Introducing Java Script In many ways Java Script
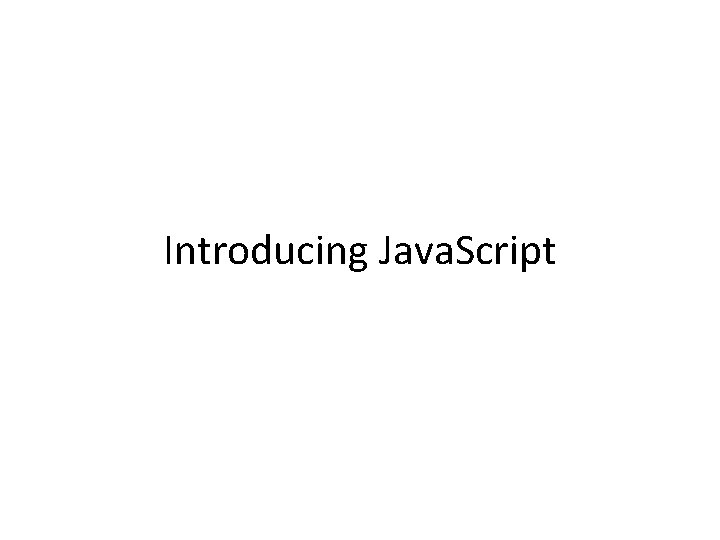
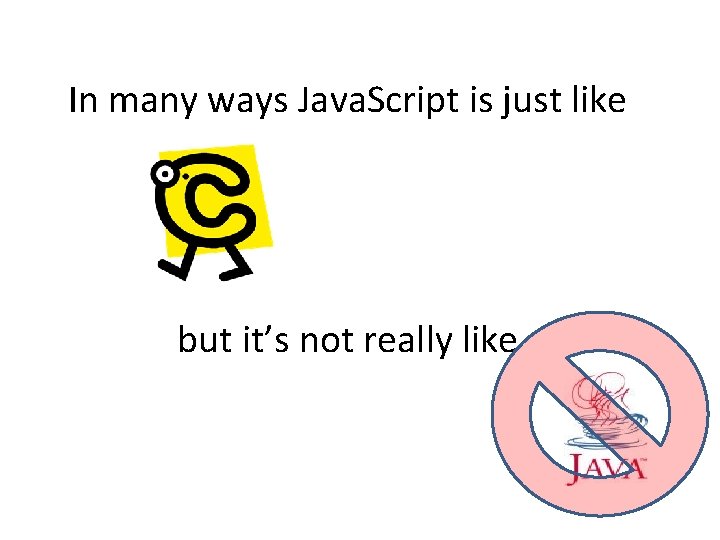
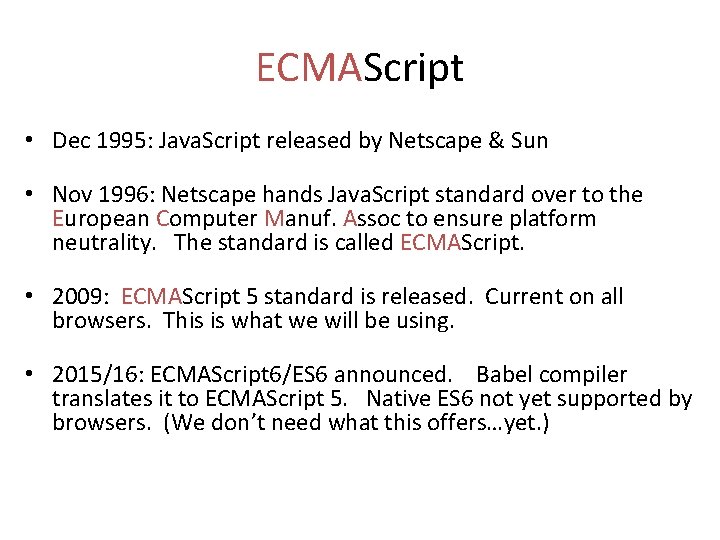
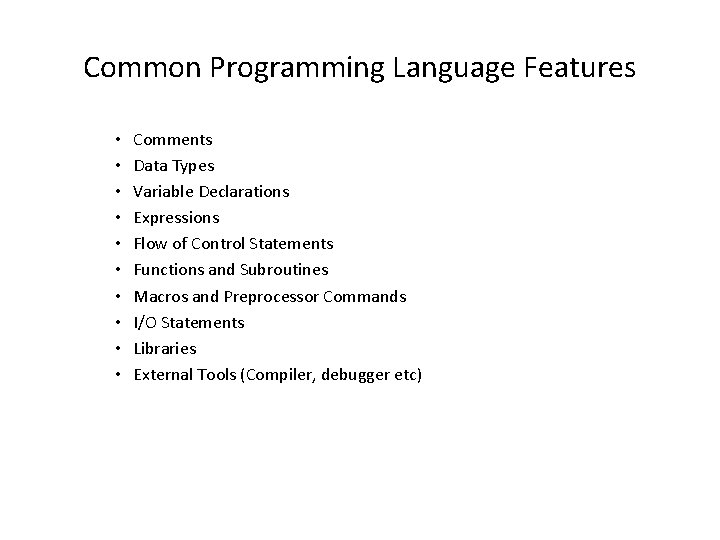
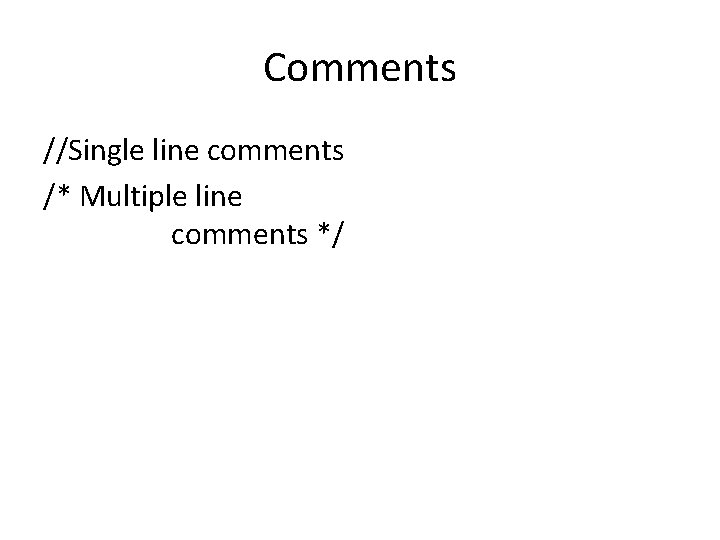
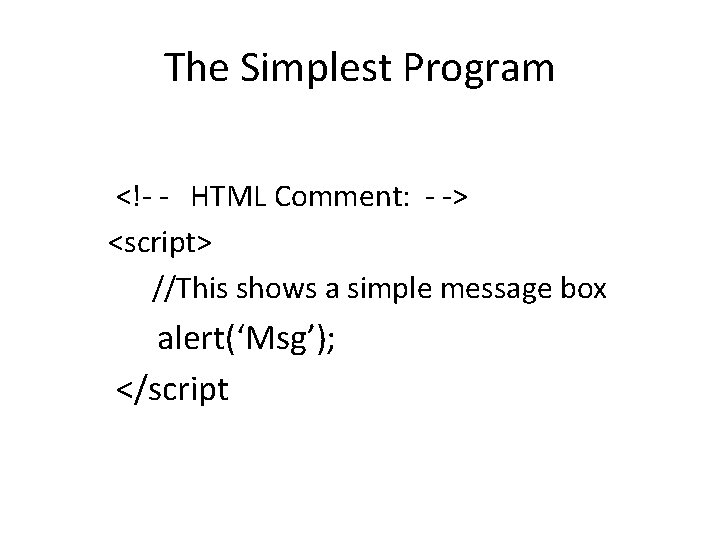
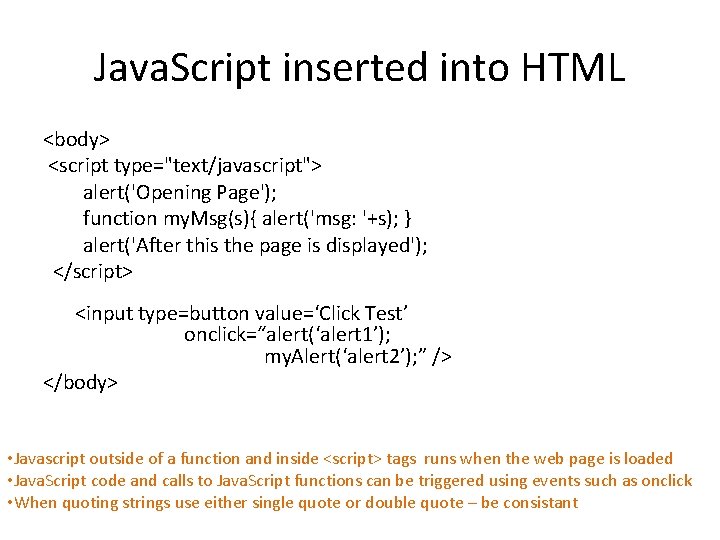
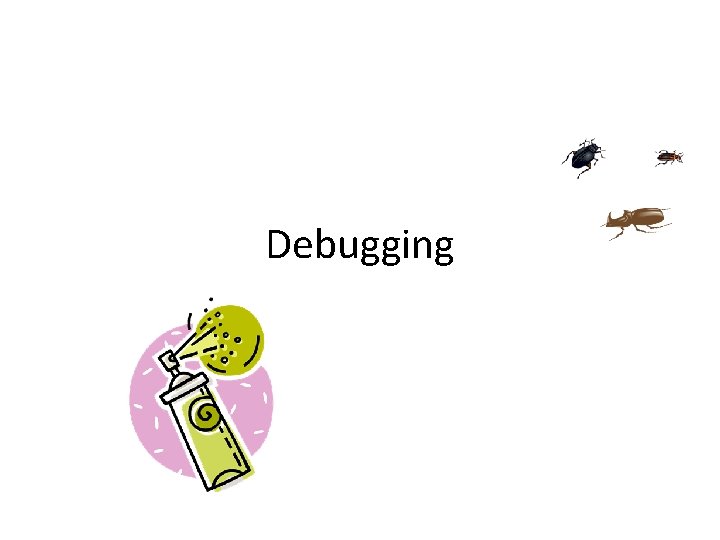
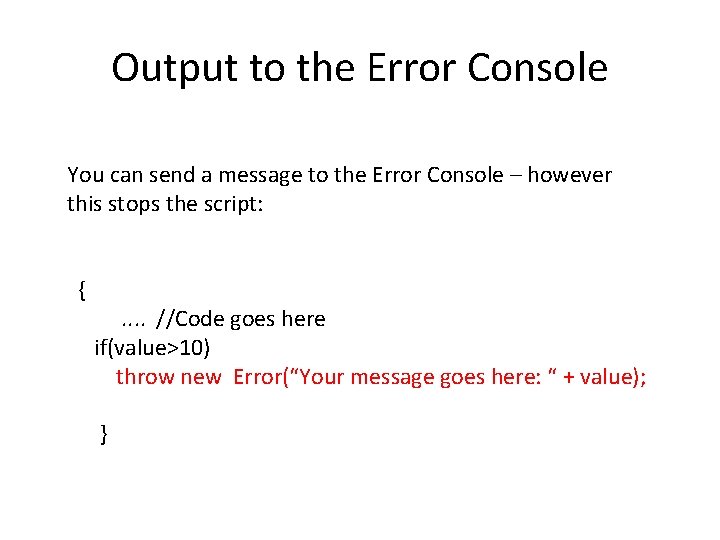
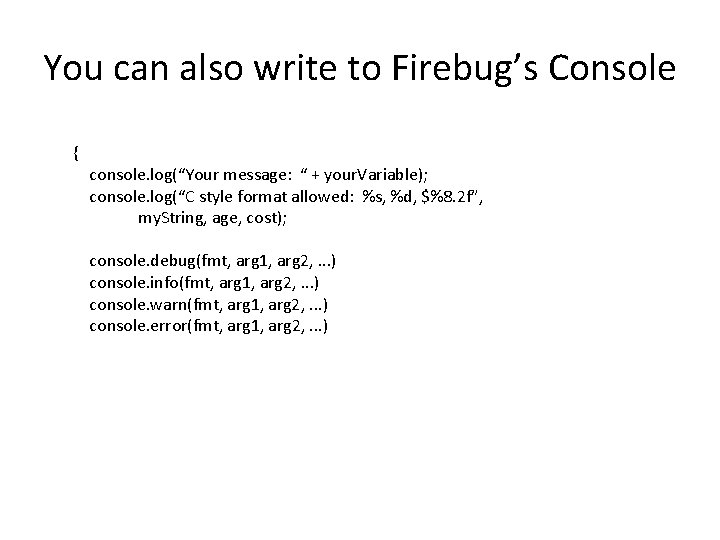
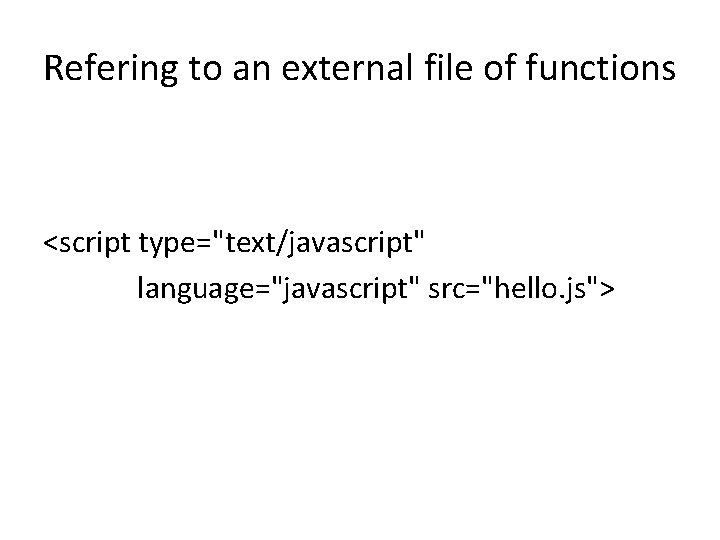
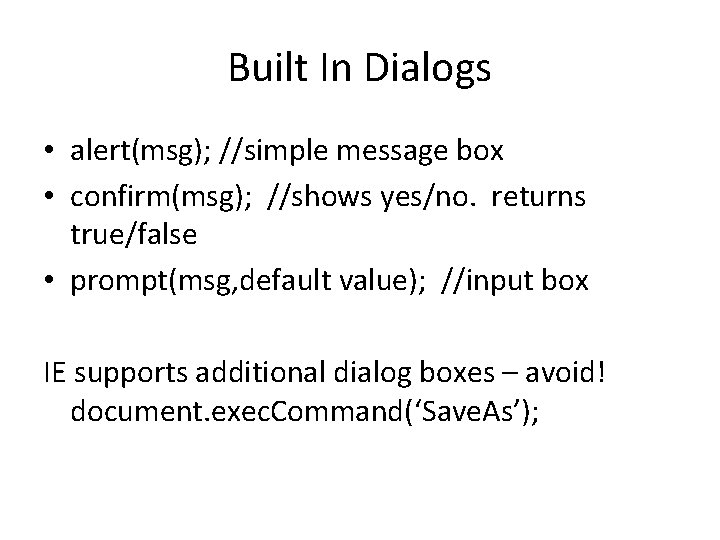
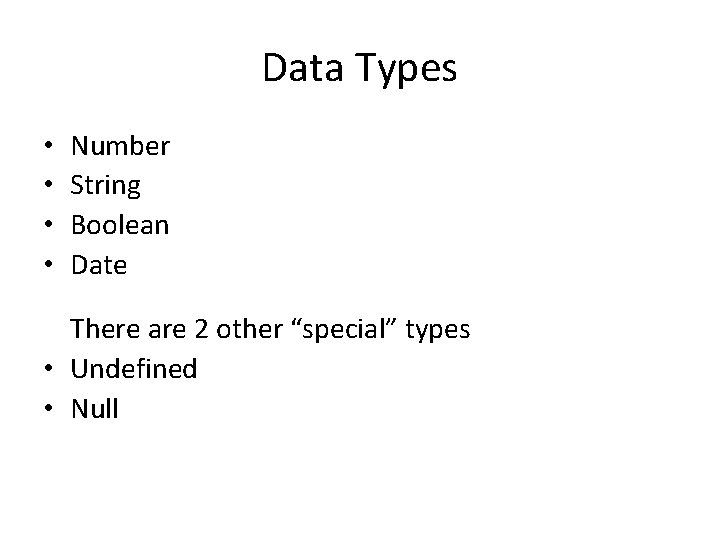
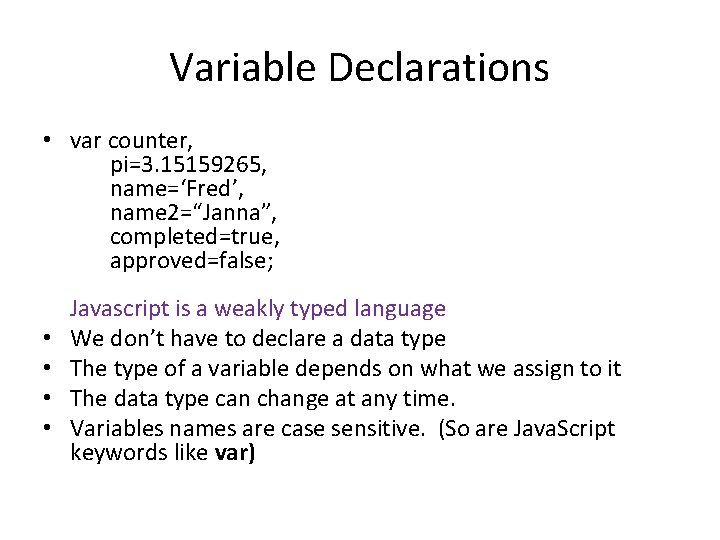
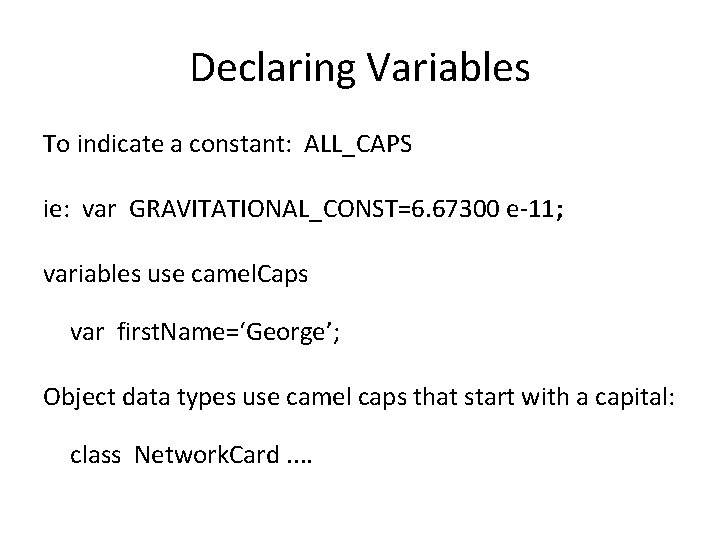
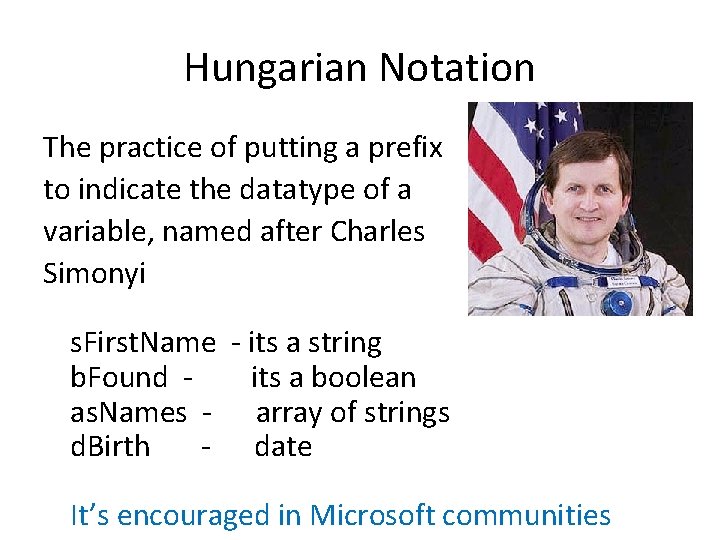
![Declaring Arrays • var names=[‘Mary’, ”Murray”, ”Jim”]; var nested. Array=[ [1, 2, 3], [4, Declaring Arrays • var names=[‘Mary’, ”Murray”, ”Jim”]; var nested. Array=[ [1, 2, 3], [4,](https://slidetodoc.com/presentation_image_h2/866320c96ebb535acbbc0ff8cb4a1755/image-17.jpg)
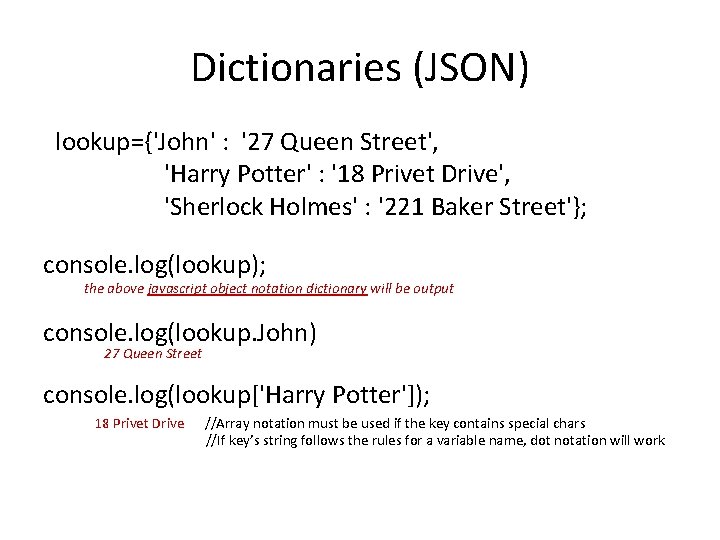
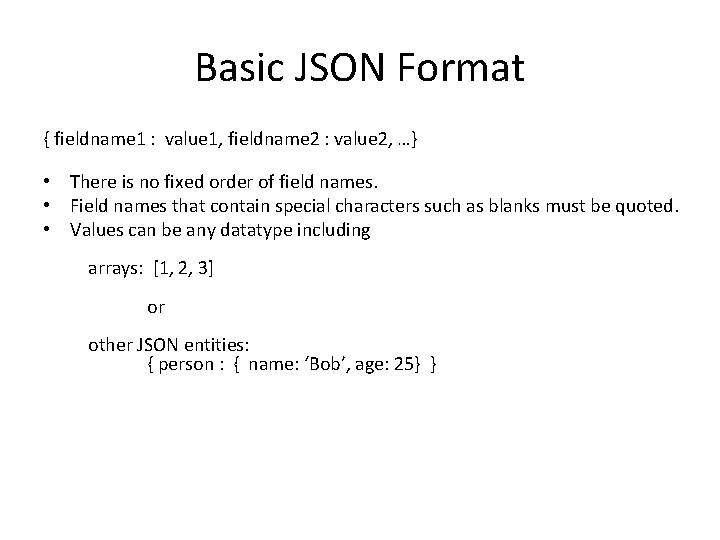
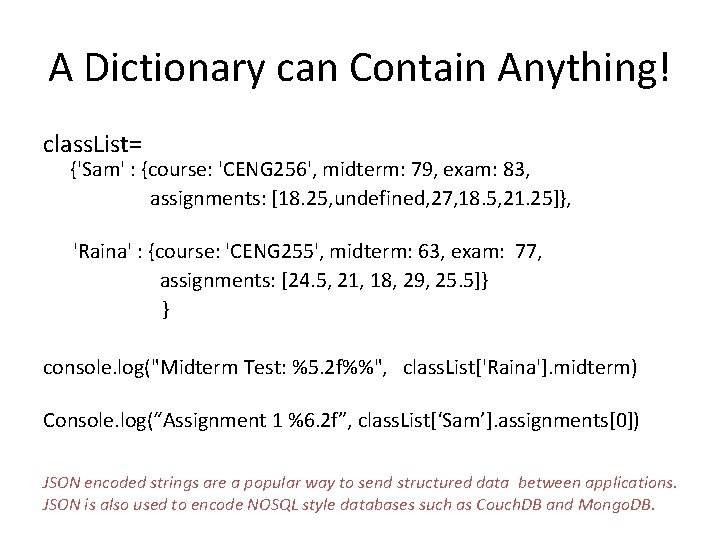
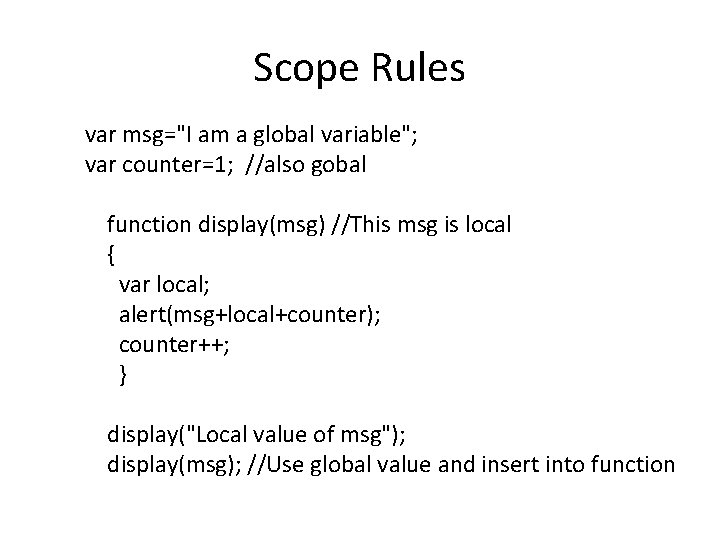
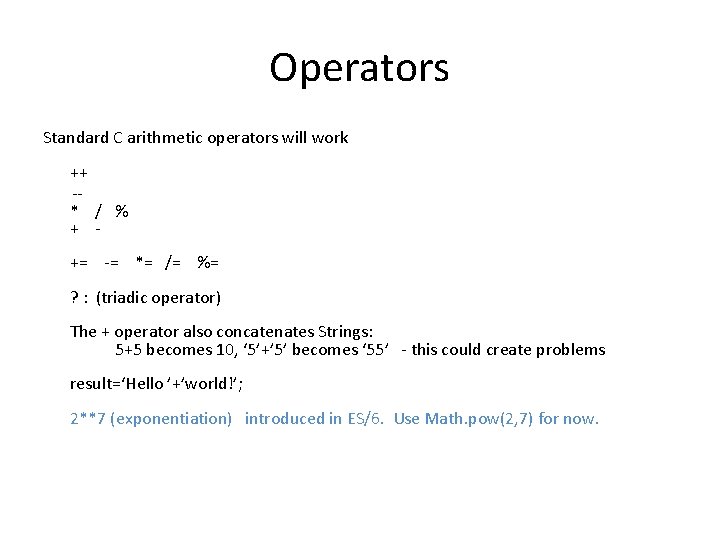
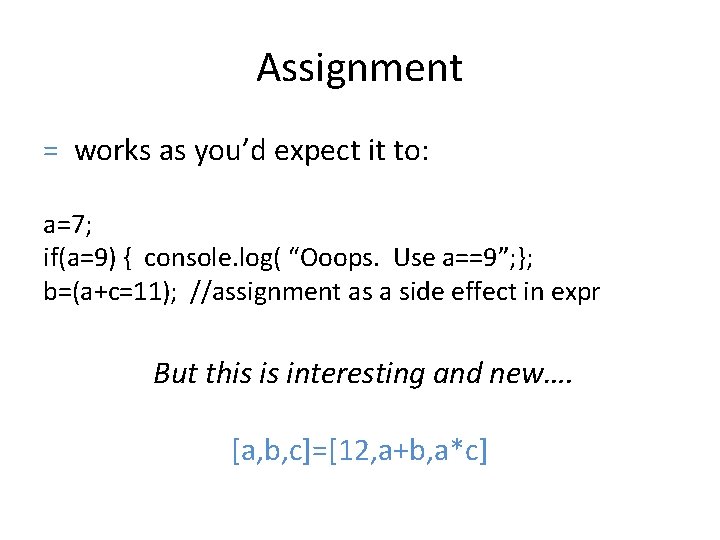
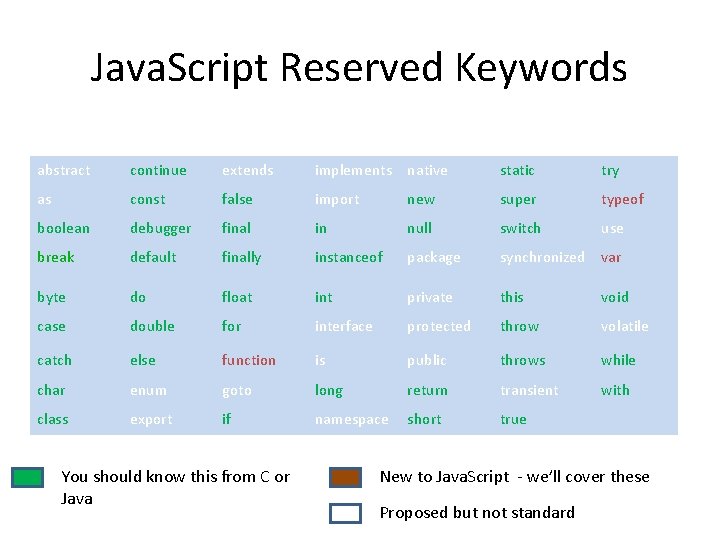
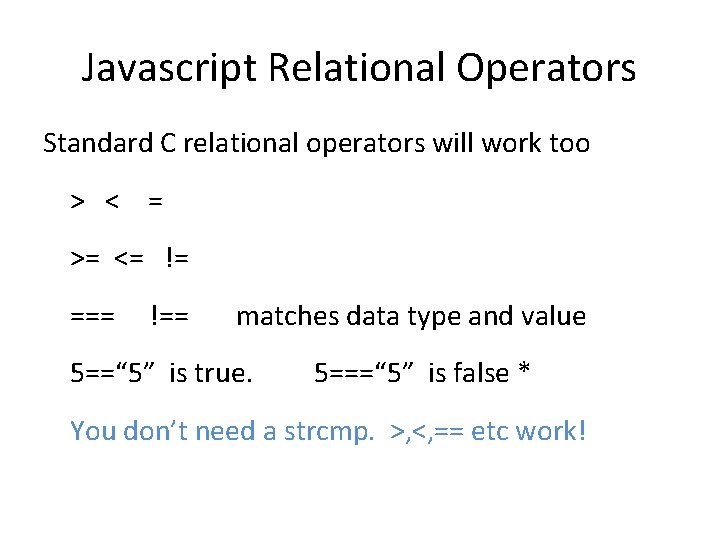
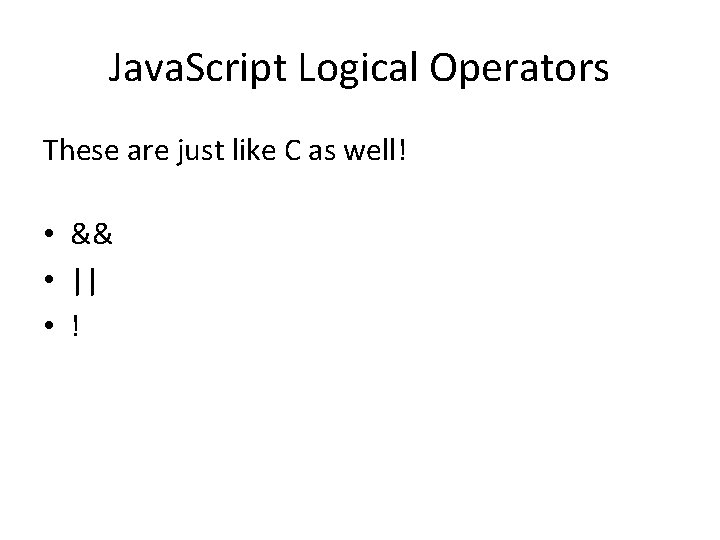
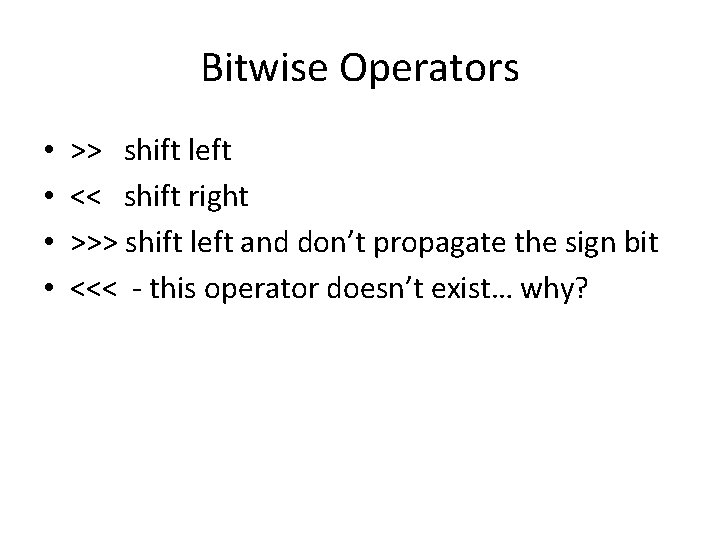
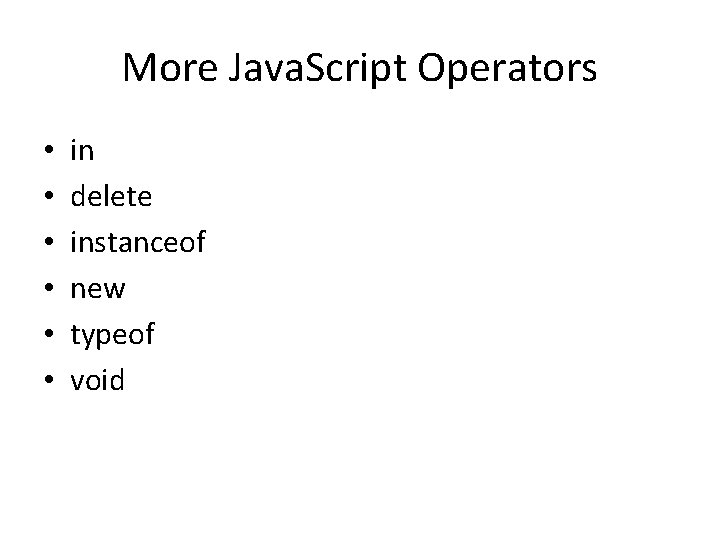
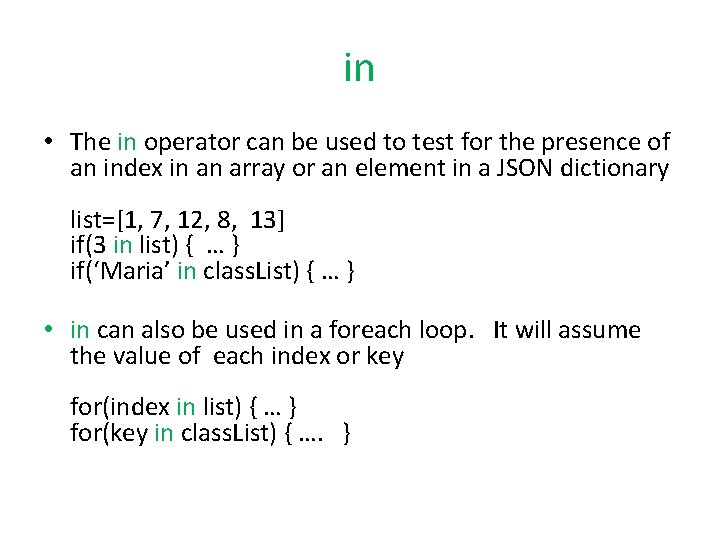
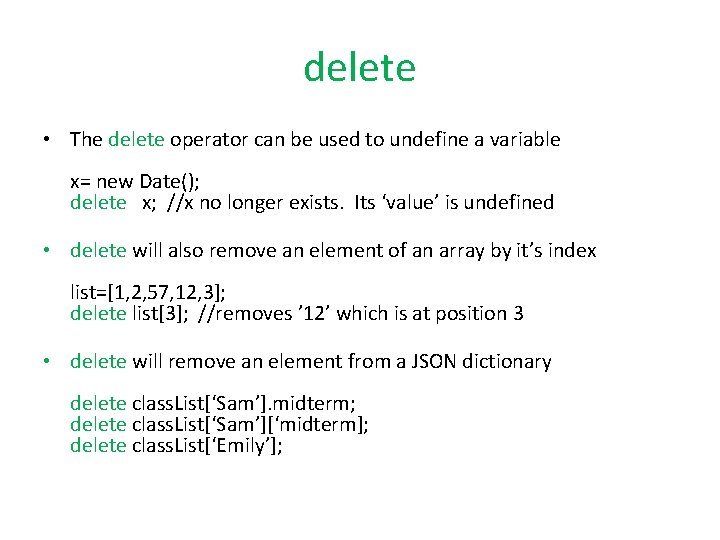
![[] . • [] and. are called the member of operators. In Java. Script [] . • [] and. are called the member of operators. In Java. Script](https://slidetodoc.com/presentation_image_h2/866320c96ebb535acbbc0ff8cb4a1755/image-31.jpg)
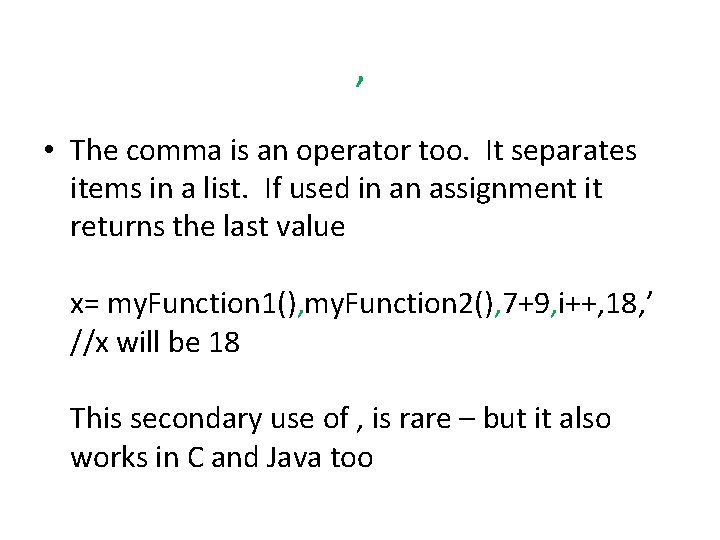
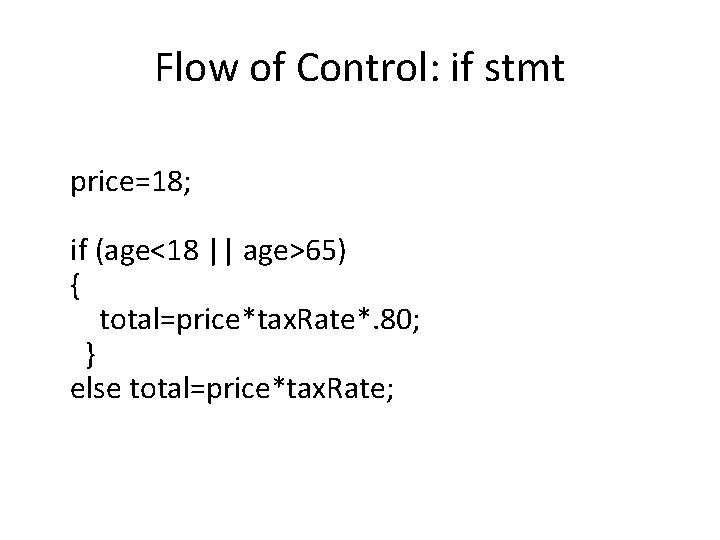
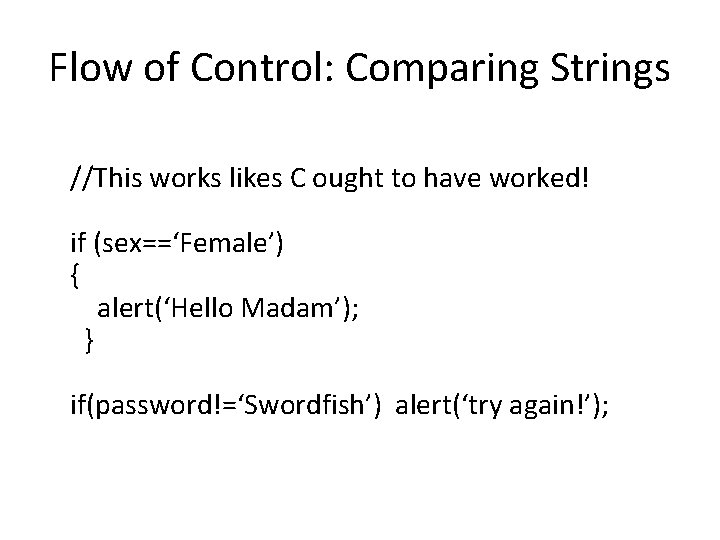
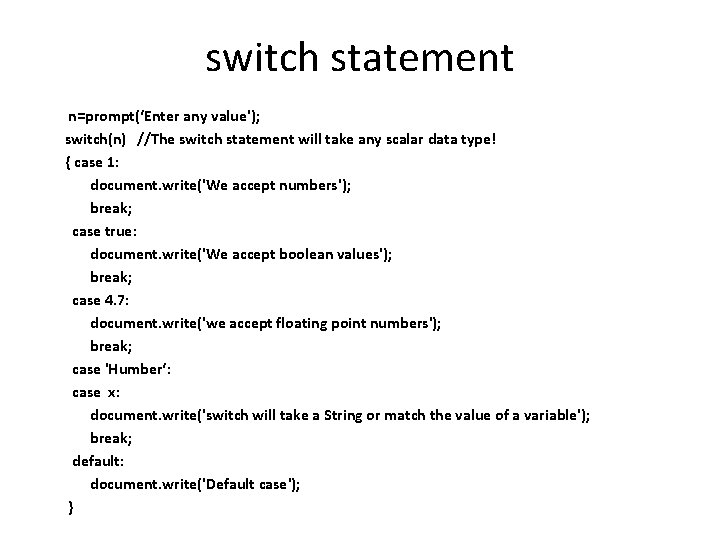
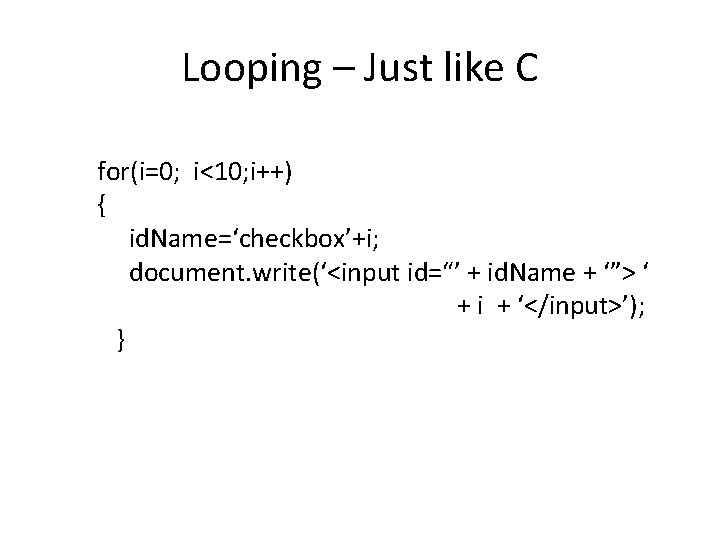
![A “for each” loop var students=['Bob', 'Mike', 'Rinky']; for(i in students) { alert(i+ ' A “for each” loop var students=['Bob', 'Mike', 'Rinky']; for(i in students) { alert(i+ '](https://slidetodoc.com/presentation_image_h2/866320c96ebb535acbbc0ff8cb4a1755/image-37.jpg)
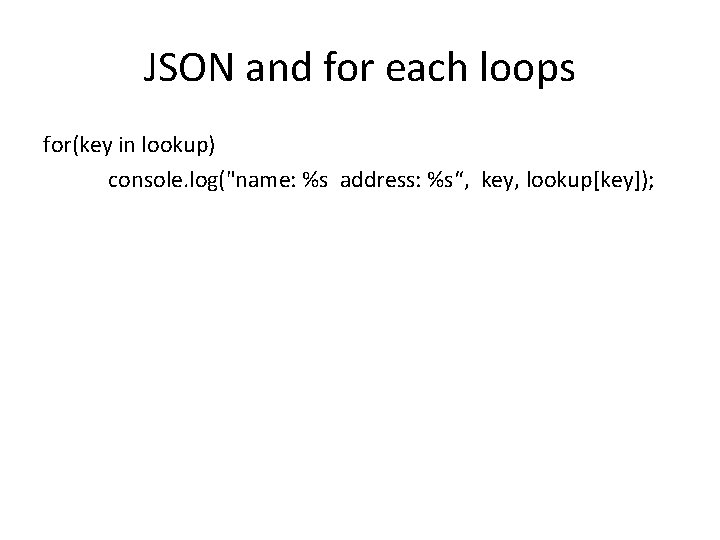
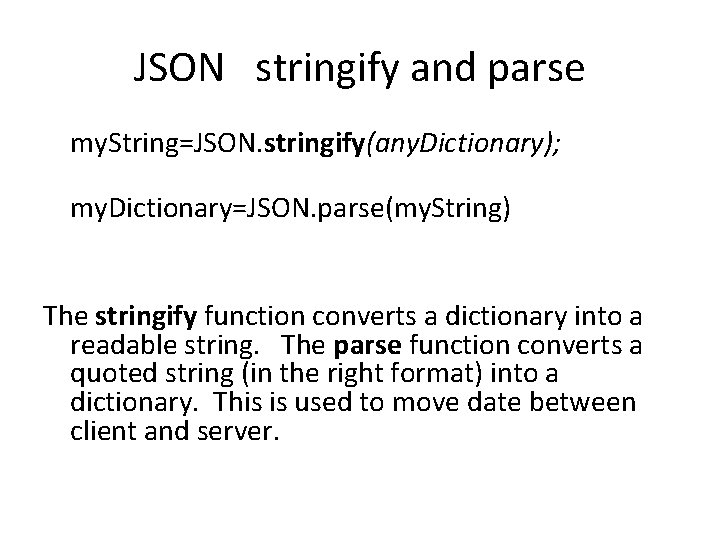
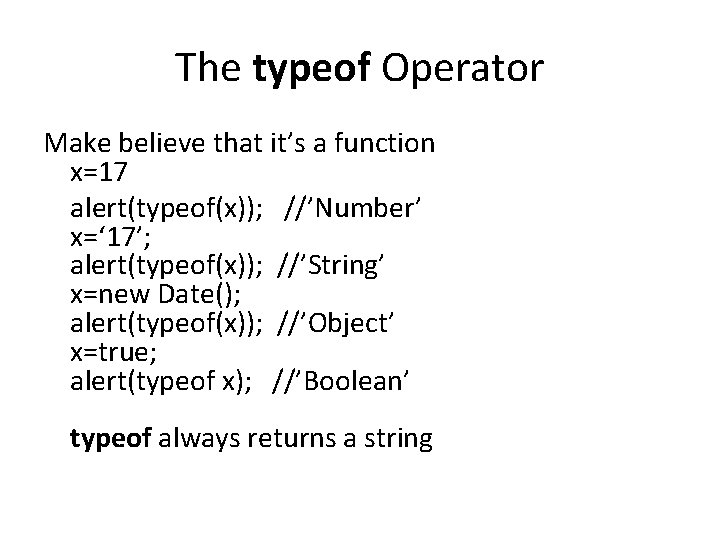
![Associative Arrays var students=['Bob', 'Mike', 'Rinky']; for(i in students) { alert(i+ ' ' +students[i]); Associative Arrays var students=['Bob', 'Mike', 'Rinky']; for(i in students) { alert(i+ ' ' +students[i]);](https://slidetodoc.com/presentation_image_h2/866320c96ebb535acbbc0ff8cb4a1755/image-41.jpg)
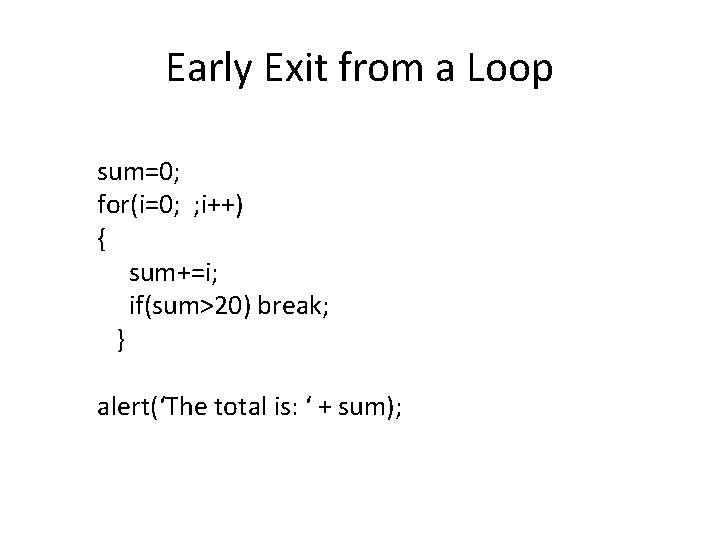
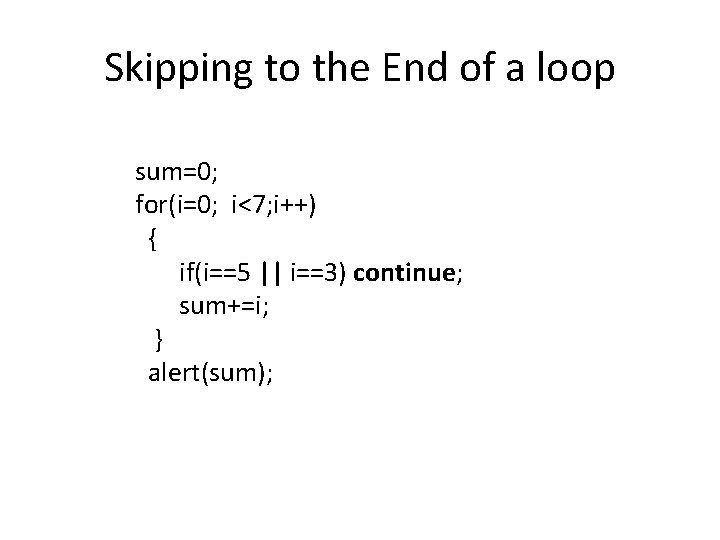
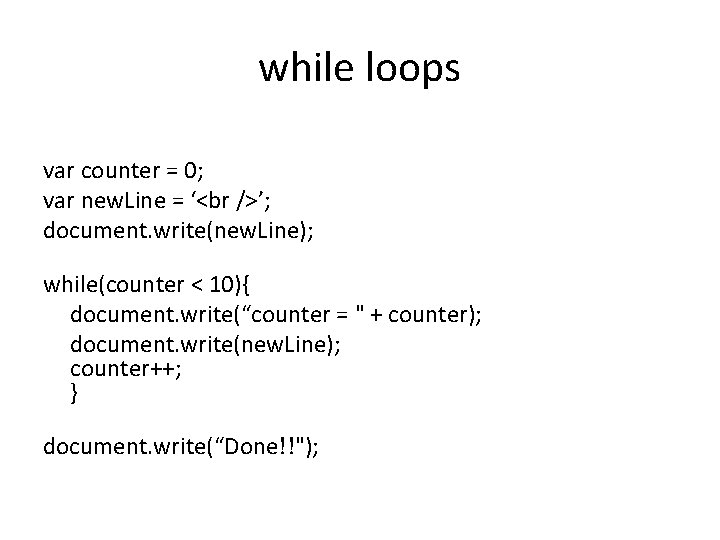
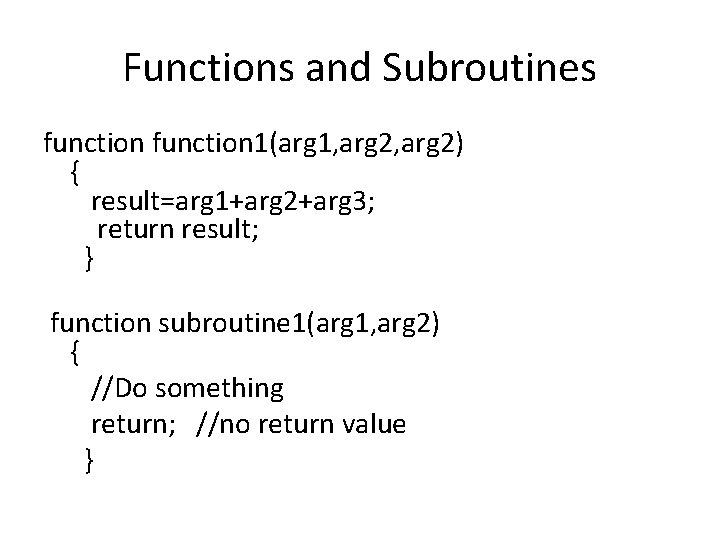
