Modern C tips tricks Kamil Szatkowski kamil szatkowskinokia
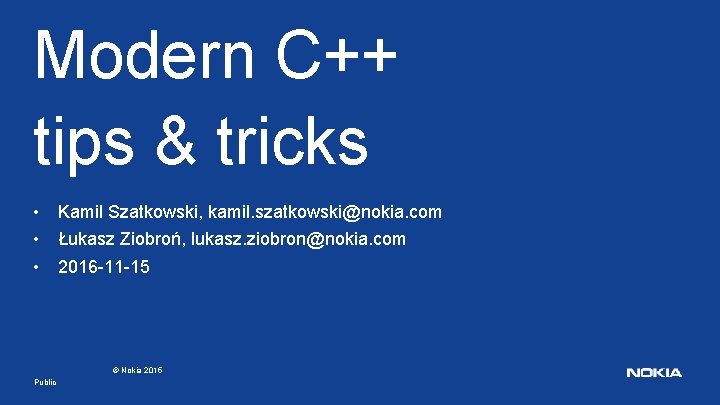
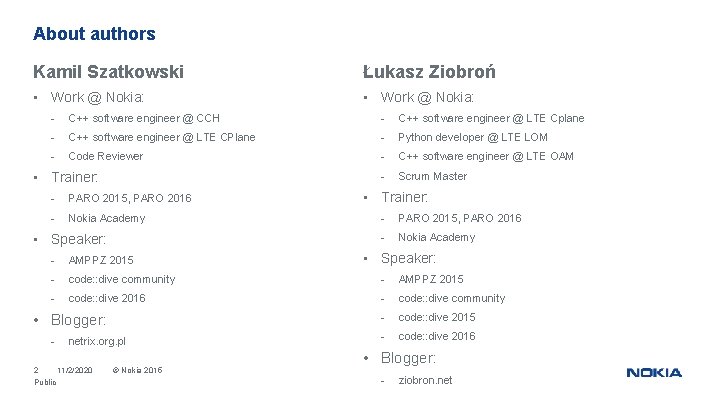
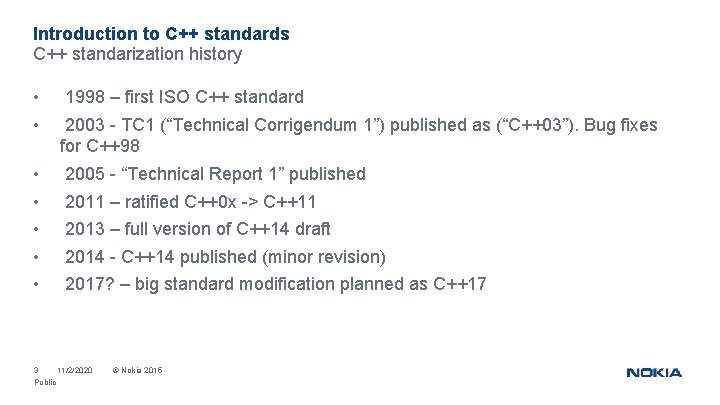
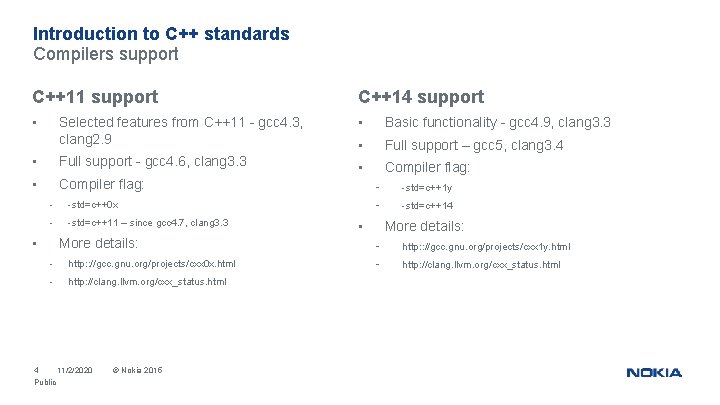
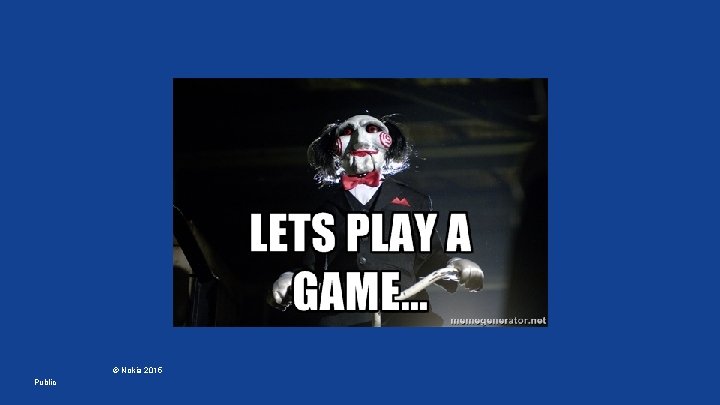
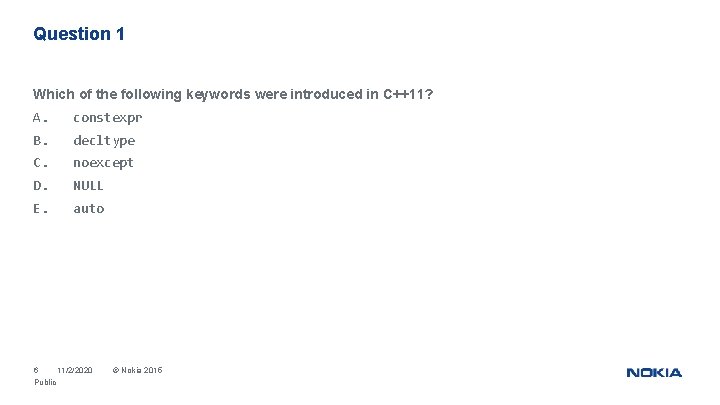
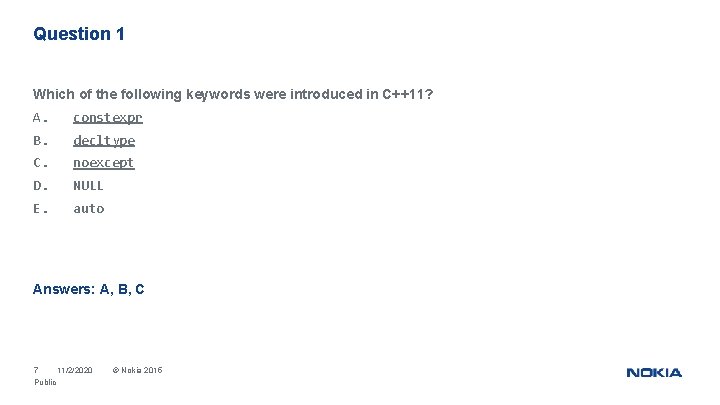
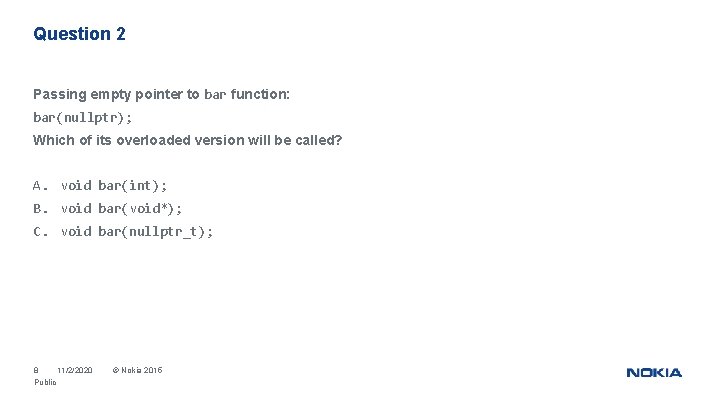
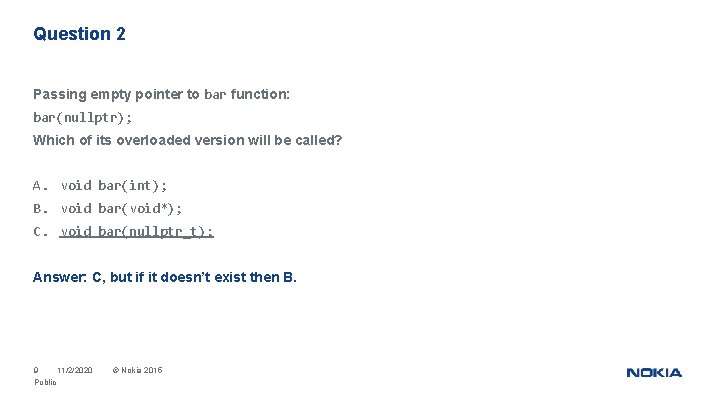
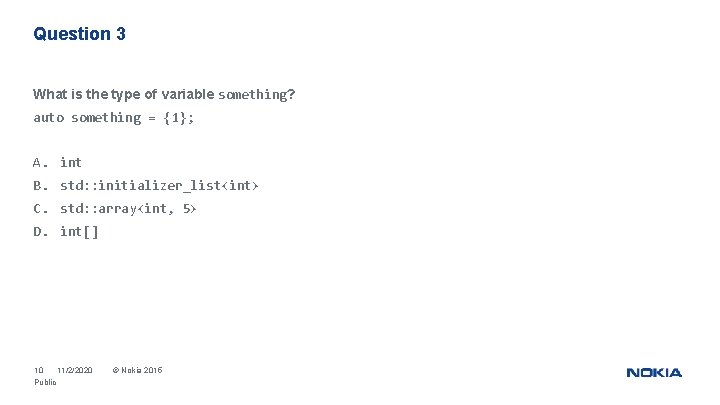
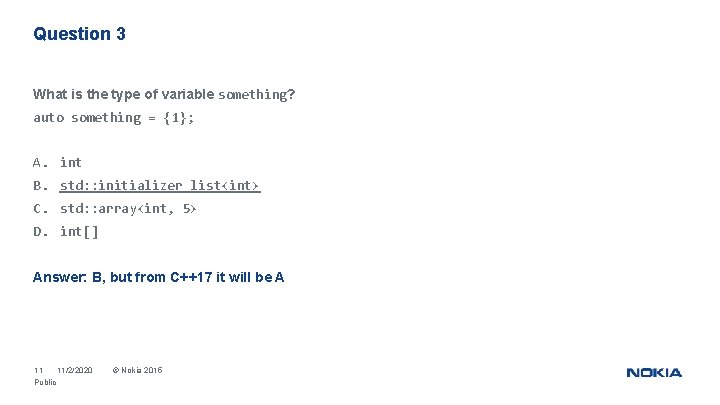
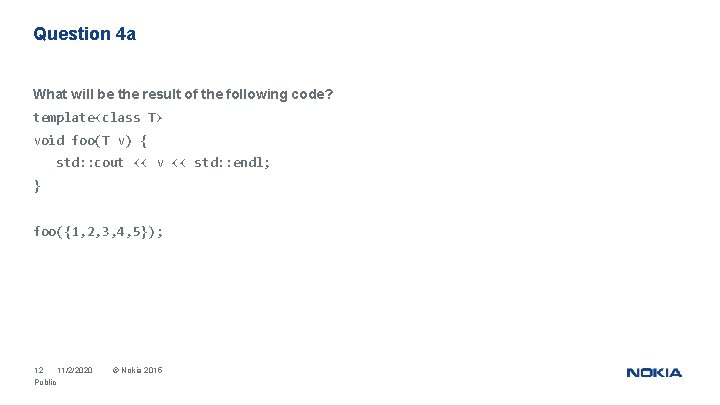
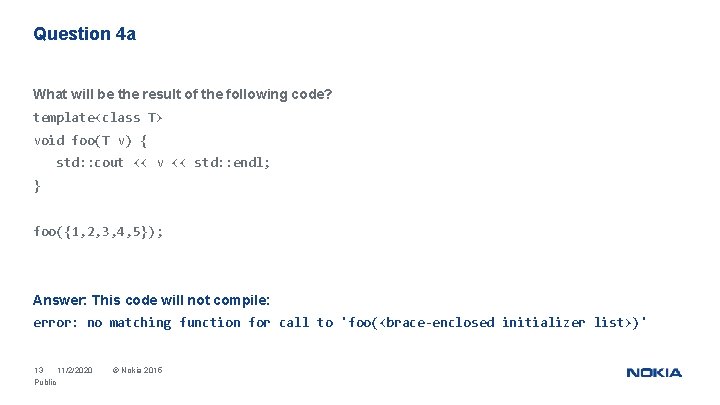
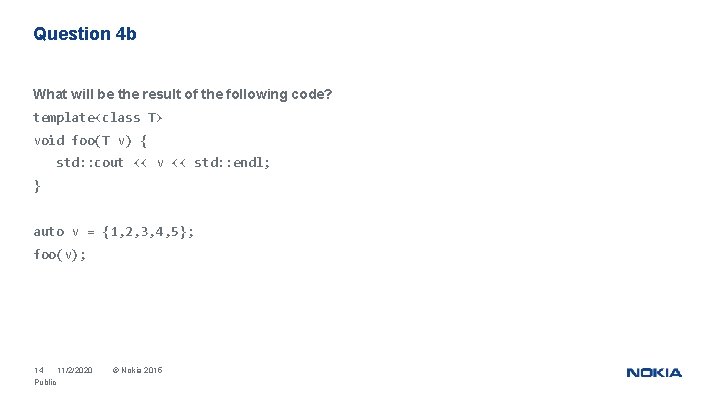
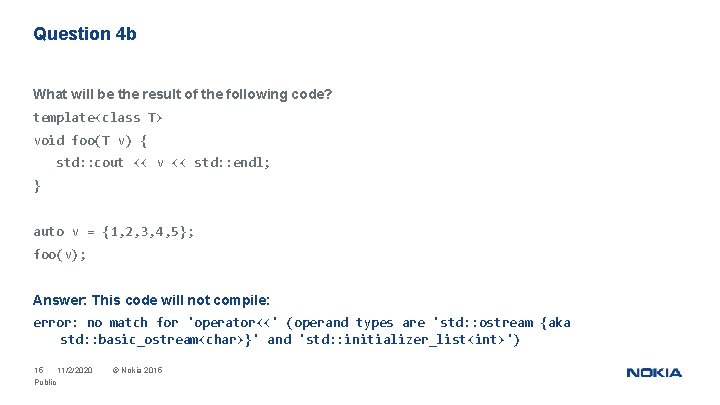
![Question 5 Will it compile? int array[] = { 1, 2, 5. 5 }; Question 5 Will it compile? int array[] = { 1, 2, 5. 5 };](https://slidetodoc.com/presentation_image/3f5829670a40bc73839a88fcfe3652d6/image-16.jpg)
![Question 5 Will it compile? int array[] = { 1, 2, 5. 5 }; Question 5 Will it compile? int array[] = { 1, 2, 5. 5 };](https://slidetodoc.com/presentation_image/3f5829670a40bc73839a88fcfe3652d6/image-17.jpg)
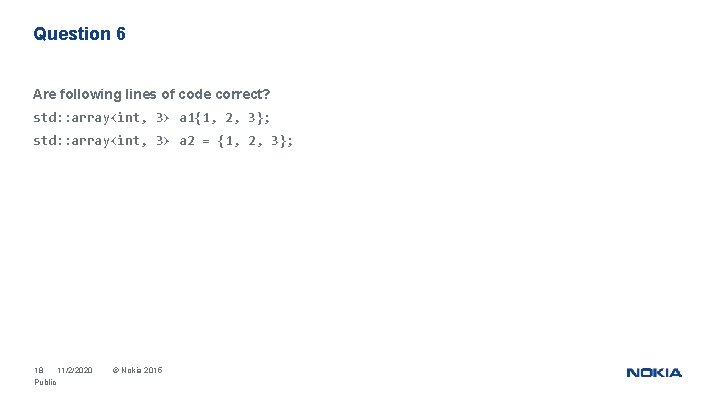
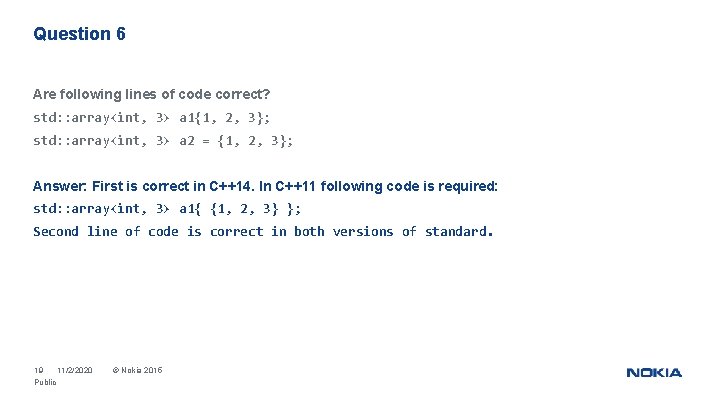
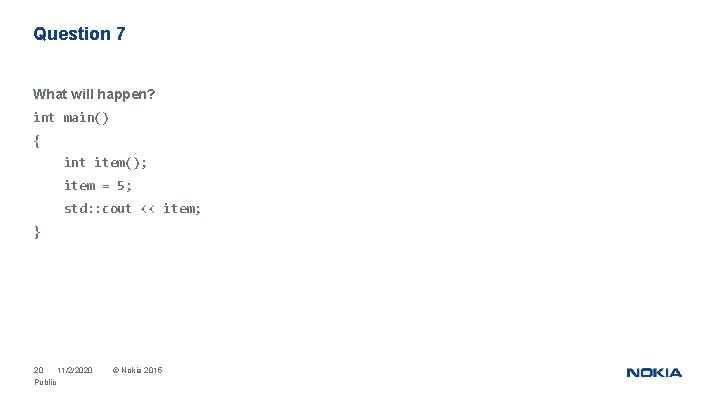
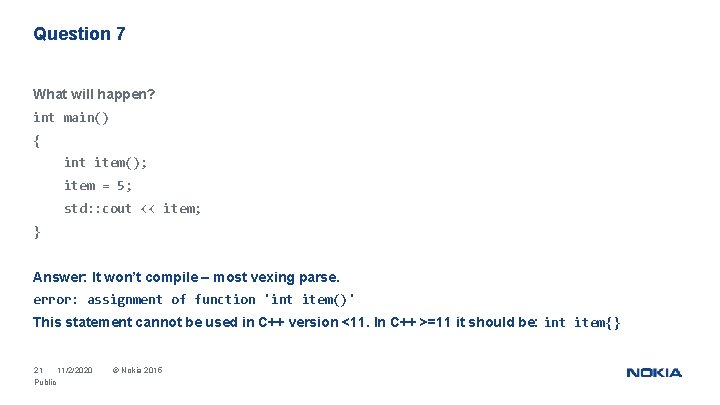
![Question 8 a What is the type of g 1? Gadget items[10]; auto g Question 8 a What is the type of g 1? Gadget items[10]; auto g](https://slidetodoc.com/presentation_image/3f5829670a40bc73839a88fcfe3652d6/image-22.jpg)
![Question 8 a What is the type of g 1? Gadget items[10]; auto g Question 8 a What is the type of g 1? Gadget items[10]; auto g](https://slidetodoc.com/presentation_image/3f5829670a40bc73839a88fcfe3652d6/image-23.jpg)
![Question 8 b What is the type of g 2? Gadget items[10]; auto & Question 8 b What is the type of g 2? Gadget items[10]; auto &](https://slidetodoc.com/presentation_image/3f5829670a40bc73839a88fcfe3652d6/image-24.jpg)
![Question 8 b What is the type of g 2? Gadget items[10]; auto & Question 8 b What is the type of g 2? Gadget items[10]; auto &](https://slidetodoc.com/presentation_image/3f5829670a40bc73839a88fcfe3652d6/image-25.jpg)
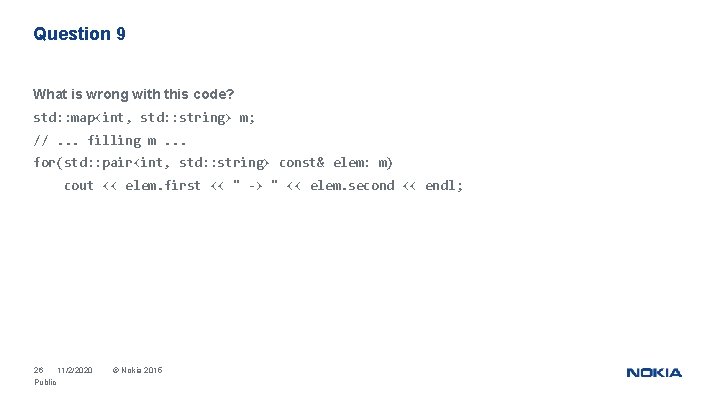
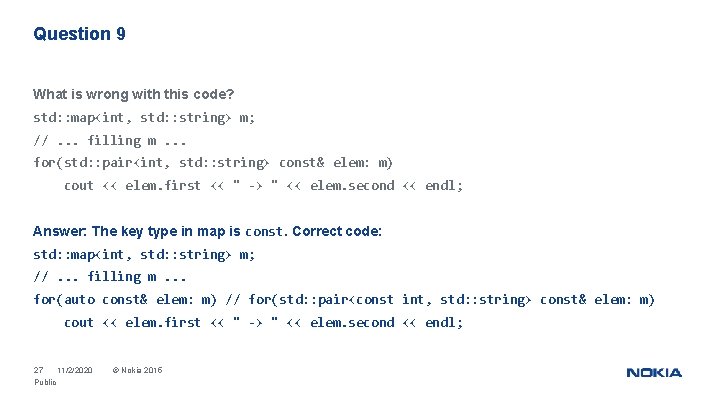
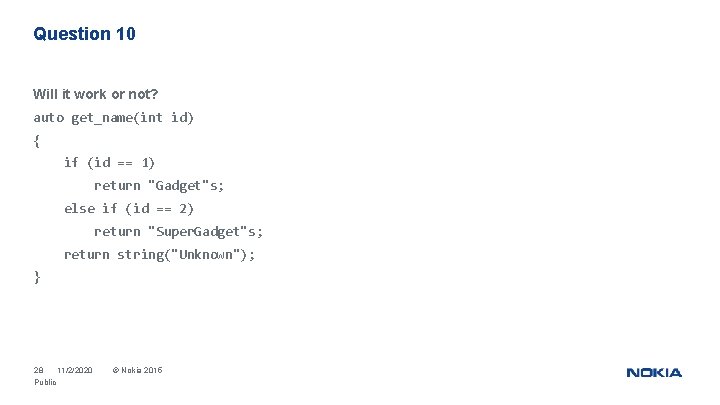
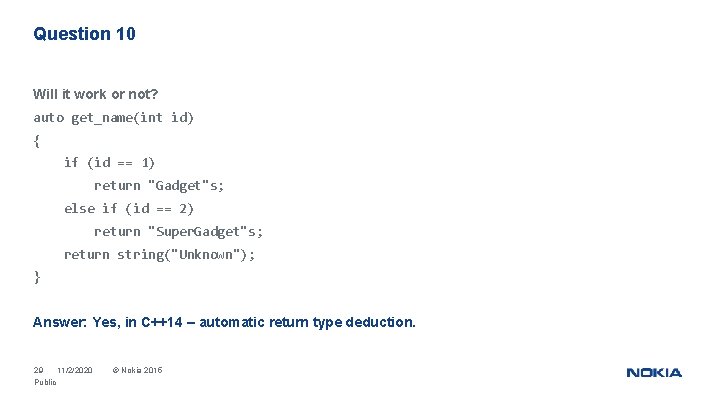
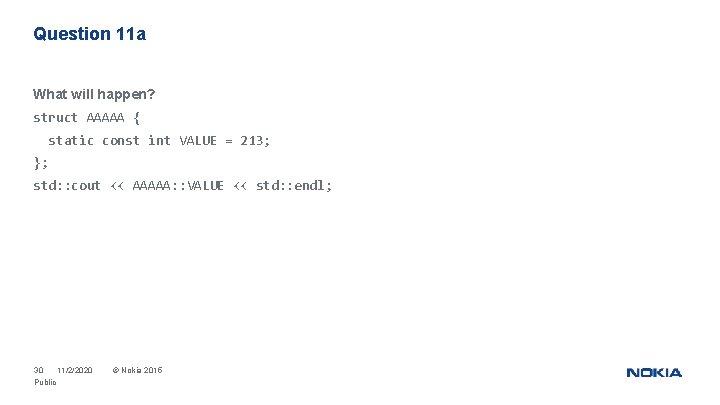
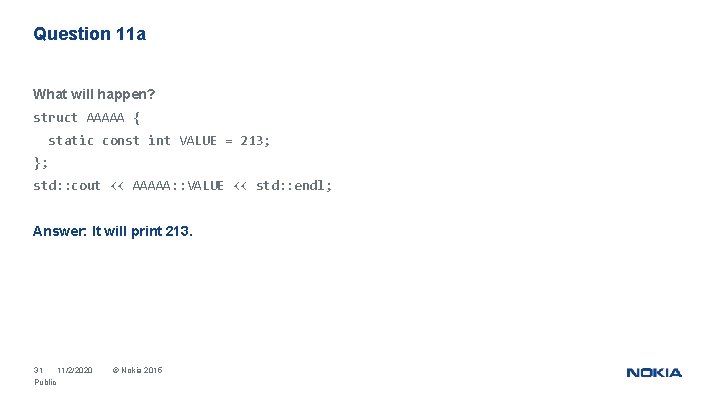
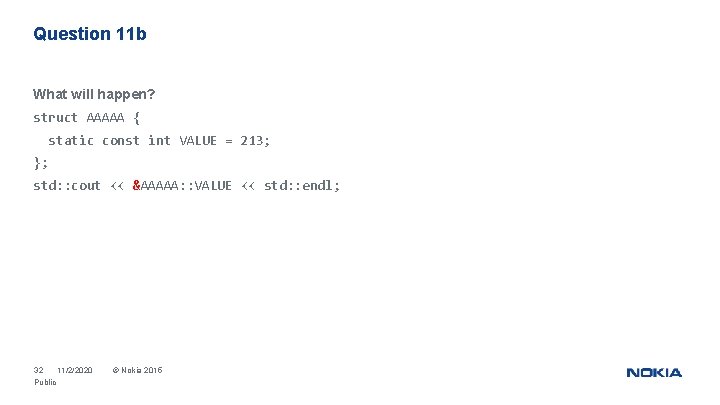
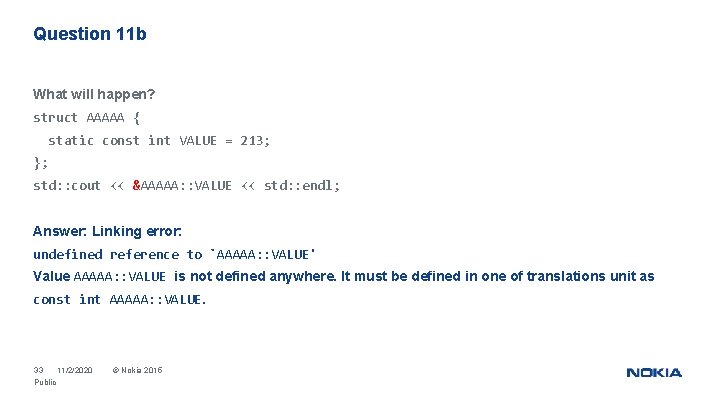
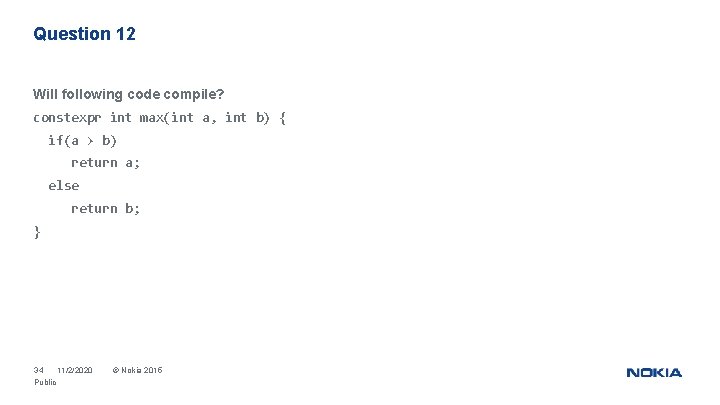
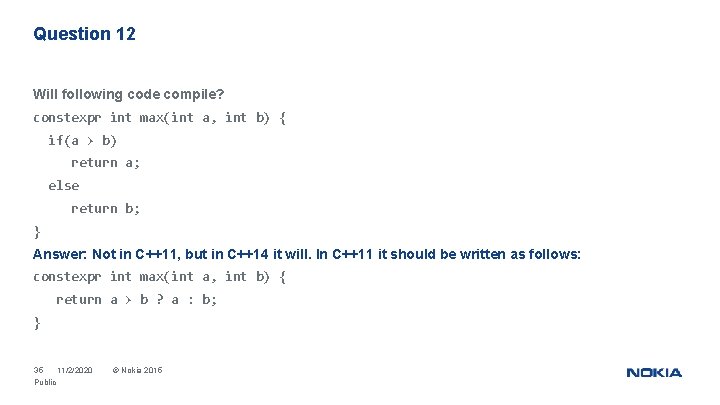
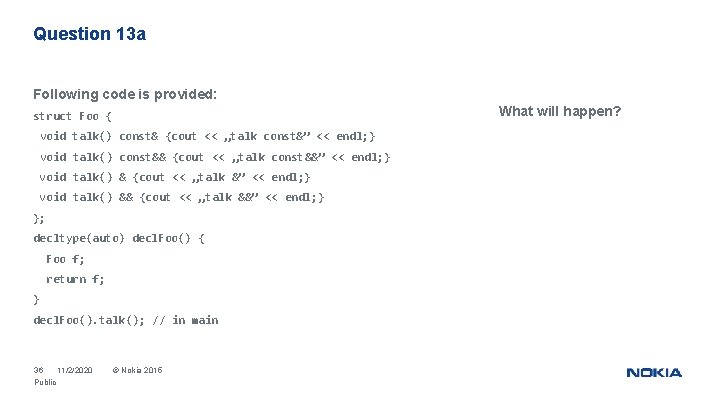
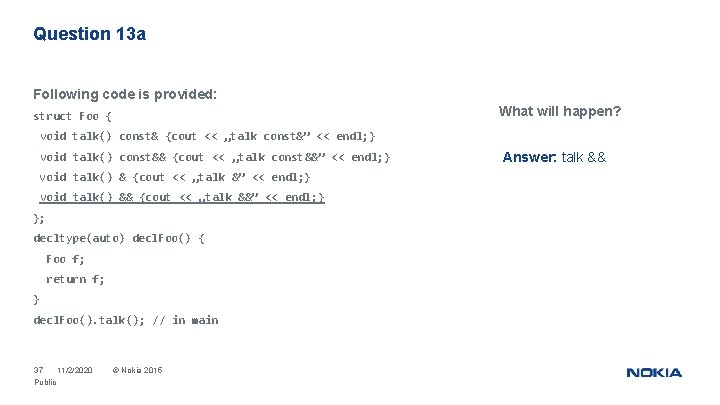
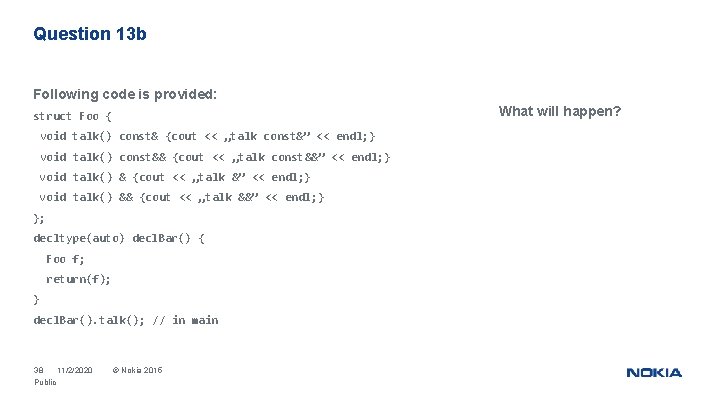
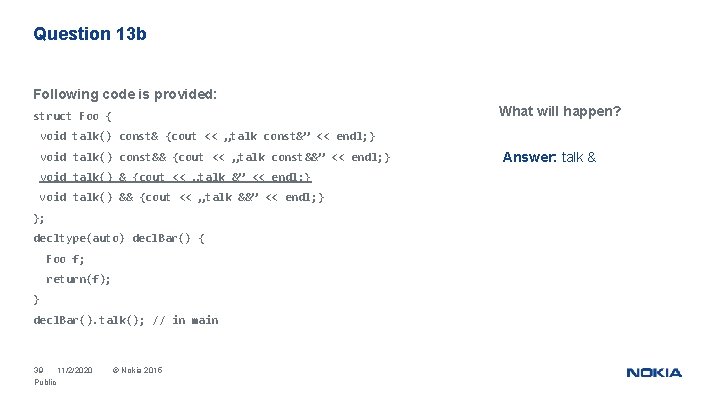
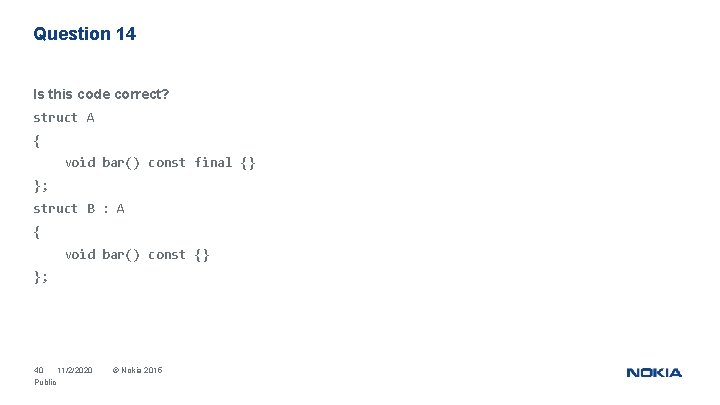
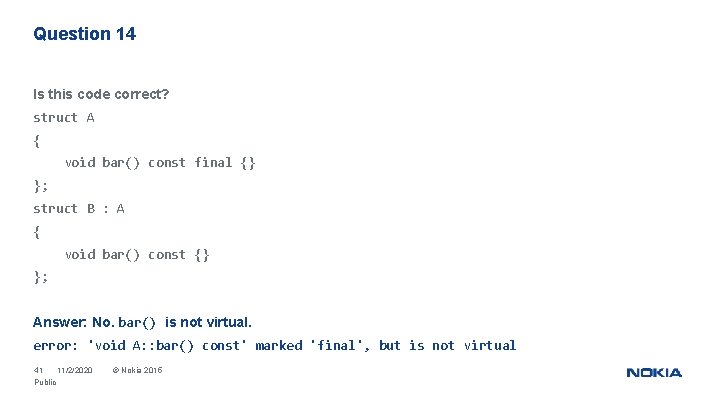
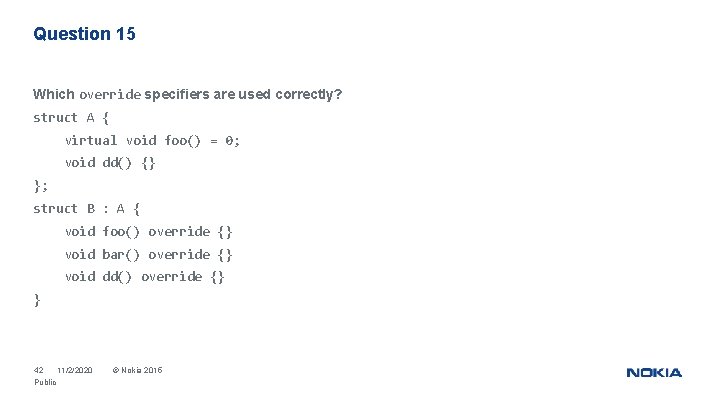
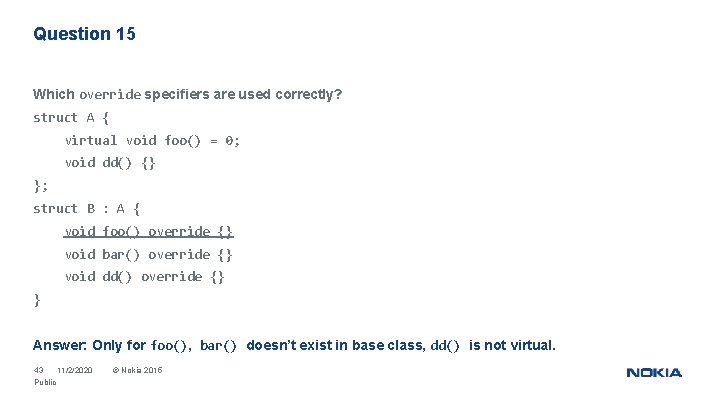
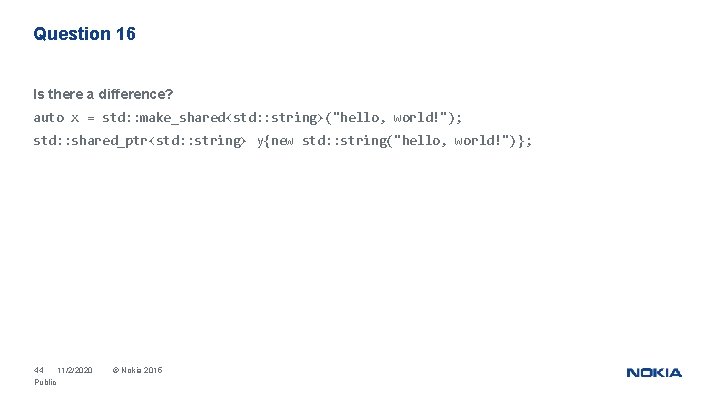
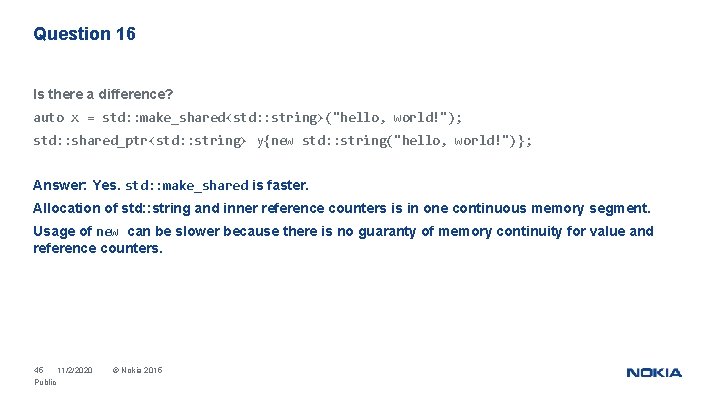
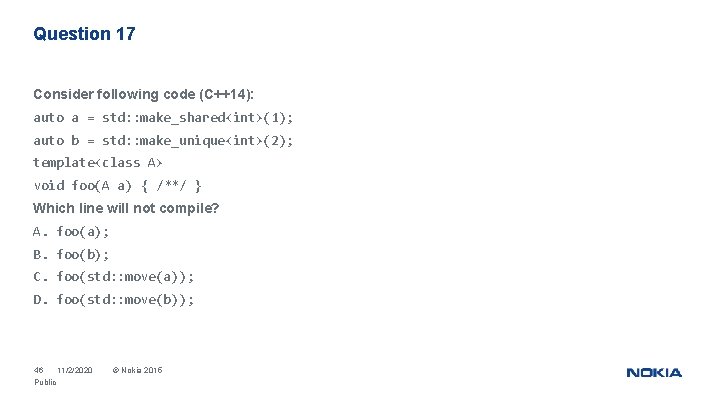
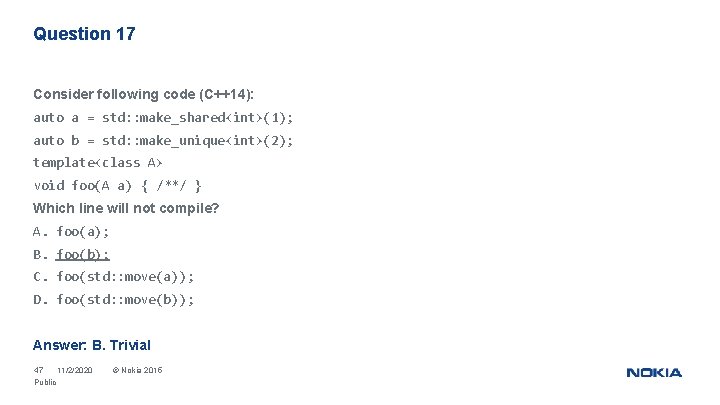
![Question 18 Which line will not compile? A. [](){}; B. []{}; C. {}; D. Question 18 Which line will not compile? A. [](){}; B. []{}; C. {}; D.](https://slidetodoc.com/presentation_image/3f5829670a40bc73839a88fcfe3652d6/image-48.jpg)
![Question 18 Which line will not compile? A. [](){}; B. []{}; C. {}; D. Question 18 Which line will not compile? A. [](){}; B. []{}; C. {}; D.](https://slidetodoc.com/presentation_image/3f5829670a40bc73839a88fcfe3652d6/image-49.jpg)
![Question 19 Which lambda function is correct? A. []() -> int { return 4; Question 19 Which lambda function is correct? A. []() -> int { return 4;](https://slidetodoc.com/presentation_image/3f5829670a40bc73839a88fcfe3652d6/image-50.jpg)
