Concurrent Programming Without Locks Keir Fraser Tim Harris
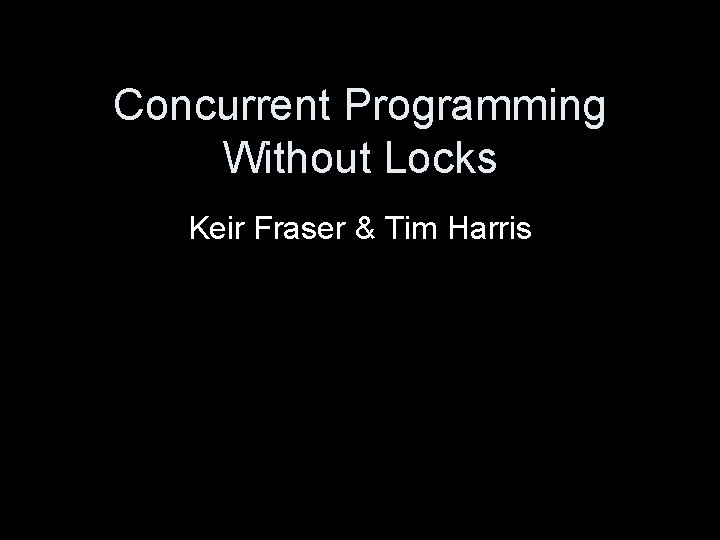
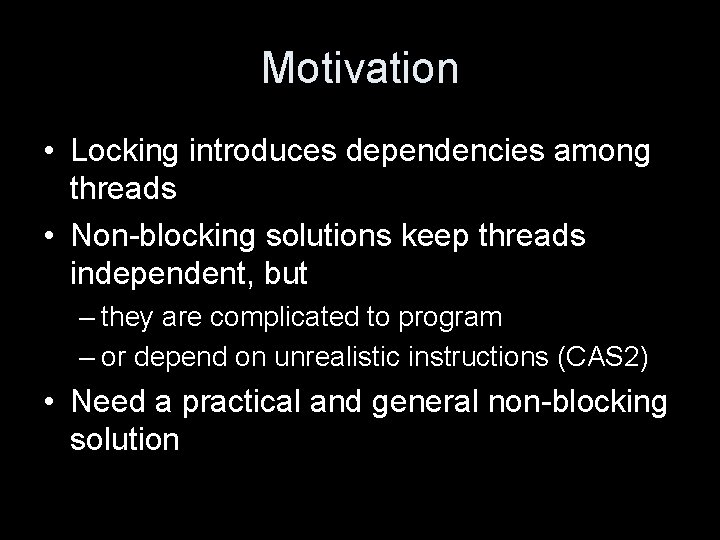
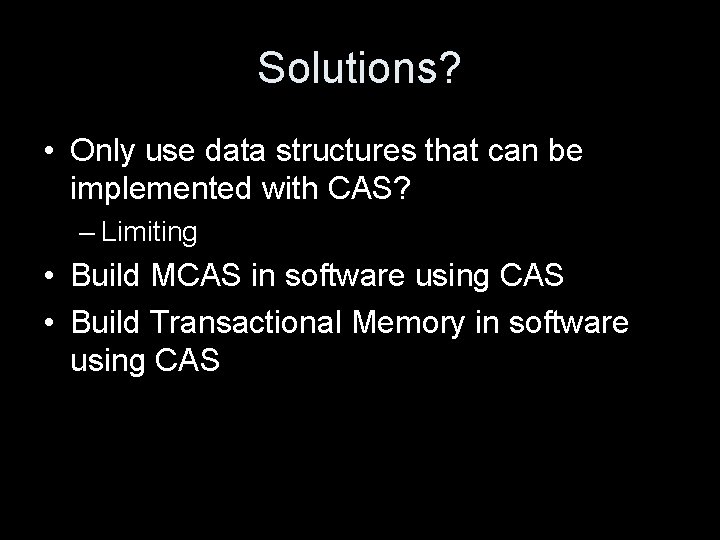
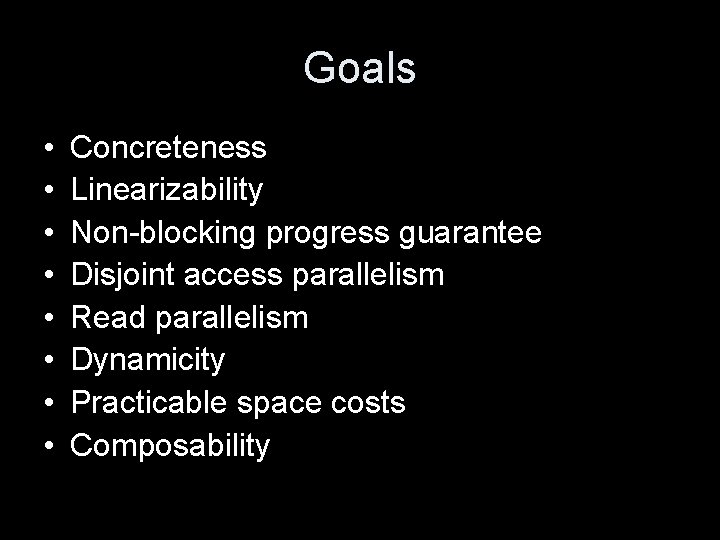
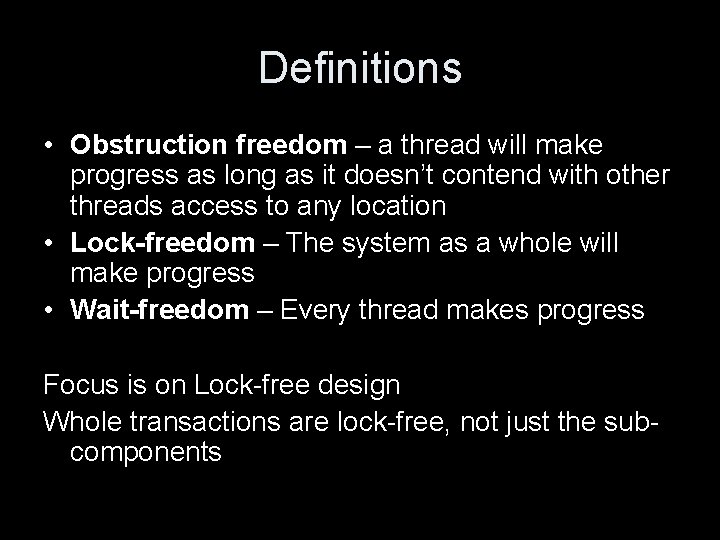
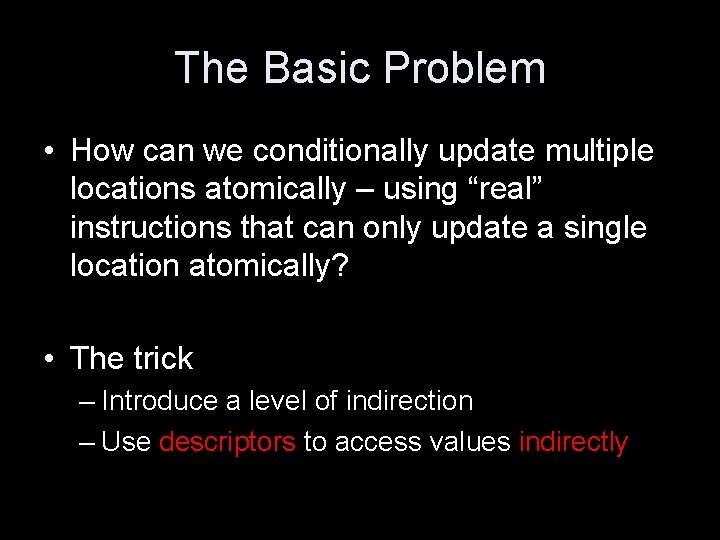
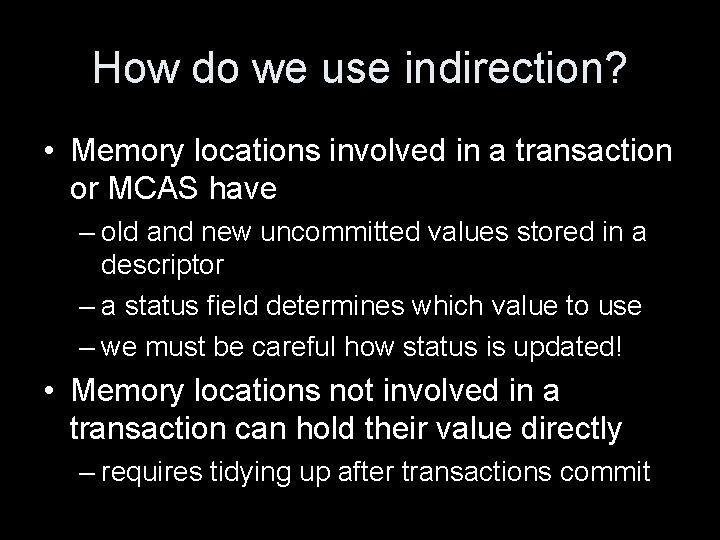
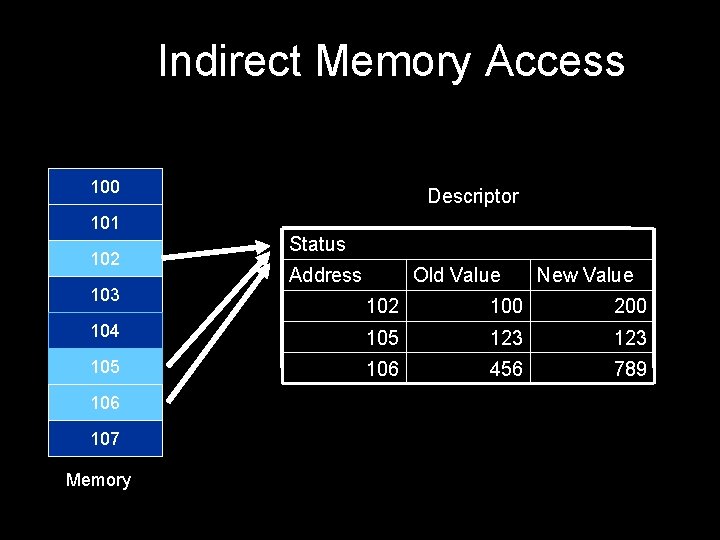
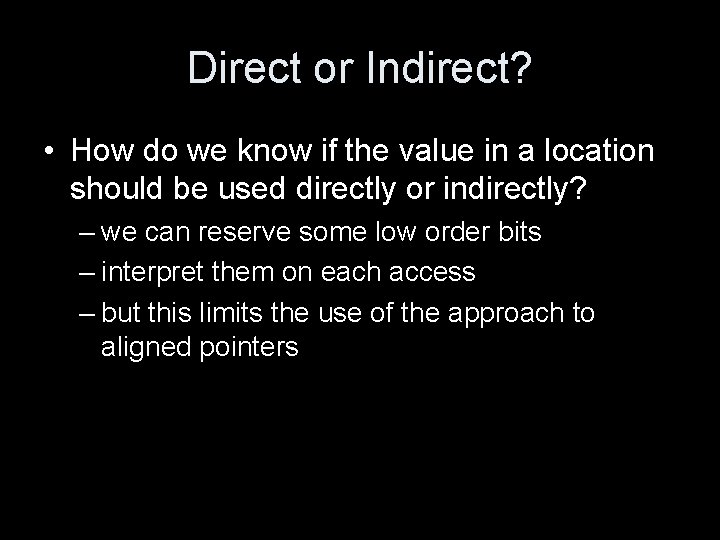
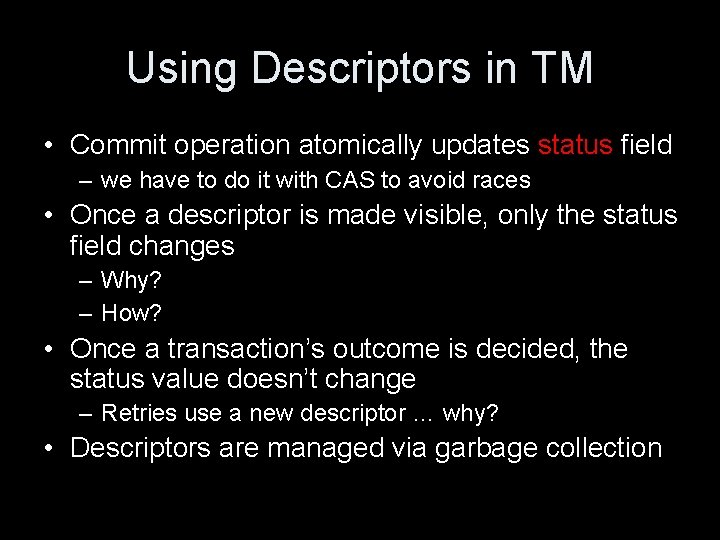
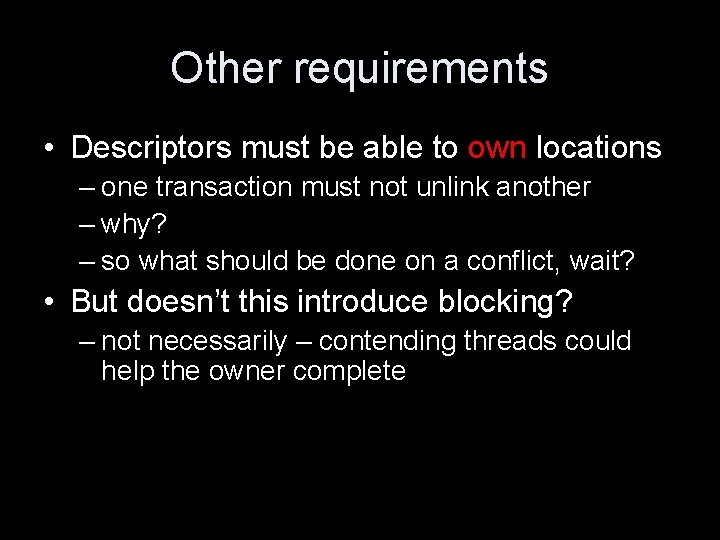
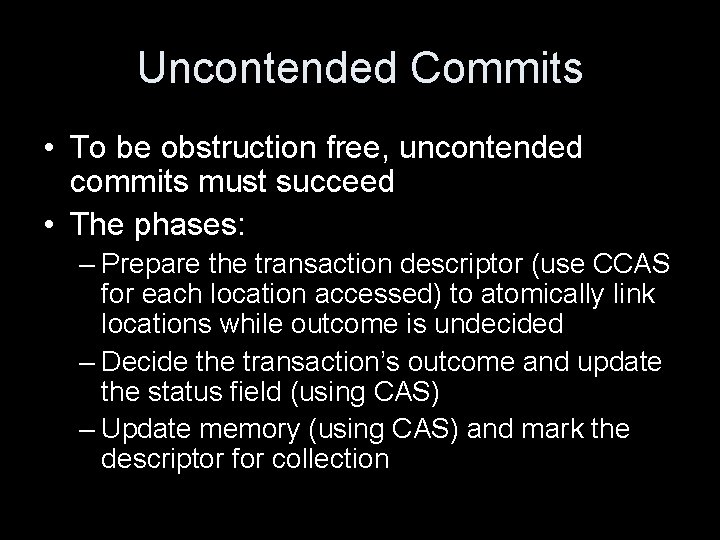
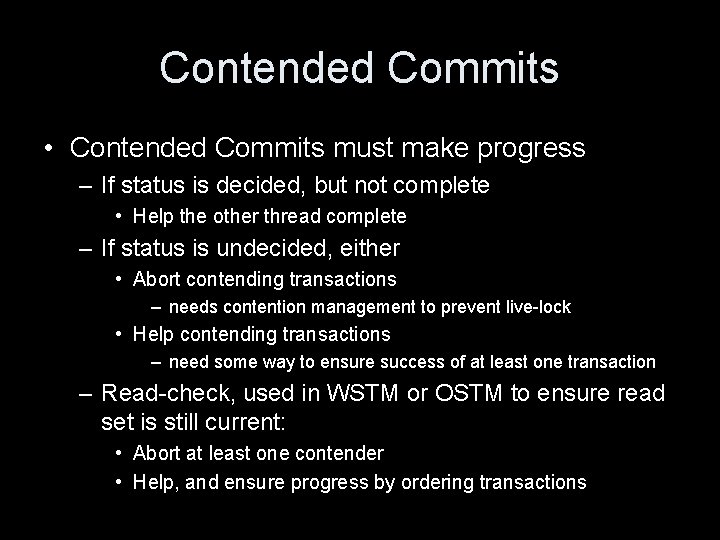
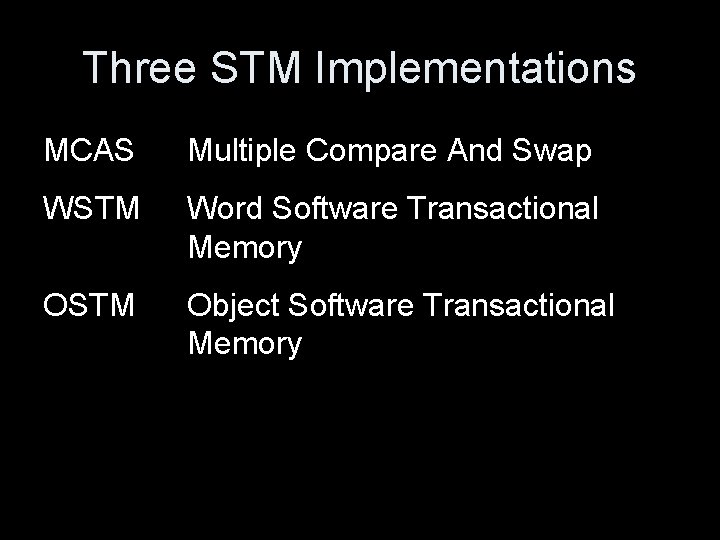
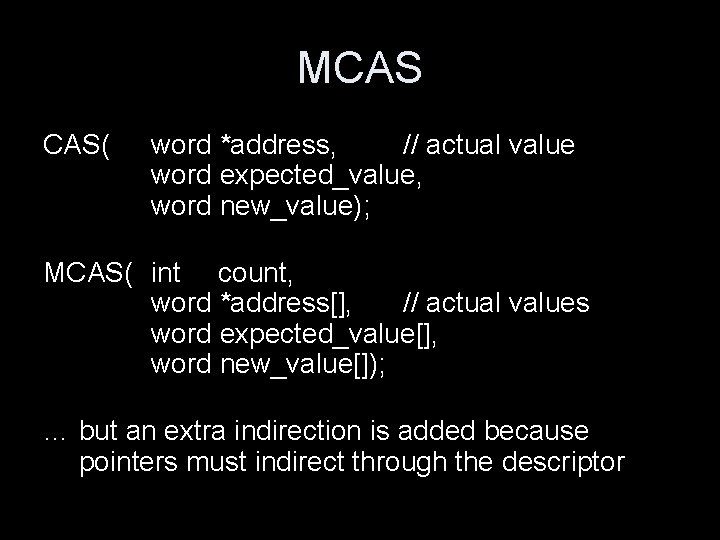
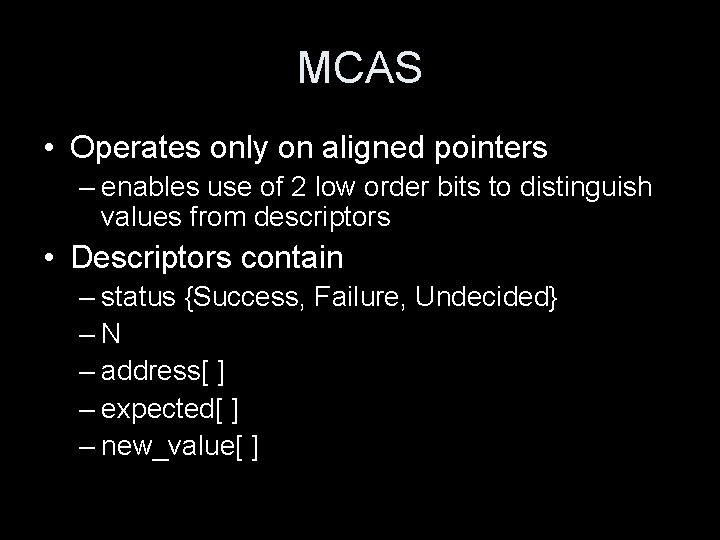
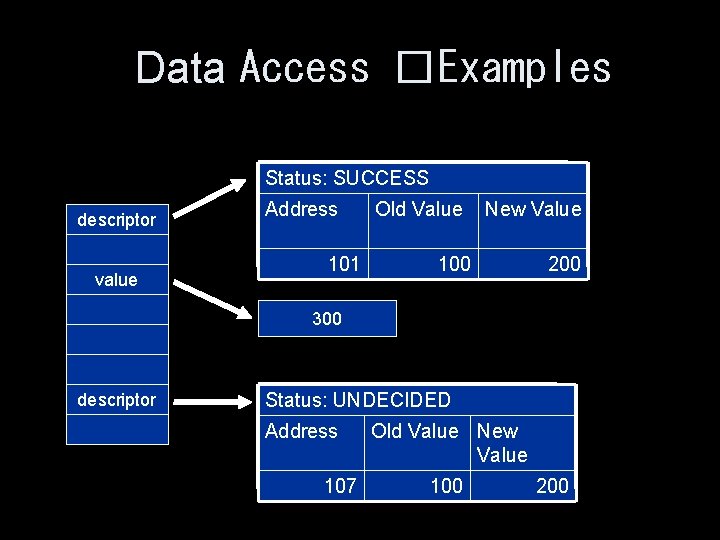
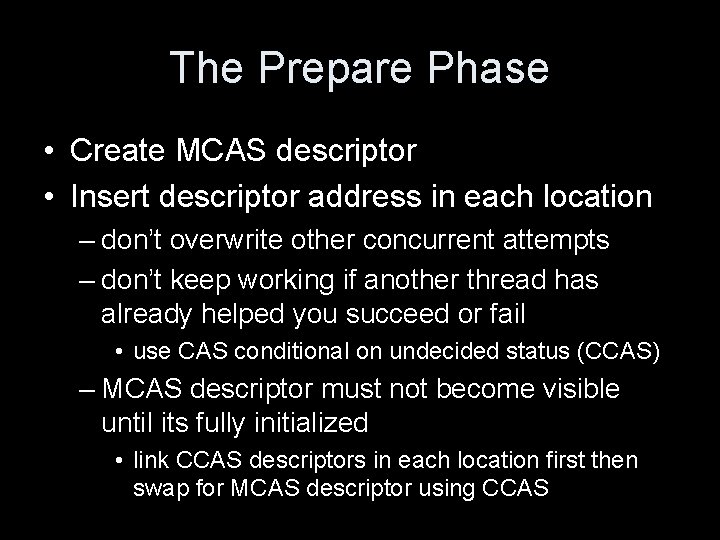
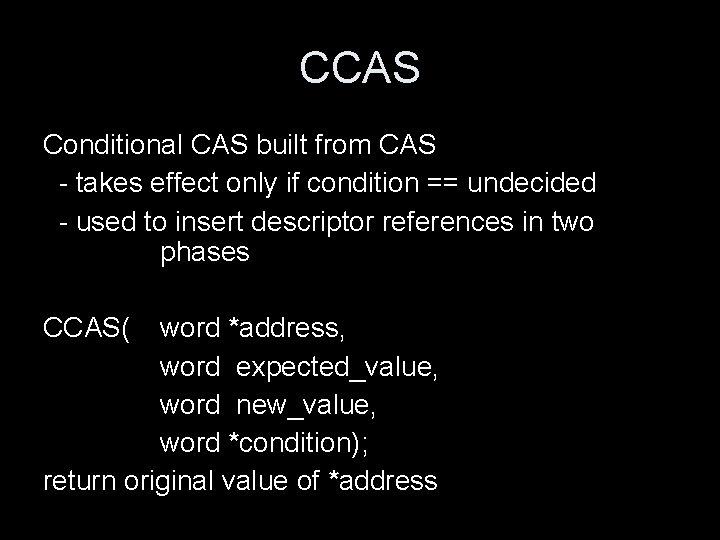
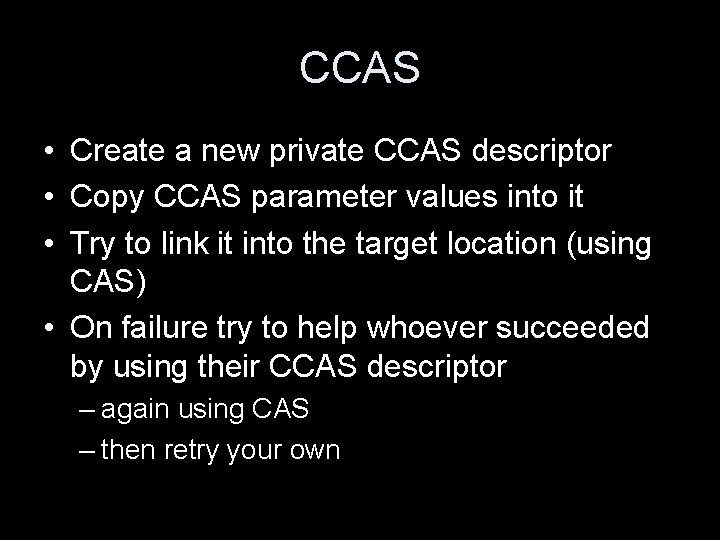
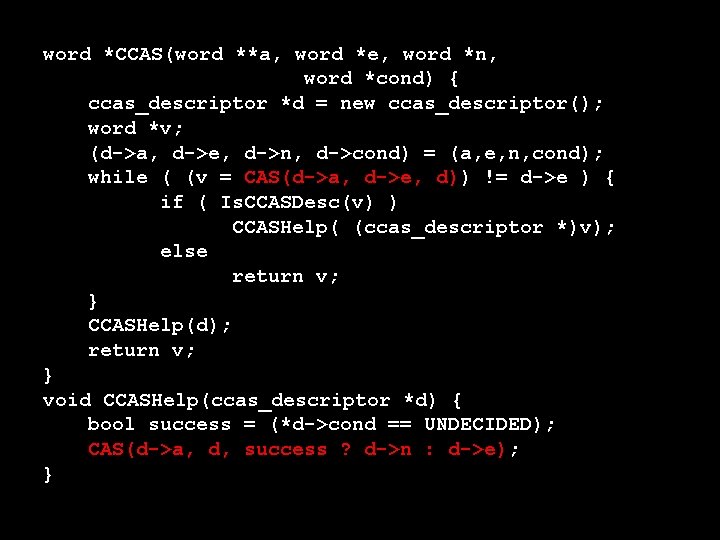
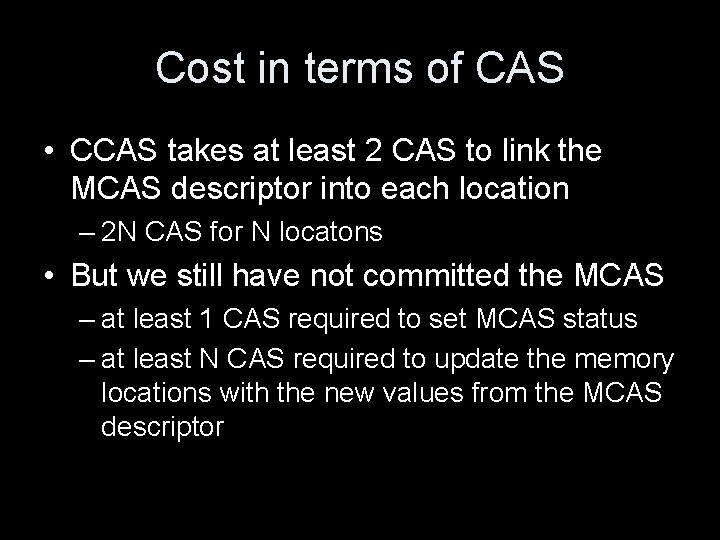
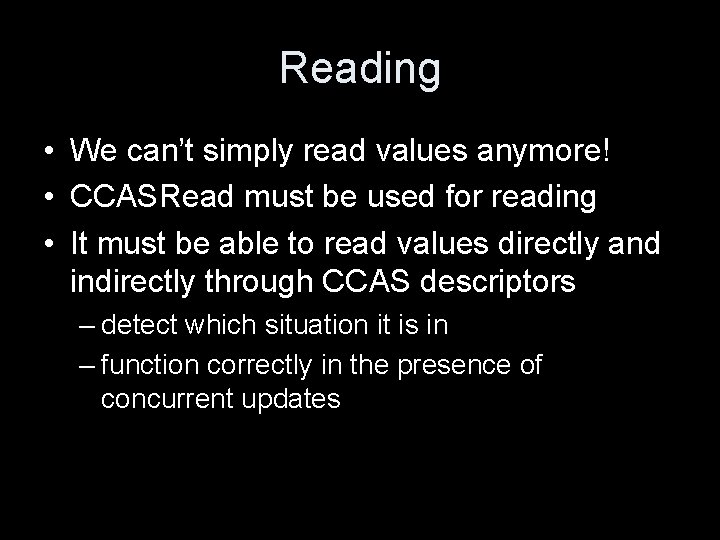
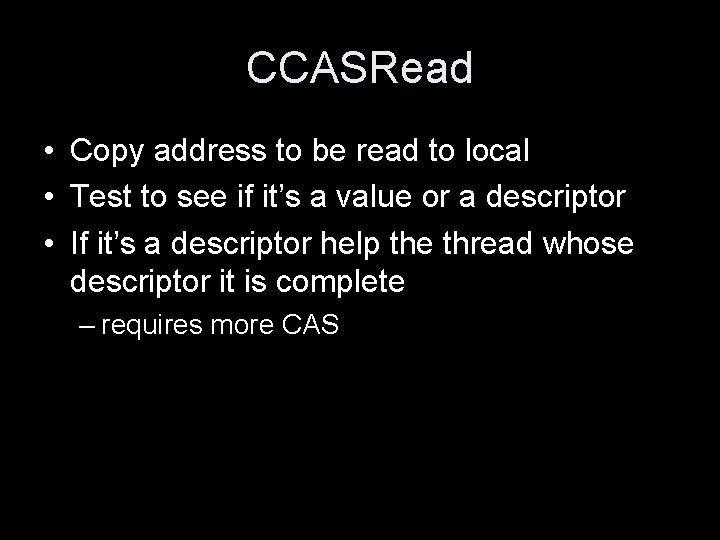
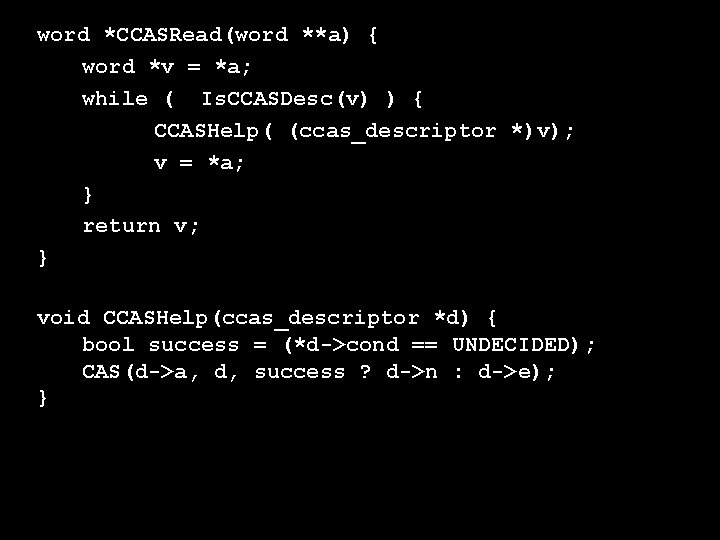
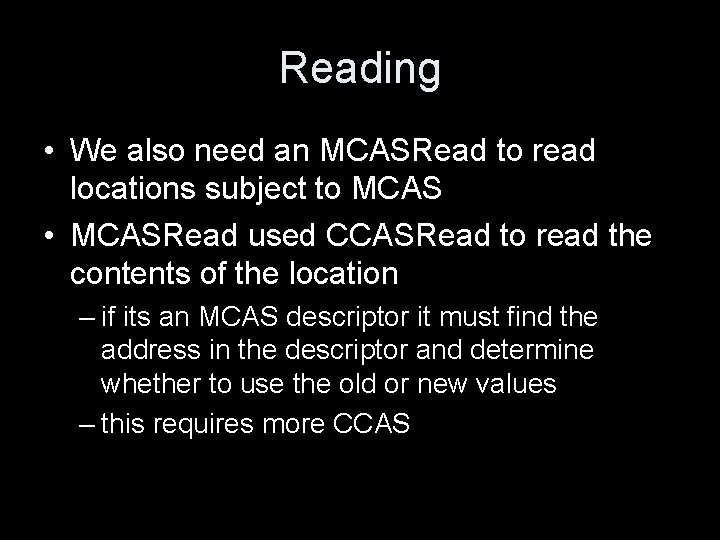
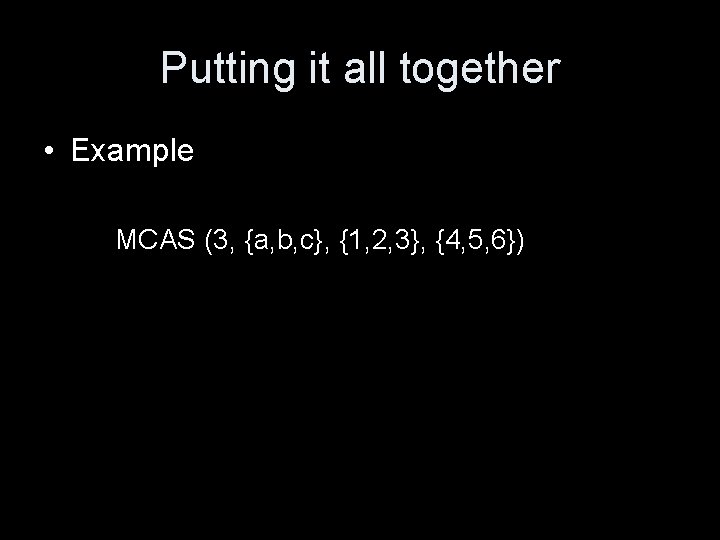
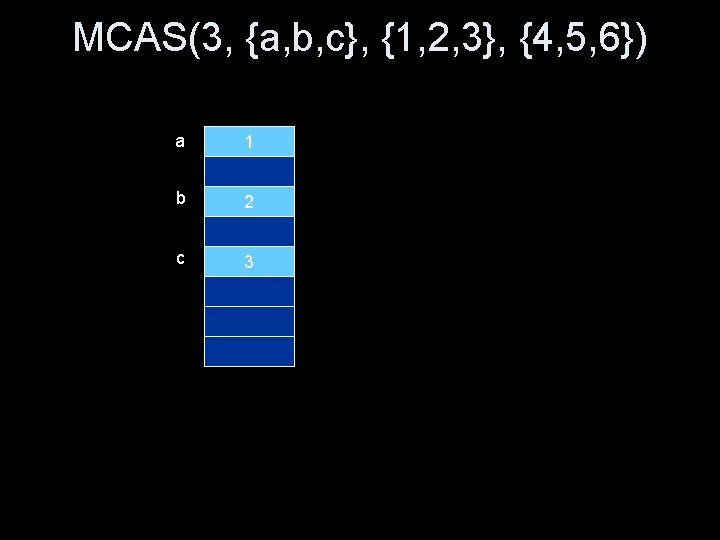
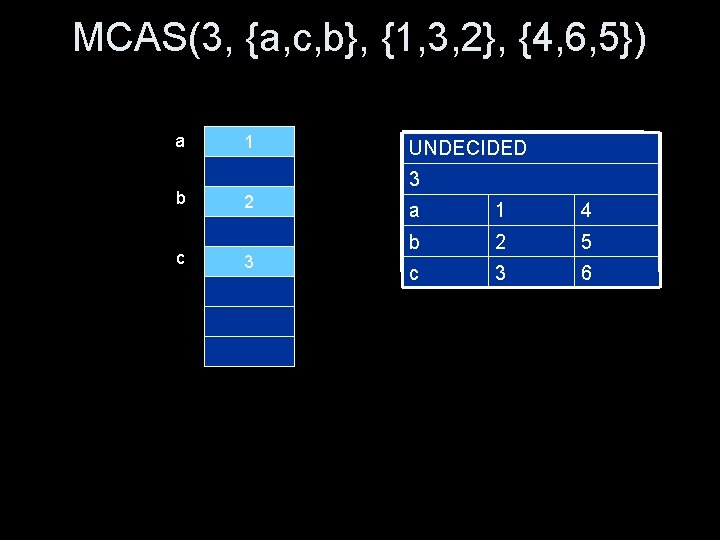
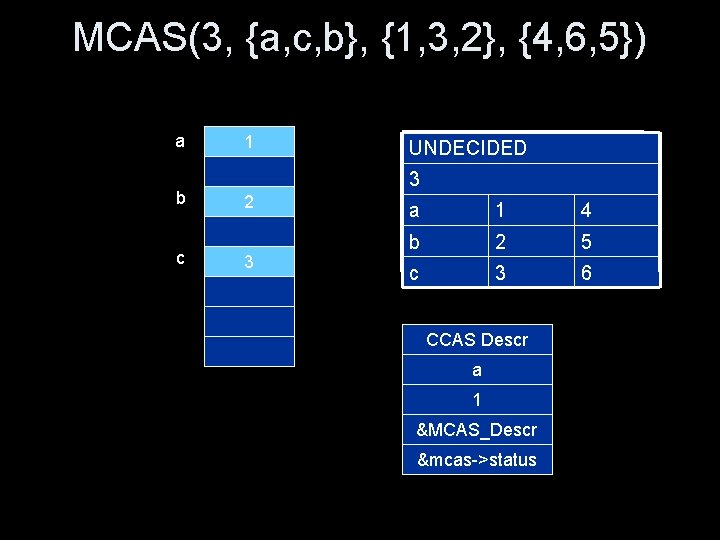
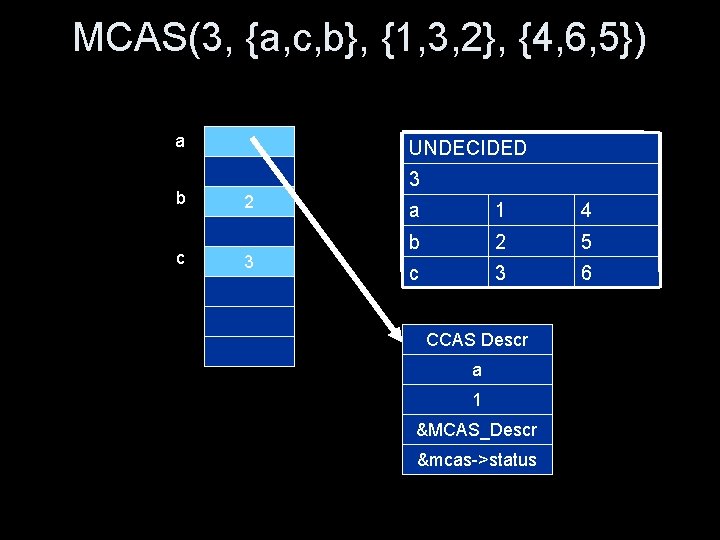
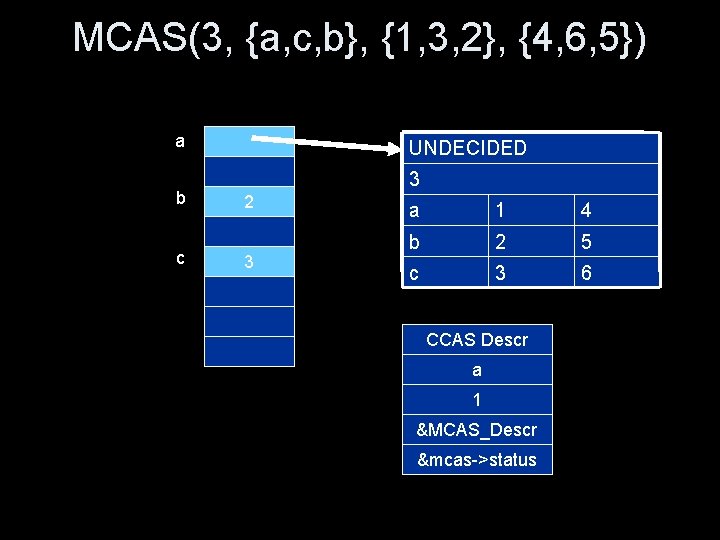
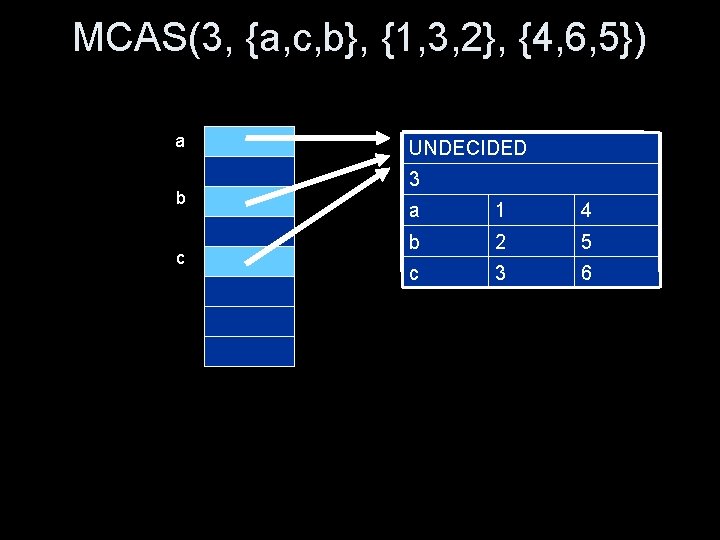
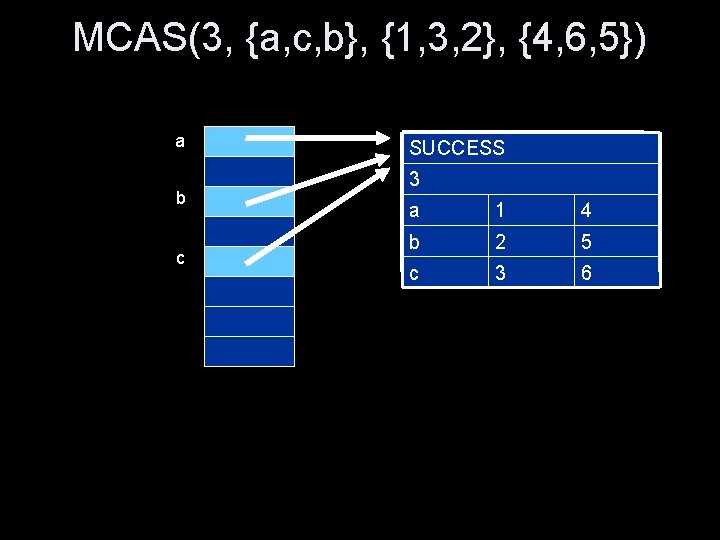
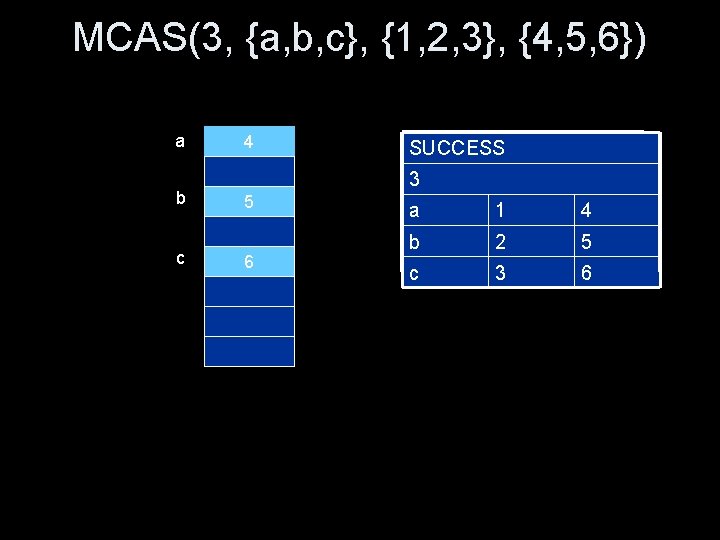
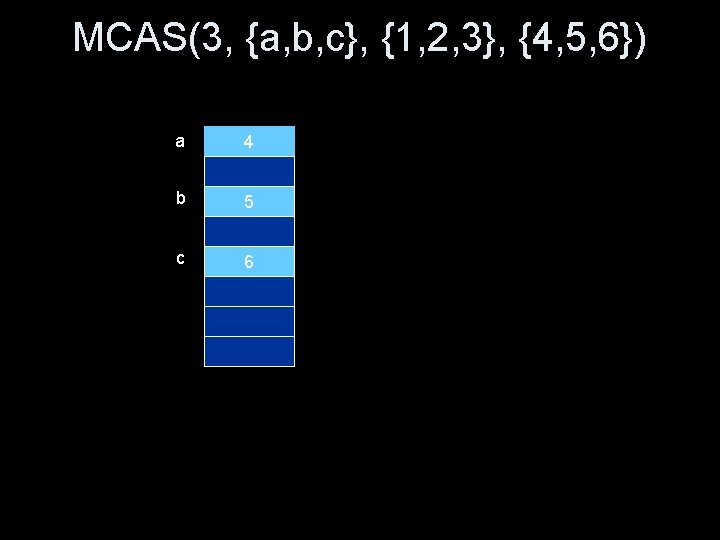
![bool MCAS(int N, word **a[], word *e[], word *n[]) { mcas_descriptor *d = new bool MCAS(int N, word **a[], word *e[], word *n[]) { mcas_descriptor *d = new](https://slidetodoc.com/presentation_image/4533dd76dbf4131d08d3af8bbf270b53/image-37.jpg)
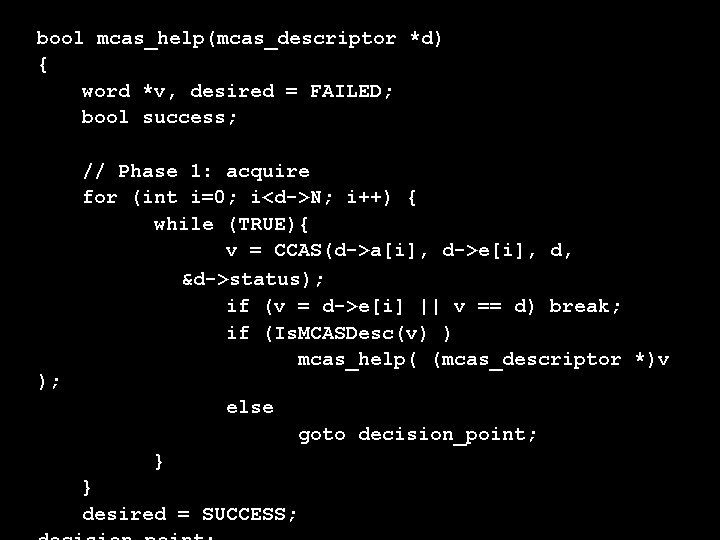
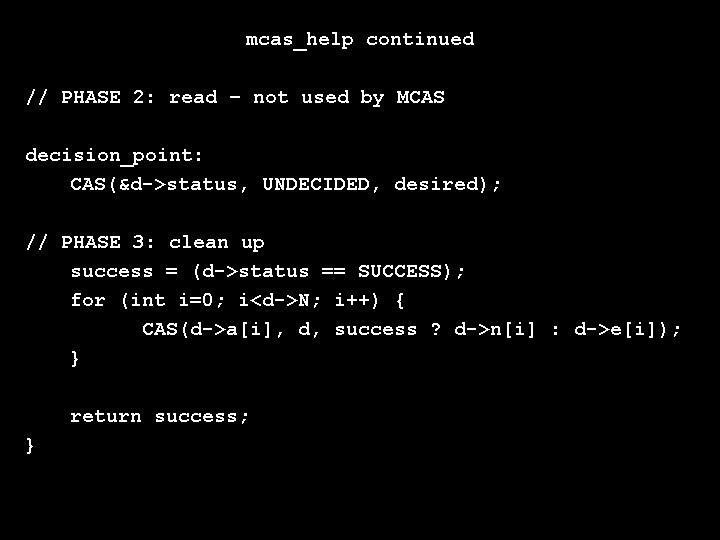
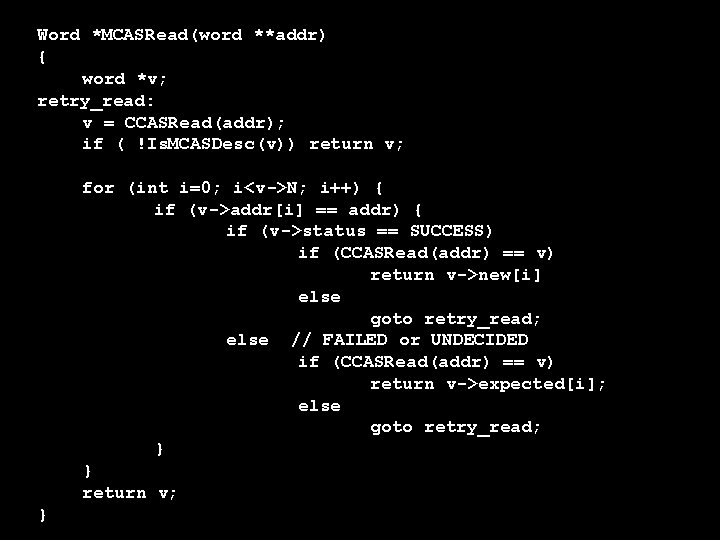
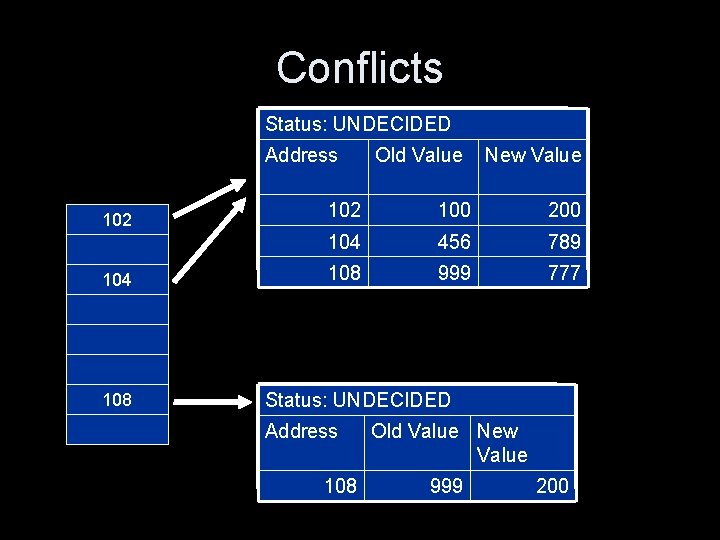
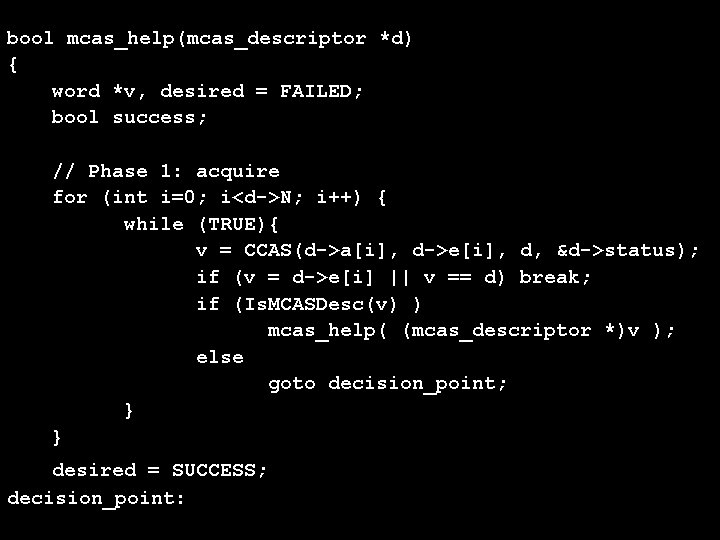
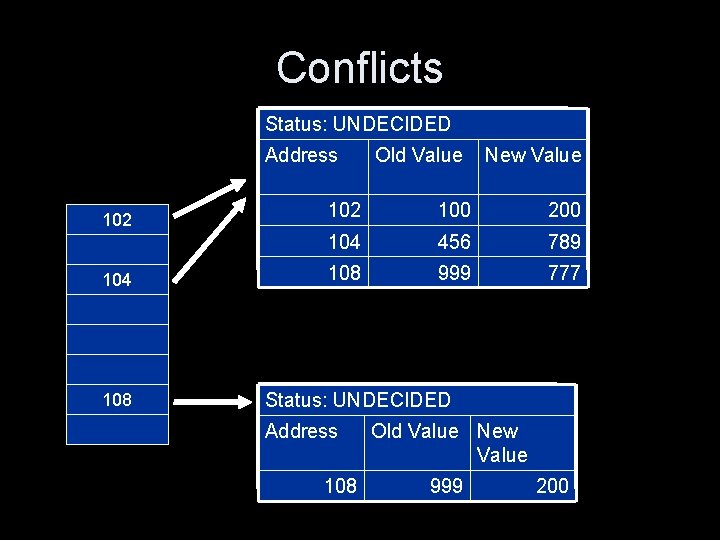
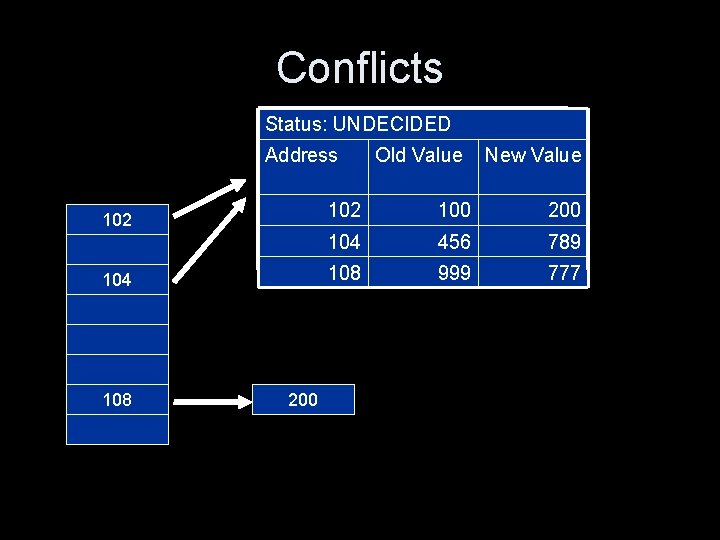
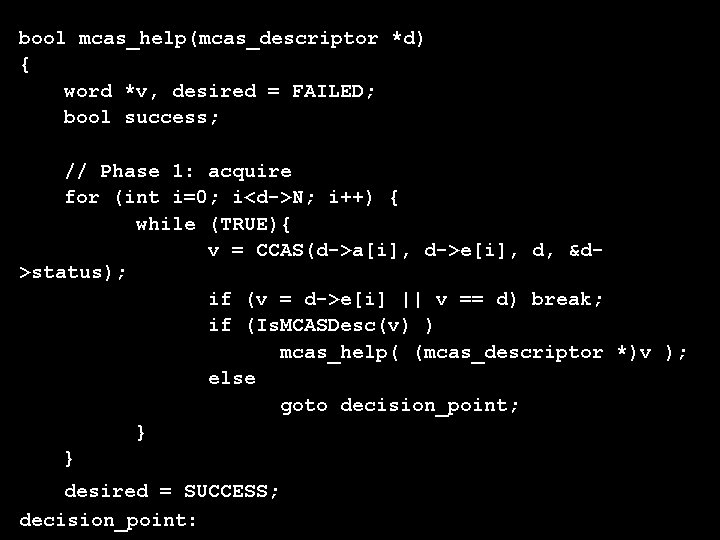
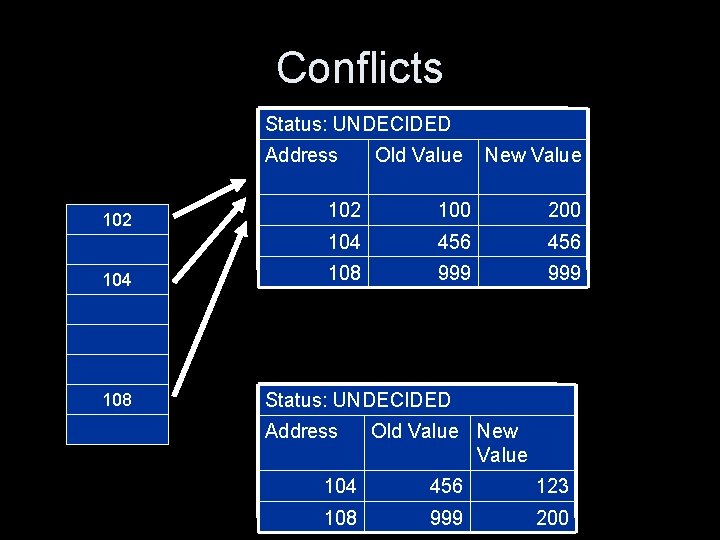
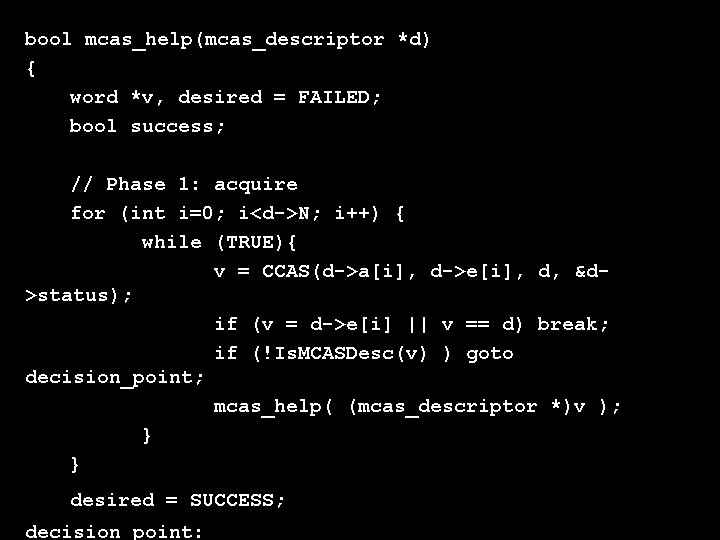
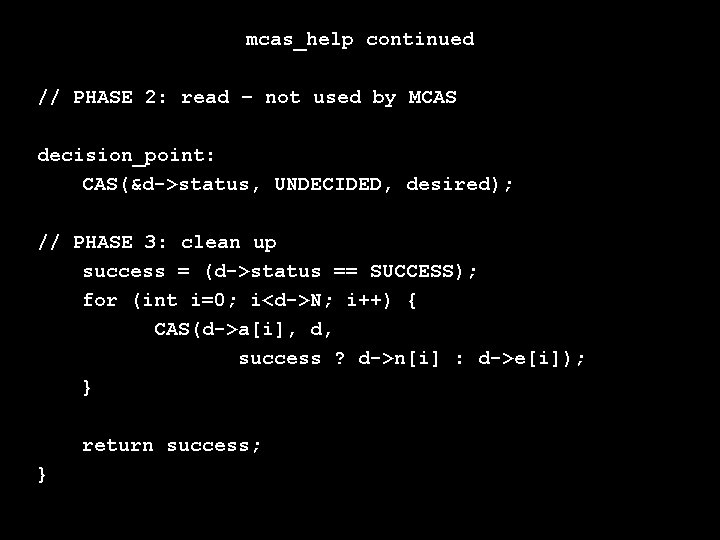
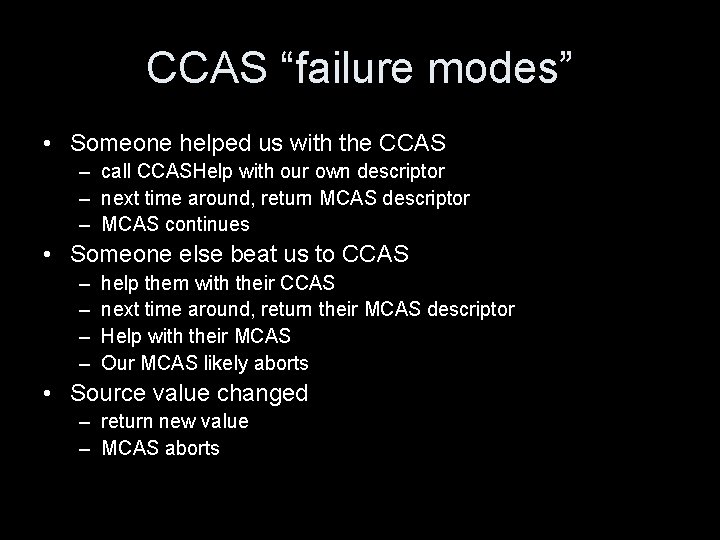
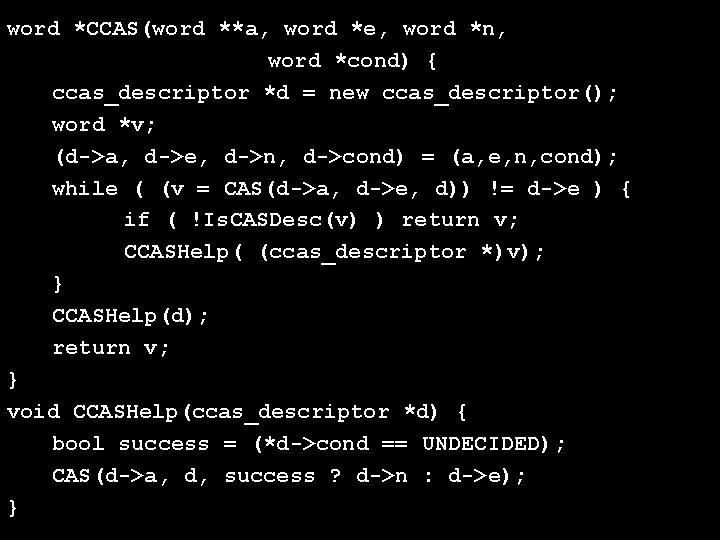
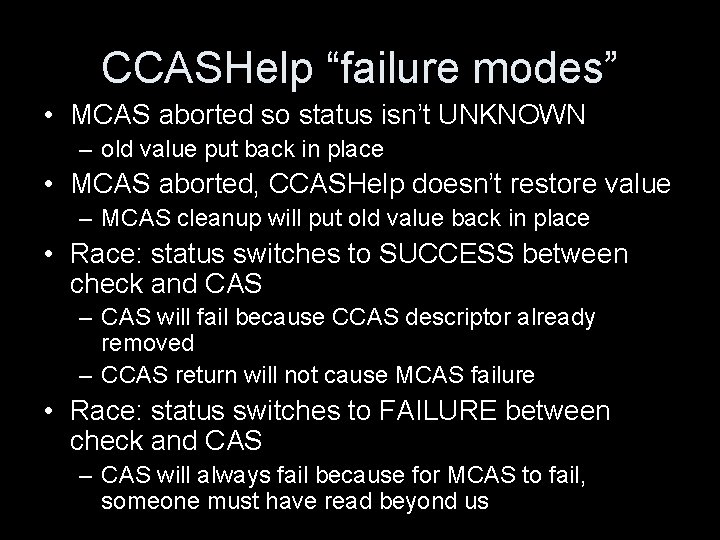
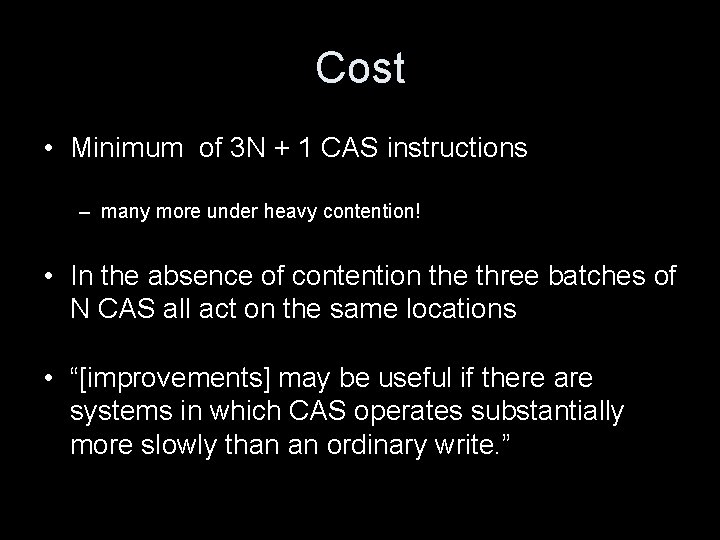
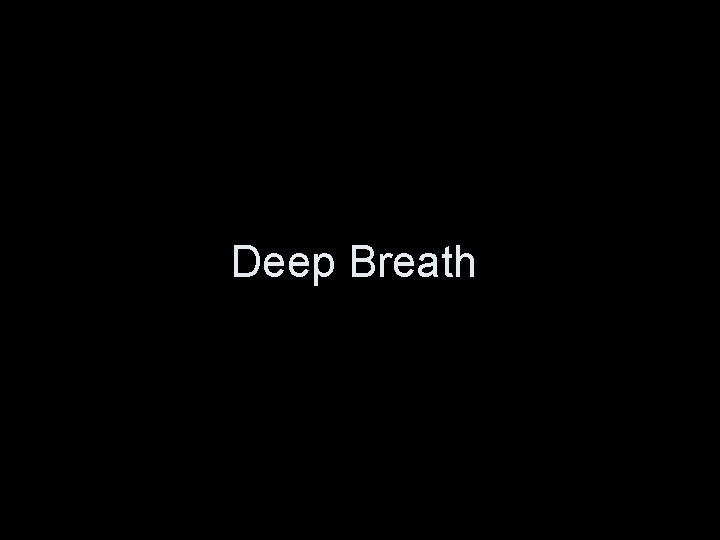
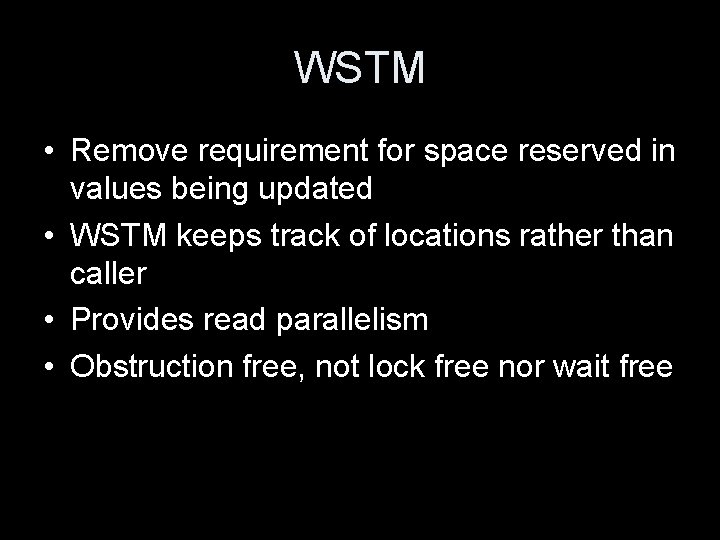
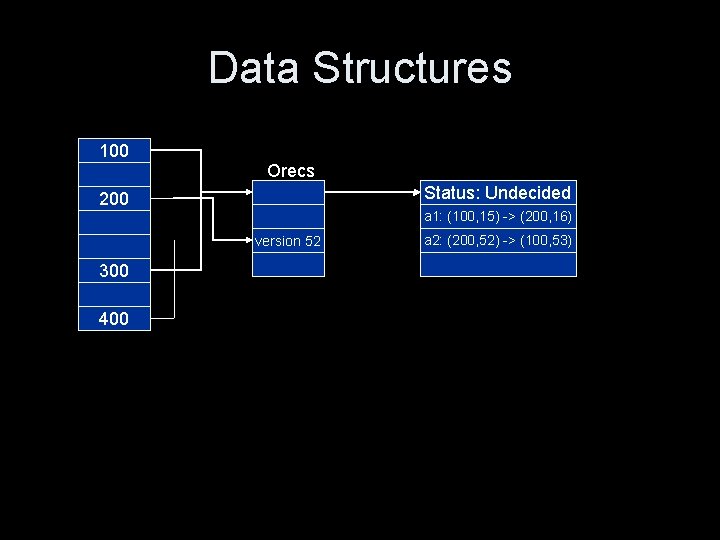
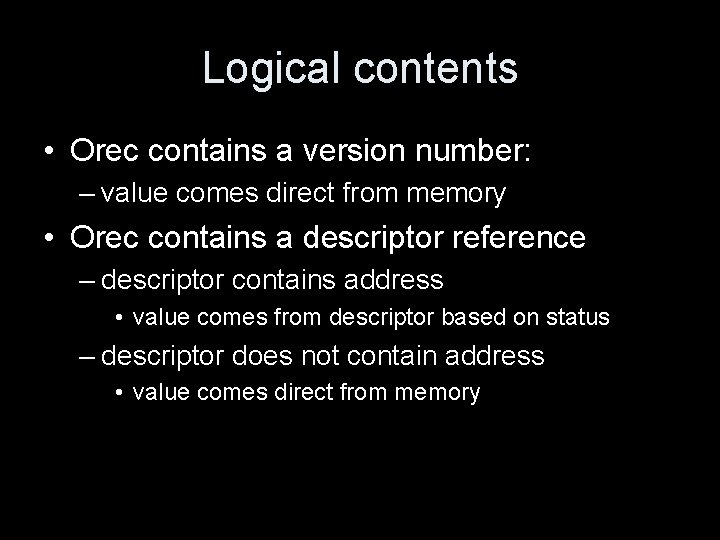
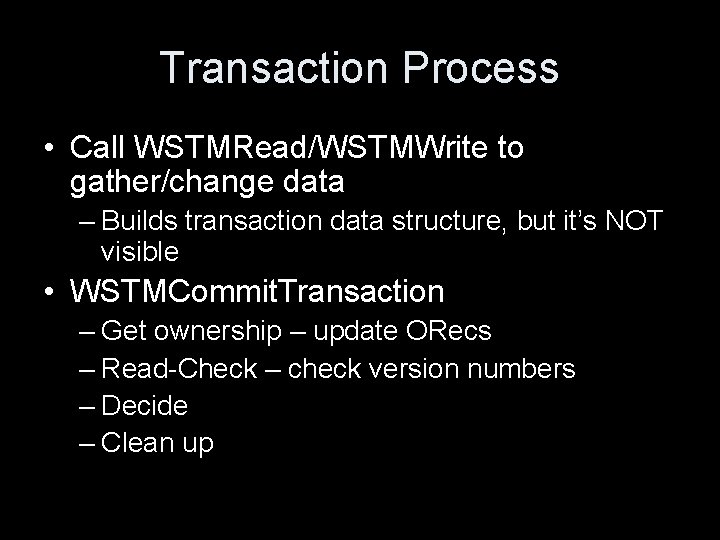
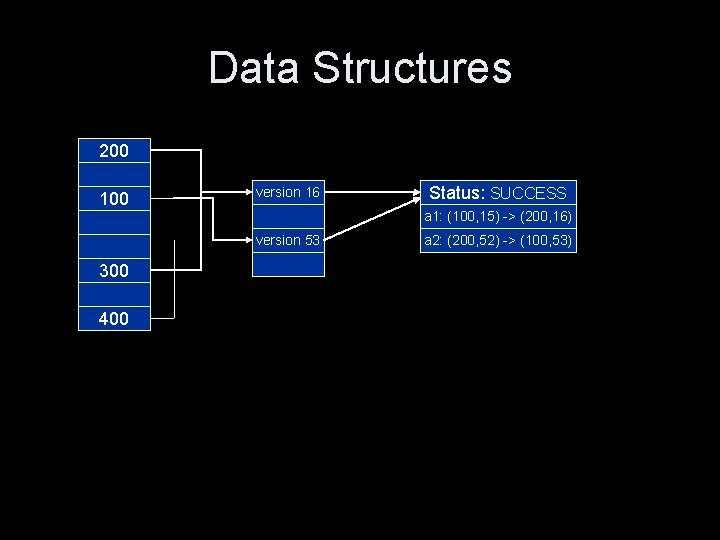
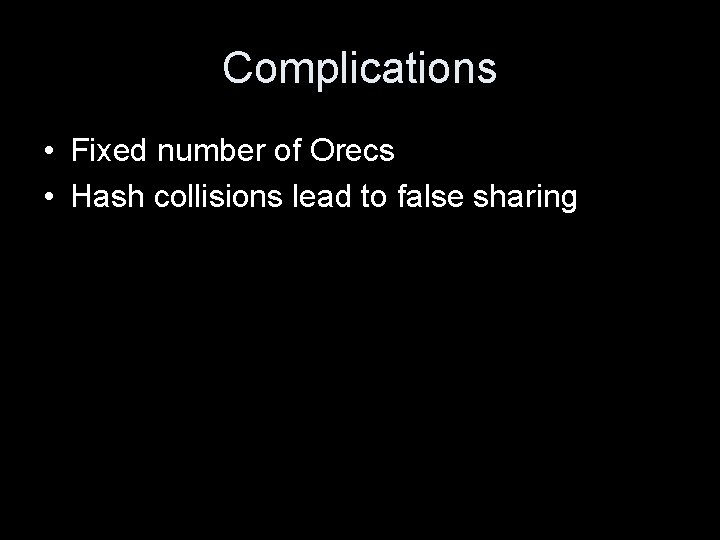
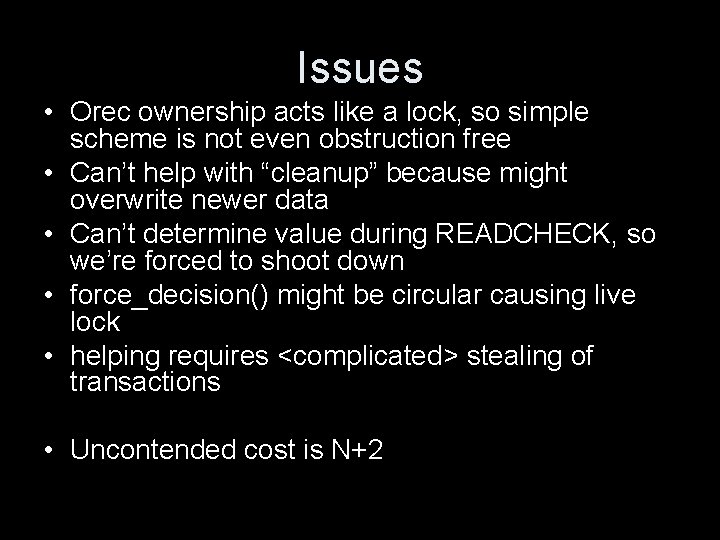
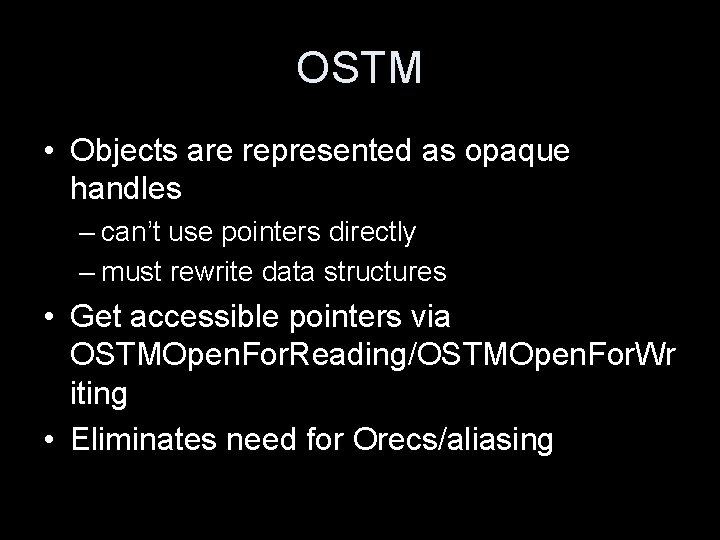
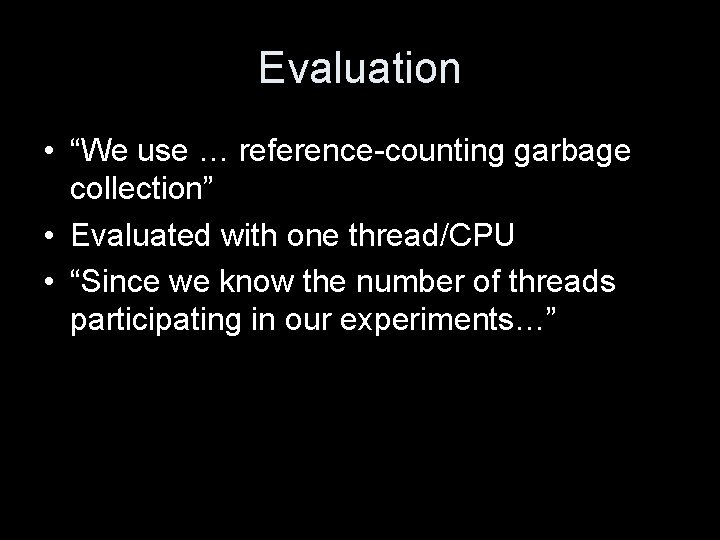
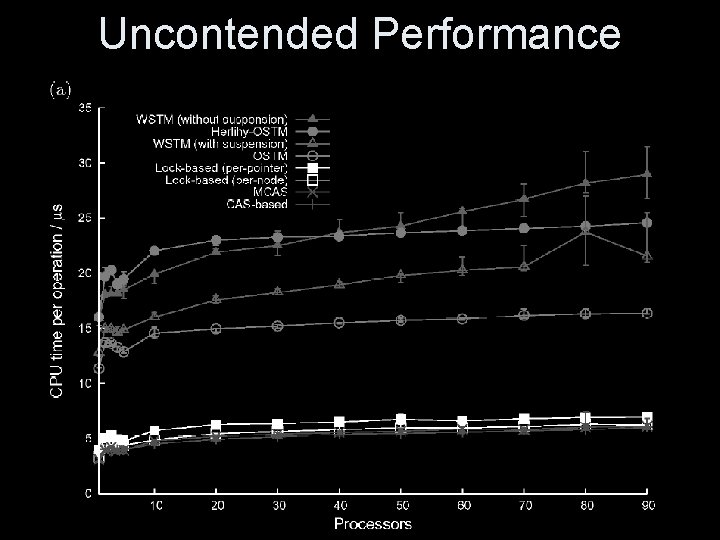
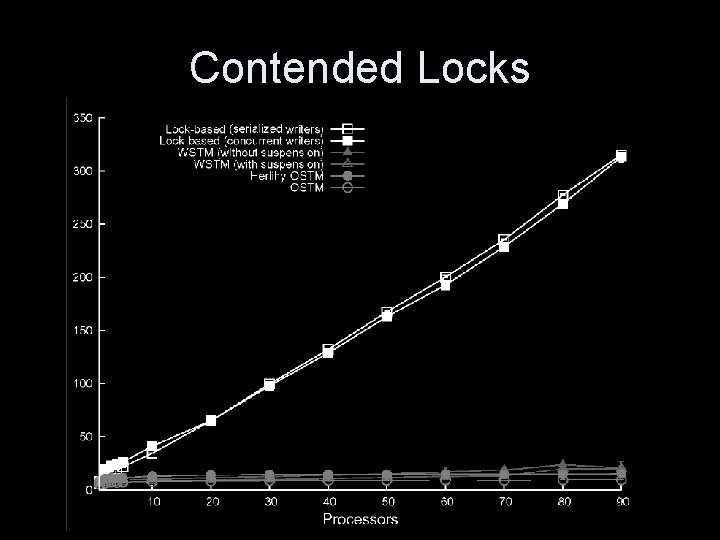
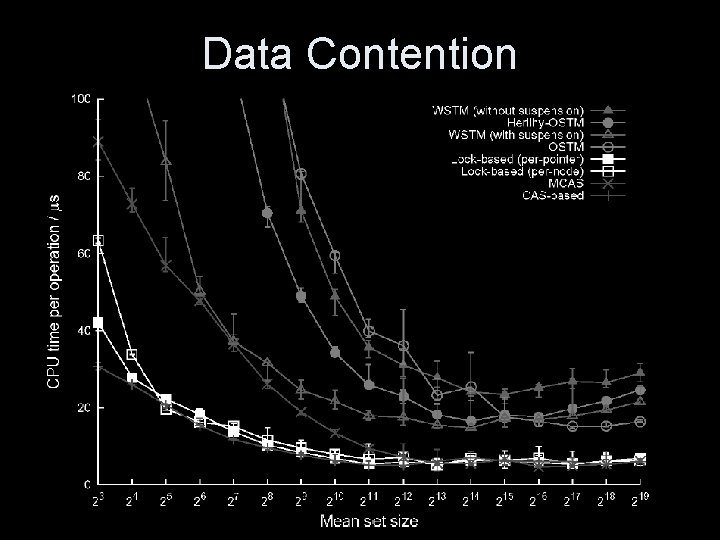
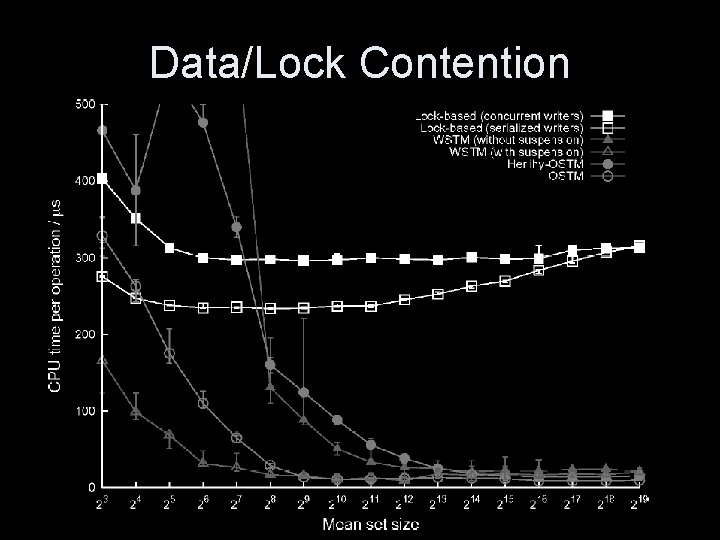
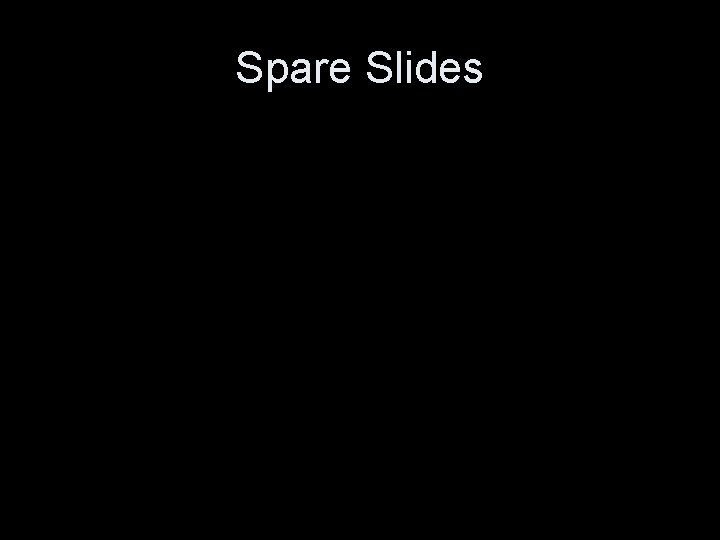
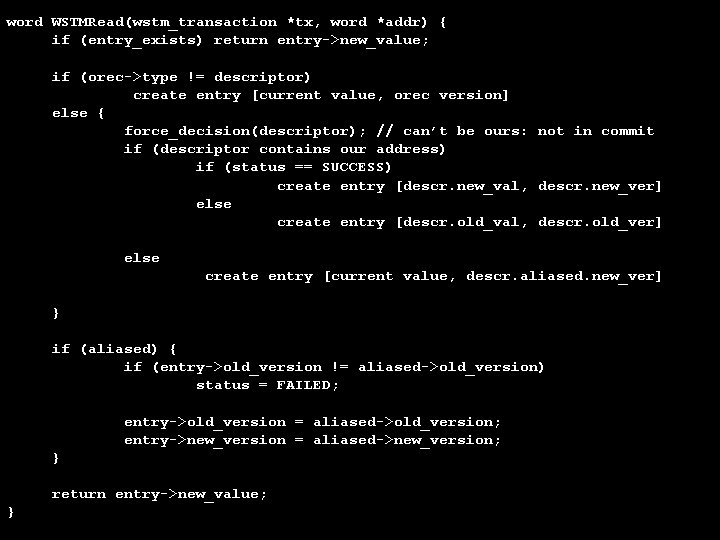
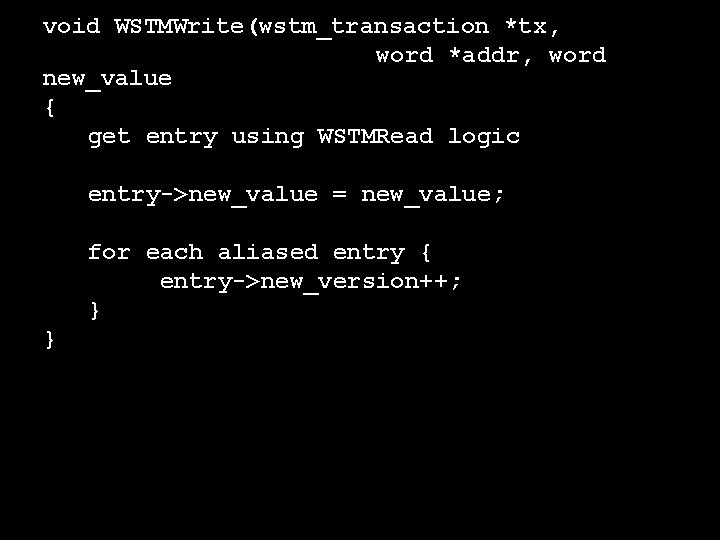
