Java Threads and Java Collections Four kinds of
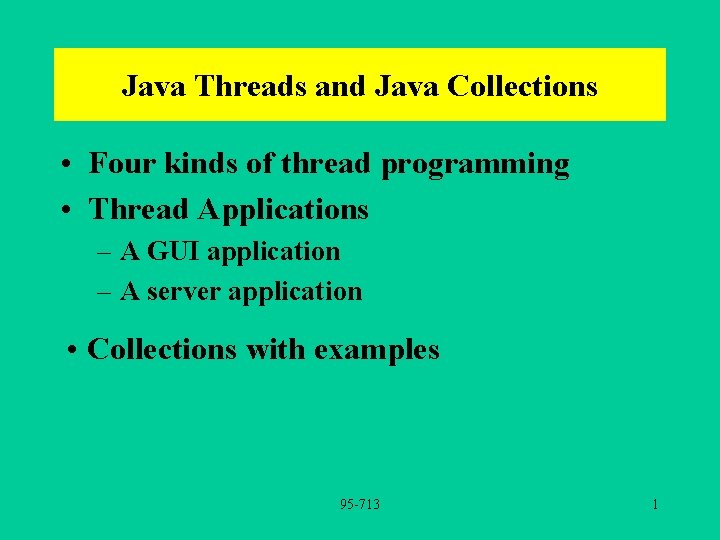
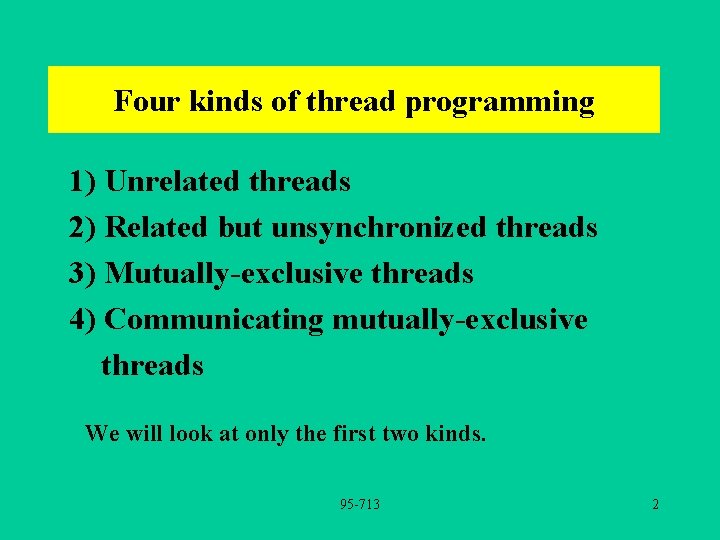
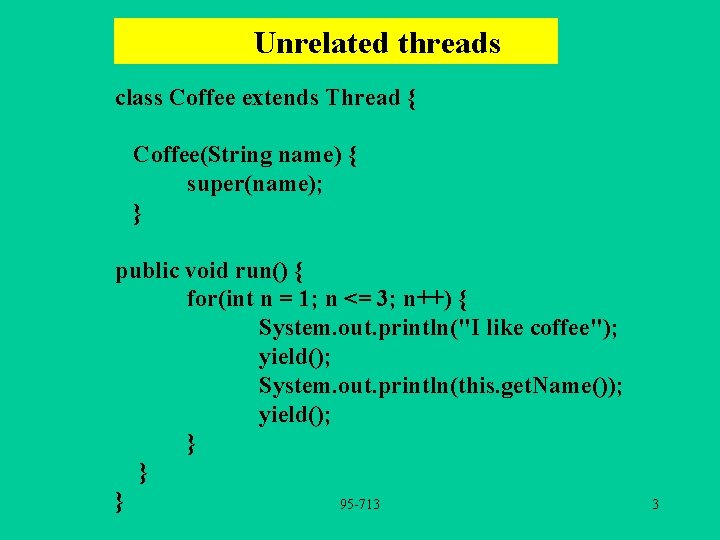
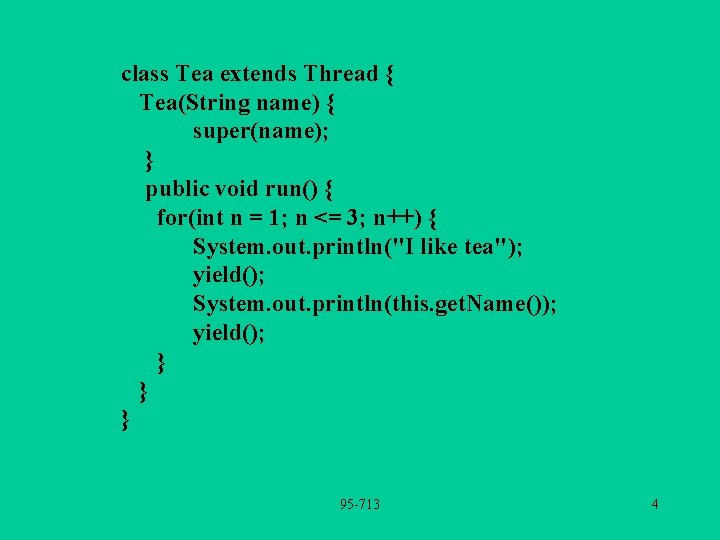
![public class Drinks { public static void main(String args[]) { System. out. println("I am public class Drinks { public static void main(String args[]) { System. out. println("I am](https://slidetodoc.com/presentation_image_h/31cf08bc45fc415bb1238376aea30ede/image-5.jpg)
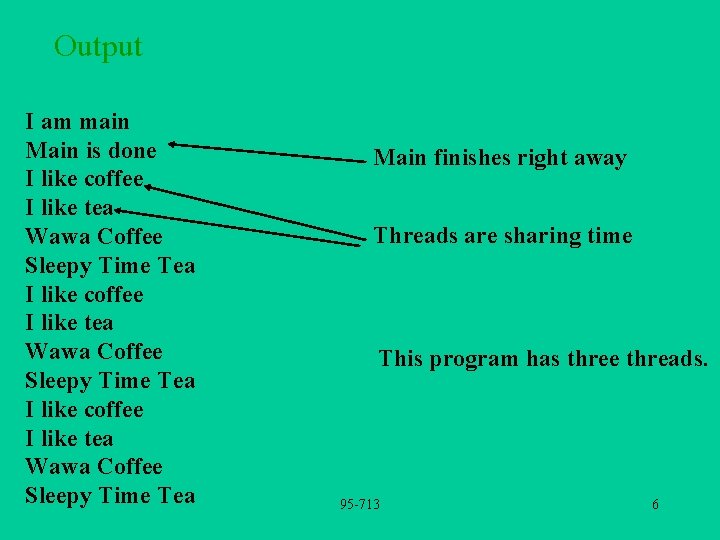
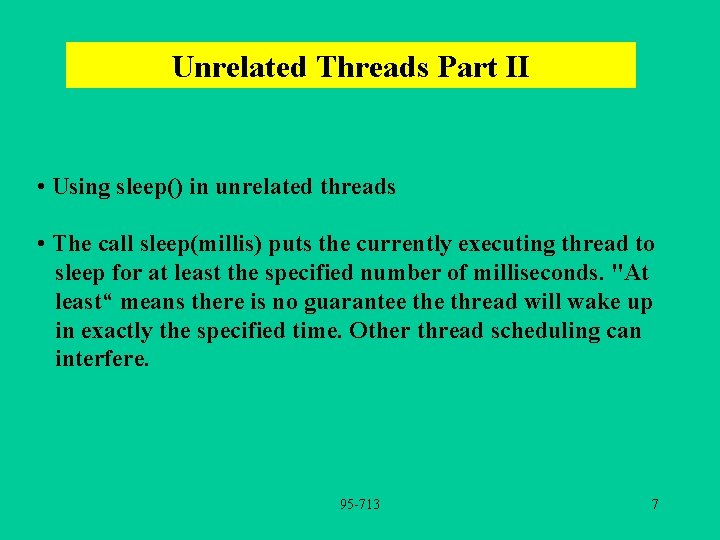
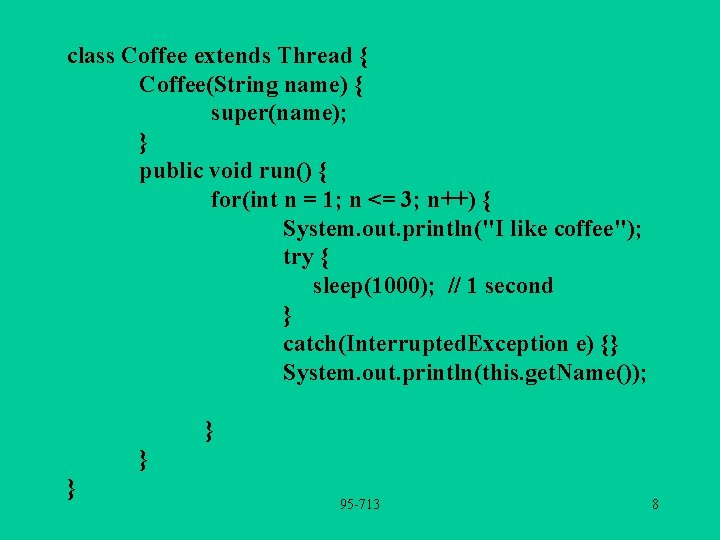
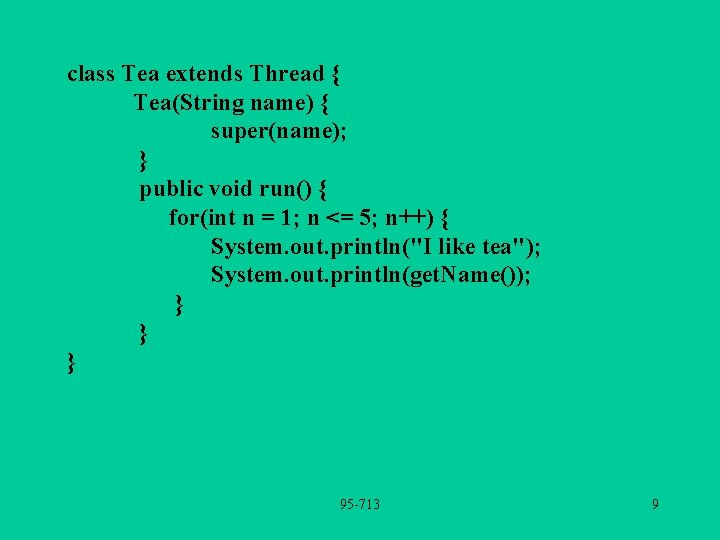
![public class Drinks 2 { public static void main(String args[]) { System. out. println("I public class Drinks 2 { public static void main(String args[]) { System. out. println("I](https://slidetodoc.com/presentation_image_h/31cf08bc45fc415bb1238376aea30ede/image-10.jpg)
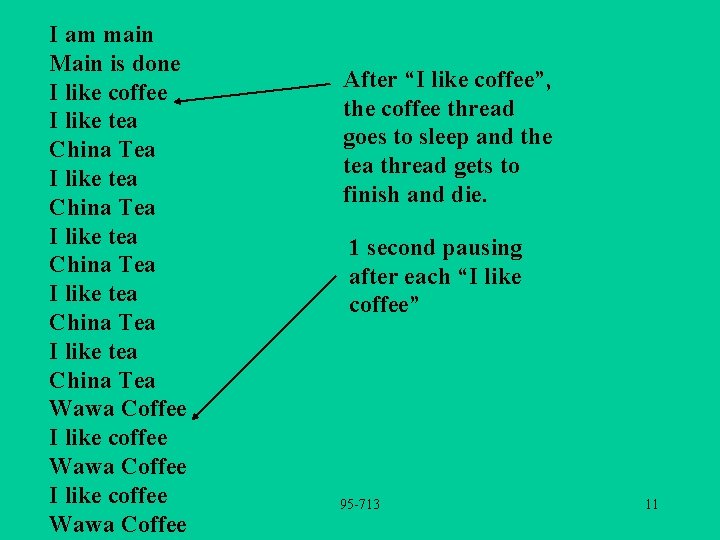
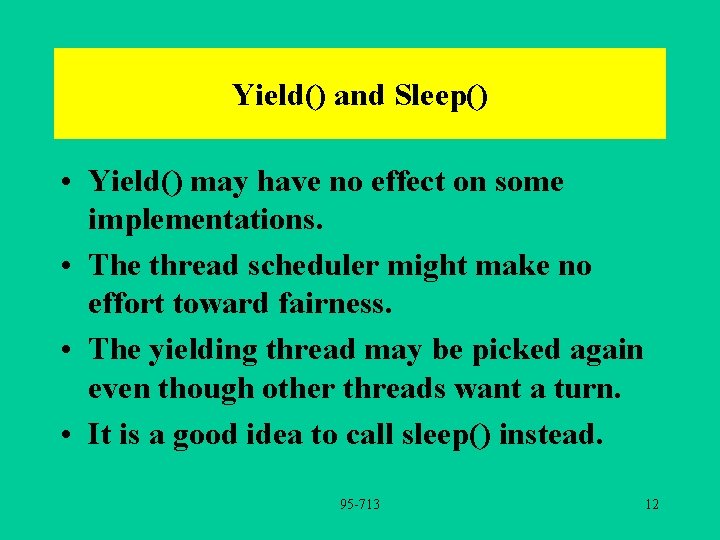
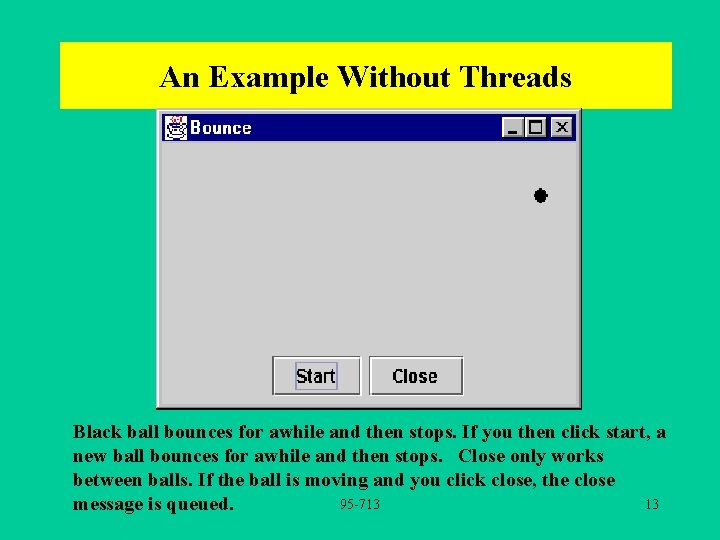
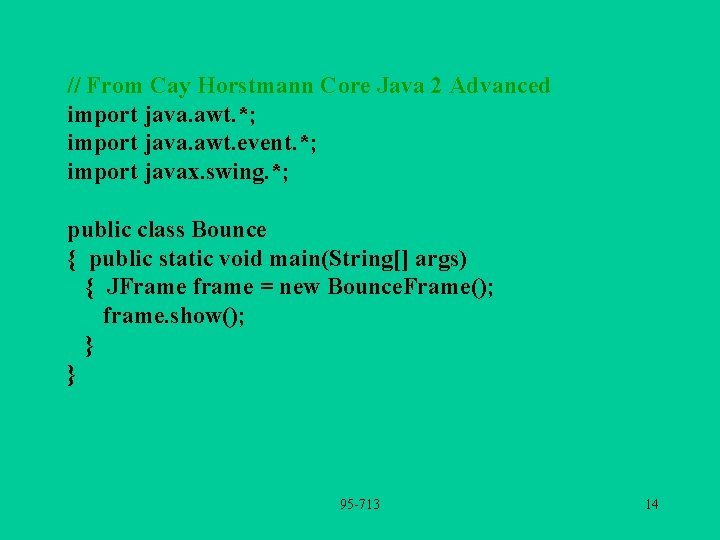
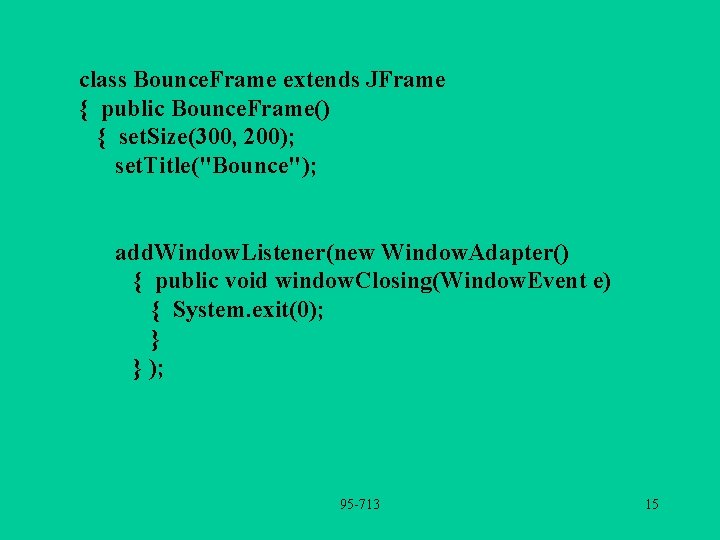
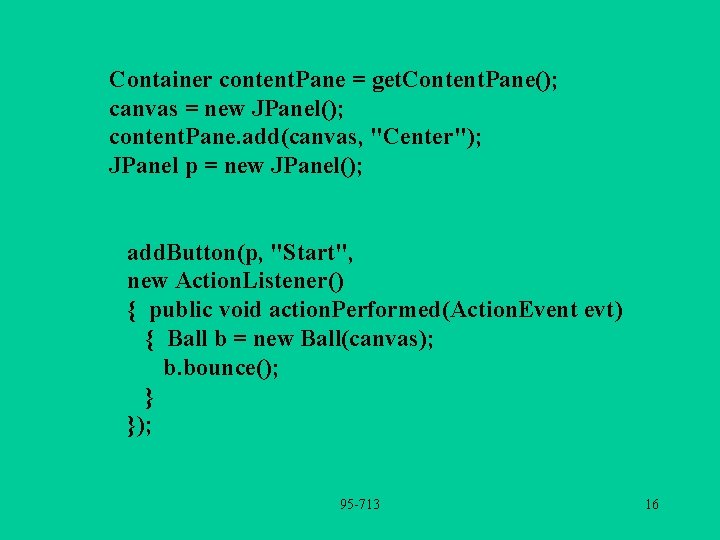
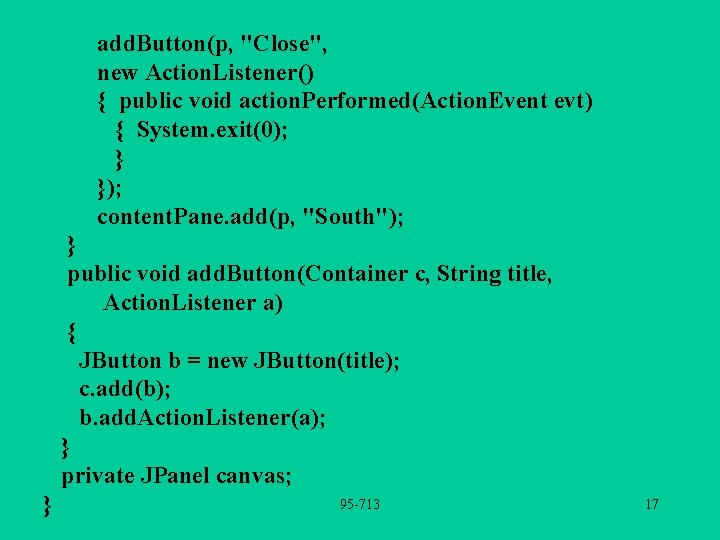
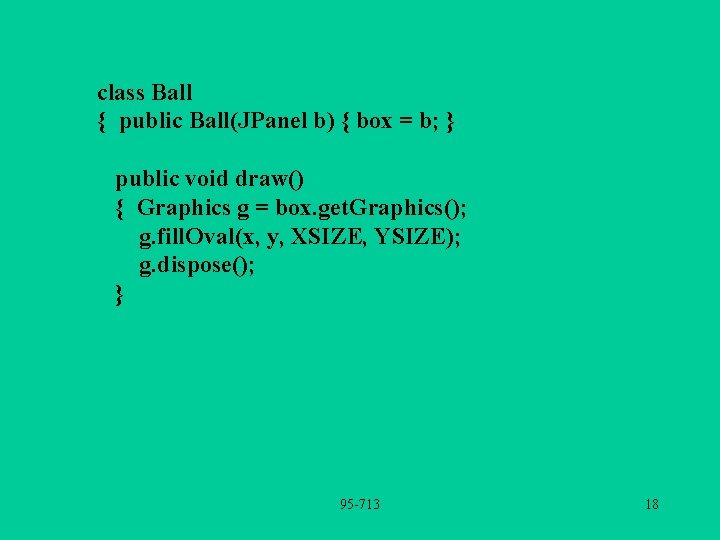
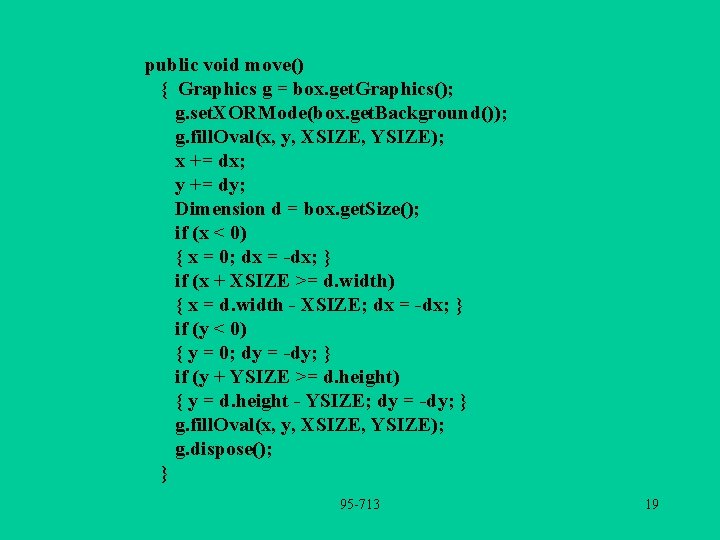
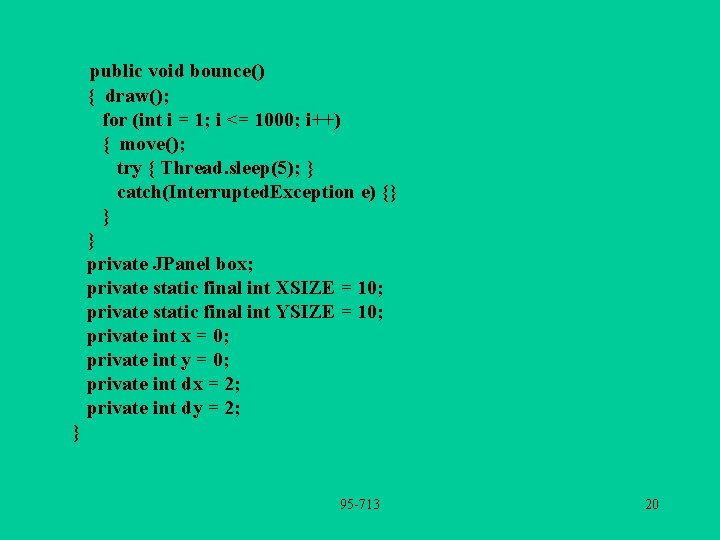
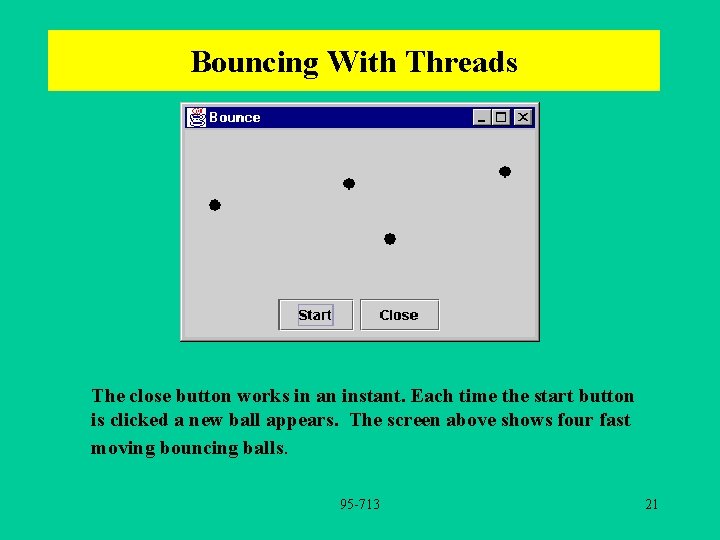
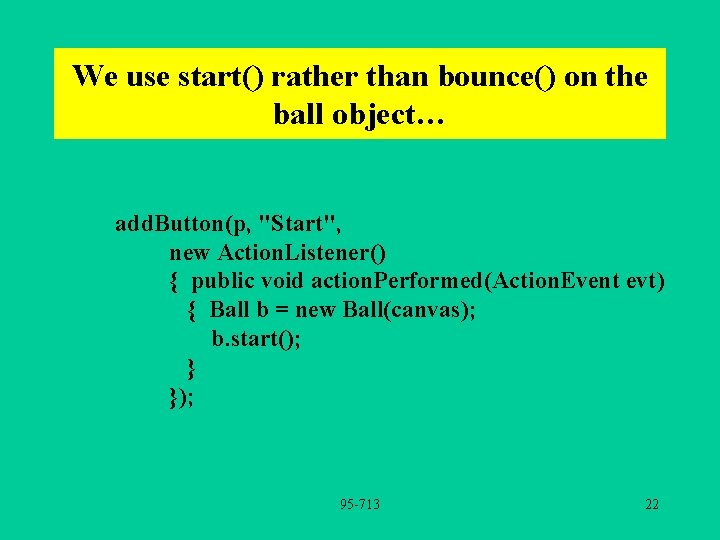
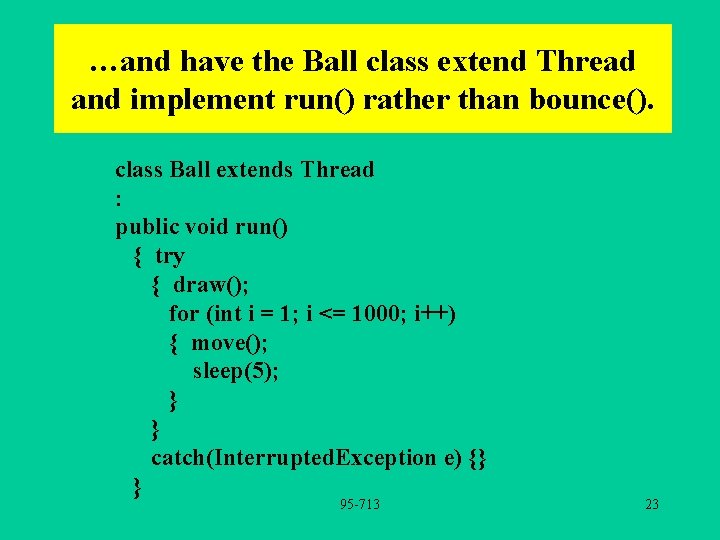
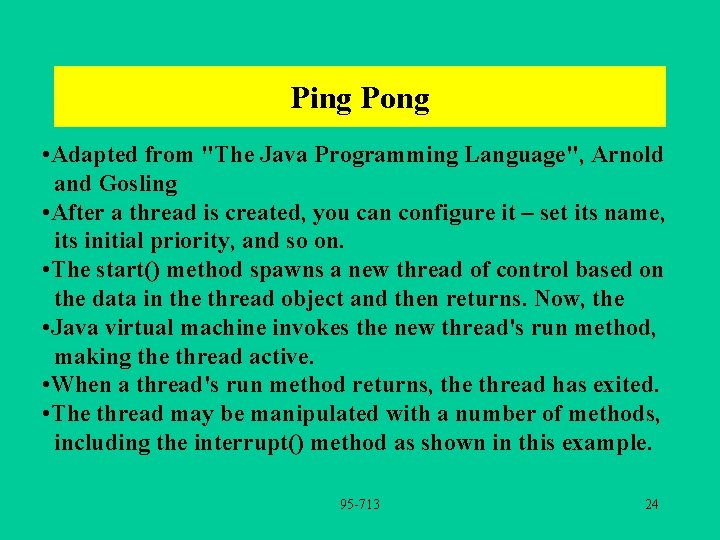
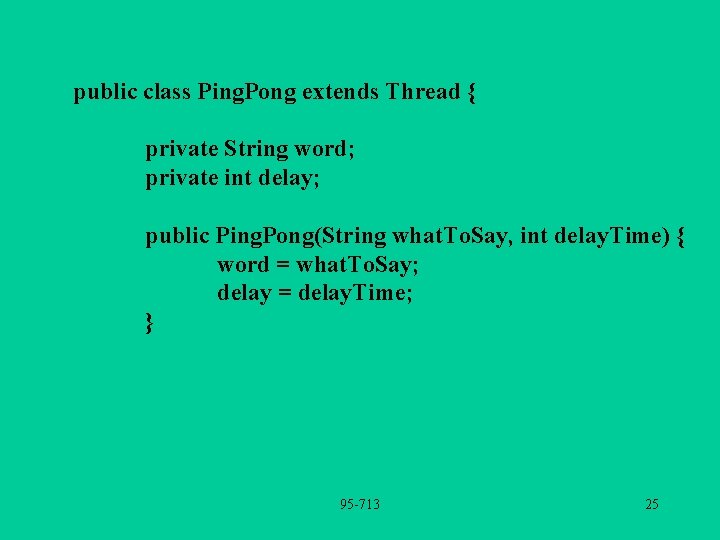
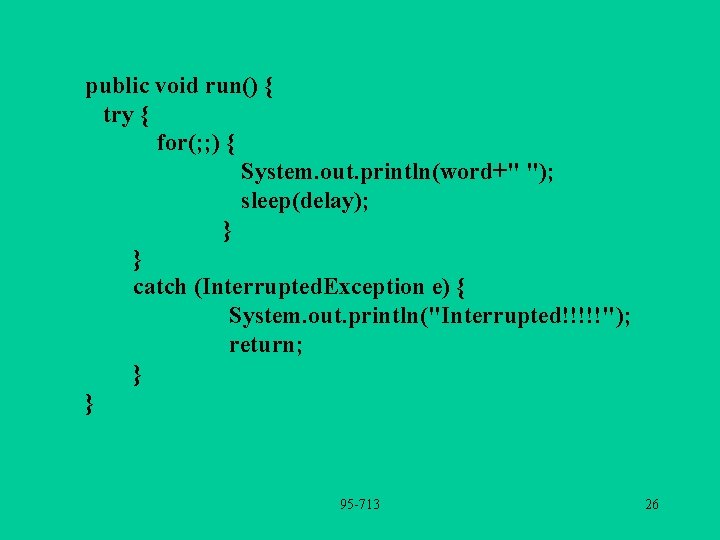
![public static void main(String args[]) { Ping. Pong t 1 = new Ping. Pong("tping", public static void main(String args[]) { Ping. Pong t 1 = new Ping. Pong("tping",](https://slidetodoc.com/presentation_image_h/31cf08bc45fc415bb1238376aea30ede/image-27.jpg)
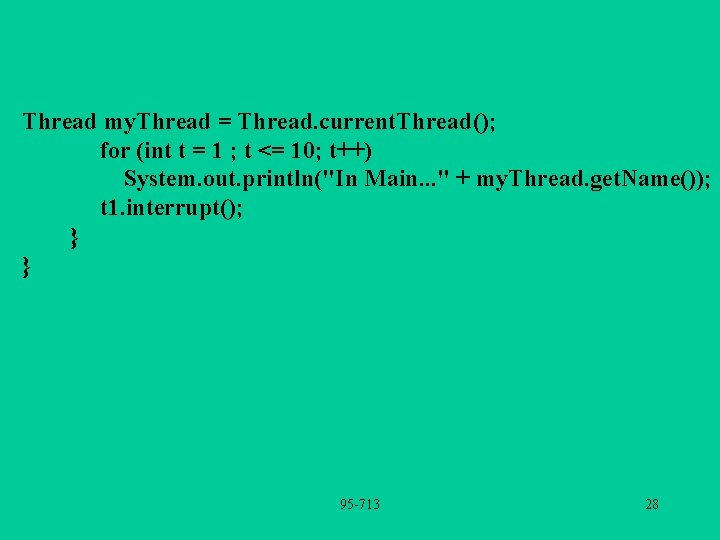
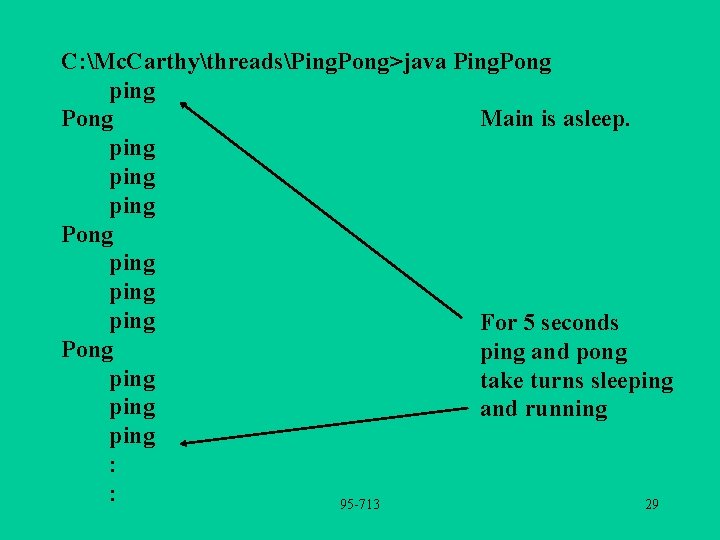
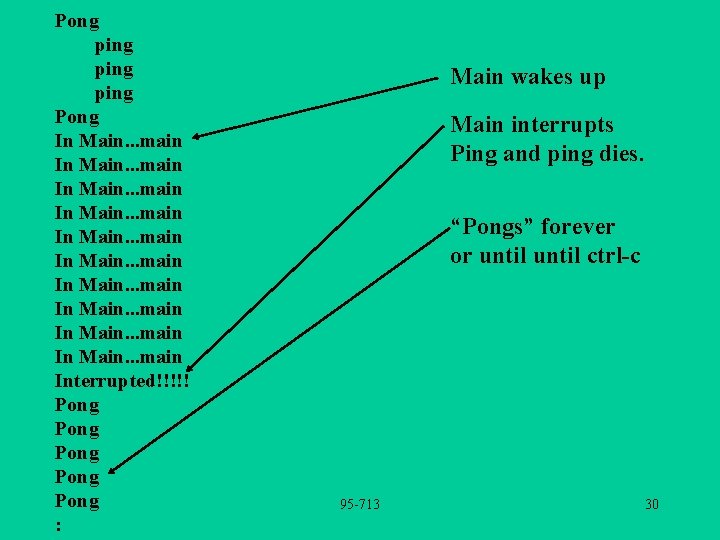
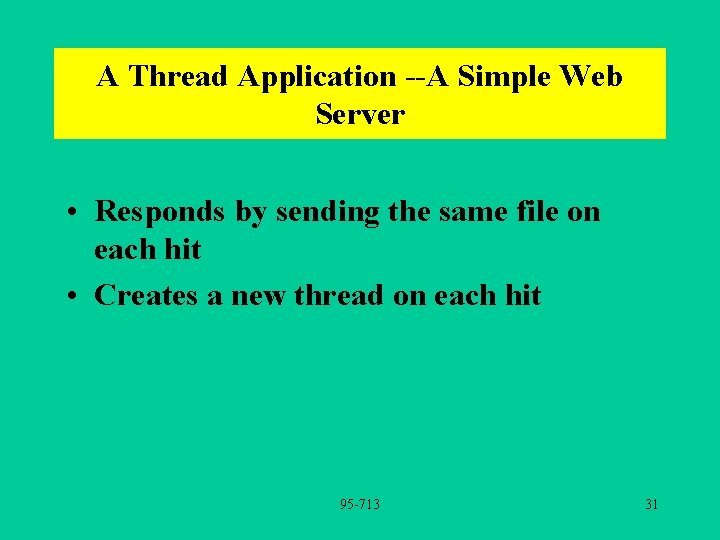
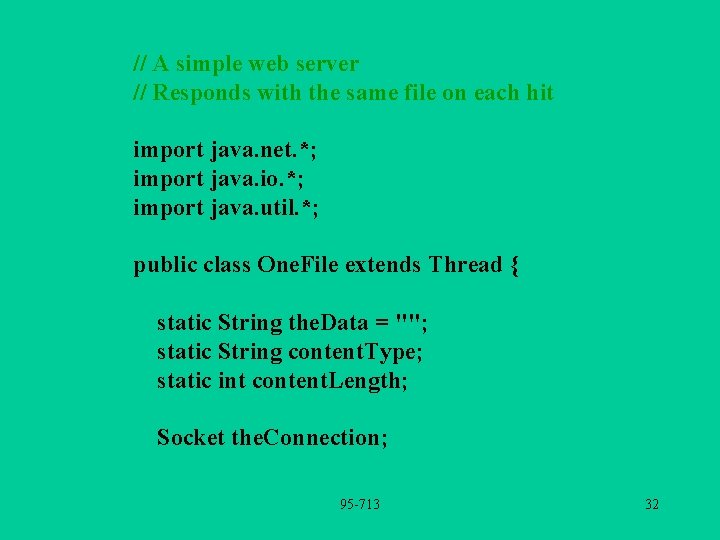
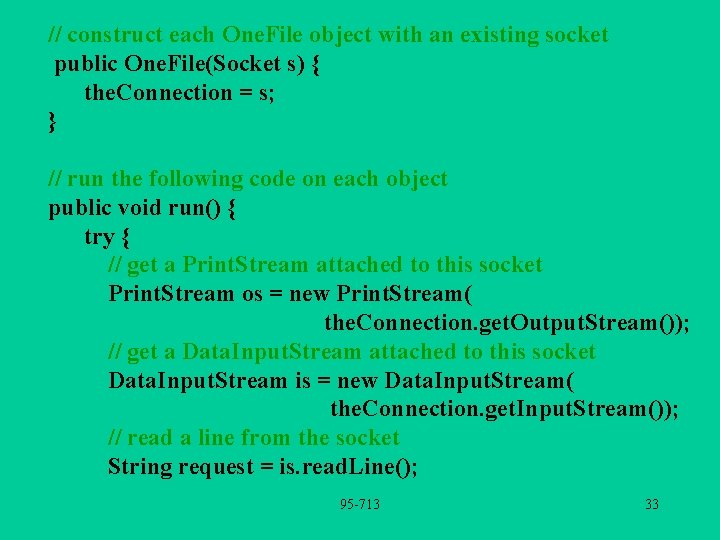
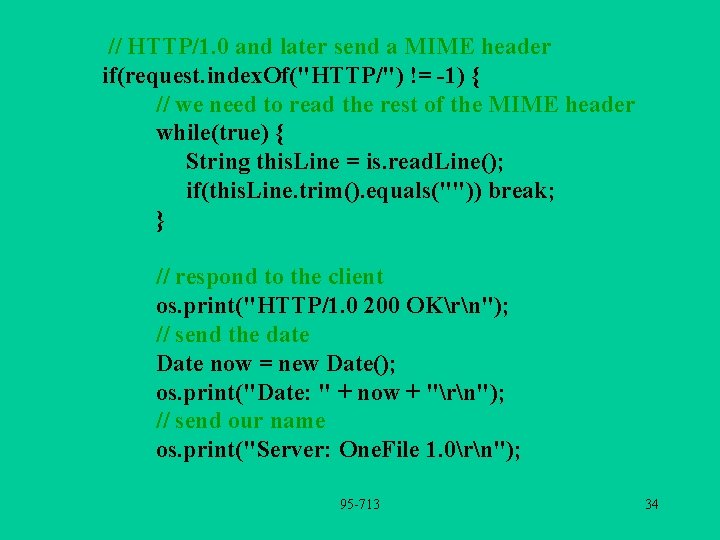
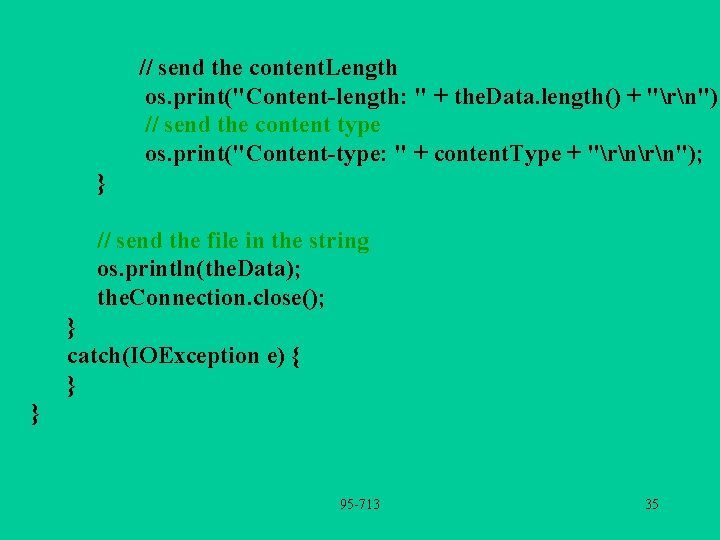
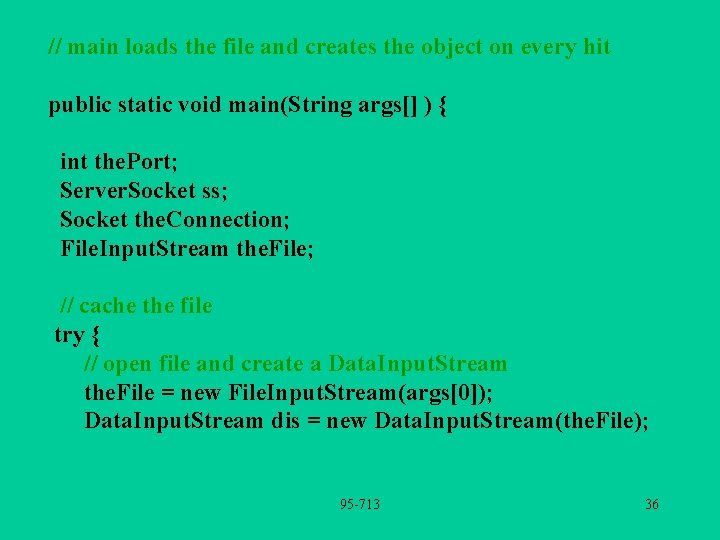
![} // determine the content type of this file if(args[0]. ends. With(". html") || } // determine the content type of this file if(args[0]. ends. With(". html") ||](https://slidetodoc.com/presentation_image_h/31cf08bc45fc415bb1238376aea30ede/image-37.jpg)
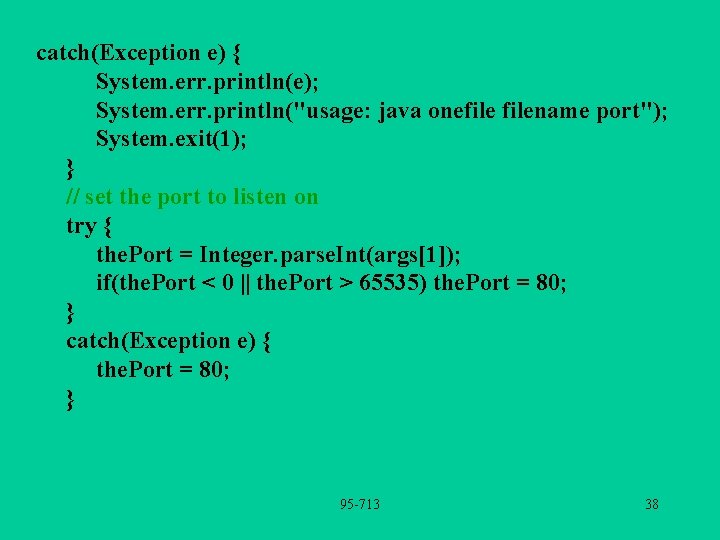
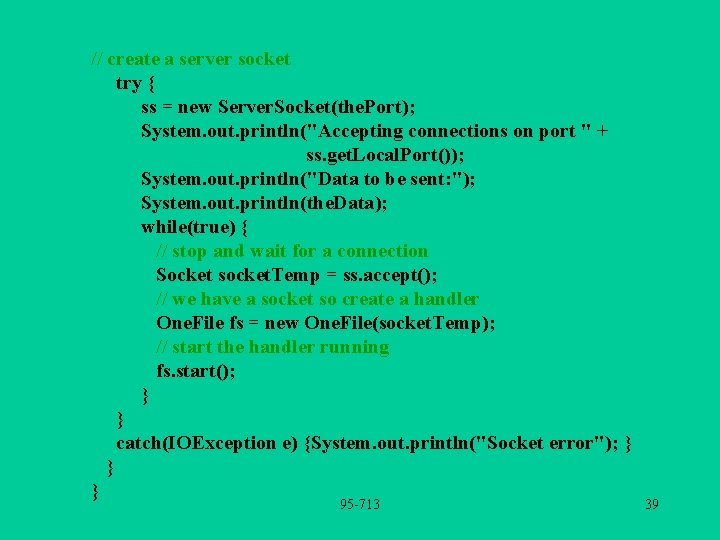
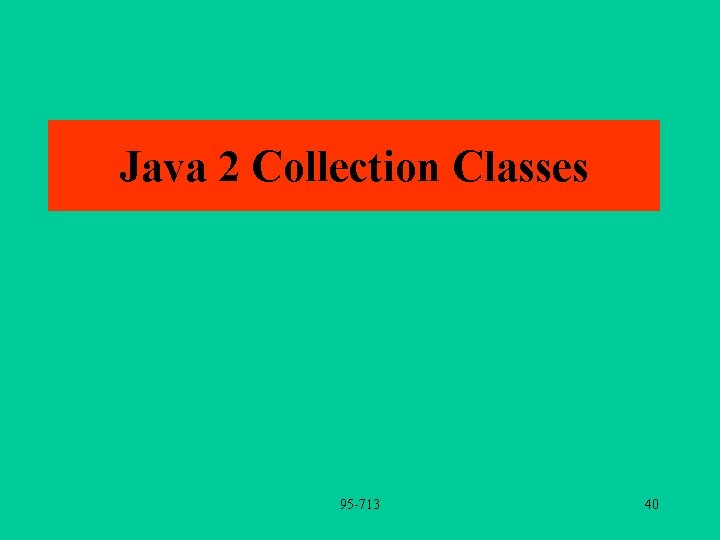
