Programming Language Concepts Its Applications Lecture 4 Computer
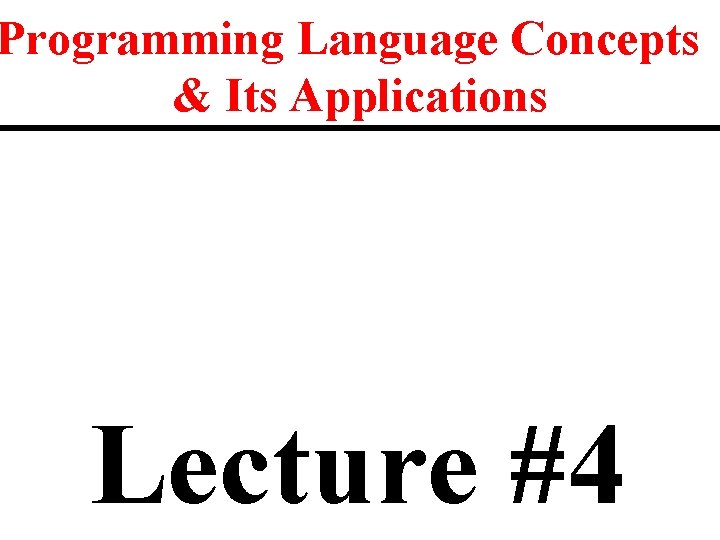
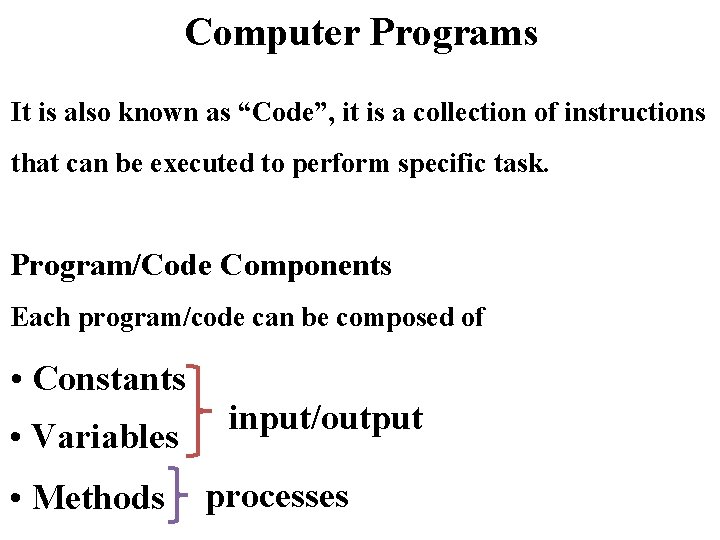
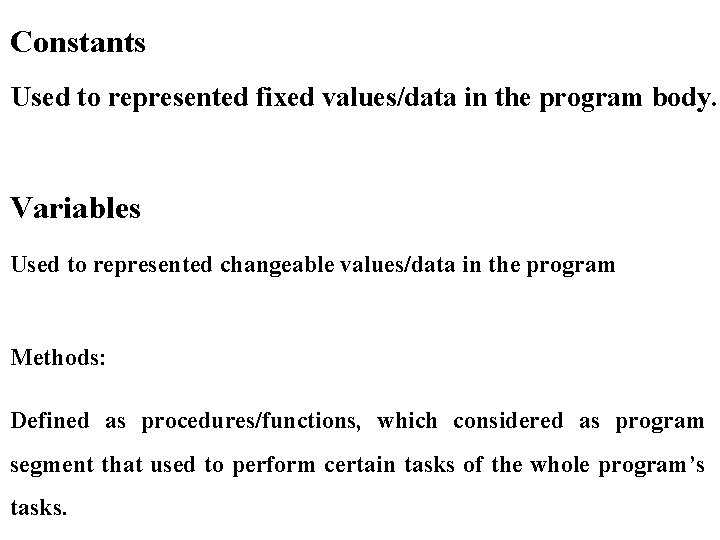
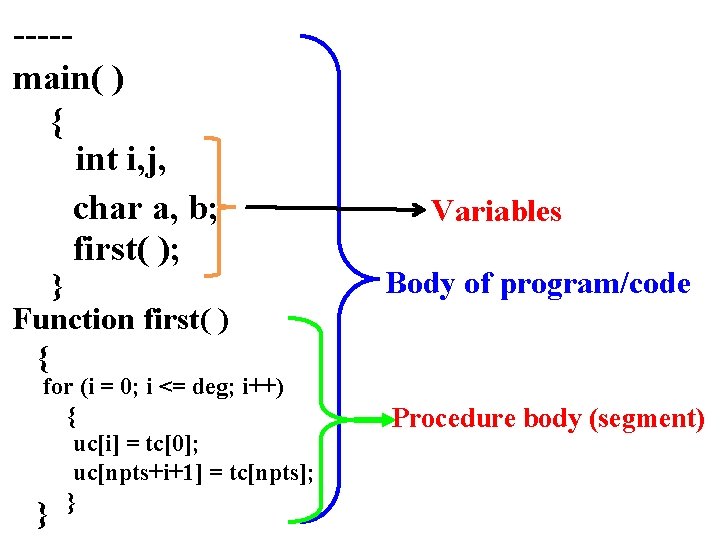
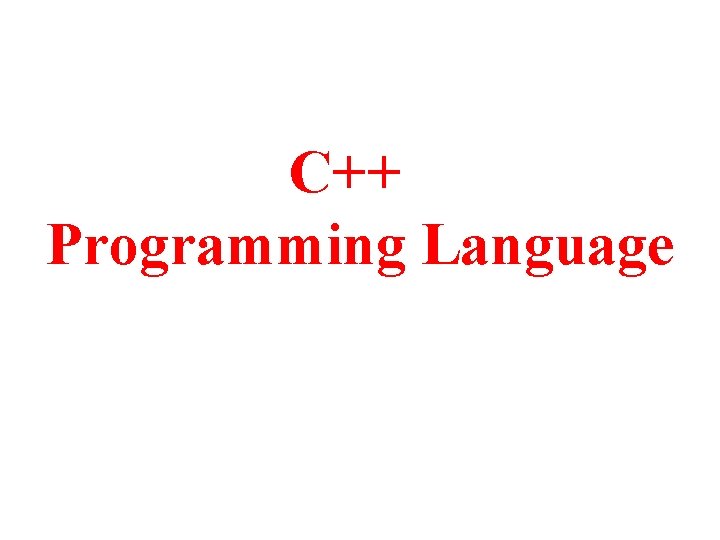
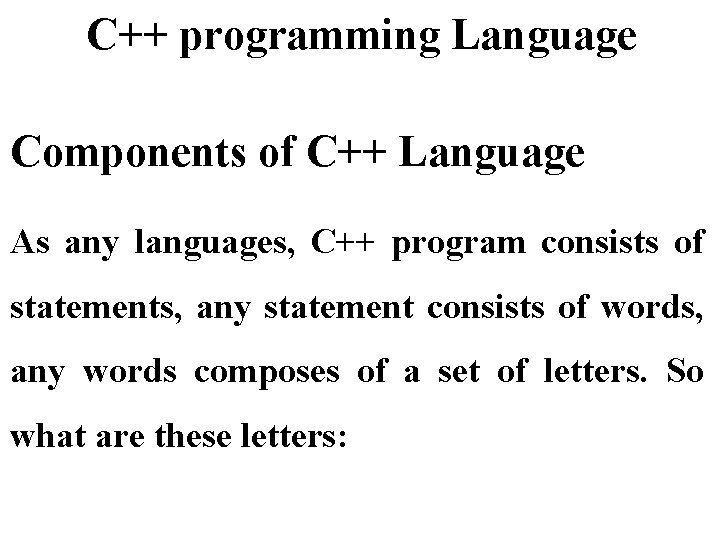
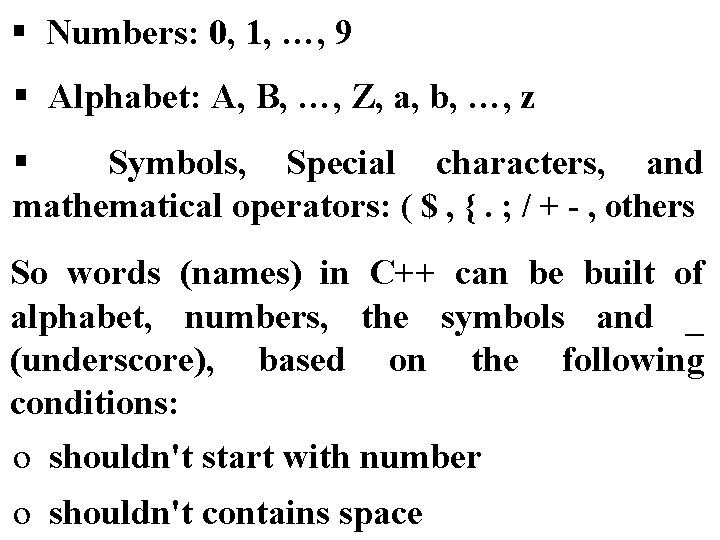
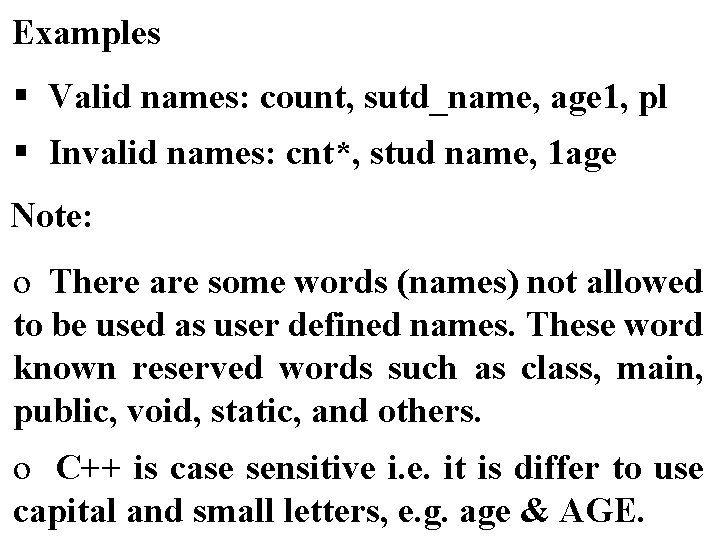
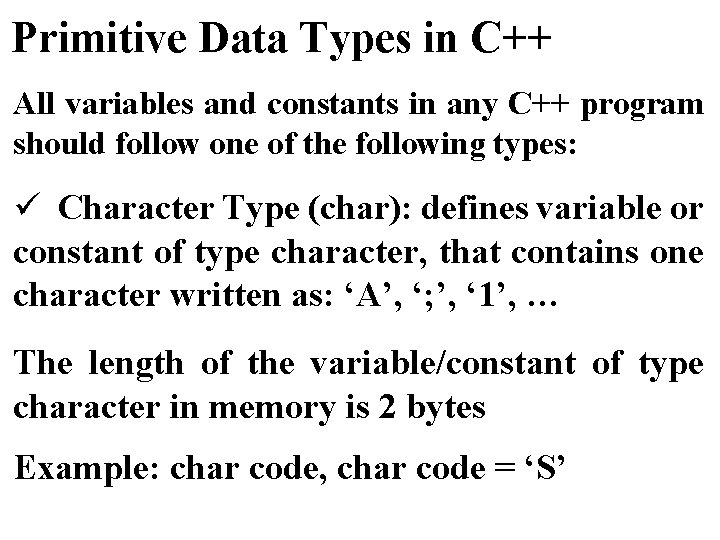
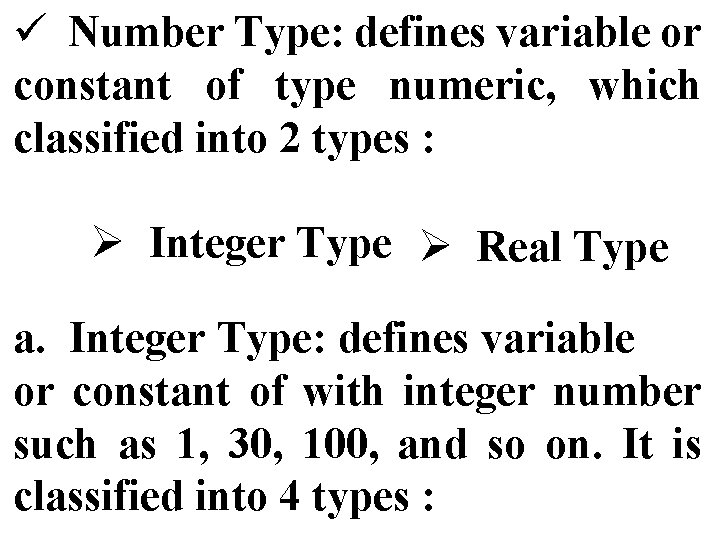
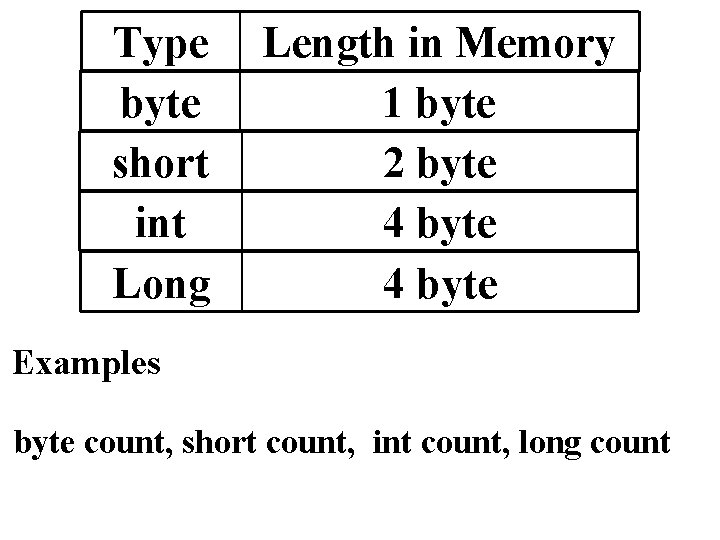
