JAVA THREADS Threads Overview Creating threads in Java
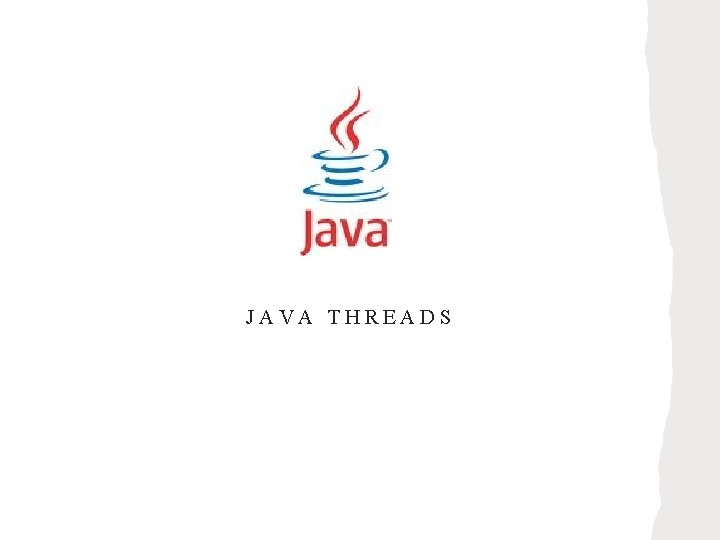
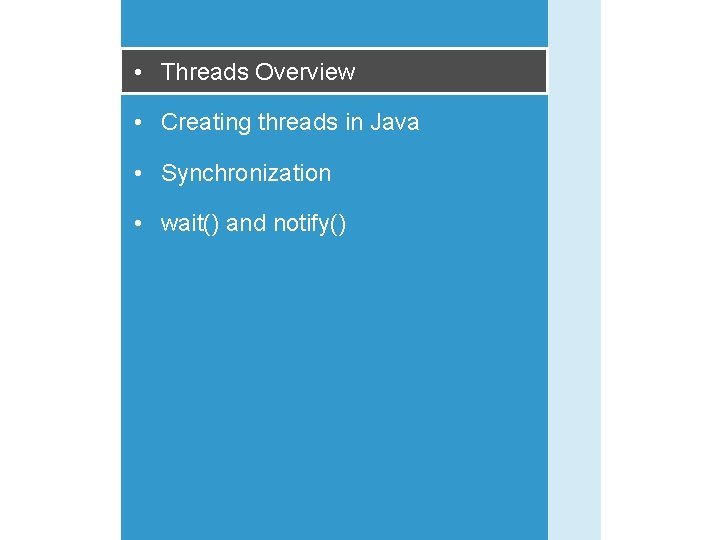
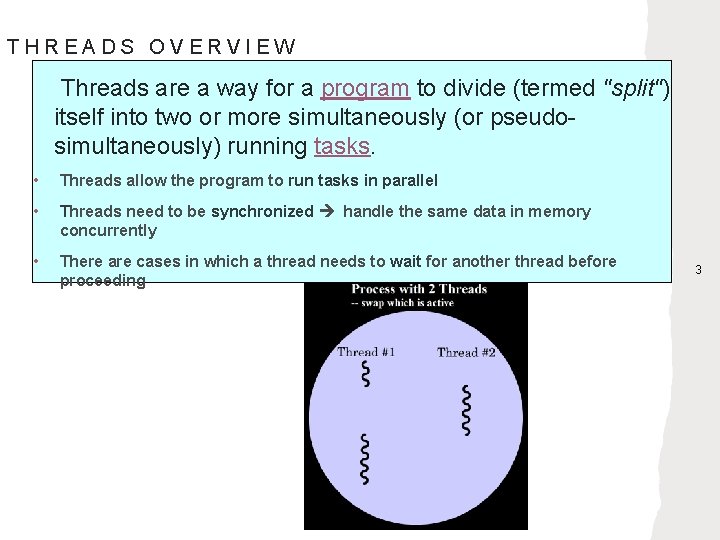
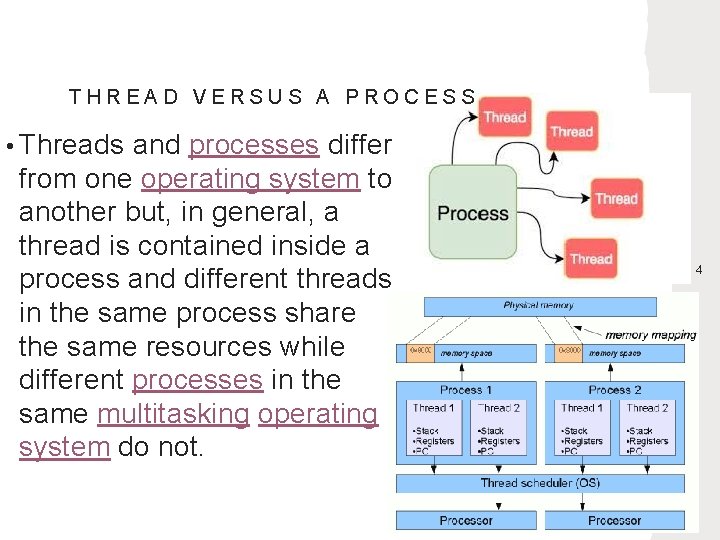
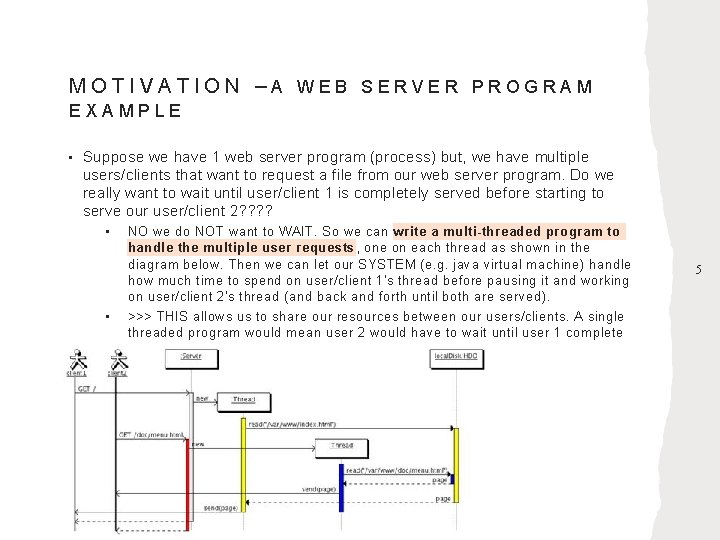
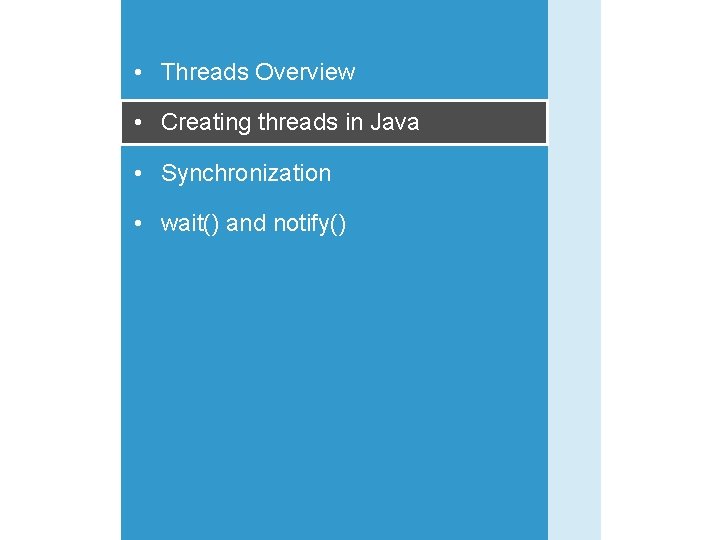
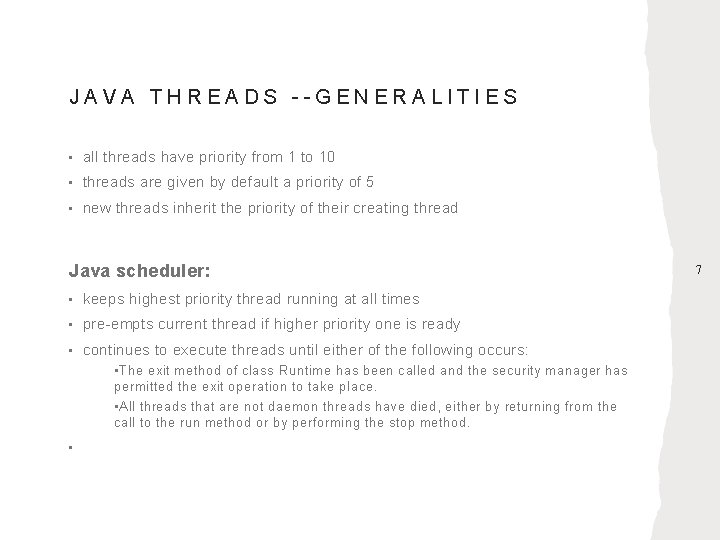
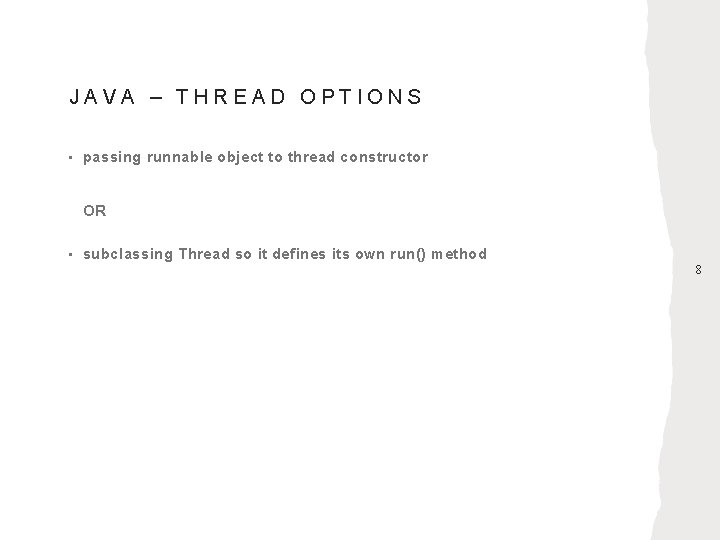
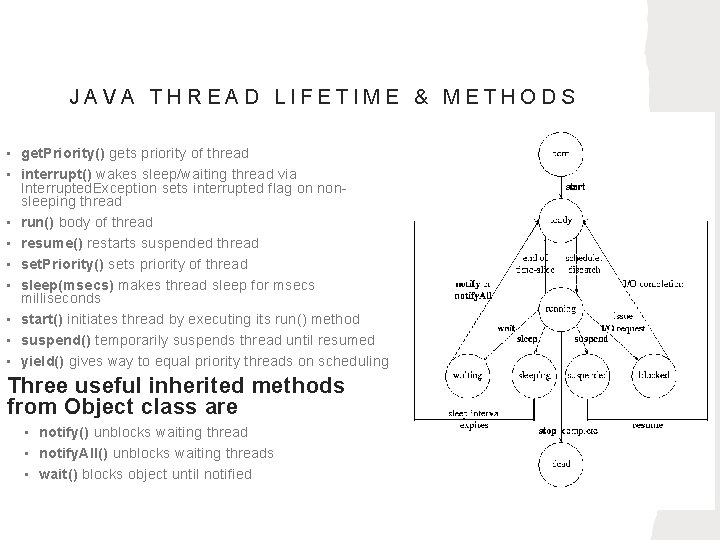
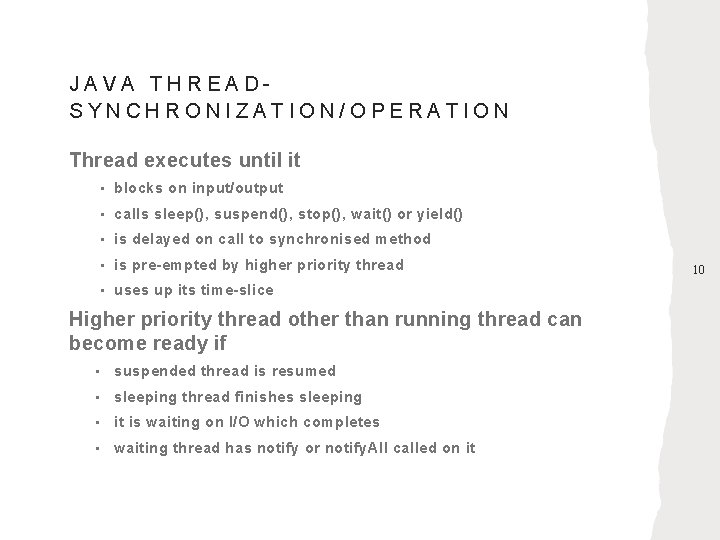
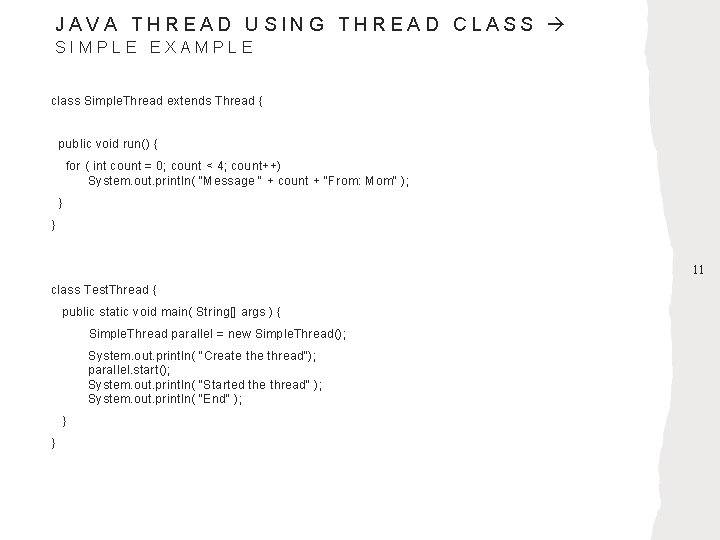
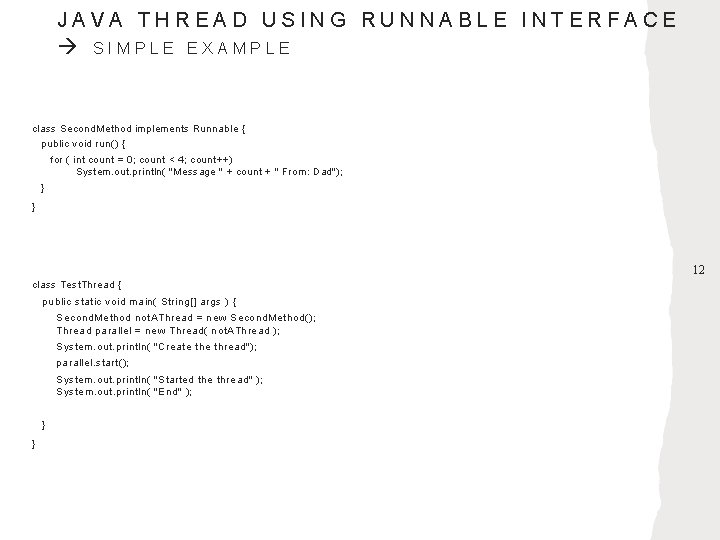
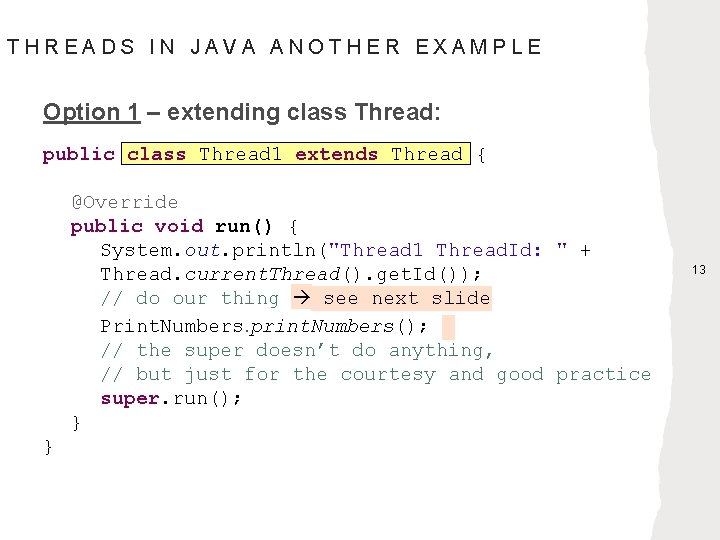
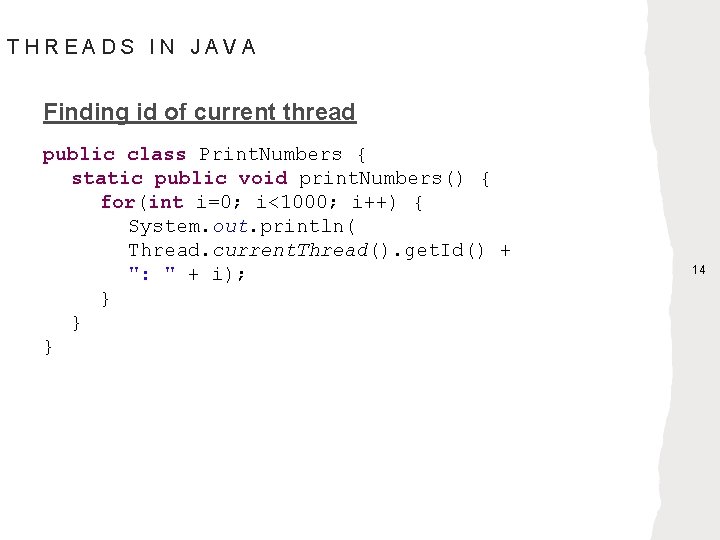
![THREADS IN JAVA Option 1 – extending class Thread (cont’): static public void main(String[] THREADS IN JAVA Option 1 – extending class Thread (cont’): static public void main(String[]](https://slidetodoc.com/presentation_image_h2/59e5a7338f020821eff9ff59d972fd80/image-15.jpg)
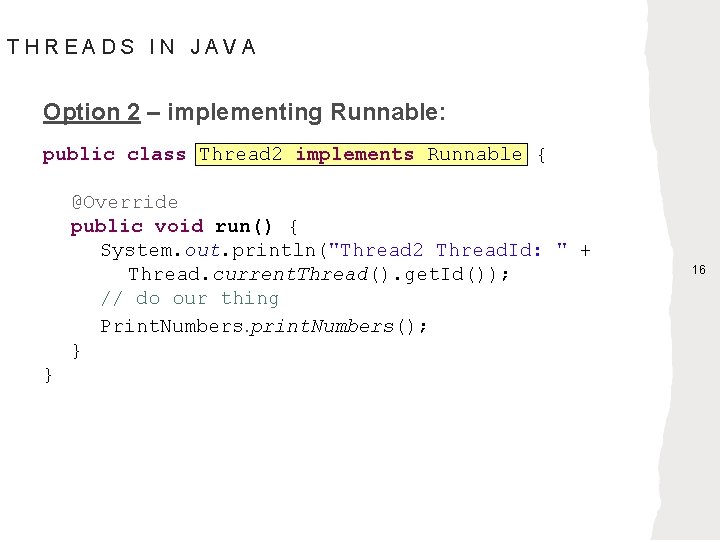
![THREADS IN JAVA Option 2 – implementing Runnable (cont’): static public void main(String[] args) THREADS IN JAVA Option 2 – implementing Runnable (cont’): static public void main(String[] args)](https://slidetodoc.com/presentation_image_h2/59e5a7338f020821eff9ff59d972fd80/image-17.jpg)
![THREADS IN JAVA “Option 3” – implementing Runnable as Anonymous: static public void main(String[] THREADS IN JAVA “Option 3” – implementing Runnable as Anonymous: static public void main(String[]](https://slidetodoc.com/presentation_image_h2/59e5a7338f020821eff9ff59d972fd80/image-18.jpg)
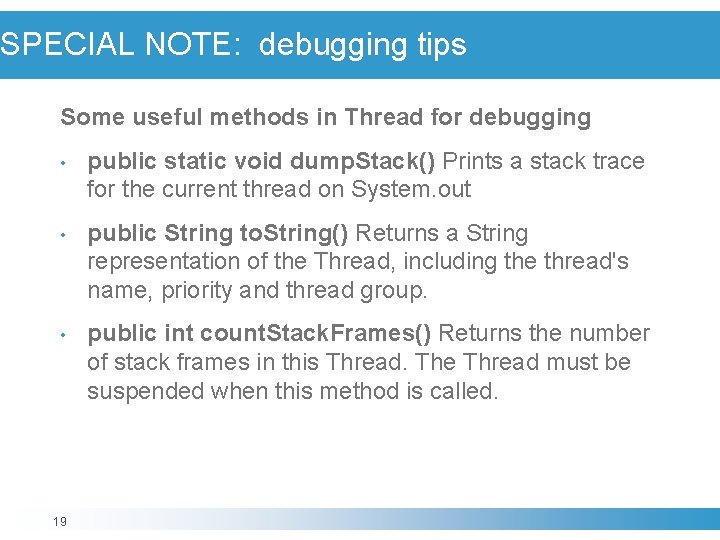
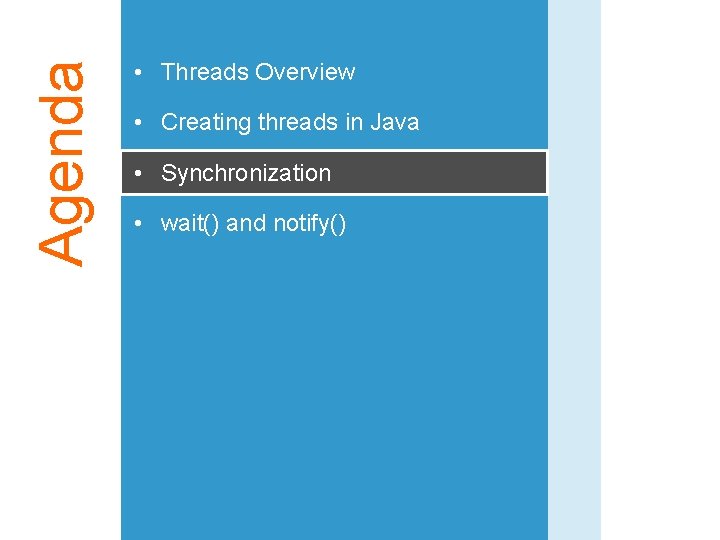
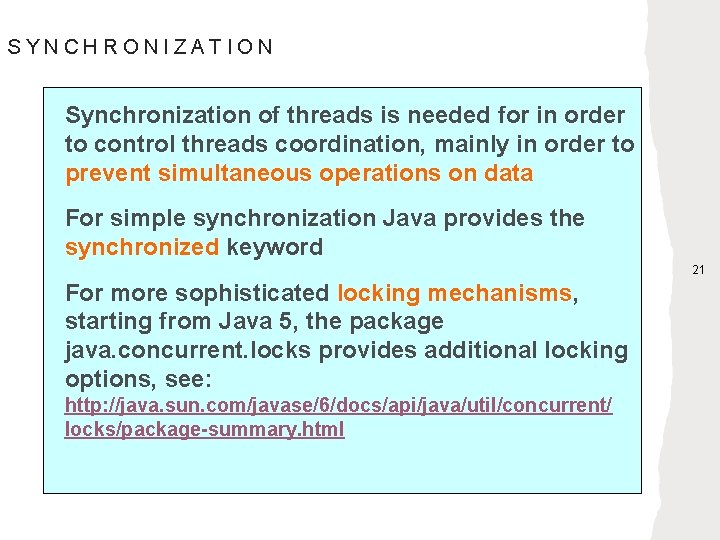
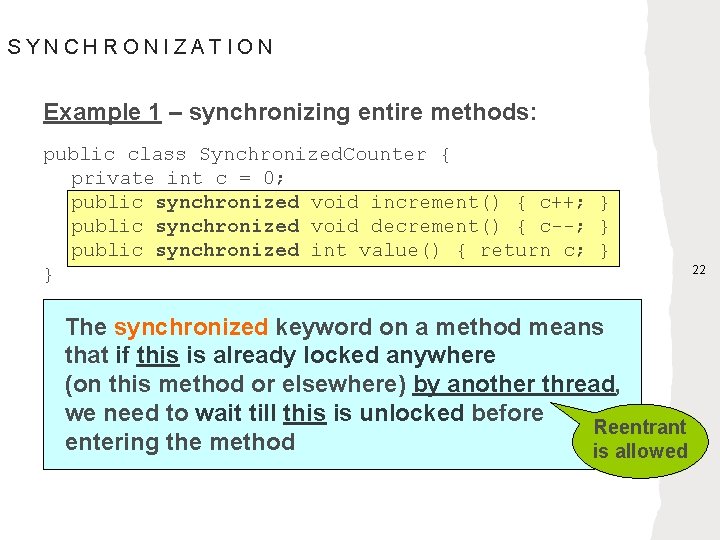
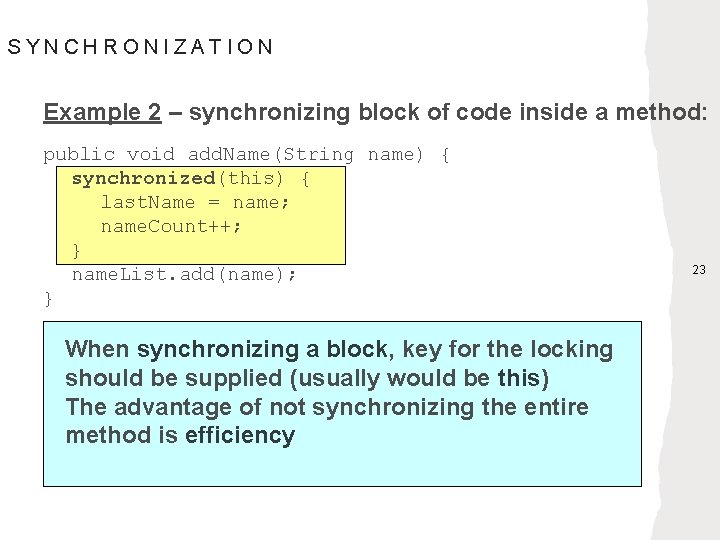
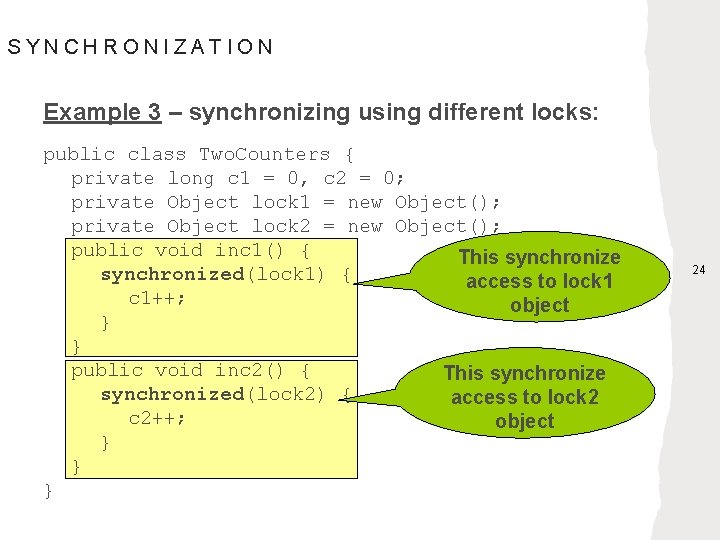
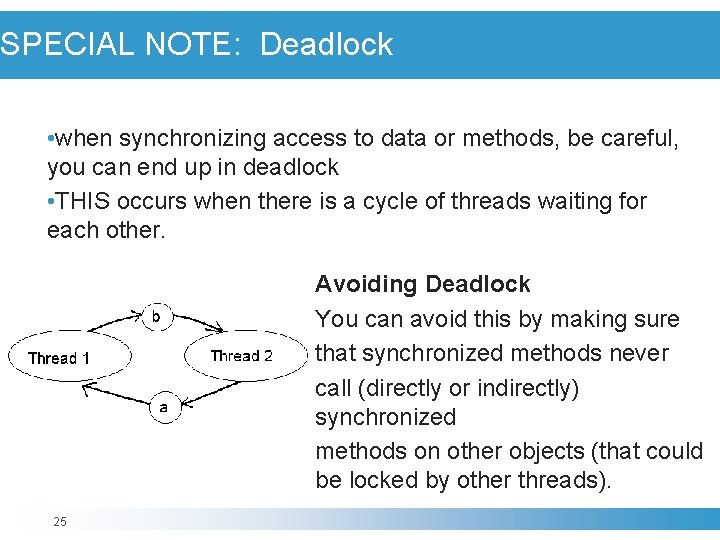
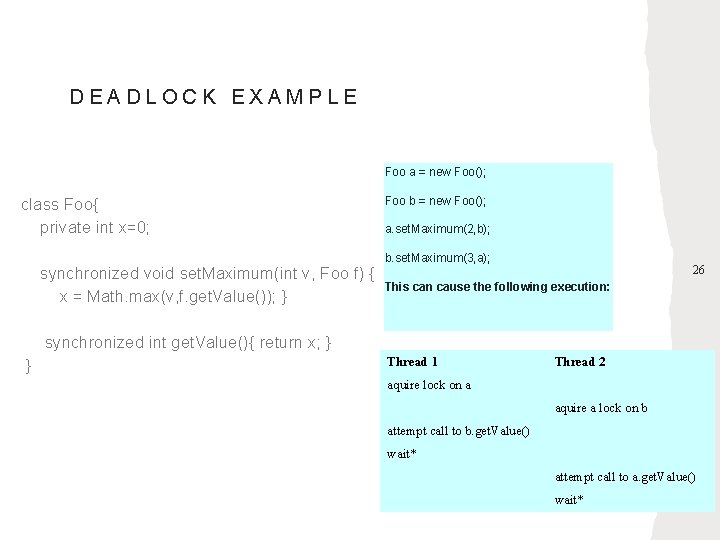
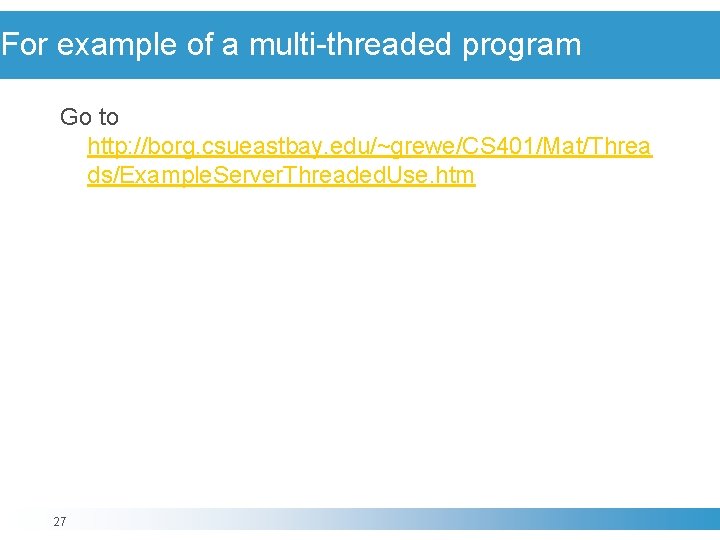
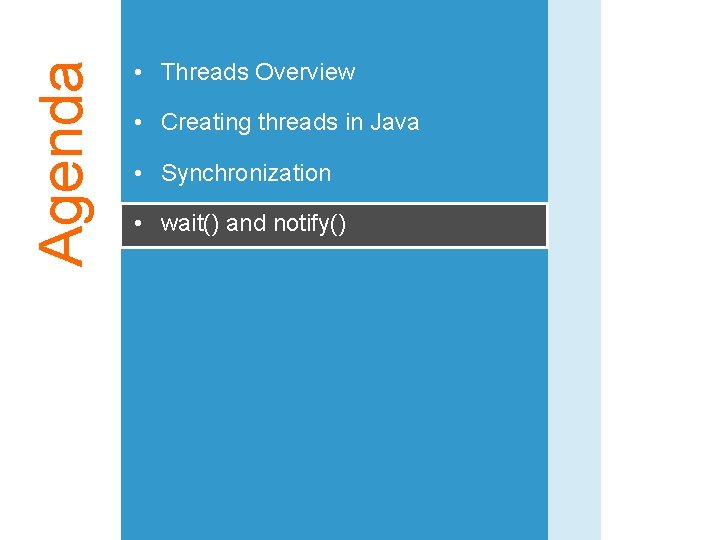
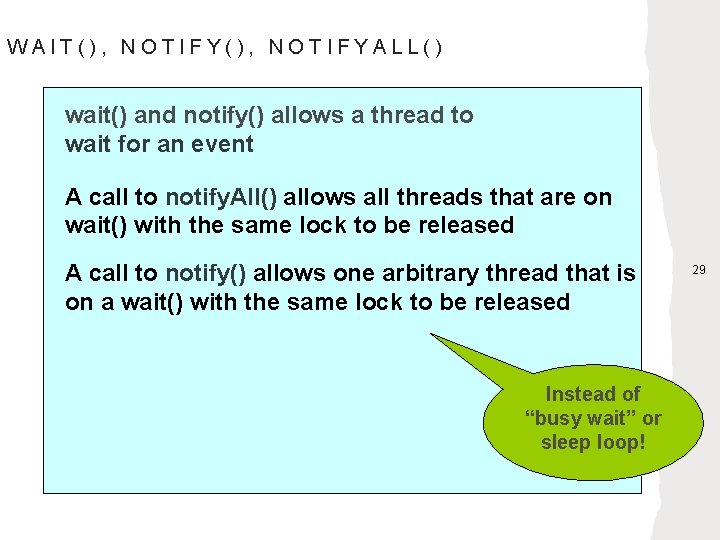
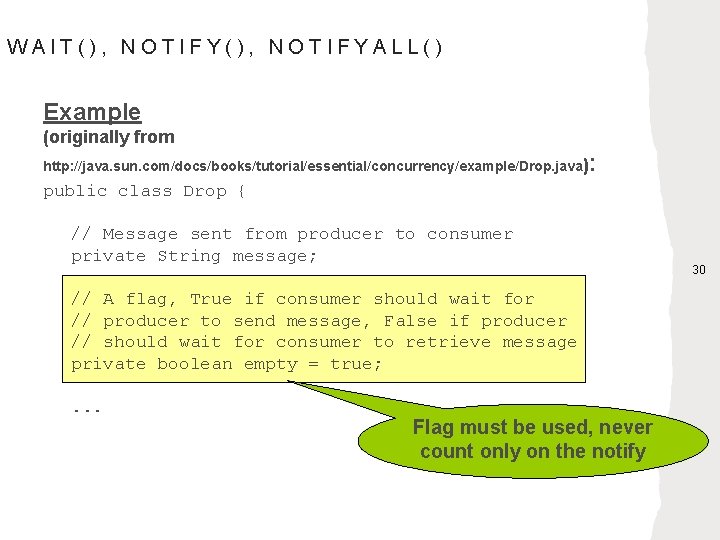
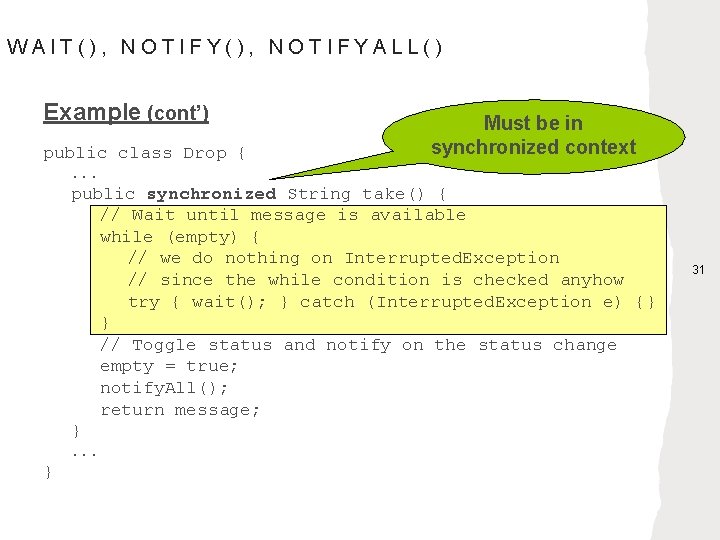
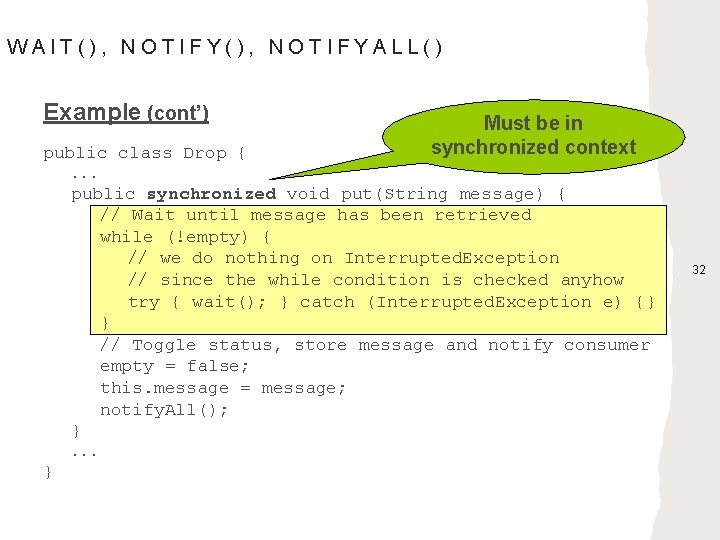
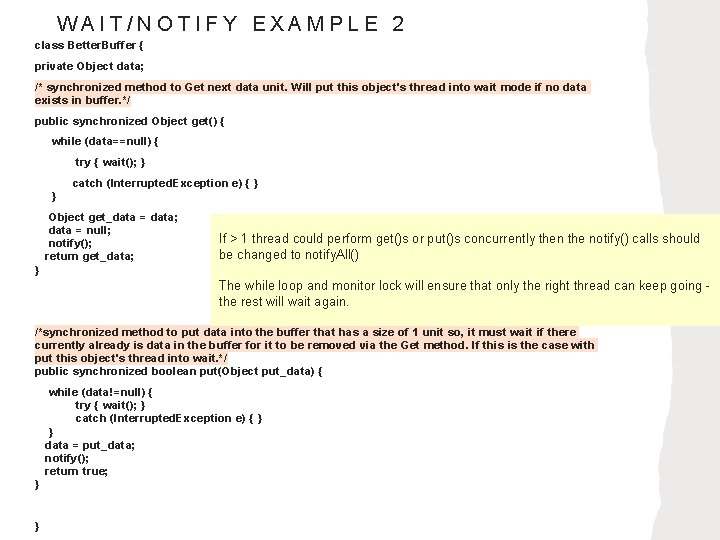
- Slides: 33
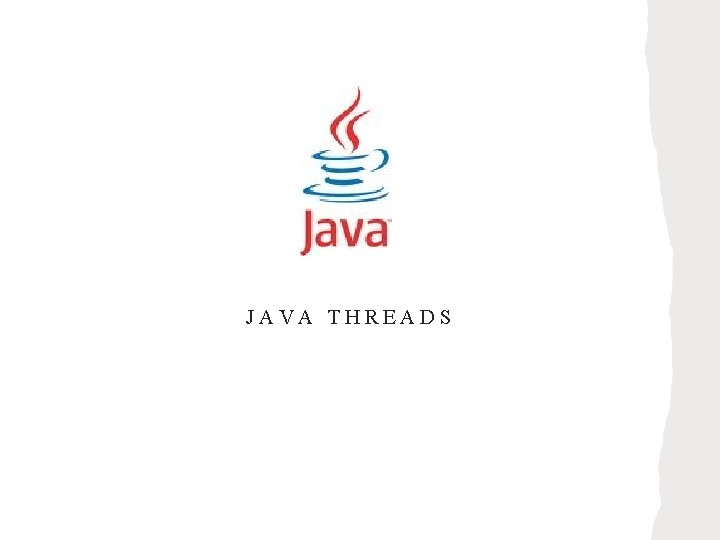