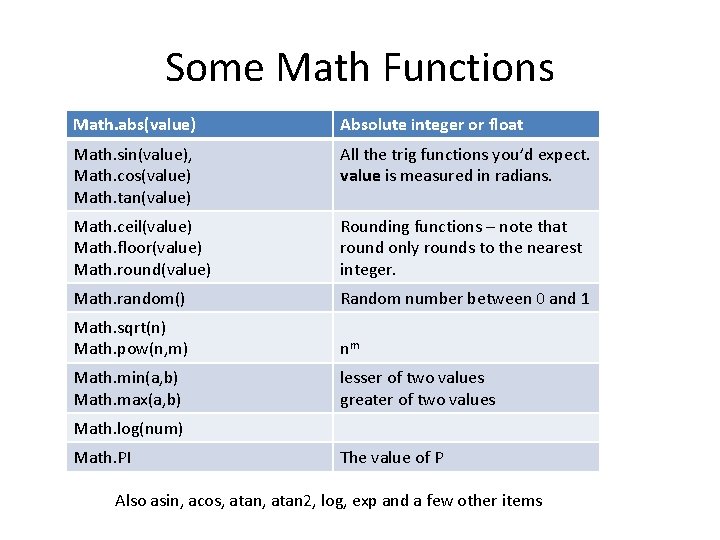
![String Functions “one, two, three”. split(‘, ’) Creates the array: [“one”, ”two”, ”three”] my. String Functions “one, two, three”. split(‘, ’) Creates the array: [“one”, ”two”, ”three”] my.](https://slidetodoc.com/presentation_image_h2/866320c96ebb535acbbc0ff8cb4a1755/image-47.jpg)
![Regular Expressions Regular expressions are directly part of the language reg. Expr=/([a-z]+)/gi; //Match all Regular Expressions Regular expressions are directly part of the language reg. Expr=/([a-z]+)/gi; //Match all](https://slidetodoc.com/presentation_image_h2/866320c96ebb535acbbc0ff8cb4a1755/image-48.jpg)
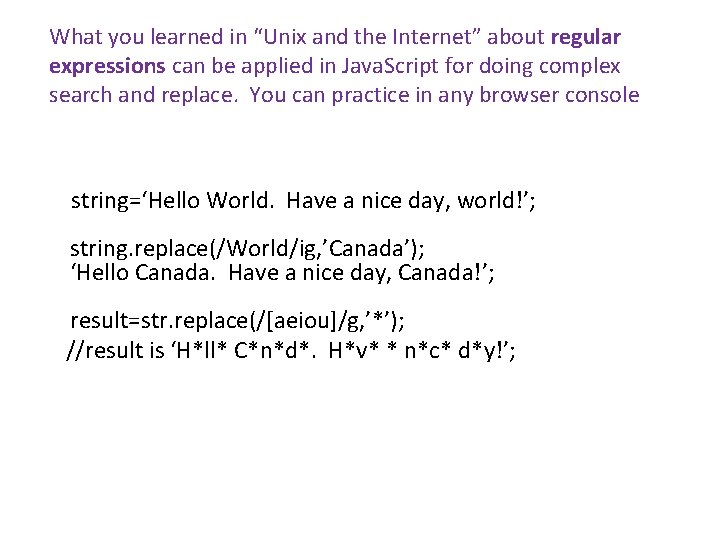
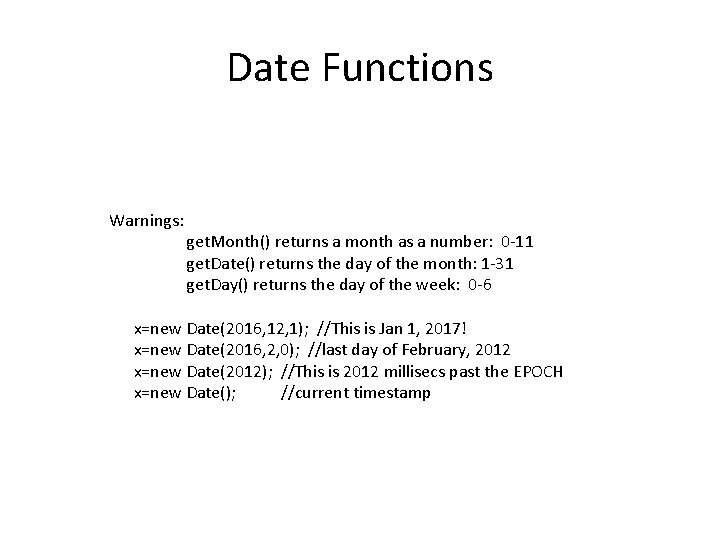
![Array Functions [“one”, ”two”, ”three”]. join(“; ”) Creates a string: one; two; three (it’s Array Functions [“one”, ”two”, ”three”]. join(“; ”) Creates a string: one; two; three (it’s](https://slidetodoc.com/presentation_image_h2/866320c96ebb535acbbc0ff8cb4a1755/image-51.jpg)
![The Array slice Function var fruits = ["Banana", "Orange", "Apple", "Mango"]; document. write(fruits. slice(0, The Array slice Function var fruits = ["Banana", "Orange", "Apple", "Mango"]; document. write(fruits. slice(0,](https://slidetodoc.com/presentation_image_h2/866320c96ebb535acbbc0ff8cb4a1755/image-52.jpg)
![The Array splice Function var fruits = ["Banana", "Orange", "Apple", "Mango"]; document. write("Removed: " The Array splice Function var fruits = ["Banana", "Orange", "Apple", "Mango"]; document. write("Removed: "](https://slidetodoc.com/presentation_image_h2/866320c96ebb535acbbc0ff8cb4a1755/image-53.jpg)
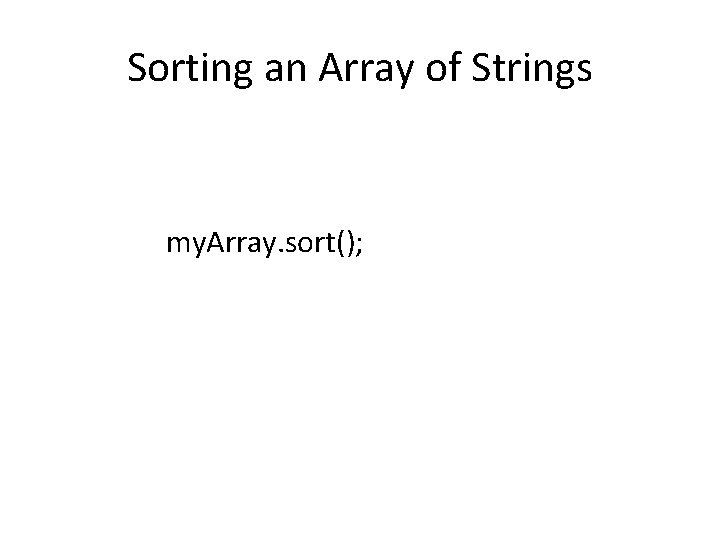
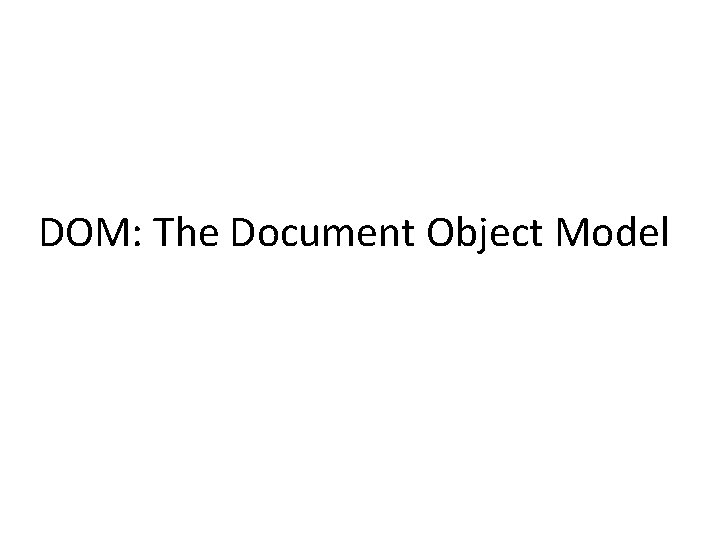
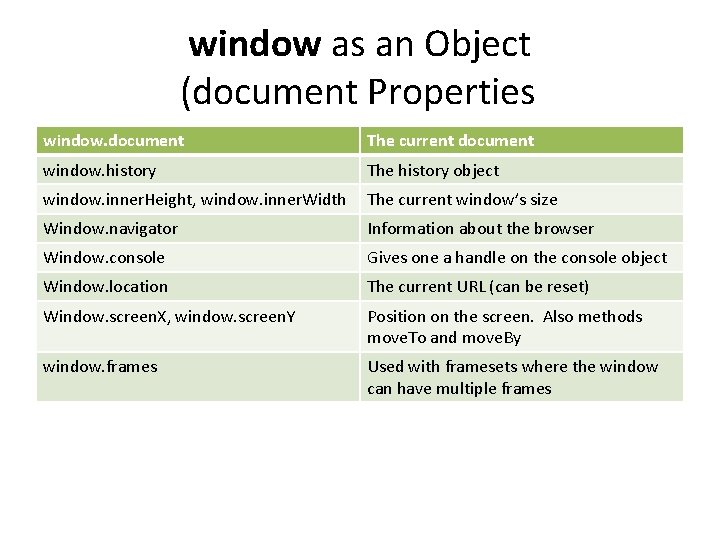
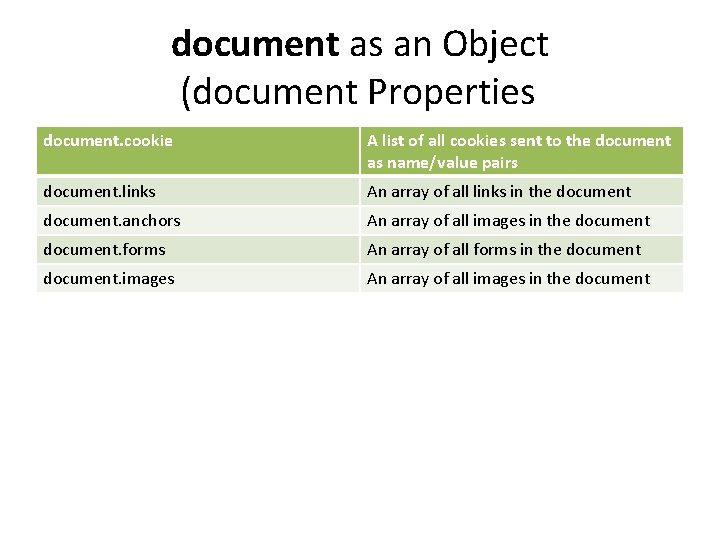
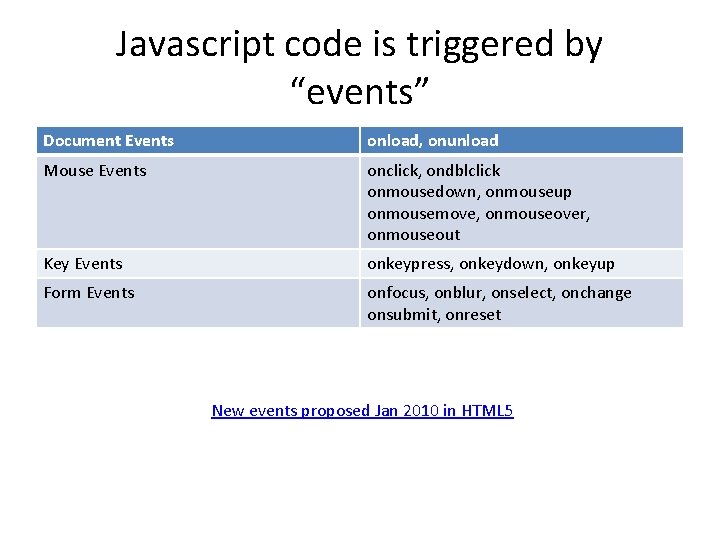
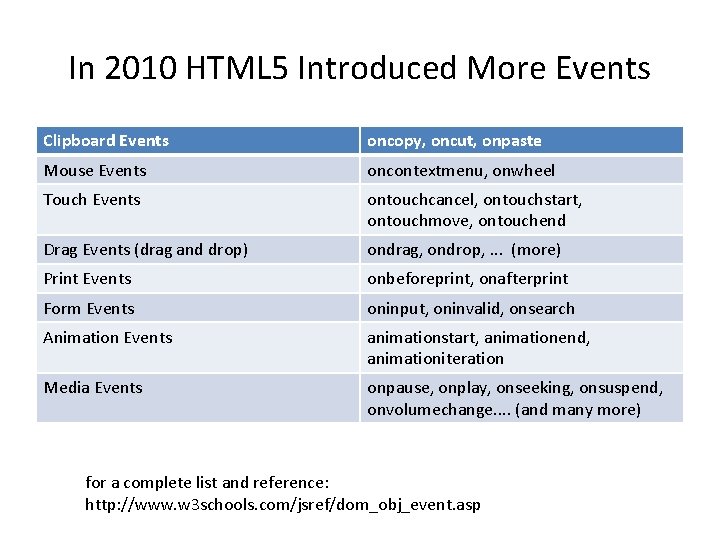
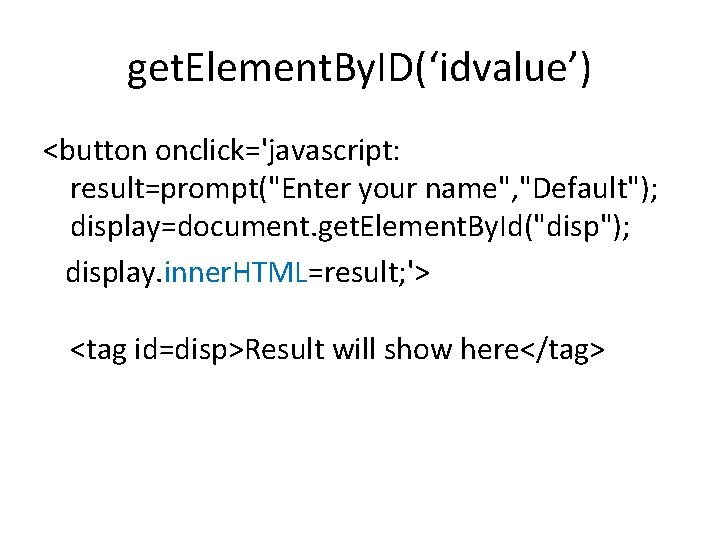
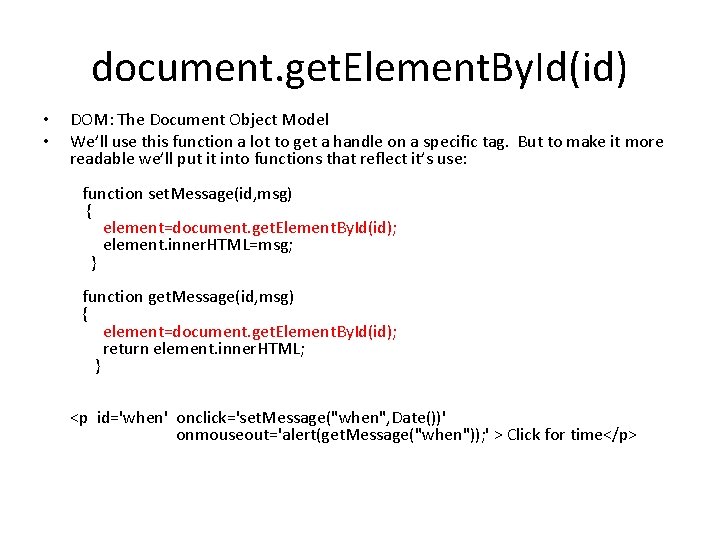
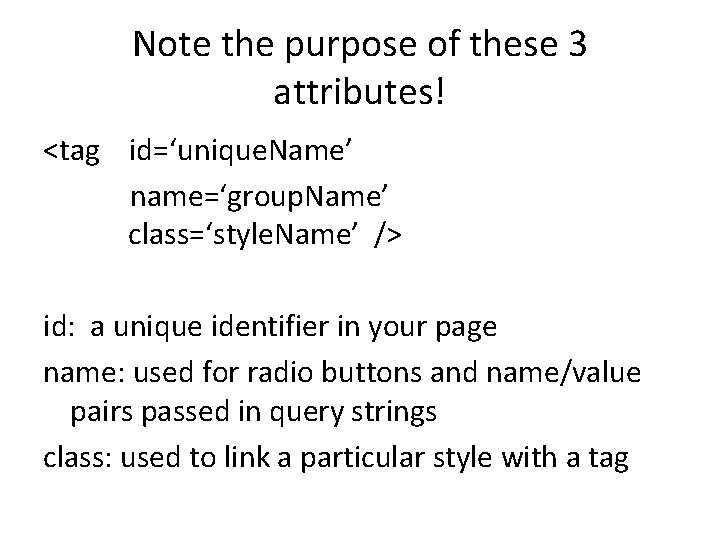
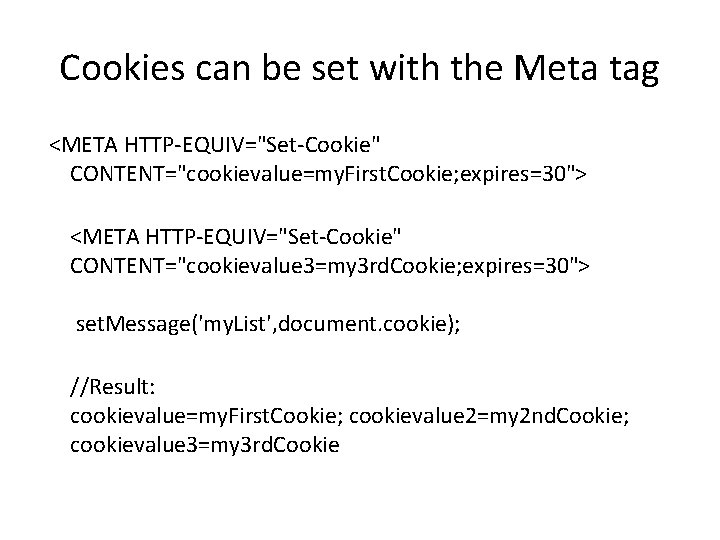
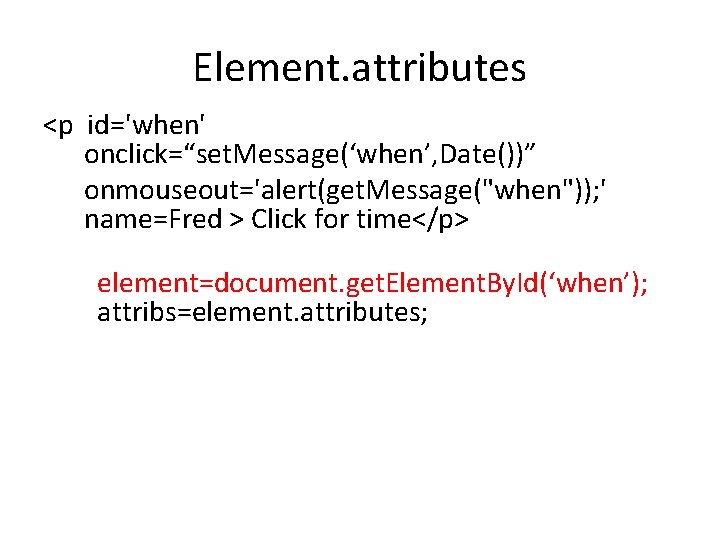
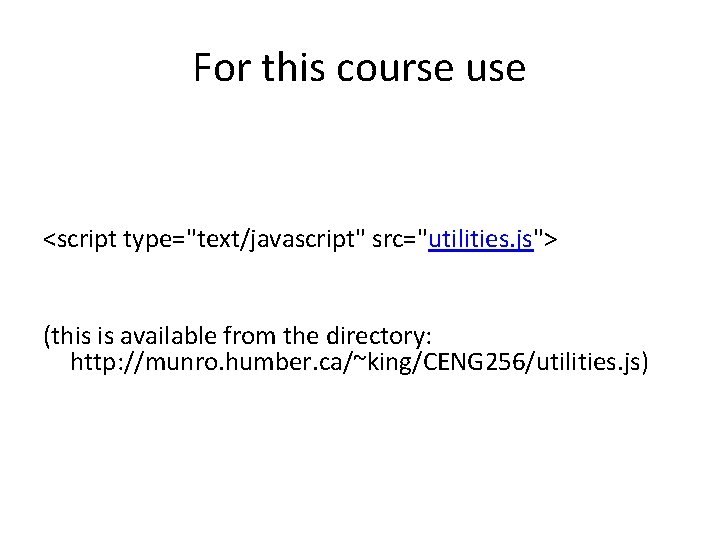
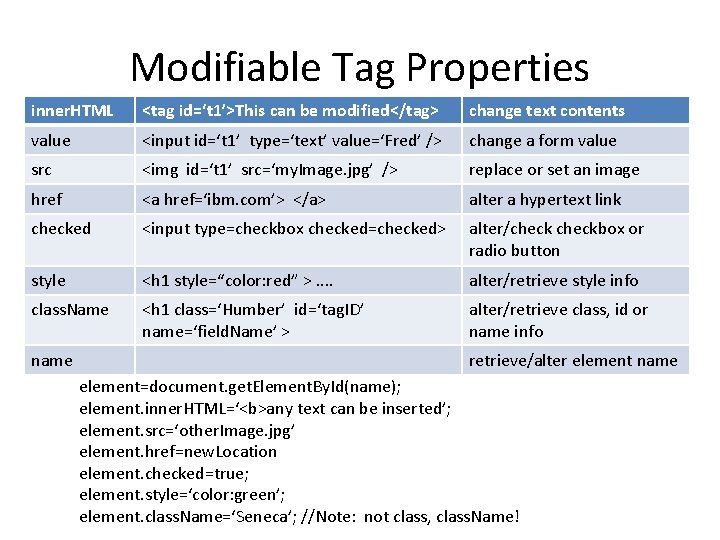
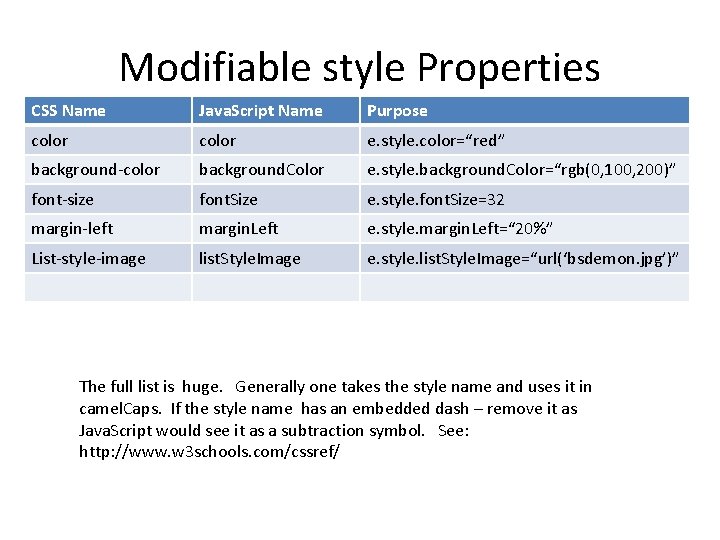
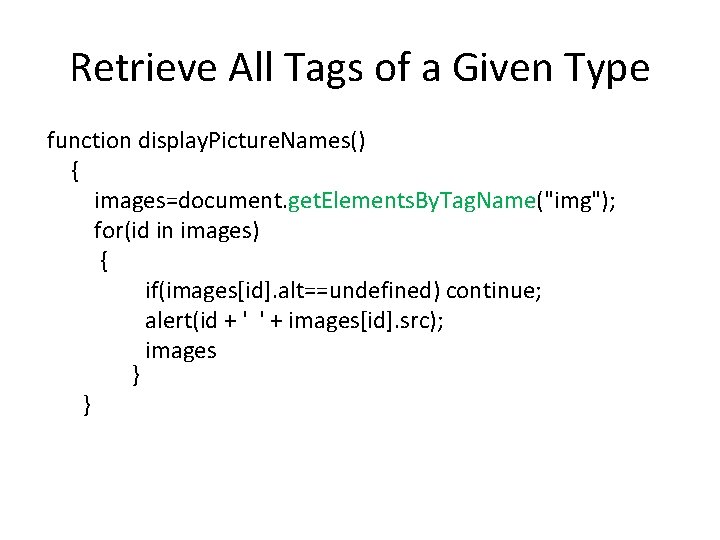
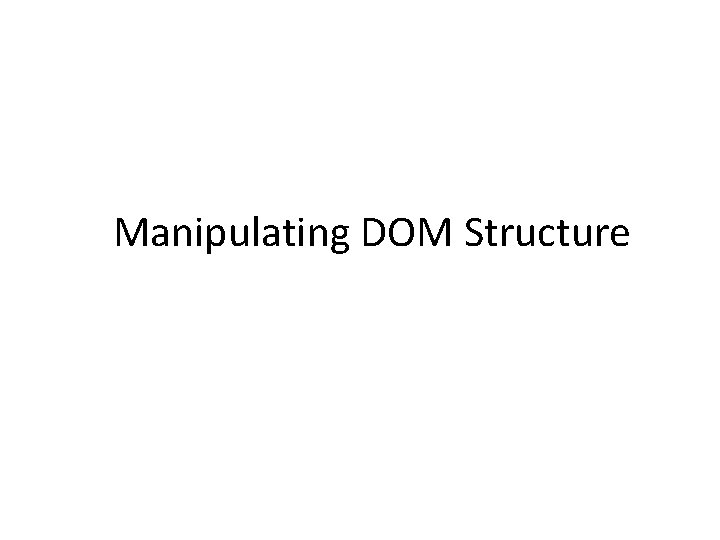
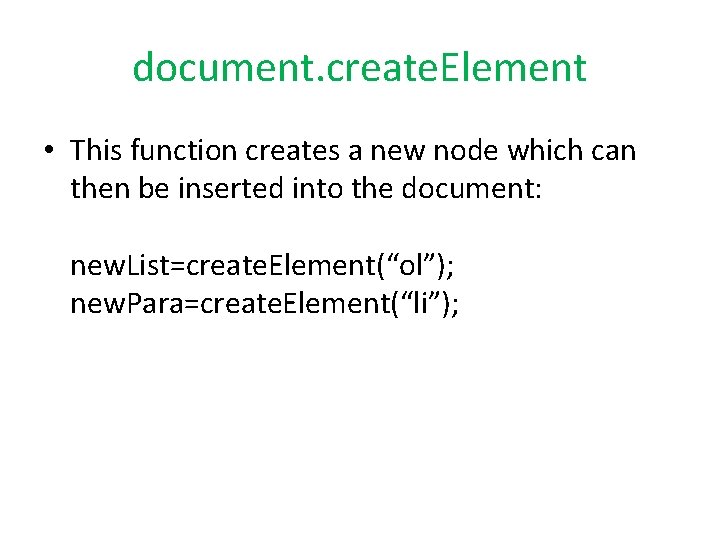
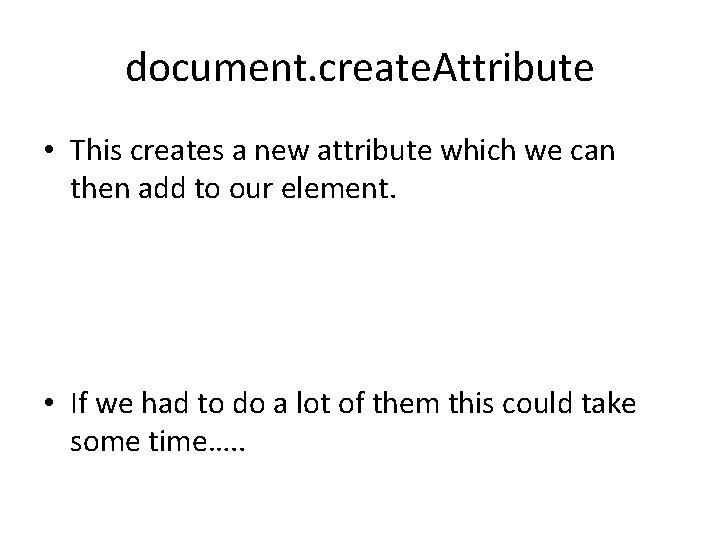
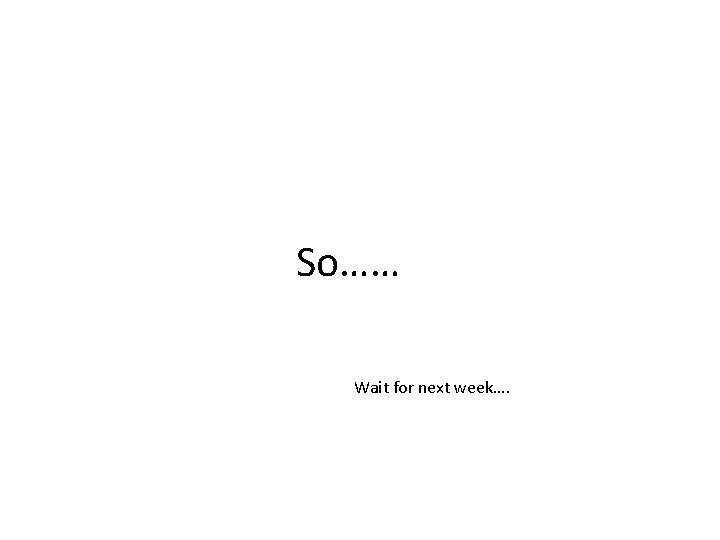
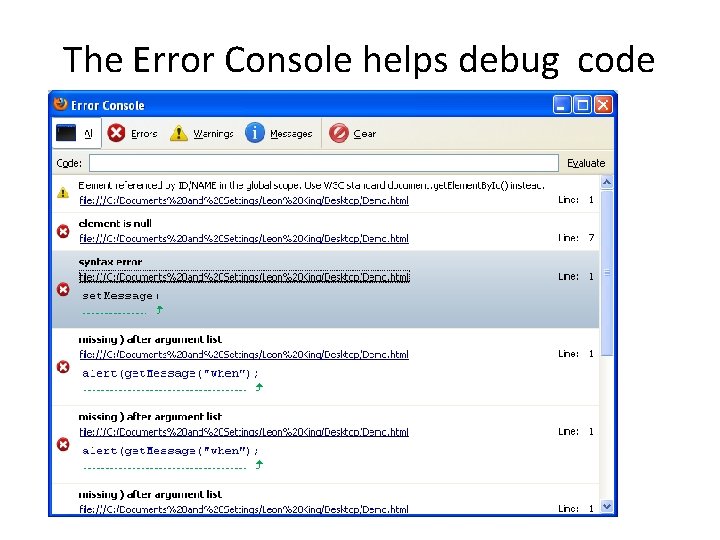
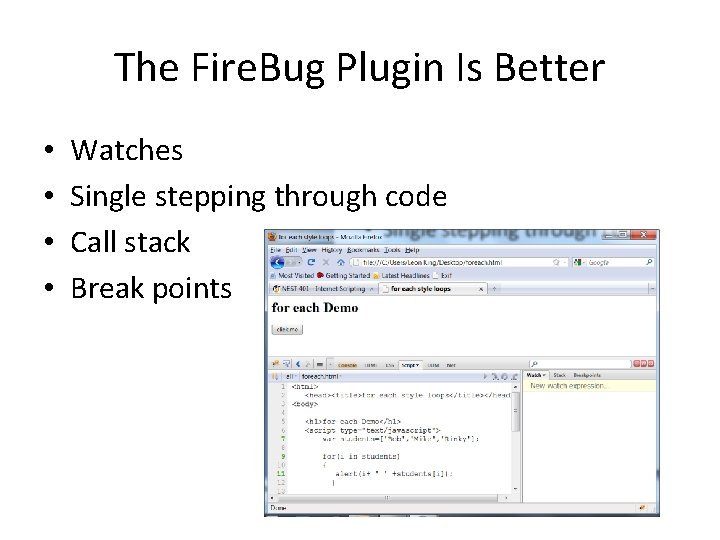
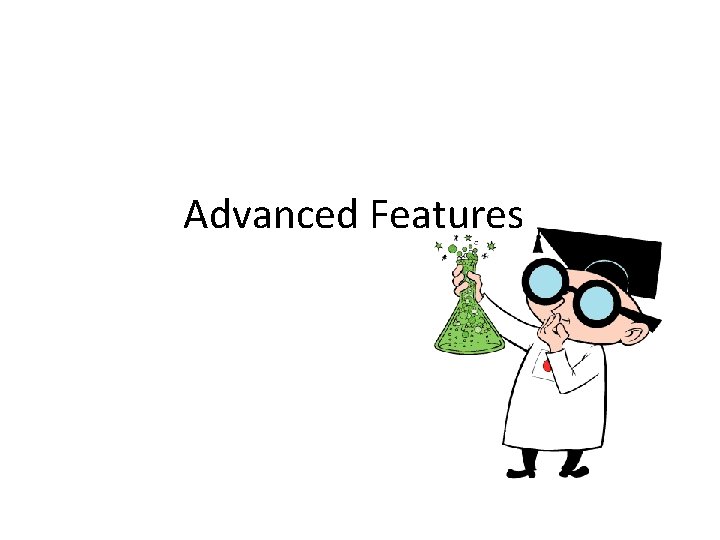
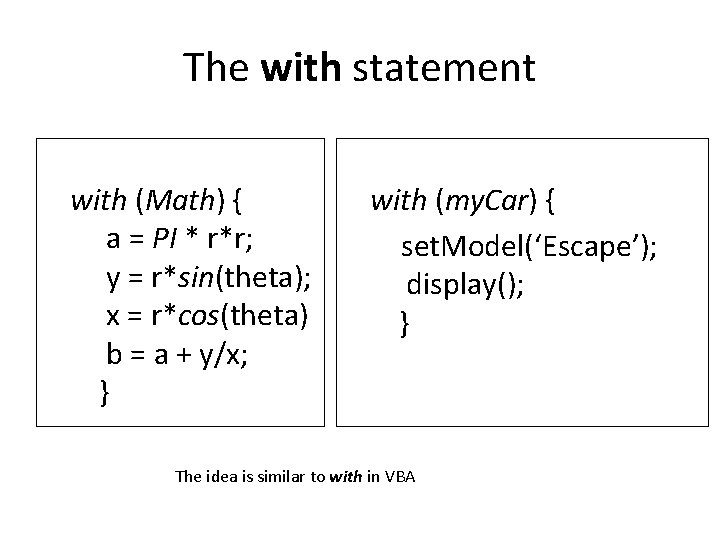
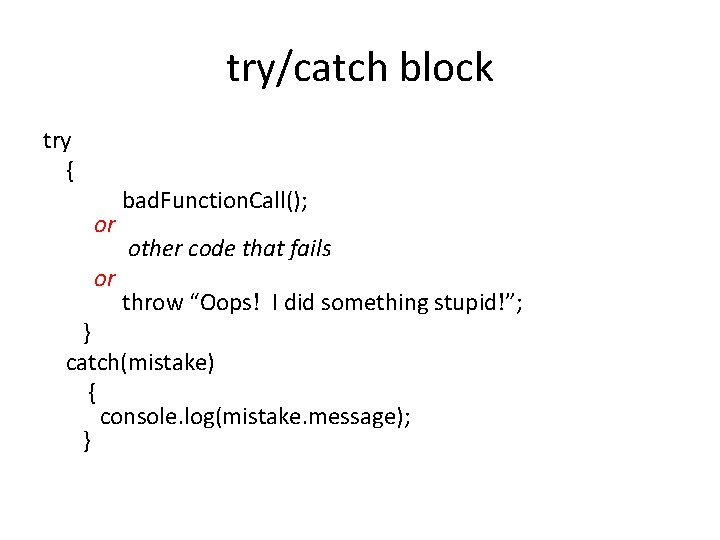
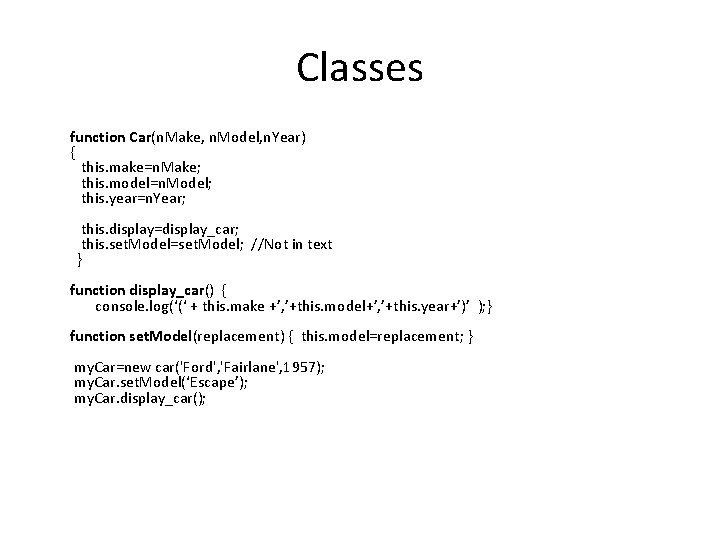
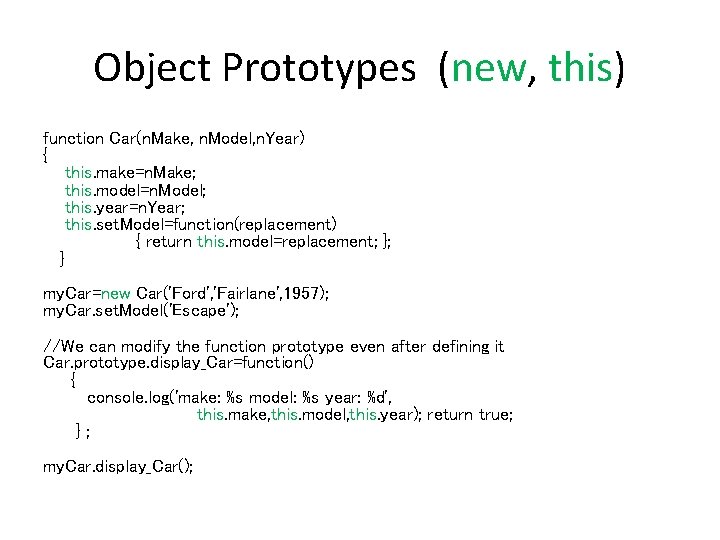
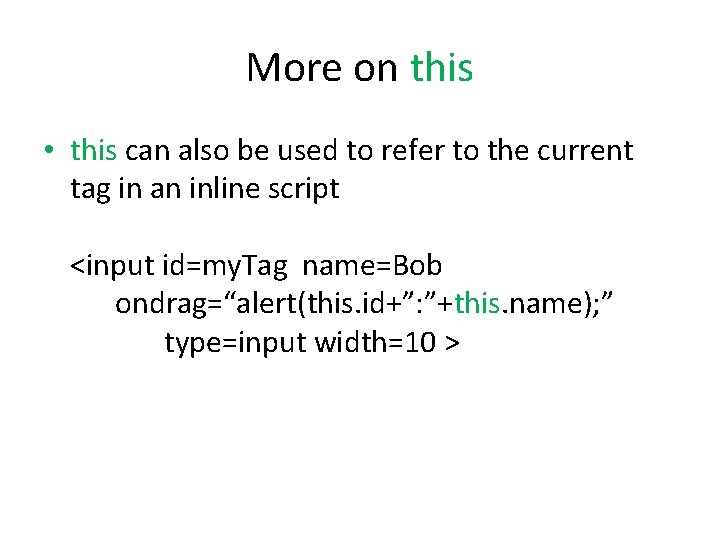
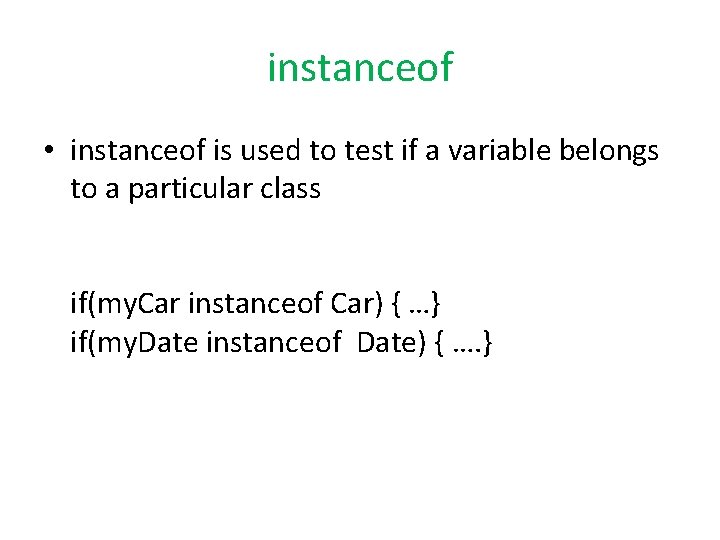
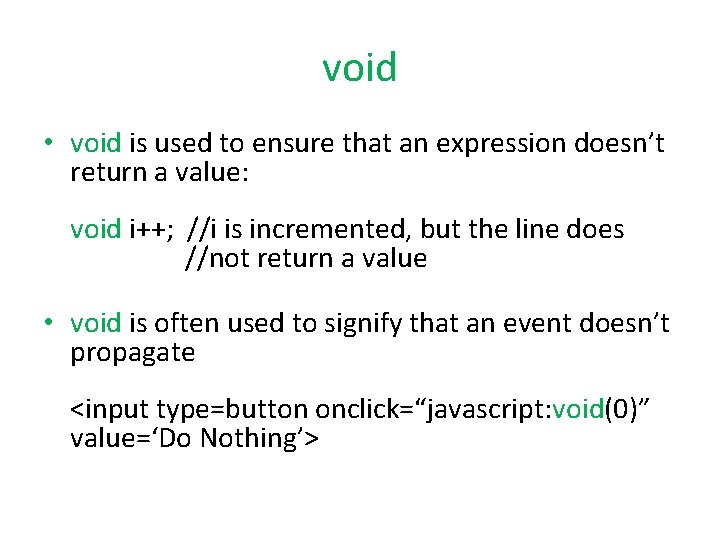