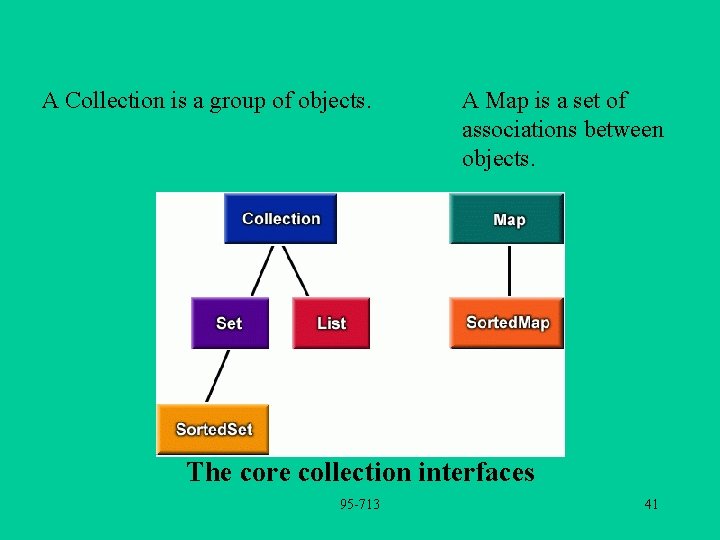
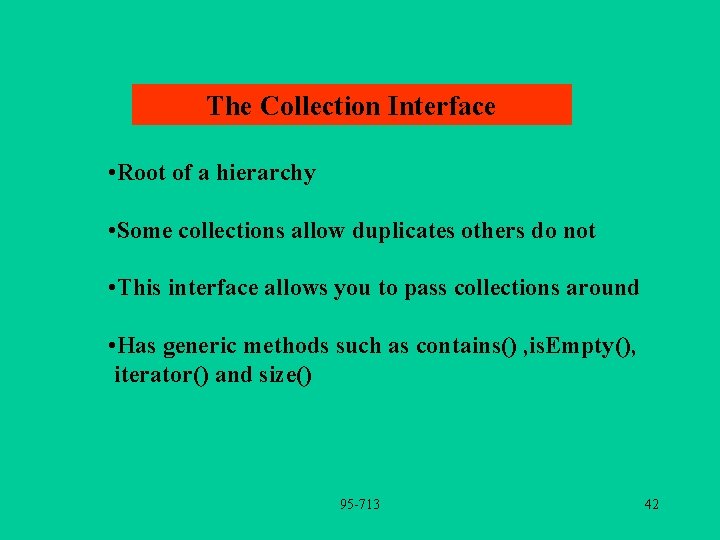
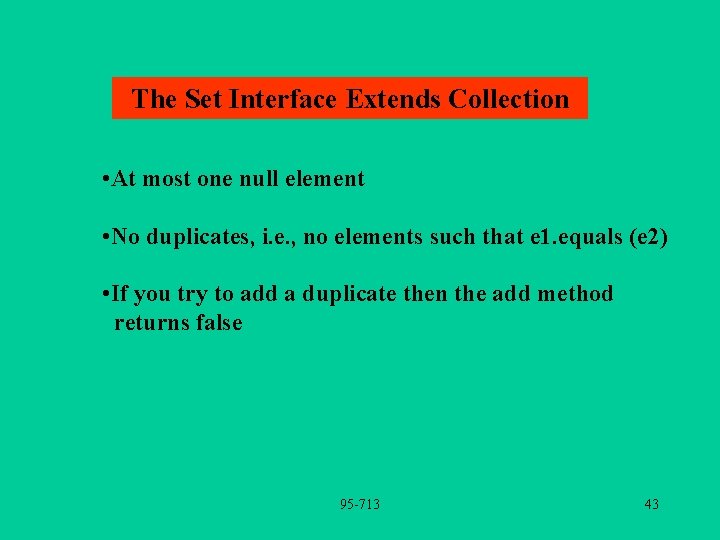
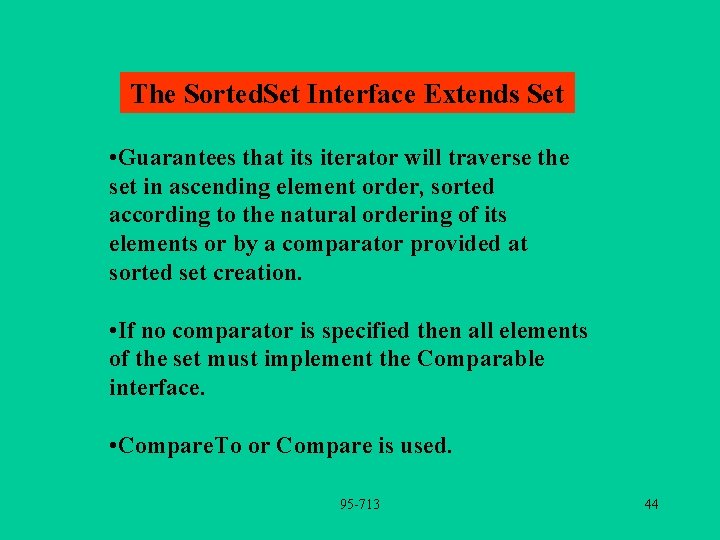
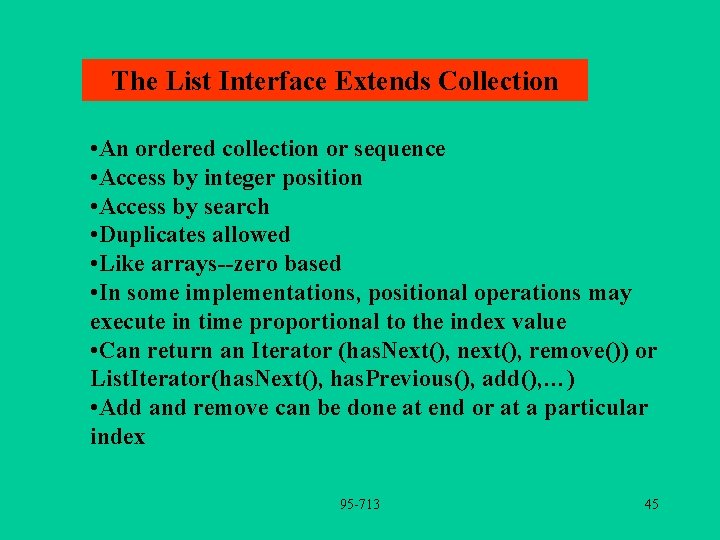
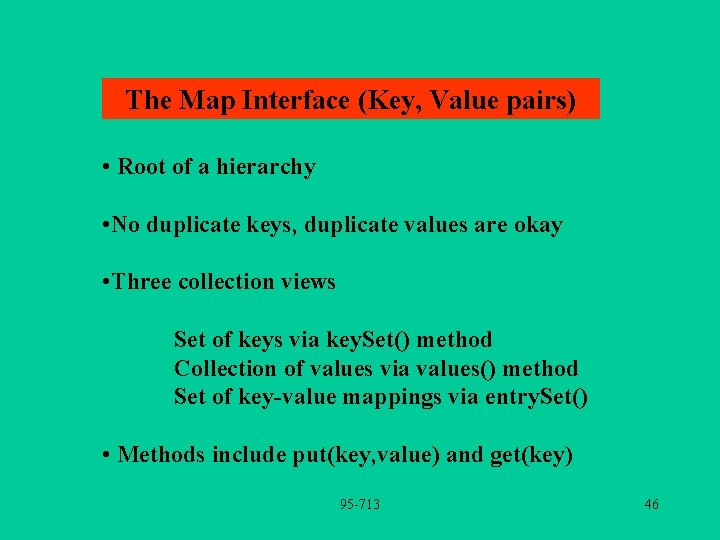
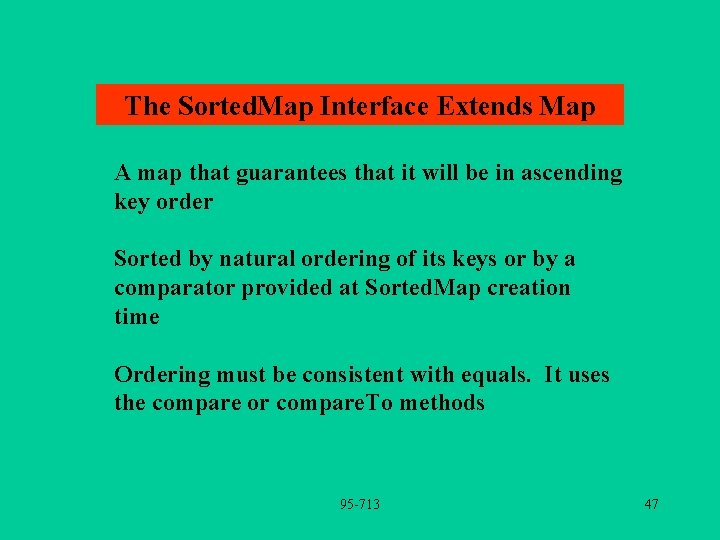
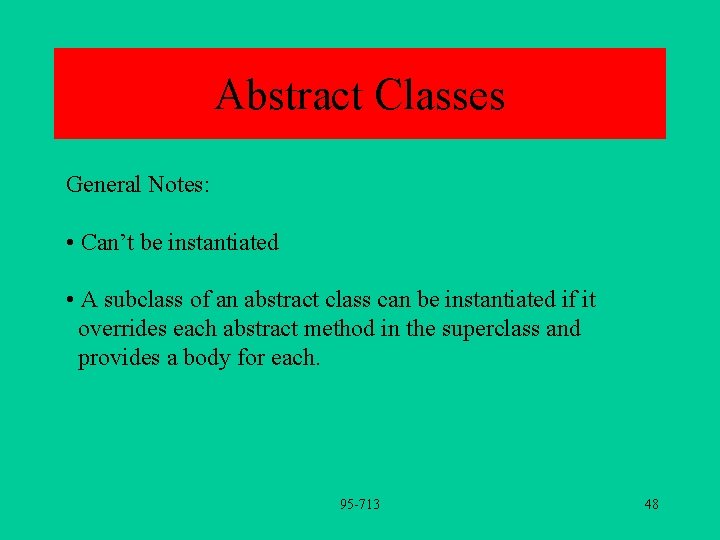
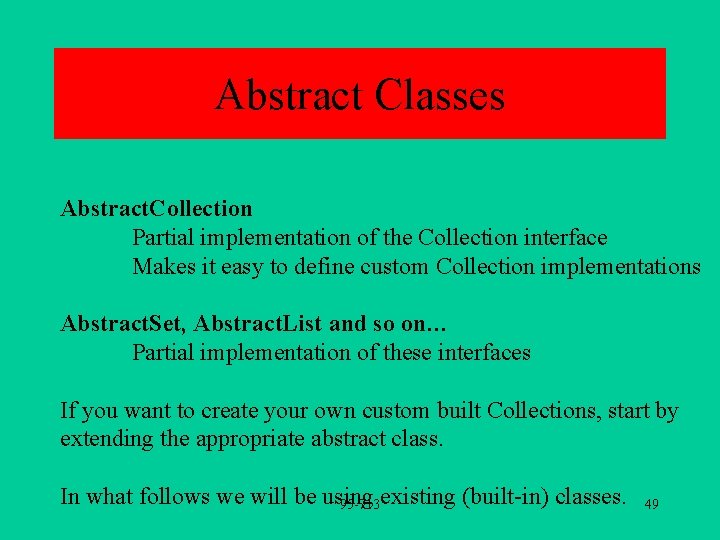
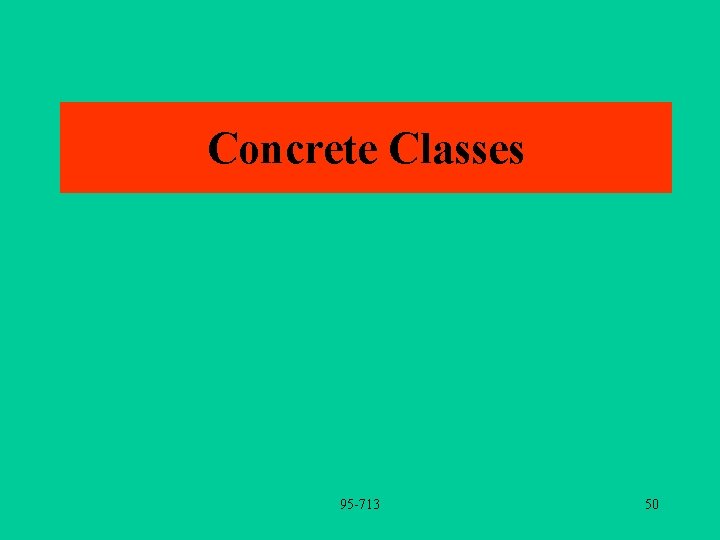
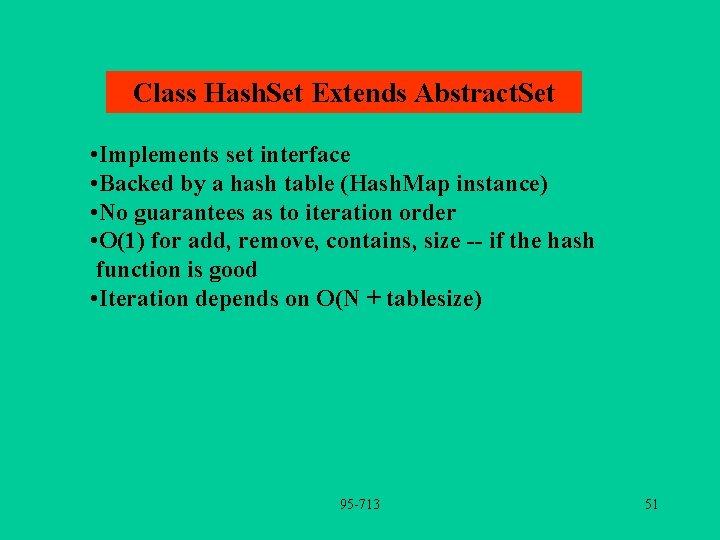
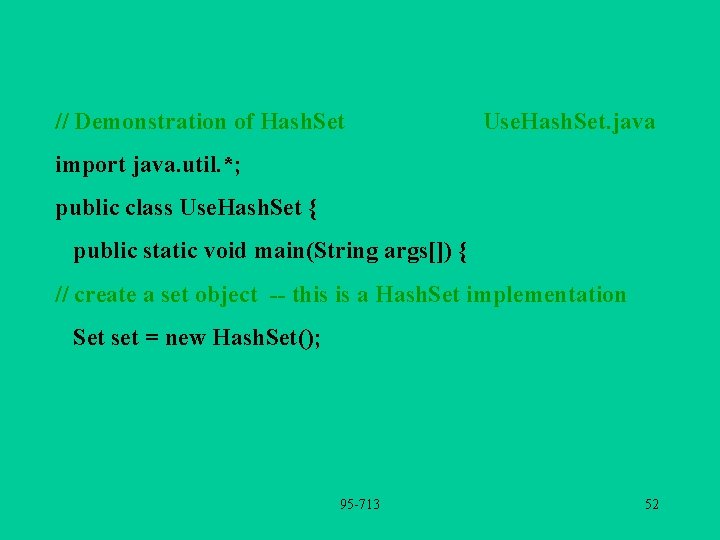
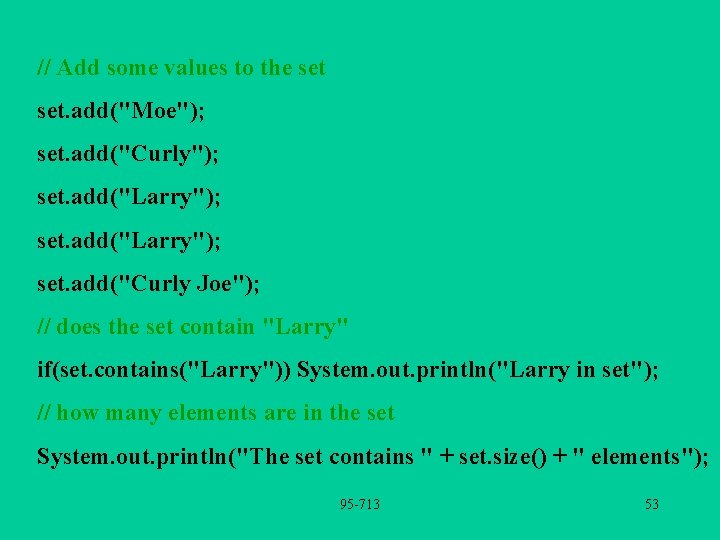
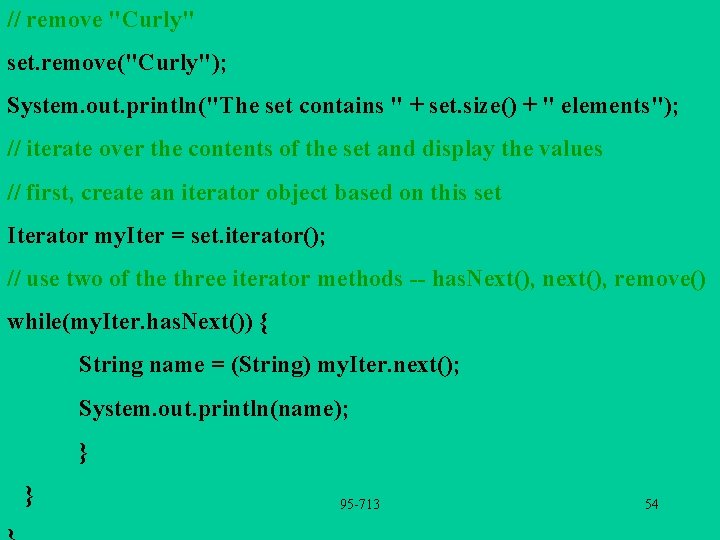
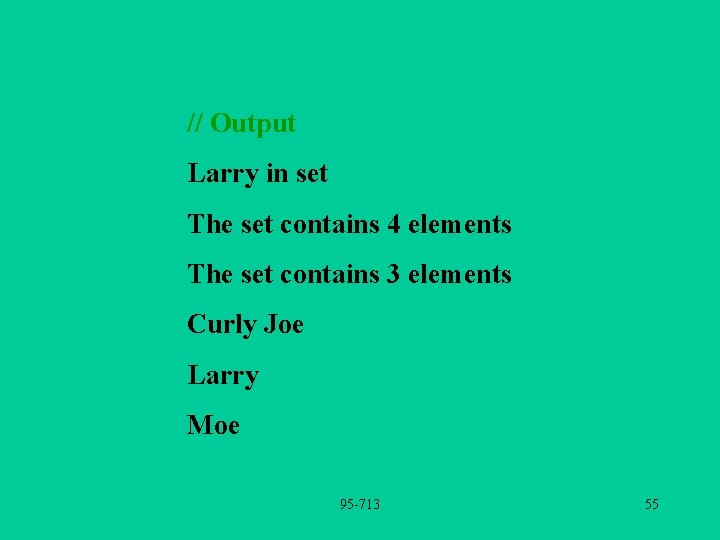
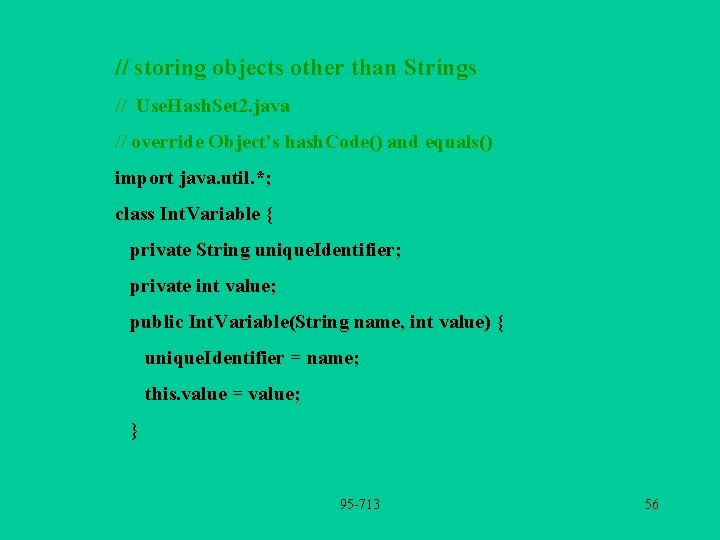
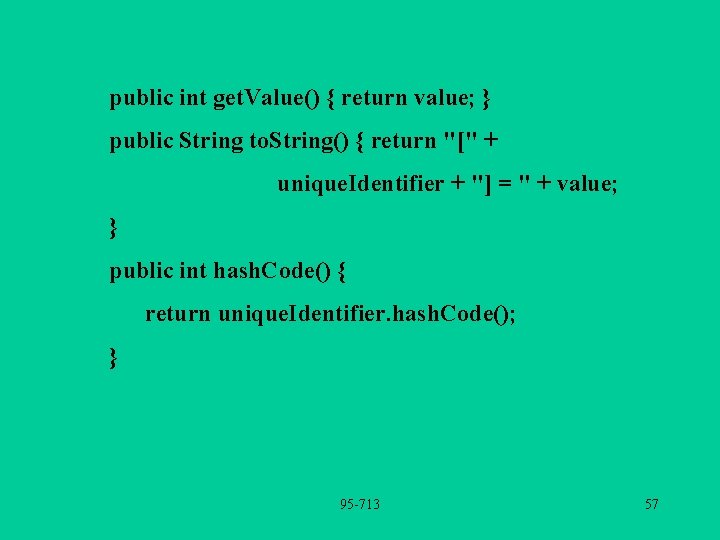
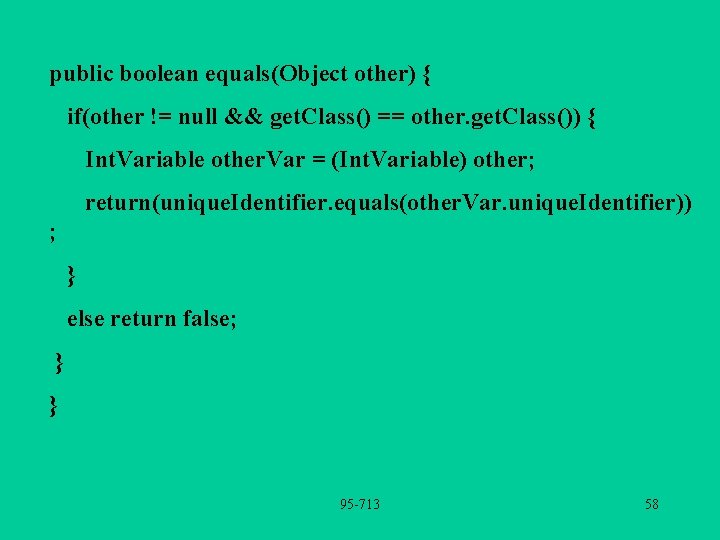
![public class Use. Hash. Set 2 { public static void main(String args[]) { Set public class Use. Hash. Set 2 { public static void main(String args[]) { Set](https://slidetodoc.com/presentation_image_h/31cf08bc45fc415bb1238376aea30ede/image-59.jpg)
![//Output [Y] = 45 [X] = 23 95 -713 60 //Output [Y] = 45 [X] = 23 95 -713 60](https://slidetodoc.com/presentation_image_h/31cf08bc45fc415bb1238376aea30ede/image-60.jpg)
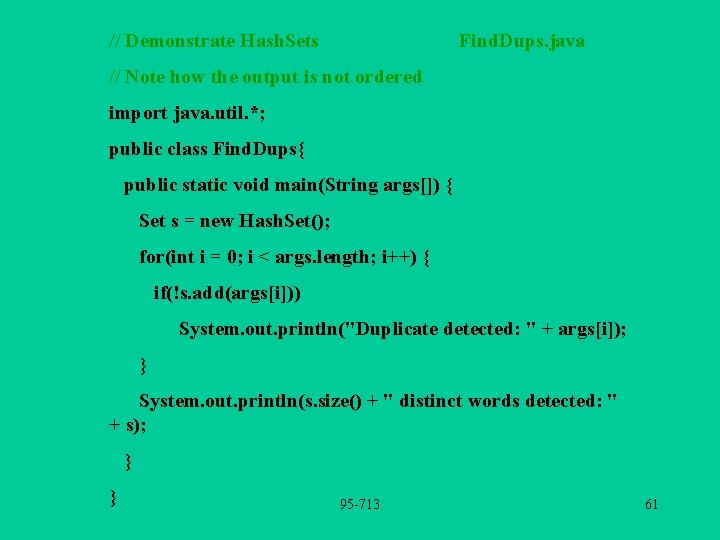
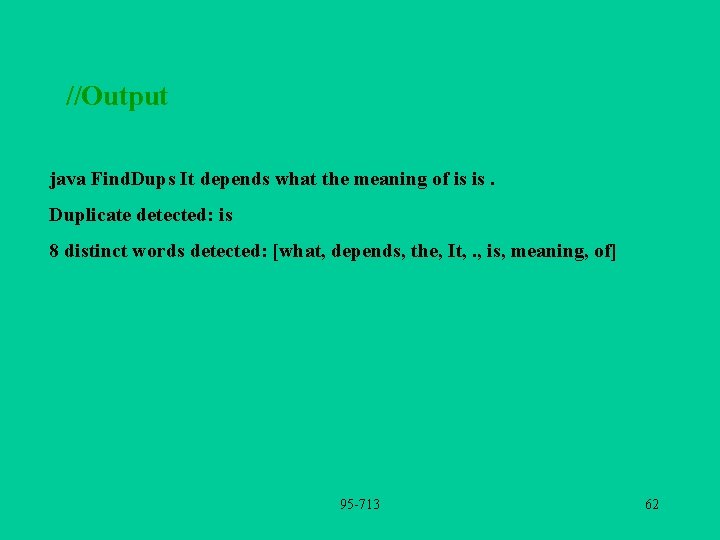
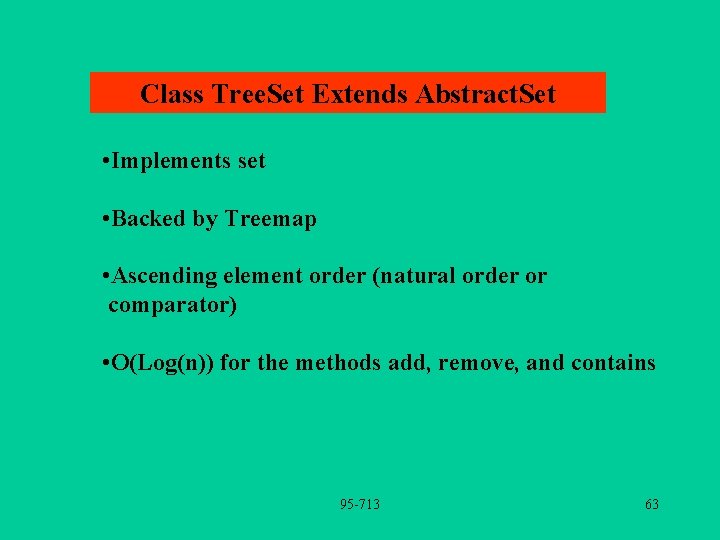
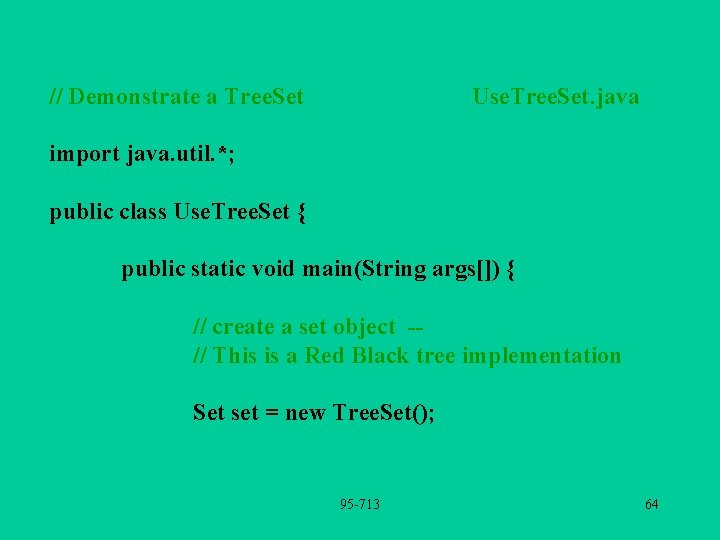
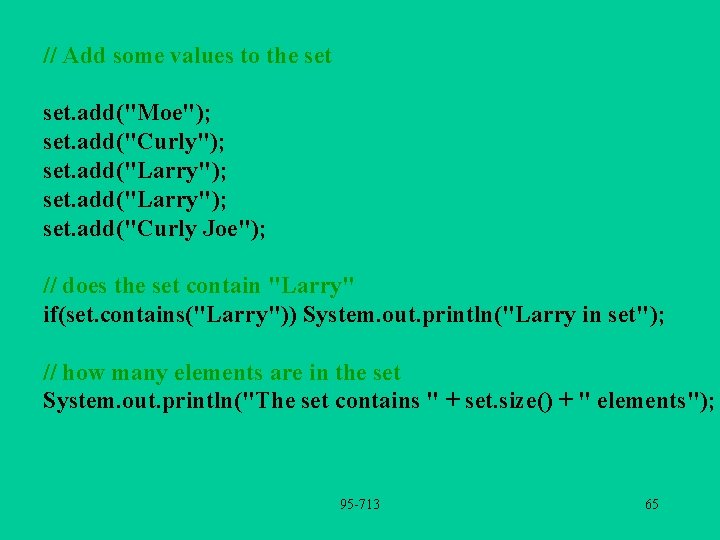
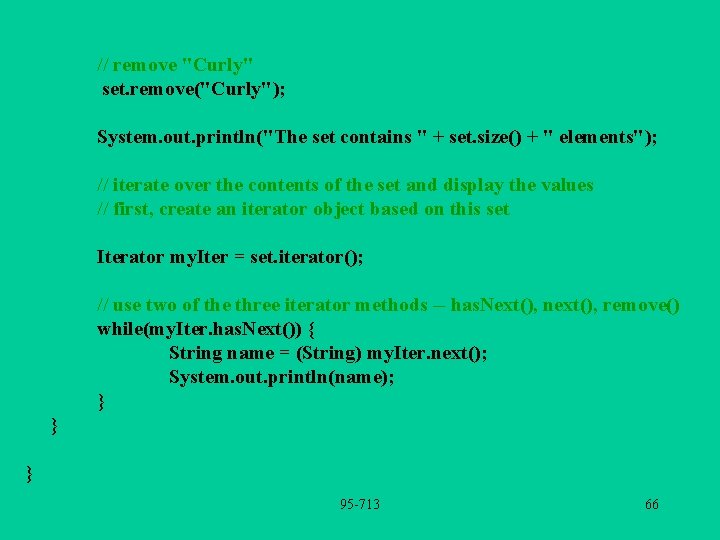
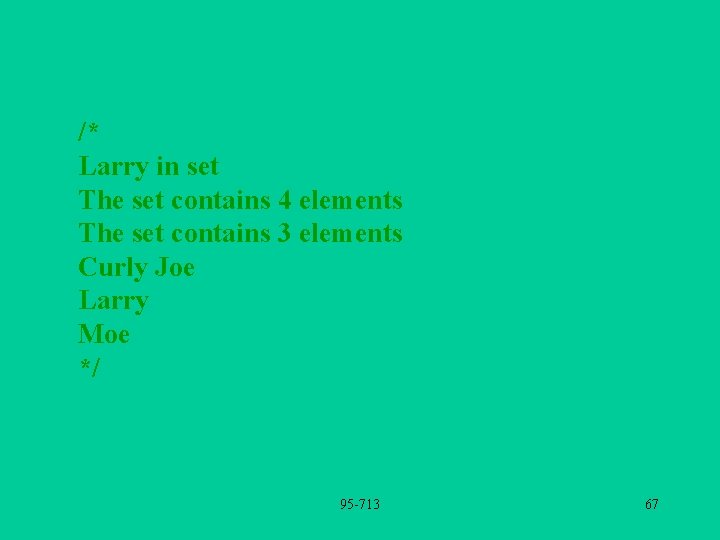
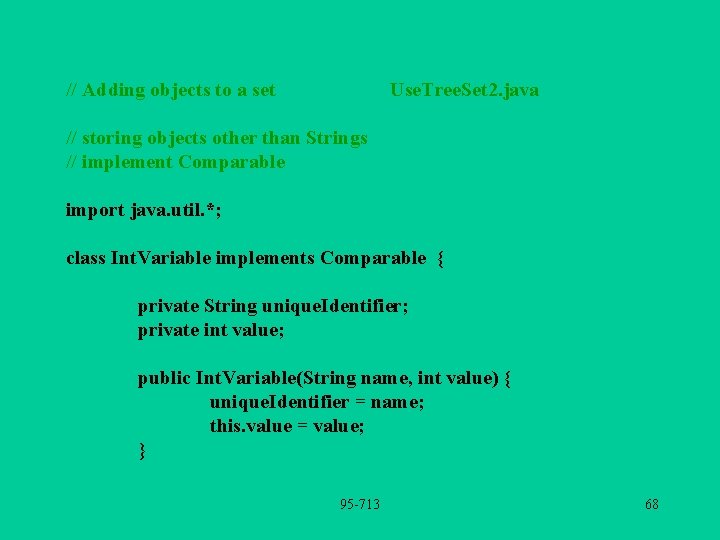
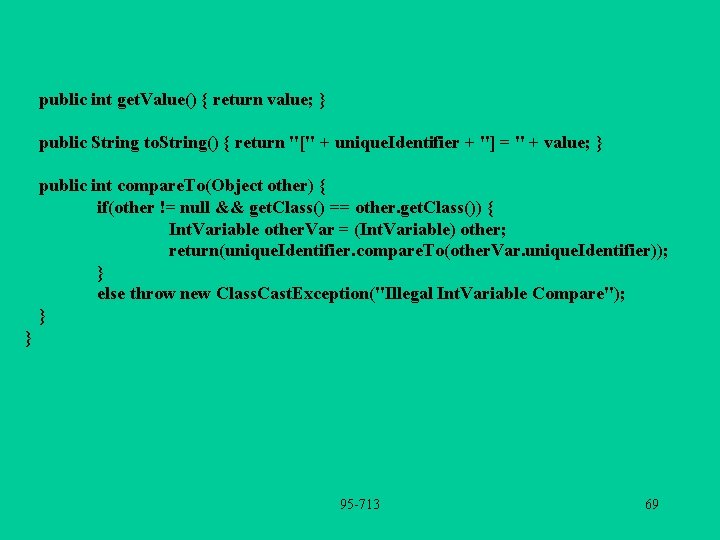
![public class Use. Tree. Set 2 { public static void main(String args[]) { Set public class Use. Tree. Set 2 { public static void main(String args[]) { Set](https://slidetodoc.com/presentation_image_h/31cf08bc45fc415bb1238376aea30ede/image-70.jpg)
![/* [X] = 23 [Y] = 45 */ 95 -713 71 /* [X] = 23 [Y] = 45 */ 95 -713 71](https://slidetodoc.com/presentation_image_h/31cf08bc45fc415bb1238376aea30ede/image-71.jpg)
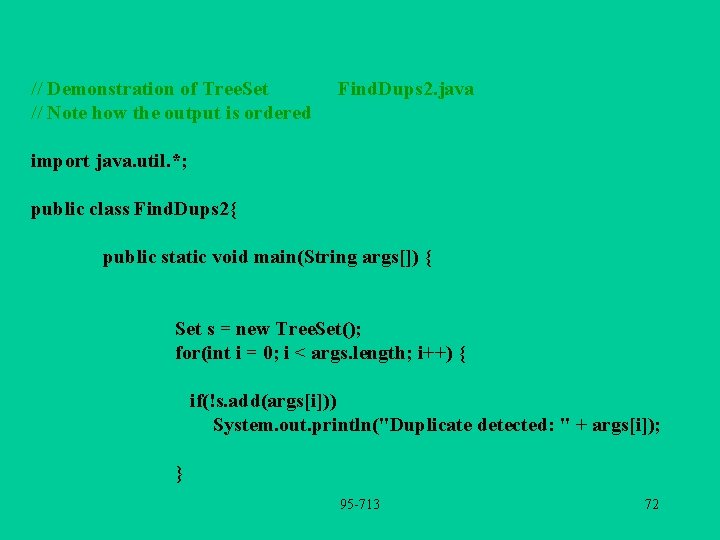
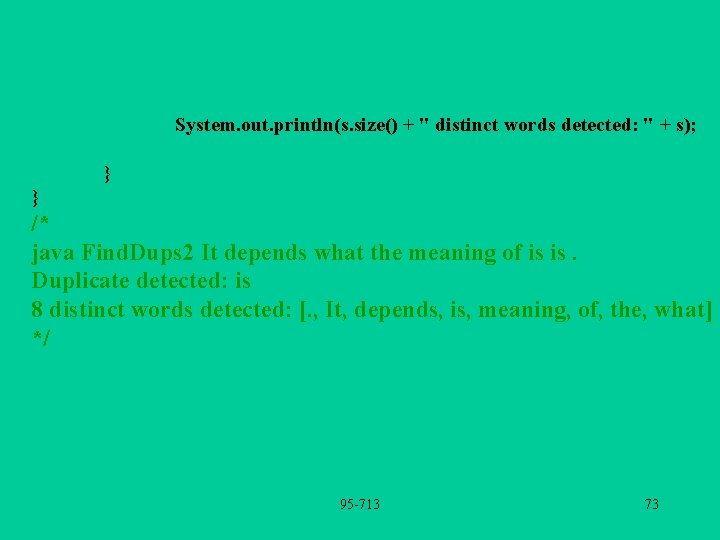
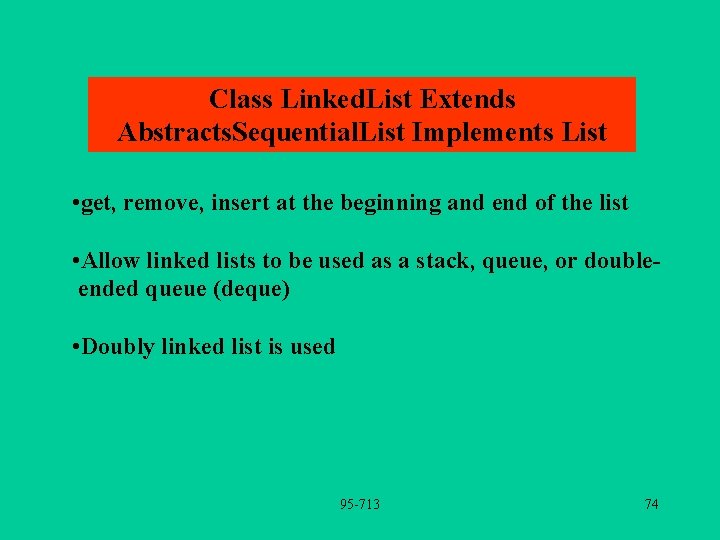
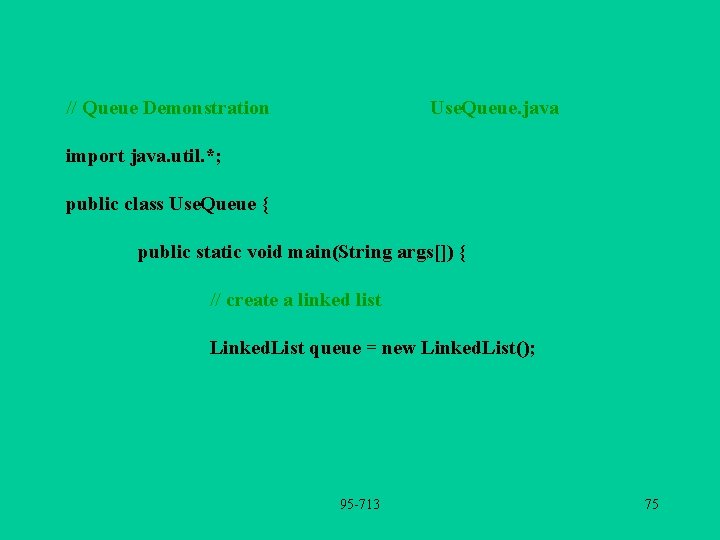
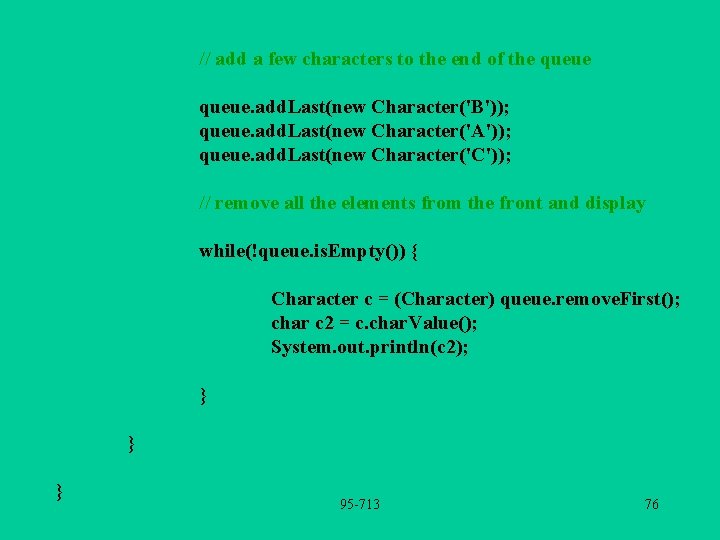
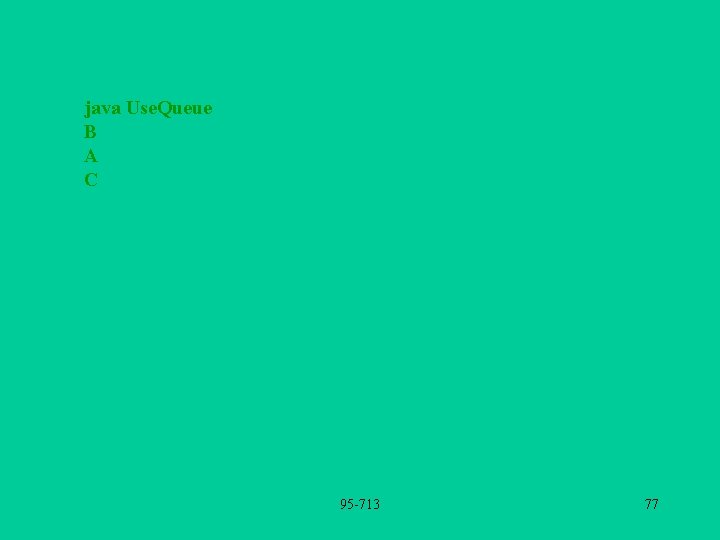
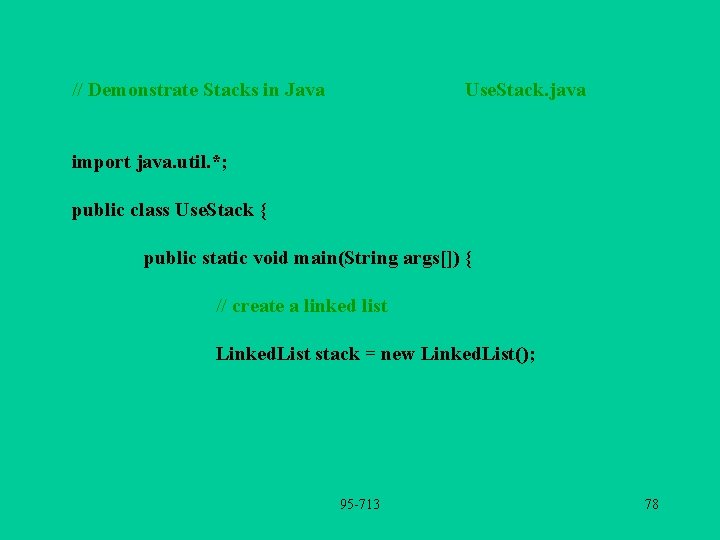
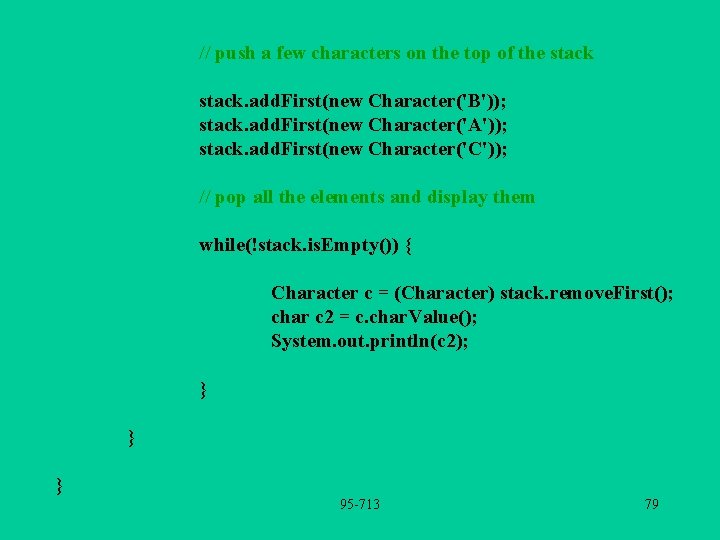
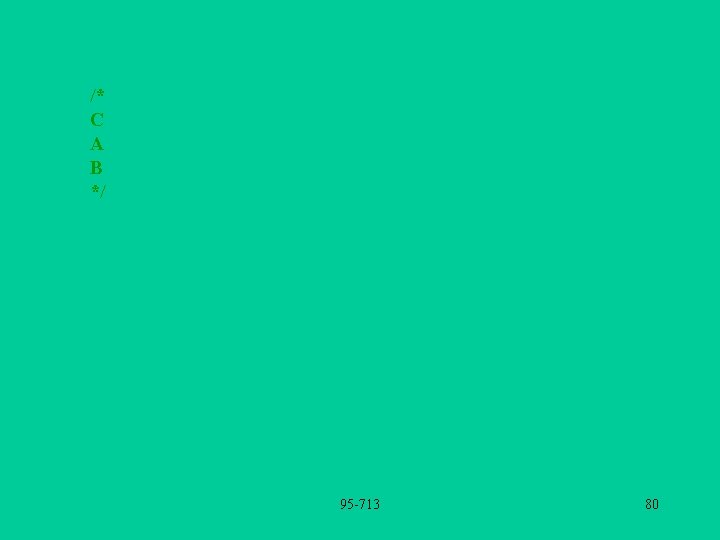
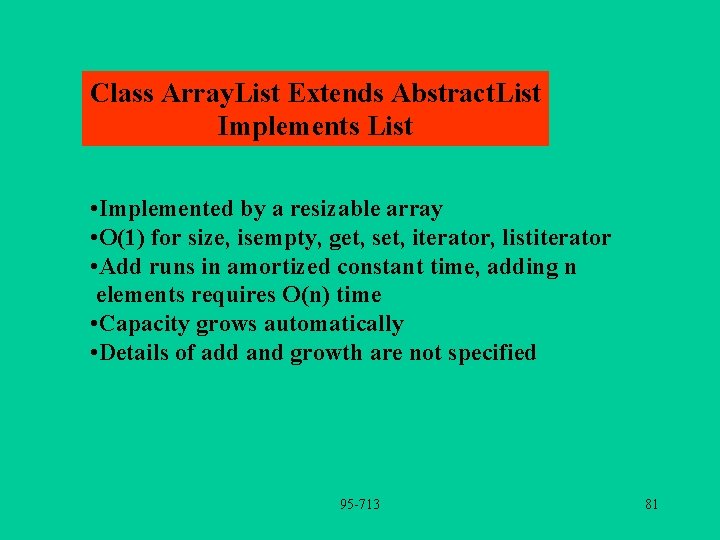
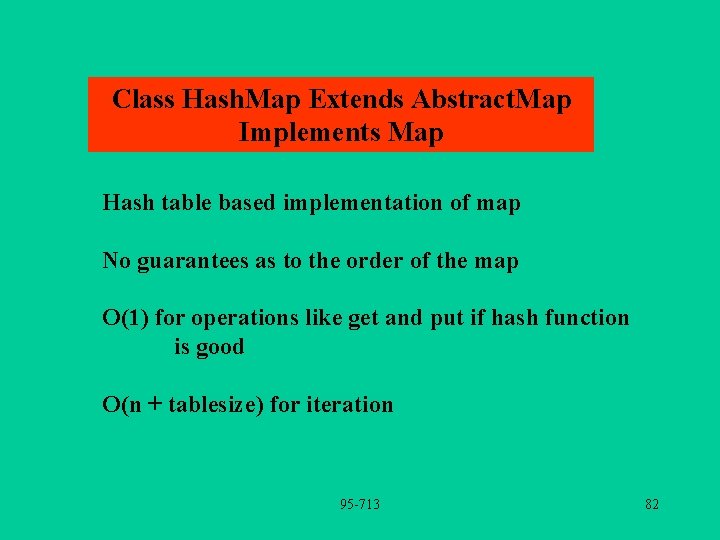
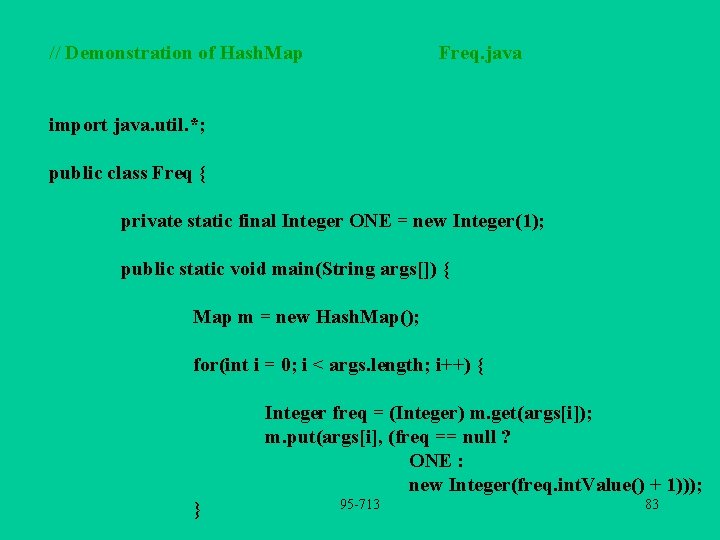
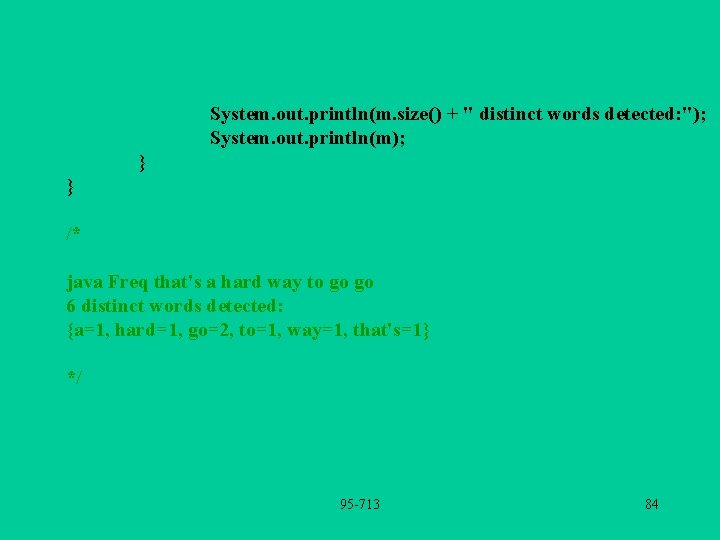
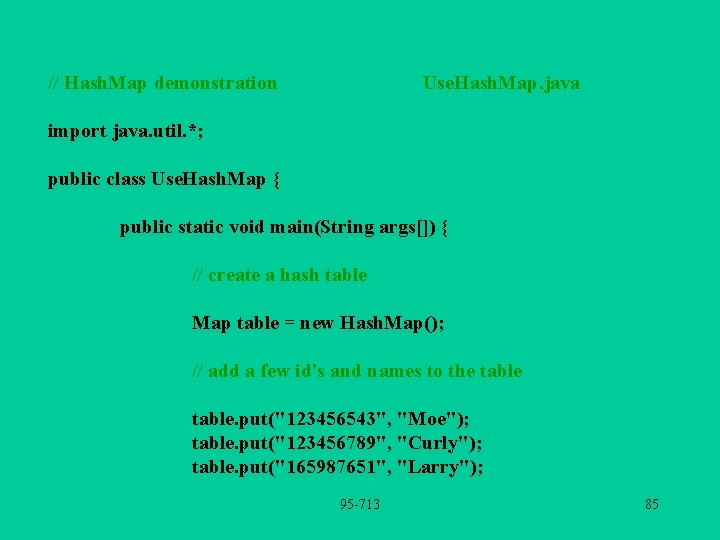
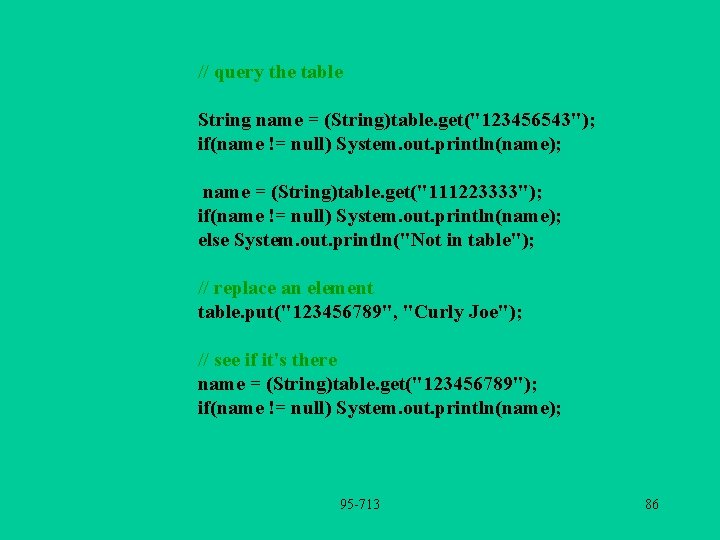
