Java Persistence API Mario Peshev National Academy for
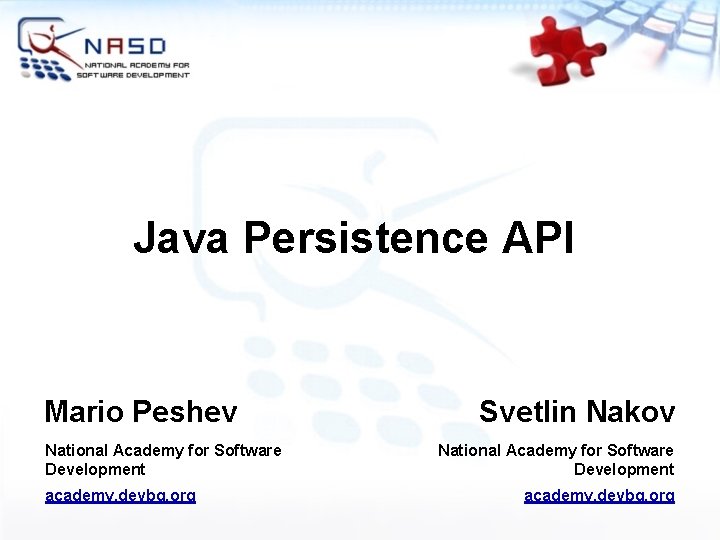
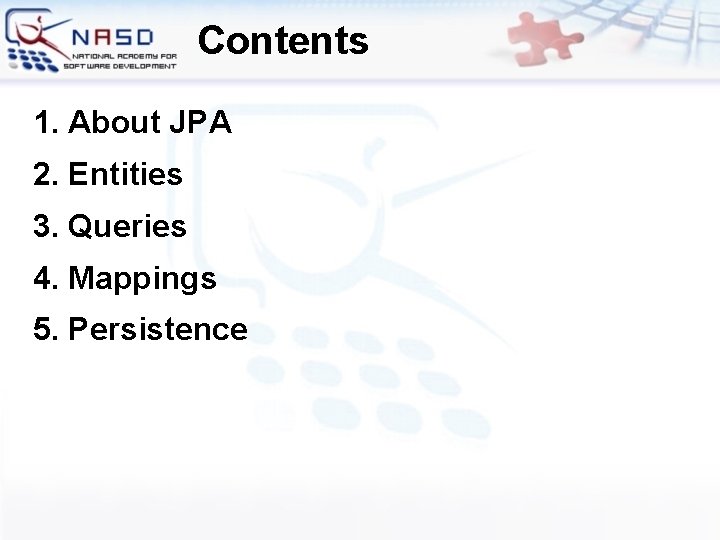
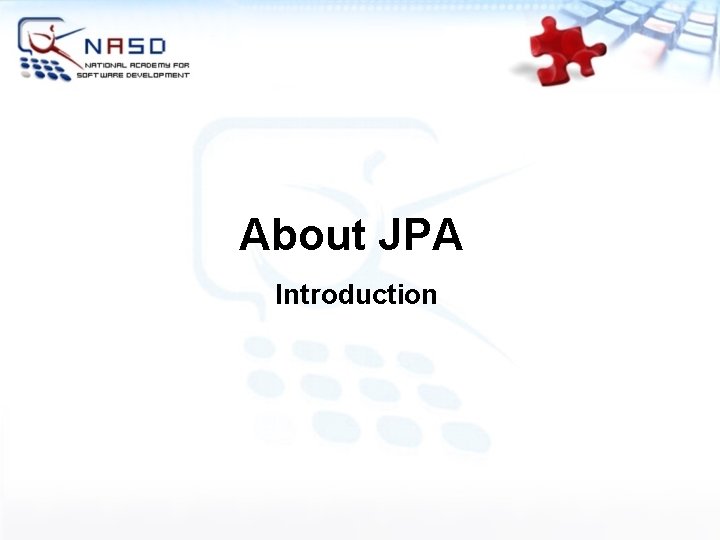
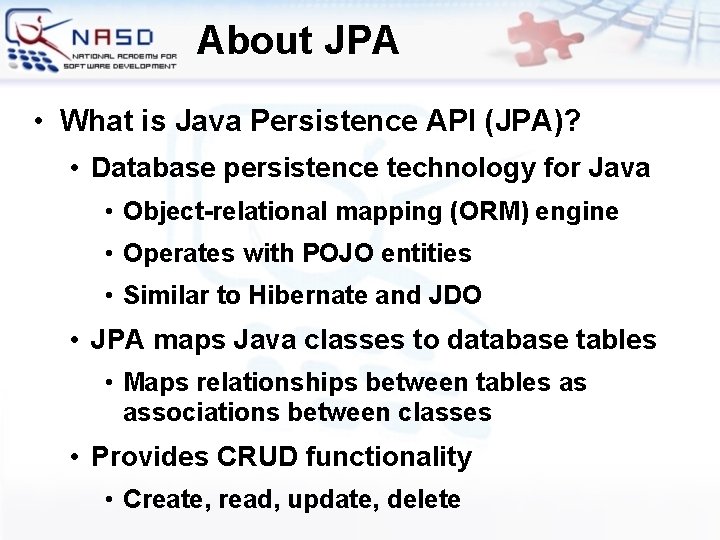
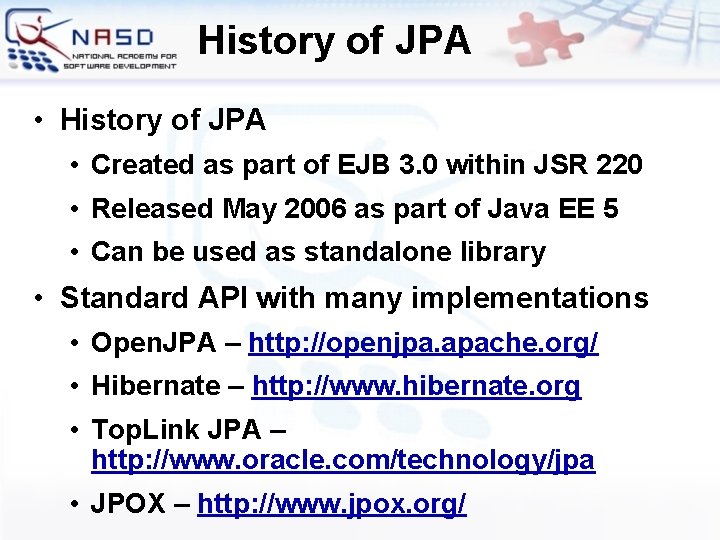
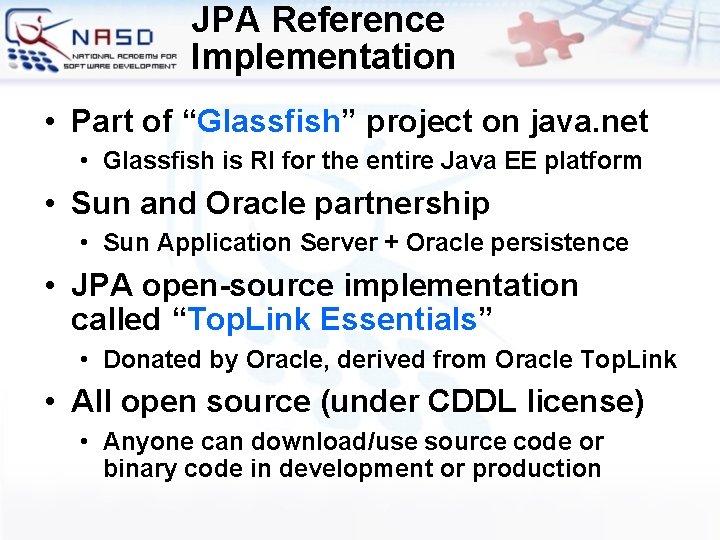
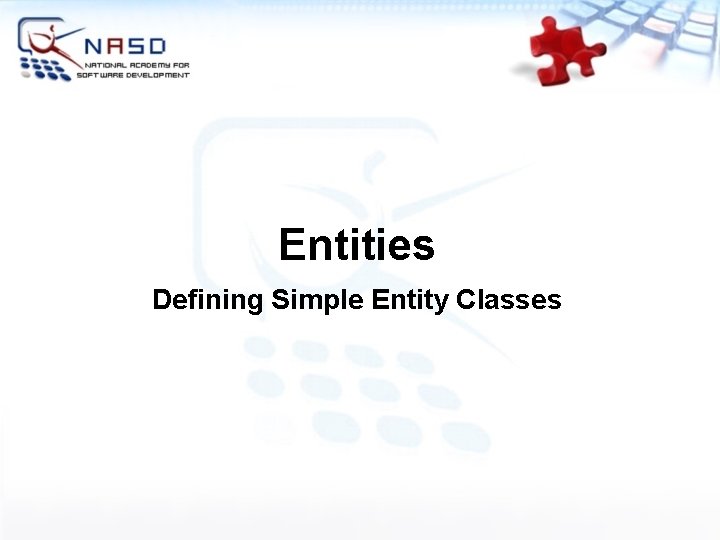
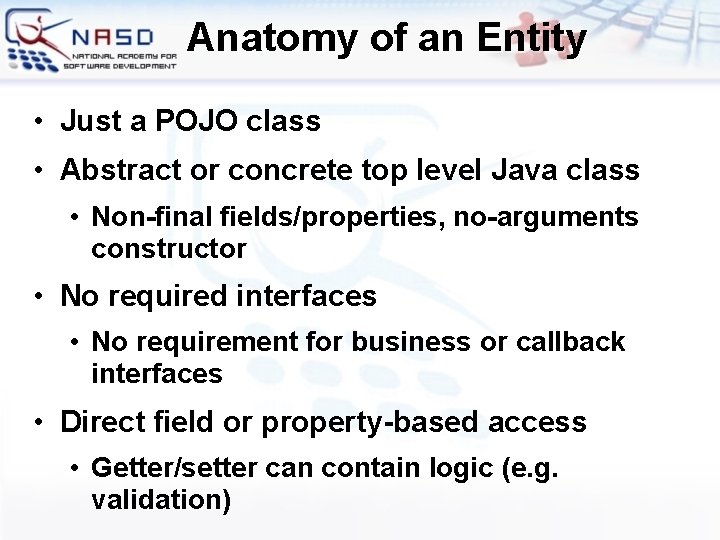
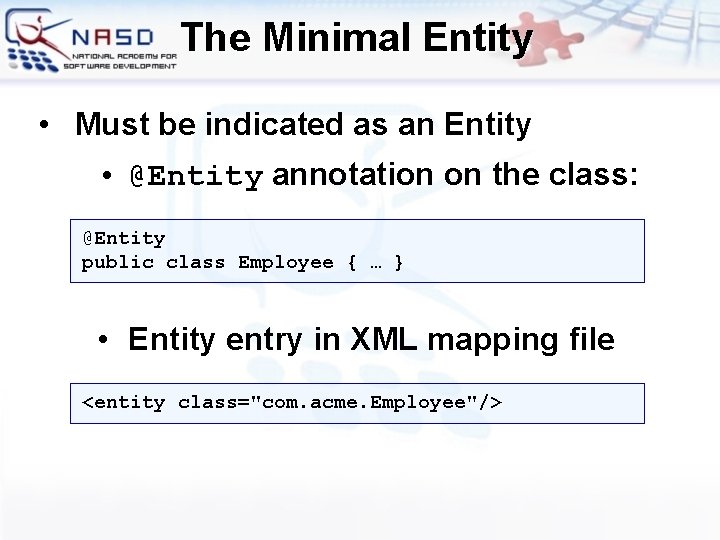
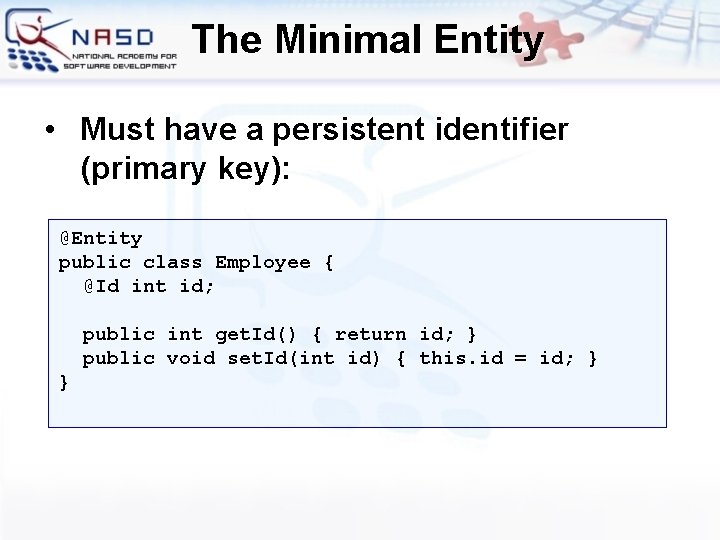
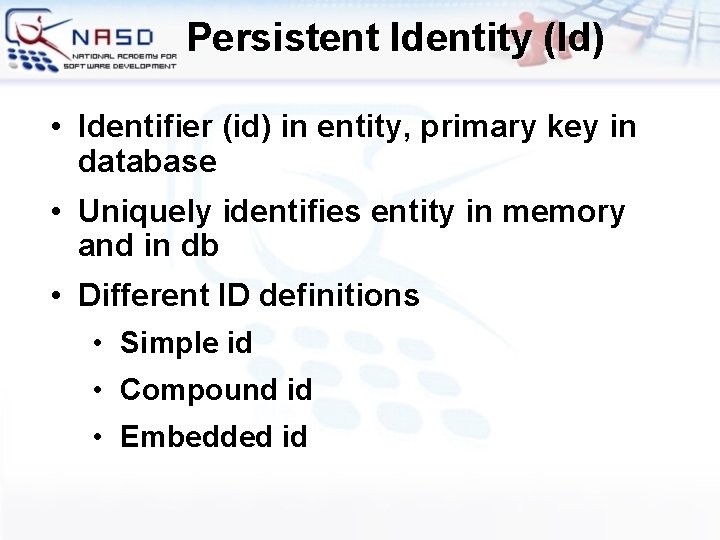
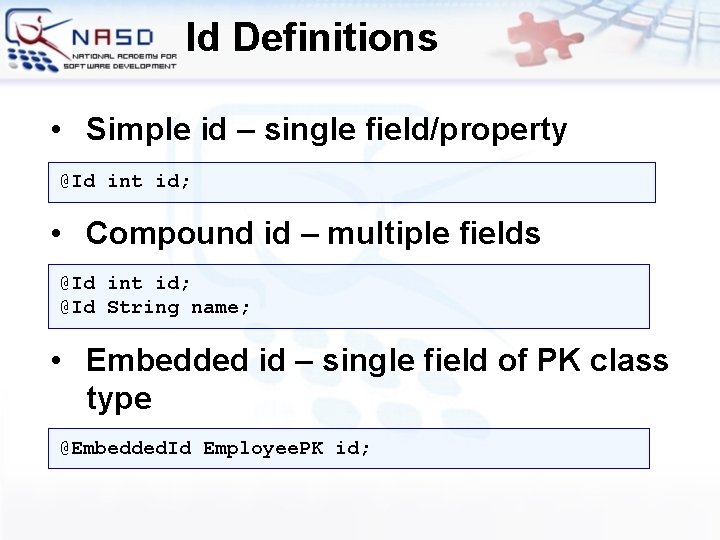
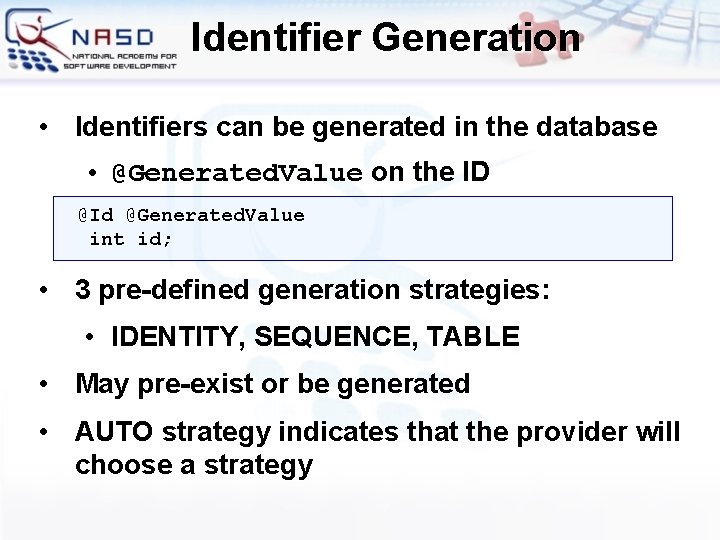
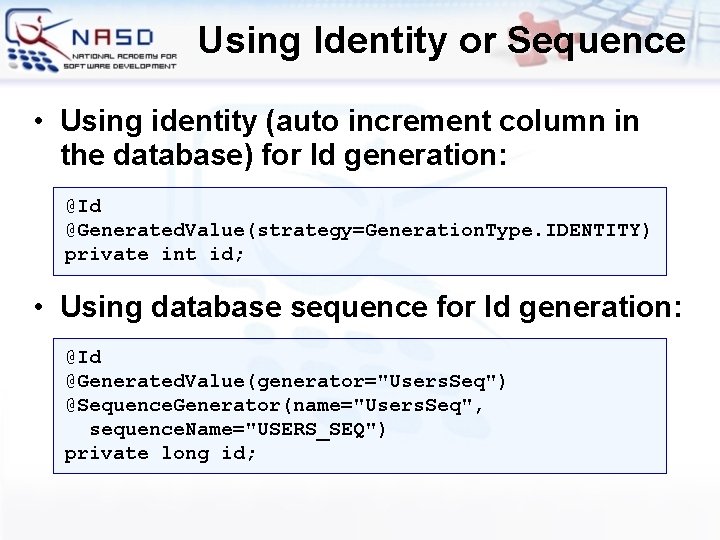
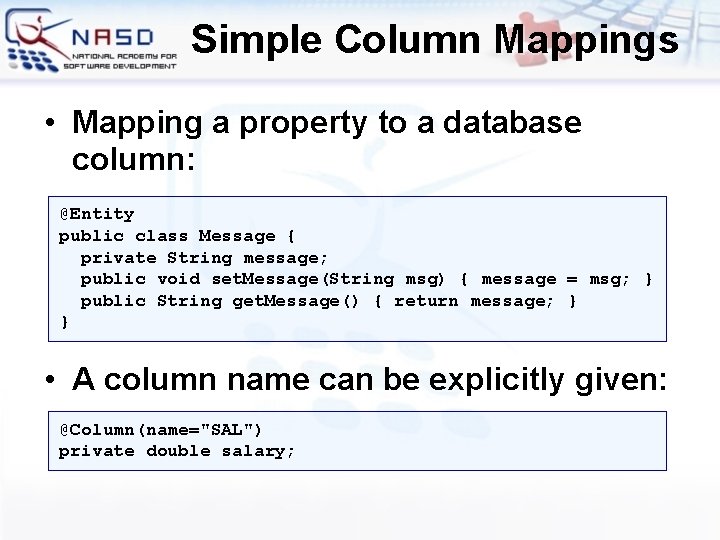
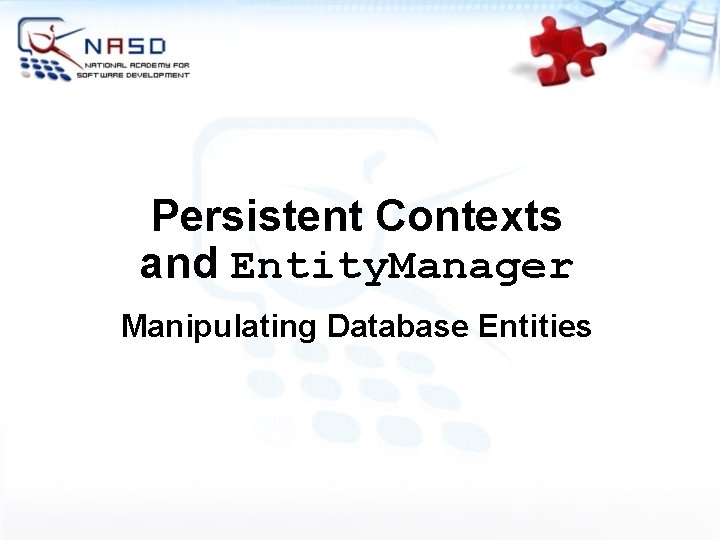
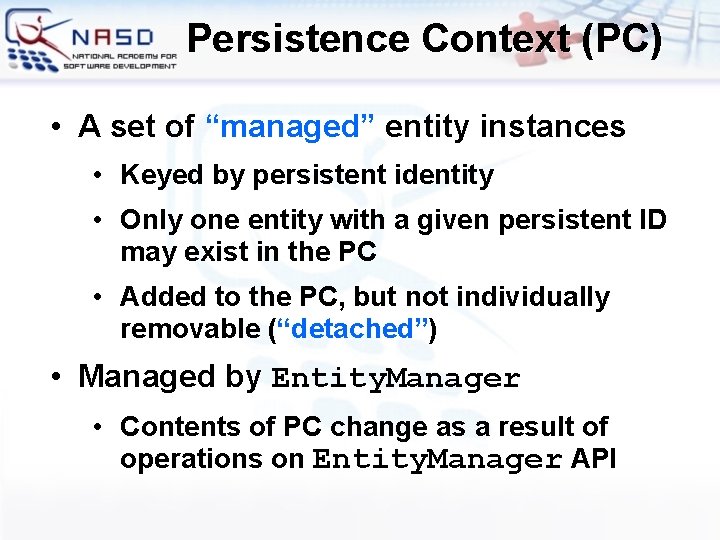
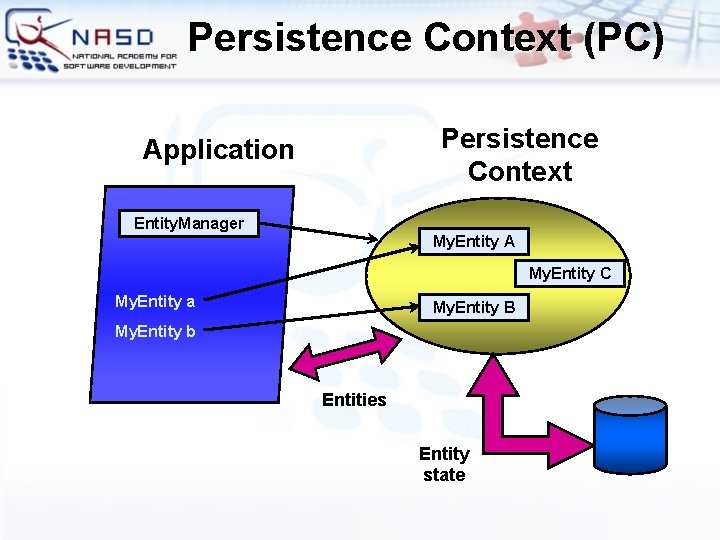
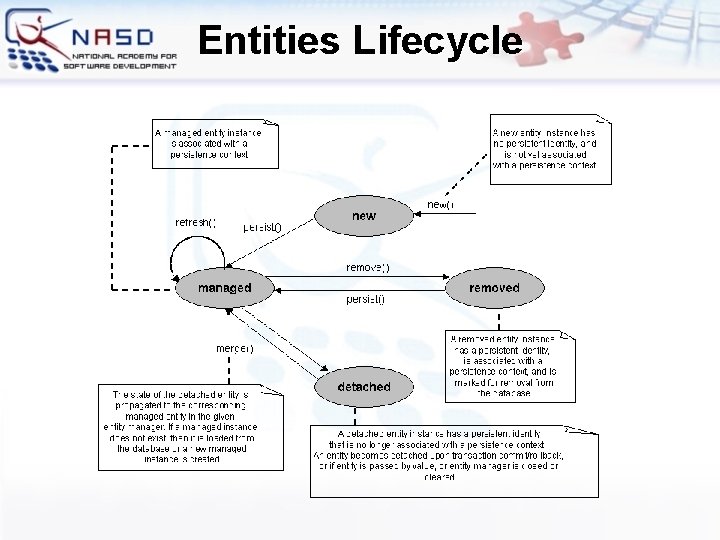
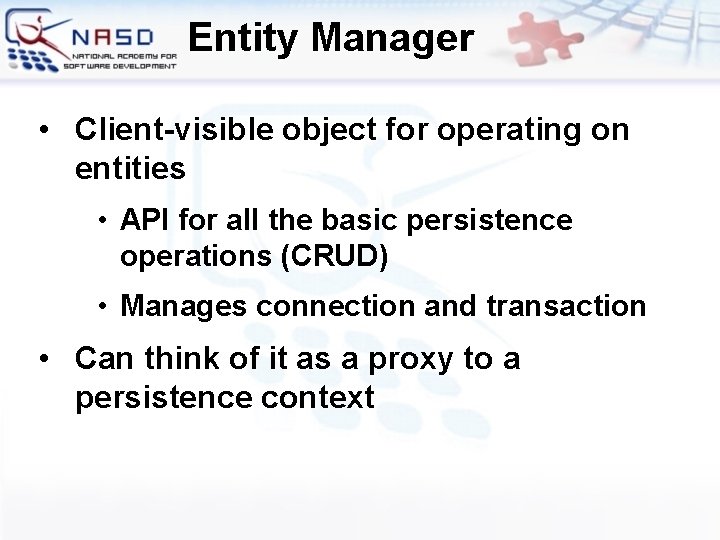
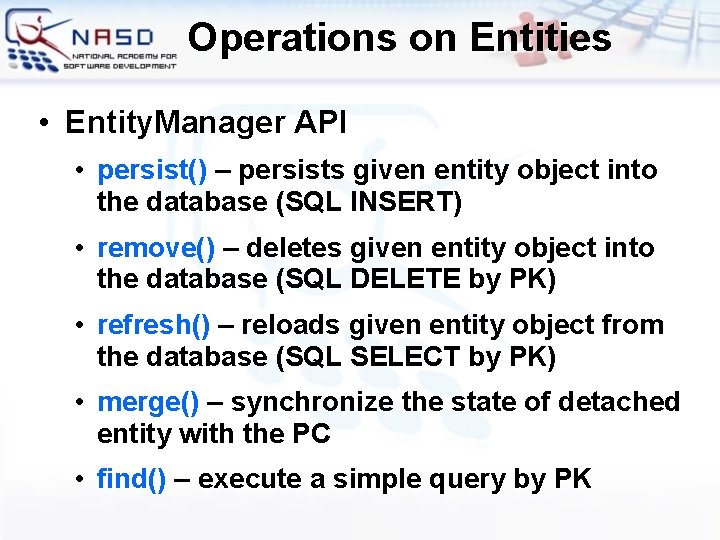
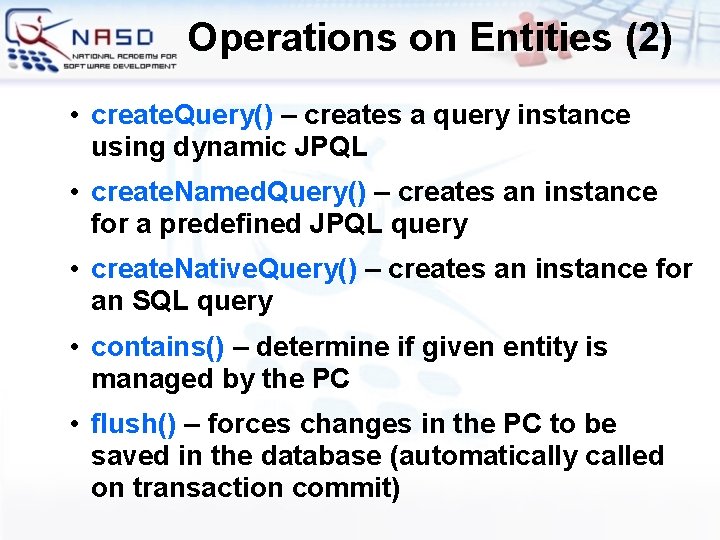
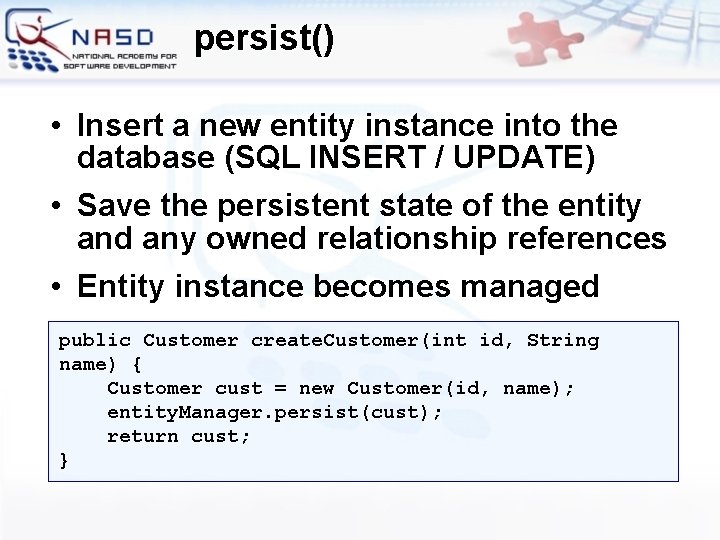
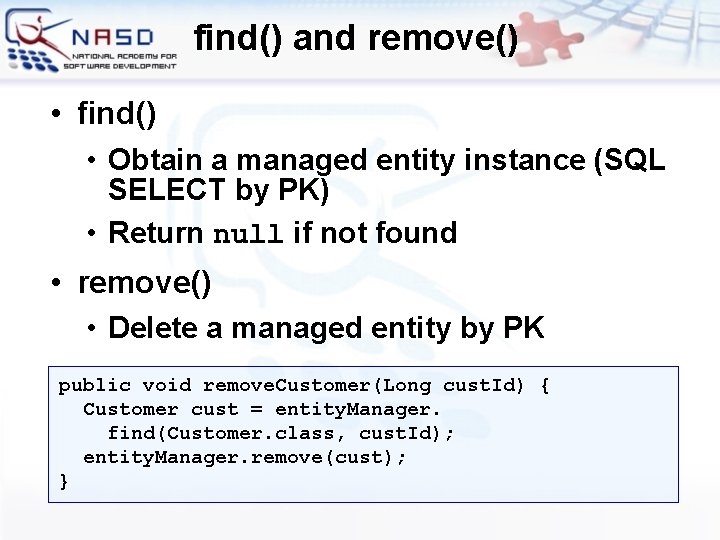
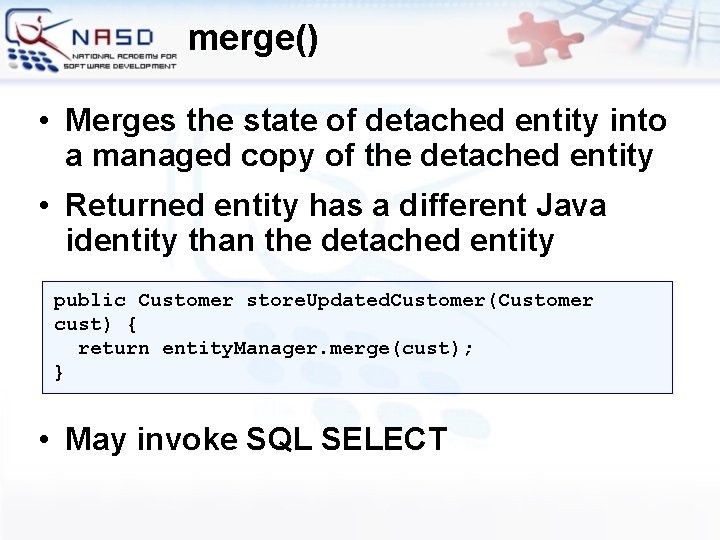
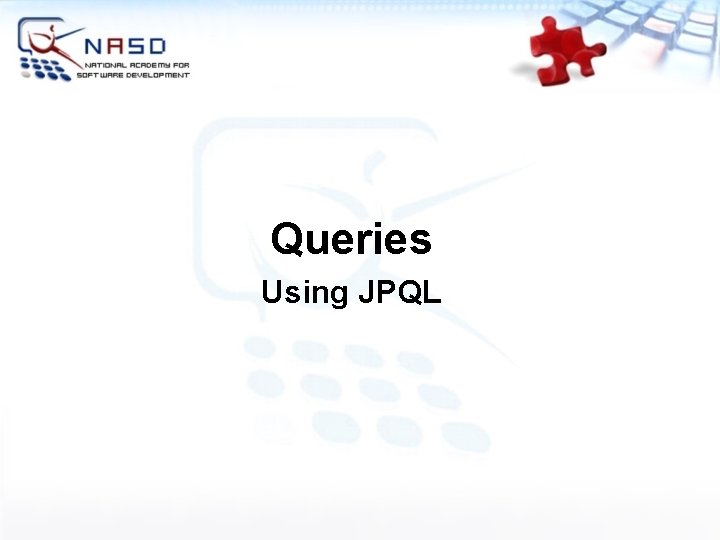
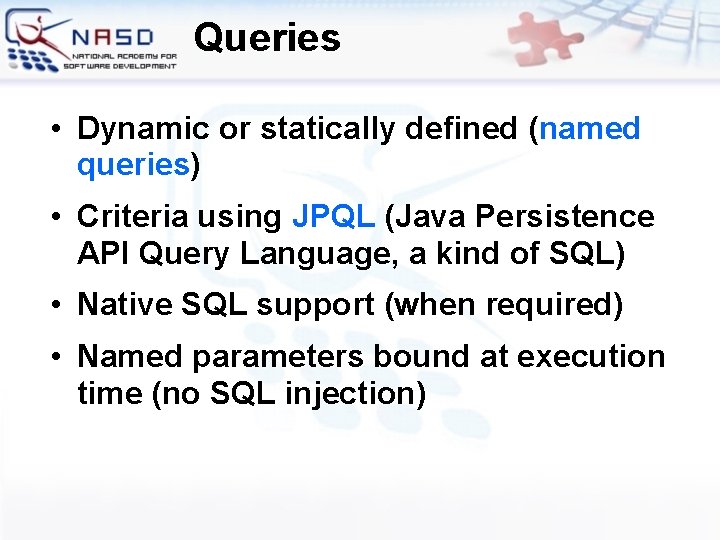
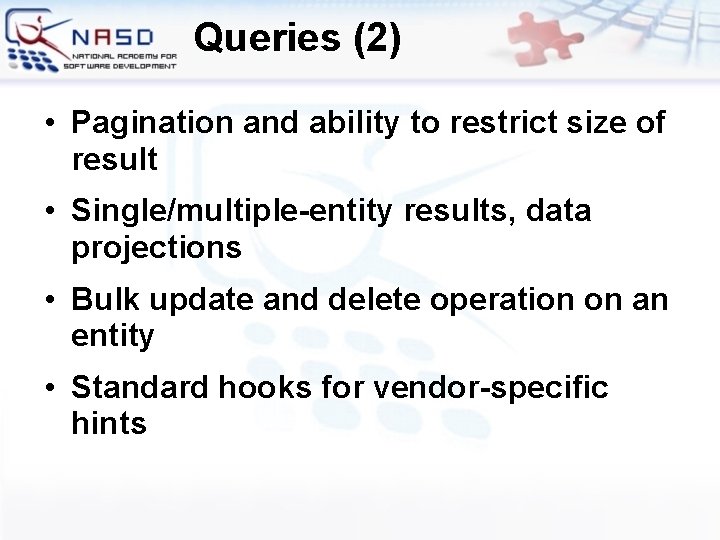
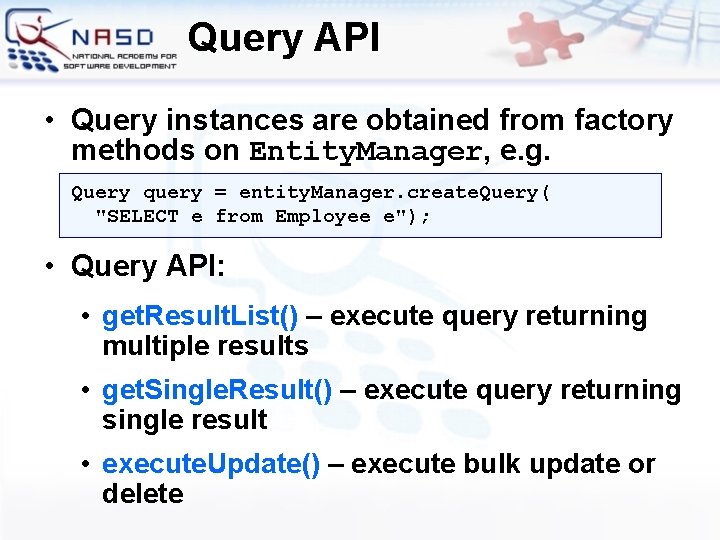
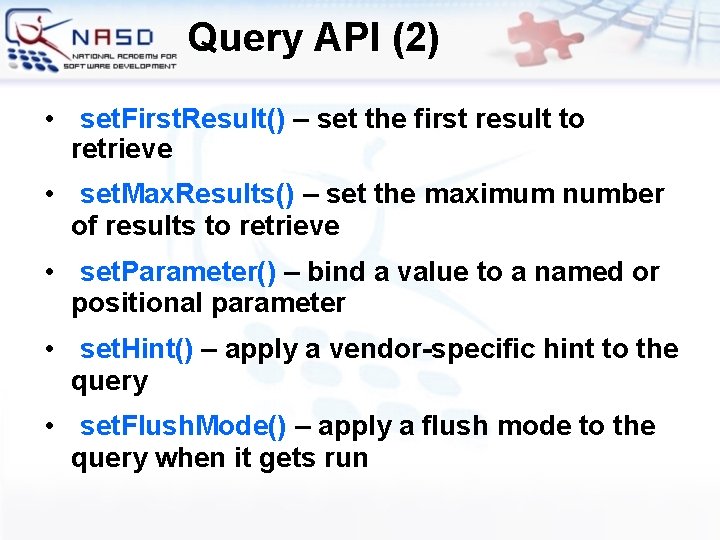
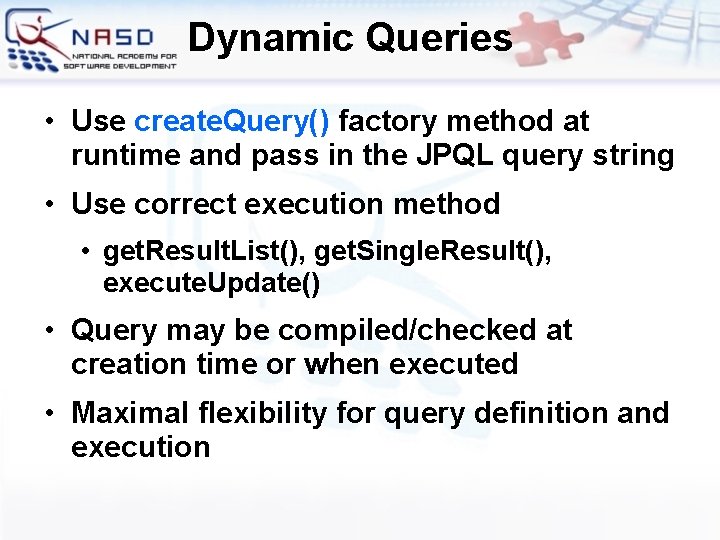
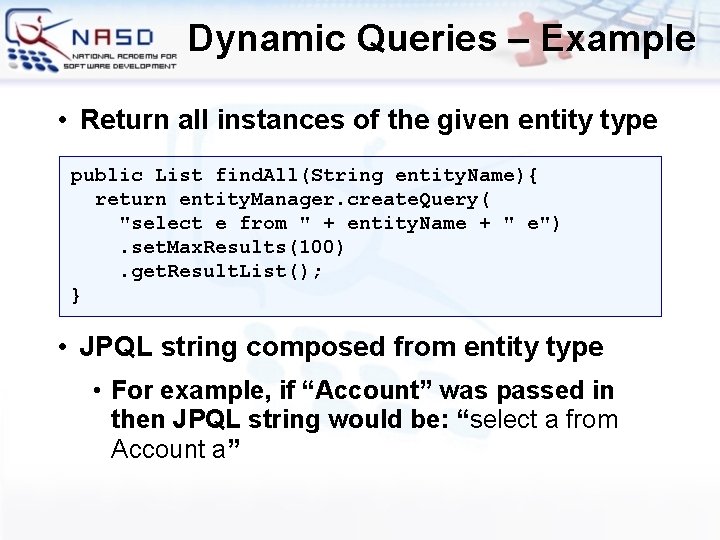
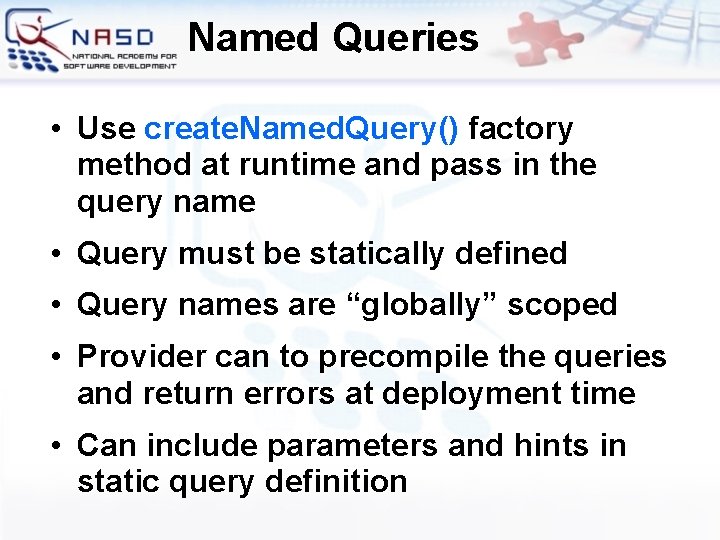
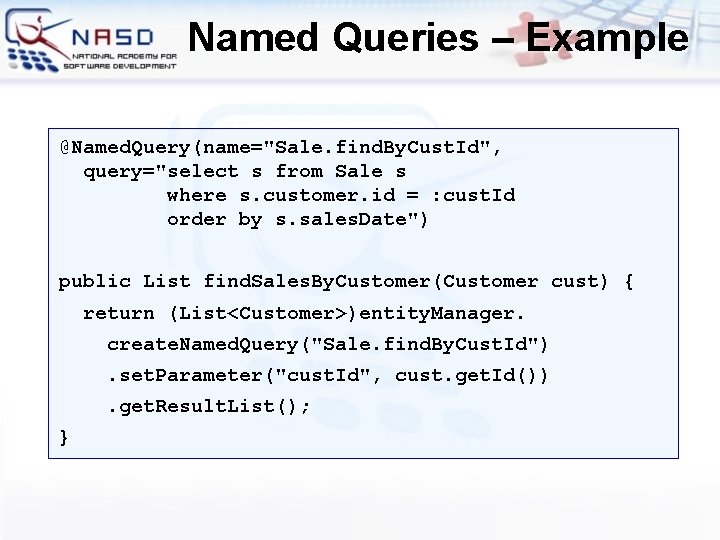
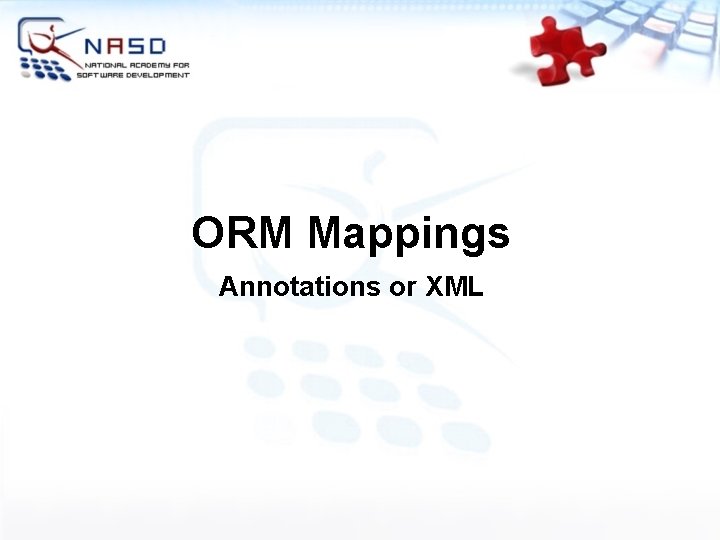
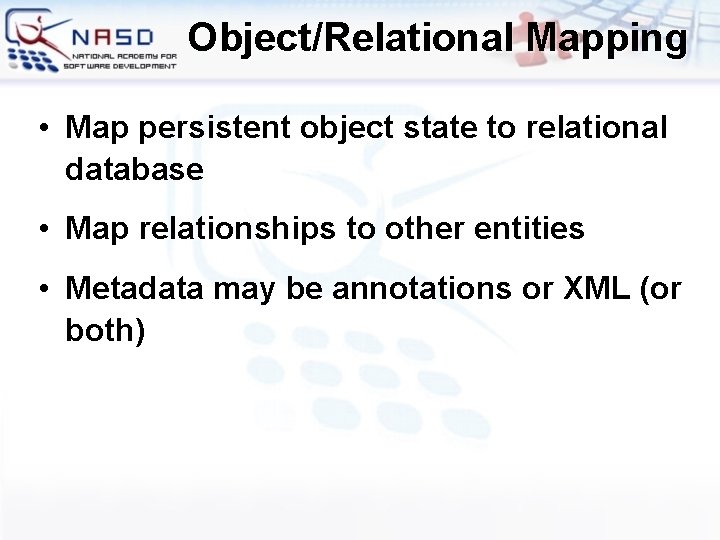
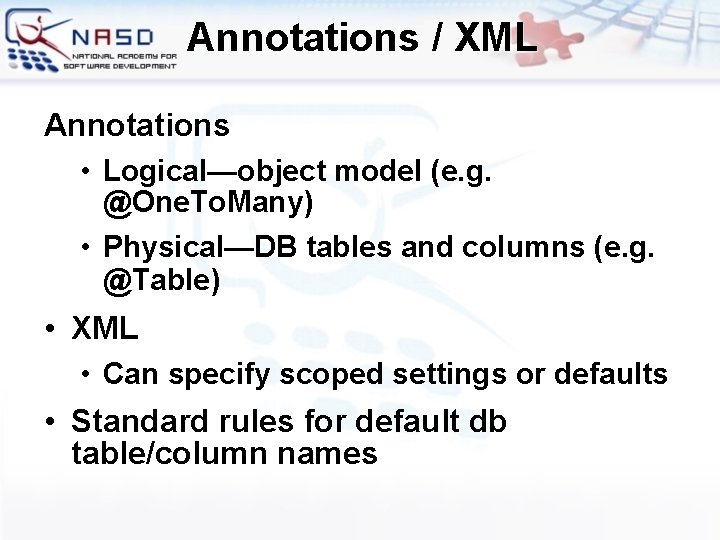
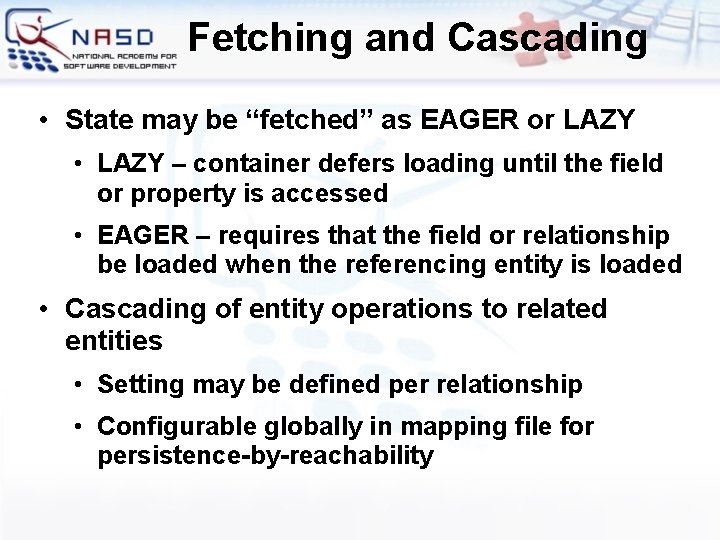
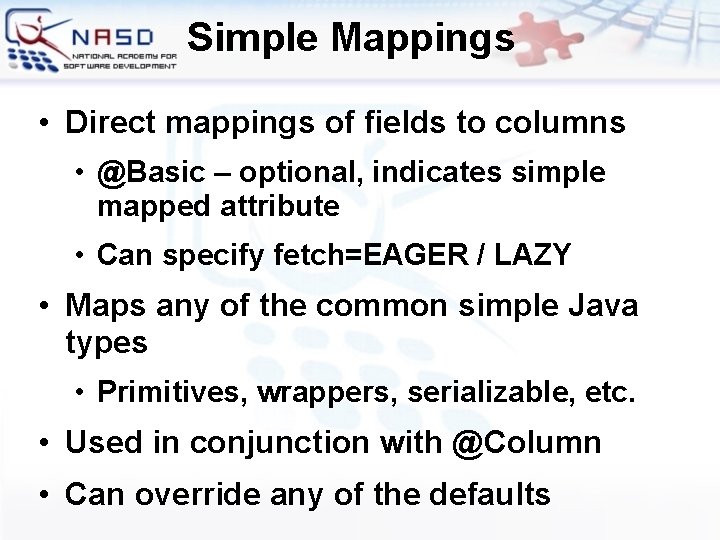
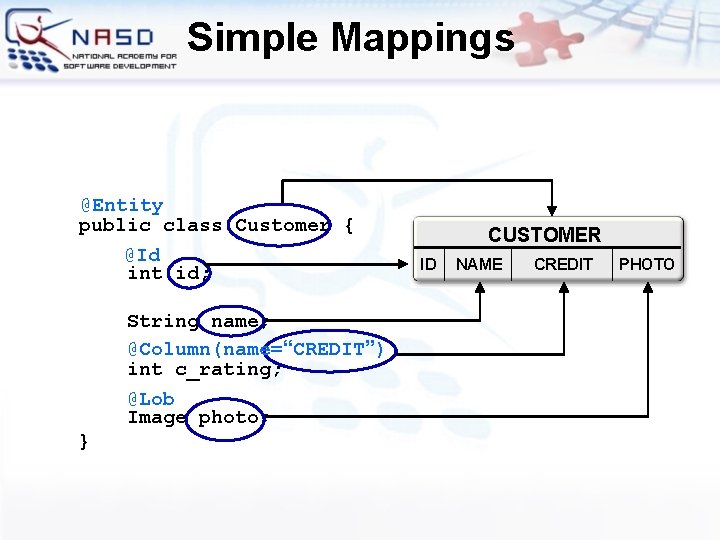
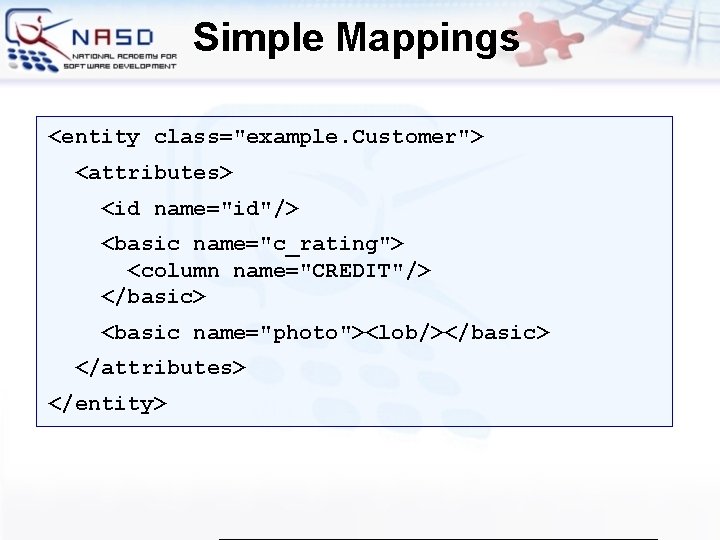
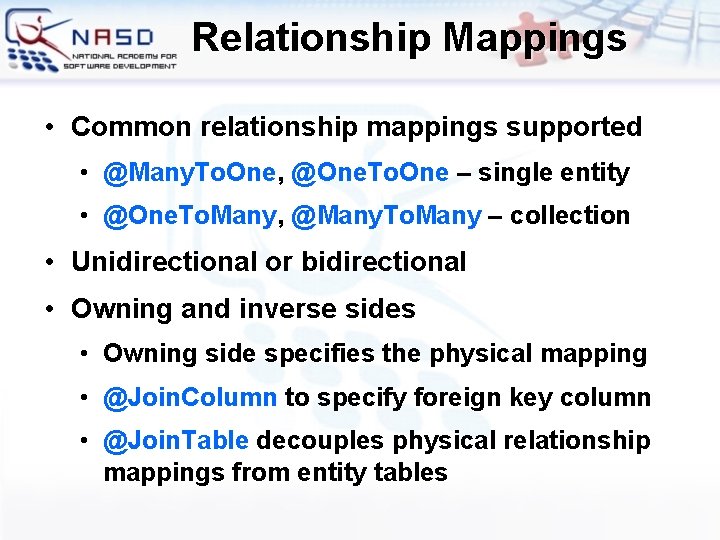
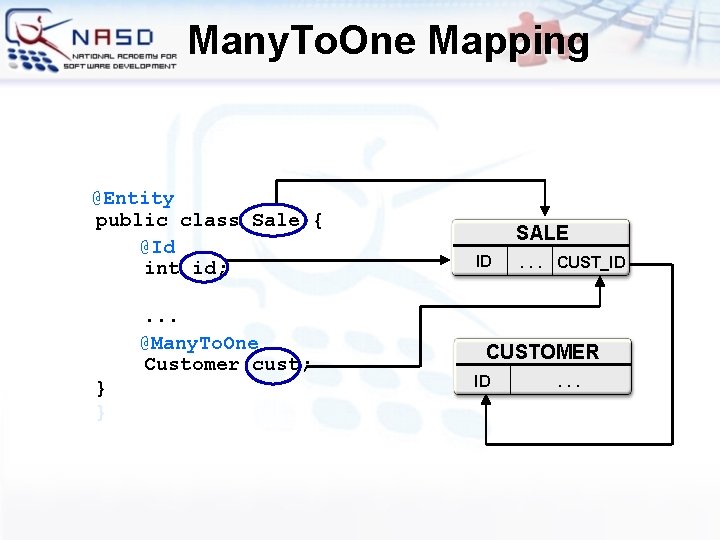
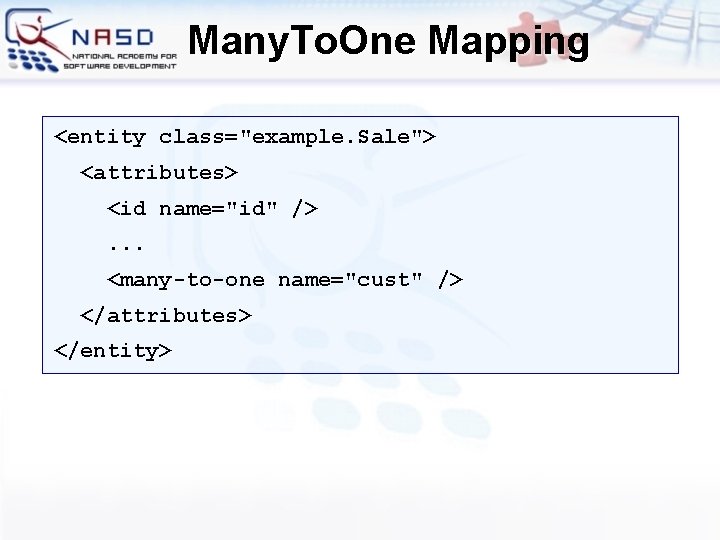
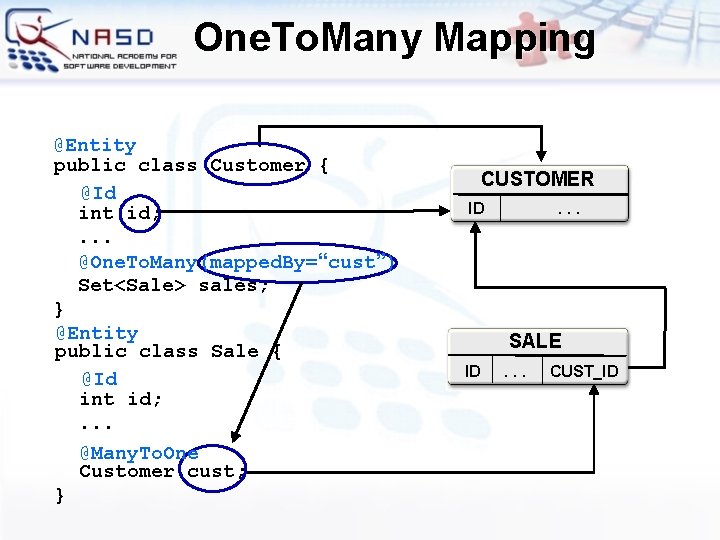
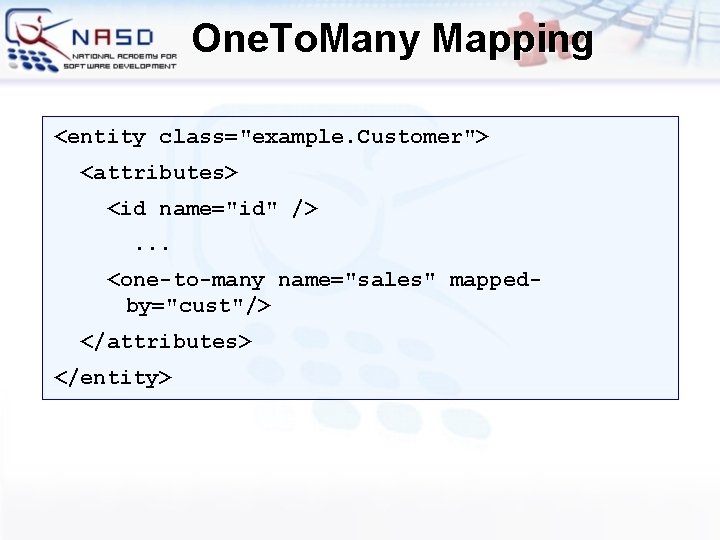
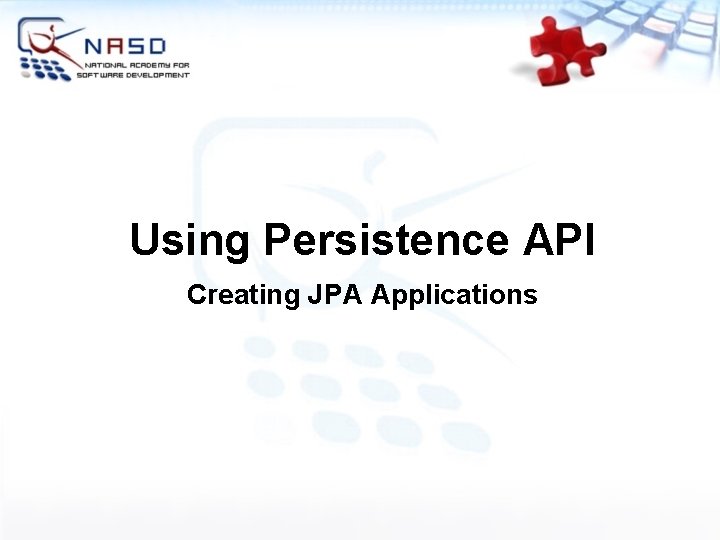
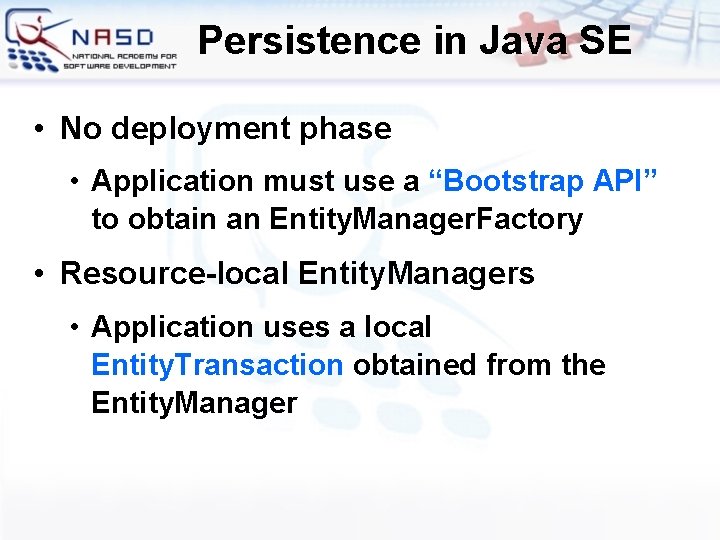
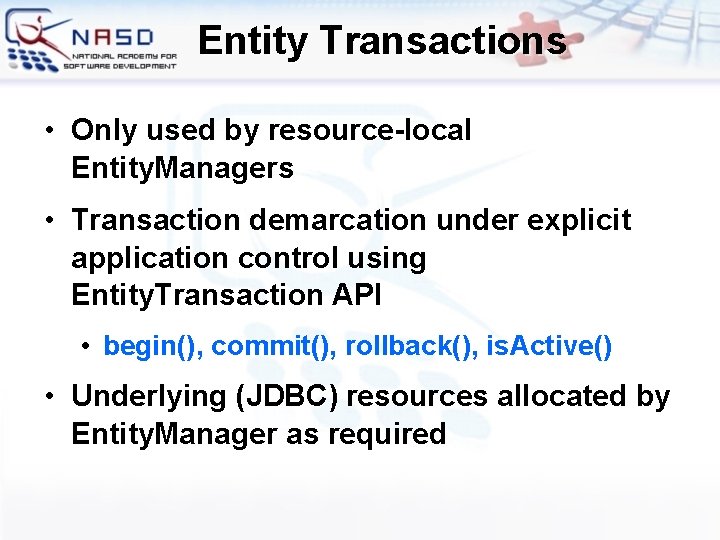
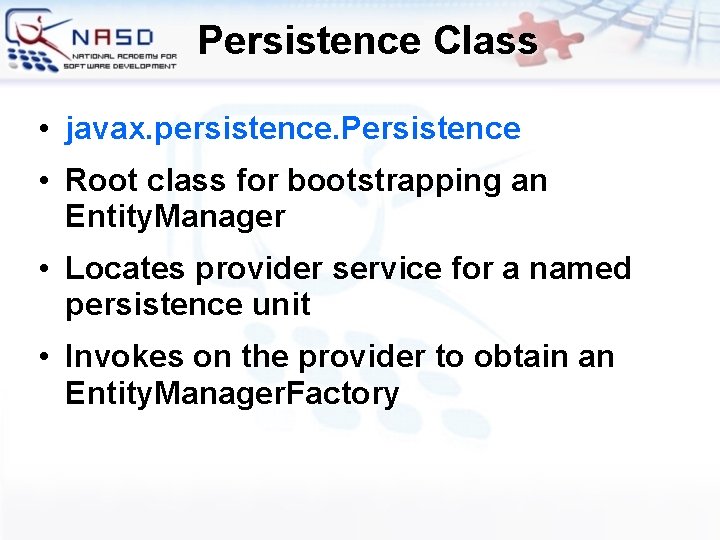
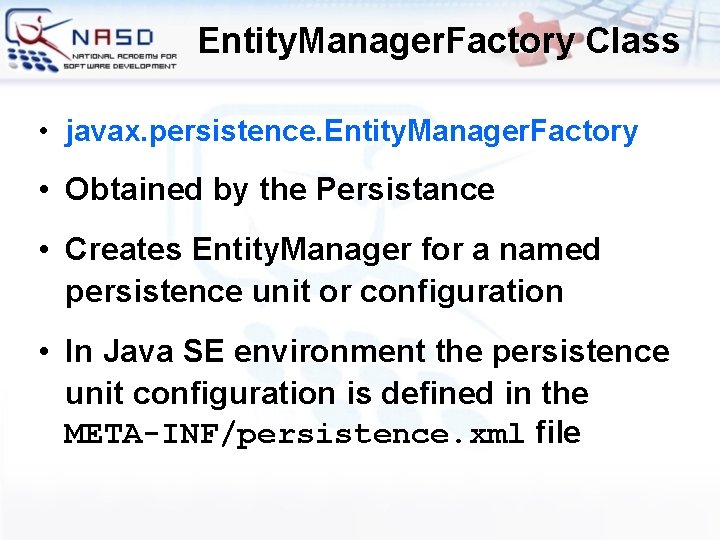
