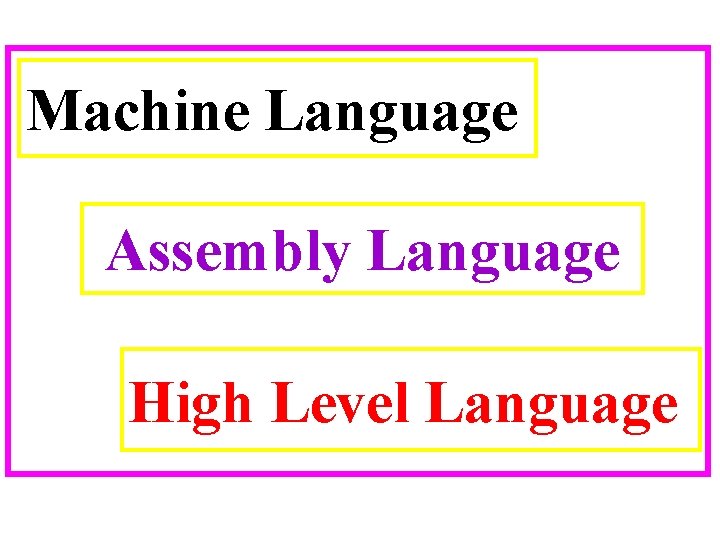
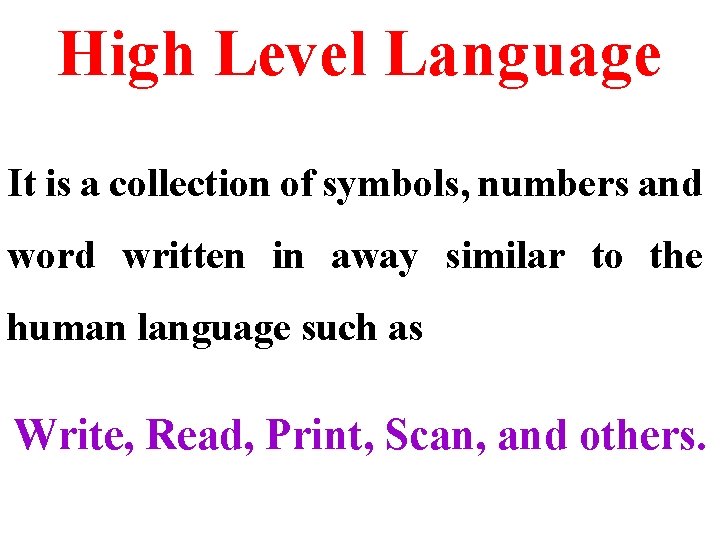
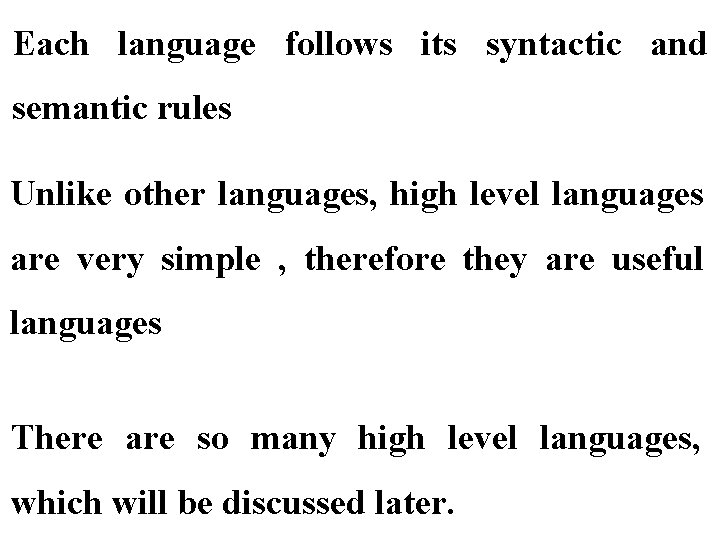
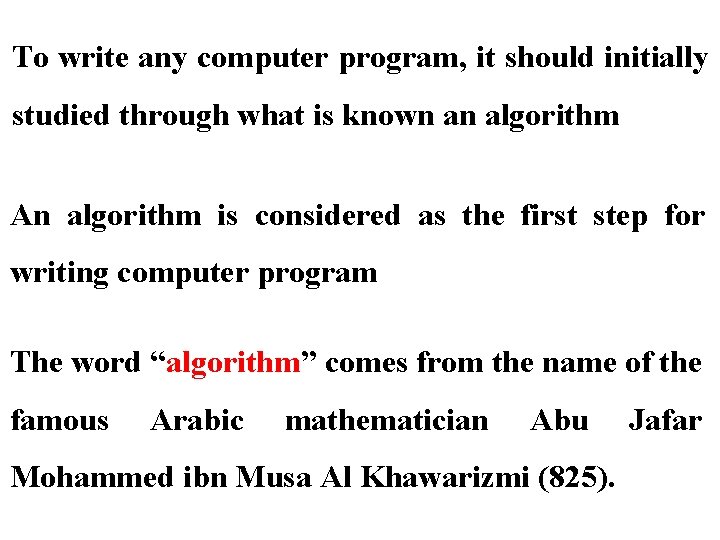
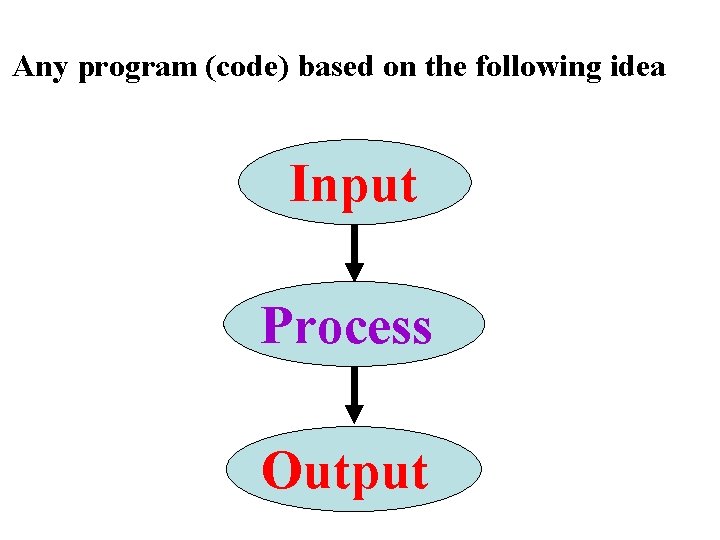
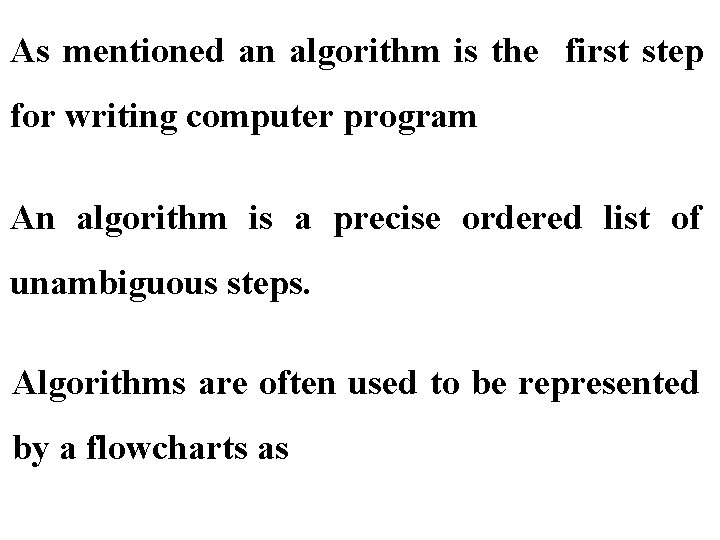
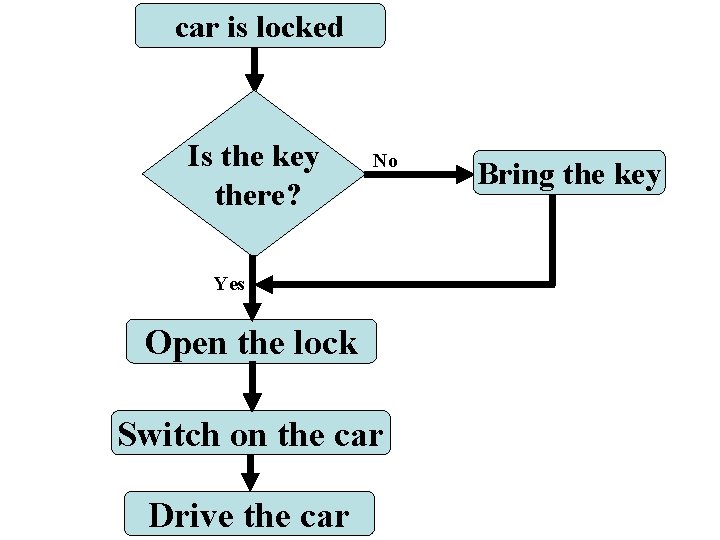
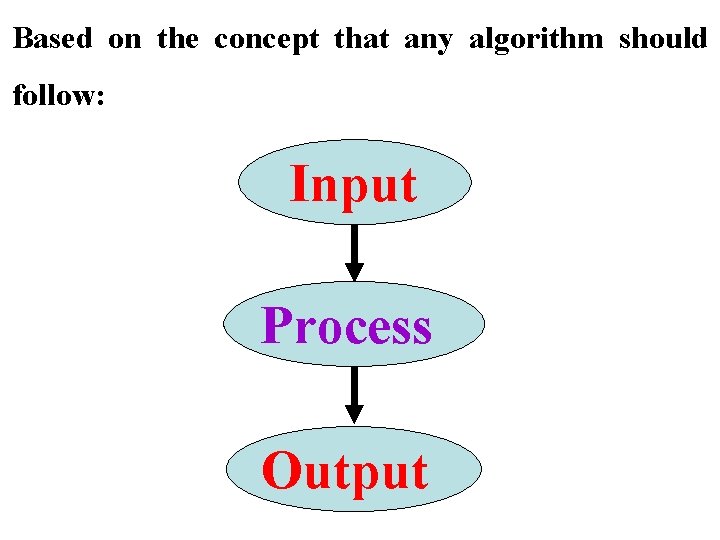
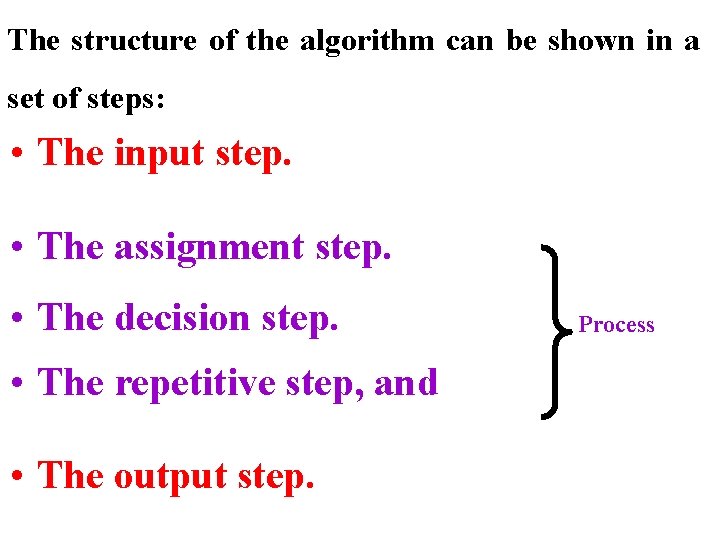
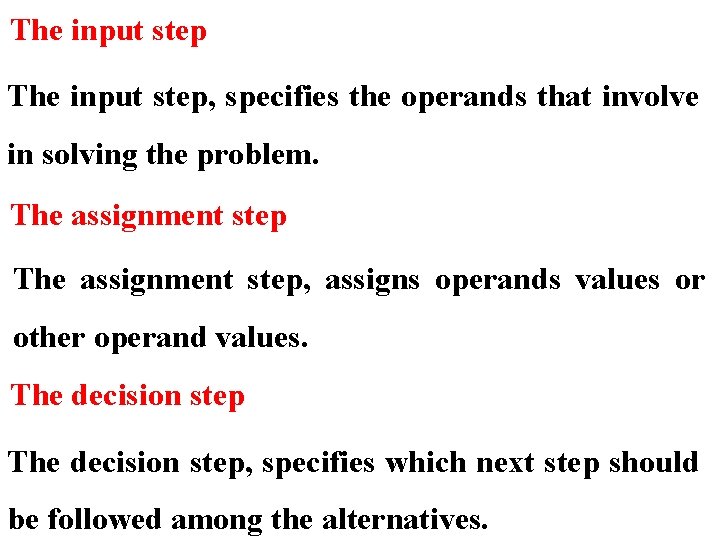
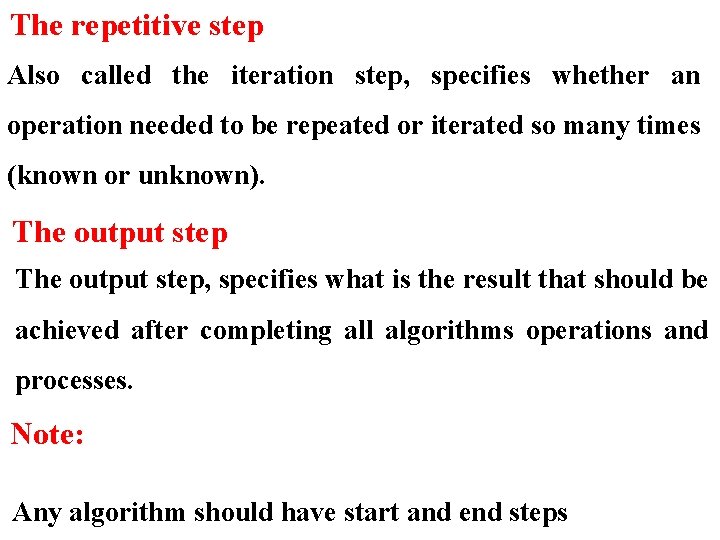
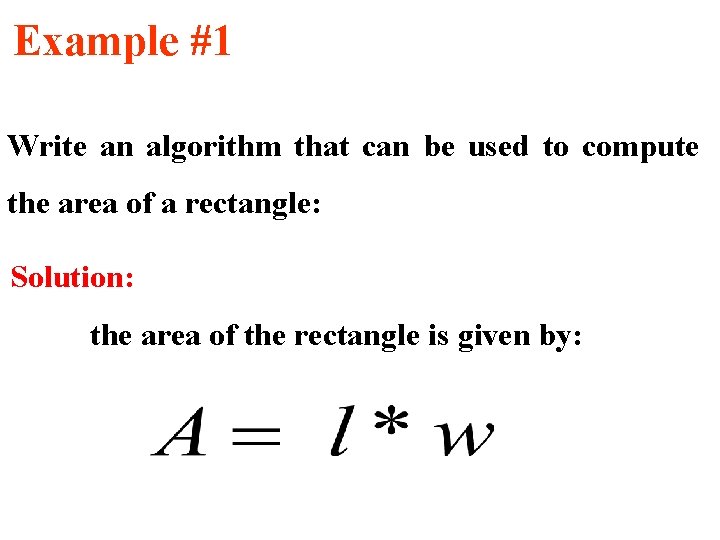
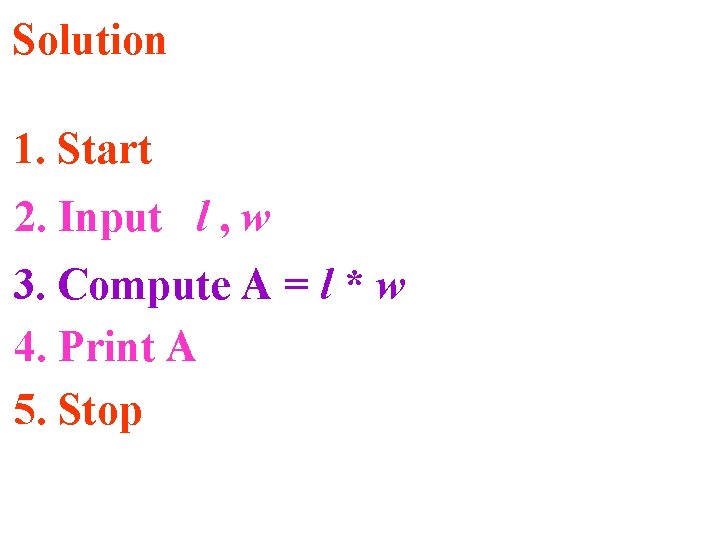
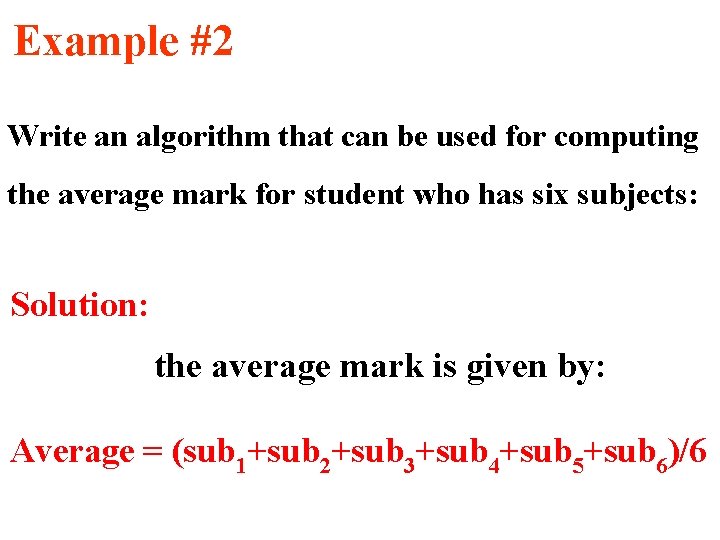
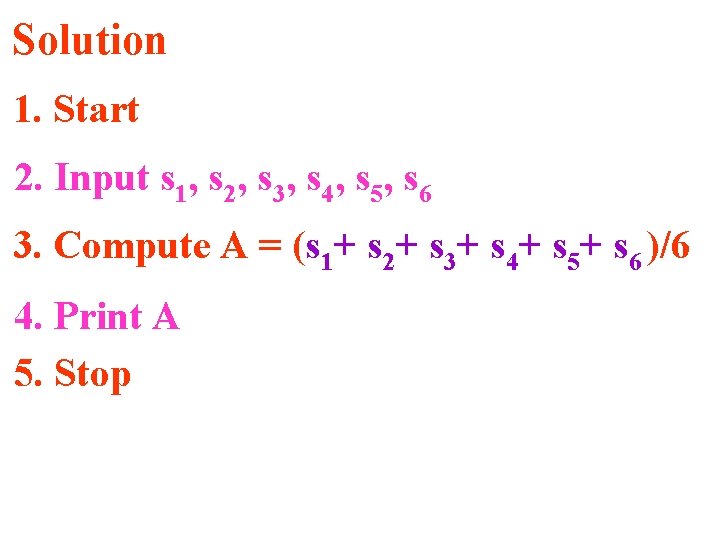
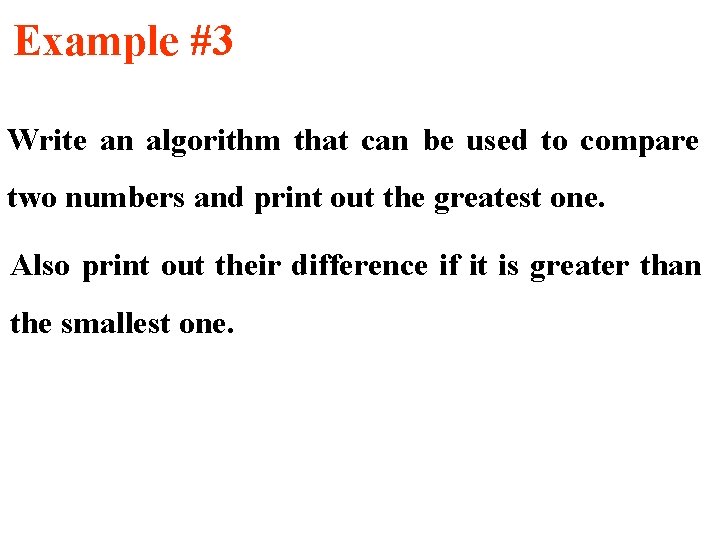
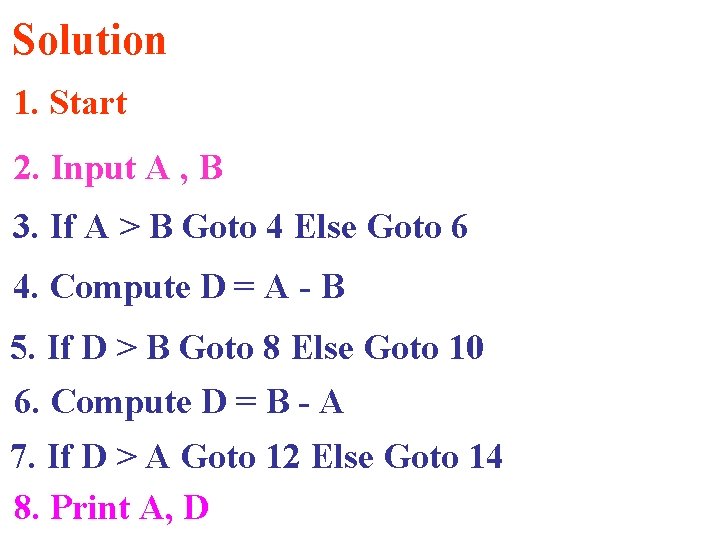
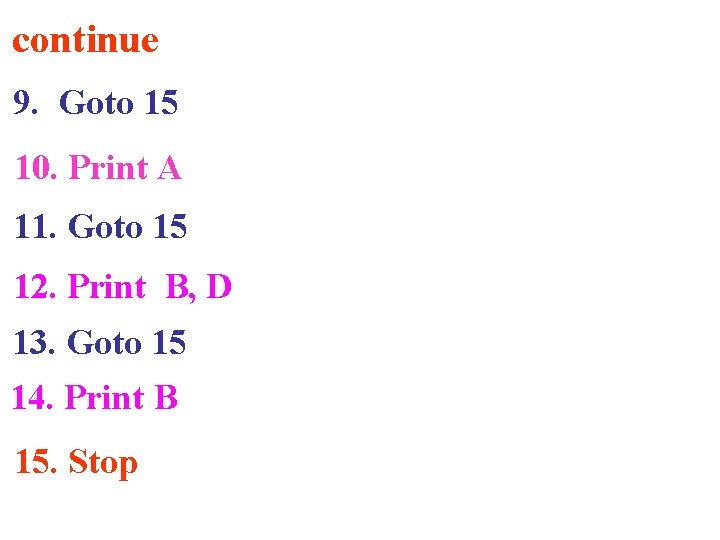
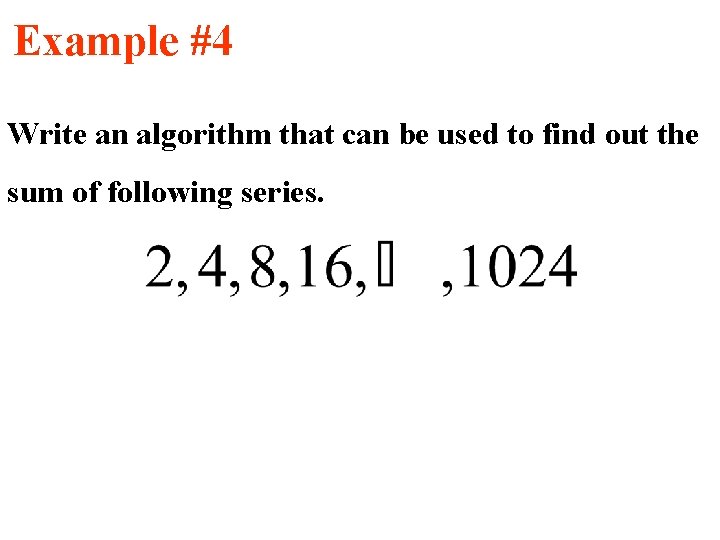
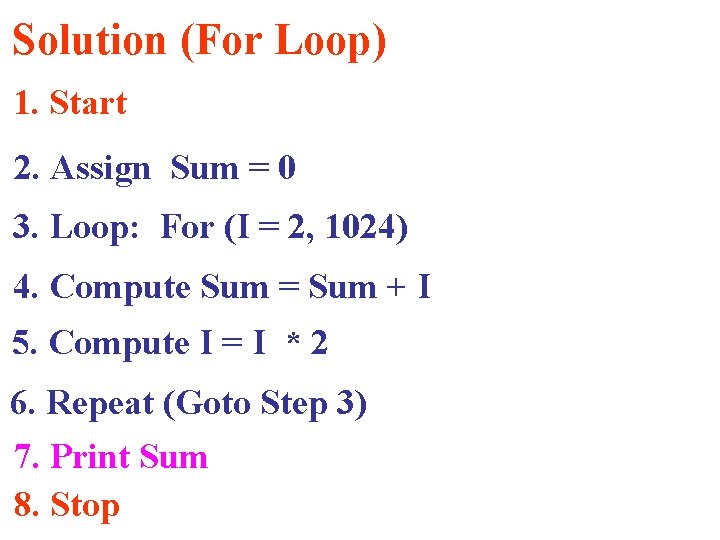
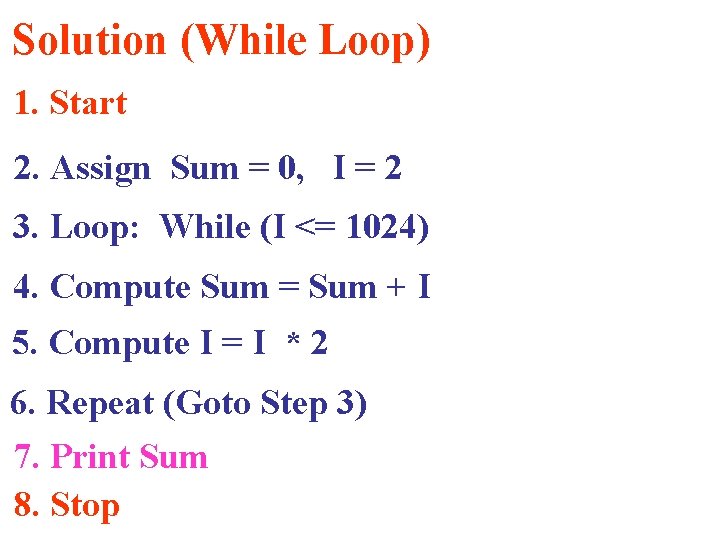
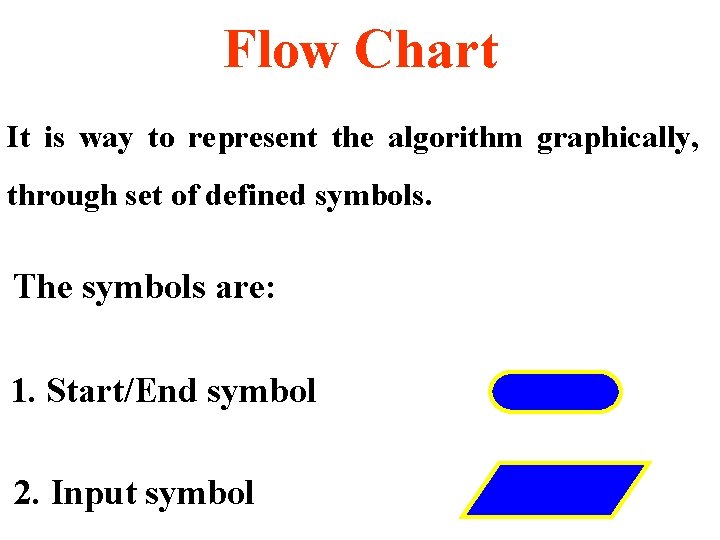
