Java Threads Introduction Processes and Threads Process 1
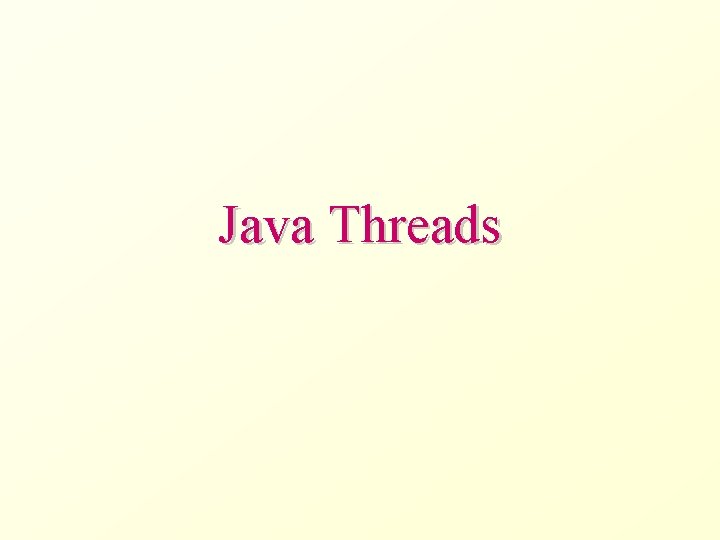
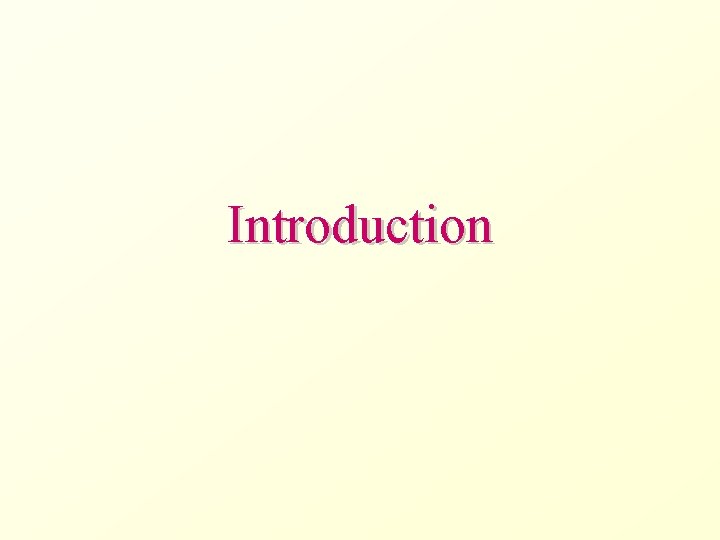
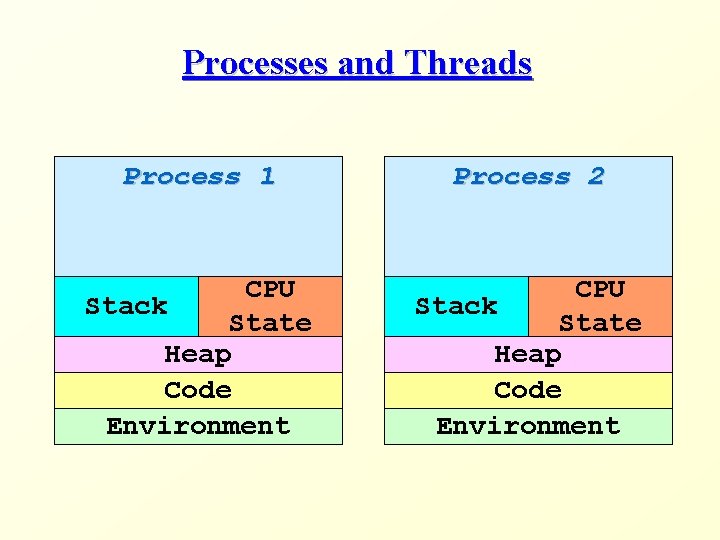
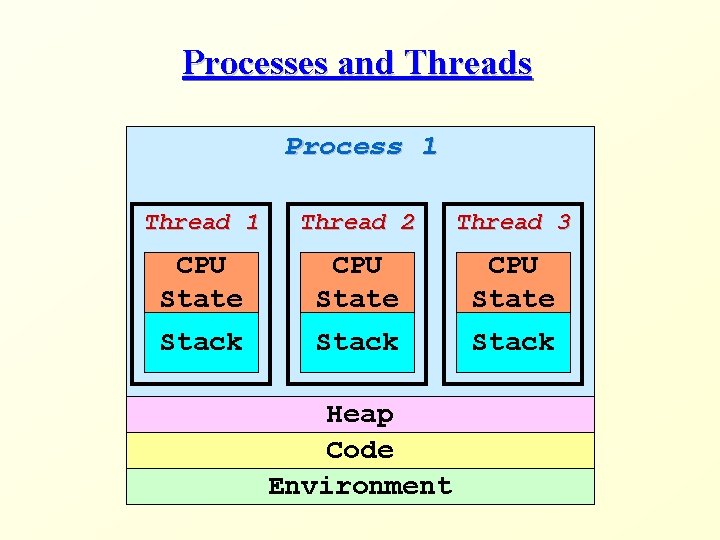
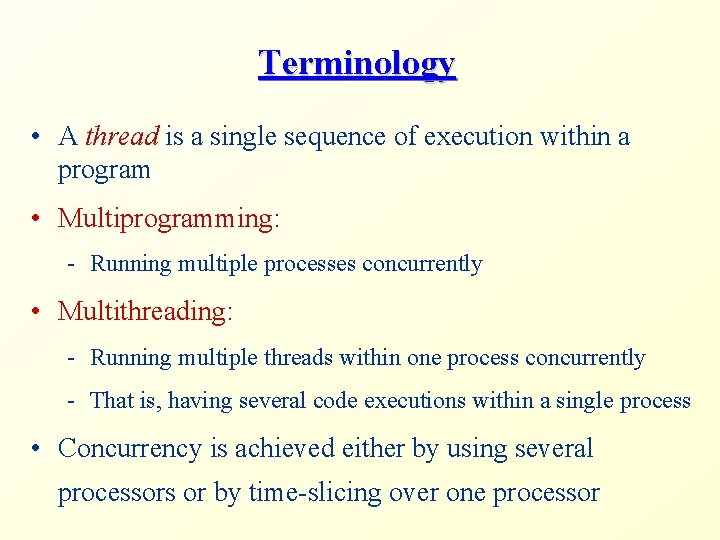
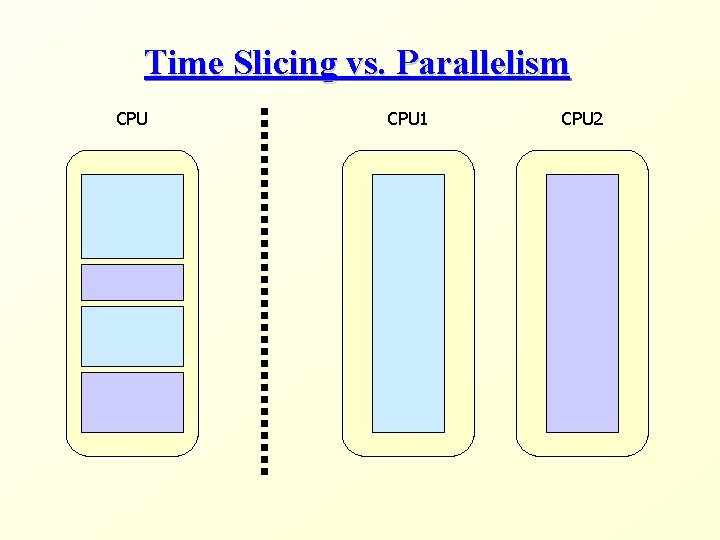
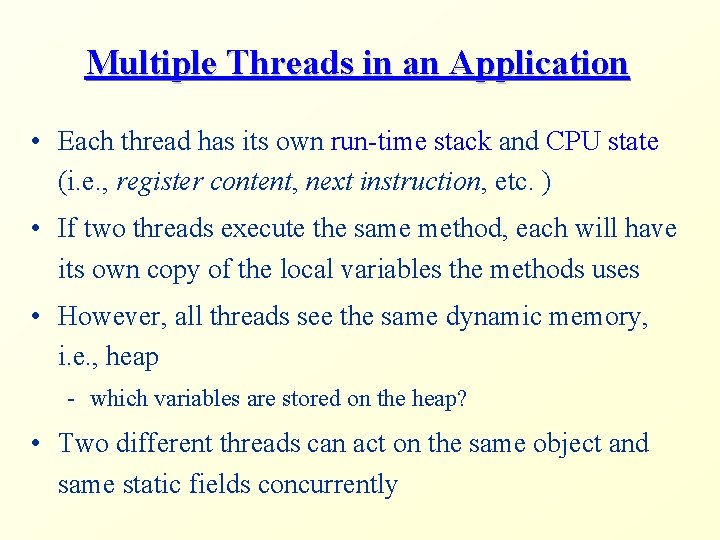
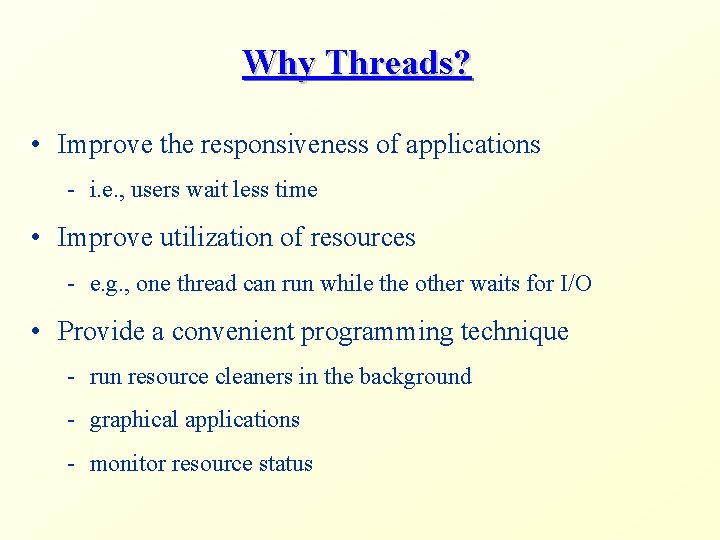
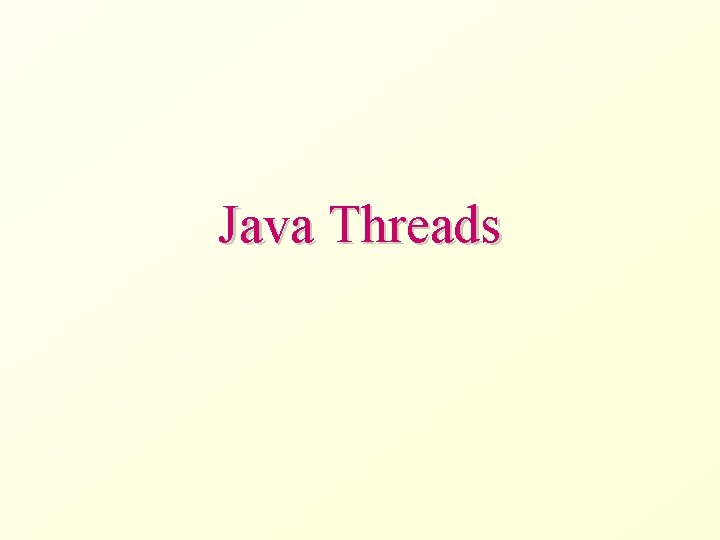
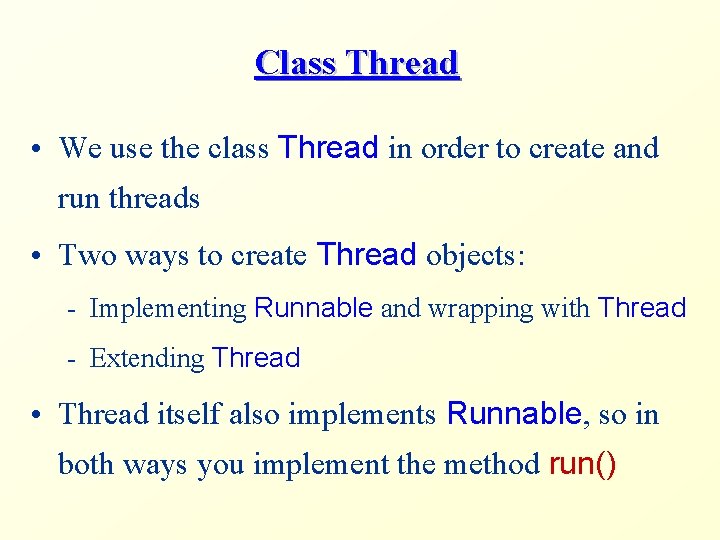
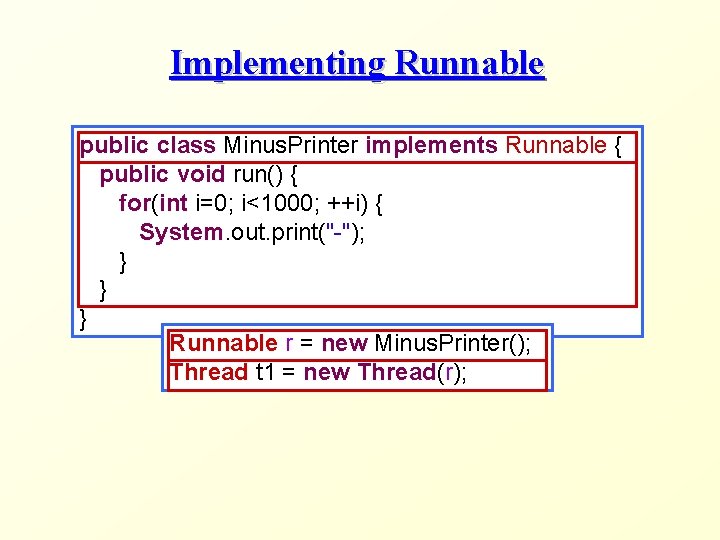
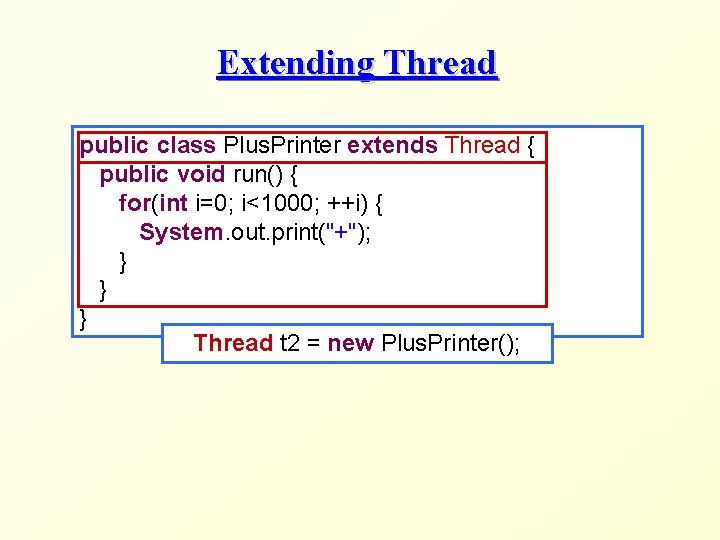
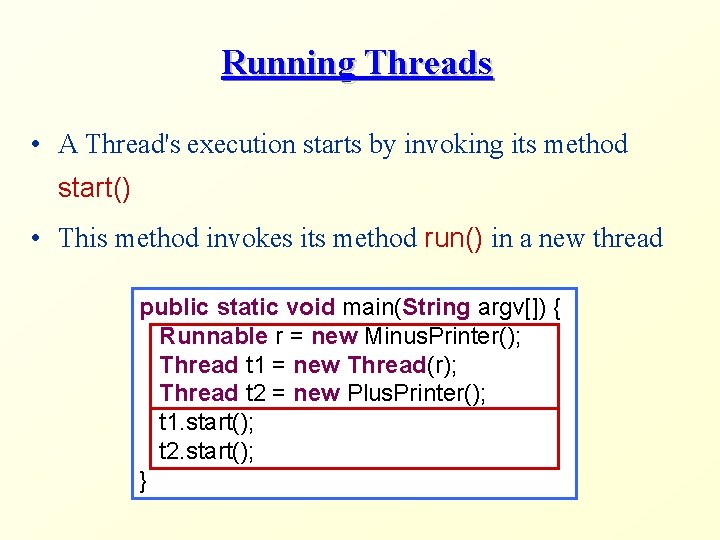
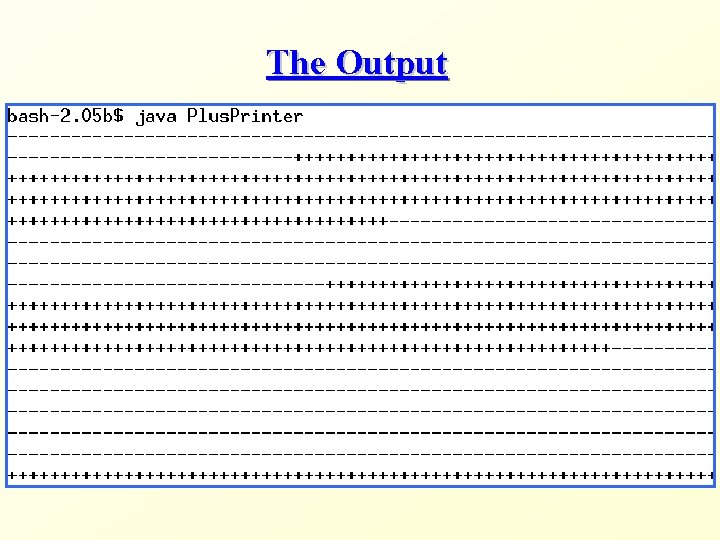
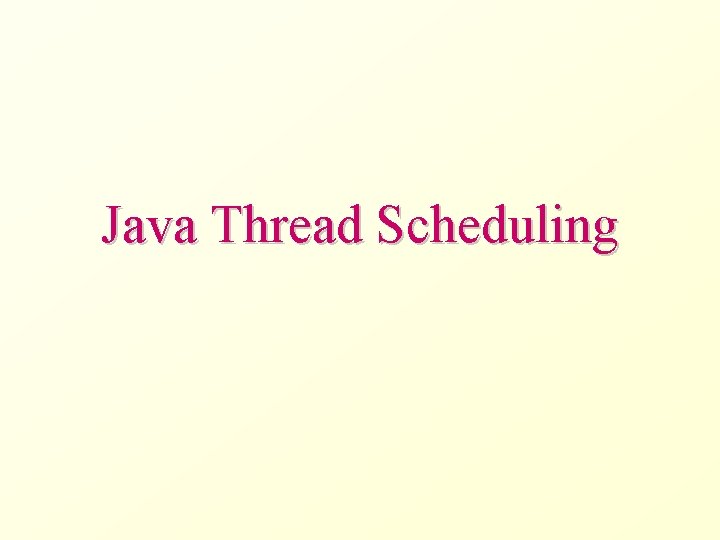
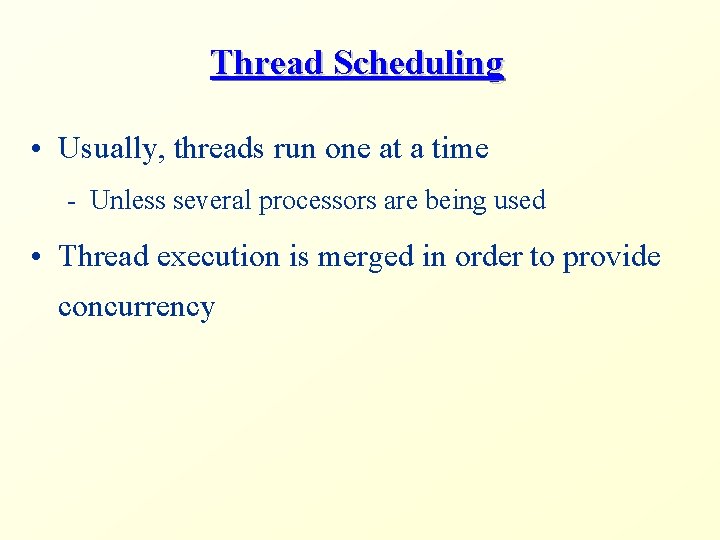
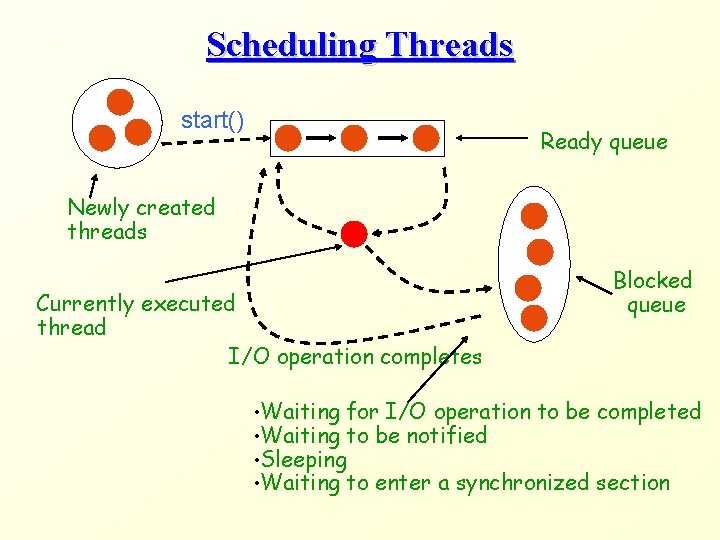
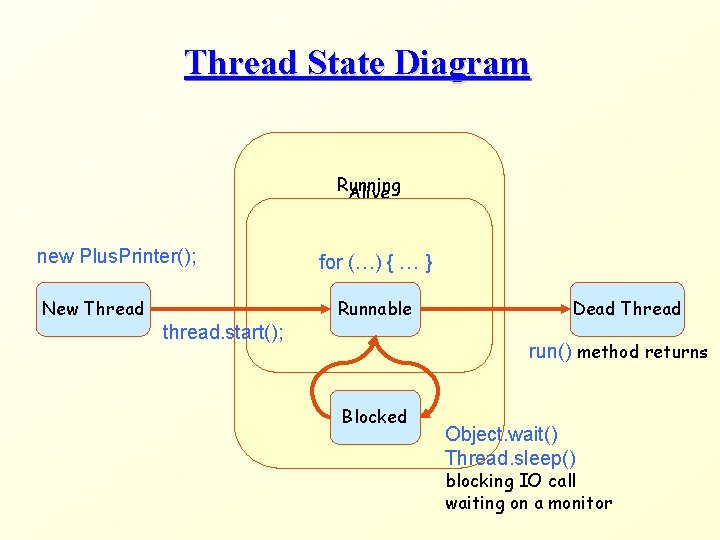
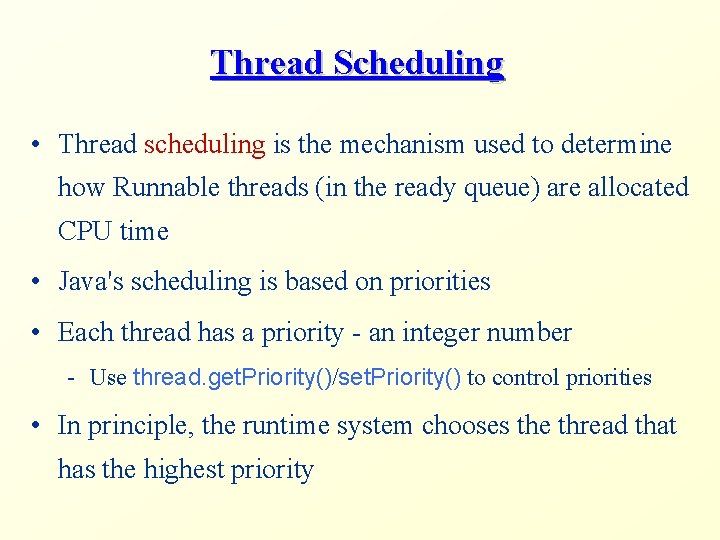
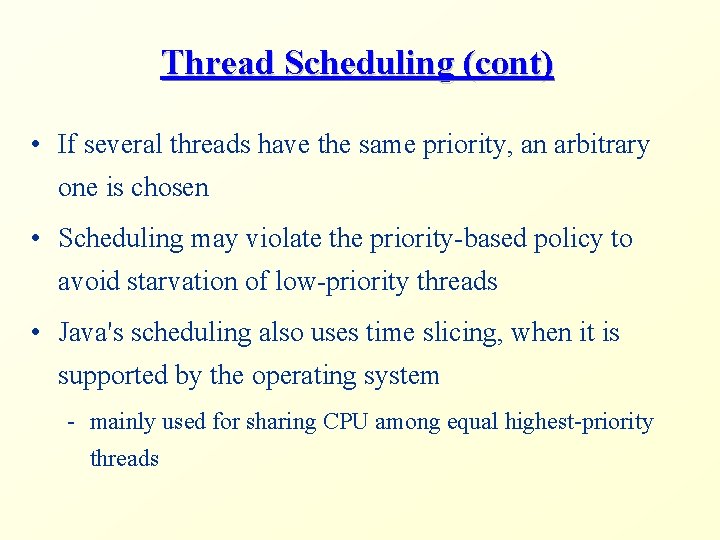
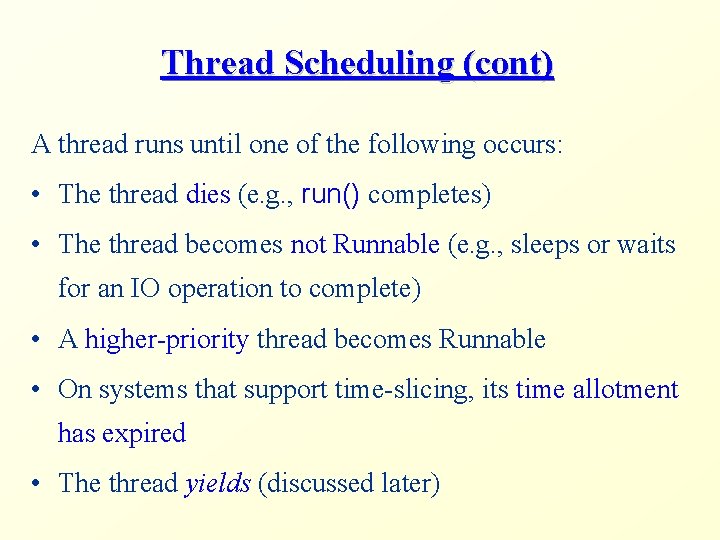
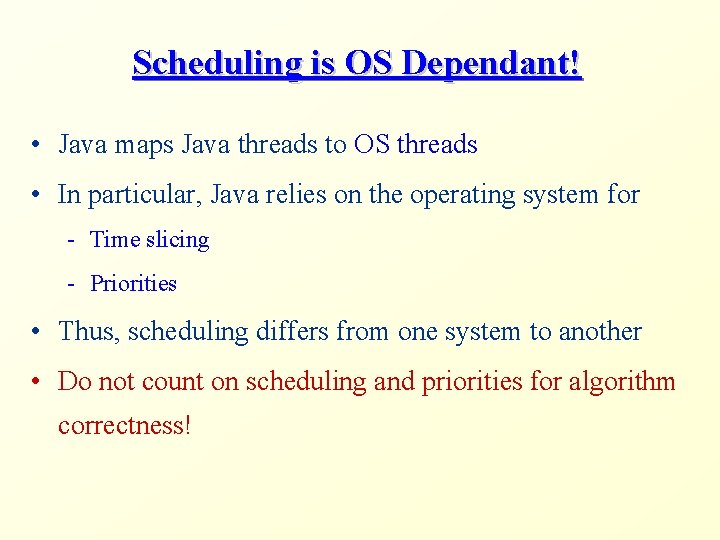
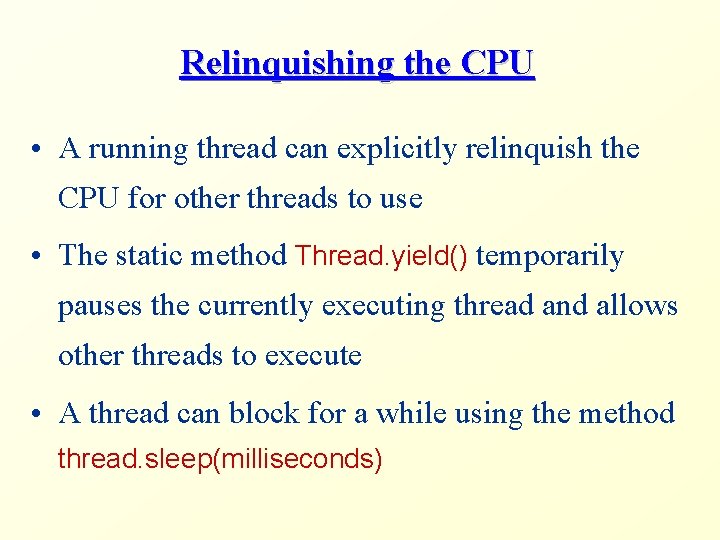
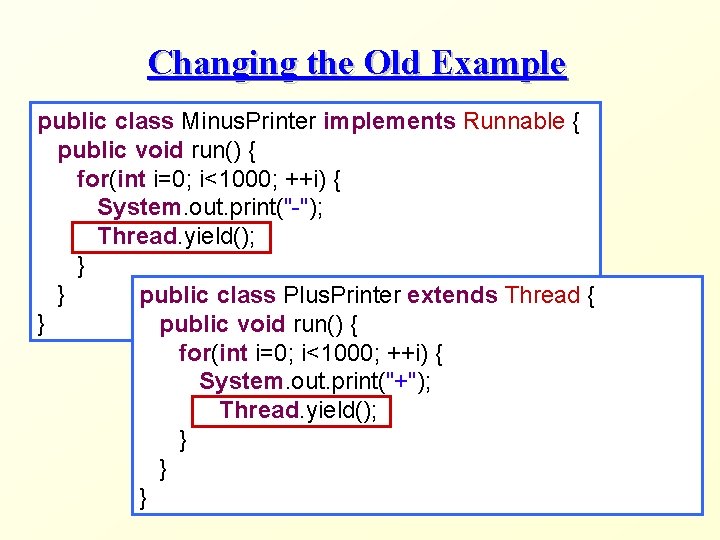
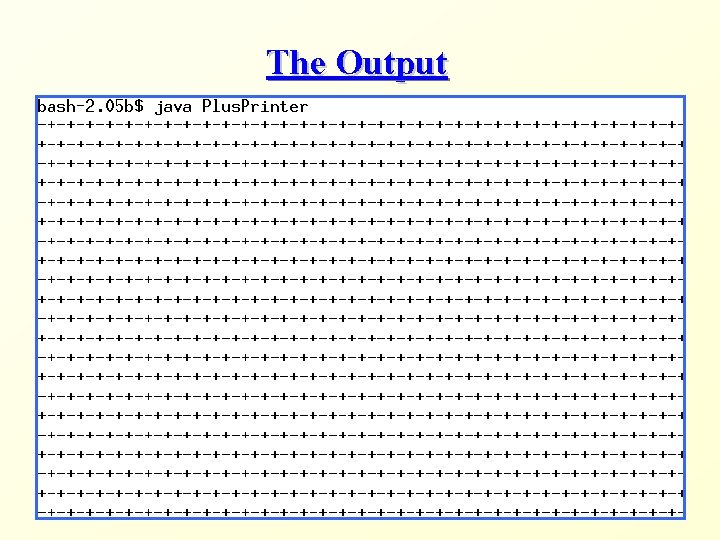
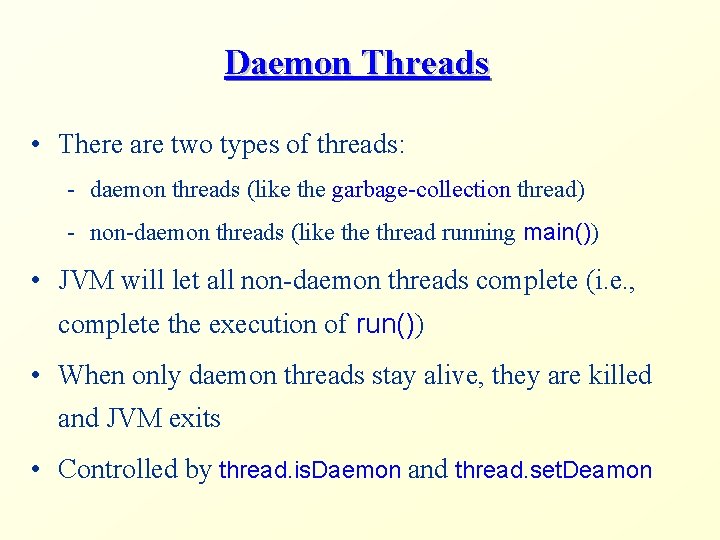
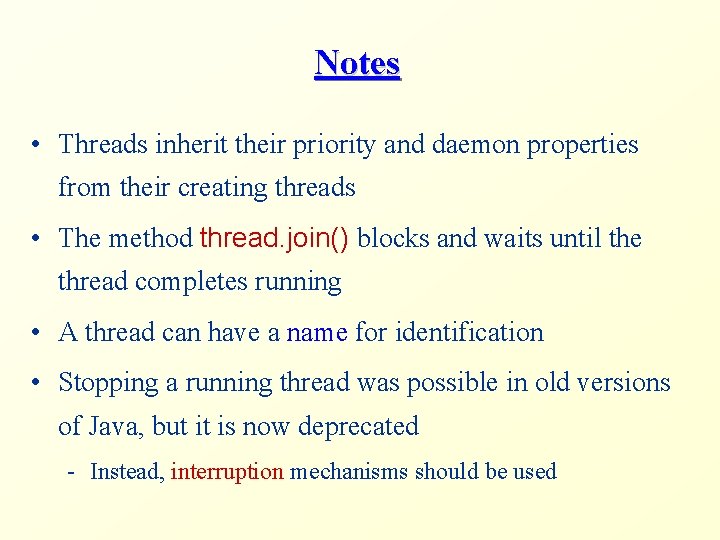
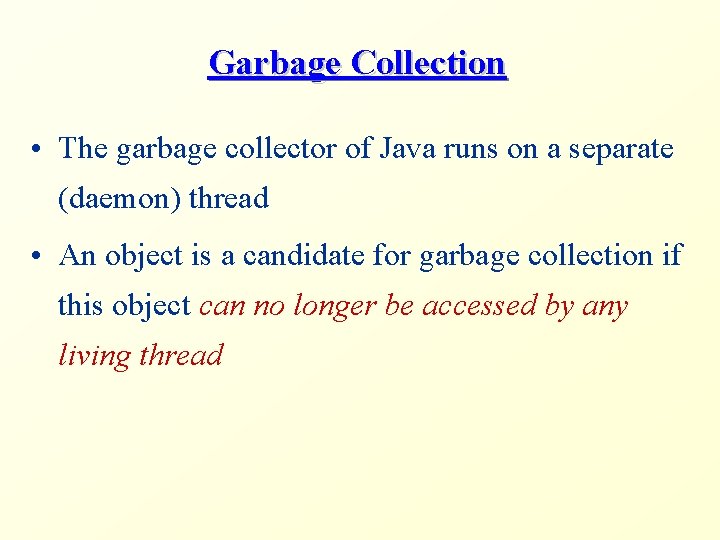
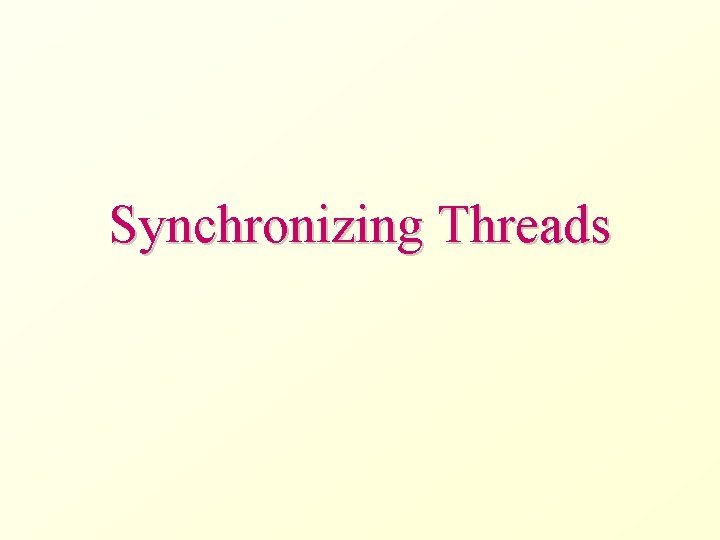
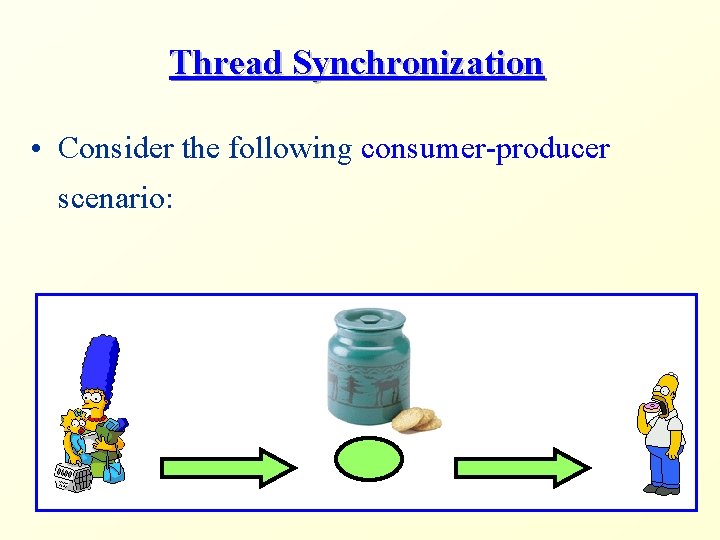
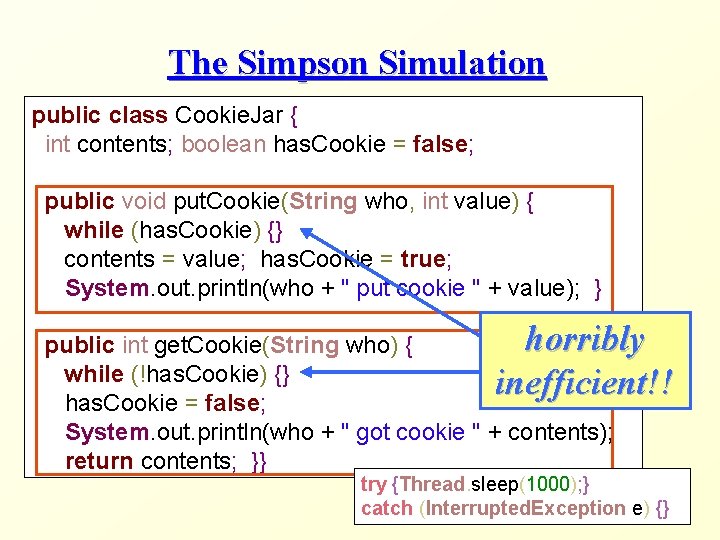
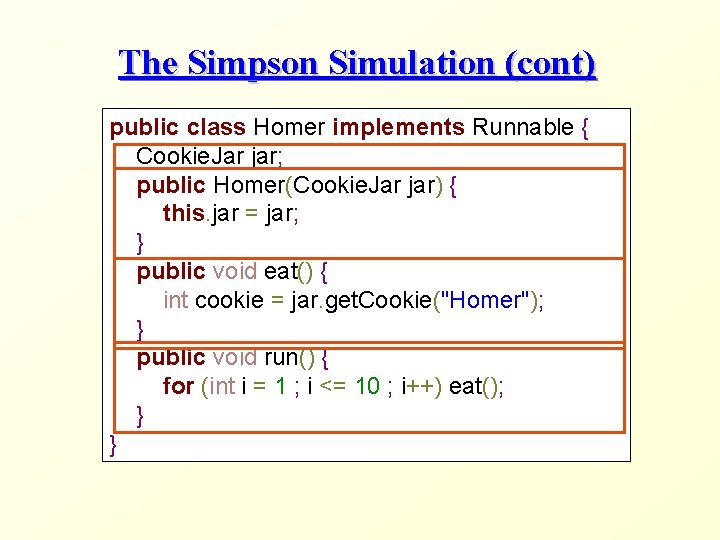
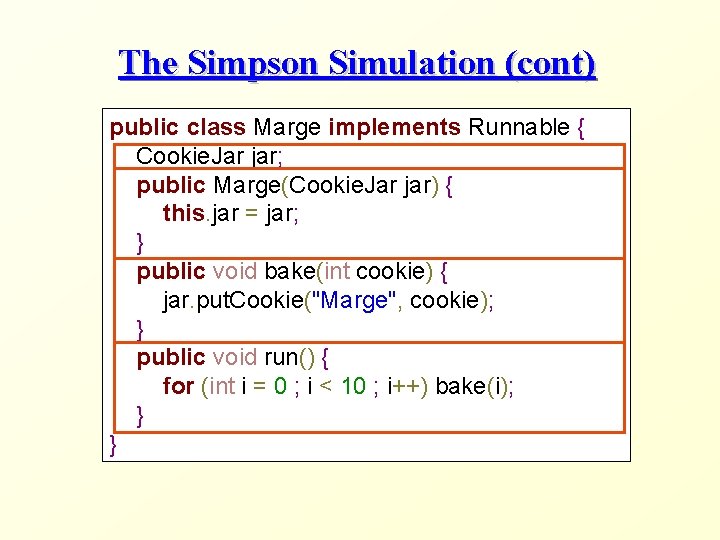
![The Simpson Simulation (cont) public class Run. Simpsons { public static void main(String[] args) The Simpson Simulation (cont) public class Run. Simpsons { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/cb794a921530f1109890c072a18da491/image-34.jpg)
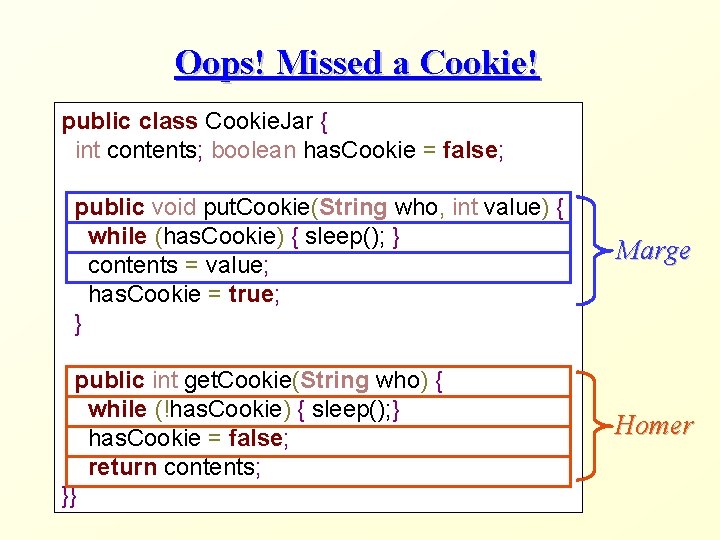
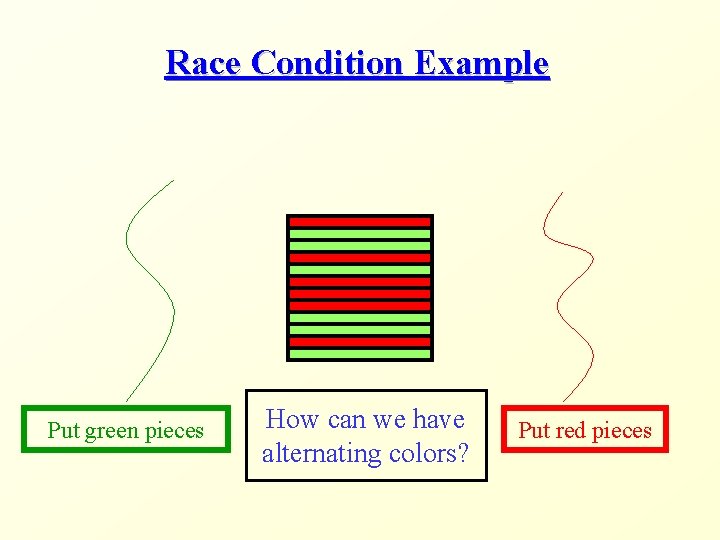
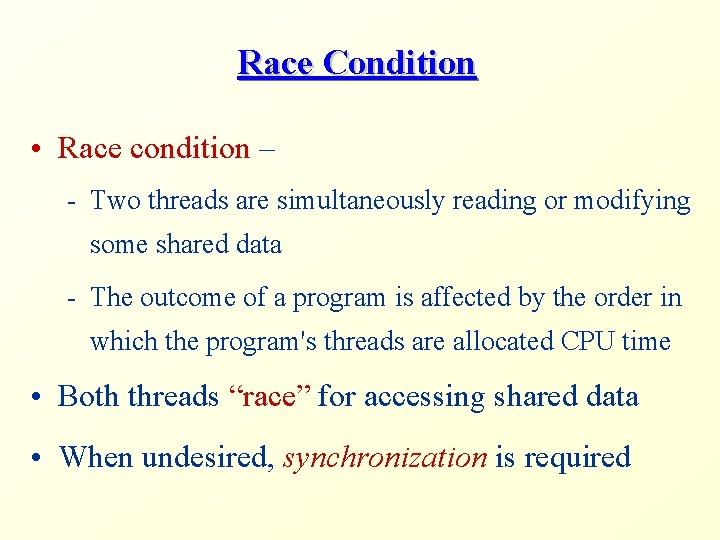
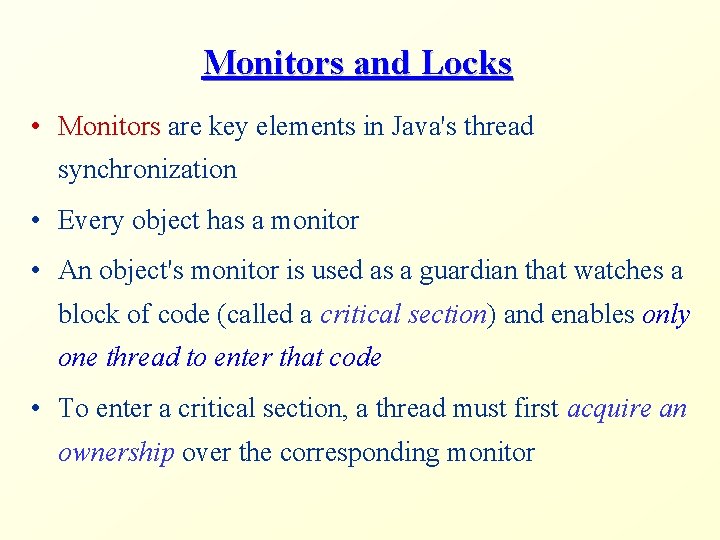