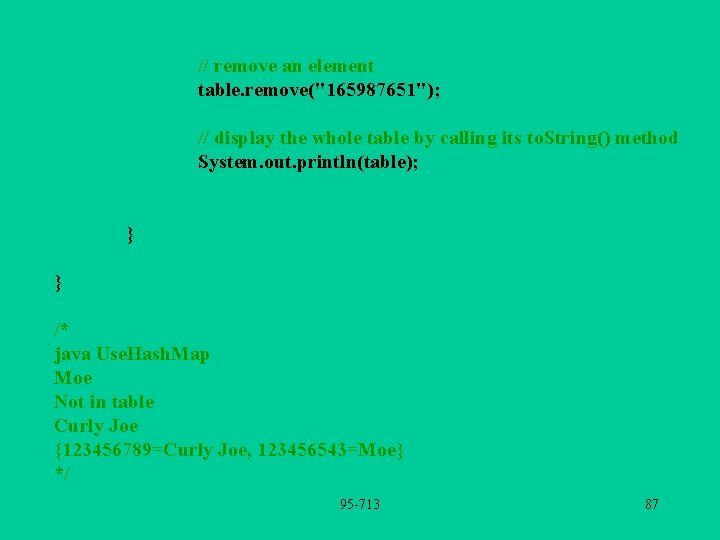
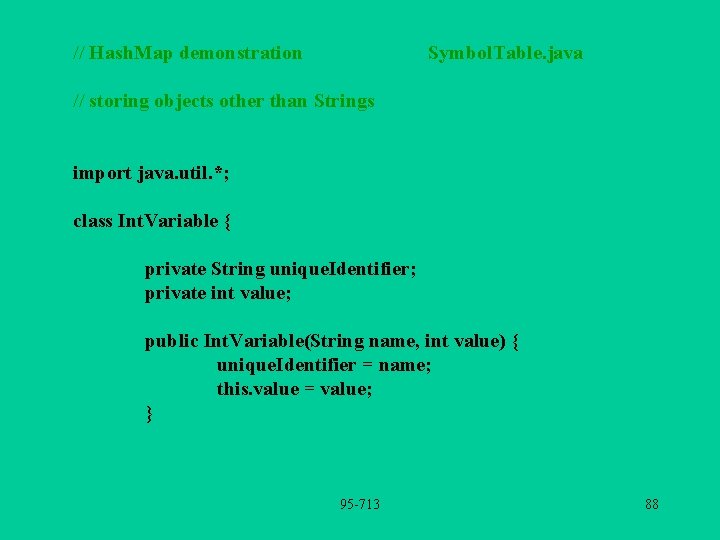
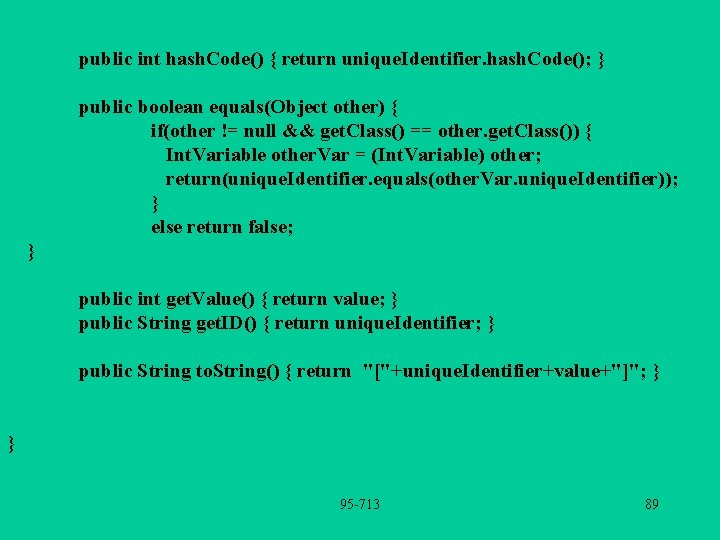
![public class Symbol. Table { public static void main(String args[]) { Map symbol. Table public class Symbol. Table { public static void main(String args[]) { Map symbol. Table](https://slidetodoc.com/presentation_image_h/31cf08bc45fc415bb1238376aea30ede/image-90.jpg)
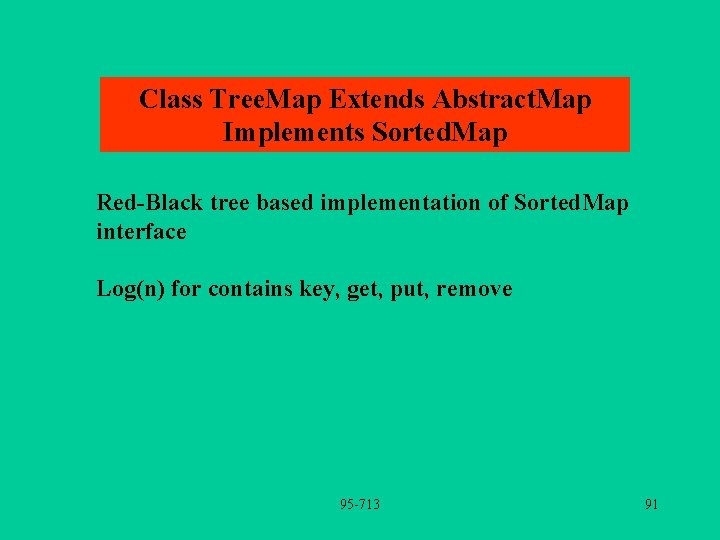
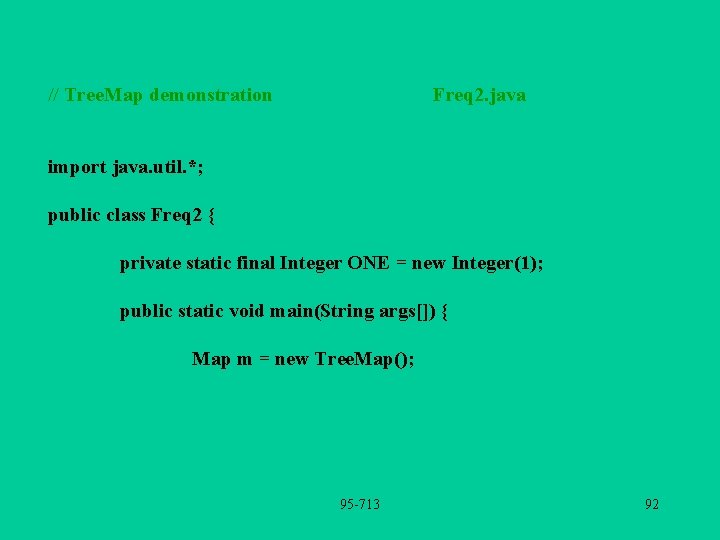
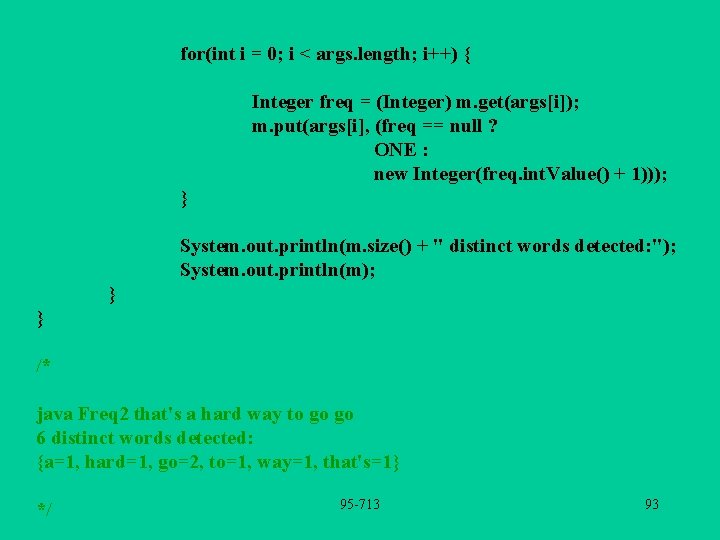
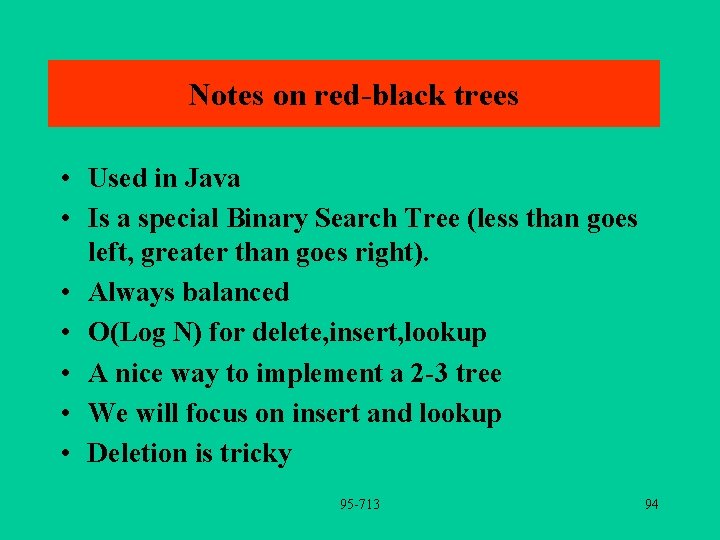
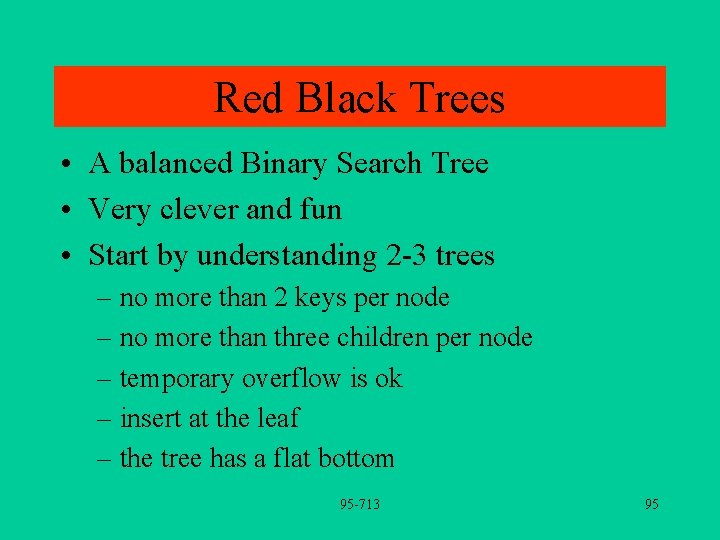
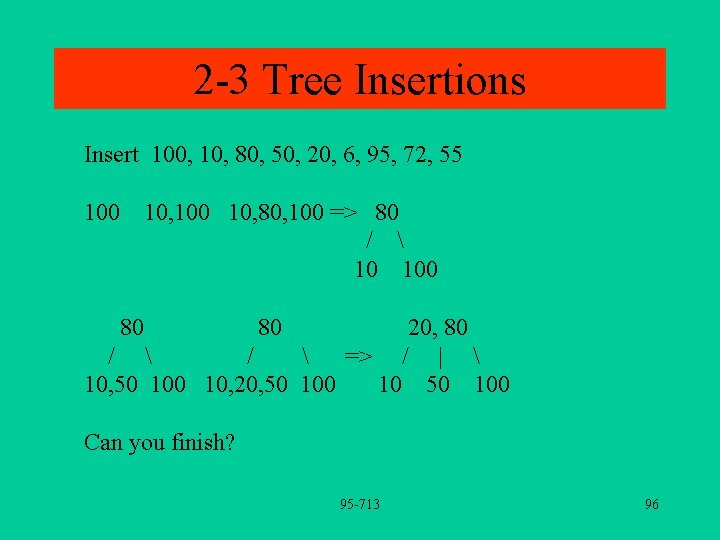
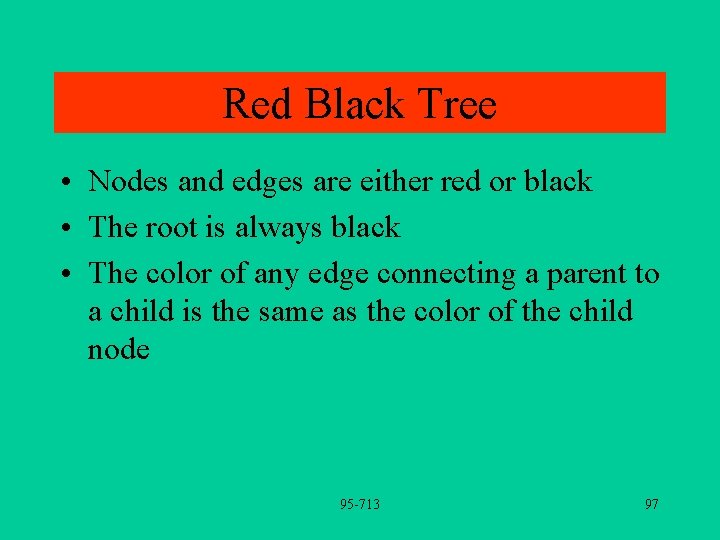
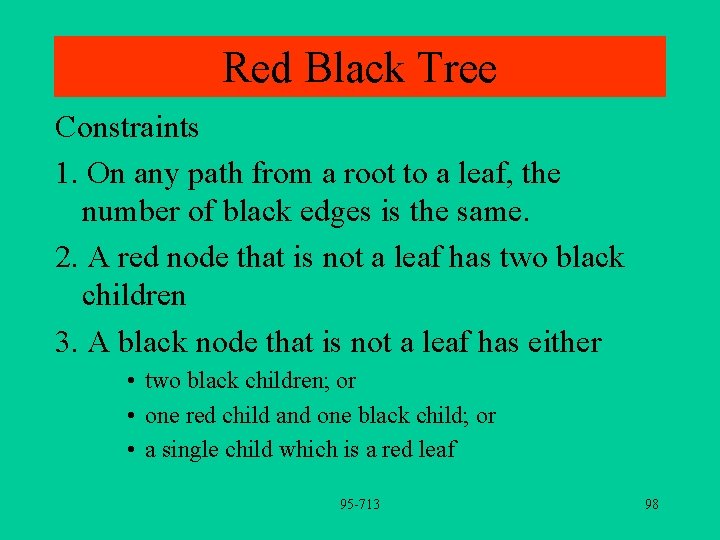
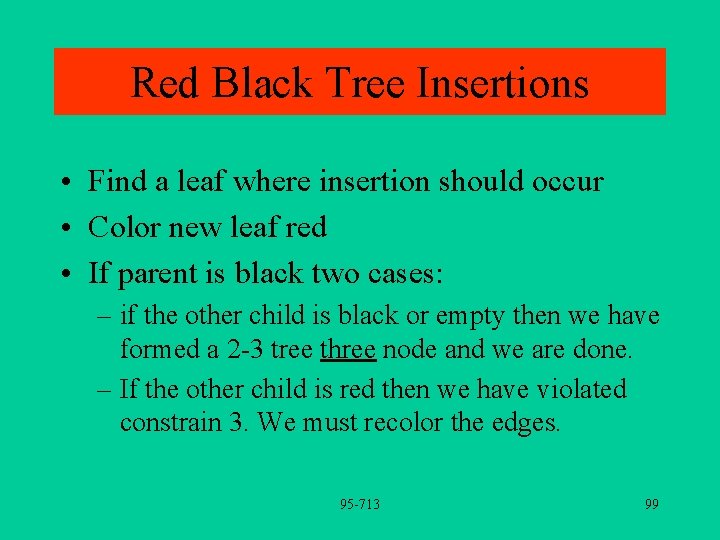
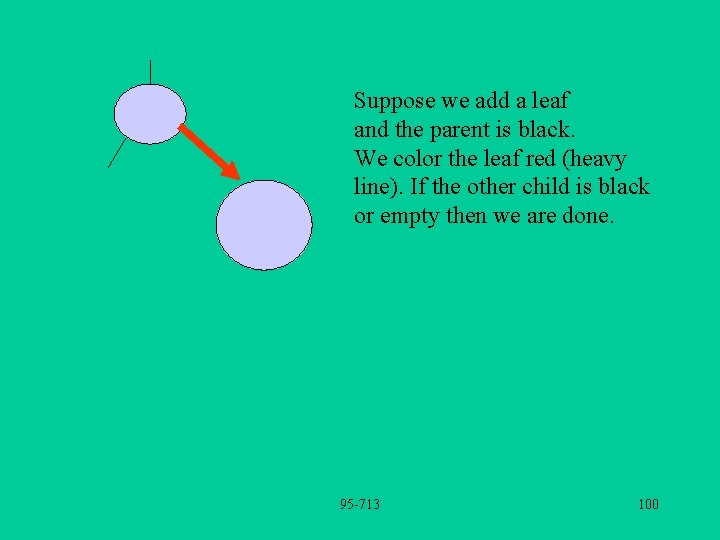
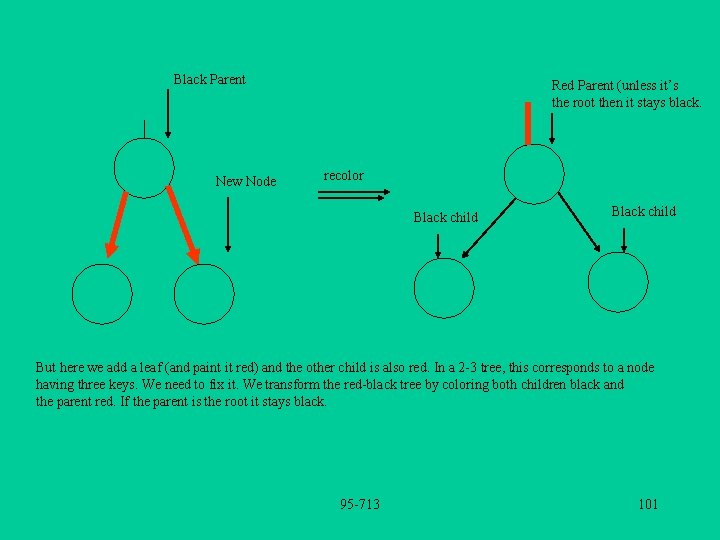
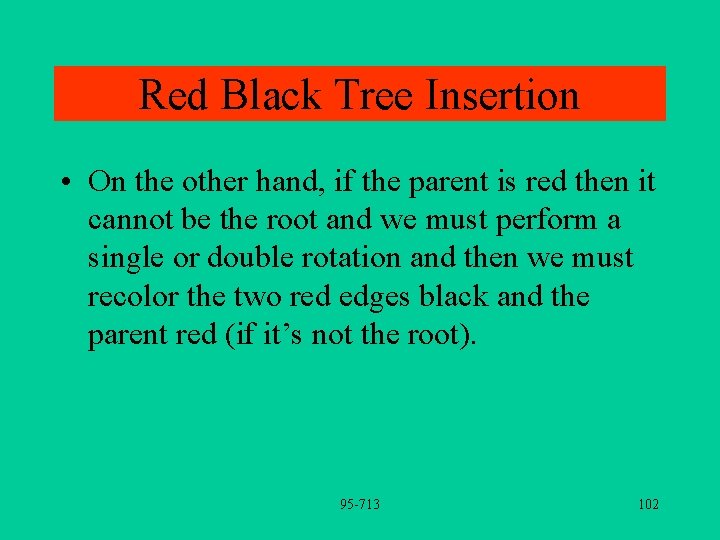
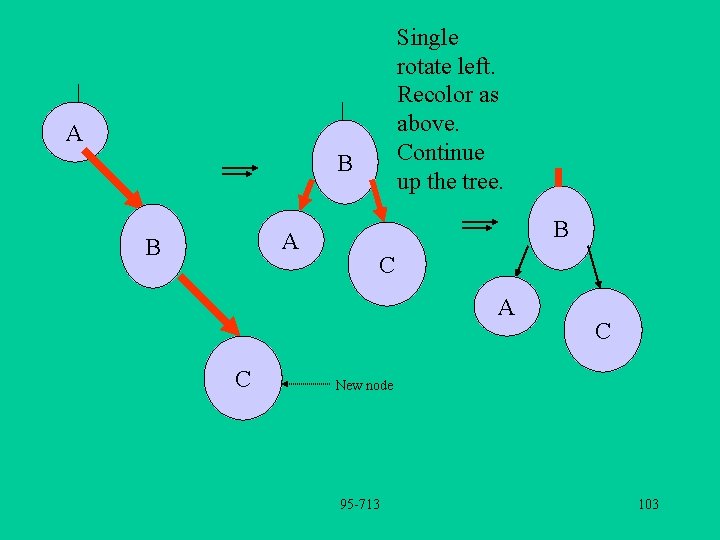
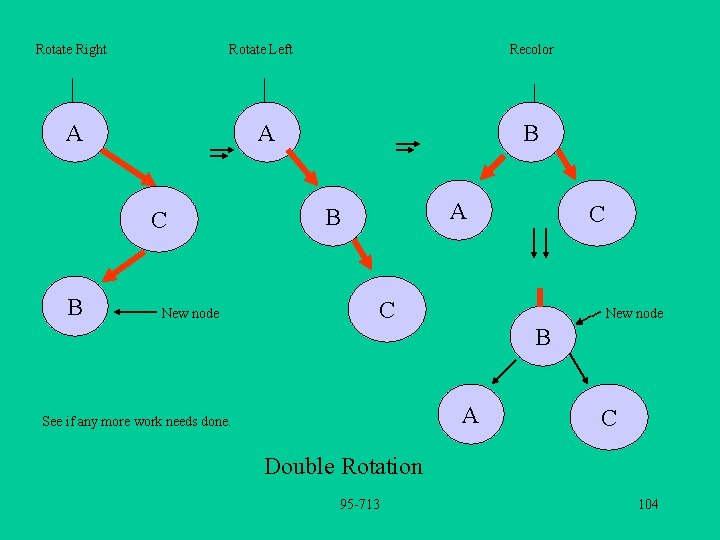
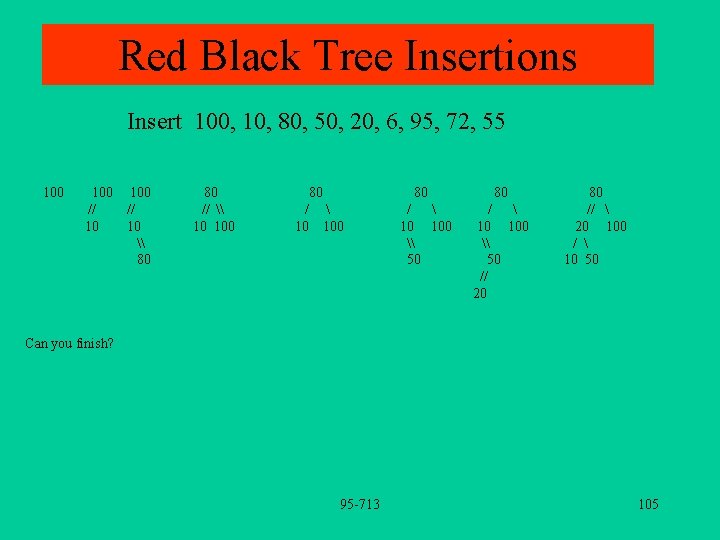
- Slides: 105
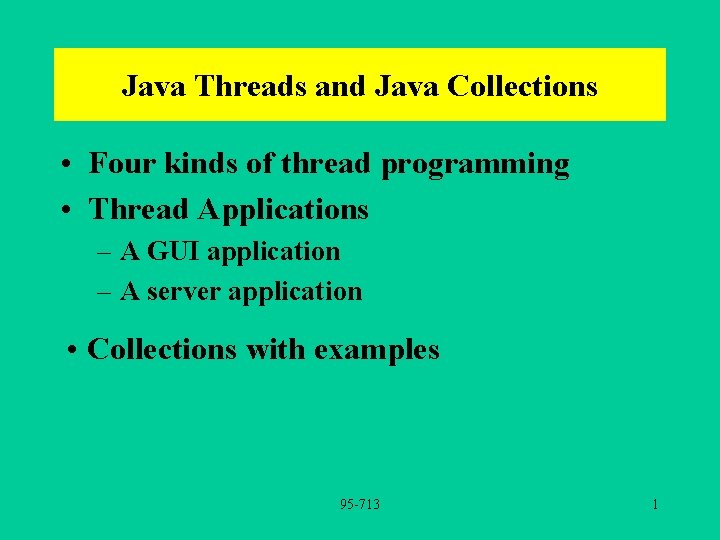
Java Threads and Java Collections • Four kinds of thread programming • Thread Applications – A GUI application – A server application • Collections with examples 95 -713 1
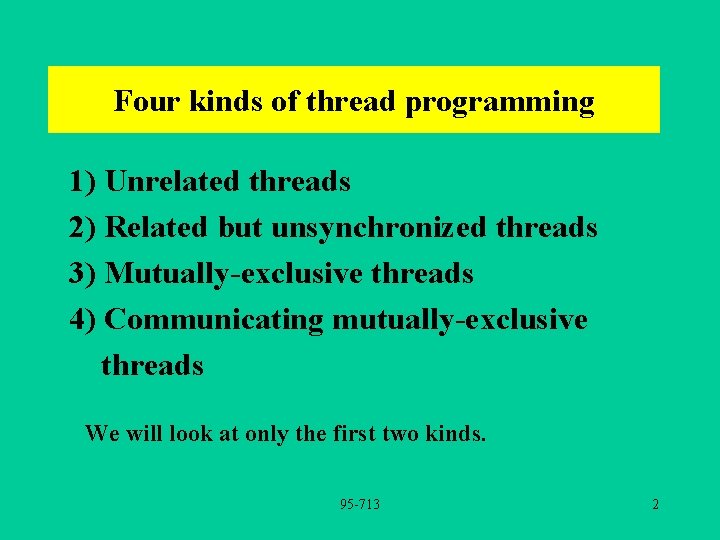
Four kinds of thread programming 1) Unrelated threads 2) Related but unsynchronized threads 3) Mutually-exclusive threads 4) Communicating mutually-exclusive threads We will look at only the first two kinds. 95 -713 2
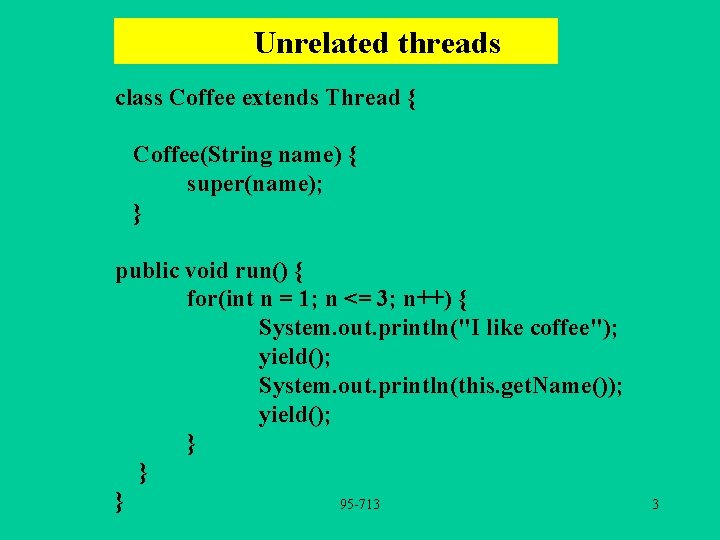
Unrelated threads class Coffee extends Thread { Coffee(String name) { super(name); } public void run() { for(int n = 1; n <= 3; n++) { System. out. println("I like coffee"); yield(); System. out. println(this. get. Name()); yield(); } } } 95 -713 3
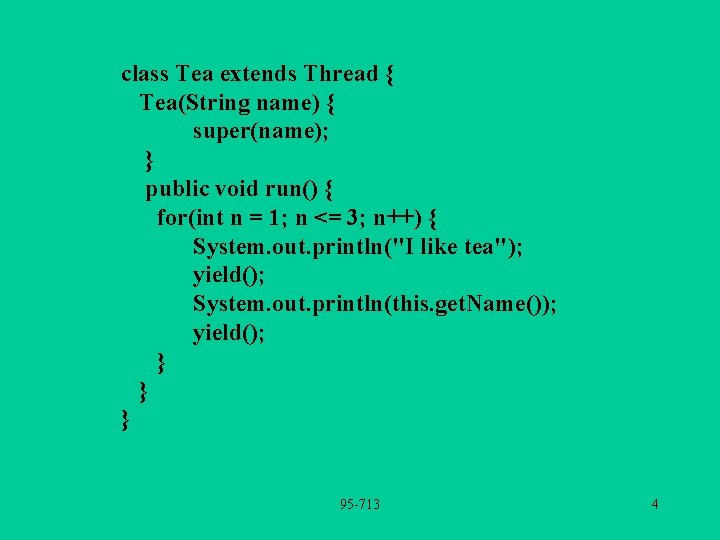
class Tea extends Thread { Tea(String name) { super(name); } public void run() { for(int n = 1; n <= 3; n++) { System. out. println("I like tea"); yield(); System. out. println(this. get. Name()); yield(); } } } 95 -713 4
![public class Drinks public static void mainString args System out printlnI am public class Drinks { public static void main(String args[]) { System. out. println("I am](https://slidetodoc.com/presentation_image_h/31cf08bc45fc415bb1238376aea30ede/image-5.jpg)
public class Drinks { public static void main(String args[]) { System. out. println("I am main"); Coffee t 1 = new Coffee("Wawa Coffee"); Tea t 2 = new Tea(“Sleepy Time Tea"); t 1. start(); t 2. start(); System. out. println("Main is done"); } } 95 -713 5
