Introduction to Java API Java API Specifications java
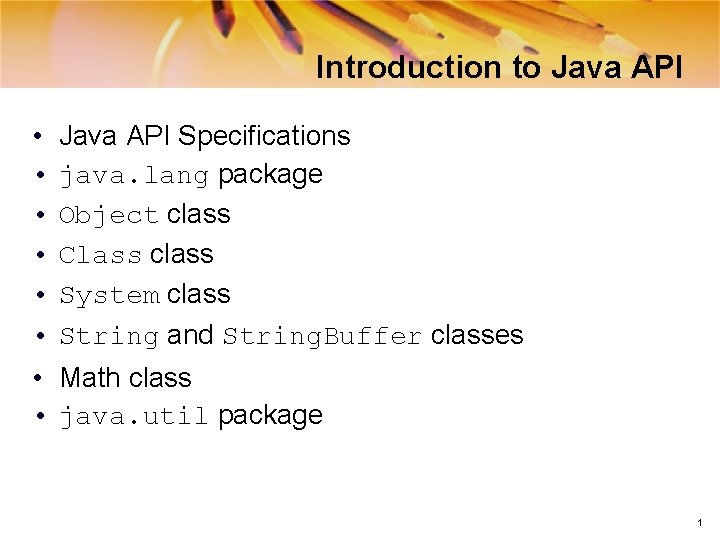
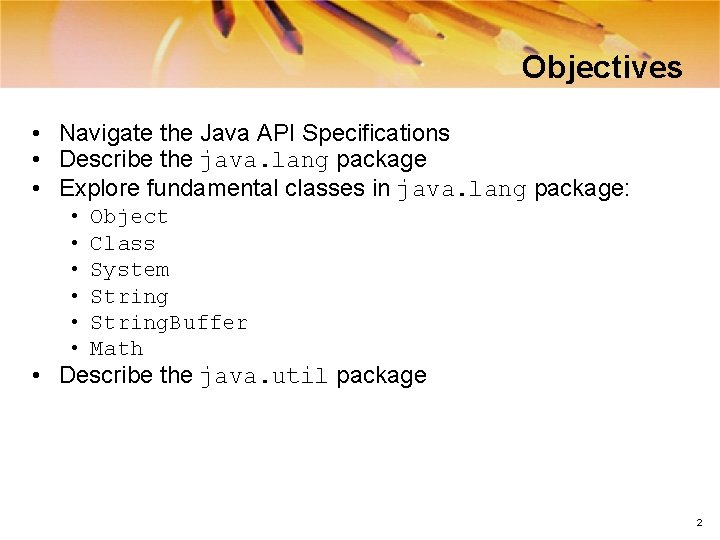
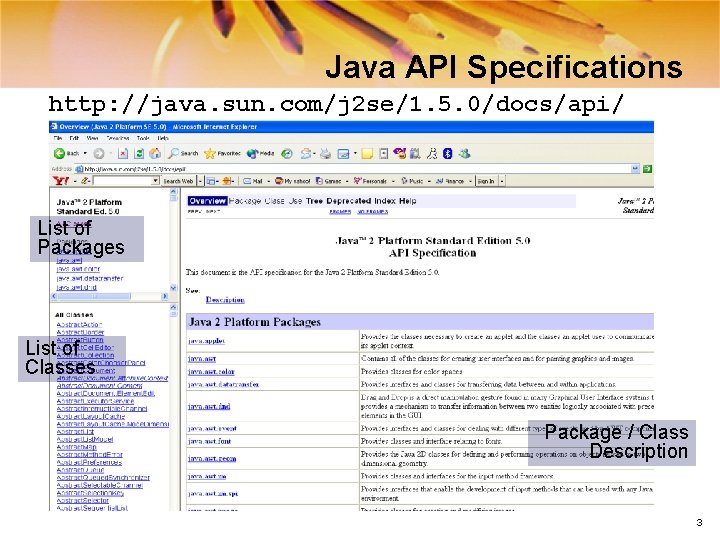
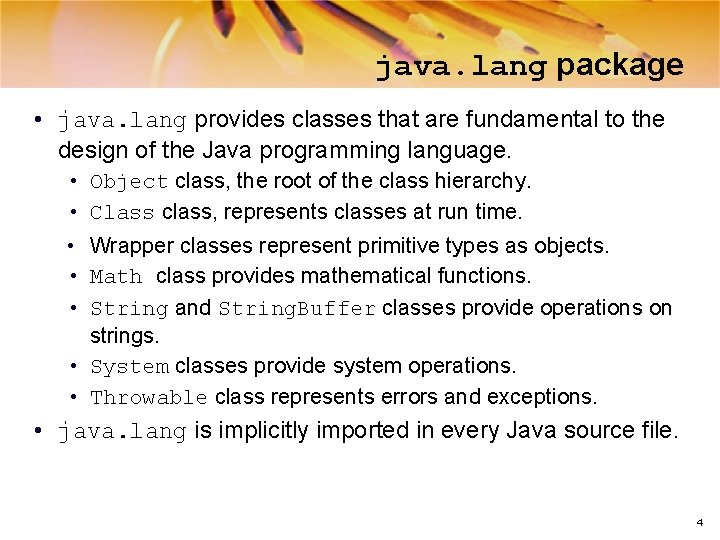
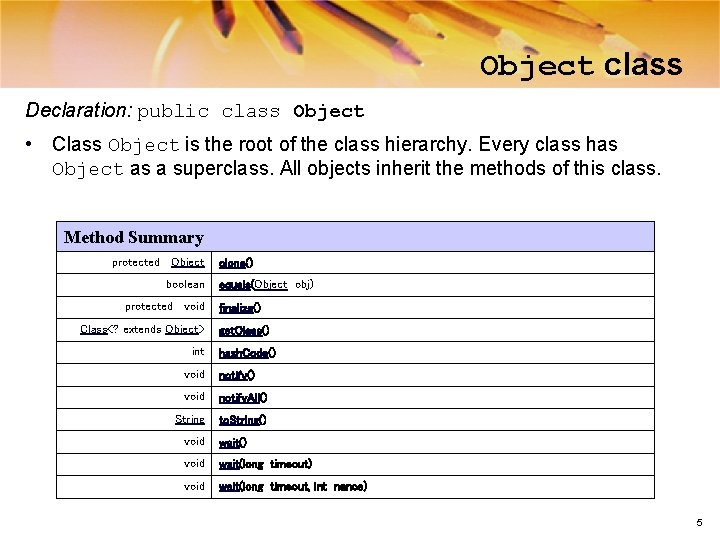
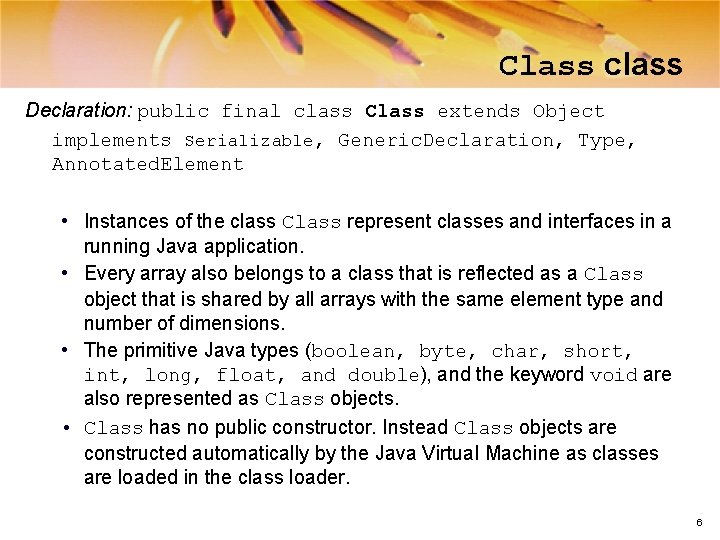
![Class class Method Summary (Partial List) static Class[] Constructor[] Field[] Class[] for. Name(String class. Class class Method Summary (Partial List) static Class[] Constructor[] Field[] Class[] for. Name(String class.](https://slidetodoc.com/presentation_image/2bd8aa05d2bb9ffa8c9404c218a3acb2/image-7.jpg)
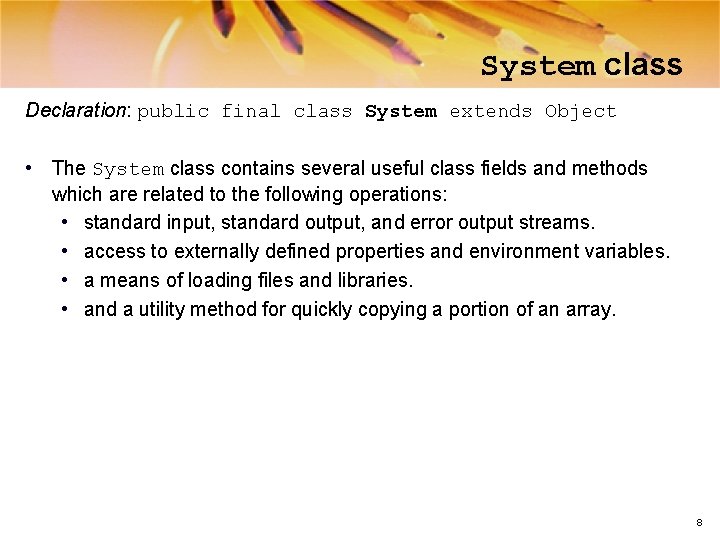
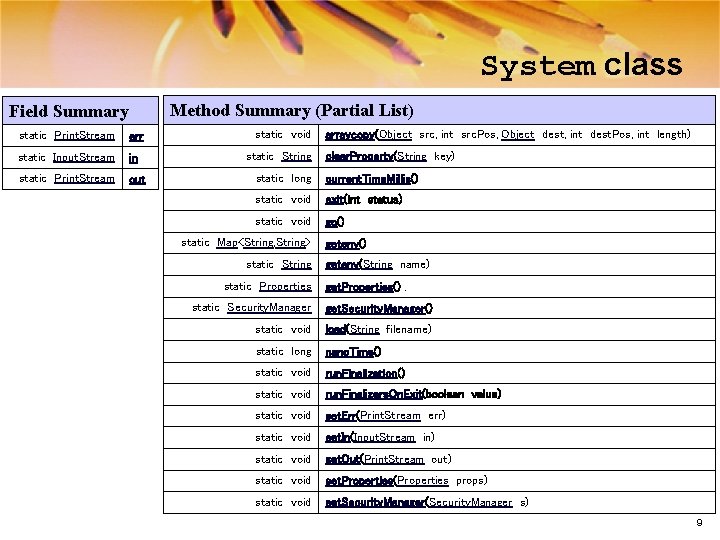
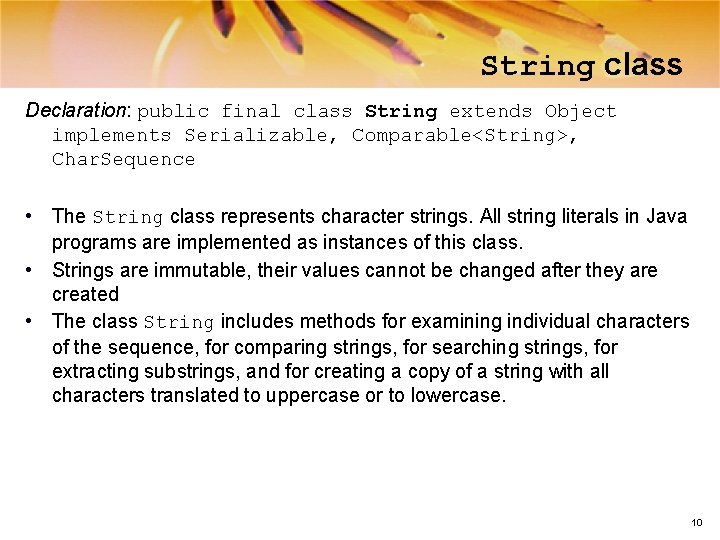
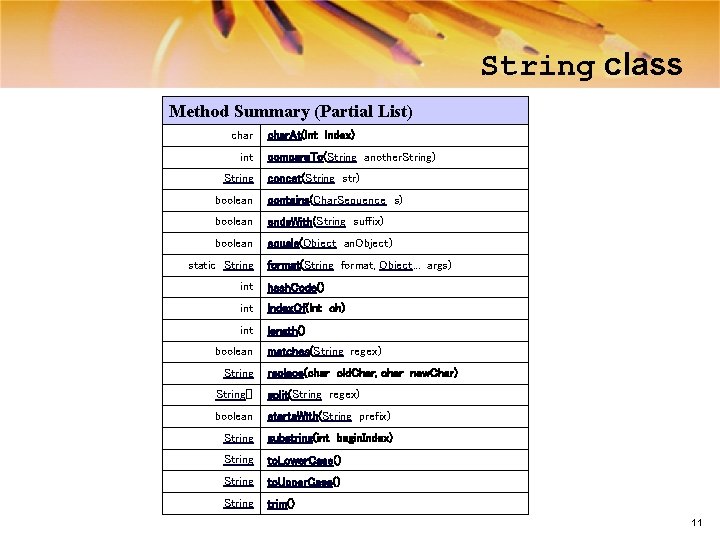
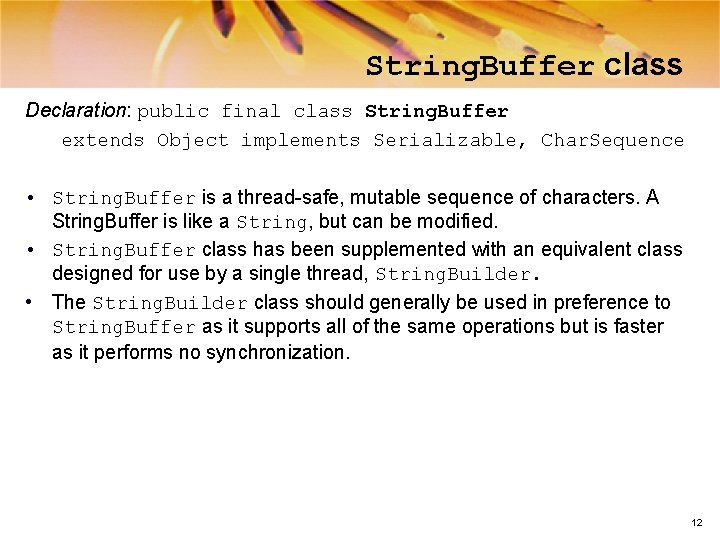
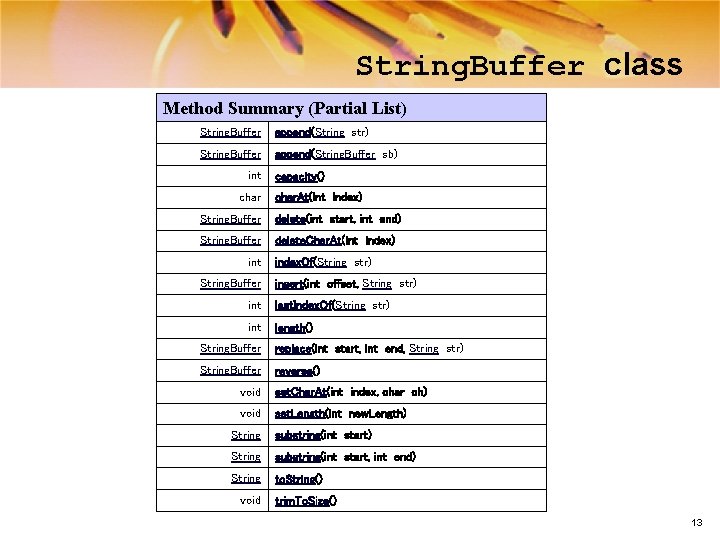
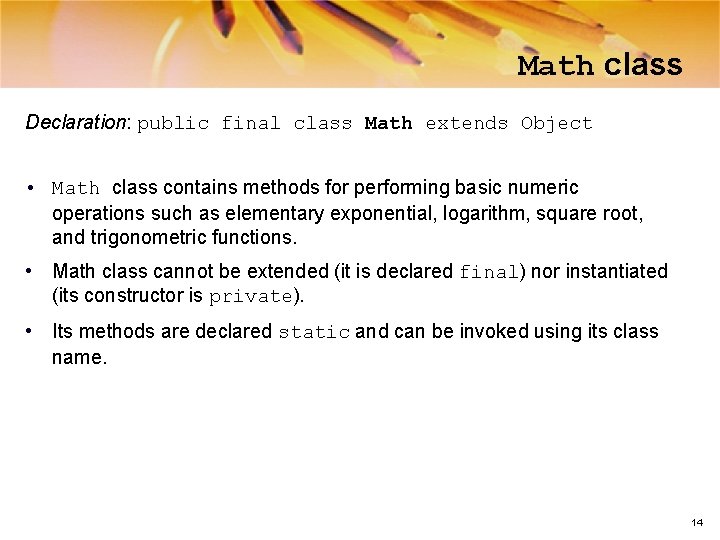
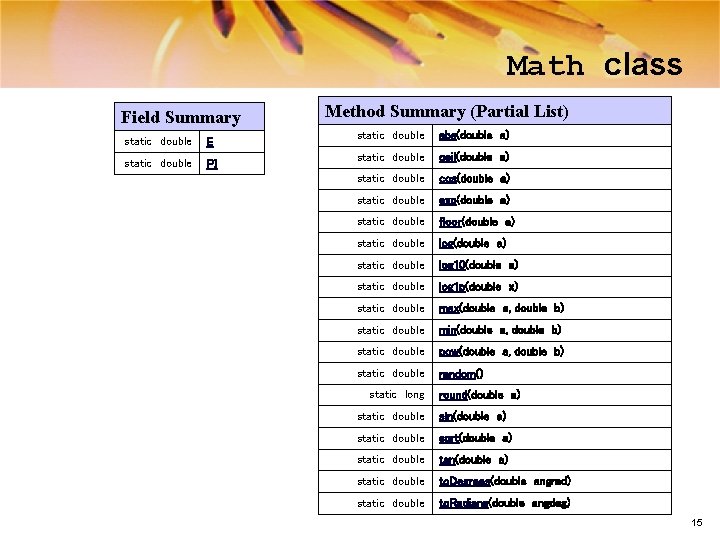
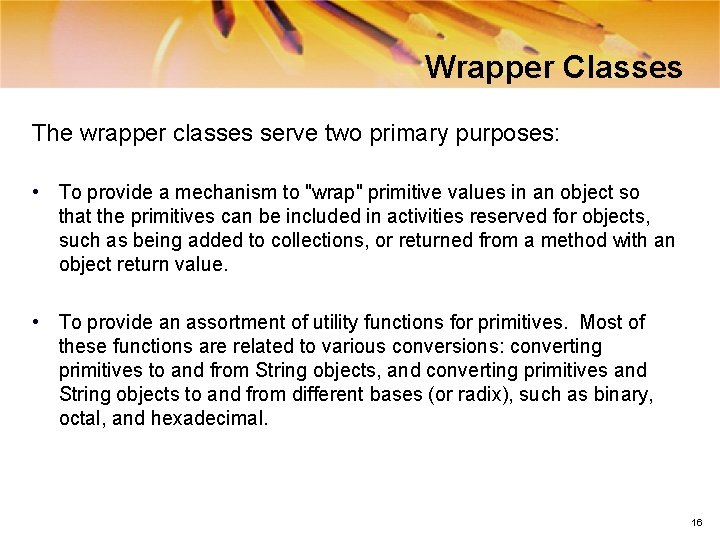
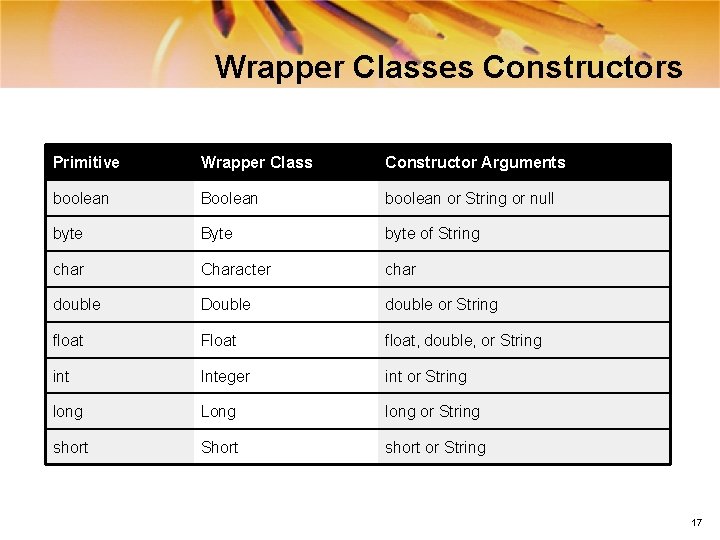
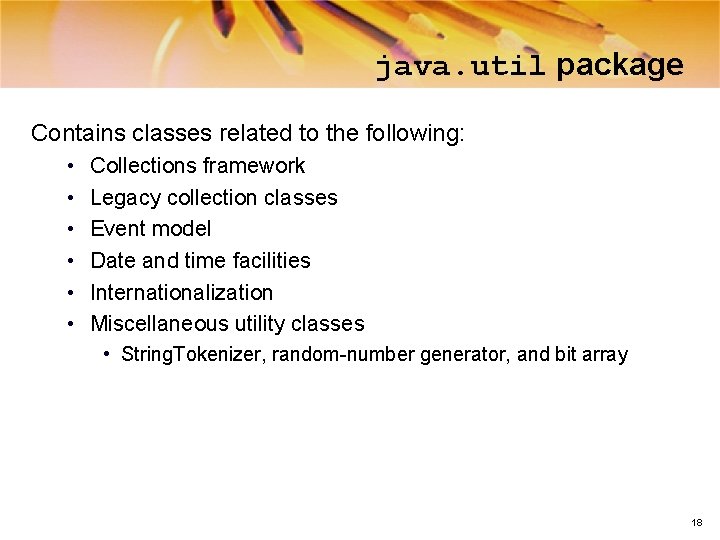
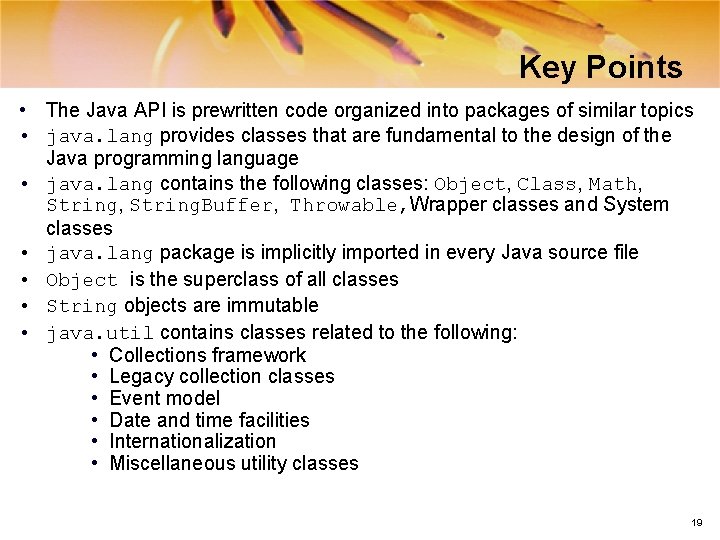
- Slides: 19
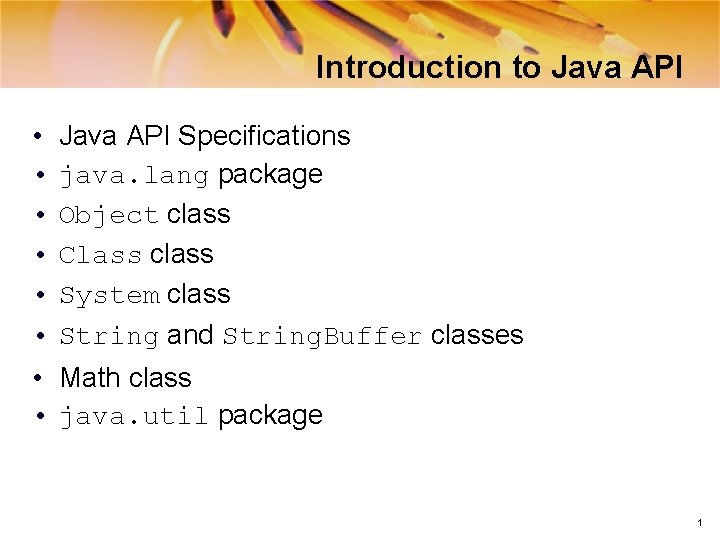
Introduction to Java API • • • Java API Specifications java. lang package Object class Class class System class String and String. Buffer classes • Math class • java. util package 1
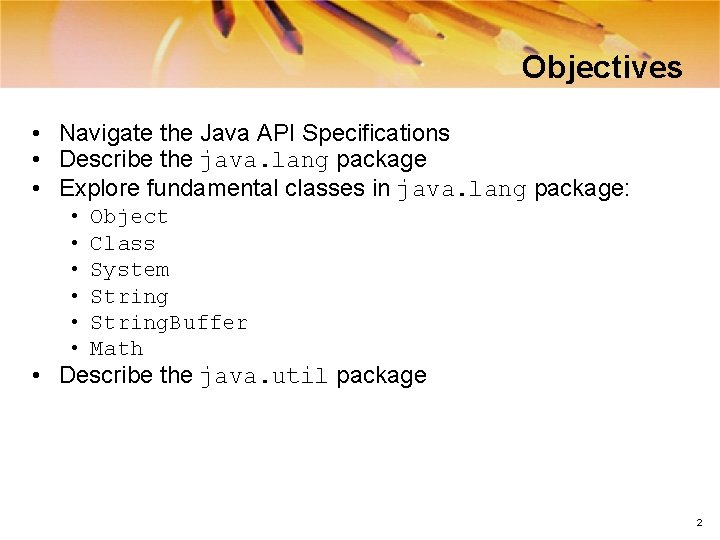