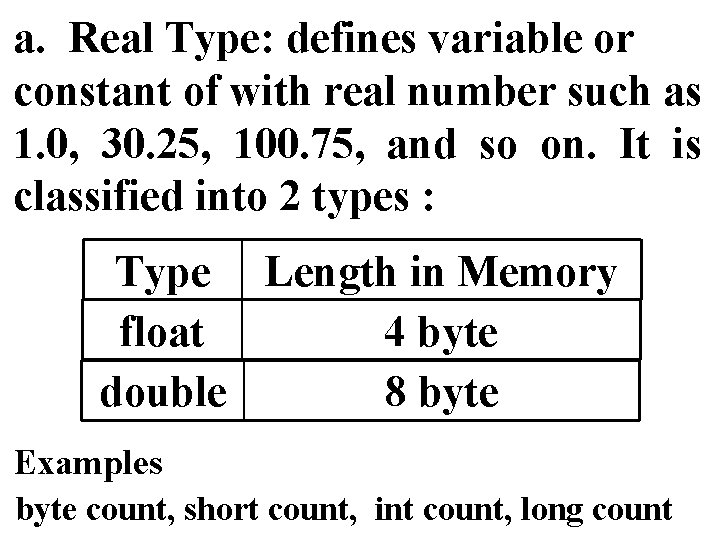
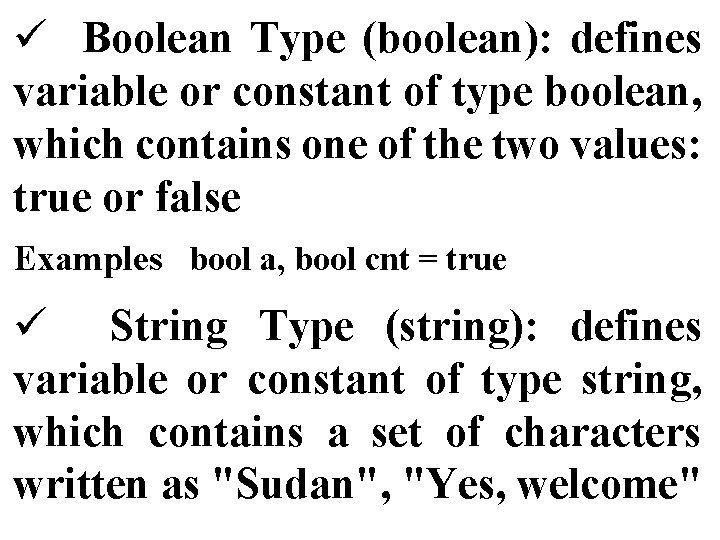
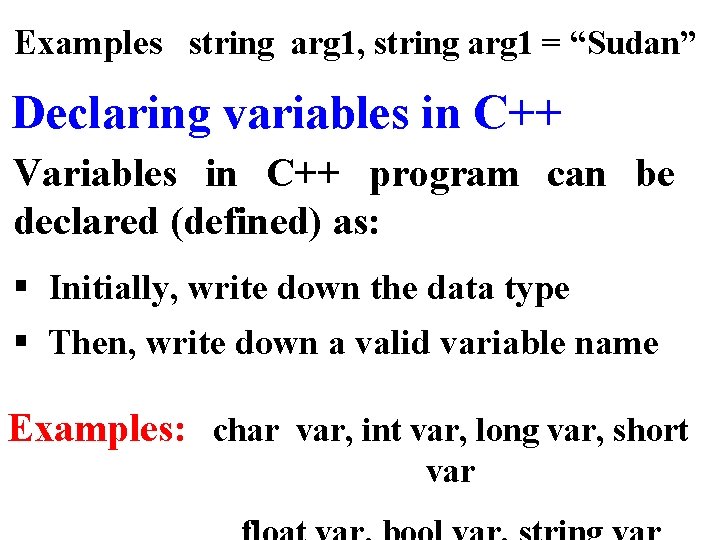
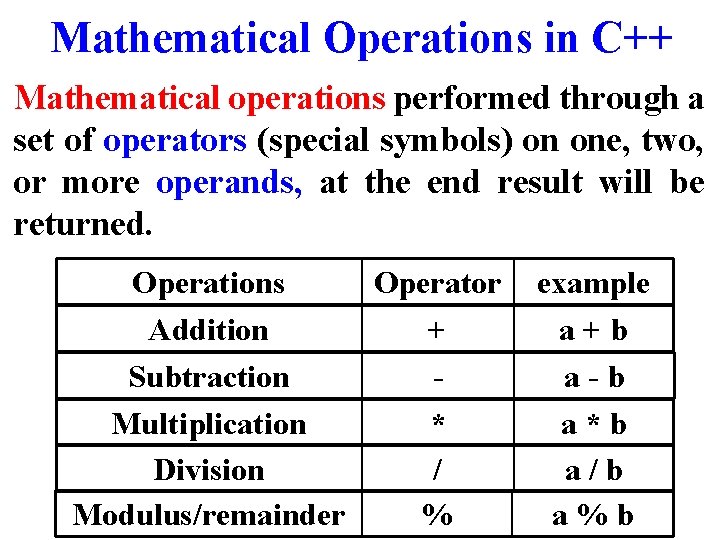
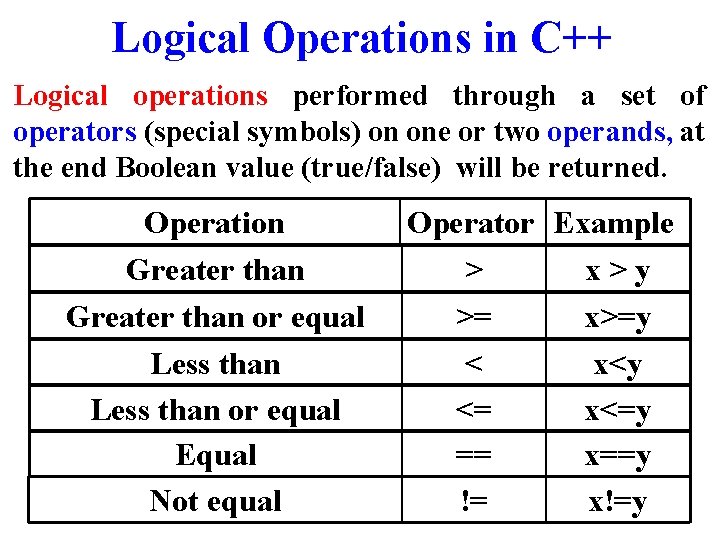
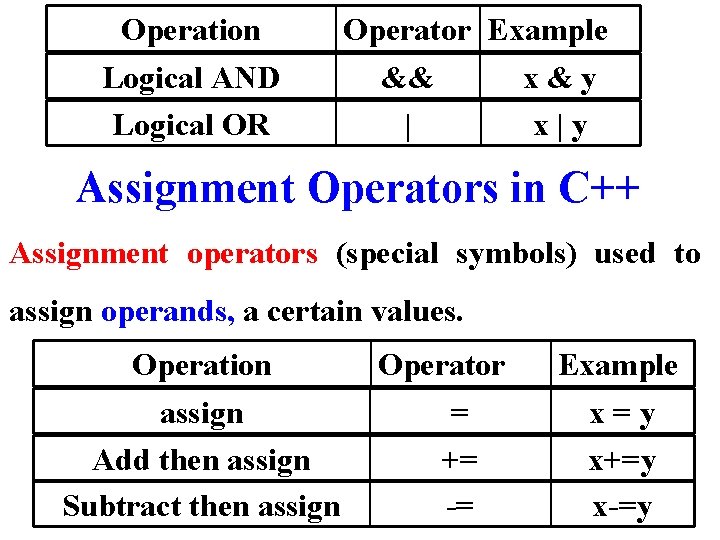
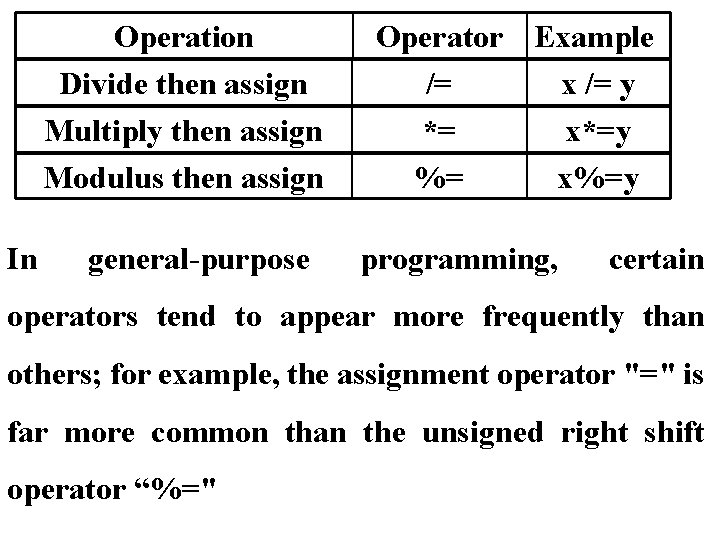
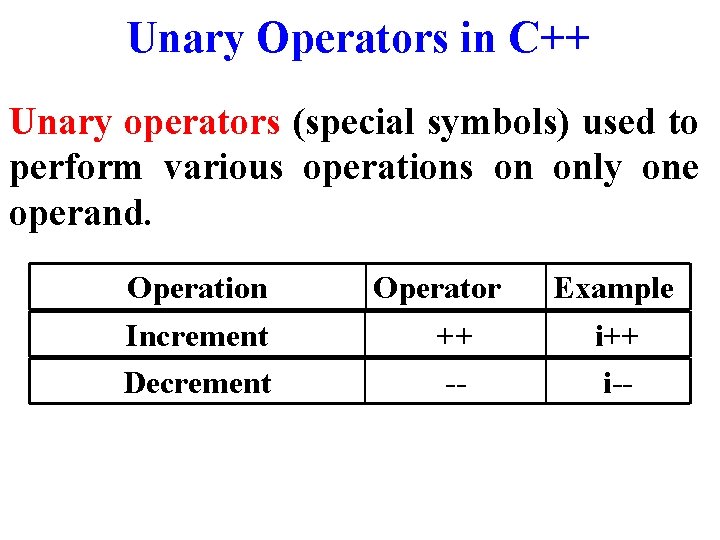
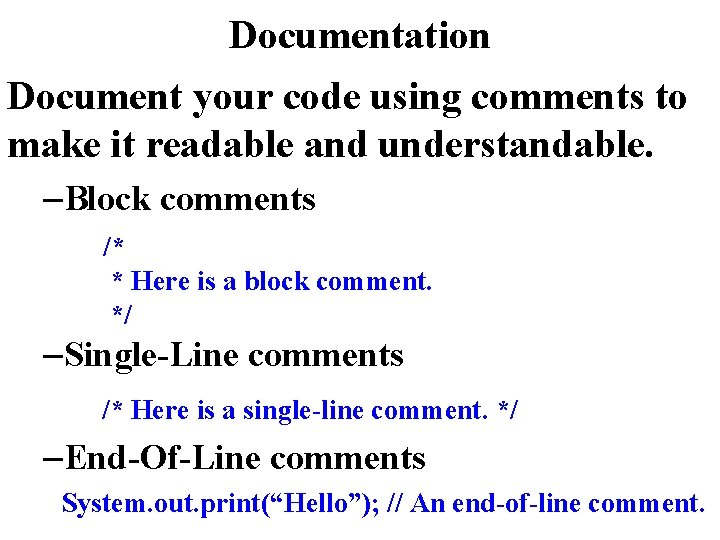
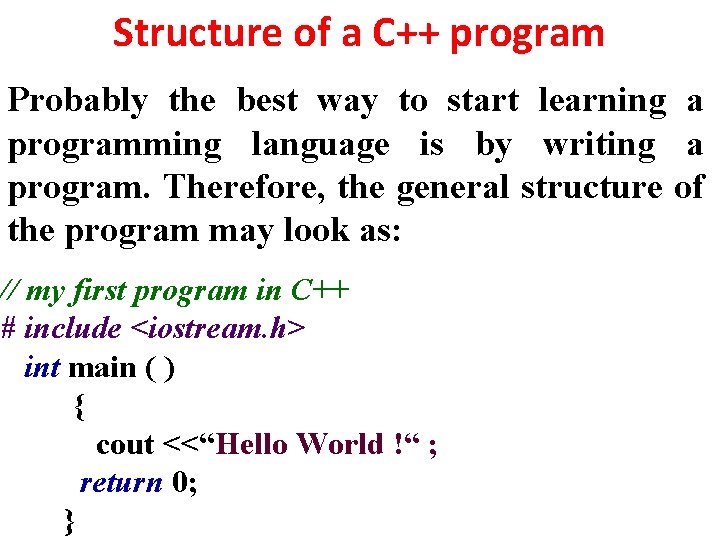
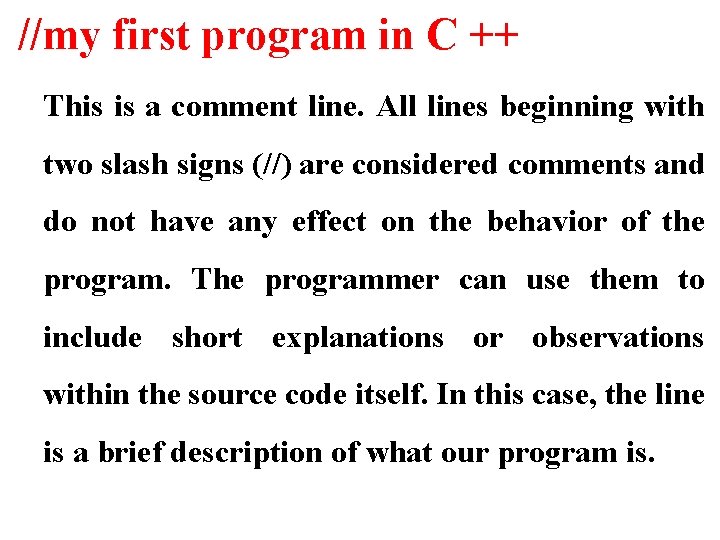
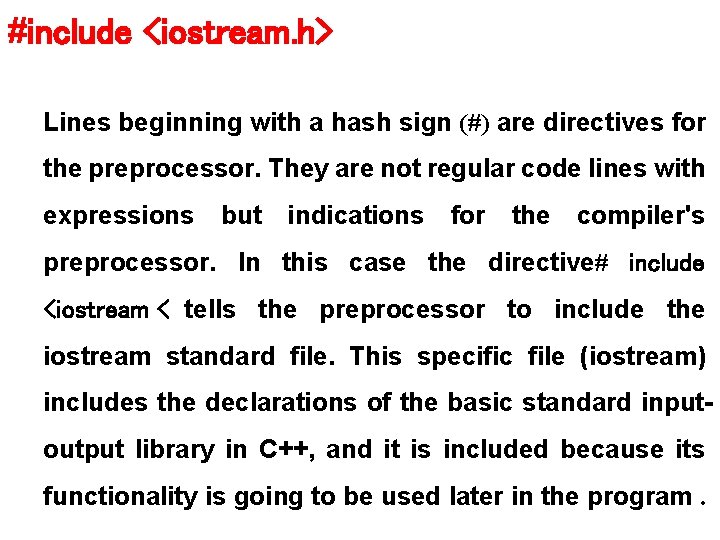