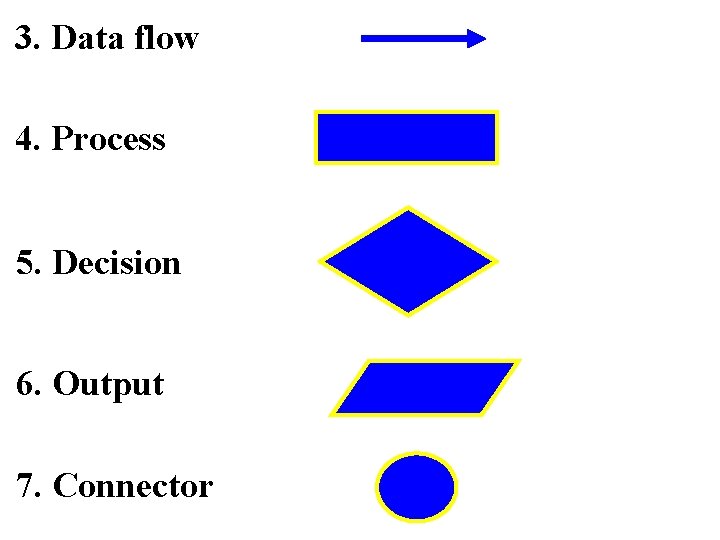
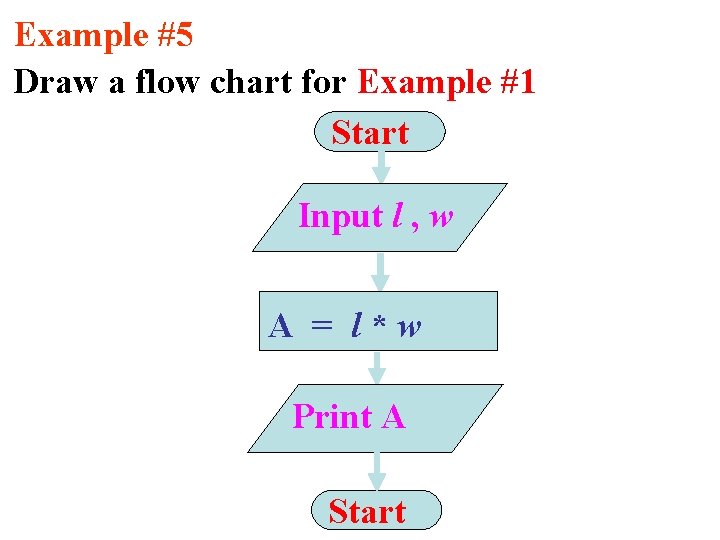
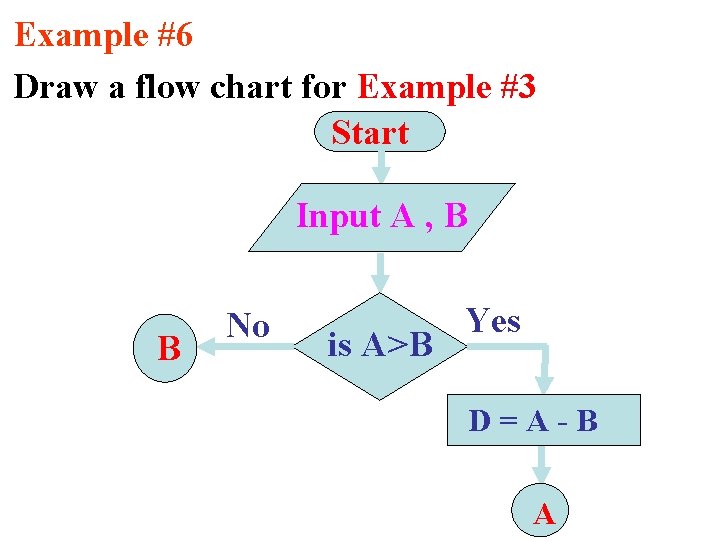
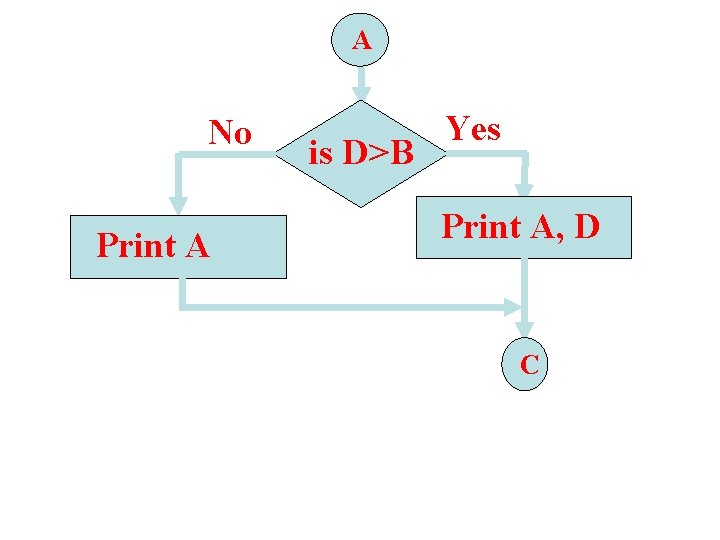
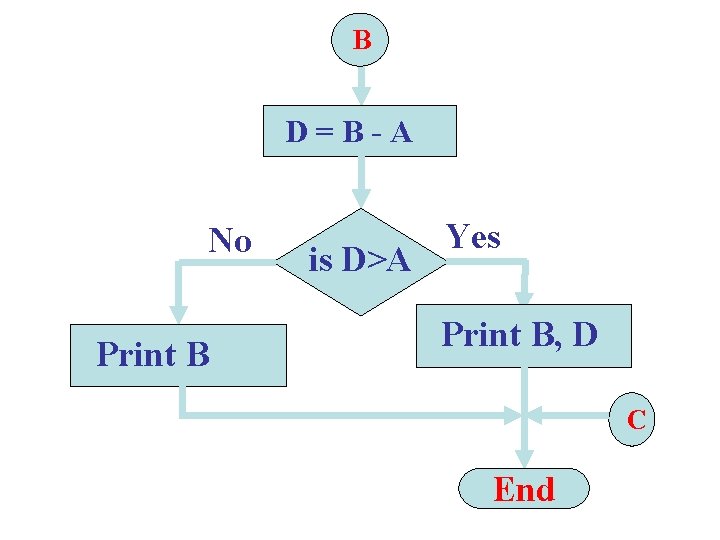
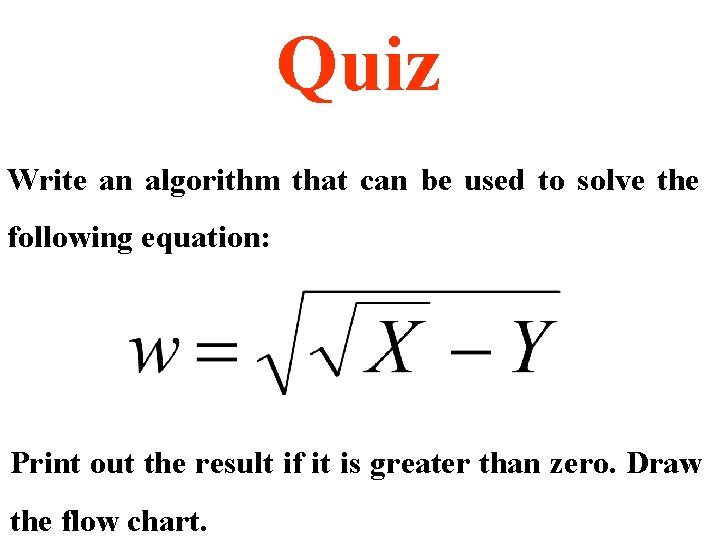
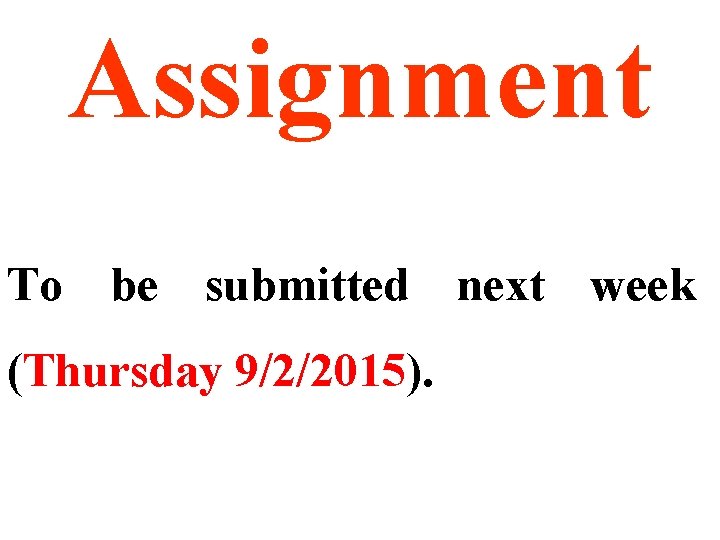
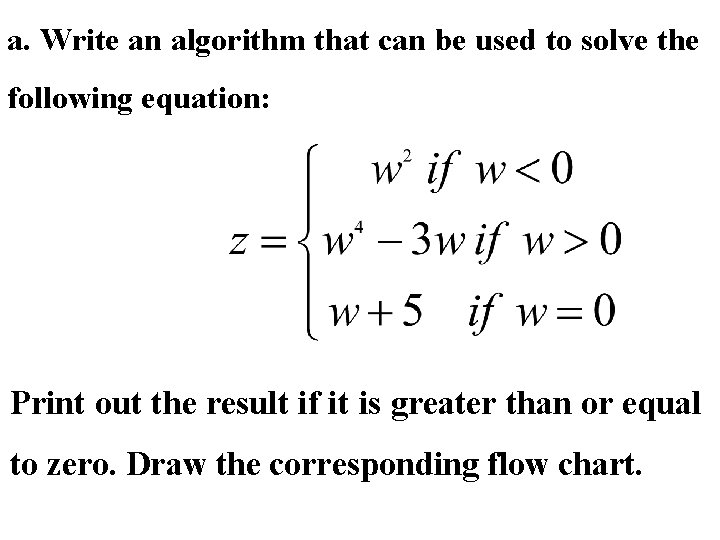
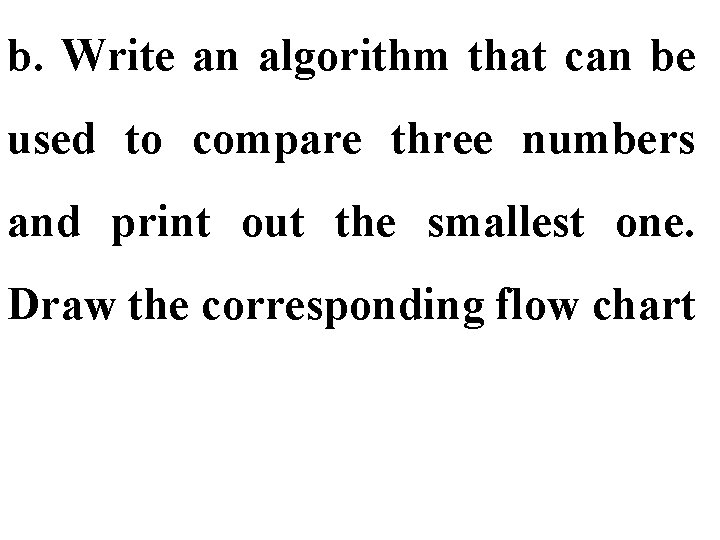
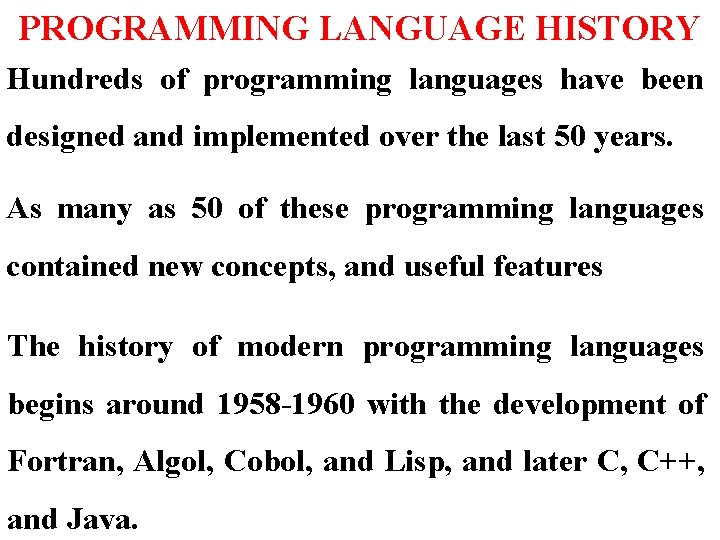
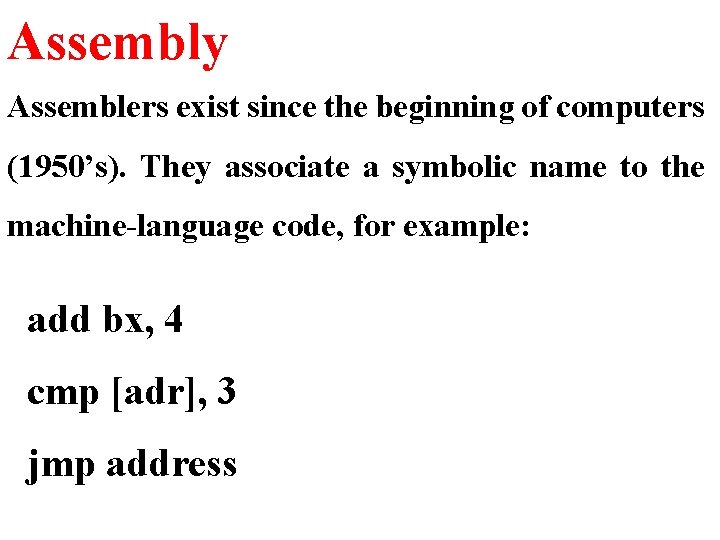
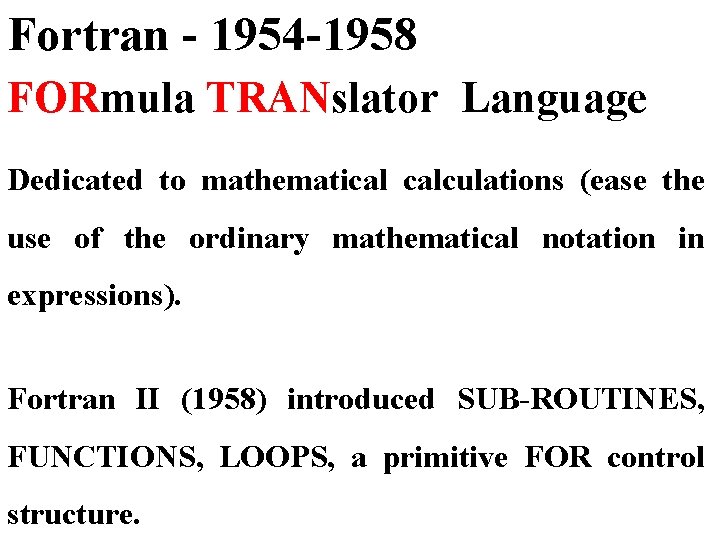
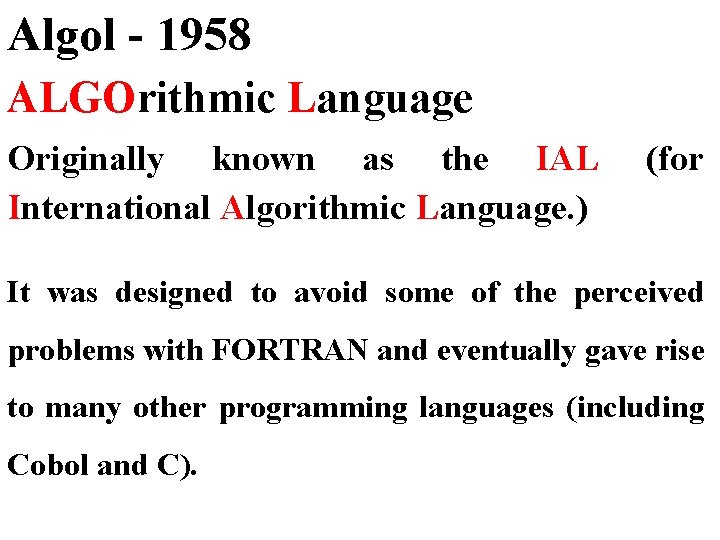
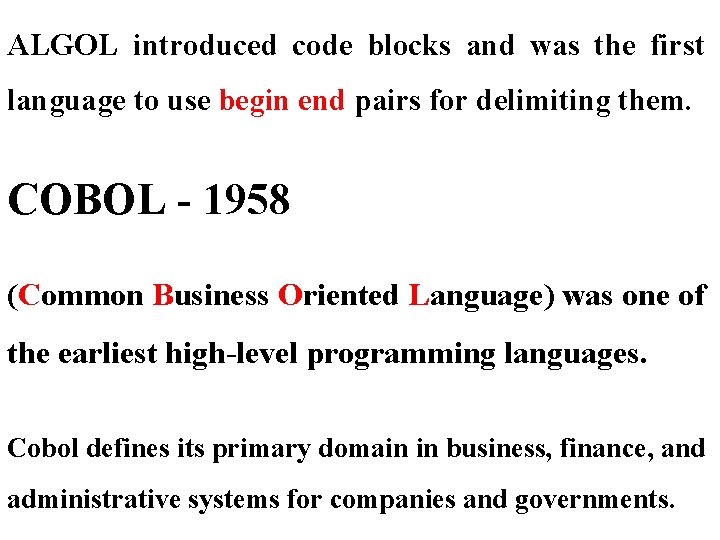
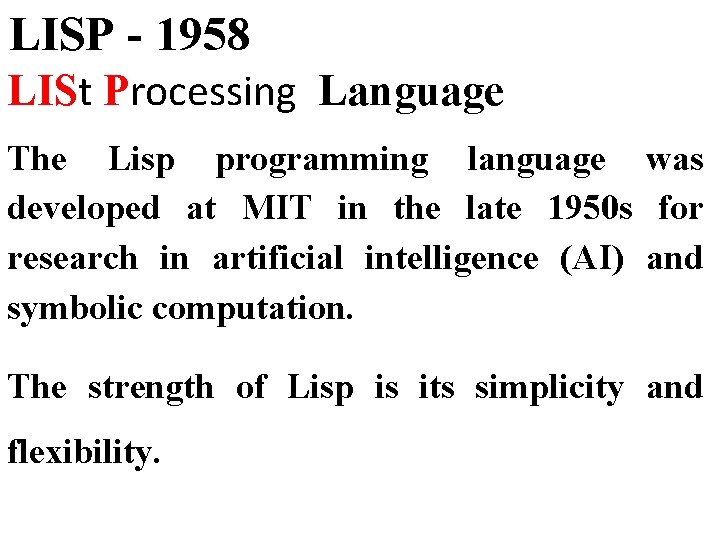
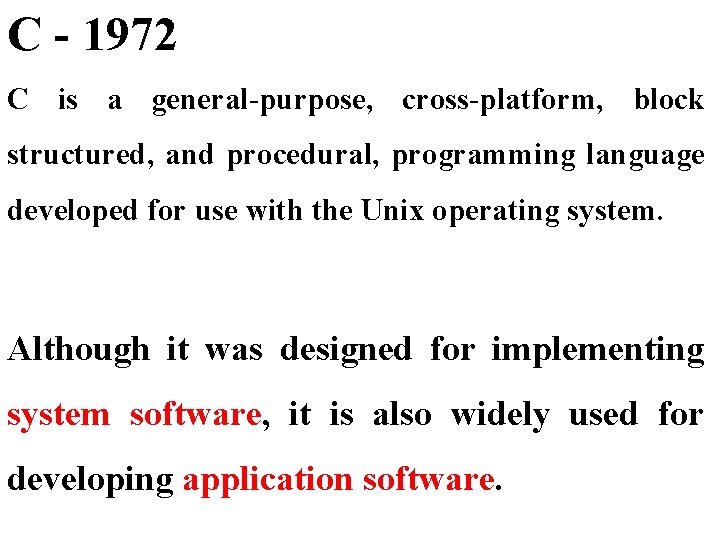
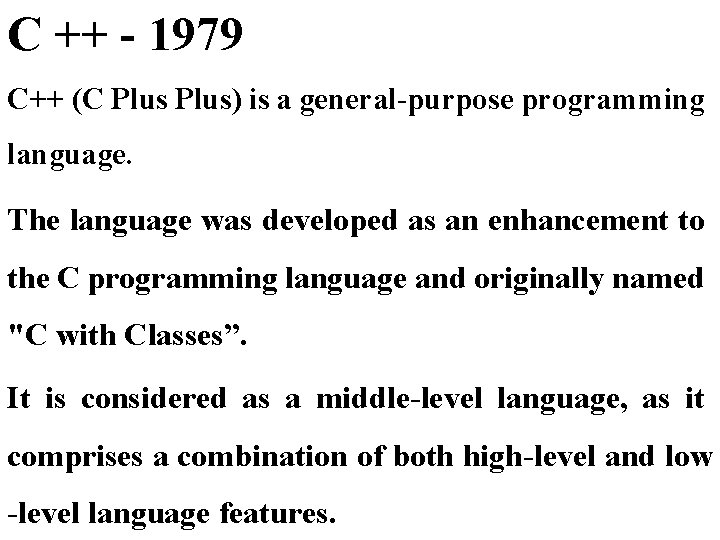
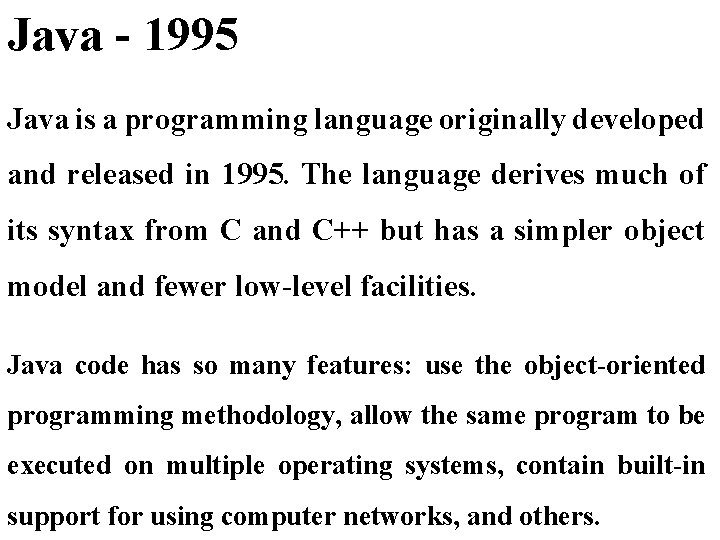
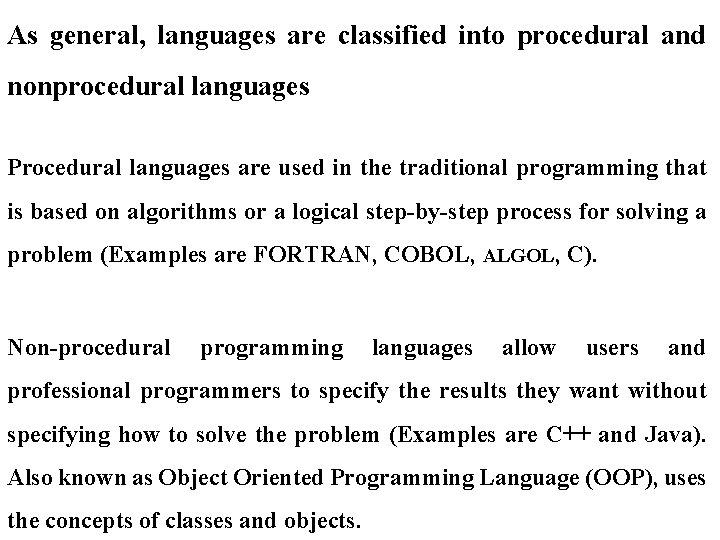
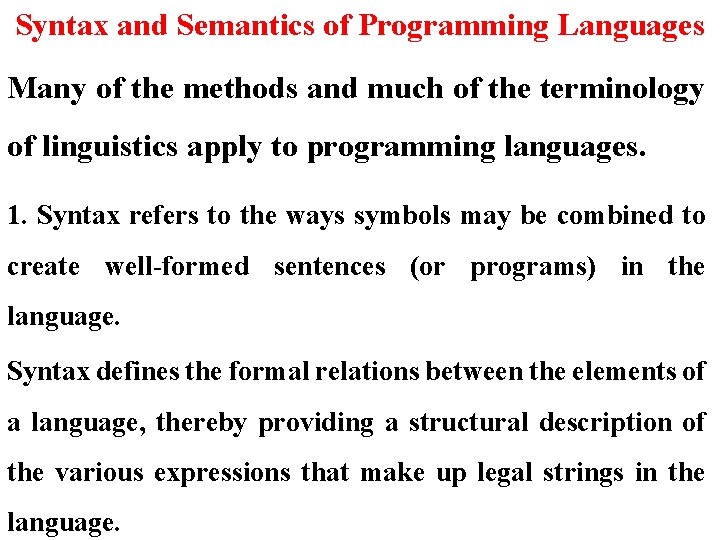
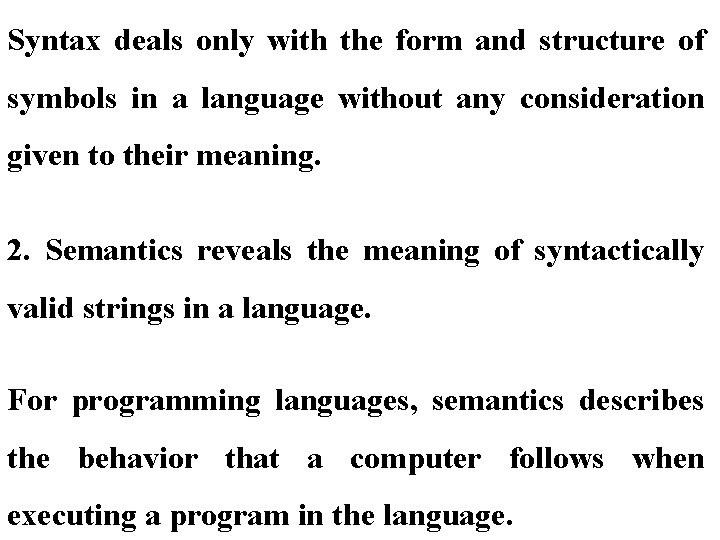
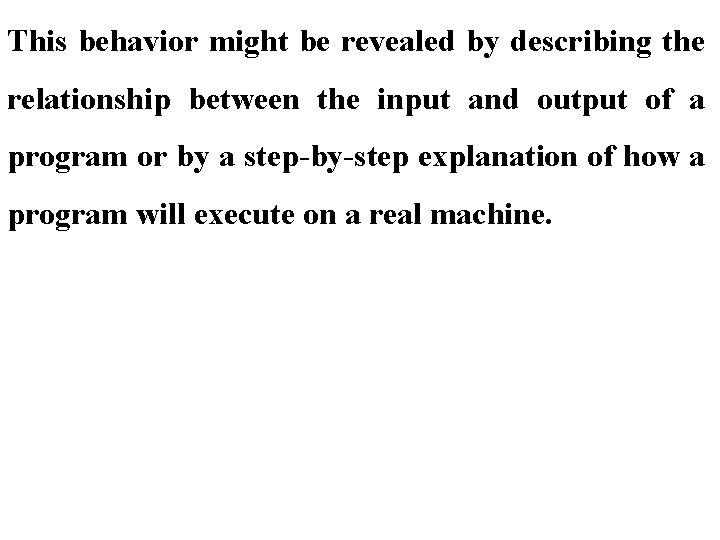
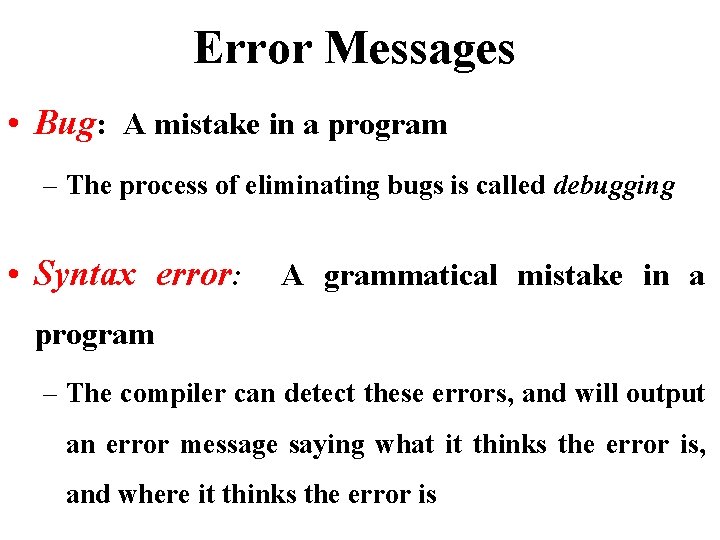