![Question 19 Which lambda function is correct? A. []() -> int { return 4; Question 19 Which lambda function is correct? A. []() -> int { return 4;](https://slidetodoc.com/presentation_image/3f5829670a40bc73839a88fcfe3652d6/image-51.jpg)
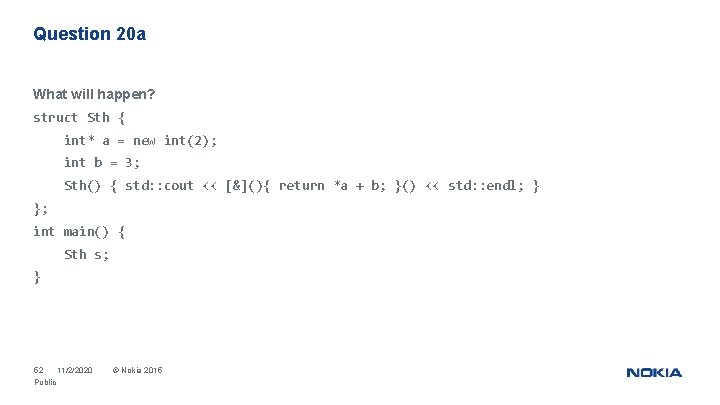
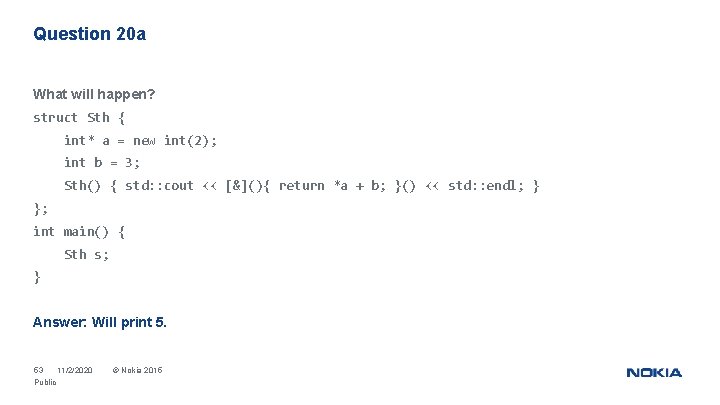
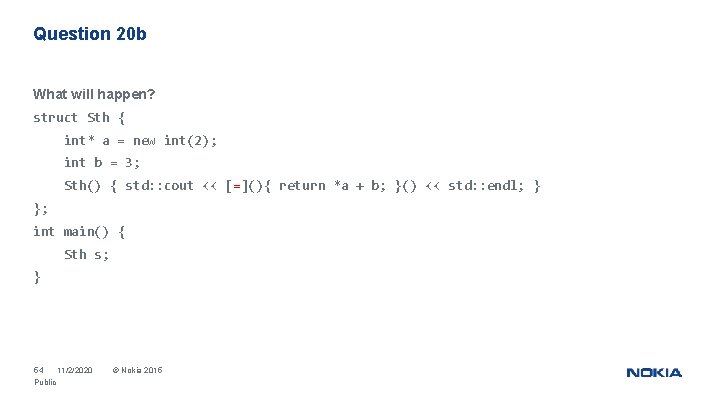
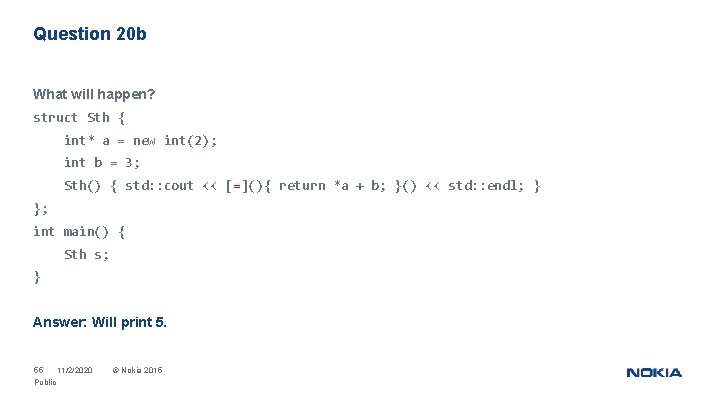
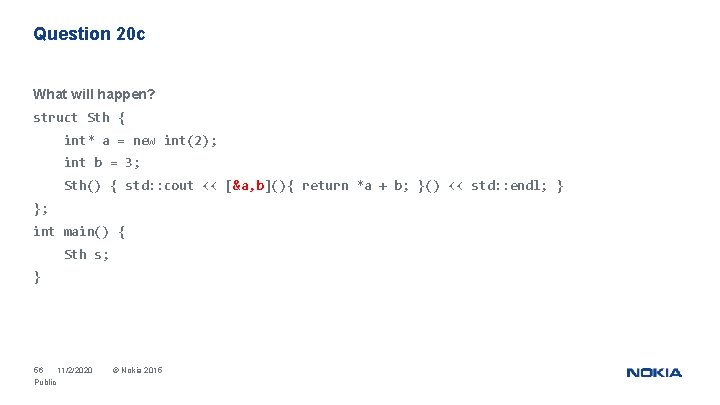
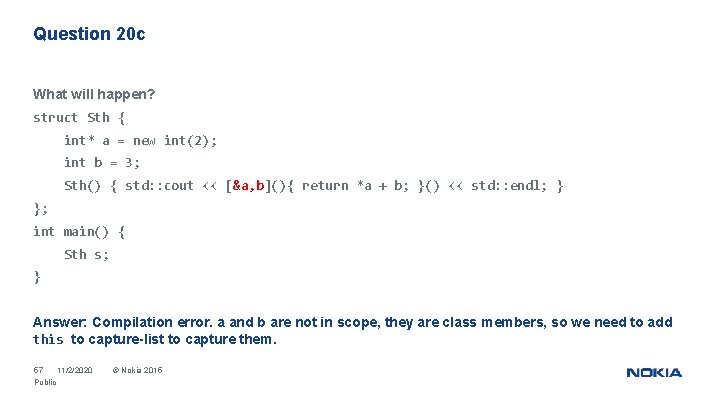
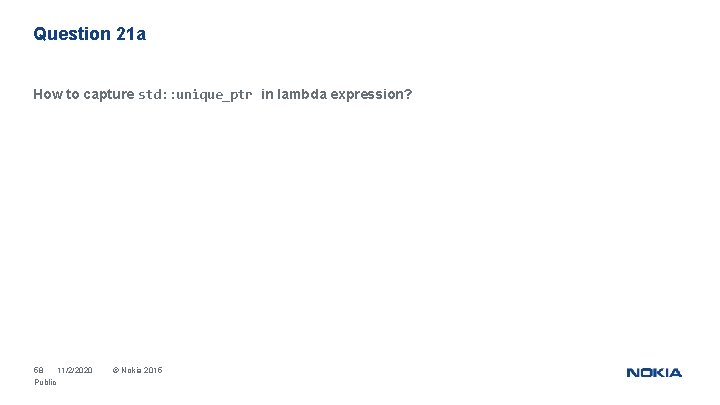
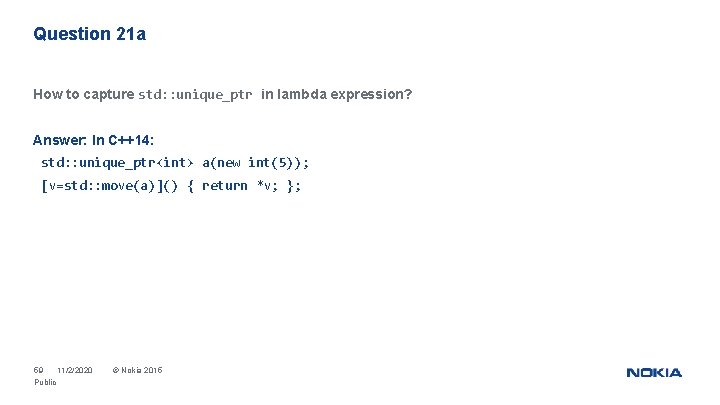
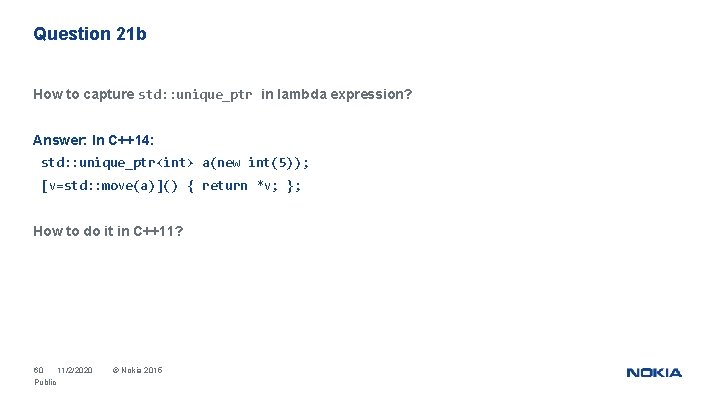
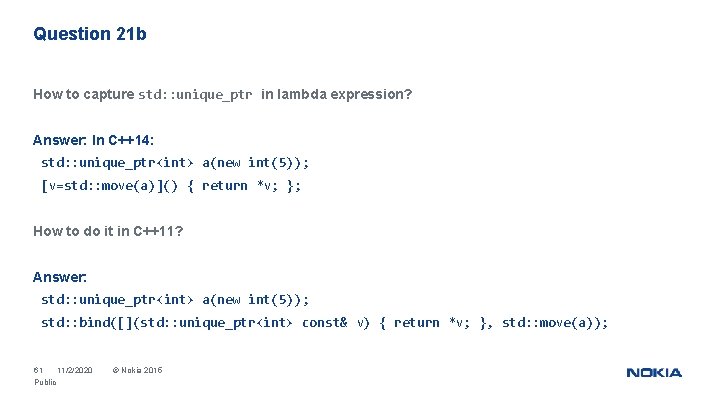
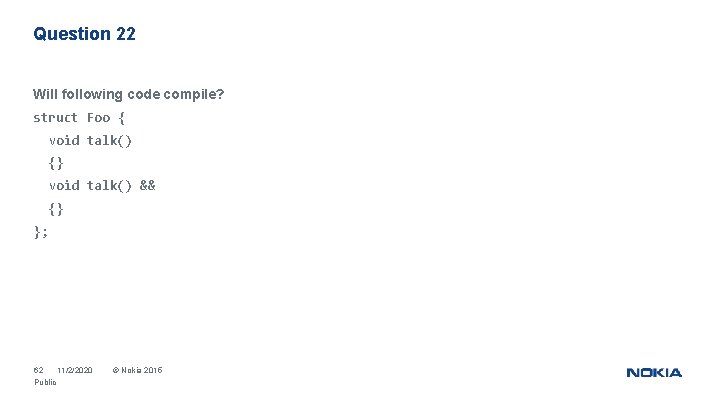
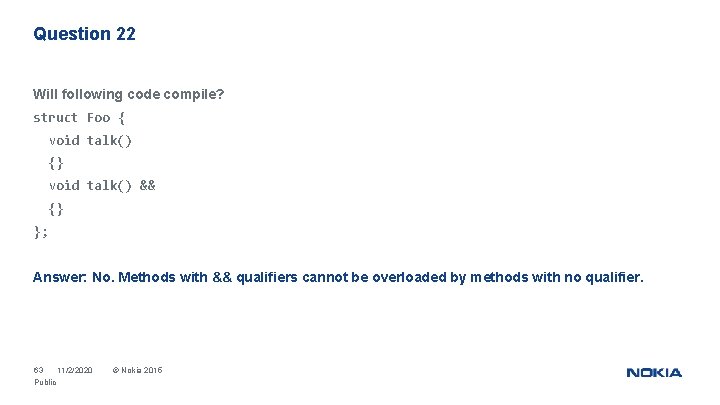
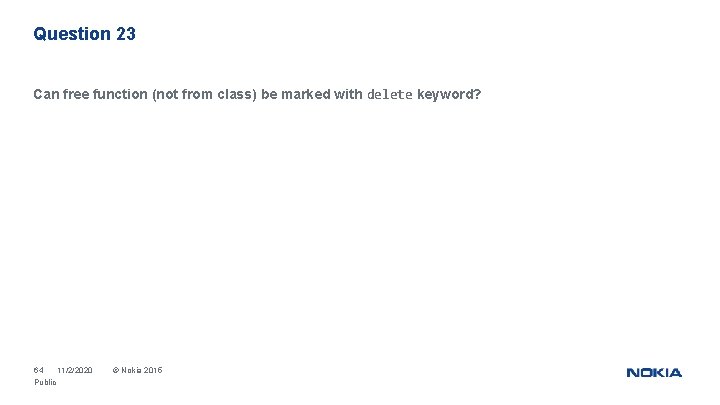
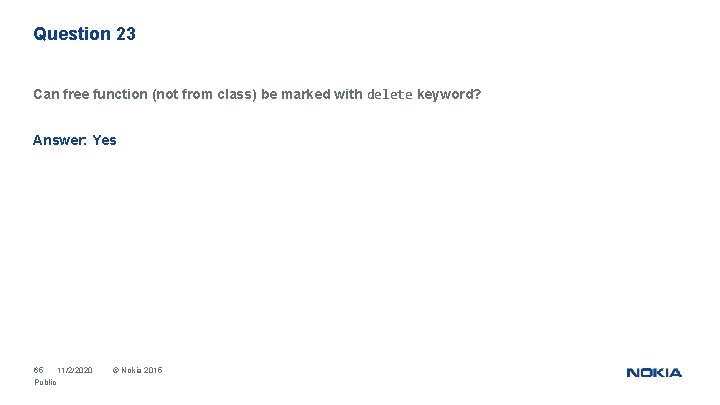
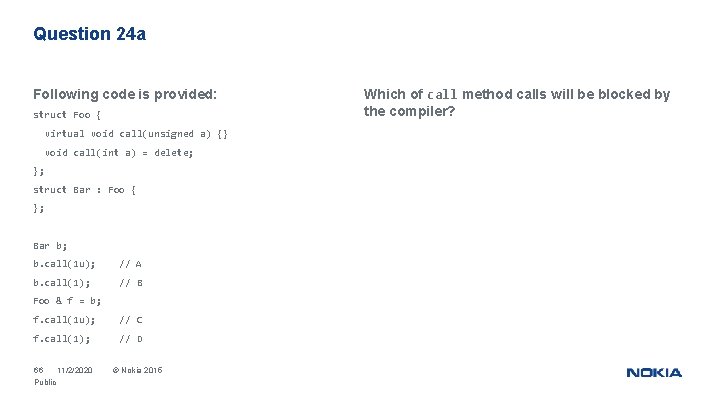
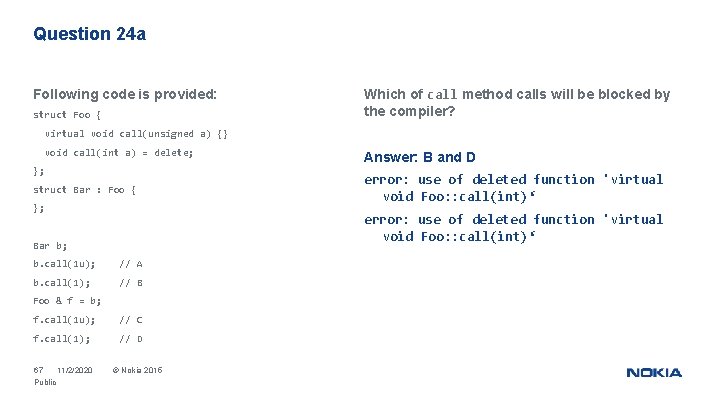
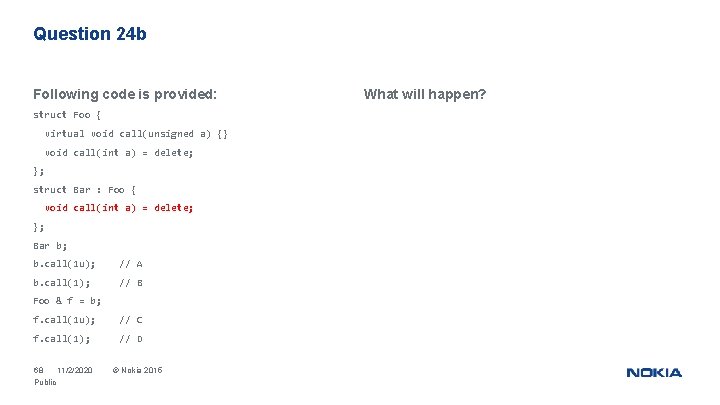
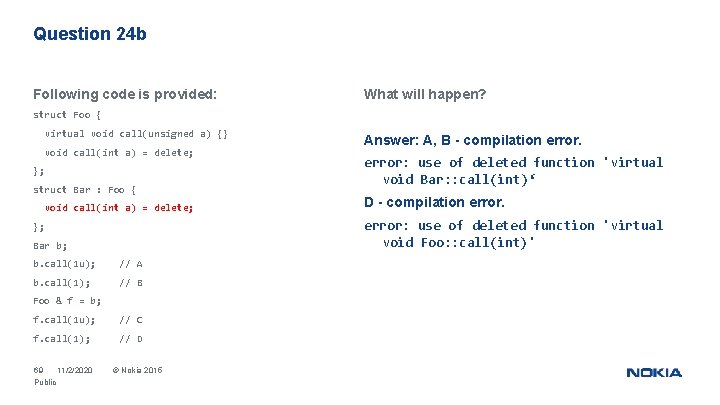
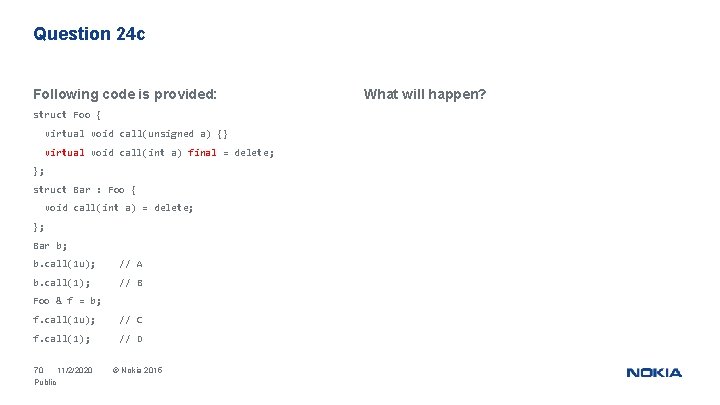
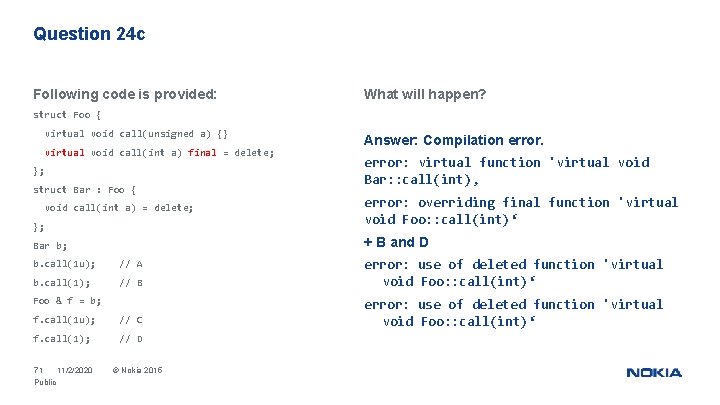
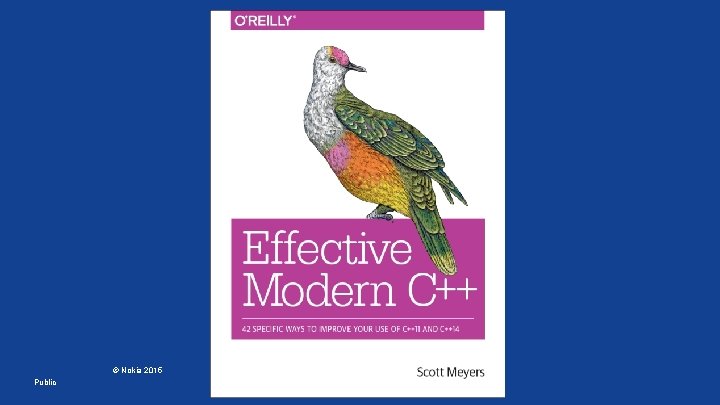
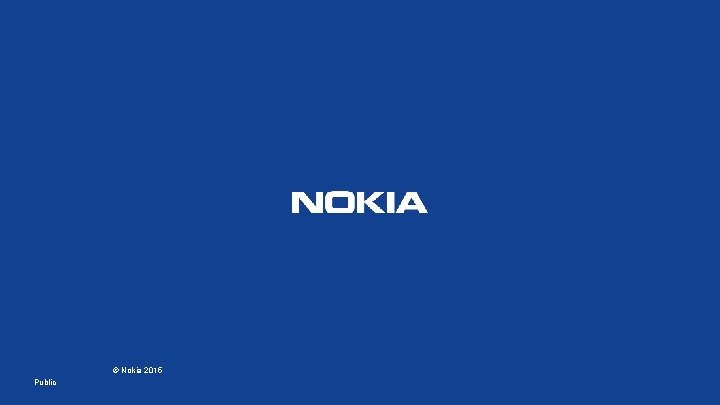
- Slides: 73
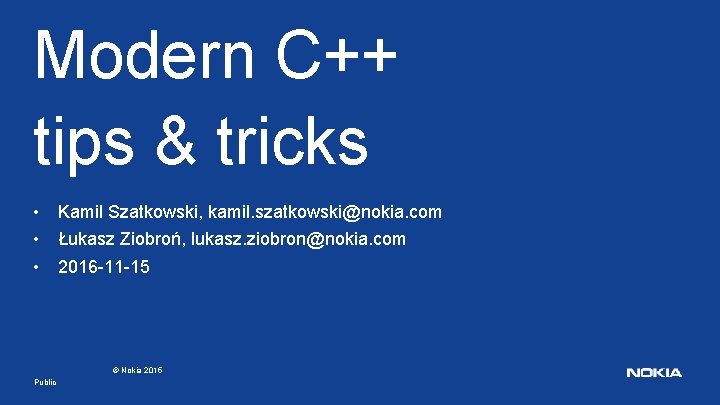
Modern C++ tips & tricks • • • Kamil Szatkowski, kamil. szatkowski@nokia. com Łukasz Ziobroń, lukasz. ziobron@nokia. com 2016 -11 -15 1 11/2/2020 Public © Nokia 2015
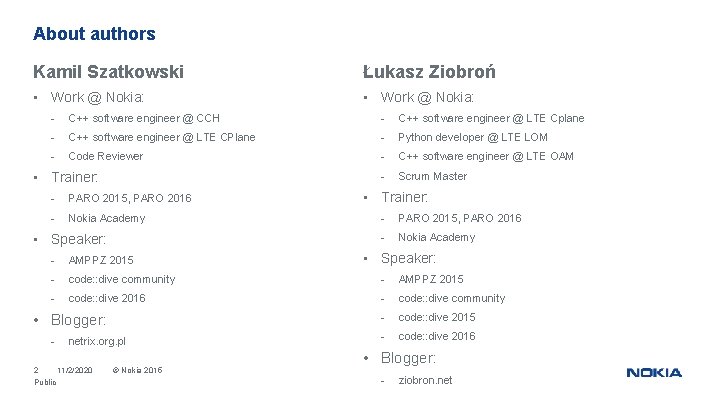
About authors Kamil Szatkowski Łukasz Ziobroń • Work @ Nokia: - C++ software engineer @ CCH - C++ software engineer @ LTE Cplane - C++ software engineer @ LTE CPlane - Python developer @ LTE LOM - Code Reviewer - C++ software engineer @ LTE OAM - Scrum Master • Trainer: - PARO 2015, PARO 2016 - Nokia Academy • Speaker: - AMPPZ 2015 - code: : dive community - AMPPZ 2015 - code: : dive 2016 - code: : dive community - code: : dive 2015 - code: : dive 2016 • Blogger: - netrix. org. pl 2 11/2/2020 Public © Nokia 2015 • Blogger: - ziobron. net
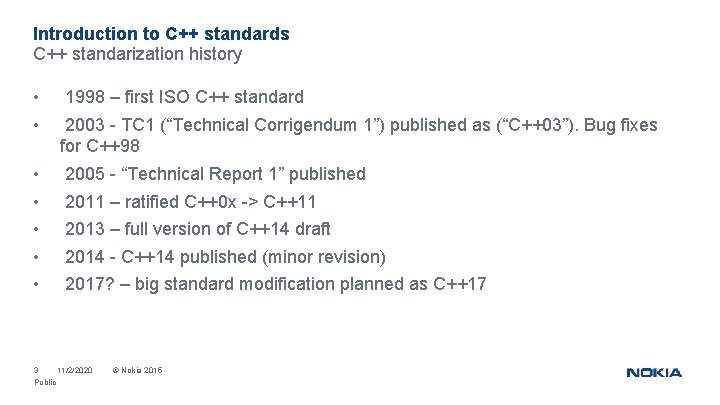
Introduction to C++ standards C++ standarization history • • 1998 – first ISO C++ standard 2003 - TC 1 (“Technical Corrigendum 1”) published as (“C++03”). Bug fixes for C++98 2005 - “Technical Report 1” published 2011 – ratified C++0 x -> C++11 2013 – full version of C++14 draft 2014 - C++14 published (minor revision) 2017? – big standard modification planned as C++17 3 11/2/2020 Public © Nokia 2015
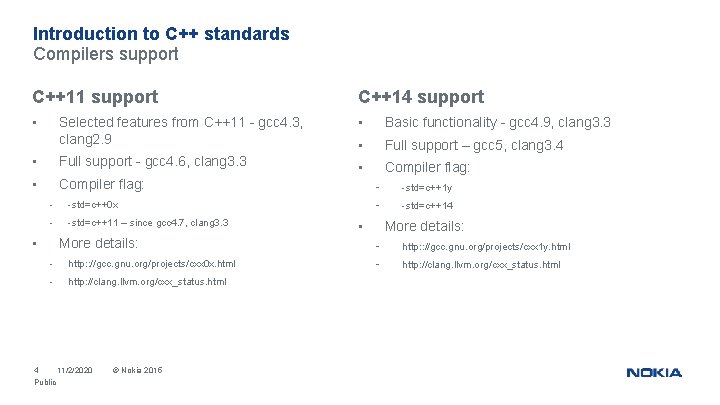
Introduction to C++ standards Compilers support C++11 support C++14 support • Selected features from C++11 - gcc 4. 3, clang 2. 9 • Basic functionality - gcc 4. 9, clang 3. 3 • Full support – gcc 5, clang 3. 4 • Full support - gcc 4. 6, clang 3. 3 • Compiler flag: - -std=c++0 x - -std=c++11 – since gcc 4. 7, clang 3. 3 • More details: - http: : //gcc. gnu. org/projects/cxx 0 x. html - http: //clang. llvm. org/cxx_status. html 4 11/2/2020 Public © Nokia 2015 ‑ -std=c++1 y ‑ -std=c++14 • More details: ‑ http: : //gcc. gnu. org/projects/cxx 1 y. html ‑ http: //clang. llvm. org/cxx_status. html
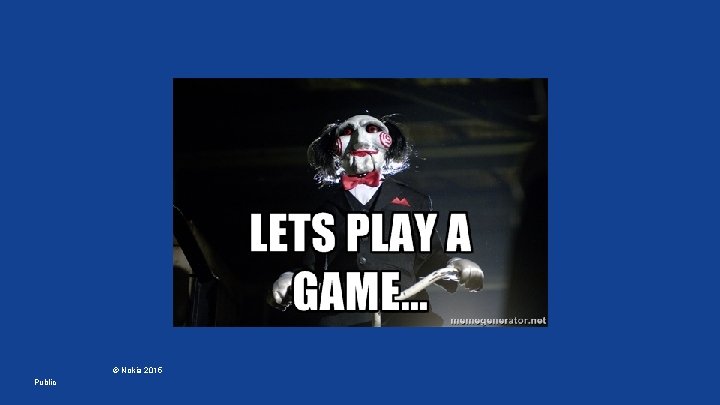
5 11/2/2020 Public © Nokia 2015
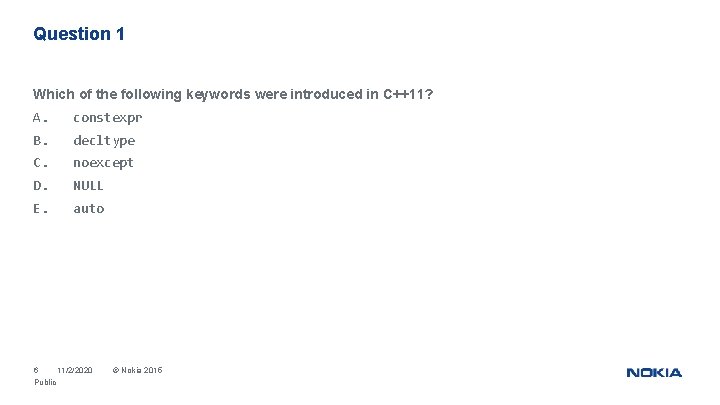
Question 1 Which of the following keywords were introduced in C++11? A. constexpr B. decltype C. noexcept D. NULL E. auto 6 11/2/2020 Public © Nokia 2015
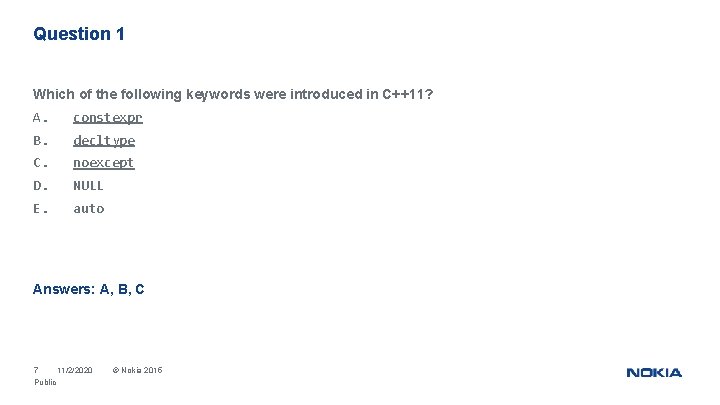
Question 1 Which of the following keywords were introduced in C++11? A. constexpr B. decltype C. noexcept D. NULL E. auto Answers: A, B, C 7 11/2/2020 Public © Nokia 2015
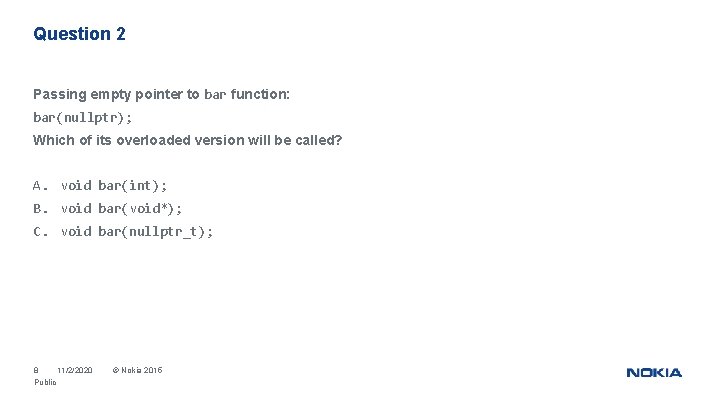
