Inheritance CSC 171 FALL 2004 LECTURE 18 READING
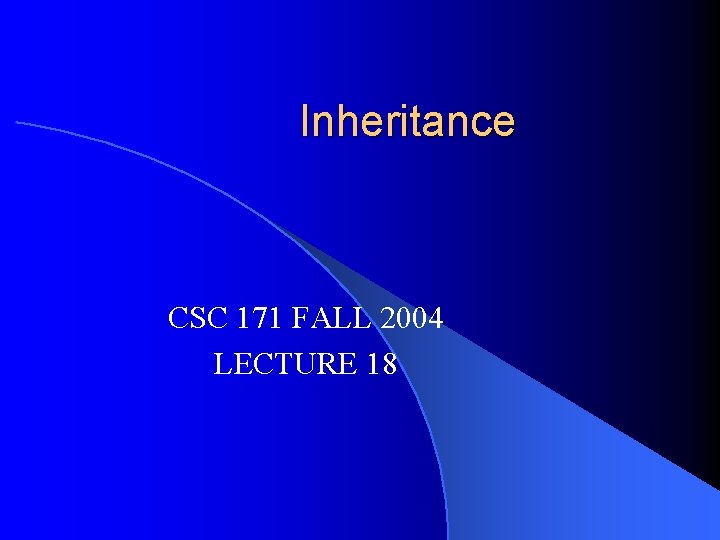
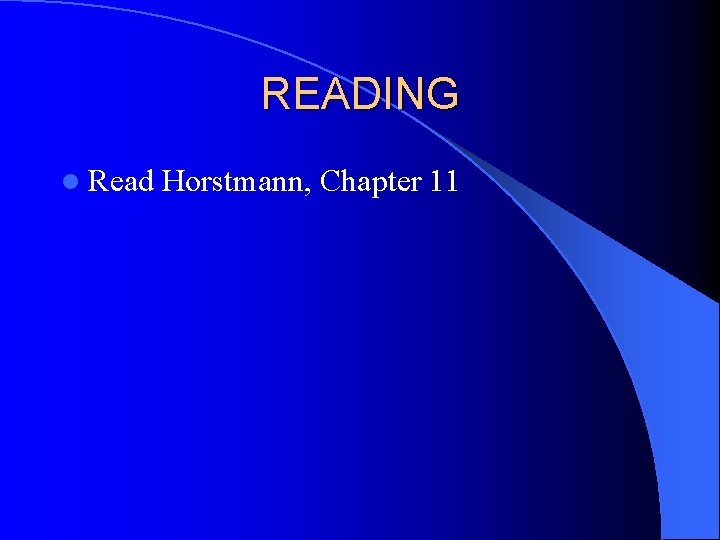
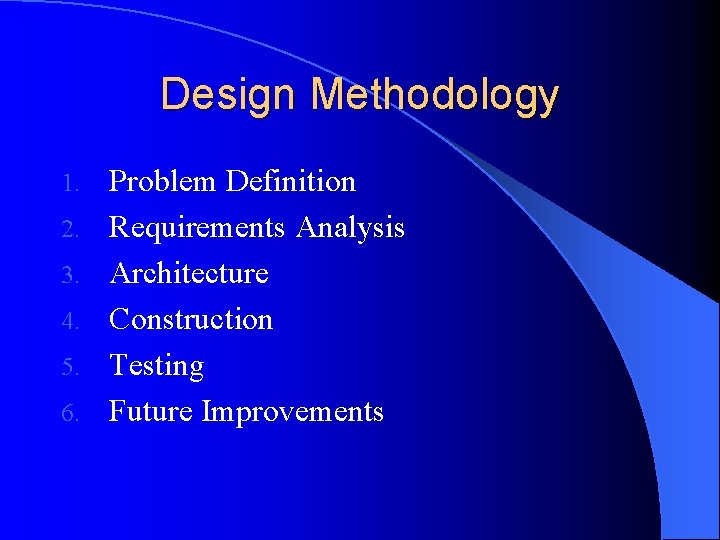
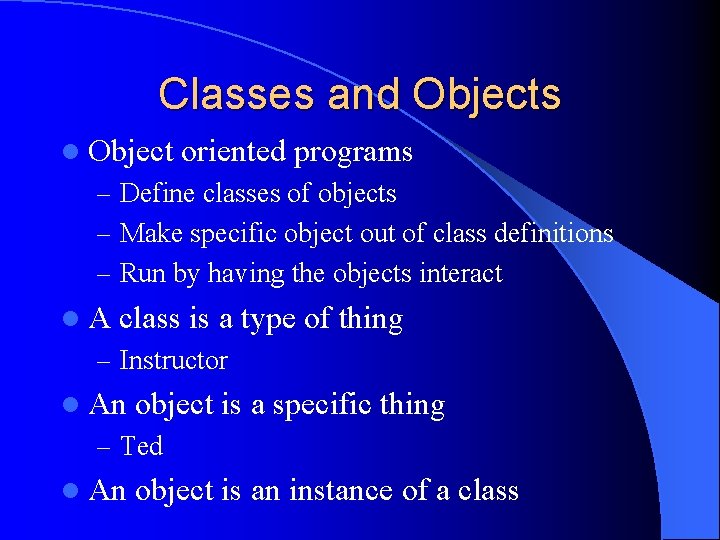
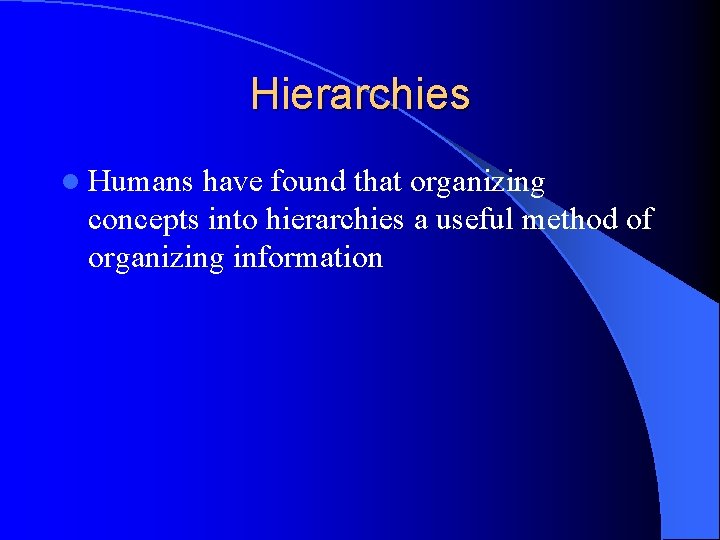
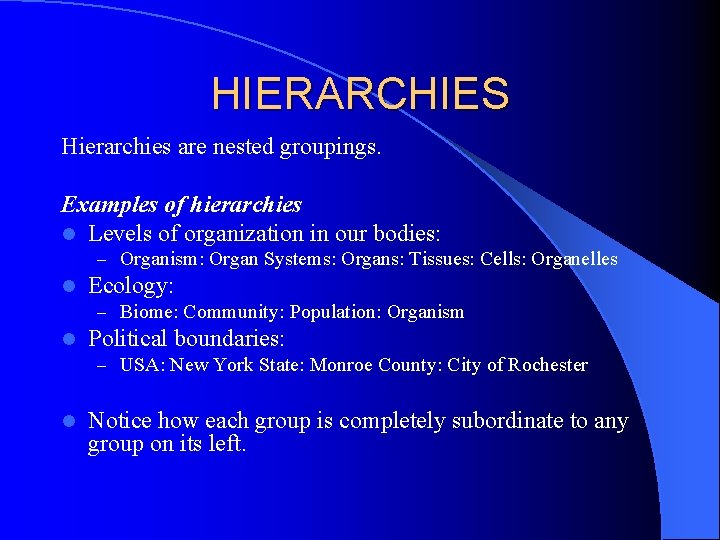
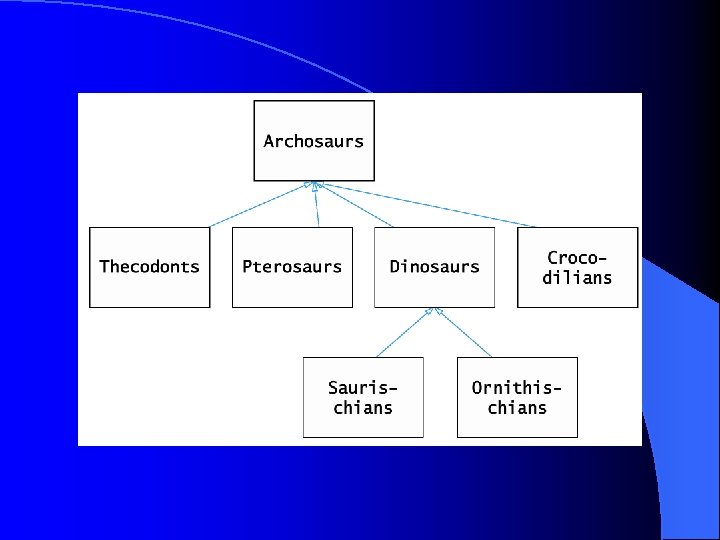
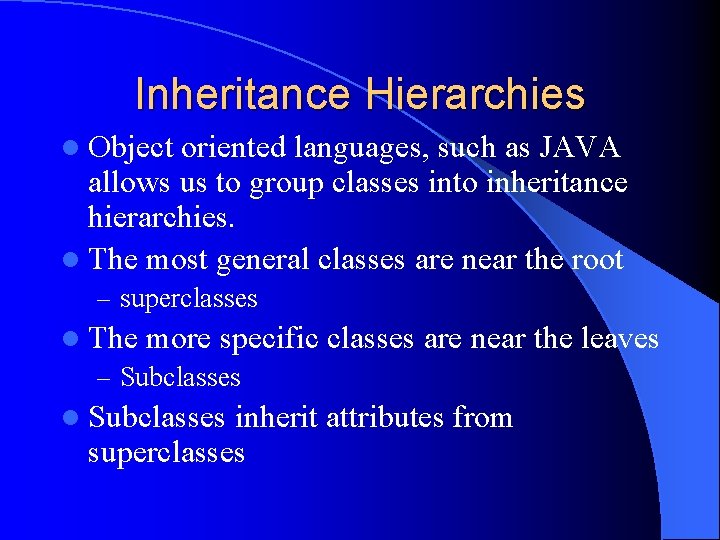
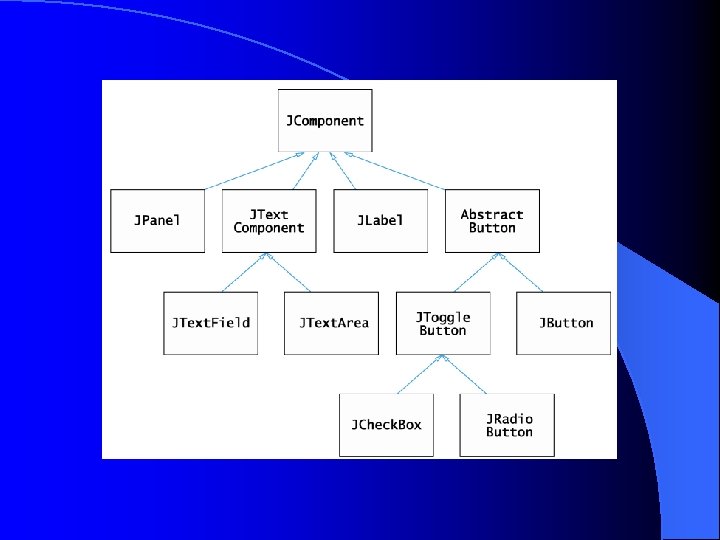
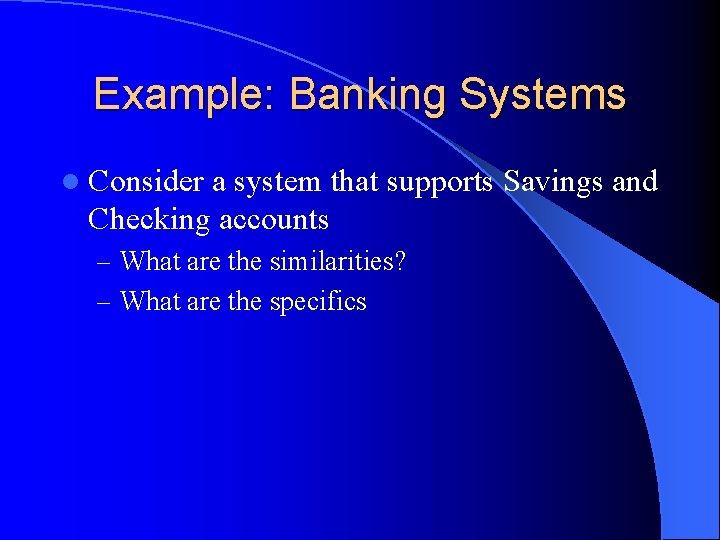
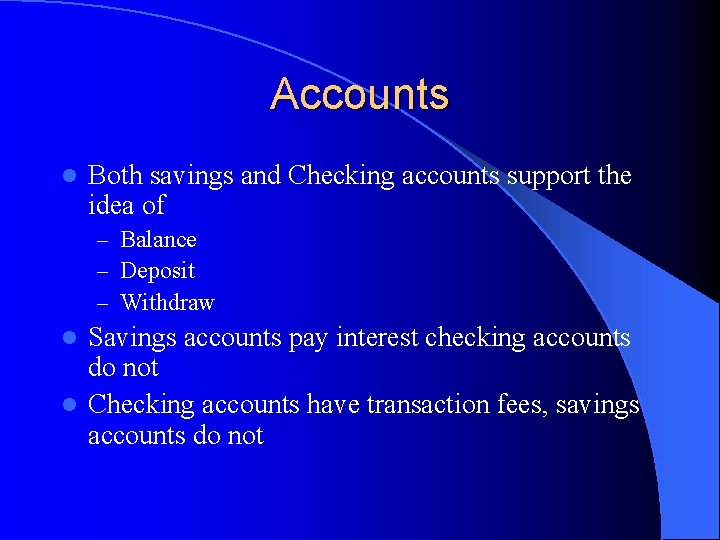
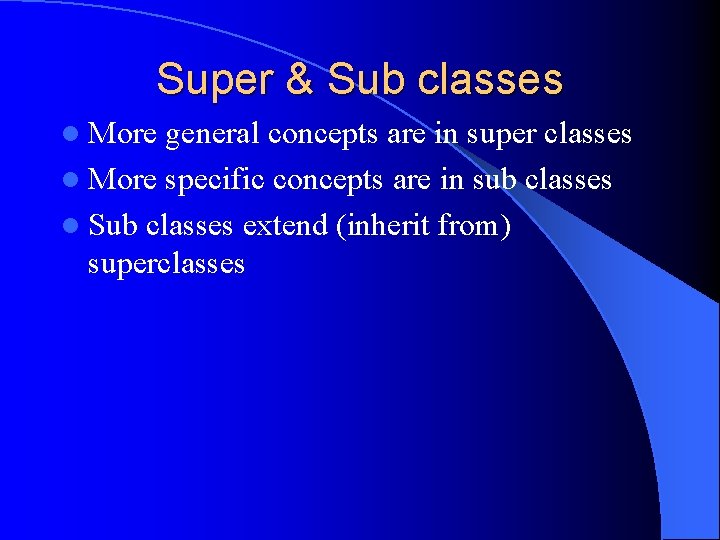