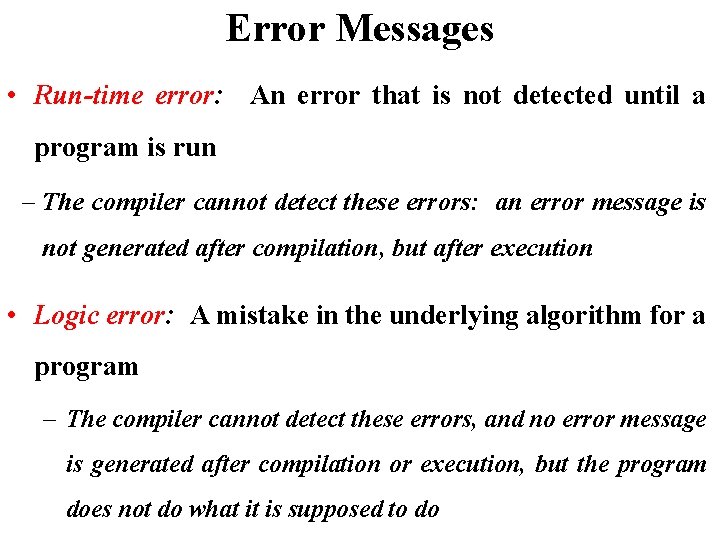
- Slides: 55
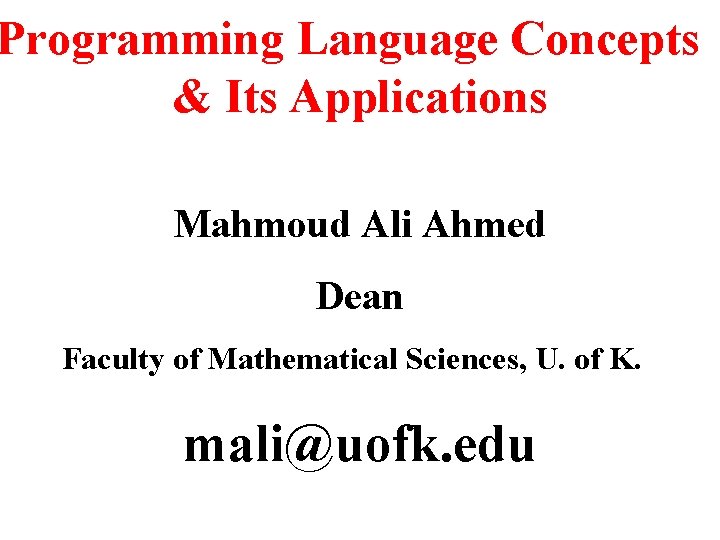
Programming Language Concepts & Its Applications Mahmoud Ali Ahmed Dean Faculty of Mathematical Sciences, U. of K. mali@uofk. edu
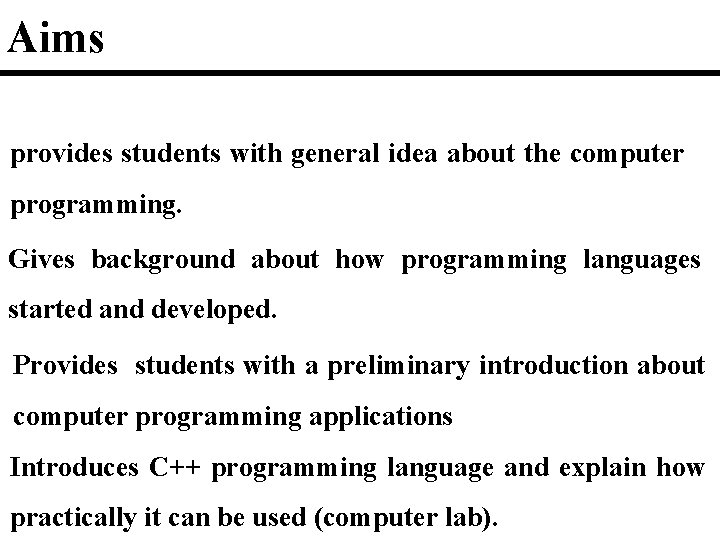
Aims provides students with general idea about the computer programming. Gives background about how programming languages started and developed. Provides students with a preliminary introduction about computer programming applications Introduces C++ programming language and explain how practically it can be used (computer lab).
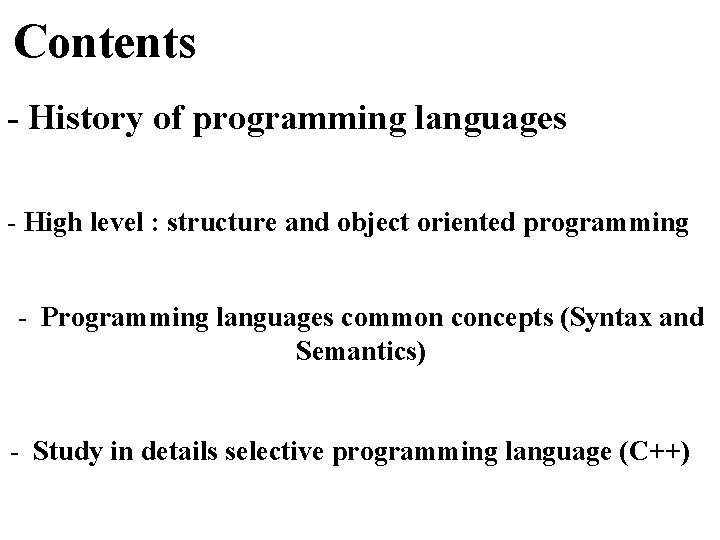
Contents - History of programming languages - High level : structure and object oriented programming - Programming languages common concepts (Syntax and Semantics) - Study in details selective programming language (C++)
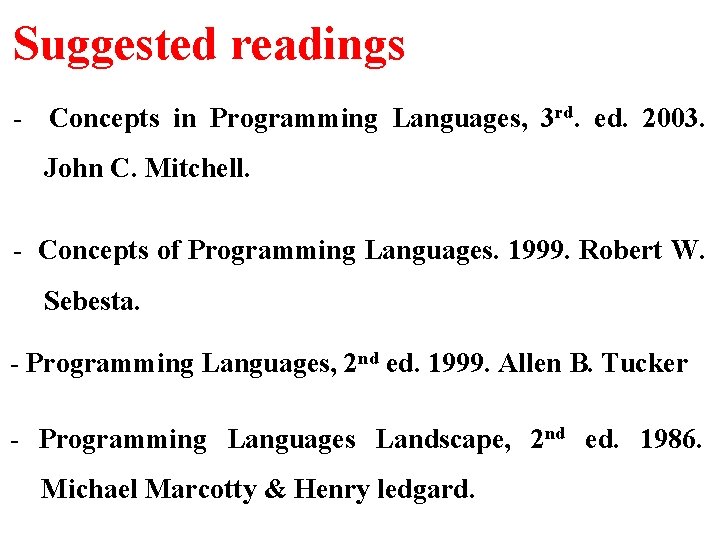