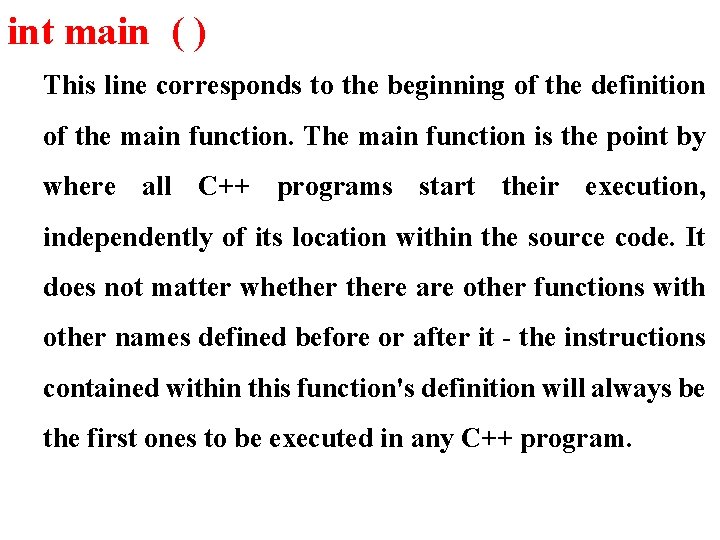
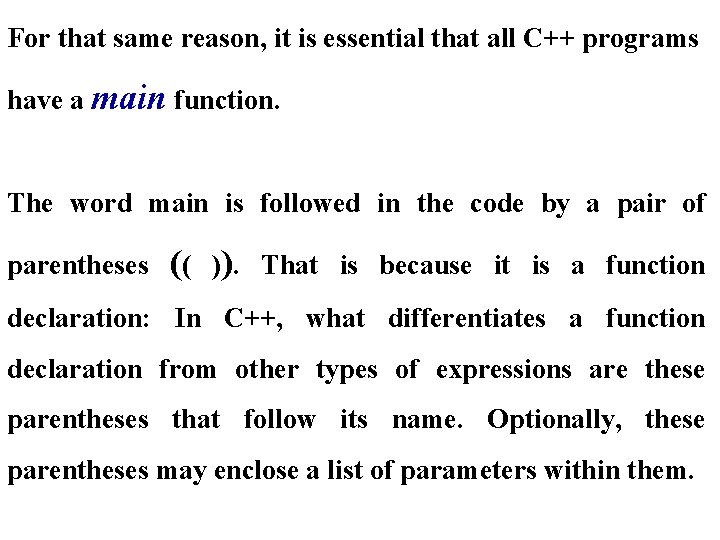
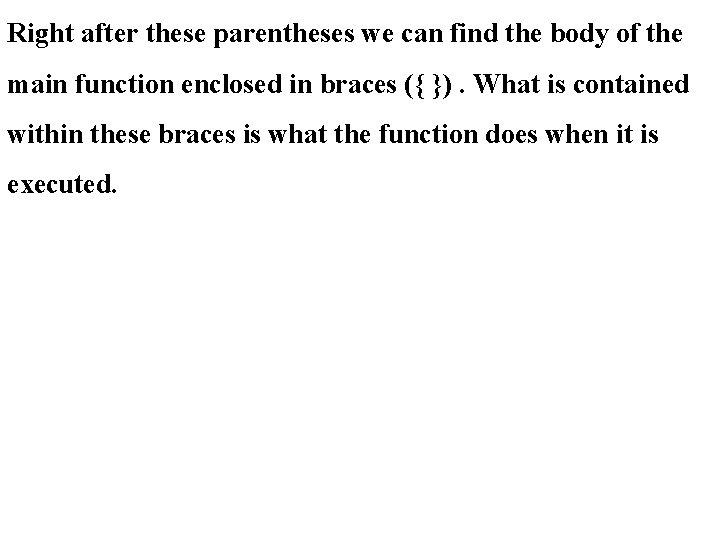
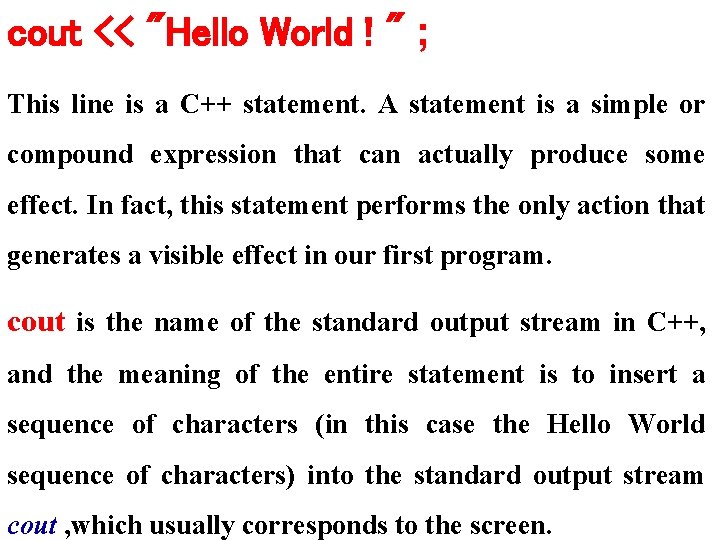
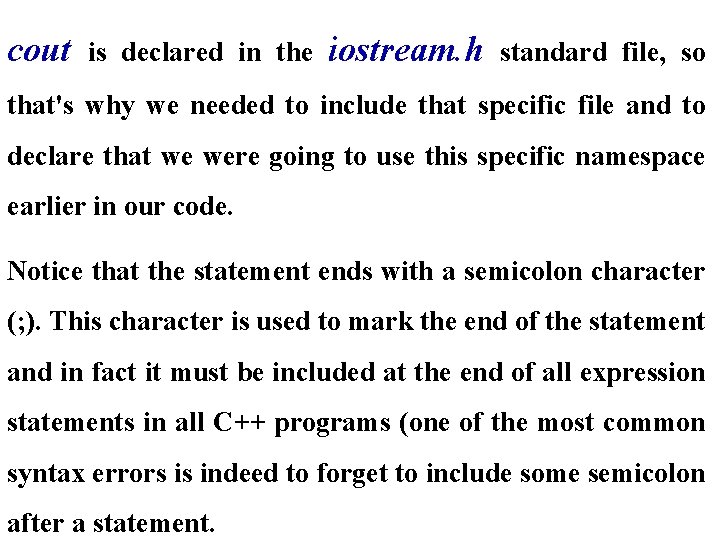
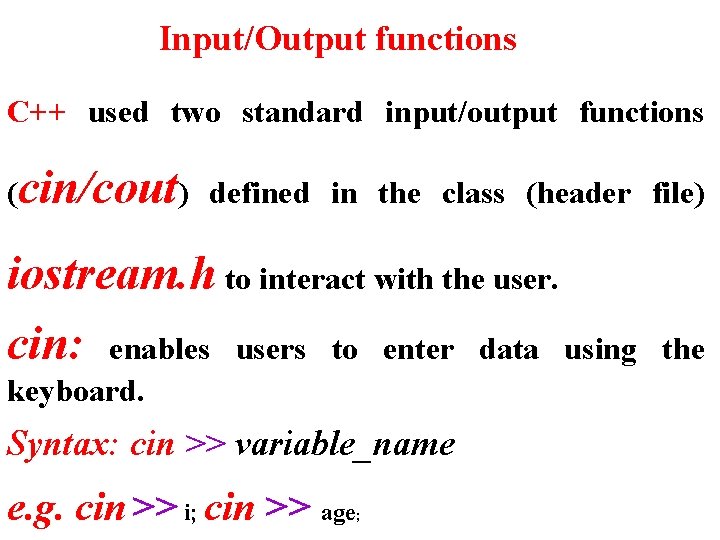
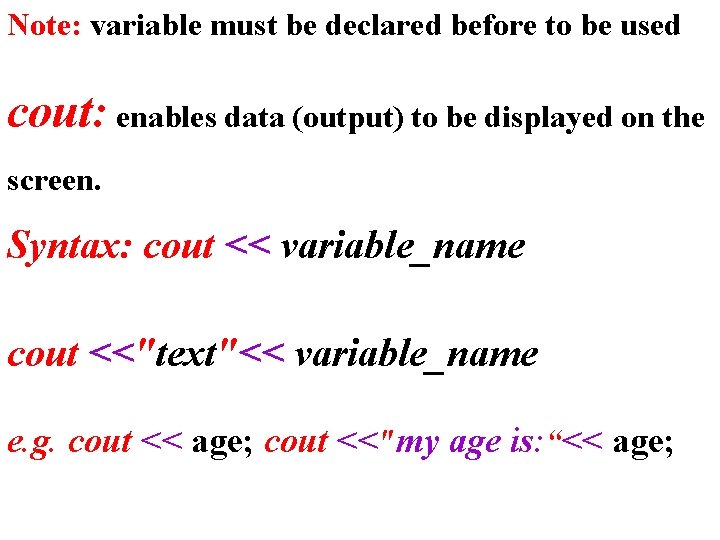
- Slides: 30
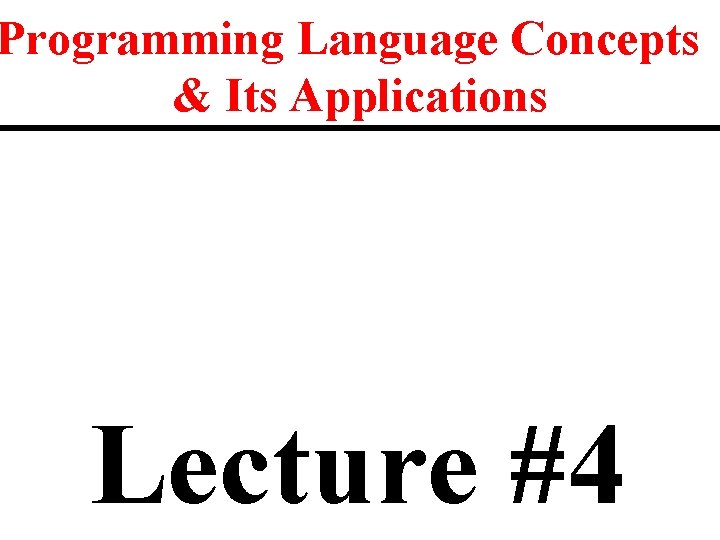
Programming Language Concepts & Its Applications Lecture #4
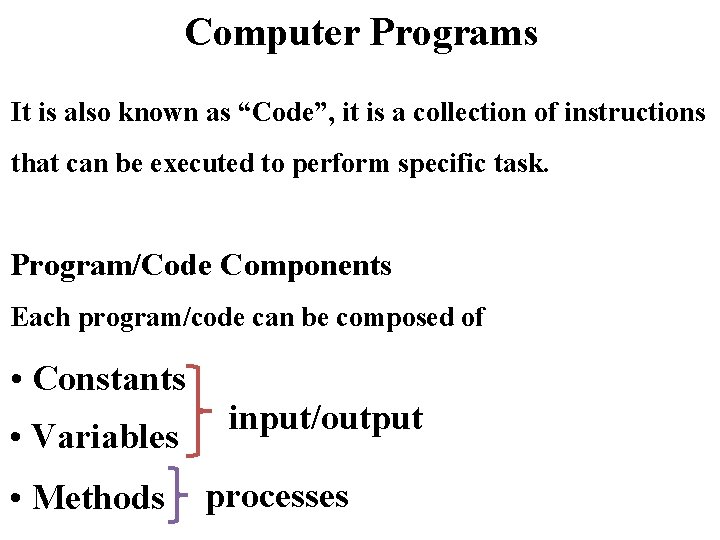