Objectives • Navigate the Java API Specifications • Describe the java. lang package • Explore fundamental classes in java. lang package: • • • Object Class System String. Buffer Math • Describe the java. util package 2
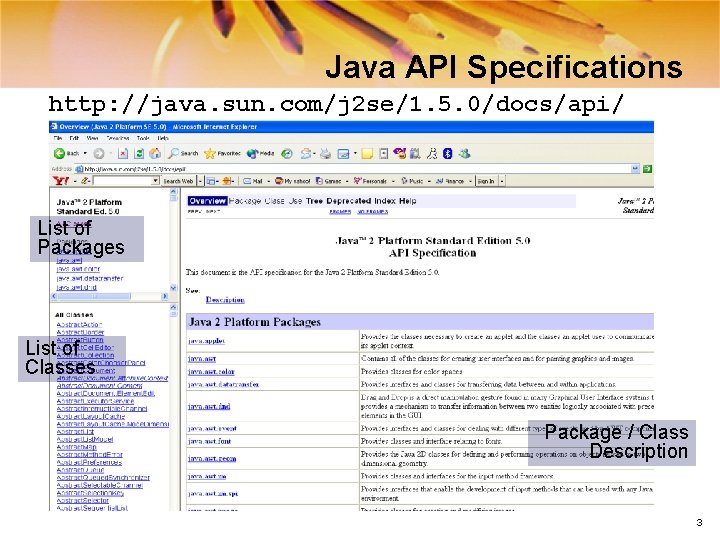
Java API Specifications http: //java. sun. com/j 2 se/1. 5. 0/docs/api/ List of Packages List of Classes Package / Class Description 3
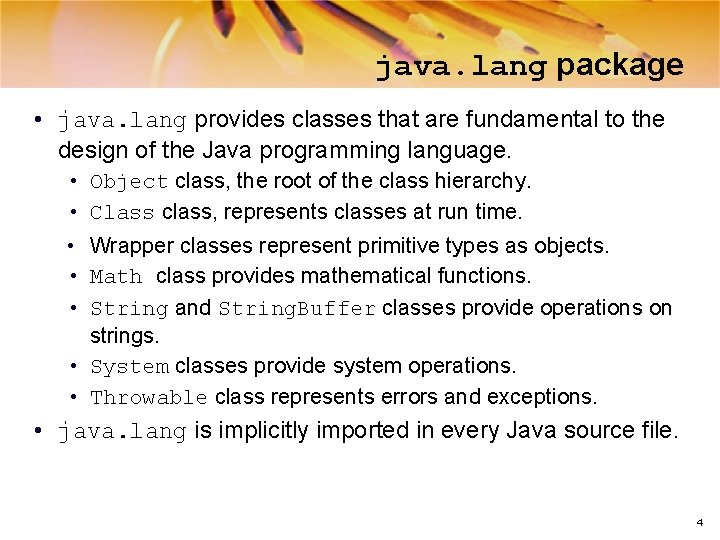
java. lang package • java. lang provides classes that are fundamental to the design of the Java programming language. • • • Object class, the root of the class hierarchy. Class class, represents classes at run time. Wrapper classes represent primitive types as objects. Math class provides mathematical functions. String and String. Buffer classes provide operations on strings. • System classes provide system operations. • Throwable class represents errors and exceptions. • java. lang is implicitly imported in every Java source file. 4
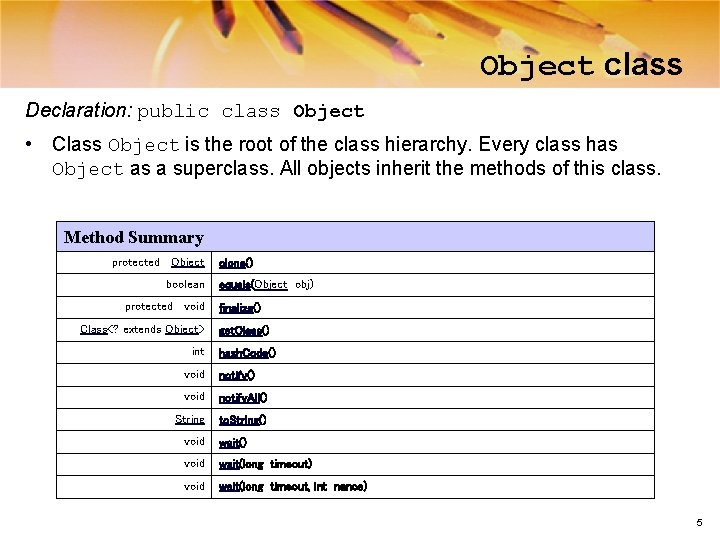
Object class Declaration: public class Object • Class Object is the root of the class hierarchy. Every class has Object as a superclass. All objects inherit the methods of this class. Method Summary protected Object boolean protected void Class<? extends Object> int clone() equals(Object obj) finalize() get. Class() hash. Code() void notify. All() String to. String() void wait(long timeout) void wait(long timeout, int nanos) 5