Question 2 Passing empty pointer to bar function: bar(nullptr); Which of its overloaded version will be called? A. void bar(int); B. void bar(void*); C. void bar(nullptr_t); 8 11/2/2020 Public © Nokia 2015
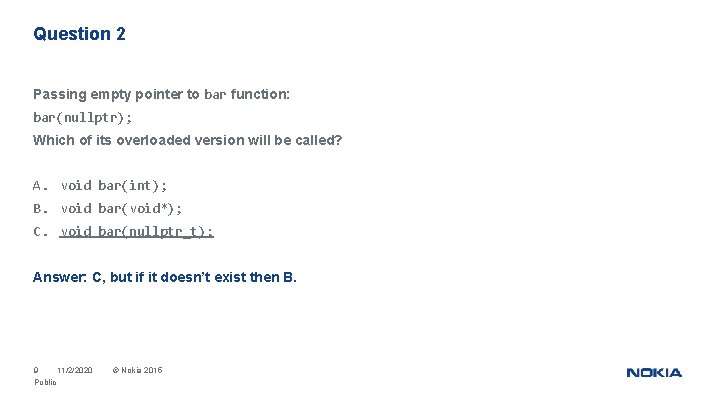
Question 2 Passing empty pointer to bar function: bar(nullptr); Which of its overloaded version will be called? A. void bar(int); B. void bar(void*); C. void bar(nullptr_t); Answer: C, but if it doesn’t exist then B. 9 11/2/2020 Public © Nokia 2015
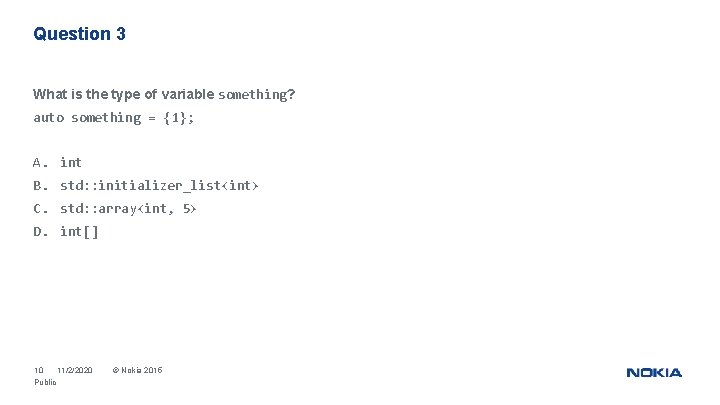
Question 3 What is the type of variable something? auto something = {1}; A. int B. std: : initializer_list<int> C. std: : array<int, 5> D. int[] 10 11/2/2020 Public © Nokia 2015
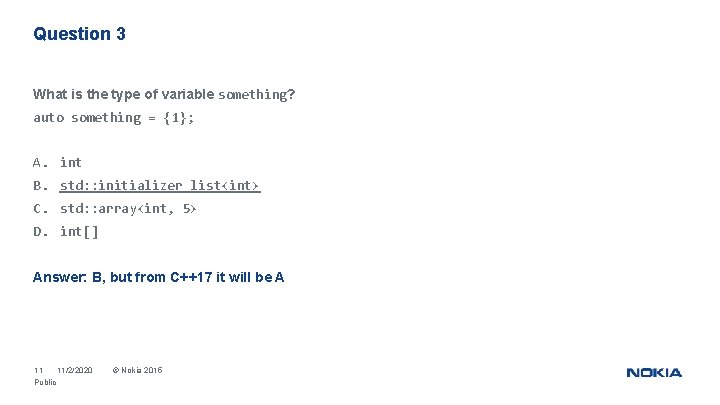
Question 3 What is the type of variable something? auto something = {1}; A. int B. std: : initializer_list<int> C. std: : array<int, 5> D. int[] Answer: B, but from C++17 it will be A 11 11/2/2020 Public © Nokia 2015
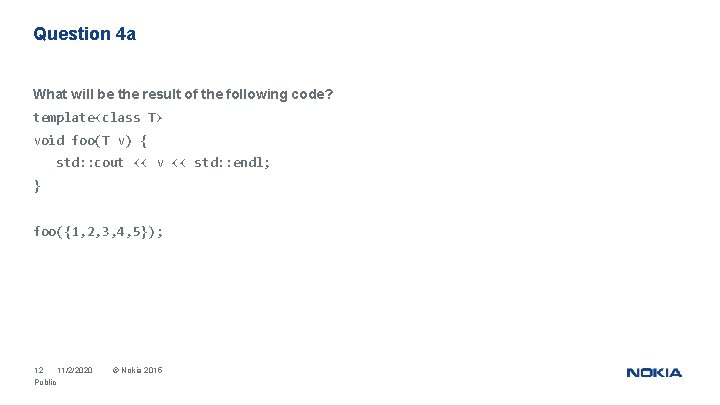
Question 4 a What will be the result of the following code? template<class T> void foo(T v) { std: : cout << v << std: : endl; } foo({1, 2, 3, 4, 5}); 12 11/2/2020 Public © Nokia 2015
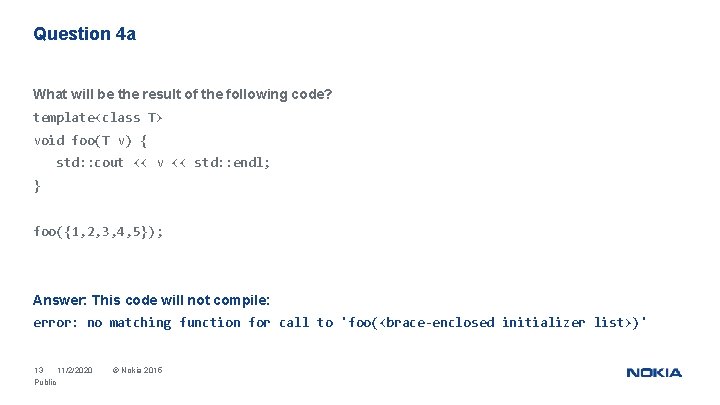
Question 4 a What will be the result of the following code? template<class T> void foo(T v) { std: : cout << v << std: : endl; } foo({1, 2, 3, 4, 5}); Answer: This code will not compile: error: no matching function for call to 'foo(<brace-enclosed initializer list>)' 13 11/2/2020 Public © Nokia 2015
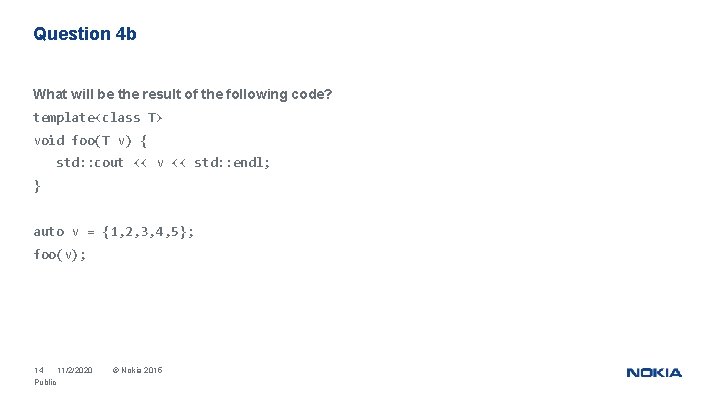
Question 4 b What will be the result of the following code? template<class T> void foo(T v) { std: : cout << v << std: : endl; } auto v = {1, 2, 3, 4, 5}; foo(v); 14 11/2/2020 Public © Nokia 2015
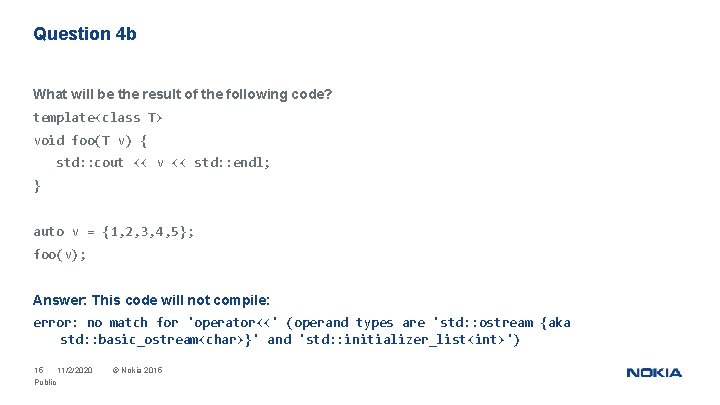
Question 4 b What will be the result of the following code? template<class T> void foo(T v) { std: : cout << v << std: : endl; } auto v = {1, 2, 3, 4, 5}; foo(v); Answer: This code will not compile: error: no match for 'operator<<' (operand types are 'std: : ostream {aka std: : basic_ostream<char>}' and 'std: : initializer_list<int>') 15 11/2/2020 Public © Nokia 2015
![Question 5 Will it compile int array 1 2 5 5 Question 5 Will it compile? int array[] = { 1, 2, 5. 5 };](https://slidetodoc.com/presentation_image/3f5829670a40bc73839a88fcfe3652d6/image-16.jpg)
Question 5 Will it compile? int array[] = { 1, 2, 5. 5 }; 16 11/2/2020 Public © Nokia 2015
![Question 5 Will it compile int array 1 2 5 5 Question 5 Will it compile? int array[] = { 1, 2, 5. 5 };](https://slidetodoc.com/presentation_image/3f5829670a40bc73839a88fcfe3652d6/image-17.jpg)
Question 5 Will it compile? int array[] = { 1, 2, 5. 5 }; Answer: C++98 - OK, C++11: error - implicit type narrowing. 17 11/2/2020 Public © Nokia 2015
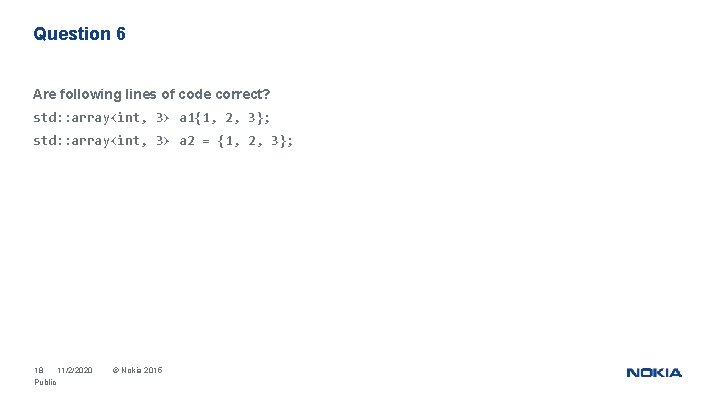
Question 6 Are following lines of code correct? std: : array<int, 3> a 1{1, 2, 3}; std: : array<int, 3> a 2 = {1, 2, 3}; 18 11/2/2020 Public © Nokia 2015
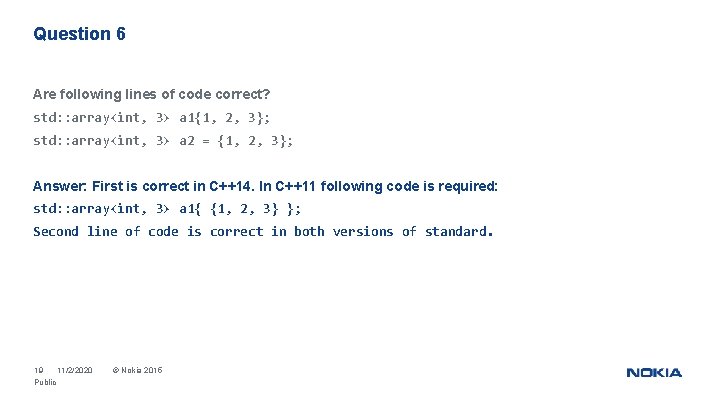
Question 6 Are following lines of code correct? std: : array<int, 3> a 1{1, 2, 3}; std: : array<int, 3> a 2 = {1, 2, 3}; Answer: First is correct in C++14. In C++11 following code is required: std: : array<int, 3> a 1{ {1, 2, 3} }; Second line of code is correct in both versions of standard. 19 11/2/2020 Public © Nokia 2015
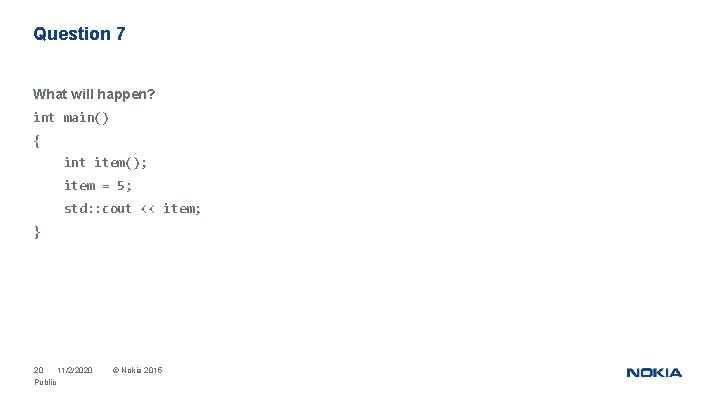
Question 7 What will happen? int main() { int item(); item = 5; std: : cout << item; } 20 11/2/2020 Public © Nokia 2015
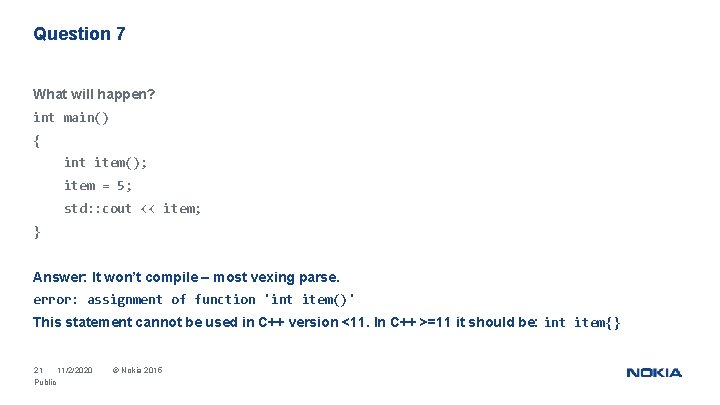
Question 7 What will happen? int main() { int item(); item = 5; std: : cout << item; } Answer: It won’t compile – most vexing parse. error: assignment of function 'int item()' This statement cannot be used in C++ version <11. In C++ >=11 it should be: int item{} 21 11/2/2020 Public © Nokia 2015