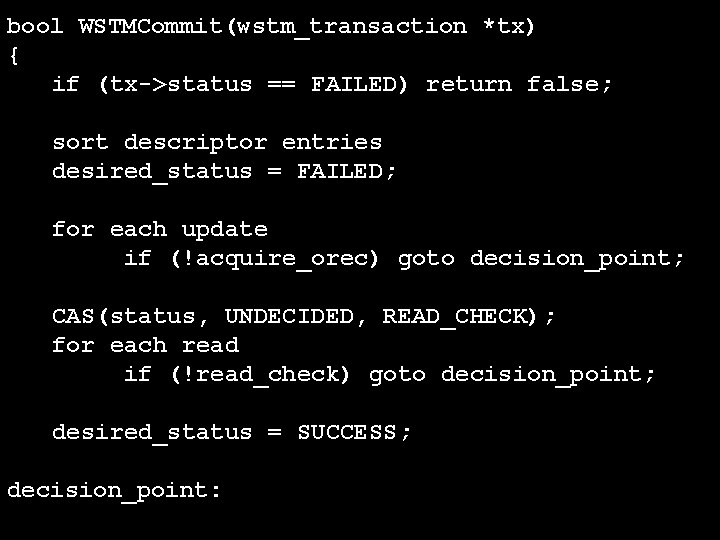
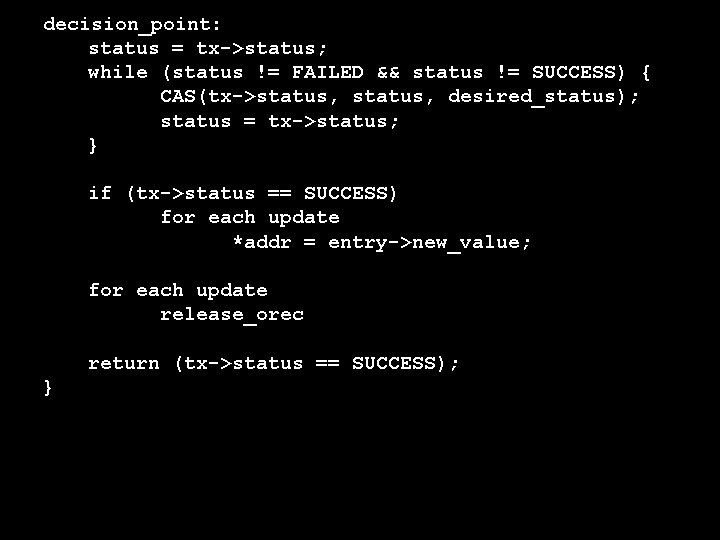
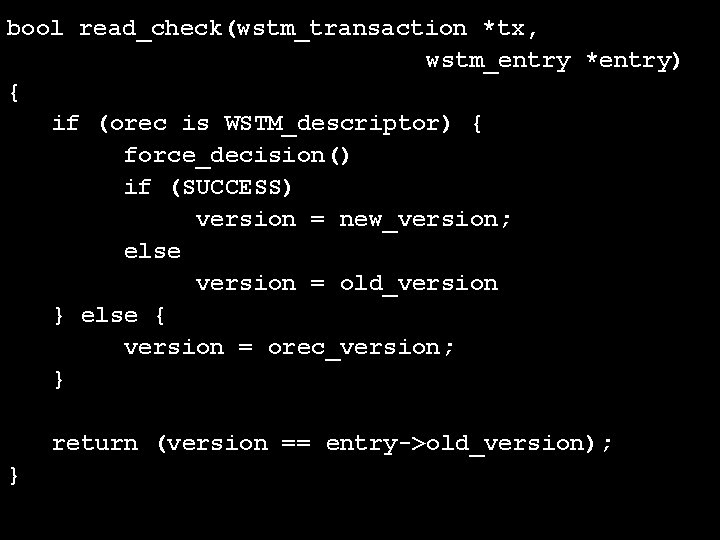
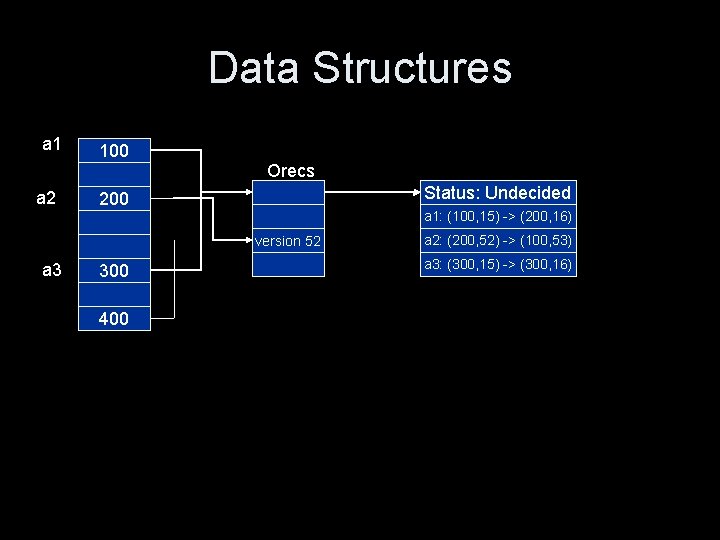
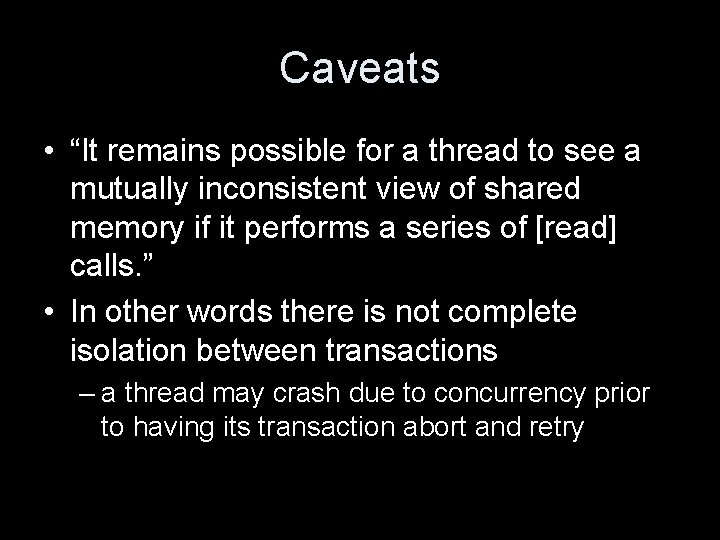
- Slides: 74
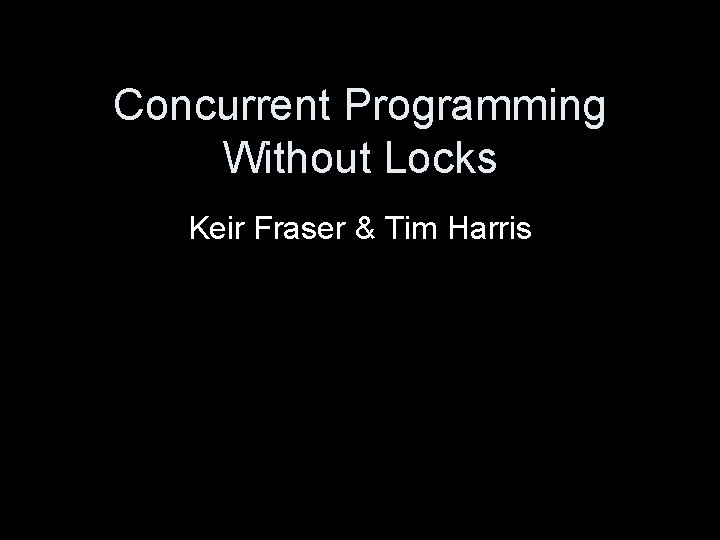
Concurrent Programming Without Locks Keir Fraser & Tim Harris
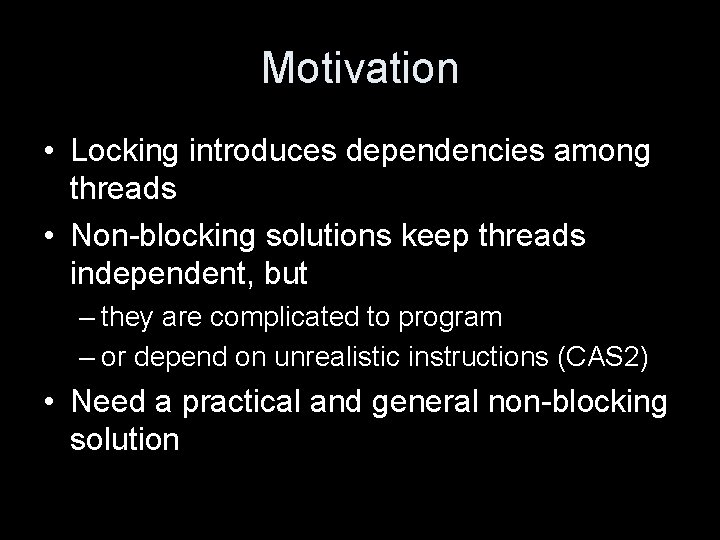
Motivation • Locking introduces dependencies among threads • Non-blocking solutions keep threads independent, but – they are complicated to program – or depend on unrealistic instructions (CAS 2) • Need a practical and general non-blocking solution
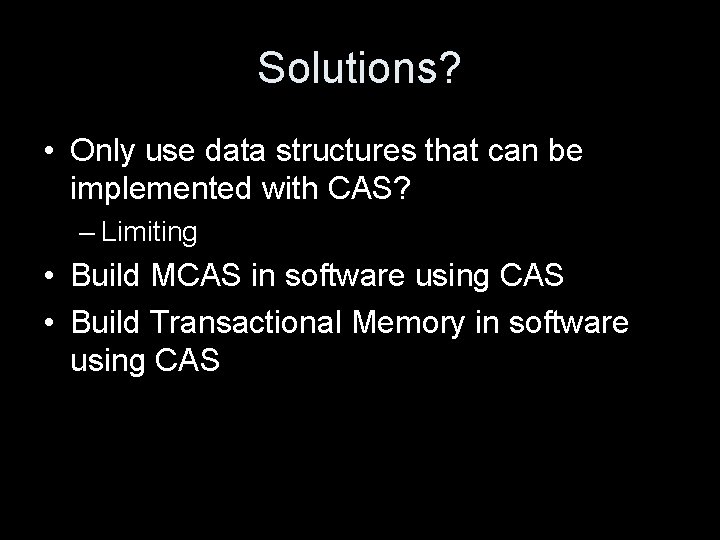
Solutions? • Only use data structures that can be implemented with CAS? – Limiting • Build MCAS in software using CAS • Build Transactional Memory in software using CAS
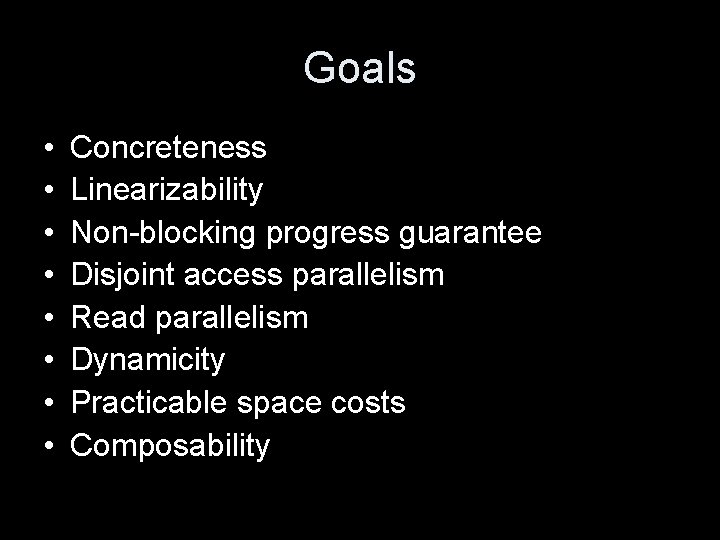
Goals • • Concreteness Linearizability Non-blocking progress guarantee Disjoint access parallelism Read parallelism Dynamicity Practicable space costs Composability
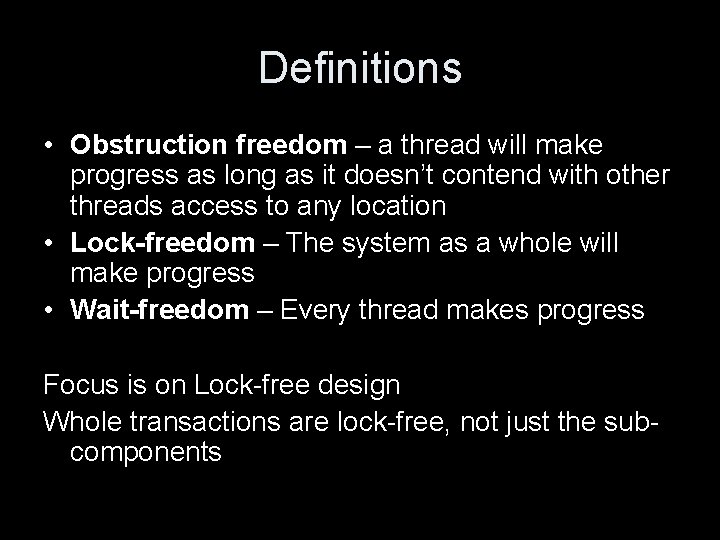
Definitions • Obstruction freedom – a thread will make progress as long as it doesn’t contend with other threads access to any location • Lock-freedom – The system as a whole will make progress • Wait-freedom – Every thread makes progress Focus is on Lock-free design Whole transactions are lock-free, not just the subcomponents
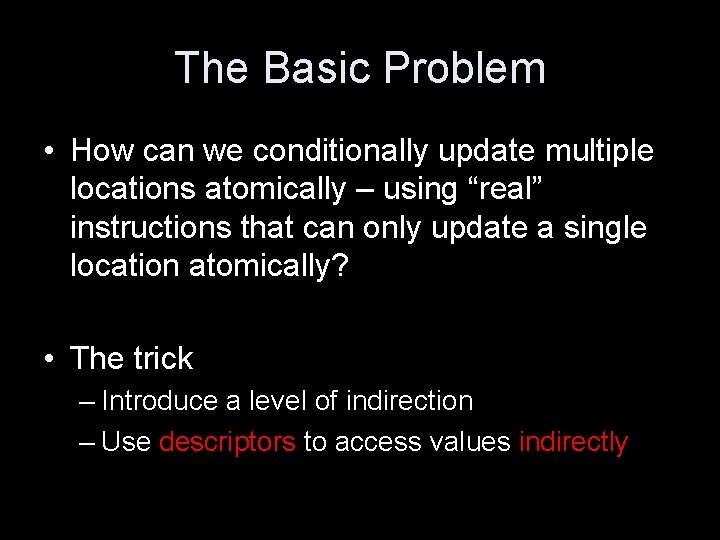
The Basic Problem • How can we conditionally update multiple locations atomically – using “real” instructions that can only update a single location atomically? • The trick – Introduce a level of indirection – Use descriptors to access values indirectly
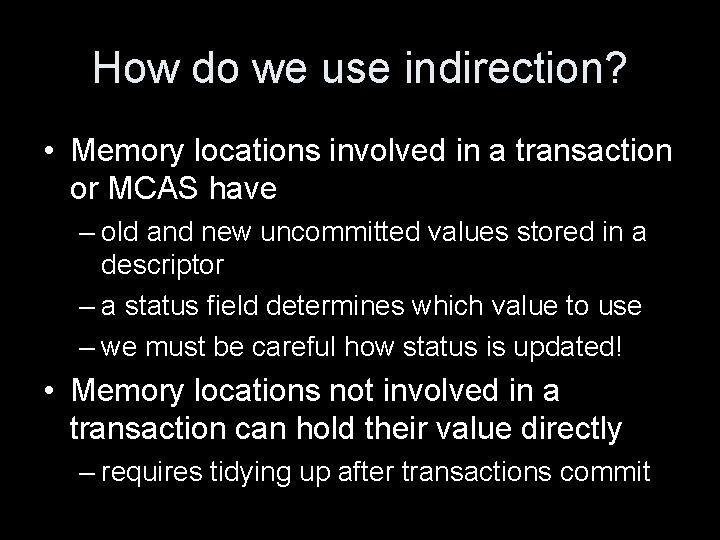
How do we use indirection? • Memory locations involved in a transaction or MCAS have – old and new uncommitted values stored in a descriptor – a status field determines which value to use – we must be careful how status is updated! • Memory locations not involved in a transaction can hold their value directly – requires tidying up after transactions commit
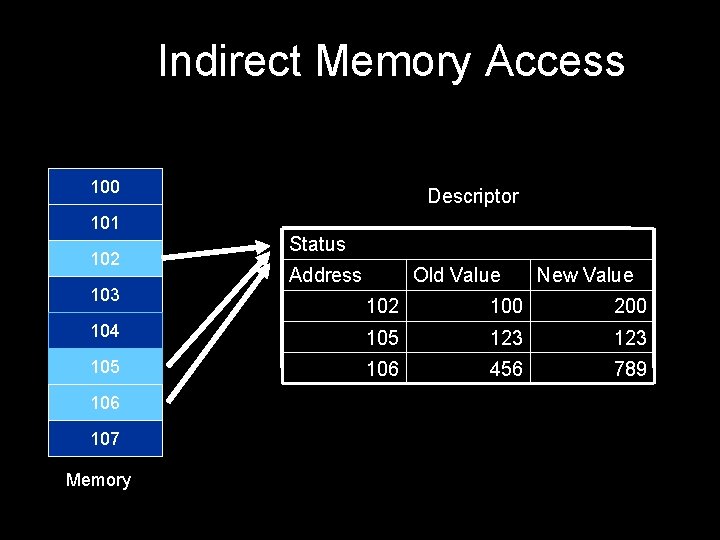
Indirect Memory Access 100 Descriptor 101 102 103 Status Address Old Value New Value 102 100 200 104 105 123 105 106 456 789 106 107 Memory
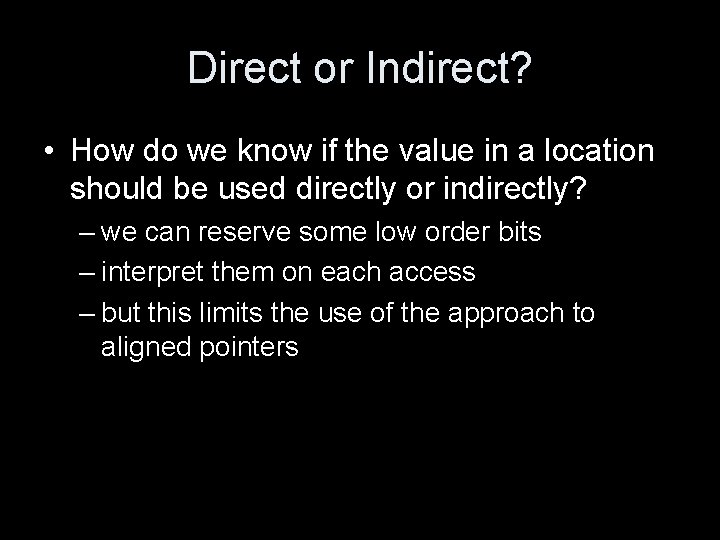
Direct or Indirect? • How do we know if the value in a location should be used directly or indirectly? – we can reserve some low order bits – interpret them on each access – but this limits the use of the approach to aligned pointers
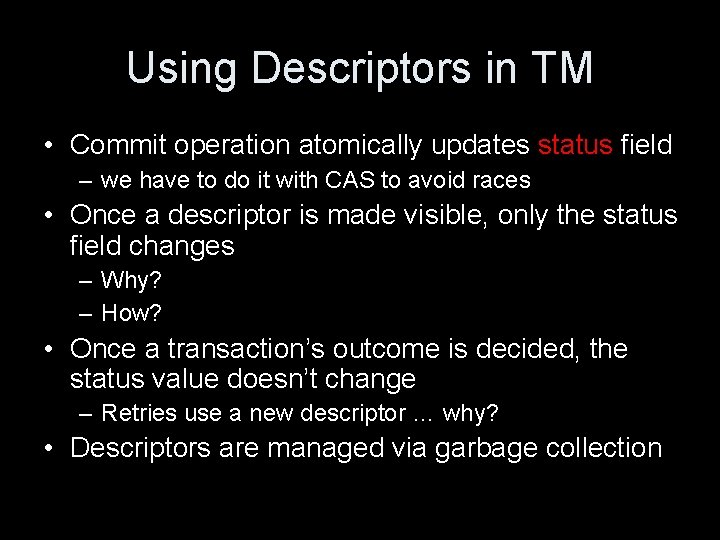
Using Descriptors in TM • Commit operation atomically updates status field – we have to do it with CAS to avoid races • Once a descriptor is made visible, only the status field changes – Why? – How? • Once a transaction’s outcome is decided, the status value doesn’t change – Retries use a new descriptor … why? • Descriptors are managed via garbage collection
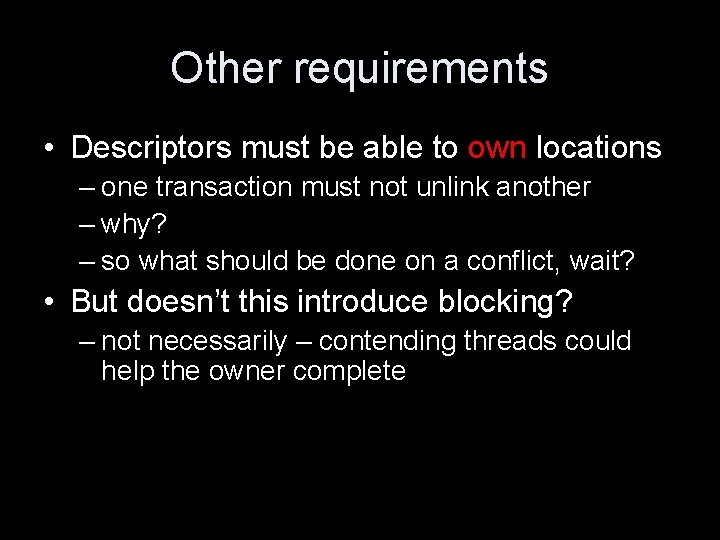
Other requirements • Descriptors must be able to own locations – one transaction must not unlink another – why? – so what should be done on a conflict, wait? • But doesn’t this introduce blocking? – not necessarily – contending threads could help the owner complete
