Inheritance Review CSC 171 FALL 2004 LECTURE 19
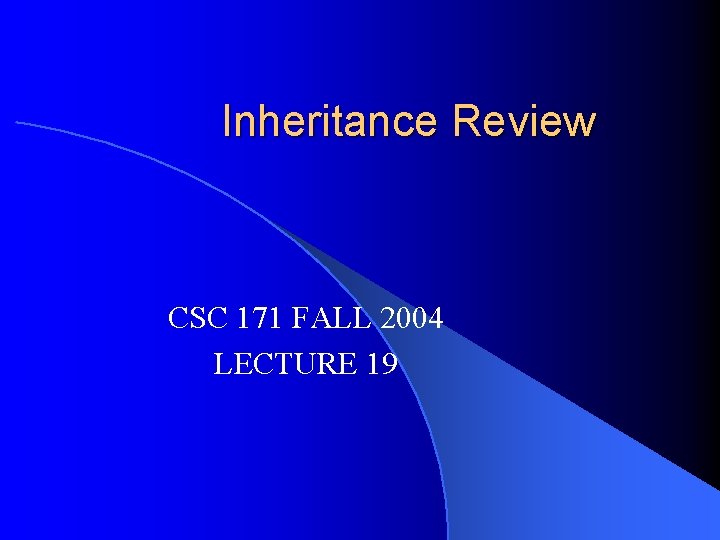
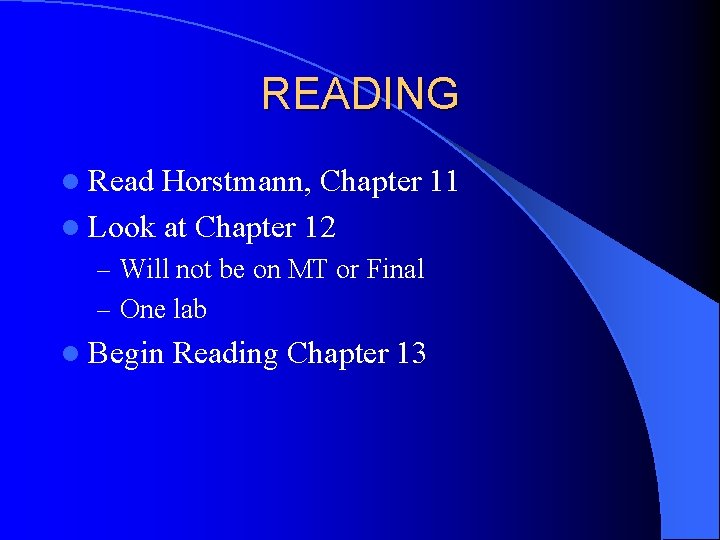
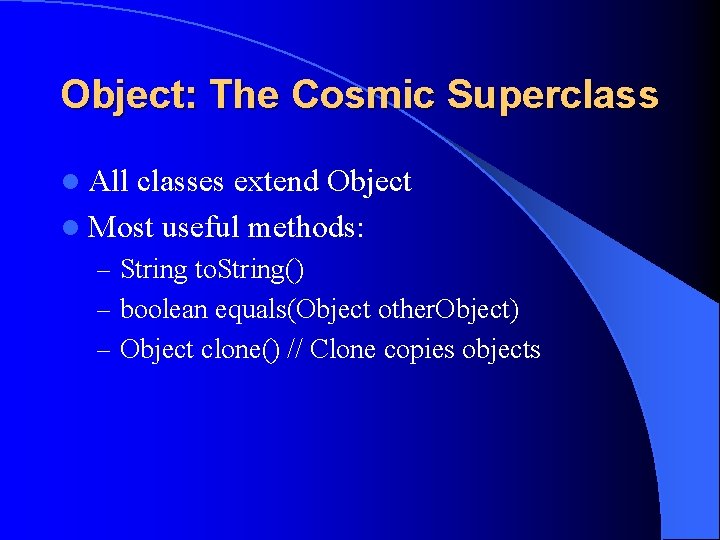
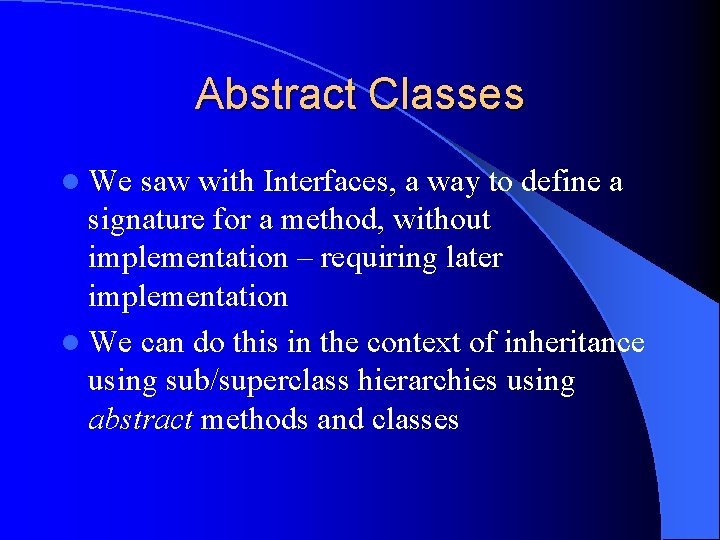
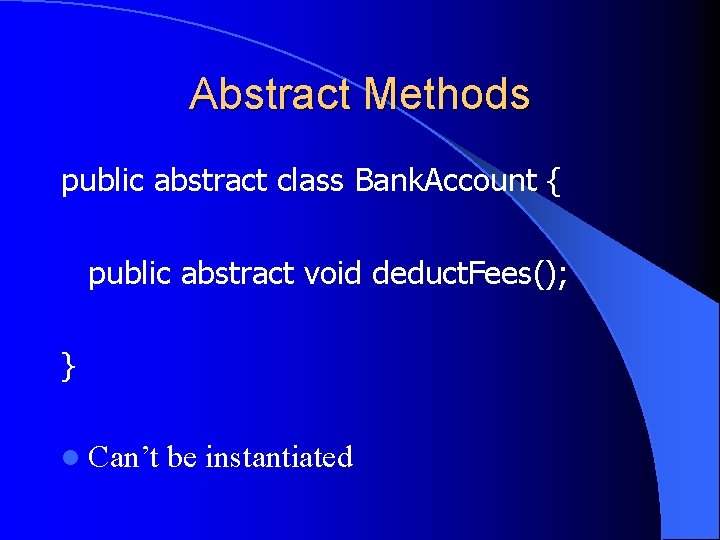
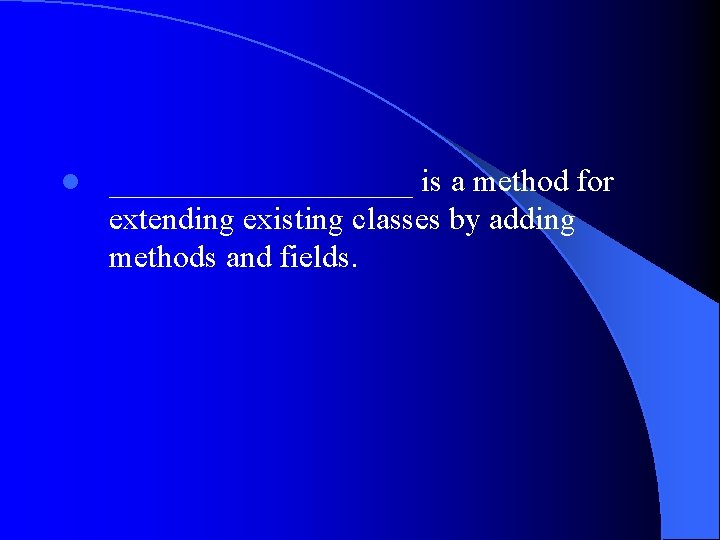
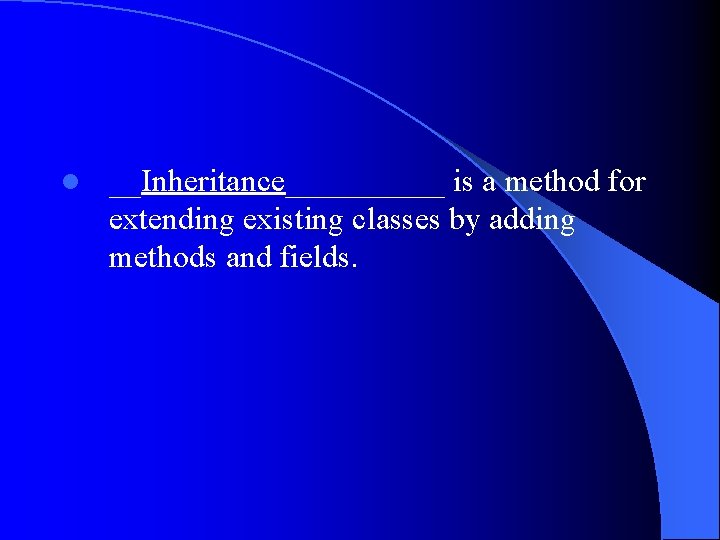
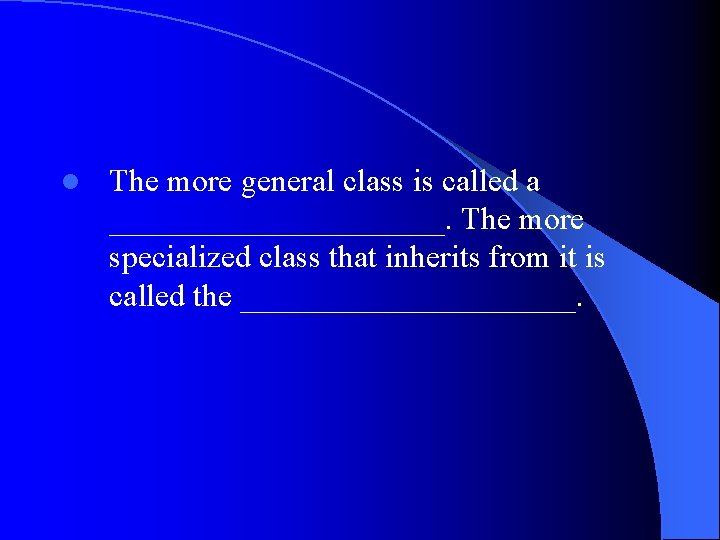
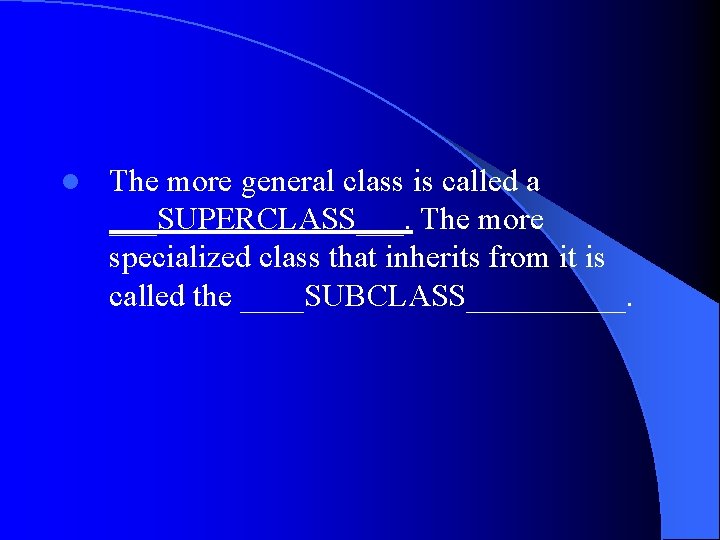
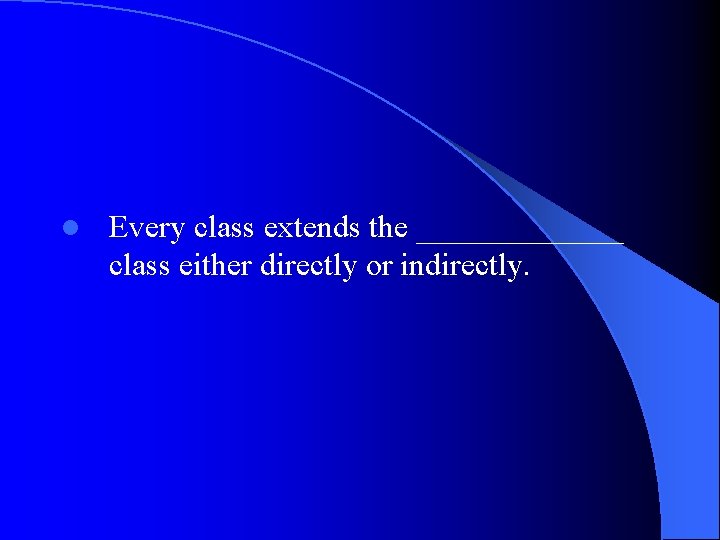
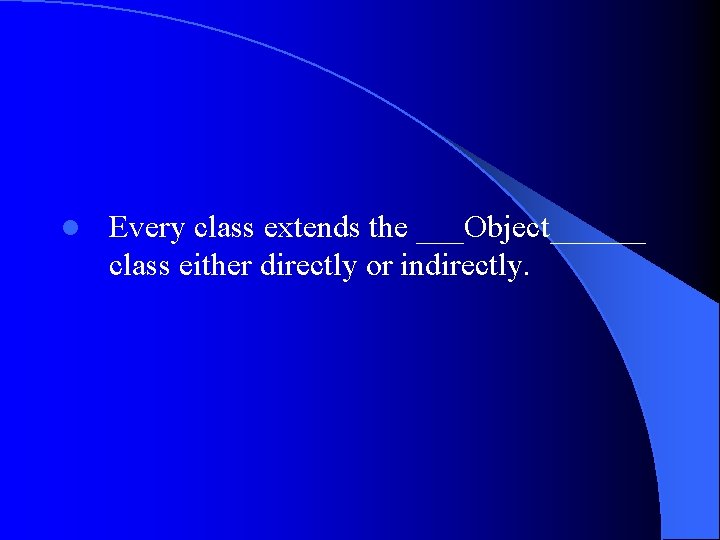
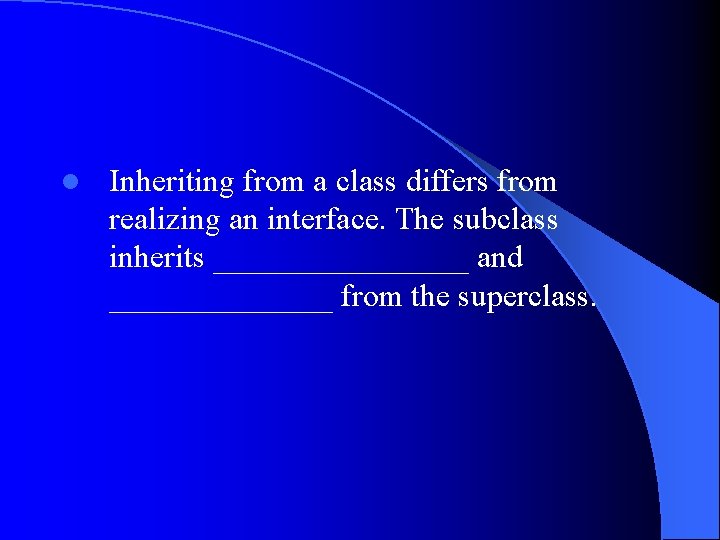
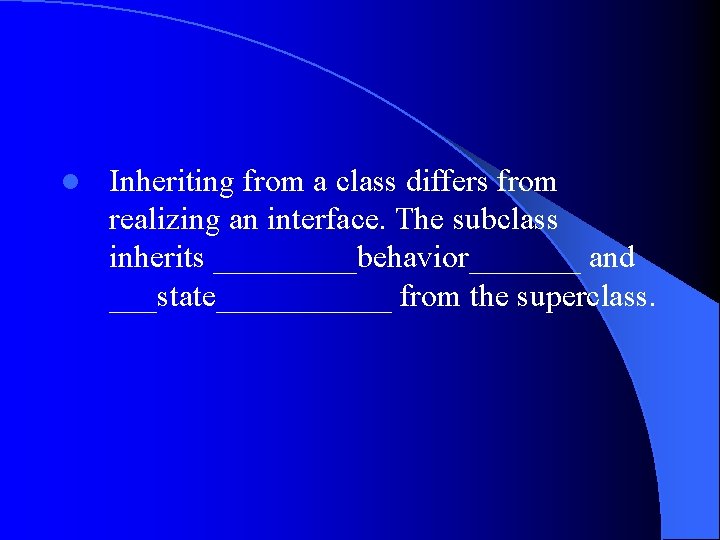
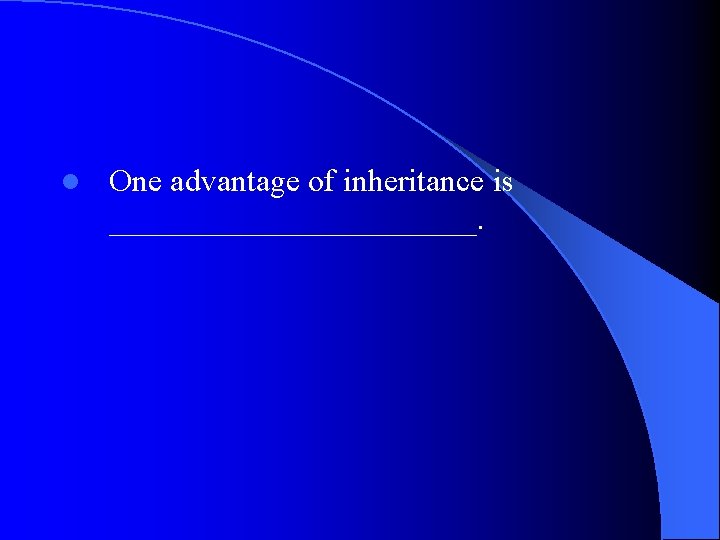
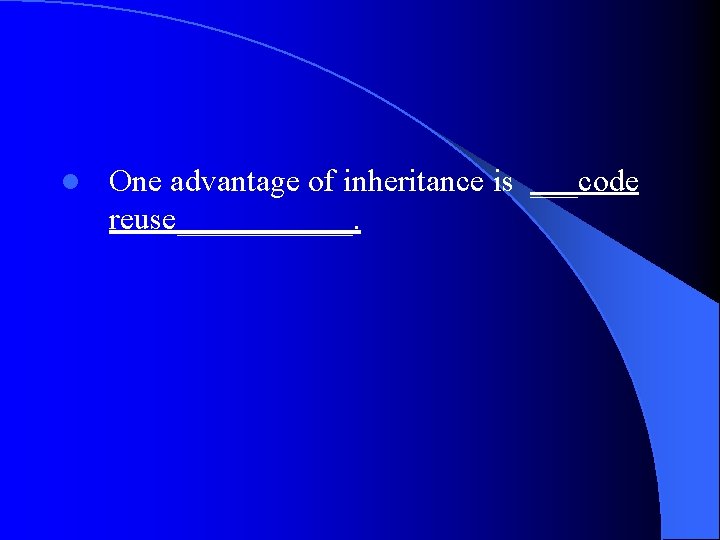
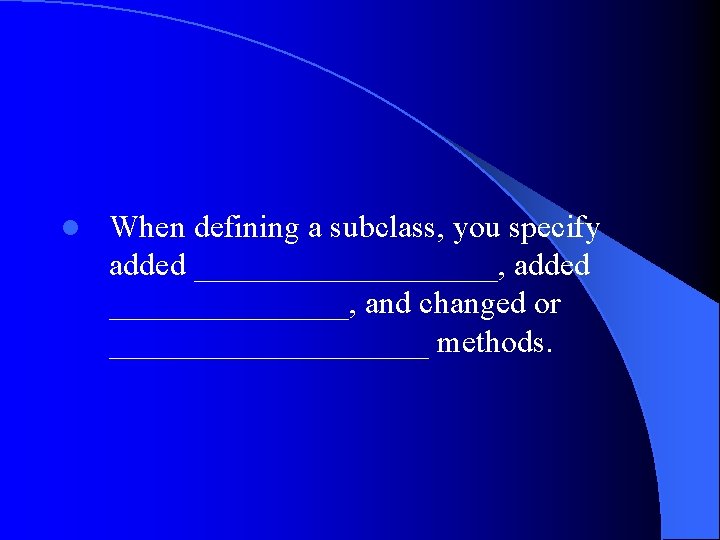
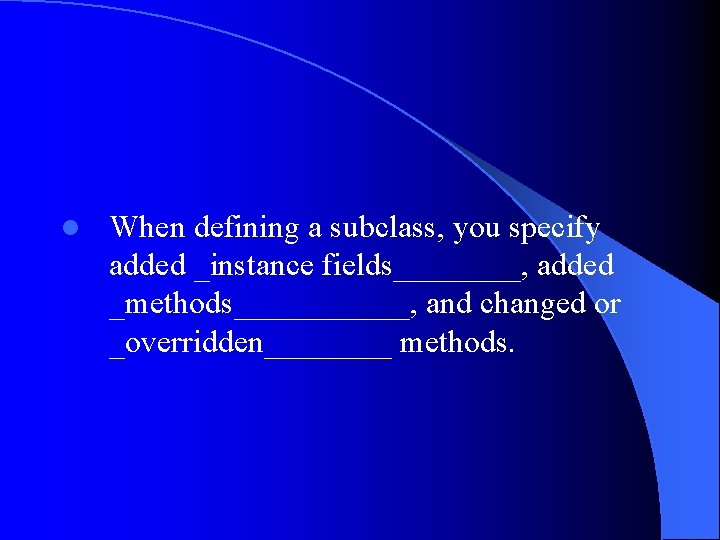
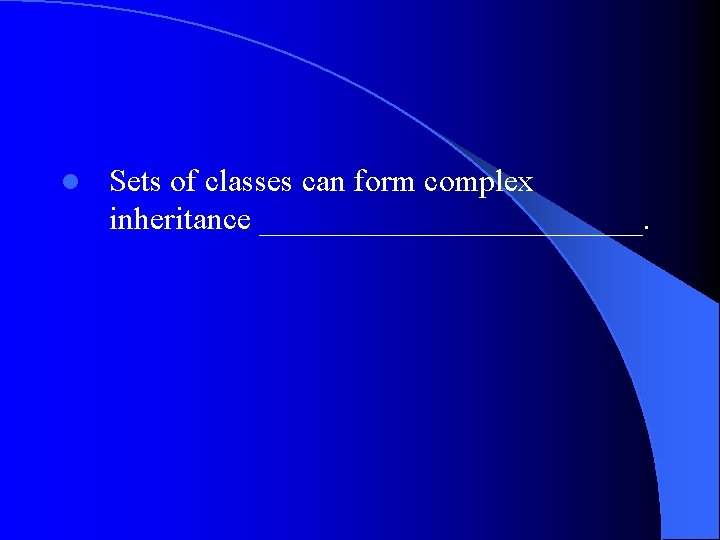
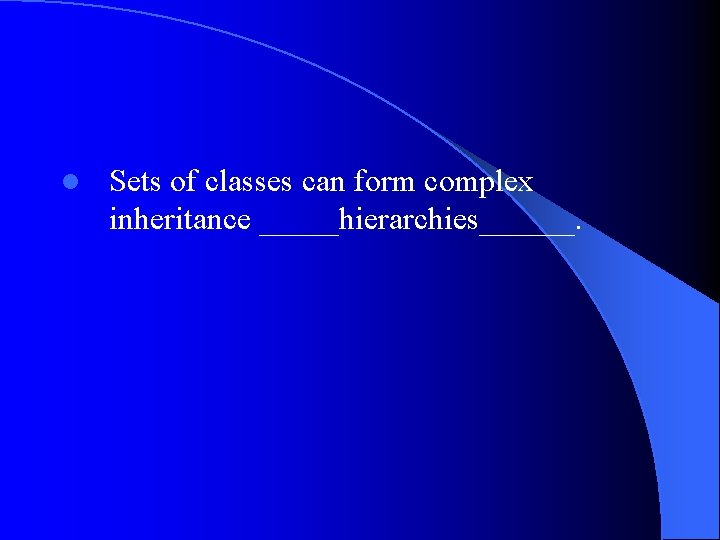
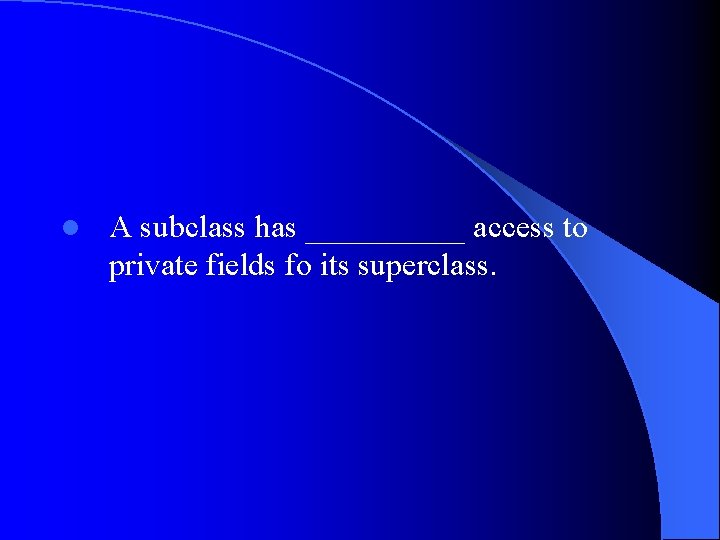
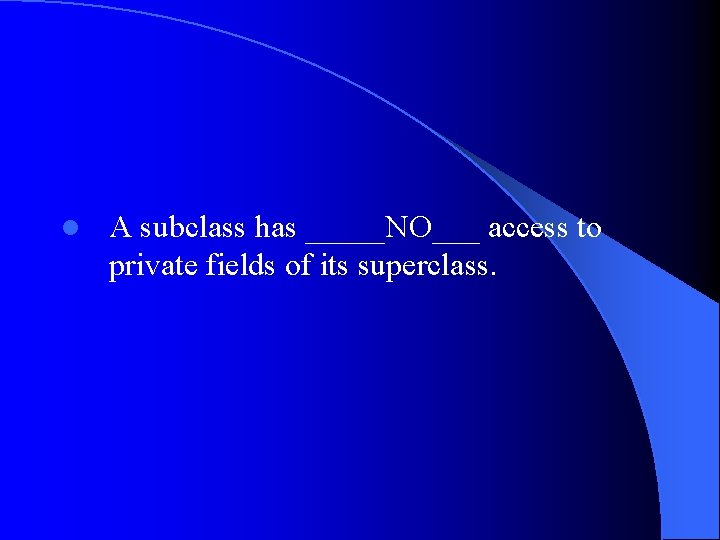