![Question 8 a What is the type of g 1 Gadget items10 auto g Question 8 a What is the type of g 1? Gadget items[10]; auto g](https://slidetodoc.com/presentation_image/3f5829670a40bc73839a88fcfe3652d6/image-22.jpg)
Question 8 a What is the type of g 1? Gadget items[10]; auto g 1 = items; 22 11/2/2020 Public © Nokia 2015
![Question 8 a What is the type of g 1 Gadget items10 auto g Question 8 a What is the type of g 1? Gadget items[10]; auto g](https://slidetodoc.com/presentation_image/3f5829670a40bc73839a88fcfe3652d6/image-23.jpg)
Question 8 a What is the type of g 1? Gadget items[10]; auto g 1 = items; Answer: Gadget* 23 11/2/2020 Public © Nokia 2015
![Question 8 b What is the type of g 2 Gadget items10 auto Question 8 b What is the type of g 2? Gadget items[10]; auto &](https://slidetodoc.com/presentation_image/3f5829670a40bc73839a88fcfe3652d6/image-24.jpg)
Question 8 b What is the type of g 2? Gadget items[10]; auto & g 2 = items; 24 11/2/2020 Public © Nokia 2015
![Question 8 b What is the type of g 2 Gadget items10 auto Question 8 b What is the type of g 2? Gadget items[10]; auto &](https://slidetodoc.com/presentation_image/3f5829670a40bc73839a88fcfe3652d6/image-25.jpg)
Question 8 b What is the type of g 2? Gadget items[10]; auto & g 2 = items; Answer: Gadget(&)[10] 25 11/2/2020 Public © Nokia 2015
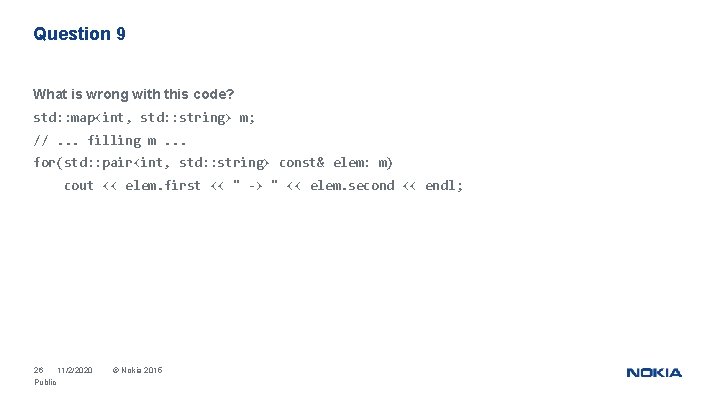
Question 9 What is wrong with this code? std: : map<int, std: : string> m; //. . . filling m. . . for(std: : pair<int, std: : string> const& elem: m) cout << elem. first << " -> " << elem. second << endl; 26 11/2/2020 Public © Nokia 2015
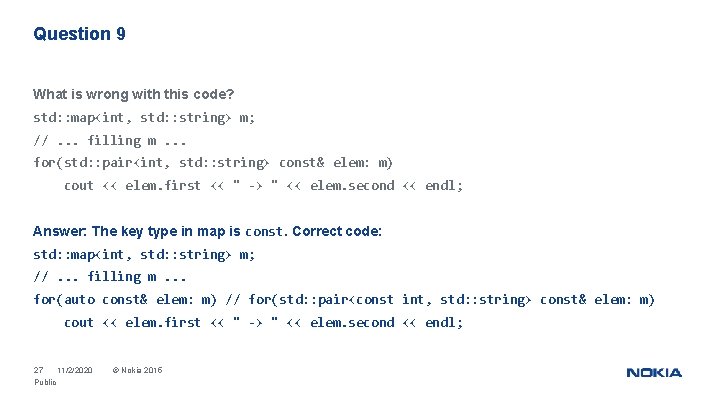
Question 9 What is wrong with this code? std: : map<int, std: : string> m; //. . . filling m. . . for(std: : pair<int, std: : string> const& elem: m) cout << elem. first << " -> " << elem. second << endl; Answer: The key type in map is const. Correct code: std: : map<int, std: : string> m; //. . . filling m. . . for(auto const& elem: m) // for(std: : pair<const int, std: : string> const& elem: m) cout << elem. first << " -> " << elem. second << endl; 27 11/2/2020 Public © Nokia 2015
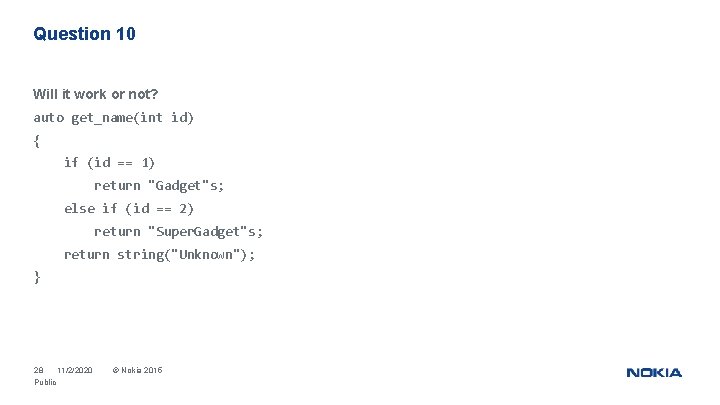
Question 10 Will it work or not? auto get_name(int id) { if (id == 1) return "Gadget"s; else if (id == 2) return "Super. Gadget"s; return string("Unknown"); } 28 11/2/2020 Public © Nokia 2015
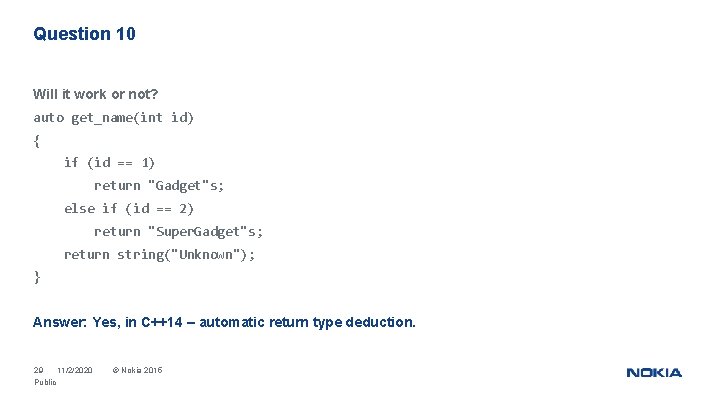
Question 10 Will it work or not? auto get_name(int id) { if (id == 1) return "Gadget"s; else if (id == 2) return "Super. Gadget"s; return string("Unknown"); } Answer: Yes, in C++14 – automatic return type deduction. 29 11/2/2020 Public © Nokia 2015
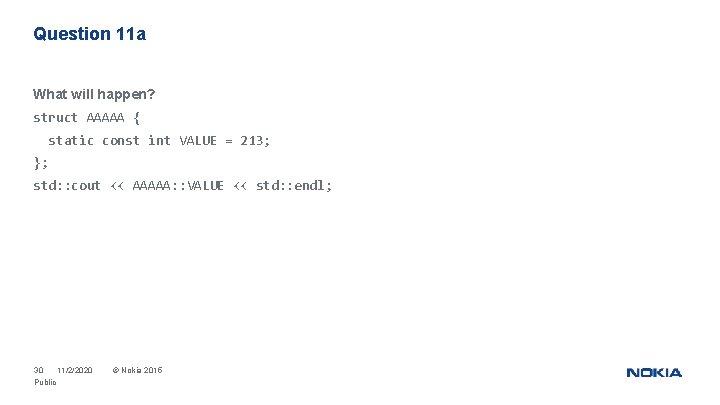
Question 11 a What will happen? struct AAAAA { static const int VALUE = 213; }; std: : cout << AAAAA: : VALUE << std: : endl; 30 11/2/2020 Public © Nokia 2015
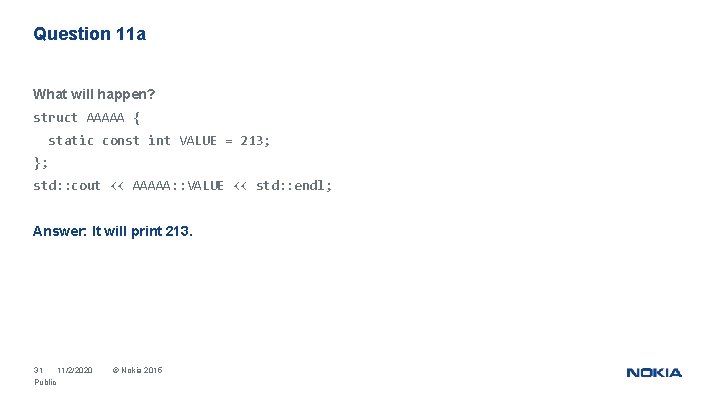
Question 11 a What will happen? struct AAAAA { static const int VALUE = 213; }; std: : cout << AAAAA: : VALUE << std: : endl; Answer: It will print 213. 31 11/2/2020 Public © Nokia 2015
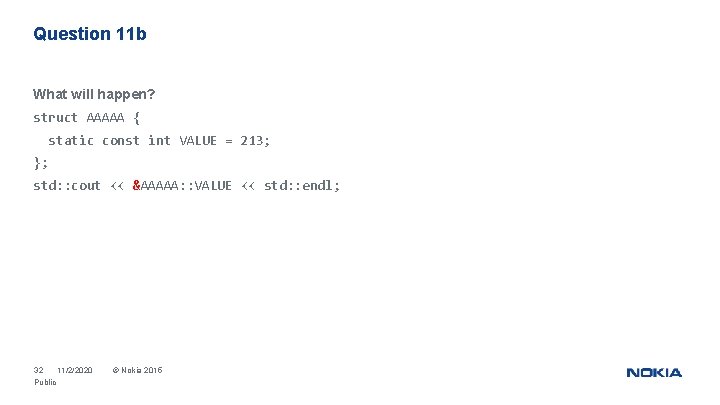
Question 11 b What will happen? struct AAAAA { static const int VALUE = 213; }; std: : cout << &AAAAA: : VALUE << std: : endl; 32 11/2/2020 Public © Nokia 2015
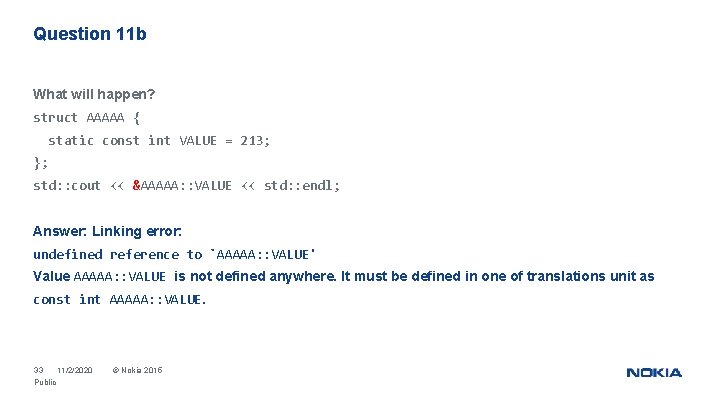
Question 11 b What will happen? struct AAAAA { static const int VALUE = 213; }; std: : cout << &AAAAA: : VALUE << std: : endl; Answer: Linking error: undefined reference to `AAAAA: : VALUE' Value AAAAA: : VALUE is not defined anywhere. It must be defined in one of translations unit as const int AAAAA: : VALUE. 33 11/2/2020 Public © Nokia 2015