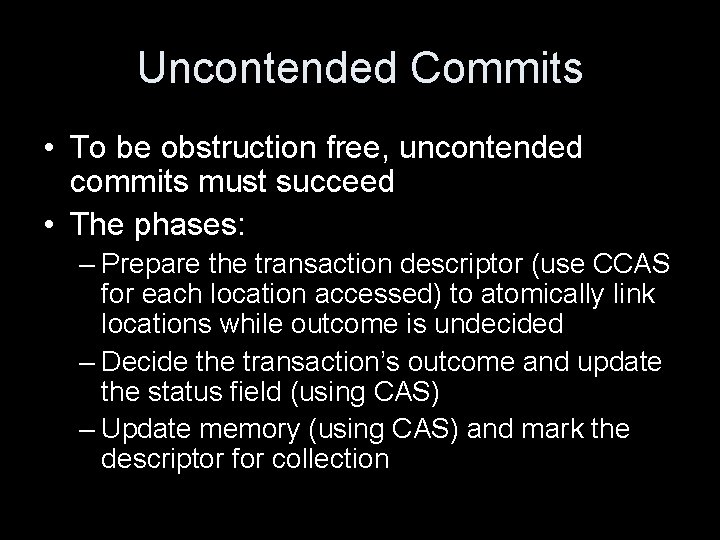
Uncontended Commits • To be obstruction free, uncontended commits must succeed • The phases: – Prepare the transaction descriptor (use CCAS for each location accessed) to atomically link locations while outcome is undecided – Decide the transaction’s outcome and update the status field (using CAS) – Update memory (using CAS) and mark the descriptor for collection
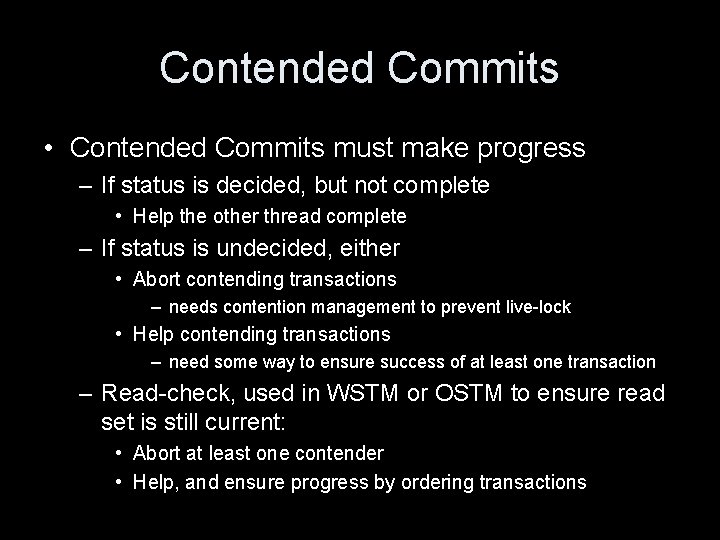
Contended Commits • Contended Commits must make progress – If status is decided, but not complete • Help the other thread complete – If status is undecided, either • Abort contending transactions – needs contention management to prevent live-lock • Help contending transactions – need some way to ensure success of at least one transaction – Read-check, used in WSTM or OSTM to ensure read set is still current: • Abort at least one contender • Help, and ensure progress by ordering transactions
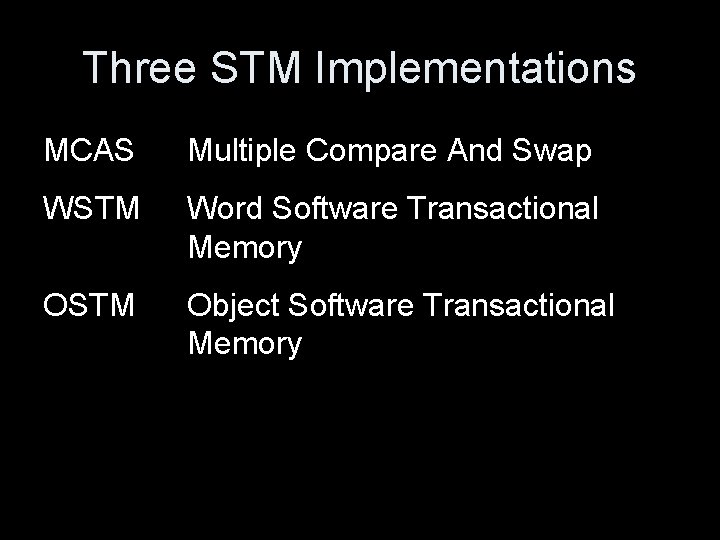
Three STM Implementations MCAS Multiple Compare And Swap WSTM Word Software Transactional Memory OSTM Object Software Transactional Memory
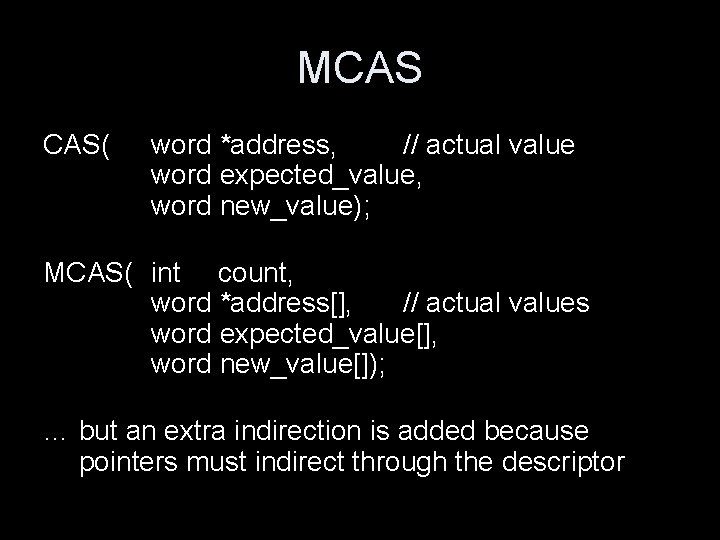
MCAS CAS( word *address, // actual value word expected_value, word new_value); MCAS( int count, word *address[], // actual values word expected_value[], word new_value[]); … but an extra indirection is added because pointers must indirect through the descriptor
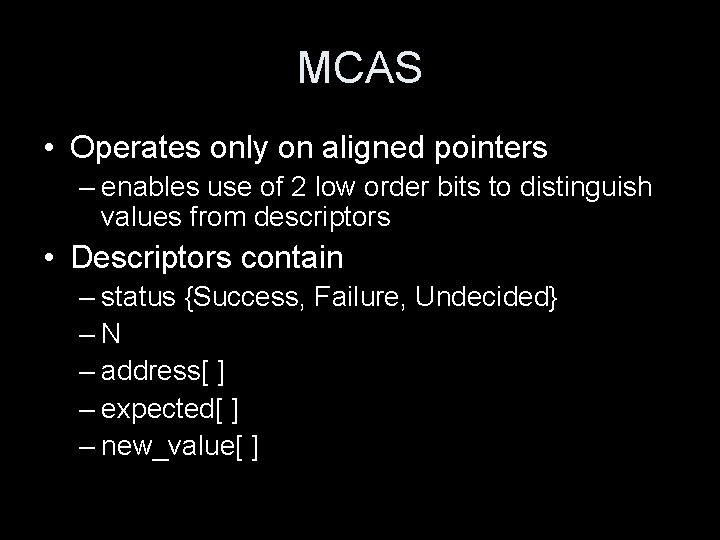
MCAS • Operates only on aligned pointers – enables use of 2 low order bits to distinguish values from descriptors • Descriptors contain – status {Success, Failure, Undecided} –N – address[ ] – expected[ ] – new_value[ ]
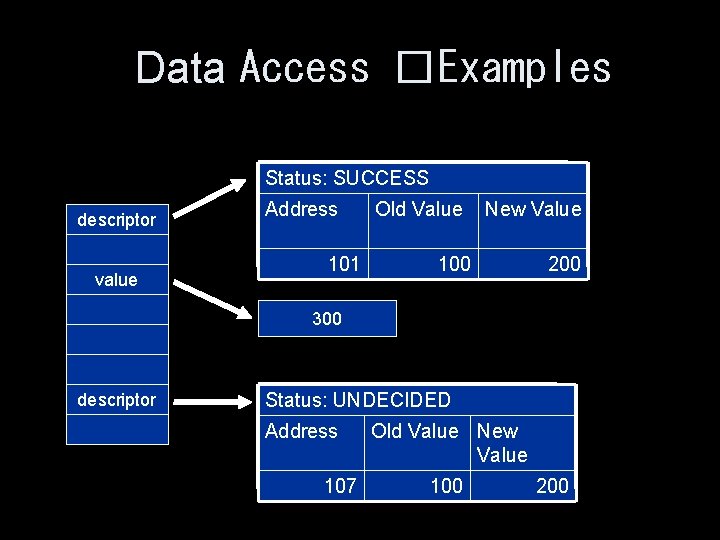
Data Access �Examples Status: SUCCESS descriptor value Address 101 Old Value New Value 100 200 300 descriptor Status: UNDECIDED Address 107 Old Value New Value 100 200
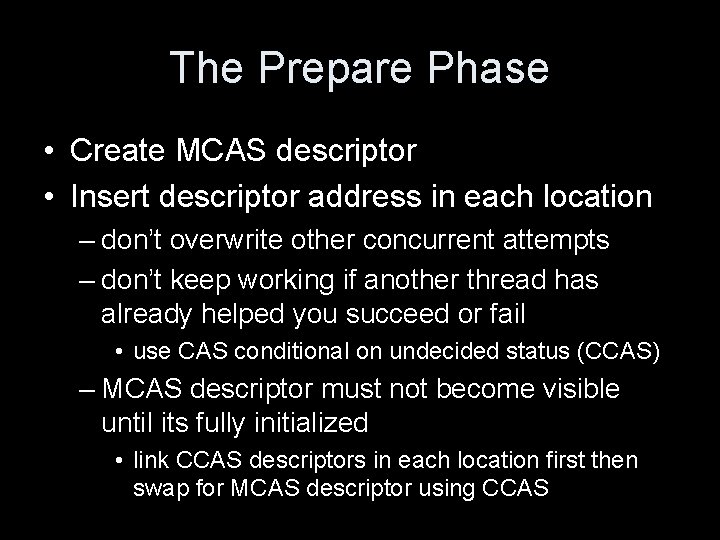
The Prepare Phase • Create MCAS descriptor • Insert descriptor address in each location – don’t overwrite other concurrent attempts – don’t keep working if another thread has already helped you succeed or fail • use CAS conditional on undecided status (CCAS) – MCAS descriptor must not become visible until its fully initialized • link CCAS descriptors in each location first then swap for MCAS descriptor using CCAS
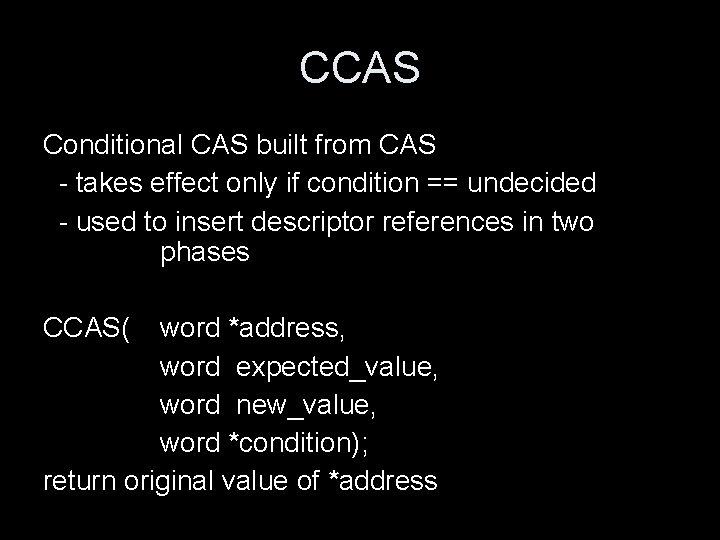
CCAS Conditional CAS built from CAS - takes effect only if condition == undecided - used to insert descriptor references in two phases CCAS( word *address, word expected_value, word new_value, word *condition); return original value of *address
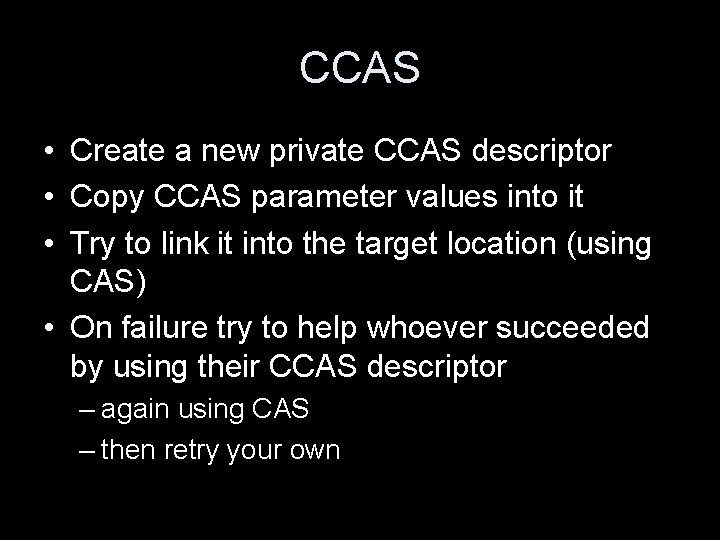
CCAS • Create a new private CCAS descriptor • Copy CCAS parameter values into it • Try to link it into the target location (using CAS) • On failure try to help whoever succeeded by using their CCAS descriptor – again using CAS – then retry your own
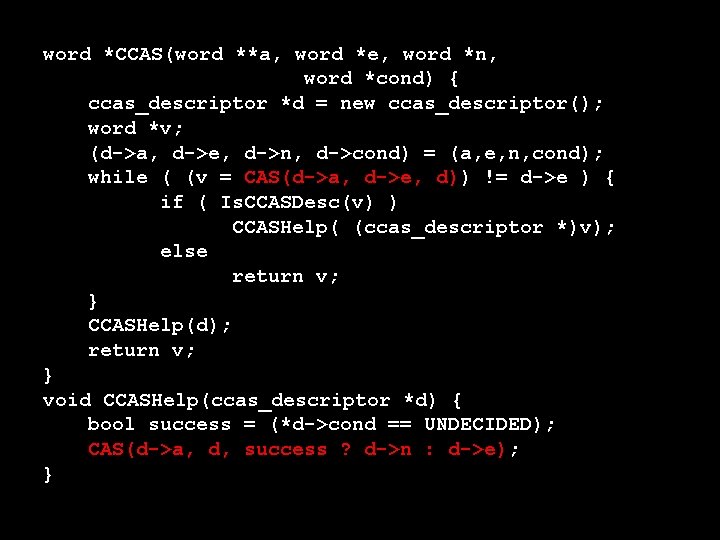