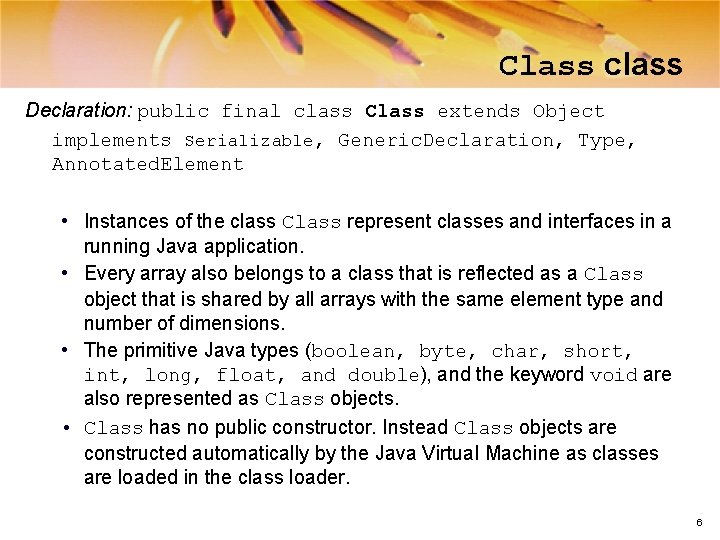
Class class Declaration: public final class Class extends Object implements Serializable, Generic. Declaration, Type, Annotated. Element • Instances of the class Class represent classes and interfaces in a running Java application. • Every array also belongs to a class that is reflected as a Class object that is shared by all arrays with the same element type and number of dimensions. • The primitive Java types (boolean, byte, char, short, int, long, float, and double), and the keyword void are also represented as Class objects. • Class has no public constructor. Instead Class objects are constructed automatically by the Java Virtual Machine as classes are loaded in the class loader. 6
![Class class Method Summary Partial List static Class Constructor Field Class for NameString class Class class Method Summary (Partial List) static Class[] Constructor[] Field[] Class[] for. Name(String class.](https://slidetodoc.com/presentation_image/2bd8aa05d2bb9ffa8c9404c218a3acb2/image-7.jpg)
Class class Method Summary (Partial List) static Class[] Constructor[] Field[] Class[] for. Name(String class. Name) get. Classes() get. Constructors() get. Fields() get. Interfaces() Method[] get. Methods() int get. Modifiers() String Package get. Name() get. Package() String get. Simple. Name() Class get. Superclass() boolean is. Array() boolean is. Instance(Object obj) boolean is. Interface() boolean is. Local. Class() boolean is. Member. Class() boolean is. Primitive() 7