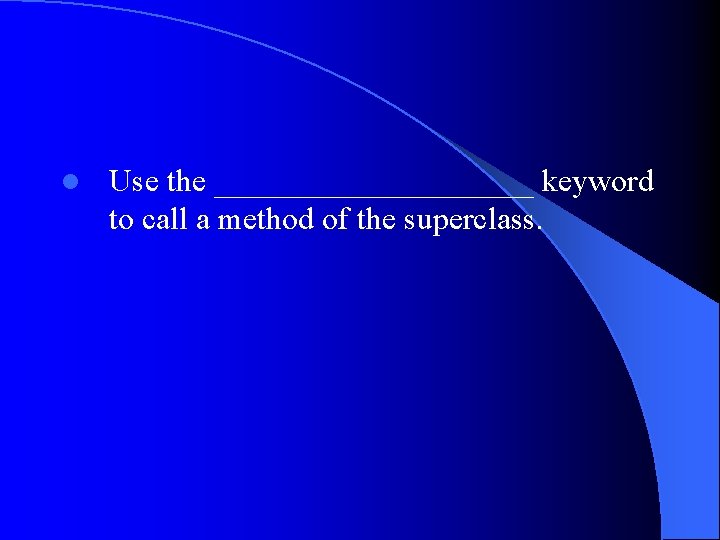
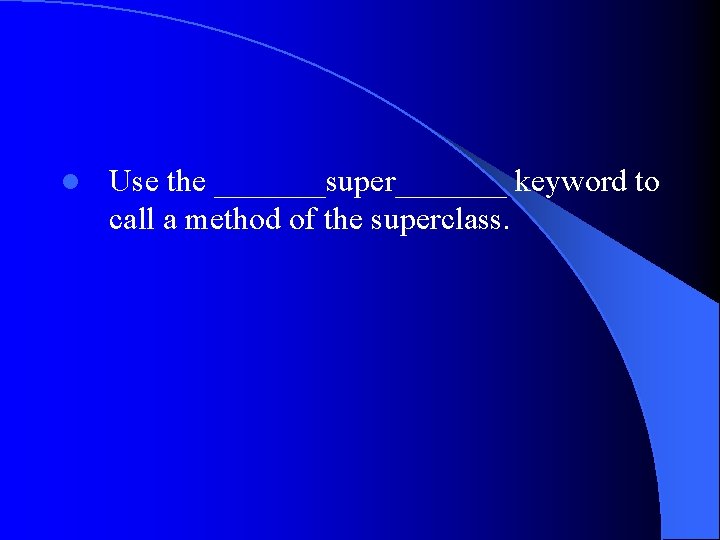
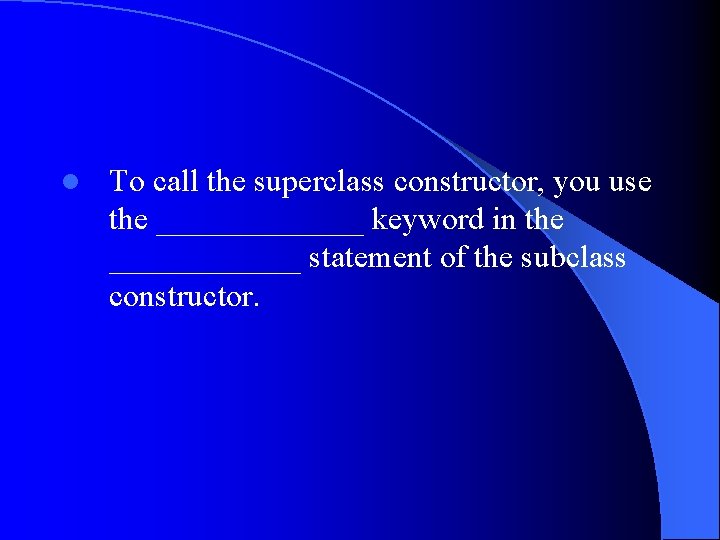
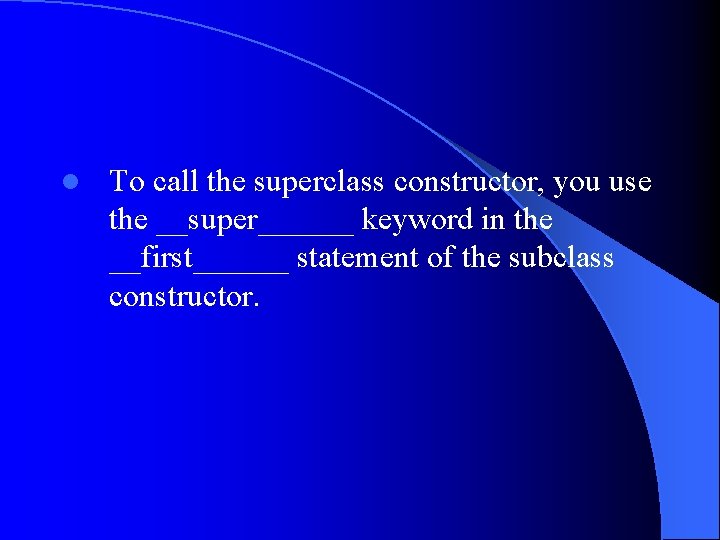
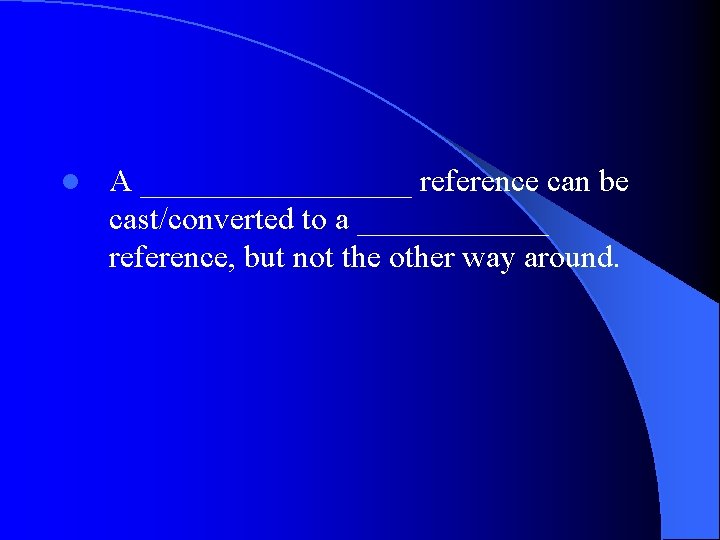
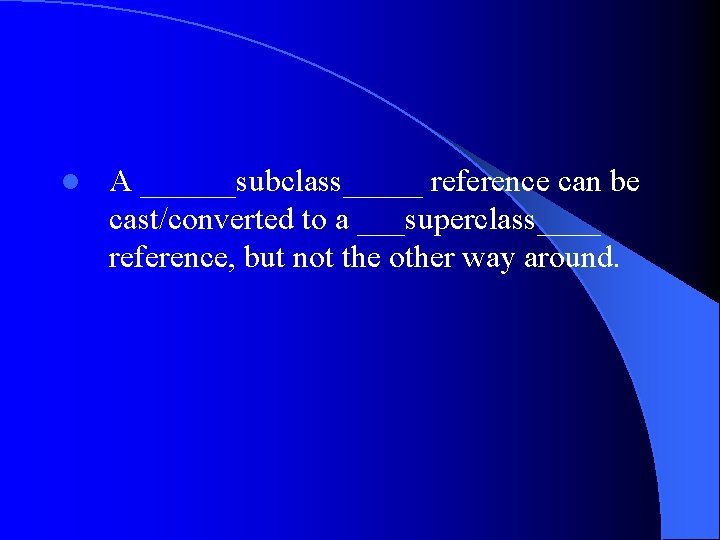
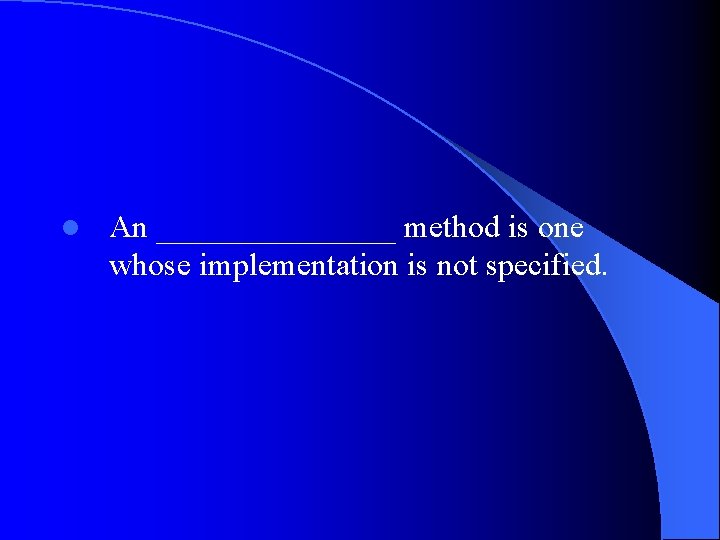
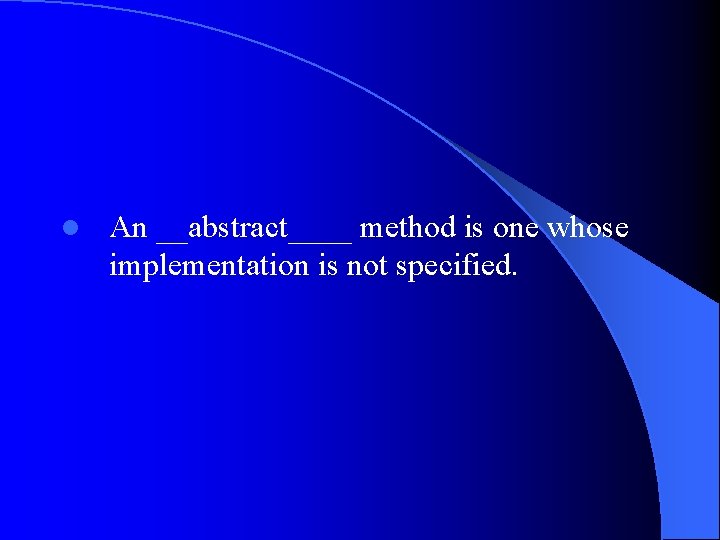
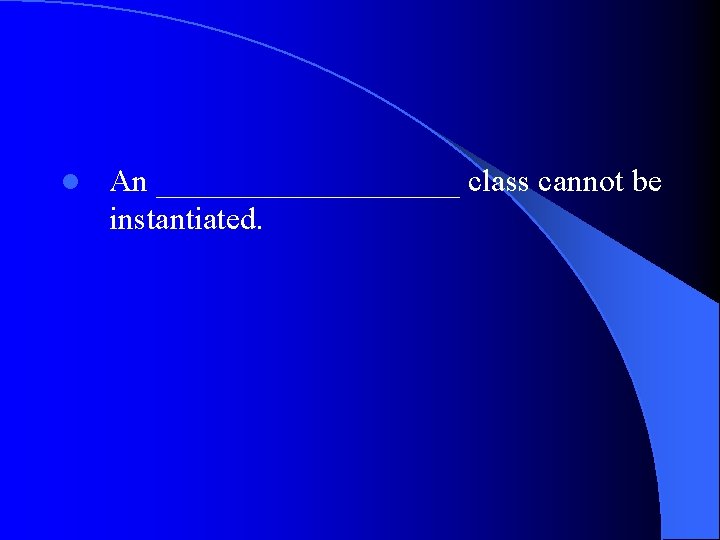
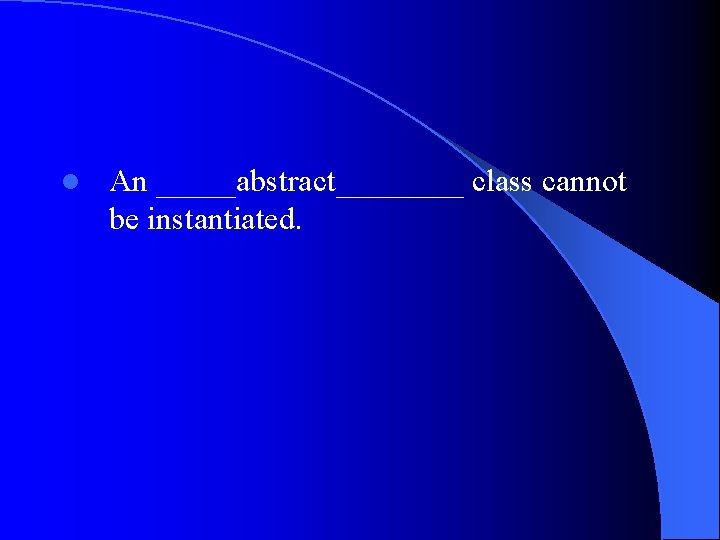
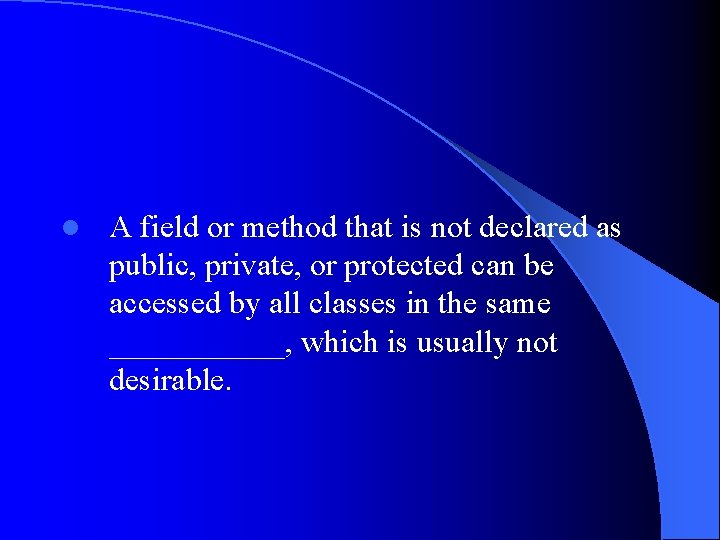
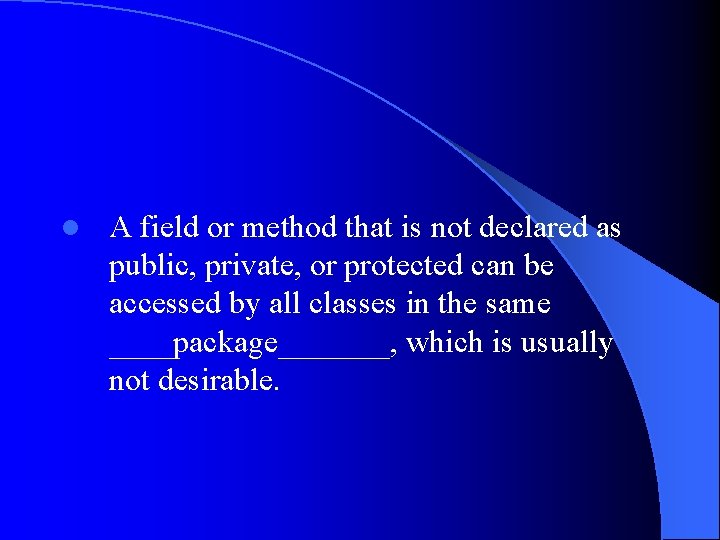
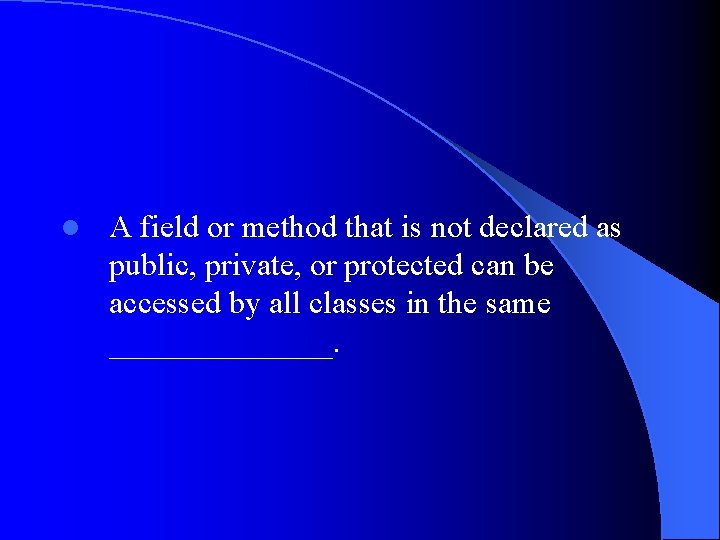
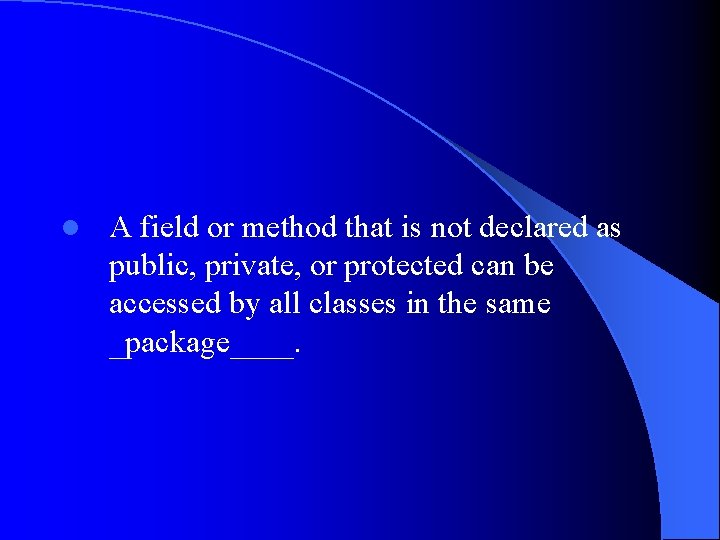
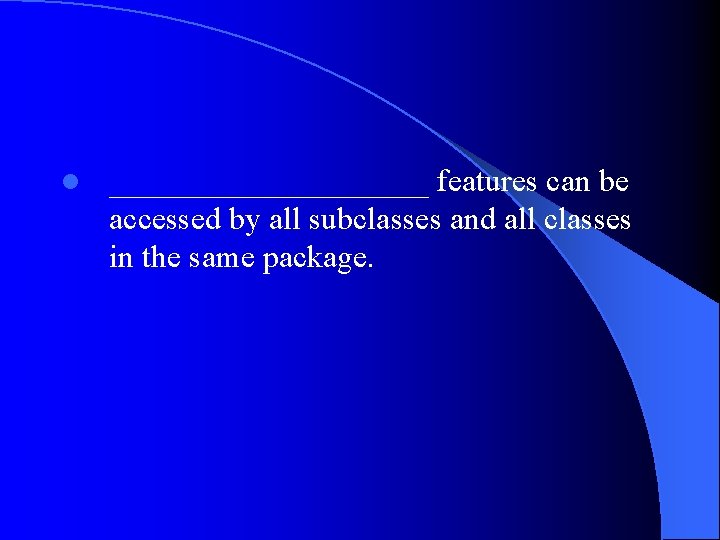
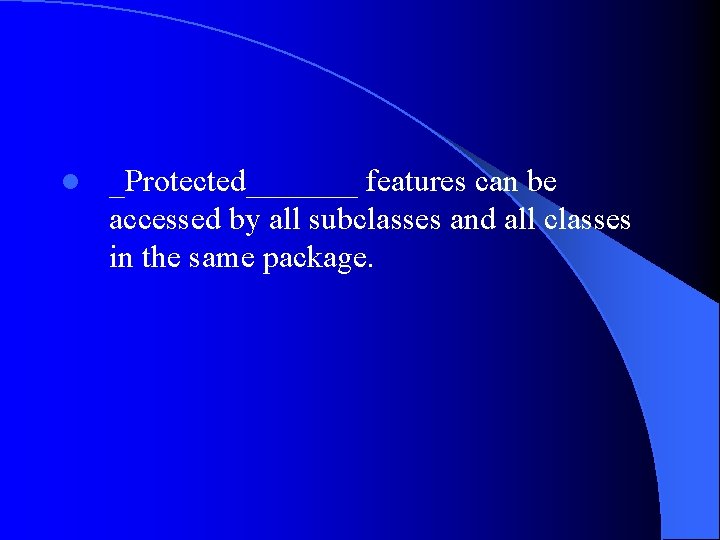
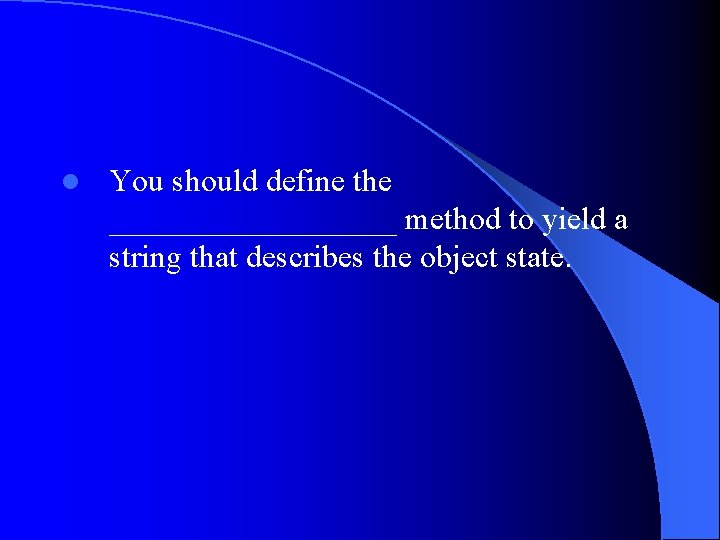
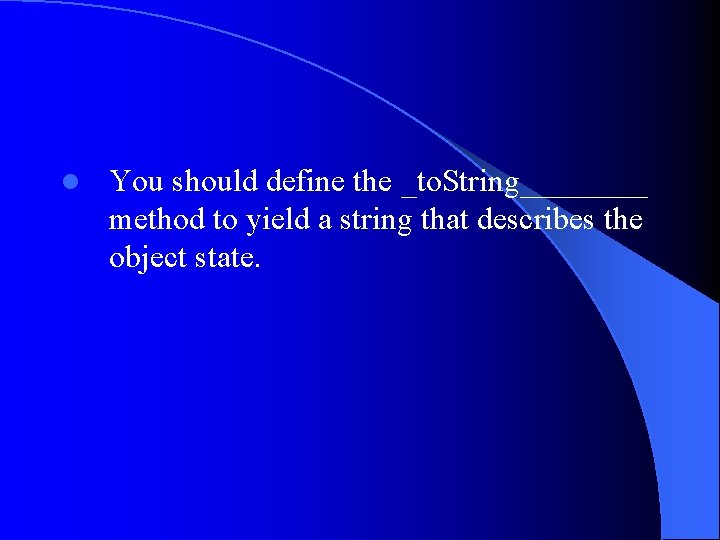
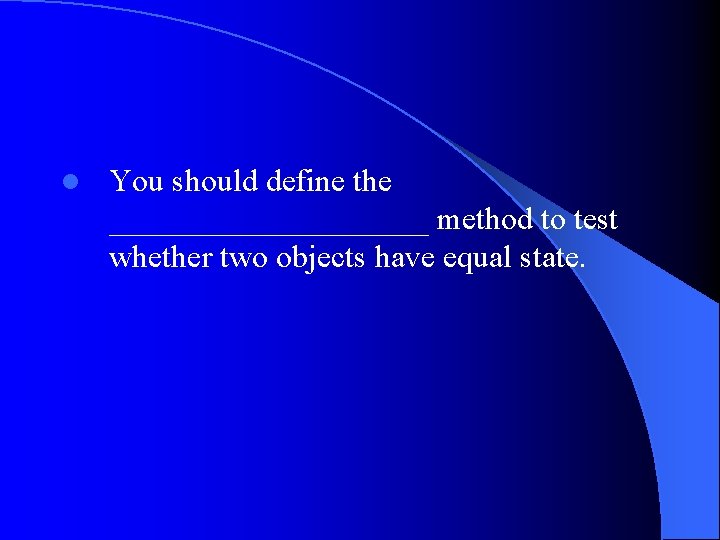
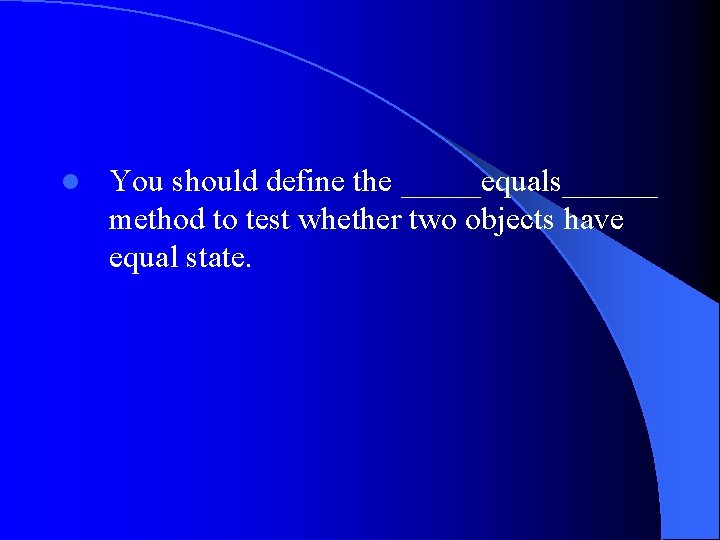
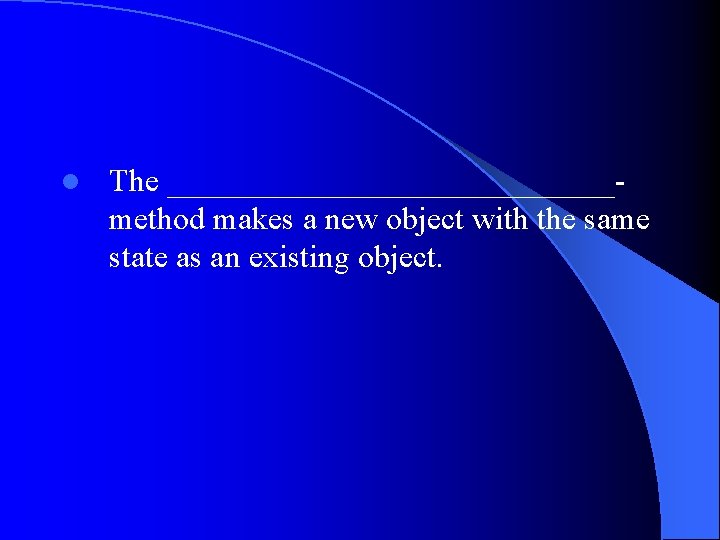
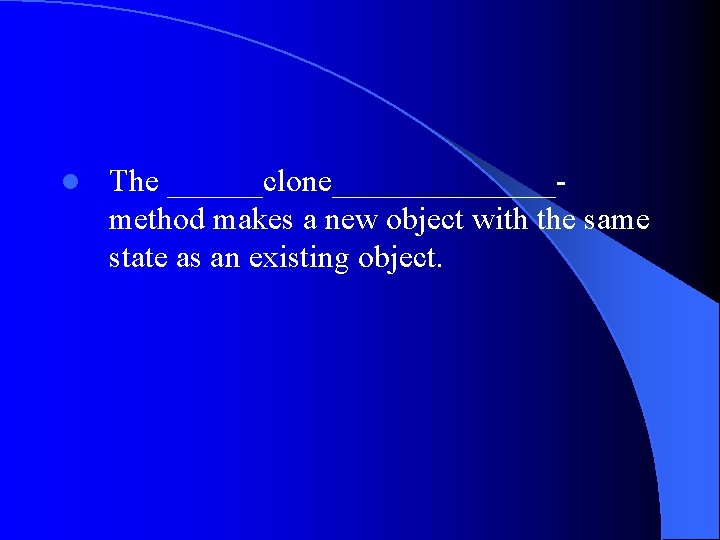