JAVA THREADS
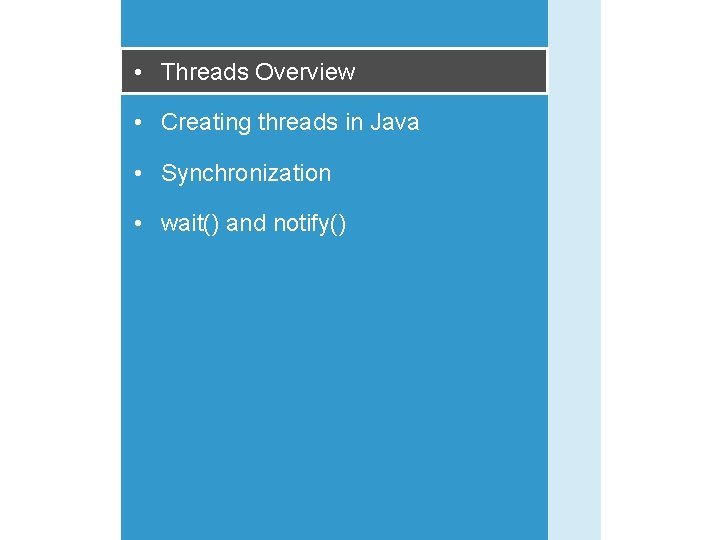
• Threads Overview • Creating threads in Java • Synchronization • wait() and notify()
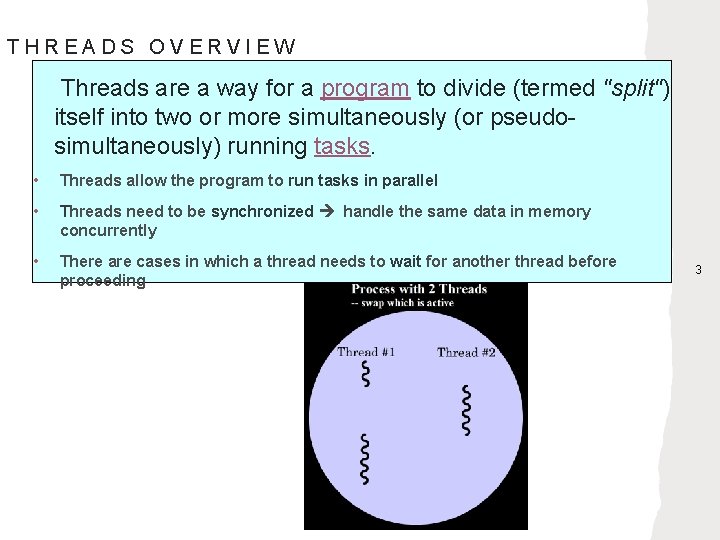
THREADS OVERVIEW Threads are a way for a program to divide (termed "split") itself into two or more simultaneously (or pseudosimultaneously) running tasks. • Threads allow the program to run tasks in parallel • Threads need to be synchronized handle the same data in memory concurrently • There are cases in which a thread needs to wait for another thread before proceeding 3
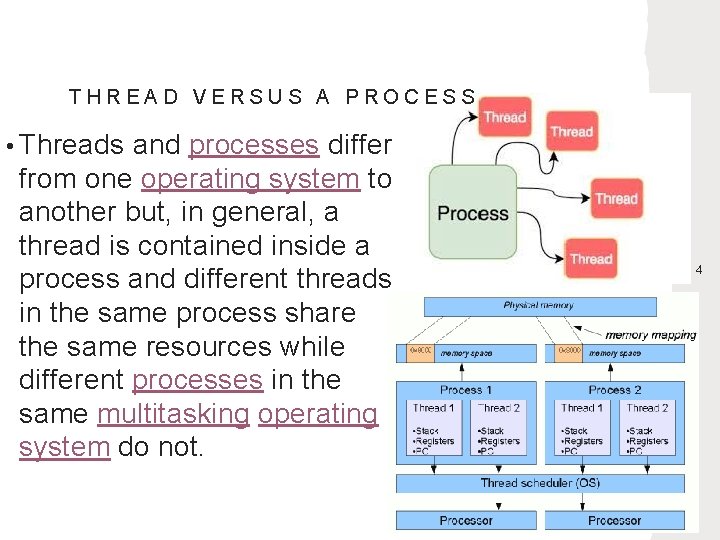
THREAD VERSUS A PROCESS • Threads and processes differ from one operating system to another but, in general, a thread is contained inside a process and different threads in the same process share the same resources while different processes in the same multitasking operating system do not. 4
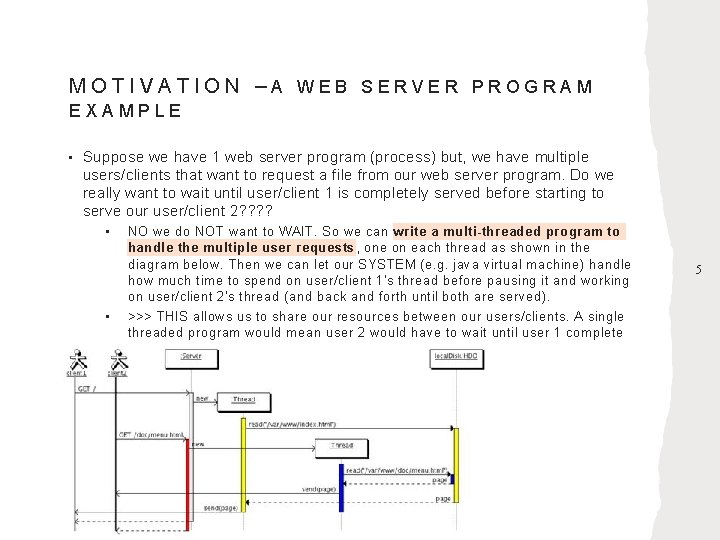
MOTIVATION –A WEB SERVER PROGRAM EXAMPLE • Suppose we have 1 web server program (process) but, we have multiple users/clients that want to request a file from our web server program. Do we really want to wait until user/client 1 is completely served before starting to serve our user/client 2? ? • • NO we do NOT want to WAIT. So we can write a multi-threaded program to handle the multiple user requests, one on each thread as shown in the diagram below. Then we can let our SYSTEM (e. g. java virtual machine) handle how much time to spend on user/client 1's thread before pausing it and working on user/client 2's thread (and back and forth until both are served). >>> THIS allows us to share our resources between our users/clients. A single threaded program would mean user 2 would have to wait until user 1 complete served. 5
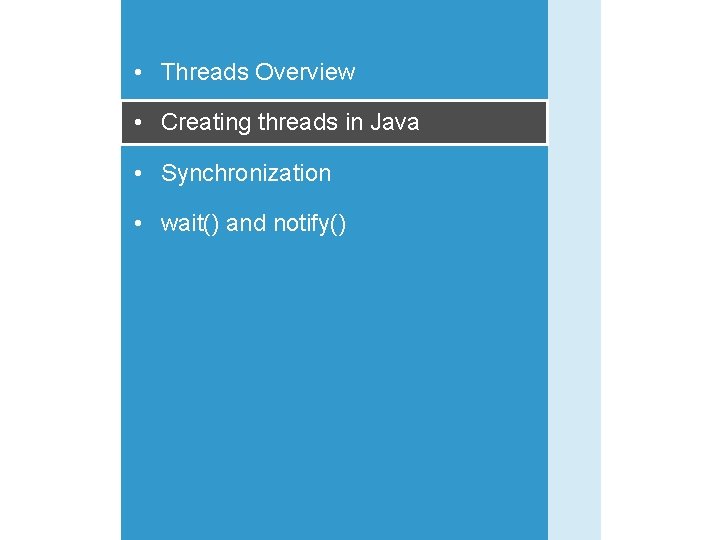
• Threads Overview • Creating threads in Java • Synchronization • wait() and notify()
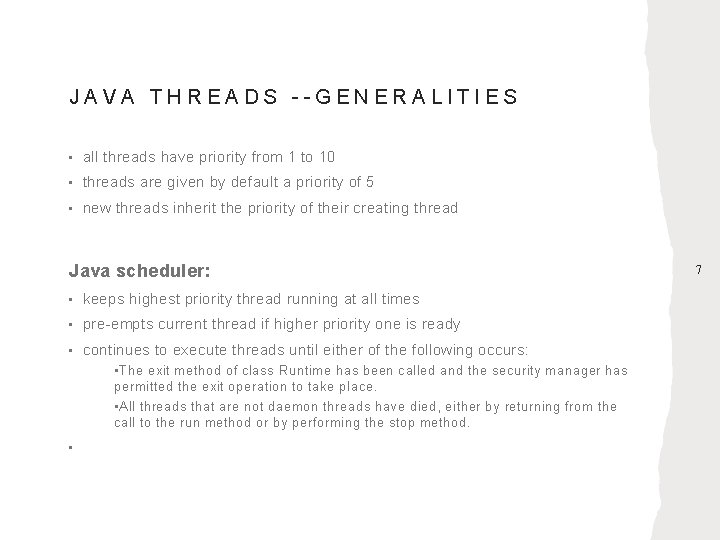