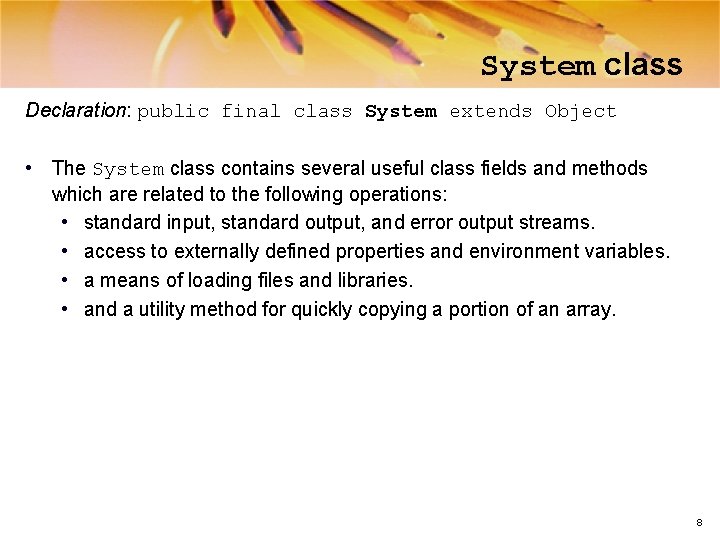
System class Declaration: public final class System extends Object • The System class contains several useful class fields and methods which are related to the following operations: • standard input, standard output, and error output streams. • access to externally defined properties and environment variables. • a means of loading files and libraries. • and a utility method for quickly copying a portion of an array. 8
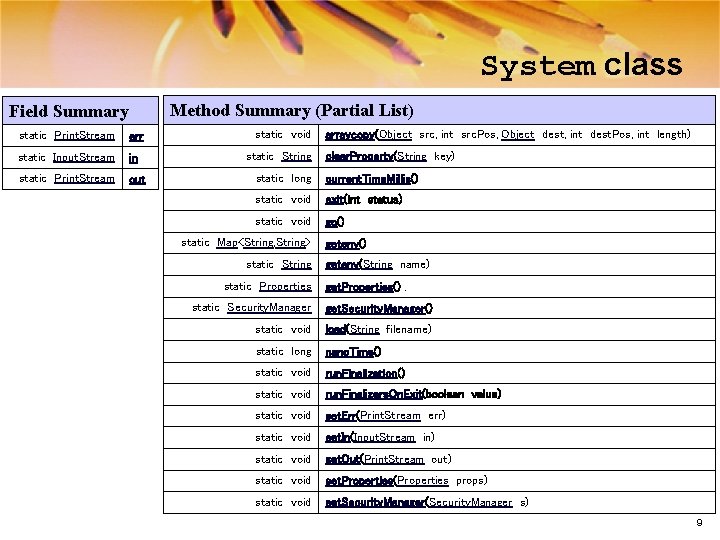
System class Method Summary (Partial List) Field Summary static Print. Stream err static Input. Stream in static Print. Stream out static void static String arraycopy(Object src, int src. Pos, Object dest, int dest. Pos, int length) clear. Property(String key) static long current. Time. Millis() static void exit(int status) static void gc() static Map<String, String> static String static Properties getenv() getenv(String name) get. Properties(). static Security. Manager get. Security. Manager() static void load(String filename) static long nano. Time() static void run. Finalization() static void run. Finalizers. On. Exit(boolean value) static void set. Err(Print. Stream err) static void set. In(Input. Stream in) static void set. Out(Print. Stream out) static void set. Properties(Properties props) static void set. Security. Manager(Security. Manager s) 9