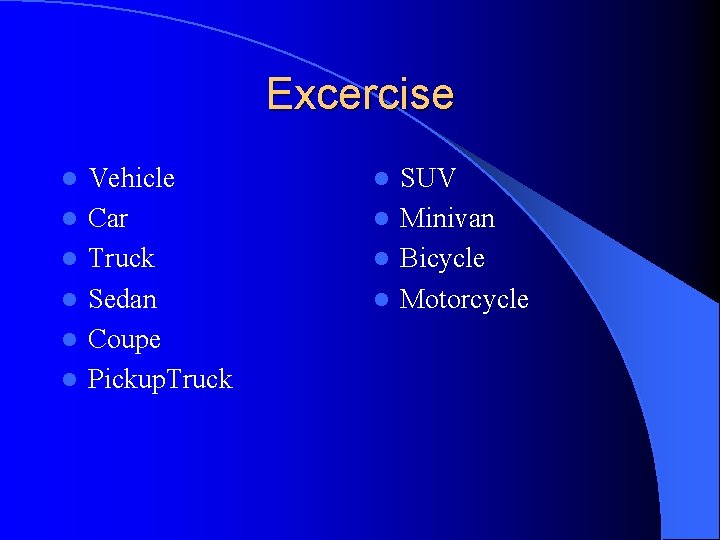
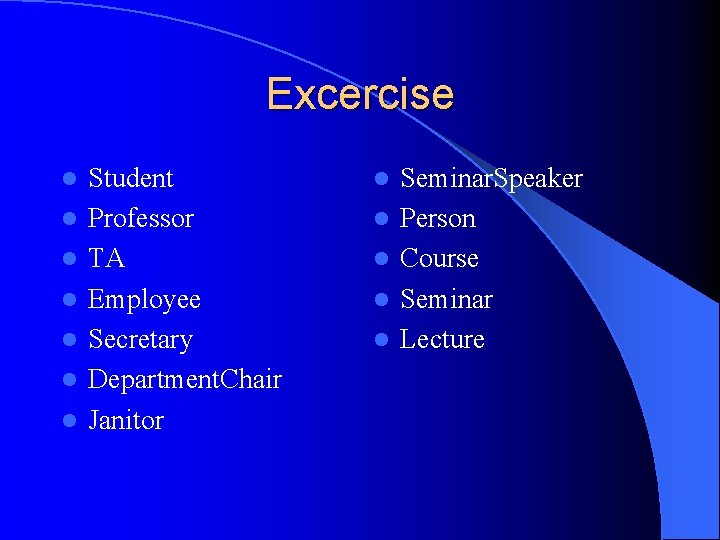
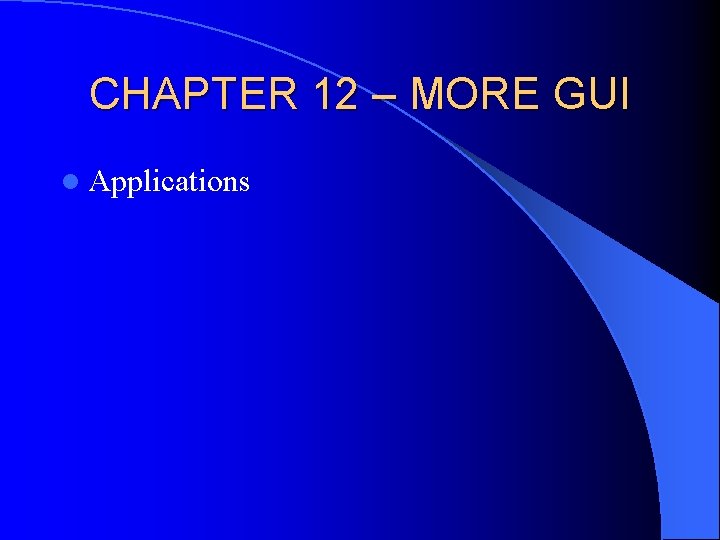
![FRAME BASED APPLICATION public static void main(String[] args) { JFrame app. Frame = new FRAME BASED APPLICATION public static void main(String[] args) { JFrame app. Frame = new](https://slidetodoc.com/presentation_image_h2/f4c171615104fa562512d9a8f53b7959/image-47.jpg)
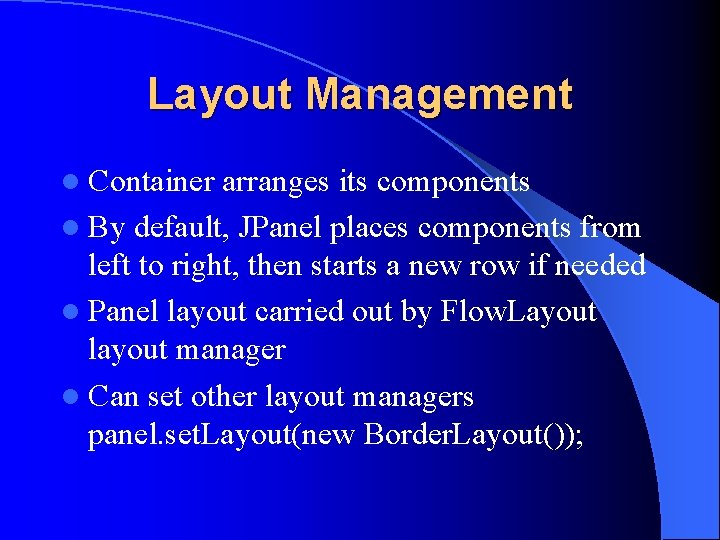
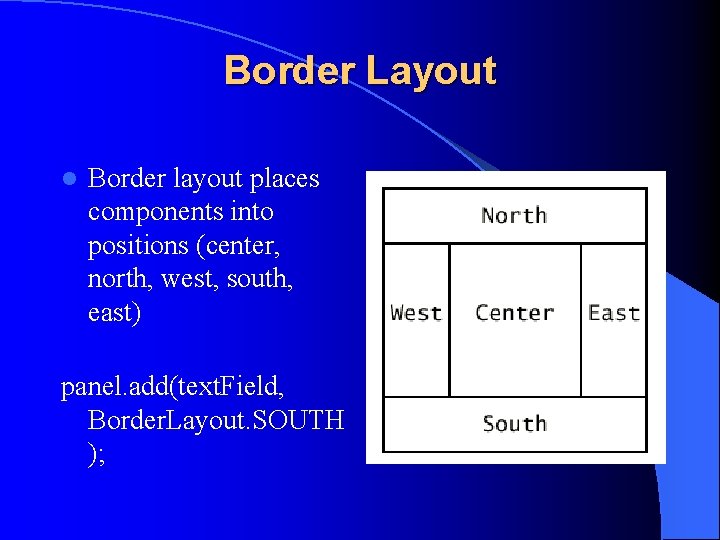
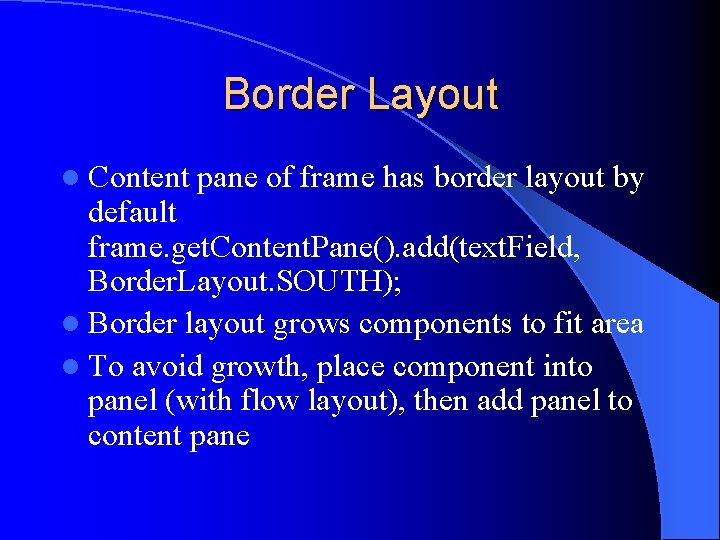
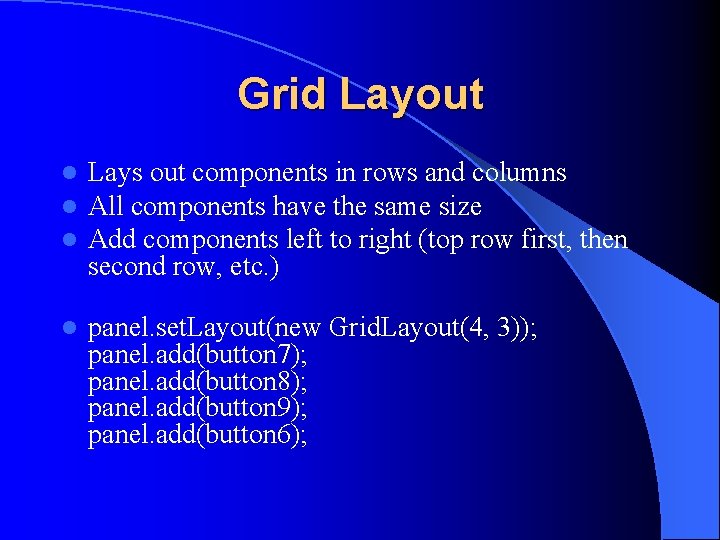
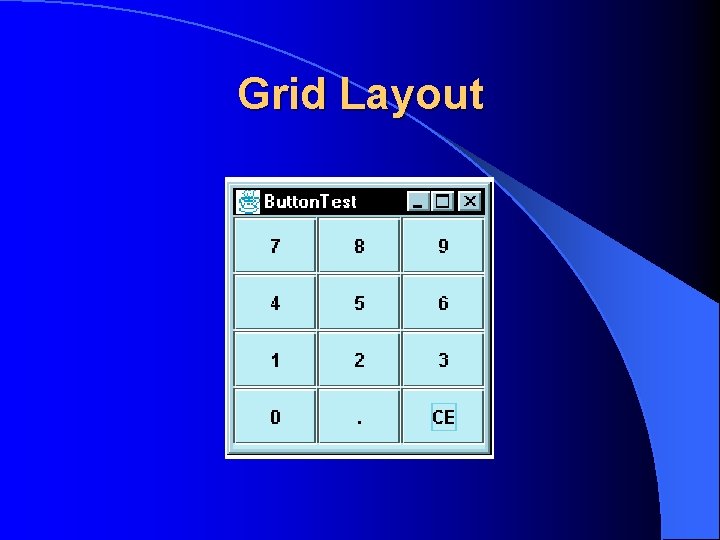
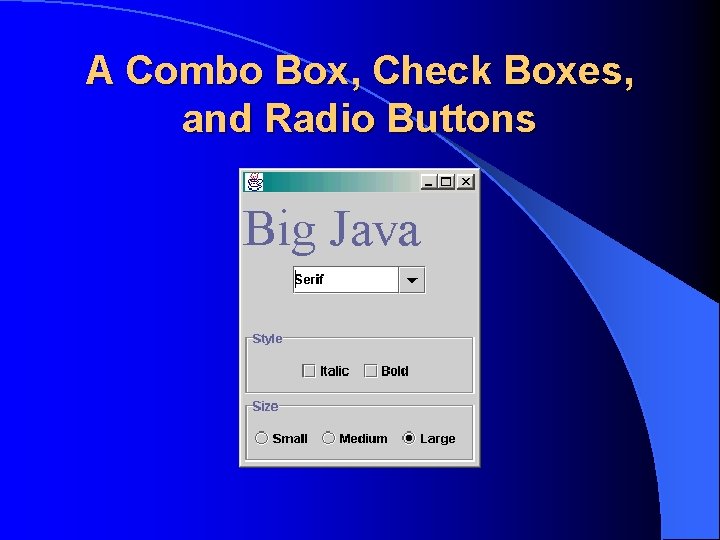
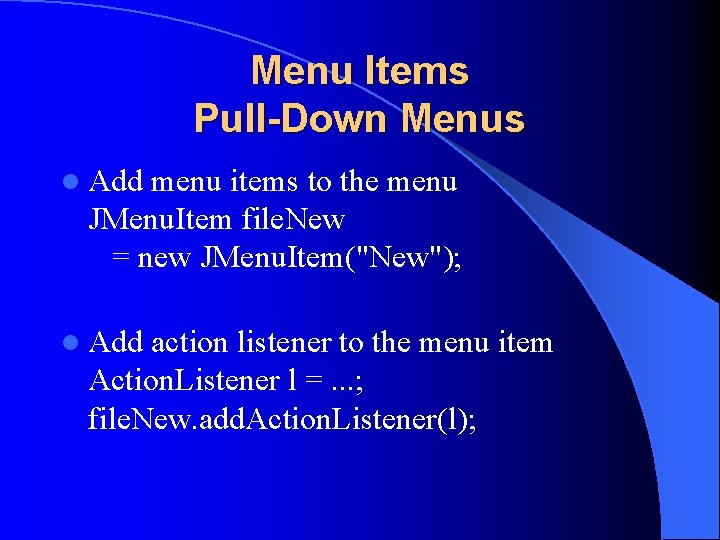
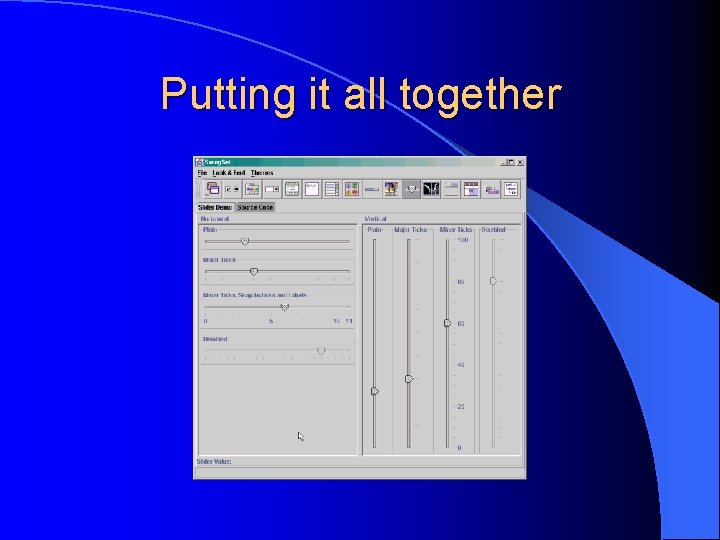
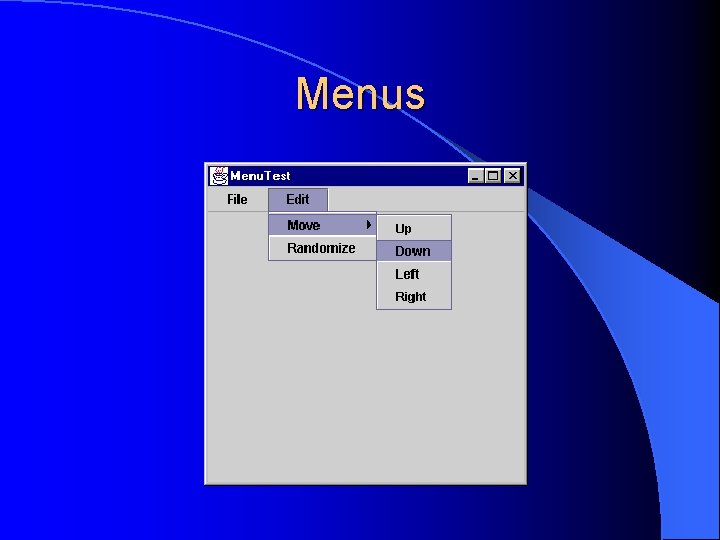
- Slides: 56
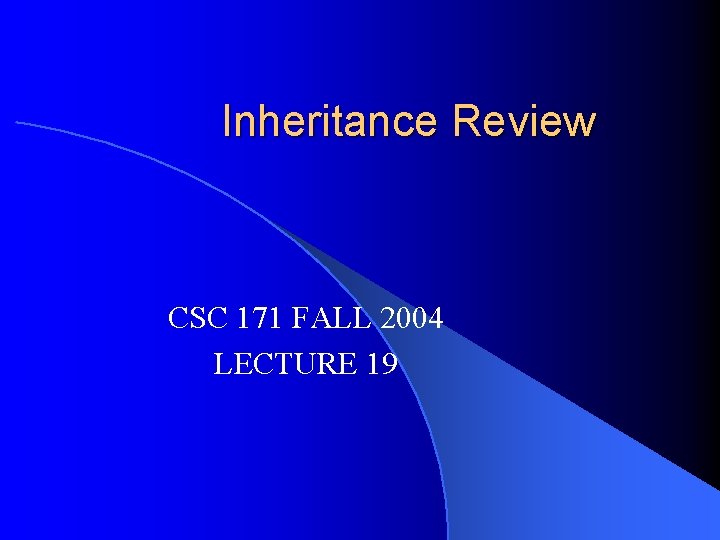
Inheritance Review CSC 171 FALL 2004 LECTURE 19
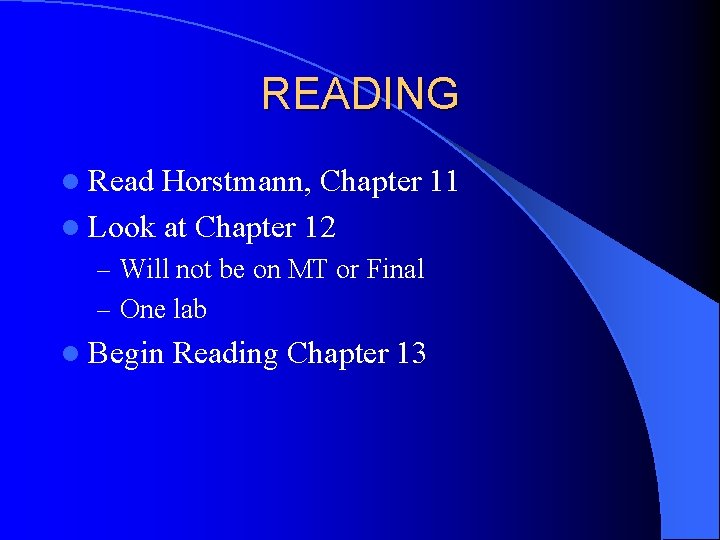