Computer Programs It is also known as “Code”, it is a collection of instructions that can be executed to perform specific task. Program/Code Components Each program/code can be composed of • Constants • Variables • Methods input/output processes
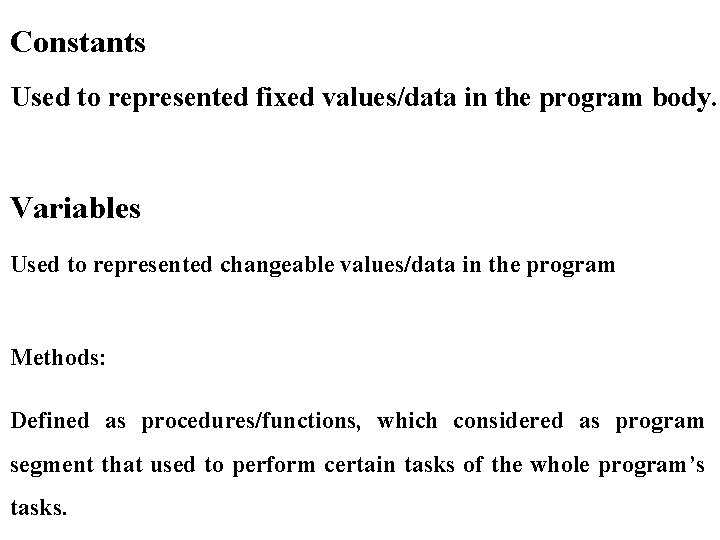
Constants Used to represented fixed values/data in the program body. Variables Used to represented changeable values/data in the program Methods: Defined as procedures/functions, which considered as program segment that used to perform certain tasks of the whole program’s tasks.