Suggested readings - Concepts in Programming Languages, 3 rd. ed. 2003. John C. Mitchell. - Concepts of Programming Languages. 1999. Robert W. Sebesta. - Programming Languages, 2 nd ed. 1999. Allen B. Tucker - Programming Languages Landscape, 2 nd ed. 1986. Michael Marcotty & Henry ledgard.
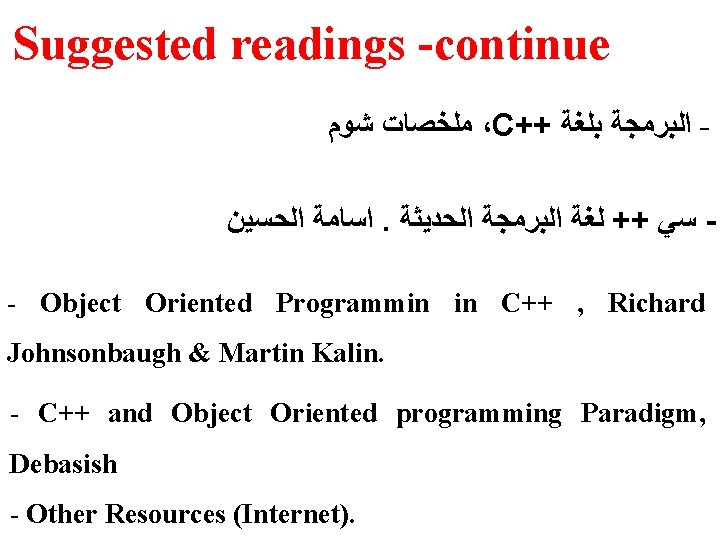
Suggested readings -continue ﻣﻠﺨﺼﺎﺕ ﺷﻮﻡ ،C++ ﺍﻟﺒﺮﻣﺠﺔ ﺑﻠﻐﺔ ﺍﺳﺎﻣﺔ ﺍﻟﺤﺴﻴﻦ. ﻟﻐﺔ ﺍﻟﺒﺮﻣﺠﺔ ﺍﻟﺤﺪﻳﺜﺔ ++ ﺳﻲ - Object Oriented Programmin in C++ , Richard Johnsonbaugh & Martin Kalin. - C++ and Object Oriented programming Paradigm, Debasish - Other Resources (Internet).
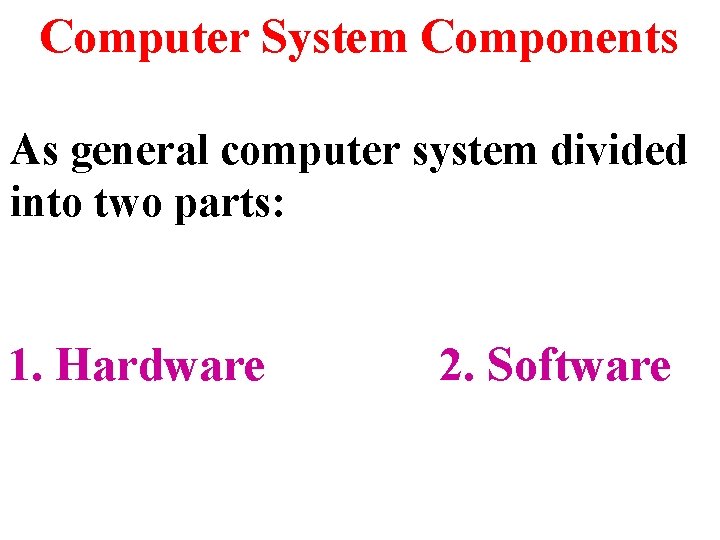
Computer System Components As general computer system divided into two parts: 1. Hardware 2. Software
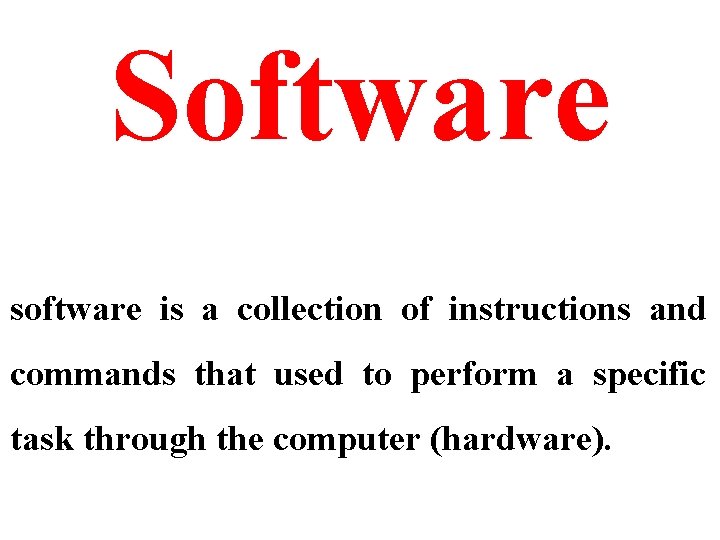
Software software is a collection of instructions and commands that used to perform a specific task through the computer (hardware).