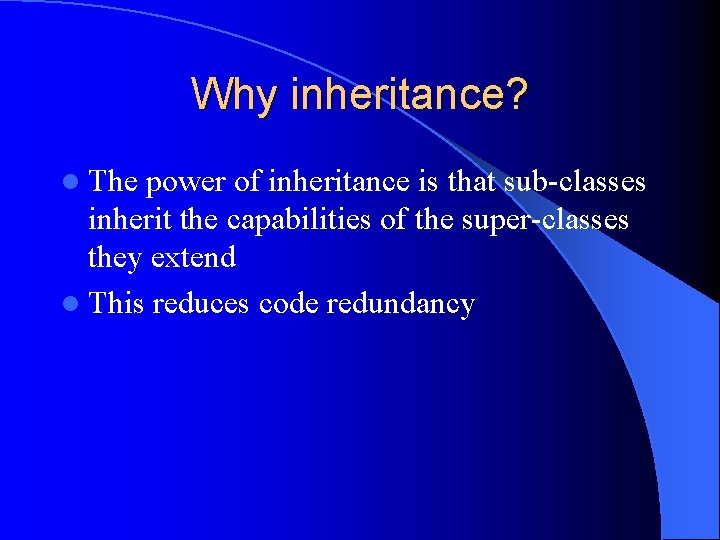
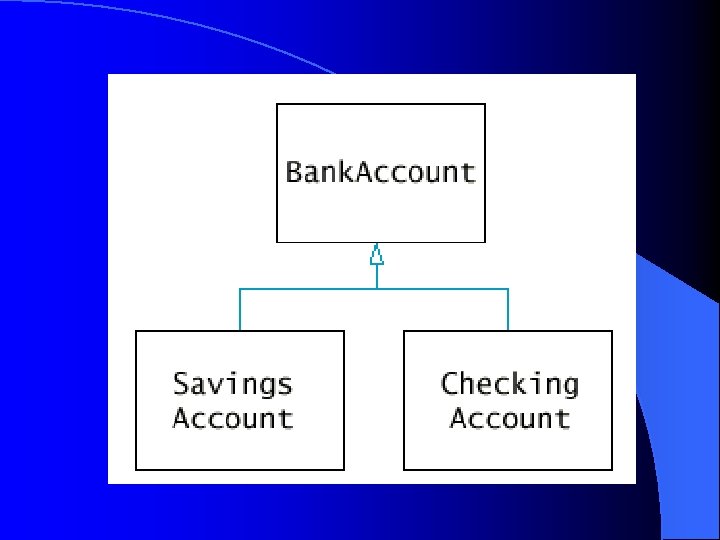
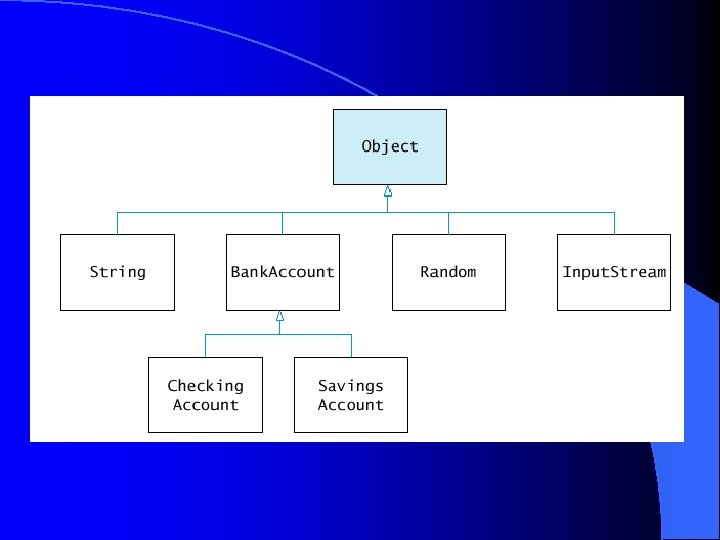
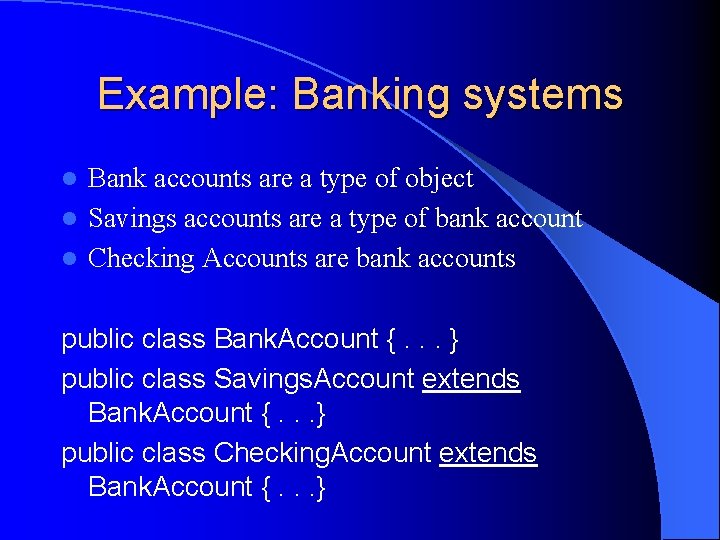
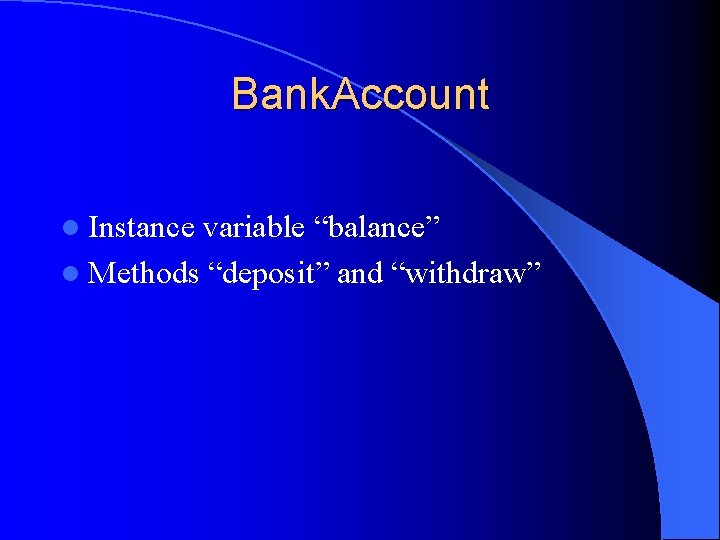
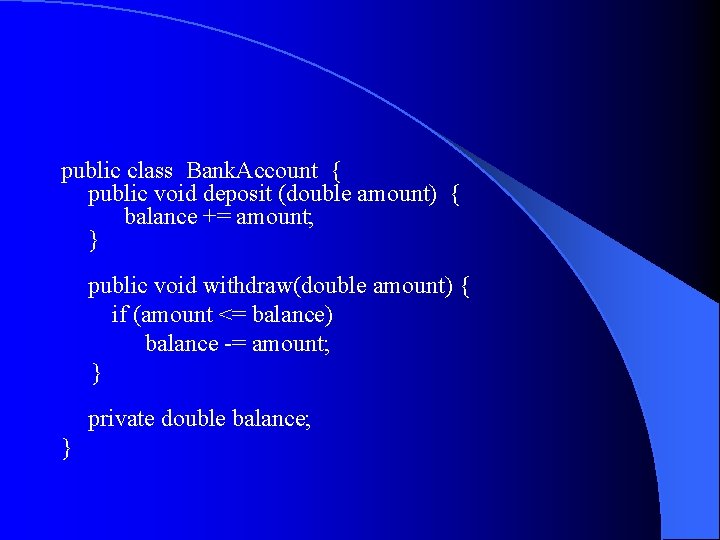
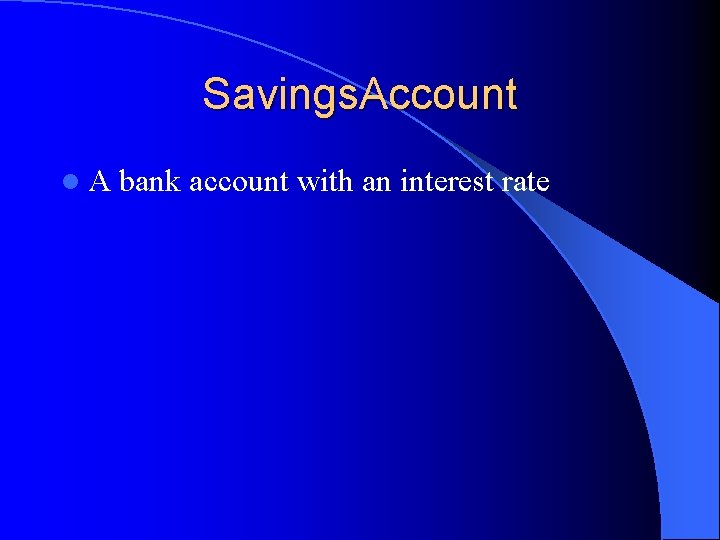
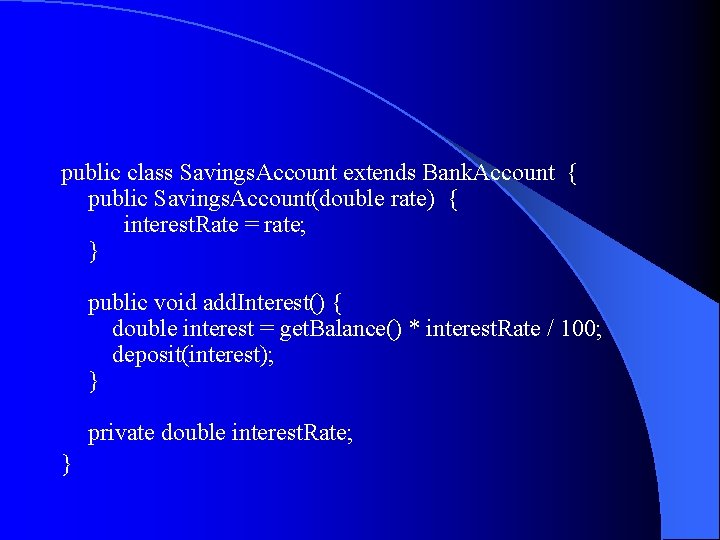
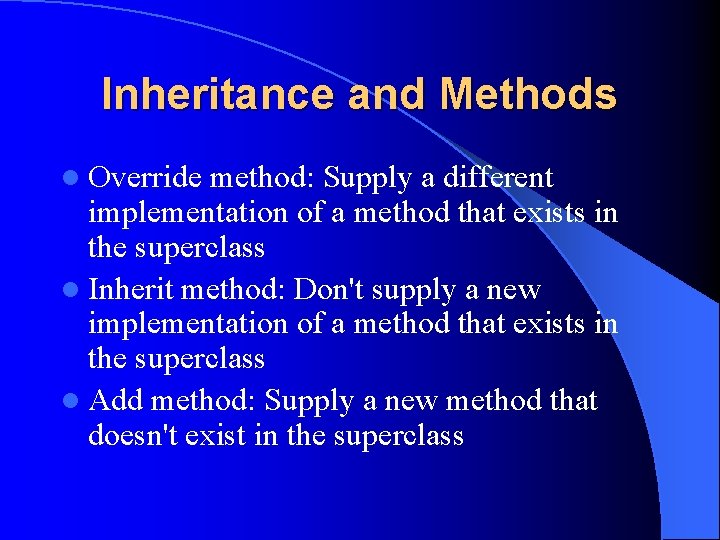
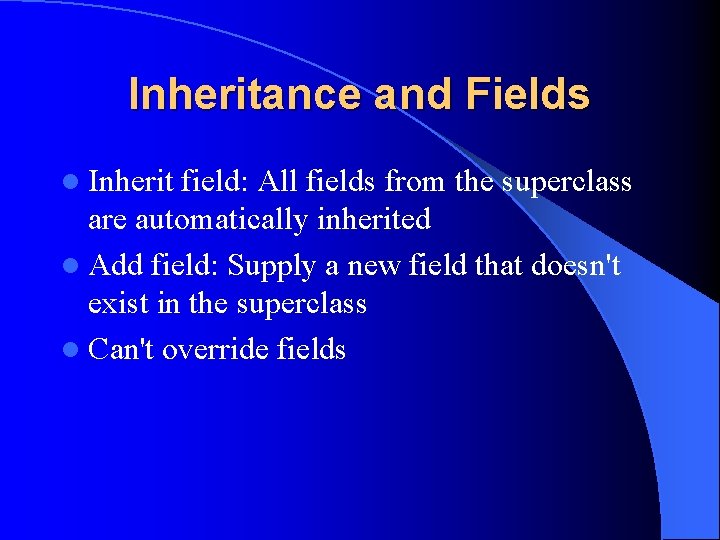
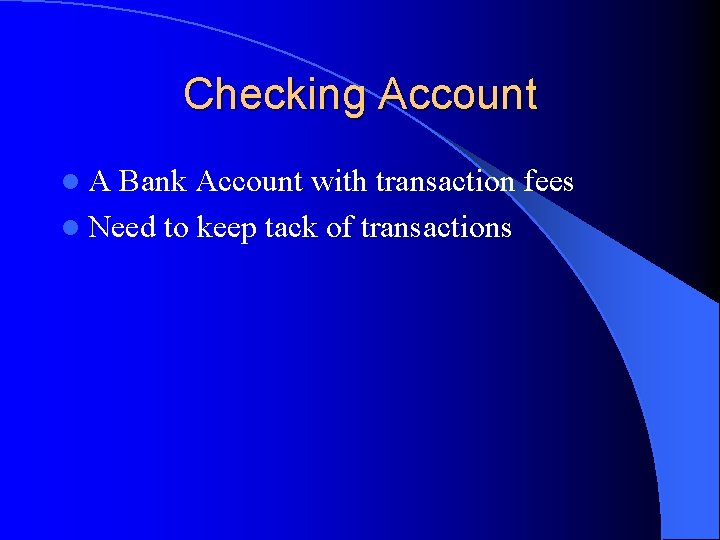
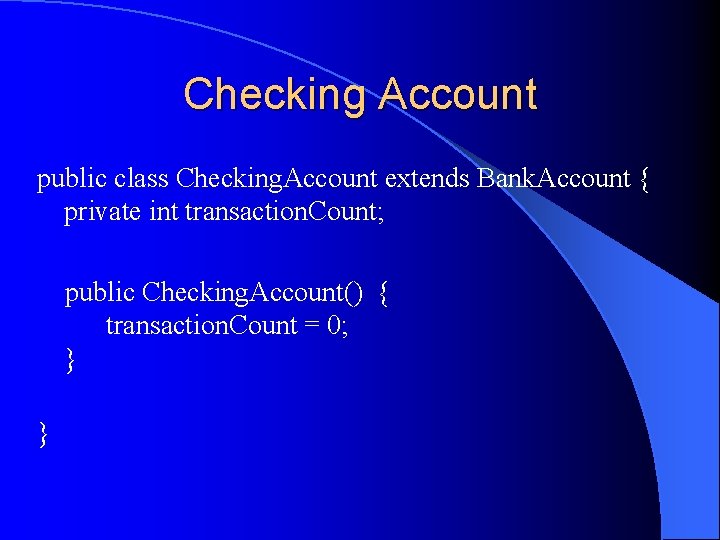
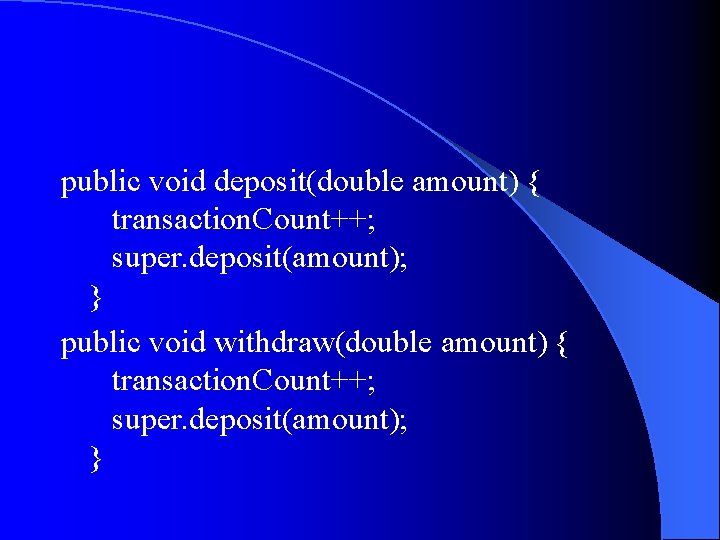