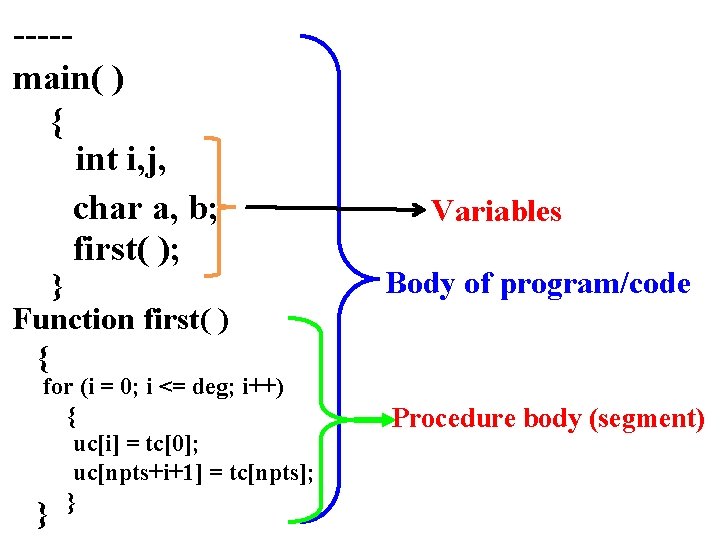
----main( ) { int i, j, char a, b; first( ); } Function first( ) { for (i = 0; i <= deg; i++) { uc[i] = tc[0]; uc[npts+i+1] = tc[npts]; } } Variables Body of program/code Procedure body (segment)
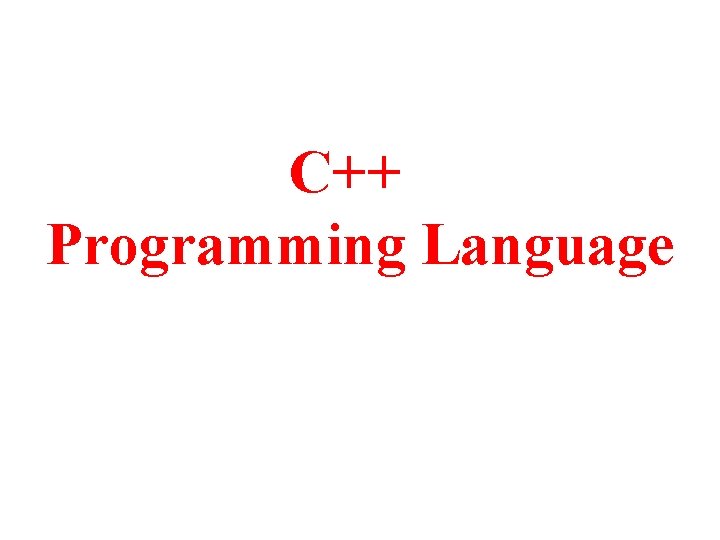
C++ Programming Language
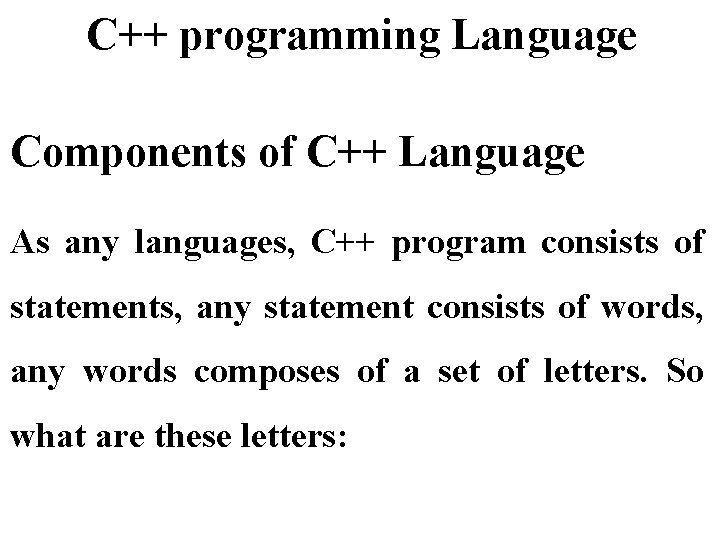
C++ programming Language Components of C++ Language As any languages, C++ program consists of statements, any statement consists of words, any words composes of a set of letters. So what are these letters:
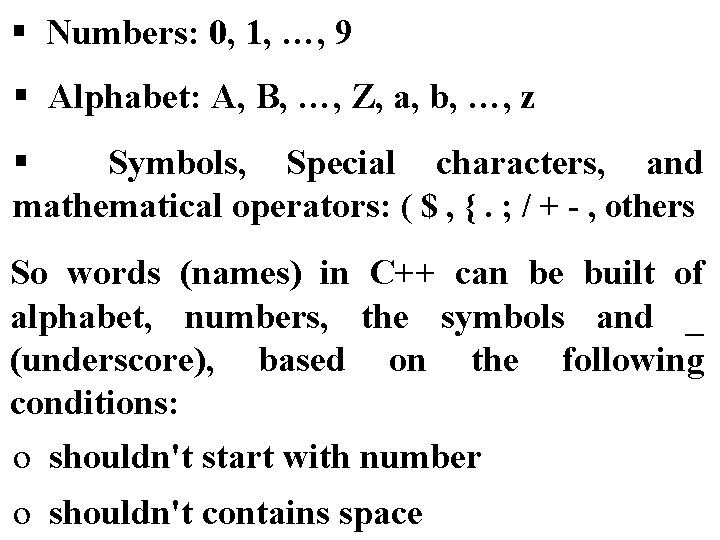
§ Numbers: 0, 1, …, 9 § Alphabet: A, B, …, Z, a, b, …, z § Symbols, Special characters, and mathematical operators: ( $ , {. ; / + - , others So words (names) in C++ can be built of alphabet, numbers, the symbols and _ (underscore), based on the following conditions: o shouldn't start with number o shouldn't contains space
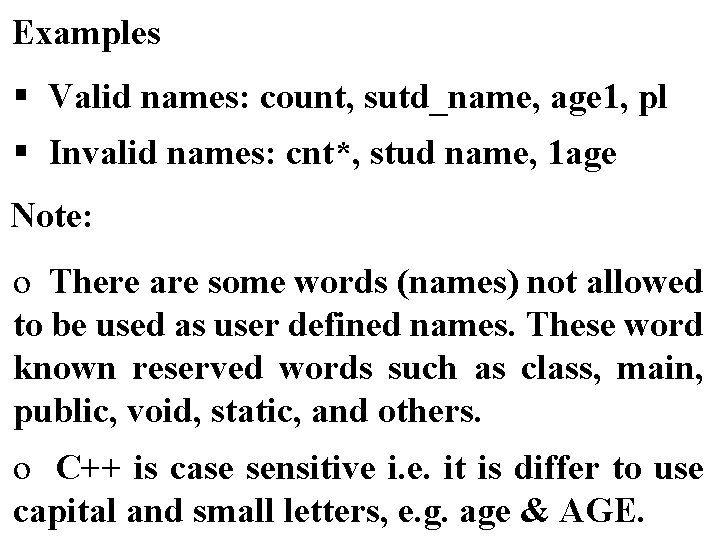
Examples § Valid names: count, sutd_name, age 1, pl § Invalid names: cnt*, stud name, 1 age Note: o There are some words (names) not allowed to be used as user defined names. These word known reserved words such as class, main, public, void, static, and others. o C++ is case sensitive i. e. it is differ to use capital and small letters, e. g. age & AGE.