word *CCAS(word **a, word *e, word *n, word *cond) { ccas_descriptor *d = new ccas_descriptor(); word *v; (d->a, d->e, d->n, d->cond) = (a, e, n, cond); while ( (v = CAS(d->a, d->e, d)) != d->e ) { if ( Is. CCASDesc(v) ) CCASHelp( (ccas_descriptor *)v); else return v; } CCASHelp(d); return v; } void CCASHelp(ccas_descriptor *d) { bool success = (*d->cond == UNDECIDED); CAS(d->a, d, success ? d->n : d->e); }
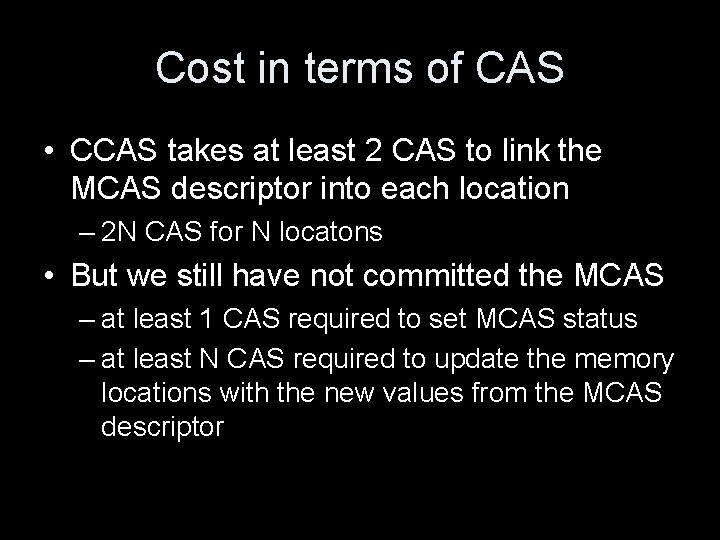
Cost in terms of CAS • CCAS takes at least 2 CAS to link the MCAS descriptor into each location – 2 N CAS for N locatons • But we still have not committed the MCAS – at least 1 CAS required to set MCAS status – at least N CAS required to update the memory locations with the new values from the MCAS descriptor
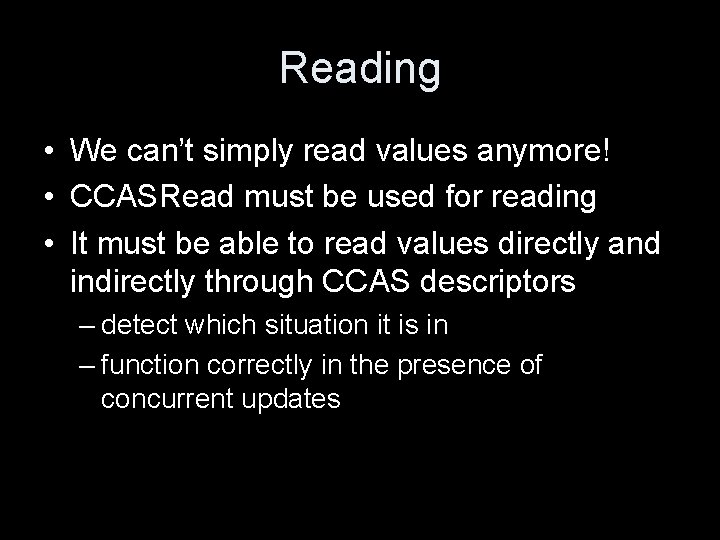
Reading • We can’t simply read values anymore! • CCASRead must be used for reading • It must be able to read values directly and indirectly through CCAS descriptors – detect which situation it is in – function correctly in the presence of concurrent updates
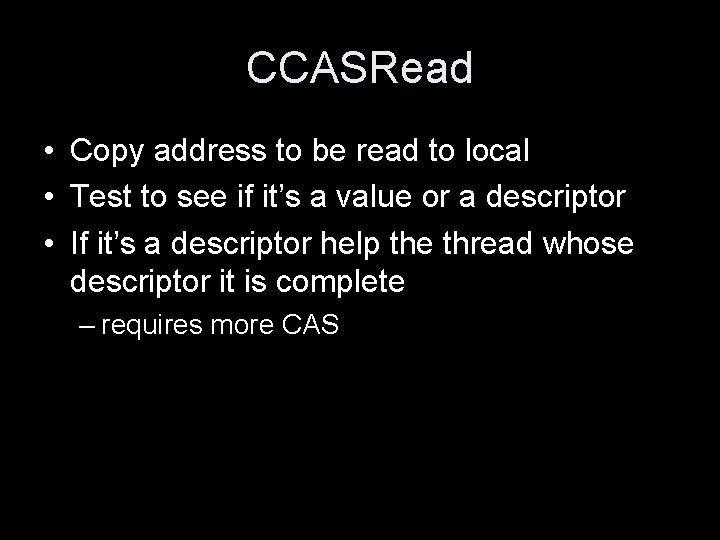
CCASRead • Copy address to be read to local • Test to see if it’s a value or a descriptor • If it’s a descriptor help the thread whose descriptor it is complete – requires more CAS
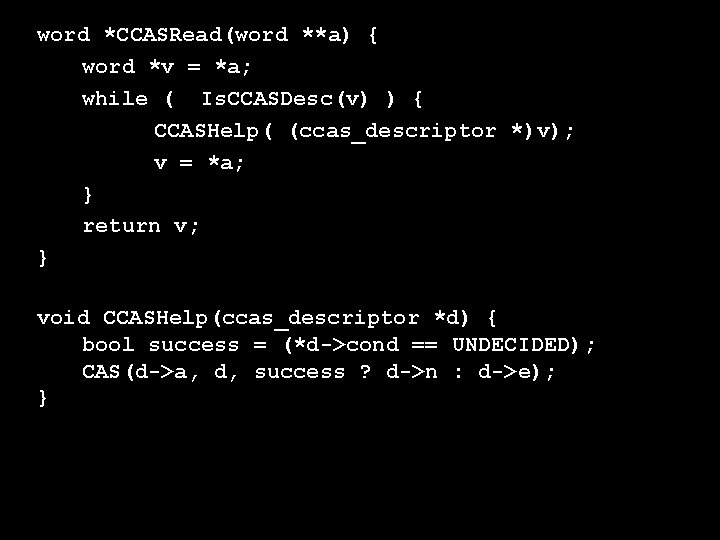
word *CCASRead(word **a) { word *v = *a; while ( Is. CCASDesc(v) ) { CCASHelp( (ccas_descriptor *)v); v = *a; } return v; } void CCASHelp(ccas_descriptor *d) { bool success = (*d->cond == UNDECIDED); CAS(d->a, d, success ? d->n : d->e); }
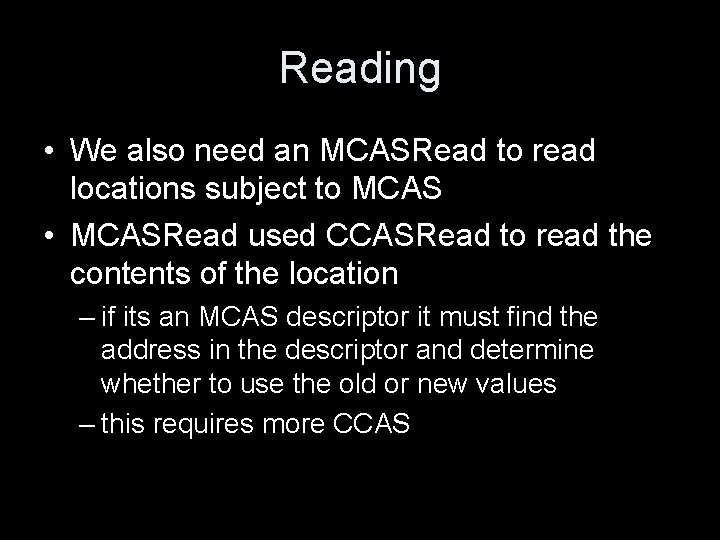
Reading • We also need an MCASRead to read locations subject to MCAS • MCASRead used CCASRead to read the contents of the location – if its an MCAS descriptor it must find the address in the descriptor and determine whether to use the old or new values – this requires more CCAS
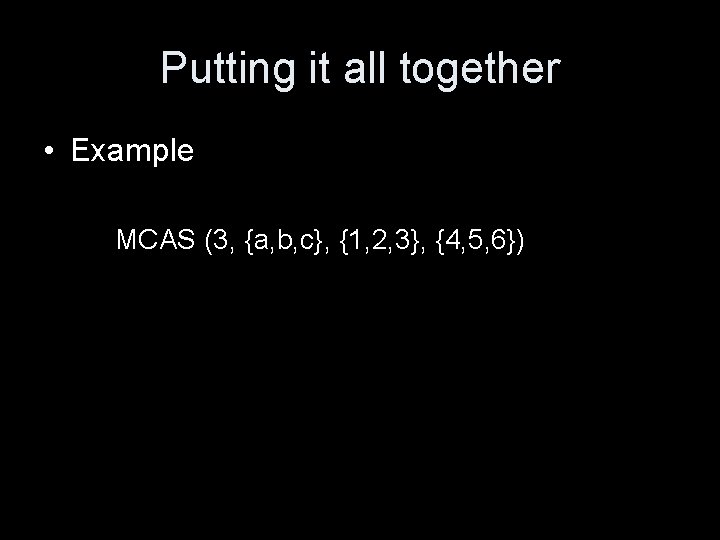
Putting it all together • Example MCAS (3, {a, b, c}, {1, 2, 3}, {4, 5, 6})
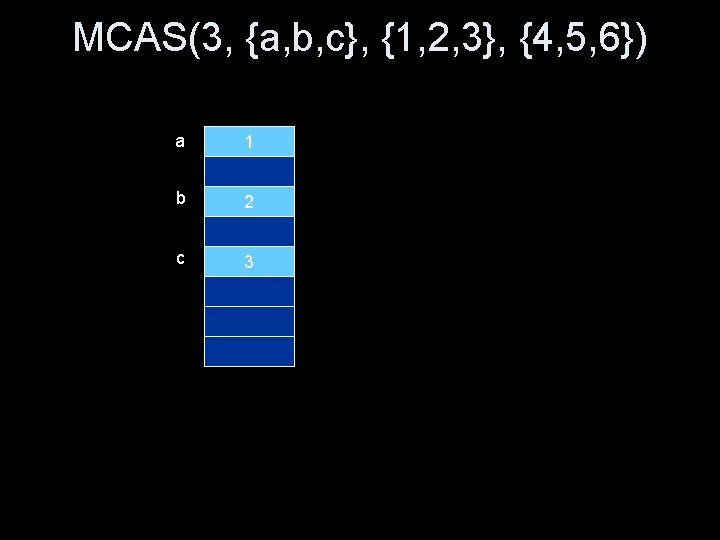
MCAS(3, {a, b, c}, {1, 2, 3}, {4, 5, 6}) a 1 b 2 c 3
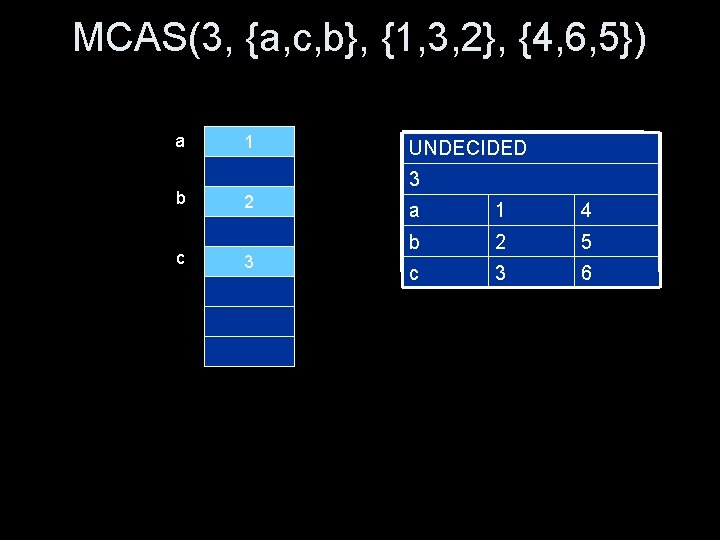
MCAS(3, {a, c, b}, {1, 3, 2}, {4, 6, 5}) a b c 1 UNDECIDED 3 2 3 a 1 4 b 2 5 c 3 6
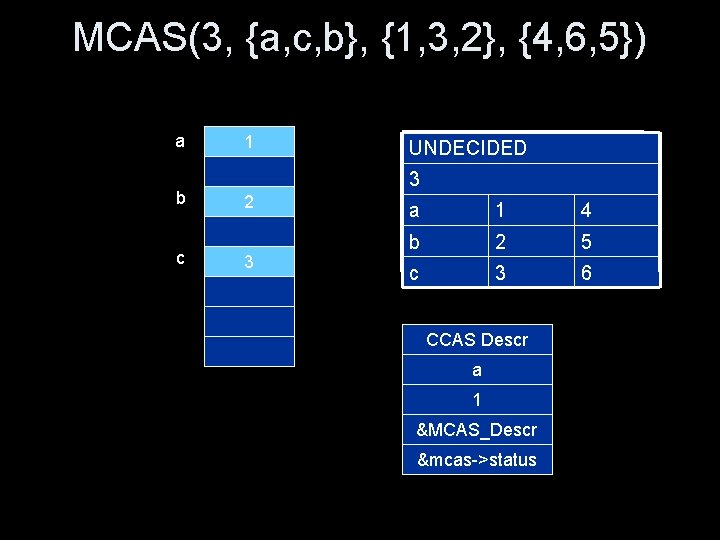
MCAS(3, {a, c, b}, {1, 3, 2}, {4, 6, 5}) a b c 1 UNDECIDED 3 2 3 a 1 4 b 2 5 c 3 6 CCAS Descr a 1 &MCAS_Descr &mcas->status
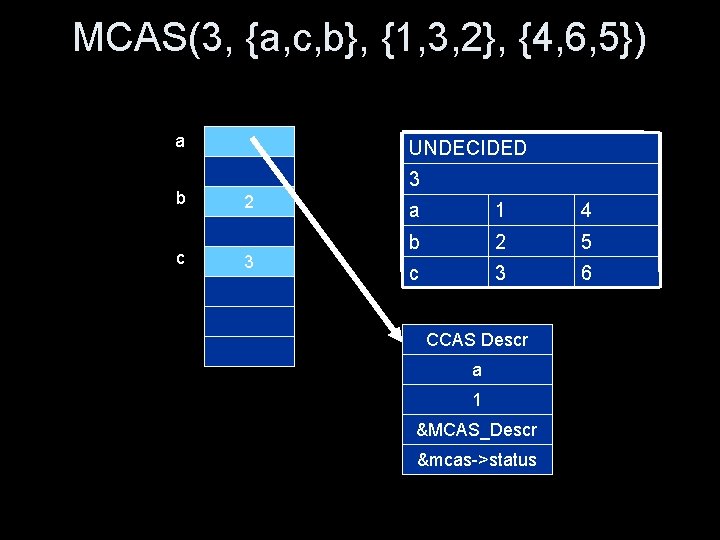
MCAS(3, {a, c, b}, {1, 3, 2}, {4, 6, 5}) a b c UNDECIDED 3 2 3 a 1 4 b 2 5 c 3 6 CCAS Descr a 1 &MCAS_Descr &mcas->status
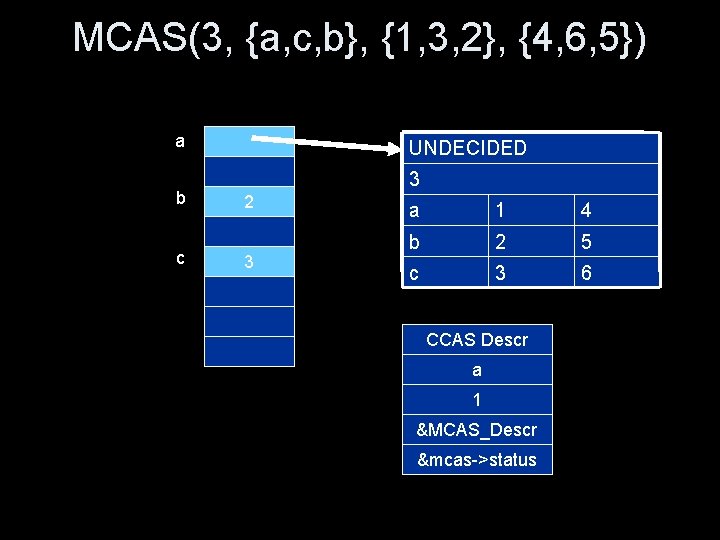
MCAS(3, {a, c, b}, {1, 3, 2}, {4, 6, 5}) a b c UNDECIDED 3 2 3 a 1 4 b 2 5 c 3 6 CCAS Descr a 1 &MCAS_Descr &mcas->status
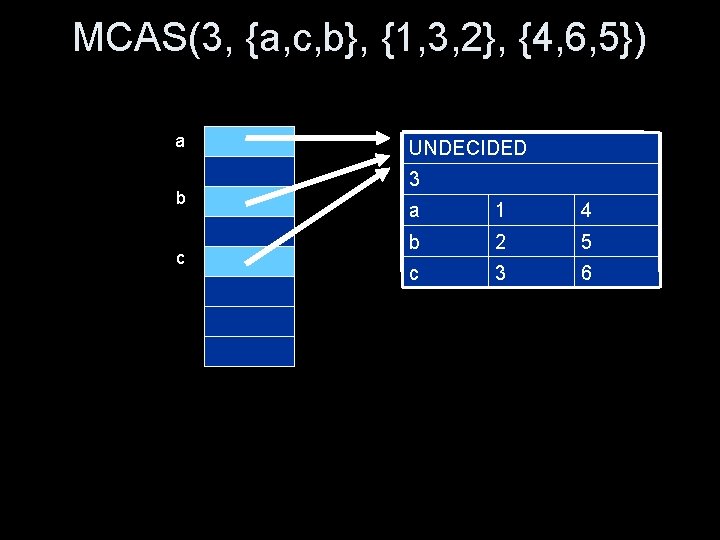
MCAS(3, {a, c, b}, {1, 3, 2}, {4, 6, 5}) a b c UNDECIDED 3 a 1 4 b 2 5 c 3 6
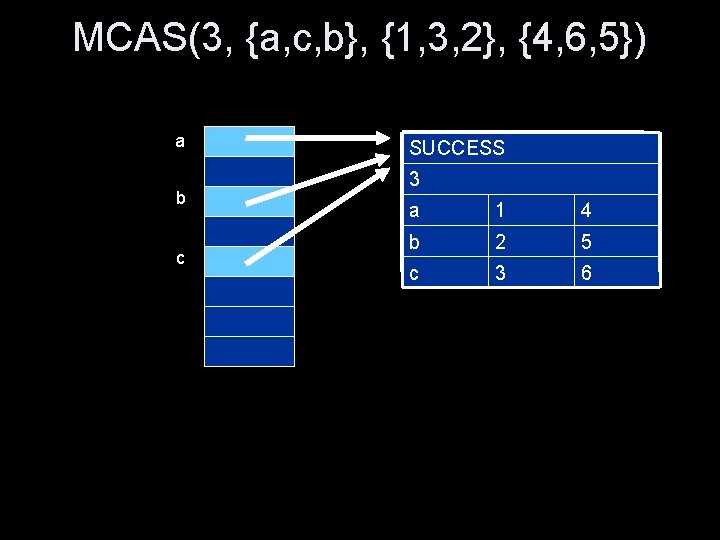
MCAS(3, {a, c, b}, {1, 3, 2}, {4, 6, 5}) a b c SUCCESS 3 a 1 4 b 2 5 c 3 6
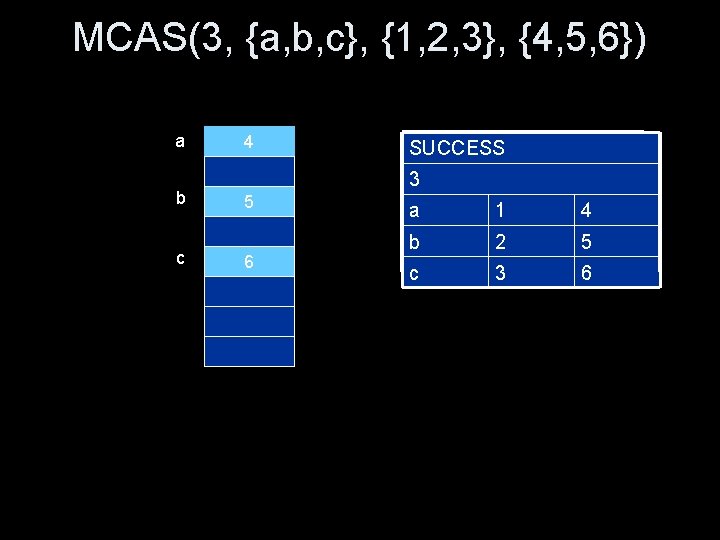
MCAS(3, {a, b, c}, {1, 2, 3}, {4, 5, 6}) a b c 1 4 SUCCESS 3 2 5 3 6 a 1 4 b 2 5 c 3 6
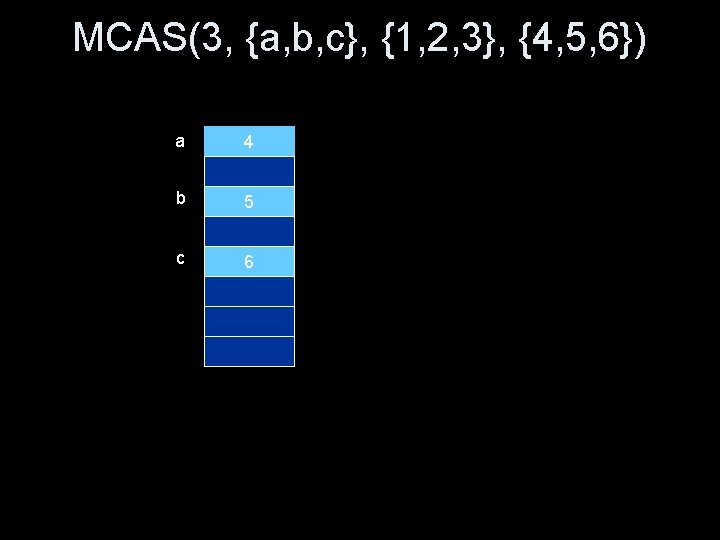