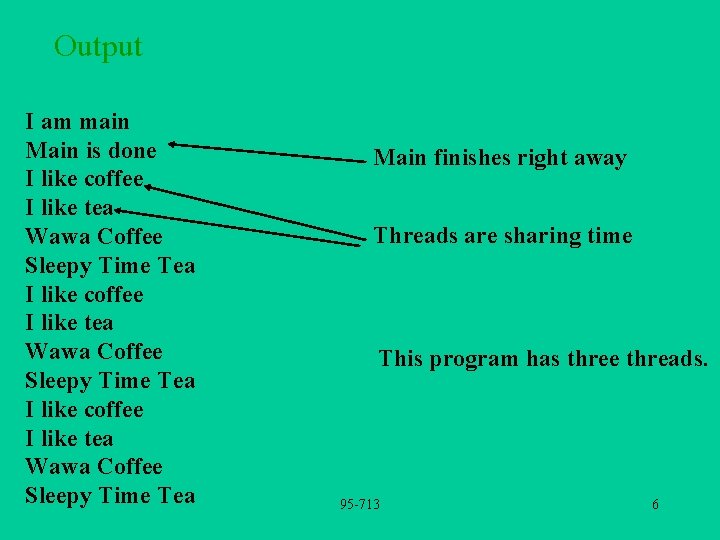
Output I am main Main is done I like coffee I like tea Wawa Coffee Sleepy Time Tea Main finishes right away Threads are sharing time This program has three threads. 95 -713 6
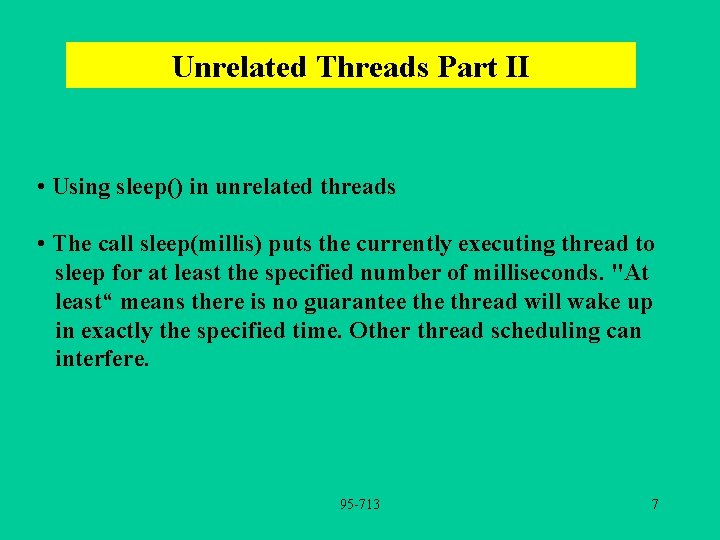
Unrelated Threads Part II • Using sleep() in unrelated threads • The call sleep(millis) puts the currently executing thread to sleep for at least the specified number of milliseconds. "At least“ means there is no guarantee thread will wake up in exactly the specified time. Other thread scheduling can interfere. 95 -713 7
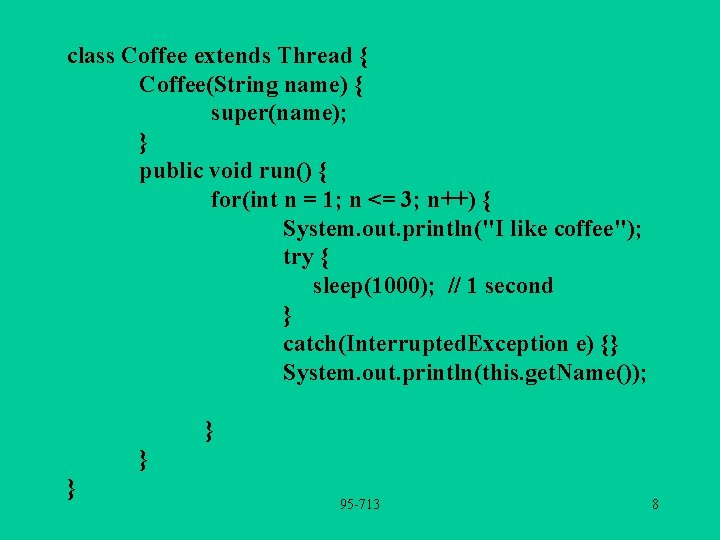
class Coffee extends Thread { Coffee(String name) { super(name); } public void run() { for(int n = 1; n <= 3; n++) { System. out. println("I like coffee"); try { sleep(1000); // 1 second } catch(Interrupted. Exception e) {} System. out. println(this. get. Name()); } } } 95 -713 8
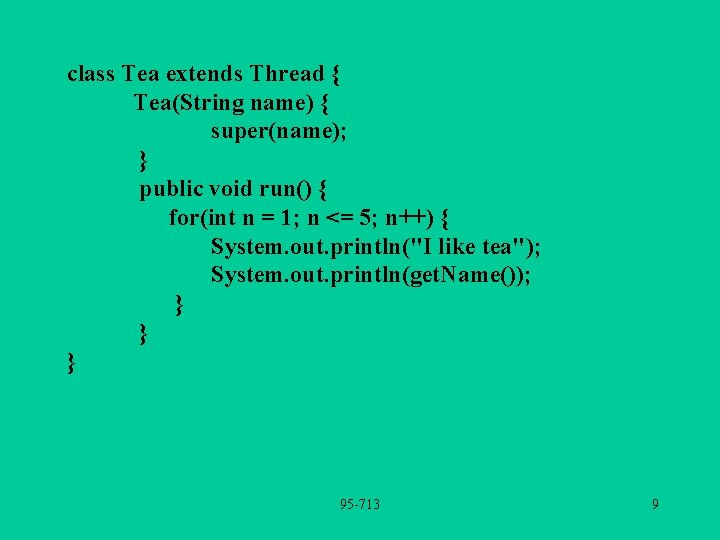
class Tea extends Thread { Tea(String name) { super(name); } public void run() { for(int n = 1; n <= 5; n++) { System. out. println("I like tea"); System. out. println(get. Name()); } } } 95 -713 9
![public class Drinks 2 public static void mainString args System out printlnI public class Drinks 2 { public static void main(String args[]) { System. out. println("I](https://slidetodoc.com/presentation_image_h/31cf08bc45fc415bb1238376aea30ede/image-10.jpg)
public class Drinks 2 { public static void main(String args[]) { System. out. println("I am main"); Coffee t 1 = new Coffee("Wawa Coffee"); Tea t 2 = new Tea("China Tea"); t 1. start(); t 2. start(); System. out. println("Main is done"); } } 95 -713 10
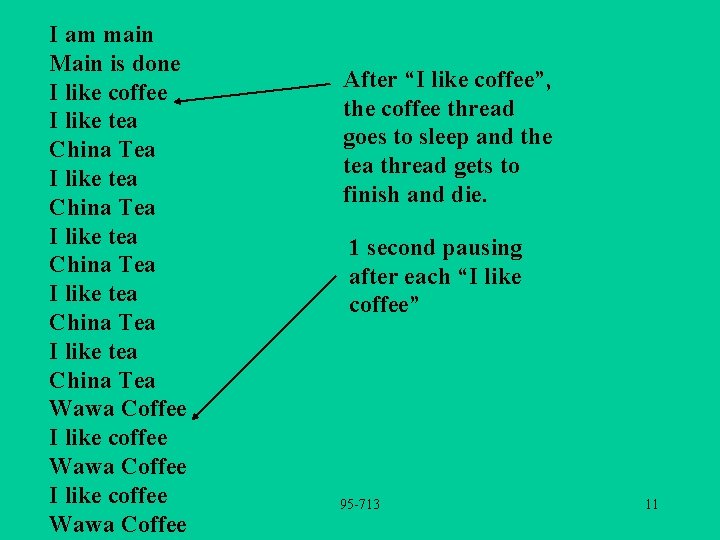
I am main Main is done I like coffee I like tea China Tea I like tea China Tea Wawa Coffee I like coffee Wawa Coffee After “I like coffee”, the coffee thread goes to sleep and the tea thread gets to finish and die. 1 second pausing after each “I like coffee” 95 -713 11
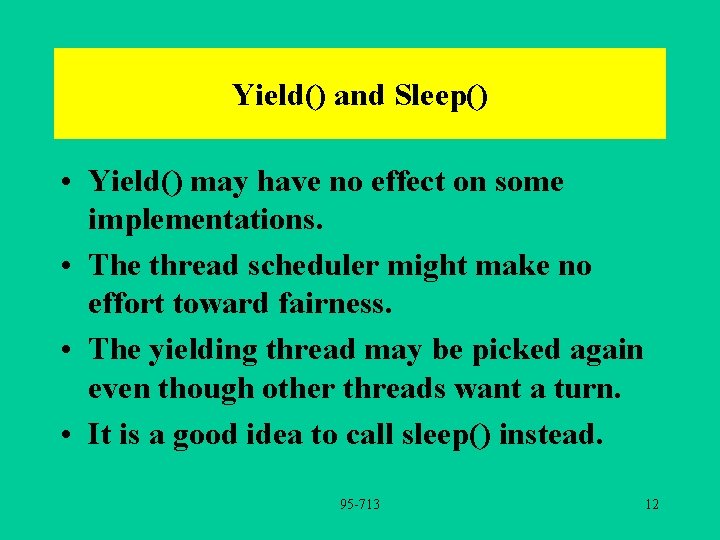
Yield() and Sleep() • Yield() may have no effect on some implementations. • The thread scheduler might make no effort toward fairness. • The yielding thread may be picked again even though other threads want a turn. • It is a good idea to call sleep() instead. 95 -713 12
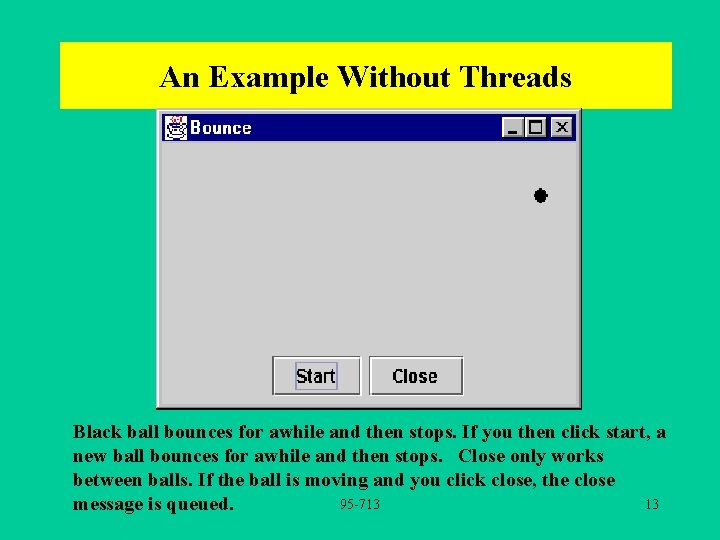
An Example Without Threads Black ball bounces for awhile and then stops. If you then click start, a new ball bounces for awhile and then stops. Close only works between balls. If the ball is moving and you click close, the close 95 -713 13 message is queued.