![Closures: map list=[1, 2, 3, 4]; console. log(list); Array [ 1, 2, 3, 4 Closures: map list=[1, 2, 3, 4]; console. log(list); Array [ 1, 2, 3, 4](https://slidetodoc.com/presentation_image_h2/866320c96ebb535acbbc0ff8cb4a1755/image-83.jpg)
![Closures: reduce list=[1, 2, 57, 3, 4] //Return the odd numbers only odd. Nums=list. Closures: reduce list=[1, 2, 57, 3, 4] //Return the odd numbers only odd. Nums=list.](https://slidetodoc.com/presentation_image_h2/866320c96ebb535acbbc0ff8cb4a1755/image-84.jpg)
- Slides: 84
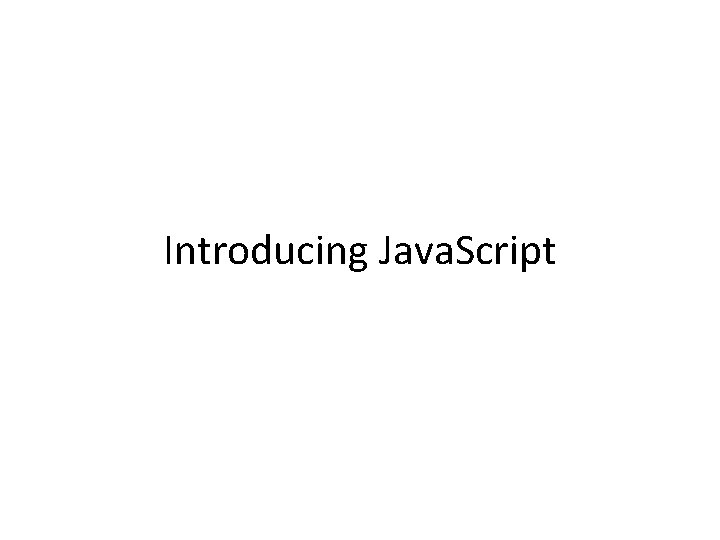
Introducing Java. Script
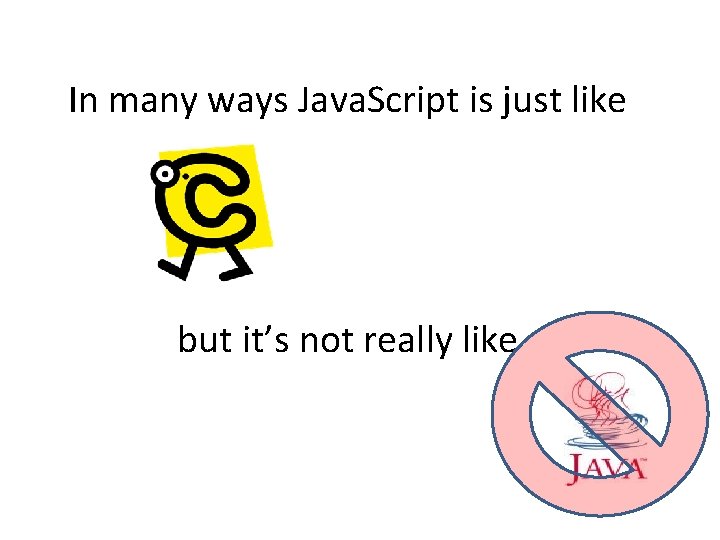
In many ways Java. Script is just like but it’s not really like
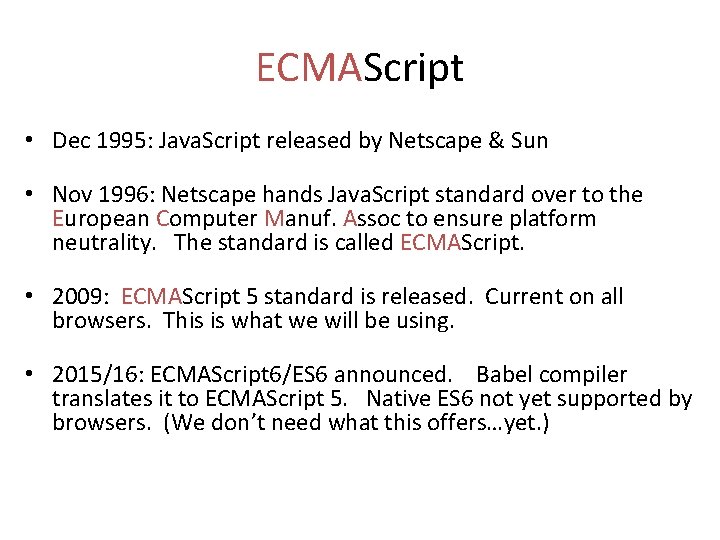
ECMAScript • Dec 1995: Java. Script released by Netscape & Sun • Nov 1996: Netscape hands Java. Script standard over to the European Computer Manuf. Assoc to ensure platform neutrality. The standard is called ECMAScript. • 2009: ECMAScript 5 standard is released. Current on all browsers. This is what we will be using. • 2015/16: ECMAScript 6/ES 6 announced. Babel compiler translates it to ECMAScript 5. Native ES 6 not yet supported by browsers. (We don’t need what this offers…yet. )
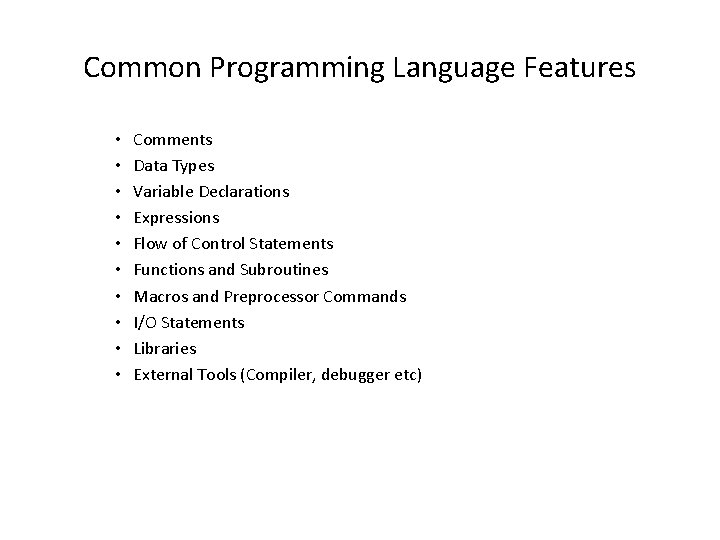
Common Programming Language Features • • • Comments Data Types Variable Declarations Expressions Flow of Control Statements Functions and Subroutines Macros and Preprocessor Commands I/O Statements Libraries External Tools (Compiler, debugger etc)
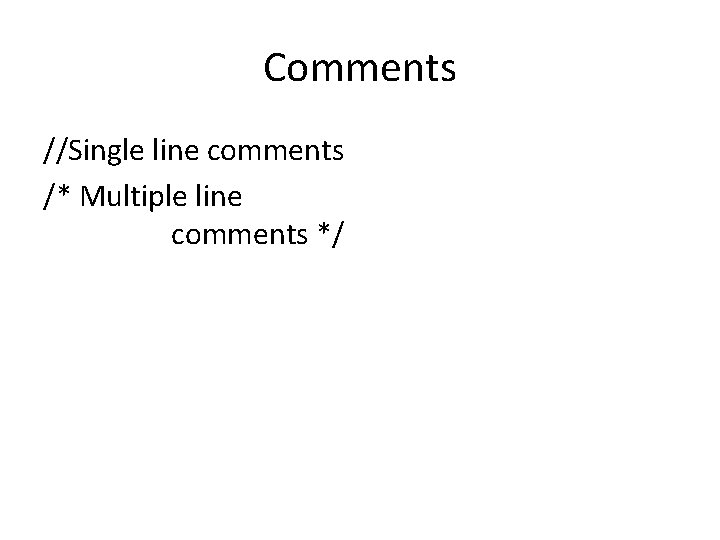
Comments //Single line comments /* Multiple line comments */
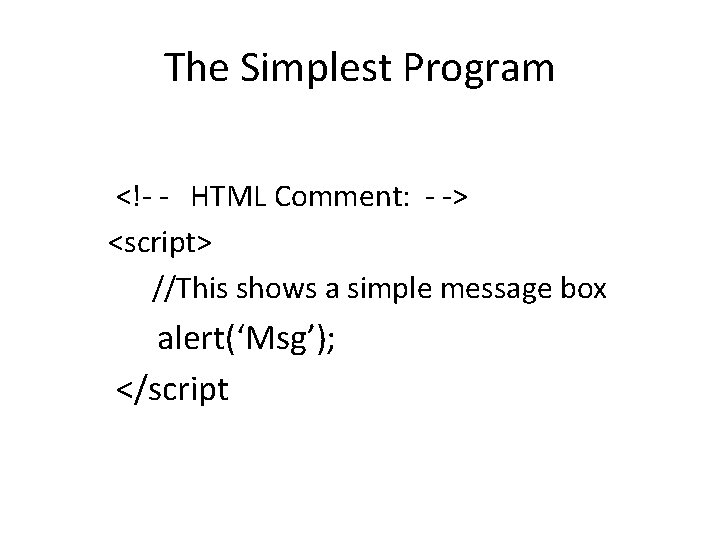
The Simplest Program <!- - HTML Comment: - -> <script> //This shows a simple message box alert(‘Msg’); </script
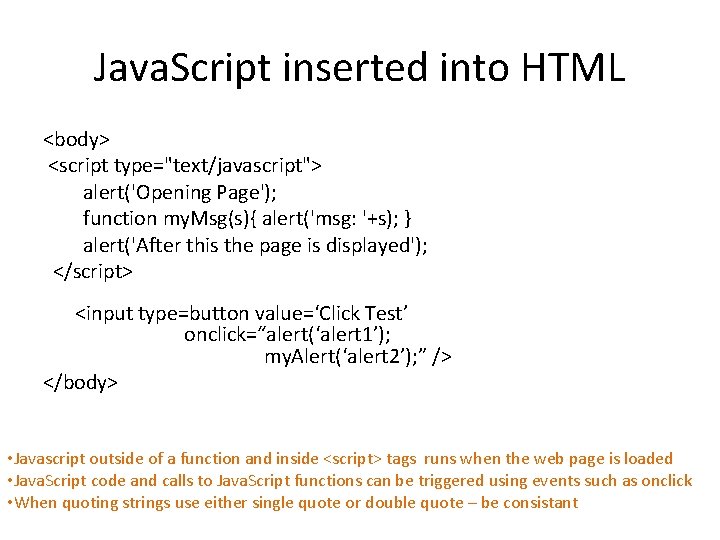
Java. Script inserted into HTML <body> <script type="text/javascript"> alert('Opening Page'); function my. Msg(s){ alert('msg: '+s); } alert('After this the page is displayed'); </script> <input type=button value=‘Click Test’ onclick=“alert(‘alert 1’); my. Alert(‘alert 2’); ” /> </body> • Javascript outside of a function and inside <script> tags runs when the web page is loaded • Java. Script code and calls to Java. Script functions can be triggered using events such as onclick • When quoting strings use either single quote or double quote – be consistant
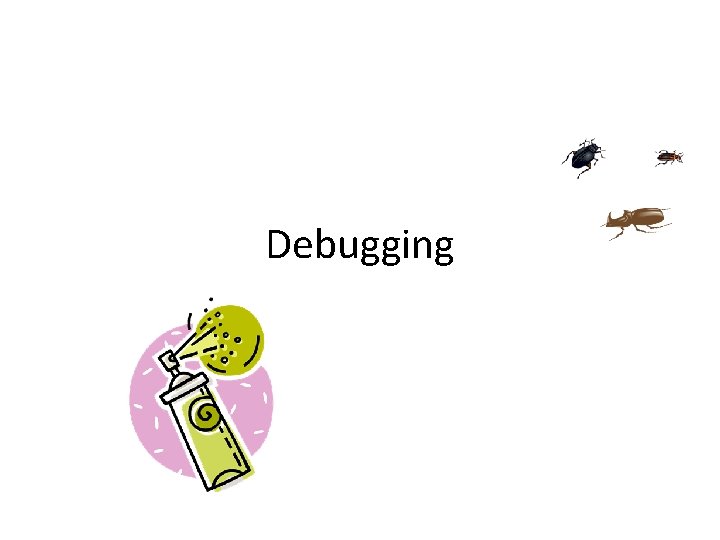
Debugging
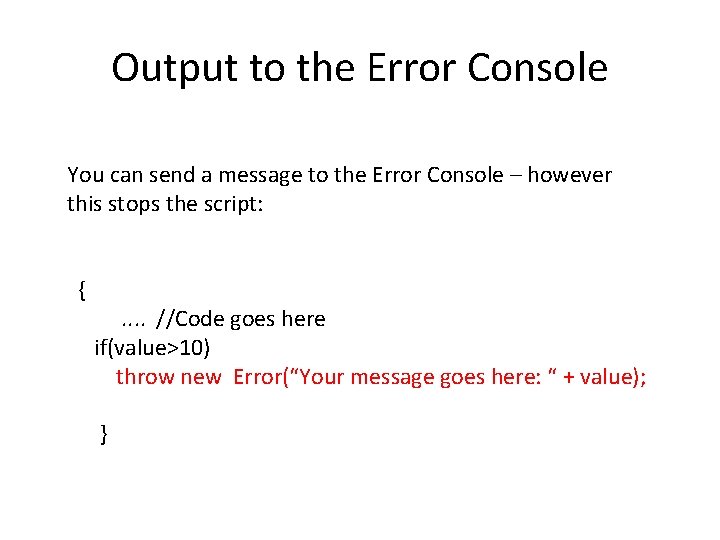
Output to the Error Console You can send a message to the Error Console – however this stops the script: { . . //Code goes here if(value>10) throw new Error(“Your message goes here: “ + value); }
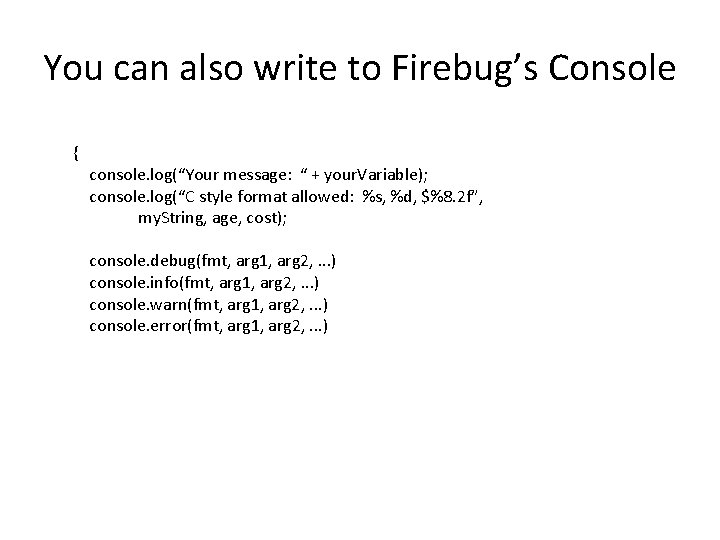