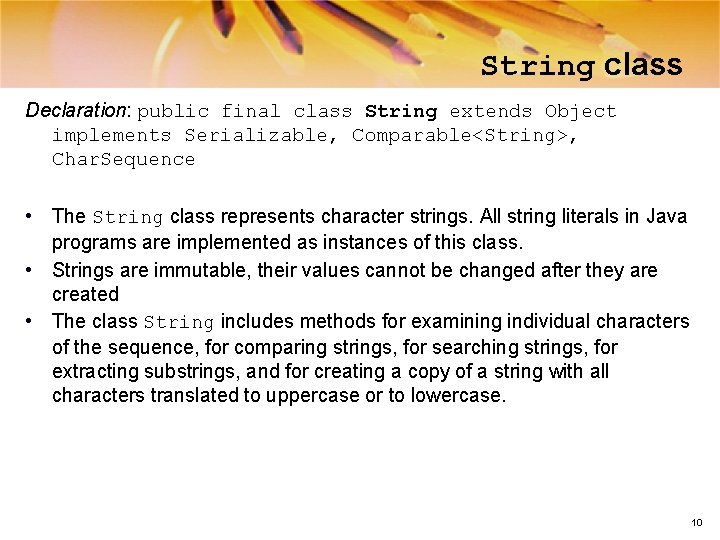
String class Declaration: public final class String extends Object implements Serializable, Comparable<String>, Char. Sequence • The String class represents character strings. All string literals in Java programs are implemented as instances of this class. • Strings are immutable, their values cannot be changed after they are created • The class String includes methods for examining individual characters of the sequence, for comparing strings, for searching strings, for extracting substrings, and for creating a copy of a string with all characters translated to uppercase or to lowercase. 10
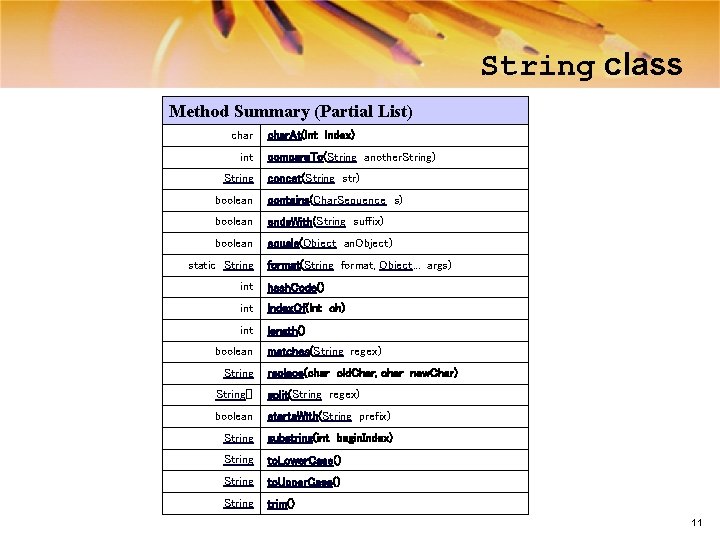
String class Method Summary (Partial List) char int String char. At(int index) compare. To(String another. String) concat(String str) boolean contains(Char. Sequence s) boolean ends. With(String suffix) boolean equals(Object an. Object) static String format(String format, Object. . . args) int hash. Code() int index. Of(int ch) int length() boolean String matches(String regex) replace(char old. Char, char new. Char) String[] split(String regex) boolean starts. With(String prefix) String substring(int begin. Index) String to. Lower. Case() String to. Upper. Case() String trim() 11
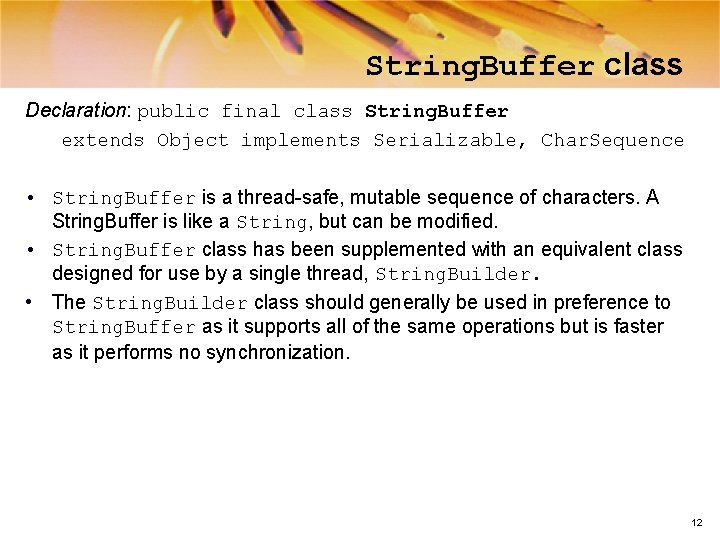