JAVA THREADS --GENERALITIES • all threads have priority from 1 to 10 • threads are given by default a priority of 5 • new threads inherit the priority of their creating thread Java scheduler: • keeps highest priority thread running at all times • pre-empts current thread if higher priority one is ready • continues to execute threads until either of the following occurs: • The exit method of class Runtime has been called and the security manager has permitted the exit operation to take place. • All threads that are not daemon threads have died, either by returning from the call to the run method or by performing the stop method. • 7
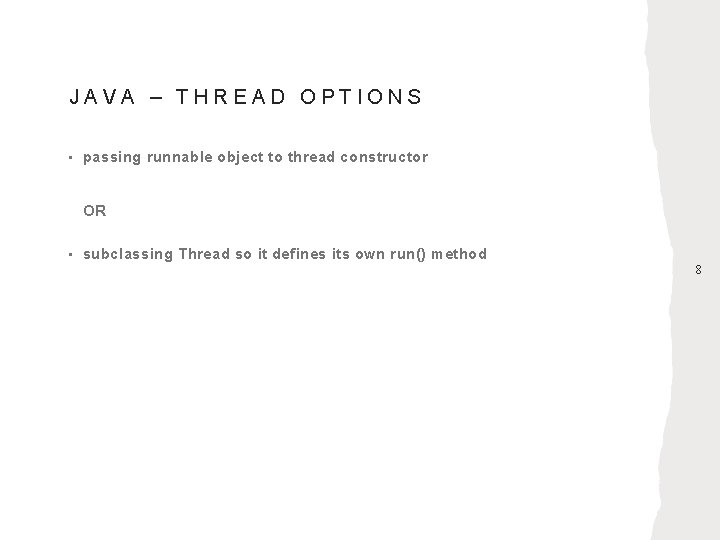
JAVA – THREAD OPTIONS • passing runnable object to thread constructor OR • subclassing Thread so it defines its own run() method 8
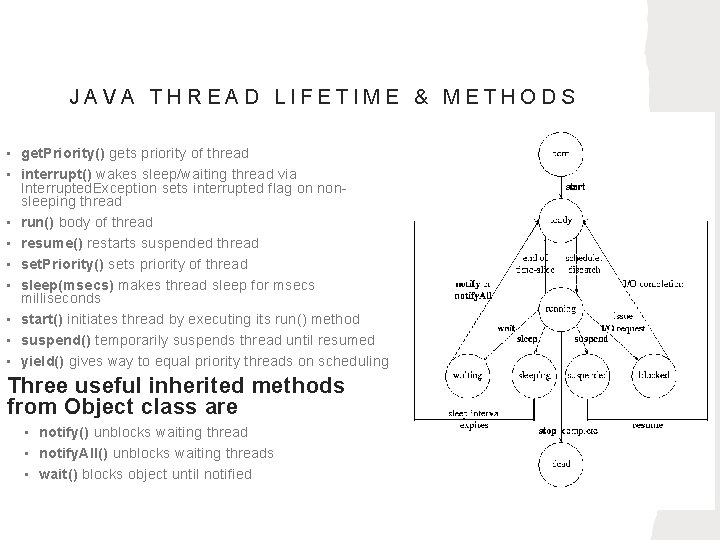
JAVA THREAD LIFETIME & METHODS • get. Priority() gets priority of thread • interrupt() wakes sleep/waiting thread via • • Interrupted. Exception sets interrupted flag on nonsleeping thread run() body of thread resume() restarts suspended thread set. Priority() sets priority of thread sleep(msecs) makes thread sleep for msecs milliseconds start() initiates thread by executing its run() method suspend() temporarily suspends thread until resumed yield() gives way to equal priority threads on scheduling Three useful inherited methods from Object class are • notify() unblocks waiting thread • notify. All() unblocks waiting threads • wait() blocks object until notified 9
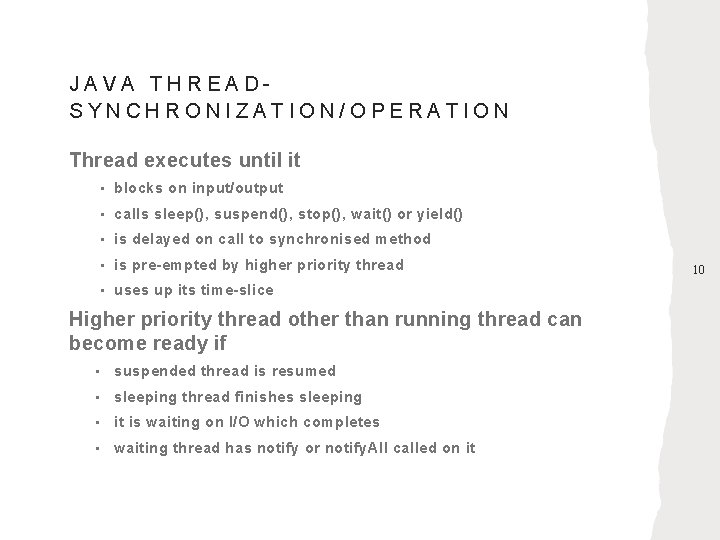
JAVA THREADSYNCHRONIZATION/OPERATION Thread executes until it • blocks on input/output • calls sleep(), suspend(), stop(), wait() or yield() • is delayed on call to synchronised method • is pre-empted by higher priority thread • uses up its time-slice Higher priority thread other than running thread can become ready if • suspended thread is resumed • sleeping thread finishes sleeping • it is waiting on I/O which completes • waiting thread has notify or notify. All called on it 10
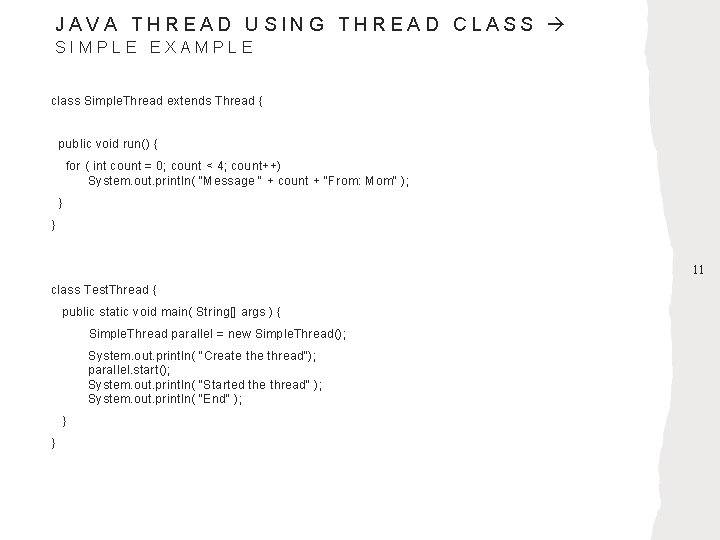
JAVA THREAD USING THREAD CLASS SIMPLE EXAMPLE class Simple. Thread extends Thread { public void run() { for ( int count = 0; count < 4; count++) System. out. println( "Message " + count + "From: Mom" ); } } 11 class Test. Thread { public static void main( String[] args ) { Simple. Thread parallel = new Simple. Thread(); System. out. println( "Create thread"); parallel. start(); System. out. println( "Started the thread" ); System. out. println( "End" ); } }