You can also write to Firebug’s Console { console. log(“Your message: “ + your. Variable); console. log(“C style format allowed: %s, %d, $%8. 2 f”, my. String, age, cost); console. debug(fmt, arg 1, arg 2, . . . ) console. info(fmt, arg 1, arg 2, . . . ) console. warn(fmt, arg 1, arg 2, . . . ) console. error(fmt, arg 1, arg 2, . . . )
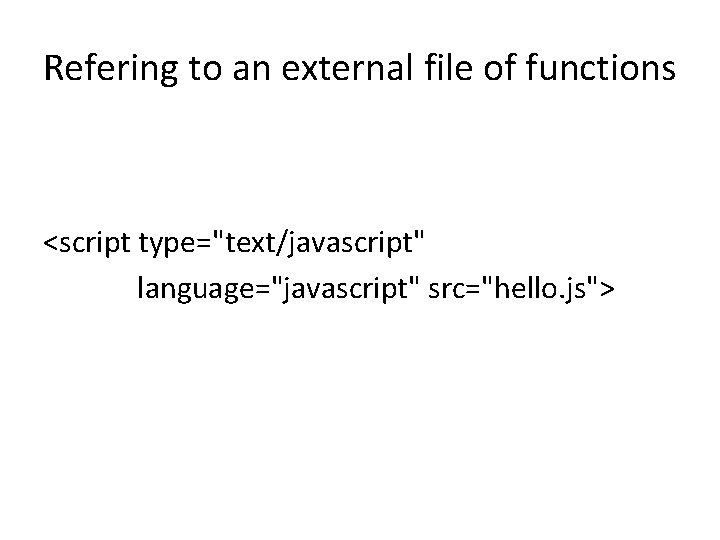
Refering to an external file of functions <script type="text/javascript" language="javascript" src="hello. js">
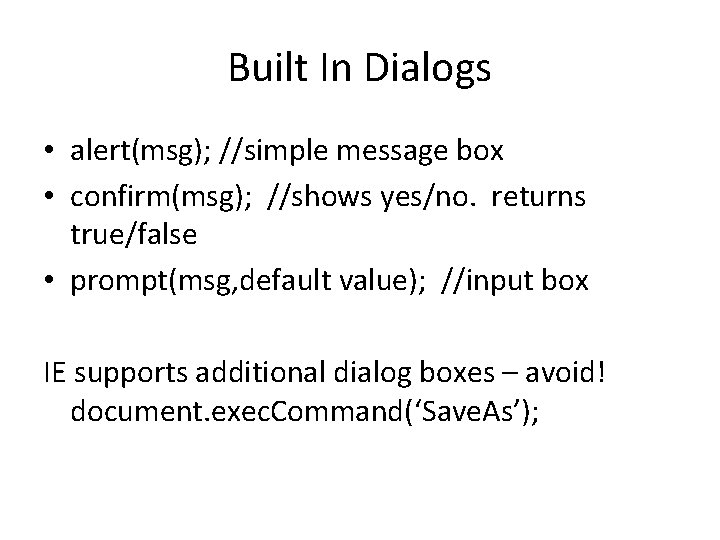
Built In Dialogs • alert(msg); //simple message box • confirm(msg); //shows yes/no. returns true/false • prompt(msg, default value); //input box IE supports additional dialog boxes – avoid! document. exec. Command(‘Save. As’);
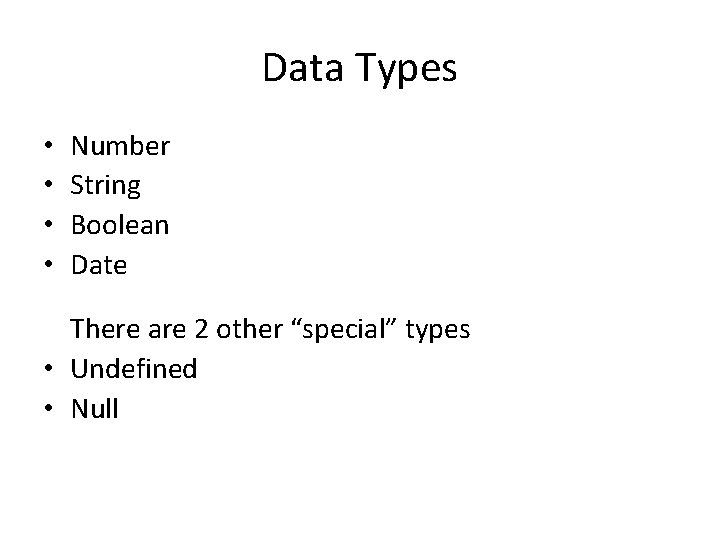
Data Types • • Number String Boolean Date There are 2 other “special” types • Undefined • Null
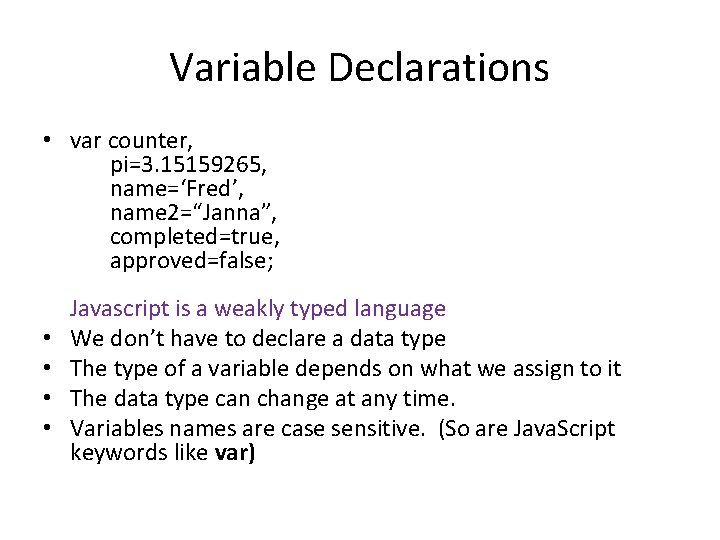
Variable Declarations • var counter, pi=3. 15159265, name=‘Fred’, name 2=“Janna”, completed=true, approved=false; • • Javascript is a weakly typed language We don’t have to declare a data type The type of a variable depends on what we assign to it The data type can change at any time. Variables names are case sensitive. (So are Java. Script keywords like var)
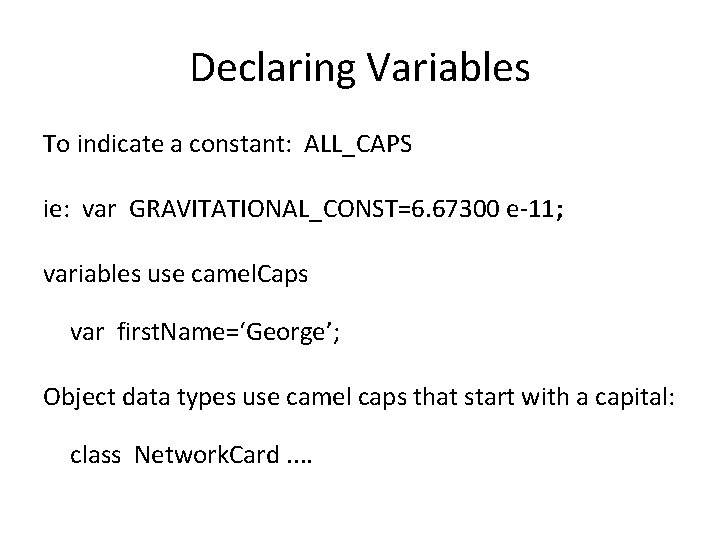
Declaring Variables To indicate a constant: ALL_CAPS ie: var GRAVITATIONAL_CONST=6. 67300 e-11; variables use camel. Caps var first. Name=‘George’; Object data types use camel caps that start with a capital: class Network. Card. .
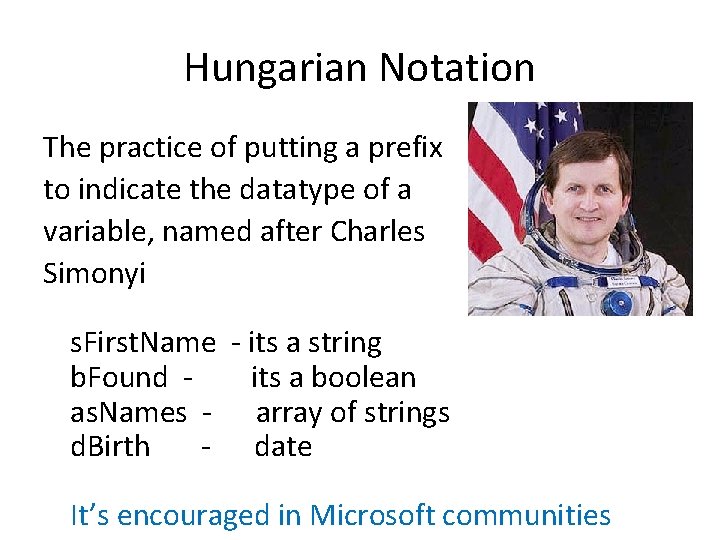
Hungarian Notation The practice of putting a prefix to indicate the datatype of a variable, named after Charles Simonyi s. First. Name - its a string b. Found its a boolean as. Names - array of strings d. Birth - date It’s encouraged in Microsoft communities
![Declaring Arrays var namesMary Murray Jim var nested Array 1 2 3 4 Declaring Arrays • var names=[‘Mary’, ”Murray”, ”Jim”]; var nested. Array=[ [1, 2, 3], [4,](https://slidetodoc.com/presentation_image_h2/866320c96ebb535acbbc0ff8cb4a1755/image-17.jpg)
Declaring Arrays • var names=[‘Mary’, ”Murray”, ”Jim”]; var nested. Array=[ [1, 2, 3], [4, 5, 6], [7, 8, 9]]; var list=new Array(‘Martha’, ’Muffin’, ’Donut’, 18); var my. Array=new Array(); for(i=1; i<10; i++) my. Array[i]=i*i; my. Array[100] = 42; We don’t have to say how big an array is in advance of its use! We can mix different data types in an array!
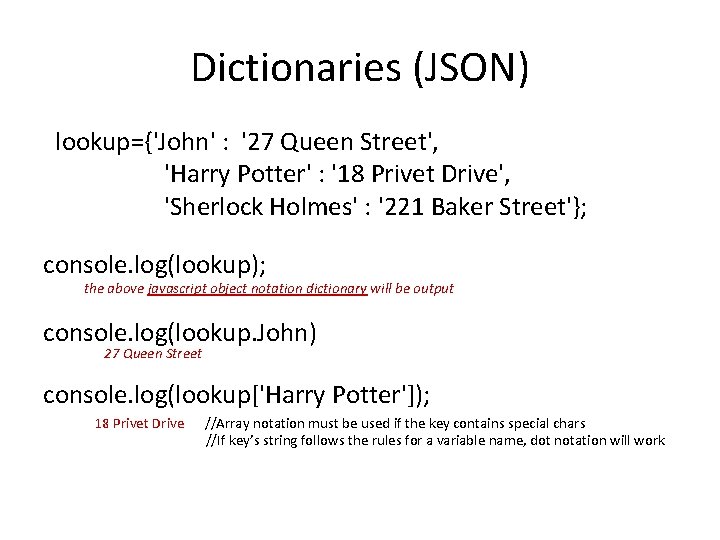
Dictionaries (JSON) lookup={'John' : '27 Queen Street', 'Harry Potter' : '18 Privet Drive', 'Sherlock Holmes' : '221 Baker Street'}; console. log(lookup); the above javascript object notation dictionary will be output console. log(lookup. John) 27 Queen Street console. log(lookup['Harry Potter']); 18 Privet Drive //Array notation must be used if the key contains special chars //If key’s string follows the rules for a variable name, dot notation will work