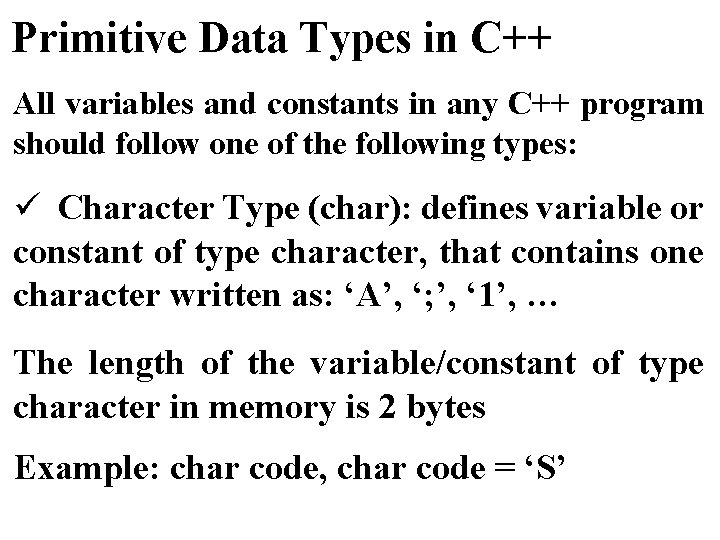
Primitive Data Types in C++ All variables and constants in any C++ program should follow one of the following types: ü Character Type (char): defines variable or constant of type character, that contains one character written as: ‘A’, ‘; ’, ‘ 1’, … The length of the variable/constant of type character in memory is 2 bytes Example: char code, char code = ‘S’
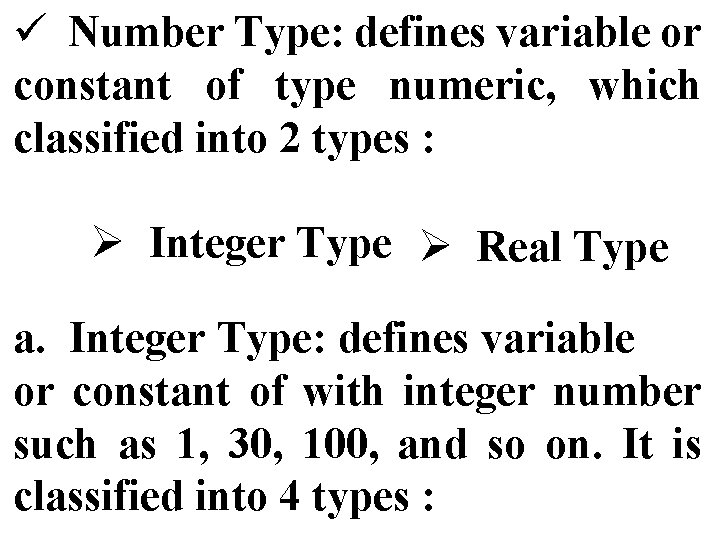
ü Number Type: defines variable or constant of type numeric, which classified into 2 types : Ø Integer Type Ø Real Type a. Integer Type: defines variable or constant of with integer number such as 1, 30, 100, and so on. It is classified into 4 types :
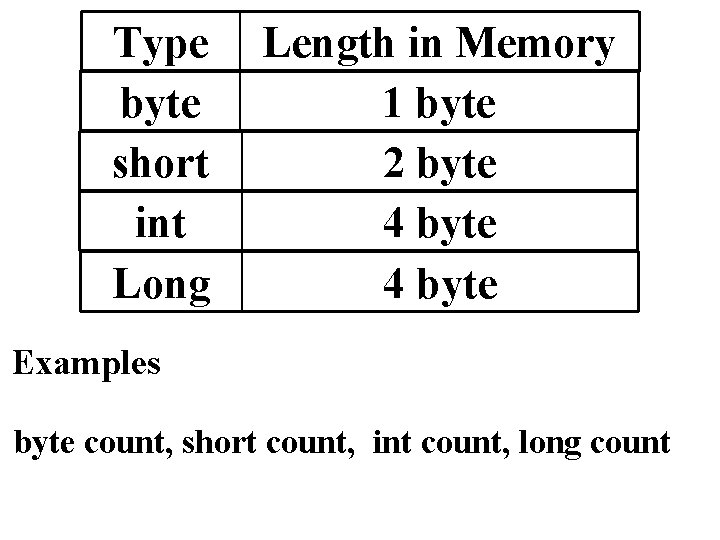
Type byte short int Long Length in Memory 1 byte 2 byte 4 byte Examples byte count, short count, int count, long count
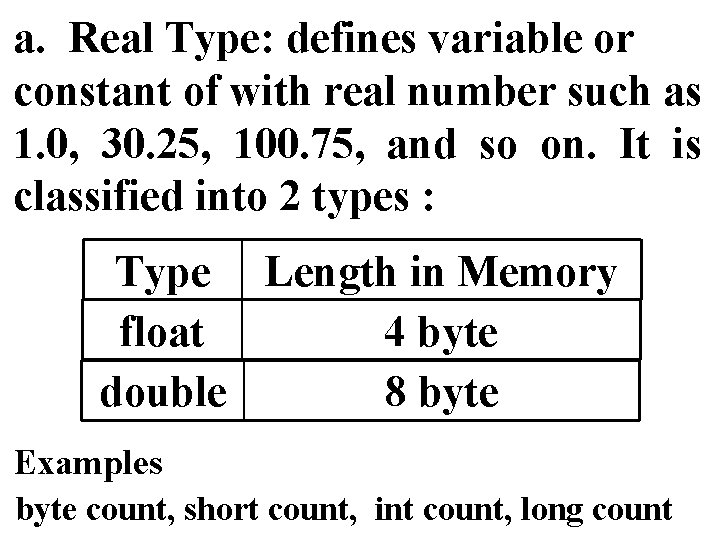
a. Real Type: defines variable or constant of with real number such as 1. 0, 30. 25, 100. 75, and so on. It is classified into 2 types : Type Length in Memory float 4 byte double 8 byte Examples byte count, short count, int count, long count
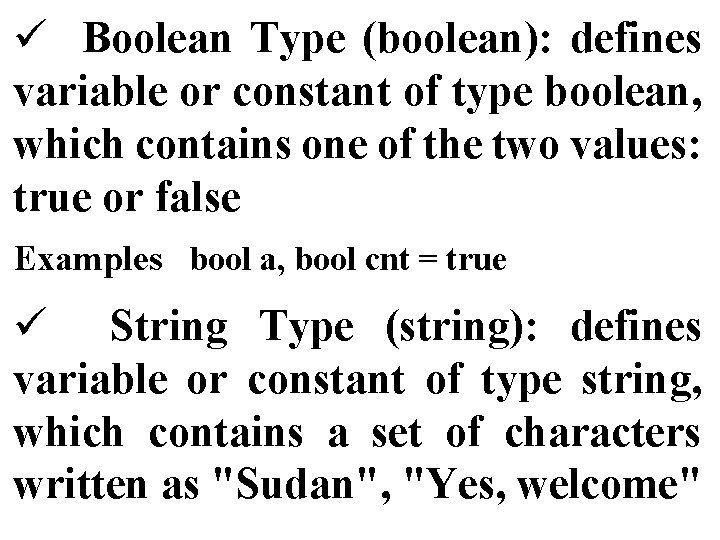
ü Boolean Type (boolean): defines variable or constant of type boolean, which contains one of the two values: true or false Examples bool a, bool cnt = true ü String Type (string): defines variable or constant of type string, which contains a set of characters written as "Sudan", "Yes, welcome"
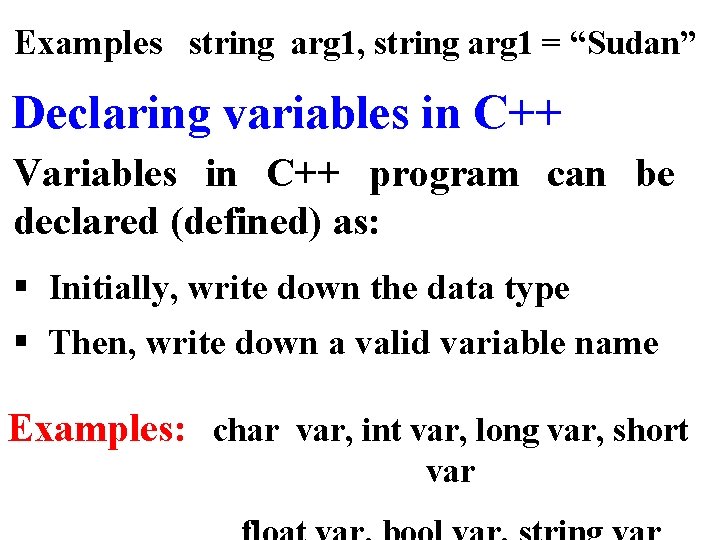
Examples string arg 1, string arg 1 = “Sudan” Declaring variables in C++ Variables in C++ program can be declared (defined) as: § Initially, write down the data type § Then, write down a valid variable name Examples: char var, int var, long var, short var
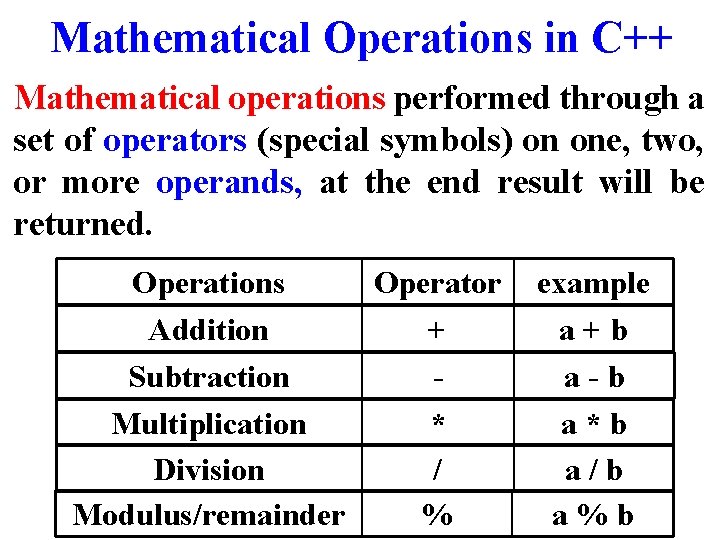
Mathematical Operations in C++ Mathematical operations performed through a set of operators (special symbols) on one, two, or more operands, at the end result will be returned. Operations Operator Addition + Subtraction Multiplication * Division / Modulus/remainder % example a+b a-b a*b a/b a%b
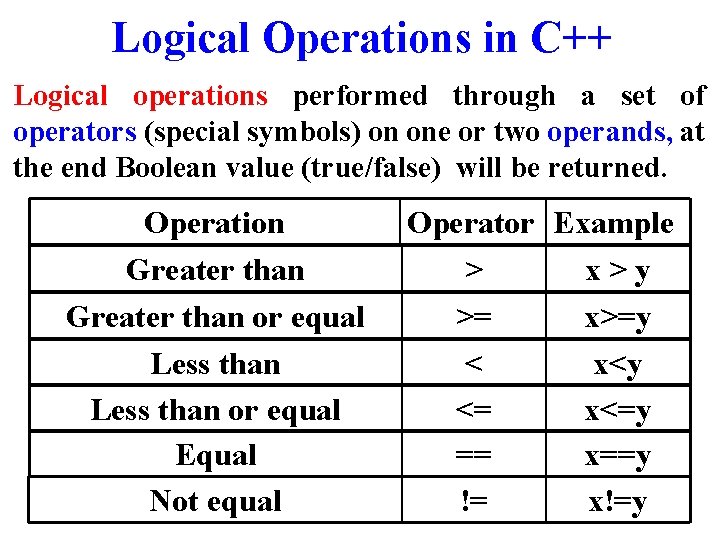
Logical Operations in C++ Logical operations performed through a set of operators (special symbols) on one or two operands, at the end Boolean value (true/false) will be returned. Operation Greater than or equal Less than or equal Equal Not equal Operator Example > x>y >= x>=y < x<y <= x<=y == x==y != x!=y