MCAS(3, {a, b, c}, {1, 2, 3}, {4, 5, 6}) a 1 4 b 2 5 c 3 6
![bool MCASint N word a word e word n mcasdescriptor d new bool MCAS(int N, word **a[], word *e[], word *n[]) { mcas_descriptor *d = new](https://slidetodoc.com/presentation_image/4533dd76dbf4131d08d3af8bbf270b53/image-37.jpg)
bool MCAS(int N, word **a[], word *e[], word *n[]) { mcas_descriptor *d = new mcas_descriptor(); d->N = N; d->status = UNDECIDED; for (int i=0; i<N; i++) { d->a[i] = a[i]; d->e[i] = e[i]; d->n[i] = n[i]; } address_sort(d); return mcas_help(d); }
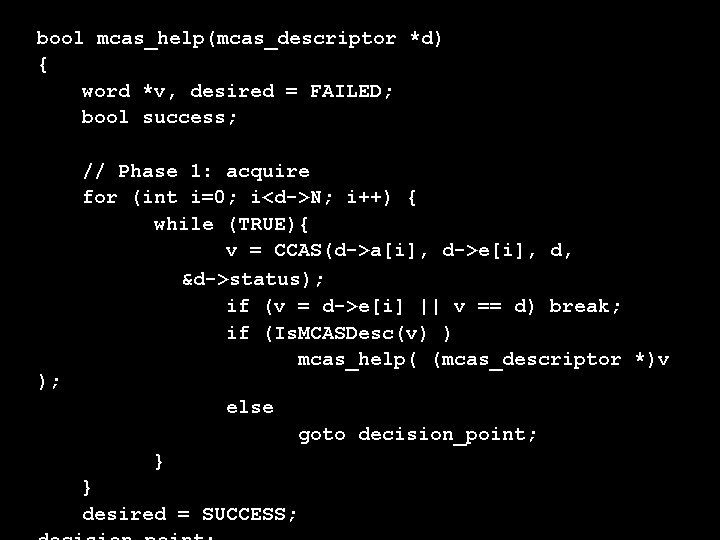
bool mcas_help(mcas_descriptor *d) { word *v, desired = FAILED; bool success; ); // Phase 1: acquire for (int i=0; i<d->N; i++) { while (TRUE){ v = CCAS(d->a[i], d->e[i], d, &d->status); if (v = d->e[i] || v == d) break; if (Is. MCASDesc(v) ) mcas_help( (mcas_descriptor *)v else goto decision_point; } } desired = SUCCESS;
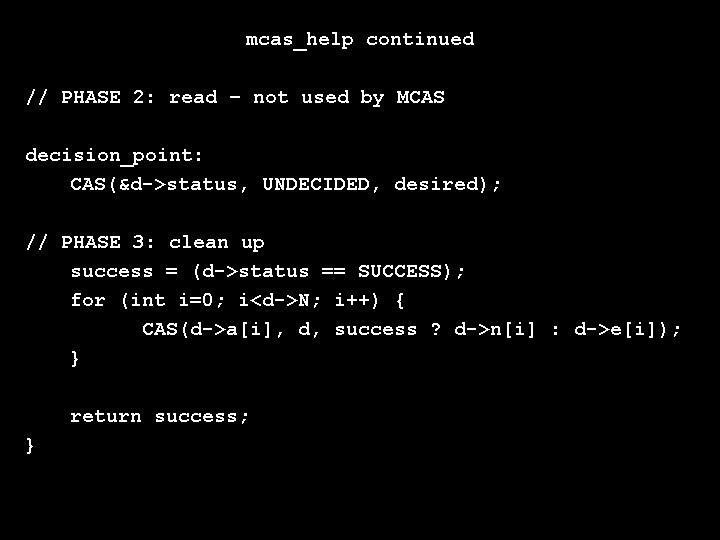
mcas_help continued // PHASE 2: read – not used by MCAS decision_point: CAS(&d->status, UNDECIDED, desired); // PHASE 3: clean up success = (d->status == SUCCESS); for (int i=0; i<d->N; i++) { CAS(d->a[i], d, success ? d->n[i] : d->e[i]); } return success; }
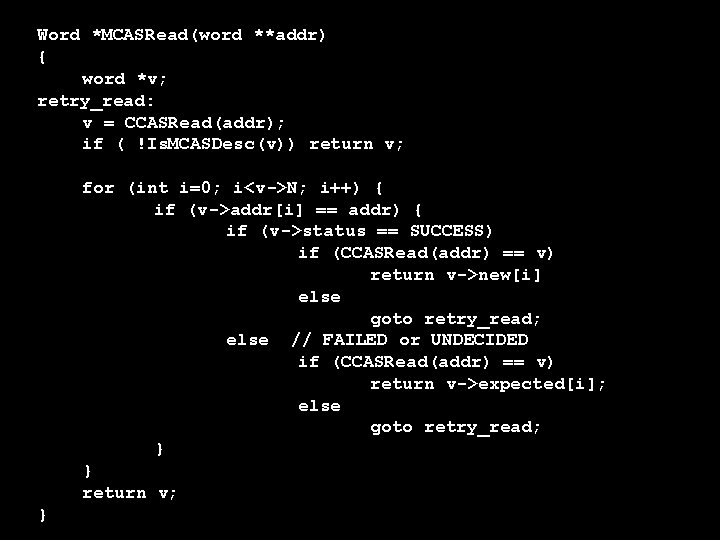
Word *MCASRead(word **addr) { word *v; retry_read: v = CCASRead(addr); if ( !Is. MCASDesc(v)) return v; for (int i=0; i<v->N; i++) { if (v->addr[i] == addr) { if (v->status == SUCCESS) if (CCASRead(addr) == v) return v->new[i] else goto retry_read; else // FAILED or UNDECIDED if (CCASRead(addr) == v) return v->expected[i]; else goto retry_read; } } return v; }
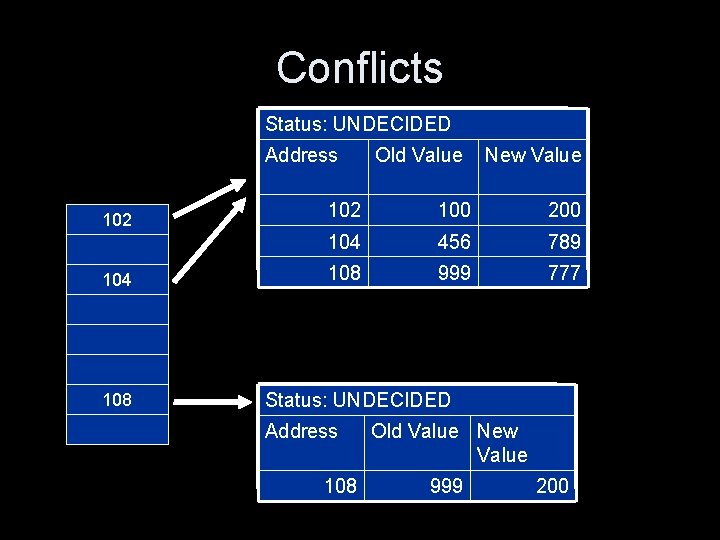
Conflicts Status: UNDECIDED Address 102 104 108 Old Value New Value 102 100 200 104 456 789 108 999 777 Status: UNDECIDED Address 108 Old Value New Value 999 200
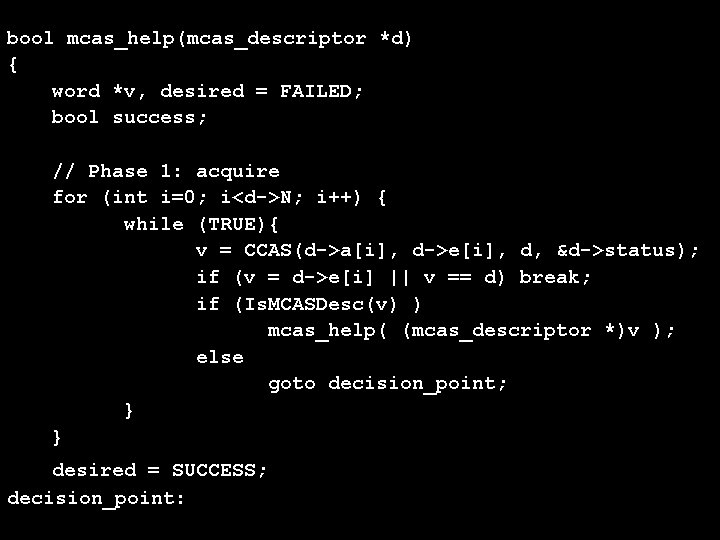
bool mcas_help(mcas_descriptor *d) { word *v, desired = FAILED; bool success; // Phase 1: acquire for (int i=0; i<d->N; i++) { while (TRUE){ v = CCAS(d->a[i], d->e[i], d, &d->status); if (v = d->e[i] || v == d) break; if (Is. MCASDesc(v) ) mcas_help( (mcas_descriptor *)v ); else goto decision_point; } } desired = SUCCESS; decision_point:
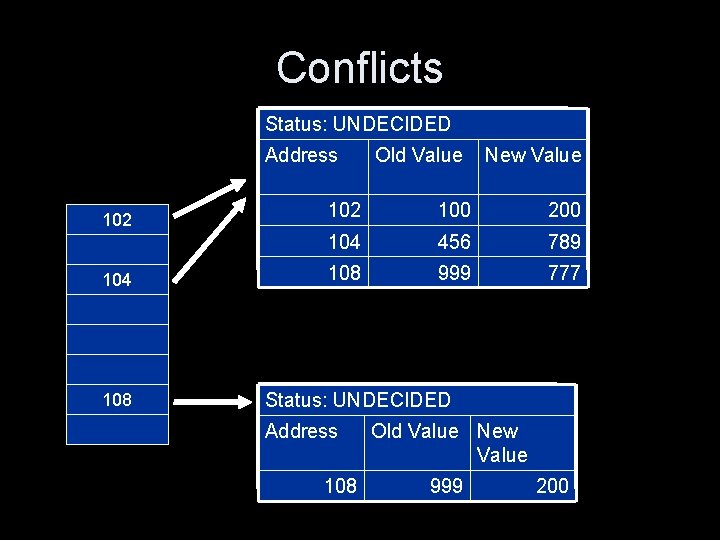
Conflicts Status: UNDECIDED Address 102 104 108 Old Value New Value 102 100 200 104 456 789 108 999 777 Status: UNDECIDED Address 108 Old Value New Value 999 200
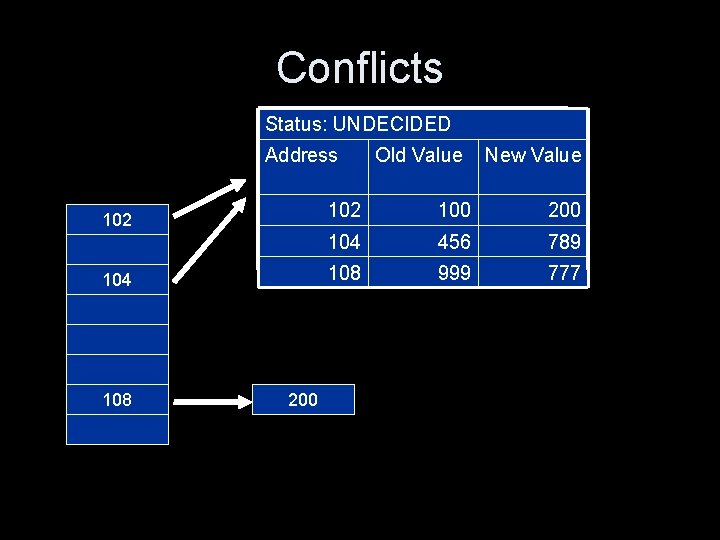
Conflicts Status: UNDECIDED Address 102 104 108 200 Old Value New Value 102 100 200 104 456 789 108 999 777
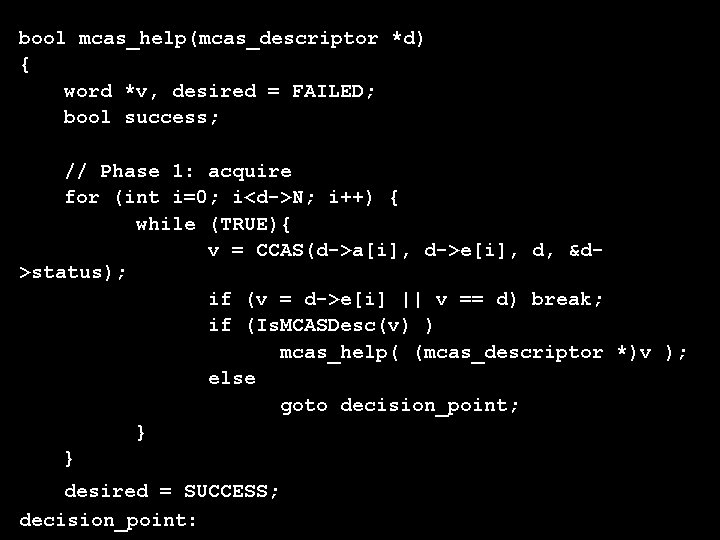
bool mcas_help(mcas_descriptor *d) { word *v, desired = FAILED; bool success; // Phase 1: acquire for (int i=0; i<d->N; i++) { while (TRUE){ v = CCAS(d->a[i], d->e[i], d, &d>status); if (v = d->e[i] || v == d) break; if (Is. MCASDesc(v) ) mcas_help( (mcas_descriptor *)v ); else goto decision_point; } } desired = SUCCESS; decision_point:
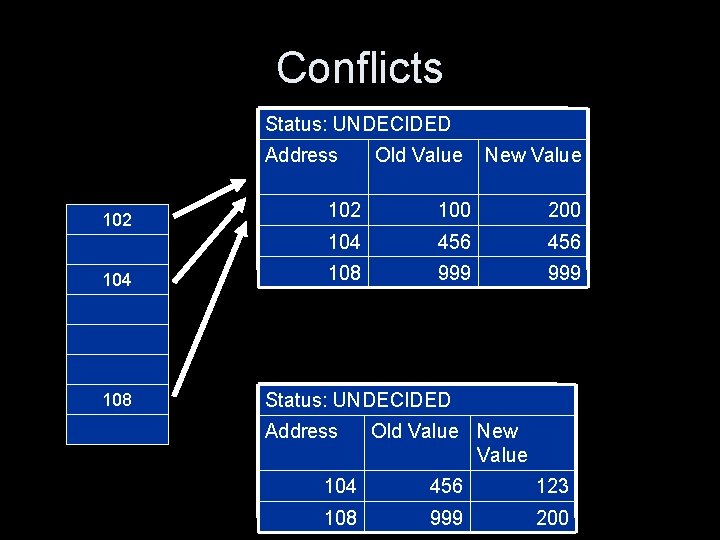
Conflicts Status: UNDECIDED Address 102 104 108 Old Value New Value 102 100 200 104 456 108 999 Status: UNDECIDED Address Old Value New Value 104 456 123 108 999 200