String. Buffer class Declaration: public final class String. Buffer extends Object implements Serializable, Char. Sequence • String. Buffer is a thread-safe, mutable sequence of characters. A String. Buffer is like a String, but can be modified. • String. Buffer class has been supplemented with an equivalent class designed for use by a single thread, String. Builder. • The String. Builder class should generally be used in preference to String. Buffer as it supports all of the same operations but is faster as it performs no synchronization. 12
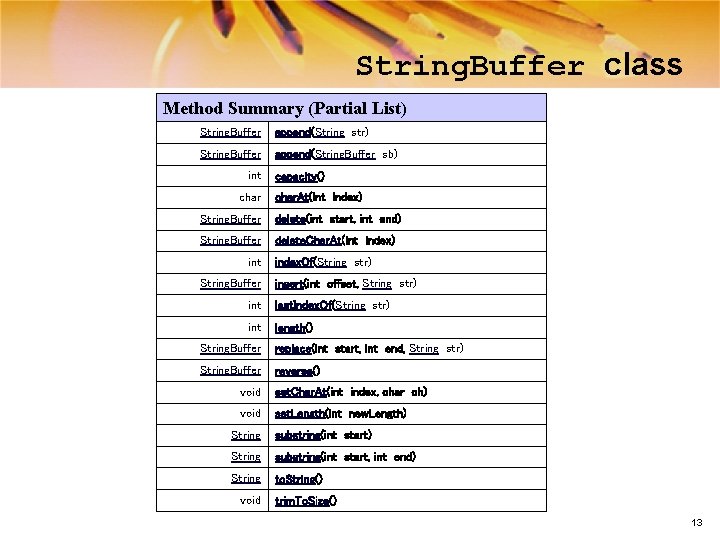
String. Buffer class Method Summary (Partial List) String. Buffer append(String str) String. Buffer append(String. Buffer sb) int char capacity() char. At(int index) String. Buffer delete(int start, int end) String. Buffer delete. Char. At(int index) int String. Buffer index. Of(String str) insert(int offset, String str) int last. Index. Of(String str) int length() String. Buffer replace(int start, int end, String str) String. Buffer reverse() void set. Char. At(int index, char ch) void set. Length(int new. Length) String substring(int start, int end) String to. String() void trim. To. Size() 13
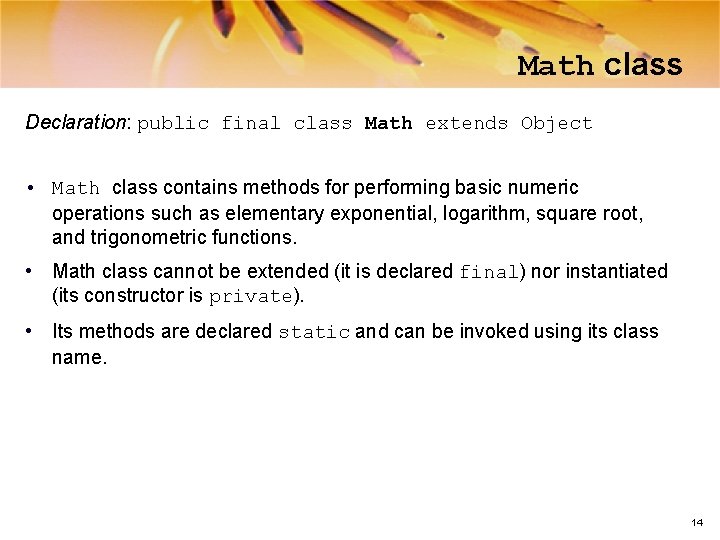
Math class Declaration: public final class Math extends Object • Math class contains methods for performing basic numeric operations such as elementary exponential, logarithm, square root, and trigonometric functions. • Math class cannot be extended (it is declared final) nor instantiated (its constructor is private). • Its methods are declared static and can be invoked using its class name. 14