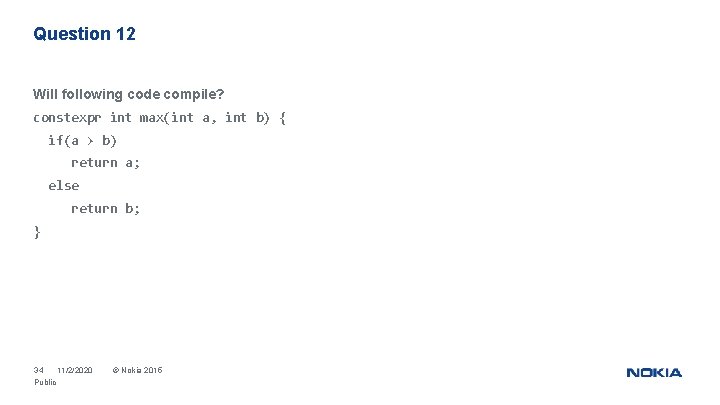
Question 12 Will following code compile? constexpr int max(int a, int b) { if(a > b) return a; else return b; } 34 11/2/2020 Public © Nokia 2015
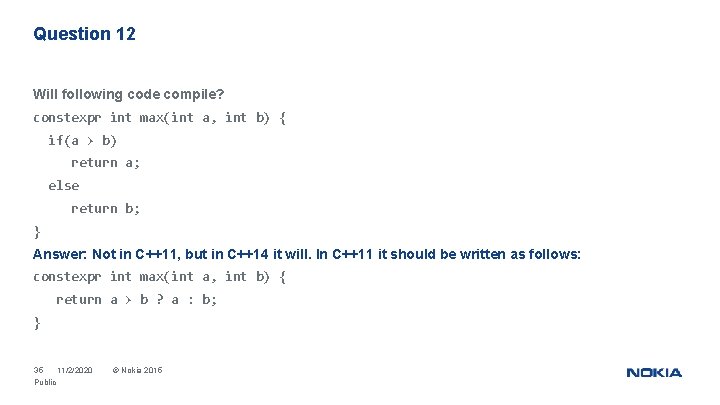
Question 12 Will following code compile? constexpr int max(int a, int b) { if(a > b) return a; else return b; } Answer: Not in C++11, but in C++14 it will. In C++11 it should be written as follows: constexpr int max(int a, int b) { return a > b ? a : b; } 35 11/2/2020 Public © Nokia 2015
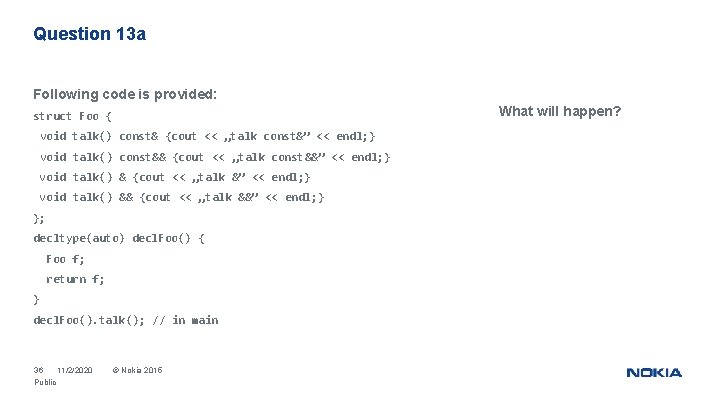
Question 13 a Following code is provided: What will happen? struct Foo { void talk() const& {cout << „talk const&” << endl; } void talk() const&& {cout << „talk const&&” << endl; } void talk() & {cout << „talk &” << endl; } void talk() && {cout << „talk &&” << endl; } }; decltype(auto) decl. Foo() { Foo f; return f; } decl. Foo(). talk(); // in main 36 11/2/2020 Public © Nokia 2015
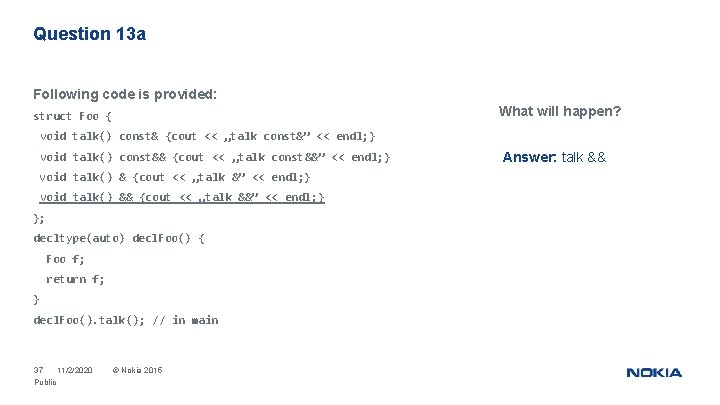
Question 13 a Following code is provided: What will happen? struct Foo { void talk() const& {cout << „talk const&” << endl; } void talk() const&& {cout << „talk const&&” << endl; } void talk() & {cout << „talk &” << endl; } void talk() && {cout << „talk &&” << endl; } }; decltype(auto) decl. Foo() { Foo f; return f; } decl. Foo(). talk(); // in main 37 11/2/2020 Public © Nokia 2015 Answer: talk &&
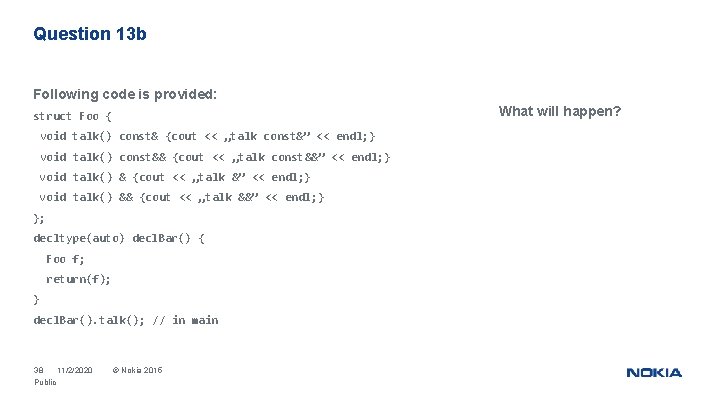
Question 13 b Following code is provided: What will happen? struct Foo { void talk() const& {cout << „talk const&” << endl; } void talk() const&& {cout << „talk const&&” << endl; } void talk() & {cout << „talk &” << endl; } void talk() && {cout << „talk &&” << endl; } }; decltype(auto) decl. Bar() { Foo f; return(f); } decl. Bar(). talk(); // in main 38 11/2/2020 Public © Nokia 2015
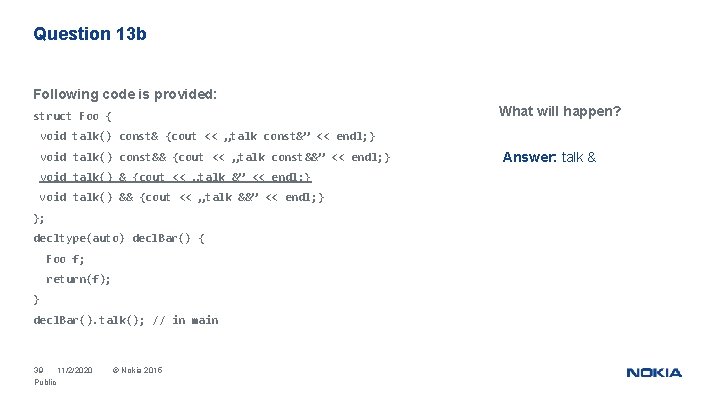
Question 13 b Following code is provided: What will happen? struct Foo { void talk() const& {cout << „talk const&” << endl; } void talk() const&& {cout << „talk const&&” << endl; } void talk() & {cout << „talk &” << endl; } void talk() && {cout << „talk &&” << endl; } }; decltype(auto) decl. Bar() { Foo f; return(f); } decl. Bar(). talk(); // in main 39 11/2/2020 Public © Nokia 2015 Answer: talk &
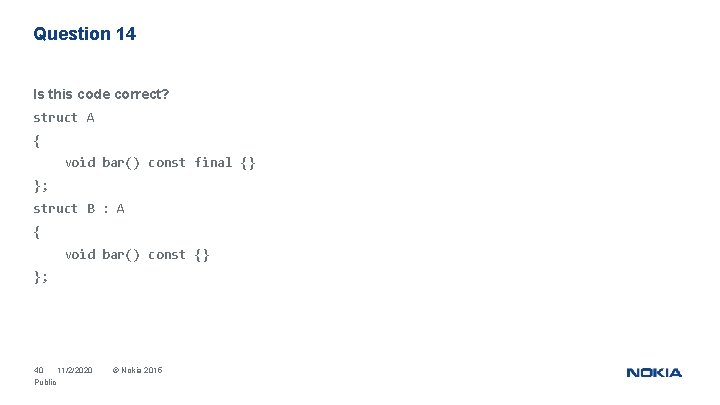
Question 14 Is this code correct? struct A { void bar() const final {} }; struct B : A { void bar() const {} }; 40 11/2/2020 Public © Nokia 2015
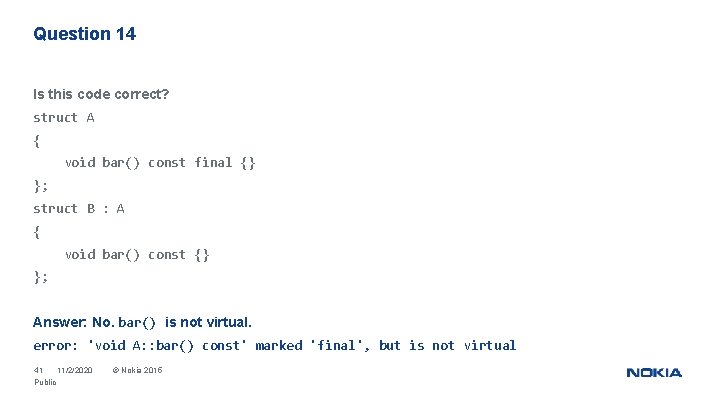
Question 14 Is this code correct? struct A { void bar() const final {} }; struct B : A { void bar() const {} }; Answer: No. bar() is not virtual. error: 'void A: : bar() const' marked 'final', but is not virtual 41 11/2/2020 Public © Nokia 2015
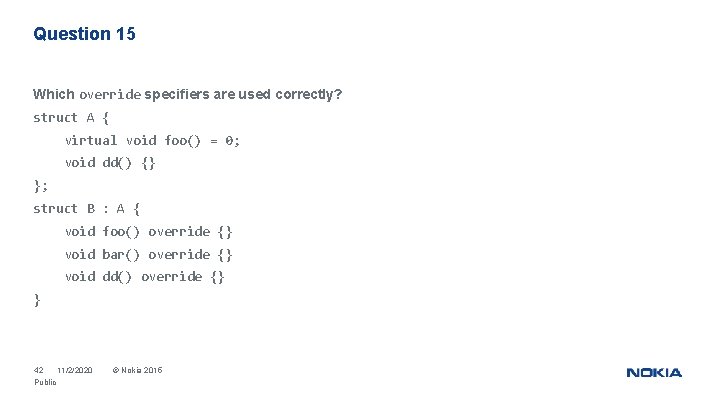
Question 15 Which override specifiers are used correctly? struct A { virtual void foo() = 0; void dd() {} }; struct B : A { void foo() override {} void bar() override {} void dd() override {} } 42 11/2/2020 Public © Nokia 2015
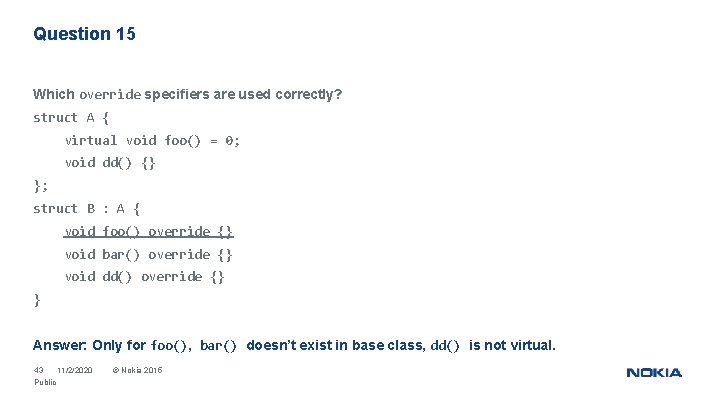
Question 15 Which override specifiers are used correctly? struct A { virtual void foo() = 0; void dd() {} }; struct B : A { void foo() override {} void bar() override {} void dd() override {} } Answer: Only for foo(), bar() doesn’t exist in base class, dd() is not virtual. 43 11/2/2020 Public © Nokia 2015
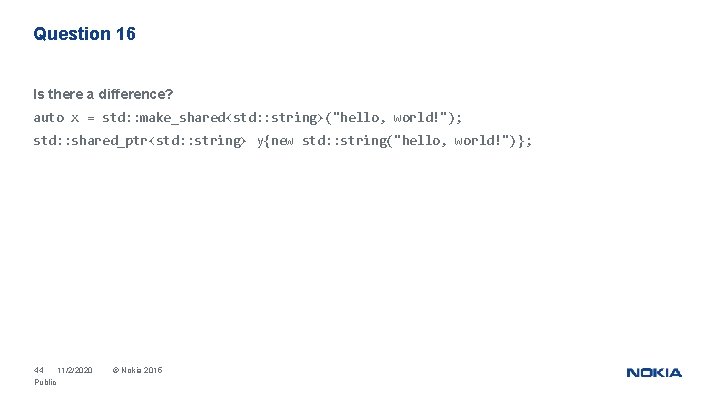
Question 16 Is there a difference? auto x = std: : make_shared<std: : string>("hello, world!"); std: : shared_ptr<std: : string> y{new std: : string("hello, world!")}; 44 11/2/2020 Public © Nokia 2015