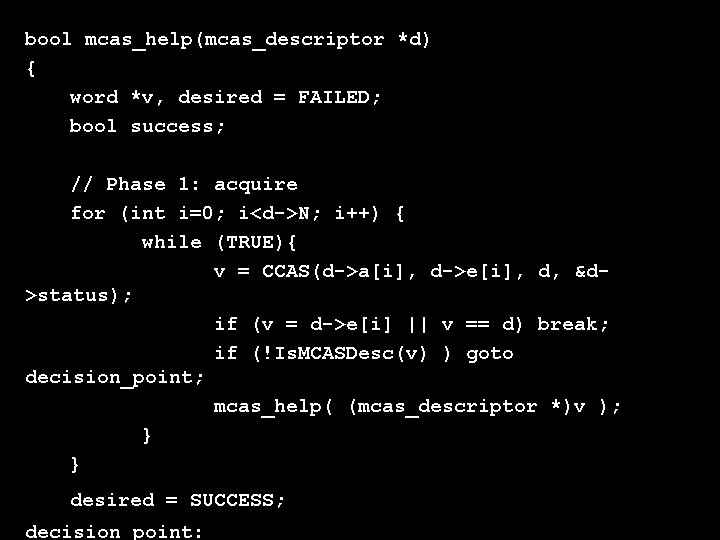
bool mcas_help(mcas_descriptor *d) { word *v, desired = FAILED; bool success; // Phase 1: acquire for (int i=0; i<d->N; i++) { while (TRUE){ v = CCAS(d->a[i], d->e[i], d, &d>status); if (v = d->e[i] || v == d) break; if (!Is. MCASDesc(v) ) goto decision_point; mcas_help( (mcas_descriptor *)v ); } } desired = SUCCESS; decision_point:
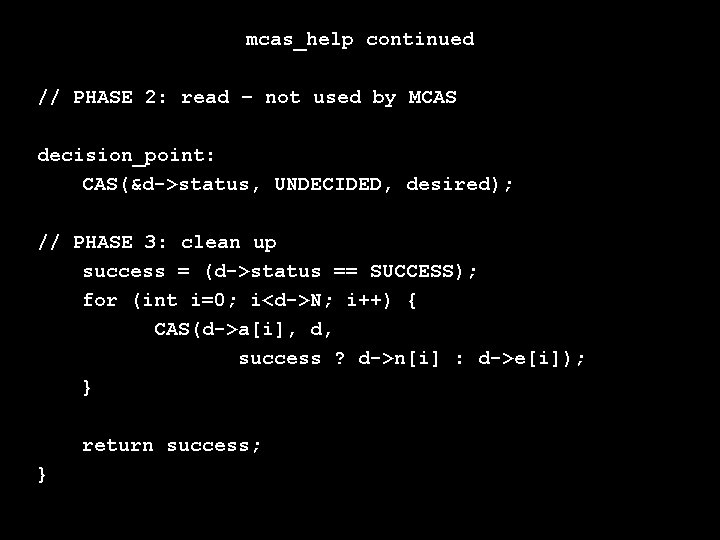
mcas_help continued // PHASE 2: read – not used by MCAS decision_point: CAS(&d->status, UNDECIDED, desired); // PHASE 3: clean up success = (d->status == SUCCESS); for (int i=0; i<d->N; i++) { CAS(d->a[i], d, success ? d->n[i] : d->e[i]); } return success; }
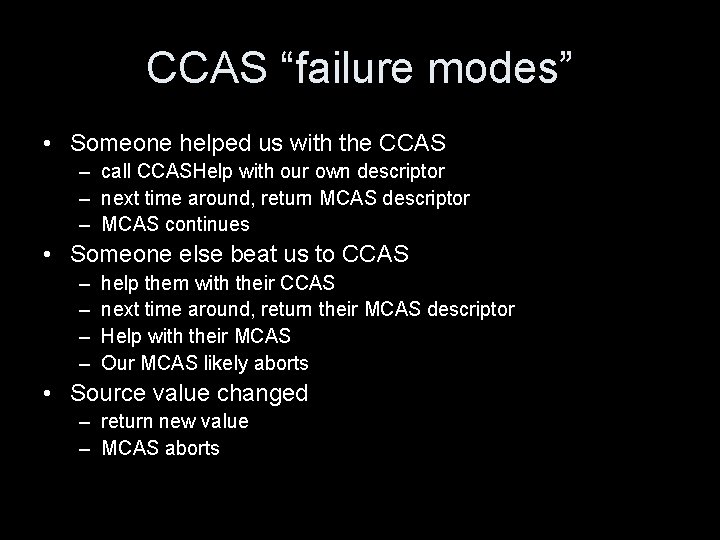
CCAS “failure modes” • Someone helped us with the CCAS – call CCASHelp with our own descriptor – next time around, return MCAS descriptor – MCAS continues • Someone else beat us to CCAS – – help them with their CCAS next time around, return their MCAS descriptor Help with their MCAS Our MCAS likely aborts • Source value changed – return new value – MCAS aborts
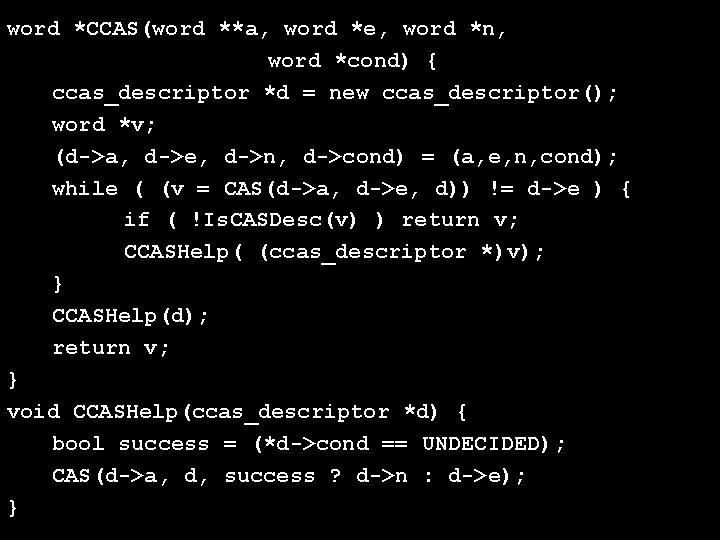
word *CCAS(word **a, word *e, word *n, word *cond) { ccas_descriptor *d = new ccas_descriptor(); word *v; (d->a, d->e, d->n, d->cond) = (a, e, n, cond); while ( (v = CAS(d->a, d->e, d)) != d->e ) { if ( !Is. CASDesc(v) ) return v; CCASHelp( (ccas_descriptor *)v); } CCASHelp(d); return v; } void CCASHelp(ccas_descriptor *d) { bool success = (*d->cond == UNDECIDED); CAS(d->a, d, success ? d->n : d->e); }
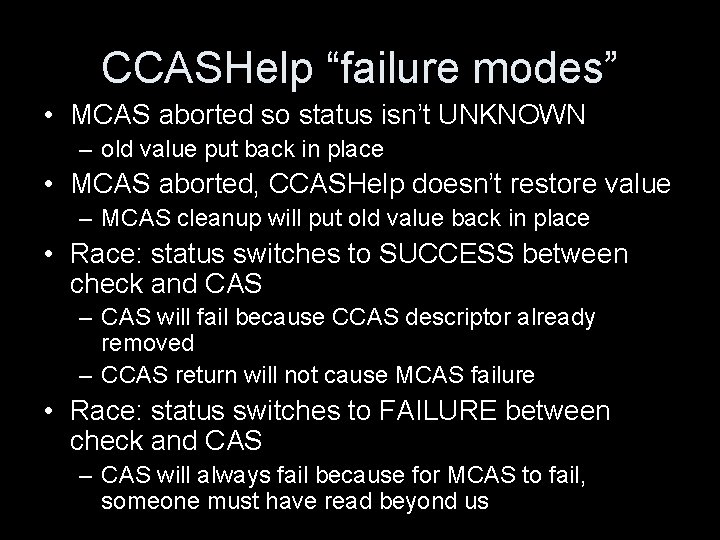
CCASHelp “failure modes” • MCAS aborted so status isn’t UNKNOWN – old value put back in place • MCAS aborted, CCASHelp doesn’t restore value – MCAS cleanup will put old value back in place • Race: status switches to SUCCESS between check and CAS – CAS will fail because CCAS descriptor already removed – CCAS return will not cause MCAS failure • Race: status switches to FAILURE between check and CAS – CAS will always fail because for MCAS to fail, someone must have read beyond us
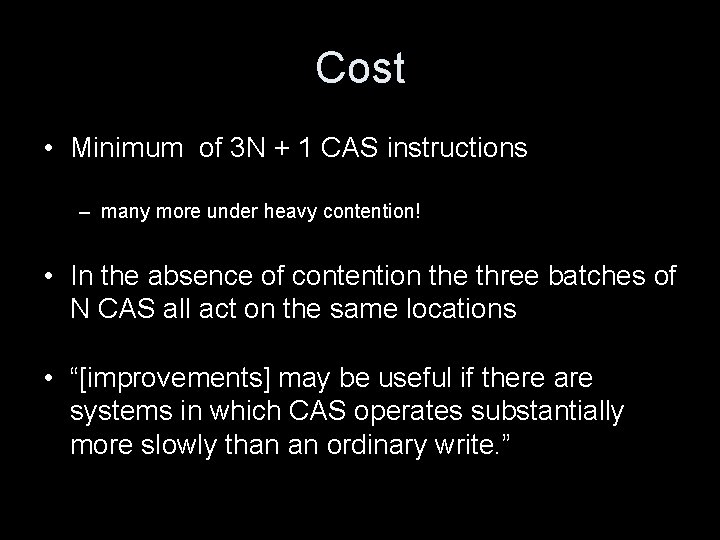
Cost • Minimum of 3 N + 1 CAS instructions – many more under heavy contention! • In the absence of contention the three batches of N CAS all act on the same locations • “[improvements] may be useful if there are systems in which CAS operates substantially more slowly than an ordinary write. ”
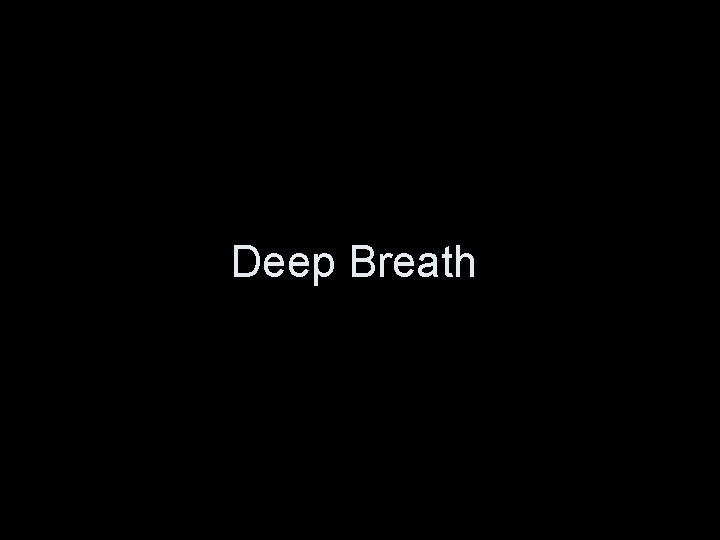
Deep Breath
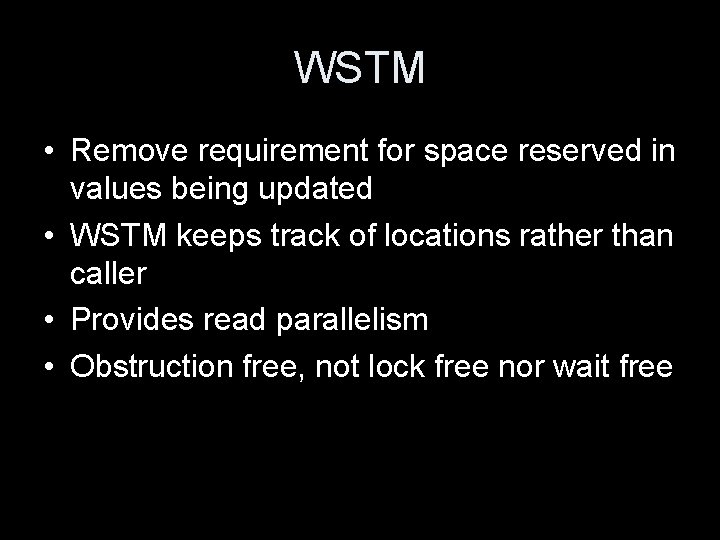
WSTM • Remove requirement for space reserved in values being updated • WSTM keeps track of locations rather than caller • Provides read parallelism • Obstruction free, not lock free nor wait free
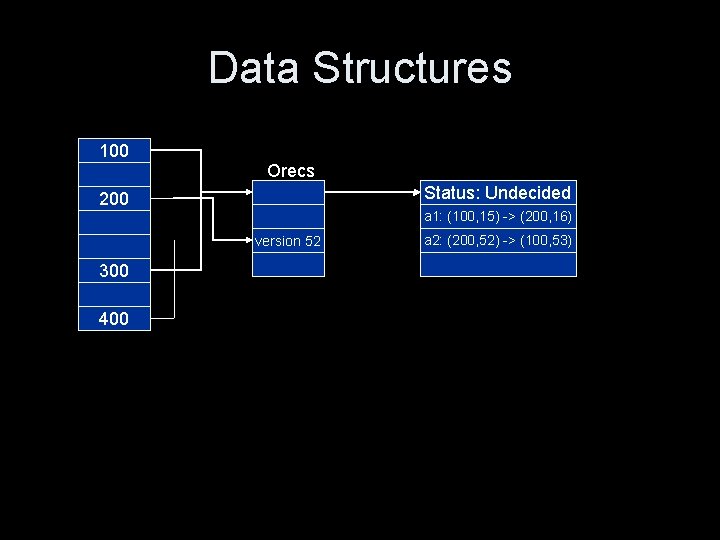
Data Structures 100 Orecs Status: Undecided 200 a 1: (100, 15) -> (200, 16) version 52 300 400 a 2: (200, 52) -> (100, 53)
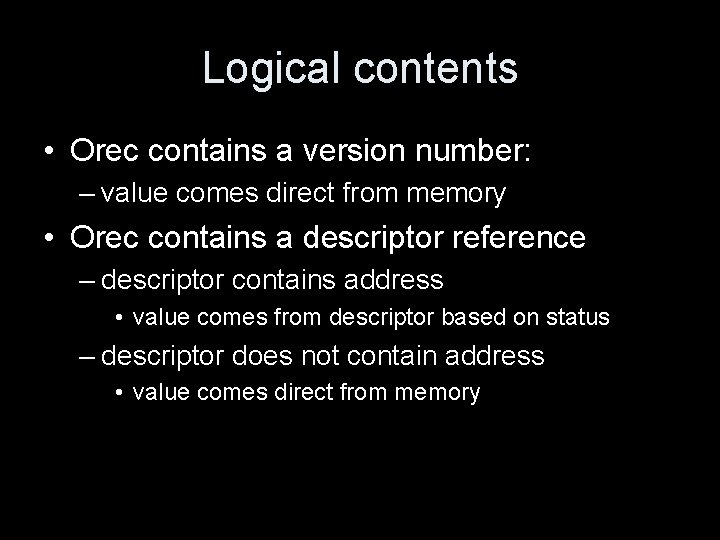
Logical contents • Orec contains a version number: – value comes direct from memory • Orec contains a descriptor reference – descriptor contains address • value comes from descriptor based on status – descriptor does not contain address • value comes direct from memory