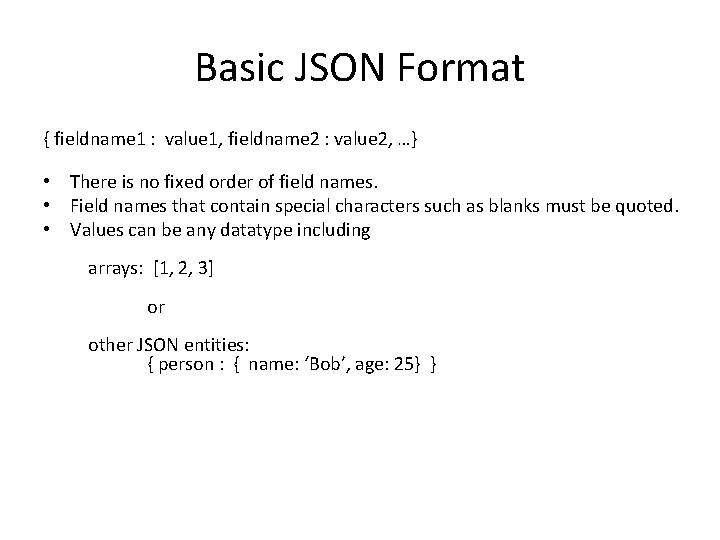
Basic JSON Format { fieldname 1 : value 1, fieldname 2 : value 2, …} • There is no fixed order of field names. • Field names that contain special characters such as blanks must be quoted. • Values can be any datatype including arrays: [1, 2, 3] or other JSON entities: { person : { name: ‘Bob’, age: 25} }
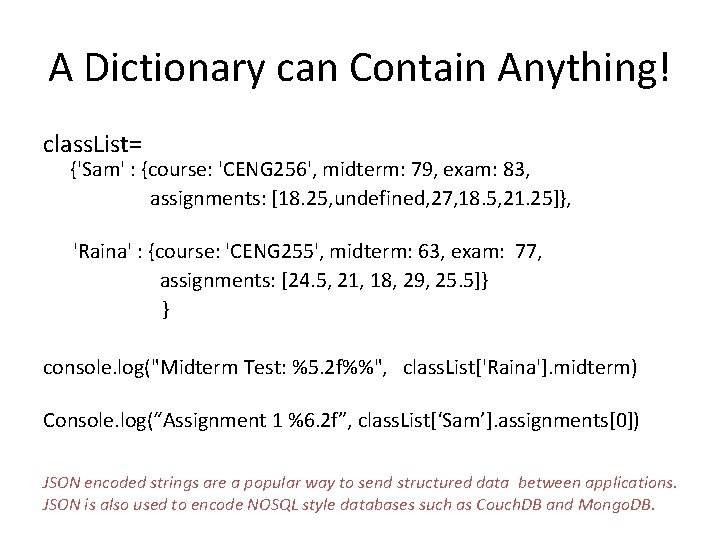
A Dictionary can Contain Anything! class. List= {'Sam' : {course: 'CENG 256', midterm: 79, exam: 83, assignments: [18. 25, undefined, 27, 18. 5, 21. 25]}, 'Raina' : {course: 'CENG 255', midterm: 63, exam: 77, assignments: [24. 5, 21, 18, 29, 25. 5]} } console. log("Midterm Test: %5. 2 f%%", class. List['Raina']. midterm) Console. log(“Assignment 1 %6. 2 f”, class. List[‘Sam’]. assignments[0]) JSON encoded strings are a popular way to send structured data between applications. JSON is also used to encode NOSQL style databases such as Couch. DB and Mongo. DB.
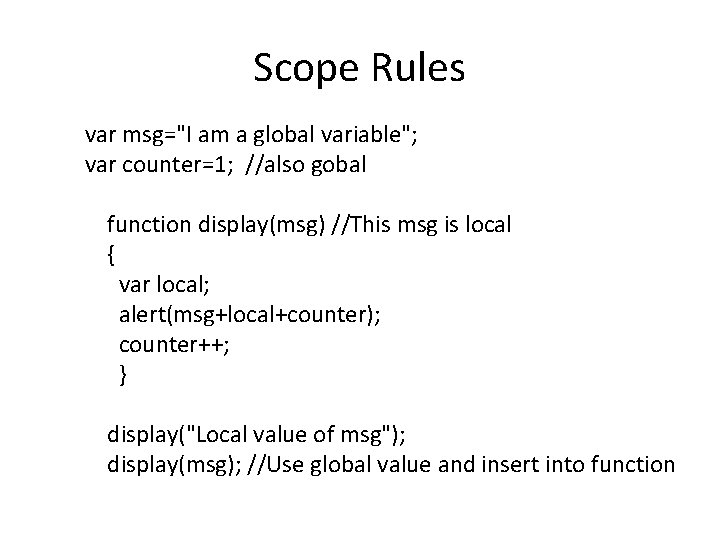
Scope Rules var msg="I am a global variable"; var counter=1; //also gobal function display(msg) //This msg is local { var local; alert(msg+local+counter); counter++; } display("Local value of msg"); display(msg); //Use global value and insert into function
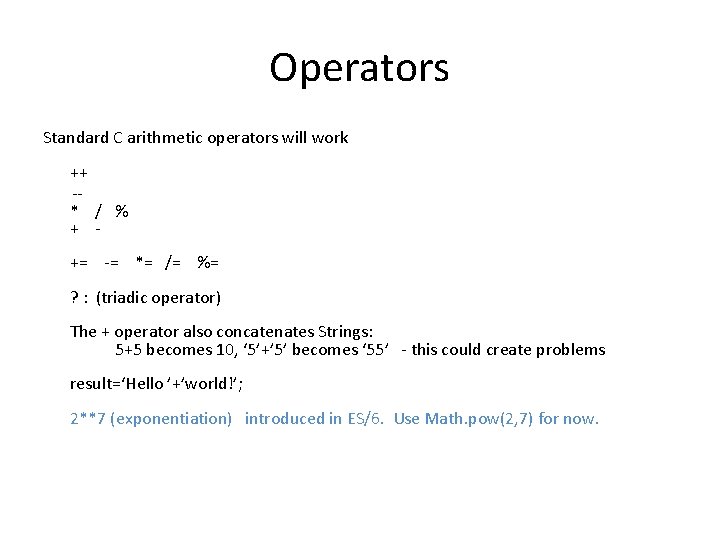
Operators Standard C arithmetic operators will work ++ -* / % + += -= *= /= %= ? : (triadic operator) The + operator also concatenates Strings: 5+5 becomes 10, ‘ 5’+’ 5’ becomes ‘ 55’ - this could create problems result=‘Hello ’+’world!’; 2**7 (exponentiation) introduced in ES/6. Use Math. pow(2, 7) for now.
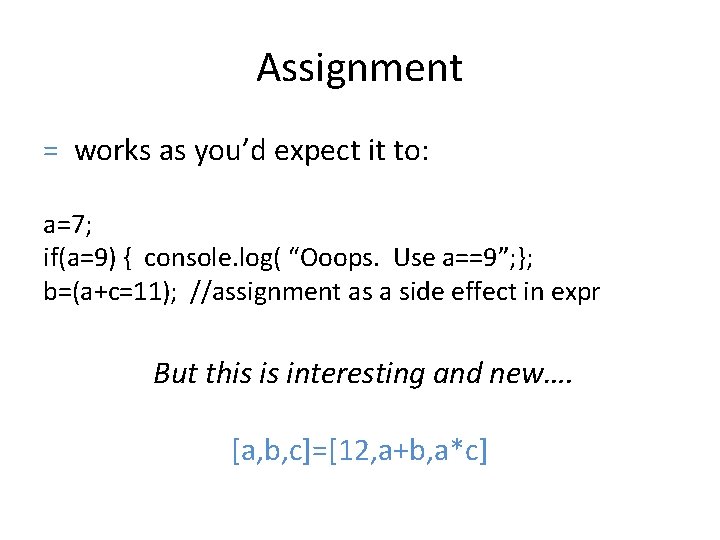
Assignment = works as you’d expect it to: a=7; if(a=9) { console. log( “Ooops. Use a==9”; }; b=(a+c=11); //assignment as a side effect in expr But this is interesting and new…. [a, b, c]=[12, a+b, a*c]
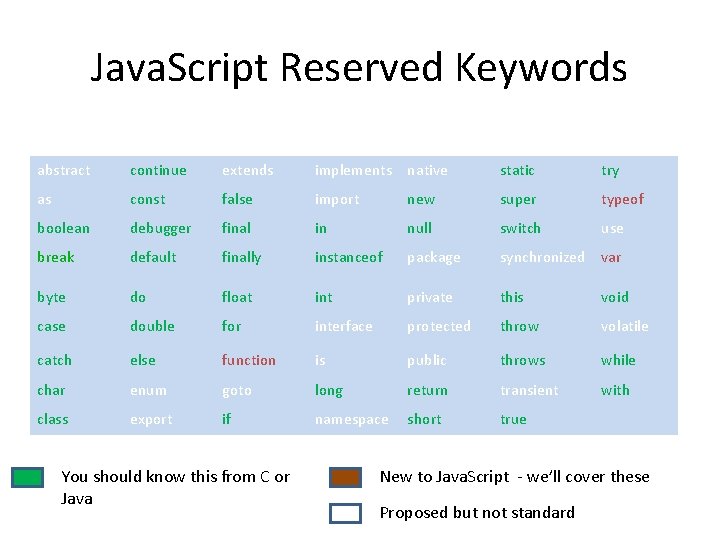
Java. Script Reserved Keywords abstract continue extends implements native static try as const false import new super typeof boolean debugger final in null switch use break default finally instanceof package synchronized var byte do float int private this void case double for interface protected throw volatile catch else function is public throws while char enum goto long return transient with class export if namespace short true You should know this from C or Java New to Java. Script - we’ll cover these Proposed but not standard
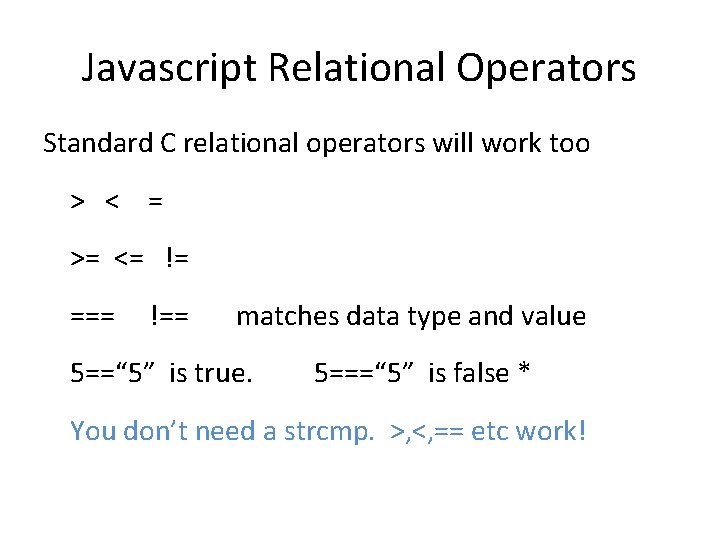
Javascript Relational Operators Standard C relational operators will work too > < = >= <= != === !== matches data type and value 5==“ 5” is true. 5===“ 5” is false * You don’t need a strcmp. >, <, == etc work!
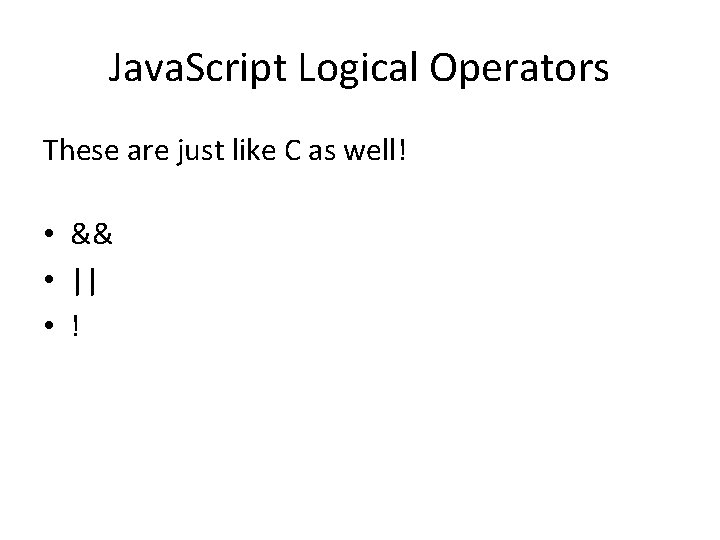
Java. Script Logical Operators These are just like C as well! • && • || • !