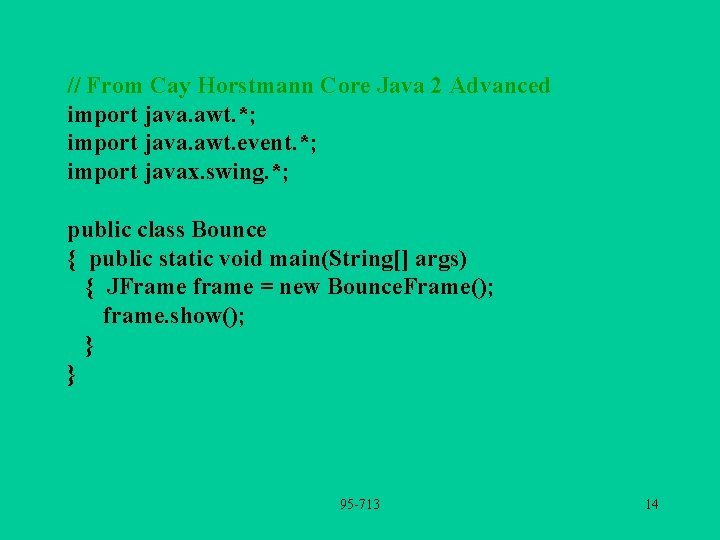
// From Cay Horstmann Core Java 2 Advanced import java. awt. *; import java. awt. event. *; import javax. swing. *; public class Bounce { public static void main(String[] args) { JFrame frame = new Bounce. Frame(); frame. show(); } } 95 -713 14
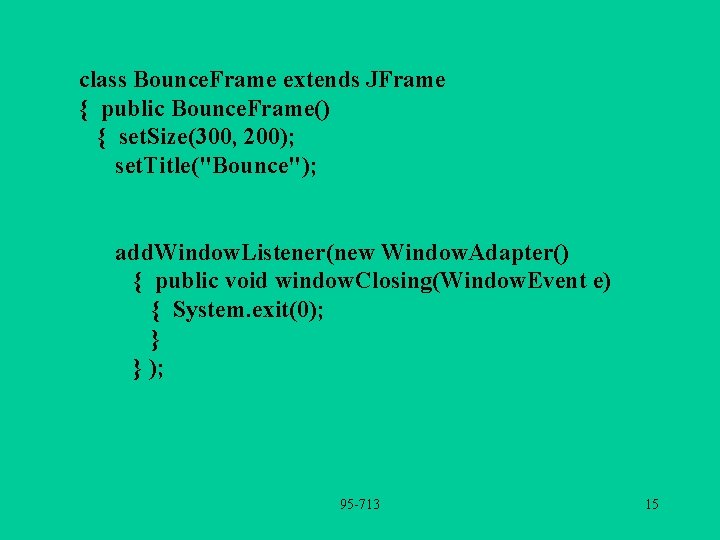
class Bounce. Frame extends JFrame { public Bounce. Frame() { set. Size(300, 200); set. Title("Bounce"); add. Window. Listener(new Window. Adapter() { public void window. Closing(Window. Event e) { System. exit(0); } } ); 95 -713 15
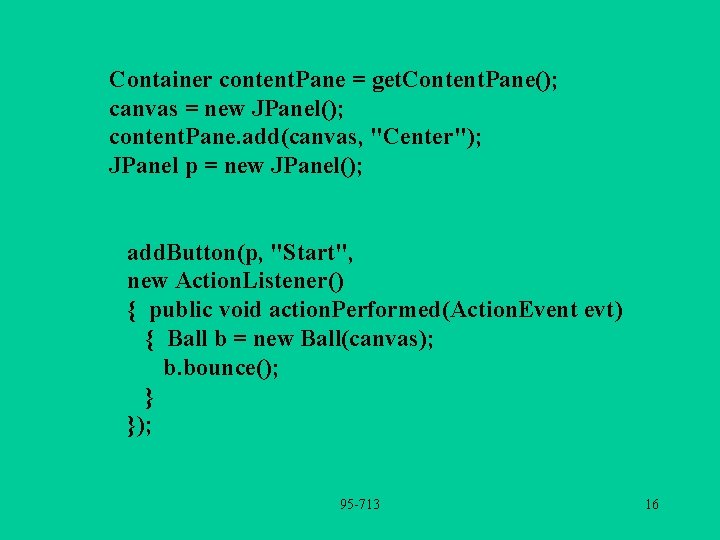
Container content. Pane = get. Content. Pane(); canvas = new JPanel(); content. Pane. add(canvas, "Center"); JPanel p = new JPanel(); add. Button(p, "Start", new Action. Listener() { public void action. Performed(Action. Event evt) { Ball b = new Ball(canvas); b. bounce(); } }); 95 -713 16
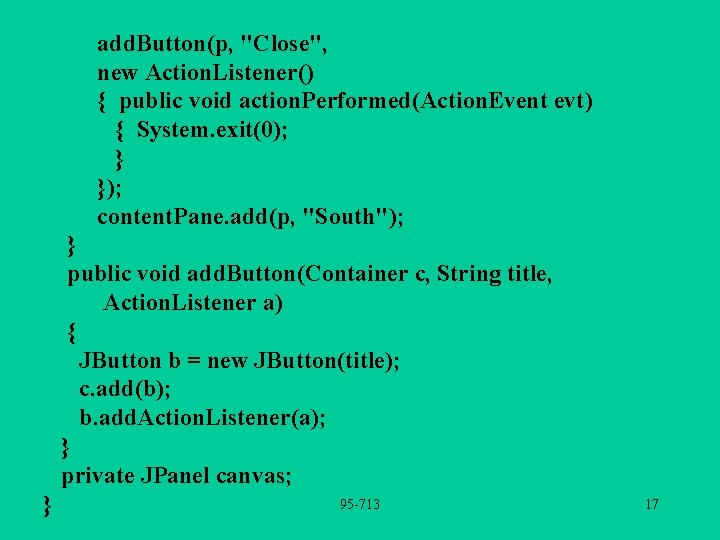
add. Button(p, "Close", new Action. Listener() { public void action. Performed(Action. Event evt) { System. exit(0); } }); content. Pane. add(p, "South"); } public void add. Button(Container c, String title, Action. Listener a) { JButton b = new JButton(title); c. add(b); b. add. Action. Listener(a); } private JPanel canvas; } 95 -713 17
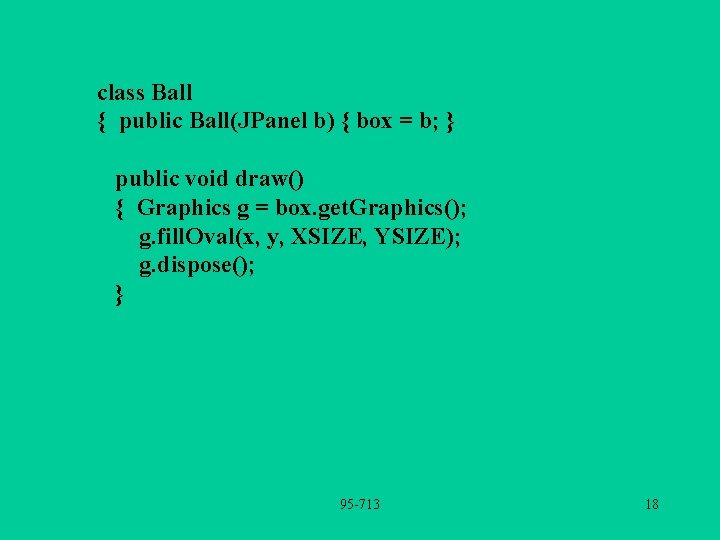
class Ball { public Ball(JPanel b) { box = b; } public void draw() { Graphics g = box. get. Graphics(); g. fill. Oval(x, y, XSIZE, YSIZE); g. dispose(); } 95 -713 18
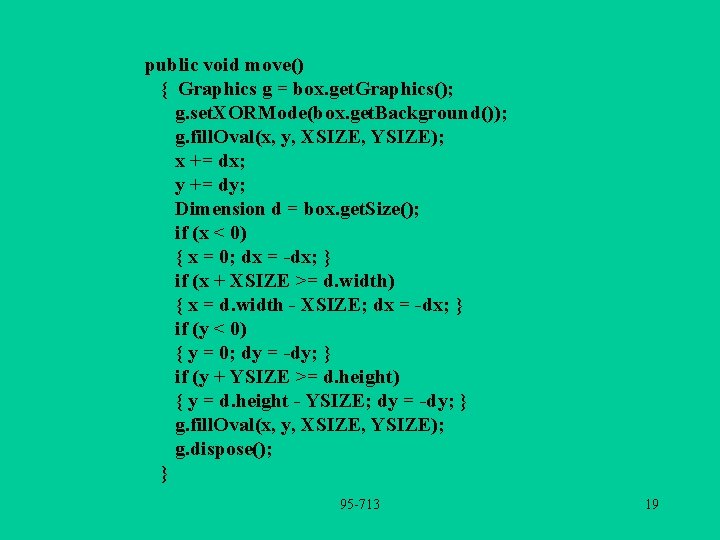
public void move() { Graphics g = box. get. Graphics(); g. set. XORMode(box. get. Background()); g. fill. Oval(x, y, XSIZE, YSIZE); x += dx; y += dy; Dimension d = box. get. Size(); if (x < 0) { x = 0; dx = -dx; } if (x + XSIZE >= d. width) { x = d. width - XSIZE; dx = -dx; } if (y < 0) { y = 0; dy = -dy; } if (y + YSIZE >= d. height) { y = d. height - YSIZE; dy = -dy; } g. fill. Oval(x, y, XSIZE, YSIZE); g. dispose(); } 95 -713 19
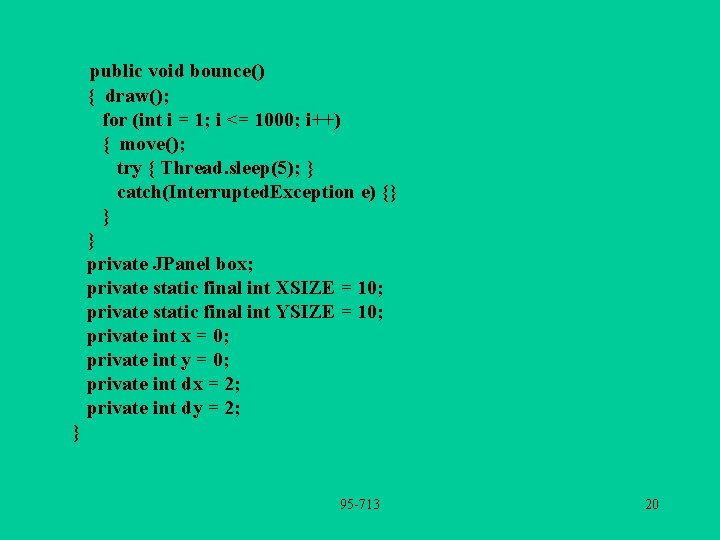
public void bounce() { draw(); for (int i = 1; i <= 1000; i++) { move(); try { Thread. sleep(5); } catch(Interrupted. Exception e) {} } } private JPanel box; private static final int XSIZE = 10; private static final int YSIZE = 10; private int x = 0; private int y = 0; private int dx = 2; private int dy = 2; } 95 -713 20
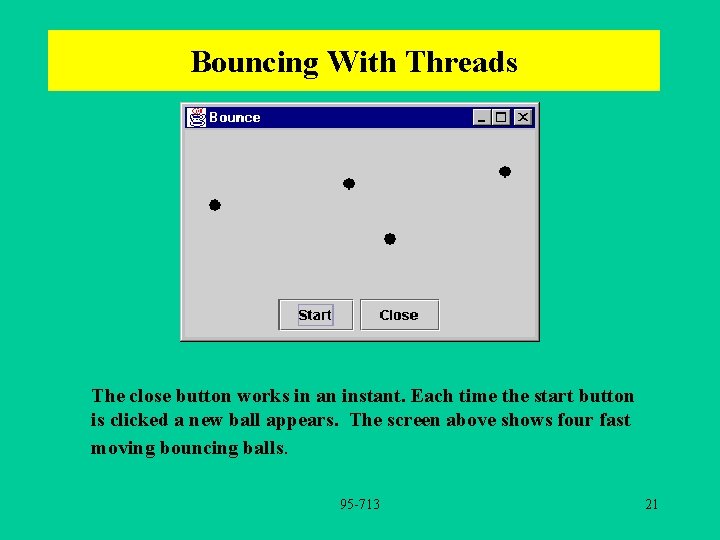
Bouncing With Threads The close button works in an instant. Each time the start button is clicked a new ball appears. The screen above shows four fast moving bouncing balls. 95 -713 21
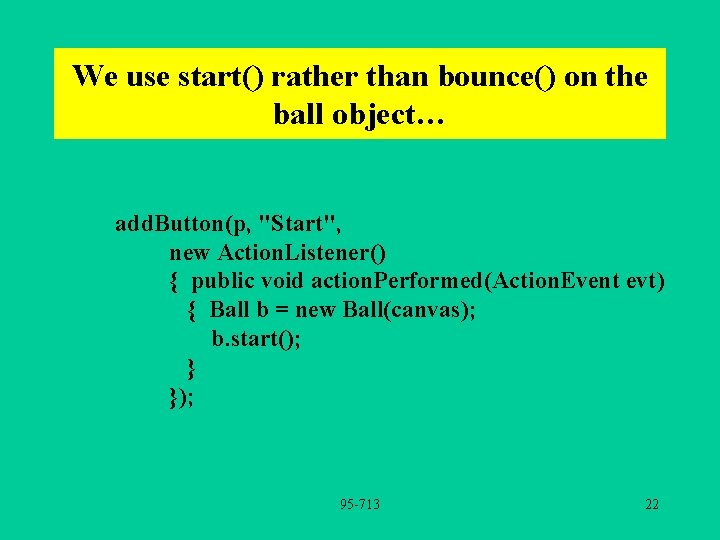
We use start() rather than bounce() on the ball object… add. Button(p, "Start", new Action. Listener() { public void action. Performed(Action. Event evt) { Ball b = new Ball(canvas); b. start(); } }); 95 -713 22
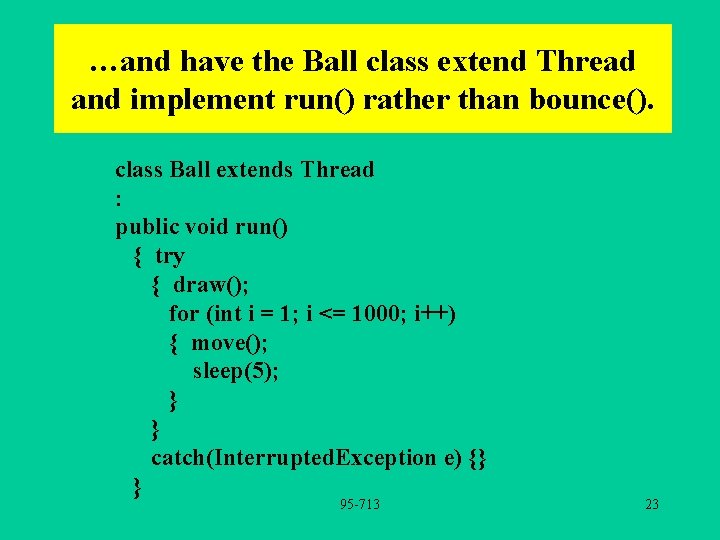
…and have the Ball class extend Thread and implement run() rather than bounce(). class Ball extends Thread : public void run() { try { draw(); for (int i = 1; i <= 1000; i++) { move(); sleep(5); } } catch(Interrupted. Exception e) {} } 95 -713 23
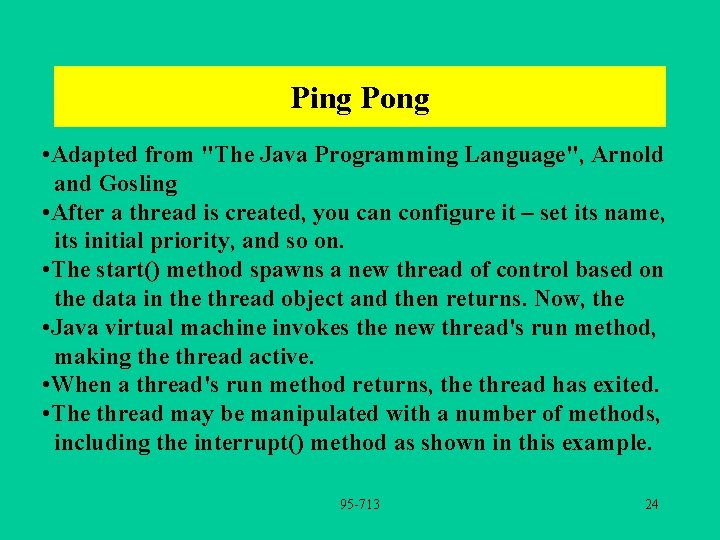
Ping Pong • Adapted from "The Java Programming Language", Arnold and Gosling • After a thread is created, you can configure it – set its name, its initial priority, and so on. • The start() method spawns a new thread of control based on the data in the thread object and then returns. Now, the • Java virtual machine invokes the new thread's run method, making the thread active. • When a thread's run method returns, the thread has exited. • The thread may be manipulated with a number of methods, including the interrupt() method as shown in this example. 95 -713 24
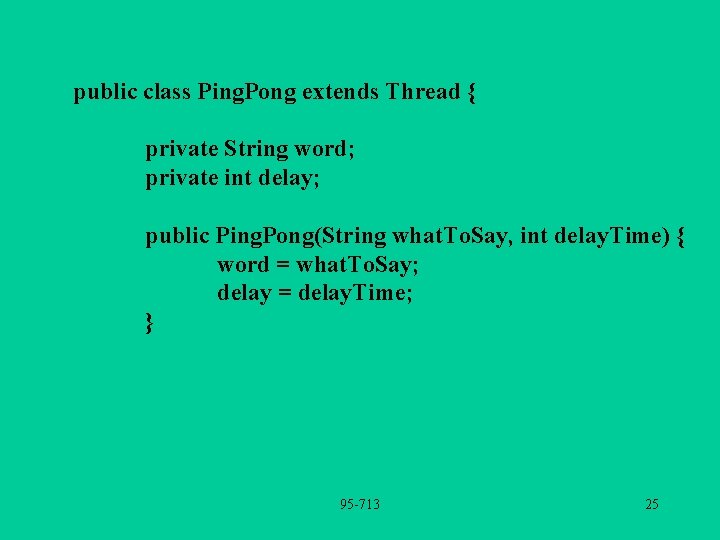
public class Ping. Pong extends Thread { private String word; private int delay; public Ping. Pong(String what. To. Say, int delay. Time) { word = what. To. Say; delay = delay. Time; } 95 -713 25
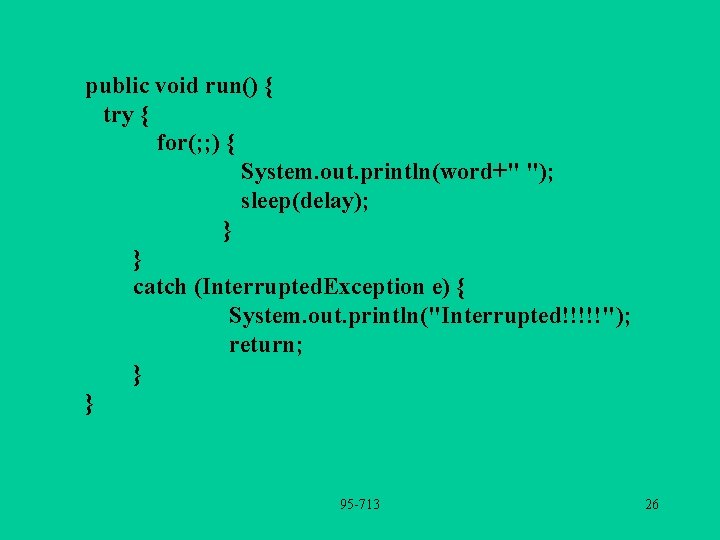
public void run() { try { for(; ; ) { System. out. println(word+" "); sleep(delay); } } catch (Interrupted. Exception e) { System. out. println("Interrupted!!!!!"); return; } } 95 -713 26
![public static void mainString args Ping Pong t 1 new Ping Pongtping public static void main(String args[]) { Ping. Pong t 1 = new Ping. Pong("tping",](https://slidetodoc.com/presentation_image_h/31cf08bc45fc415bb1238376aea30ede/image-27.jpg)
public static void main(String args[]) { Ping. Pong t 1 = new Ping. Pong("tping", 33); t 1. start(); Ping. Pong t 2 = new Ping. Pong("Pong", 100); t 2. start(); try { Thread. sleep(5000); } catch(Interrupted. Exception e) { // will not be printed System. out. println("Good morning"); return; } 95 -713 27
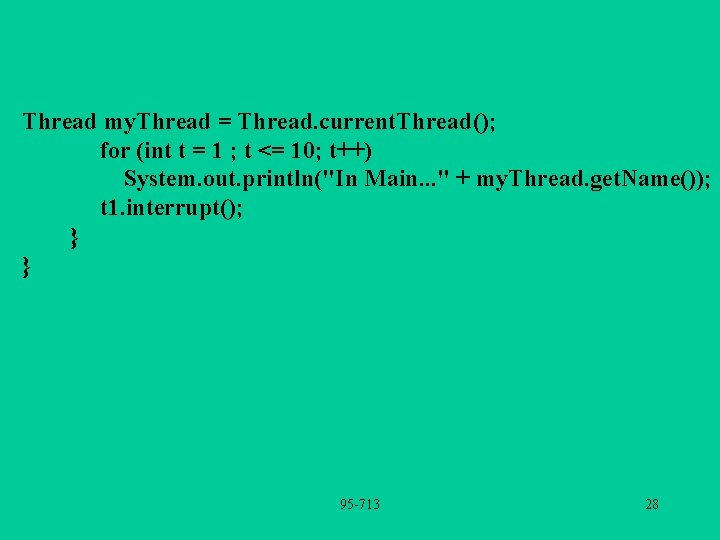
Thread my. Thread = Thread. current. Thread(); for (int t = 1 ; t <= 10; t++) System. out. println("In Main. . . " + my. Thread. get. Name()); t 1. interrupt(); } } 95 -713 28
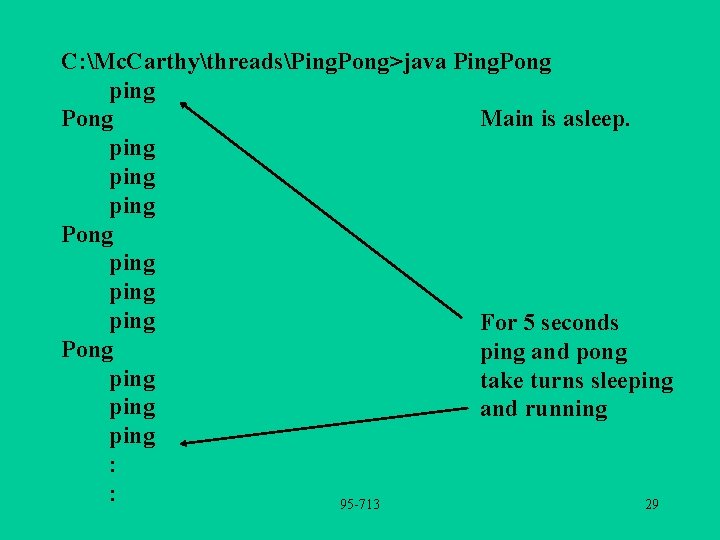
C: Mc. CarthythreadsPing. Pong>java Ping. Pong ping Main is asleep. Pong ping ping For 5 seconds Pong ping and pong ping take turns sleeping and running ping : : 95 -713 29
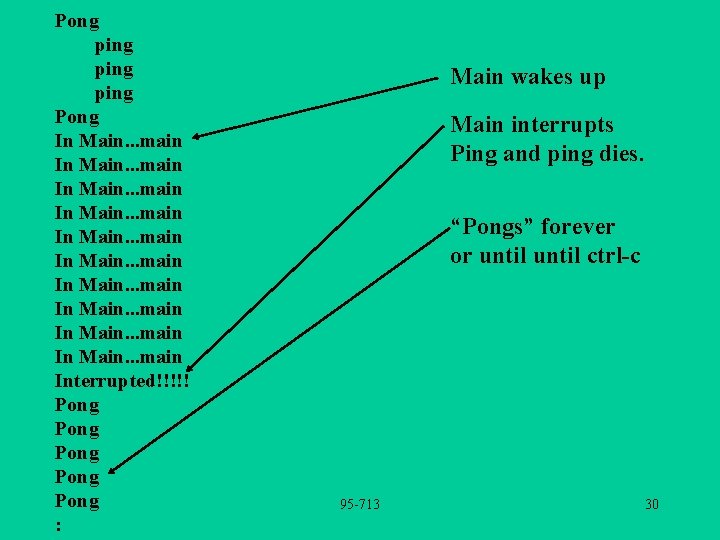
Pong ping Pong In Main. . . main In Main. . . main Interrupted!!!!! Pong Pong : Main wakes up Main interrupts Ping and ping dies. “Pongs” forever or until ctrl-c 95 -713 30
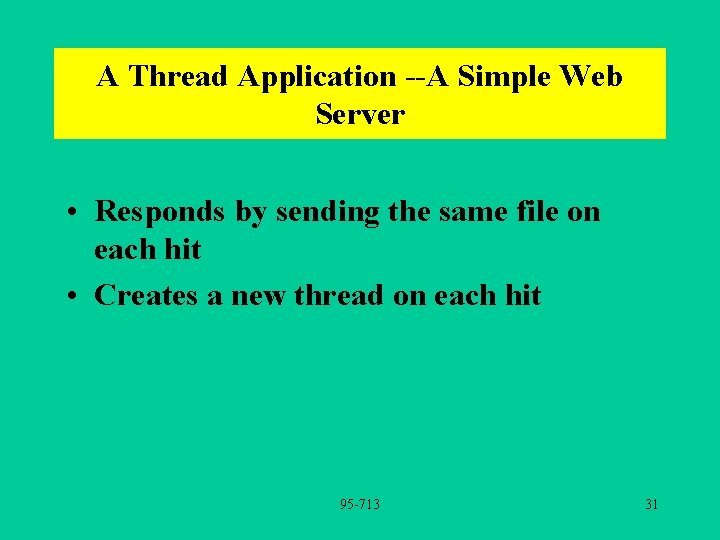
A Thread Application --A Simple Web Server • Responds by sending the same file on each hit • Creates a new thread on each hit 95 -713 31
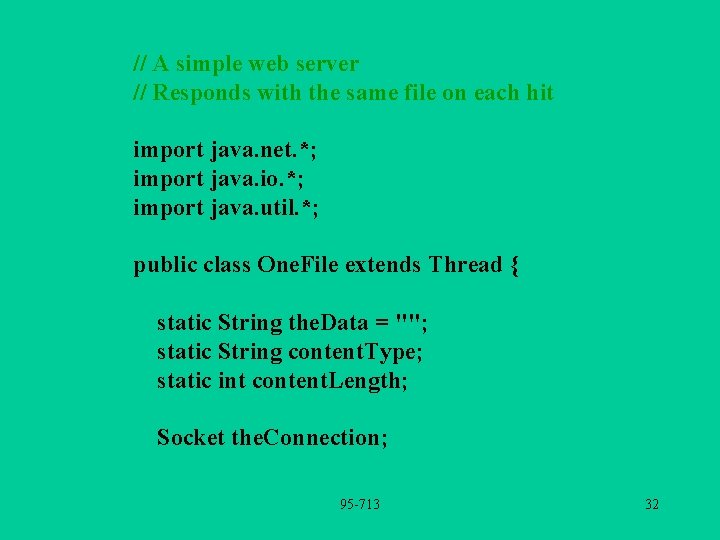
// A simple web server // Responds with the same file on each hit import java. net. *; import java. io. *; import java. util. *; public class One. File extends Thread { static String the. Data = ""; static String content. Type; static int content. Length; Socket the. Connection; 95 -713 32
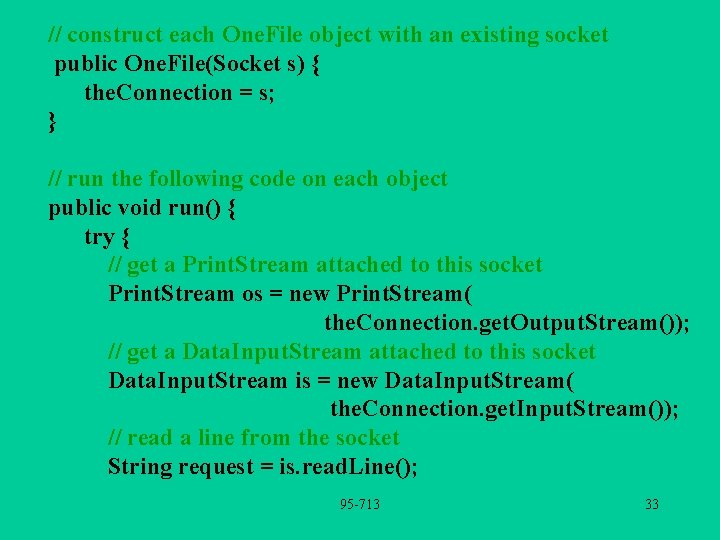
// construct each One. File object with an existing socket public One. File(Socket s) { the. Connection = s; } // run the following code on each object public void run() { try { // get a Print. Stream attached to this socket Print. Stream os = new Print. Stream( the. Connection. get. Output. Stream()); // get a Data. Input. Stream attached to this socket Data. Input. Stream is = new Data. Input. Stream( the. Connection. get. Input. Stream()); // read a line from the socket String request = is. read. Line(); 95 -713 33
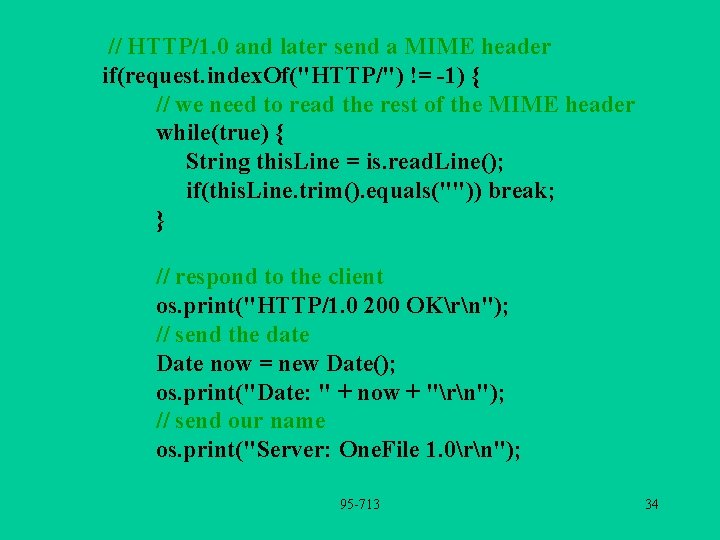
// HTTP/1. 0 and later send a MIME header if(request. index. Of("HTTP/") != -1) { // we need to read the rest of the MIME header while(true) { String this. Line = is. read. Line(); if(this. Line. trim(). equals("")) break; } // respond to the client os. print("HTTP/1. 0 200 OKrn"); // send the date Date now = new Date(); os. print("Date: " + now + "rn"); // send our name os. print("Server: One. File 1. 0rn"); 95 -713 34
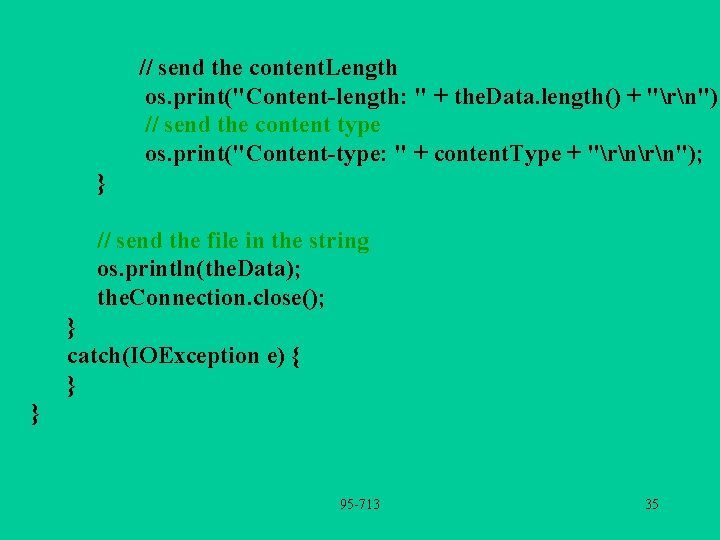
// send the content. Length os. print("Content-length: " + the. Data. length() + "rn"); // send the content type os. print("Content-type: " + content. Type + "rn"); } // send the file in the string os. println(the. Data); the. Connection. close(); } catch(IOException e) { } } 95 -713 35
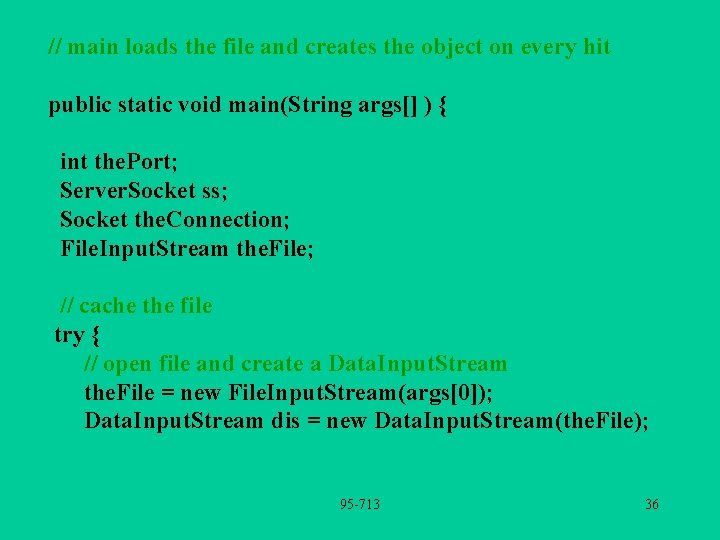
// main loads the file and creates the object on every hit public static void main(String args[] ) { int the. Port; Server. Socket ss; Socket the. Connection; File. Input. Stream the. File; // cache the file try { // open file and create a Data. Input. Stream the. File = new File. Input. Stream(args[0]); Data. Input. Stream dis = new Data. Input. Stream(the. File); 95 -713 36
![determine the content type of this file ifargs0 ends With html } // determine the content type of this file if(args[0]. ends. With(". html") ||](https://slidetodoc.com/presentation_image_h/31cf08bc45fc415bb1238376aea30ede/image-37.jpg)
} // determine the content type of this file if(args[0]. ends. With(". html") || args[0]. ends. With(". htm") ) { content. Type = "text/html"; } else { content. Type = "text/plain"; } // read the file into the string the. Data try { String this. Line; while((this. Line = dis. read. Line()) != null) { the. Data += this. Line + "n"; } } catch(Exception e) { System. err. println("Error " + e); } 95 -713 37