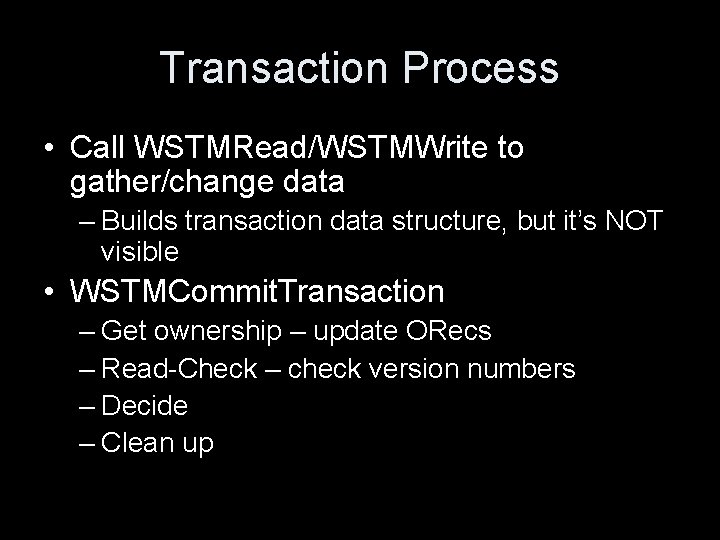
Transaction Process • Call WSTMRead/WSTMWrite to gather/change data – Builds transaction data structure, but it’s NOT visible • WSTMCommit. Transaction – Get ownership – update ORecs – Read-Check – check version numbers – Decide – Clean up
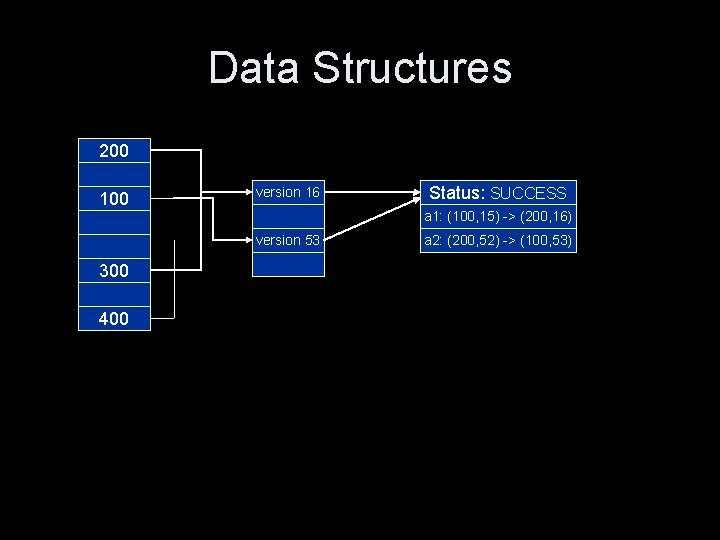
Data Structures 100 200 100 version 16 15 Status: UNKNOWN SUCCESS a 1: (100, 15) -> (200, 16) version 53 52 300 400 a 2: (200, 52) -> (100, 53) (200, 52)
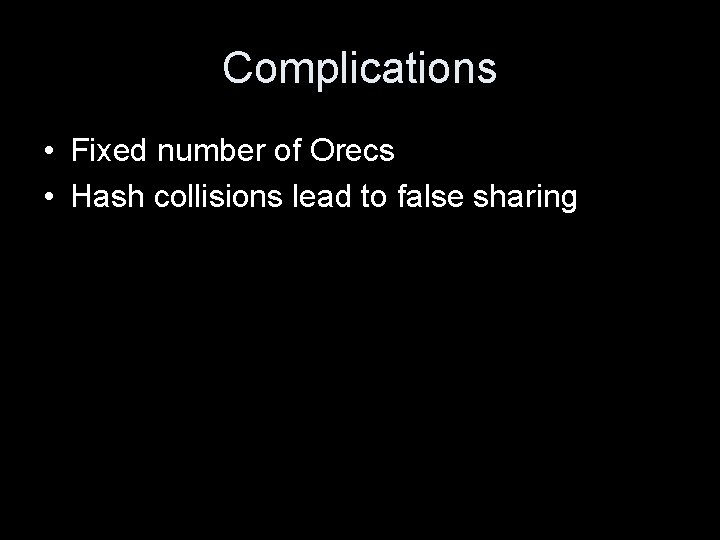
Complications • Fixed number of Orecs • Hash collisions lead to false sharing
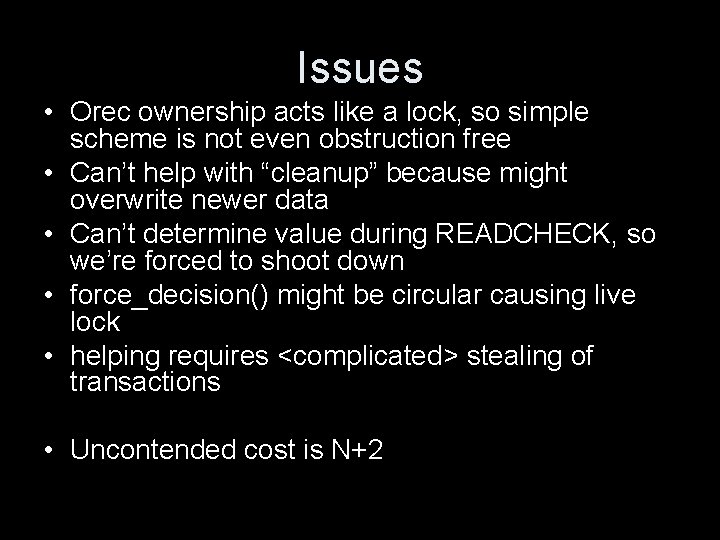
Issues • Orec ownership acts like a lock, so simple scheme is not even obstruction free • Can’t help with “cleanup” because might overwrite newer data • Can’t determine value during READCHECK, so we’re forced to shoot down • force_decision() might be circular causing live lock • helping requires <complicated> stealing of transactions • Uncontended cost is N+2
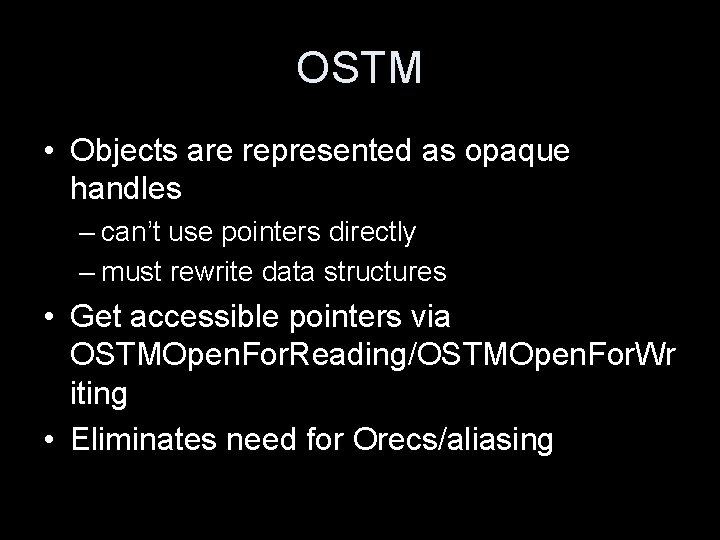
OSTM • Objects are represented as opaque handles – can’t use pointers directly – must rewrite data structures • Get accessible pointers via OSTMOpen. For. Reading/OSTMOpen. For. Wr iting • Eliminates need for Orecs/aliasing
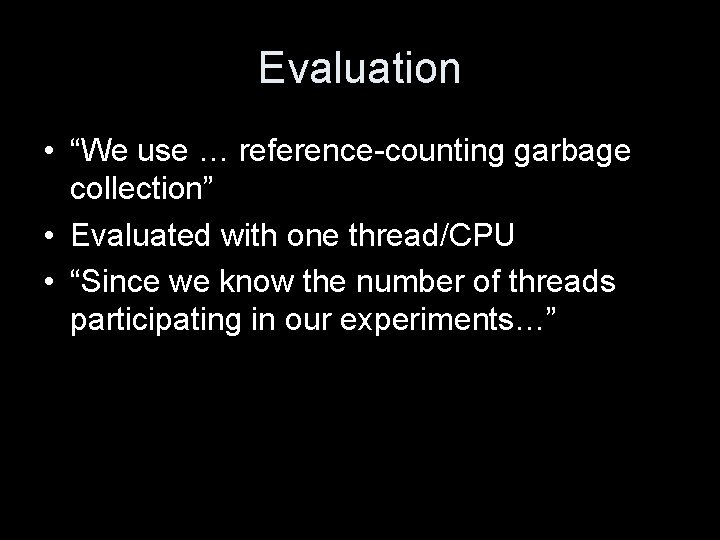
Evaluation • “We use … reference-counting garbage collection” • Evaluated with one thread/CPU • “Since we know the number of threads participating in our experiments…”
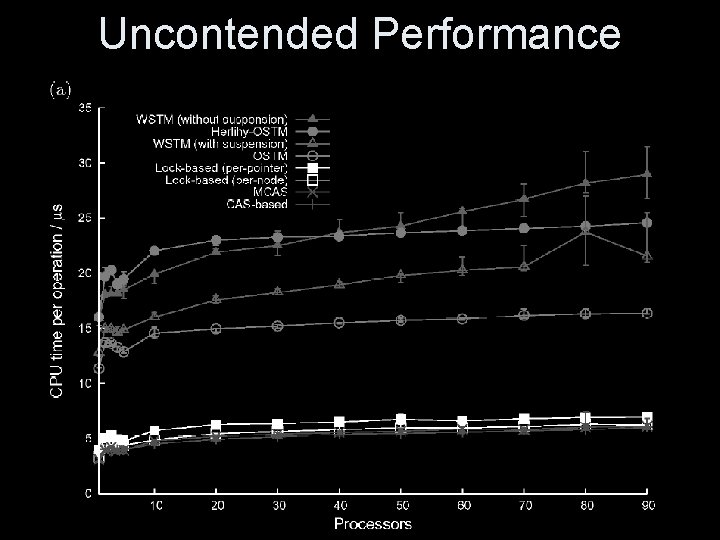
Uncontended Performance
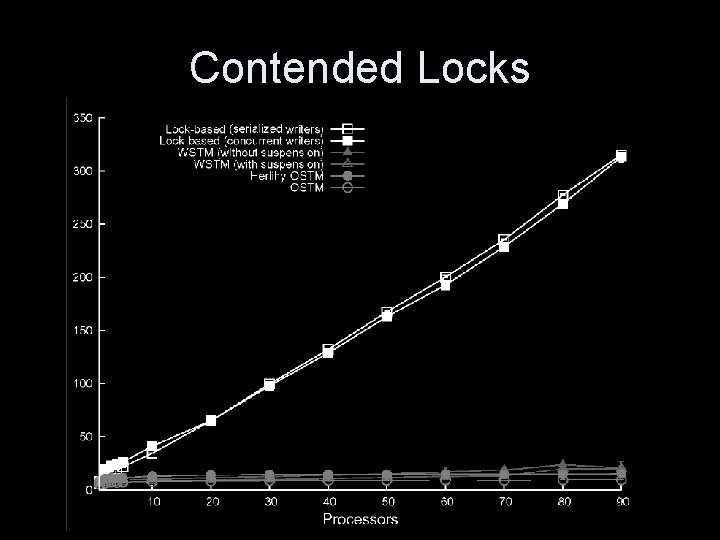
Contended Locks
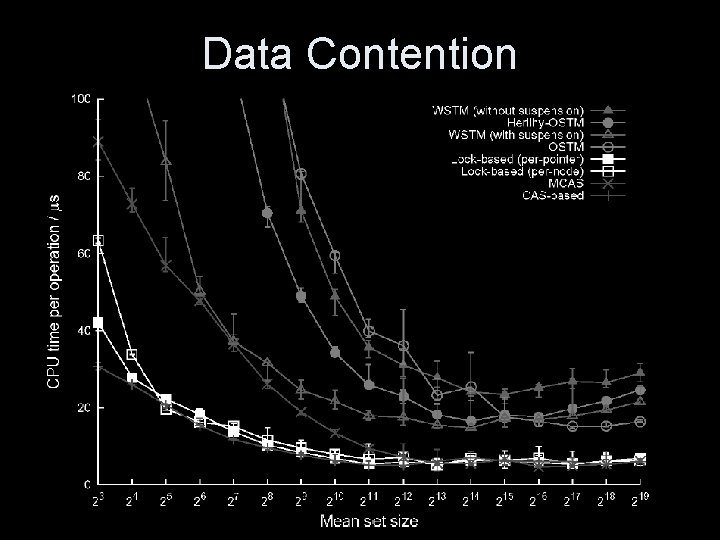
Data Contention
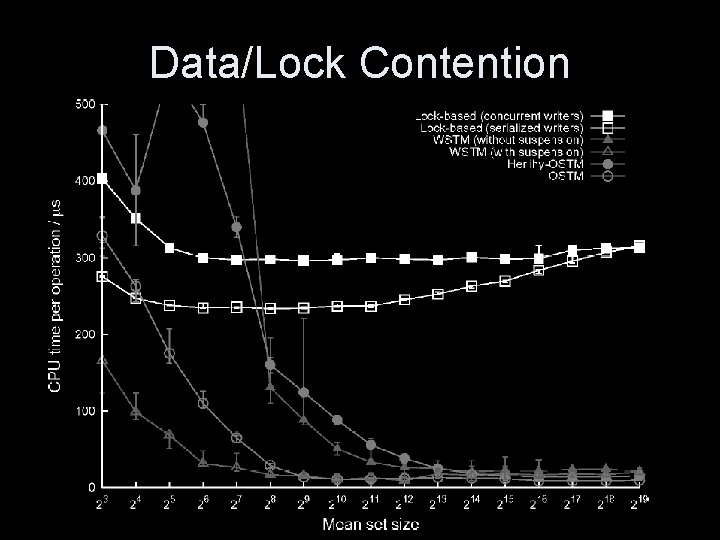
Data/Lock Contention
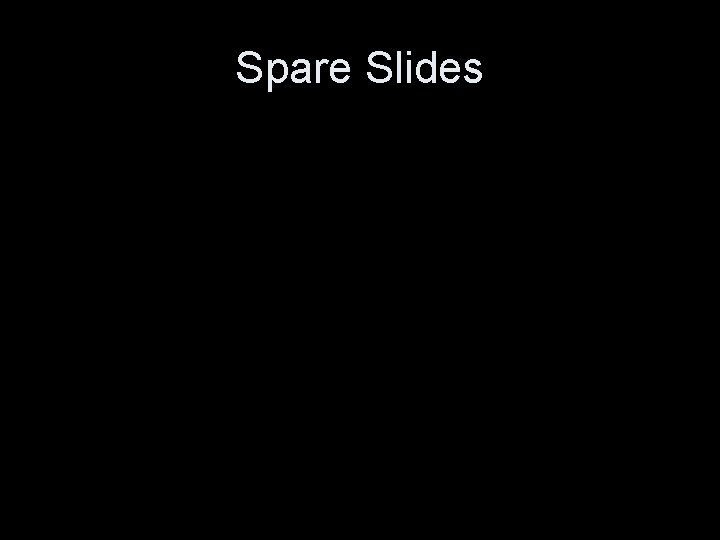
Spare Slides
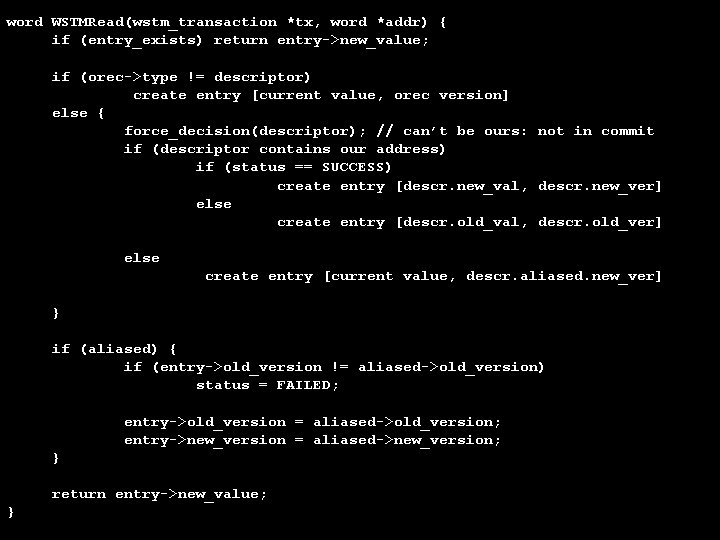
word WSTMRead(wstm_transaction *tx, word *addr) { if (entry_exists) return entry->new_value; if (orec->type != descriptor) create entry [current value, orec version] else { force_decision(descriptor); // can’t be ours: not in commit if (descriptor contains our address) if (status == SUCCESS) create entry [descr. new_val, descr. new_ver] else create entry [descr. old_val, descr. old_ver] else create entry [current value, descr. aliased. new_ver] } if (aliased) { if (entry->old_version != aliased->old_version) status = FAILED; entry->old_version = aliased->old_version; entry->new_version = aliased->new_version; } return entry->new_value; }
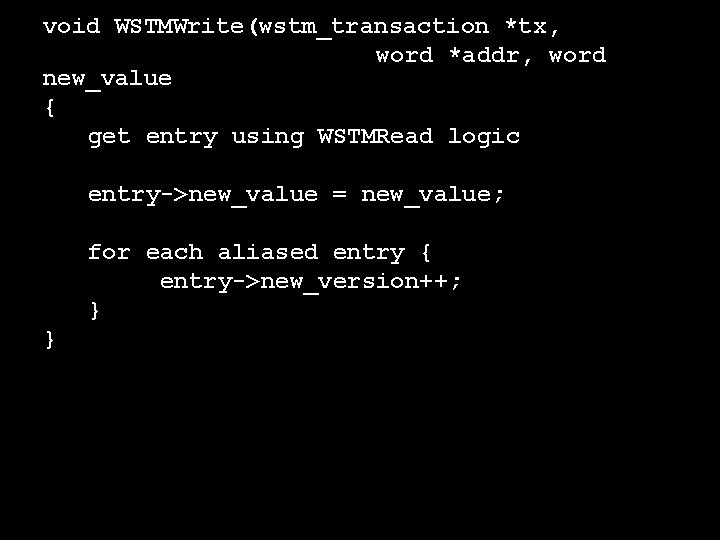
void WSTMWrite(wstm_transaction *tx, word *addr, word new_value { get entry using WSTMRead logic entry->new_value = new_value; for each aliased entry { entry->new_version++; } }
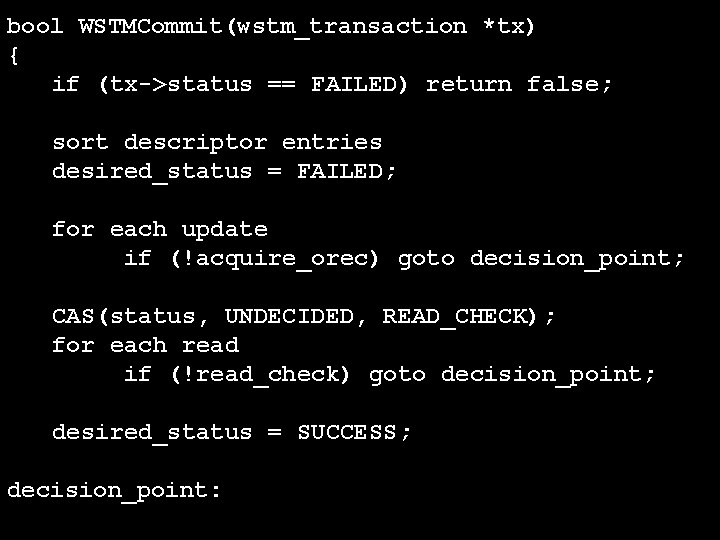
bool WSTMCommit(wstm_transaction *tx) { if (tx->status == FAILED) return false; sort descriptor entries desired_status = FAILED; for each update if (!acquire_orec) goto decision_point; CAS(status, UNDECIDED, READ_CHECK); for each read if (!read_check) goto decision_point; desired_status = SUCCESS; decision_point:
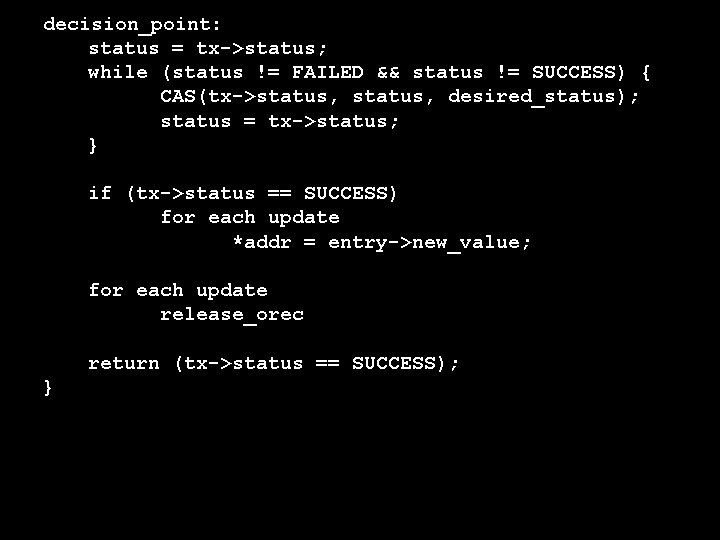