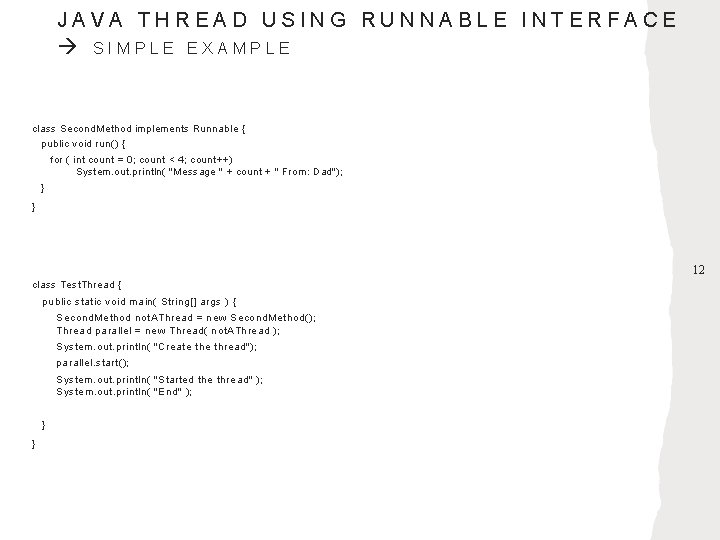
JAVA THREAD USING RUNNABLE INTERFACE SIMPLE EXAMPLE class Second. Method implements Runnable { public void run() { for ( int count = 0; count < 4; count++) System. out. println( "Message " + count + " From: Dad"); } } 12 class Test. Thread { public static void main( String[] args ) { Second. Method not. AThread = new Second. Method(); Thread parallel = new Thread( not. AThread ); System. out. println( "Create thread"); parallel. start(); System. out. println( "Started the thread" ); System. out. println( "End" ); } }
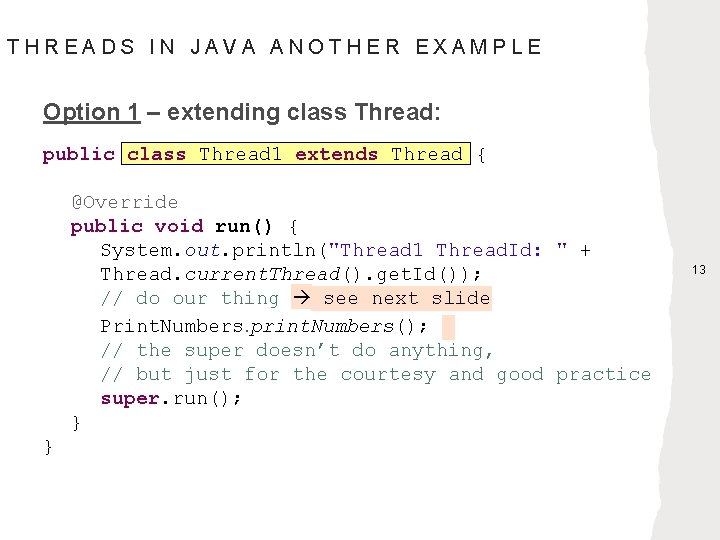
THREADS IN JAVA ANOTHER EXAMPLE Option 1 – extending class Thread: public class Thread 1 extends Thread { @Override public void run() { System. out. println("Thread 1 Thread. Id: " + Thread. current. Thread(). get. Id()); // do our thing see next slide Print. Numbers. print. Numbers(); // the super doesn’t do anything, // but just for the courtesy and good practice super. run(); } } 13
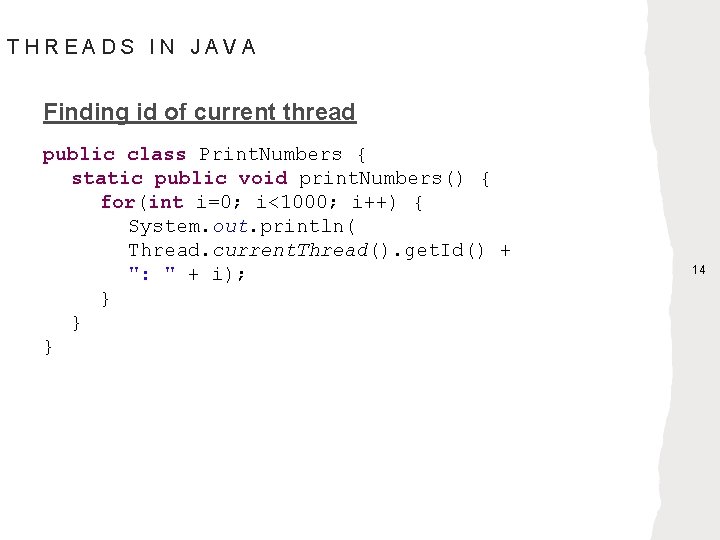
THREADS IN JAVA Finding id of current thread public class Print. Numbers { static public void print. Numbers() { for(int i=0; i<1000; i++) { System. out. println( Thread. current. Thread(). get. Id() + ": " + i); } } } 14
![THREADS IN JAVA Option 1 extending class Thread cont static public void mainString THREADS IN JAVA Option 1 – extending class Thread (cont’): static public void main(String[]](https://slidetodoc.com/presentation_image_h2/59e5a7338f020821eff9ff59d972fd80/image-15.jpg)
THREADS IN JAVA Option 1 – extending class Thread (cont’): static public void main(String[] args) { System. out. println("Main Thread. Id: " + Thread. current. Thread(). get. Id()); for(int i=0; i<3; i++) { new Thread 1(). start(); // don't call run! // (if you want a separate thread) } print. Numbers(); } 15
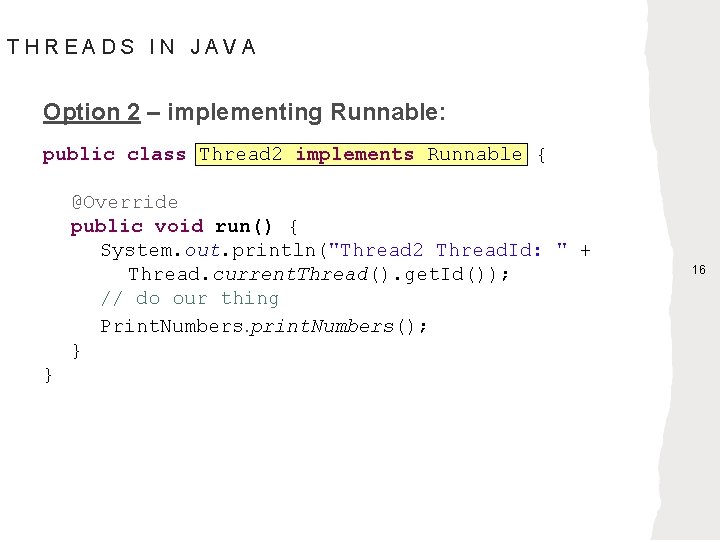
THREADS IN JAVA Option 2 – implementing Runnable: public class Thread 2 implements Runnable { @Override public void run() { System. out. println("Thread 2 Thread. Id: " + Thread. current. Thread(). get. Id()); // do our thing Print. Numbers. print. Numbers(); } } 16