READING l Read Horstmann, Chapter 11 l Look at Chapter 12 – Will not be on MT or Final – One lab l Begin Reading Chapter 13
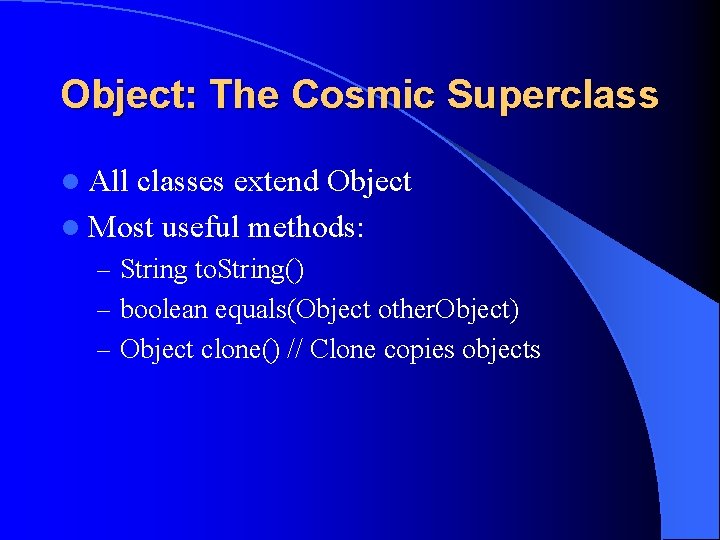
Object: The Cosmic Superclass l All classes extend Object l Most useful methods: – String to. String() – boolean equals(Object other. Object) – Object clone() // Clone copies objects
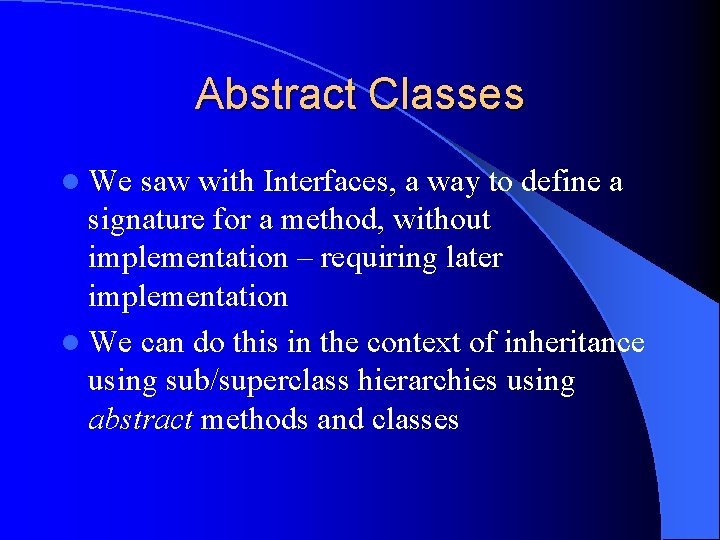
Abstract Classes l We saw with Interfaces, a way to define a signature for a method, without implementation – requiring later implementation l We can do this in the context of inheritance using sub/superclass hierarchies using abstract methods and classes
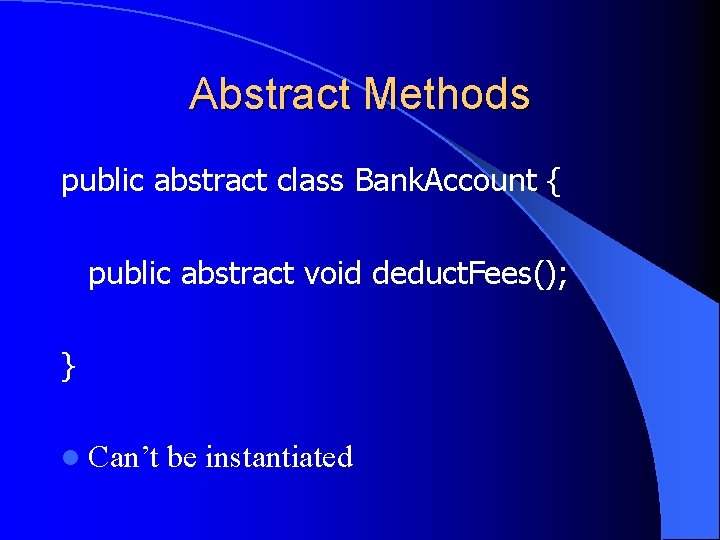
Abstract Methods public abstract class Bank. Account { public abstract void deduct. Fees(); } l Can’t be instantiated
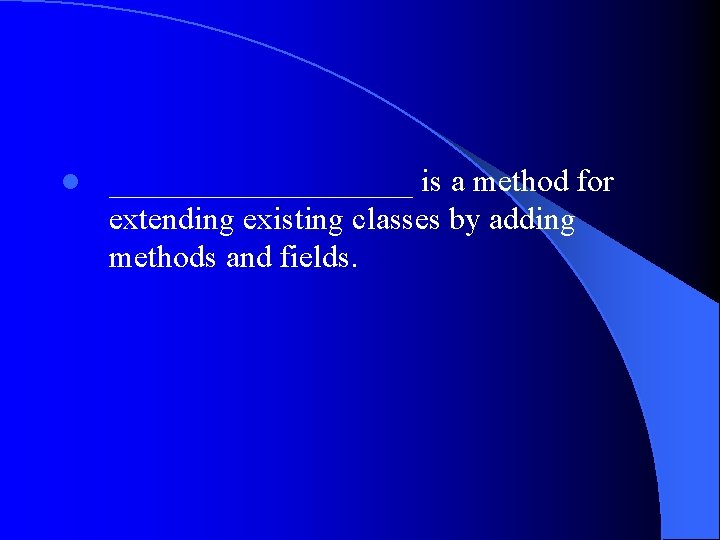
l __________ is a method for extending existing classes by adding methods and fields.