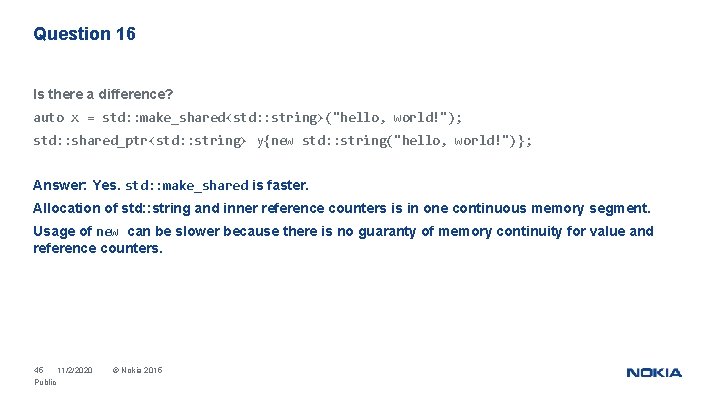
Question 16 Is there a difference? auto x = std: : make_shared<std: : string>("hello, world!"); std: : shared_ptr<std: : string> y{new std: : string("hello, world!")}; Answer: Yes. std: : make_shared is faster. Allocation of std: : string and inner reference counters is in one continuous memory segment. Usage of new can be slower because there is no guaranty of memory continuity for value and reference counters. 45 11/2/2020 Public © Nokia 2015
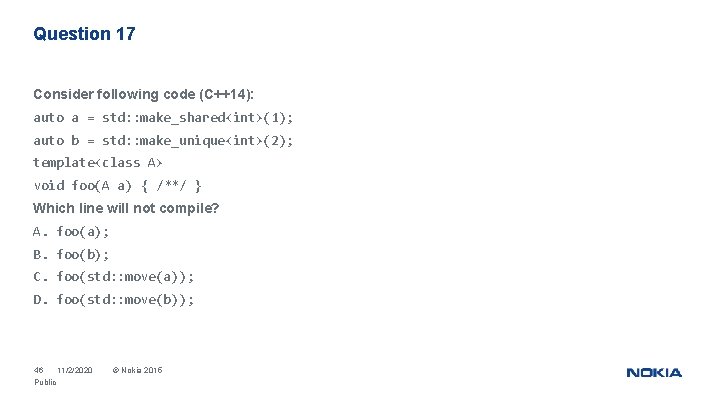
Question 17 Consider following code (C++14): auto a = std: : make_shared<int>(1); auto b = std: : make_unique<int>(2); template<class A> void foo(A a) { /**/ } Which line will not compile? A. foo(a); B. foo(b); C. foo(std: : move(a)); D. foo(std: : move(b)); 46 11/2/2020 Public © Nokia 2015
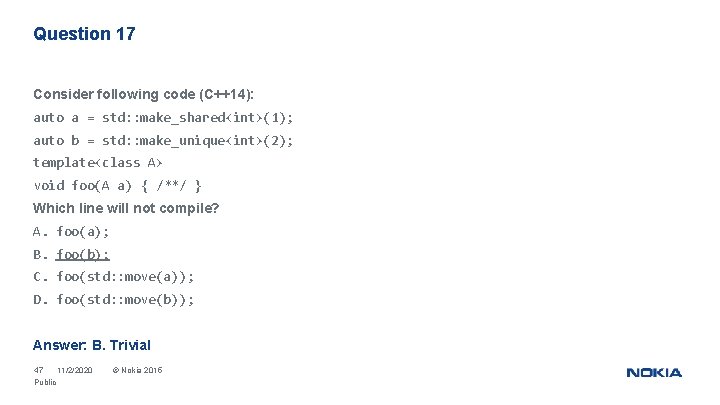
Question 17 Consider following code (C++14): auto a = std: : make_shared<int>(1); auto b = std: : make_unique<int>(2); template<class A> void foo(A a) { /**/ } Which line will not compile? A. foo(a); B. foo(b); C. foo(std: : move(a)); D. foo(std: : move(b)); Answer: B. Trivial 47 11/2/2020 Public © Nokia 2015
![Question 18 Which line will not compile A B C D Question 18 Which line will not compile? A. [](){}; B. []{}; C. {}; D.](https://slidetodoc.com/presentation_image/3f5829670a40bc73839a88fcfe3652d6/image-48.jpg)
Question 18 Which line will not compile? A. [](){}; B. []{}; C. {}; D. [](); E. []; F. (); G. [](){}(); 48 11/2/2020 Public © Nokia 2015
![Question 18 Which line will not compile A B C D Question 18 Which line will not compile? A. [](){}; B. []{}; C. {}; D.](https://slidetodoc.com/presentation_image/3f5829670a40bc73839a88fcfe3652d6/image-49.jpg)
Question 18 Which line will not compile? A. [](){}; B. []{}; C. {}; D. [](); E. []; F. (); G. [](){}(); Answer: D, E, F won’t compile. A i B are correct lambdas. C are scope braces, which do nothing. G is a correct lambda called after definition. 49 11/2/2020 Public © Nokia 2015
![Question 19 Which lambda function is correct A int return 4 Question 19 Which lambda function is correct? A. []() -> int { return 4;](https://slidetodoc.com/presentation_image/3f5829670a40bc73839a88fcfe3652d6/image-50.jpg)
Question 19 Which lambda function is correct? A. []() -> int { return 4; }; B. int [](){ return 4; }; C. auto [](){ return 4; }; D. []() -> auto { return 4; }; E. [](){ return 4; }; F. []{ return 4; }; G. [] -> int { return 4; }; H. int []{ return 4; }; 50 11/2/2020 Public © Nokia 2015
![Question 19 Which lambda function is correct A int return 4 Question 19 Which lambda function is correct? A. []() -> int { return 4;](https://slidetodoc.com/presentation_image/3f5829670a40bc73839a88fcfe3652d6/image-51.jpg)
Question 19 Which lambda function is correct? A. []() -> int { return 4; }; B. int [](){ return 4; }; C. auto [](){ return 4; }; D. []() -> auto { return 4; }; E. [](){ return 4; }; F. []{ return 4; }; G. [] -> int { return 4; }; H. int []{ return 4; }; Answers: A, D, E, F 51 11/2/2020 Public © Nokia 2015
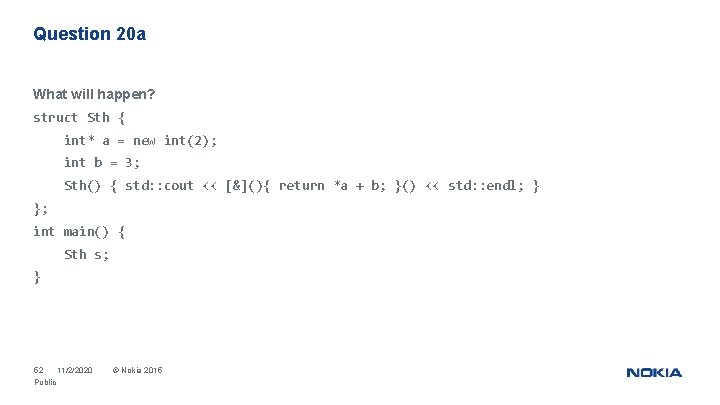
Question 20 a What will happen? struct Sth { int* a = new int(2); int b = 3; Sth() { std: : cout << [&](){ return *a + b; }() << std: : endl; } }; int main() { Sth s; } 52 11/2/2020 Public © Nokia 2015
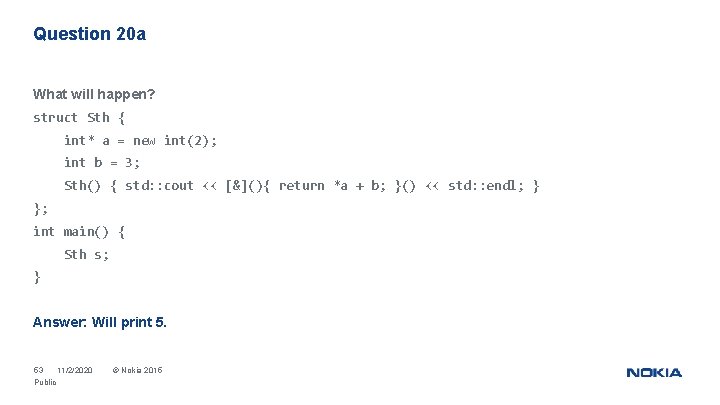
Question 20 a What will happen? struct Sth { int* a = new int(2); int b = 3; Sth() { std: : cout << [&](){ return *a + b; }() << std: : endl; } }; int main() { Sth s; } Answer: Will print 5. 53 11/2/2020 Public © Nokia 2015
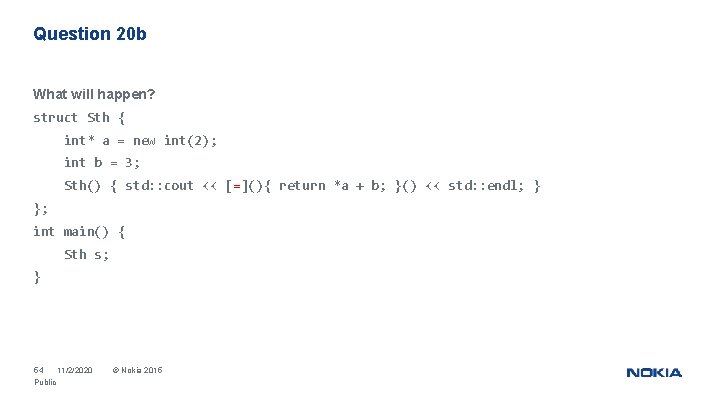