![THREADS IN JAVA Option 2 implementing Runnable cont static public void mainString args THREADS IN JAVA Option 2 – implementing Runnable (cont’): static public void main(String[] args)](https://slidetodoc.com/presentation_image_h2/59e5a7338f020821eff9ff59d972fd80/image-17.jpg)
THREADS IN JAVA Option 2 – implementing Runnable (cont’): static public void main(String[] args) { System. out. println("Main Thread. Id: " + Thread. current. Thread(). get. Id()); for(int i=0; i<3; i++) { new Thread(new Thread 2()). start(); // again, don't call run! // (if you want a separate thread) } print. Numbers(); } 17
![THREADS IN JAVA Option 3 implementing Runnable as Anonymous static public void mainString THREADS IN JAVA “Option 3” – implementing Runnable as Anonymous: static public void main(String[]](https://slidetodoc.com/presentation_image_h2/59e5a7338f020821eff9ff59d972fd80/image-18.jpg)
THREADS IN JAVA “Option 3” – implementing Runnable as Anonymous: static public void main(String[] args) { System. out. println("Main Thread. Id: " + Thread. current. Thread(). get. Id()); new Thread(new Runnable() { @Override public void run() { System. out. println("Thread 3 Thread. Id: " + Thread. current. Thread(). get. Id()); // do our thing print. Numbers(); } }). start(); // don't call run!. . . print. Numbers(); } 18
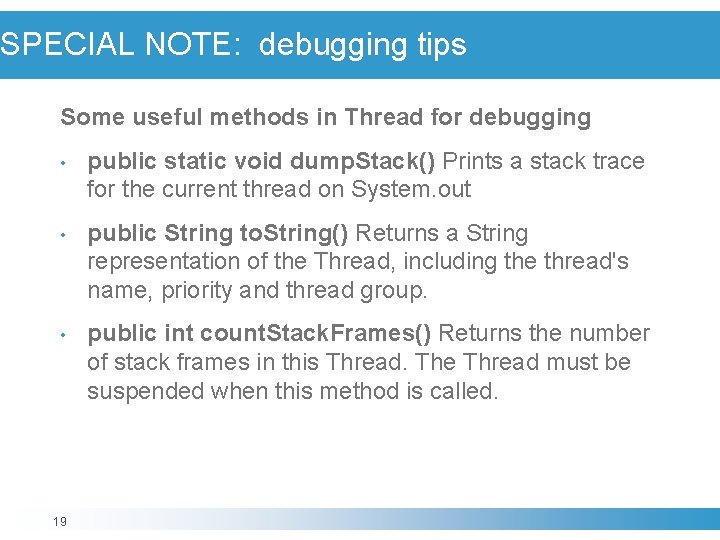
SPECIAL NOTE: debugging tips Some useful methods in Thread for debugging • public static void dump. Stack() Prints a stack trace for the current thread on System. out • public String to. String() Returns a String representation of the Thread, including the thread's name, priority and thread group. • public int count. Stack. Frames() Returns the number of stack frames in this Thread. The Thread must be suspended when this method is called. 19
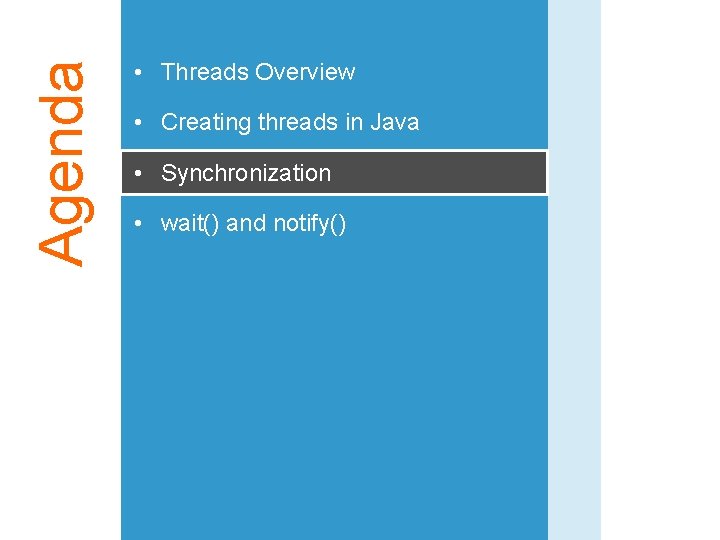
Agenda • Threads Overview • Creating threads in Java • Synchronization • wait() and notify()
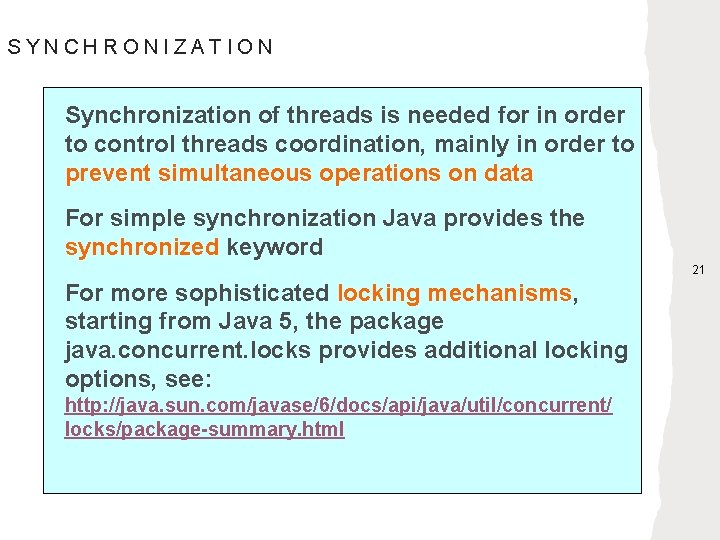