decision_point: status = tx->status; while (status != FAILED && status != SUCCESS) { CAS(tx->status, desired_status); status = tx->status; } if (tx->status == SUCCESS) for each update *addr = entry->new_value; for each update release_orec return (tx->status == SUCCESS); }
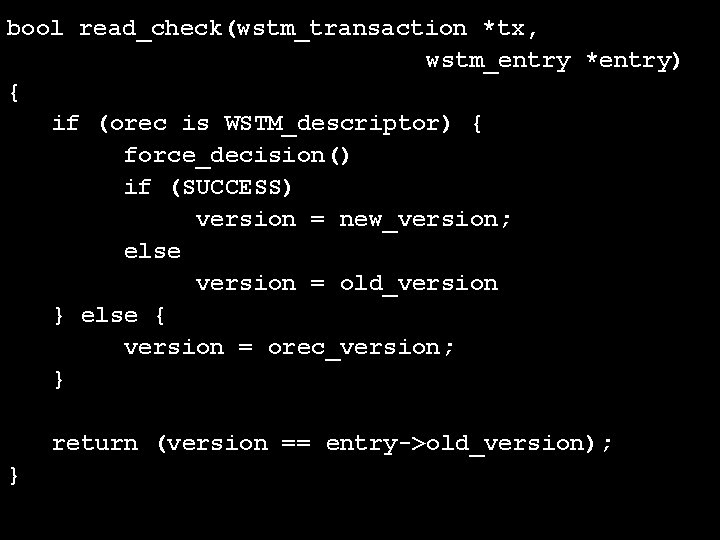
bool read_check(wstm_transaction *tx, wstm_entry *entry) { if (orec is WSTM_descriptor) { force_decision() if (SUCCESS) version = new_version; else version = old_version } else { version = orec_version; } return (version == entry->old_version); }
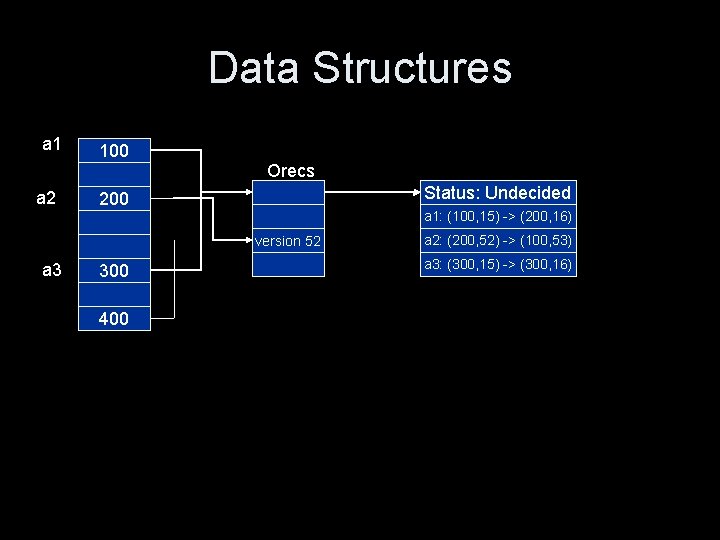
Data Structures a 1 100 Orecs a 2 Status: Undecided 200 a 1: (100, 15) -> (200, 16) version 52 a 3 300 400 a 2: (200, 52) -> (100, 53) a 3: (300, 15) -> (300, 16)
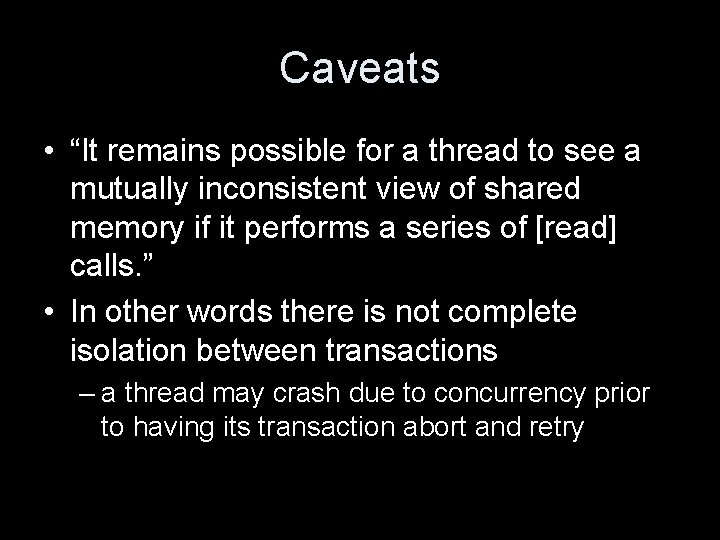
Caveats • “It remains possible for a thread to see a mutually inconsistent view of shared memory if it performs a series of [read] calls. ” • In other words there is not complete isolation between transactions – a thread may crash due to concurrency prior to having its transaction abort and retry
Keir hardie primary school
Laura keir
Keir thelander
Keir hardie primary school
Digital design and computer architecture
Synchronization algorithms and concurrent programming
A lock that allows concurrent transactions
Concurrency control in distributed transactions
Proximal ditch technique
Hydraulic forcible entry tool
Atoms escape room digital locks answers
Acsys locks
Proper term for channel locks
Secondary resistance and retention form features
Forcible entry magnetic locks
Advisory lock postgres
Occ systems
Retention coves
For my father who lived without ceremony
Without a title poem
Without rush without engines poetic device
Linear vs integer programming
Perbedaan linear programming dan integer programming
Definisi integer
Greedy vs dynamic
System programming vs application programming
Karl fraser
Fraser doherty jam
Munro fraser 5 point plan
Simon fraser university statistics
Stuart fraser winnipeg
Double vision
Bethel v fraser summary
Bethel v fraser oyez
Leslye fraser
Fraser doherty superjam
Jennifer fraser
Hosanna brooke fraser
Alison fraser
Mariam fraser body download
Bc fraser health region map
James fraser md mph
Ap john fraser
C dalrymple-fraser
Sfu surrey library
The practice of public relations
Samuel l jackson mbti
Aspect en toit de pagode
Mesa disociativa de fraser
Adcb aed to inr
James fraser naval aviator
Jamilah fraser
Hemsley fraser project management
Sara strauß upb
Itslearning fraser
Dr fraser duncan
Bethel school district #43 v. fraser
Bethel school district #43 v. fraser (1987)
Postal rule
Zerspanungsgrößen
Hussey fraser
James fraser naval aviator
Parallel processing vs concurrent processing
Transaction management in dbms
Concurrent engineering in project management
Force system statics
Example of concurrent powers
Swot redbull
Types of validity and reliability
Concurrent activity in network diagram
Contoh pemrograman konkuren
Concurrent process model in embedded systems
Concurrent planning definition
York university my file
Retrospective validation