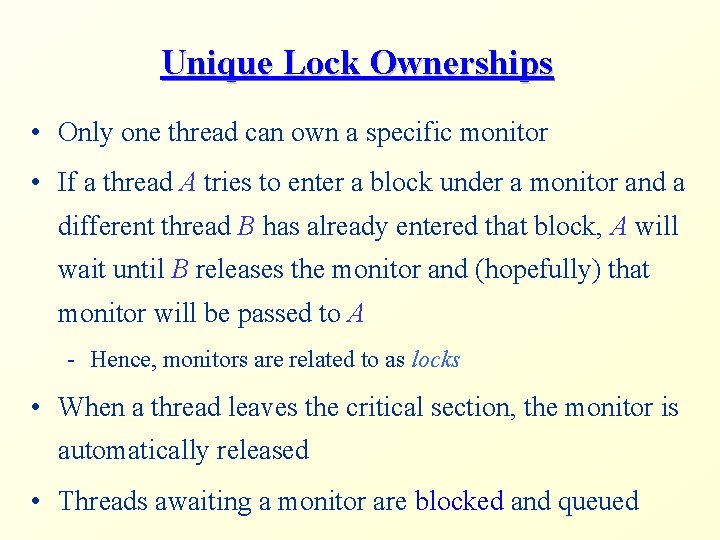
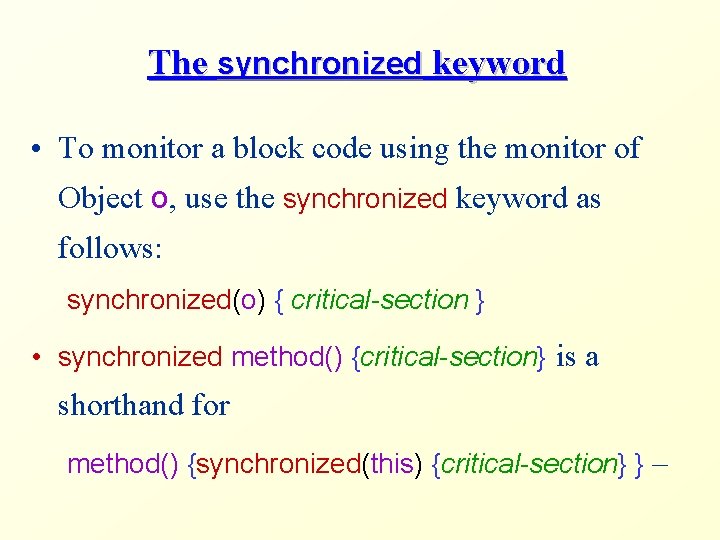
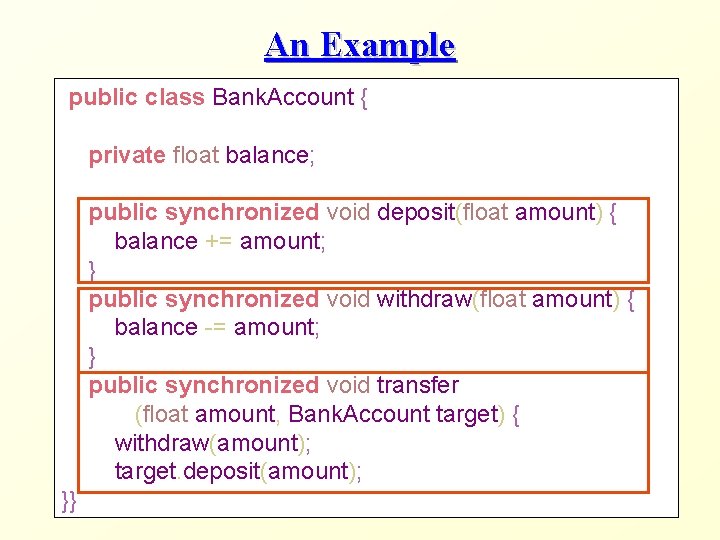
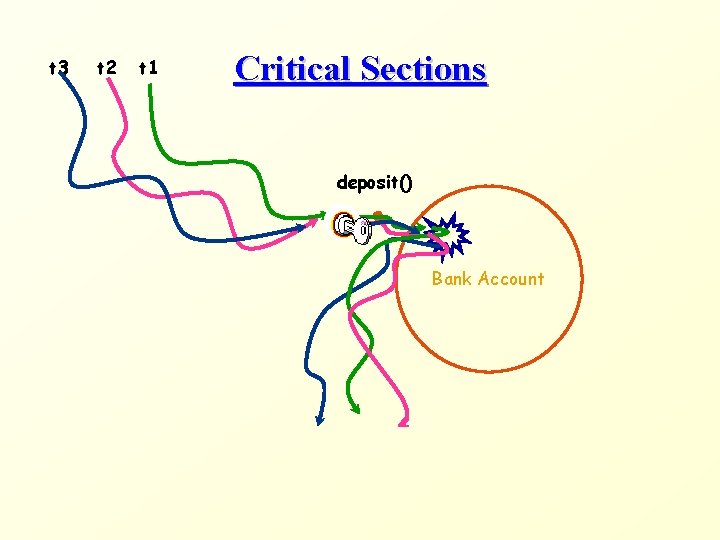
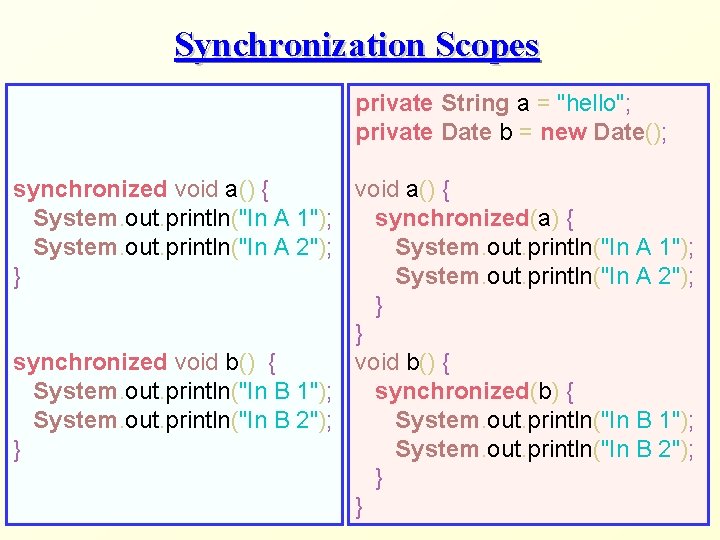
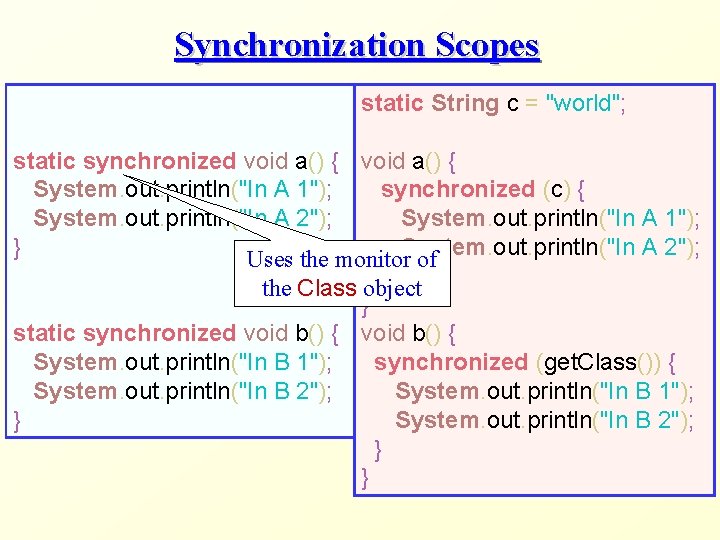
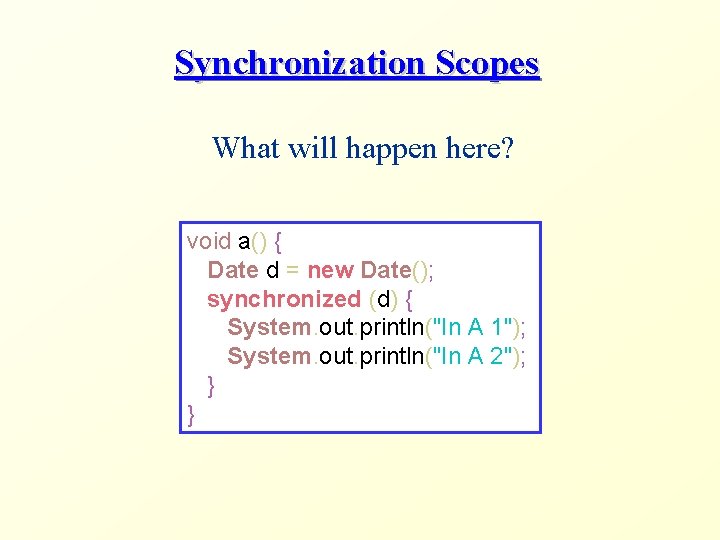
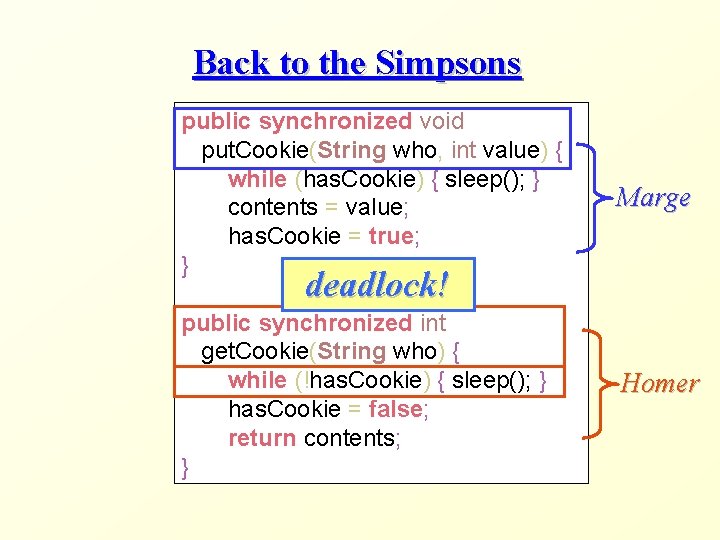
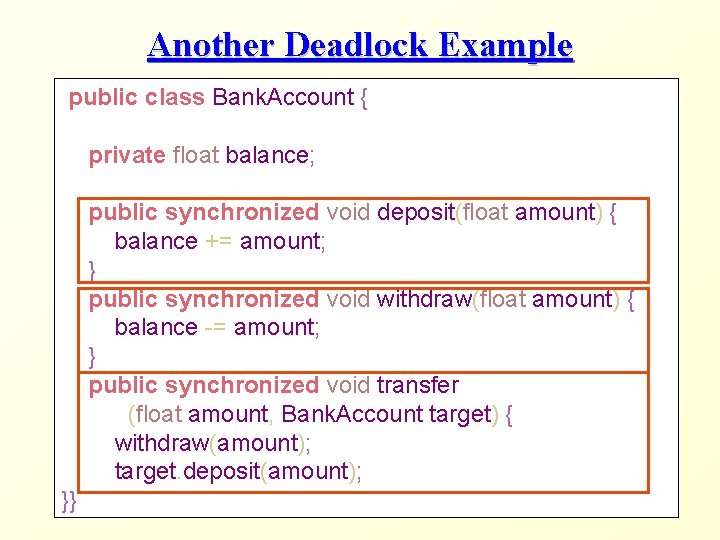
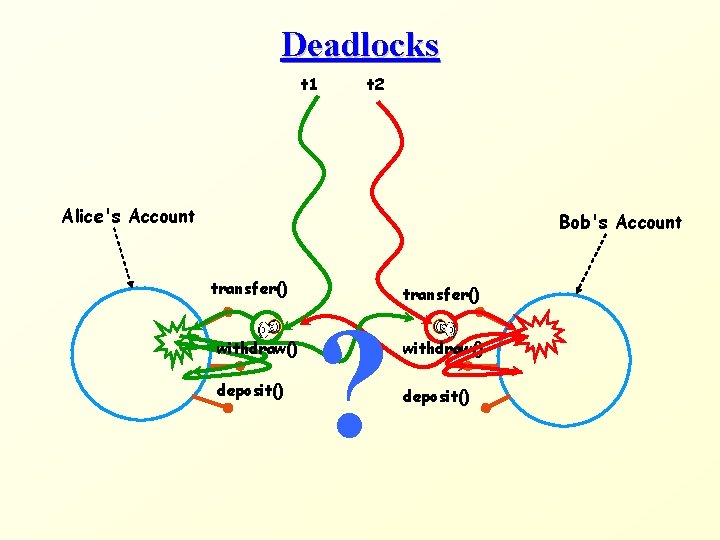
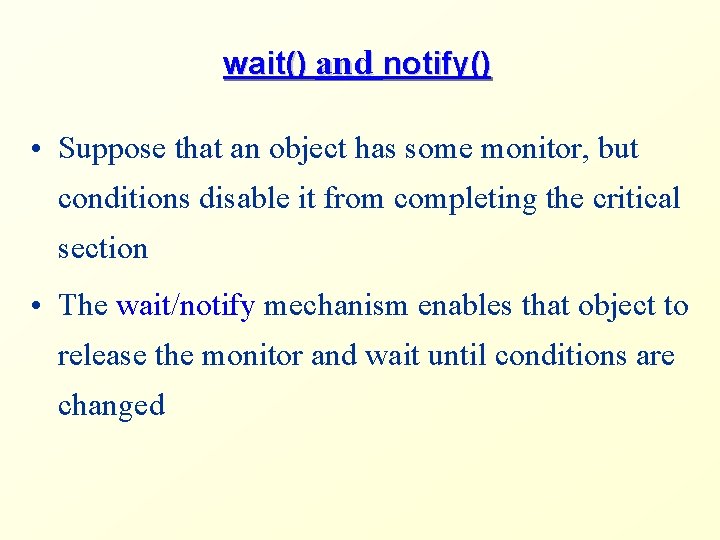
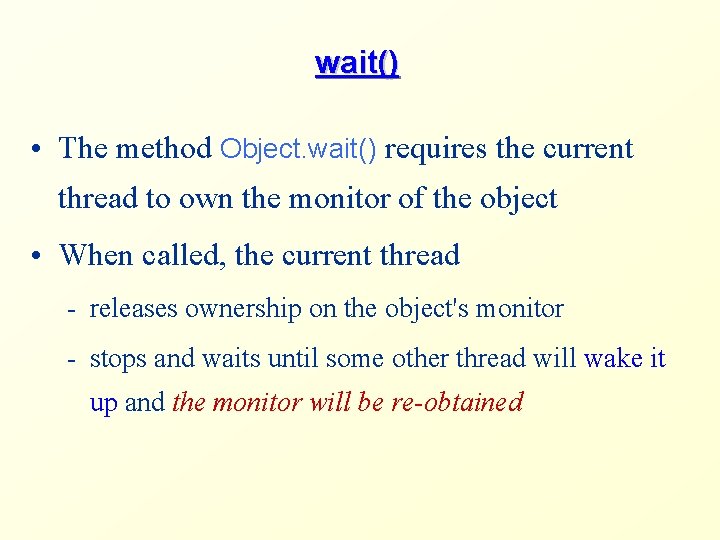
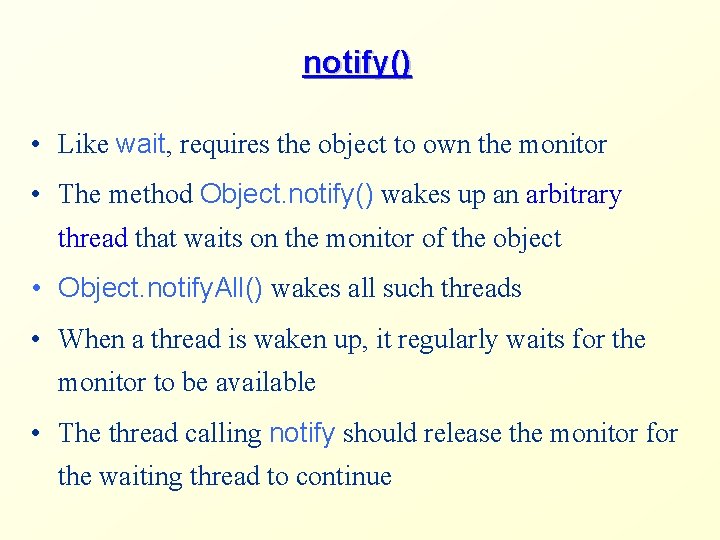
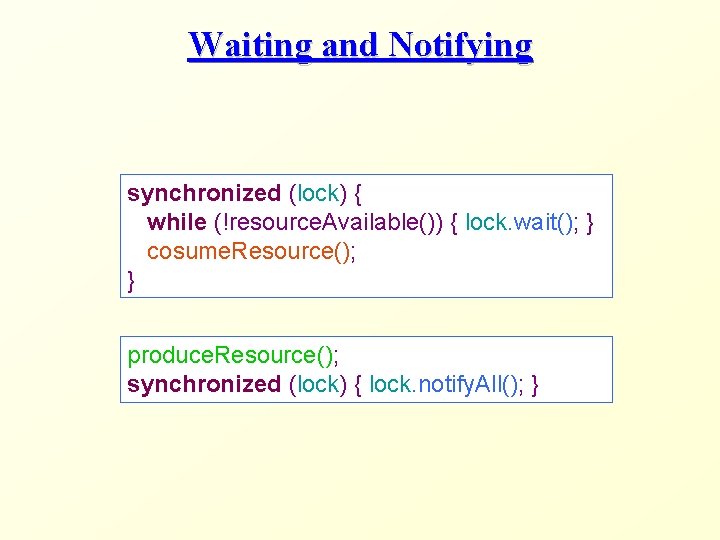
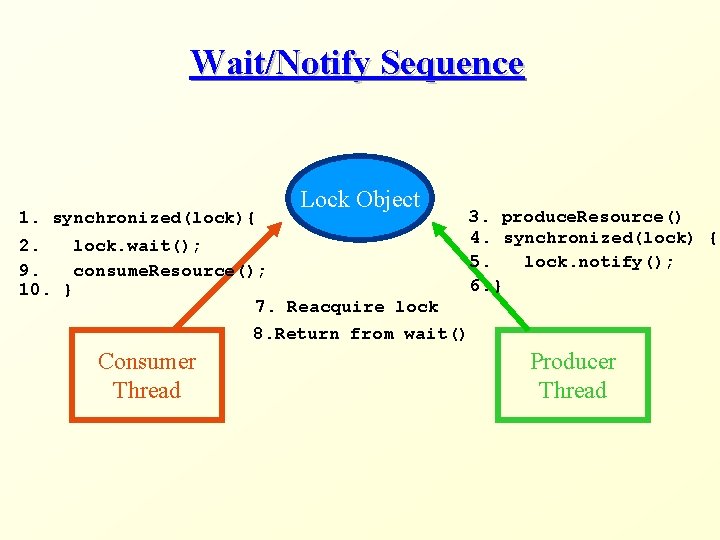
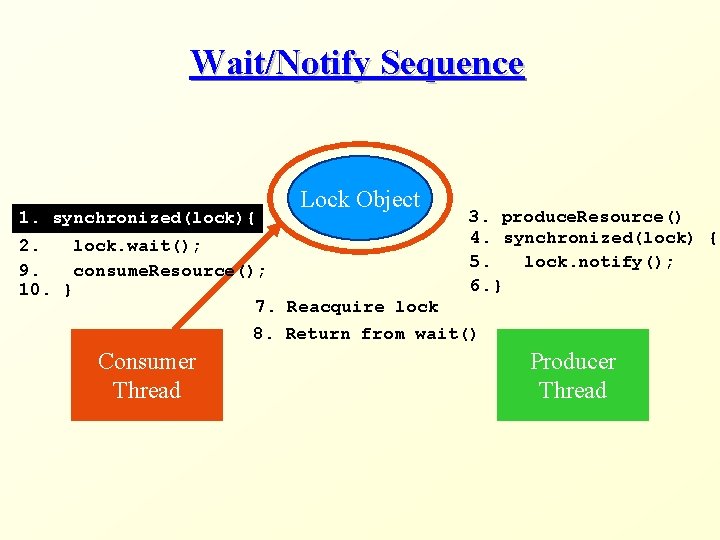
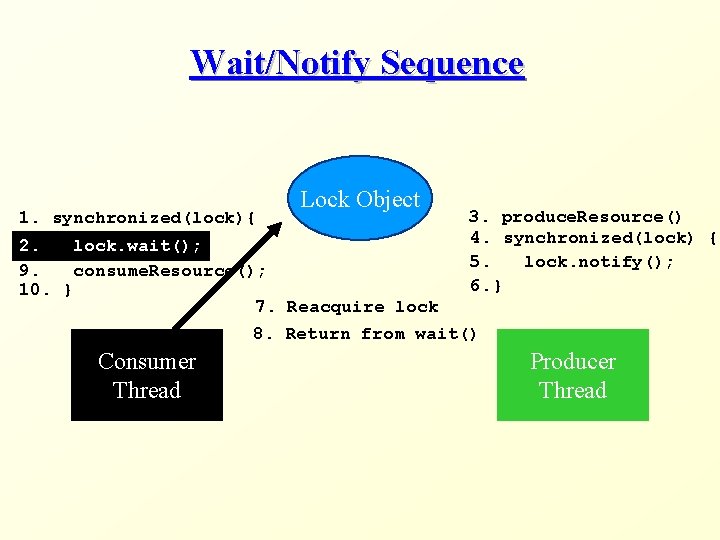
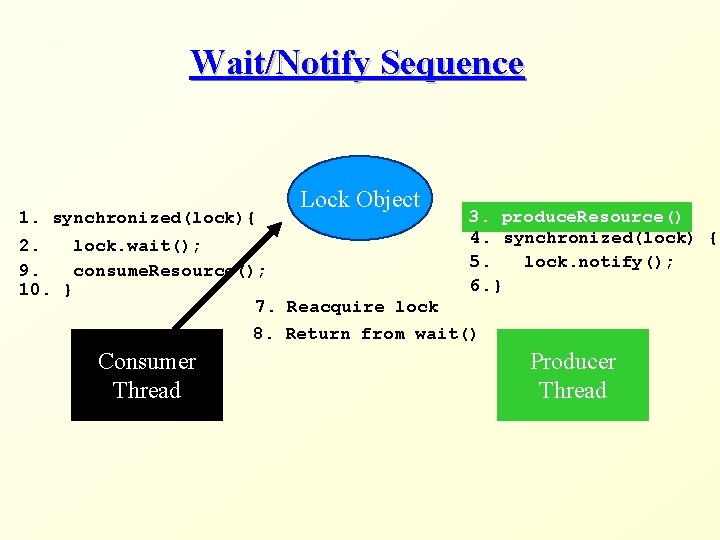
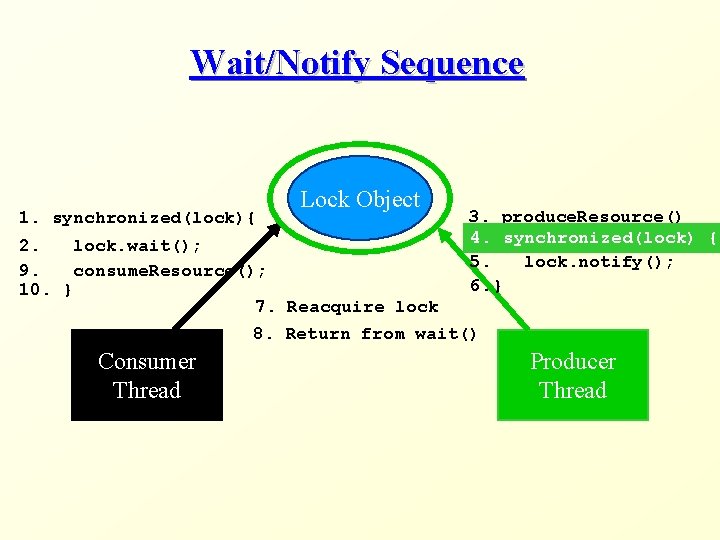
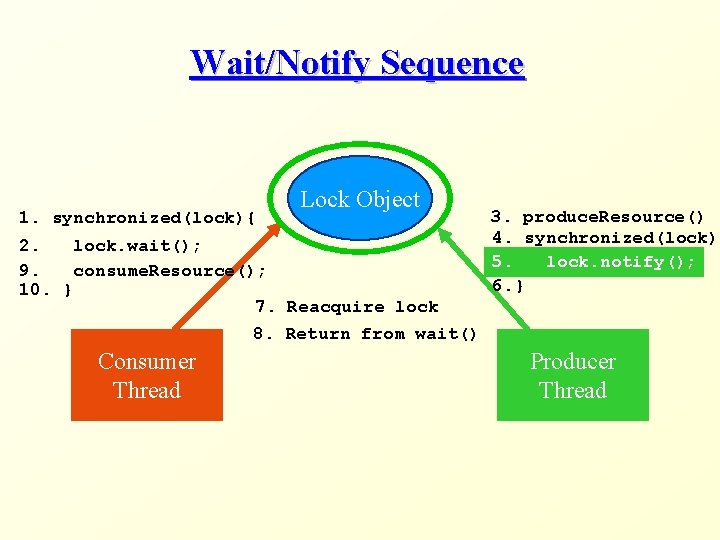
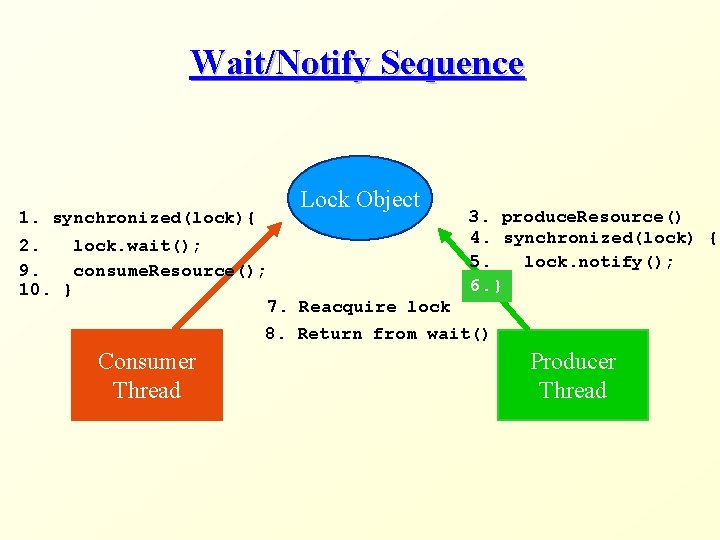
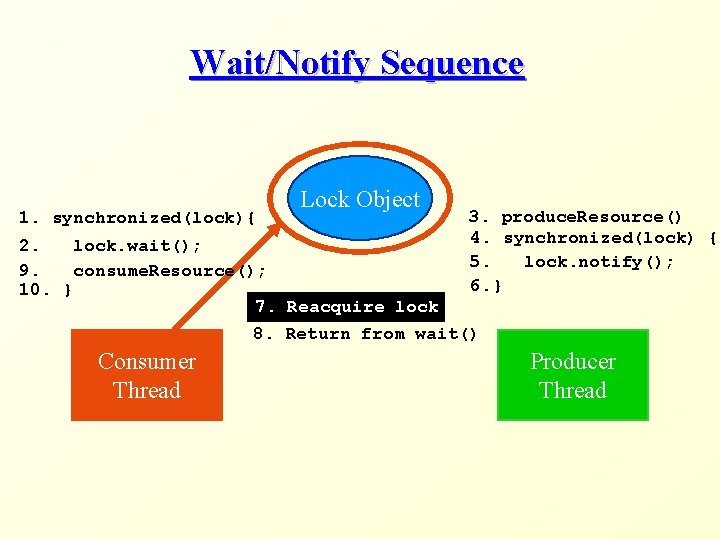
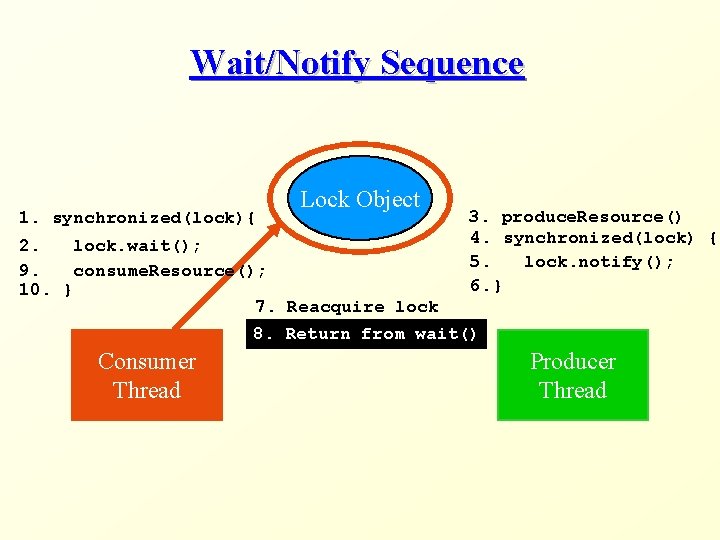
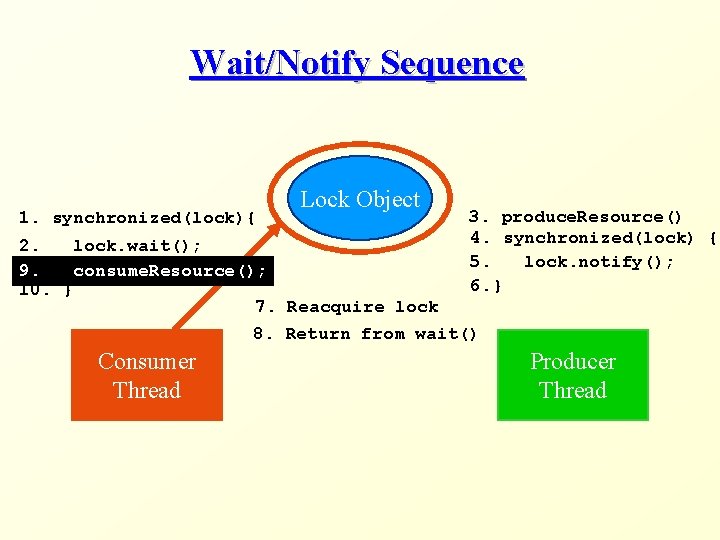
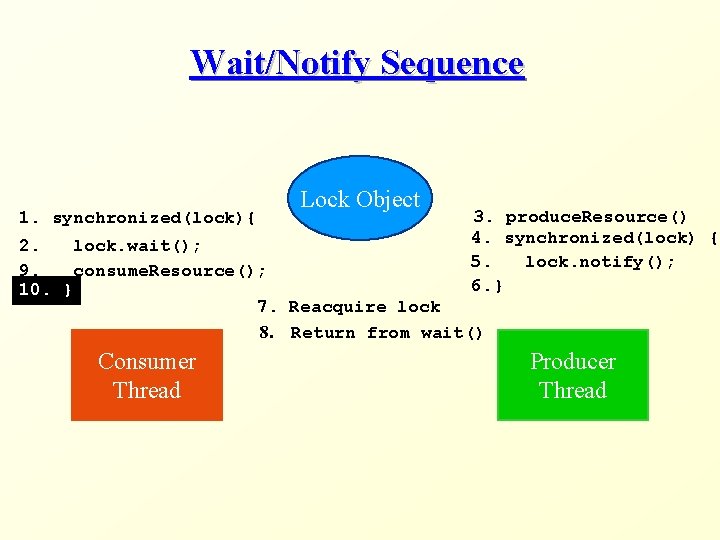
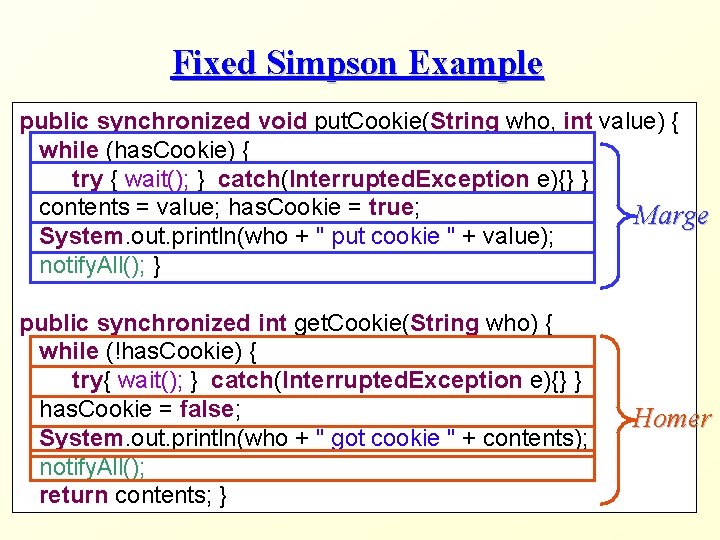
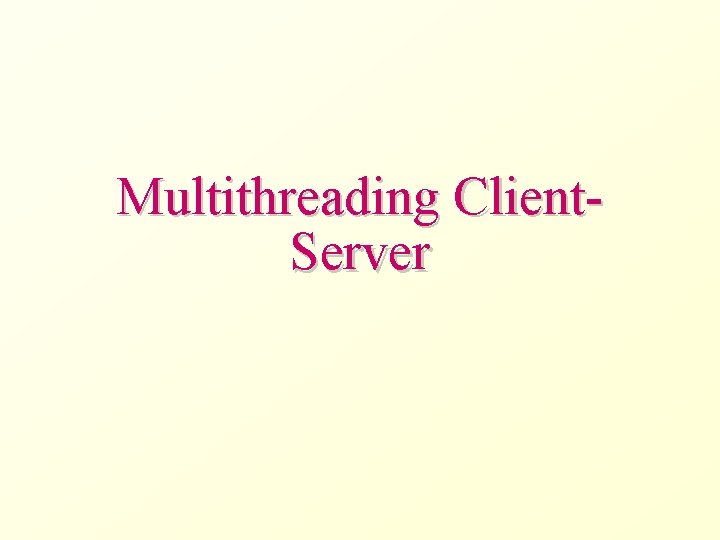
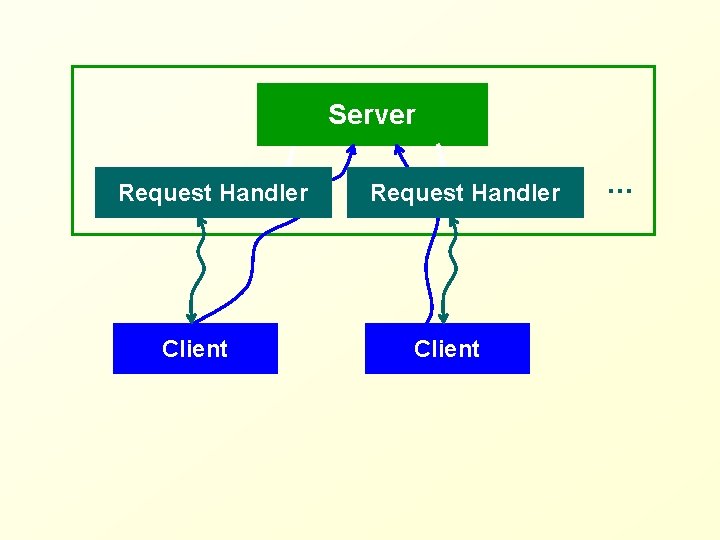
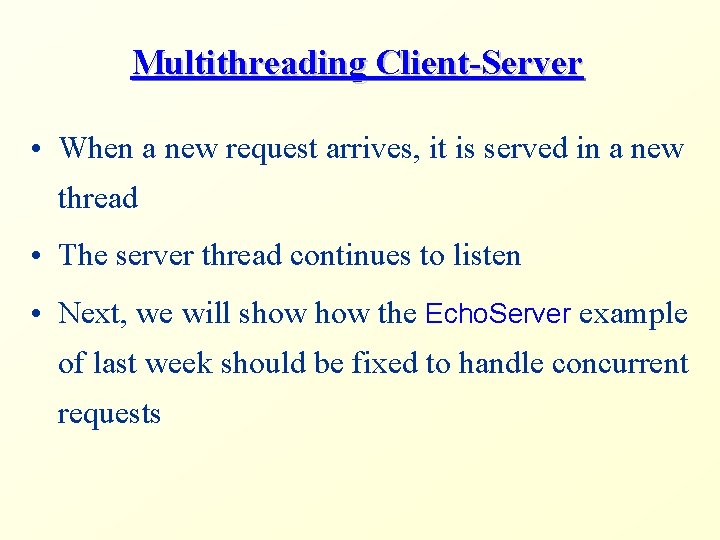
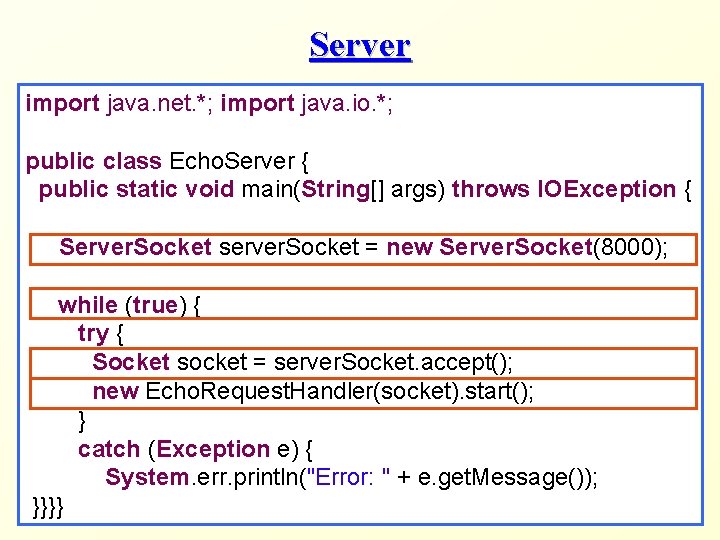
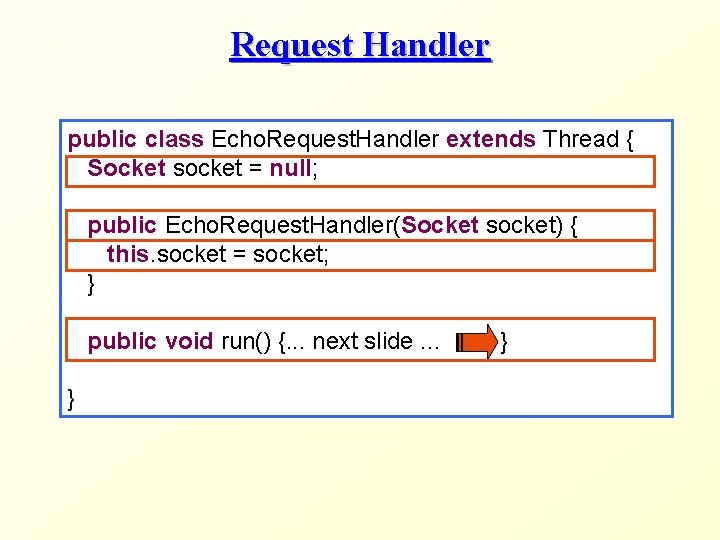
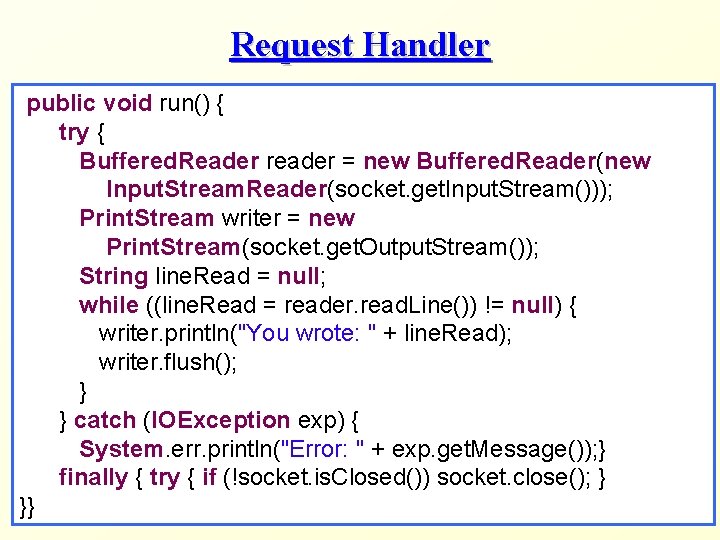
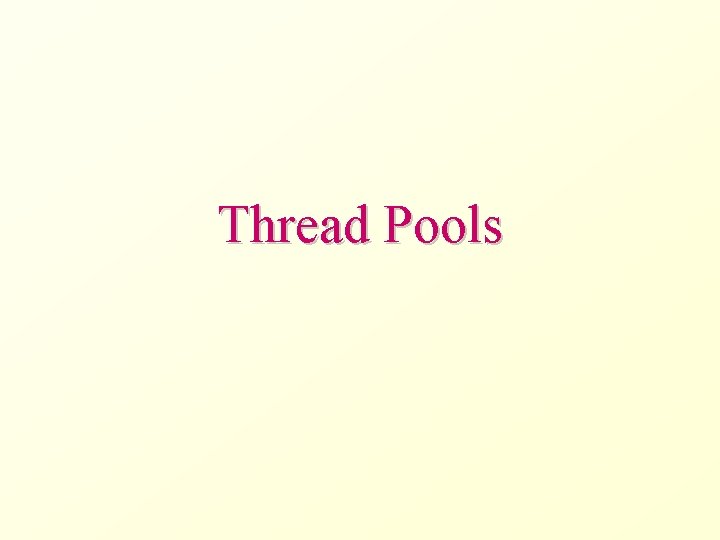
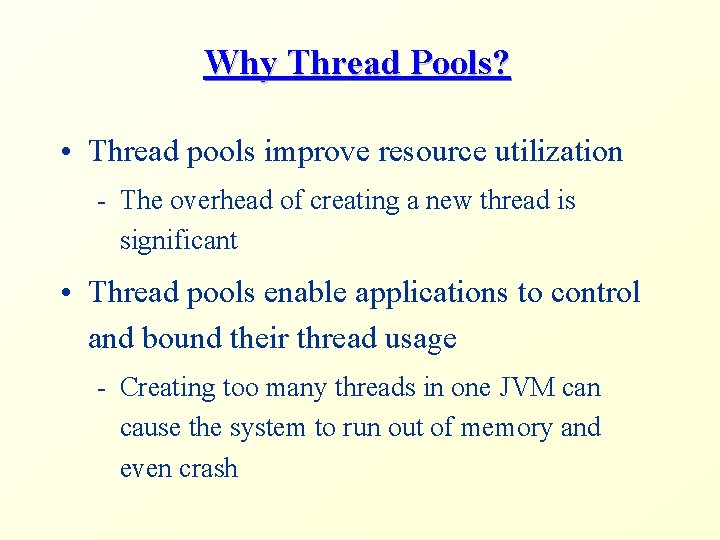
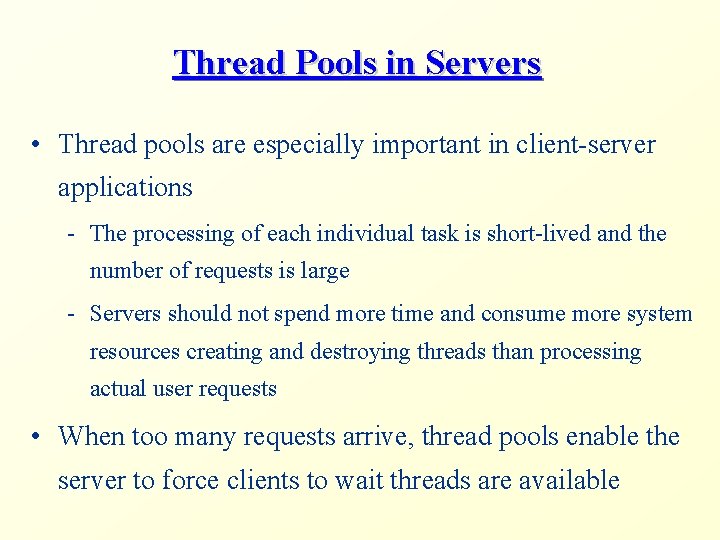
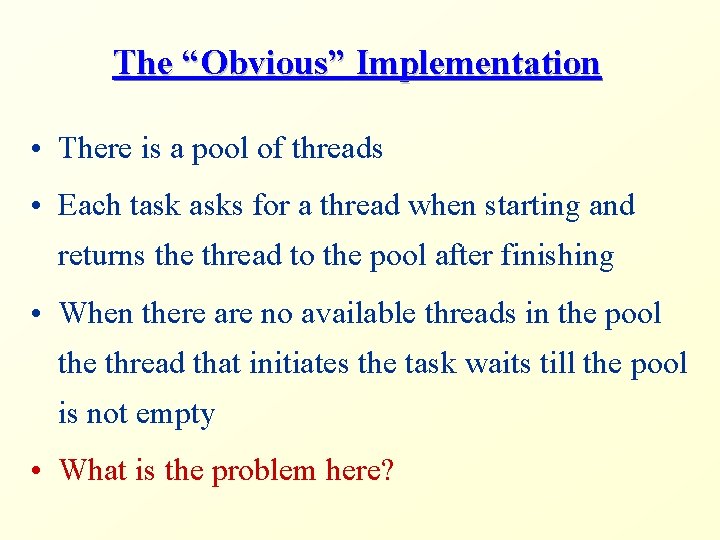
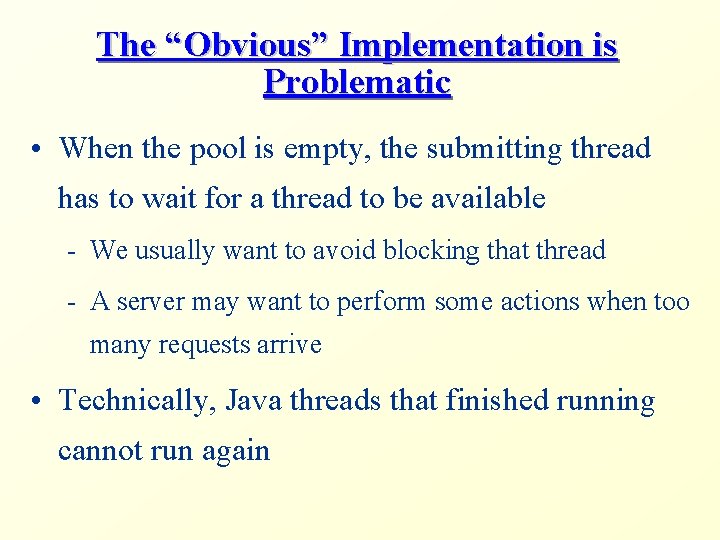
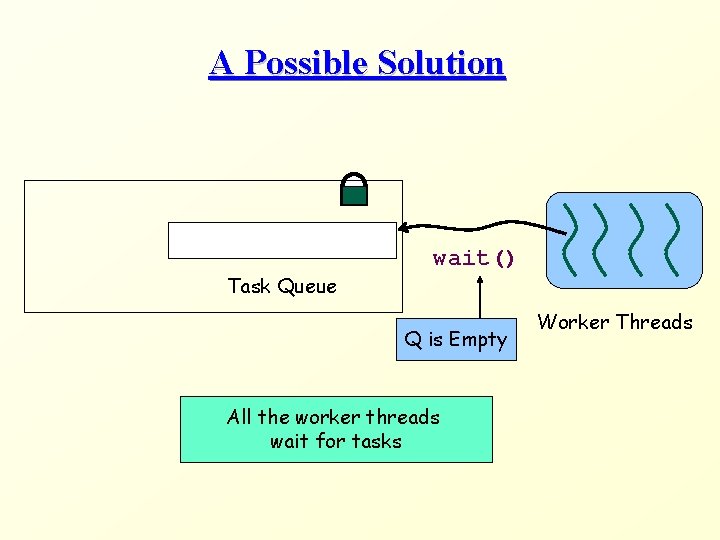
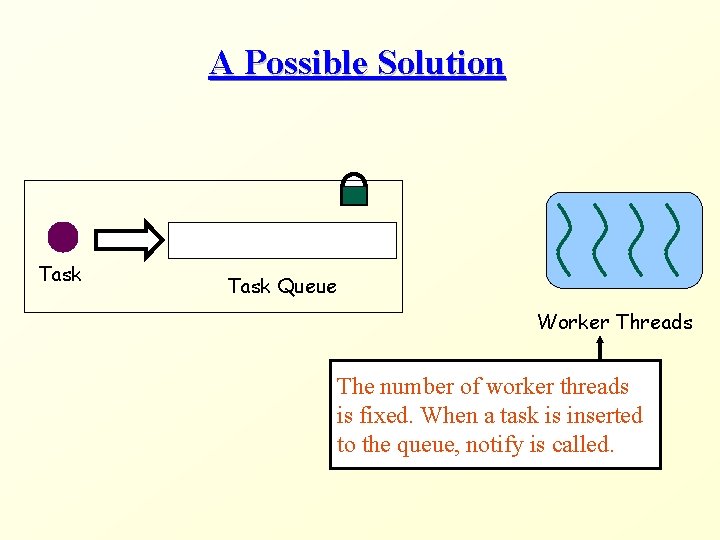
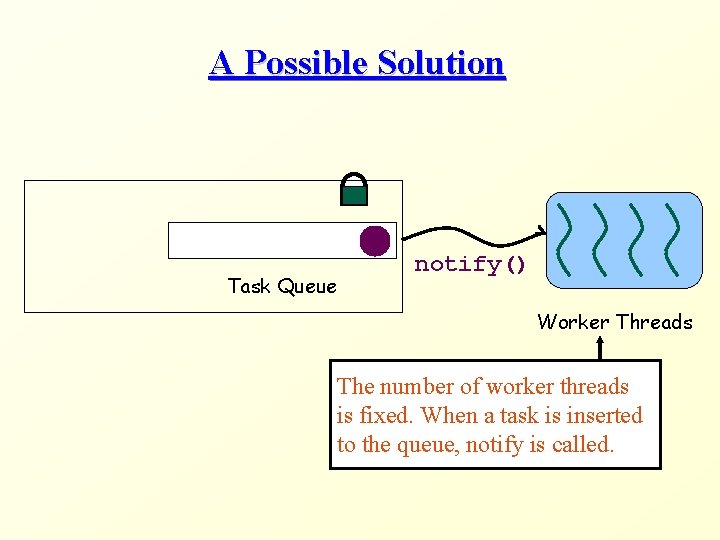
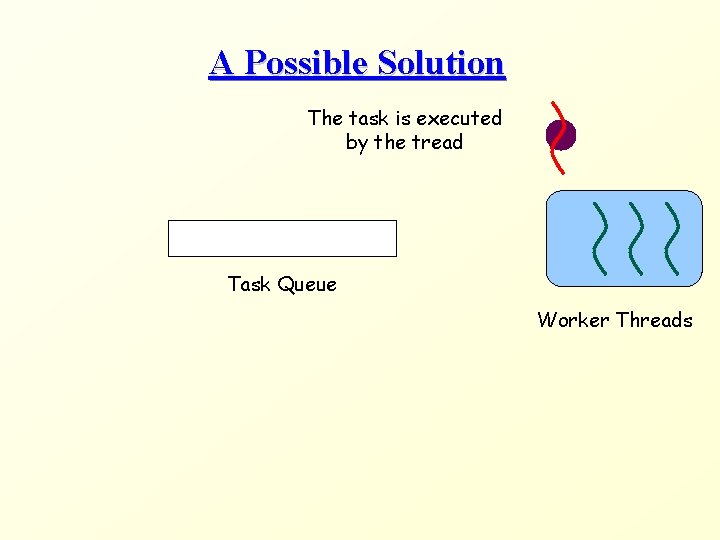
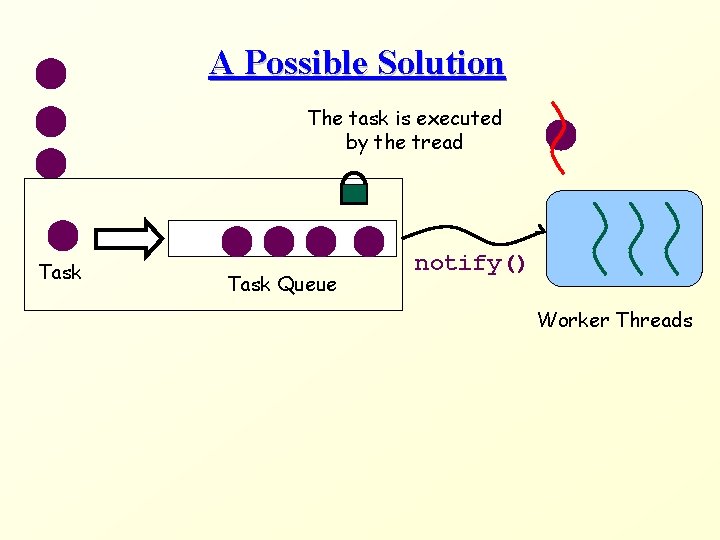
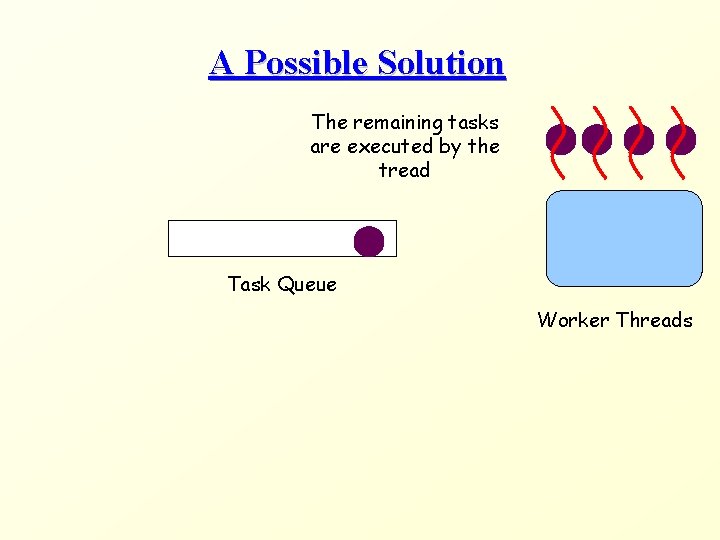
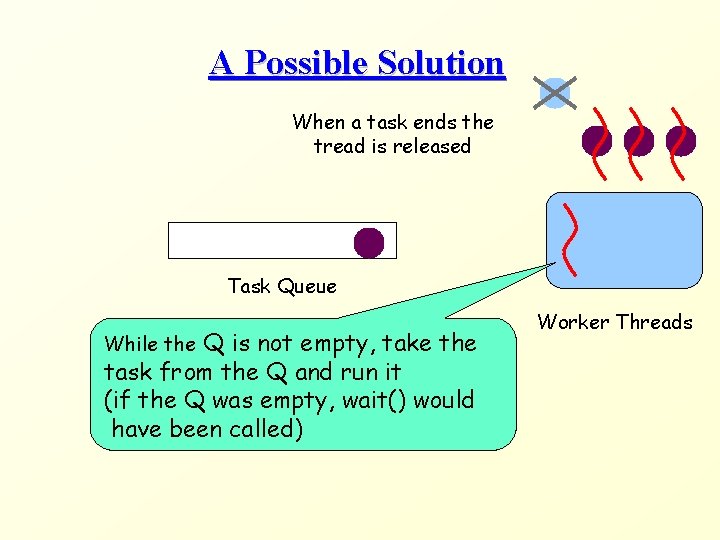