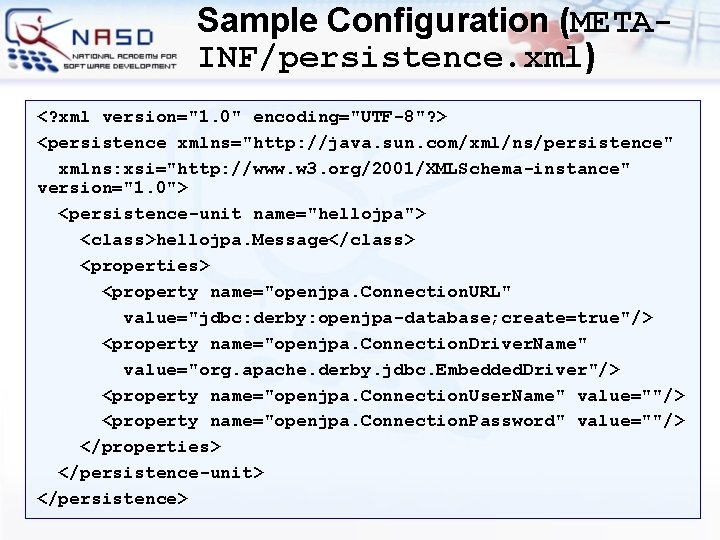
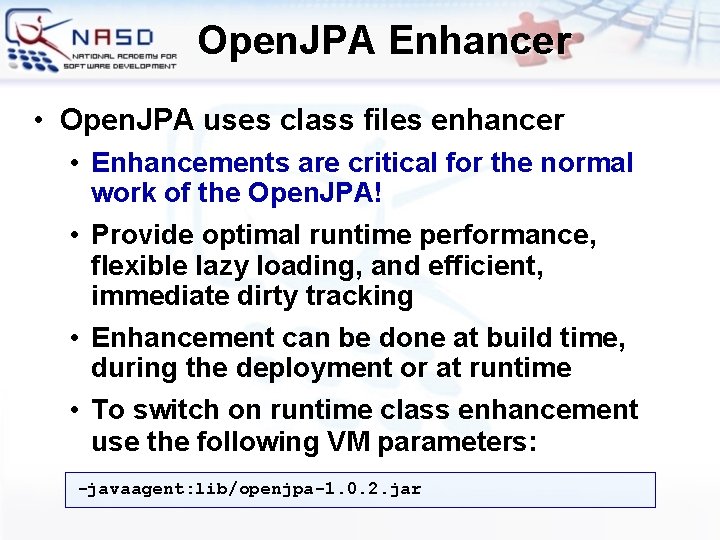
![JPA Bootstrap – Example public class Persistence. Example { public static void main(String[] args) JPA Bootstrap – Example public class Persistence. Example { public static void main(String[] args)](https://slidetodoc.com/presentation_image/da3e0636d2f19fae2ce92b078ad5f6be/image-54.jpg)
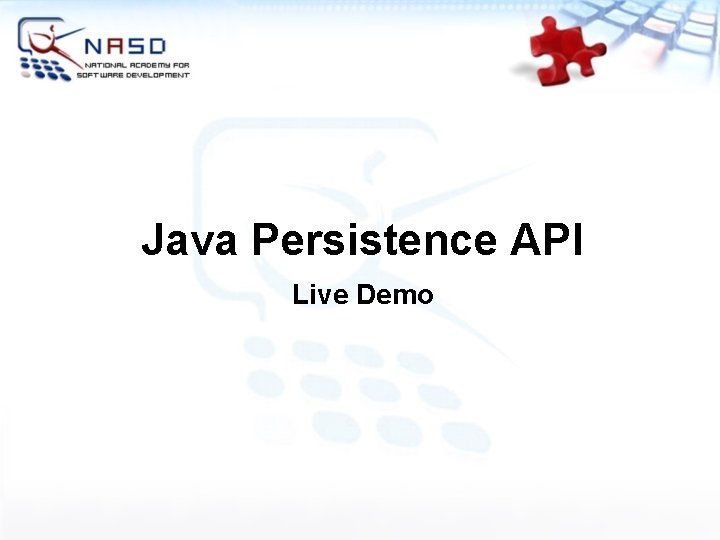
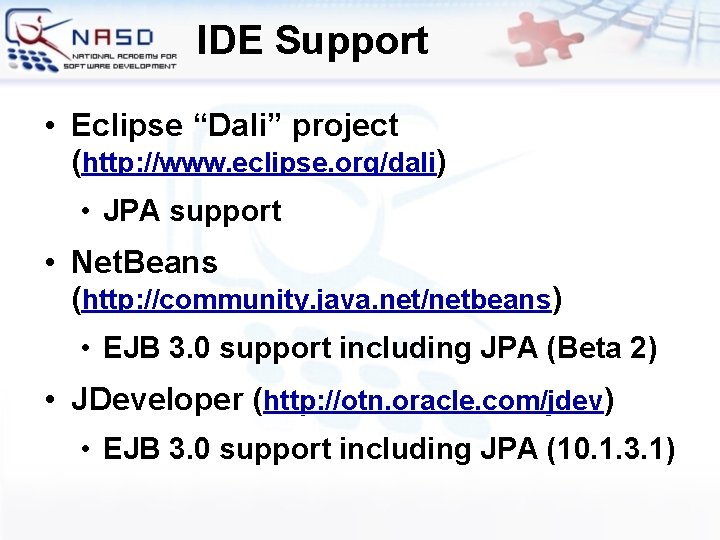
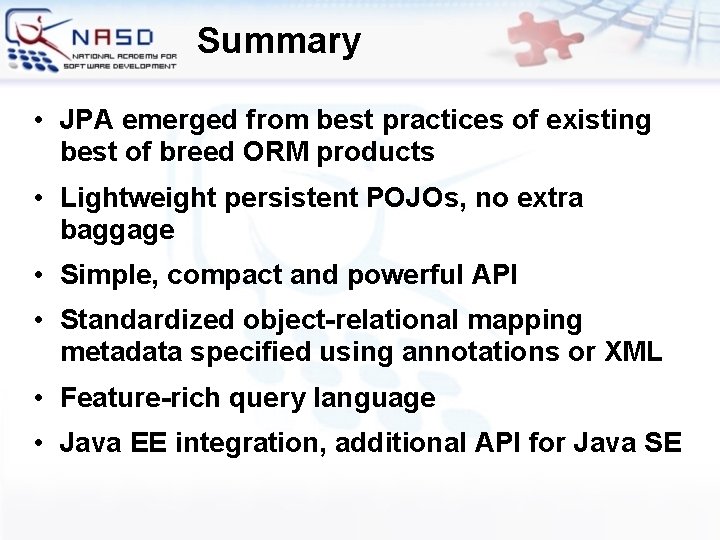
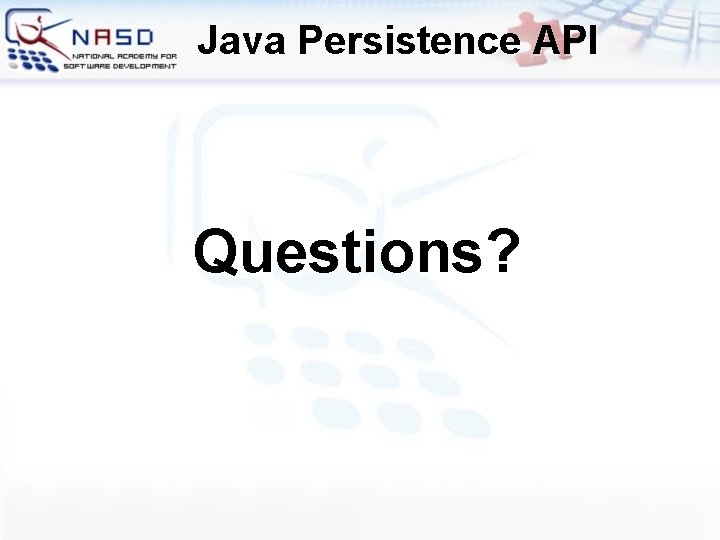
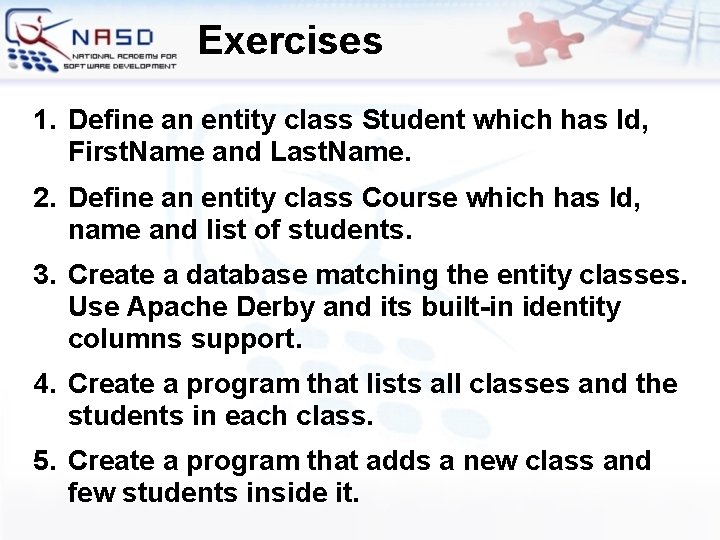
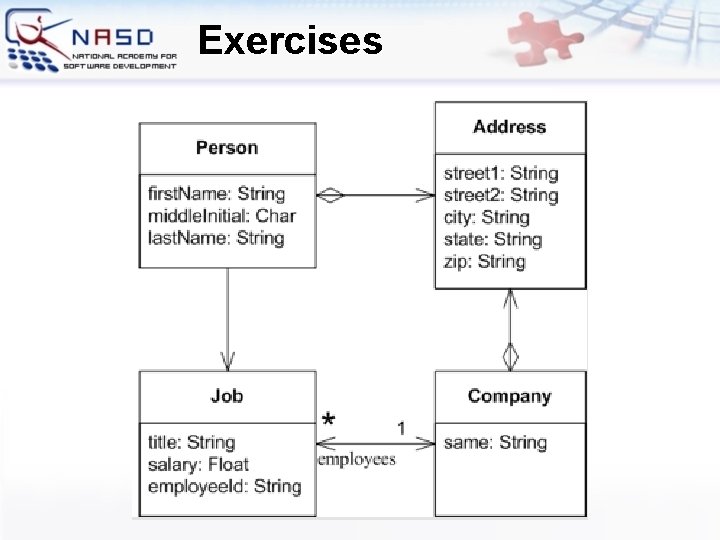
- Slides: 60
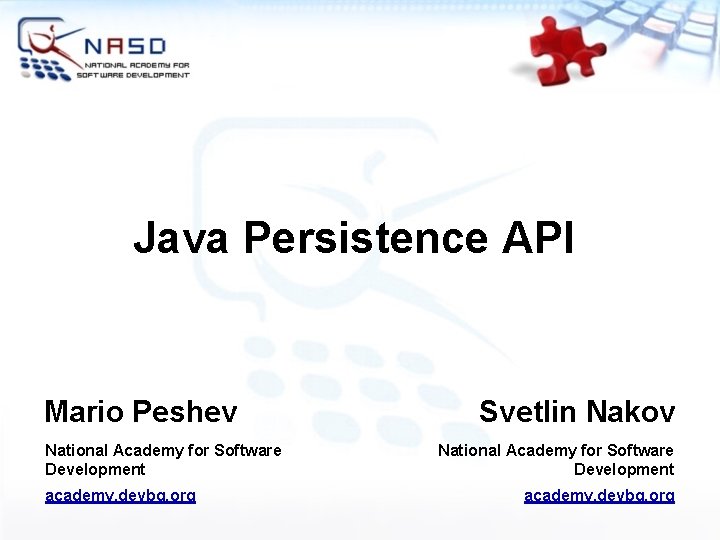
Java Persistence API Mario Peshev National Academy for Software Development academy. devbg. org Svetlin Nakov National Academy for Software Development academy. devbg. org
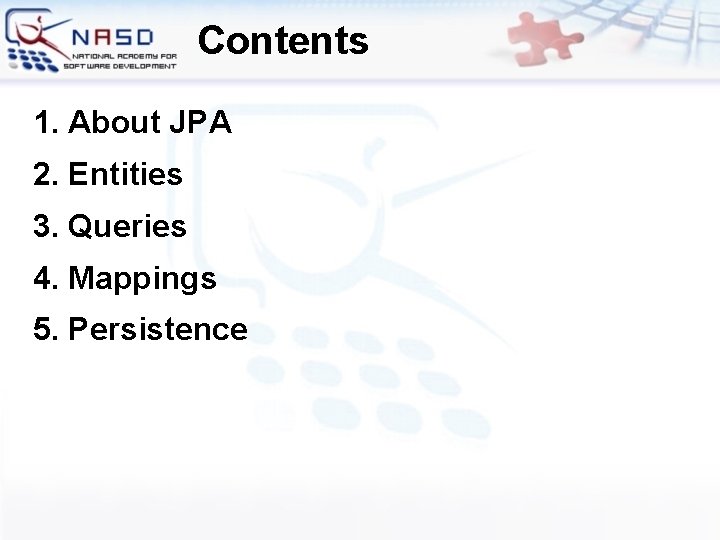
Contents 1. About JPA 2. Entities 3. Queries 4. Mappings 5. Persistence
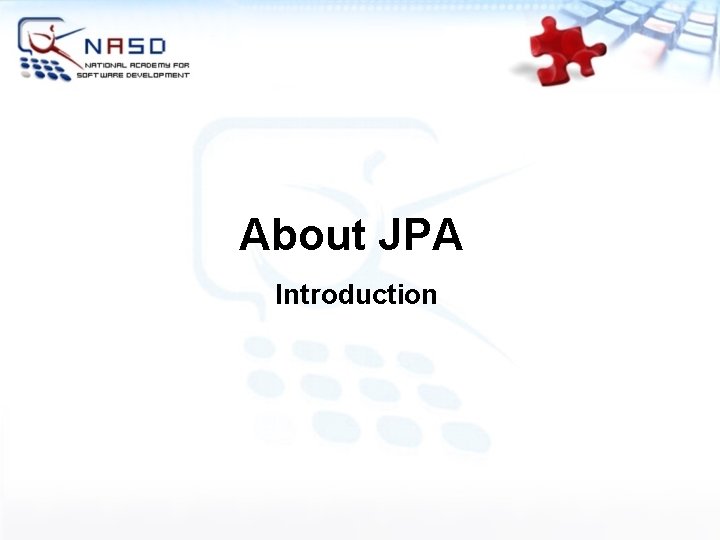
About JPA Introduction
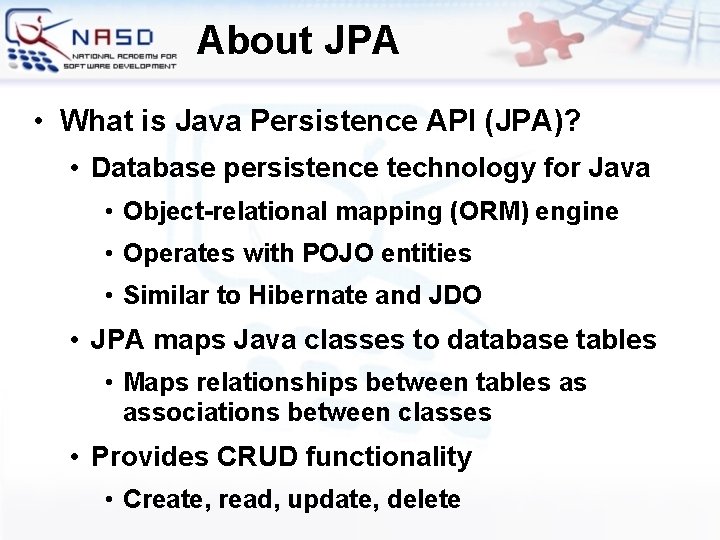
About JPA • What is Java Persistence API (JPA)? • Database persistence technology for Java • Object-relational mapping (ORM) engine • Operates with POJO entities • Similar to Hibernate and JDO • JPA maps Java classes to database tables • Maps relationships between tables as associations between classes • Provides CRUD functionality • Create, read, update, delete
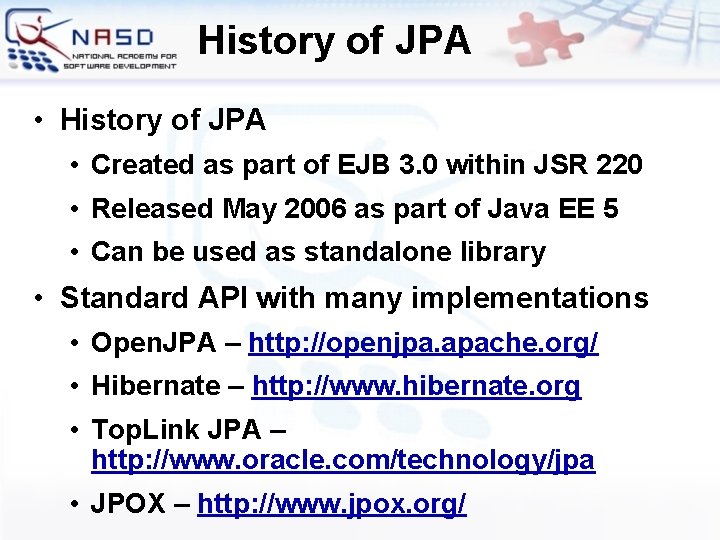
History of JPA • Created as part of EJB 3. 0 within JSR 220 • Released May 2006 as part of Java EE 5 • Can be used as standalone library • Standard API with many implementations • Open. JPA – http: //openjpa. apache. org/ • Hibernate – http: //www. hibernate. org • Top. Link JPA – http: //www. oracle. com/technology/jpa • JPOX – http: //www. jpox. org/
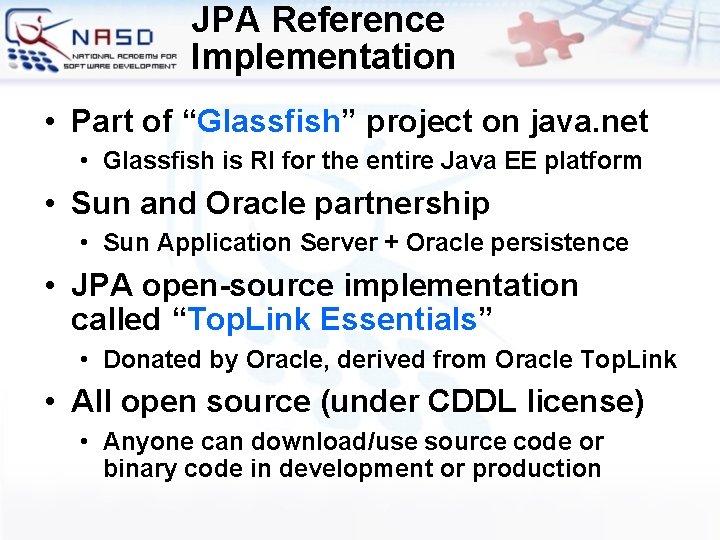
JPA Reference Implementation • Part of “Glassfish” project on java. net • Glassfish is RI for the entire Java EE platform • Sun and Oracle partnership • Sun Application Server + Oracle persistence • JPA open-source implementation called “Top. Link Essentials” • Donated by Oracle, derived from Oracle Top. Link • All open source (under CDDL license) • Anyone can download/use source code or binary code in development or production