SYNCHRONIZATION Synchronization of threads is needed for in order to control threads coordination, mainly in order to prevent simultaneous operations on data For simple synchronization Java provides the synchronized keyword 21 For more sophisticated locking mechanisms, starting from Java 5, the package java. concurrent. locks provides additional locking options, see: http: //java. sun. com/javase/6/docs/api/java/util/concurrent/ locks/package-summary. html
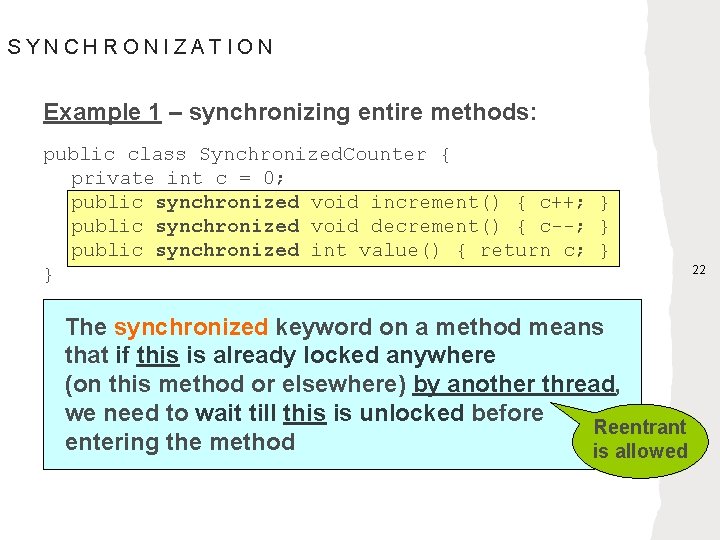
SYNCHRONIZATION Example 1 – synchronizing entire methods: public class Synchronized. Counter { private int c = 0; public synchronized void increment() { c++; } public synchronized void decrement() { c--; } public synchronized int value() { return c; } } The synchronized keyword on a method means that if this is already locked anywhere (on this method or elsewhere) by another thread, we need to wait till this is unlocked before Reentrant entering the method is allowed 22
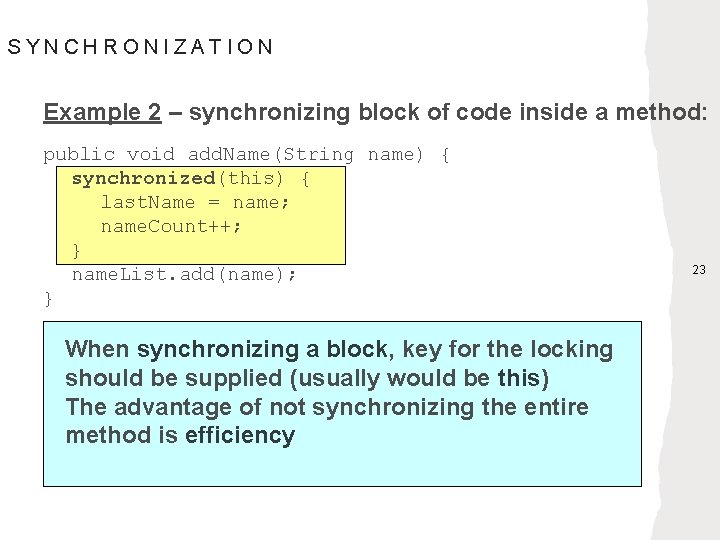
SYNCHRONIZATION Example 2 – synchronizing block of code inside a method: public void add. Name(String name) { synchronized(this) { last. Name = name; name. Count++; } name. List. add(name); } When synchronizing a block, key for the locking should be supplied (usually would be this) The advantage of not synchronizing the entire method is efficiency 23
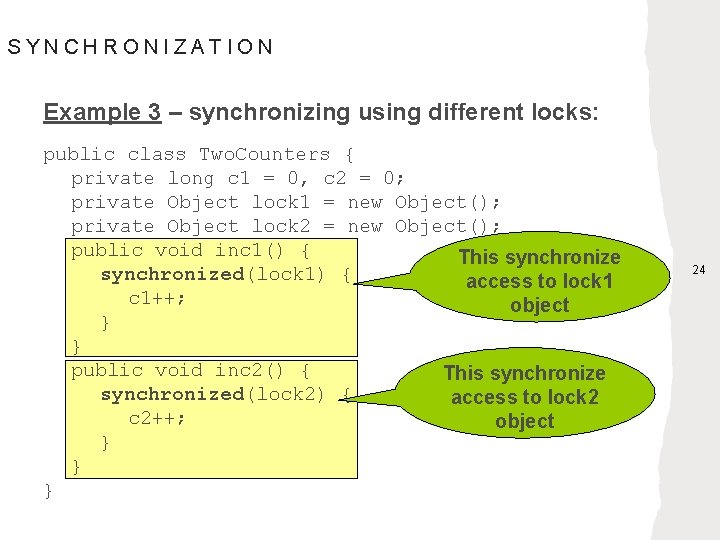
SYNCHRONIZATION Example 3 – synchronizing using different locks: public class Two. Counters { private long c 1 = 0, c 2 = 0; private Object lock 1 = new Object(); private Object lock 2 = new Object(); public void inc 1() { This synchronized(lock 1) { access to lock 1 c 1++; object } } public void inc 2() { This synchronized(lock 2) { access to lock 2 c 2++; object } } } 24
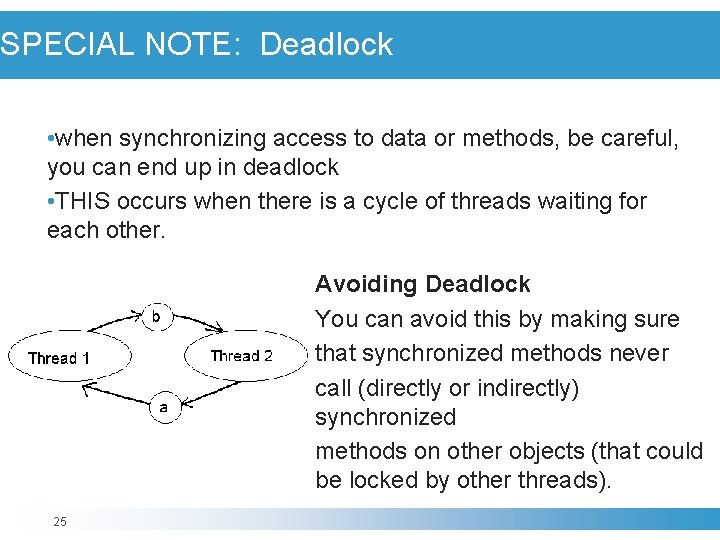
SPECIAL NOTE: Deadlock • when synchronizing access to data or methods, be careful, you can end up in deadlock • THIS occurs when there is a cycle of threads waiting for each other. Avoiding Deadlock You can avoid this by making sure that synchronized methods never call (directly or indirectly) synchronized methods on other objects (that could be locked by other threads). 25
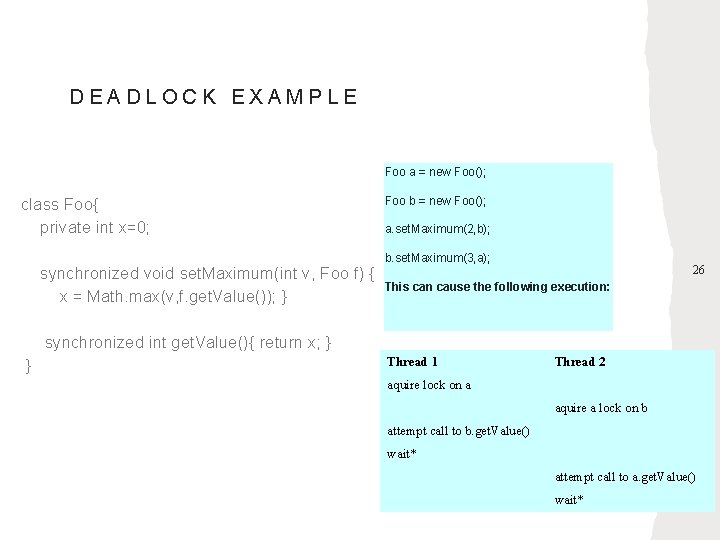