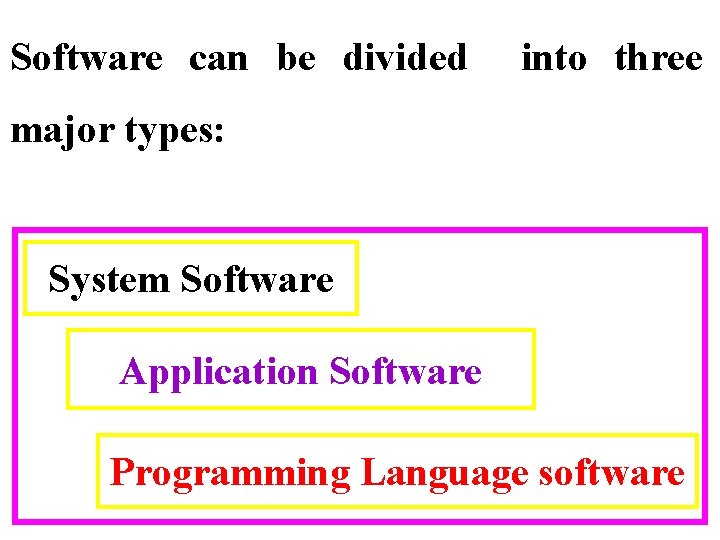
Software can be divided into three major types: System Software Application Software Programming Language software
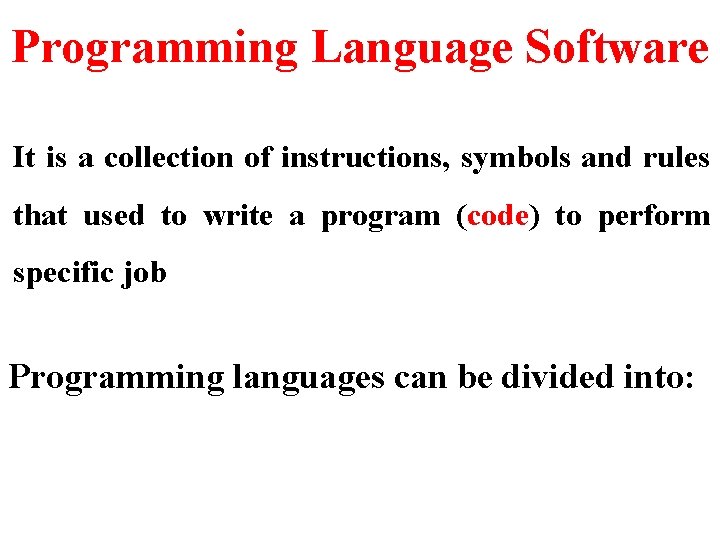
Programming Language Software It is a collection of instructions, symbols and rules that used to write a program (code) to perform specific job Programming languages can be divided into:
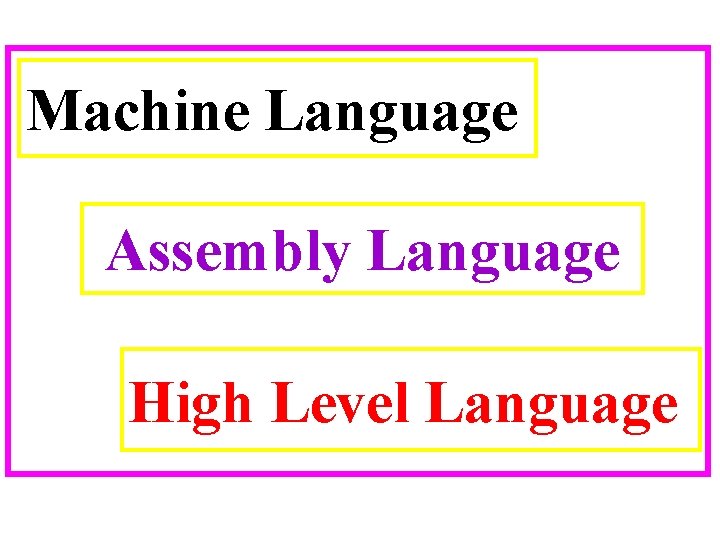
Machine Language Assembly Language High Level Language
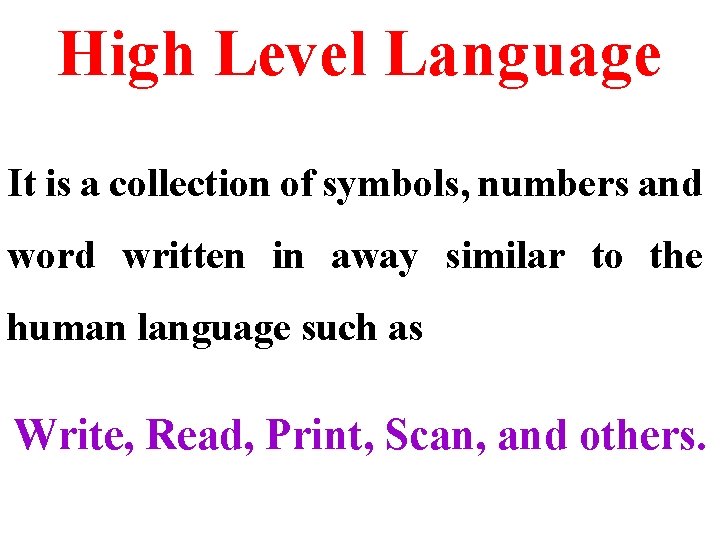
High Level Language It is a collection of symbols, numbers and word written in away similar to the human language such as Write, Read, Print, Scan, and others.
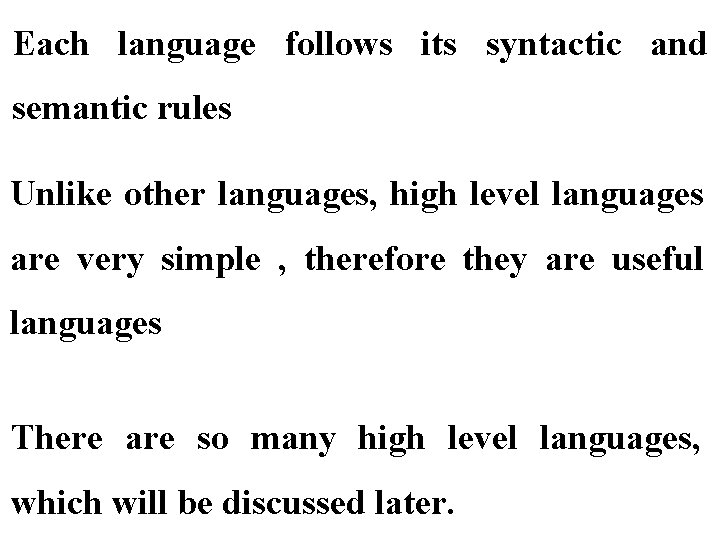
Each language follows its syntactic and semantic rules Unlike other languages, high level languages are very simple , therefore they are useful languages There are so many high level languages, which will be discussed later.
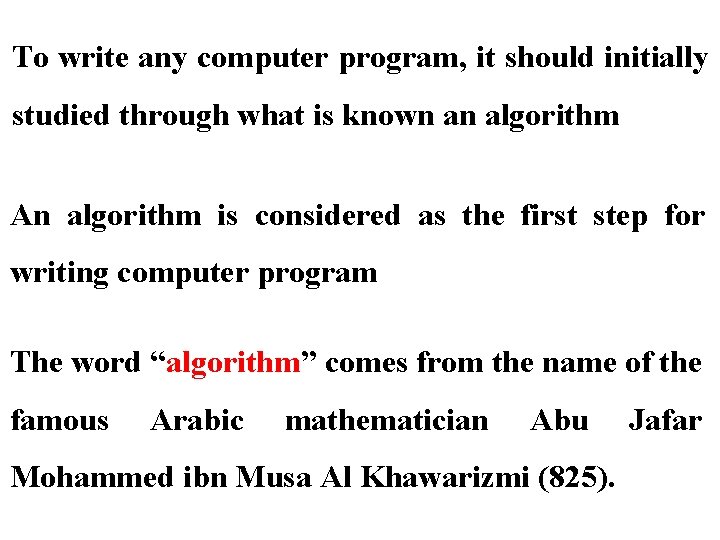
To write any computer program, it should initially studied through what is known an algorithm An algorithm is considered as the first step for writing computer program The word “algorithm” comes from the name of the famous Arabic mathematician Abu Mohammed ibn Musa Al Khawarizmi (825). Jafar
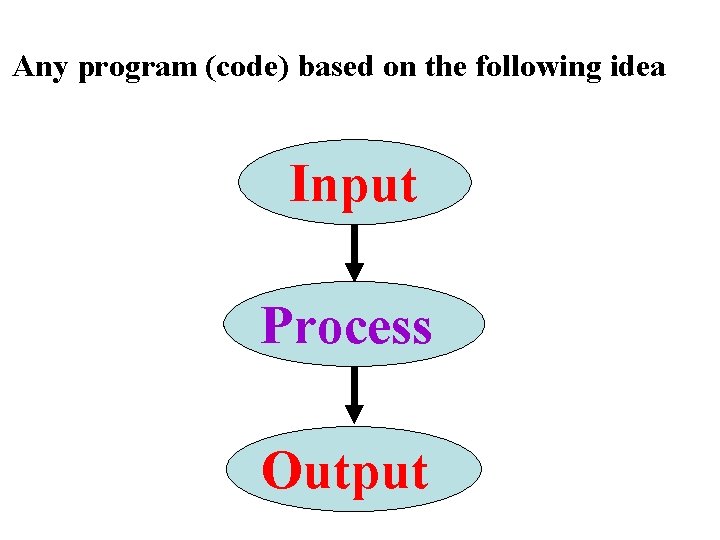
Any program (code) based on the following idea Input Process Output
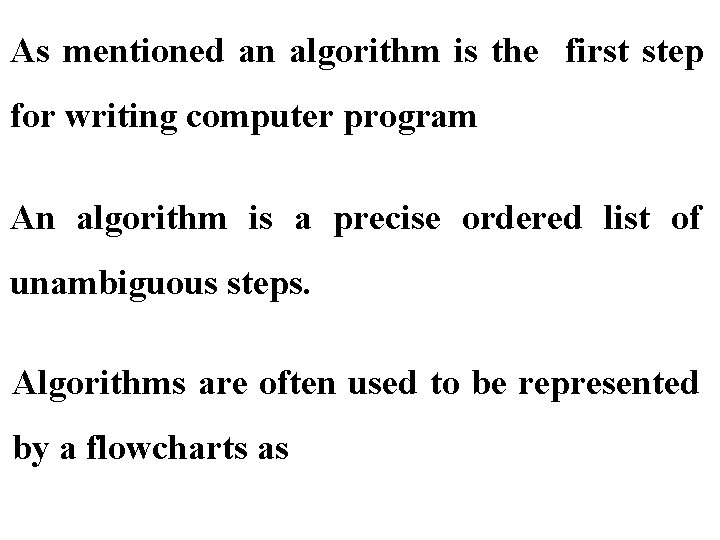
As mentioned an algorithm is the first step for writing computer program An algorithm is a precise ordered list of unambiguous steps. Algorithms are often used to be represented by a flowcharts as
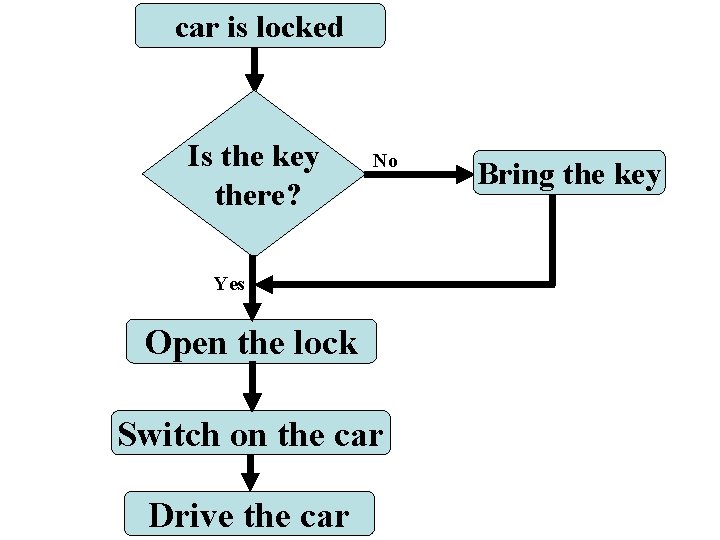
car is locked Is the key there? No Yes Open the lock Switch on the car Drive the car Bring the key
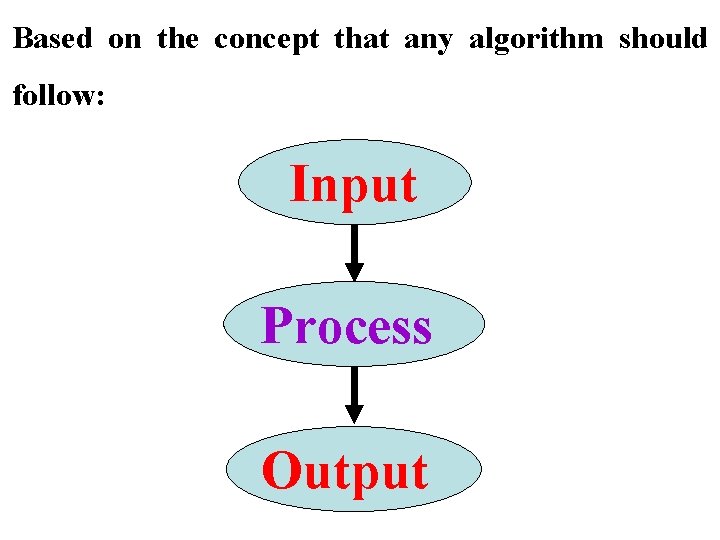
Based on the concept that any algorithm should follow: Input Process Output
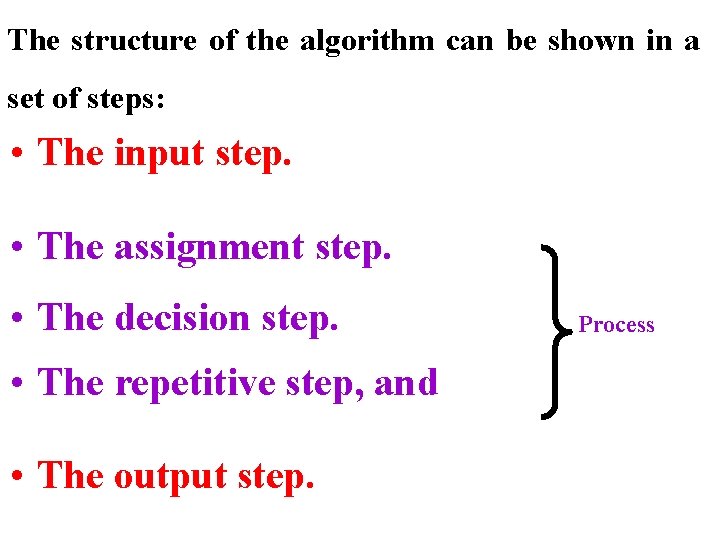
The structure of the algorithm can be shown in a set of steps: • The input step. • The assignment step. • The decision step. • The repetitive step, and • The output step. Process
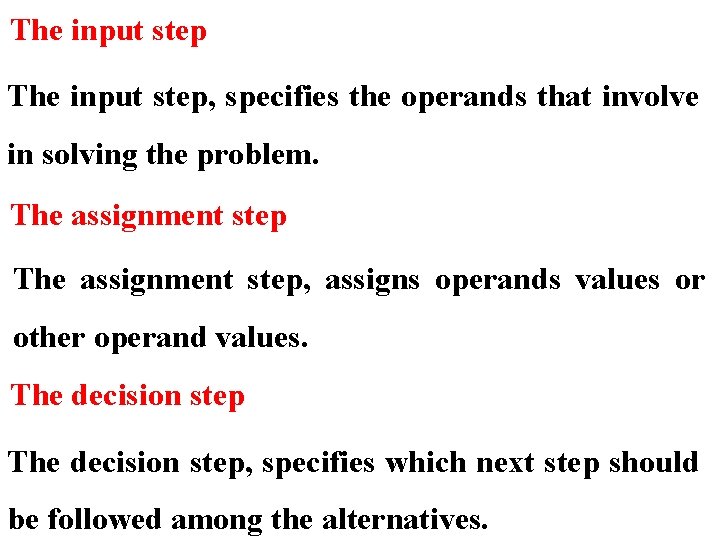
The input step, specifies the operands that involve in solving the problem. The assignment step, assigns operands values or other operand values. The decision step, specifies which next step should be followed among the alternatives.