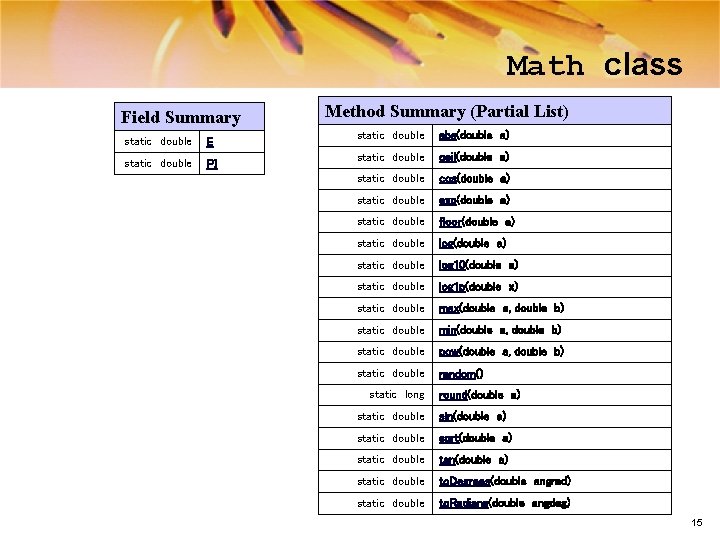
Math class Field Summary static double E static double PI Method Summary (Partial List) static double abs(double a) static double ceil(double a) static double cos(double a) static double exp(double a) static double floor(double a) static double log 10(double a) static double log 1 p(double x) static double max(double a, double b) static double min(double a, double b) static double pow(double a, double b) static double random() static long round(double a) static double sin(double a) static double sqrt(double a) static double tan(double a) static double to. Degrees(double angrad) static double to. Radians(double angdeg) 15
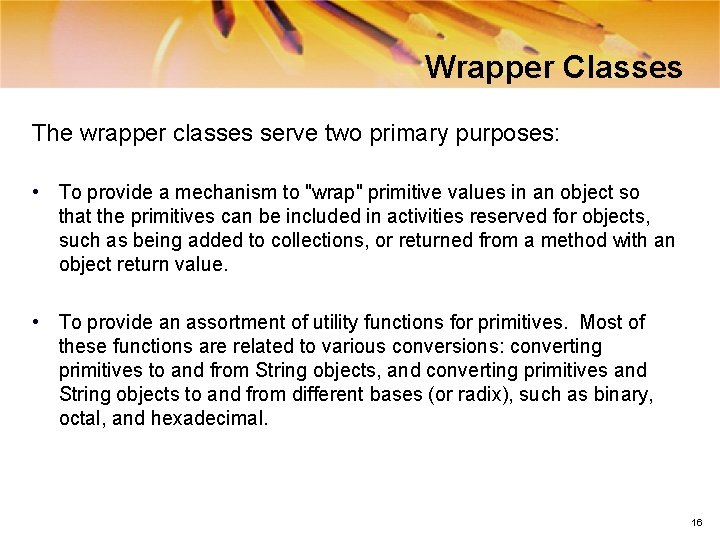
Wrapper Classes The wrapper classes serve two primary purposes: • To provide a mechanism to "wrap" primitive values in an object so that the primitives can be included in activities reserved for objects, such as being added to collections, or returned from a method with an object return value. • To provide an assortment of utility functions for primitives. Most of these functions are related to various conversions: converting primitives to and from String objects, and converting primitives and String objects to and from different bases (or radix), such as binary, octal, and hexadecimal. 16