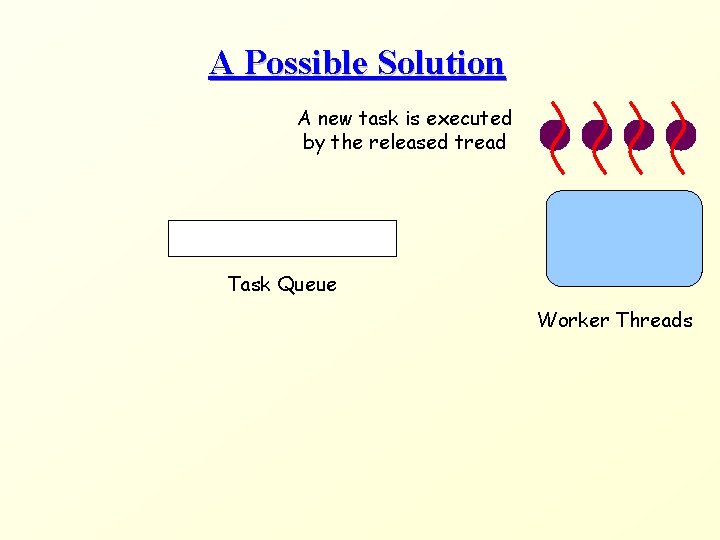
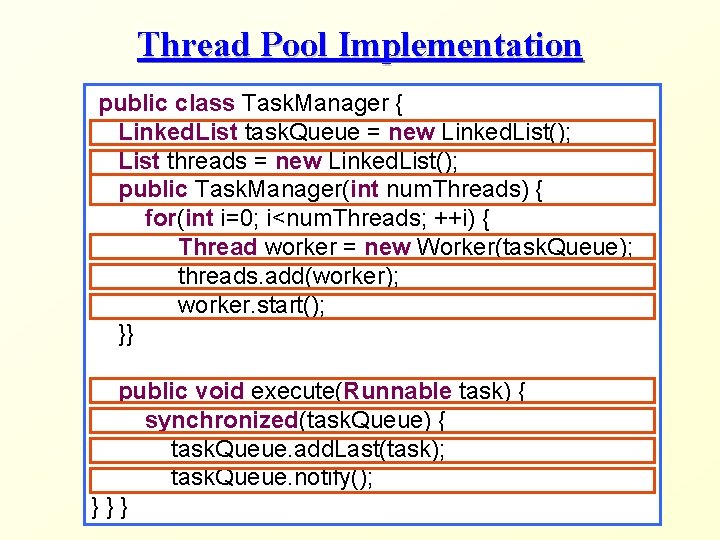
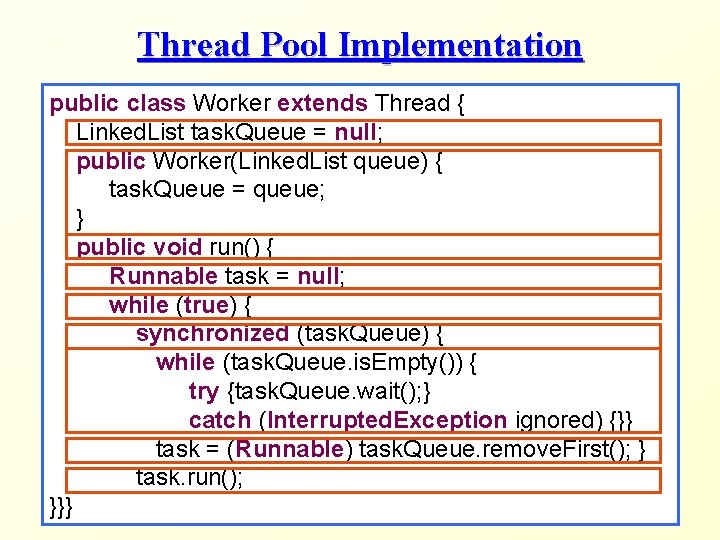
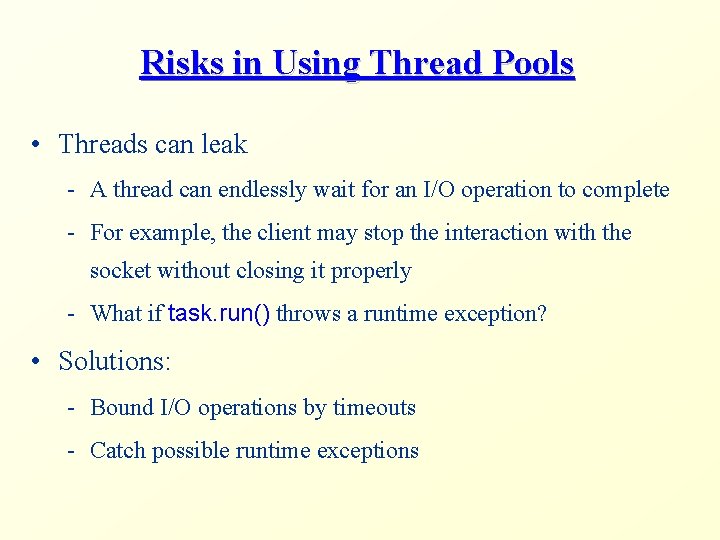
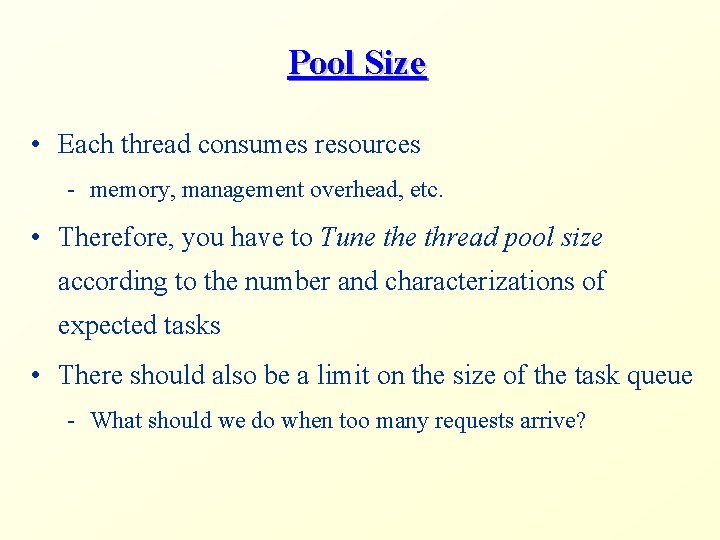
- Slides: 87
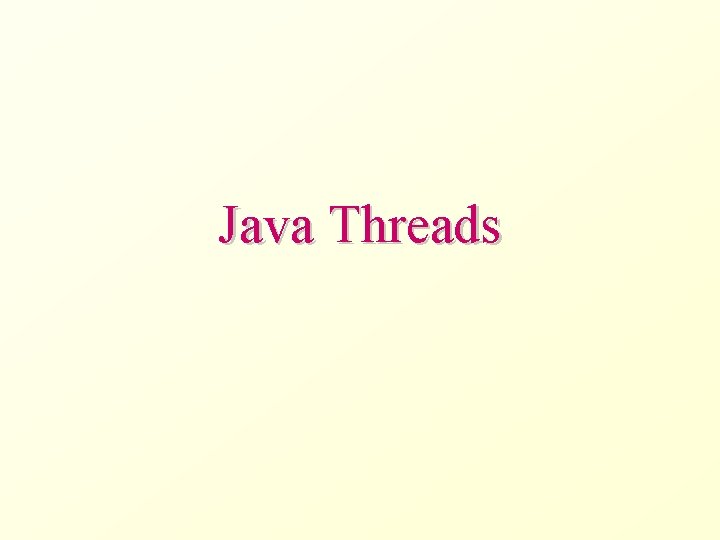
Java Threads
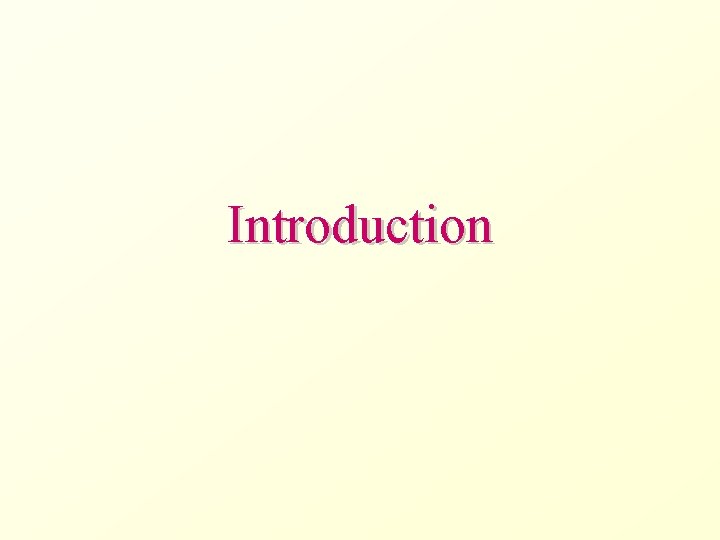
Introduction
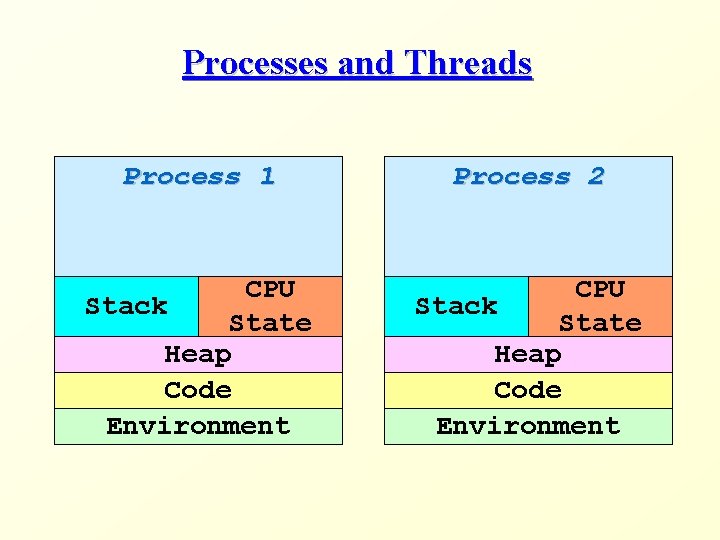
Processes and Threads Process 1 Process 2 CPU Stack State Heap Code Environment
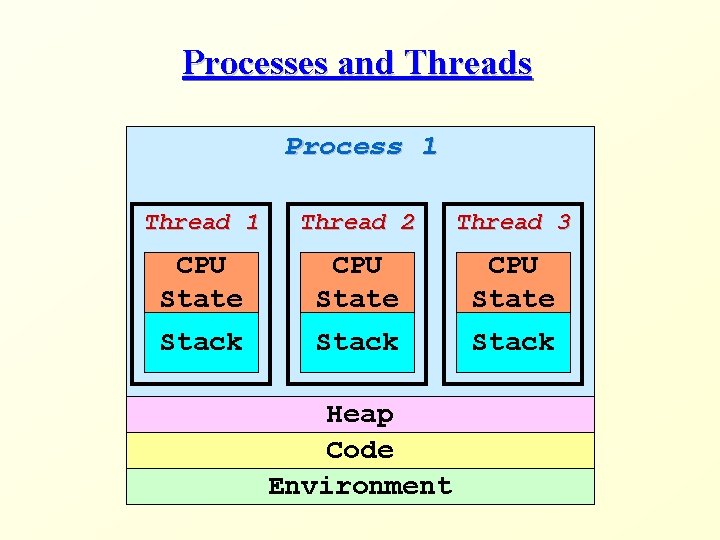
Processes and Threads Process 1 Thread 2 Thread 3 CPU State Stack Heap Code Environment
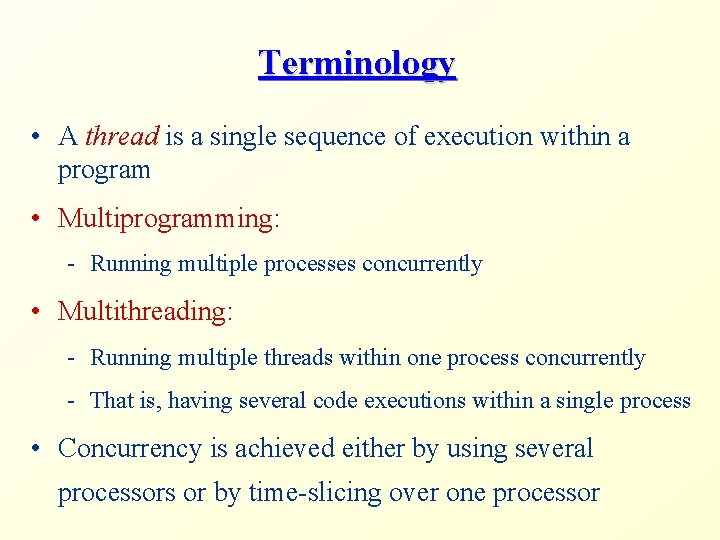
Terminology • A thread is a single sequence of execution within a program • Multiprogramming: - Running multiple processes concurrently • Multithreading: - Running multiple threads within one process concurrently - That is, having several code executions within a single process • Concurrency is achieved either by using several processors or by time-slicing over one processor
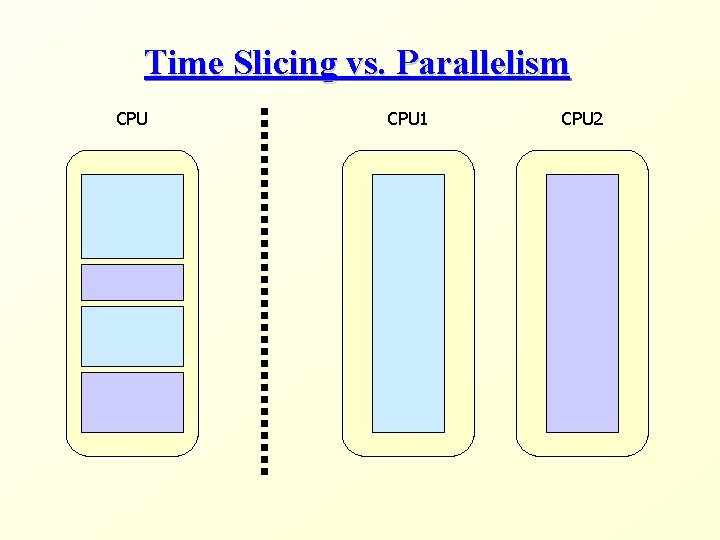
Time Slicing vs. Parallelism CPU 1 CPU 2
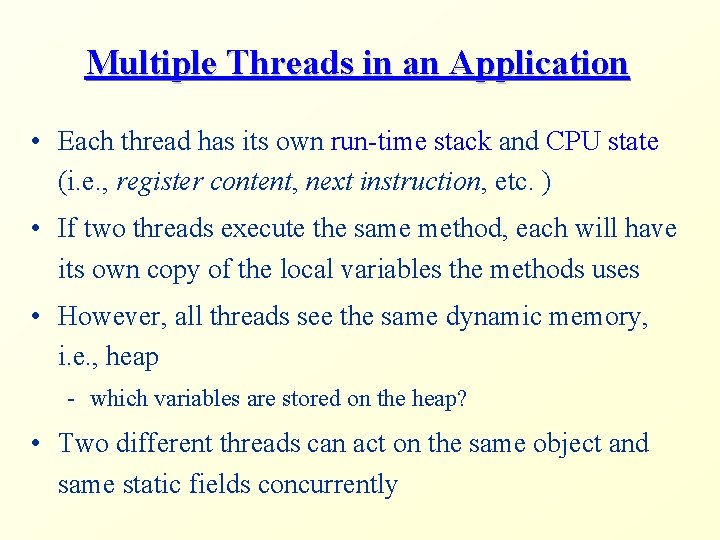
Multiple Threads in an Application • Each thread has its own run-time stack and CPU state (i. e. , register content, next instruction, etc. ) • If two threads execute the same method, each will have its own copy of the local variables the methods uses • However, all threads see the same dynamic memory, i. e. , heap - which variables are stored on the heap? • Two different threads can act on the same object and same static fields concurrently
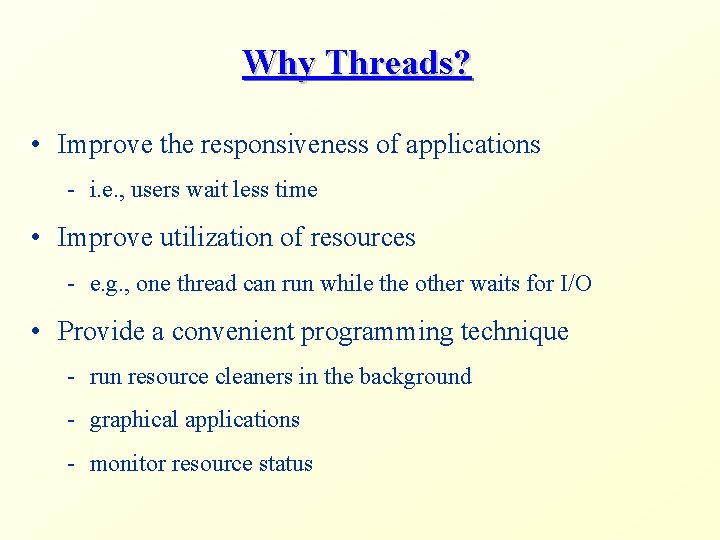
Why Threads? • Improve the responsiveness of applications - i. e. , users wait less time • Improve utilization of resources - e. g. , one thread can run while the other waits for I/O • Provide a convenient programming technique - run resource cleaners in the background - graphical applications - monitor resource status