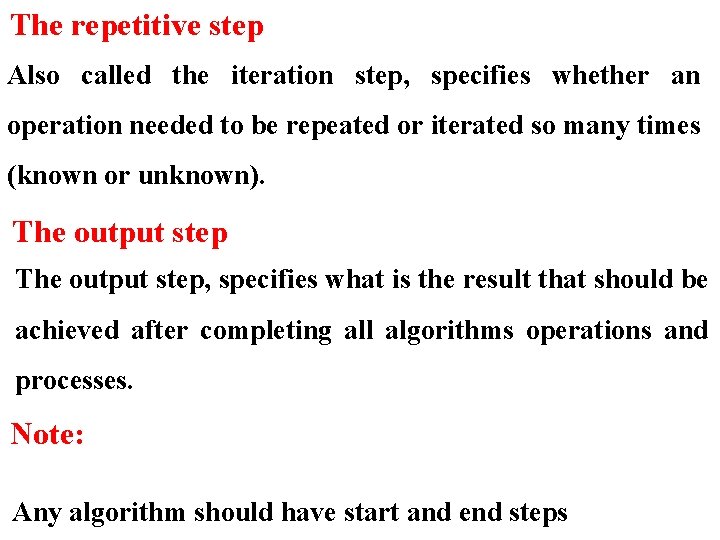
The repetitive step Also called the iteration step, specifies whether an operation needed to be repeated or iterated so many times (known or unknown). The output step, specifies what is the result that should be achieved after completing all algorithms operations and processes. Note: Any algorithm should have start and end steps
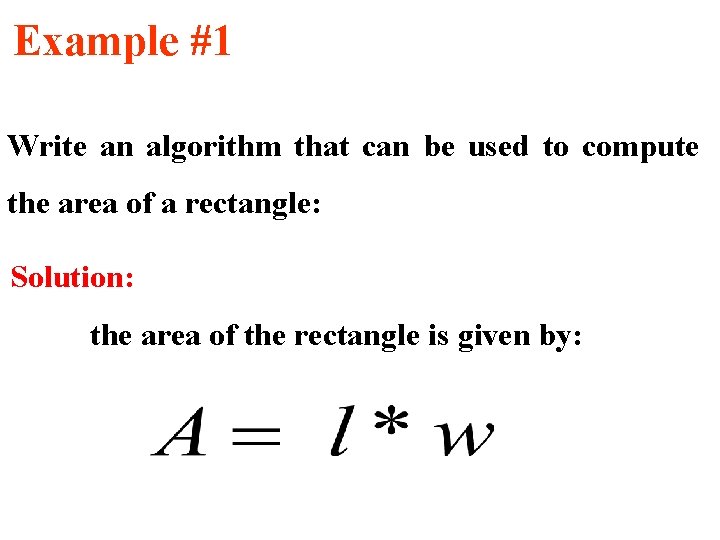
Example #1 Write an algorithm that can be used to compute the area of a rectangle: Solution: the area of the rectangle is given by:
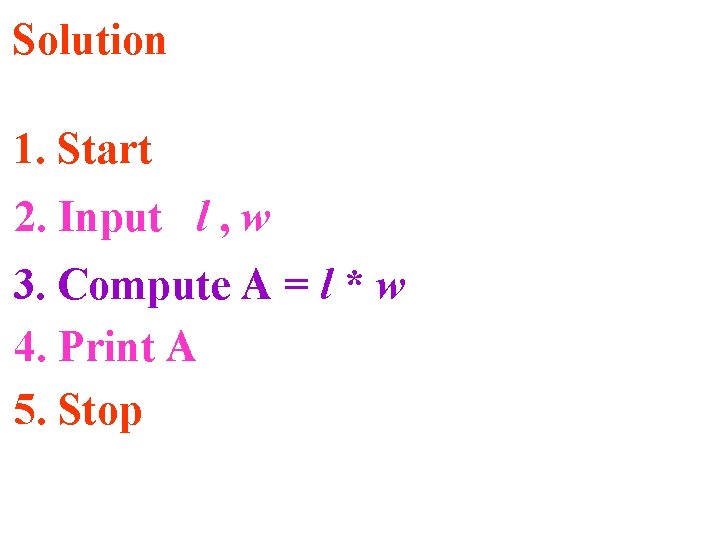
Solution 1. Start 2. Input l , w 3. Compute A = l * w 4. Print A 5. Stop
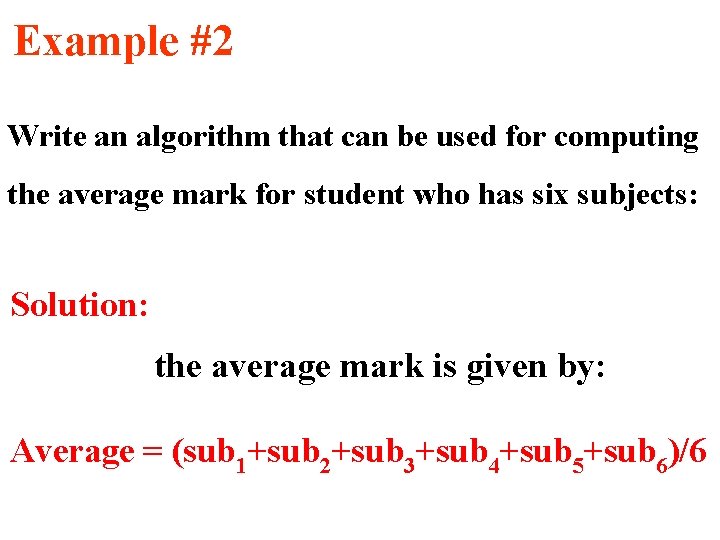
Example #2 Write an algorithm that can be used for computing the average mark for student who has six subjects: Solution: the average mark is given by: Average = (sub 1+sub 2+sub 3+sub 4+sub 5+sub 6)/6
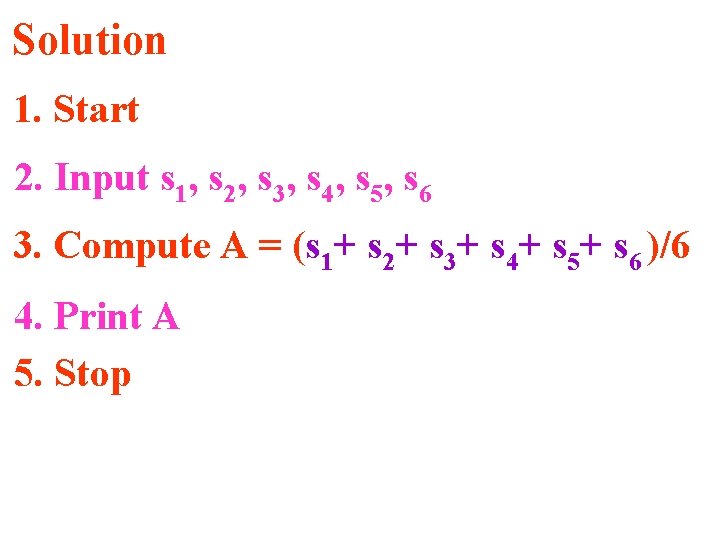
Solution 1. Start 2. Input s 1, s 2, s 3, s 4, s 5, s 6 3. Compute A = (s 1+ s 2+ s 3+ s 4+ s 5+ s 6 )/6 4. Print A 5. Stop
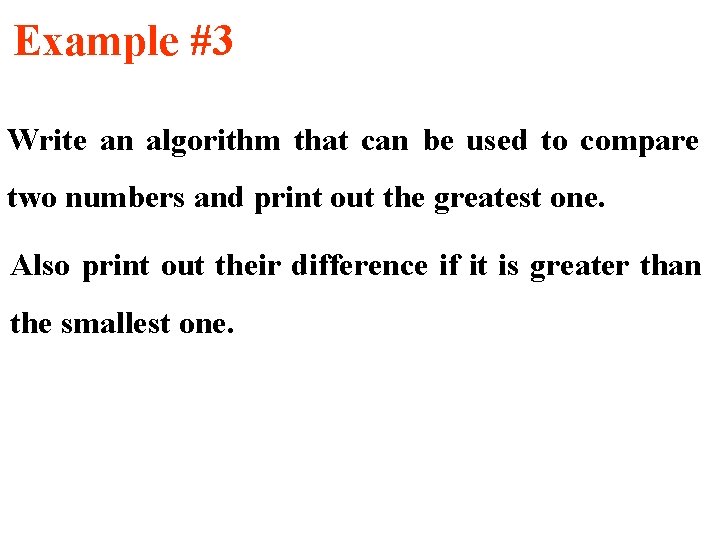
Example #3 Write an algorithm that can be used to compare two numbers and print out the greatest one. Also print out their difference if it is greater than the smallest one.
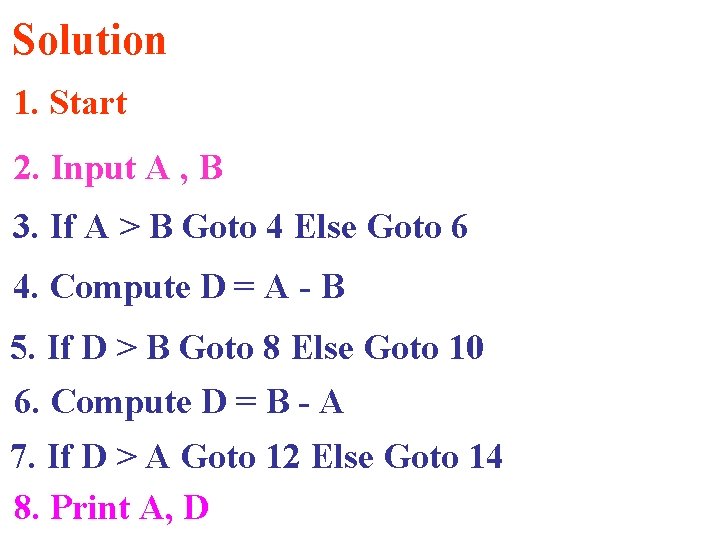
Solution 1. Start 2. Input A , B 3. If A > B Goto 4 Else Goto 6 4. Compute D = A - B 5. If D > B Goto 8 Else Goto 10 6. Compute D = B - A 7. If D > A Goto 12 Else Goto 14 8. Print A, D
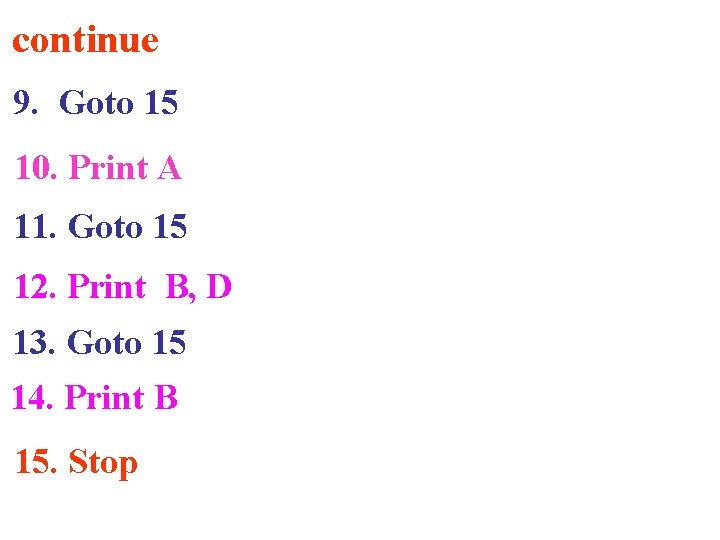
continue 9. Goto 15 10. Print A 11. Goto 15 12. Print B, D 13. Goto 15 14. Print B 15. Stop
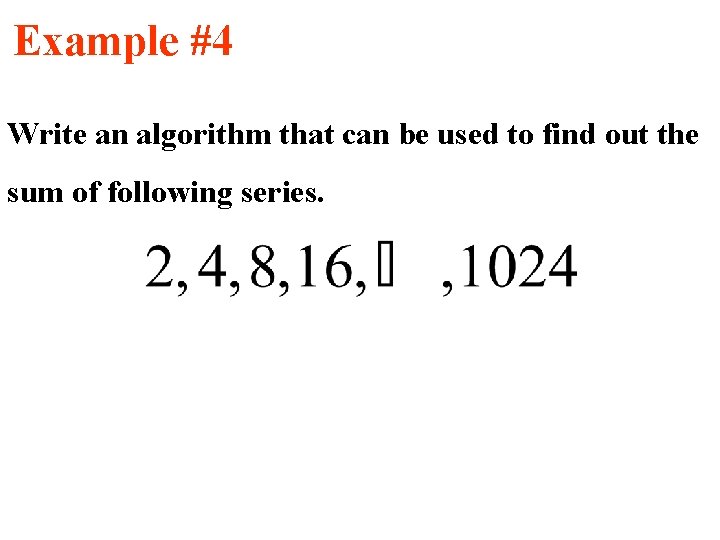
Example #4 Write an algorithm that can be used to find out the sum of following series.
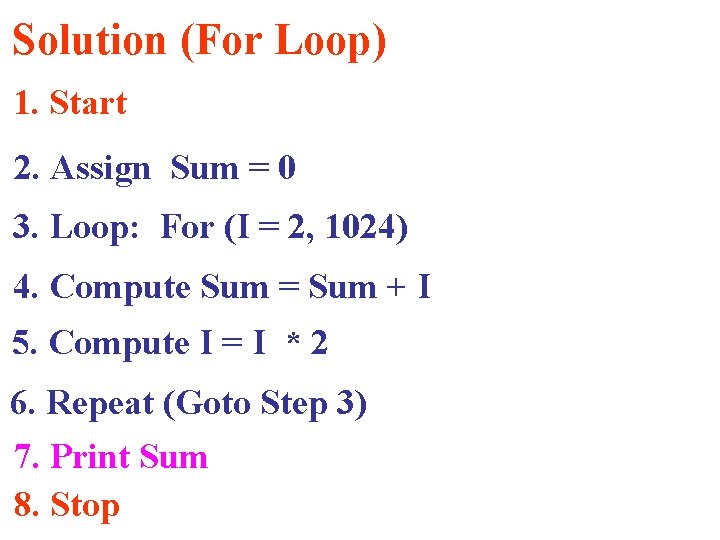
Solution (For Loop) 1. Start 2. Assign Sum = 0 3. Loop: For (I = 2, 1024) 4. Compute Sum = Sum + I 5. Compute I = I * 2 6. Repeat (Goto Step 3) 7. Print Sum 8. Stop
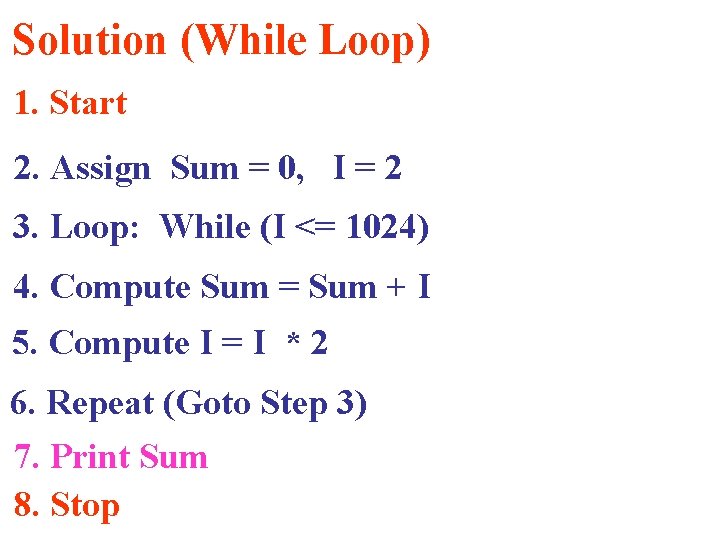
Solution (While Loop) 1. Start 2. Assign Sum = 0, I = 2 3. Loop: While (I <= 1024) 4. Compute Sum = Sum + I 5. Compute I = I * 2 6. Repeat (Goto Step 3) 7. Print Sum 8. Stop
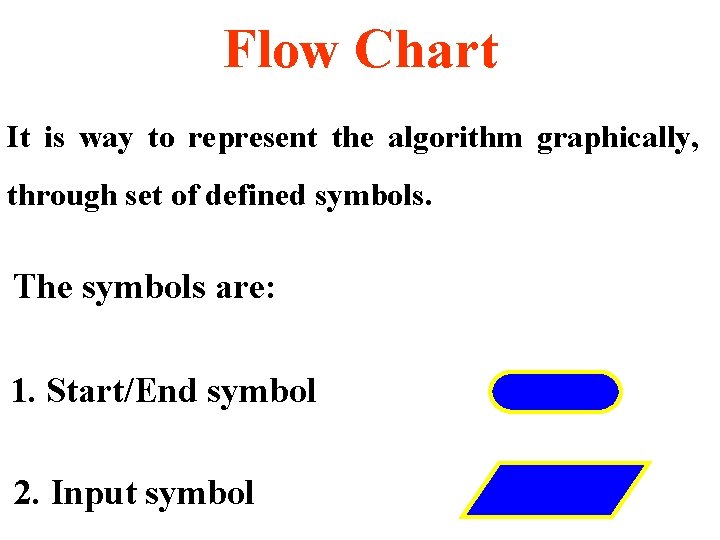
Flow Chart It is way to represent the algorithm graphically, through set of defined symbols. The symbols are: 1. Start/End symbol 2. Input symbol
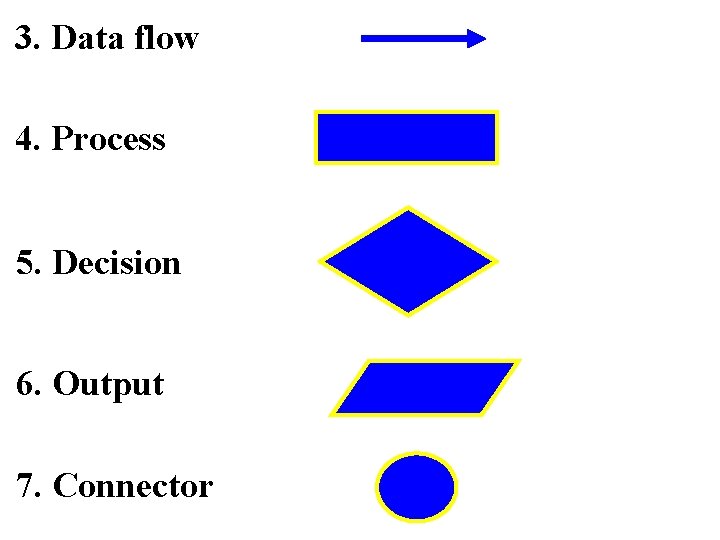
3. Data flow 4. Process 5. Decision 6. Output 7. Connector
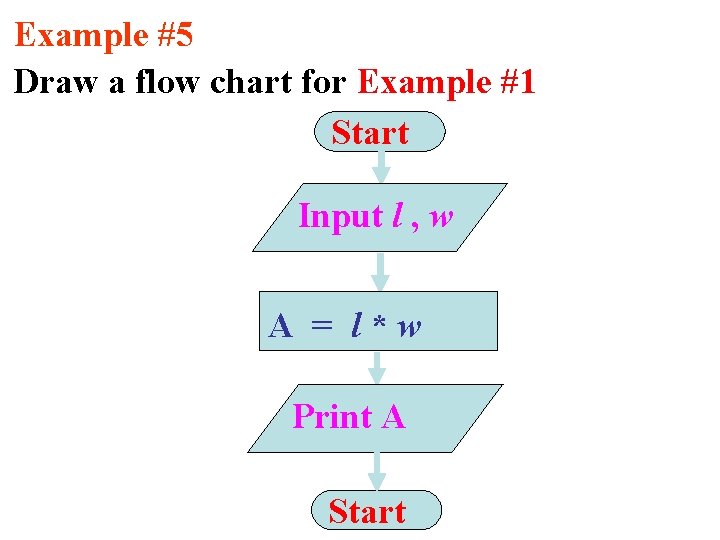
Example #5 Draw a flow chart for Example #1 Start Input l , w A = l*w Print A Start