DEADLOCK EXAMPLE Foo a = new Foo(); class Foo{ private int x=0; synchronized void set. Maximum(int v, Foo f) { x = Math. max(v, f. get. Value()); } Foo b = new Foo(); a. set. Maximum(2, b); b. set. Maximum(3, a); 26 This can cause the following execution: synchronized int get. Value(){ return x; } } Thread 1 Thread 2 aquire lock on a aquire a lock on b attempt call to b. get. Value() wait* attempt call to a. get. Value() wait*
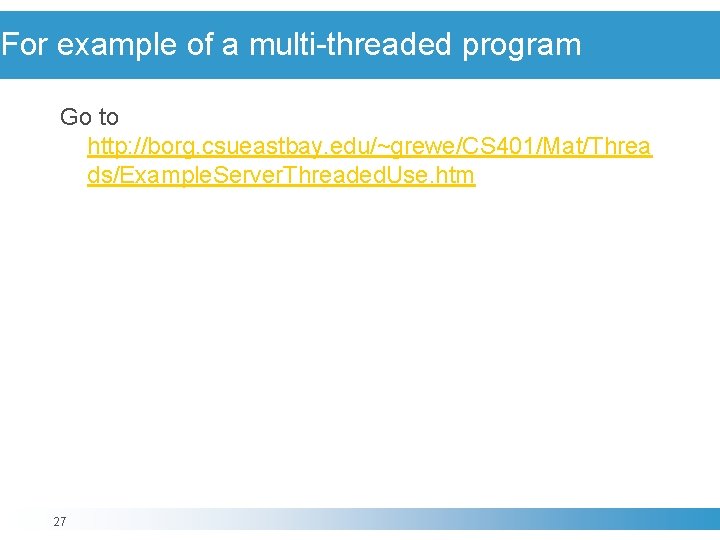
For example of a multi-threaded program Go to http: //borg. csueastbay. edu/~grewe/CS 401/Mat/Threa ds/Example. Server. Threaded. Use. htm 27
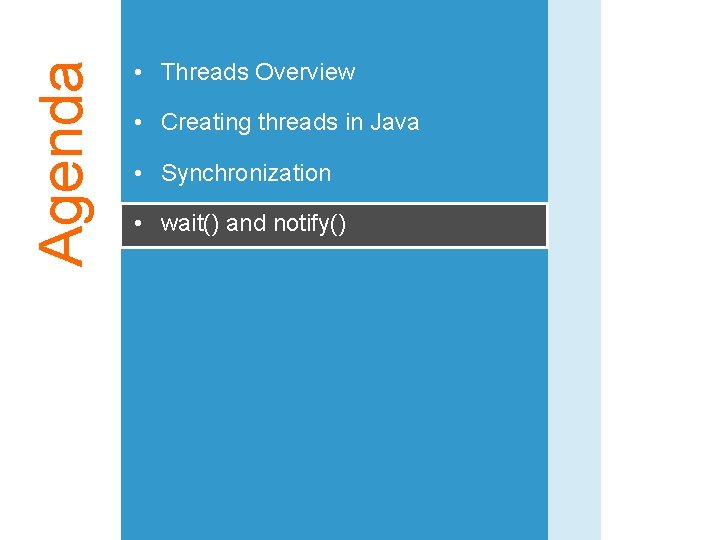
Agenda • Threads Overview • Creating threads in Java • Synchronization • wait() and notify()
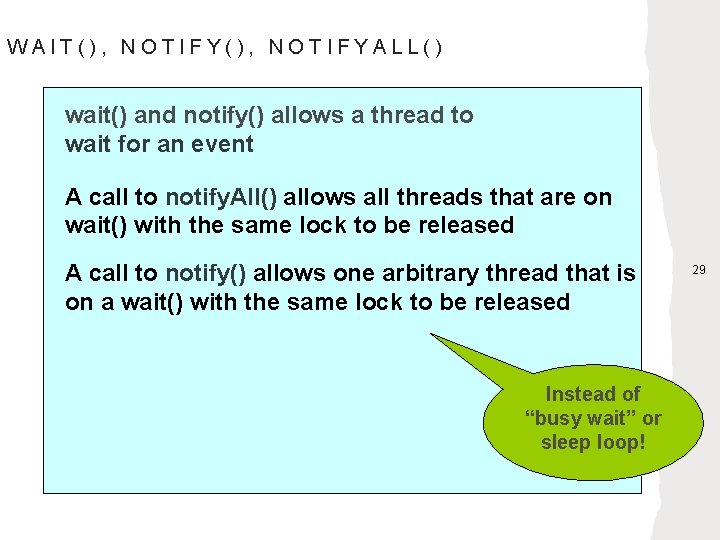
WAIT(), NOTIFYALL() wait() and notify() allows a thread to wait for an event A call to notify. All() allows all threads that are on wait() with the same lock to be released A call to notify() allows one arbitrary thread that is on a wait() with the same lock to be released Instead of “busy wait” or sleep loop! 29
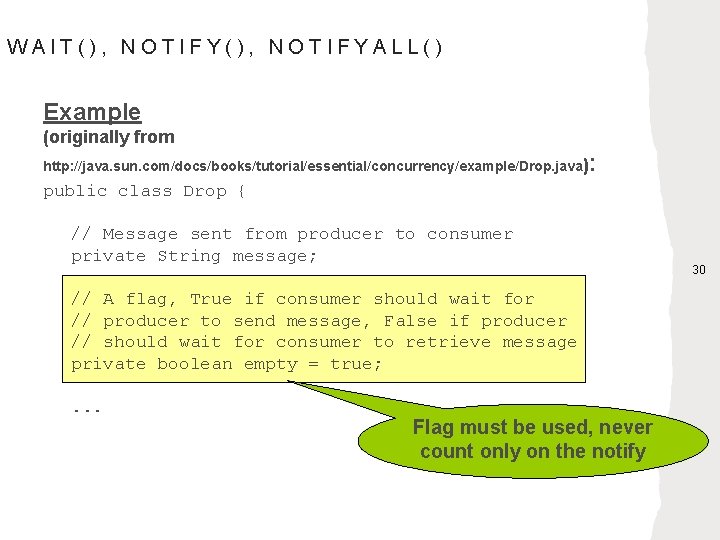
WAIT(), NOTIFYALL() Example (originally from http: //java. sun. com/docs/books/tutorial/essential/concurrency/example/Drop. java ) : public class Drop { // Message sent from producer to consumer private String message; // A flag, True if consumer should wait for // producer to send message, False if producer // should wait for consumer to retrieve message private boolean empty = true; . . . Flag must be used, never count only on the notify 30
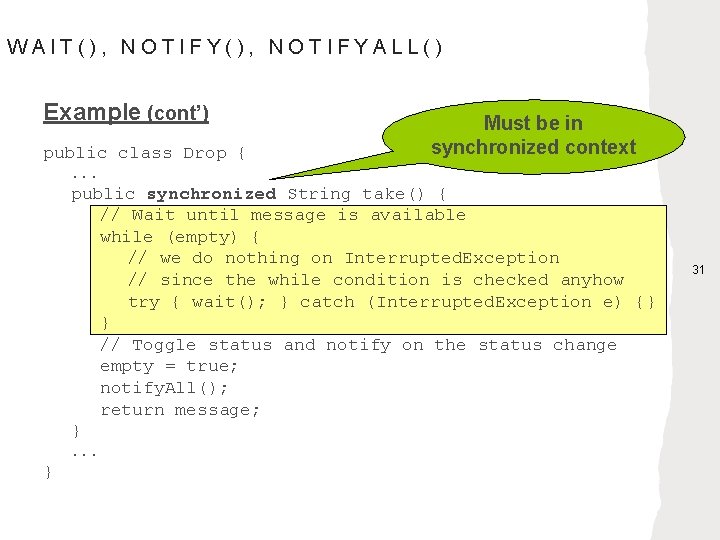
WAIT(), NOTIFYALL() Example (cont’) public class Drop { Must be in synchronized context . . . public synchronized String take() { // Wait until message is available while (empty) { // we do nothing on Interrupted. Exception // since the while condition is checked anyhow try { wait(); } catch (Interrupted. Exception e) {} } // Toggle status and notify on the status change empty = true; notify. All(); return message; }. . . } 31
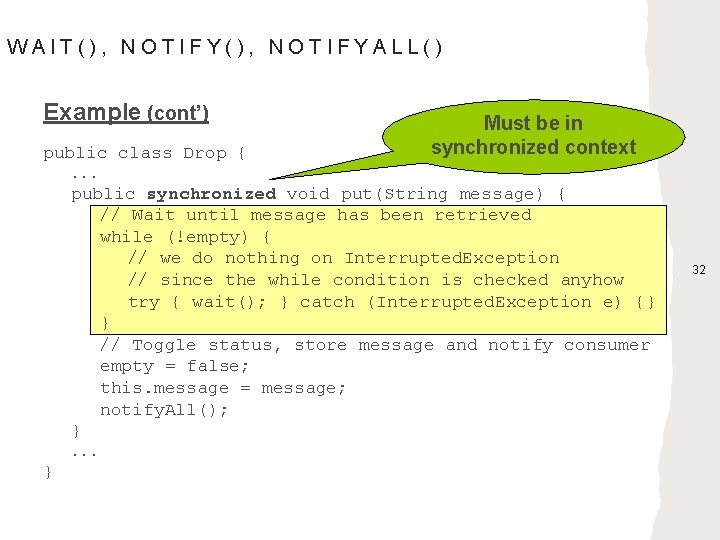