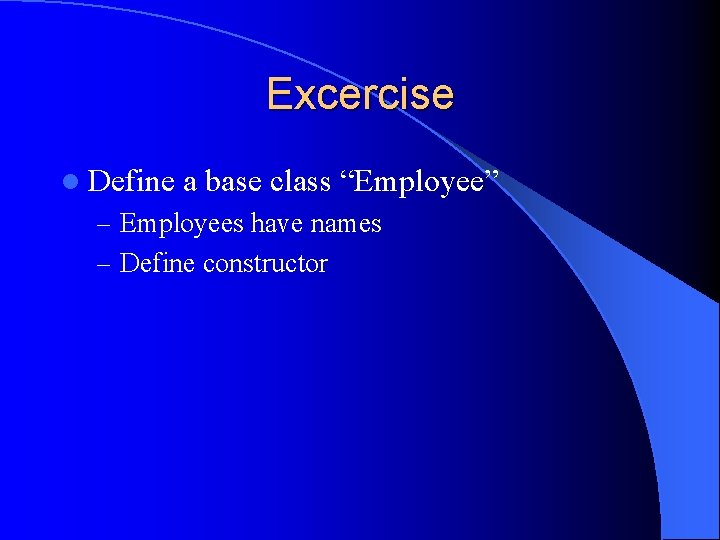
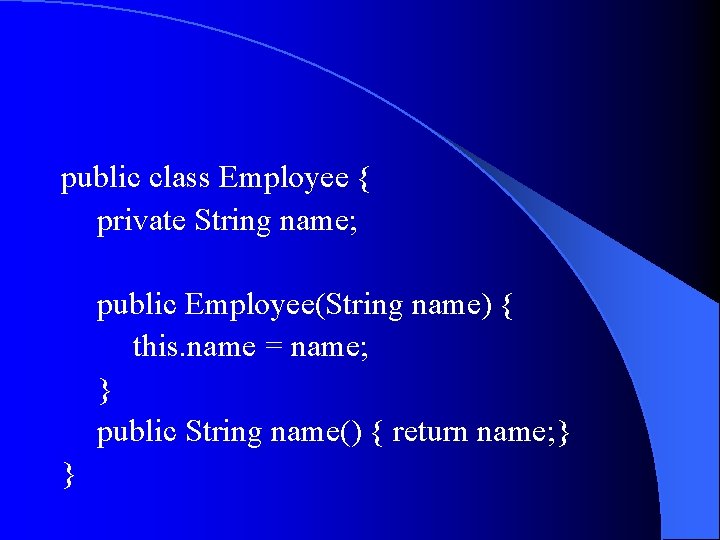
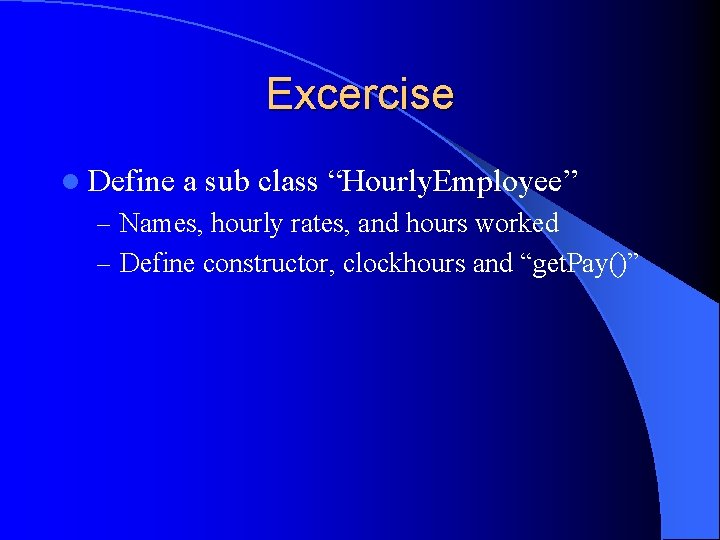
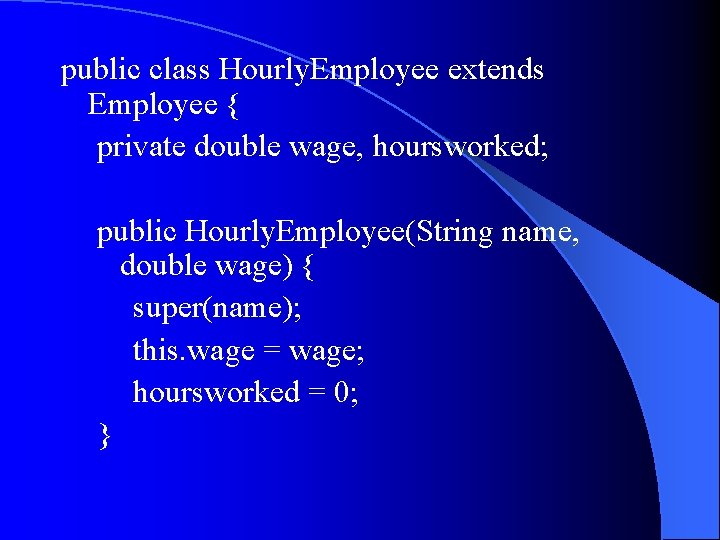
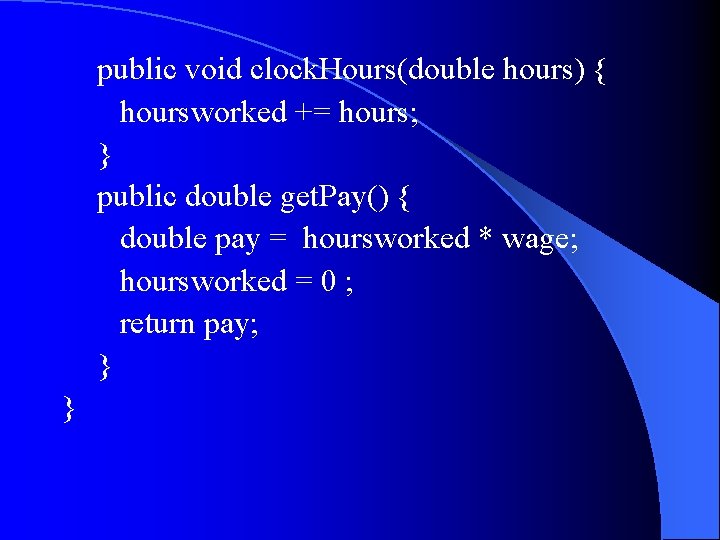
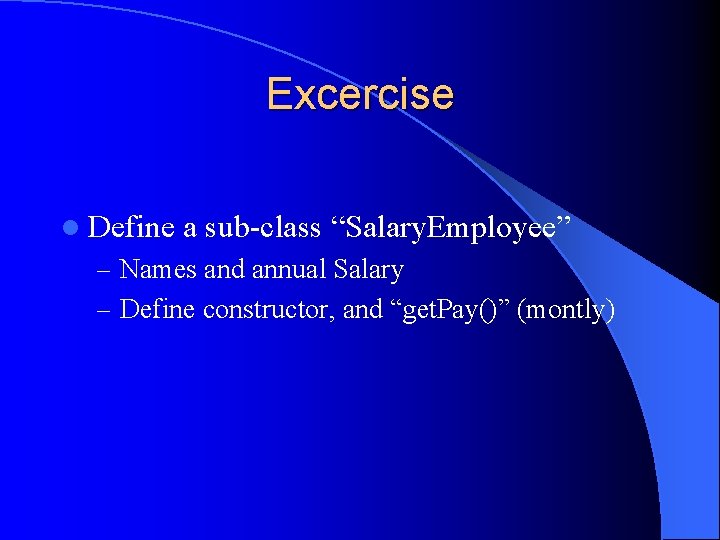
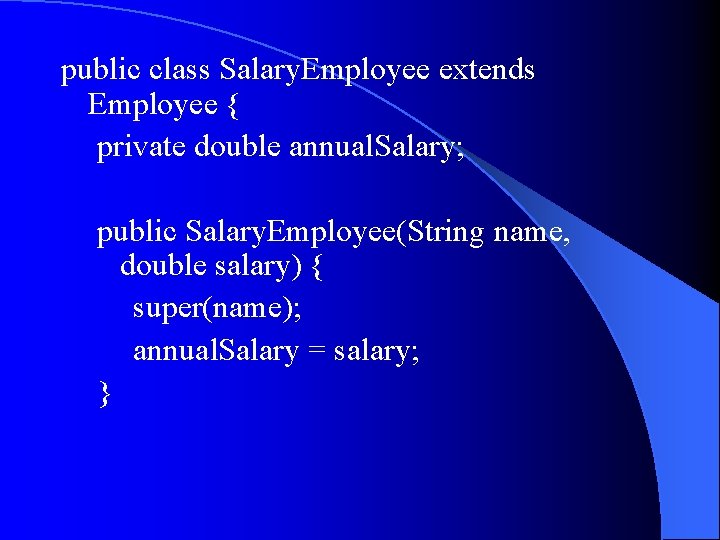
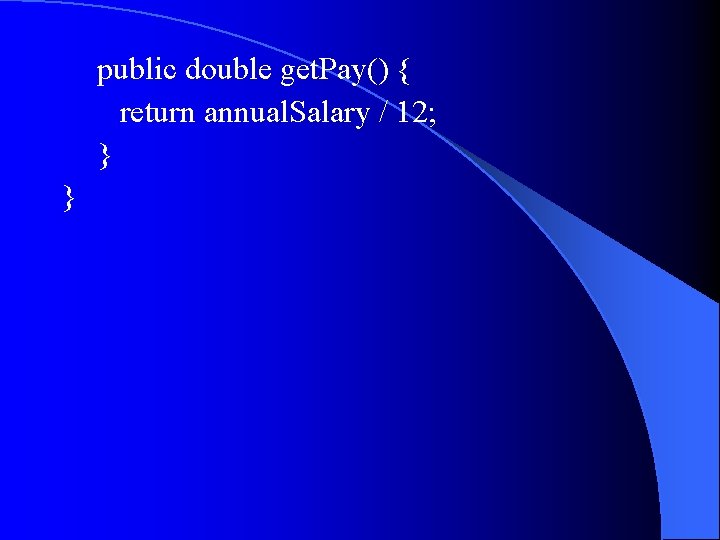
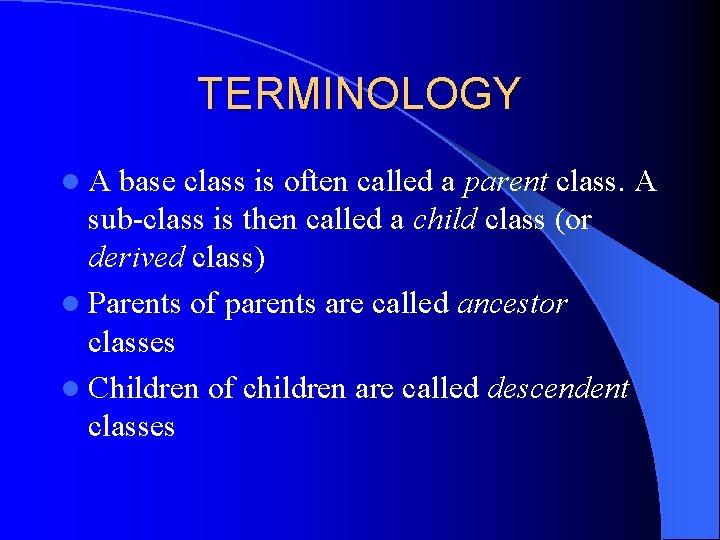
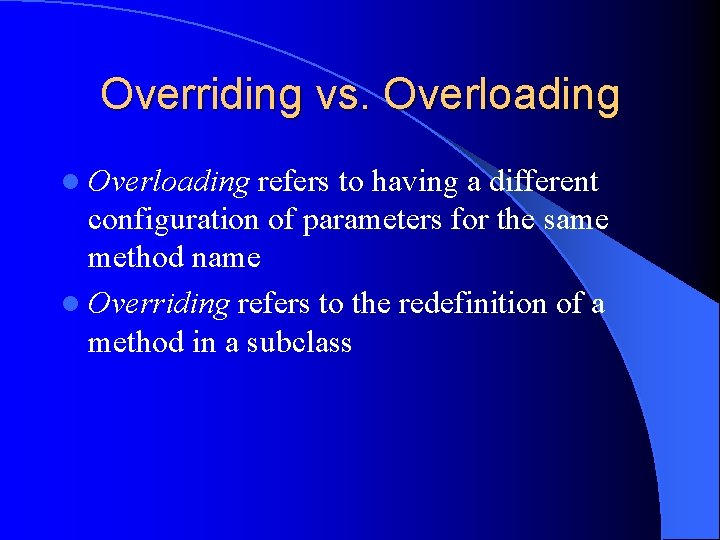
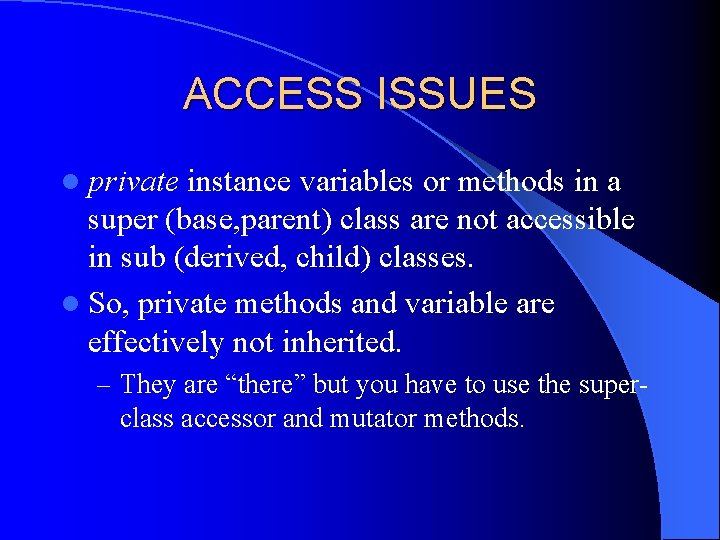
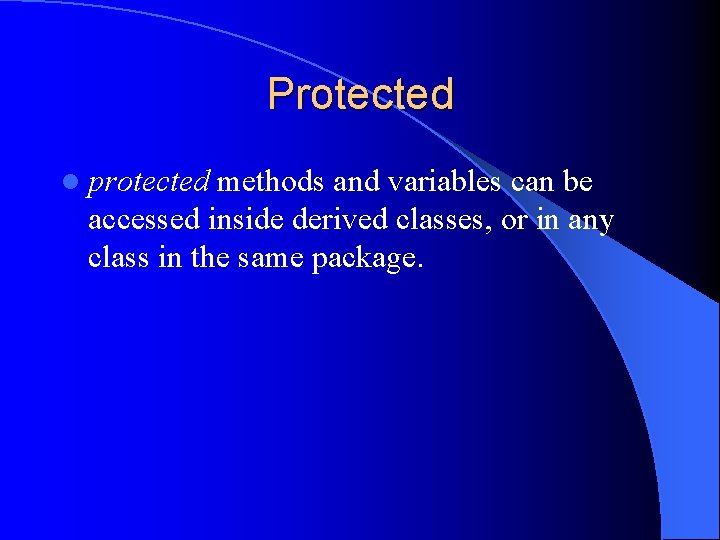
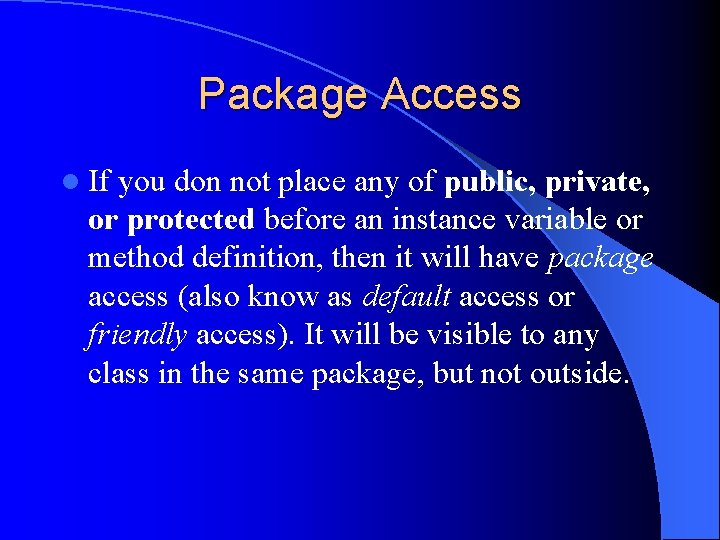
- Slides: 38