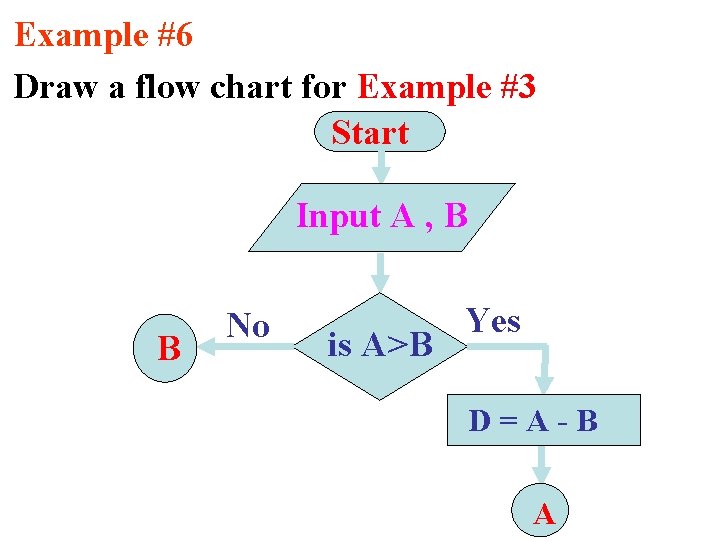
Example #6 Draw a flow chart for Example #3 Start Input A , B B No is A>B Yes D=A-B A
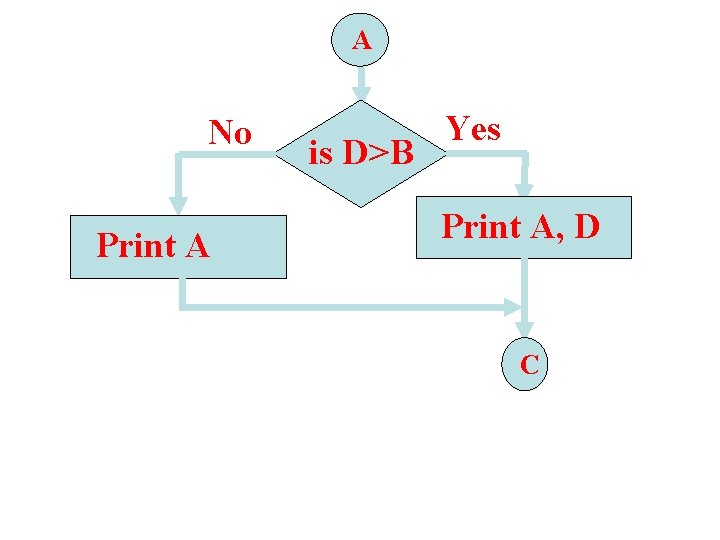
A No Print A is D>B Yes Print A, D C
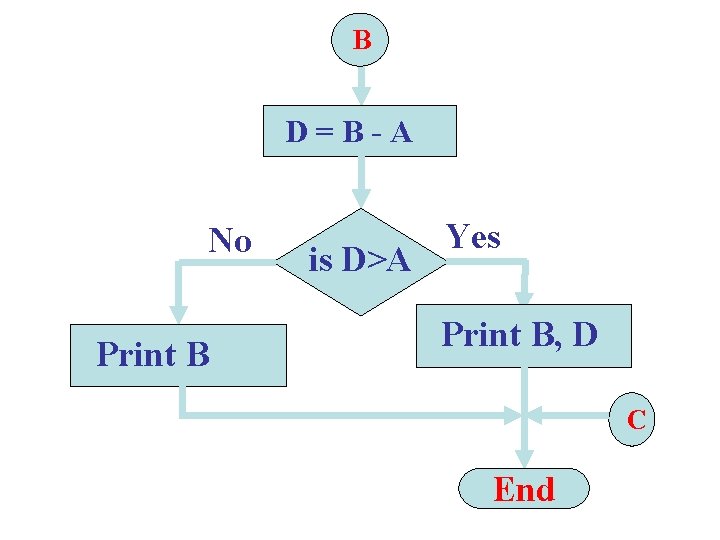
B D=B-A No Print B is D>A Yes Print B, D C End
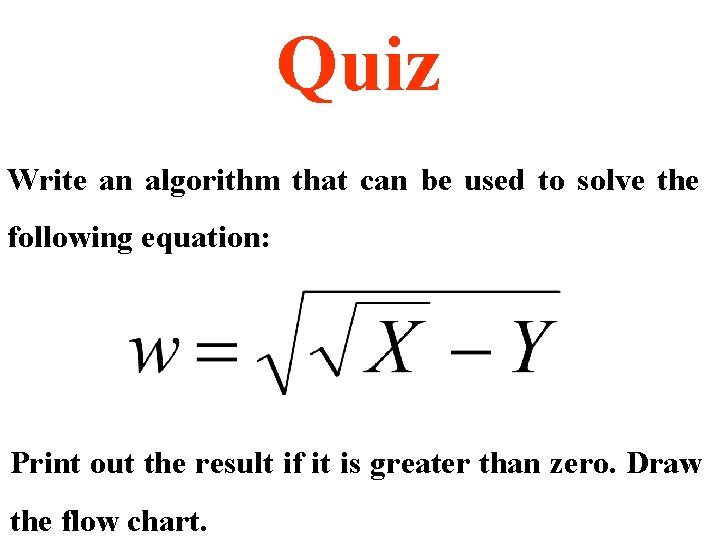
Quiz Write an algorithm that can be used to solve the following equation: Print out the result if it is greater than zero. Draw the flow chart.
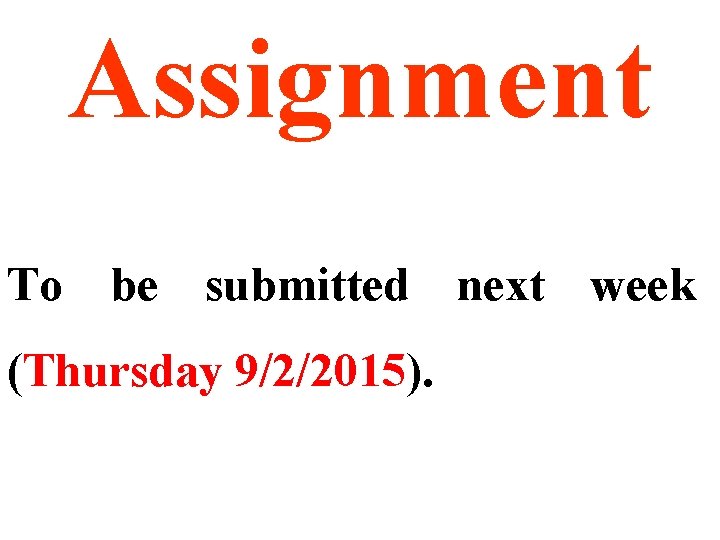
Assignment To be submitted next week (Thursday 9/2/2015).
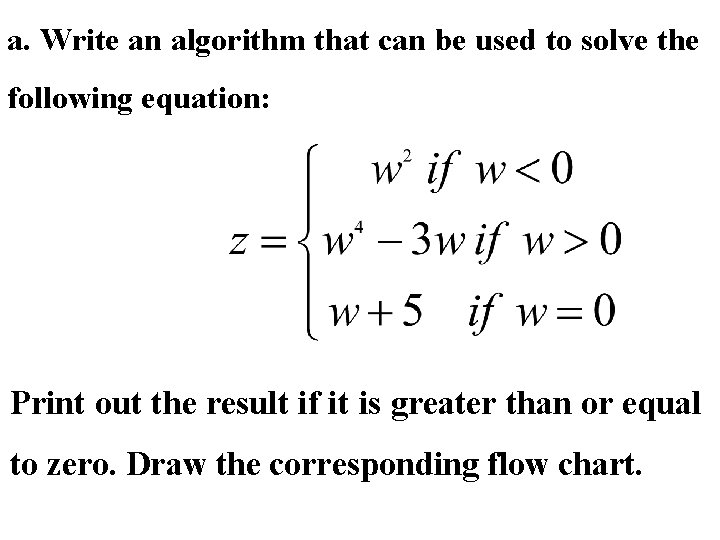
a. Write an algorithm that can be used to solve the following equation: Print out the result if it is greater than or equal to zero. Draw the corresponding flow chart.
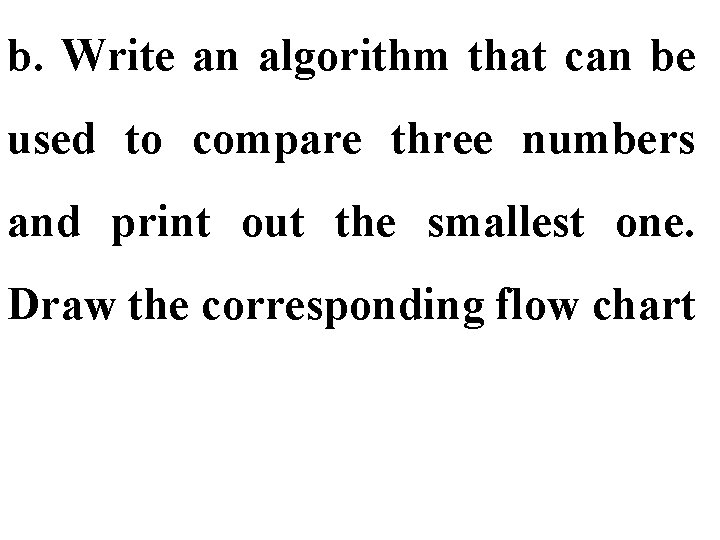
b. Write an algorithm that can be used to compare three numbers and print out the smallest one. Draw the corresponding flow chart
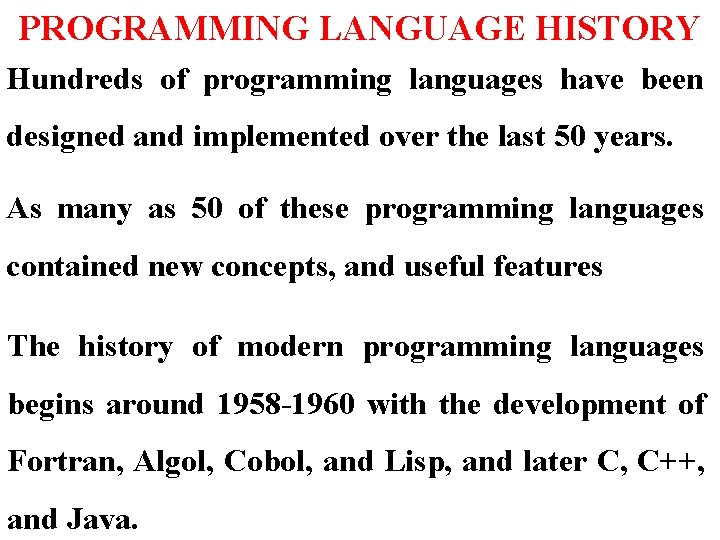
PROGRAMMING LANGUAGE HISTORY Hundreds of programming languages have been designed and implemented over the last 50 years. As many as 50 of these programming languages contained new concepts, and useful features The history of modern programming languages begins around 1958 -1960 with the development of Fortran, Algol, Cobol, and Lisp, and later C, C++, and Java.
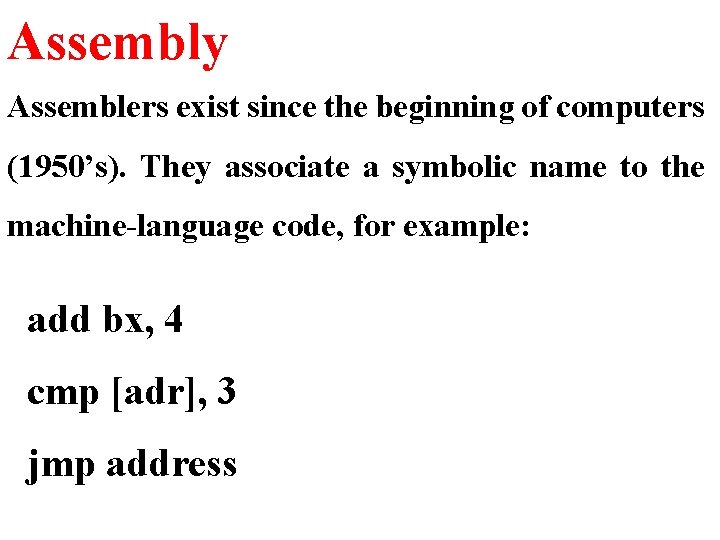
Assembly Assemblers exist since the beginning of computers (1950’s). They associate a symbolic name to the machine-language code, for example: add bx, 4 cmp [adr], 3 jmp address
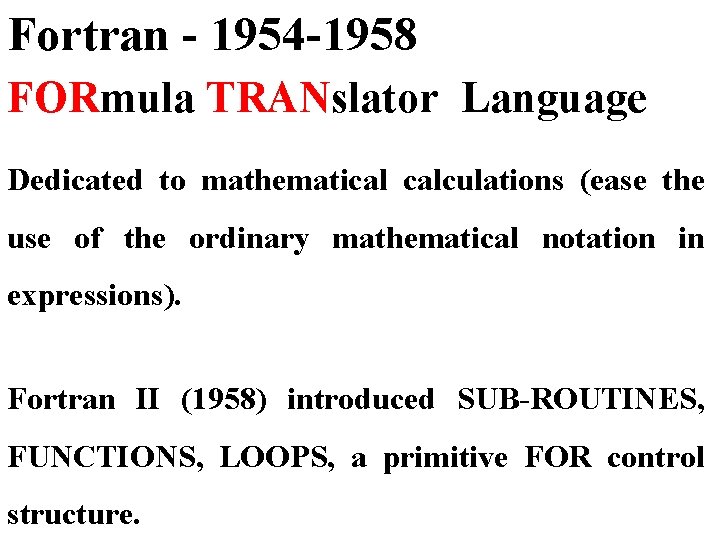
Fortran - 1954 -1958 FORmula TRANslator Language Dedicated to mathematical calculations (ease the use of the ordinary mathematical notation in expressions). Fortran II (1958) introduced SUB-ROUTINES, FUNCTIONS, LOOPS, a primitive FOR control structure.
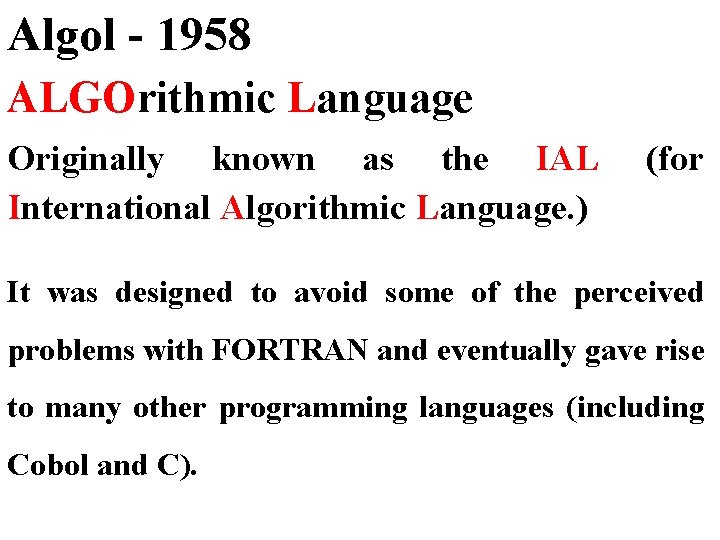
Algol - 1958 ALGOrithmic Language Originally known as the IAL International Algorithmic Language. ) (for It was designed to avoid some of the perceived problems with FORTRAN and eventually gave rise to many other programming languages (including Cobol and C).
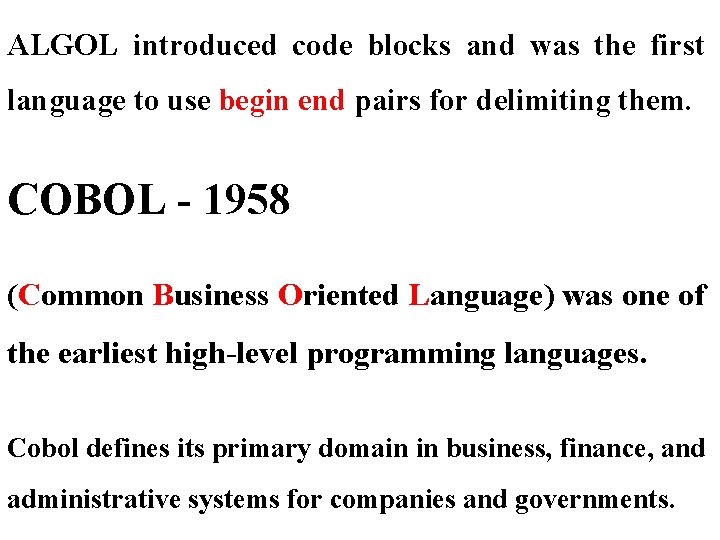
ALGOL introduced code blocks and was the first language to use begin end pairs for delimiting them. COBOL - 1958 (Common Business Oriented Language) was one of the earliest high-level programming languages. Cobol defines its primary domain in business, finance, and administrative systems for companies and governments.