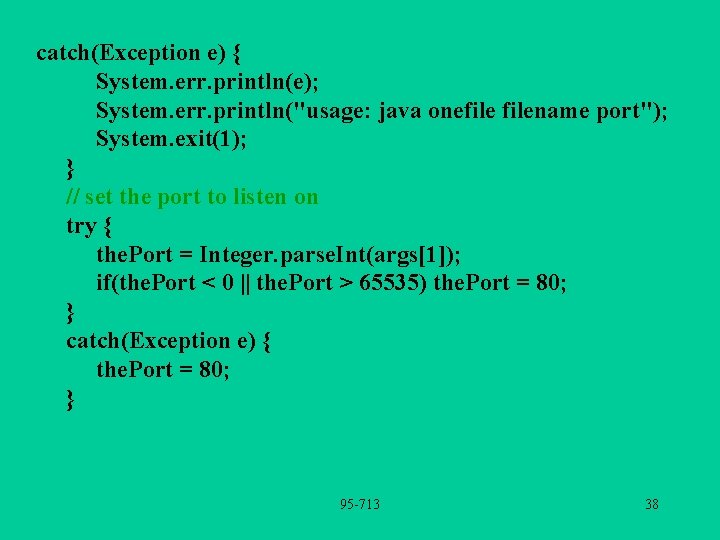
catch(Exception e) { System. err. println(e); System. err. println("usage: java onefilename port"); System. exit(1); } // set the port to listen on try { the. Port = Integer. parse. Int(args[1]); if(the. Port < 0 || the. Port > 65535) the. Port = 80; } catch(Exception e) { the. Port = 80; } 95 -713 38
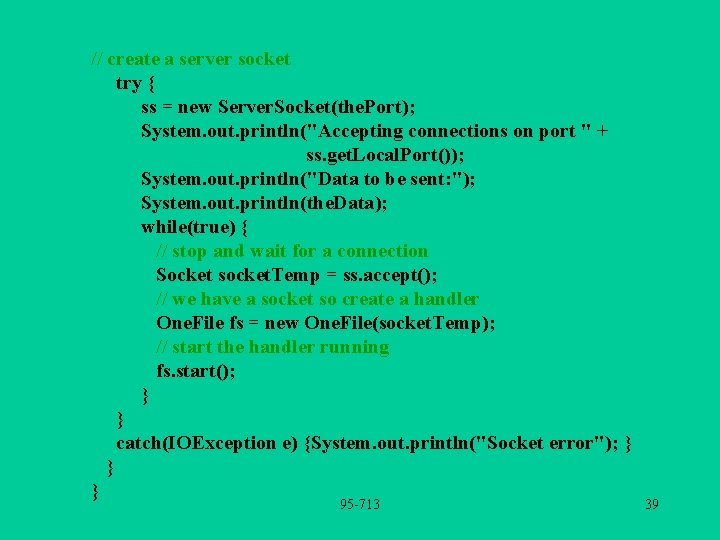
// create a server socket try { ss = new Server. Socket(the. Port); System. out. println("Accepting connections on port " + ss. get. Local. Port()); System. out. println("Data to be sent: "); System. out. println(the. Data); while(true) { // stop and wait for a connection Socket socket. Temp = ss. accept(); // we have a socket so create a handler One. File fs = new One. File(socket. Temp); // start the handler running fs. start(); } } catch(IOException e) {System. out. println("Socket error"); } } } 95 -713 39
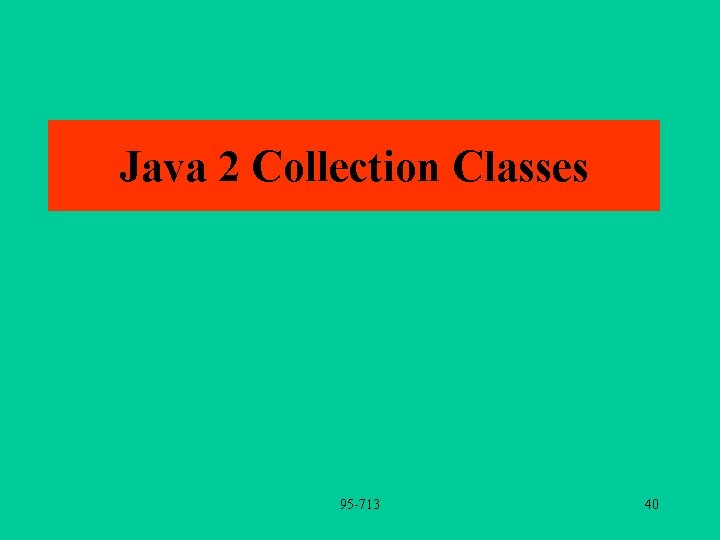
Java 2 Collection Classes 95 -713 40
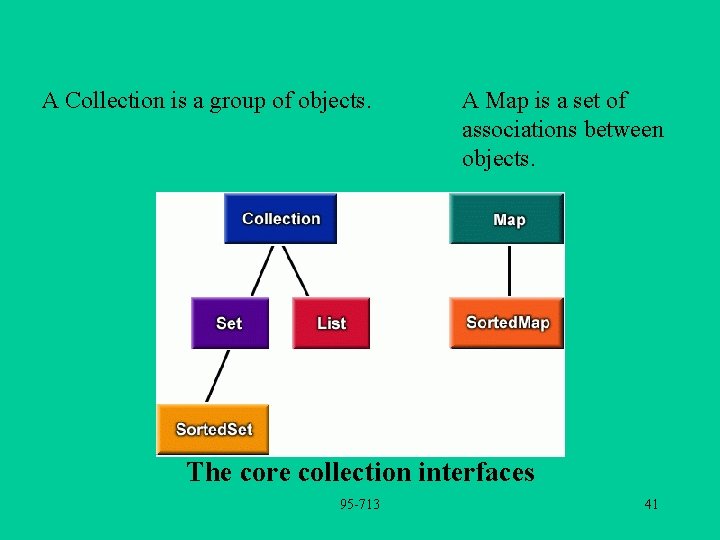
A Collection is a group of objects. A Map is a set of associations between objects. The core collection interfaces 95 -713 41
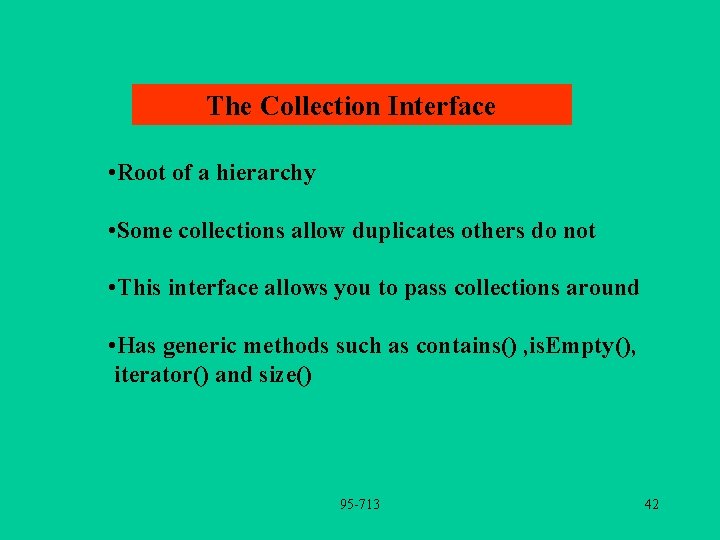
The Collection Interface • Root of a hierarchy • Some collections allow duplicates others do not • This interface allows you to pass collections around • Has generic methods such as contains() , is. Empty(), iterator() and size() 95 -713 42
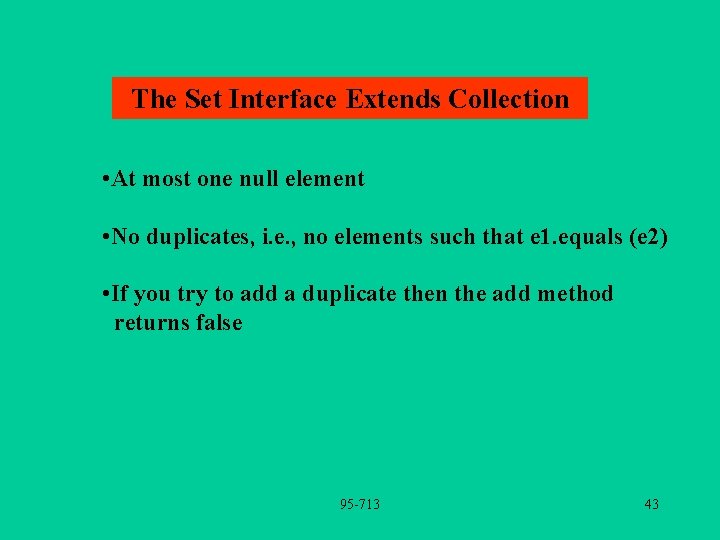
The Set Interface Extends Collection • At most one null element • No duplicates, i. e. , no elements such that e 1. equals (e 2) • If you try to add a duplicate then the add method returns false 95 -713 43
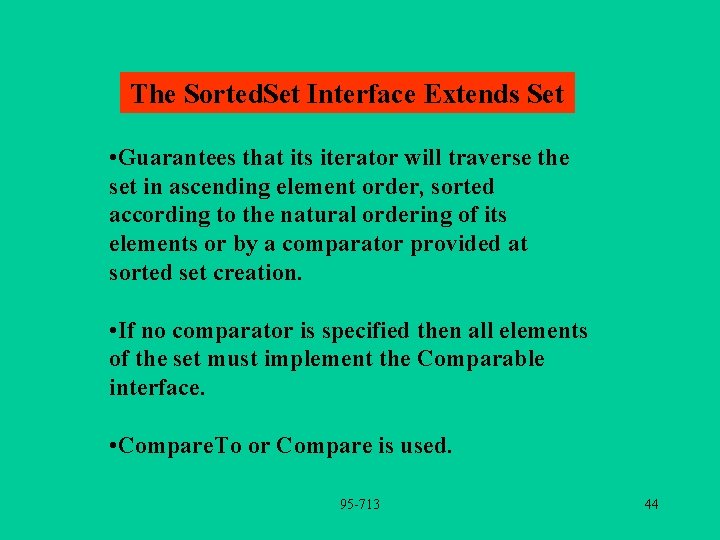
The Sorted. Set Interface Extends Set • Guarantees that its iterator will traverse the set in ascending element order, sorted according to the natural ordering of its elements or by a comparator provided at sorted set creation. • If no comparator is specified then all elements of the set must implement the Comparable interface. • Compare. To or Compare is used. 95 -713 44
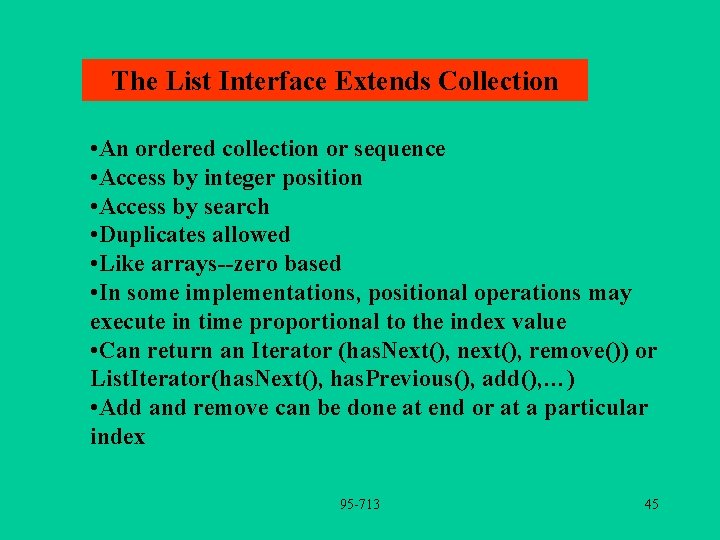
The List Interface Extends Collection • An ordered collection or sequence • Access by integer position • Access by search • Duplicates allowed • Like arrays--zero based • In some implementations, positional operations may execute in time proportional to the index value • Can return an Iterator (has. Next(), next(), remove()) or List. Iterator(has. Next(), has. Previous(), add(), …) • Add and remove can be done at end or at a particular index 95 -713 45
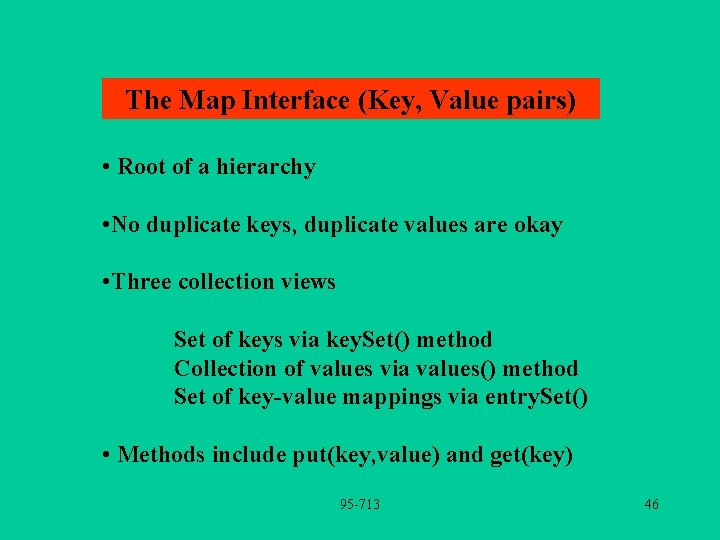
The Map Interface (Key, Value pairs) • Root of a hierarchy • No duplicate keys, duplicate values are okay • Three collection views Set of keys via key. Set() method Collection of values via values() method Set of key-value mappings via entry. Set() • Methods include put(key, value) and get(key) 95 -713 46
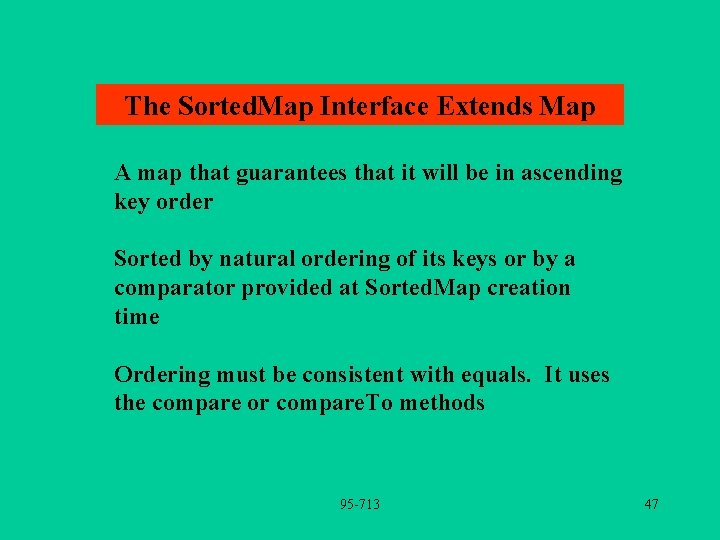
The Sorted. Map Interface Extends Map A map that guarantees that it will be in ascending key order Sorted by natural ordering of its keys or by a comparator provided at Sorted. Map creation time Ordering must be consistent with equals. It uses the compare or compare. To methods 95 -713 47
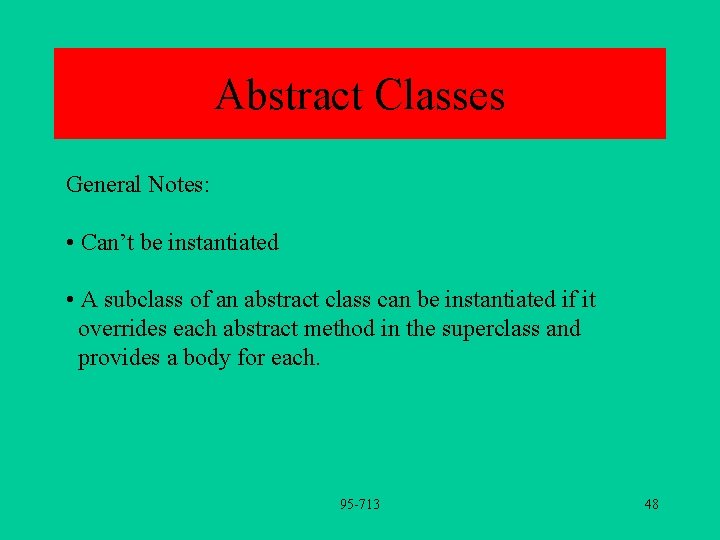
Abstract Classes General Notes: • Can’t be instantiated • A subclass of an abstract class can be instantiated if it overrides each abstract method in the superclass and provides a body for each. 95 -713 48
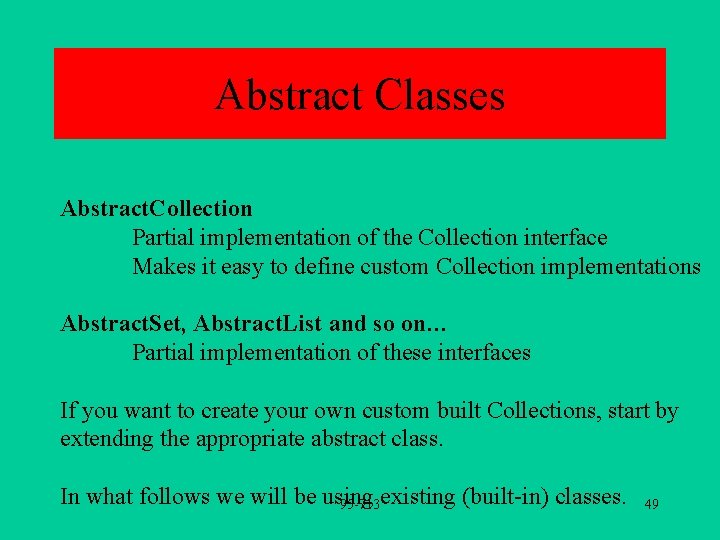
Abstract Classes Abstract. Collection Partial implementation of the Collection interface Makes it easy to define custom Collection implementations Abstract. Set, Abstract. List and so on… Partial implementation of these interfaces If you want to create your own custom built Collections, start by extending the appropriate abstract class. In what follows we will be using 95 -713 existing (built-in) classes. 49
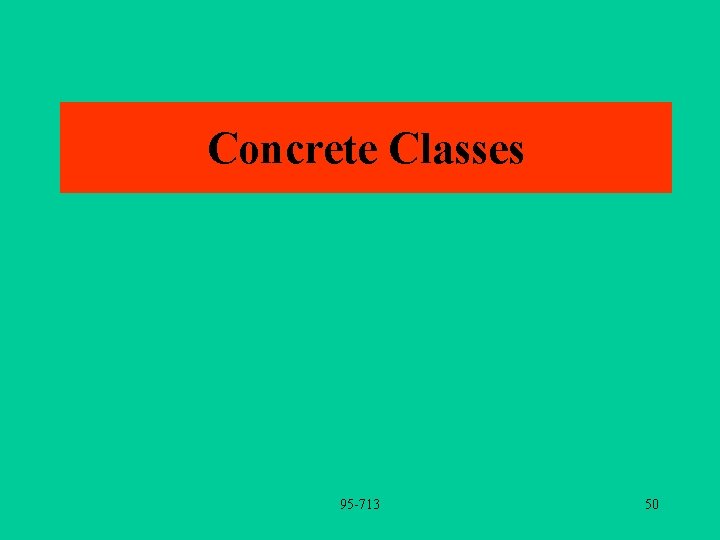
Concrete Classes 95 -713 50
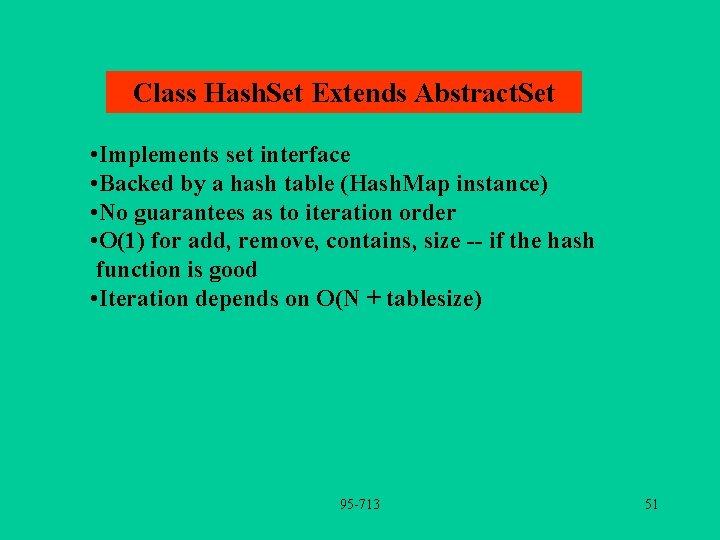
Class Hash. Set Extends Abstract. Set • Implements set interface • Backed by a hash table (Hash. Map instance) • No guarantees as to iteration order • O(1) for add, remove, contains, size -- if the hash function is good • Iteration depends on O(N + tablesize) 95 -713 51
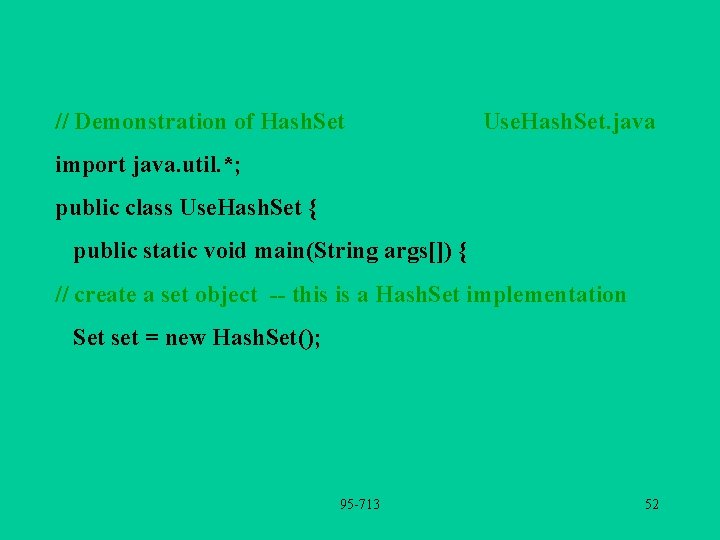
// Demonstration of Hash. Set Use. Hash. Set. java import java. util. *; public class Use. Hash. Set { public static void main(String args[]) { // create a set object -- this is a Hash. Set implementation Set set = new Hash. Set(); 95 -713 52
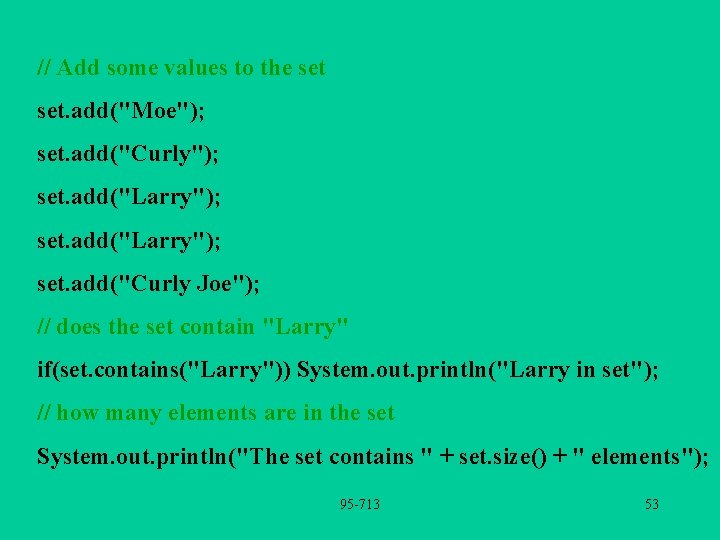
// Add some values to the set. add("Moe"); set. add("Curly"); set. add("Larry"); set. add("Curly Joe"); // does the set contain "Larry" if(set. contains("Larry")) System. out. println("Larry in set"); // how many elements are in the set System. out. println("The set contains " + set. size() + " elements"); 95 -713 53
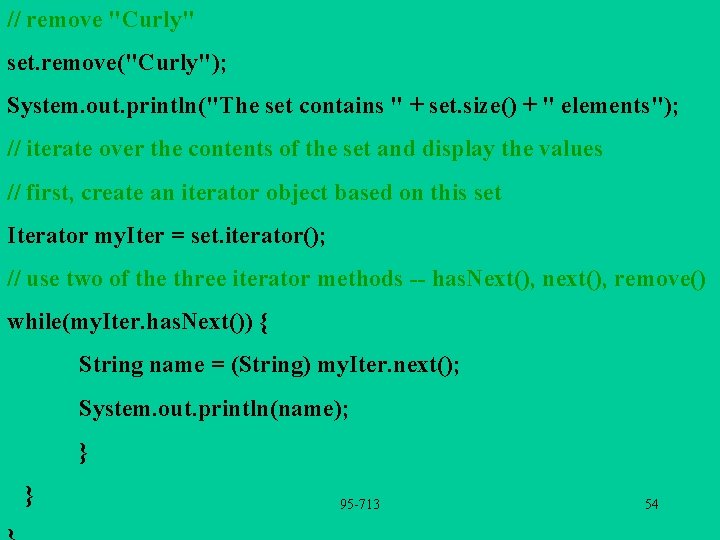
// remove "Curly" set. remove("Curly"); System. out. println("The set contains " + set. size() + " elements"); // iterate over the contents of the set and display the values // first, create an iterator object based on this set Iterator my. Iter = set. iterator(); // use two of the three iterator methods -- has. Next(), next(), remove() while(my. Iter. has. Next()) { String name = (String) my. Iter. next(); System. out. println(name); } } 95 -713 54
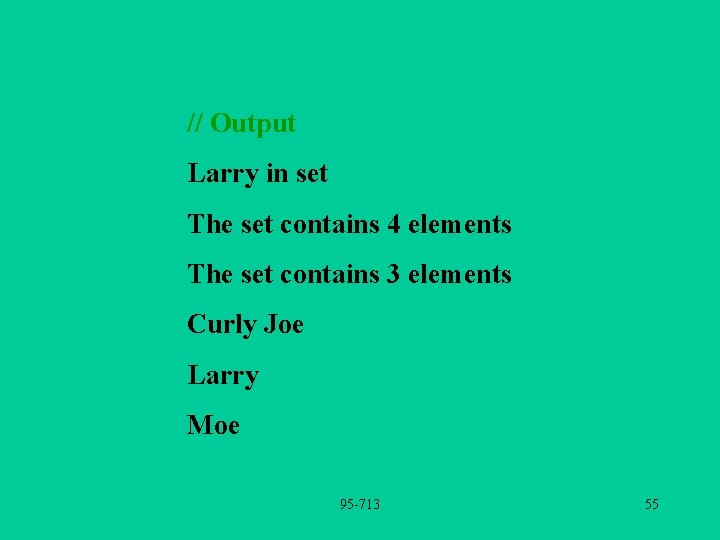
// Output Larry in set The set contains 4 elements The set contains 3 elements Curly Joe Larry Moe 95 -713 55
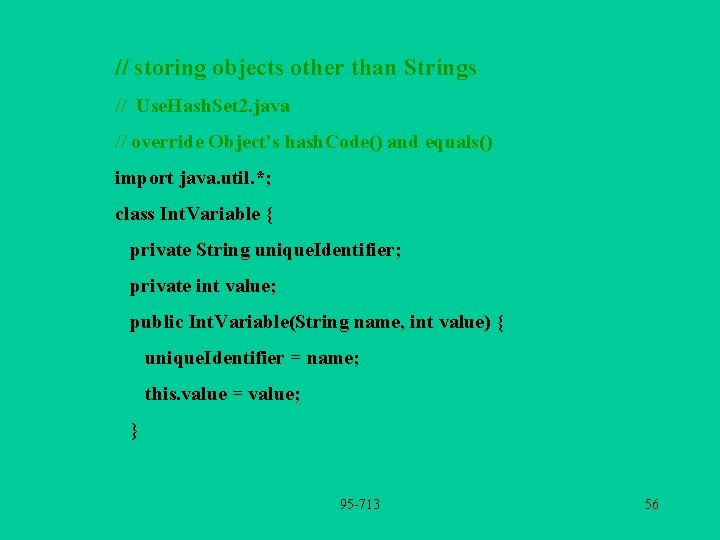
// storing objects other than Strings // Use. Hash. Set 2. java // override Object's hash. Code() and equals() import java. util. *; class Int. Variable { private String unique. Identifier; private int value; public Int. Variable(String name, int value) { unique. Identifier = name; this. value = value; } 95 -713 56
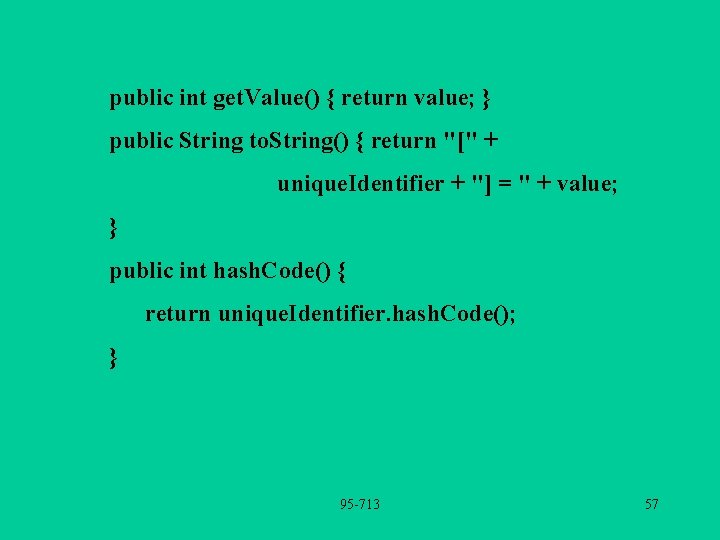
public int get. Value() { return value; } public String to. String() { return "[" + unique. Identifier + "] = " + value; } public int hash. Code() { return unique. Identifier. hash. Code(); } 95 -713 57
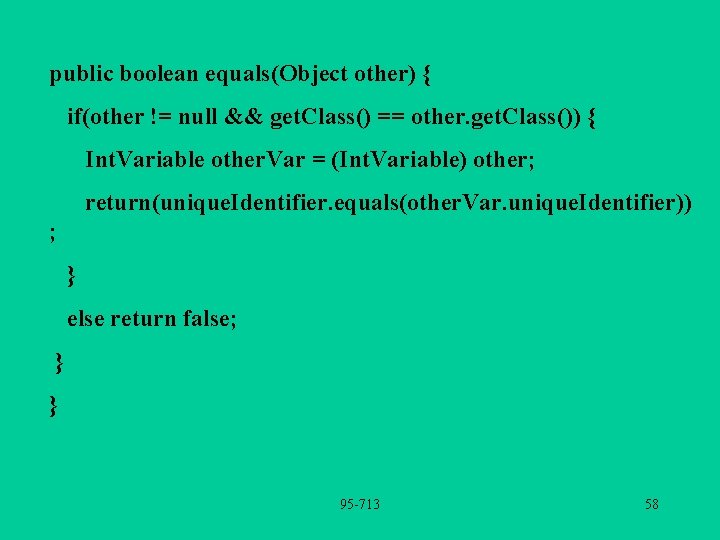
public boolean equals(Object other) { if(other != null && get. Class() == other. get. Class()) { Int. Variable other. Var = (Int. Variable) other; return(unique. Identifier. equals(other. Var. unique. Identifier)) ; } else return false; } } 95 -713 58
![public class Use Hash Set 2 public static void mainString args Set public class Use. Hash. Set 2 { public static void main(String args[]) { Set](https://slidetodoc.com/presentation_image_h/31cf08bc45fc415bb1238376aea30ede/image-59.jpg)
public class Use. Hash. Set 2 { public static void main(String args[]) { Set symbol. Table = new Hash. Set(); Int. Variable x = new Int. Variable("X", 23), y = new Int. Variable("Y", 45); symbol. Table. add(x); symbol. Table. add(y); Iterator iter = symbol. Table. iterator(); while(iter. has. Next()) { System. out. println(iter. next()); } } } 95 -713 59
![Output Y 45 X 23 95 713 60 //Output [Y] = 45 [X] = 23 95 -713 60](https://slidetodoc.com/presentation_image_h/31cf08bc45fc415bb1238376aea30ede/image-60.jpg)
//Output [Y] = 45 [X] = 23 95 -713 60
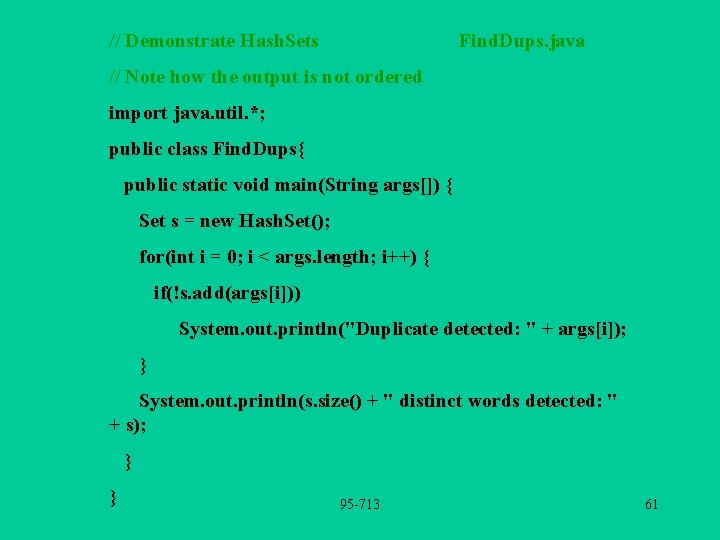
// Demonstrate Hash. Sets Find. Dups. java // Note how the output is not ordered import java. util. *; public class Find. Dups{ public static void main(String args[]) { Set s = new Hash. Set(); for(int i = 0; i < args. length; i++) { if(!s. add(args[i])) System. out. println("Duplicate detected: " + args[i]); } System. out. println(s. size() + " distinct words detected: " + s); } } 95 -713 61
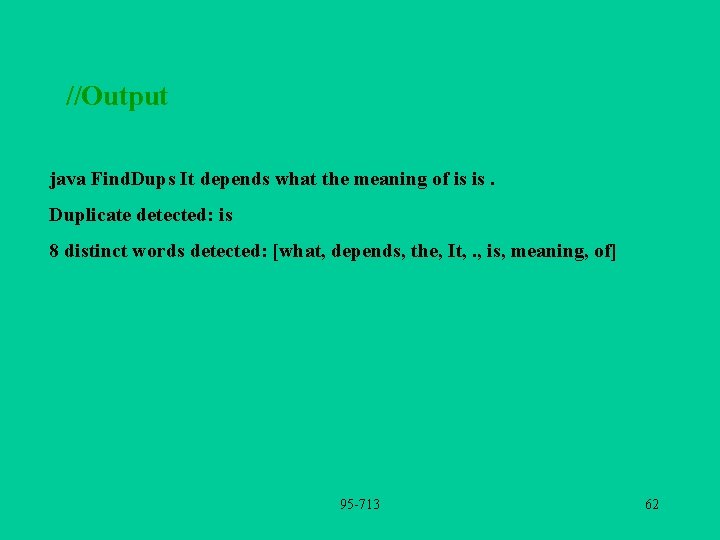
//Output java Find. Dups It depends what the meaning of is is. Duplicate detected: is 8 distinct words detected: [what, depends, the, It, . , is, meaning, of] 95 -713 62
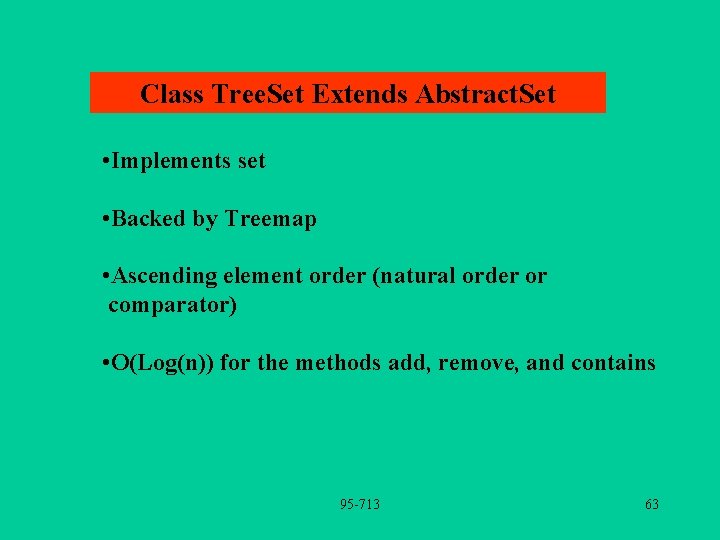