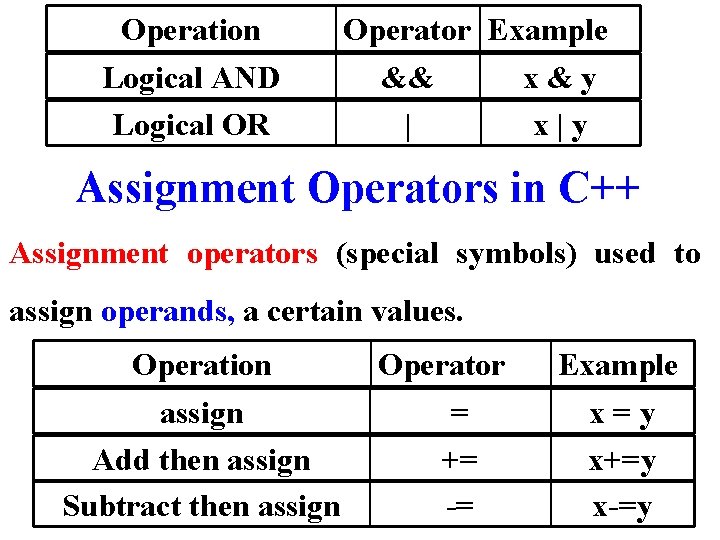
Operation Logical AND Logical OR Operator Example && x&y | x|y Assignment Operators in C++ Assignment operators (special symbols) used to assign operands, a certain values. Operation assign Add then assign Subtract then assign Operator = += -= Example x=y x+=y x-=y
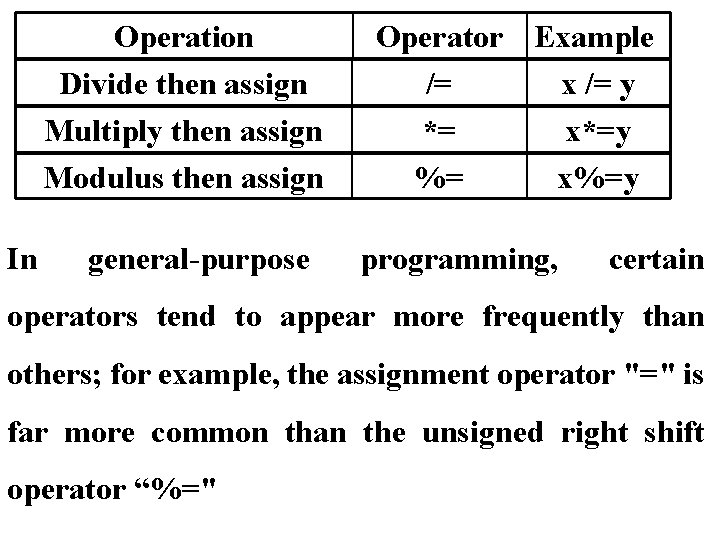
Operation Divide then assign Multiply then assign Modulus then assign In general-purpose Operator Example /= x /= y *= x*=y %= x%=y programming, certain operators tend to appear more frequently than others; for example, the assignment operator "=" is far more common than the unsigned right shift operator “%="
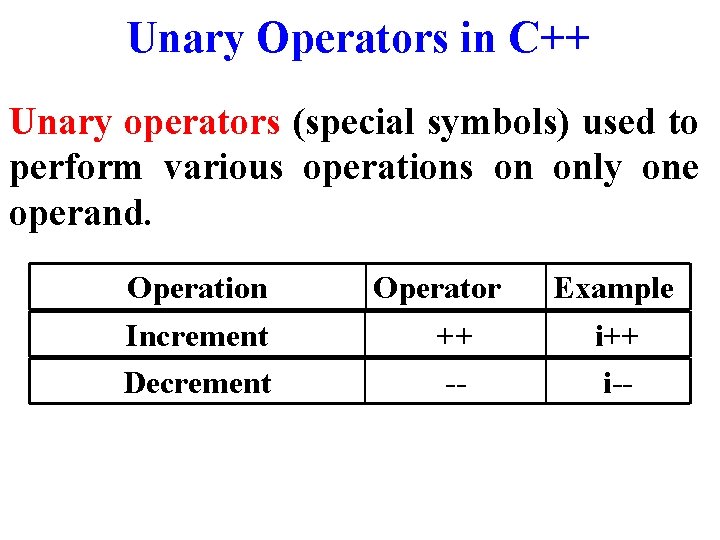
Unary Operators in C++ Unary operators (special symbols) used to perform various operations on only one operand. Operation Increment Decrement Operator ++ -- Example i++ i--
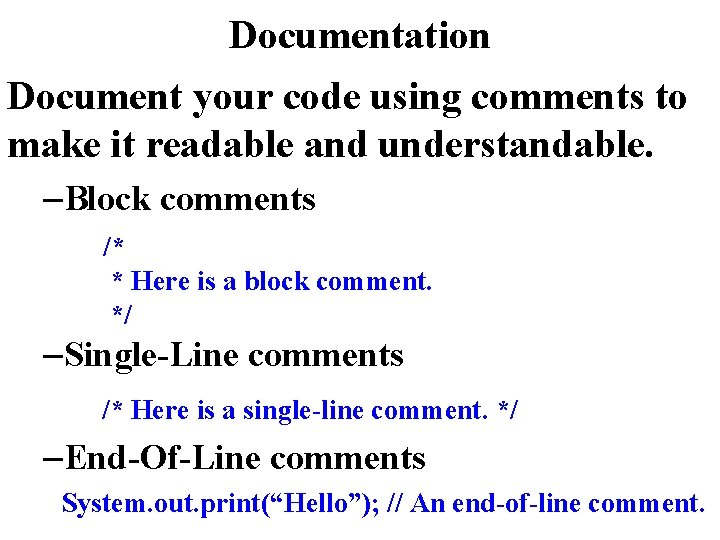
Documentation Document your code using comments to make it readable and understandable. –Block comments /* * Here is a block comment. */ –Single-Line comments /* Here is a single-line comment. */ –End-Of-Line comments System. out. print(“Hello”); // An end-of-line comment.
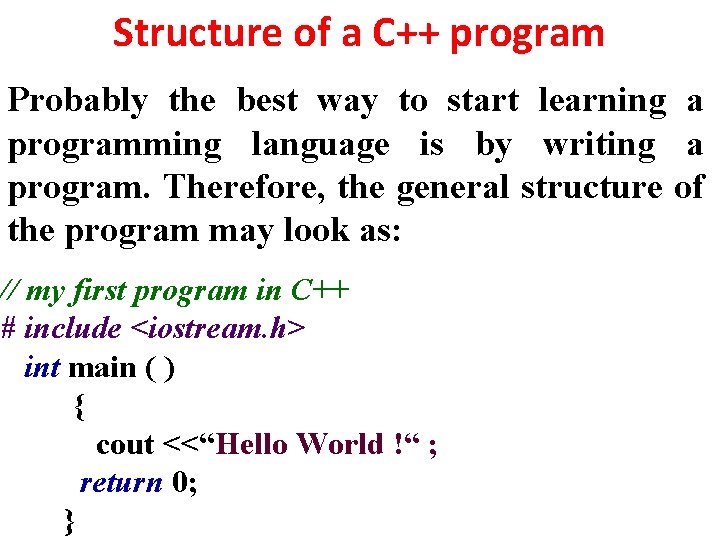
Structure of a C++ program Probably the best way to start learning a programming language is by writing a program. Therefore, the general structure of the program may look as: // my first program in C++ # include <iostream. h> int main ( ) { cout <<“Hello World !“ ; return 0; }
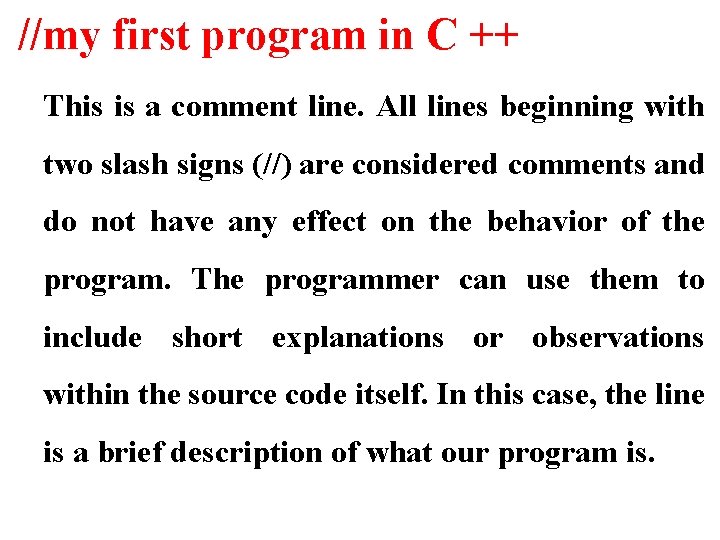
//my first program in C ++ This is a comment line. All lines beginning with two slash signs (//) are considered comments and do not have any effect on the behavior of the program. The programmer can use them to include short explanations or observations within the source code itself. In this case, the line is a brief description of what our program is.
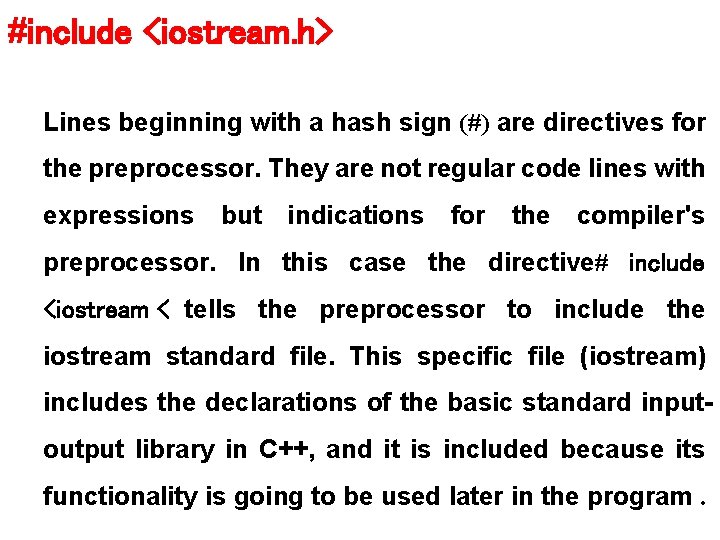
#include <iostream. h> Lines beginning with a hash sign (#) are directives for the preprocessor. They are not regular code lines with expressions but indications for the compiler's preprocessor. In this case the directive# include <iostream < tells the preprocessor to include the iostream standard file. This specific file (iostream) includes the declarations of the basic standard inputoutput library in C++, and it is included because its functionality is going to be used later in the program.