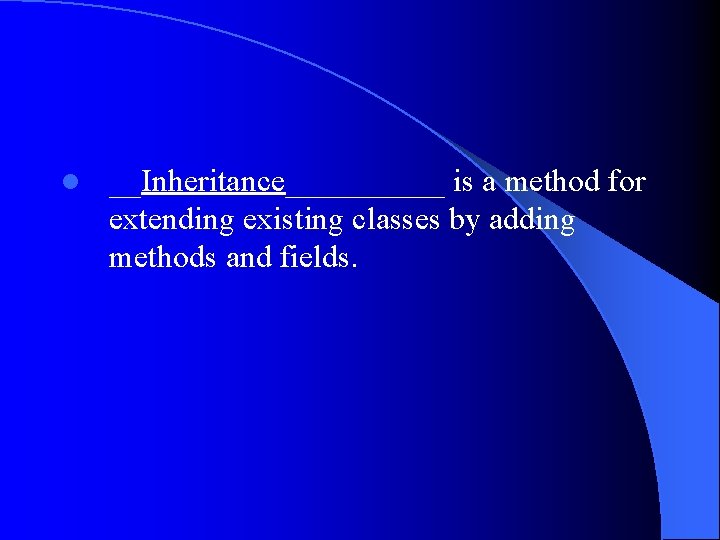
l __Inheritance_____ is a method for extending existing classes by adding methods and fields.
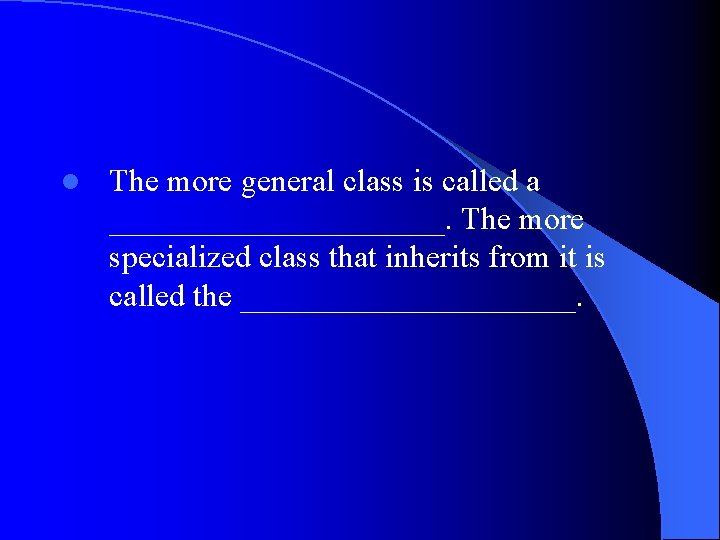
l The more general class is called a ___________. The more specialized class that inherits from it is called the ___________.
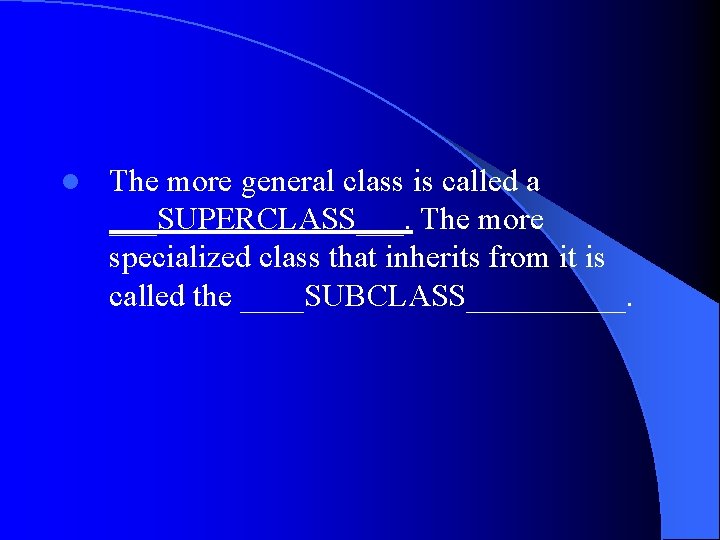
l The more general class is called a ___SUPERCLASS___. The more specialized class that inherits from it is called the ____SUBCLASS_____.
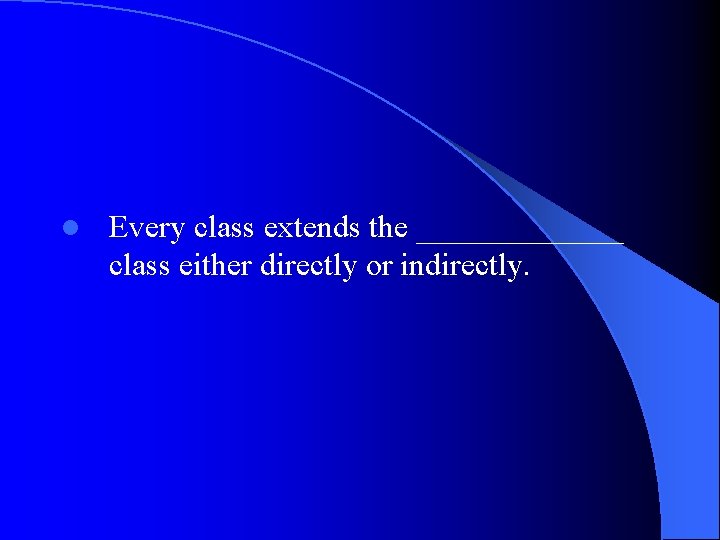
l Every class extends the _______ class either directly or indirectly.
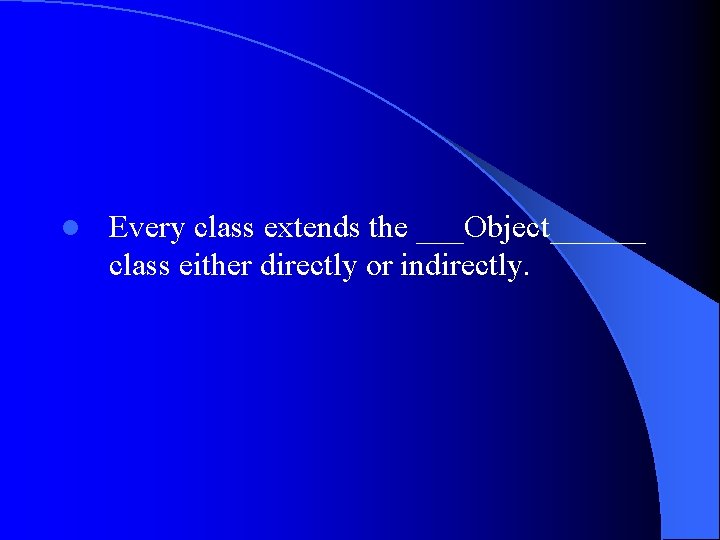
l Every class extends the ___Object______ class either directly or indirectly.
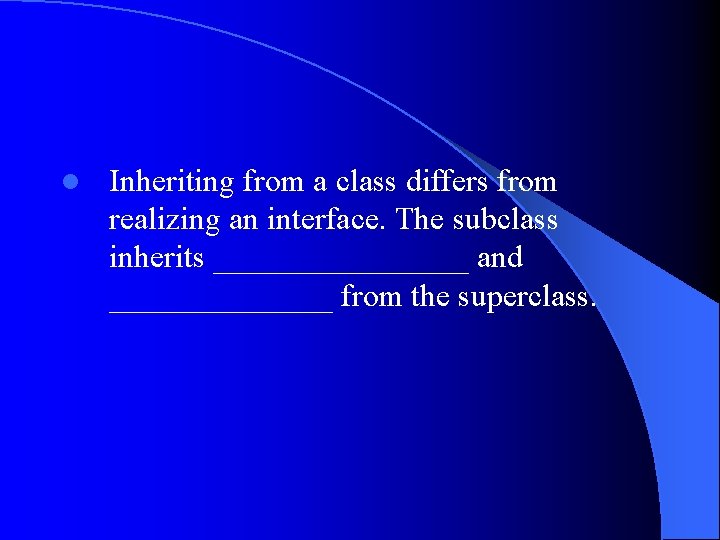
l Inheriting from a class differs from realizing an interface. The subclass inherits ________ and _______ from the superclass.
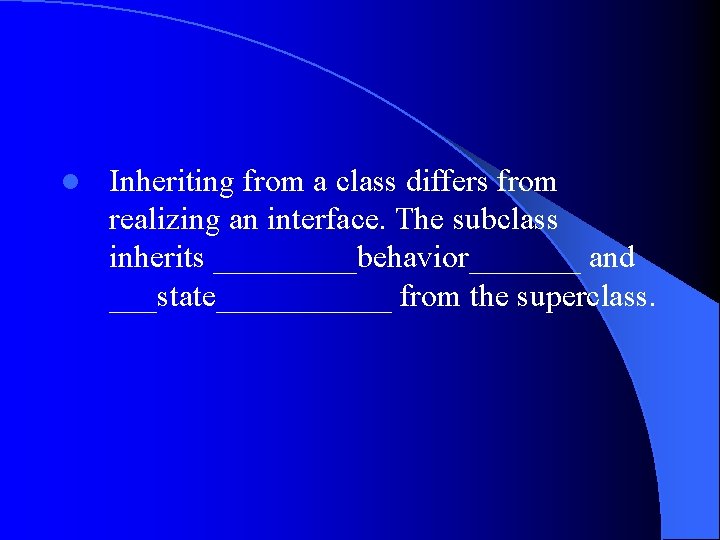
l Inheriting from a class differs from realizing an interface. The subclass inherits _____behavior_______ and ___state______ from the superclass.
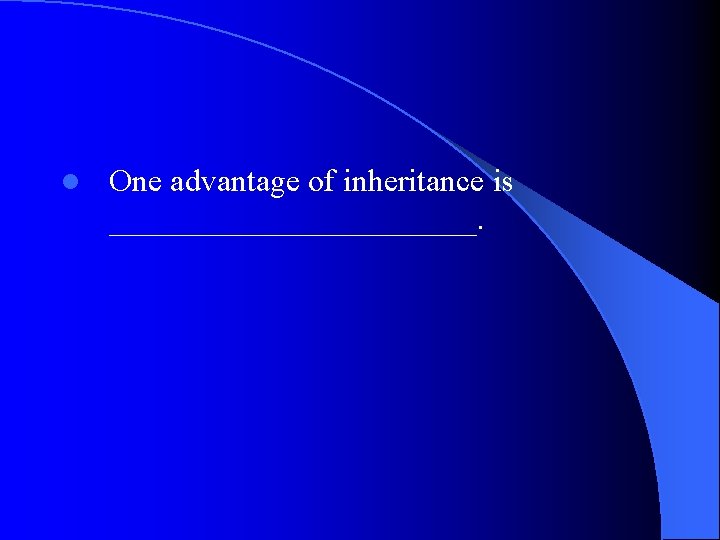
l One advantage of inheritance is ____________.
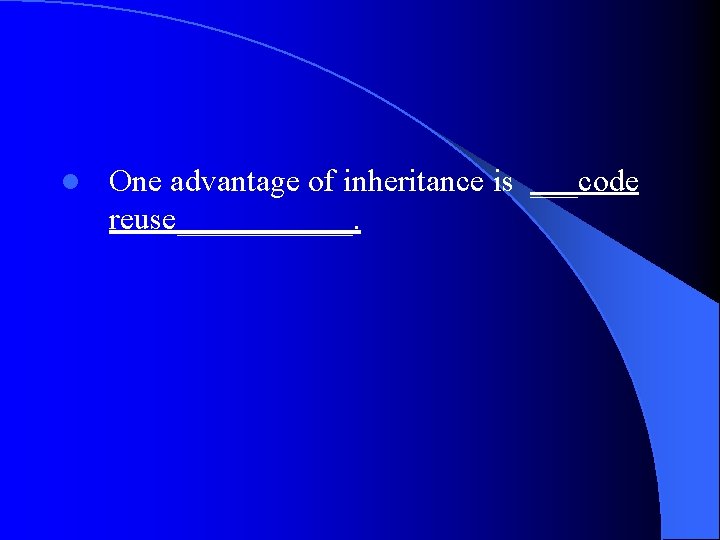
l One advantage of inheritance is ___code reuse______.
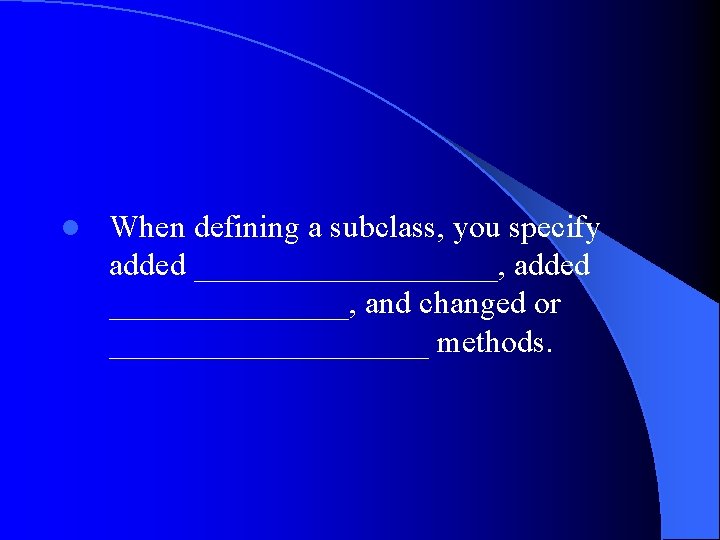
l When defining a subclass, you specify added __________, added ________, and changed or __________ methods.