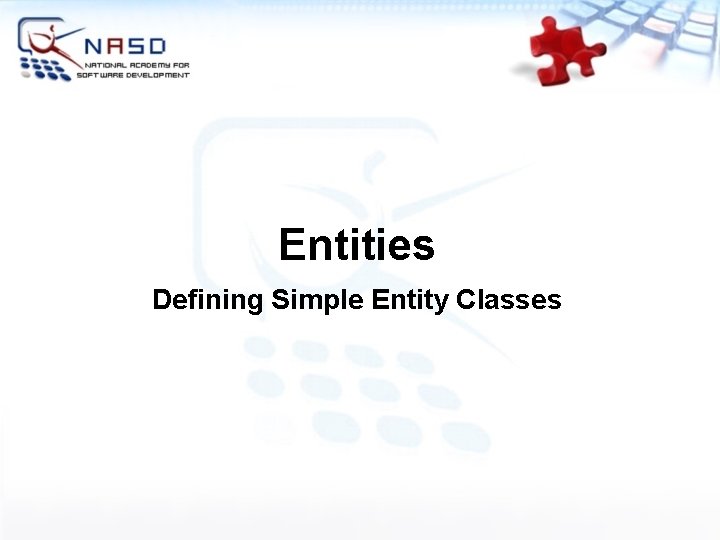
Entities Defining Simple Entity Classes
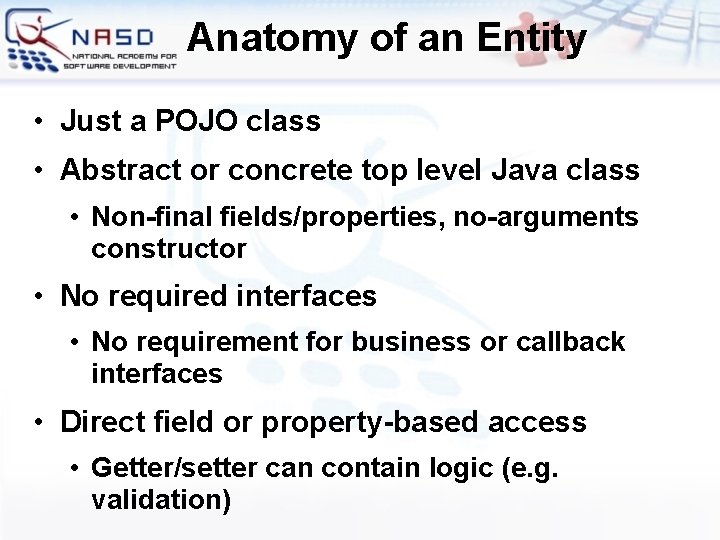
Anatomy of an Entity • Just a POJO class • Abstract or concrete top level Java class • Non-final fields/properties, no-arguments constructor • No required interfaces • No requirement for business or callback interfaces • Direct field or property-based access • Getter/setter can contain logic (e. g. validation)
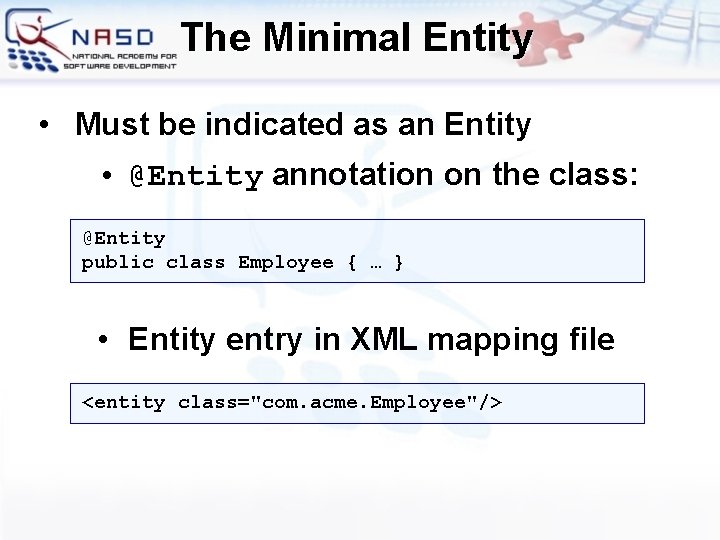
The Minimal Entity • Must be indicated as an Entity • @Entity annotation on the class: @Entity public class Employee { … } • Entity entry in XML mapping file <entity class="com. acme. Employee"/>
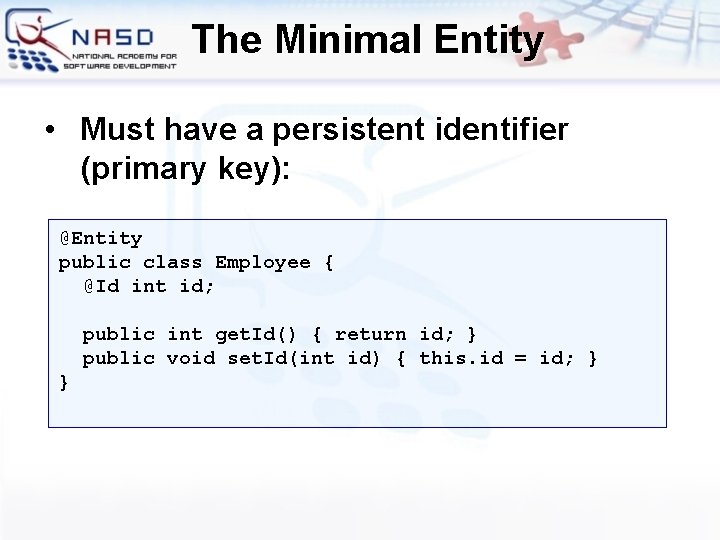
The Minimal Entity • Must have a persistent identifier (primary key): @Entity public class Employee { @Id int id; public } int get. Id() { return void set. Id(int id) { id; } this. id = id; }
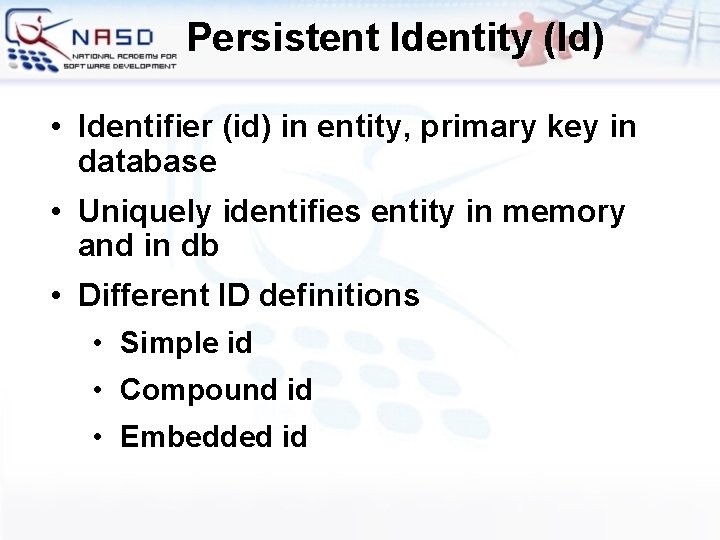
Persistent Identity (Id) • Identifier (id) in entity, primary key in database • Uniquely identifies entity in memory and in db • Different ID definitions • Simple id • Compound id • Embedded id
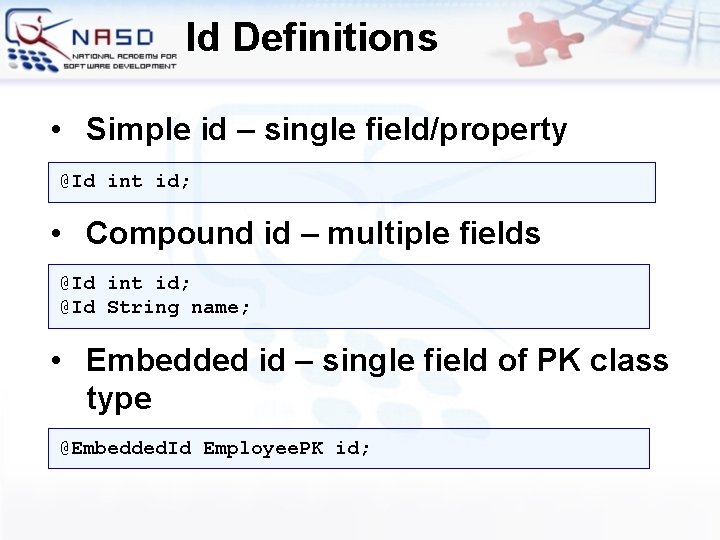
Id Definitions • Simple id – single field/property @Id int id; • Compound id – multiple fields @Id int id; @Id String name; • Embedded id – single field of PK class type @Embedded. Id Employee. PK id;
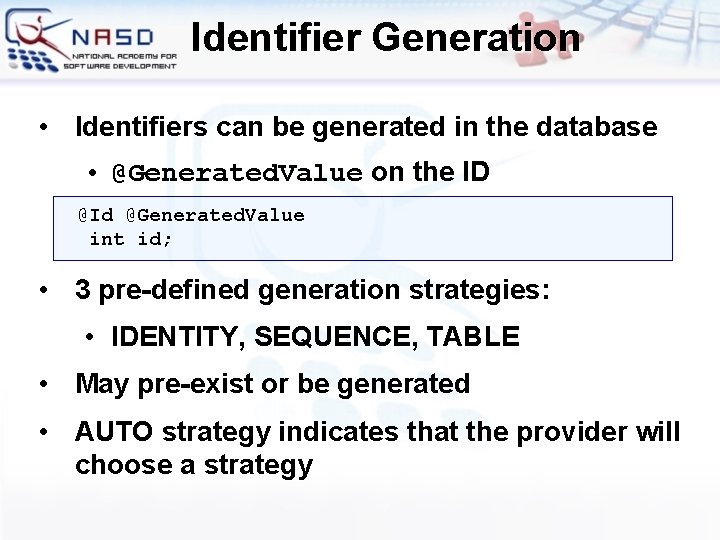
Identifier Generation • Identifiers can be generated in the database • @Generated. Value on the ID @Id @Generated. Value int id; • 3 pre-defined generation strategies: • IDENTITY, SEQUENCE, TABLE • May pre-exist or be generated • AUTO strategy indicates that the provider will choose a strategy
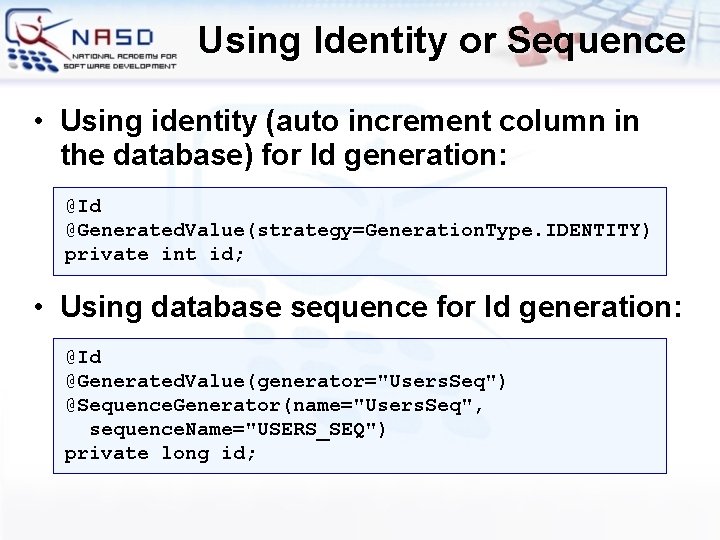
Using Identity or Sequence • Using identity (auto increment column in the database) for Id generation: @Id @Generated. Value(strategy=Generation. Type. IDENTITY) private int id; • Using database sequence for Id generation: @Id @Generated. Value(generator="Users. Seq") @Sequence. Generator(name="Users. Seq", sequence. Name="USERS_SEQ") private long id;