Class Tree. Set Extends Abstract. Set • Implements set • Backed by Treemap • Ascending element order (natural order or comparator) • O(Log(n)) for the methods add, remove, and contains 95 -713 63
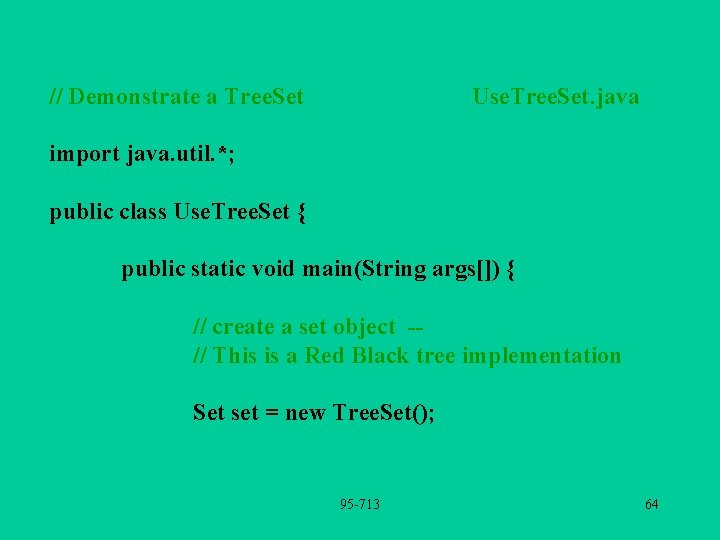
// Demonstrate a Tree. Set Use. Tree. Set. java import java. util. *; public class Use. Tree. Set { public static void main(String args[]) { // create a set object -// This is a Red Black tree implementation Set set = new Tree. Set(); 95 -713 64
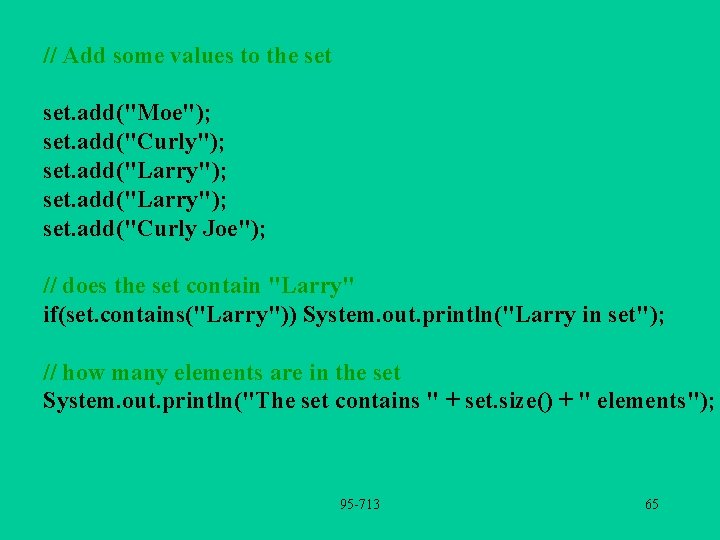
// Add some values to the set. add("Moe"); set. add("Curly"); set. add("Larry"); set. add("Curly Joe"); // does the set contain "Larry" if(set. contains("Larry")) System. out. println("Larry in set"); // how many elements are in the set System. out. println("The set contains " + set. size() + " elements"); 95 -713 65
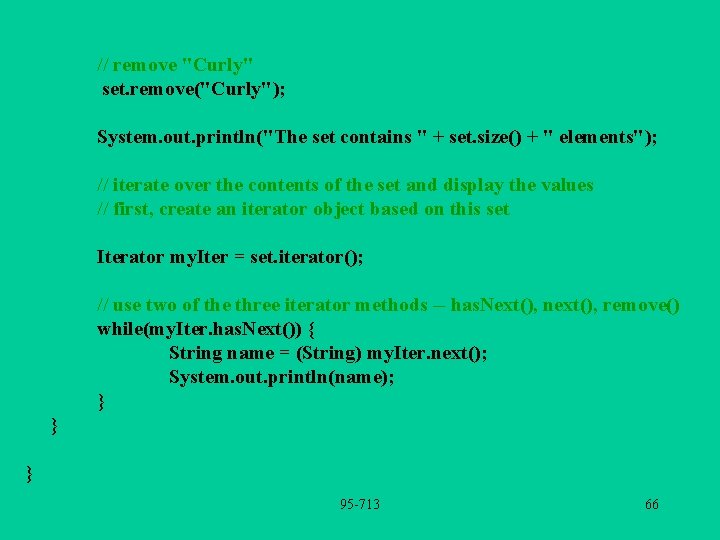
// remove "Curly" set. remove("Curly"); System. out. println("The set contains " + set. size() + " elements"); // iterate over the contents of the set and display the values // first, create an iterator object based on this set Iterator my. Iter = set. iterator(); // use two of the three iterator methods -- has. Next(), next(), remove() while(my. Iter. has. Next()) { String name = (String) my. Iter. next(); System. out. println(name); } } } 95 -713 66
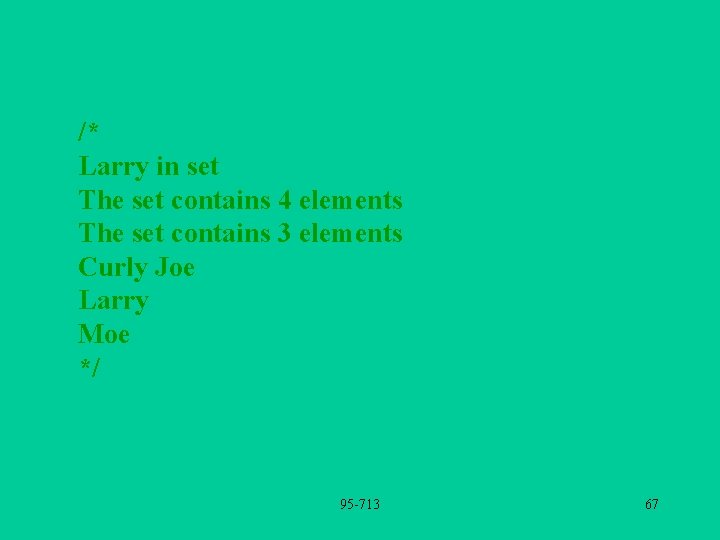
/* Larry in set The set contains 4 elements The set contains 3 elements Curly Joe Larry Moe */ 95 -713 67
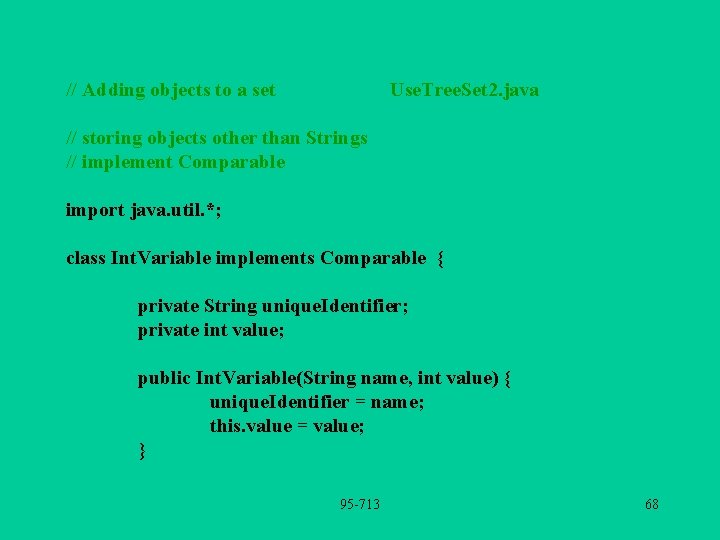
// Adding objects to a set Use. Tree. Set 2. java // storing objects other than Strings // implement Comparable import java. util. *; class Int. Variable implements Comparable { private String unique. Identifier; private int value; public Int. Variable(String name, int value) { unique. Identifier = name; this. value = value; } 95 -713 68
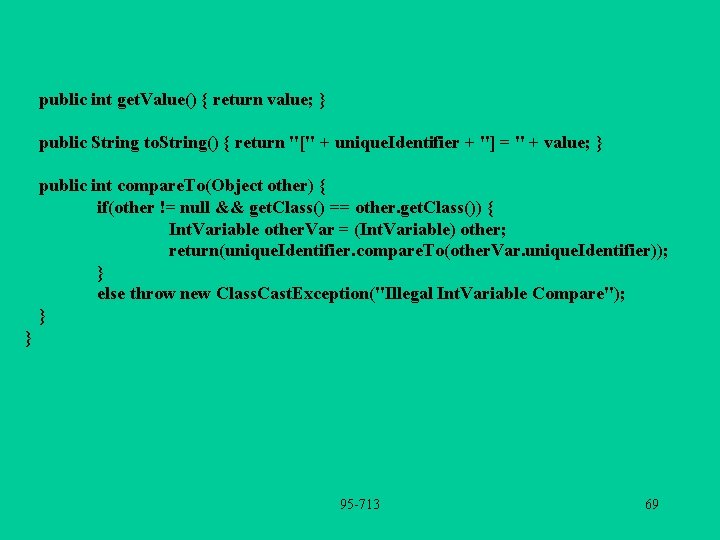
public int get. Value() { return value; } public String to. String() { return "[" + unique. Identifier + "] = " + value; } public int compare. To(Object other) { if(other != null && get. Class() == other. get. Class()) { Int. Variable other. Var = (Int. Variable) other; return(unique. Identifier. compare. To(other. Var. unique. Identifier)); } else throw new Class. Cast. Exception("Illegal Int. Variable Compare"); } } 95 -713 69
![public class Use Tree Set 2 public static void mainString args Set public class Use. Tree. Set 2 { public static void main(String args[]) { Set](https://slidetodoc.com/presentation_image_h/31cf08bc45fc415bb1238376aea30ede/image-70.jpg)
public class Use. Tree. Set 2 { public static void main(String args[]) { Set symbol. Table = new Tree. Set(); Int. Variable x = new Int. Variable("X", 23), y = new Int. Variable("Y", 45); symbol. Table. add(x); symbol. Table. add(y); Iterator iter = symbol. Table. iterator(); while(iter. has. Next()) { System. out. println(iter. next()); } } } 95 -713 70
![X 23 Y 45 95 713 71 /* [X] = 23 [Y] = 45 */ 95 -713 71](https://slidetodoc.com/presentation_image_h/31cf08bc45fc415bb1238376aea30ede/image-71.jpg)
/* [X] = 23 [Y] = 45 */ 95 -713 71
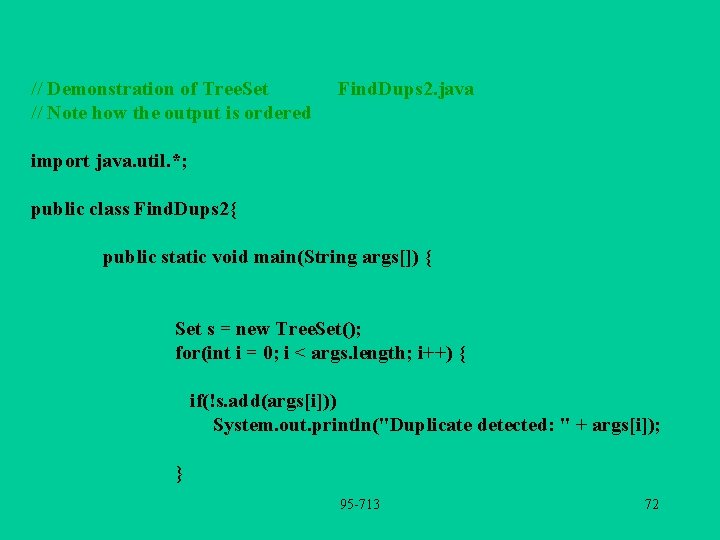
// Demonstration of Tree. Set // Note how the output is ordered Find. Dups 2. java import java. util. *; public class Find. Dups 2{ public static void main(String args[]) { Set s = new Tree. Set(); for(int i = 0; i < args. length; i++) { if(!s. add(args[i])) System. out. println("Duplicate detected: " + args[i]); } 95 -713 72
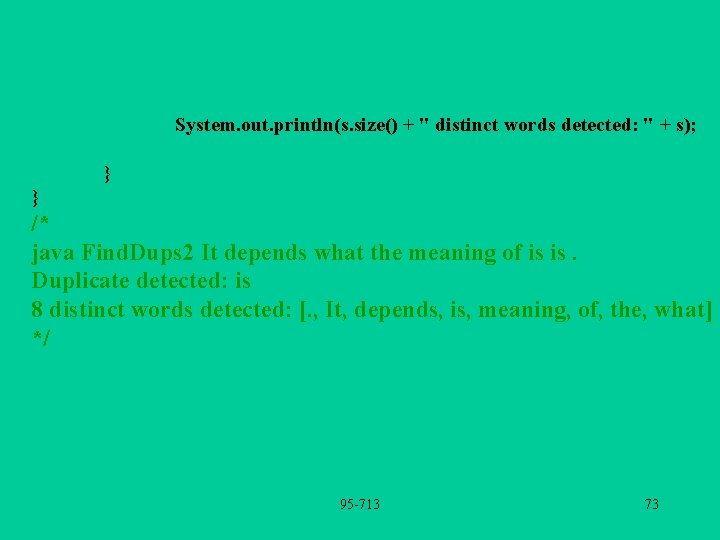
System. out. println(s. size() + " distinct words detected: " + s); } } /* java Find. Dups 2 It depends what the meaning of is is. Duplicate detected: is 8 distinct words detected: [. , It, depends, is, meaning, of, the, what] */ 95 -713 73
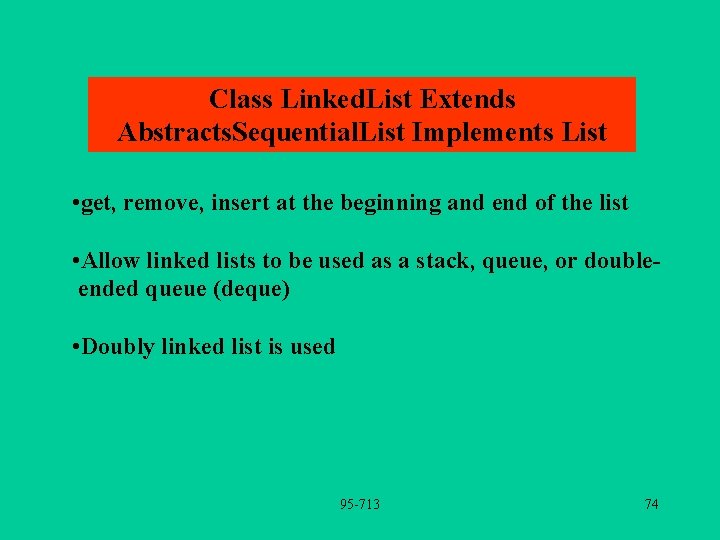
Class Linked. List Extends Abstracts. Sequential. List Implements List • get, remove, insert at the beginning and end of the list • Allow linked lists to be used as a stack, queue, or doubleended queue (deque) • Doubly linked list is used 95 -713 74
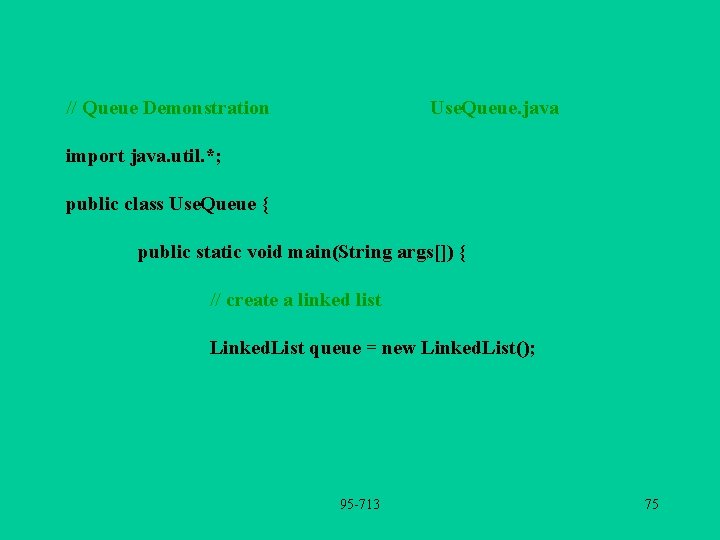
// Queue Demonstration Use. Queue. java import java. util. *; public class Use. Queue { public static void main(String args[]) { // create a linked list Linked. List queue = new Linked. List(); 95 -713 75
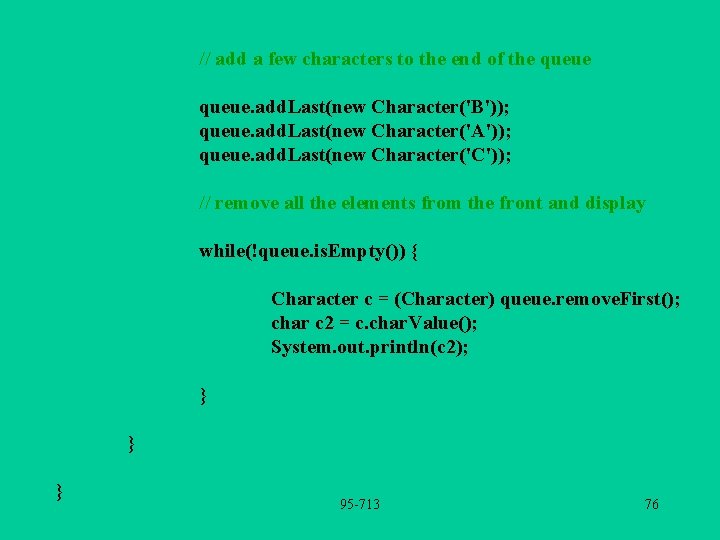
// add a few characters to the end of the queue. add. Last(new Character('B')); queue. add. Last(new Character('A')); queue. add. Last(new Character('C')); // remove all the elements from the front and display while(!queue. is. Empty()) { Character c = (Character) queue. remove. First(); char c 2 = c. char. Value(); System. out. println(c 2); } } } 95 -713 76
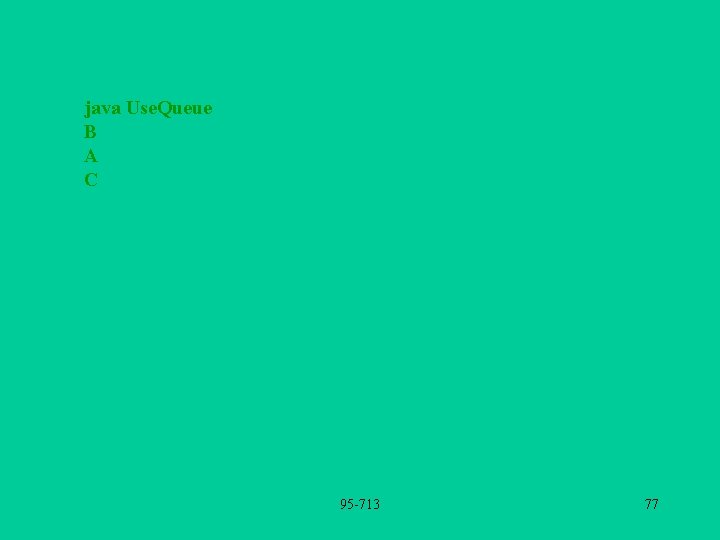
java Use. Queue B A C 95 -713 77
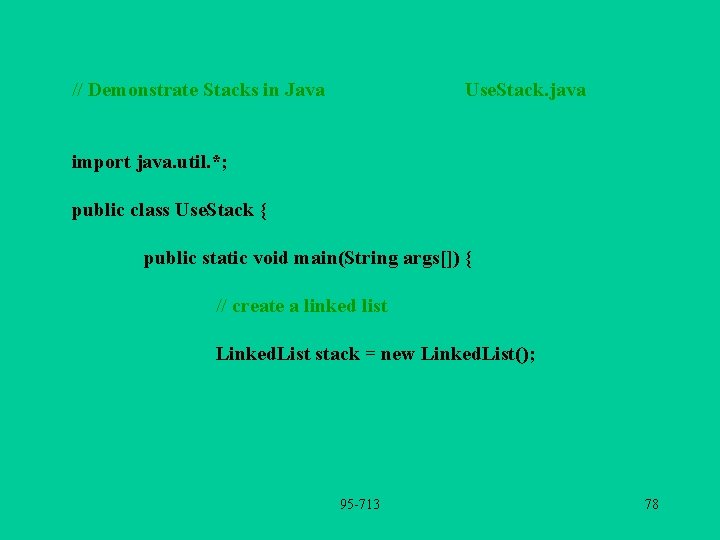
// Demonstrate Stacks in Java Use. Stack. java import java. util. *; public class Use. Stack { public static void main(String args[]) { // create a linked list Linked. List stack = new Linked. List(); 95 -713 78
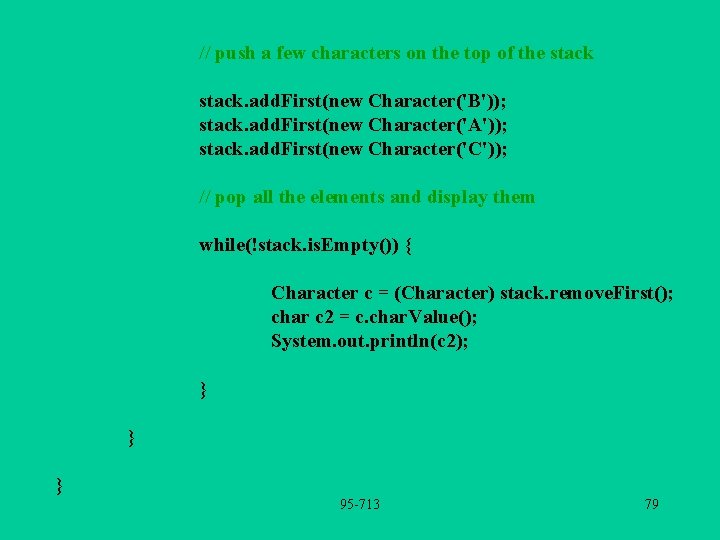
// push a few characters on the top of the stack. add. First(new Character('B')); stack. add. First(new Character('A')); stack. add. First(new Character('C')); // pop all the elements and display them while(!stack. is. Empty()) { Character c = (Character) stack. remove. First(); char c 2 = c. char. Value(); System. out. println(c 2); } } } 95 -713 79
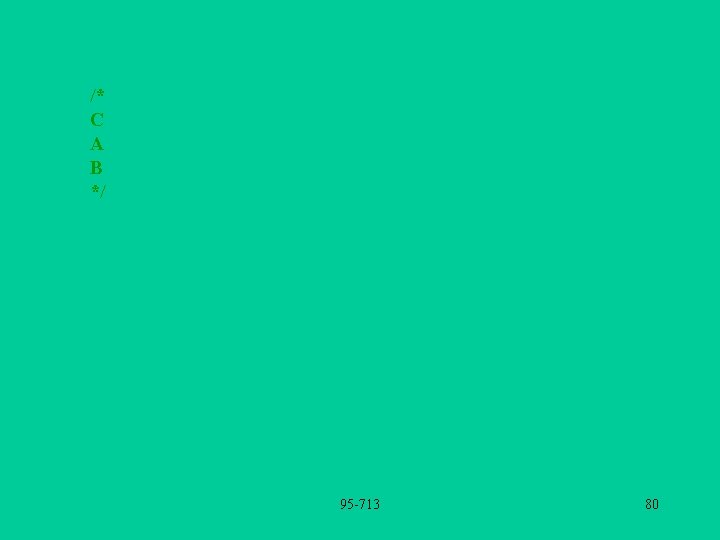
/* C A B */ 95 -713 80
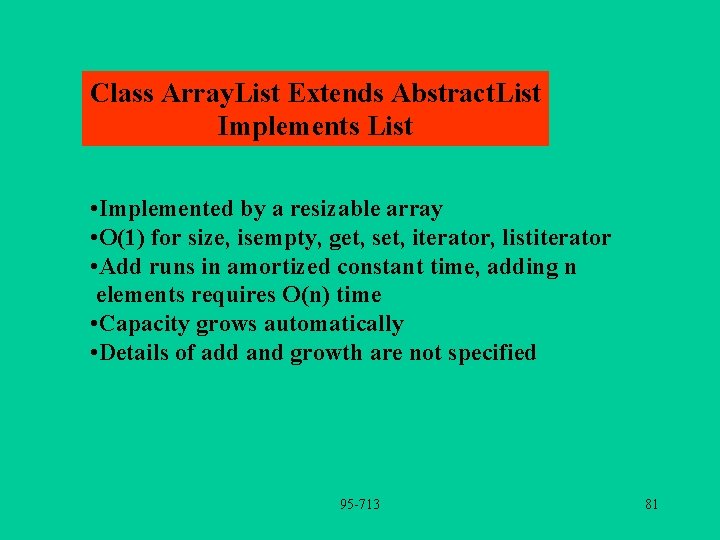
Class Array. List Extends Abstract. List Implements List • Implemented by a resizable array • O(1) for size, isempty, get, set, iterator, listiterator • Add runs in amortized constant time, adding n elements requires O(n) time • Capacity grows automatically • Details of add and growth are not specified 95 -713 81
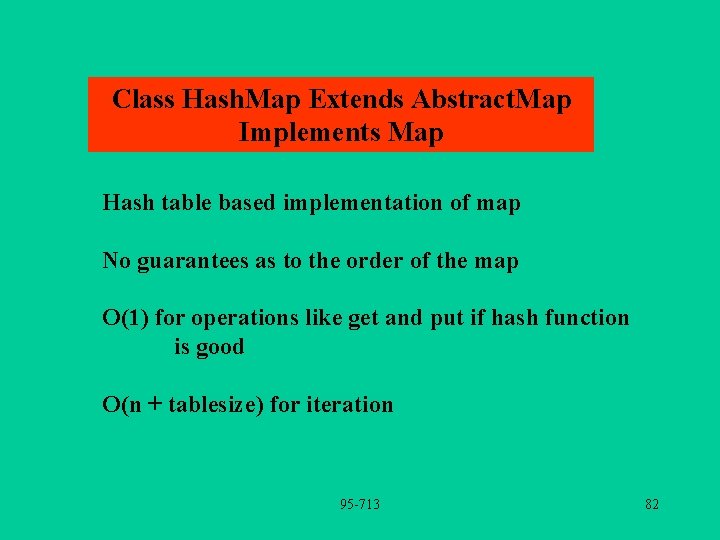
Class Hash. Map Extends Abstract. Map Implements Map Hash table based implementation of map No guarantees as to the order of the map O(1) for operations like get and put if hash function is good O(n + tablesize) for iteration 95 -713 82
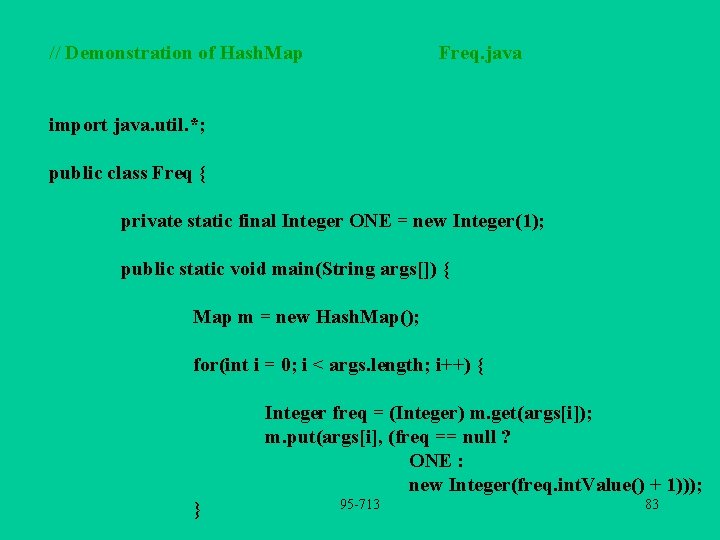
// Demonstration of Hash. Map Freq. java import java. util. *; public class Freq { private static final Integer ONE = new Integer(1); public static void main(String args[]) { Map m = new Hash. Map(); for(int i = 0; i < args. length; i++) { Integer freq = (Integer) m. get(args[i]); m. put(args[i], (freq == null ? ONE : new Integer(freq. int. Value() + 1))); } 95 -713 83
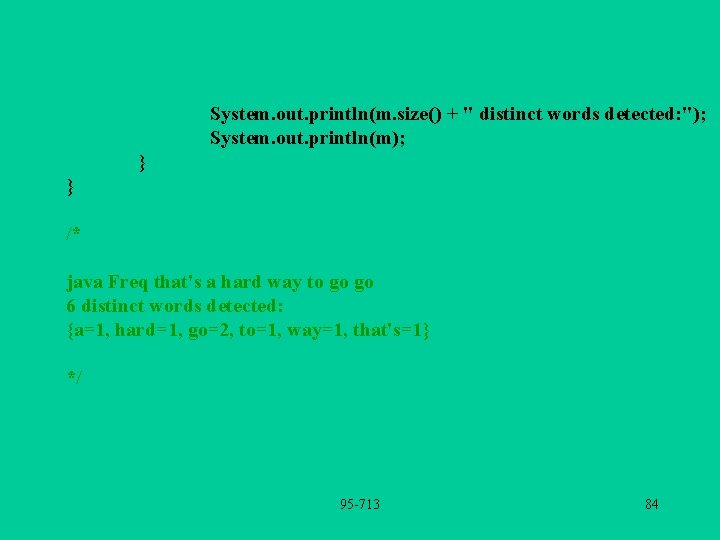
System. out. println(m. size() + " distinct words detected: "); System. out. println(m); } } /* java Freq that's a hard way to go go 6 distinct words detected: {a=1, hard=1, go=2, to=1, way=1, that's=1} */ 95 -713 84
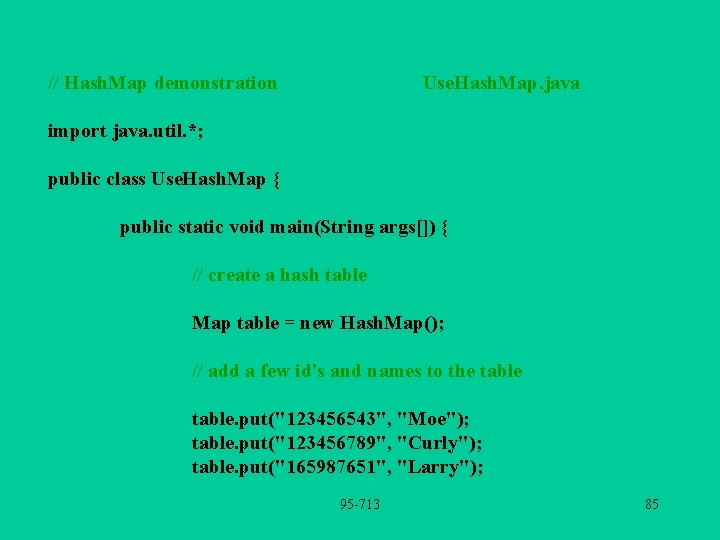
// Hash. Map demonstration Use. Hash. Map. java import java. util. *; public class Use. Hash. Map { public static void main(String args[]) { // create a hash table Map table = new Hash. Map(); // add a few id's and names to the table. put("123456543", "Moe"); table. put("123456789", "Curly"); table. put("165987651", "Larry"); 95 -713 85
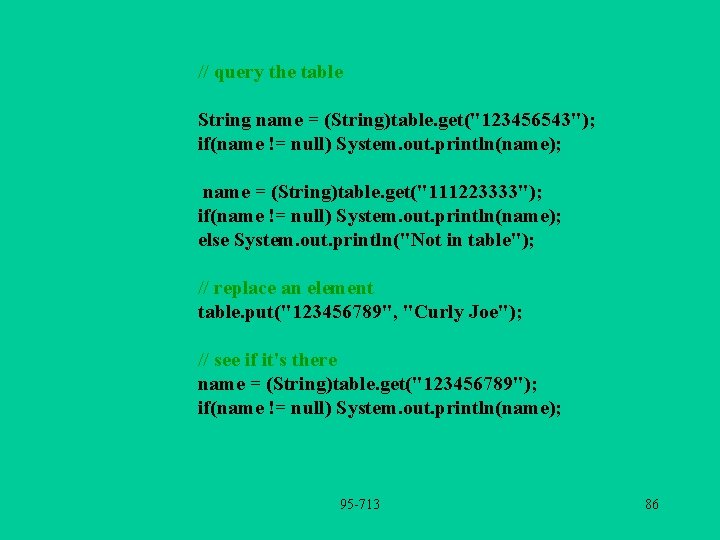
// query the table String name = (String)table. get("123456543"); if(name != null) System. out. println(name); name = (String)table. get("111223333"); if(name != null) System. out. println(name); else System. out. println("Not in table"); // replace an element table. put("123456789", "Curly Joe"); // see if it's there name = (String)table. get("123456789"); if(name != null) System. out. println(name); 95 -713 86
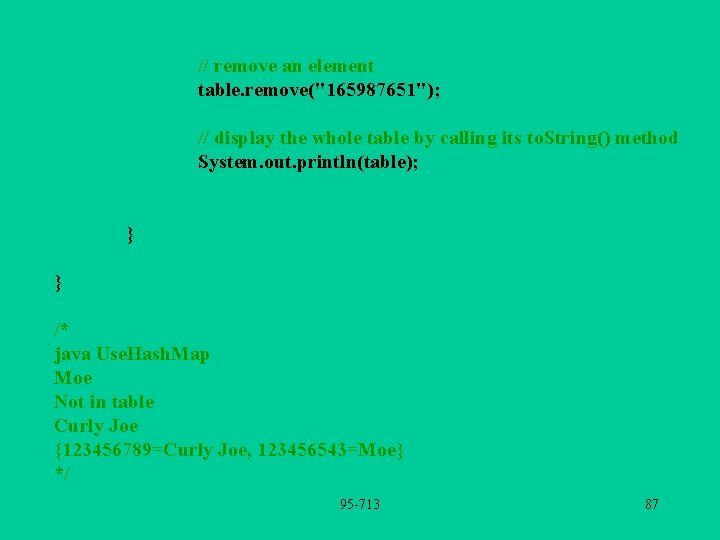
// remove an element table. remove("165987651"); // display the whole table by calling its to. String() method System. out. println(table); } } /* java Use. Hash. Map Moe Not in table Curly Joe {123456789=Curly Joe, 123456543=Moe} */ 95 -713 87
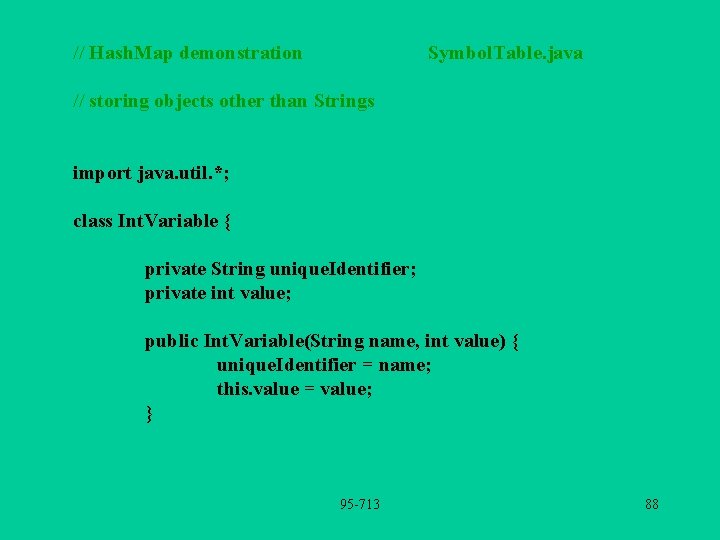
// Hash. Map demonstration Symbol. Table. java // storing objects other than Strings import java. util. *; class Int. Variable { private String unique. Identifier; private int value; public Int. Variable(String name, int value) { unique. Identifier = name; this. value = value; } 95 -713 88