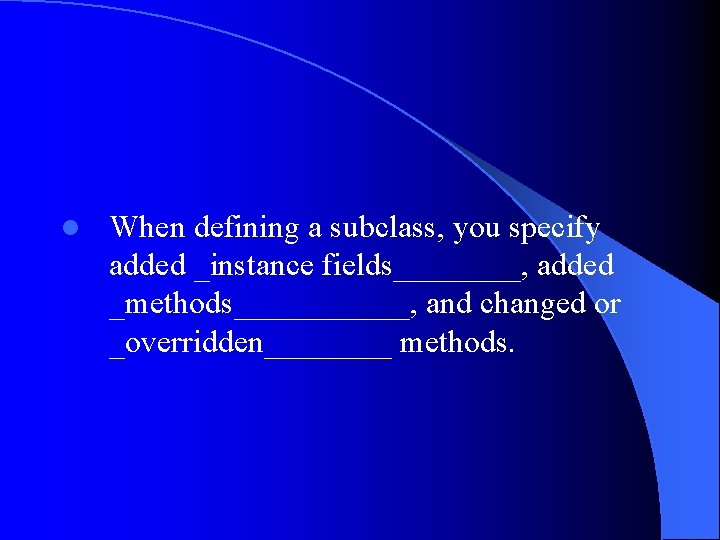
l When defining a subclass, you specify added _instance fields____, added _methods______, and changed or _overridden____ methods.
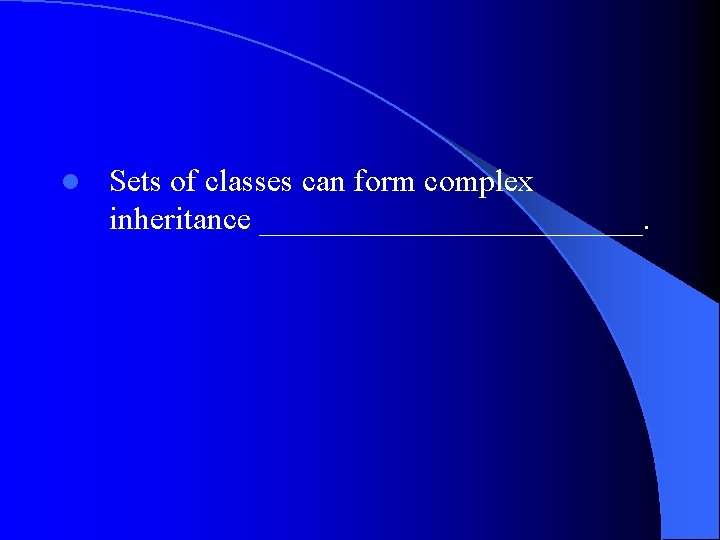
l Sets of classes can form complex inheritance ____________.
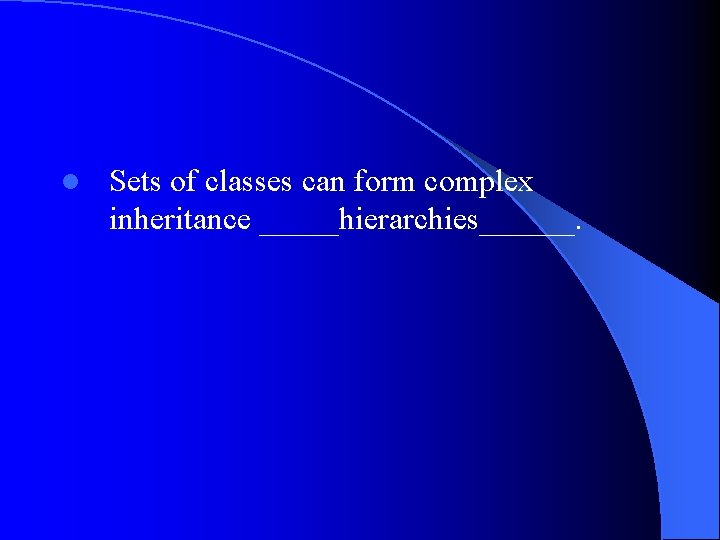
l Sets of classes can form complex inheritance _____hierarchies______.
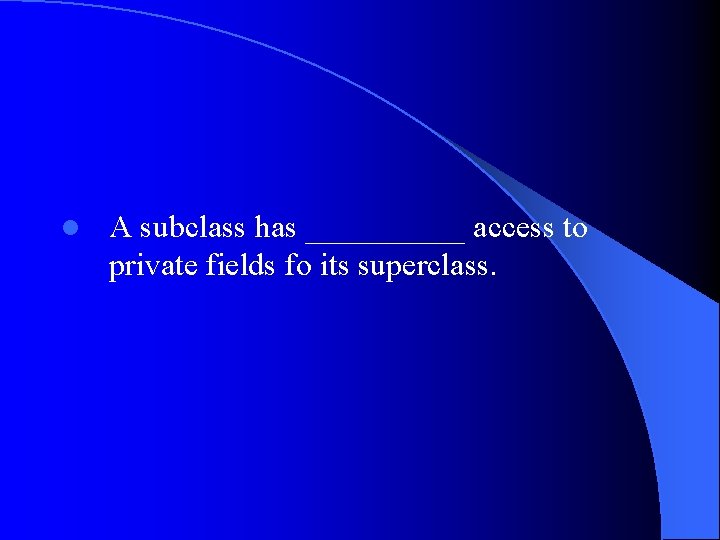
l A subclass has _____ access to private fields fo its superclass.
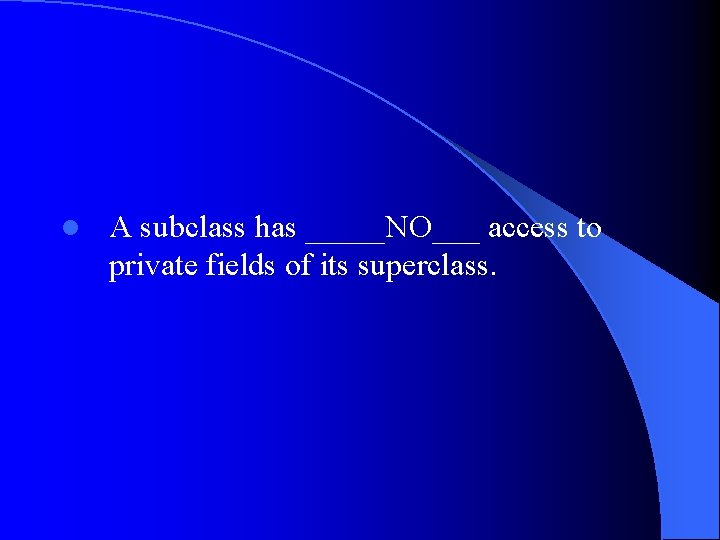
l A subclass has _____NO___ access to private fields of its superclass.
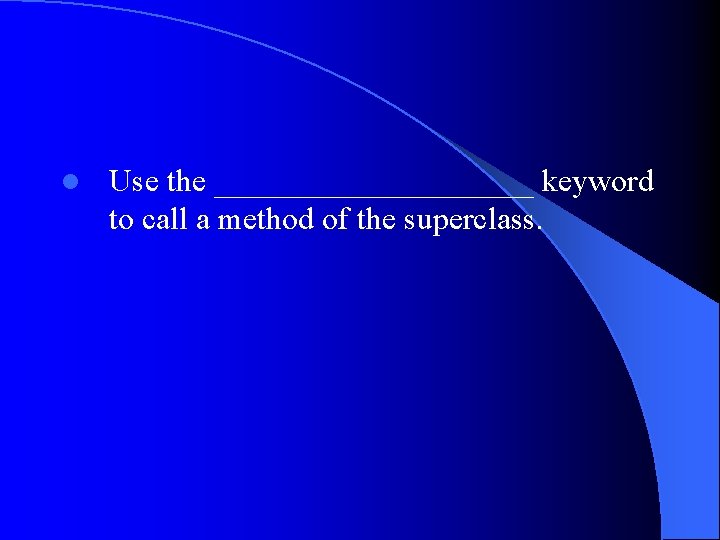
l Use the __________ keyword to call a method of the superclass.
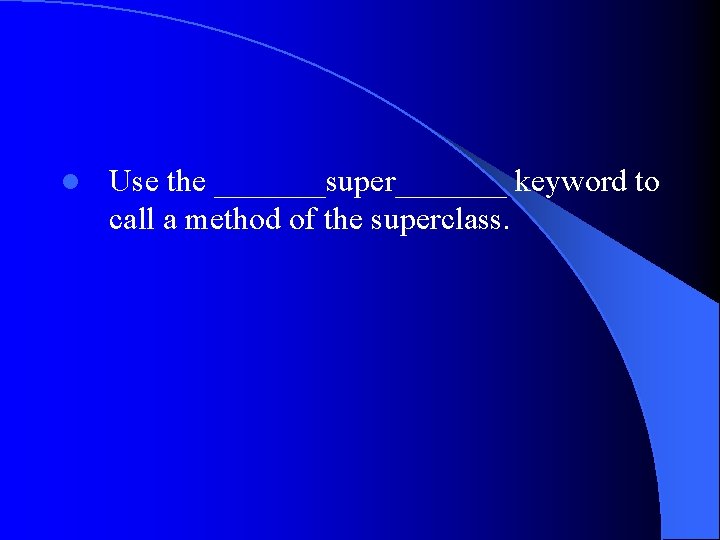
l Use the _______super_______ keyword to call a method of the superclass.
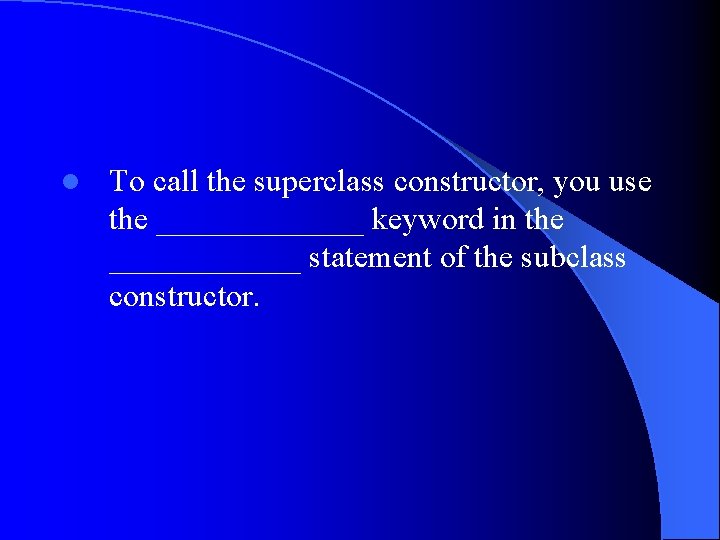
l To call the superclass constructor, you use the _______ keyword in the ______ statement of the subclass constructor.
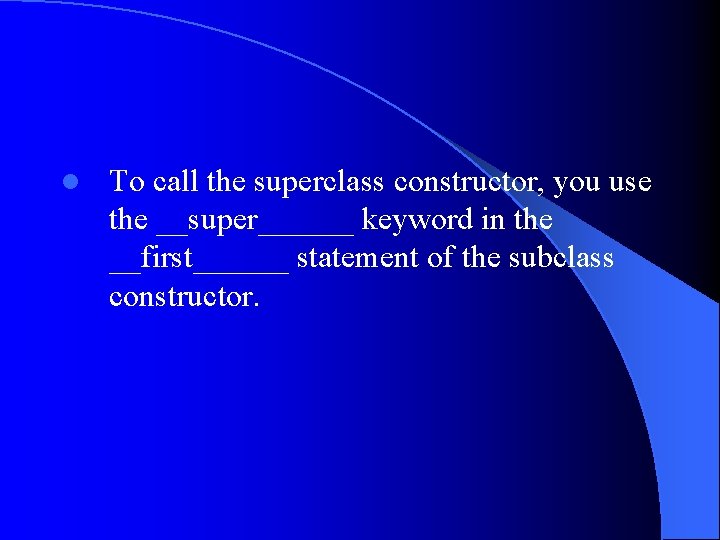
l To call the superclass constructor, you use the __super______ keyword in the __first______ statement of the subclass constructor.
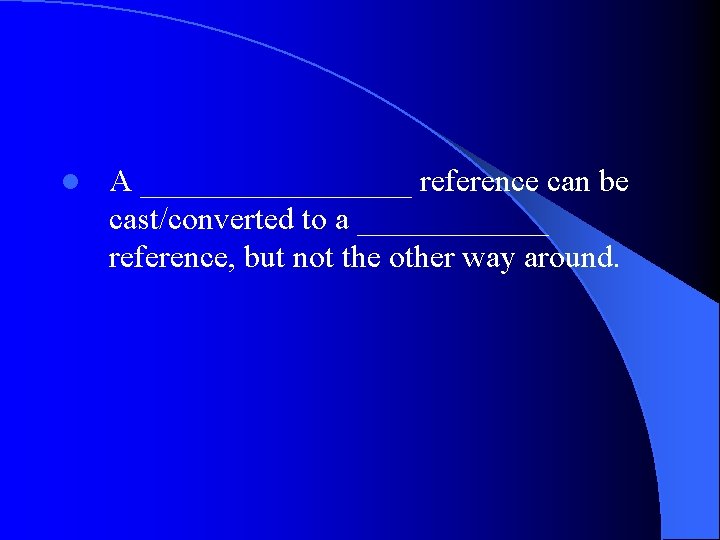
l A _________ reference can be cast/converted to a ______ reference, but not the other way around.
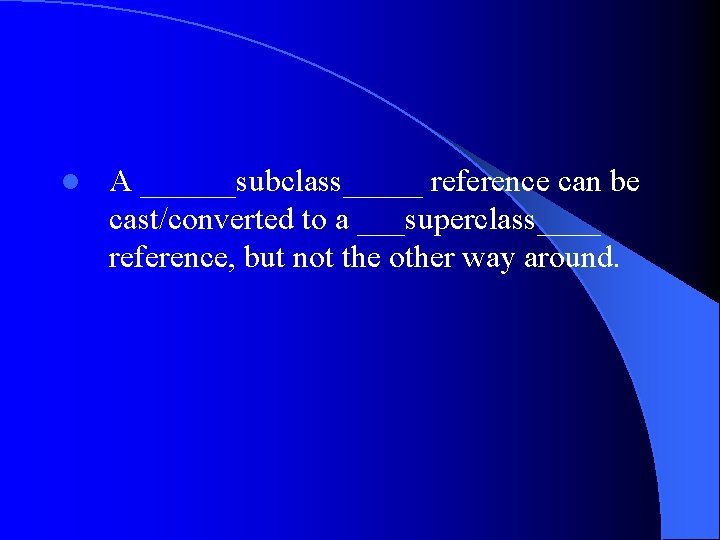
l A ______subclass_____ reference can be cast/converted to a ___superclass____ reference, but not the other way around.
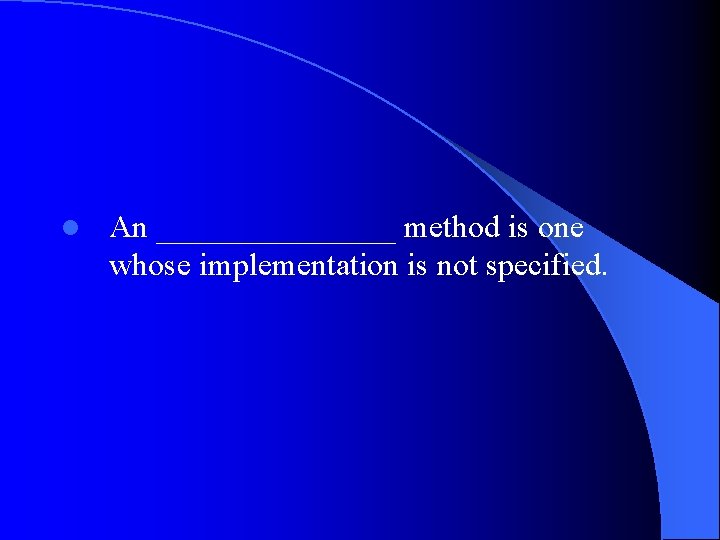
l An ________ method is one whose implementation is not specified.
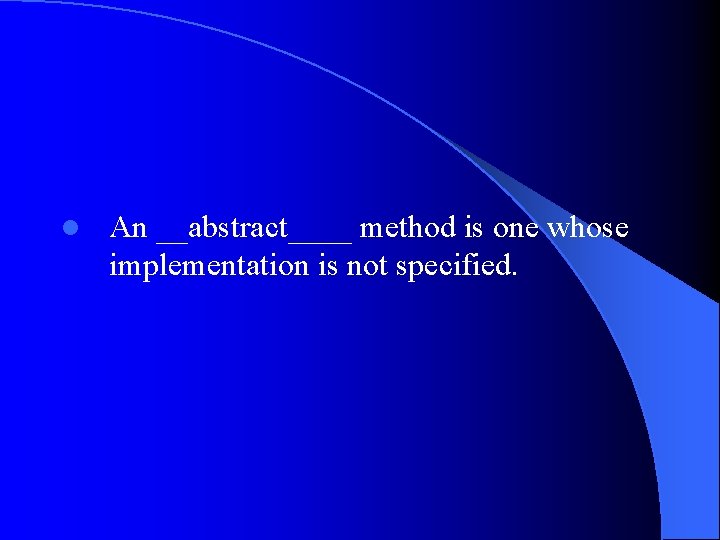
l An __abstract____ method is one whose implementation is not specified.
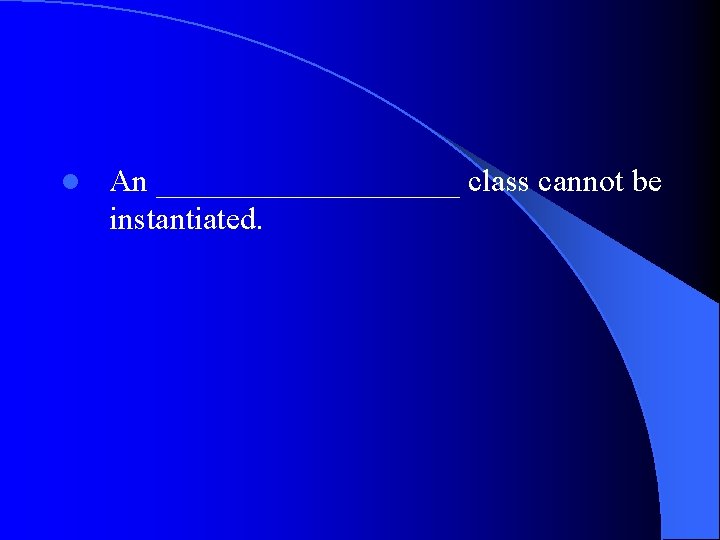
l An __________ class cannot be instantiated.