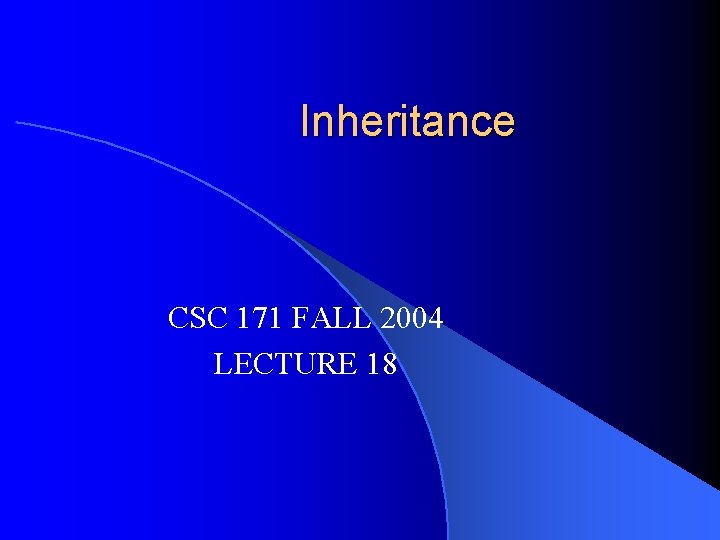
Inheritance CSC 171 FALL 2004 LECTURE 18
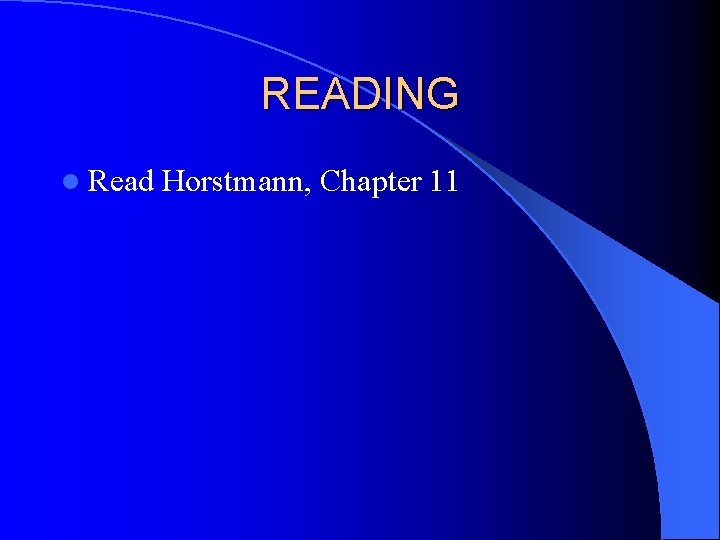
READING l Read Horstmann, Chapter 11
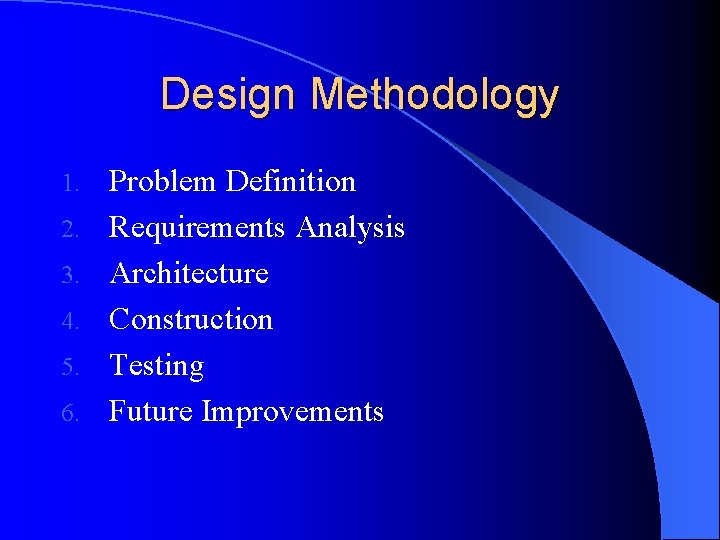
Design Methodology 1. 2. 3. 4. 5. 6. Problem Definition Requirements Analysis Architecture Construction Testing Future Improvements
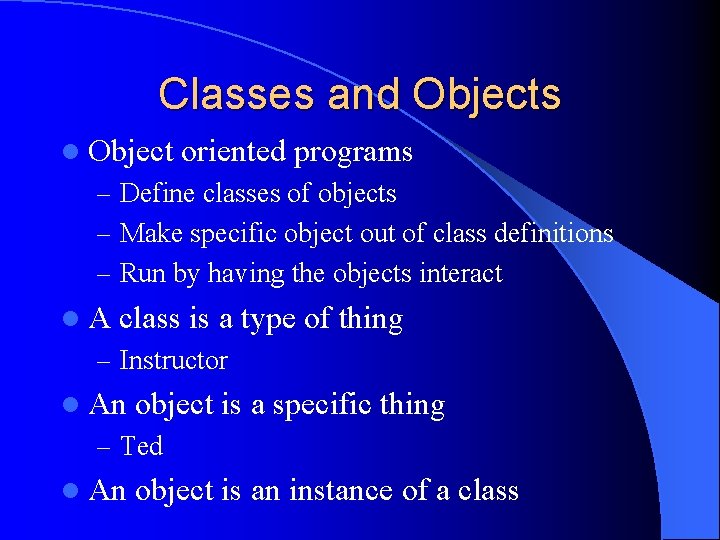
Classes and Objects l Object oriented programs – Define classes of objects – Make specific object out of class definitions – Run by having the objects interact l. A class is a type of thing – Instructor l An object is a specific thing – Ted l An object is an instance of a class
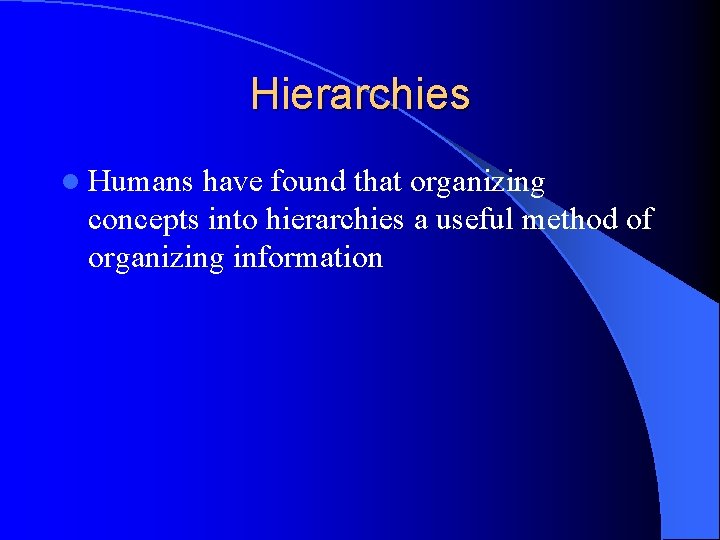
Hierarchies l Humans have found that organizing concepts into hierarchies a useful method of organizing information
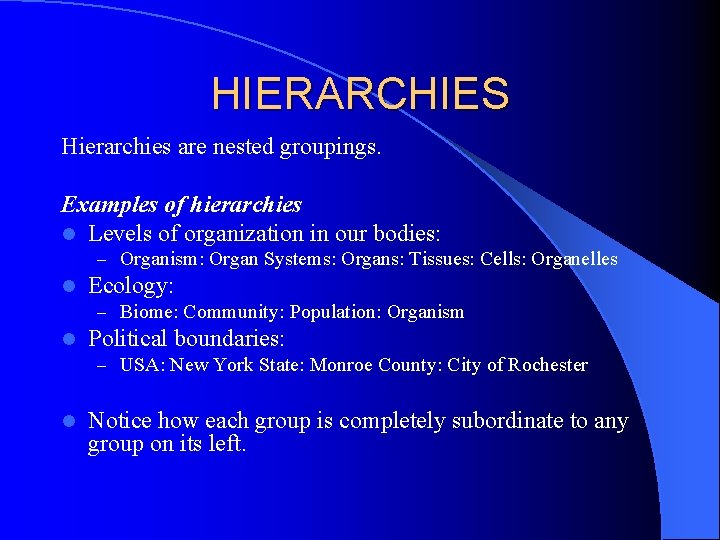
HIERARCHIES Hierarchies are nested groupings. Examples of hierarchies l Levels of organization in our bodies: – Organism: Organ Systems: Organs: Tissues: Cells: Organelles l Ecology: – Biome: Community: Population: Organism l Political boundaries: – USA: New York