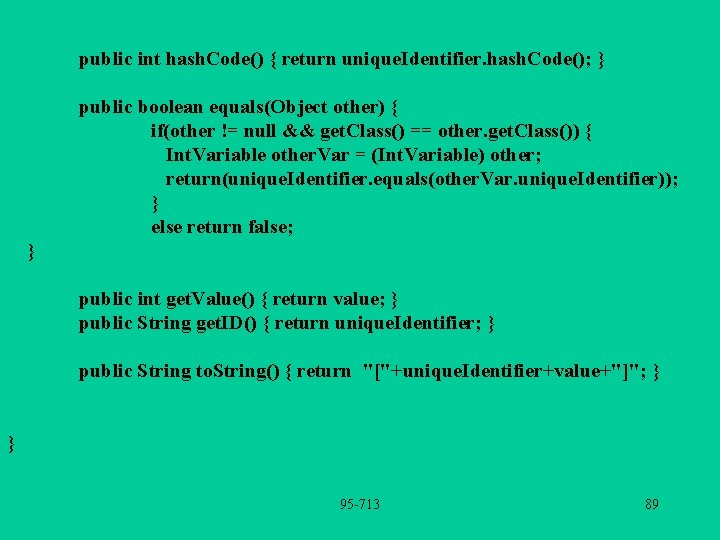
public int hash. Code() { return unique. Identifier. hash. Code(); } public boolean equals(Object other) { if(other != null && get. Class() == other. get. Class()) { Int. Variable other. Var = (Int. Variable) other; return(unique. Identifier. equals(other. Var. unique. Identifier)); } else return false; } public int get. Value() { return value; } public String get. ID() { return unique. Identifier; } public String to. String() { return "["+unique. Identifier+value+"]"; } } 95 -713 89
![public class Symbol Table public static void mainString args Map symbol Table public class Symbol. Table { public static void main(String args[]) { Map symbol. Table](https://slidetodoc.com/presentation_image_h/31cf08bc45fc415bb1238376aea30ede/image-90.jpg)
public class Symbol. Table { public static void main(String args[]) { Map symbol. Table = new Hash. Map(); Int. Variable x = new Int. Variable("X", 23), y = new Int. Variable("Y", 45); symbol. Table. put(x. get. ID(), x); symbol. Table. put(y. get. ID(), y); Set s = symbol. Table. entry. Set(); Iterator iter = s. iterator(); while(iter. has. Next()) { System. out. println(iter. next()); } } } 95 -713 90
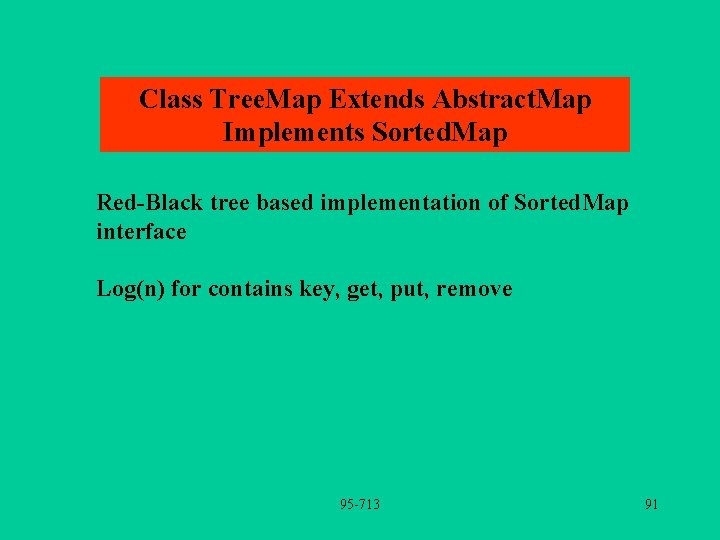
Class Tree. Map Extends Abstract. Map Implements Sorted. Map Red-Black tree based implementation of Sorted. Map interface Log(n) for contains key, get, put, remove 95 -713 91
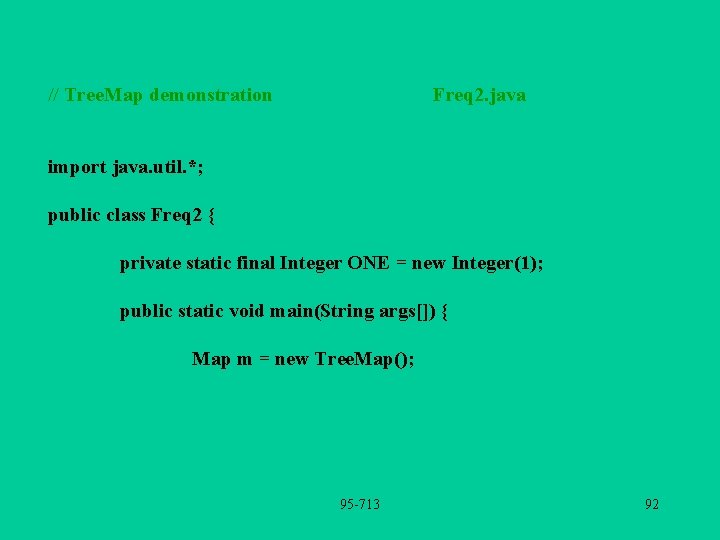
// Tree. Map demonstration Freq 2. java import java. util. *; public class Freq 2 { private static final Integer ONE = new Integer(1); public static void main(String args[]) { Map m = new Tree. Map(); 95 -713 92
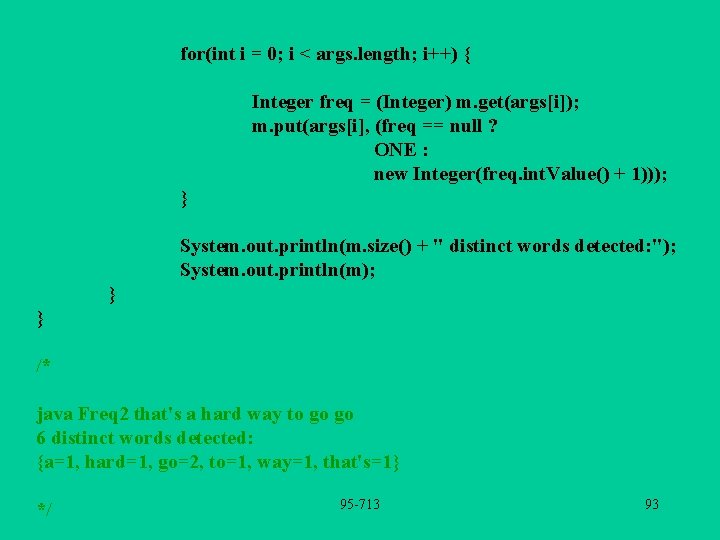
for(int i = 0; i < args. length; i++) { Integer freq = (Integer) m. get(args[i]); m. put(args[i], (freq == null ? ONE : new Integer(freq. int. Value() + 1))); } System. out. println(m. size() + " distinct words detected: "); System. out. println(m); } } /* java Freq 2 that's a hard way to go go 6 distinct words detected: {a=1, hard=1, go=2, to=1, way=1, that's=1} */ 95 -713 93
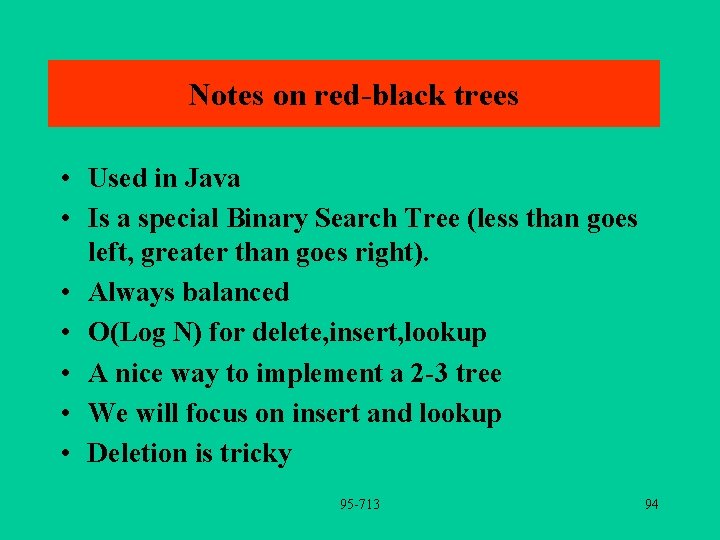
Notes on red-black trees • Used in Java • Is a special Binary Search Tree (less than goes left, greater than goes right). • Always balanced • O(Log N) for delete, insert, lookup • A nice way to implement a 2 -3 tree • We will focus on insert and lookup • Deletion is tricky 95 -713 94
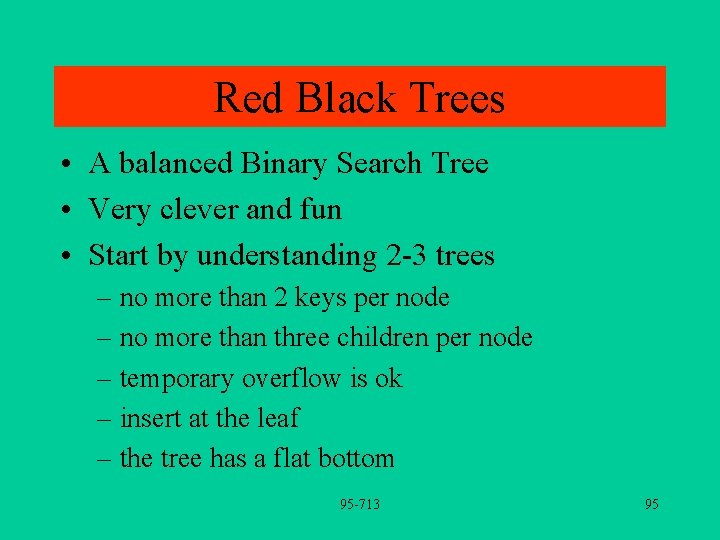
Red Black Trees • A balanced Binary Search Tree • Very clever and fun • Start by understanding 2 -3 trees – no more than 2 keys per node – no more than three children per node – temporary overflow is ok – insert at the leaf – the tree has a flat bottom 95 -713 95
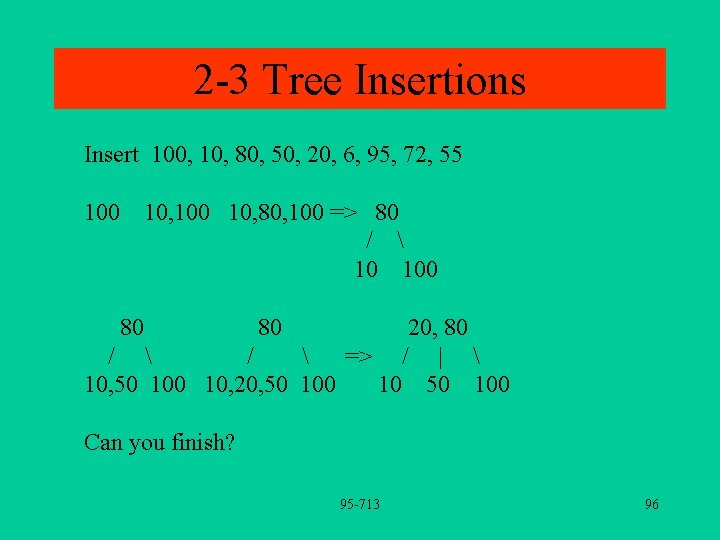
2 -3 Tree Insertions Insert 100, 10, 80, 50, 20, 6, 95, 72, 55 100 10, 80, 100 => 80 / 10 100 80 80 20, 80 / => / | 10, 50 10, 20, 50 100 Can you finish? 95 -713 96
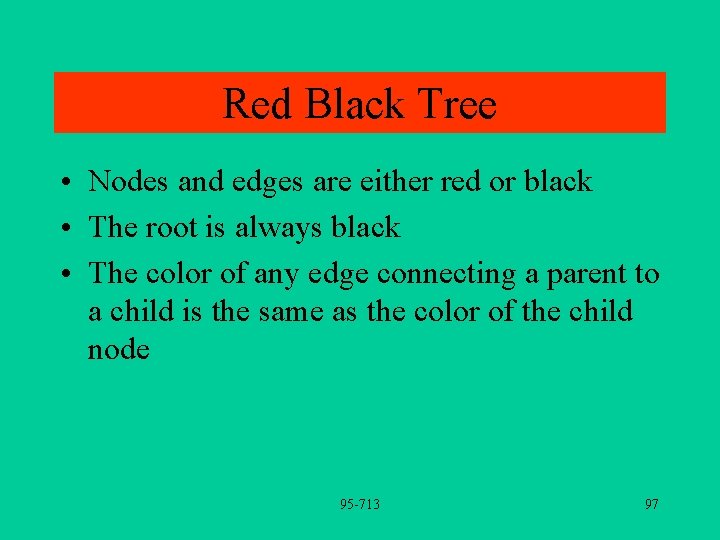
Red Black Tree • Nodes and edges are either red or black • The root is always black • The color of any edge connecting a parent to a child is the same as the color of the child node 95 -713 97
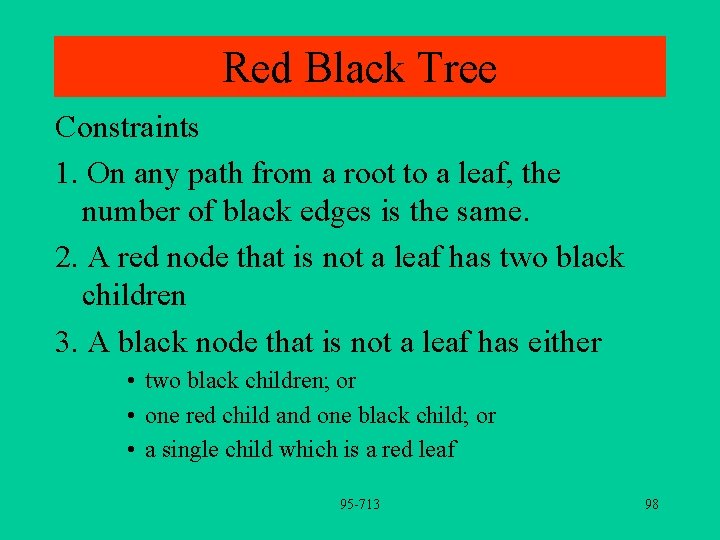
Red Black Tree Constraints 1. On any path from a root to a leaf, the number of black edges is the same. 2. A red node that is not a leaf has two black children 3. A black node that is not a leaf has either • two black children; or • one red child and one black child; or • a single child which is a red leaf 95 -713 98
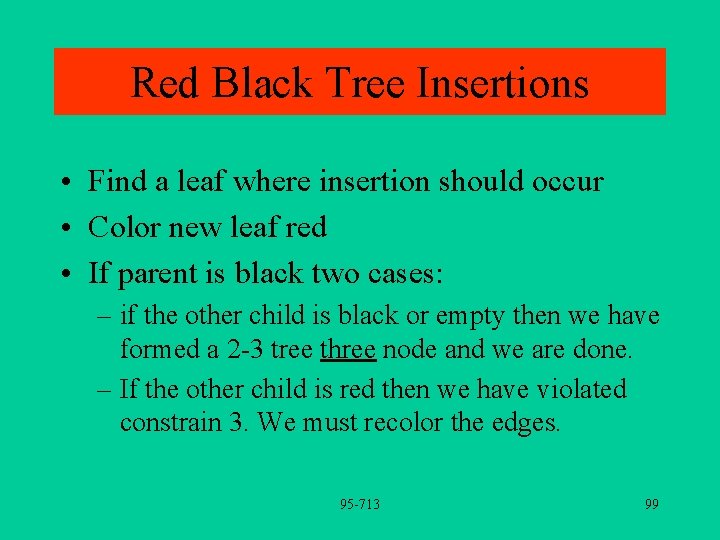
Red Black Tree Insertions • Find a leaf where insertion should occur • Color new leaf red • If parent is black two cases: – if the other child is black or empty then we have formed a 2 -3 tree three node and we are done. – If the other child is red then we have violated constrain 3. We must recolor the edges. 95 -713 99
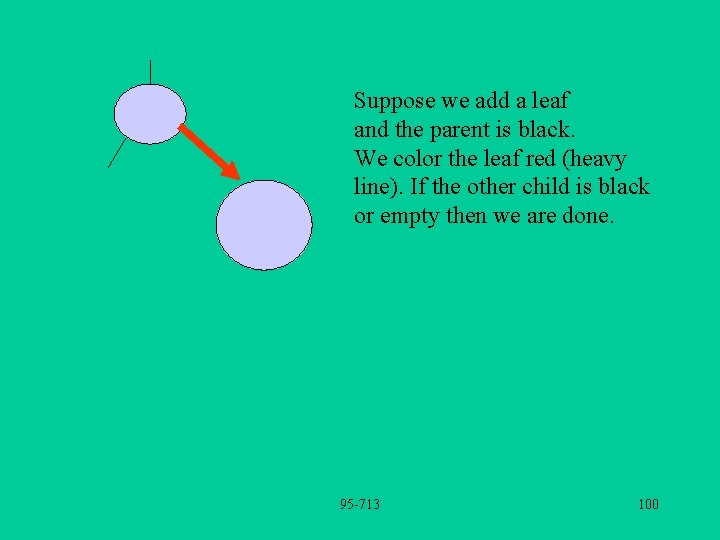
Suppose we add a leaf and the parent is black. We color the leaf red (heavy line). If the other child is black or empty then we are done. 95 -713 100
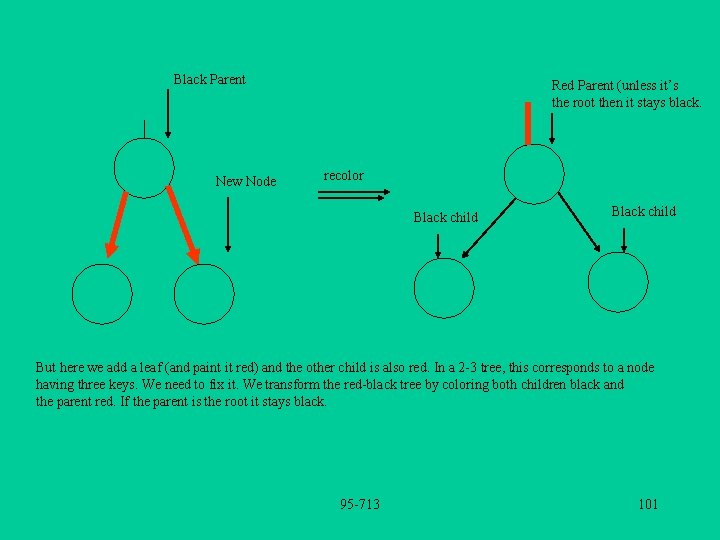
Black Parent New Node Red Parent (unless it’s the root then it stays black. recolor Black child But here we add a leaf (and paint it red) and the other child is also red. In a 2 -3 tree, this corresponds to a node having three keys. We need to fix it. We transform the red-black tree by coloring both children black and the parent red. If the parent is the root it stays black. 95 -713 101
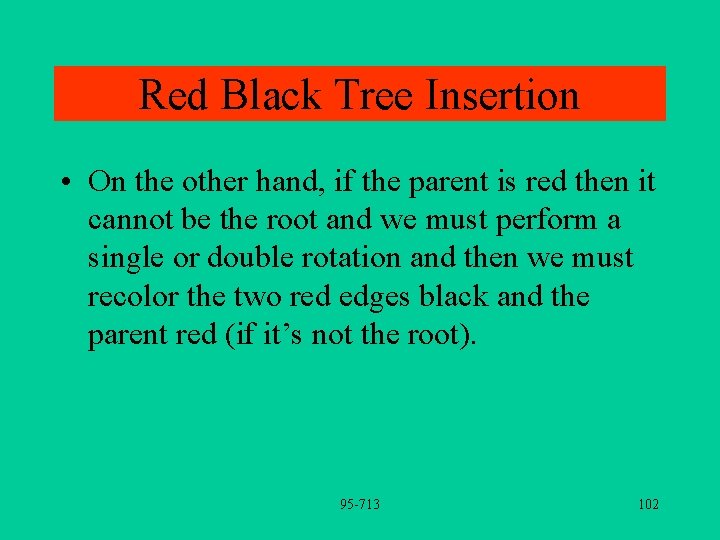
Red Black Tree Insertion • On the other hand, if the parent is red then it cannot be the root and we must perform a single or double rotation and then we must recolor the two red edges black and the parent red (if it’s not the root). 95 -713 102
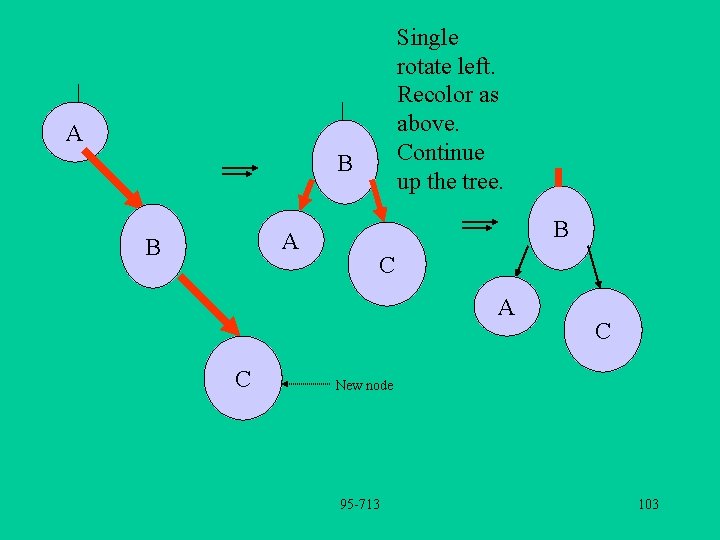
Single rotate left. Recolor as above. Continue up the tree. A B B C A C C New node 95 -713 103
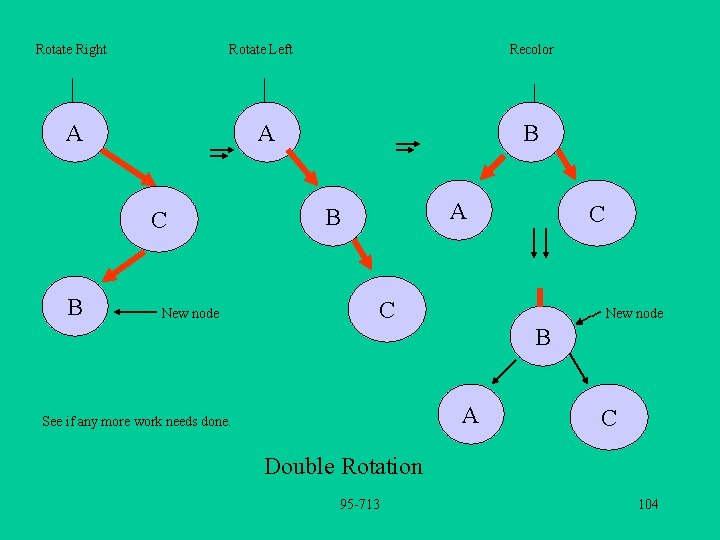
Rotate Right Rotate Left A A C B Recolor New node B A B C C New node B A See if any more work needs done. C Double Rotation 95 -713 104
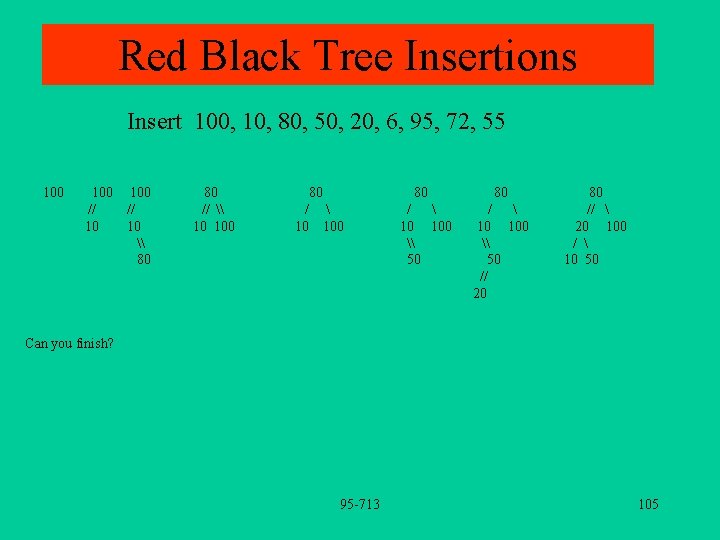
Red Black Tree Insertions Insert 100, 10, 80, 50, 20, 6, 95, 72, 55 100 // 10 \ 80 80 // \ 10 100 80 / 10 80 100 / 10 100 \ 50 80 / 10 100 \ 50 // 20 80 // 20 100 / 10 50 Can you finish? 95 -713 105
Shared memory java
Threads java
Threads em java
Java collections tree
Java collections overview
Java collections framework diagram
Collection hierarchy in java
Import java.collections
Google guava collections
7 types of pronoun
Declarative sentence
Four types of paragraphs
There are four kinds of sentence
Straight lines in art
Fluid friction
Process and threads
Process and threads
Sockets and threads
What is thread in operating system
Pseg credit and collections
Chapter 20 patient collections and financial management
Object model and collections in dhtml
Collection of specialized cells and cell products
Aba therapy billing and collections
Williams and fudge make a payment
Tonbridge refuse collection
Shapes with four sides and four corners
C11 threads
Assembly drawing
A flexible flat material made by interlacing threads/fibers
Escalonamento de threads
Os threads
The vinaya pitaka is a sacred text of
Needle like threads of spongy bone
Waxy coated paper which transfers pattern markings
Golden thread strategy
Pintos manual
Pthreads
Osteospermum white lightning
Forum.unity.com/threads/game-over.54735
/threads/ fiji
Threads consumes cpu in best possible manner
Posix threads
Forum.unity.com/threads/game-over.54735
Process vs thread
Sequence diagram threads
Threads cannot be implemented as a library
Threads.h
The tapper turning gives shape to job mcq
Cuda threads per block
Threads cannot be implemented as a library
The kernel is unaware of user threads
Cuda synchronize threads
Threads in distributed systems
Ece api
What is thread in operating system
Ece threads
Chia 3 threads
Threads vs processes
Scheduler activation
Pintos advanced scheduler
Joint mission threads
Os threads
Using system using system.collections.generic
Collections trust spectrum
Certificate iii in pathology collections
Largest galaxy
Static collection
631-828-3160
Sap collections management
Financial data systems collections
Fungsi movie task pane
Collection (abstract data type)
Using system collections generic
Lessons from hadith 25
Jisc collections manager
Jennifer weintraub
Shared services of alaska collections
Fms collections
Collections
Eton college collections
Sruthika collections
I dig bio
Collections
Reflectionit
Revenue cycle sales to cash collections
Arm industry collections
Iupui digital collections
Benchmarks in collections care
Processes of neurons
Dda collections
Set interface in java
Revenue cycle sales to cash collections
C# collections overview
4 eyes in 4 hours
Gang of four java
Weakness of public relations
Forms of fish
What market forms of meat does not undergo chilling
Axial dance movement
Market forms of meat
Author is common noun
What are the polygons
Kinds of elements and principles of arts
Import java.lang.*
Java import java.util.*