Question 20 b What will happen? struct Sth { int* a = new int(2); int b = 3; Sth() { std: : cout << [=](){ return *a + b; }() << std: : endl; } }; int main() { Sth s; } 54 11/2/2020 Public © Nokia 2015
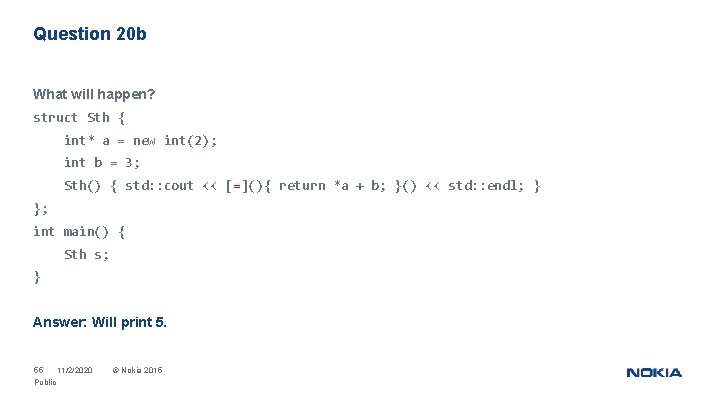
Question 20 b What will happen? struct Sth { int* a = new int(2); int b = 3; Sth() { std: : cout << [=](){ return *a + b; }() << std: : endl; } }; int main() { Sth s; } Answer: Will print 5. 55 11/2/2020 Public © Nokia 2015
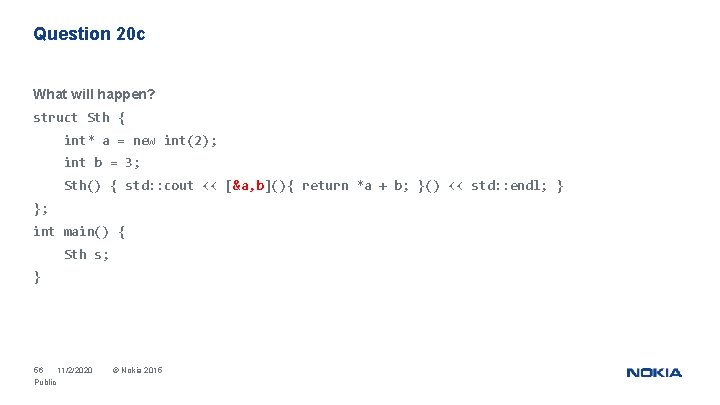
Question 20 c What will happen? struct Sth { int* a = new int(2); int b = 3; Sth() { std: : cout << [&a, b](){ return *a + b; }() << std: : endl; } }; int main() { Sth s; } 56 11/2/2020 Public © Nokia 2015
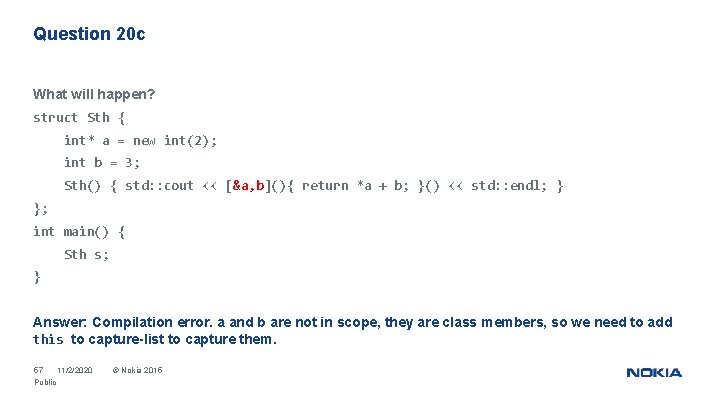
Question 20 c What will happen? struct Sth { int* a = new int(2); int b = 3; Sth() { std: : cout << [&a, b](){ return *a + b; }() << std: : endl; } }; int main() { Sth s; } Answer: Compilation error. a and b are not in scope, they are class members, so we need to add this to capture-list to capture them. 57 11/2/2020 Public © Nokia 2015
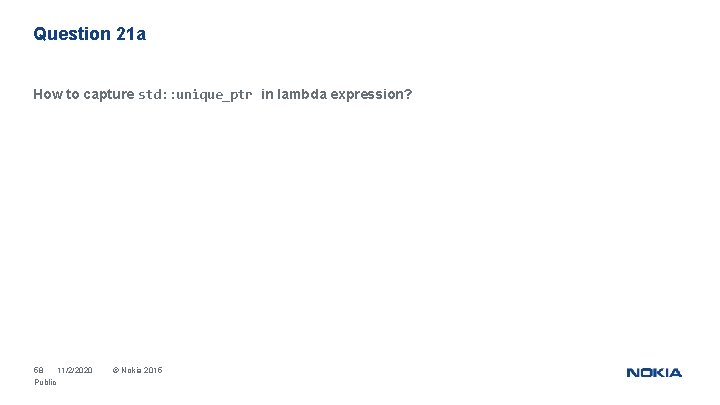
Question 21 a How to capture std: : unique_ptr in lambda expression? 58 11/2/2020 Public © Nokia 2015
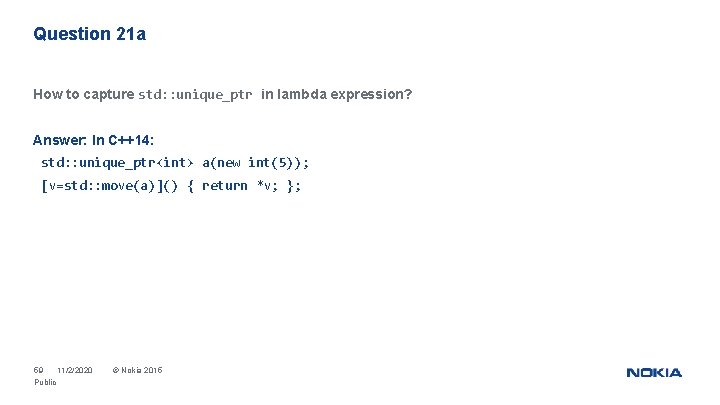
Question 21 a How to capture std: : unique_ptr in lambda expression? Answer: In C++14: std: : unique_ptr<int> a(new int(5)); [v=std: : move(a)]() { return *v; }; 59 11/2/2020 Public © Nokia 2015
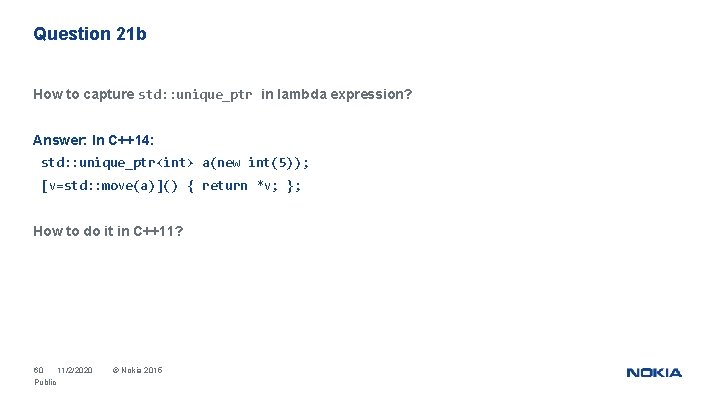
Question 21 b How to capture std: : unique_ptr in lambda expression? Answer: In C++14: std: : unique_ptr<int> a(new int(5)); [v=std: : move(a)]() { return *v; }; How to do it in C++11? 60 11/2/2020 Public © Nokia 2015
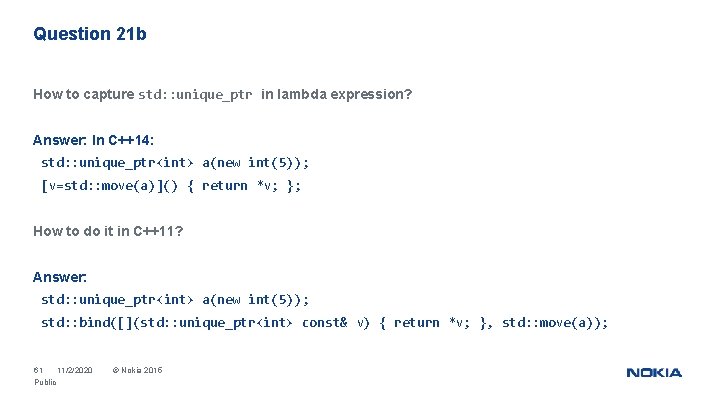
Question 21 b How to capture std: : unique_ptr in lambda expression? Answer: In C++14: std: : unique_ptr<int> a(new int(5)); [v=std: : move(a)]() { return *v; }; How to do it in C++11? Answer: std: : unique_ptr<int> a(new int(5)); std: : bind([](std: : unique_ptr<int> const& v) { return *v; }, std: : move(a)); 61 11/2/2020 Public © Nokia 2015
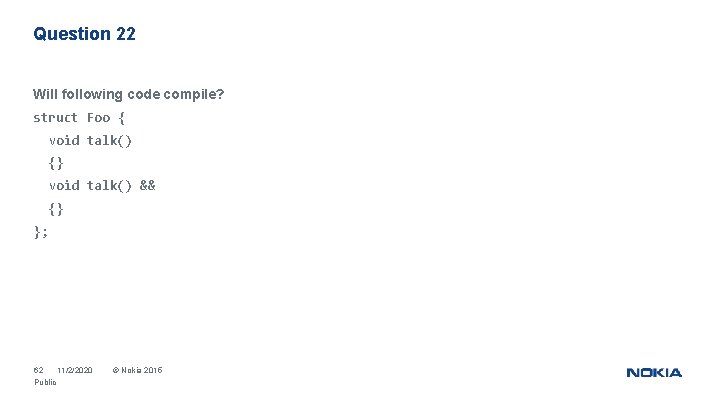
Question 22 Will following code compile? struct Foo { void talk() {} void talk() && {} }; 62 11/2/2020 Public © Nokia 2015
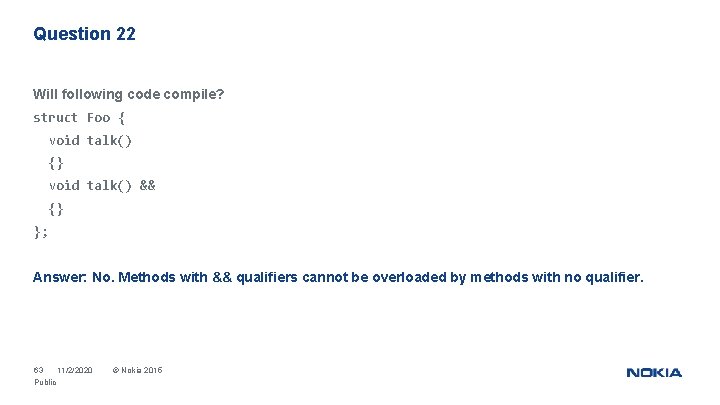
Question 22 Will following code compile? struct Foo { void talk() {} void talk() && {} }; Answer: No. Methods with && qualifiers cannot be overloaded by methods with no qualifier. 63 11/2/2020 Public © Nokia 2015
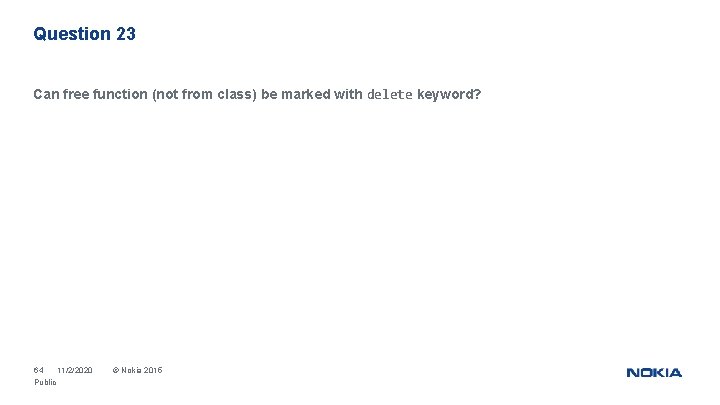
Question 23 Can free function (not from class) be marked with delete keyword? 64 11/2/2020 Public © Nokia 2015
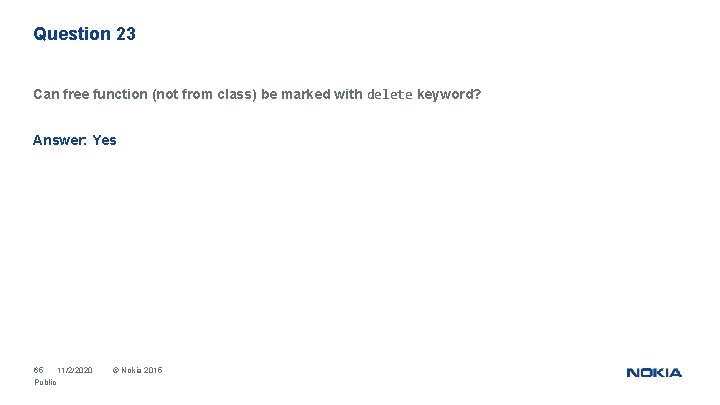
Question 23 Can free function (not from class) be marked with delete keyword? Answer: Yes 65 11/2/2020 Public © Nokia 2015
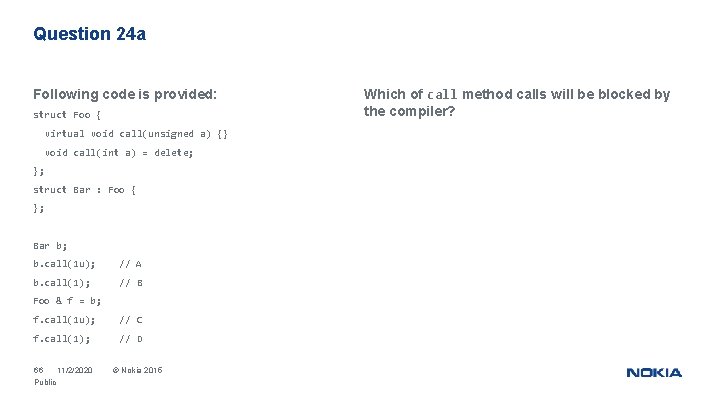
Question 24 a Following code is provided: struct Foo { virtual void call(unsigned a) {} void call(int a) = delete; }; struct Bar : Foo { }; Bar b; b. call(1 u); // A b. call(1); // B Foo & f = b; f. call(1 u); // C f. call(1); // D 66 11/2/2020 Public © Nokia 2015 Which of call method calls will be blocked by the compiler?
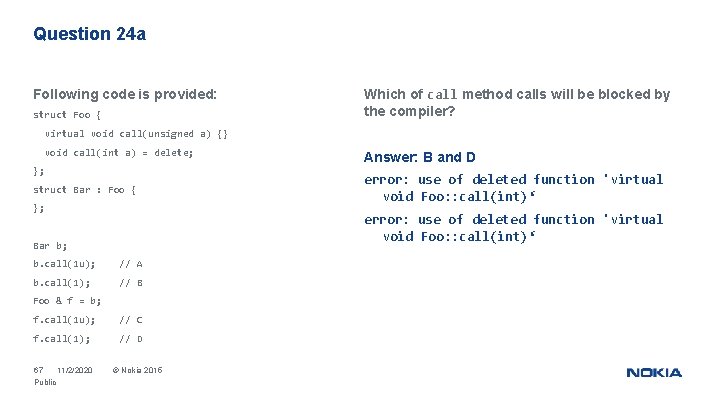
Question 24 a Following code is provided: struct Foo { Which of call method calls will be blocked by the compiler? virtual void call(unsigned a) {} void call(int a) = delete; }; struct Bar : Foo { }; error: use of deleted function 'virtual void Foo: : call(int)‘ Bar b; b. call(1 u); // A b. call(1); // B Foo & f = b; f. call(1 u); // C f. call(1); // D 67 11/2/2020 Public Answer: B and D © Nokia 2015
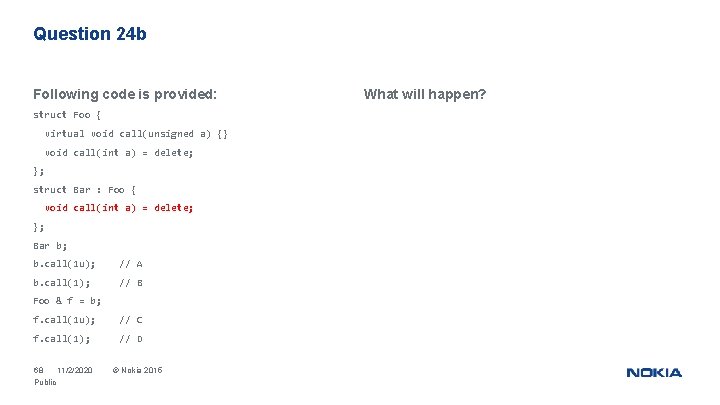