State: Monroe County: City of Rochester l Notice how each group is completely subordinate to any group on its left.
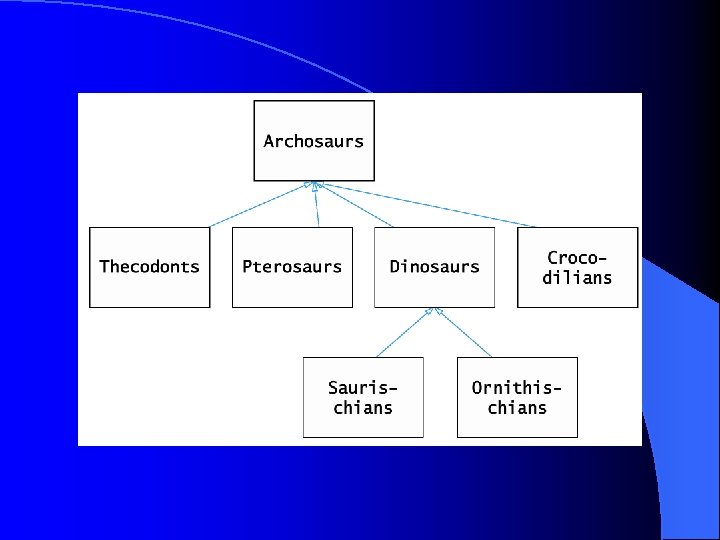
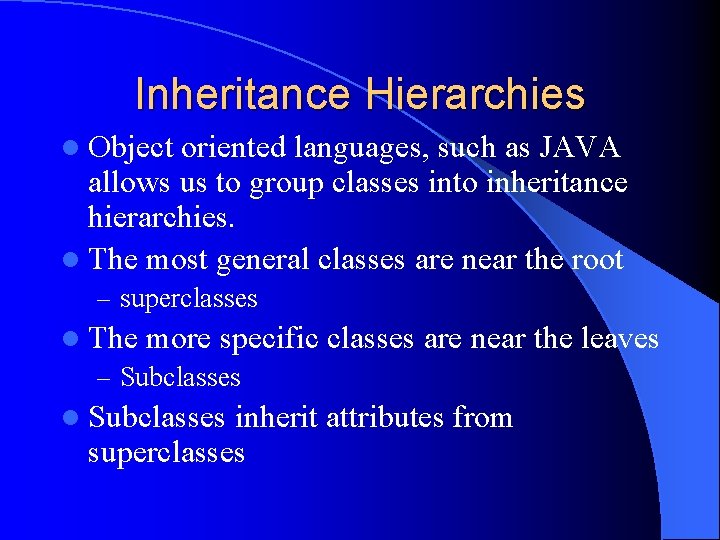
Inheritance Hierarchies l Object oriented languages, such as JAVA allows us to group classes into inheritance hierarchies. l The most general classes are near the root – superclasses l The more specific – Subclasses l Subclasses are near the leaves inherit attributes from superclasses
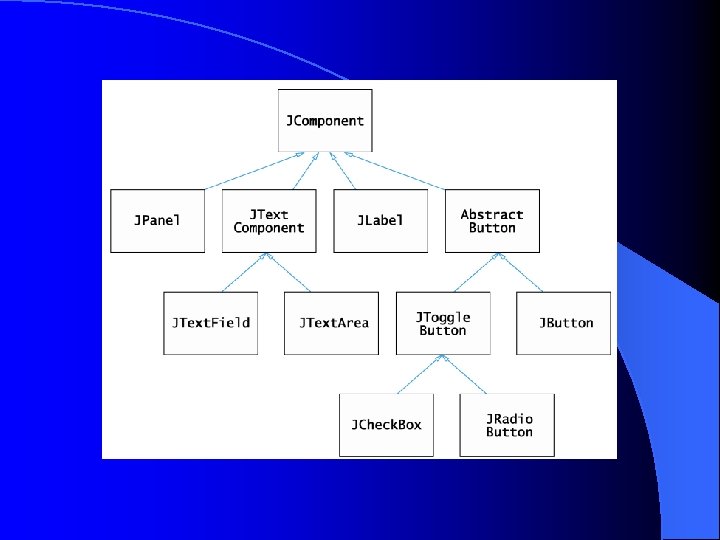