WAIT(), NOTIFYALL() Example (cont’) public class Drop { Must be in synchronized context . . . public synchronized void put(String message) { // Wait until message has been retrieved while (!empty) { // we do nothing on Interrupted. Exception // since the while condition is checked anyhow try { wait(); } catch (Interrupted. Exception e) {} } // Toggle status, store message and notify consumer empty = false; this. message = message; notify. All(); }. . . } 32
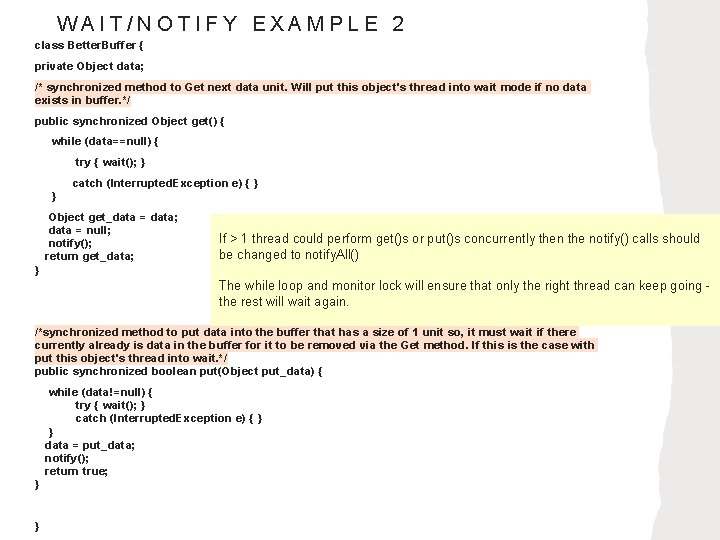
WAIT/NOTIFY EXAMPLE 2 class Better. Buffer { private Object data; /* synchronized method to Get next data unit. Will put this object's thread into wait mode if no data exists in buffer. */ public synchronized Object get() { while (data==null) { try { wait(); } catch (Interrupted. Exception e) { } } Object get_data = data; data = null; notify(); return get_data; If > 1 thread could perform get()s or put()s concurrently then the notify() calls should be changed to notify. All() 33 } The while loop and monitor lock will ensure that only the right thread can keep going the rest will wait again. /*synchronized method to put data into the buffer that has a size of 1 unit so, it must wait if there currently already is data in the buffer for it to be removed via the Get method. If this is the case with put this object's thread into wait. */ public synchronized boolean put(Object put_data) { while (data!=null) { try { wait(); } catch (Interrupted. Exception e) { } } data = put_data; notify(); return true; } }
Threads java
Threads em java
Java shared memory
Java collections overview
Advantages of user level threads
Syncthreads
Threads cannot be implemented as a library
Escalonamento por prioridades
Threads fiji
Ece threads
Cuprintf
Needle like threads of spongy bone
Internal screw thread
Forum.unity.com/threads/game-over.54735
Posix threads in os
Pintos threads
Threads in distributed systems
Threads.h
Process and threads
Mustafa cem kasapbaşı
Chia 3 threads
Threads cannot be implemented as a library
Waxy coated paper which transfers pattern markings
Process thread
Process and threads
Osteospermum white lightning
Mission thread example
Ece threads
Carriage of a lathe consists of__________.
Os threads
Sockets and threads
Priority donation pintos
Threads vs processes
Two level model thread