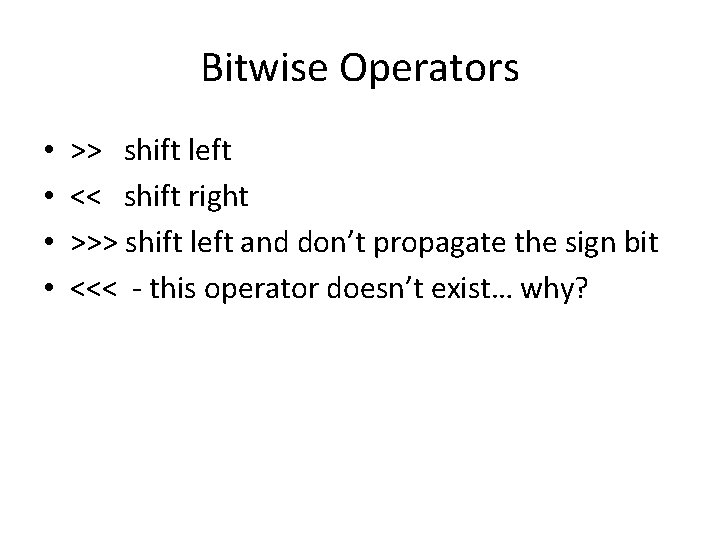
Bitwise Operators • • >> shift left << shift right >>> shift left and don’t propagate the sign bit <<< - this operator doesn’t exist… why?
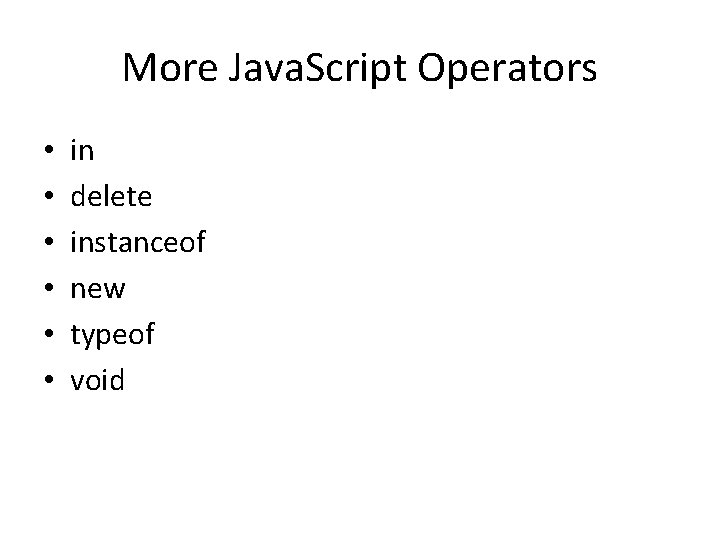
More Java. Script Operators • • • in delete instanceof new typeof void
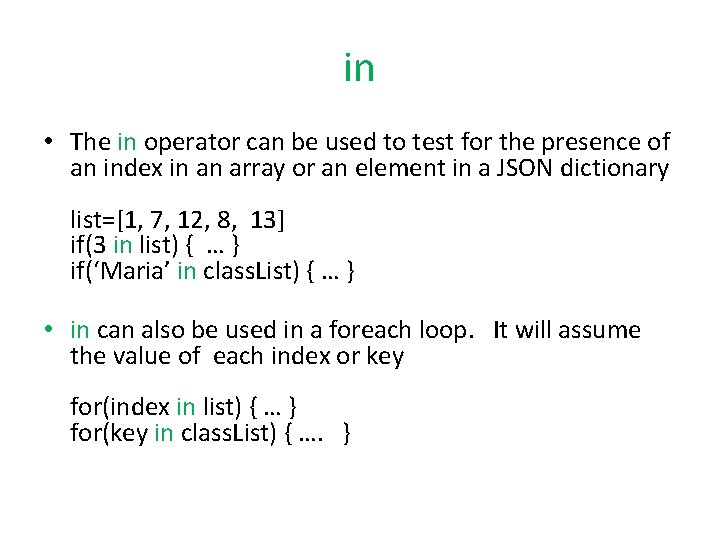
in • The in operator can be used to test for the presence of an index in an array or an element in a JSON dictionary list=[1, 7, 12, 8, 13] if(3 in list) { … } if(‘Maria’ in class. List) { … } • in can also be used in a foreach loop. It will assume the value of each index or key for(index in list) { … } for(key in class. List) { …. }
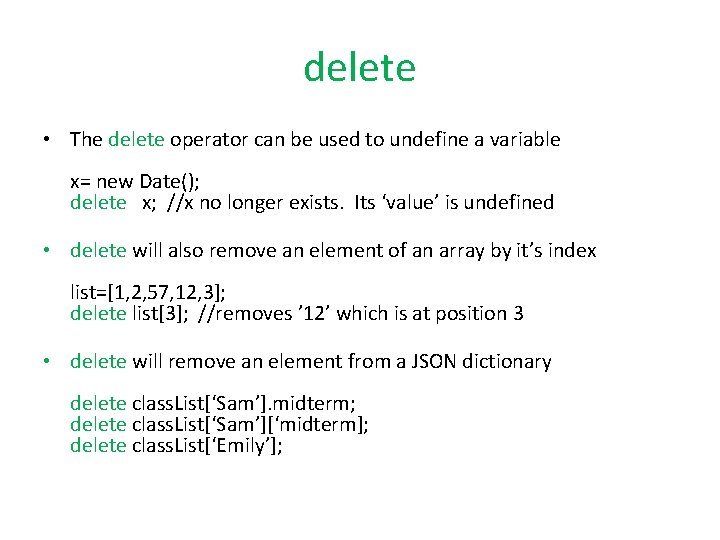
delete • The delete operator can be used to undefine a variable x= new Date(); delete x; //x no longer exists. Its ‘value’ is undefined • delete will also remove an element of an array by it’s index list=[1, 2, 57, 12, 3]; delete list[3]; //removes ’ 12’ which is at position 3 • delete will remove an element from a JSON dictionary delete class. List[‘Sam’]. midterm; delete class. List[‘Sam’][‘midterm]; delete class. List[‘Emily’];
![and are called the member of operators In Java Script [] . • [] and. are called the member of operators. In Java. Script](https://slidetodoc.com/presentation_image_h2/866320c96ebb535acbbc0ff8cb4a1755/image-31.jpg)
[] . • [] and. are called the member of operators. In Java. Script they do the same thing my. List[‘Sam’]= {midterm: 68, ‘final’: 79 } my. List. Raina={midterm: 68, ‘final’ : 82 } We tend not to think of [] and. As operators, but it is useful to consider them as such.
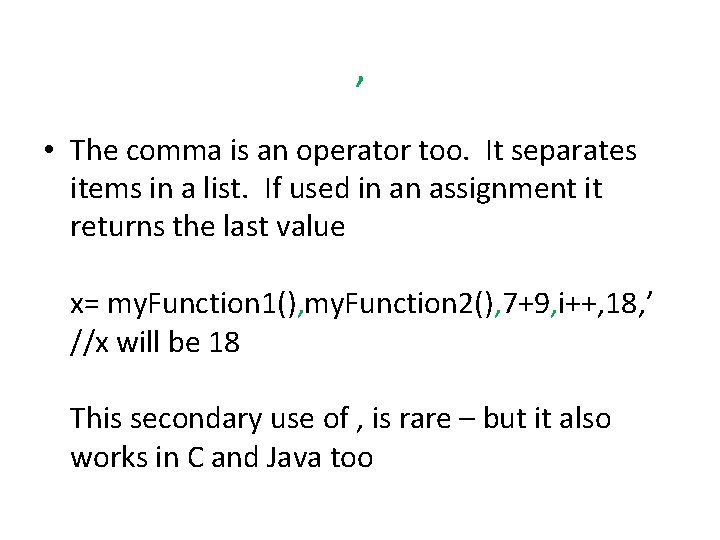
, • The comma is an operator too. It separates items in a list. If used in an assignment it returns the last value x= my. Function 1(), my. Function 2(), 7+9, i++, 18, ’ //x will be 18 This secondary use of , is rare – but it also works in C and Java too
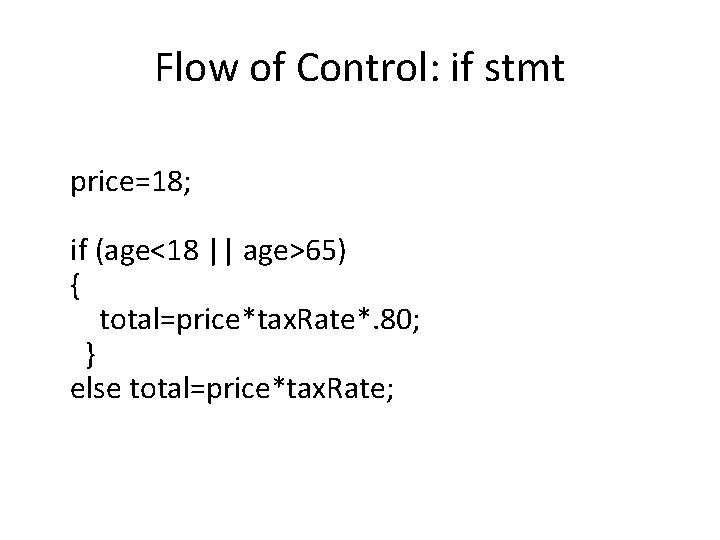
Flow of Control: if stmt price=18; if (age<18 || age>65) { total=price*tax. Rate*. 80; } else total=price*tax. Rate;
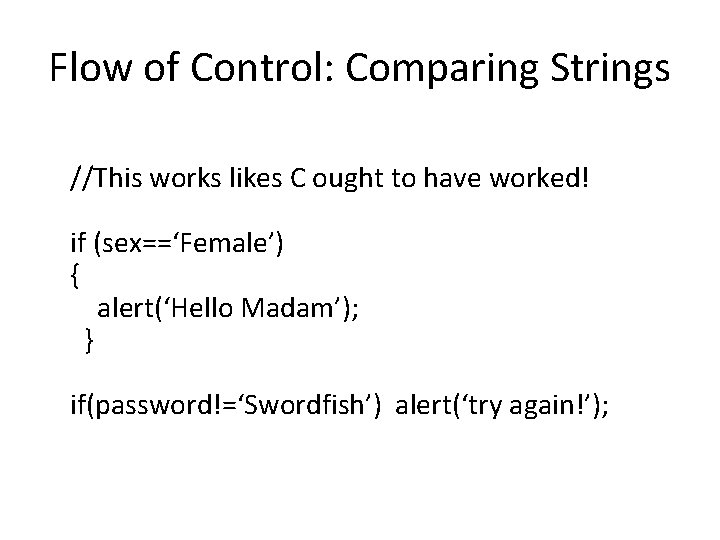
Flow of Control: Comparing Strings //This works likes C ought to have worked! if (sex==‘Female’) { alert(‘Hello Madam’); } if(password!=‘Swordfish’) alert(‘try again!’);
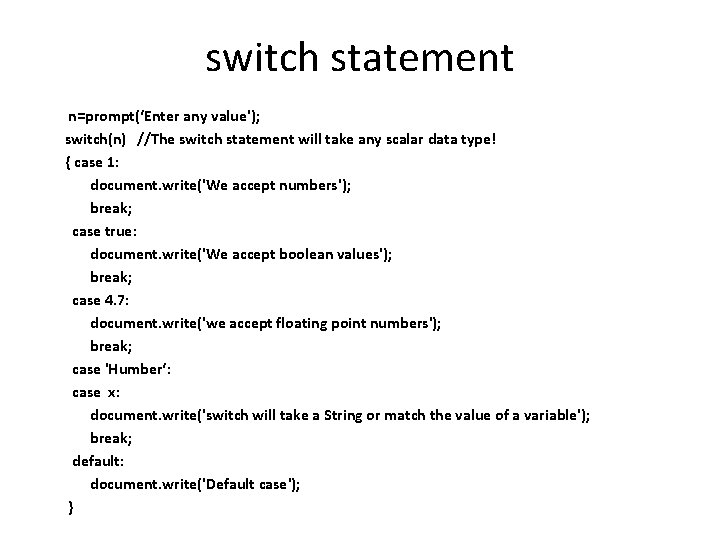
switch statement n=prompt(‘Enter any value'); switch(n) //The switch statement will take any scalar data type! { case 1: document. write('We accept numbers'); break; case true: document. write('We accept boolean values'); break; case 4. 7: document. write('we accept floating point numbers'); break; case 'Humber‘: case x: document. write('switch will take a String or match the value of a variable'); break; default: document. write('Default case'); }
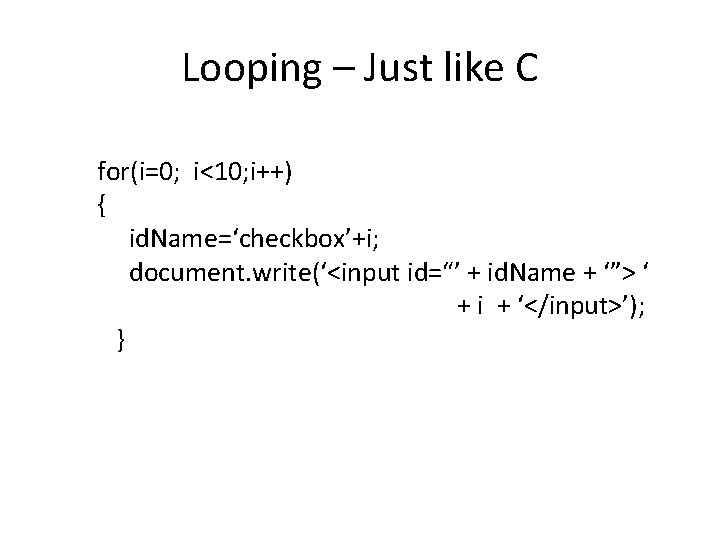
Looping – Just like C for(i=0; i<10; i++) { id. Name=‘checkbox’+i; document. write(‘<input id=“’ + id. Name + ‘”> ‘ + i + ‘</input>’); }
![A for each loop var studentsBob Mike Rinky fori in students alerti A “for each” loop var students=['Bob', 'Mike', 'Rinky']; for(i in students) { alert(i+ '](https://slidetodoc.com/presentation_image_h2/866320c96ebb535acbbc0ff8cb4a1755/image-37.jpg)
A “for each” loop var students=['Bob', 'Mike', 'Rinky']; for(i in students) { alert(i+ ' ' +students[i]); }
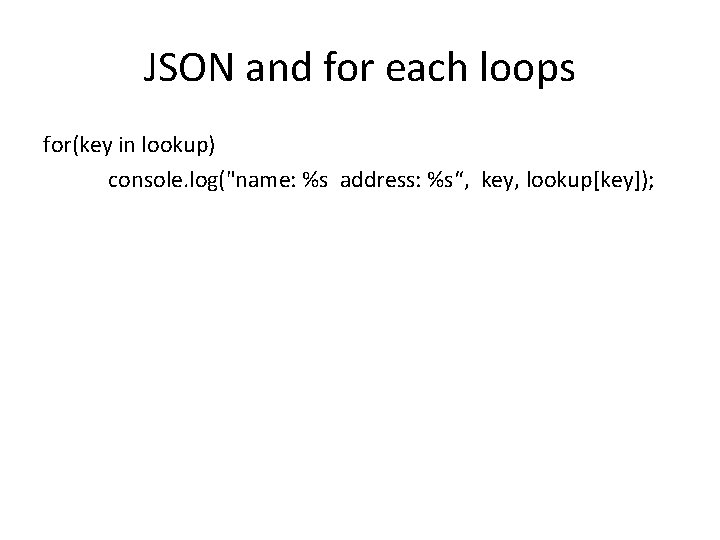
JSON and for each loops for(key in lookup) console. log("name: %s address: %s“, key, lookup[key]);
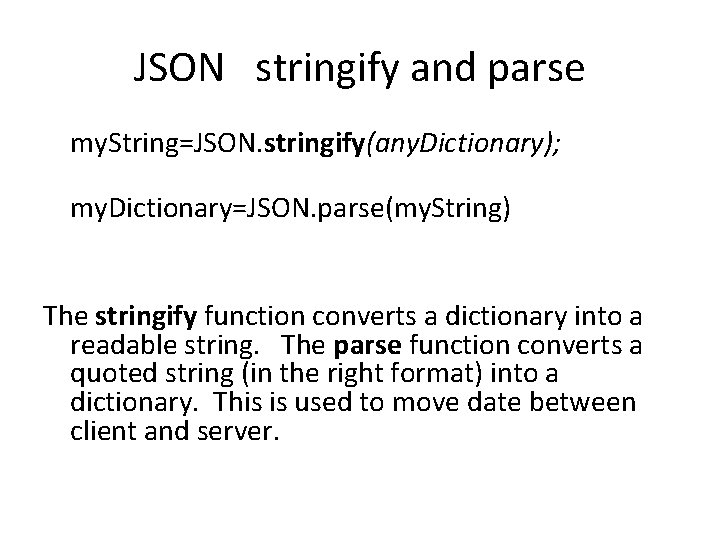
JSON stringify and parse my. String=JSON. stringify(any. Dictionary); my. Dictionary=JSON. parse(my. String) The stringify function converts a dictionary into a readable string. The parse function converts a quoted string (in the right format) into a dictionary. This is used to move date between client and server.
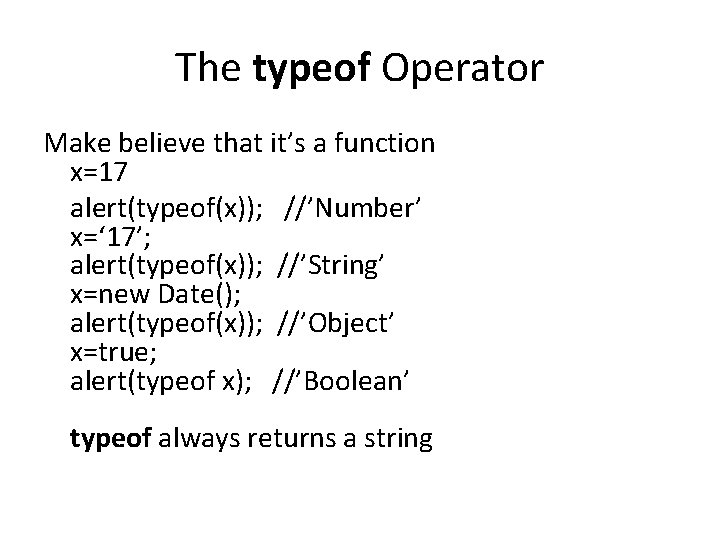
The typeof Operator Make believe that it’s a function x=17 alert(typeof(x)); //’Number’ x=‘ 17’; alert(typeof(x)); //’String’ x=new Date(); alert(typeof(x)); //’Object’ x=true; alert(typeof x); //’Boolean’ typeof always returns a string
![Associative Arrays var studentsBob Mike Rinky fori in students alerti studentsi Associative Arrays var students=['Bob', 'Mike', 'Rinky']; for(i in students) { alert(i+ ' ' +students[i]);](https://slidetodoc.com/presentation_image_h2/866320c96ebb535acbbc0ff8cb4a1755/image-41.jpg)
Associative Arrays var students=['Bob', 'Mike', 'Rinky']; for(i in students) { alert(i+ ' ' +students[i]); }
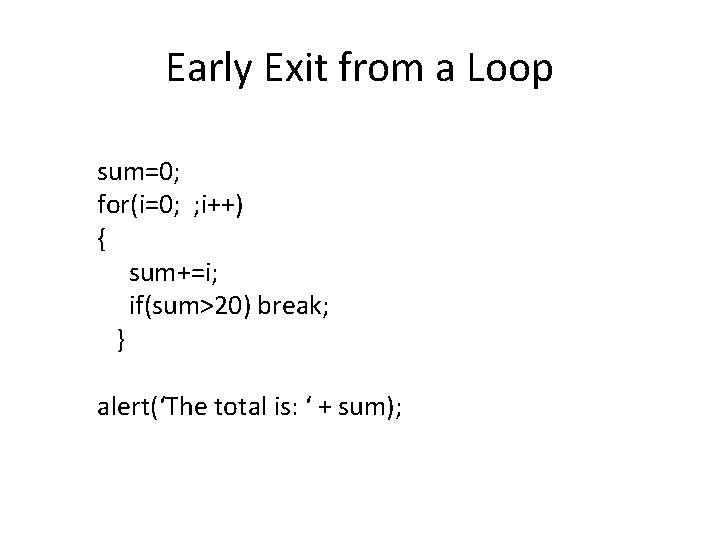
Early Exit from a Loop sum=0; for(i=0; ; i++) { sum+=i; if(sum>20) break; } alert(‘The total is: ‘ + sum);
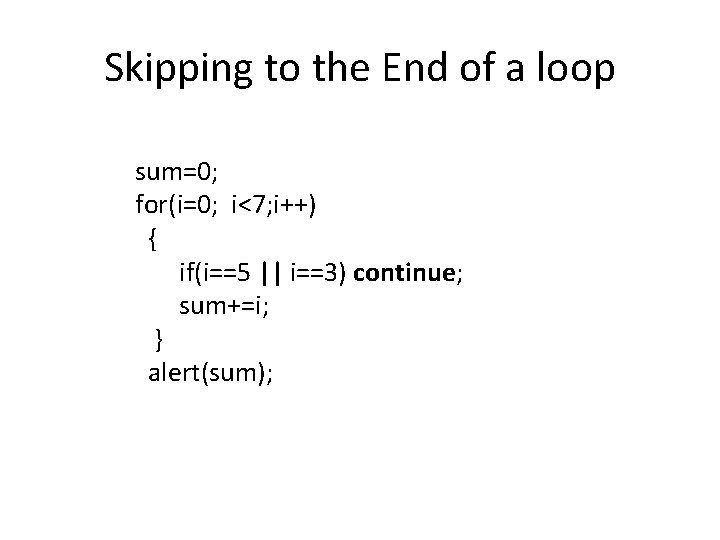
Skipping to the End of a loop sum=0; for(i=0; i<7; i++) { if(i==5 || i==3) continue; sum+=i; } alert(sum);
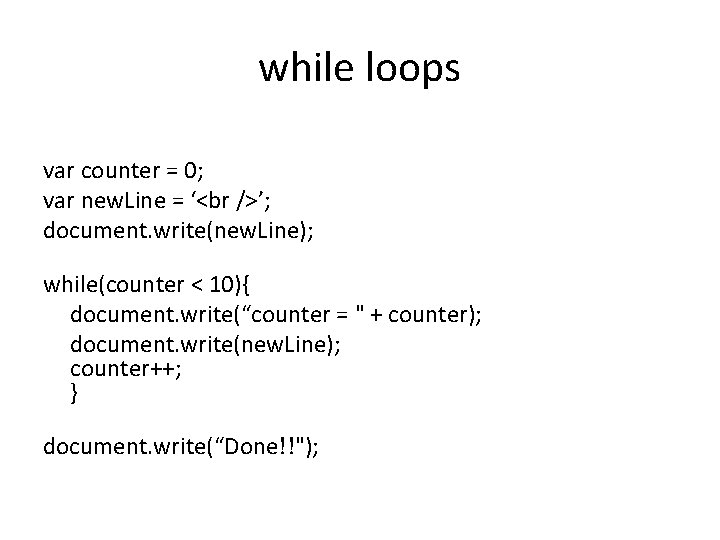
while loops var counter = 0; var new. Line = ‘ ’; document. write(new. Line); while(counter < 10){ document. write(“counter = " + counter); document. write(new. Line); counter++; } document. write(“Done!!");
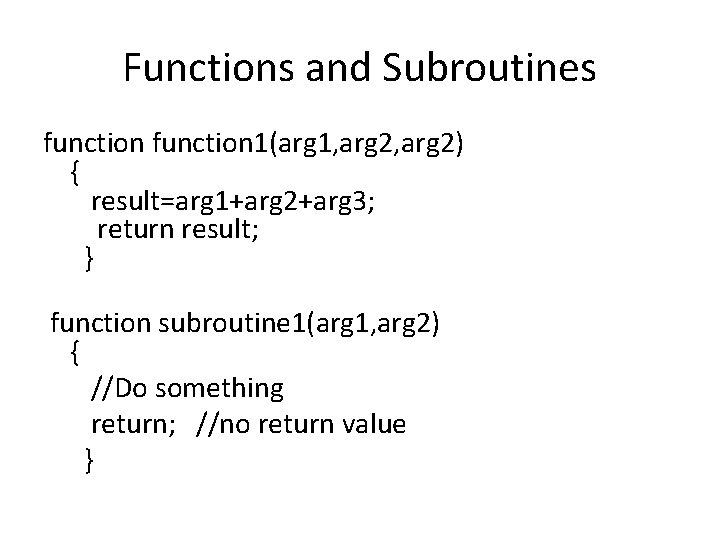
Functions and Subroutines function 1(arg 1, arg 2) { result=arg 1+arg 2+arg 3; return result; } function subroutine 1(arg 1, arg 2) { //Do something return; //no return value }
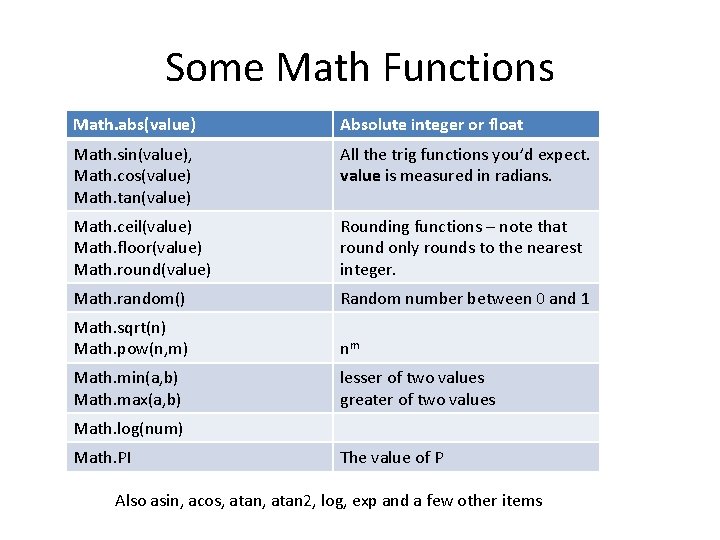
Some Math Functions Math. abs(value) Absolute integer or float Math. sin(value), Math. cos(value) Math. tan(value) All the trig functions you’d expect. value is measured in radians. Math. ceil(value) Math. floor(value) Math. round(value) Rounding functions – note that round only rounds to the nearest integer. Math. random() Random number between 0 and 1 Math. sqrt(n) Math. pow(n, m) nm Math. min(a, b) Math. max(a, b) lesser of two values greater of two values Math. log(num) Math. PI The value of P Also asin, acos, atan 2, log, exp and a few other items
![String Functions one two three split Creates the array one two three my String Functions “one, two, three”. split(‘, ’) Creates the array: [“one”, ”two”, ”three”] my.](https://slidetodoc.com/presentation_image_h2/866320c96ebb535acbbc0ff8cb4a1755/image-47.jpg)
String Functions “one, two, three”. split(‘, ’) Creates the array: [“one”, ”two”, ”three”] my. String. length of string x=my. String. to. Upper. Case() x=my. String. to. Lower. Case() x will be all upper case x will be all lower case “George”. substring(2, 4) Value is “org” - we start counting at 0 “Bananana”. index. Of(“nan”) “Bananana”. last. Index. Of(“nan”) returns location ? “George”. char. At(0) result is “G” x=escape(“<br/> This & That? ”)) Generates: %3 CBR/%3 E%20%26%20 This%20 %26%20 That%3 F%20 the inverse function y=unescape(string) These make a string portable to send over a network.