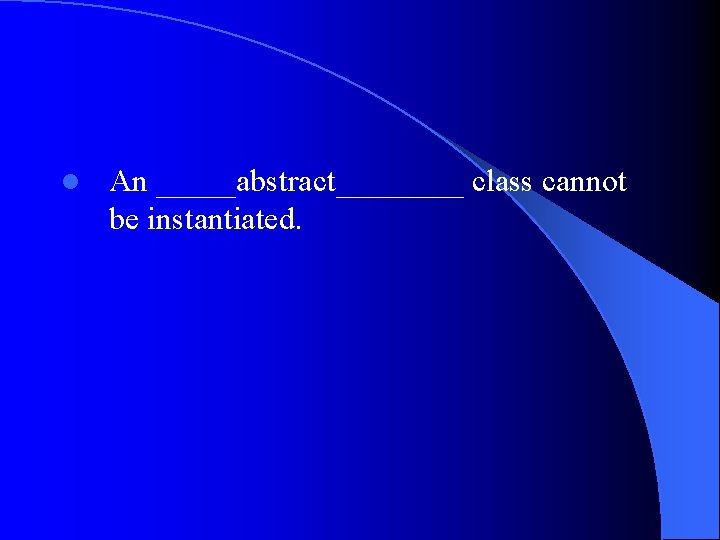
l An _____abstract____ class cannot be instantiated.
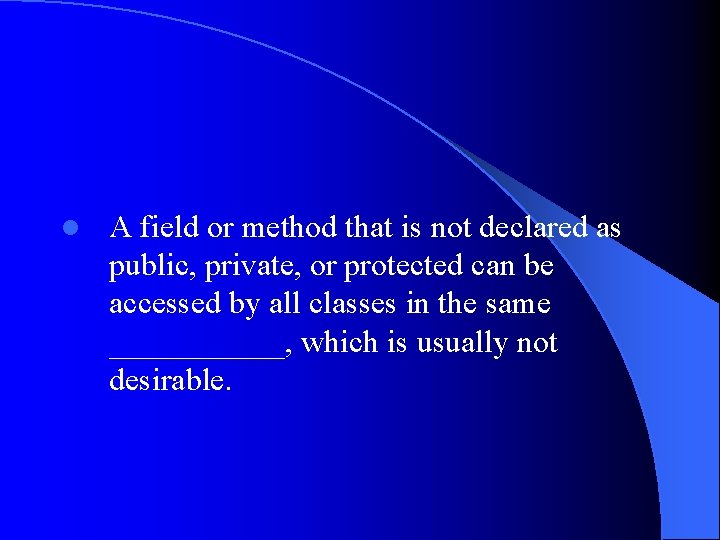
l A field or method that is not declared as public, private, or protected can be accessed by all classes in the same ______, which is usually not desirable.
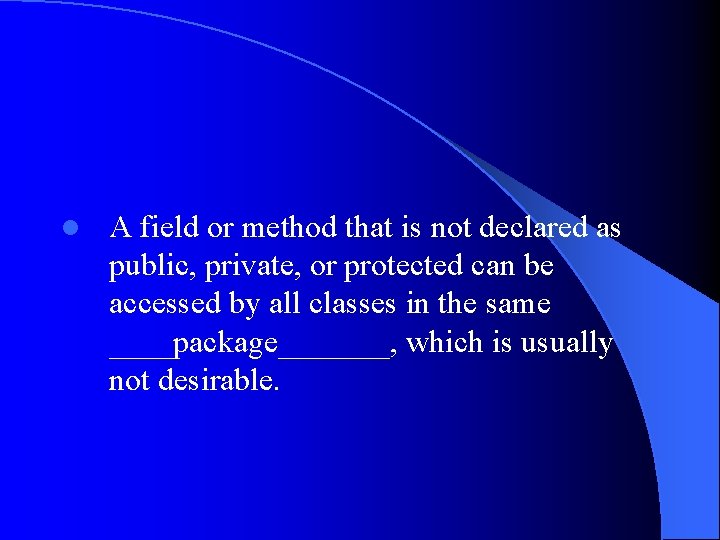
l A field or method that is not declared as public, private, or protected can be accessed by all classes in the same ____package_______, which is usually not desirable.
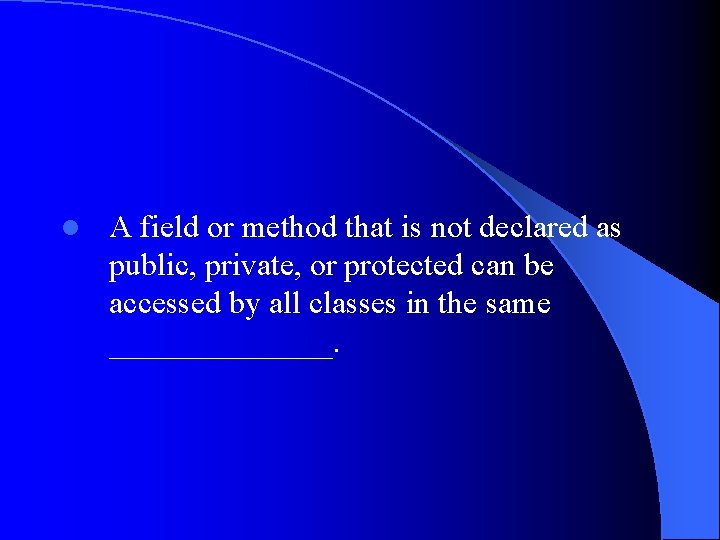
l A field or method that is not declared as public, private, or protected can be accessed by all classes in the same _______.
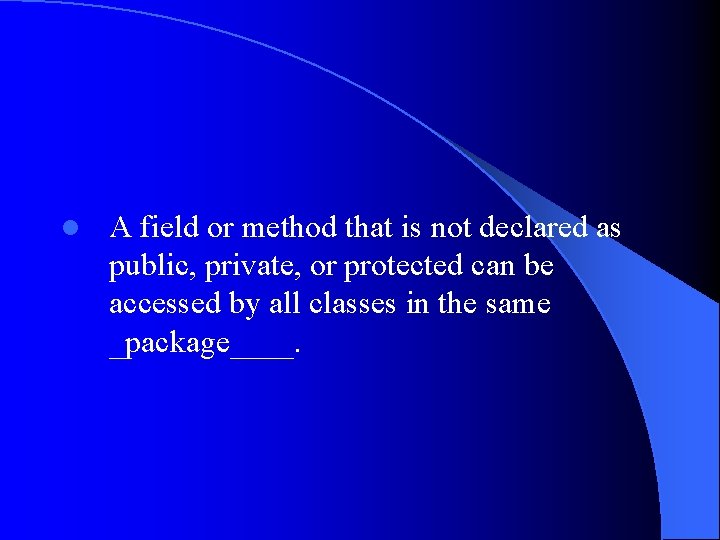
l A field or method that is not declared as public, private, or protected can be accessed by all classes in the same _package____.
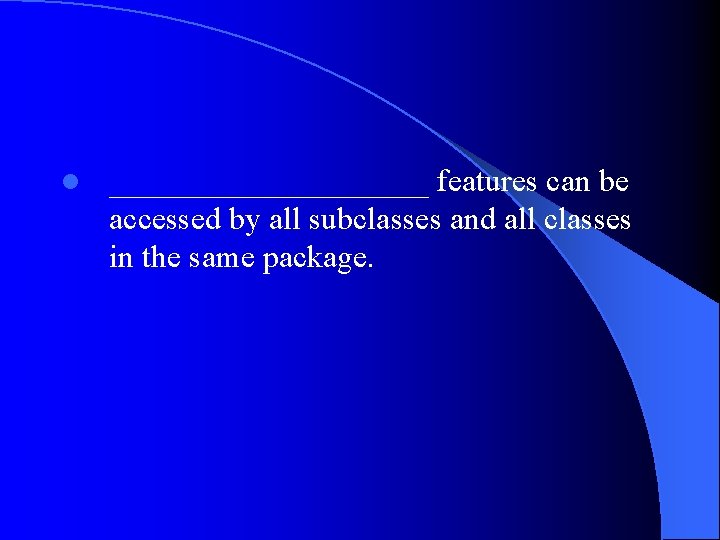
l __________ features can be accessed by all subclasses and all classes in the same package.
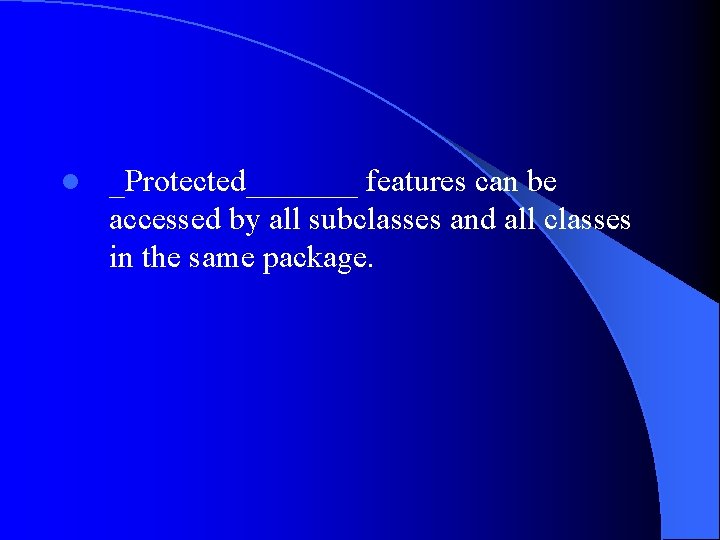
l _Protected_______ features can be accessed by all subclasses and all classes in the same package.
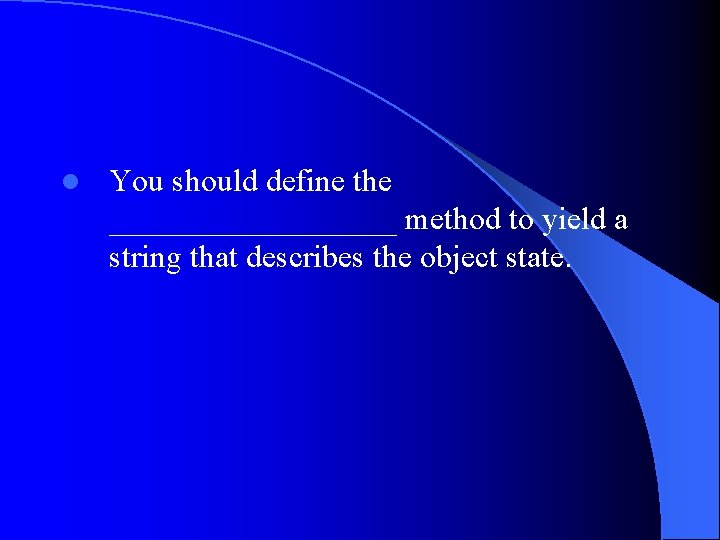
l You should define the _________ method to yield a string that describes the object state.
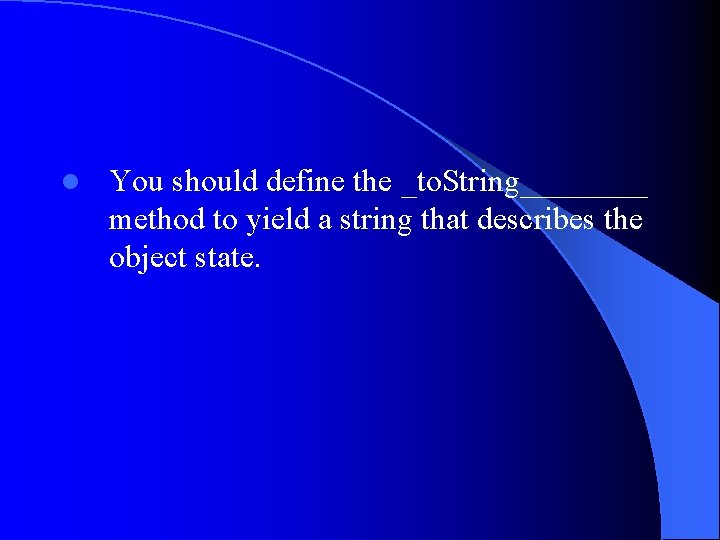
l You should define the _to. String____ method to yield a string that describes the object state.
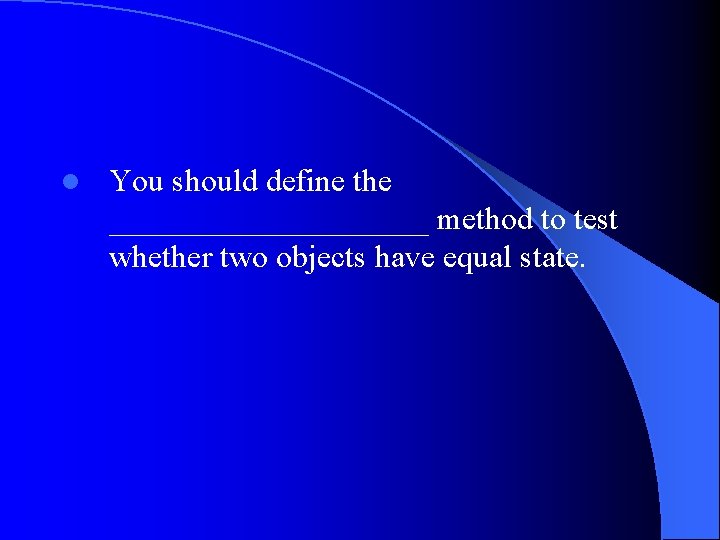
l You should define the __________ method to test whether two objects have equal state.
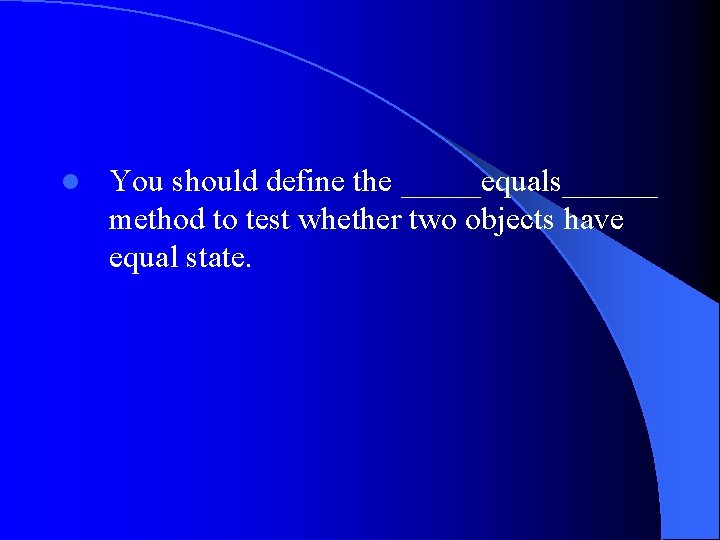
l You should define the _____equals______ method to test whether two objects have equal state.
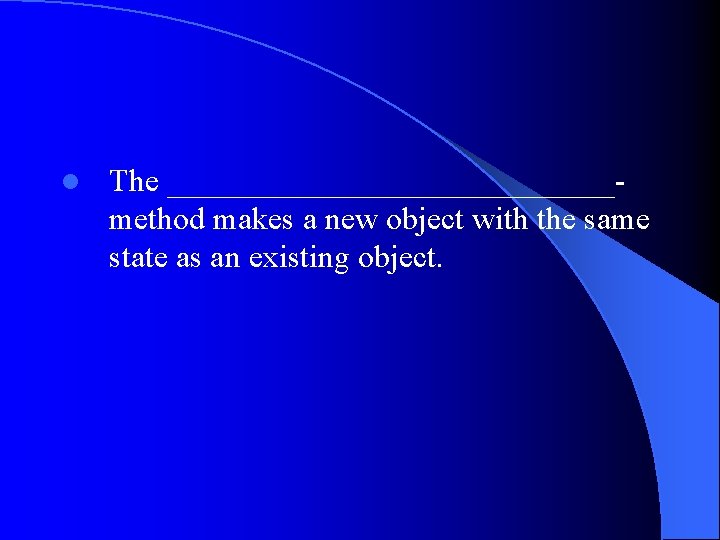
l The ______________method makes a new object with the same state as an existing object.
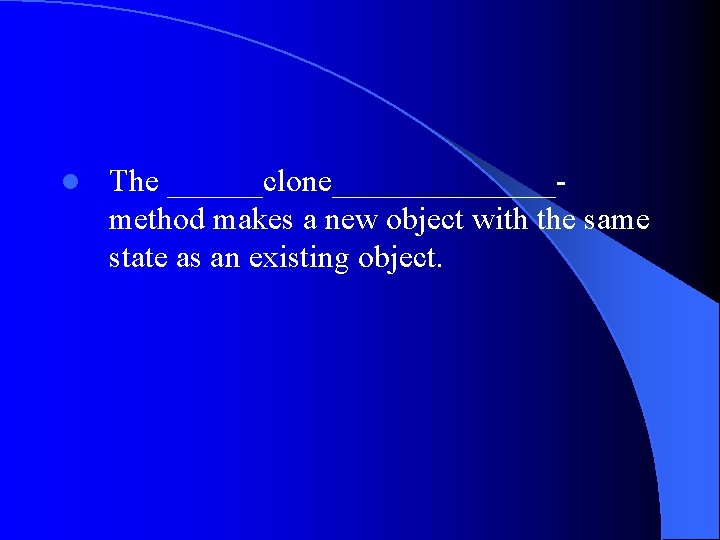
l The ______clone_______method makes a new object with the same state as an existing object.