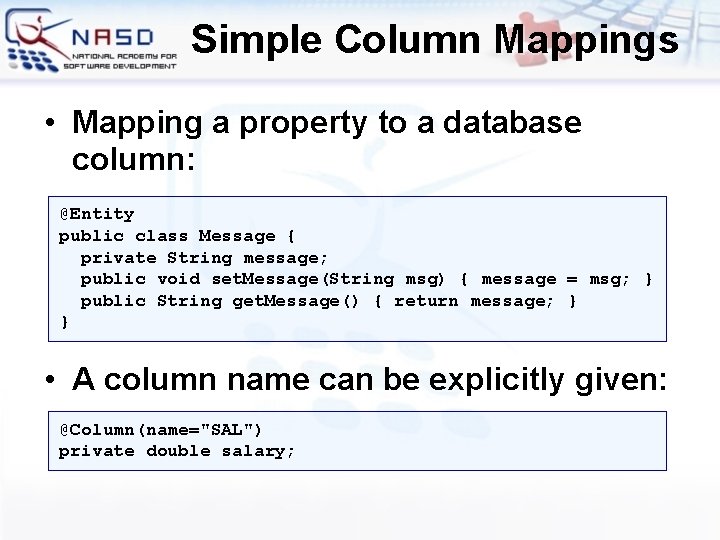
Simple Column Mappings • Mapping a property to a database column: @Entity public class Message { private String message; public void set. Message(String msg) { message = msg; } public String get. Message() { return message; } } • A column name can be explicitly given: @Column(name="SAL") private double salary;
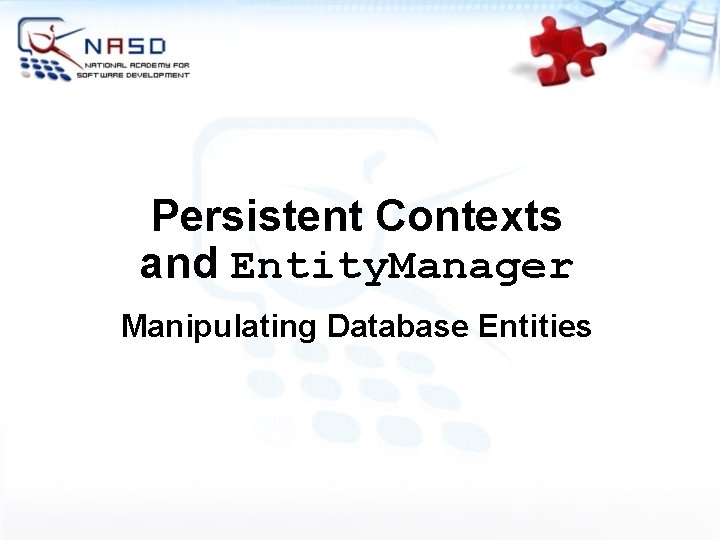
Persistent Contexts and Entity. Manager Manipulating Database Entities
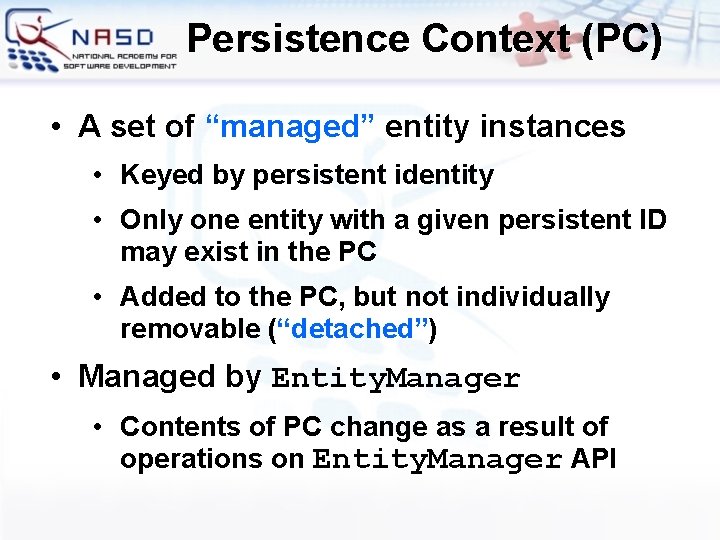
Persistence Context (PC) • A set of “managed” entity instances • Keyed by persistent identity • Only one entity with a given persistent ID may exist in the PC • Added to the PC, but not individually removable (“detached”) • Managed by Entity. Manager • Contents of PC change as a result of operations on Entity. Manager API
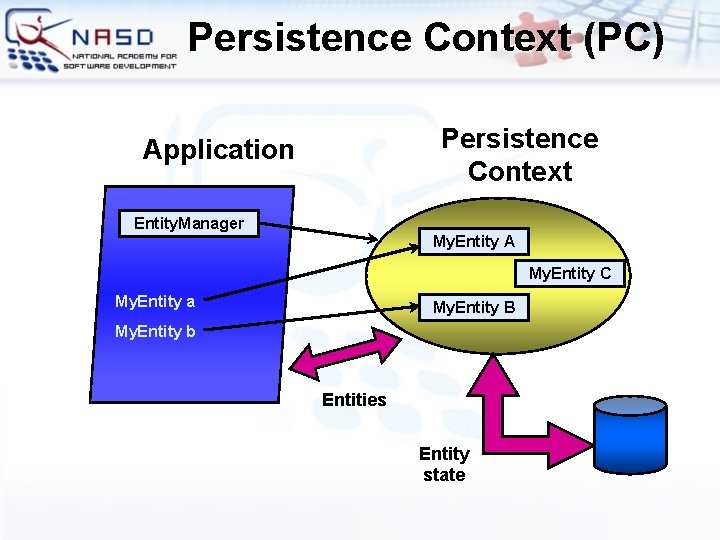
Persistence Context (PC) Persistence Context Application Entity. Manager My. Entity A My. Entity C My. Entity a My. Entity B My. Entity b Entities Entity state
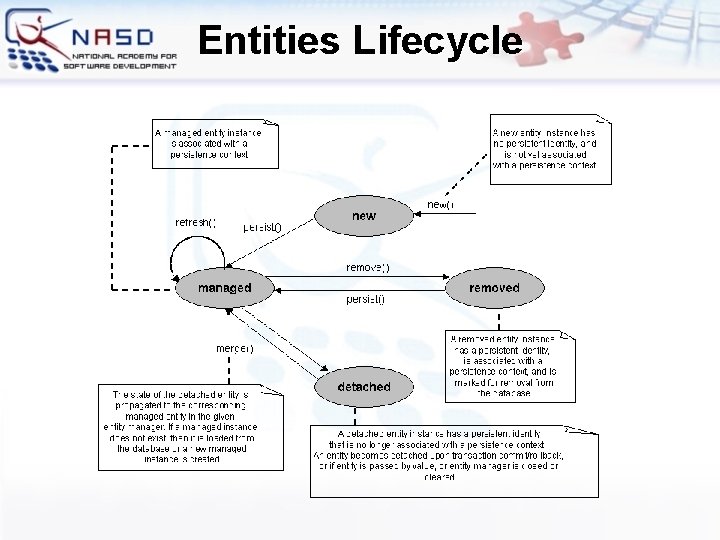
Entities Lifecycle
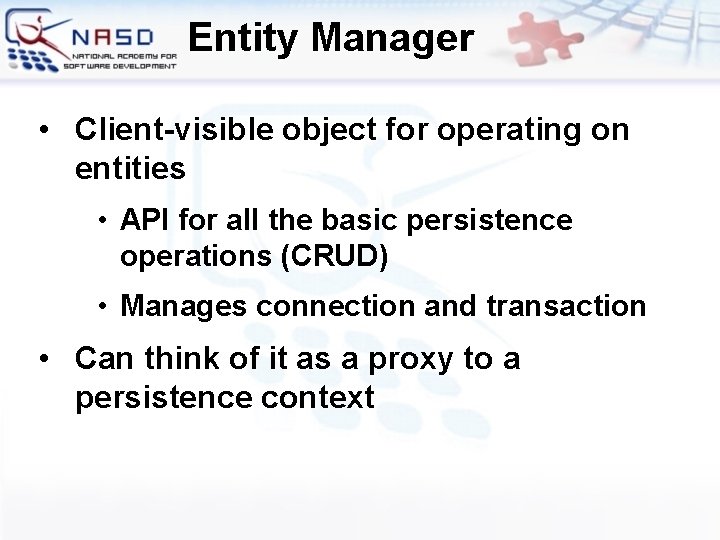
Entity Manager • Client-visible object for operating on entities • API for all the basic persistence operations (CRUD) • Manages connection and transaction • Can think of it as a proxy to a persistence context
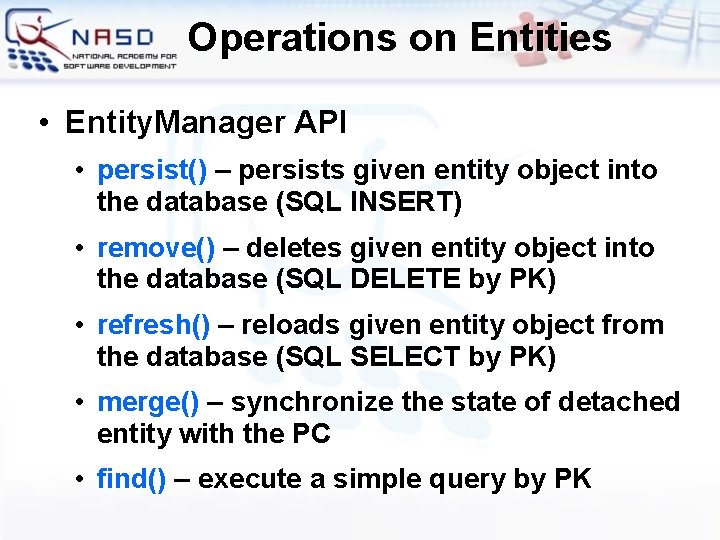
Operations on Entities • Entity. Manager API • persist() – persists given entity object into the database (SQL INSERT) • remove() – deletes given entity object into the database (SQL DELETE by PK) • refresh() – reloads given entity object from the database (SQL SELECT by PK) • merge() – synchronize the state of detached entity with the PC • find() – execute a simple query by PK
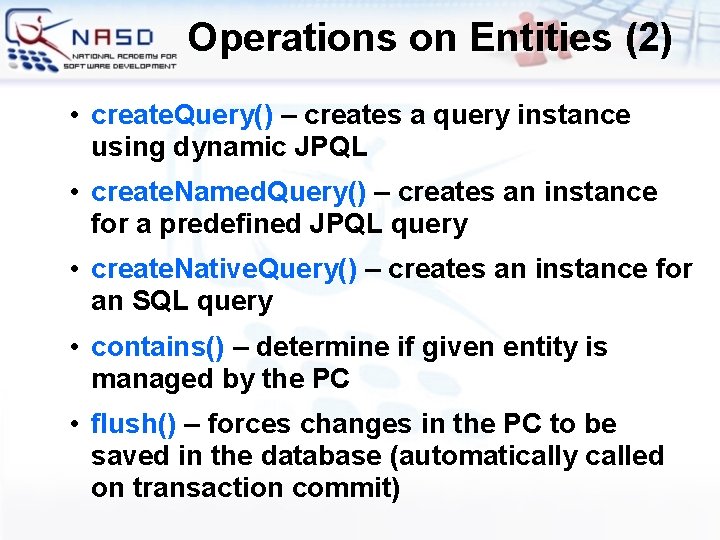
Operations on Entities (2) • create. Query() – creates a query instance using dynamic JPQL • create. Named. Query() – creates an instance for a predefined JPQL query • create. Native. Query() – creates an instance for an SQL query • contains() – determine if given entity is managed by the PC • flush() – forces changes in the PC to be saved in the database (automatically called on transaction commit)