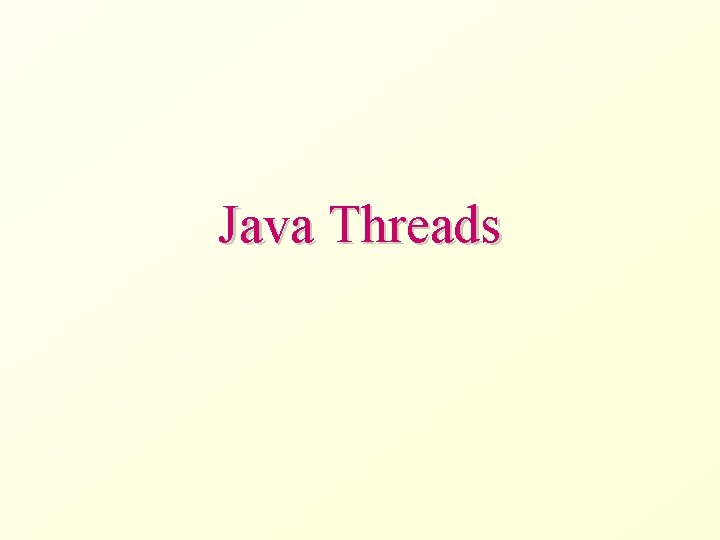
Java Threads
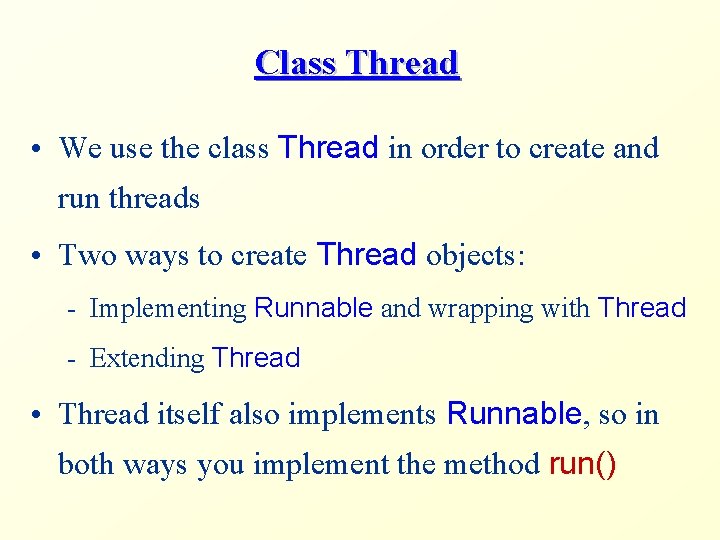
Class Thread • We use the class Thread in order to create and run threads • Two ways to create Thread objects: - Implementing Runnable and wrapping with Thread - Extending Thread • Thread itself also implements Runnable, so in both ways you implement the method run()
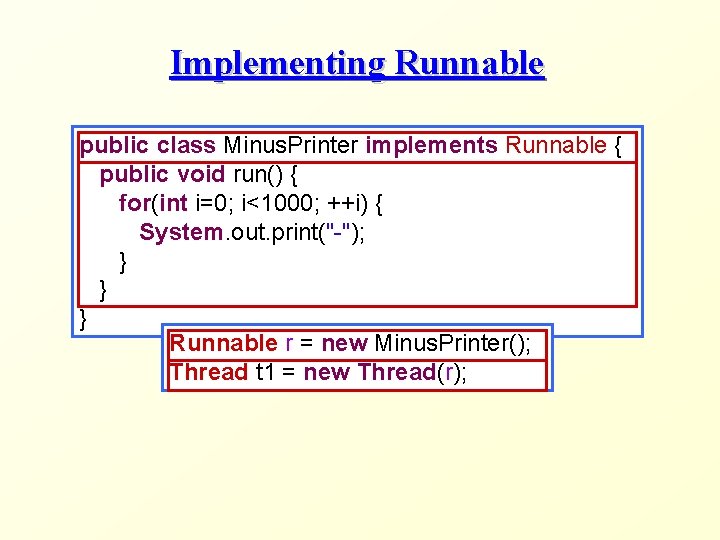
Implementing Runnable public class Minus. Printer implements Runnable { public void run() { for(int i=0; i<1000; ++i) { System. out. print("-"); } } } Runnable r = new Minus. Printer(); Thread t 1 = new Thread(r);
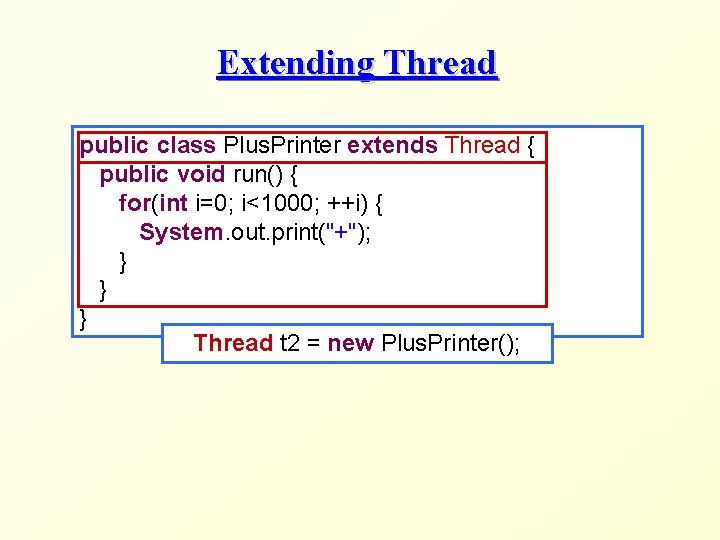
Extending Thread public class Plus. Printer extends Thread { public void run() { for(int i=0; i<1000; ++i) { System. out. print("+"); } } } Thread t 2 = new Plus. Printer();
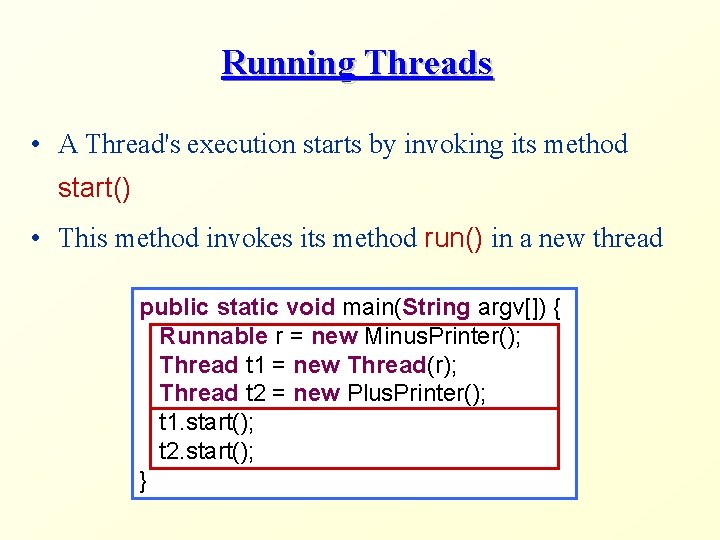
Running Threads • A Thread's execution starts by invoking its method start() • This method invokes its method run() in a new thread public static void main(String argv[]) { Runnable r = new Minus. Printer(); Thread t 1 = new Thread(r); Thread t 2 = new Plus. Printer(); t 1. start(); t 2. start(); }
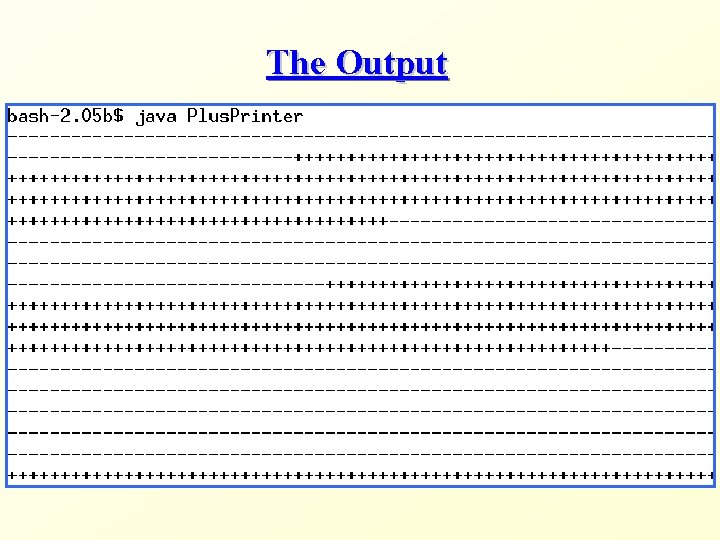
The Output
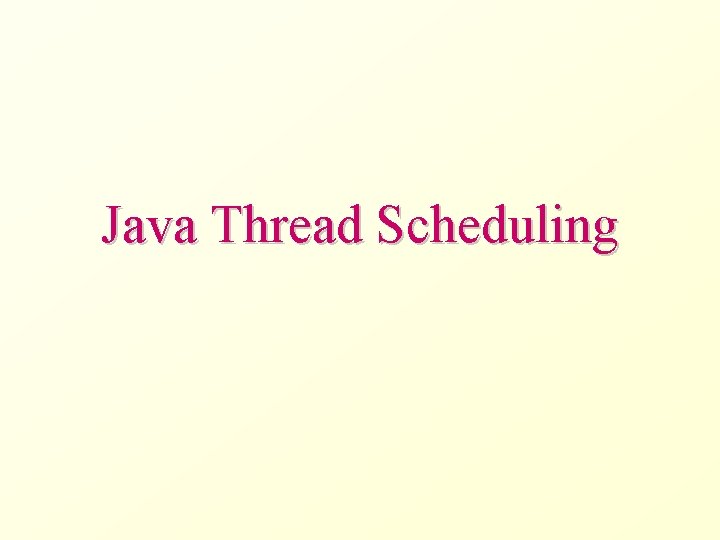
Java Thread Scheduling
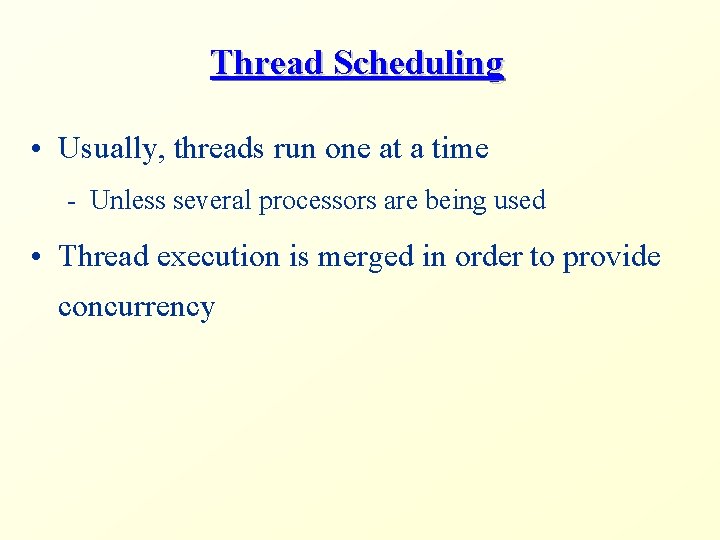
Thread Scheduling • Usually, threads run one at a time - Unless several processors are being used • Thread execution is merged in order to provide concurrency
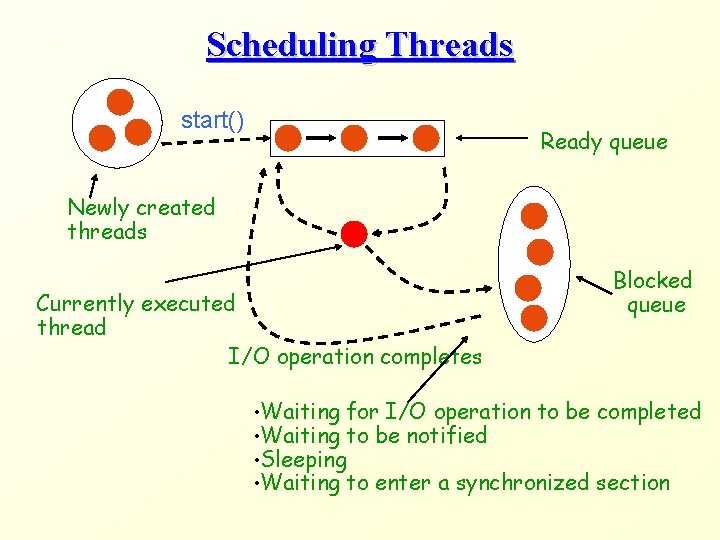
Scheduling Threads start() Ready queue Newly created threads Currently executed thread I/O operation completes • Waiting for I/O operation to be • Waiting to be notified • Sleeping • Waiting to enter a synchronized Blocked queue completed section