![Regular Expressions Regular expressions are directly part of the language reg Exprazgi Match all Regular Expressions Regular expressions are directly part of the language reg. Expr=/([a-z]+)/gi; //Match all](https://slidetodoc.com/presentation_image_h2/866320c96ebb535acbbc0ff8cb4a1755/image-48.jpg)
Regular Expressions Regular expressions are directly part of the language reg. Expr=/([a-z]+)/gi; //Match all runs of at least //1 alphabetic character “The rain, in Spain!”. match(reg. Expr) [“The”, “rain”, “Spain”] any. String. test(/regular expr/modifiers) Returns true or false any. String. search(/regular expr/modifiers) Returns the position of the 1 st match
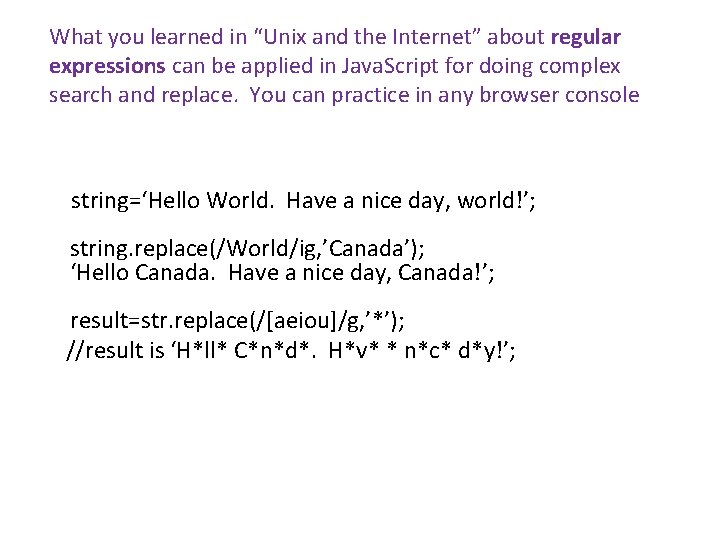
What you learned in “Unix and the Internet” about regular expressions can be applied in Java. Script for doing complex search and replace. You can practice in any browser console string=‘Hello World. Have a nice day, world!’; string. replace(/World/ig, ’Canada’); ‘Hello Canada. Have a nice day, Canada!’; result=str. replace(/[aeiou]/g, ’*’); //result is ‘H*ll* C*n*d*. H*v* * n*c* d*y!’;
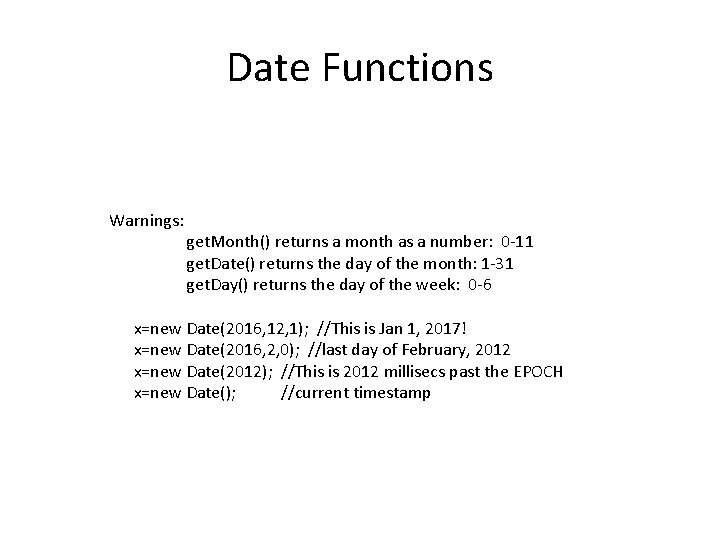
Date Functions Warnings: get. Month() returns a month as a number: 0 -11 get. Date() returns the day of the month: 1 -31 get. Day() returns the day of the week: 0 -6 x=new Date(2016, 12, 1); //This is Jan 1, 2017! x=new Date(2016, 2, 0); //last day of February, 2012 x=new Date(2012); //This is 2012 millisecs past the EPOCH x=new Date(); //current timestamp
![Array Functions one two three join Creates a string one two three its Array Functions [“one”, ”two”, ”three”]. join(“; ”) Creates a string: one; two; three (it’s](https://slidetodoc.com/presentation_image_h2/866320c96ebb535acbbc0ff8cb4a1755/image-51.jpg)
Array Functions [“one”, ”two”, ”three”]. join(“; ”) Creates a string: one; two; three (it’s the opposite of split) my. Array. length highest subscript in my. Array+1 my. Array. reverse() Reverses the order of elements in the array. my. Array. slice(2, 5) Creates a new array using elements 2 thru 5 of the old array my. Array. map(function) Creates a new array by applying the function to each of the elements of the array.
![The Array slice Function var fruits Banana Orange Apple Mango document writefruits slice0 The Array slice Function var fruits = ["Banana", "Orange", "Apple", "Mango"]; document. write(fruits. slice(0,](https://slidetodoc.com/presentation_image_h2/866320c96ebb535acbbc0ff8cb4a1755/image-52.jpg)
The Array slice Function var fruits = ["Banana", "Orange", "Apple", "Mango"]; document. write(fruits. slice(0, 1) + " "); document. write(fruits. slice(-2) + " "); document. write(fruits); //Output Banana Orange, Apple, Mango Banana, Orange, Apple, Mango Example taken from W 3 Schools. com
![The Array splice Function var fruits Banana Orange Apple Mango document writeRemoved The Array splice Function var fruits = ["Banana", "Orange", "Apple", "Mango"]; document. write("Removed: "](https://slidetodoc.com/presentation_image_h2/866320c96ebb535acbbc0ff8cb4a1755/image-53.jpg)
The Array splice Function var fruits = ["Banana", "Orange", "Apple", "Mango"]; document. write("Removed: " + fruits. splice(2, 1, "Lemon") + " "); document. write(fruits); The function returns items removed and modifies the original array. Example taken from W 3 Schools. com
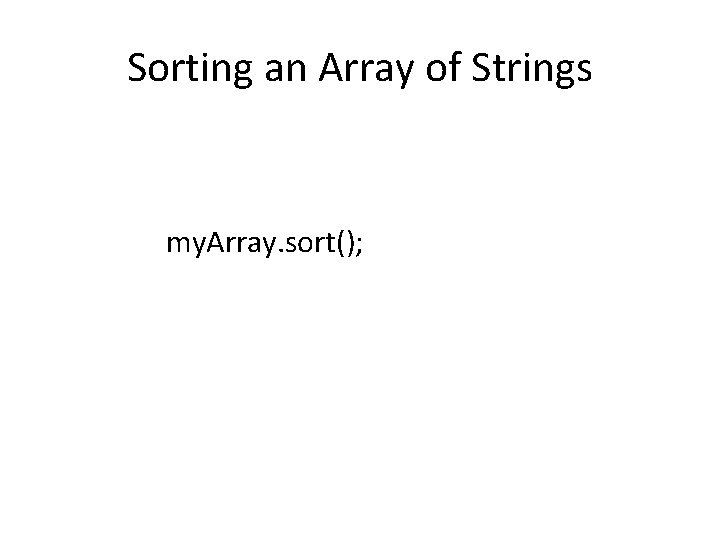
Sorting an Array of Strings my. Array. sort();
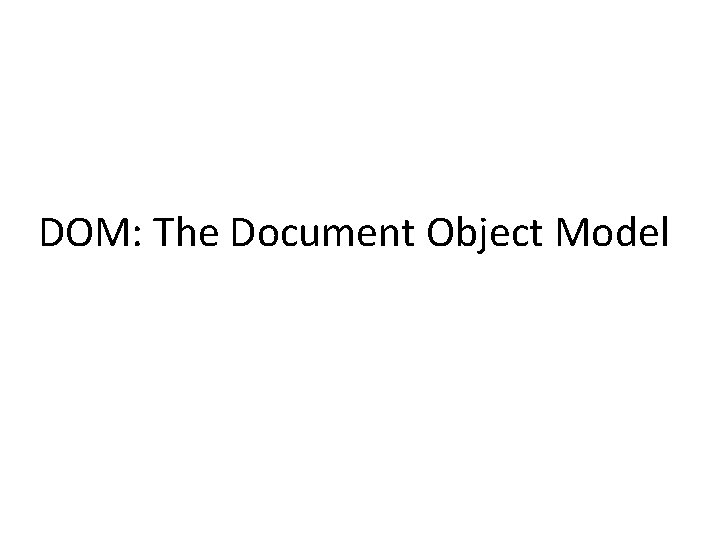
DOM: The Document Object Model
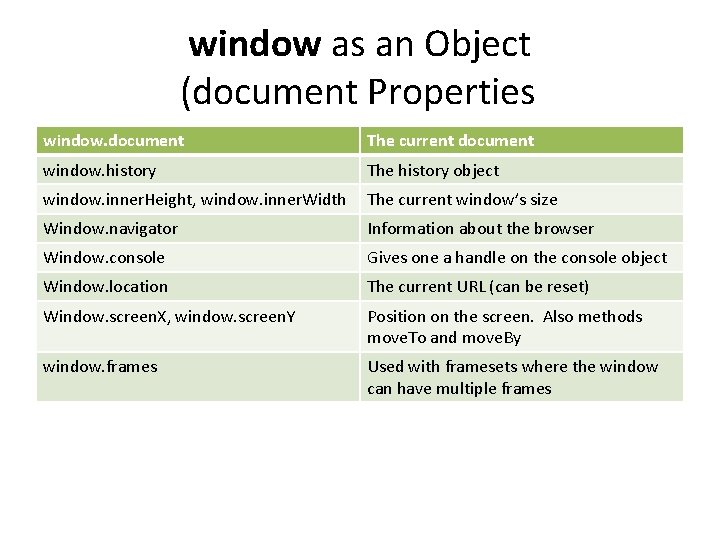
window as an Object (document Properties window. document The current document window. history The history object window. inner. Height, window. inner. Width The current window’s size Window. navigator Information about the browser Window. console Gives one a handle on the console object Window. location The current URL (can be reset) Window. screen. X, window. screen. Y Position on the screen. Also methods move. To and move. By window. frames Used with framesets where the window can have multiple frames
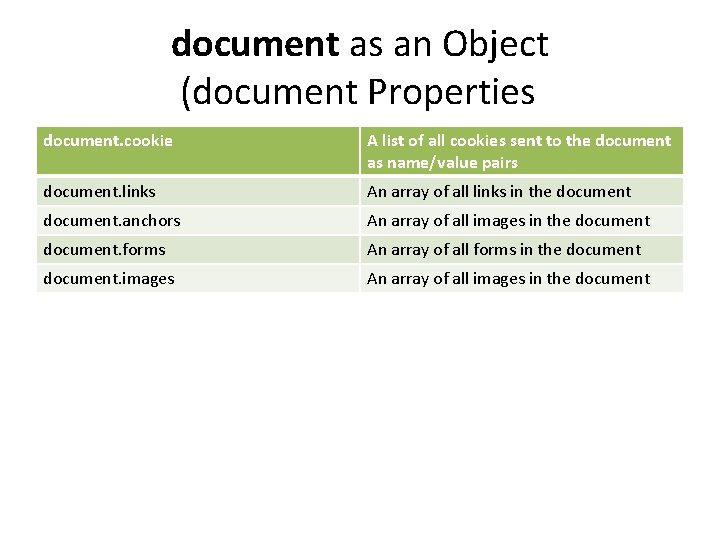
document as an Object (document Properties document. cookie A list of all cookies sent to the document as name/value pairs document. links An array of all links in the document. anchors An array of all images in the document. forms An array of all forms in the document. images An array of all images in the document
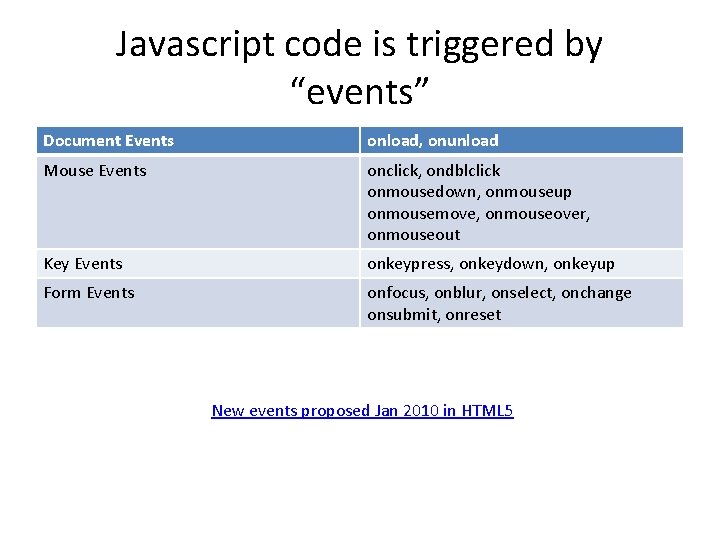
Javascript code is triggered by “events” Document Events onload, onunload Mouse Events onclick, ondblclick onmousedown, onmouseup onmousemove, onmouseover, onmouseout Key Events onkeypress, onkeydown, onkeyup Form Events onfocus, onblur, onselect, onchange onsubmit, onreset New events proposed Jan 2010 in HTML 5
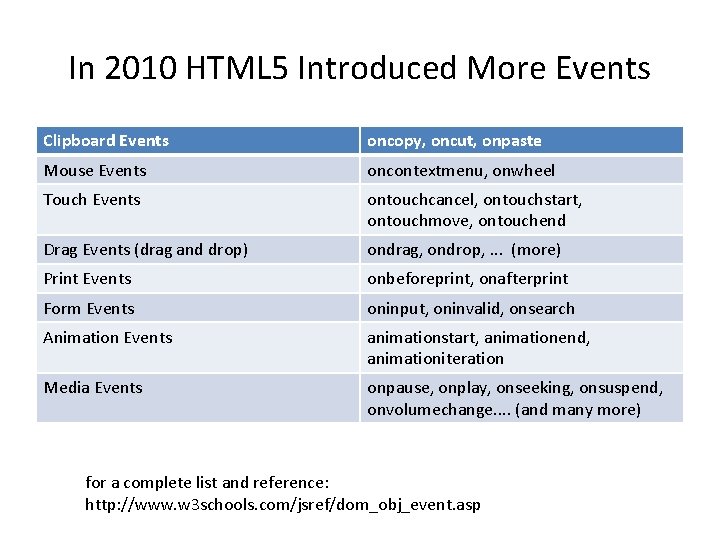
In 2010 HTML 5 Introduced More Events Clipboard Events oncopy, oncut, onpaste Mouse Events oncontextmenu, onwheel Touch Events ontouchcancel, ontouchstart, ontouchmove, ontouchend Drag Events (drag and drop) ondrag, ondrop, . . . (more) Print Events onbeforeprint, onafterprint Form Events oninput, oninvalid, onsearch Animation Events animationstart, animationend, animationiteration Media Events onpause, onplay, onseeking, onsuspend, onvolumechange. . (and many more) for a complete list and reference: http: //www. w 3 schools. com/jsref/dom_obj_event. asp
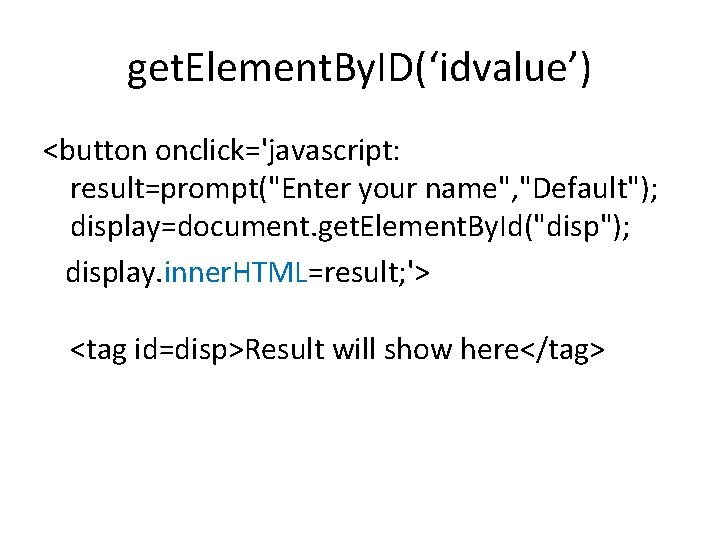
get. Element. By. ID(‘idvalue’) <button onclick='javascript: result=prompt("Enter your name", "Default"); display=document. get. Element. By. Id("disp"); display. inner. HTML=result; '> <tag id=disp>Result will show here</tag>
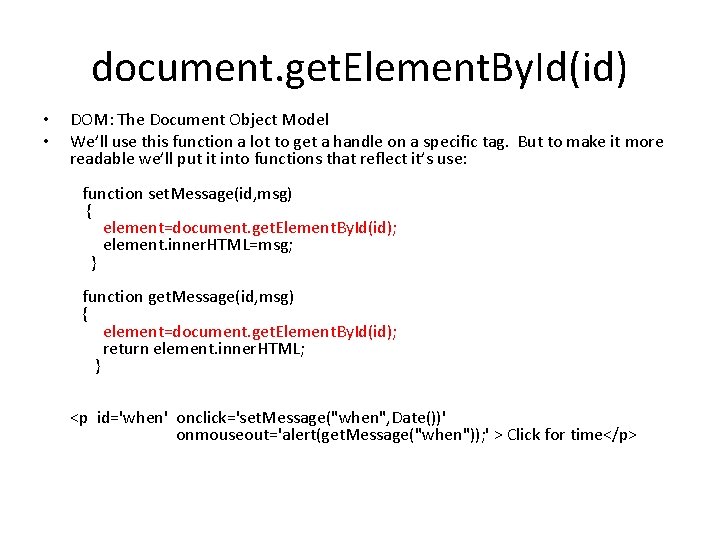
document. get. Element. By. Id(id) • • DOM: The Document Object Model We’ll use this function a lot to get a handle on a specific tag. But to make it more readable we’ll put it into functions that reflect it’s use: function set. Message(id, msg) { element=document. get. Element. By. Id(id); element. inner. HTML=msg; } function get. Message(id, msg) { element=document. get. Element. By. Id(id); return element. inner. HTML; } <p id='when' onclick='set. Message("when", Date())' onmouseout='alert(get. Message("when")); ' > Click for time</p>
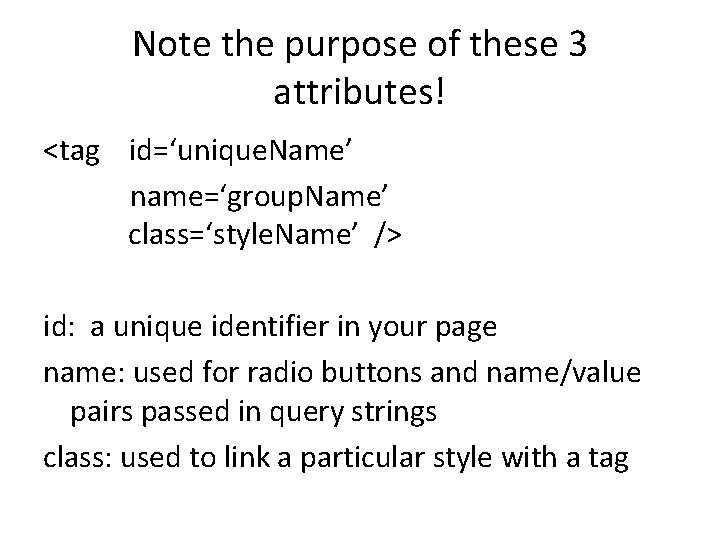
Note the purpose of these 3 attributes! <tag id=‘unique. Name’ name=‘group. Name’ class=‘style. Name’ /> id: a unique identifier in your page name: used for radio buttons and name/value pairs passed in query strings class: used to link a particular style with a tag
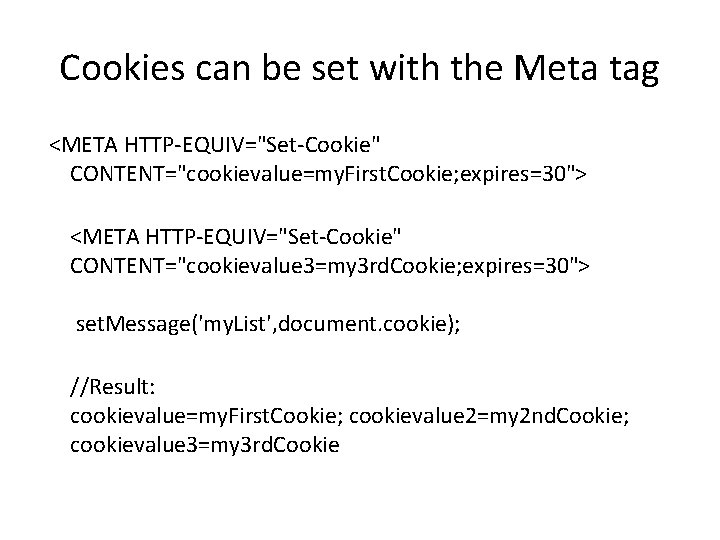
Cookies can be set with the Meta tag <META HTTP-EQUIV="Set-Cookie" CONTENT="cookievalue=my. First. Cookie; expires=30"> <META HTTP-EQUIV="Set-Cookie" CONTENT="cookievalue 3=my 3 rd. Cookie; expires=30"> set. Message('my. List', document. cookie); //Result: cookievalue=my. First. Cookie; cookievalue 2=my 2 nd. Cookie; cookievalue 3=my 3 rd. Cookie
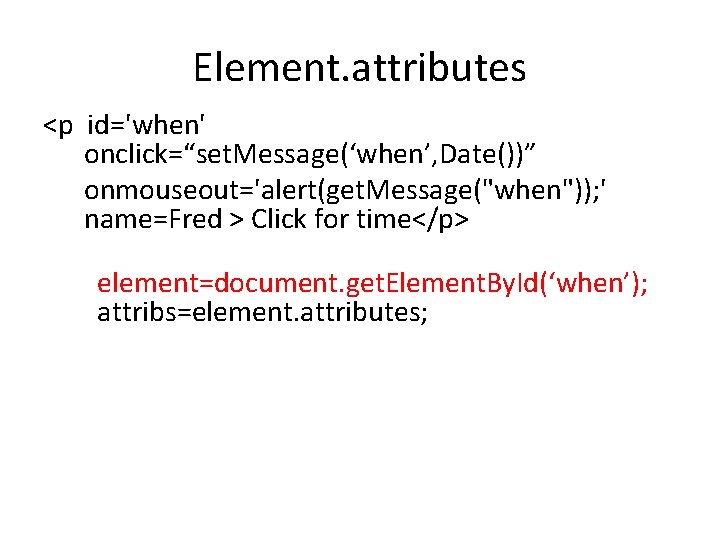
Element. attributes <p id='when' onclick=“set. Message(‘when’, Date())” onmouseout='alert(get. Message("when")); ' name=Fred > Click for time</p> element=document. get. Element. By. Id(‘when’); attribs=element. attributes;
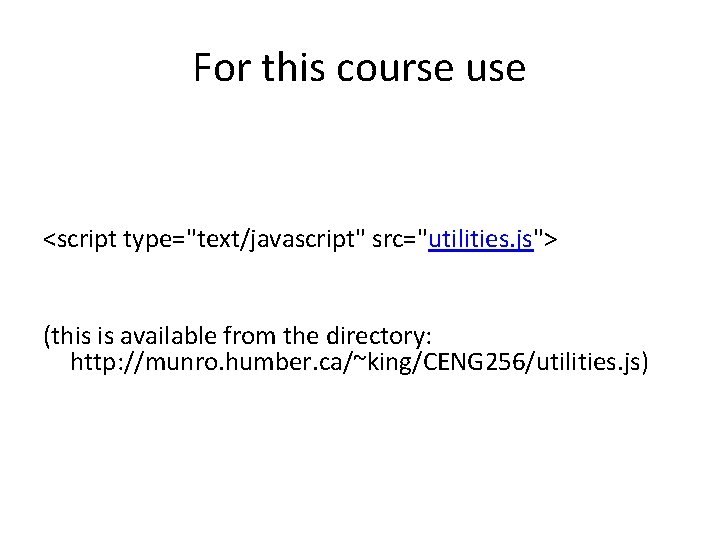
For this course use <script type="text/javascript" src="utilities. js"> (this is available from the directory: http: //munro. humber. ca/~king/CENG 256/utilities. js)
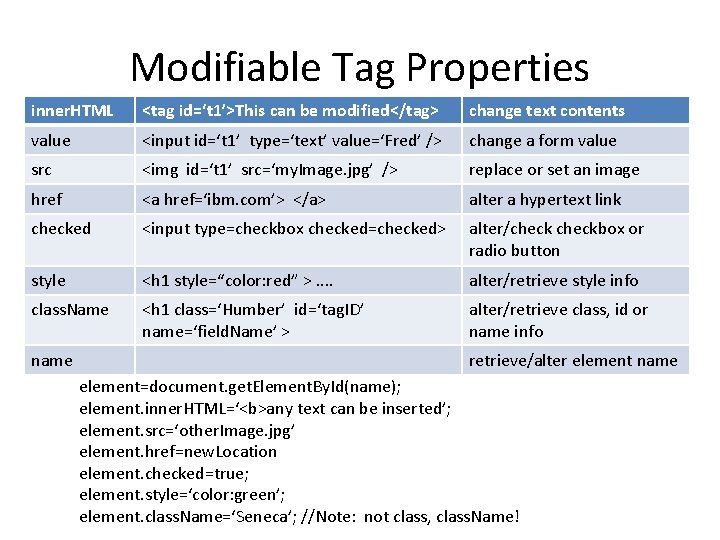
Modifiable Tag Properties inner. HTML <tag id=‘t 1’>This can be modified</tag> change text contents value <input id=‘t 1’ type=‘text’ value=‘Fred’ /> change a form value src <img id=‘t 1’ src=‘my. Image. jpg’ /> replace or set an image href <a href=‘ibm. com’> </a> alter a hypertext link checked <input type=checkbox checked=checked> alter/checkbox or radio button style <h 1 style=“color: red” >. . alter/retrieve style info class. Name <h 1 class=‘Humber’ id=‘tag. ID’ name=‘field. Name’ > alter/retrieve class, id or name info name retrieve/alter element name element=document. get. Element. By. Id(name); element. inner. HTML=‘<b>any text can be inserted’; element. src=‘other. Image. jpg’ element. href=new. Location element. checked=true; element. style=‘color: green’; element. class. Name=‘Seneca’; //Note: not class, class. Name!