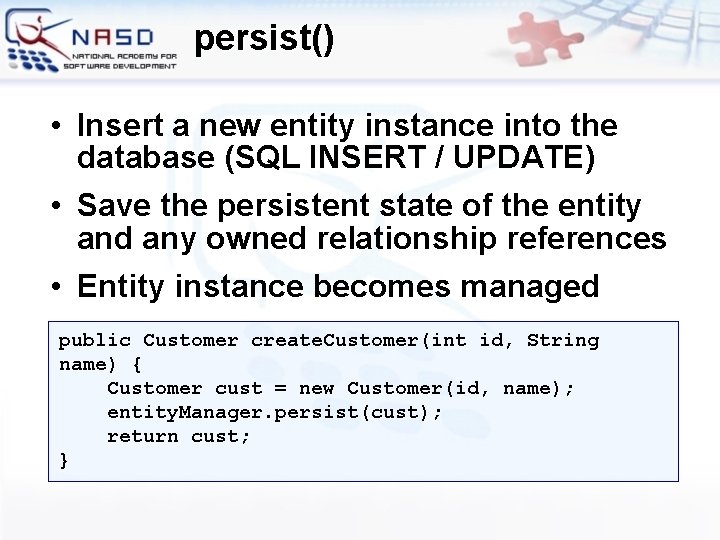
persist() • Insert a new entity instance into the database (SQL INSERT / UPDATE) • Save the persistent state of the entity and any owned relationship references • Entity instance becomes managed public Customer create. Customer(int id, String name) { Customer cust = new Customer(id, name); entity. Manager. persist(cust); return cust; }
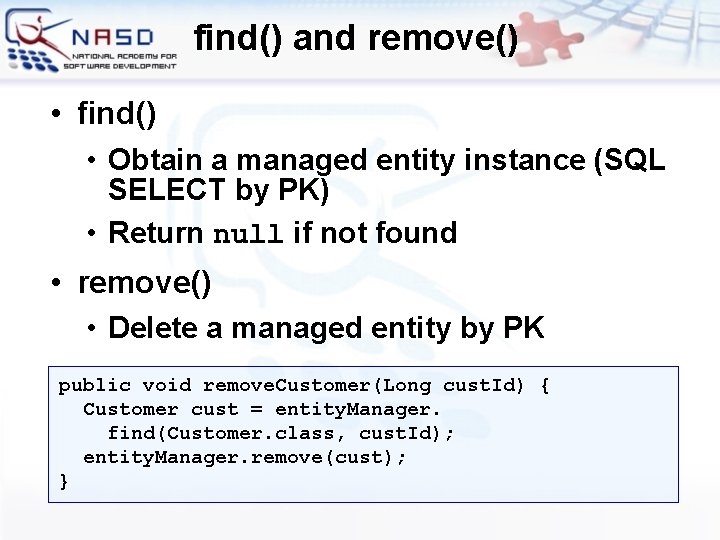
find() and remove() • find() • Obtain a managed entity instance (SQL SELECT by PK) • Return null if not found • remove() • Delete a managed entity by PK public void remove. Customer(Long cust. Id) { Customer cust = entity. Manager. find(Customer. class, cust. Id); entity. Manager. remove(cust); }
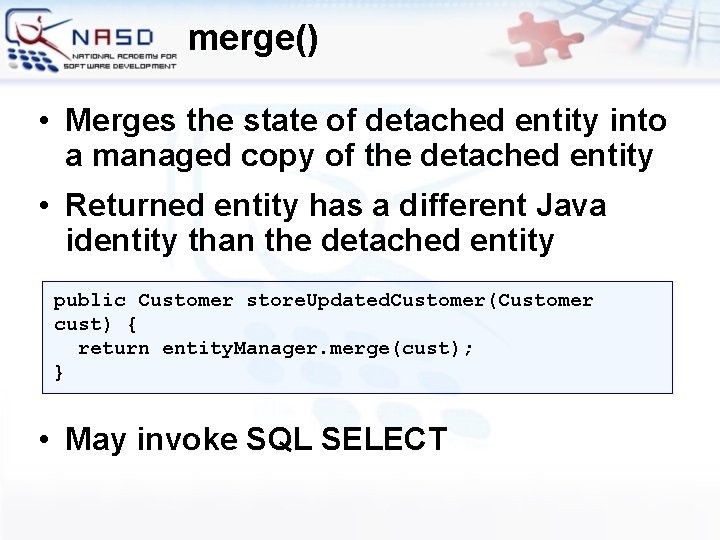
merge() • Merges the state of detached entity into a managed copy of the detached entity • Returned entity has a different Java identity than the detached entity public Customer store. Updated. Customer(Customer cust) { return entity. Manager. merge(cust); } • May invoke SQL SELECT
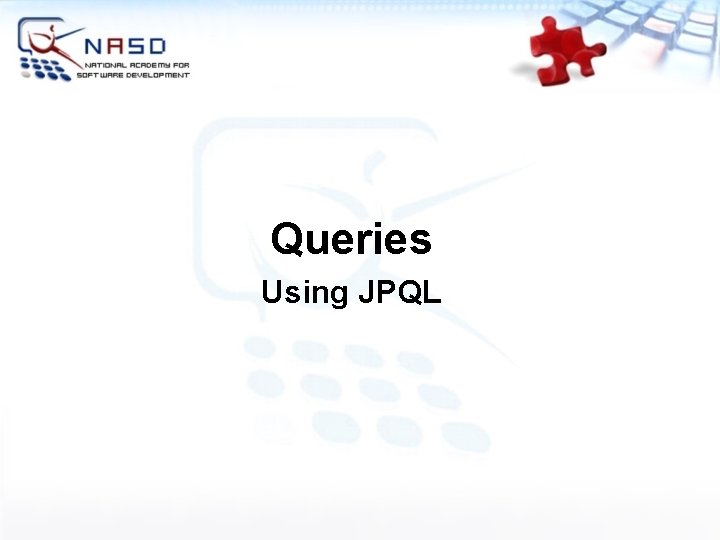
Queries Using JPQL
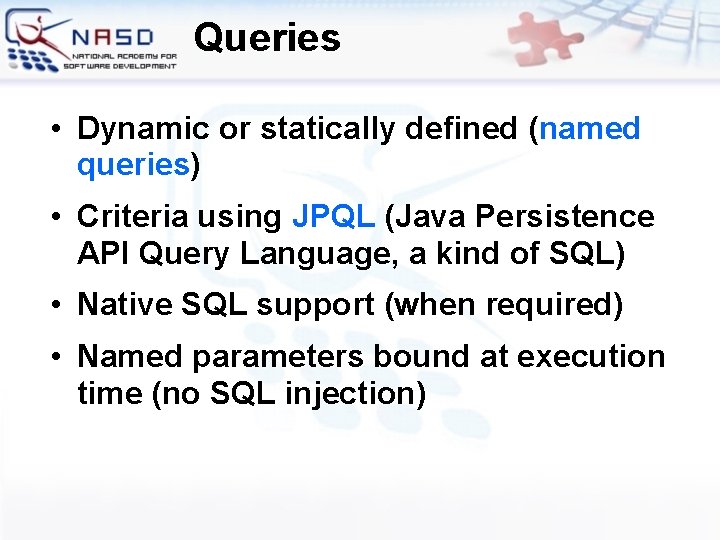
Queries • Dynamic or statically defined (named queries) • Criteria using JPQL (Java Persistence API Query Language, a kind of SQL) • Native SQL support (when required) • Named parameters bound at execution time (no SQL injection)
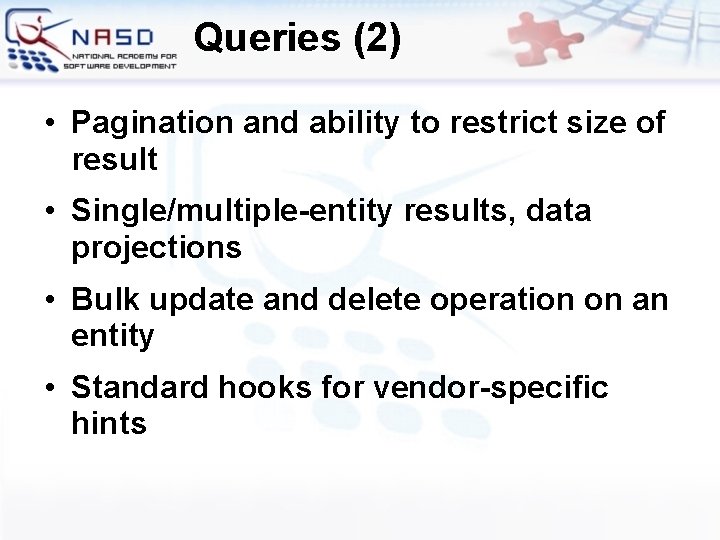
Queries (2) • Pagination and ability to restrict size of result • Single/multiple-entity results, data projections • Bulk update and delete operation on an entity • Standard hooks for vendor-specific hints
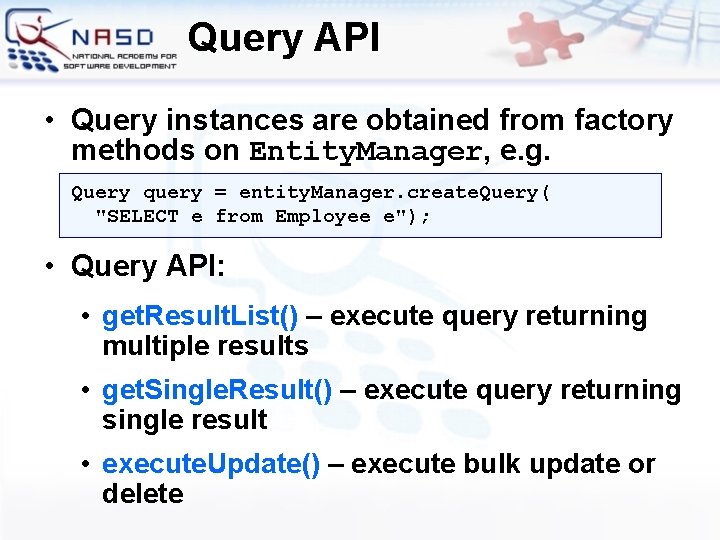
Query API • Query instances are obtained from factory methods on Entity. Manager, e. g. Query query = entity. Manager. create. Query( "SELECT e from Employee e"); • Query API: • get. Result. List() – execute query returning multiple results • get. Single. Result() – execute query returning single result • execute. Update() – execute bulk update or delete