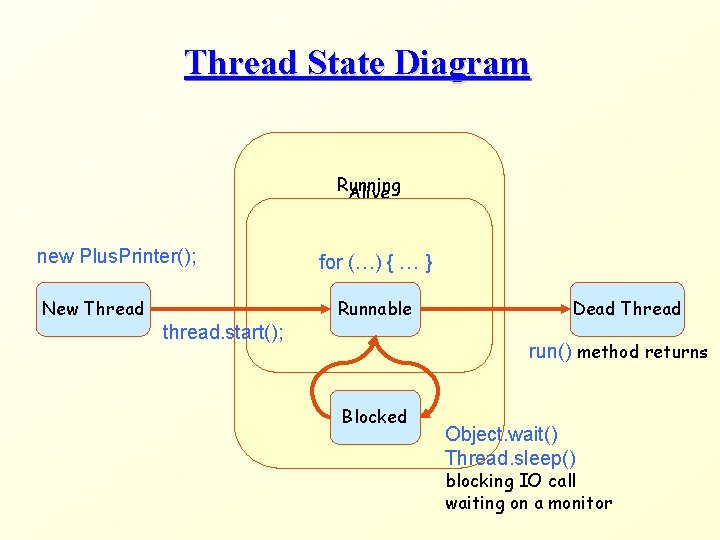
Thread State Diagram Running Alive new Plus. Printer(); New Thread for (…) { … } Runnable thread. start(); Dead Thread run() method returns Blocked Object. wait() Thread. sleep() blocking IO call waiting on a monitor
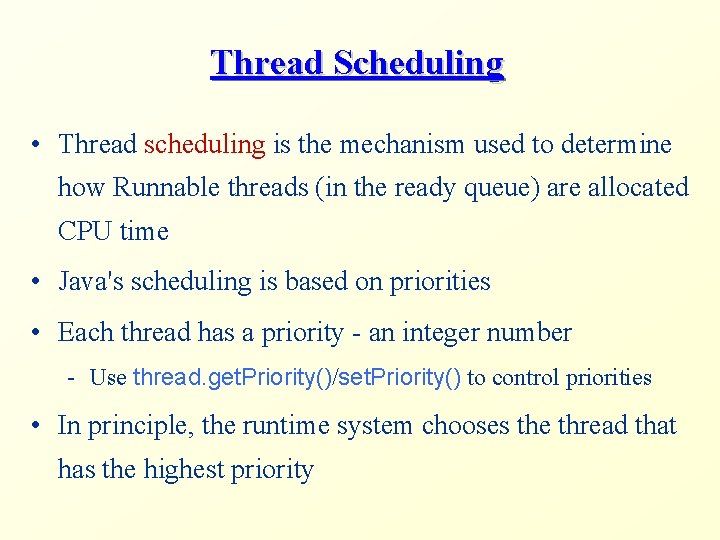
Thread Scheduling • Thread scheduling is the mechanism used to determine how Runnable threads (in the ready queue) are allocated CPU time • Java's scheduling is based on priorities • Each thread has a priority - an integer number - Use thread. get. Priority()/set. Priority() to control priorities • In principle, the runtime system chooses the thread that has the highest priority
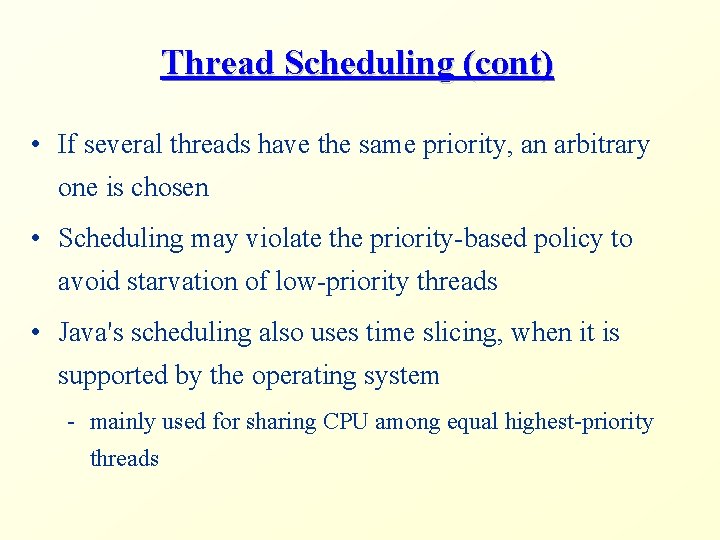
Thread Scheduling (cont) • If several threads have the same priority, an arbitrary one is chosen • Scheduling may violate the priority-based policy to avoid starvation of low-priority threads • Java's scheduling also uses time slicing, when it is supported by the operating system - mainly used for sharing CPU among equal highest-priority threads
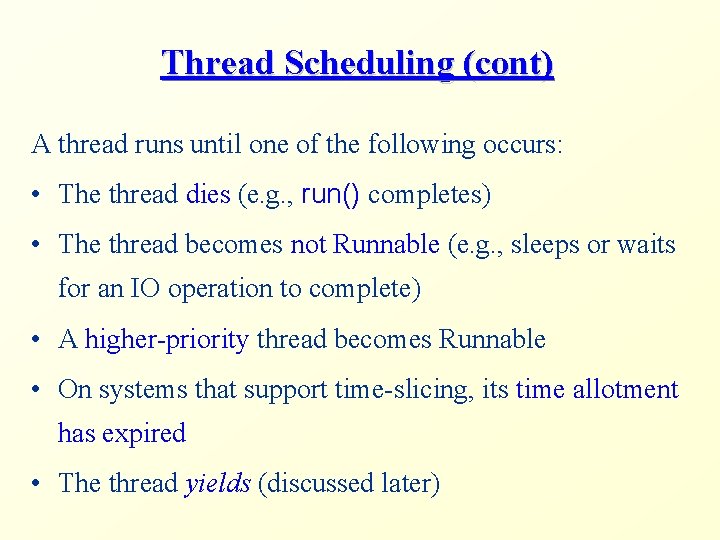
Thread Scheduling (cont) A thread runs until one of the following occurs: • The thread dies (e. g. , run() completes) • The thread becomes not Runnable (e. g. , sleeps or waits for an IO operation to complete) • A higher-priority thread becomes Runnable • On systems that support time-slicing, its time allotment has expired • The thread yields (discussed later)
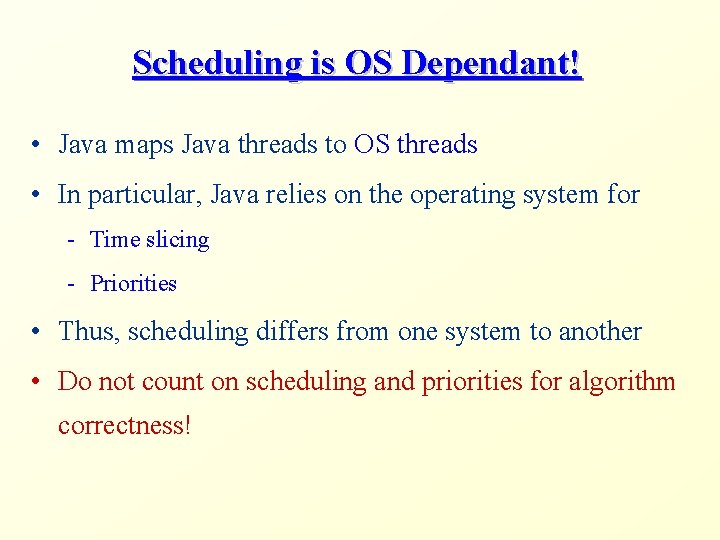
Scheduling is OS Dependant! • Java maps Java threads to OS threads • In particular, Java relies on the operating system for - Time slicing - Priorities • Thus, scheduling differs from one system to another • Do not count on scheduling and priorities for algorithm correctness!