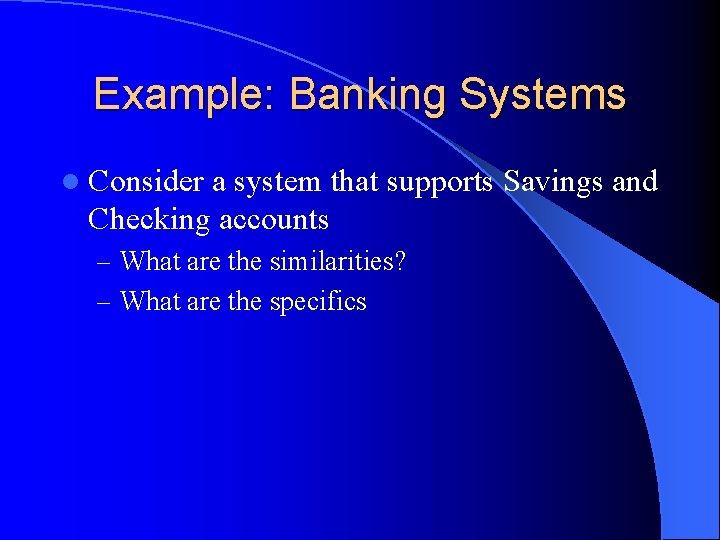
Example: Banking Systems l Consider a system that supports Savings and Checking accounts – What are the similarities? – What are the specifics
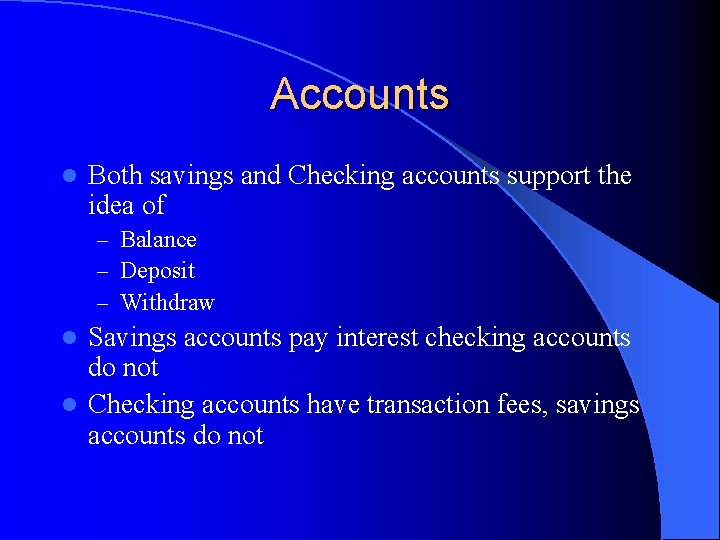
Accounts l Both savings and Checking accounts support the idea of – Balance – Deposit – Withdraw Savings accounts pay interest checking accounts do not l Checking accounts have transaction fees, savings accounts do not l
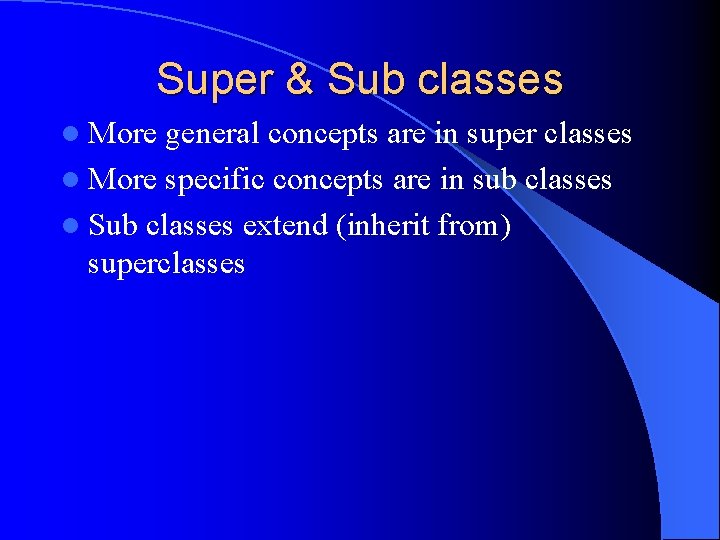
Super & Sub classes l More general concepts are in super classes l More specific concepts are in sub classes l Sub classes extend (inherit from) superclasses