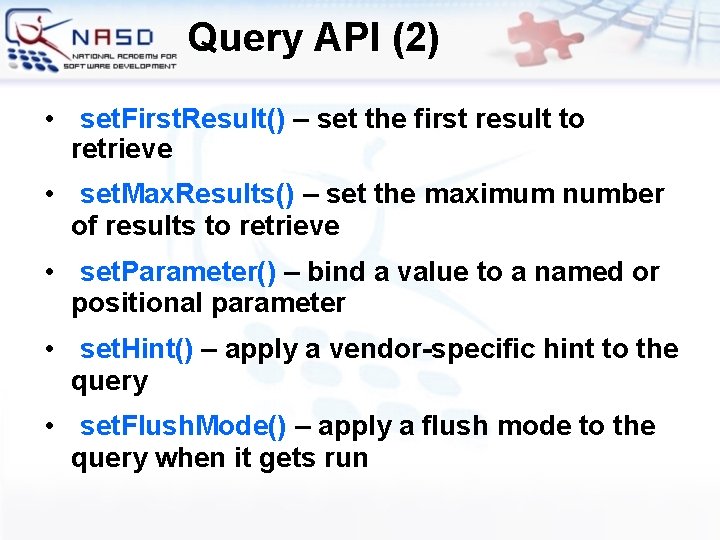
Query API (2) • set. First. Result() – set the first result to retrieve • set. Max. Results() – set the maximum number of results to retrieve • set. Parameter() – bind a value to a named or positional parameter • set. Hint() – apply a vendor-specific hint to the query • set. Flush. Mode() – apply a flush mode to the query when it gets run
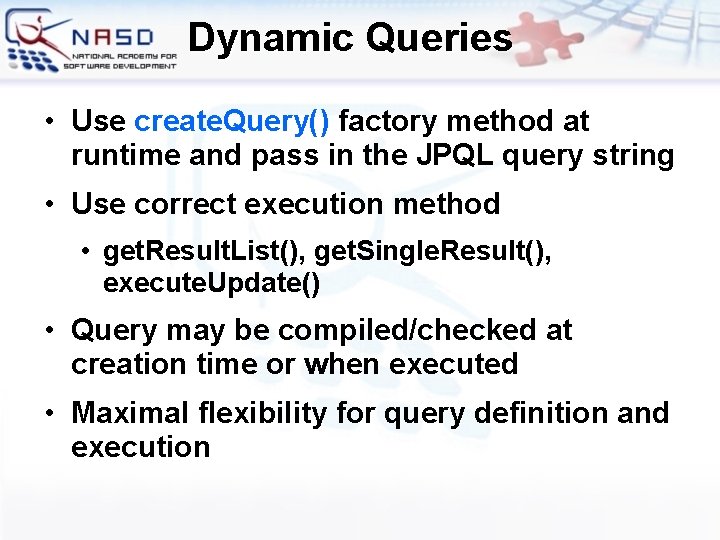
Dynamic Queries • Use create. Query() factory method at runtime and pass in the JPQL query string • Use correct execution method • get. Result. List(), get. Single. Result(), execute. Update() • Query may be compiled/checked at creation time or when executed • Maximal flexibility for query definition and execution
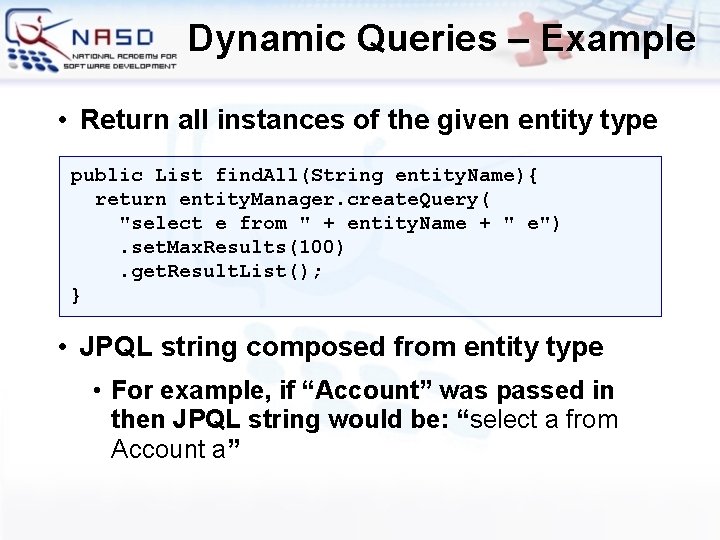
Dynamic Queries – Example • Return all instances of the given entity type public List find. All(String entity. Name){ return entity. Manager. create. Query( "select e from " + entity. Name + " e"). set. Max. Results(100). get. Result. List(); } • JPQL string composed from entity type • For example, if “Account” was passed in then JPQL string would be: “select a from Account a”
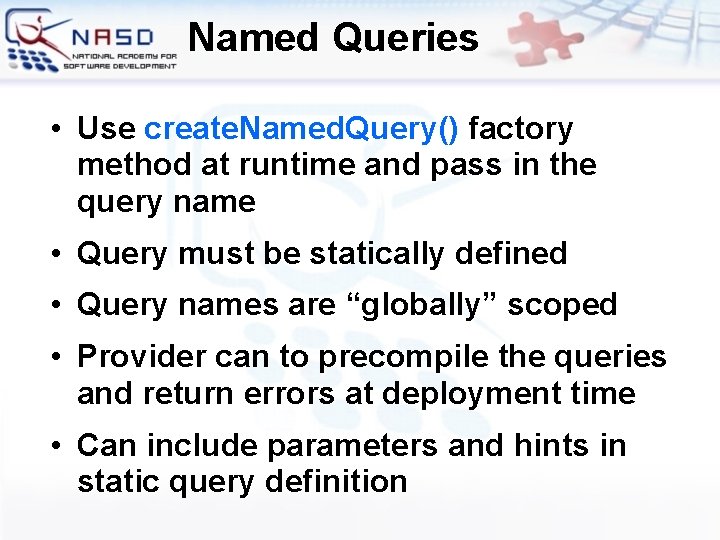
Named Queries • Use create. Named. Query() factory method at runtime and pass in the query name • Query must be statically defined • Query names are “globally” scoped • Provider can to precompile the queries and return errors at deployment time • Can include parameters and hints in static query definition
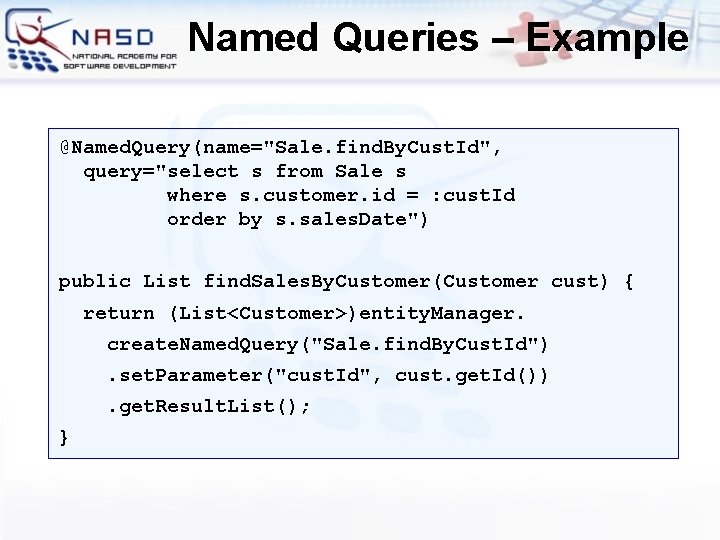
Named Queries – Example @Named. Query(name="Sale. find. By. Cust. Id", query="select s from Sale s where s. customer. id = : cust. Id order by s. sales. Date") public List find. Sales. By. Customer(Customer cust) { return (List<Customer>)entity. Manager. create. Named. Query("Sale. find. By. Cust. Id"). set. Parameter("cust. Id", cust. get. Id()). get. Result. List(); }
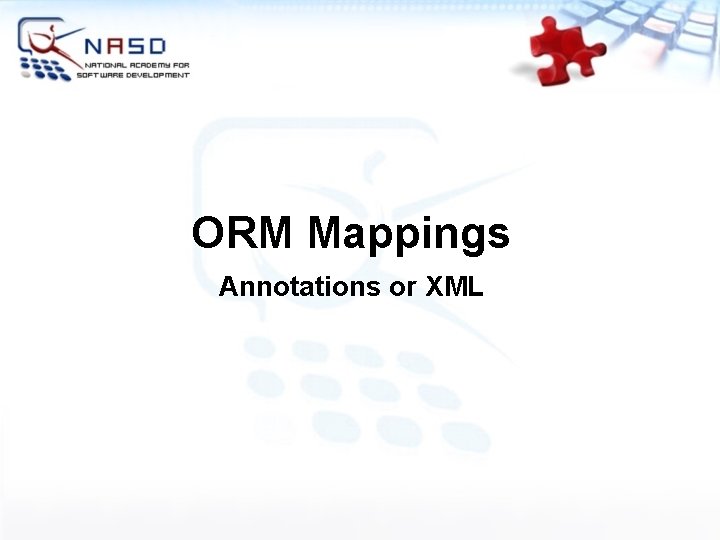
ORM Mappings Annotations or XML
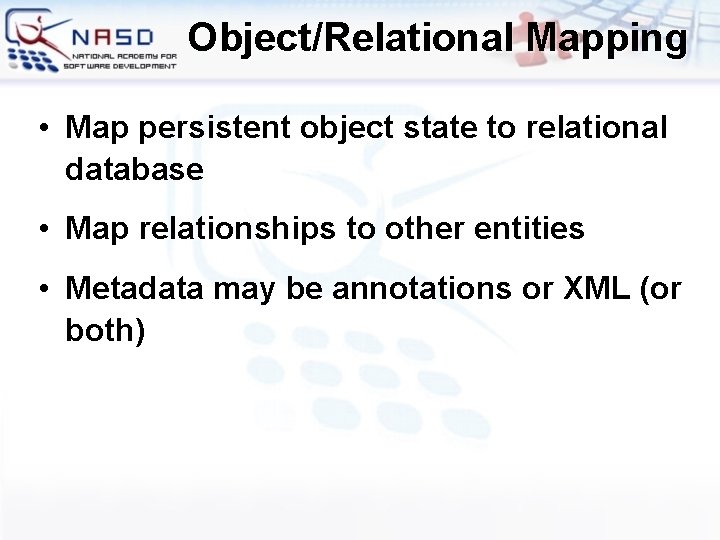
Object/Relational Mapping • Map persistent object state to relational database • Map relationships to other entities • Metadata may be annotations or XML (or both)