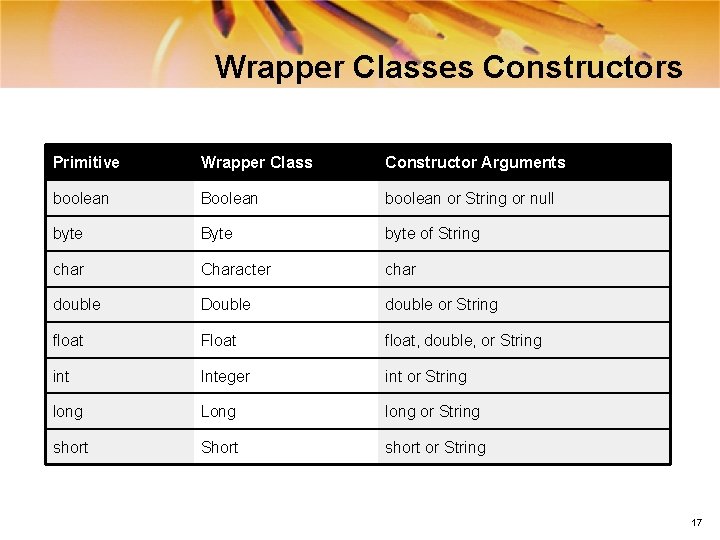
Wrapper Classes Constructors Primitive Wrapper Class Constructor Arguments boolean Boolean boolean or String or null byte Byte byte of String char Character char double Double double or String float Float float, double, or String int Integer int or String long Long long or String short Short short or String 17
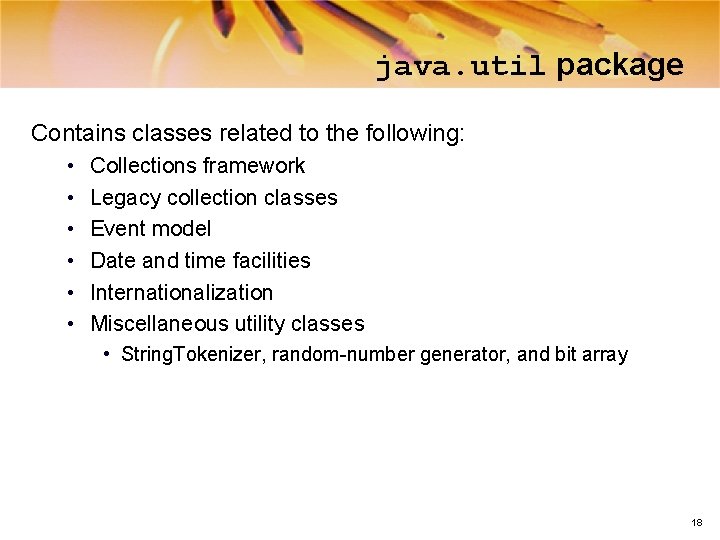
java. util package Contains classes related to the following: • • • Collections framework Legacy collection classes Event model Date and time facilities Internationalization Miscellaneous utility classes • String. Tokenizer, random-number generator, and bit array 18
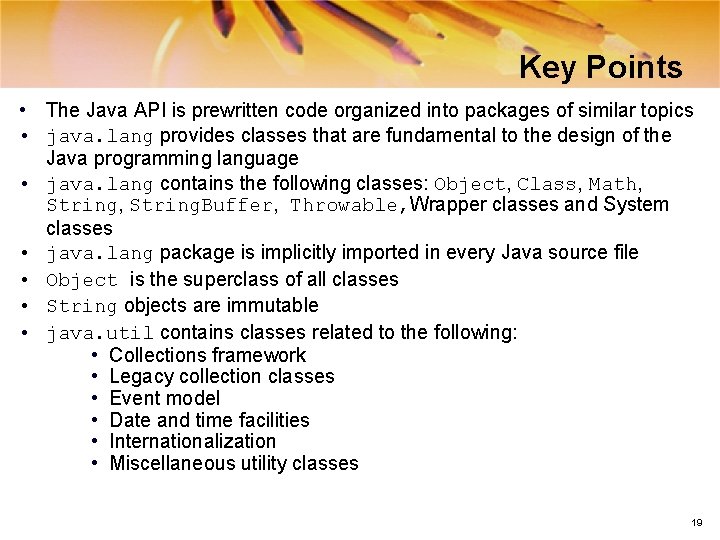
Key Points • The Java API is prewritten code organized into packages of similar topics • java. lang provides classes that are fundamental to the design of the Java programming language • java. lang contains the following classes: Object, Class, Math, String. Buffer, Throwable, Wrapper classes and System classes • java. lang package is implicitly imported in every Java source file • Object is the superclass of all classes • String objects are immutable • java. util contains classes related to the following: • Collections framework • Legacy collection classes • Event model • Date and time facilities • Internationalization • Miscellaneous utility classes 19