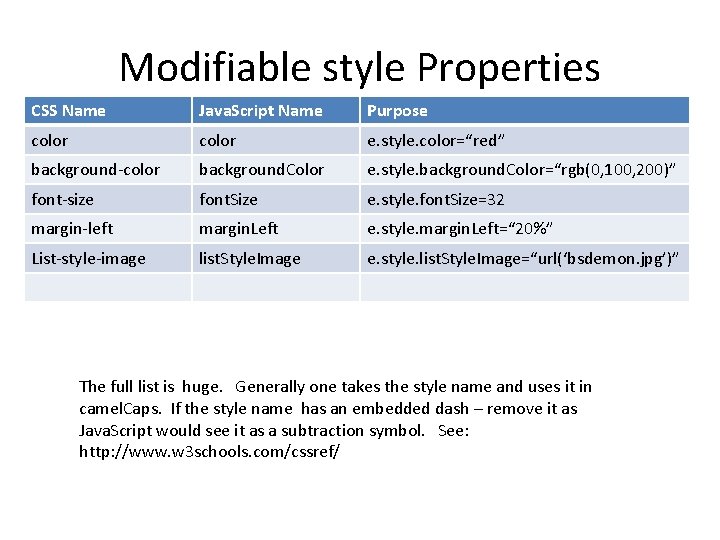
Modifiable style Properties CSS Name Java. Script Name Purpose color e. style. color=“red” background-color background. Color e. style. background. Color=“rgb(0, 100, 200)” font-size font. Size e. style. font. Size=32 margin-left margin. Left e. style. margin. Left=“ 20%” List-style-image list. Style. Image e. style. list. Style. Image=“url(‘bsdemon. jpg’)” The full list is huge. Generally one takes the style name and uses it in camel. Caps. If the style name has an embedded dash – remove it as Java. Script would see it as a subtraction symbol. See: http: //www. w 3 schools. com/cssref/
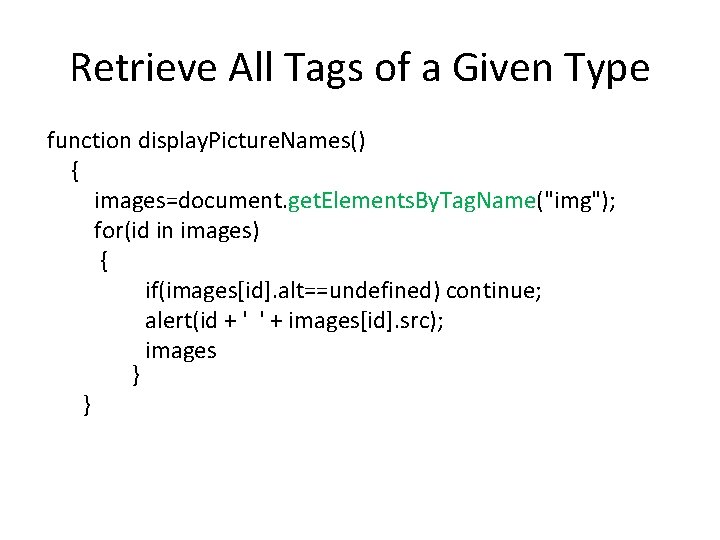
Retrieve All Tags of a Given Type function display. Picture. Names() { images=document. get. Elements. By. Tag. Name("img"); for(id in images) { if(images[id]. alt==undefined) continue; alert(id + ' ' + images[id]. src); images } }
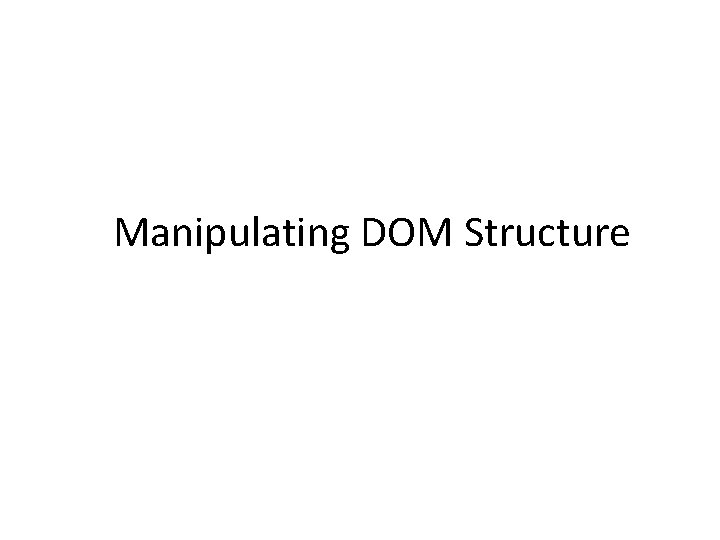
Manipulating DOM Structure
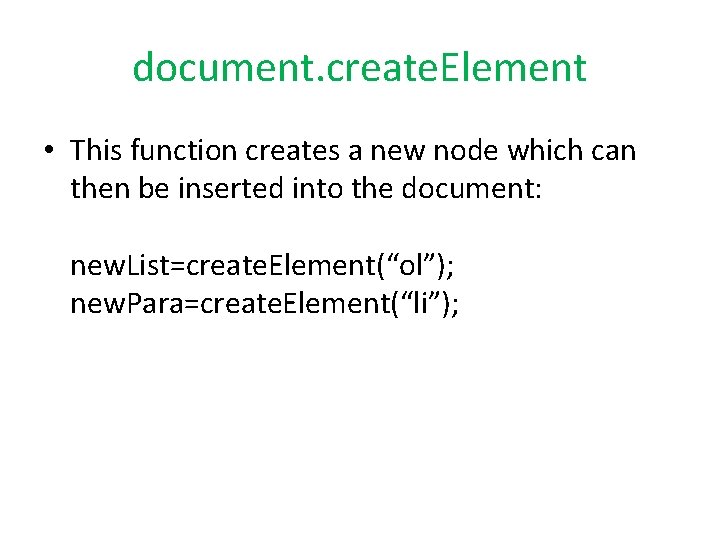
document. create. Element • This function creates a new node which can then be inserted into the document: new. List=create. Element(“ol”); new. Para=create. Element(“li”);
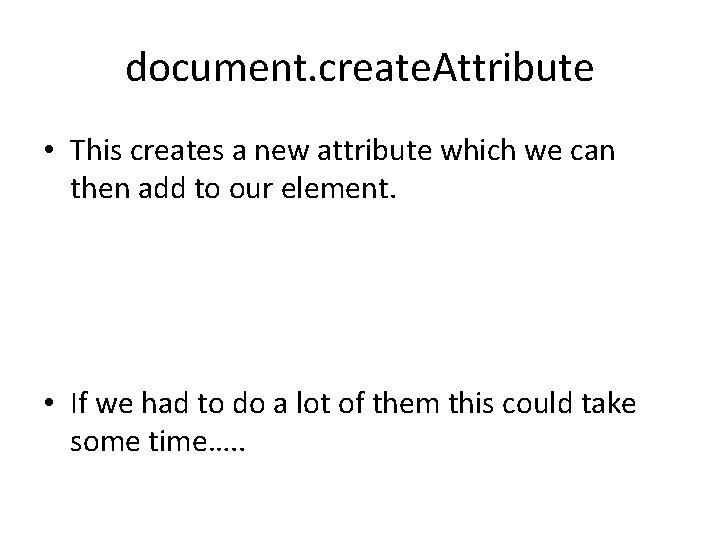
document. create. Attribute • This creates a new attribute which we can then add to our element. • If we had to do a lot of them this could take some time…. .
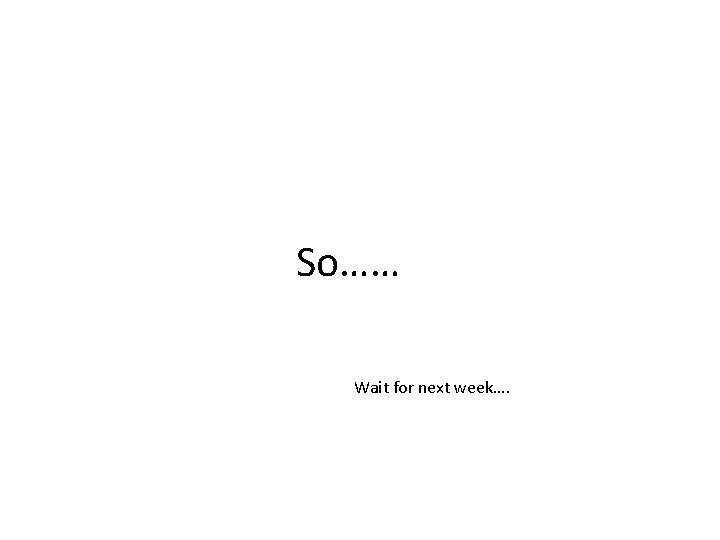
So…… Wait for next week….
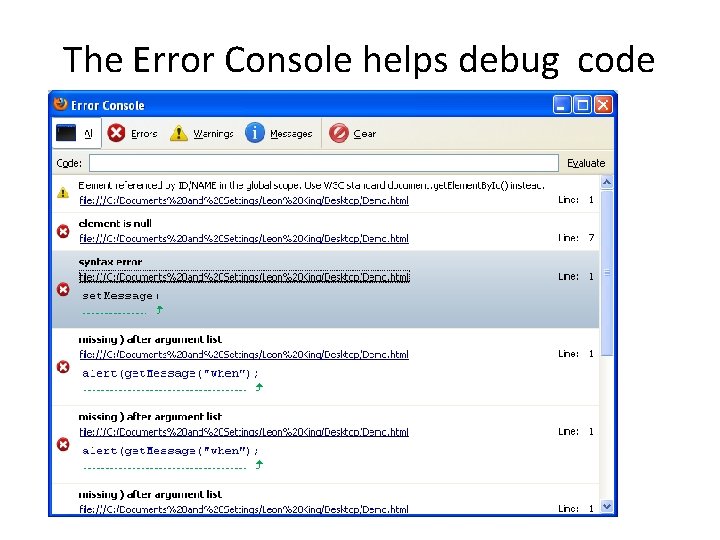
The Error Console helps debug code
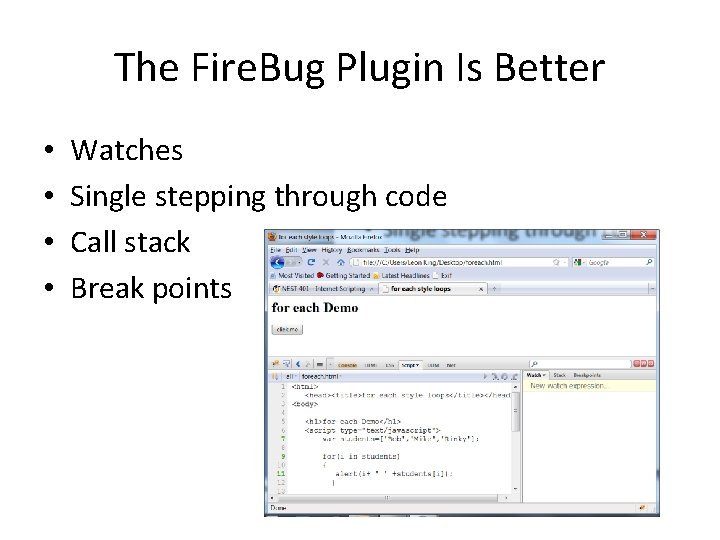
The Fire. Bug Plugin Is Better • • Watches Single stepping through code Call stack Break points
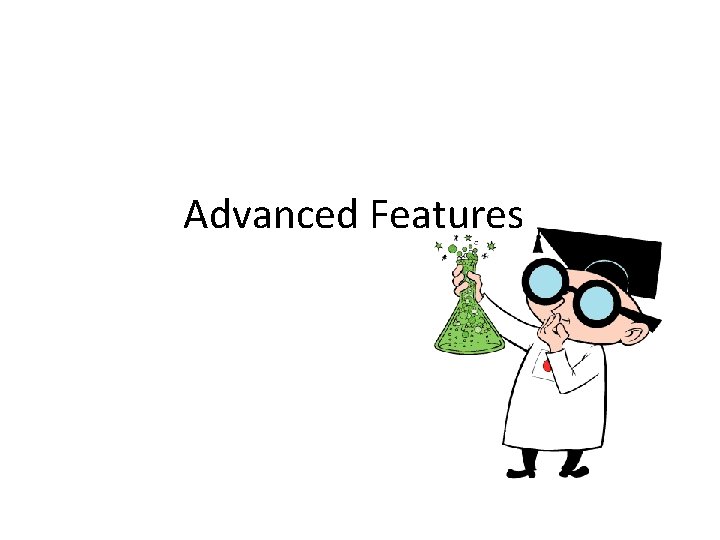
Advanced Features
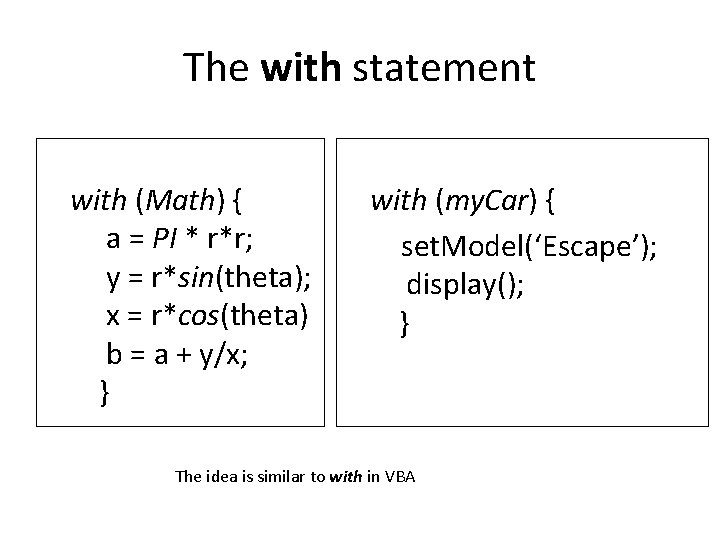
The with statement with (Math) { a = PI * r*r; y = r*sin(theta); x = r*cos(theta) b = a + y/x; } with (my. Car) { set. Model(‘Escape’); display(); } The idea is similar to with in VBA
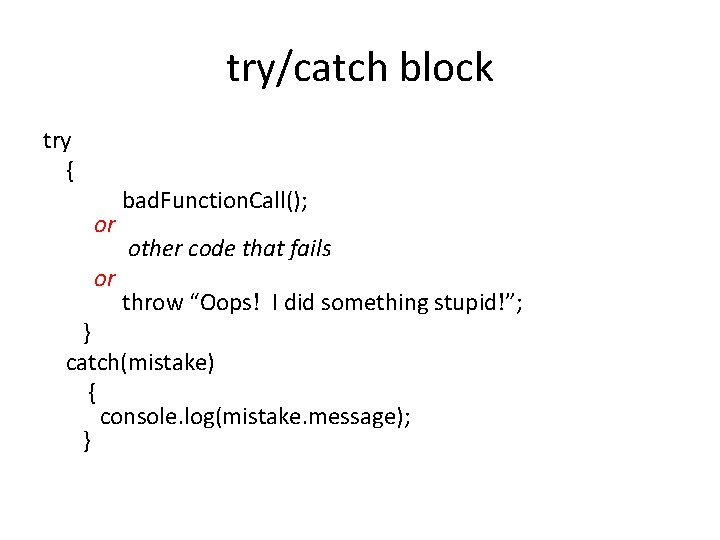
try/catch block try { or or bad. Function. Call(); other code that fails throw “Oops! I did something stupid!”; } catch(mistake) { console. log(mistake. message); }
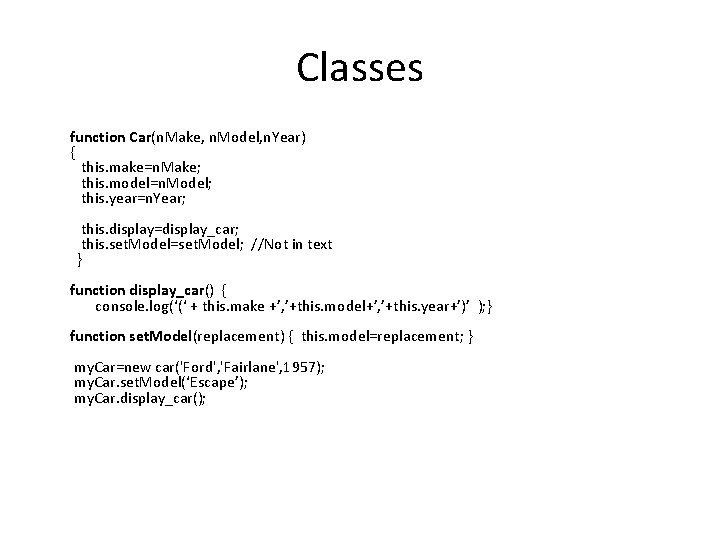
Classes function Car(n. Make, n. Model, n. Year) { this. make=n. Make; this. model=n. Model; this. year=n. Year; this. display=display_car; this. set. Model=set. Model; //Not in text } function display_car() { console. log(‘(‘ + this. make +’, ’+this. model+’, ’+this. year+’)’ ); } function set. Model(replacement) { this. model=replacement; } my. Car=new car('Ford', 'Fairlane', 1957); my. Car. set. Model(‘Escape’); my. Car. display_car();
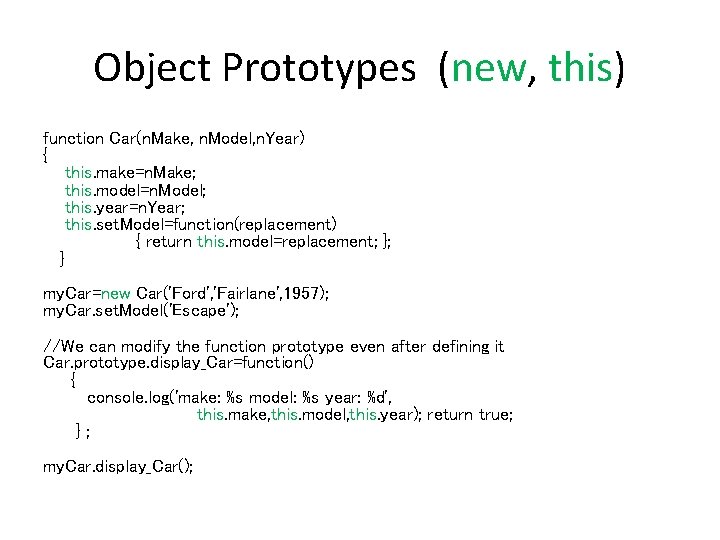
Object Prototypes (new, this) function Car(n. Make, n. Model, n. Year) { this. make=n. Make; this. model=n. Model; this. year=n. Year; this. set. Model=function(replacement) { return this. model=replacement; }; } my. Car=new Car('Ford', 'Fairlane', 1957); my. Car. set. Model('Escape'); //We can modify the function prototype even after defining it Car. prototype. display_Car=function() { console. log('make: %s model: %s year: %d', this. make, this. model, this. year); return true; }; my. Car. display_Car();
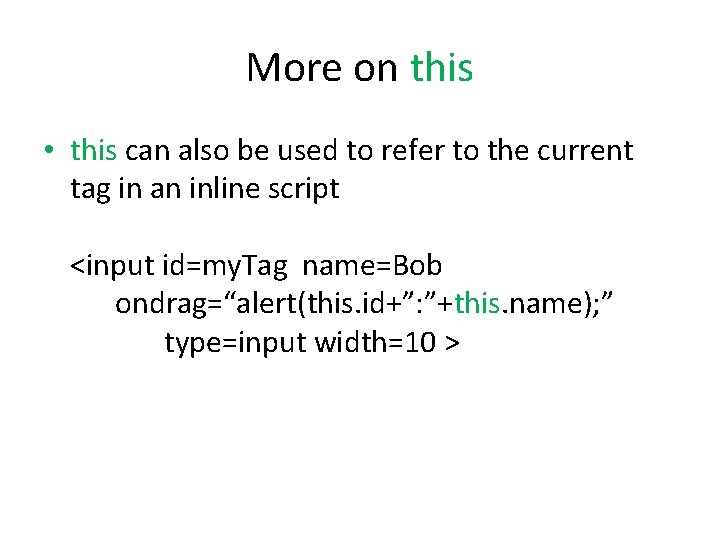
More on this • this can also be used to refer to the current tag in an inline script <input id=my. Tag name=Bob ondrag=“alert(this. id+”: ”+this. name); ” type=input width=10 >
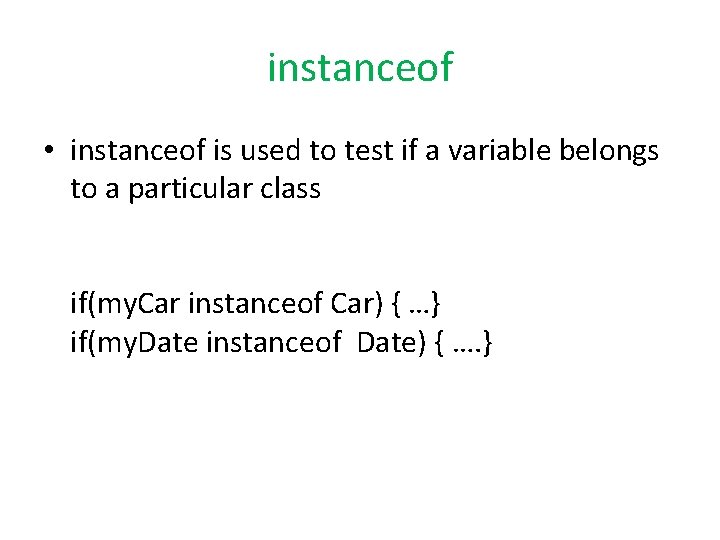
instanceof • instanceof is used to test if a variable belongs to a particular class if(my. Car instanceof Car) { …} if(my. Date instanceof Date) { …. }
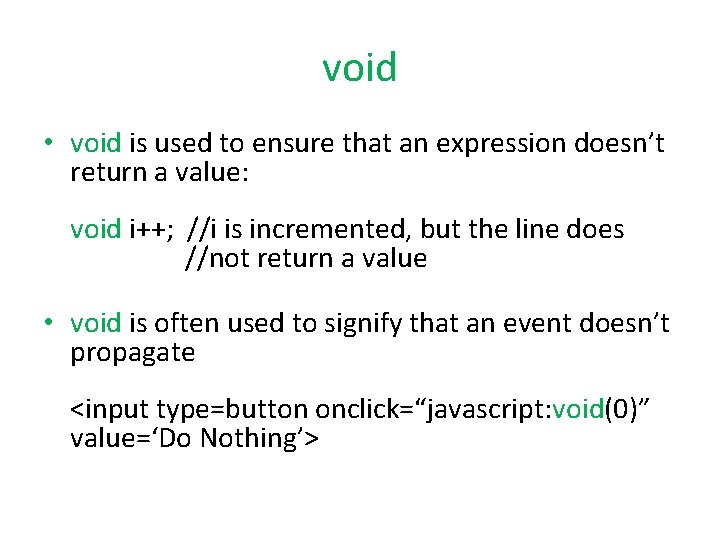
void • void is used to ensure that an expression doesn’t return a value: void i++; //i is incremented, but the line does //not return a value • void is often used to signify that an event doesn’t propagate <input type=button onclick=“javascript: void(0)” value=‘Do Nothing’>
![Closures map list1 2 3 4 console loglist Array 1 2 3 4 Closures: map list=[1, 2, 3, 4]; console. log(list); Array [ 1, 2, 3, 4](https://slidetodoc.com/presentation_image_h2/866320c96ebb535acbbc0ff8cb4a1755/image-83.jpg)
Closures: map list=[1, 2, 3, 4]; console. log(list); Array [ 1, 2, 3, 4 ] console. log(my. Closure(17)); 289 console. log(list. map(Math. sqrt)); Array [ 1, 1. 4142135623730951, 1. 7320508075688772, 2 ] console. log(list. map(my. Closure)); Array [ 1, 4, 9, 16 ]
![Closures reduce list1 2 57 3 4 Return the odd numbers only odd Numslist Closures: reduce list=[1, 2, 57, 3, 4] //Return the odd numbers only odd. Nums=list.](https://slidetodoc.com/presentation_image_h2/866320c96ebb535acbbc0ff8cb4a1755/image-84.jpg)
Closures: reduce list=[1, 2, 57, 3, 4] //Return the odd numbers only odd. Nums=list. reduce(function(prev, curr) { if(curr%2==1) return prev. concat(curr); else return prev; }, []); console. log(odd. Nums); //Find the maximum value of a list maxvalue=list. reduce(function(prev, curr) { if(prev == undefined) return curr; if(prev > curr) return prev; return curr; }); console. log("Maximum value is: ", maxvalue);
Gods ways are not our ways
Computers manipulate data in many ways
Classify each quadrilateral in as many ways as possible
Final expenditure approach
Many ways to measure
When permutation and when combination
Four ways in which ones
In many different ways
Phrases for introducing quotes
A concise introduction to linguistics answer key
Introducing james joyce
Complex sentence starters
Phrases to introduce the counterclaim
Introduce yourself sample
Talk boost tracker
Introducing family members in french
Ottava rima example
Introduction to digestive system
Introducing
Opposite numbers definition
Introducing internet
Explanation sentence starters
New market offerings
Templates for introducing quotations
Define upgrade advisor
Introducing the metric system
Short bio of yourself
Relocation diffusion definition
Blood relation definition
An introduction to the odyssey
Email to an exchange student
Introducing broker vs carrying broker
Int family
Safe relentless improvement
Define politics
Introducing flex pods
Expression greeting and response
Introducing neeta anil said
An introduction to rhetoric using the available means
Templates for introducing quotations
Templates for introducing quotations
Introducing the metric system
Introduce yourself
Introducing a quote
Psychology a journey
Introducing illustrator 2013
An organism develops active immunity as a result of
Introducing and naming new products and brand extensions
Introducing and naming new products and brand extensions
Ariel trust
Script de java
Java scrept
Java script wikipedia
Language
"java script"
"java script"
Java script course
Java script
"java script"
Khan academy object oriented programming
Java script examples
Inside which element do you put javascript
Java script email
Slido softuni
"language fundamentals"
"language fundamentals"
Java script classes
Many buyers and sellers
Er diagram in dbms
Convert conceptual model to logical model
Erd vs erm
Unary many to many
Contoh erd one to many
Unary many to many
Many-to-many communication
Sqlbi many to many
Unary relationship database
Many sellers and many buyers
Java swing form example
Import java.util.*
Import java swing
Import scanner java
Import java.io.*
Import java.util.*
Java random