Question 24 b Following code is provided: struct Foo { virtual void call(unsigned a) {} void call(int a) = delete; }; struct Bar : Foo { void call(int a) = delete; }; Bar b; b. call(1 u); // A b. call(1); // B Foo & f = b; f. call(1 u); // C f. call(1); // D 68 11/2/2020 Public © Nokia 2015 What will happen?
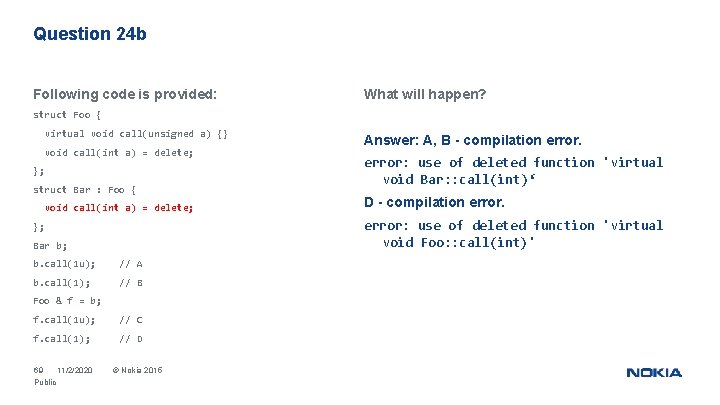
Question 24 b Following code is provided: What will happen? struct Foo { virtual void call(unsigned a) {} void call(int a) = delete; }; struct Bar : Foo { void call(int a) = delete; error: use of deleted function 'virtual void Bar: : call(int)‘ D - compilation error: use of deleted function 'virtual void Foo: : call(int)' }; Bar b; b. call(1 u); // A b. call(1); // B Foo & f = b; f. call(1 u); // C f. call(1); // D 69 11/2/2020 Public Answer: A, B - compilation error. © Nokia 2015
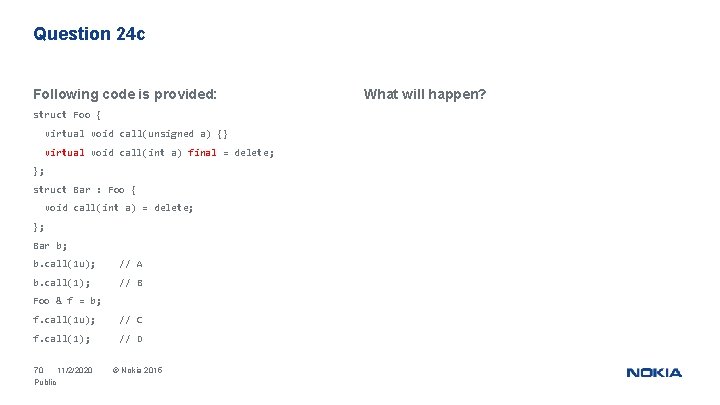
Question 24 c Following code is provided: struct Foo { virtual void call(unsigned a) {} virtual void call(int a) final = delete; }; struct Bar : Foo { void call(int a) = delete; }; Bar b; b. call(1 u); // A b. call(1); // B Foo & f = b; f. call(1 u); // C f. call(1); // D 70 11/2/2020 Public © Nokia 2015 What will happen?
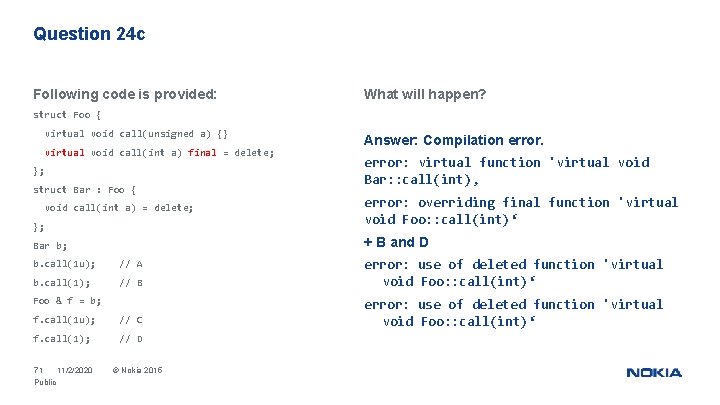
Question 24 c Following code is provided: What will happen? struct Foo { virtual void call(unsigned a) {} virtual void call(int a) final = delete; }; struct Bar : Foo { void call(int a) = delete; }; Answer: Compilation error: virtual function 'virtual void Bar: : call(int)‚ error: overriding final function 'virtual void Foo: : call(int)‘ + B and D Bar b; b. call(1 u); // A b. call(1); // B error: use of deleted function 'virtual void Foo: : call(int)‘ f. call(1 u); // C error: use of deleted function 'virtual void Foo: : call(int)‘ f. call(1); // D Foo & f = b; 71 11/2/2020 Public © Nokia 2015
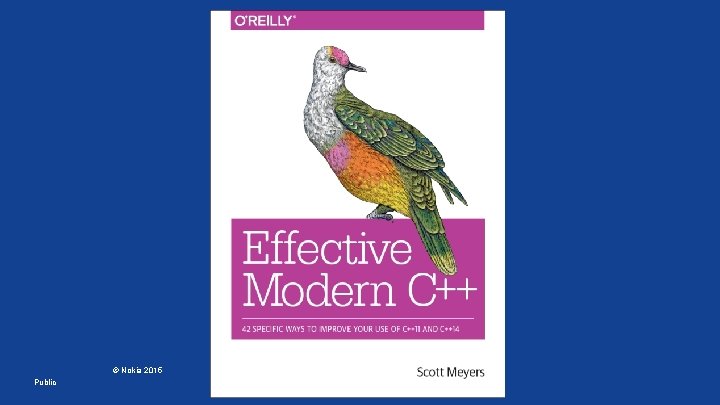
72 11/2/2020 Public © Nokia 2015
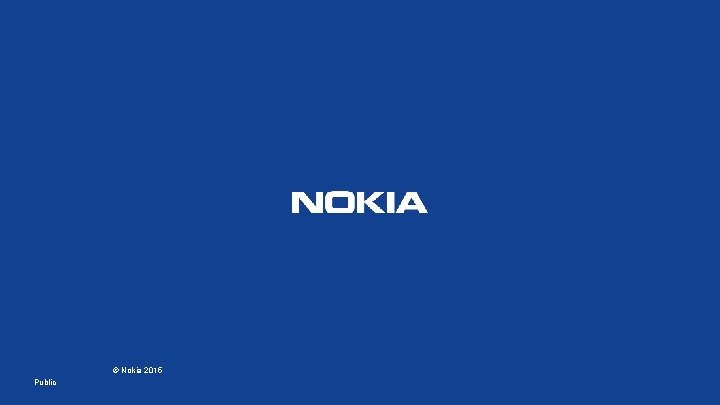
73 11/2/2020 Public © Nokia 2015
Kamil szatkowski
Accessibility tips and tricks
Ssrs tips and tricks
Database design tips and tricks
Sigma tips and tricks
Ssis-049
Advanced excel tips and tricks 2019
Navision tips and tricks
Vacuum forming process steps
Nextgen crystal reports
Stata tips and tricks
Unit agresso
Uil calculator practice tests with answers
Fmsi login
Google scholar tricks
Database design tips and tricks
Marketplace tips and tricks
Vmware tips and tricks
Kronos tips and tricks
Chemdraw clean up reaction
Xero practice manager demo
C tips and tricks
Cecos moodle
Data analysis tricks
Microchip tips and tricks
The 30 greatest lead generation tips tricks & ideas
Igcse english tips
Qlik sense tips and tricks
Western blot tips and tricks
Alma analytics tips and tricks
Machine learning tips and tricks
Sql server management studio tips and tricks
Alteryx tips and tricks
Wells fargo positive pay file format
Visual studio 2010 tips and tricks
Gogle znalac
Matlab tips and tricks
Agresso tips and tricks
Brand tips and tricks
Homework
Gp tips and tricks
Train the trainer tips and tricks
Magento 2 tips and tricks
Microsoft flow tips and tricks
Windows 7 tips and tricks
Next gen lab corp
Wpf tips and tricks
Kamil teke
Apostrofa
Kamil florek
Kamil nejezchleb
Kamil sönmez ankara üniversitesi
Palançı nədir
Kamil gupta
Toman real
Kamil trzebiatowski
Iklab hükmü
Kombinované zapojení kondenzátorů
Prof dr kamil adalet
Horehronie kamil peteraj
Ssm wisła płock plan treningowy
Zakres pracy inżynierskiej przykład
Shoaib kamil
Kamil wasilewski internet
Kamil sznajder
Kamil szadkowski srednia
Babson college mission
Arapça kamil olma olgunlaşma
Wan abdul rahim kamil
Kamil majestic age
Kamil valica
Hyperechogénny
Kamil zięba umcs
Rzymskie boginie przeznaczenia