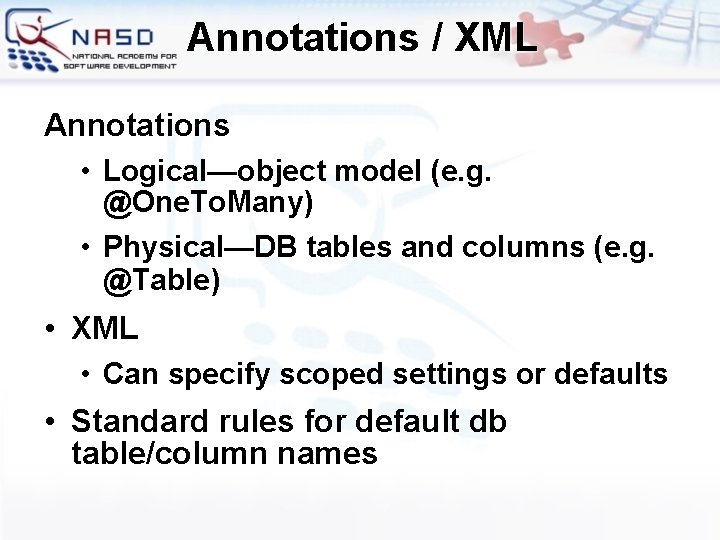
Annotations / XML Annotations • Logical—object model (e. g. @One. To. Many) • Physical—DB tables and columns (e. g. @Table) • XML • Can specify scoped settings or defaults • Standard rules for default db table/column names
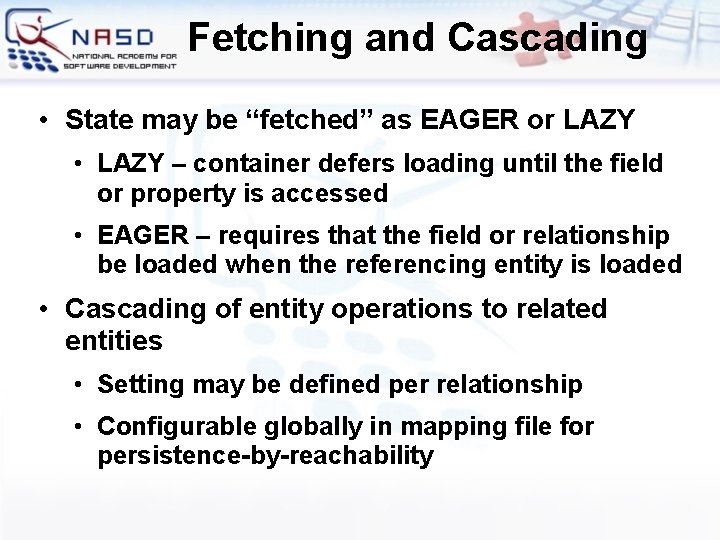
Fetching and Cascading • State may be “fetched” as EAGER or LAZY • LAZY – container defers loading until the field or property is accessed • EAGER – requires that the field or relationship be loaded when the referencing entity is loaded • Cascading of entity operations to related entities • Setting may be defined per relationship • Configurable globally in mapping file for persistence-by-reachability
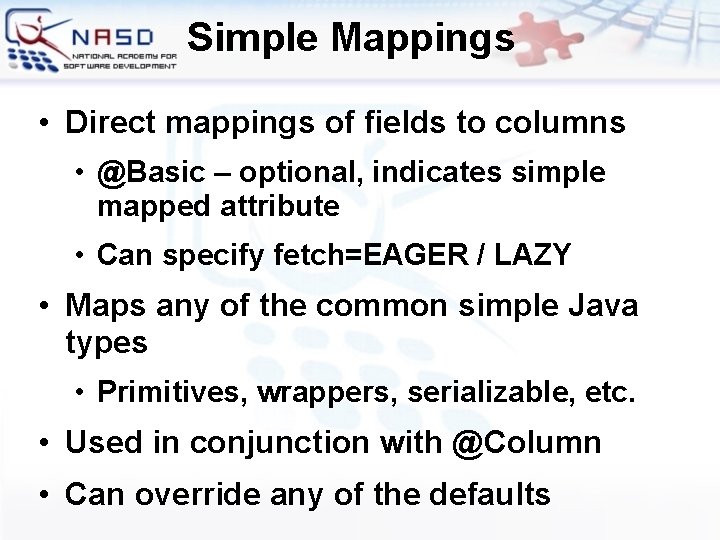
Simple Mappings • Direct mappings of fields to columns • @Basic – optional, indicates simple mapped attribute • Can specify fetch=EAGER / LAZY • Maps any of the common simple Java types • Primitives, wrappers, serializable, etc. • Used in conjunction with @Column • Can override any of the defaults
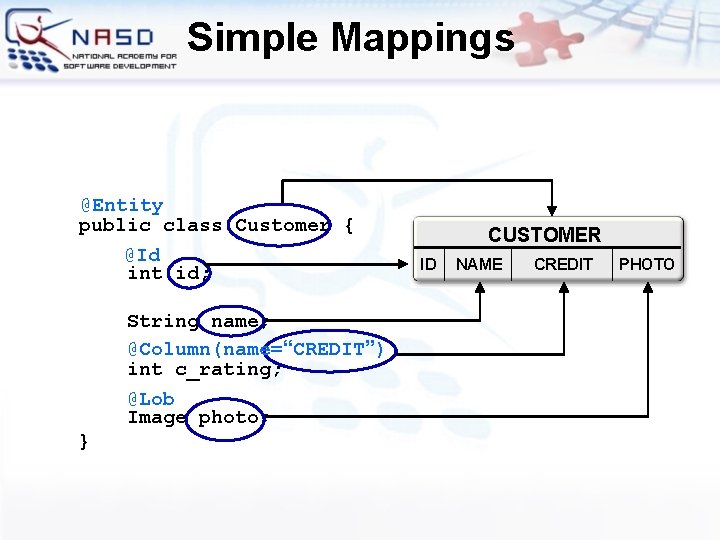
Simple Mappings @Entity public class Customer { @Id int id; String name; @Column(name=“CREDIT”) int c_rating; @Lob Image photo; } CUSTOMER ID NAME CREDIT PHOTO
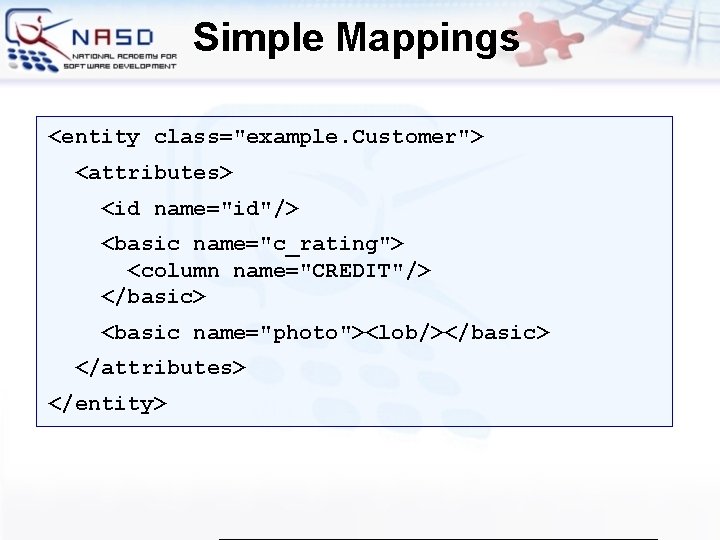
Simple Mappings <entity class="example. Customer"> <attributes> <id name="id"/> <basic name="c_rating"> <column name="CREDIT"/> </basic> <basic name="photo"><lob/></basic> </attributes> </entity>
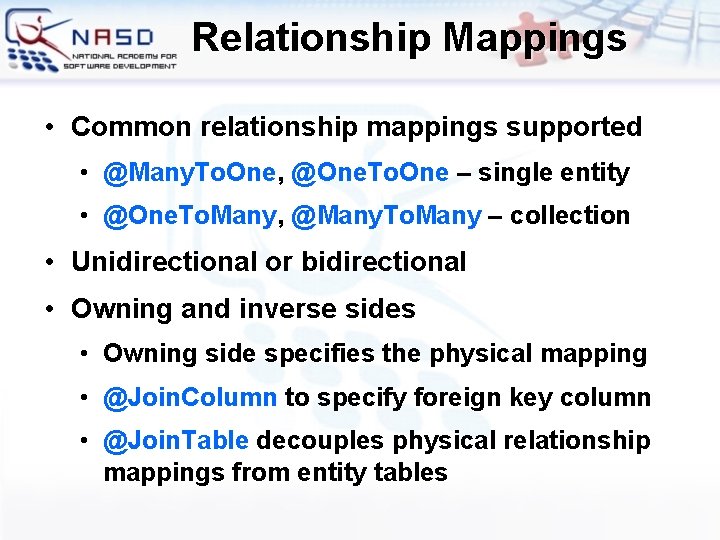
Relationship Mappings • Common relationship mappings supported • @Many. To. One, @One. To. One – single entity • @One. To. Many, @Many. To. Many – collection • Unidirectional or bidirectional • Owning and inverse sides • Owning side specifies the physical mapping • @Join. Column to specify foreign key column • @Join. Table decouples physical relationship mappings from entity tables
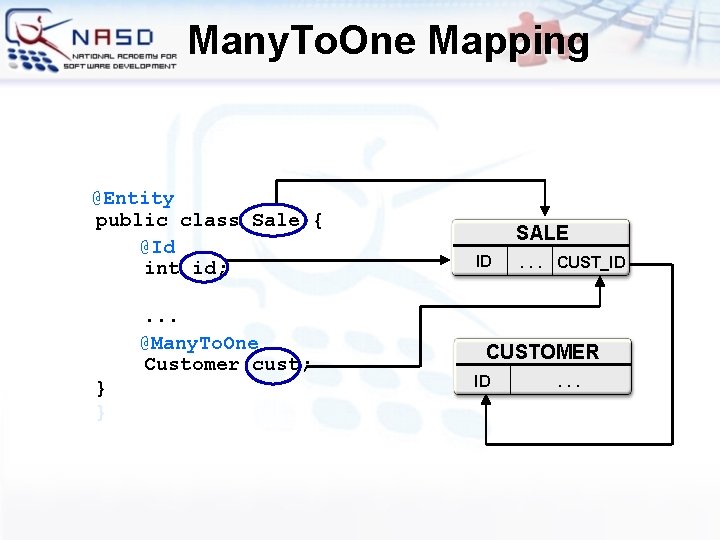
Many. To. One Mapping @Entity public class Sale { @Id int id; . . . @Many. To. One Customer cust; } } SALE ID . . . CUST_ID CUSTOMER ID . . .