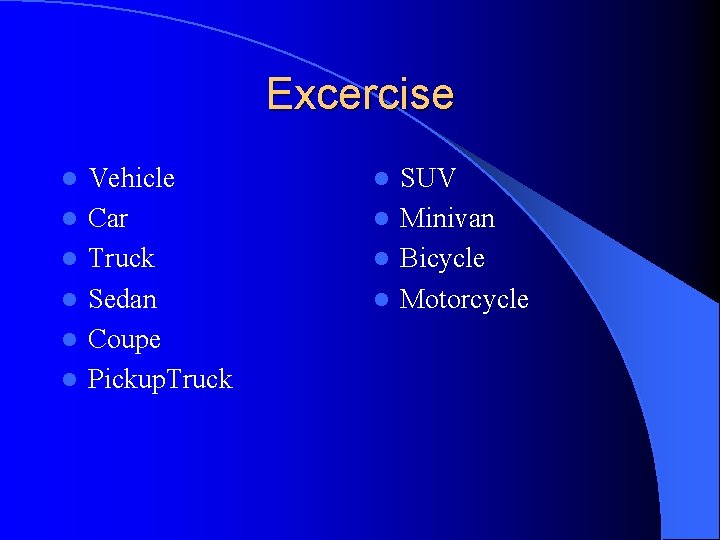
Excercise l l l Vehicle Car Truck Sedan Coupe Pickup. Truck SUV l Minivan l Bicycle l Motorcycle l
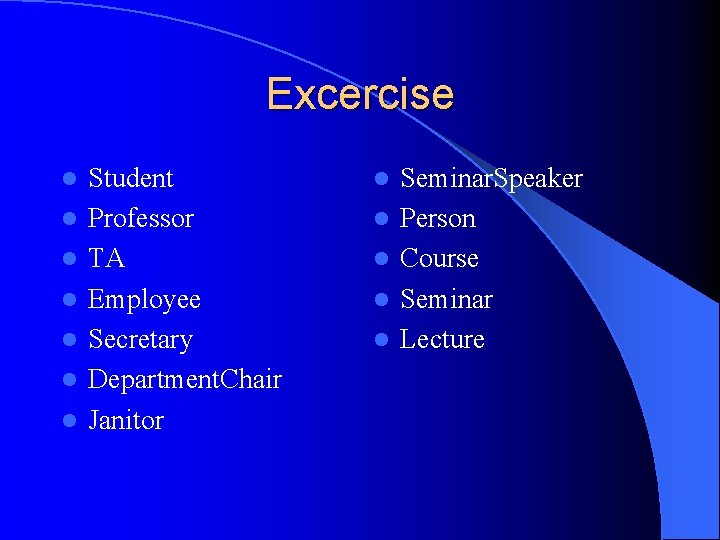
Excercise l l l l Student Professor TA Employee Secretary Department. Chair Janitor l l l Seminar. Speaker Person Course Seminar Lecture
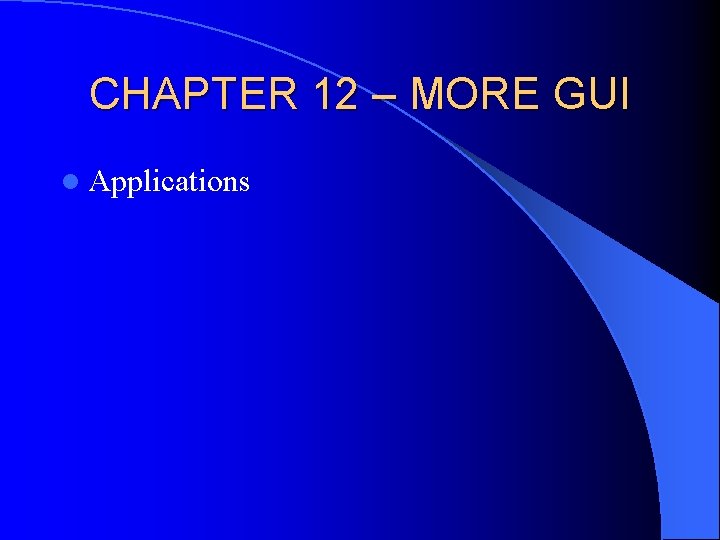
CHAPTER 12 – MORE GUI l Applications
![FRAME BASED APPLICATION public static void mainString args JFrame app Frame new FRAME BASED APPLICATION public static void main(String[] args) { JFrame app. Frame = new](https://slidetodoc.com/presentation_image_h2/f4c171615104fa562512d9a8f53b7959/image-47.jpg)
FRAME BASED APPLICATION public static void main(String[] args) { JFrame app. Frame = new JFrame(); app. Frame. set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE); app. Frame. set. Content. Pane(rect. Panel); app. Frame. pack(); app. Frame. show(); }
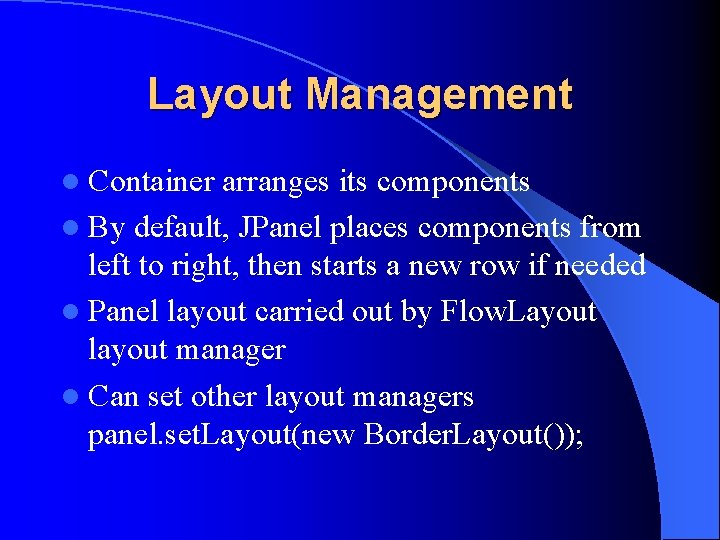
Layout Management l Container arranges its components l By default, JPanel places components from left to right, then starts a new row if needed l Panel layout carried out by Flow. Layout layout manager l Can set other layout managers panel. set. Layout(new Border. Layout());
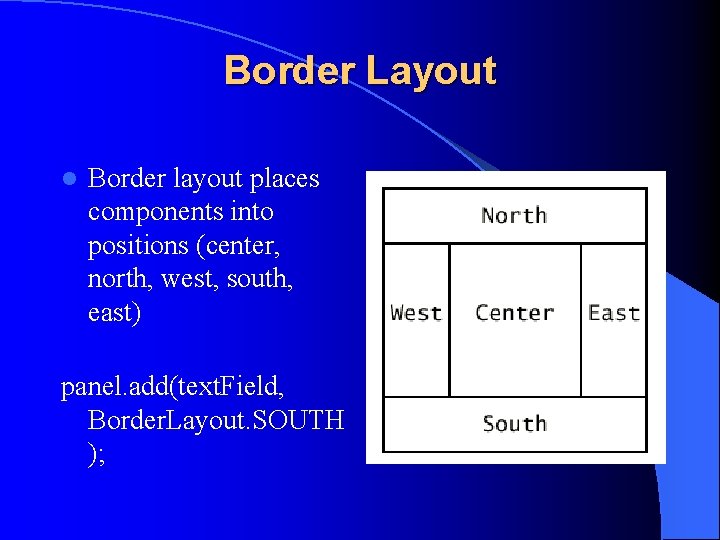
Border Layout l Border layout places components into positions (center, north, west, south, east) panel. add(text. Field, Border. Layout. SOUTH );
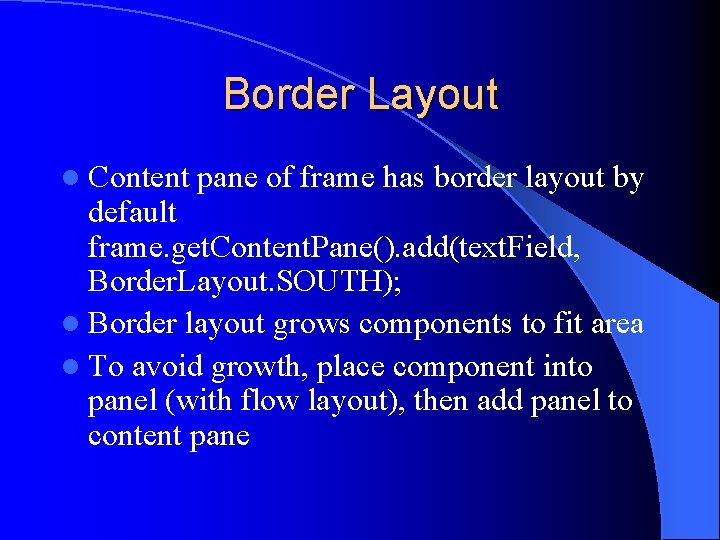
Border Layout l Content pane of frame has border layout by default frame. get. Content. Pane(). add(text. Field, Border. Layout. SOUTH); l Border layout grows components to fit area l To avoid growth, place component into panel (with flow layout), then add panel to content pane
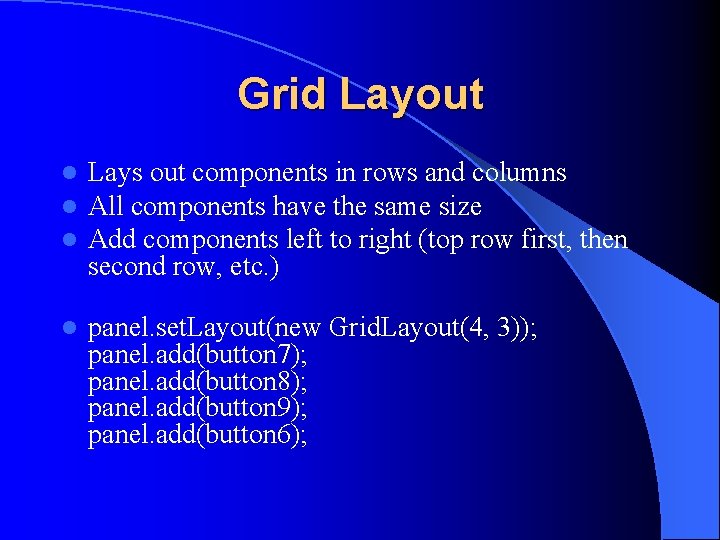
Grid Layout l l l Lays out components in rows and columns All components have the same size Add components left to right (top row first, then second row, etc. ) l panel. set. Layout(new Grid. Layout(4, 3)); panel. add(button 7); panel. add(button 8); panel. add(button 9); panel. add(button 6);
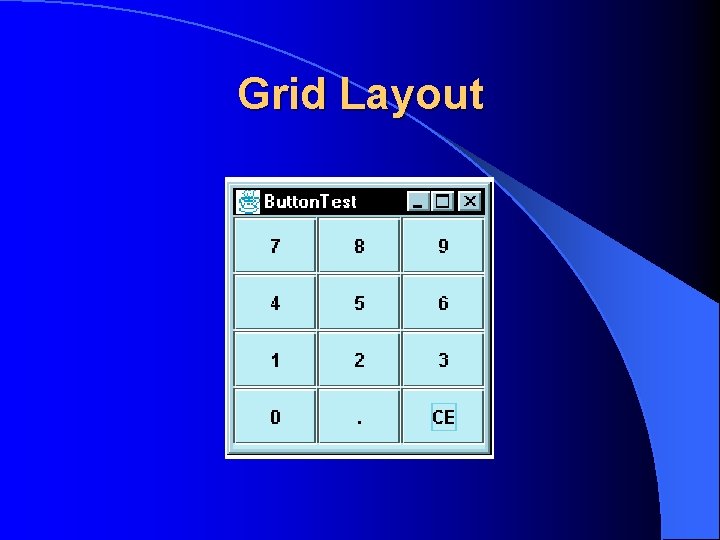
Grid Layout
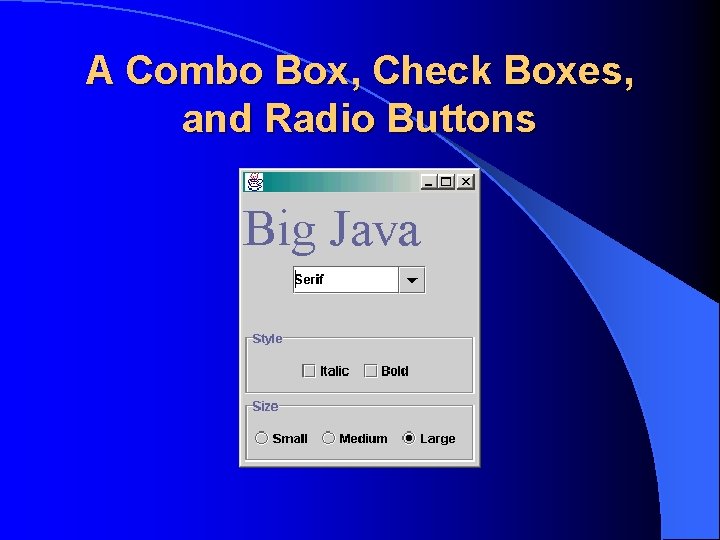
A Combo Box, Check Boxes, and Radio Buttons
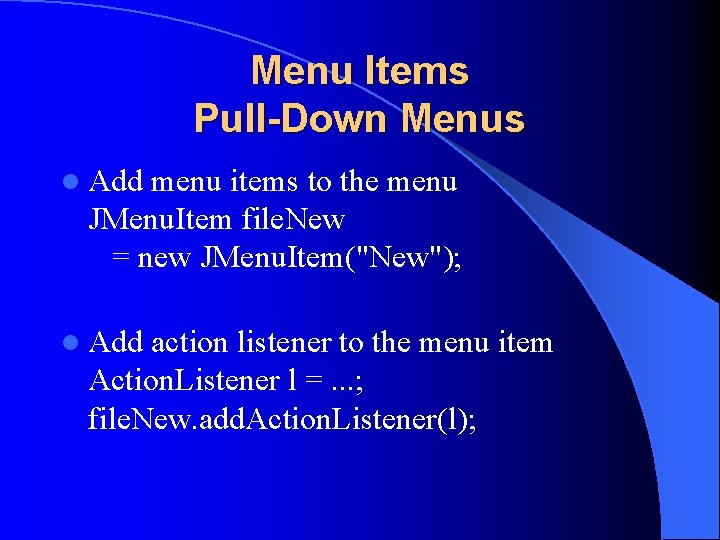
Menu Items Pull-Down Menus l Add menu items to the menu JMenu. Item file. New = new JMenu. Item("New"); l Add action listener to the menu item Action. Listener l =. . . ; file. New. add. Action. Listener(l);
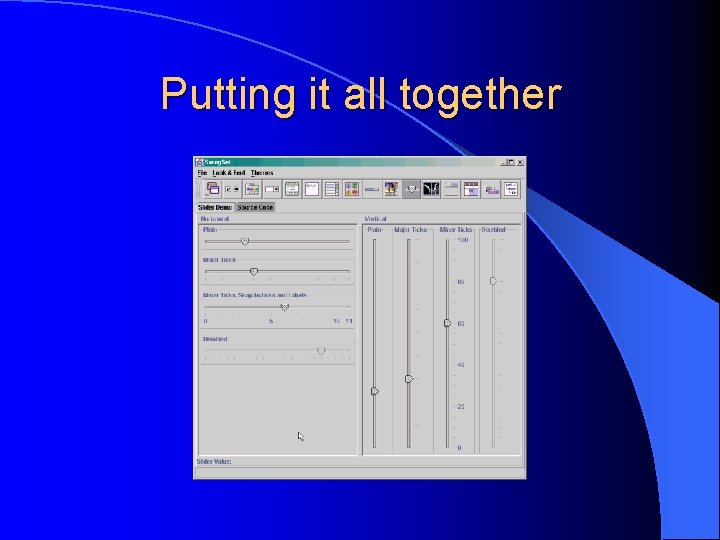
Putting it all together
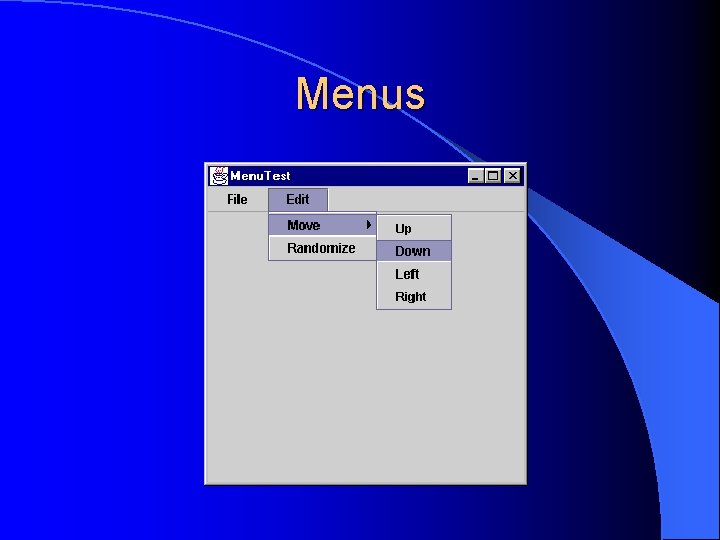
Menus
01:640:244 lecture notes - lecture 15: plat, idah, farad
Pg 171
29052007
The verbs salir decir and venir p 155 answers
Nonlinear pricing example
Ai 171
Ai 171
171 meaning
Informasiya prosesleri
171 nomreli mekteb
Decret 171/2015
Dot
Cs 171 uci
171 in binary
171 sayli mekteb
171 nomreli mekteb
Physics 20 final exam practice
Physics fall semester exam review
U.s. history semester 1 final exam
English 3 fall semester exam review
Situational irony def
World history semester exam
World history semester 1 final exam study guide answers
Chapter review motion part a vocabulary review answer key
Ap gov final review
Narrative review vs systematic review
Search strategy example
Narrative review vs systematic review
Transmission ciblée aide soignante exemple
Pearson education
Richard murray caltech
Tabel rh
T. trimpe 2004 http //sciencespot.net/
Dgc 2004
2004 dress code
Composition of grievance committee deped
Sworn statement for esales registration
Legge urbanistica regione campania 16/2004
Permenkes 33 tahun 2015
Perbedaan kurikulum 2004 dan 2013
2004 ford ranger 2.3 firing order
Age discrimination act 2004
Ley idea 2004
Victoria climbie timeline
Lancaster 2004
Age discrimination act 2004
Directorio general para la catequesis
Hg 974 din 2004
Landasan kurikulum 2004
2004-1948
Circolare miur 4099/a/4 2004
Clara morgane 2004
En 10204: 2004
Bildungsplan 2004
The age discrimination act 2004
Ordinul 154 din 2004
Xxxxxxxx 17