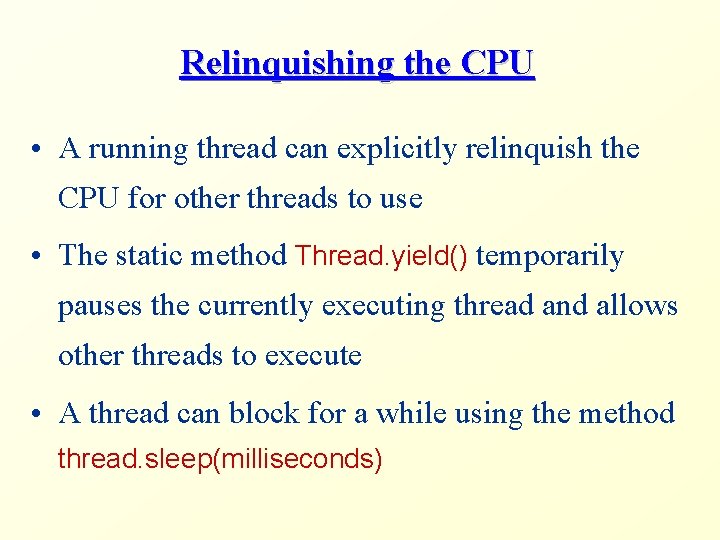
Relinquishing the CPU • A running thread can explicitly relinquish the CPU for other threads to use • The static method Thread. yield() temporarily pauses the currently executing thread and allows other threads to execute • A thread can block for a while using the method thread. sleep(milliseconds)
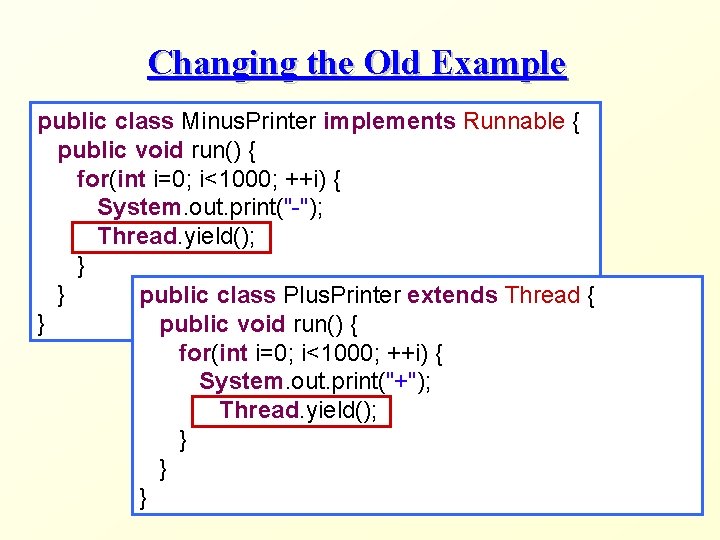
Changing the Old Example public class Minus. Printer implements Runnable { public void run() { for(int i=0; i<1000; ++i) { System. out. print("-"); Thread. yield(); } } public class Plus. Printer extends Thread { } public void run() { for(int i=0; i<1000; ++i) { System. out. print("+"); Thread. yield(); } } }
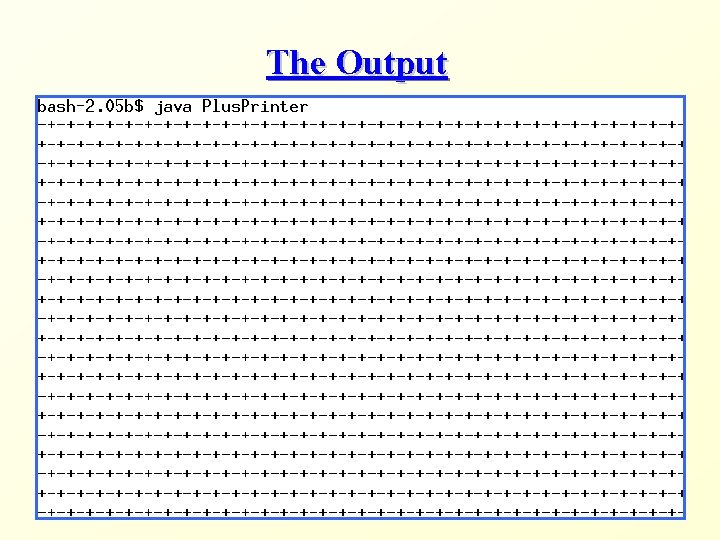
The Output
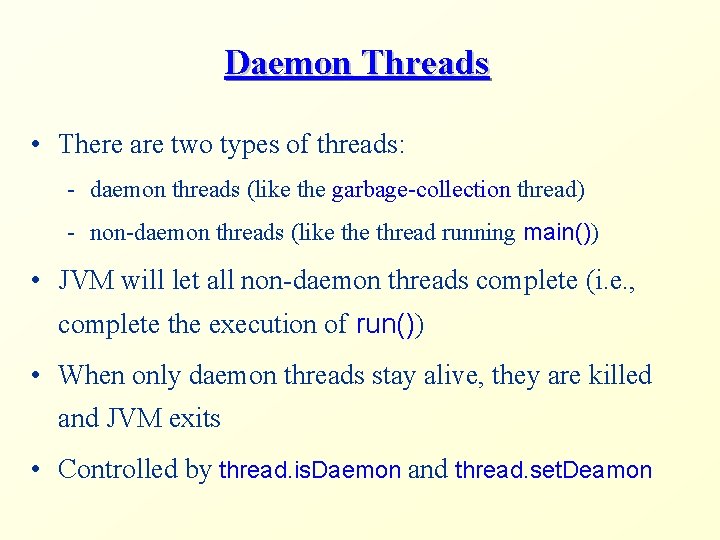
Daemon Threads • There are two types of threads: - daemon threads (like the garbage-collection thread) - non-daemon threads (like thread running main()) • JVM will let all non-daemon threads complete (i. e. , complete the execution of run()) • When only daemon threads stay alive, they are killed and JVM exits • Controlled by thread. is. Daemon and thread. set. Deamon
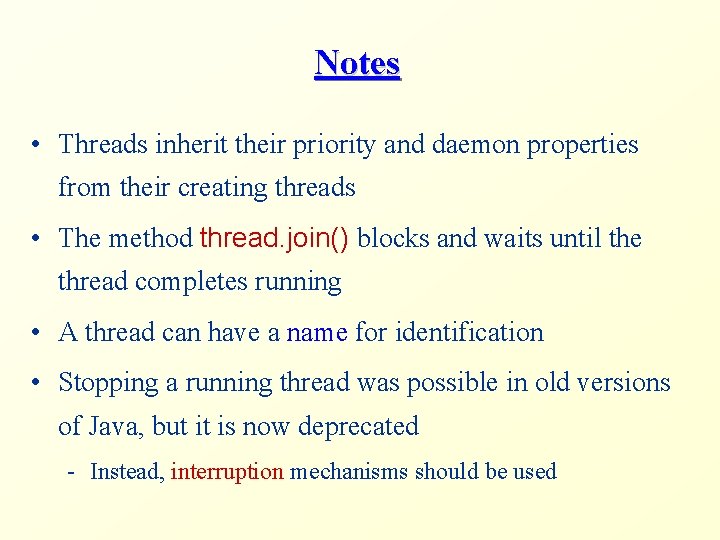
Notes • Threads inherit their priority and daemon properties from their creating threads • The method thread. join() blocks and waits until the thread completes running • A thread can have a name for identification • Stopping a running thread was possible in old versions of Java, but it is now deprecated - Instead, interruption mechanisms should be used
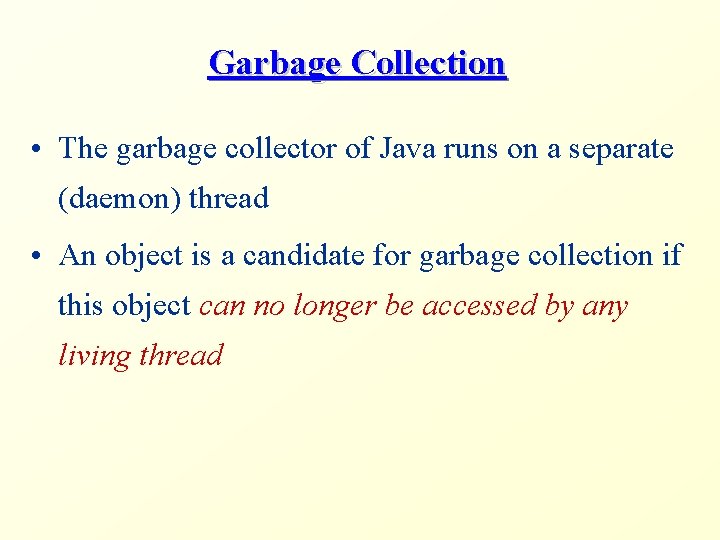
Garbage Collection • The garbage collector of Java runs on a separate (daemon) thread • An object is a candidate for garbage collection if this object can no longer be accessed by any living thread
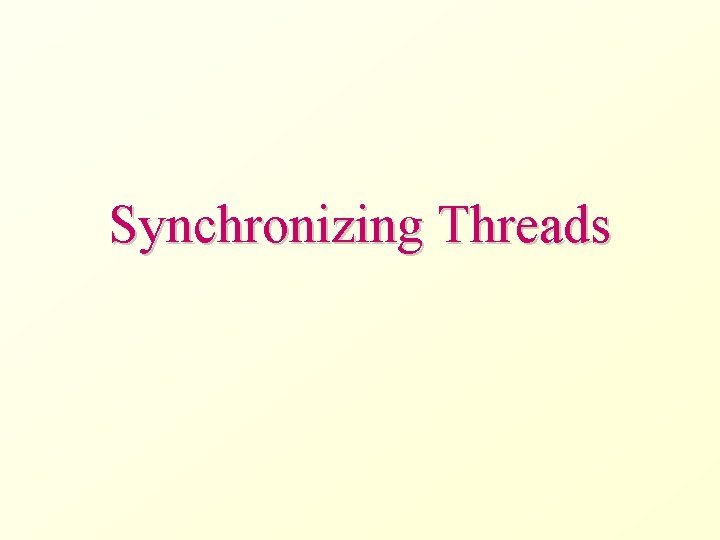
Synchronizing Threads
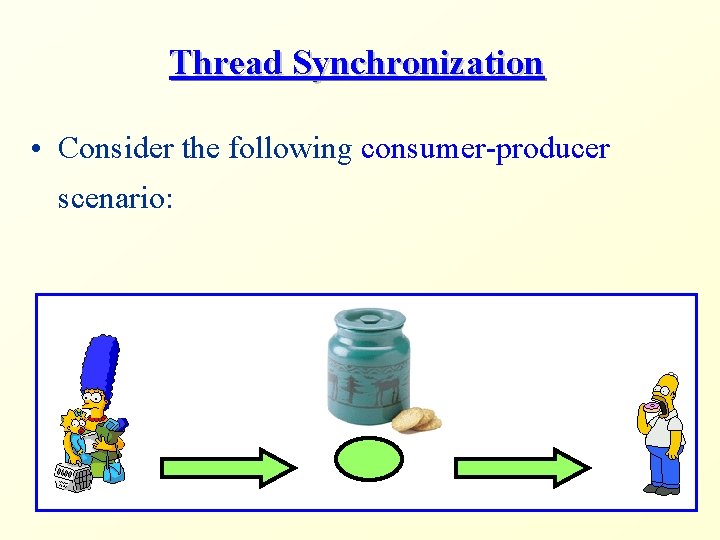
Thread Synchronization • Consider the following consumer-producer scenario:
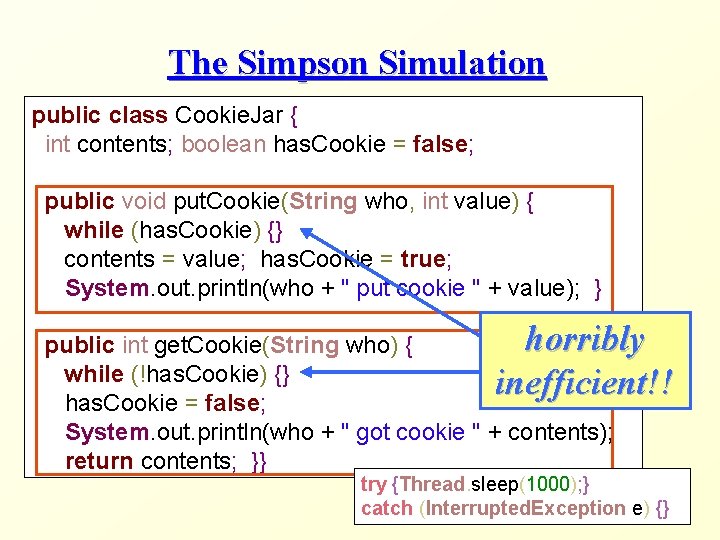
The Simpson Simulation public class Cookie. Jar { int contents; boolean has. Cookie = false; public void put. Cookie(String who, int value) { while (has. Cookie) {} contents = value; has. Cookie = true; System. out. println(who + " put cookie " + value); } horribly public int get. Cookie(String who) { while (!has. Cookie) {} inefficient!! has. Cookie = false; System. out. println(who + " got cookie " + contents); return contents; }} try {Thread. sleep(1000); } catch (Interrupted. Exception e) {}
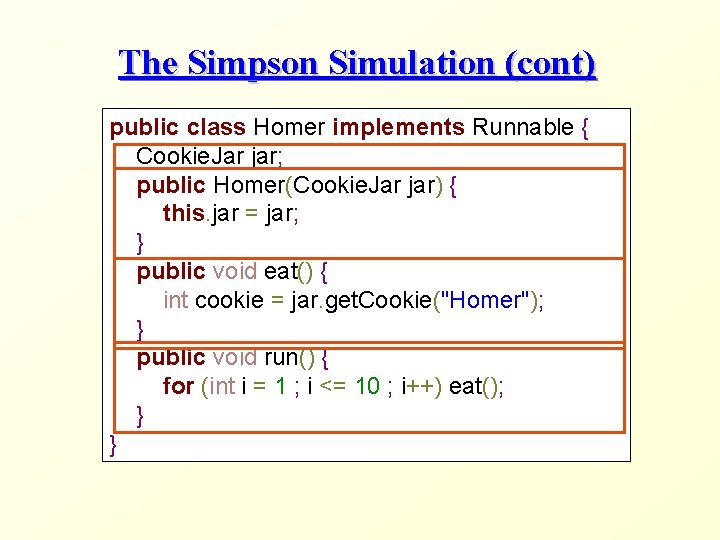
The Simpson Simulation (cont) public class Homer implements Runnable { Cookie. Jar jar; public Homer(Cookie. Jar jar) { this. jar = jar; } public void eat() { int cookie = jar. get. Cookie("Homer"); } public void run() { for (int i = 1 ; i <= 10 ; i++) eat(); } }
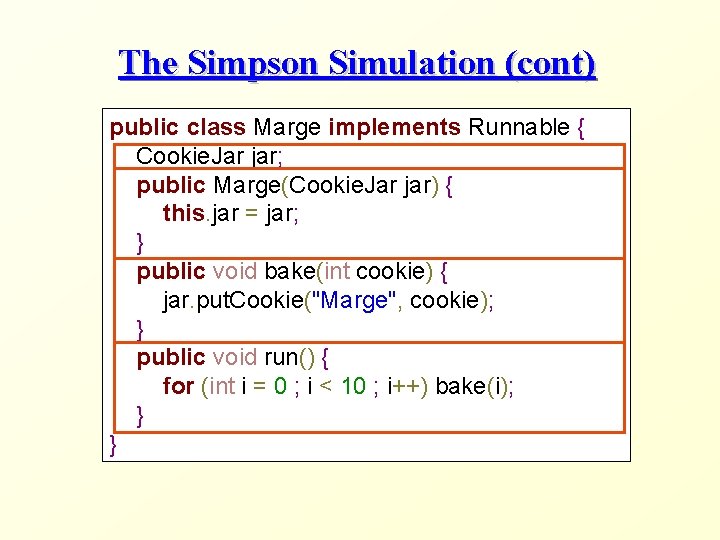
The Simpson Simulation (cont) public class Marge implements Runnable { Cookie. Jar jar; public Marge(Cookie. Jar jar) { this. jar = jar; } public void bake(int cookie) { jar. put. Cookie("Marge", cookie); } public void run() { for (int i = 0 ; i < 10 ; i++) bake(i); } }
![The Simpson Simulation cont public class Run Simpsons public static void mainString args The Simpson Simulation (cont) public class Run. Simpsons { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/cb794a921530f1109890c072a18da491/image-34.jpg)
The Simpson Simulation (cont) public class Run. Simpsons { public static void main(String[] args) { Cookie. Jar jar = new Cookie. Jar(); Homer homer = new Homer(jar); Marge marge = new Marge(jar); new Thread(homer). start(); new Thread(marge). start(); } }
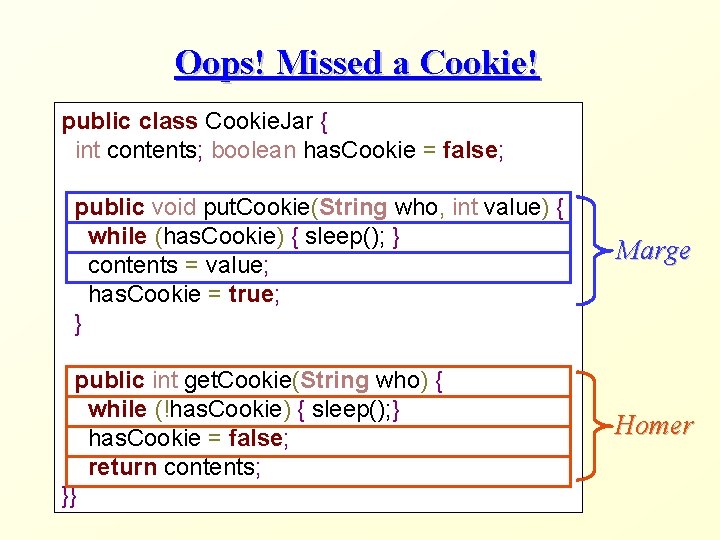
Oops! Missed a Cookie! public class Cookie. Jar { int contents; boolean has. Cookie = false; public void put. Cookie(String who, int value) { while (has. Cookie) { sleep(); } contents = value; has. Cookie = true; } public int get. Cookie(String who) { while (!has. Cookie) { sleep(); } has. Cookie = false; return contents; }} Marge Homer
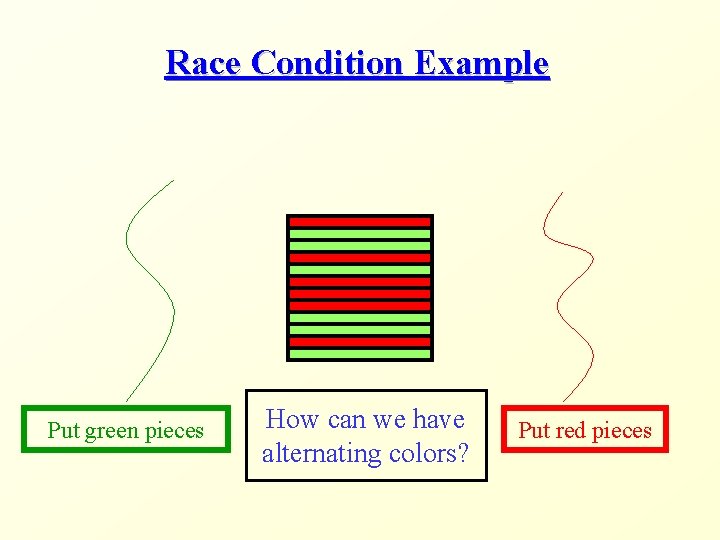
Race Condition Example Put green pieces How can we have alternating colors? Put red pieces
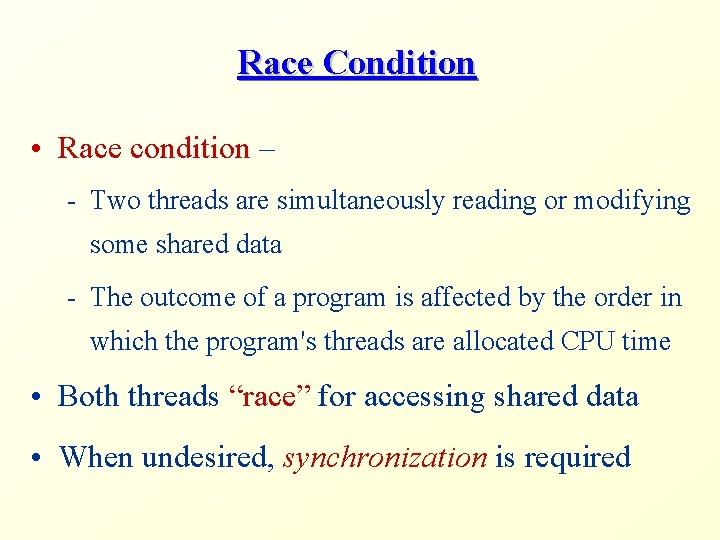
Race Condition • Race condition – - Two threads are simultaneously reading or modifying some shared data - The outcome of a program is affected by the order in which the program's threads are allocated CPU time • Both threads “race” for accessing shared data • When undesired, synchronization is required
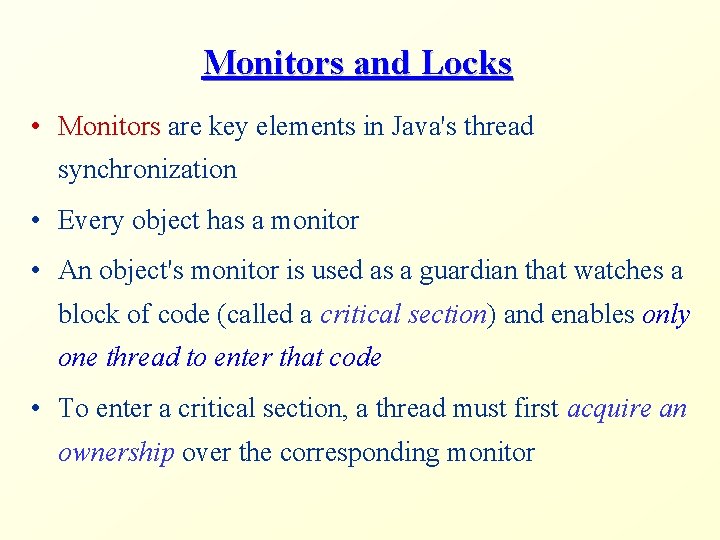
Monitors and Locks • Monitors are key elements in Java's thread synchronization • Every object has a monitor • An object's monitor is used as a guardian that watches a block of code (called a critical section) and enables only one thread to enter that code • To enter a critical section, a thread must first acquire an ownership over the corresponding monitor
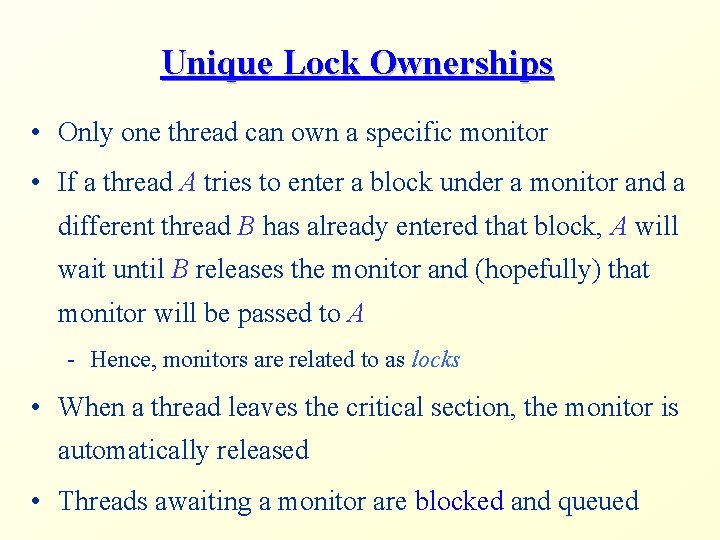
Unique Lock Ownerships • Only one thread can own a specific monitor • If a thread A tries to enter a block under a monitor and a different thread B has already entered that block, A will wait until B releases the monitor and (hopefully) that monitor will be passed to A - Hence, monitors are related to as locks • When a thread leaves the critical section, the monitor is automatically released • Threads awaiting a monitor are blocked and queued
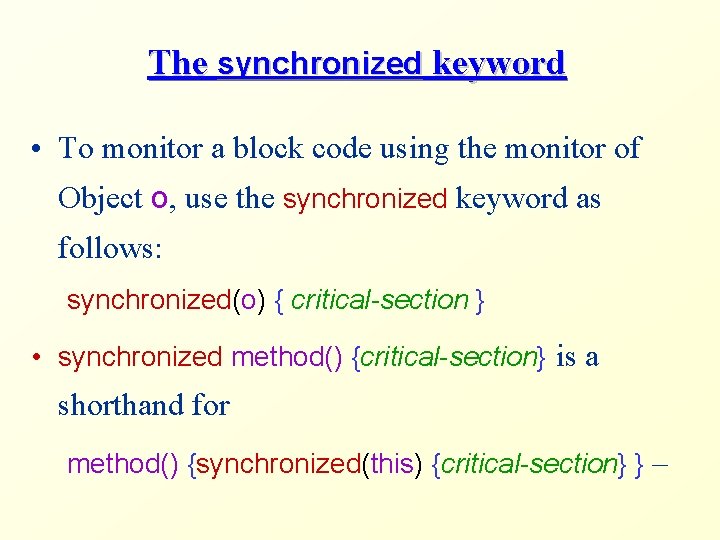
The synchronized keyword • To monitor a block code using the monitor of Object o, use the synchronized keyword as follows: synchronized(o) { critical-section } • synchronized method() {critical-section} is a shorthand for method() {synchronized(this) {critical-section} } –
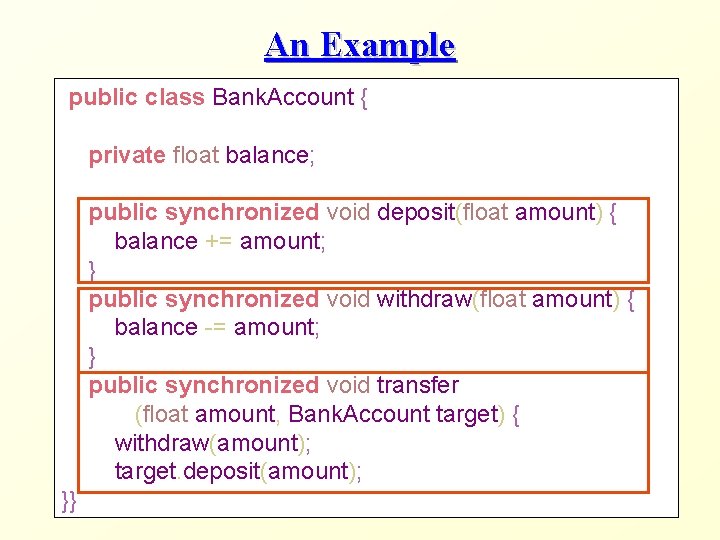
An Example public class Bank. Account { private float balance; public synchronized void deposit(float amount) { balance += amount; } public synchronized void withdraw(float amount) { balance -= amount; } public synchronized void transfer (float amount, Bank. Account target) { withdraw(amount); target. deposit(amount); }}
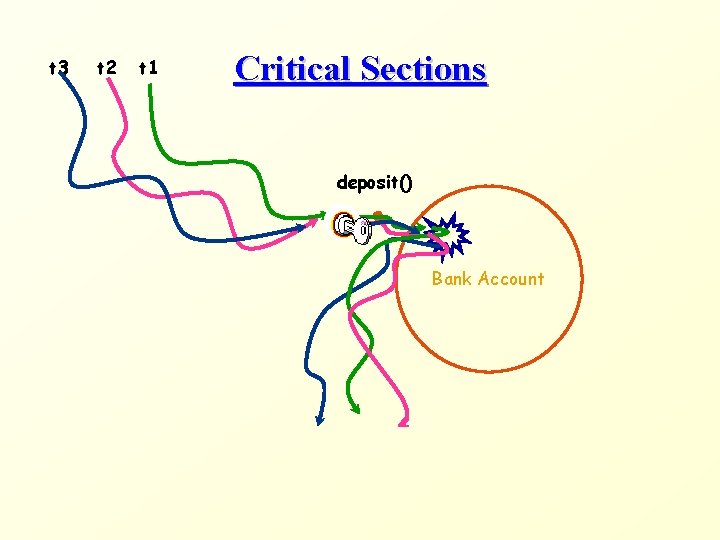
t 3 t 2 t 1 Critical Sections deposit() Bank Account
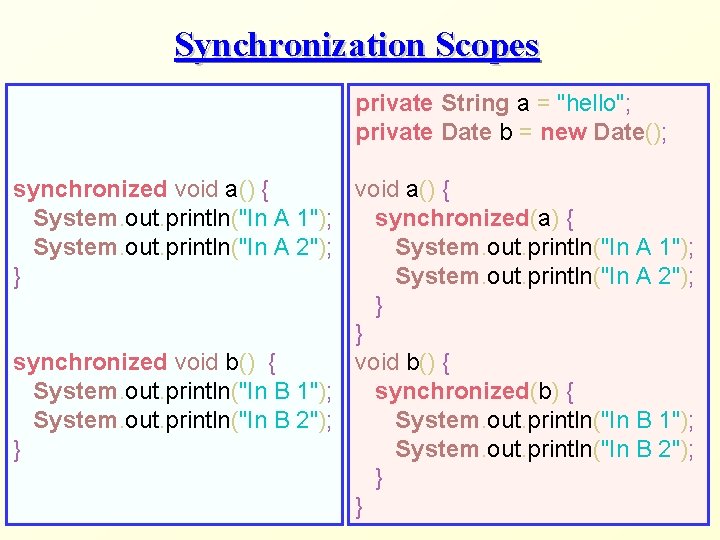
Synchronization Scopes private String a = "hello"; private Date b = new Date(); synchronized void a() { System. out. println("In A 1"); synchronized(a) { System. out. println("In A 2"); System. out. println("In A 1"); } System. out. println("In A 2"); } } synchronized void b() { System. out. println("In B 1"); synchronized(b) { System. out. println("In B 2"); System. out. println("In B 1"); } System. out. println("In B 2"); } }
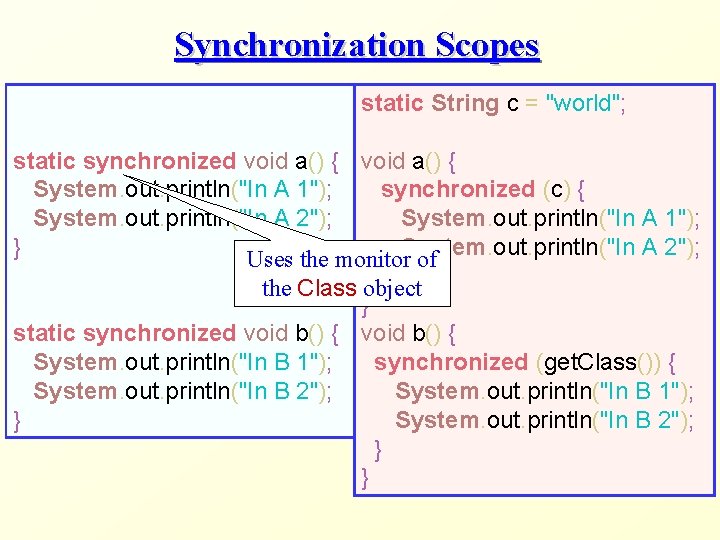
Synchronization Scopes static String c = "world"; static synchronized void a() { System. out. println("In A 1"); synchronized (c) { System. out. println("In A 2"); System. out. println("In A 1"); } System. out. println("In A 2"); Uses the monitor of } the Class object } static synchronized void b() { System. out. println("In B 1"); synchronized (get. Class()) { System. out. println("In B 2"); System. out. println("In B 1"); } System. out. println("In B 2"); } }
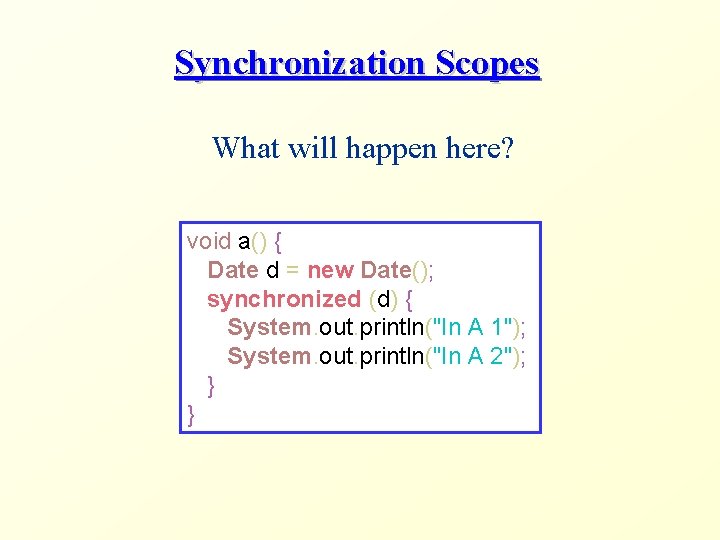
Synchronization Scopes What will happen here? void a() { Date d = new Date(); synchronized (d) { System. out. println("In A 1"); System. out. println("In A 2"); } }
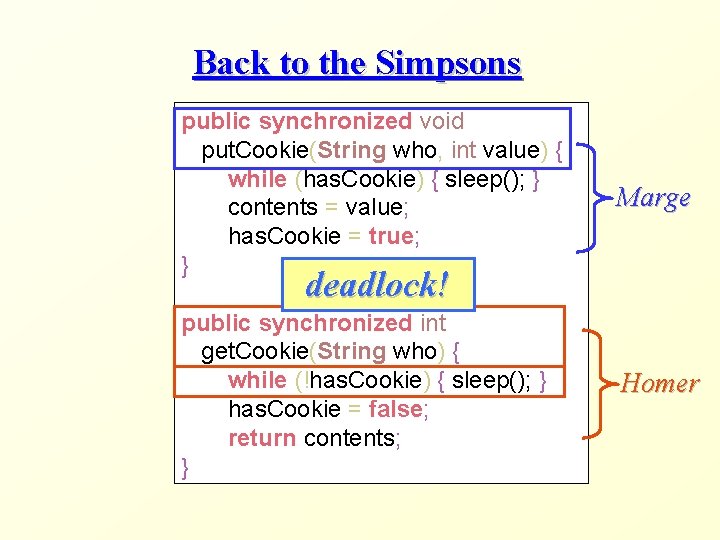
Back to the Simpsons public synchronized void put. Cookie(String who, int value) { while (has. Cookie) { sleep(); } contents = value; has. Cookie = true; } Marge public synchronized int get. Cookie(String who) { while (!has. Cookie) { sleep(); } has. Cookie = false; return contents; } Homer deadlock!