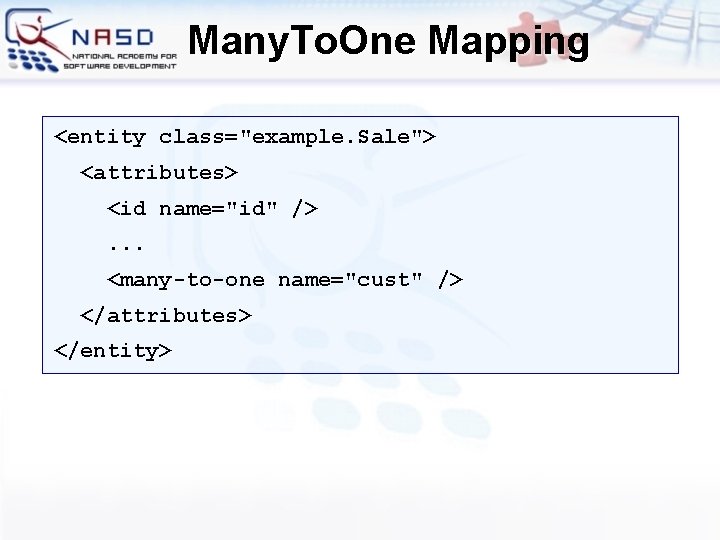
Many. To. One Mapping <entity class="example. Sale"> <attributes> <id name="id" />. . . <many-to-one name="cust" /> </attributes> </entity>
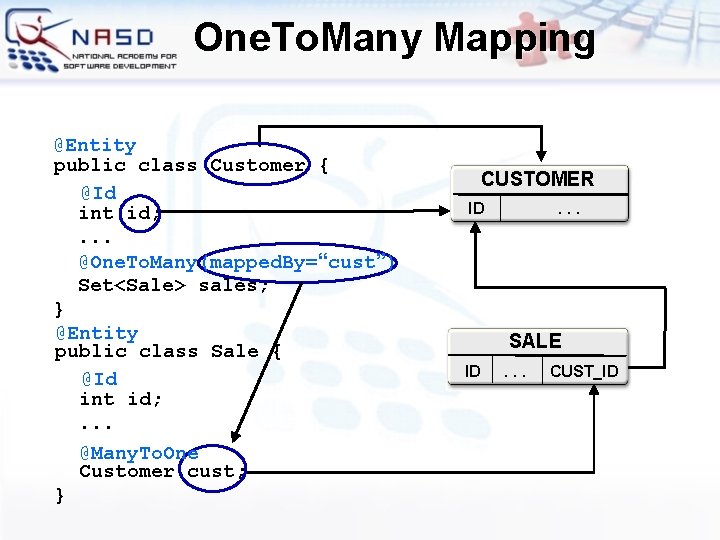
One. To. Many Mapping @Entity public class Customer { @Id int id; . . . @One. To. Many(mapped. By=“cust”) Set<Sale> sales; } @Entity public class Sale { @Id int id; . . . @Many. To. One Customer cust; } CUSTOMER ID . . . SALE ID . . . CUST_ID
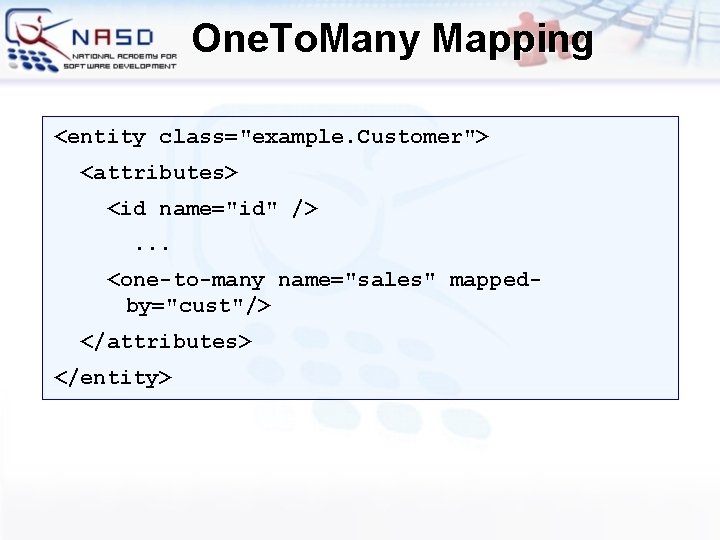
One. To. Many Mapping <entity class="example. Customer"> <attributes> <id name="id" />. . . <one-to-many name="sales" mappedby="cust"/> </attributes> </entity>
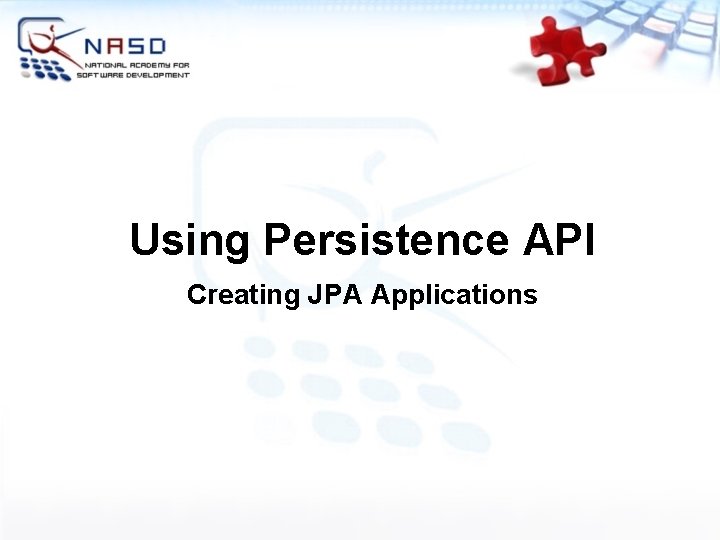
Using Persistence API Creating JPA Applications
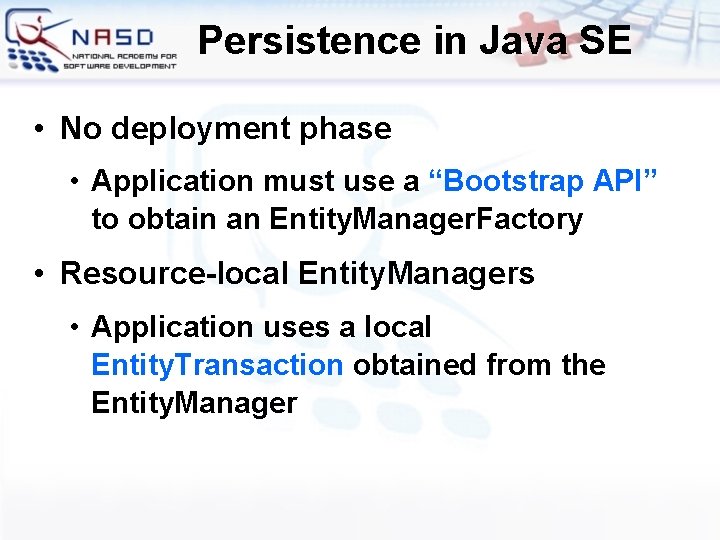
Persistence in Java SE • No deployment phase • Application must use a “Bootstrap API” to obtain an Entity. Manager. Factory • Resource-local Entity. Managers • Application uses a local Entity. Transaction obtained from the Entity. Manager
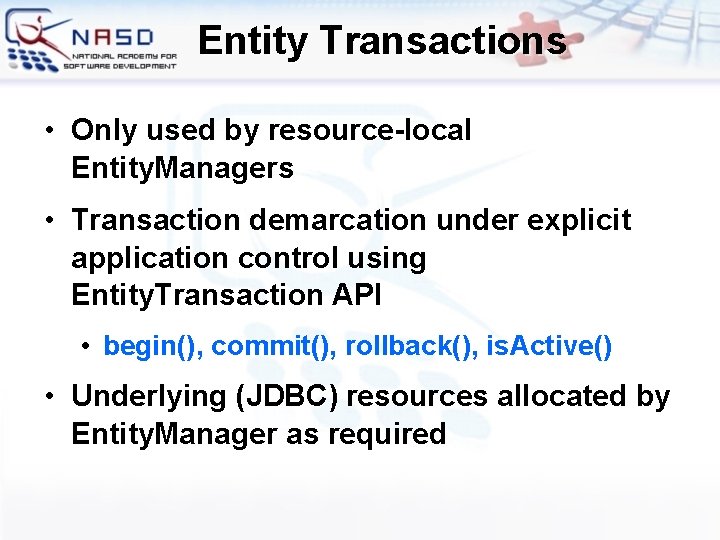
Entity Transactions • Only used by resource-local Entity. Managers • Transaction demarcation under explicit application control using Entity. Transaction API • begin(), commit(), rollback(), is. Active() • Underlying (JDBC) resources allocated by Entity. Manager as required
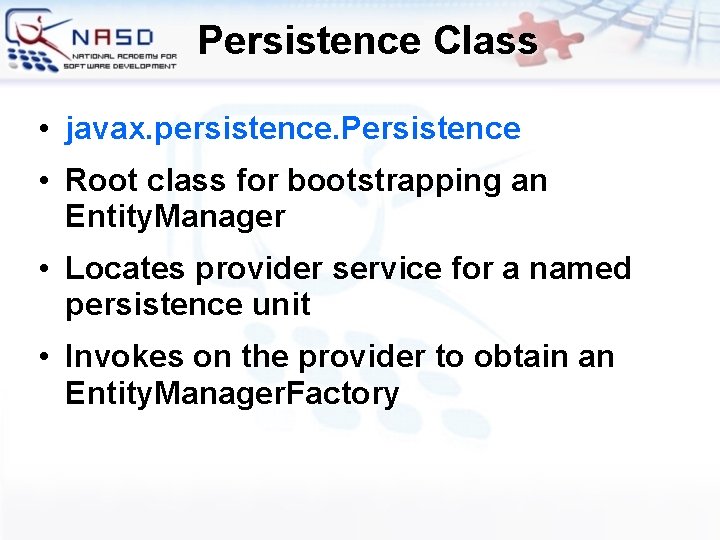
Persistence Class • javax. persistence. Persistence • Root class for bootstrapping an Entity. Manager • Locates provider service for a named persistence unit • Invokes on the provider to obtain an Entity. Manager. Factory
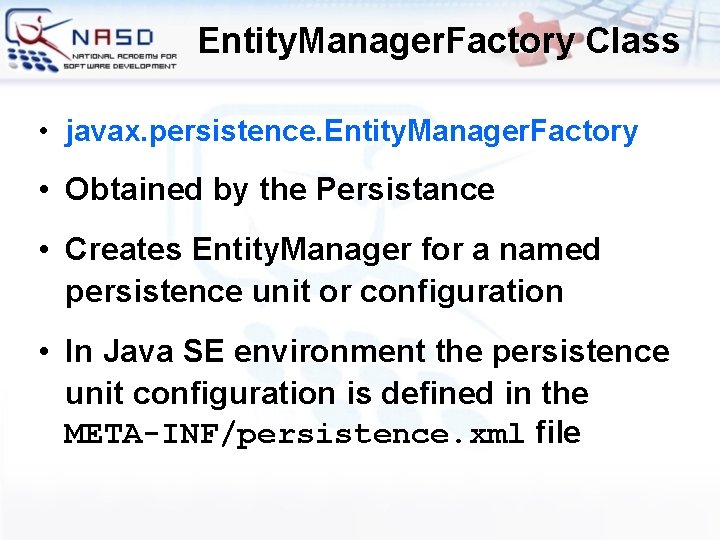
Entity. Manager. Factory Class • javax. persistence. Entity. Manager. Factory • Obtained by the Persistance • Creates Entity. Manager for a named persistence unit or configuration • In Java SE environment the persistence unit configuration is defined in the META-INF/persistence. xml file
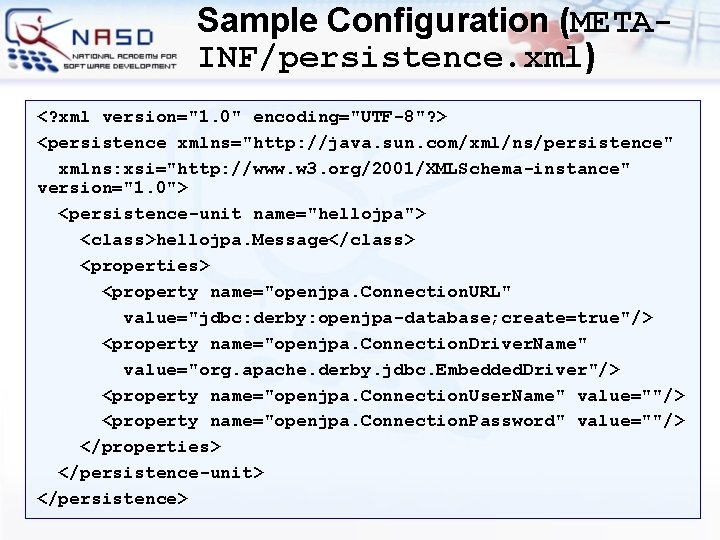
Sample Configuration (METAINF/persistence. xml) <? xml version="1. 0" encoding="UTF-8"? > <persistence xmlns="http: //java. sun. com/xml/ns/persistence" xmlns: xsi="http: //www. w 3. org/2001/XMLSchema-instance" version="1. 0"> <persistence-unit name="hellojpa"> <class>hellojpa. Message</class> <properties> <property name="openjpa. Connection. URL" value="jdbc: derby: openjpa-database; create=true"/> <property name="openjpa. Connection. Driver. Name" value="org. apache. derby. jdbc. Embedded. Driver"/> <property name="openjpa. Connection. User. Name" value=""/> <property name="openjpa. Connection. Password" value=""/> </properties> </persistence-unit> </persistence>
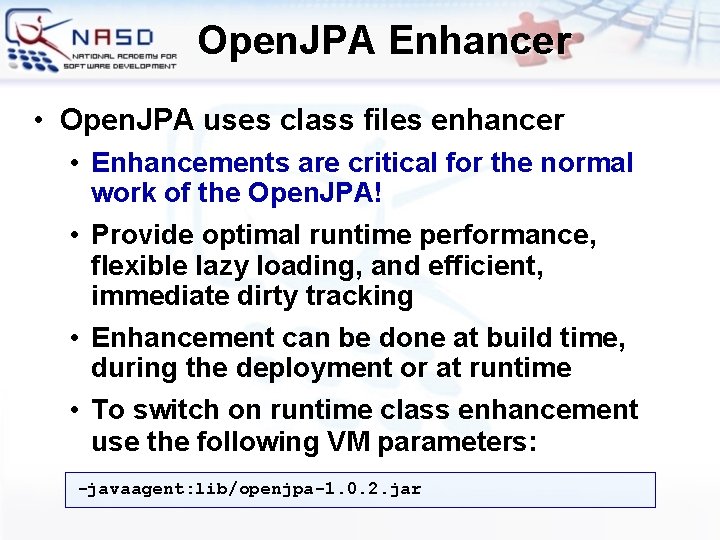
Open. JPA Enhancer • Open. JPA uses class files enhancer • Enhancements are critical for the normal work of the Open. JPA! • Provide optimal runtime performance, flexible lazy loading, and efficient, immediate dirty tracking • Enhancement can be done at build time, during the deployment or at runtime • To switch on runtime class enhancement use the following VM parameters: -javaagent: lib/openjpa-1. 0. 2. jar
![JPA Bootstrap Example public class Persistence Example public static void mainString args JPA Bootstrap – Example public class Persistence. Example { public static void main(String[] args)](https://slidetodoc.com/presentation_image/da3e0636d2f19fae2ce92b078ad5f6be/image-54.jpg)
JPA Bootstrap – Example public class Persistence. Example { public static void main(String[] args) { Entity. Manager. Factory emf = Persistence. create. Entity. Manager. Factory("Some. PUnit"); Entity. Manager em = emf. create. Entity. Manager(); em. get. Transaction(). begin(); // Perform finds, execute queries, // update entities, etc. em. get. Transaction(). commit(); em. close(); emf. close(); } }
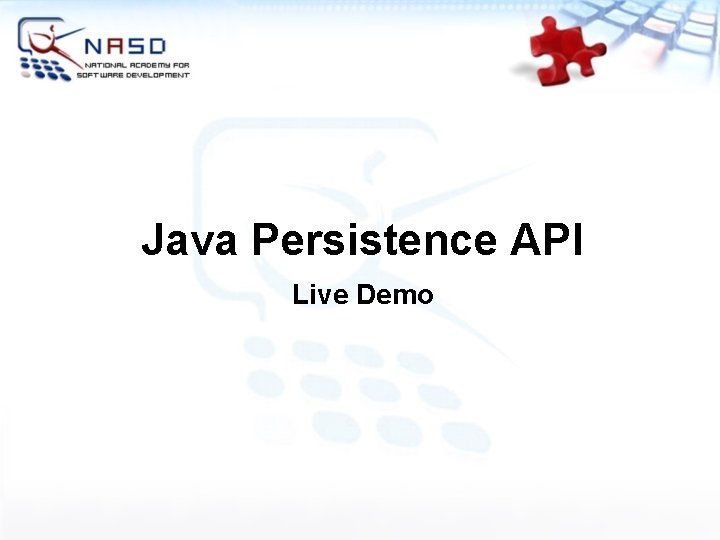
Java Persistence API Live Demo
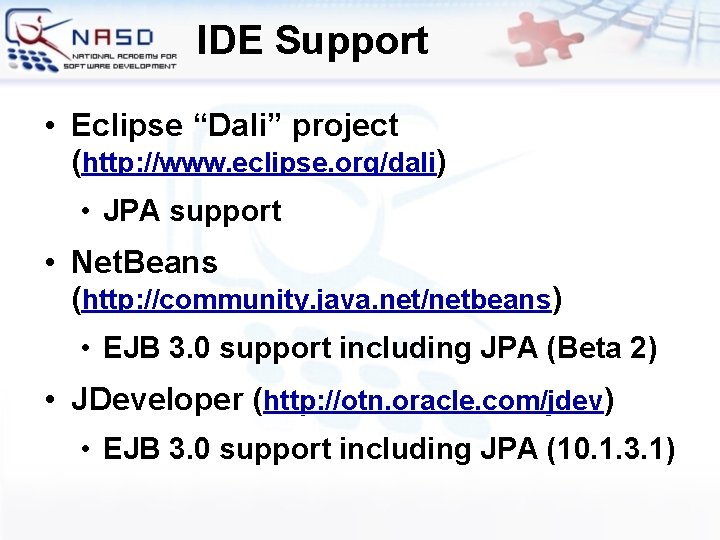
IDE Support • Eclipse “Dali” project (http: //www. eclipse. org/dali) • JPA support • Net. Beans (http: //community. java. net/netbeans) • EJB 3. 0 support including JPA (Beta 2) • JDeveloper (http: //otn. oracle. com/jdev) • EJB 3. 0 support including JPA (10. 1. 3. 1)
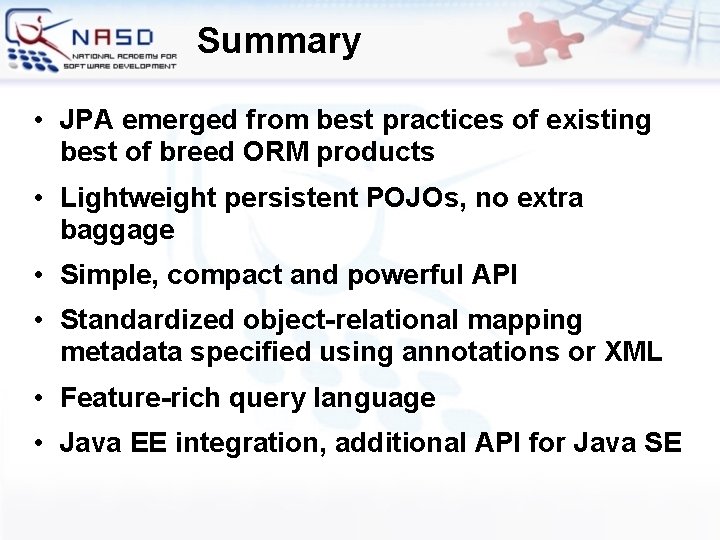
Summary • JPA emerged from best practices of existing best of breed ORM products • Lightweight persistent POJOs, no extra baggage • Simple, compact and powerful API • Standardized object-relational mapping metadata specified using annotations or XML • Feature-rich query language • Java EE integration, additional API for Java SE
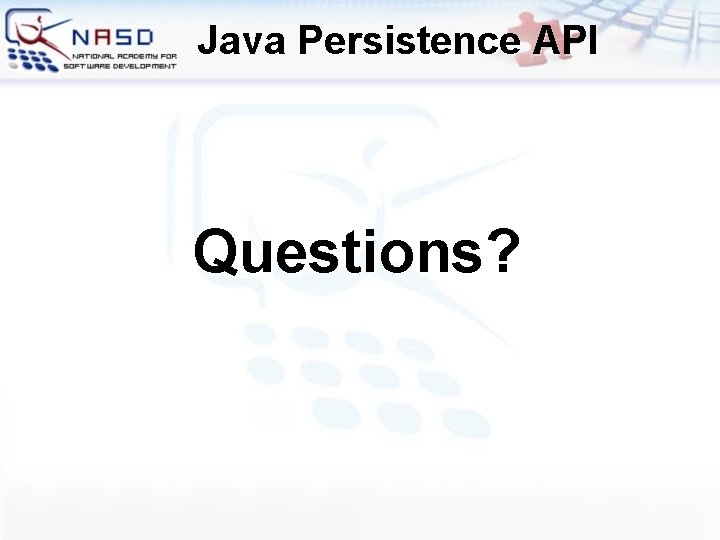
Java Persistence API Questions?
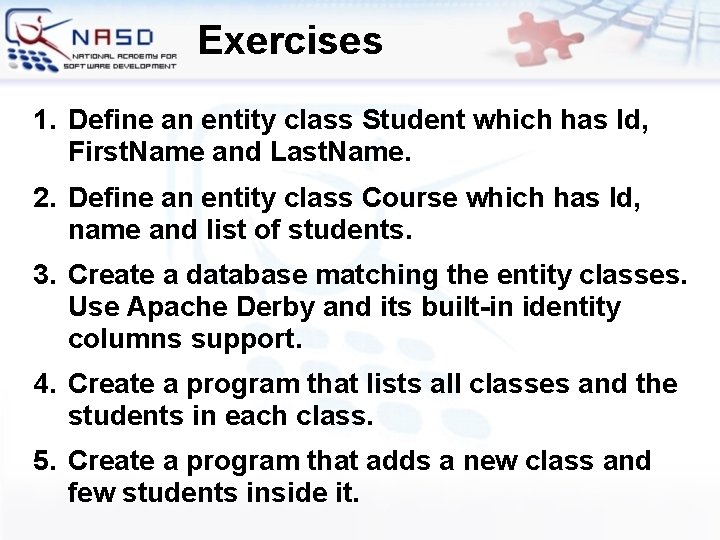
Exercises 1. Define an entity class Student which has Id, First. Name and Last. Name. 2. Define an entity class Course which has Id, name and list of students. 3. Create a database matching the entity classes. Use Apache Derby and its built-in identity columns support. 4. Create a program that lists all classes and the students in each class. 5. Create a program that adds a new class and few students inside it.
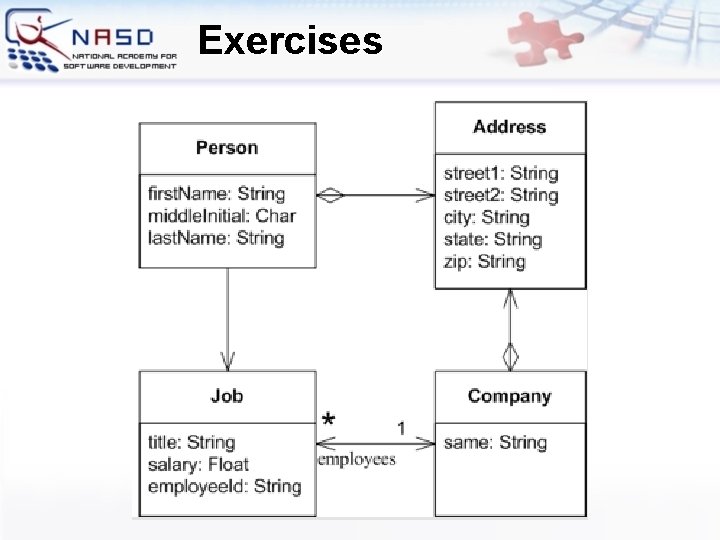
Exercises
Object persistence in java
Object persistence in java
Site:slidetodoc.com
Dali jpa tools
Java persistence architecture
Java object persistence
Java database connectivity api
Dominonappulat
Java audio api
Java sound api
Java print api
Graphics api java
Generic rest api java
Java sun api
Dynamic proxies in java
Java reflection api
Khan academy programming
National academy for public administration
National academy of statistical administration
National academy of railroad sciences
National emergency services academy
National coalition academy
National skills academy for retail
National academy of sciences forensic science
Lbsnaa mess menu
Kontinuitetshantering
Novell typiska drag
Tack för att ni lyssnade bild
Vad står k.r.å.k.a.n för
Varför kallas perioden 1918-1939 för mellankrigstiden
En lathund för arbete med kontinuitetshantering
Underlag för särskild löneskatt på pensionskostnader
Tidbok för yrkesförare
Anatomi organ reproduksi
Vad är densitet
Datorkunskap för nybörjare
Tack för att ni lyssnade bild
Hur skriver man en debattartikel
Magnetsjukhus
Nyckelkompetenser för livslångt lärande
Påbyggnader för flakfordon
Lufttryck formel
Offentlig förvaltning
I gullregnens månad
Presentera för publik crossboss
Argument för teckenspråk som minoritetsspråk
Vem räknas som jude
Treserva lathund
Epiteltyper
Bästa kameran för astrofoto
Cks
Verifikationsplan
Mat för unga idrottare
Verktyg för automatisering av utbetalningar
Rutin för avvikelsehantering
Smärtskolan kunskap för livet
Ministerstyre för och nackdelar
Tack för att ni har lyssnat
Vad är referatmarkeringar
Redogör för vad psykologi är
Matematisk modellering eksempel