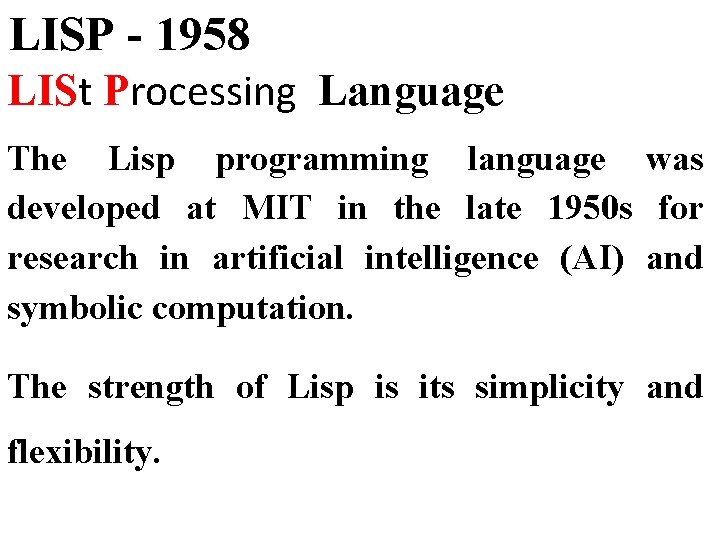
LISP - 1958 LISt Processing Language The Lisp programming language was developed at MIT in the late 1950 s for research in artificial intelligence (AI) and symbolic computation. The strength of Lisp is its simplicity and flexibility.
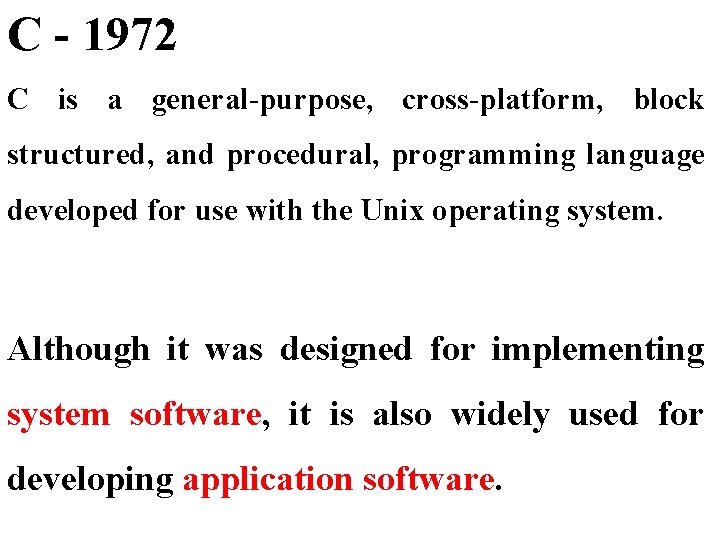
C - 1972 C is a general-purpose, cross-platform, block structured, and procedural, programming language developed for use with the Unix operating system. Although it was designed for implementing system software, it is also widely used for developing application software.
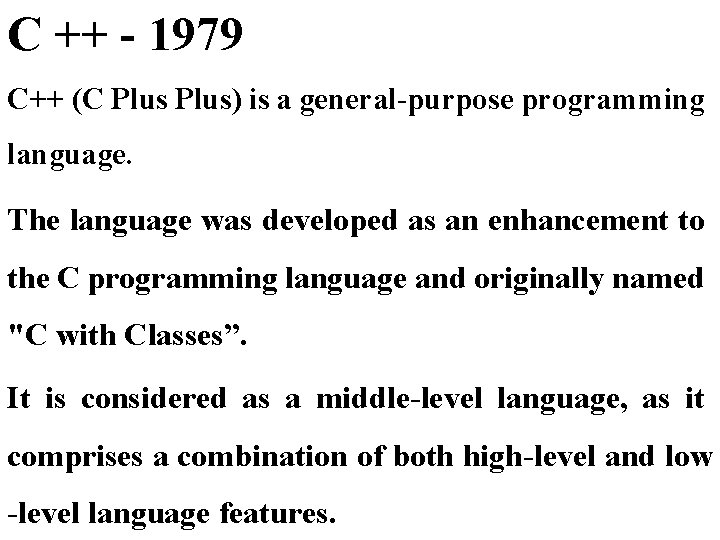
C ++ - 1979 C++ (C Plus) is a general-purpose programming language. The language was developed as an enhancement to the C programming language and originally named "C with Classes”. It is considered as a middle-level language, as it comprises a combination of both high-level and low -level language features.
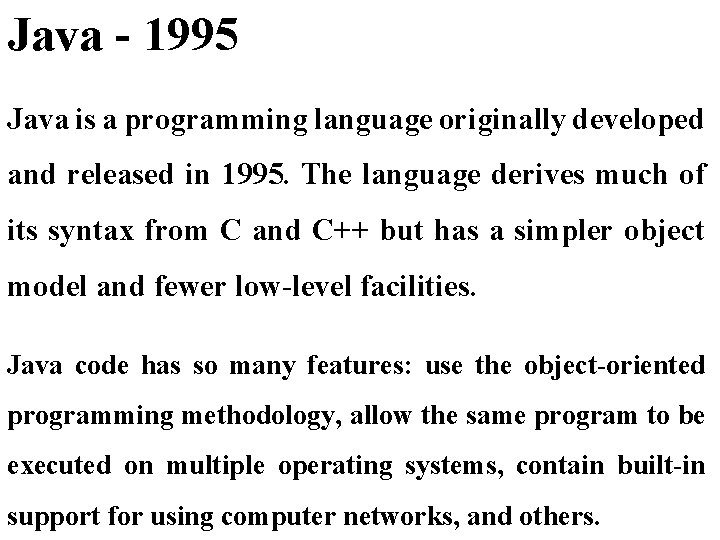
Java - 1995 Java is a programming language originally developed and released in 1995. The language derives much of its syntax from C and C++ but has a simpler object model and fewer low-level facilities. Java code has so many features: use the object-oriented programming methodology, allow the same program to be executed on multiple operating systems, contain built-in support for using computer networks, and others.
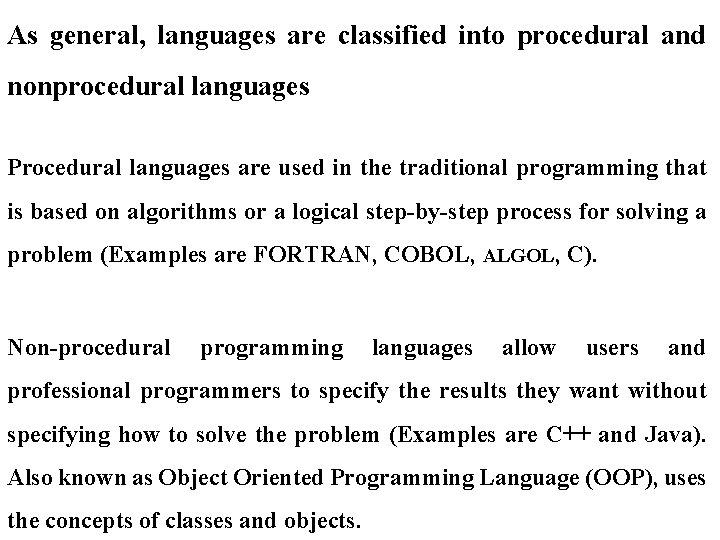
As general, languages are classified into procedural and nonprocedural languages Procedural languages are used in the traditional programming that is based on algorithms or a logical step-by-step process for solving a problem (Examples are FORTRAN, COBOL, ALGOL, C). Non-procedural programming languages allow users and professional programmers to specify the results they want without specifying how to solve the problem (Examples are C++ and Java). Also known as Object Oriented Programming Language (OOP), uses the concepts of classes and objects.
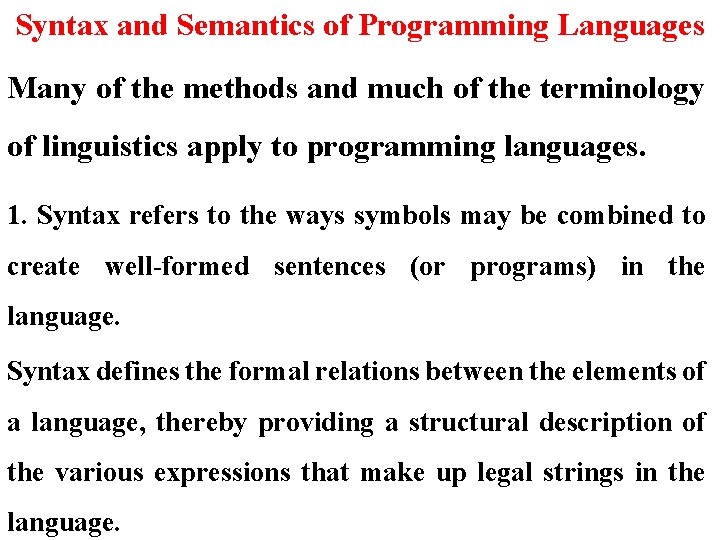
Syntax and Semantics of Programming Languages Many of the methods and much of the terminology of linguistics apply to programming languages. 1. Syntax refers to the ways symbols may be combined to create well-formed sentences (or programs) in the language. Syntax defines the formal relations between the elements of a language, thereby providing a structural description of the various expressions that make up legal strings in the language.
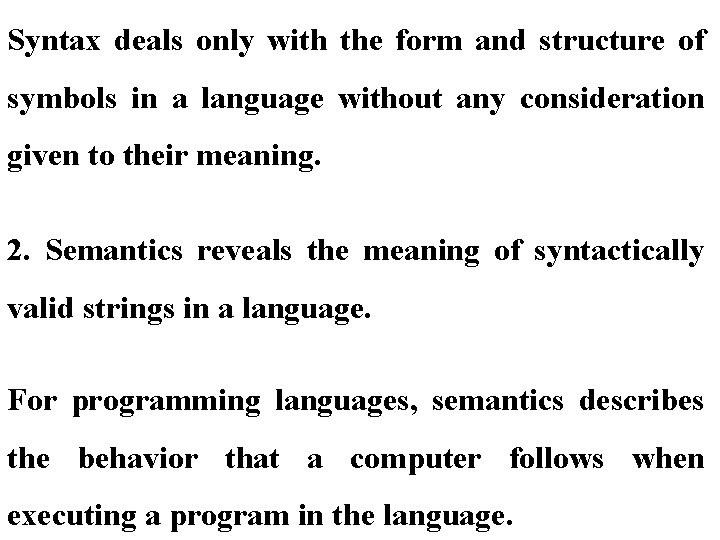
Syntax deals only with the form and structure of symbols in a language without any consideration given to their meaning. 2. Semantics reveals the meaning of syntactically valid strings in a language. For programming languages, semantics describes the behavior that a computer follows when executing a program in the language.
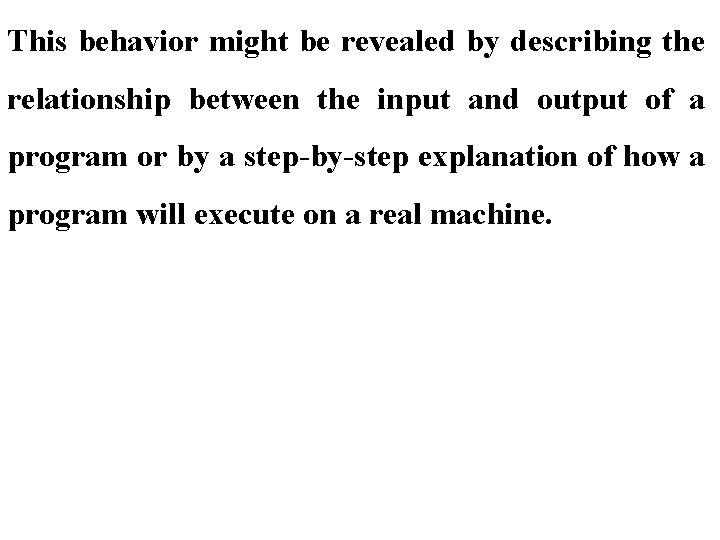
This behavior might be revealed by describing the relationship between the input and output of a program or by a step-by-step explanation of how a program will execute on a real machine.
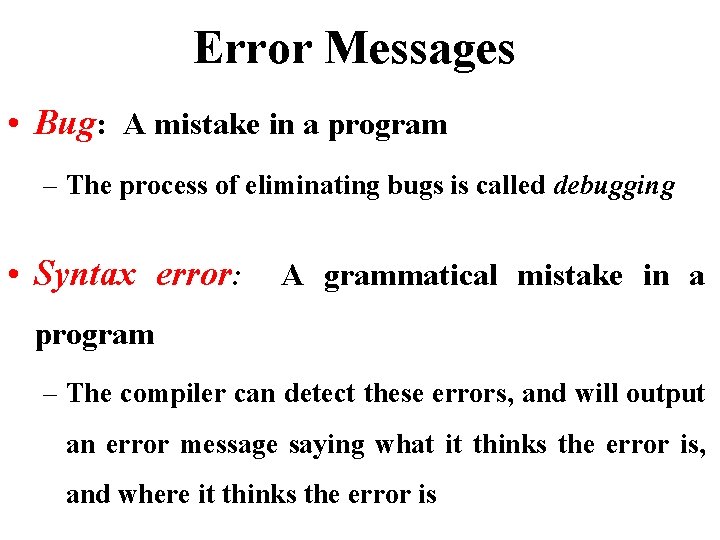
Error Messages • Bug: A mistake in a program – The process of eliminating bugs is called debugging • Syntax error: A grammatical mistake in a program – The compiler can detect these errors, and will output an error message saying what it thinks the error is, and where it thinks the error is
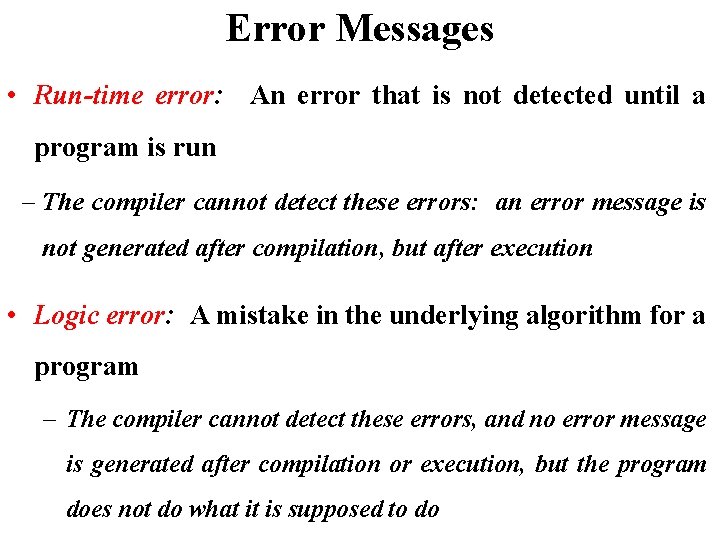
Error Messages • Run-time error: An error that is not detected until a program is run – The compiler cannot detect these errors: an error message is not generated after compilation, but after execution • Logic error: A mistake in the underlying algorithm for a program – The compiler cannot detect these errors, and no error message is generated after compilation or execution, but the program does not do what it is supposed to do
Ahmed muhudiin ahmed
Ali ahmed is a mathematics professor who tries to involve
Ahmed ali siddiqui
Ali is taller than ahmed
Mahmoud abdelfattah age
Passport mahmoud darwish analysis
Dr mahmoud salman
Mahmoud arafa
Boudarene mahmoud
Mahmoud sarmini md
Adp antagonist drugs
Mahmoud khattab md
Hana mahmoud
Human genetics concepts and applications 10th edition
Motivation from concept to application
Starr evers starr biology concepts and applications
Language
Chapter 8 linear programming applications solutions
Concepts, techniques and models of computer programming
Linear programming definition
Reasons for studying concepts of programming languages
Scratch programming concepts
Scratch programming concepts
Construction of cathode ray oscilloscope
The fourier transform and its applications
Daniel spielman spectral graph theory
Spectral graph theory and its applications
Diagonalizable matrix
Dimensional analysis and its applications
Zener diode and its application
Fast random walk with restart and its applications
Slater's rule
Cstnet
Basics of client server model and its applications
Kohlrausch law
Perbedaan linear programming dan integer programming
Greedy vs dynamic programming
Components of system programming
Linear vs integer programming
Perbedaan linear programming dan integer programming
The emigree poem analysis
When a train increases its velocity, its momentum
Weather sunny cloudy rainy windy
If its a square it's a sonnet summary
Its halloween its halloween the moon is full and bright
Its not easy but its worth it
Risala asbab-i-baghawat-i-hind
Uv khayyam
Adel ahmed alalla
United indian patriotic association in hindi
Ahmed salman rushdie
Ahmed salemi
Humbaracı ahmed paşa
Dr mohammed ahmed
Saad ahmed javed
Ahmed mustapha