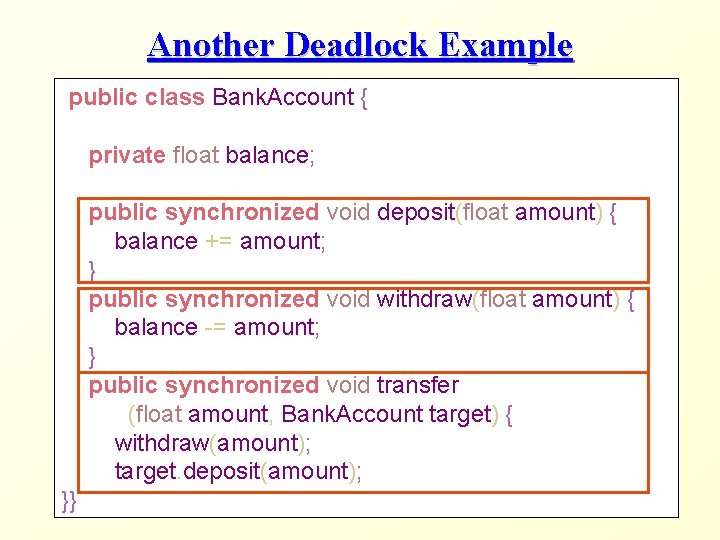
Another Deadlock Example public class Bank. Account { private float balance; public synchronized void deposit(float amount) { balance += amount; } public synchronized void withdraw(float amount) { balance -= amount; } public synchronized void transfer (float amount, Bank. Account target) { withdraw(amount); target. deposit(amount); }}
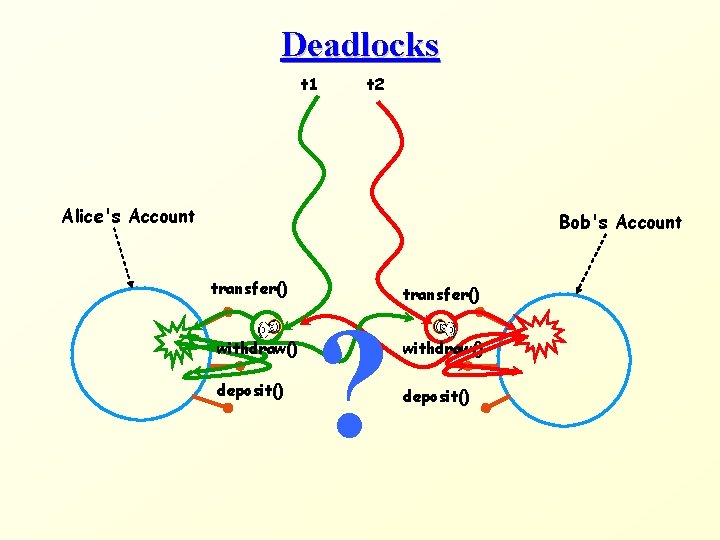
Deadlocks t 1 t 2 Alice's Account Bob's Account transfer() withdraw() deposit() ? transfer() withdraw() deposit()
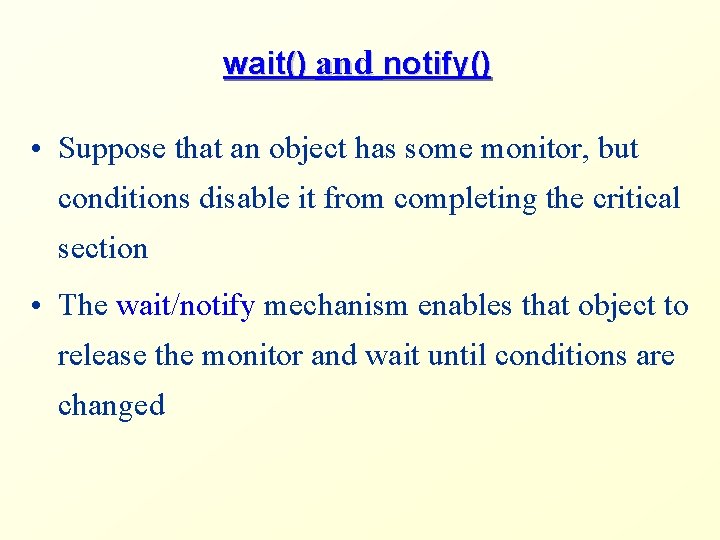
wait() and notify() • Suppose that an object has some monitor, but conditions disable it from completing the critical section • The wait/notify mechanism enables that object to release the monitor and wait until conditions are changed
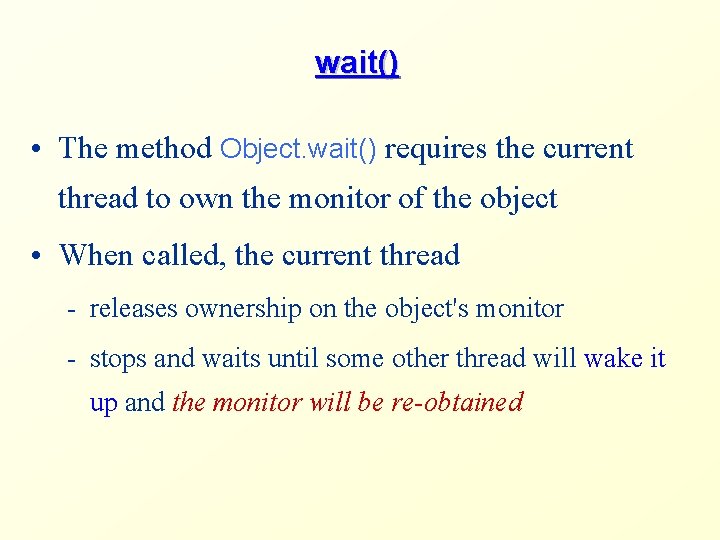
wait() • The method Object. wait() requires the current thread to own the monitor of the object • When called, the current thread - releases ownership on the object's monitor - stops and waits until some other thread will wake it up and the monitor will be re-obtained
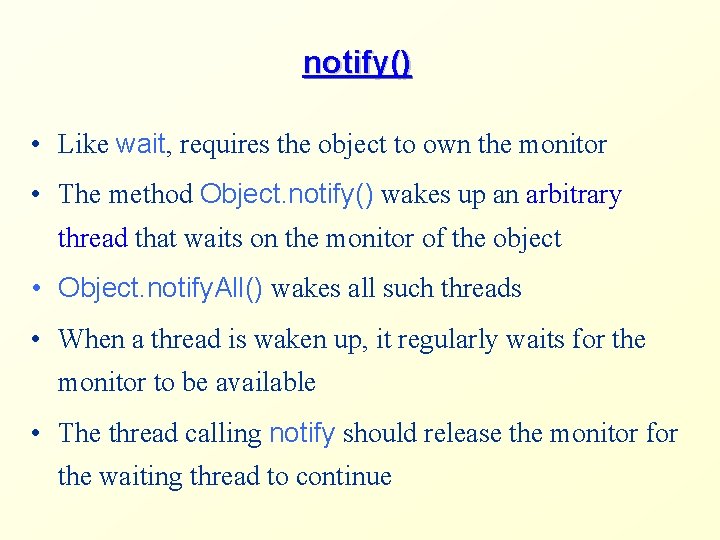
notify() • Like wait, requires the object to own the monitor • The method Object. notify() wakes up an arbitrary thread that waits on the monitor of the object • Object. notify. All() wakes all such threads • When a thread is waken up, it regularly waits for the monitor to be available • The thread calling notify should release the monitor for the waiting thread to continue
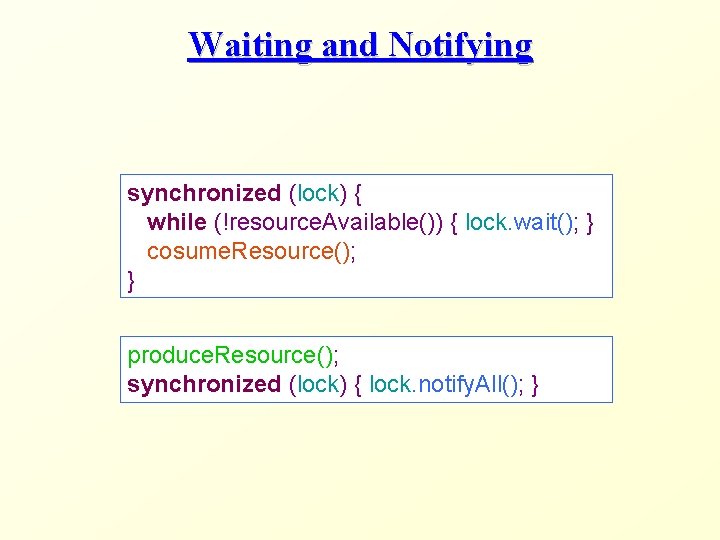
Waiting and Notifying synchronized (lock) { while (!resource. Available()) { lock. wait(); } cosume. Resource(); } produce. Resource(); synchronized (lock) { lock. notify. All(); }
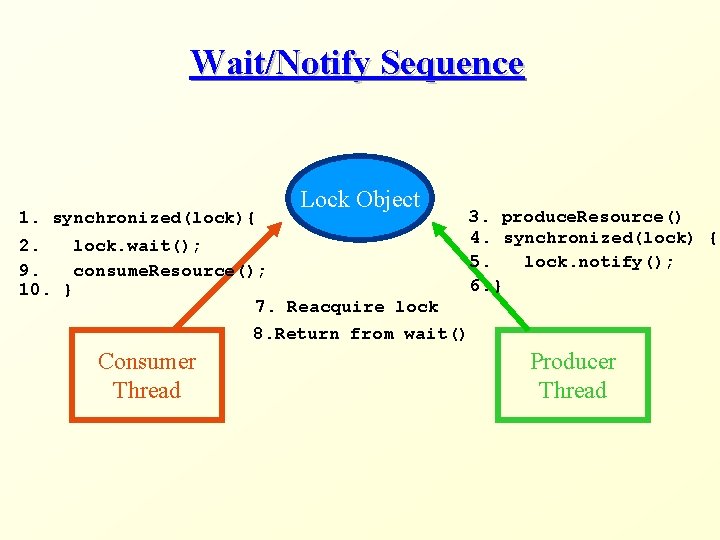
Wait/Notify Sequence 1. synchronized(lock){ Lock Object 2. lock. wait(); 9. consume. Resource(); 10. } 7. Reacquire lock 8. Return from wait() Consumer Thread 3. produce. Resource() 4. synchronized(lock) { 5. lock. notify(); 6. } Producer Thread
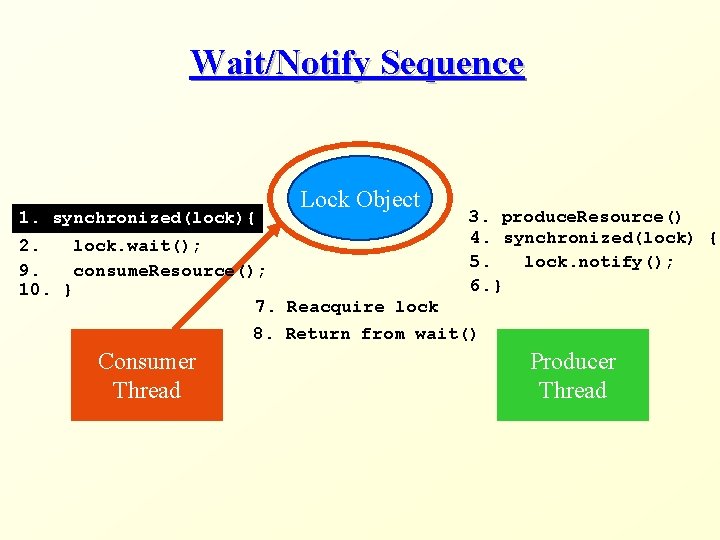
Wait/Notify Sequence 1. synchronized(lock){ Lock Object 3. produce. Resource() 4. synchronized(lock) { 5. lock. notify(); 6. } 2. lock. wait(); 9. consume. Resource(); 10. } 7. Reacquire lock 8. Return from wait() Consumer Thread Producer Thread
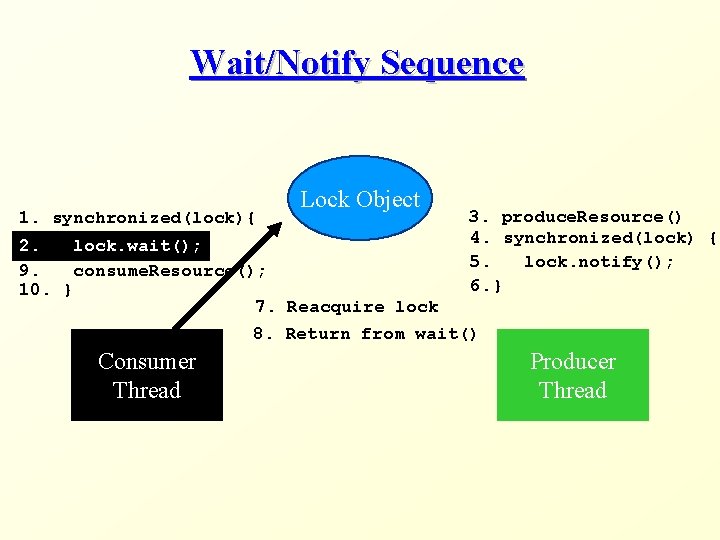
Wait/Notify Sequence 1. synchronized(lock){ Lock Object 3. produce. Resource() 4. synchronized(lock) { 5. lock. notify(); 6. } 2. lock. wait(); 9. consume. Resource(); 10. } 7. Reacquire lock 8. Return from wait() Consumer Thread Producer Thread
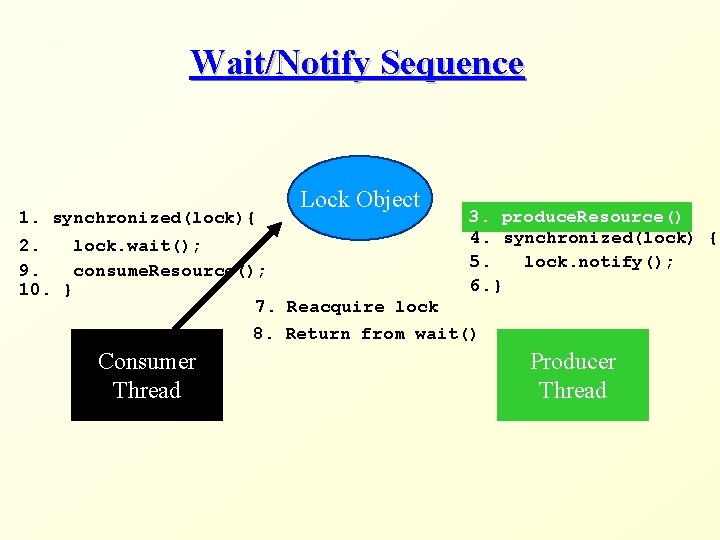
Wait/Notify Sequence 1. synchronized(lock){ Lock Object 3. produce. Resource() 4. synchronized(lock) { 5. lock. notify(); 6. } 2. lock. wait(); 9. consume. Resource(); 10. } 7. Reacquire lock 8. Return from wait() Consumer Thread Producer Thread
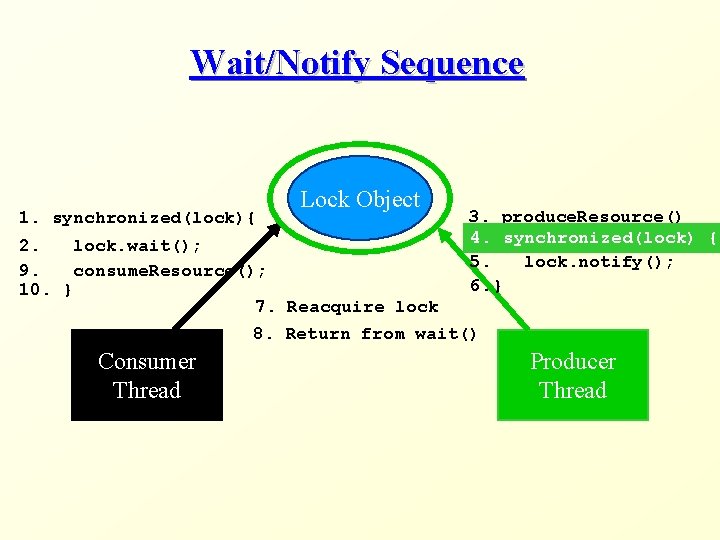
Wait/Notify Sequence 1. synchronized(lock){ Lock Object 3. produce. Resource() 4. synchronized(lock) { 5. lock. notify(); 6. } 2. lock. wait(); 9. consume. Resource(); 10. } 7. Reacquire lock 8. Return from wait() Consumer Thread Producer Thread
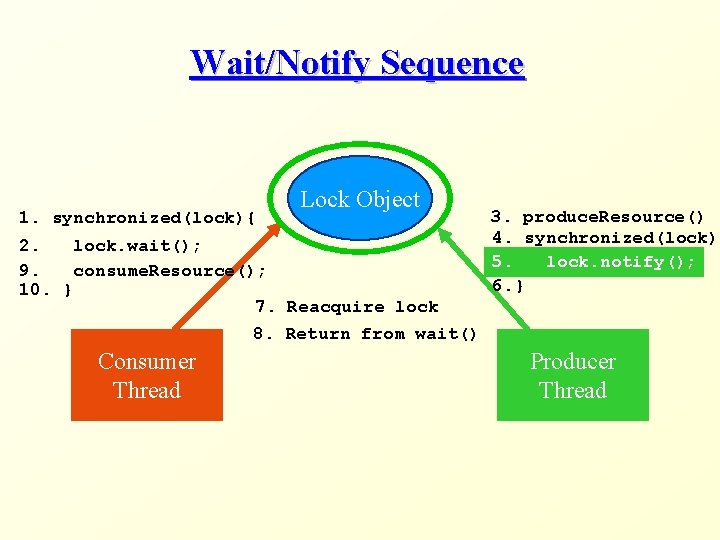
Wait/Notify Sequence 1. synchronized(lock){ Lock Object 2. lock. wait(); 9. consume. Resource(); 10. } 7. Reacquire lock 8. Return from wait() Consumer Thread 3. produce. Resource() 4. synchronized(lock) 5. lock. notify(); 6. } Producer Thread
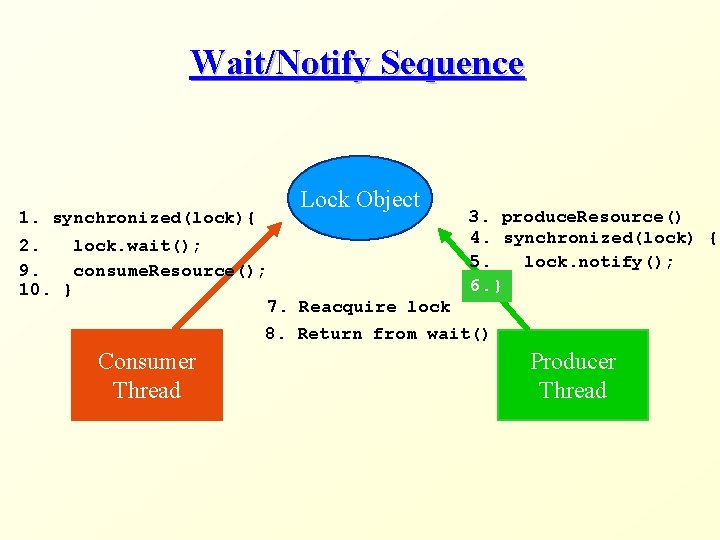
Wait/Notify Sequence 1. synchronized(lock){ Lock Object 3. produce. Resource() 4. synchronized(lock) { 5. lock. notify(); 6. } 2. lock. wait(); 9. consume. Resource(); 10. } 7. Reacquire lock 8. Return from wait() Consumer Thread Producer Thread
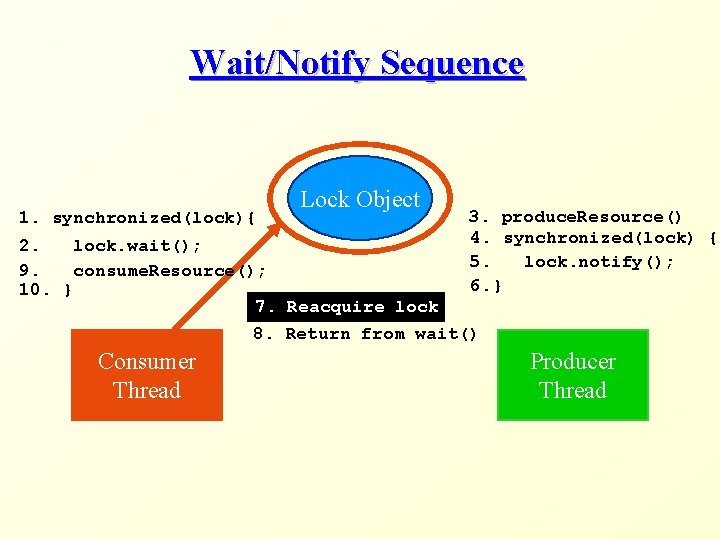
Wait/Notify Sequence 1. synchronized(lock){ Lock Object 3. produce. Resource() 4. synchronized(lock) { 5. lock. notify(); 6. } 2. lock. wait(); 9. consume. Resource(); 10. } 7. Reacquire lock 8. Return from wait() Consumer Thread Producer Thread
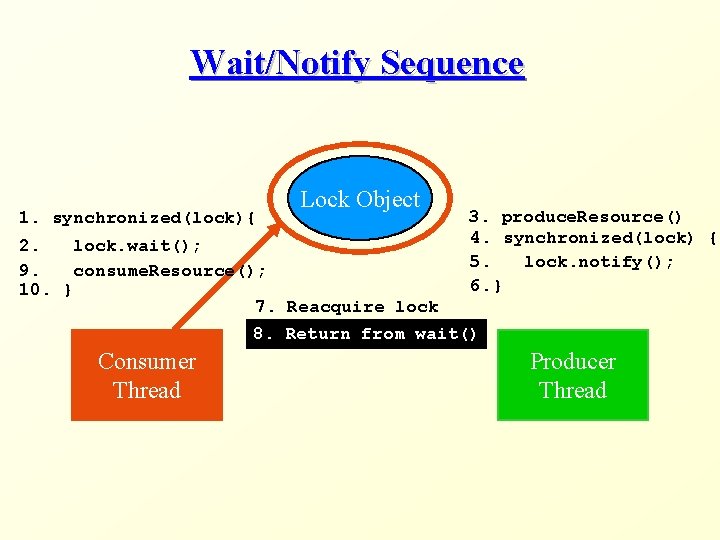
Wait/Notify Sequence 1. synchronized(lock){ Lock Object 3. produce. Resource() 4. synchronized(lock) { 5. lock. notify(); 6. } 2. lock. wait(); 9. consume. Resource(); 10. } 7. Reacquire lock 8. Return from wait() Consumer Thread Producer Thread
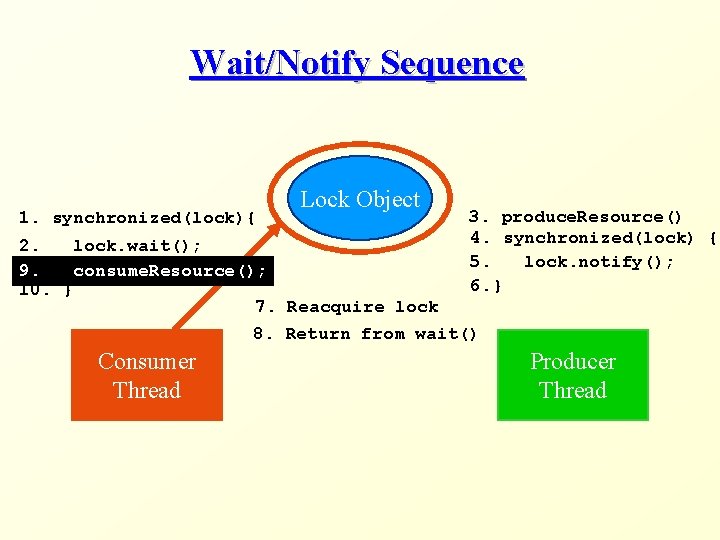
Wait/Notify Sequence 1. synchronized(lock){ Lock Object 3. produce. Resource() 4. synchronized(lock) { 5. lock. notify(); 6. } 2. lock. wait(); 9. consume. Resource(); 10. } 7. Reacquire lock 8. Return from wait() Consumer Thread Producer Thread
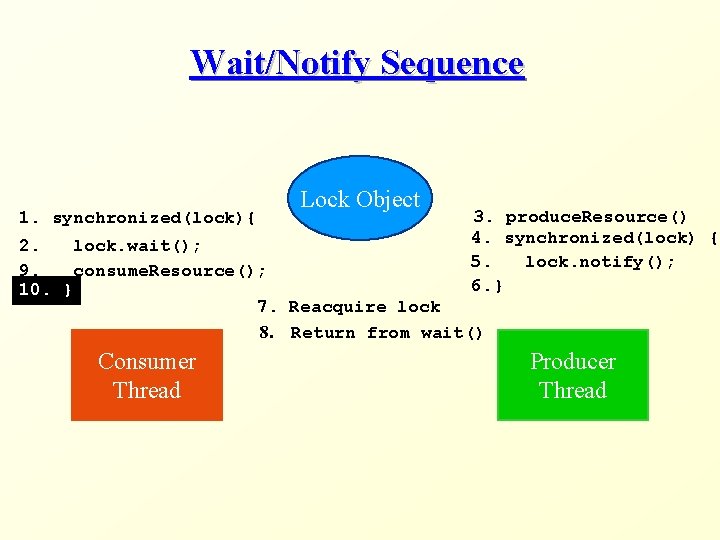
Wait/Notify Sequence 1. synchronized(lock){ Lock Object 3. produce. Resource() 4. synchronized(lock) { 5. lock. notify(); 6. } 2. lock. wait(); 9. consume. Resource(); 10. } 7. Reacquire lock 8. Return from wait() Consumer Thread Producer Thread
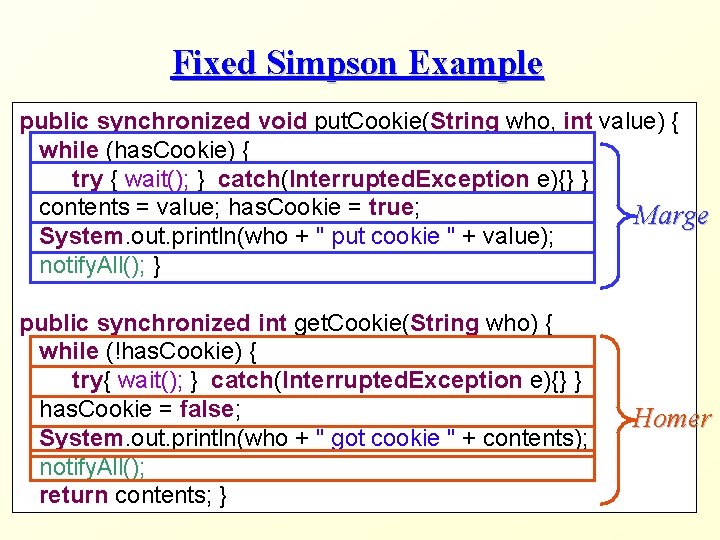
Fixed Simpson Example public synchronized void put. Cookie(String who, int value) { while (has. Cookie) { try { wait(); } catch(Interrupted. Exception e){} } contents = value; has. Cookie = true; Marge System. out. println(who + " put cookie " + value); notify. All(); } public synchronized int get. Cookie(String who) { while (!has. Cookie) { try{ wait(); } catch(Interrupted. Exception e){} } has. Cookie = false; System. out. println(who + " got cookie " + contents); notify. All(); return contents; } Homer
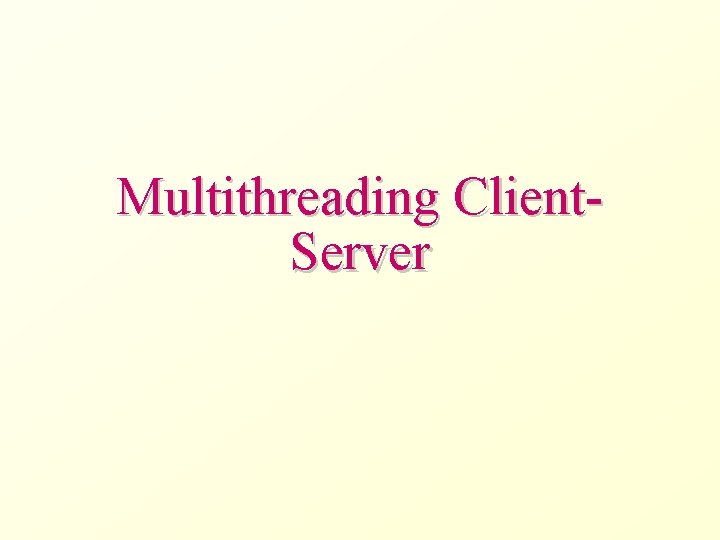
Multithreading Client. Server
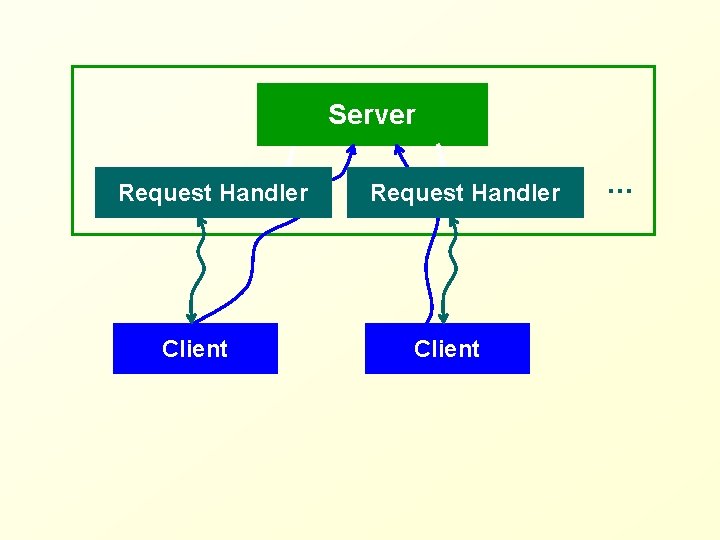
Server Request Handler Client …
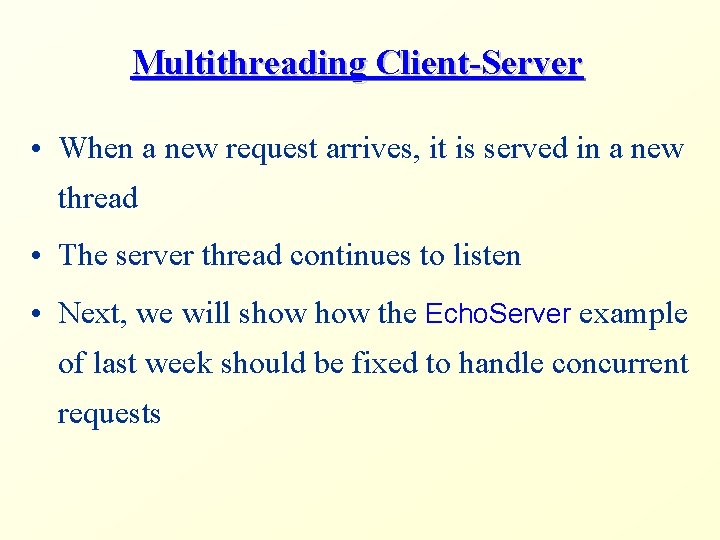
Multithreading Client-Server • When a new request arrives, it is served in a new thread • The server thread continues to listen • Next, we will show the Echo. Server example of last week should be fixed to handle concurrent requests
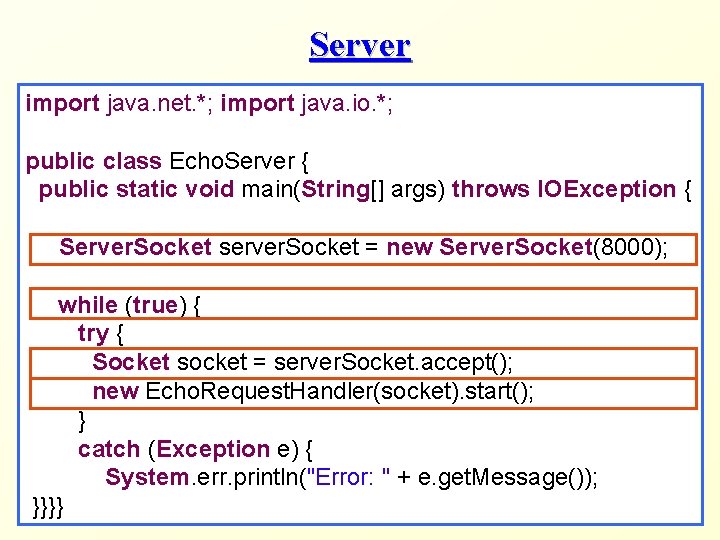
Server import java. net. *; import java. io. *; public class Echo. Server { public static void main(String[] args) throws IOException { Server. Socket server. Socket = new Server. Socket(8000); while (true) { try { Socket socket = server. Socket. accept(); new Echo. Request. Handler(socket). start(); } catch (Exception e) { System. err. println("Error: " + e. get. Message()); }}}}
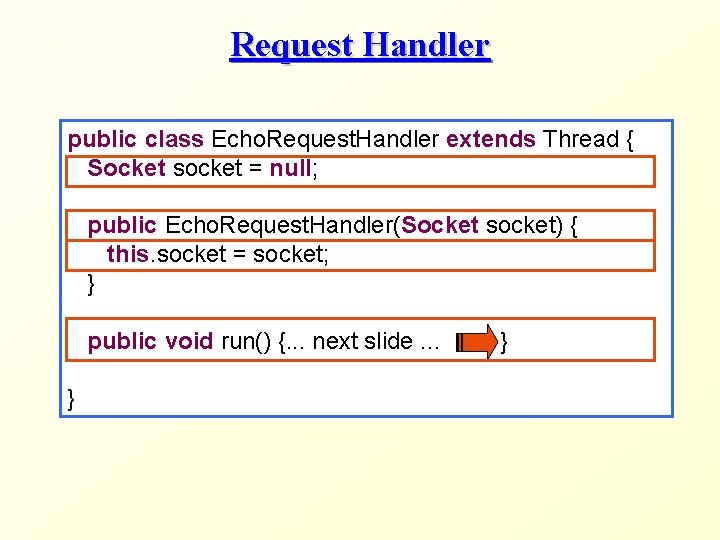
Request Handler public class Echo. Request. Handler extends Thread { Socket socket = null; public Echo. Request. Handler(Socket socket) { this. socket = socket; } public void run() {. . . next slide. . . } }
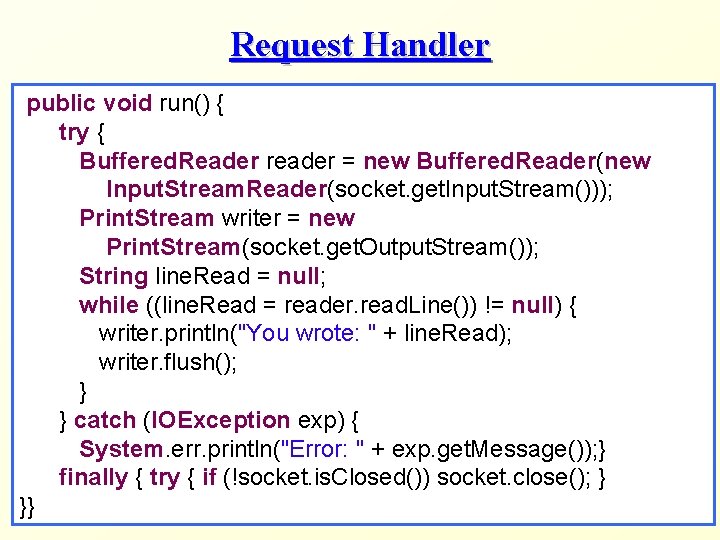
Request Handler public void run() { try { Buffered. Reader reader = new Buffered. Reader(new Input. Stream. Reader(socket. get. Input. Stream())); Print. Stream writer = new Print. Stream(socket. get. Output. Stream()); String line. Read = null; while ((line. Read = reader. read. Line()) != null) { writer. println("You wrote: " + line. Read); writer. flush(); } } catch (IOException exp) { System. err. println("Error: " + exp. get. Message()); } finally { try { if (!socket. is. Closed()) socket. close(); } }}
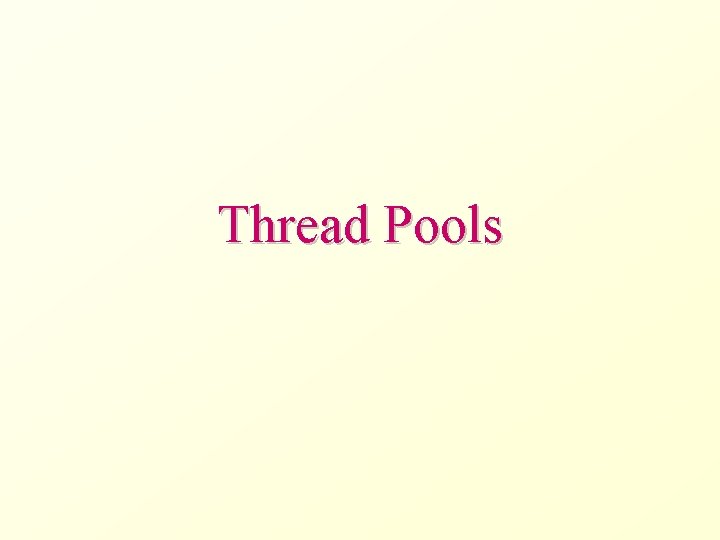
Thread Pools
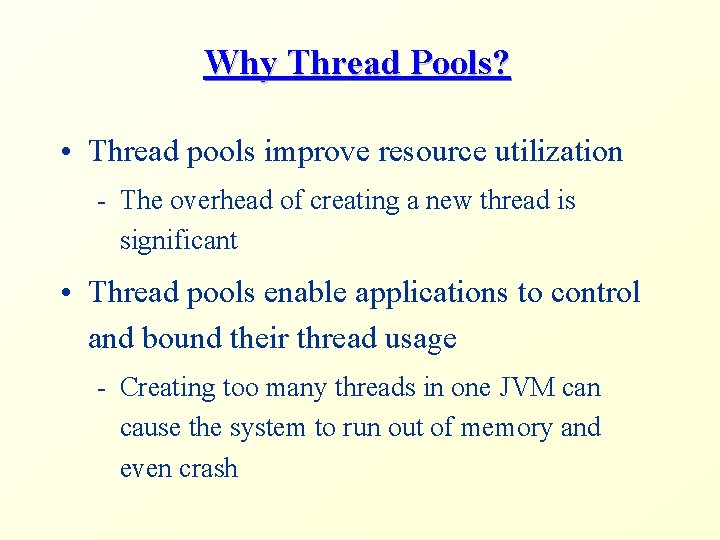
Why Thread Pools? • Thread pools improve resource utilization - The overhead of creating a new thread is significant • Thread pools enable applications to control and bound their thread usage - Creating too many threads in one JVM can cause the system to run out of memory and even crash
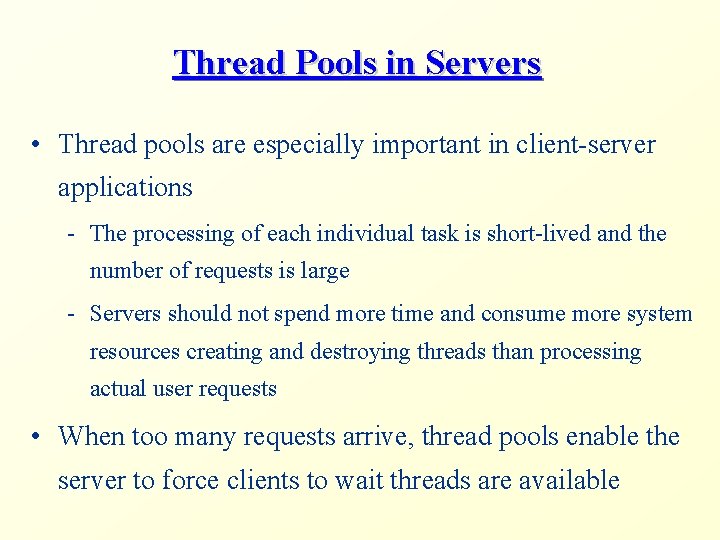
Thread Pools in Servers • Thread pools are especially important in client-server applications - The processing of each individual task is short-lived and the number of requests is large - Servers should not spend more time and consume more system resources creating and destroying threads than processing actual user requests • When too many requests arrive, thread pools enable the server to force clients to wait threads are available
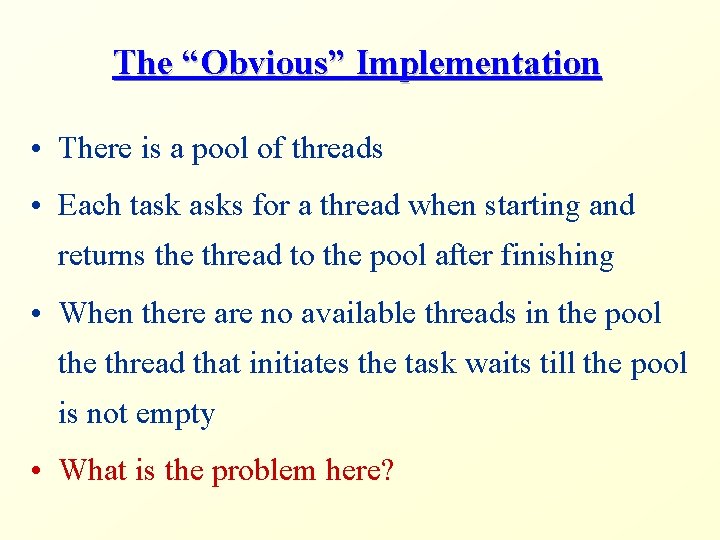
The “Obvious” Implementation • There is a pool of threads • Each task asks for a thread when starting and returns the thread to the pool after finishing • When there are no available threads in the pool the thread that initiates the task waits till the pool is not empty • What is the problem here?