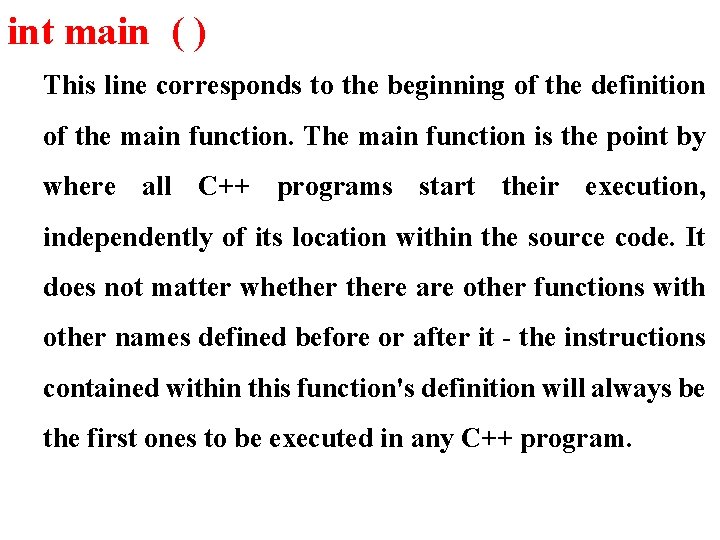
int main ( ) This line corresponds to the beginning of the definition of the main function. The main function is the point by where all C++ programs start their execution, independently of its location within the source code. It does not matter whethere are other functions with other names defined before or after it - the instructions contained within this function's definition will always be the first ones to be executed in any C++ program.
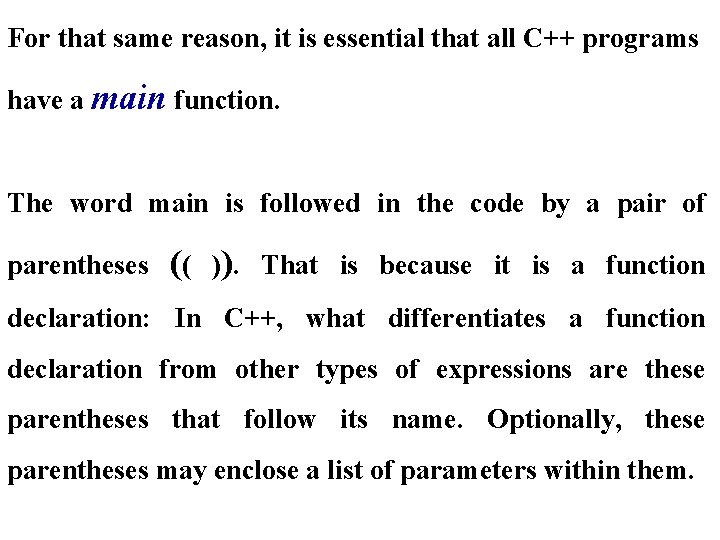
For that same reason, it is essential that all C++ programs have a main function. The word main is followed in the code by a pair of parentheses (( )). That is because it is a function declaration: In C++, what differentiates a function declaration from other types of expressions are these parentheses that follow its name. Optionally, these parentheses may enclose a list of parameters within them.
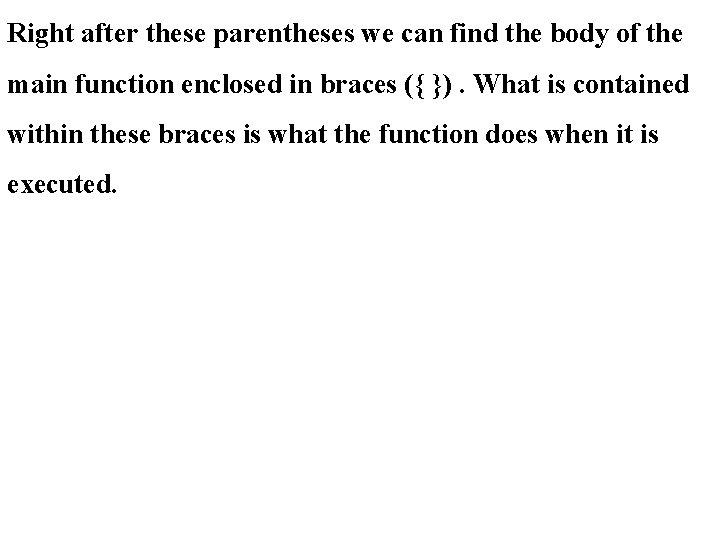
Right after these parentheses we can find the body of the main function enclosed in braces ({ }). What is contained within these braces is what the function does when it is executed.
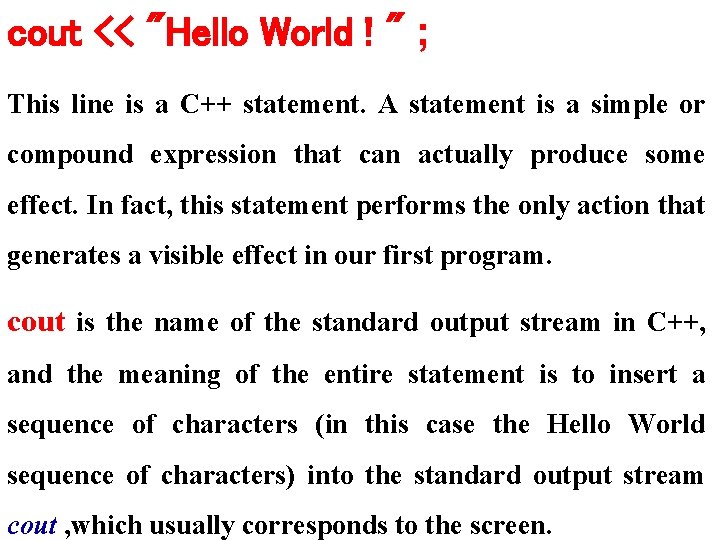
cout << "Hello World ! " ; This line is a C++ statement. A statement is a simple or compound expression that can actually produce some effect. In fact, this statement performs the only action that generates a visible effect in our first program. cout is the name of the standard output stream in C++, and the meaning of the entire statement is to insert a sequence of characters (in this case the Hello World sequence of characters) into the standard output stream cout , which usually corresponds to the screen.
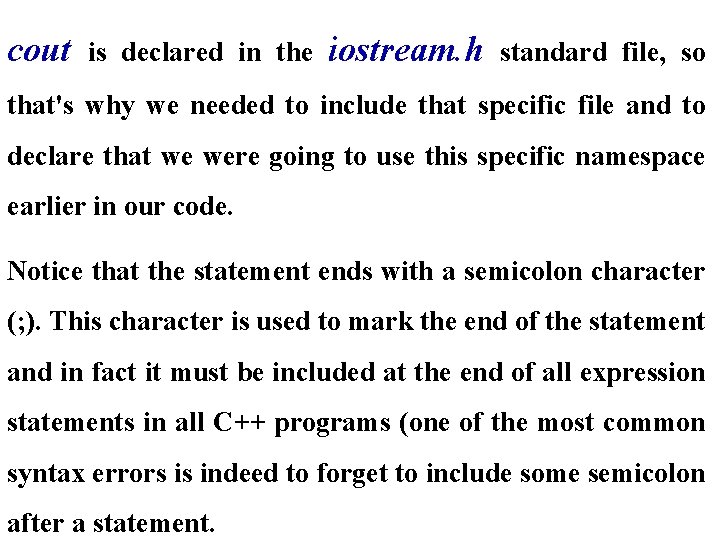
cout is declared in the iostream. h standard file, so that's why we needed to include that specific file and to declare that we were going to use this specific namespace earlier in our code. Notice that the statement ends with a semicolon character (; ). This character is used to mark the end of the statement and in fact it must be included at the end of all expression statements in all C++ programs (one of the most common syntax errors is indeed to forget to include some semicolon after a statement.
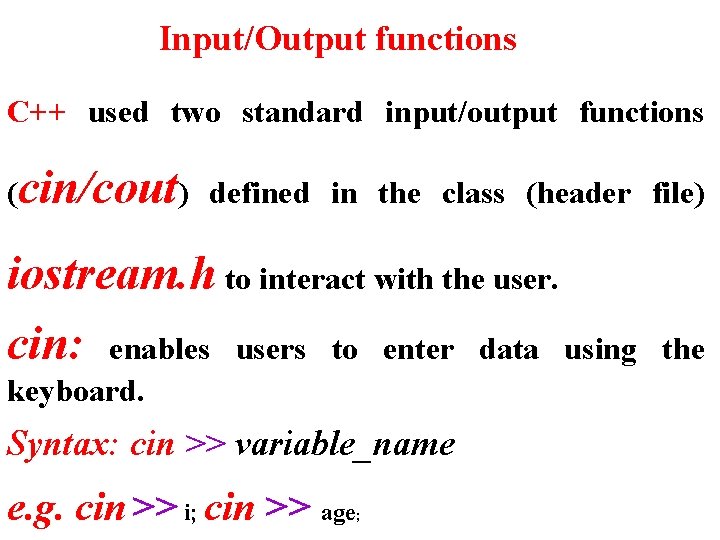
Input/Output functions C++ used two standard input/output functions (cin/cout) defined in the class (header file) iostream. h to interact with the user. cin: enables users to enter data using keyboard. Syntax: cin >> variable_name e. g. cin >> i; cin >> age ; the
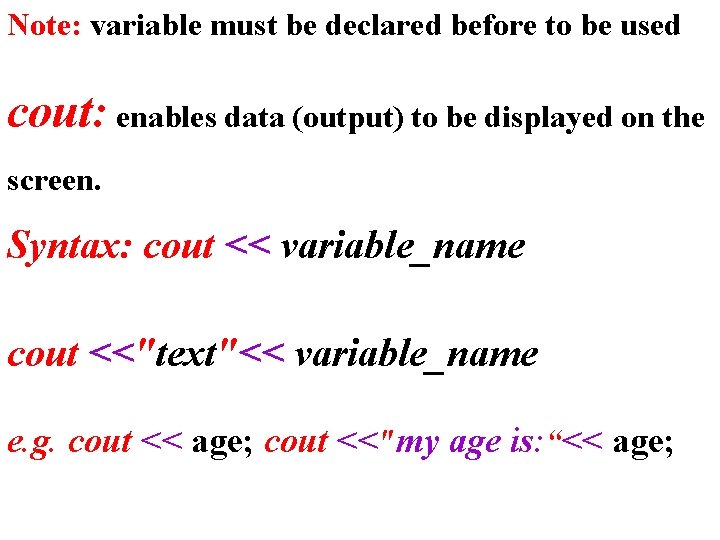
Note: variable must be declared before to be used cout: enables data (output) to be displayed on the screen. Syntax: cout << variable_name cout <<"text"<< variable_name e. g. cout << age; cout <<"my age is: “<< age;