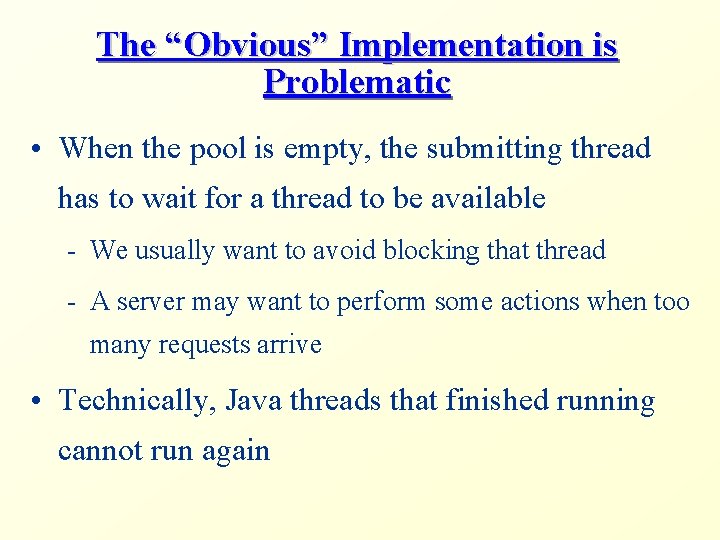
The “Obvious” Implementation is Problematic • When the pool is empty, the submitting thread has to wait for a thread to be available - We usually want to avoid blocking that thread - A server may want to perform some actions when too many requests arrive • Technically, Java threads that finished running cannot run again
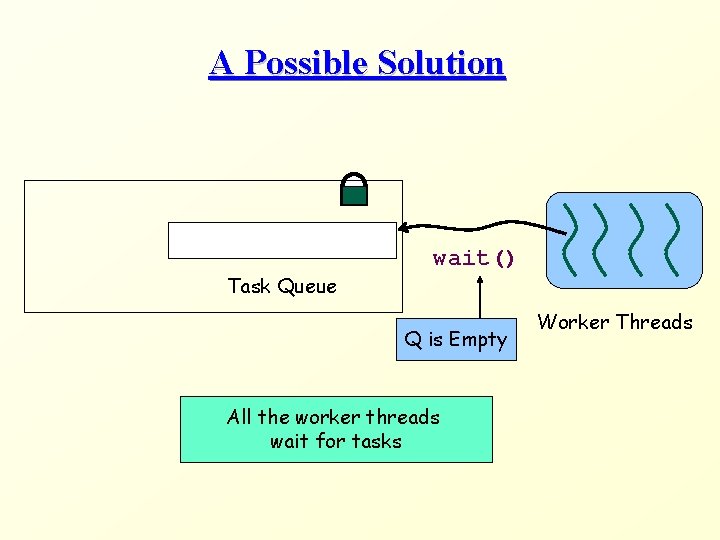
A Possible Solution Task Queue wait() Q is Empty All the worker threads wait for tasks Worker Threads
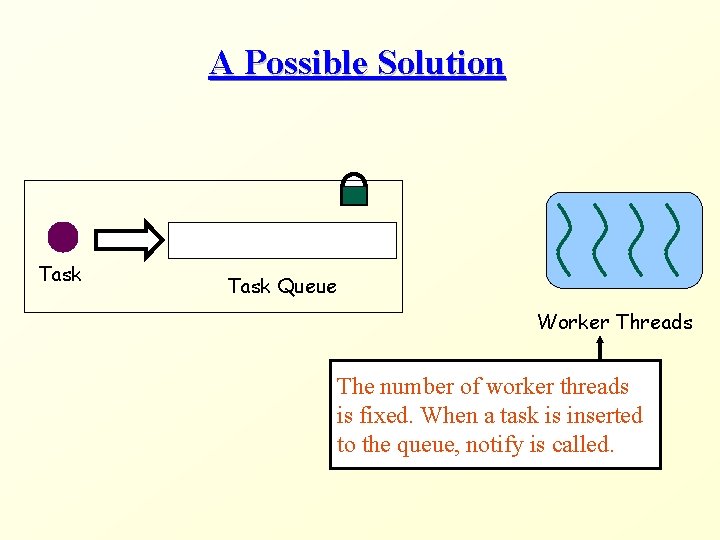
A Possible Solution Task Queue Worker Threads The number of worker threads is fixed. When a task is inserted to the queue, notify is called.
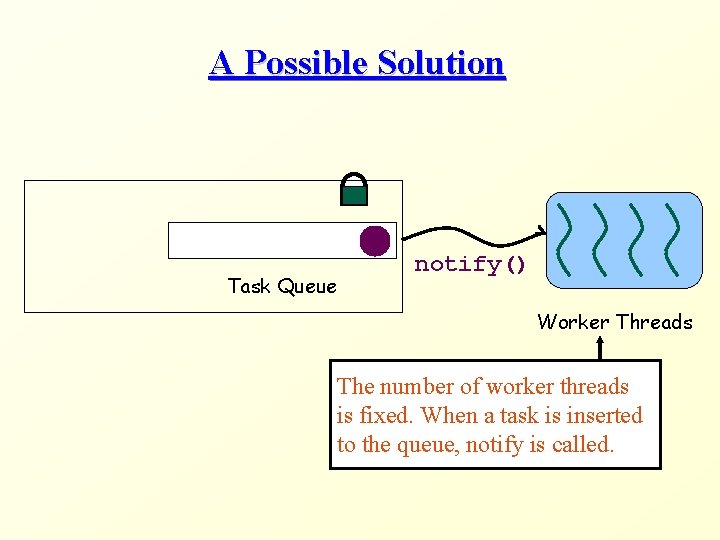
A Possible Solution Task Queue notify() Worker Threads The number of worker threads is fixed. When a task is inserted to the queue, notify is called.
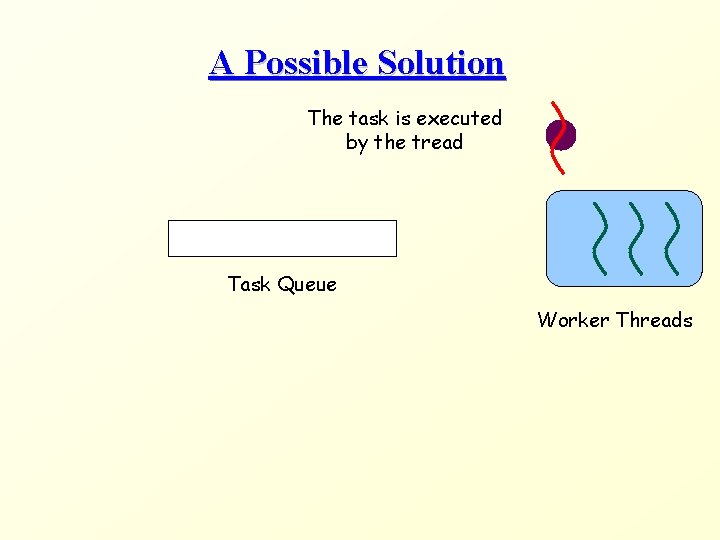
A Possible Solution The task is executed by the tread Task Queue Worker Threads
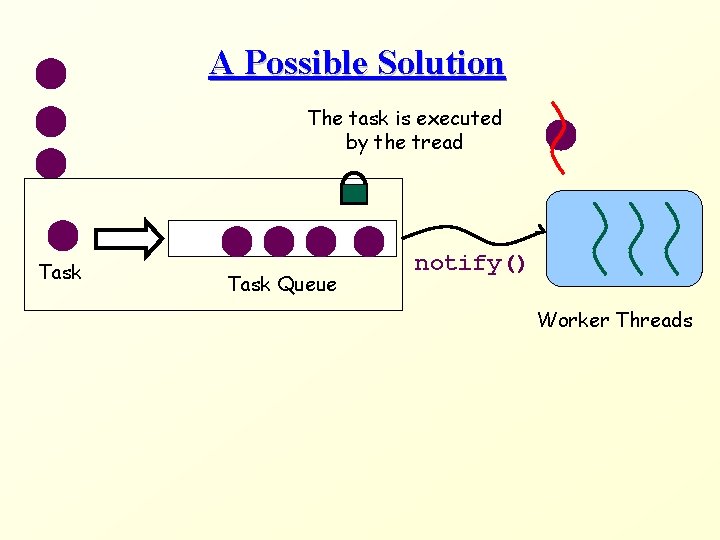
A Possible Solution The task is executed by the tread Task Queue notify() Worker Threads
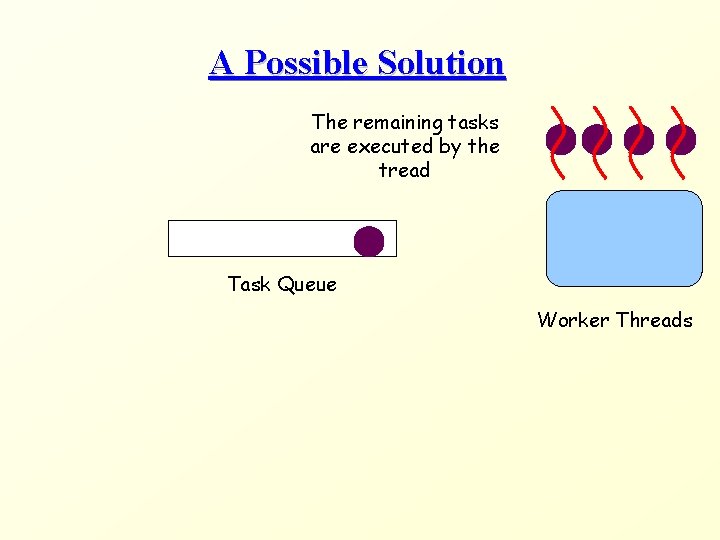
A Possible Solution The remaining tasks are executed by the tread Task Queue Worker Threads
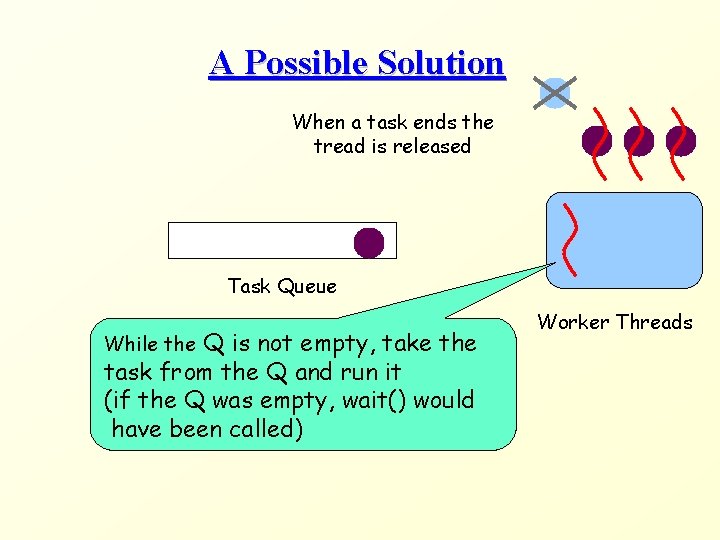
A Possible Solution When a task ends the tread is released Task Queue Q is not empty, take the task from the Q and run it (if the Q was empty, wait() would have been called) While the Worker Threads
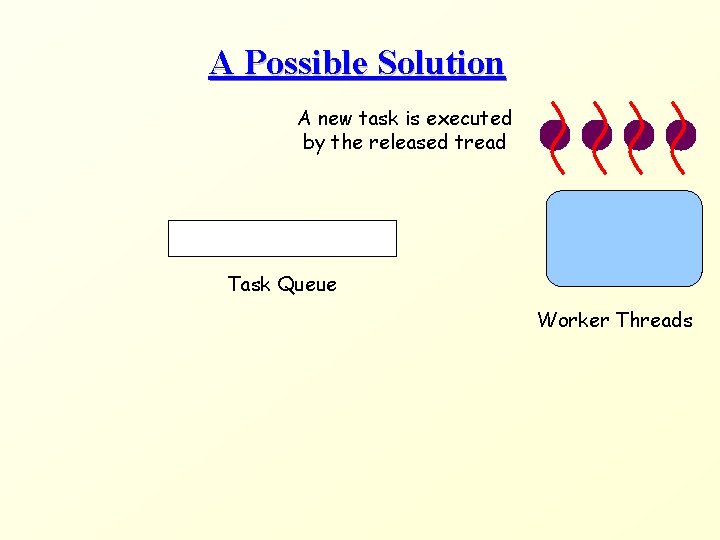
A Possible Solution A new task is executed by the released tread Task Queue Worker Threads
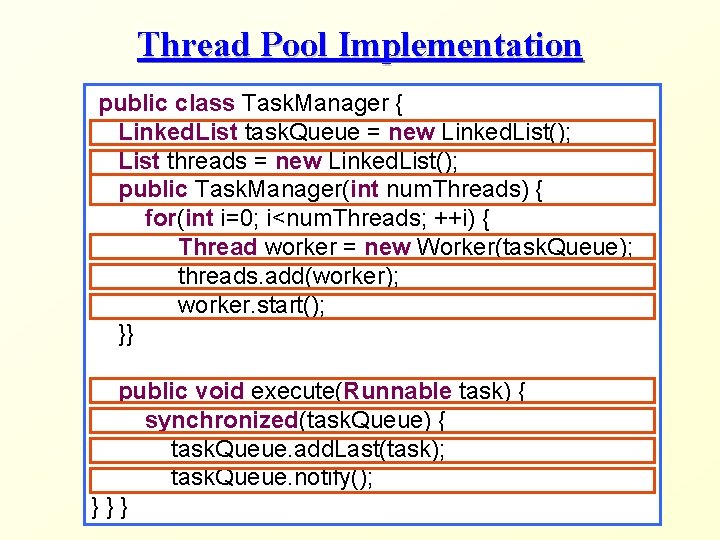
Thread Pool Implementation public class Task. Manager { Linked. List task. Queue = new Linked. List(); List threads = new Linked. List(); public Task. Manager(int num. Threads) { for(int i=0; i<num. Threads; ++i) { Thread worker = new Worker(task. Queue); threads. add(worker); worker. start(); }} public void execute(Runnable task) { synchronized(task. Queue) { task. Queue. add. Last(task); task. Queue. notify(); }}}
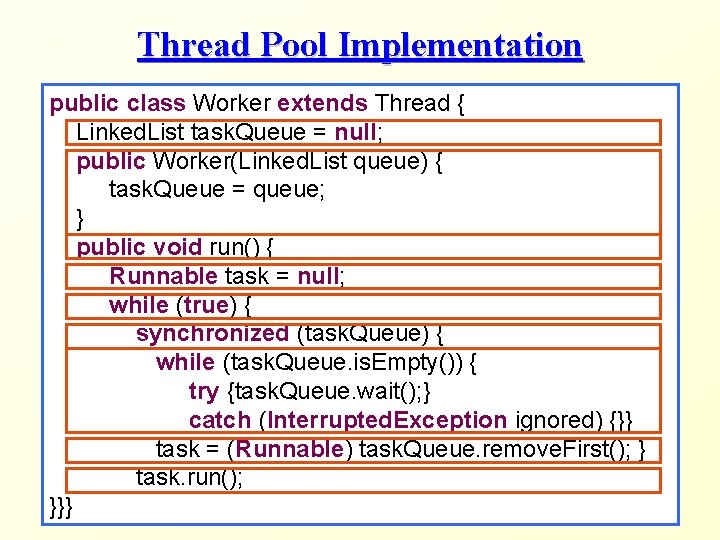
Thread Pool Implementation public class Worker extends Thread { Linked. List task. Queue = null; public Worker(Linked. List queue) { task. Queue = queue; } public void run() { Runnable task = null; while (true) { synchronized (task. Queue) { while (task. Queue. is. Empty()) { try {task. Queue. wait(); } catch (Interrupted. Exception ignored) {}} task = (Runnable) task. Queue. remove. First(); } task. run(); }}}
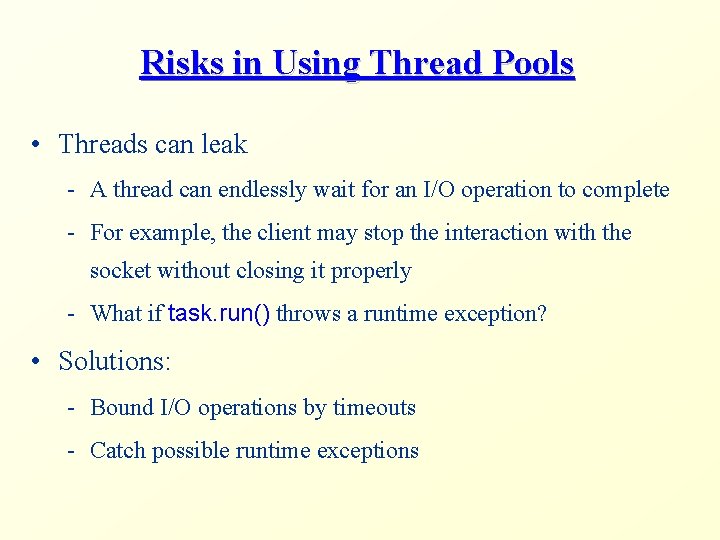
Risks in Using Thread Pools • Threads can leak - A thread can endlessly wait for an I/O operation to complete - For example, the client may stop the interaction with the socket without closing it properly - What if task. run() throws a runtime exception? • Solutions: - Bound I/O operations by timeouts - Catch possible runtime exceptions
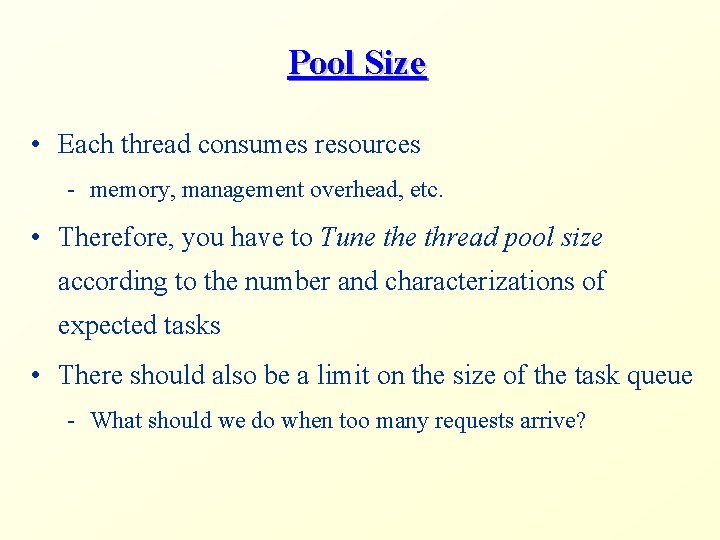
Pool Size • Each thread consumes resources - memory, management overhead, etc. • Therefore, you have to Tune thread pool size according to the number and characterizations of expected tasks • There should also be a limit on the size of the task queue - What should we do when too many requests arrive?
Process thread
Threads vs processes
Concurrent processes are processes that
Shared memory in java
Threads java
Threads em java
Process and threads
Process and threads
Process and thread management in operating system
Introduction to manufacturing processes
Introduction to stochastic processes pdf
What processes are crucial to the ipde process
Sockets and threads
Import java.lang.*
Import java.util.*
Import java swing
Import scanner java
Java 讀檔
Gcd java
Java random
Java import java.io.*
Import java.util.
Java thread import
Apa perbedaan antara java swing dengan java awt
Import java.awt.event
Java compiler translates java source code into
Rmi javatpoint
Java introduction to problem solving and programming
Programming and problem solving with java
Java introduction to problem solving and programming
C11 thread
Conventional representation of threads
Flexible flat material made by interlacing yarns
Escalonamento por prioridades
Os threads
The vinaya pitaka is a sacred text of
Needle like threads of spongy bone
Waxy coated paper which transfers pattern markings
Golden thread principle
Pintos project 1 alarm clock
Pthreads
Alternanthera red threads
Forum.unity.com/threads/game-over.54735
Threads fiji
Mustafa cem kasapbaşı
Posix threads
Forum.unity.com/threads/game-over.54735
Sequence diagram threads
Threads cannot be implemented as a library
Threads.h
For lathe operations, work piece can be hold _____
Cuda threads per block
Threads cannot be implemented as a library
The kernel is unaware of user threads
Cuda thread synchronization
Threads in distributed systems
Ece api
Ece threads
Ece threads
Chia number of threads
Scheduler activation
Pintos advanced scheduler
Mission thread example
Os threads
Elementary programming in java
Llll bean
Introduction to java programming 10th edition quizzes
Introduction to swing in java
Interprocess communication in java
Olecranon process vs fossa
Condylar process and coronoid process
Study of behavior and mental processes
Wharton risk management program
A system has 12 magnetic tape drives and 3 processes
How does the dynamics of community operate as a process
Tools equipment and processes
Irreversible process in thermodynamics
Information processes and technology past papers
Helping students practice skills, strategies, and processes
Why designing and managing service processes
Word formation process
Marketing processes and consumer behavior
Participants processes and circumstances
How to designing and managing service processes
The scientific study of behavior and mental processes
Input-transformation-output model restaurant
Glacier terms
Cultural patterns and processes