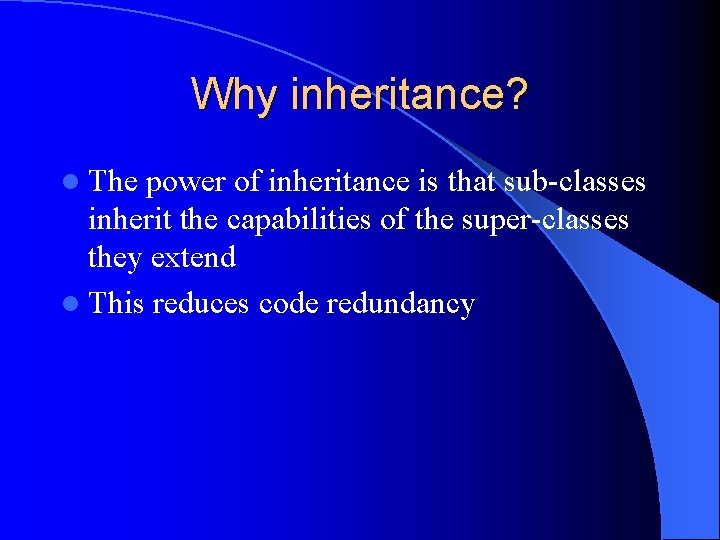
Why inheritance? l The power of inheritance is that sub-classes inherit the capabilities of the super-classes they extend l This reduces code redundancy
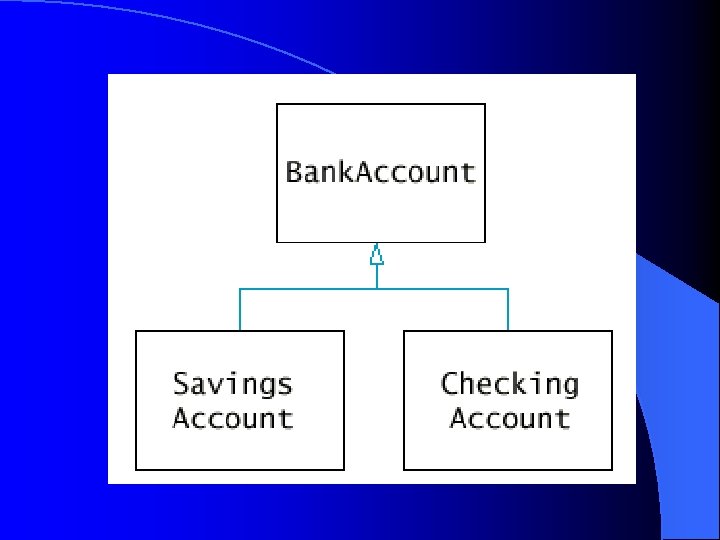
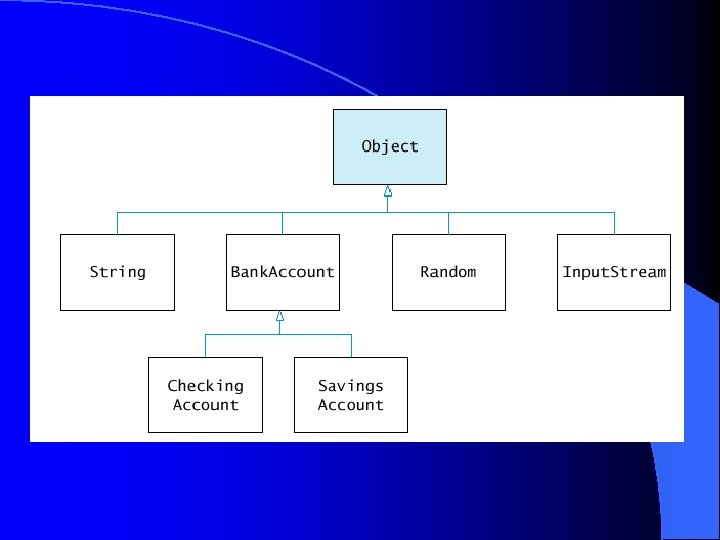
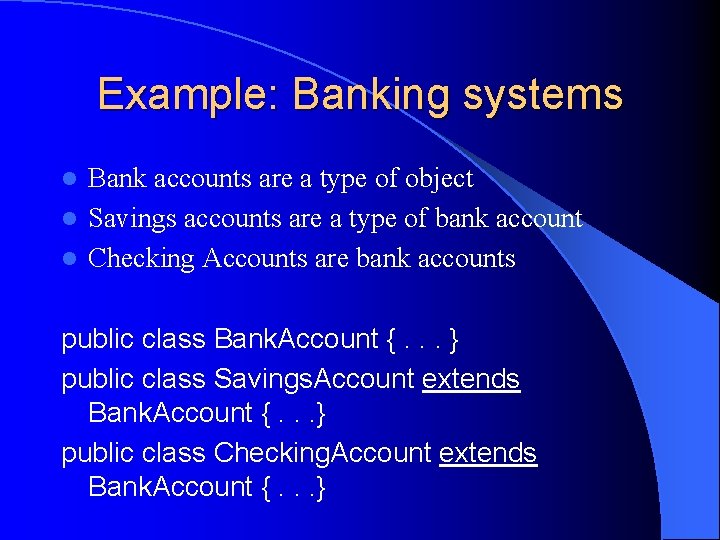
Example: Banking systems Bank accounts are a type of object l Savings accounts are a type of bank account l Checking Accounts are bank accounts l public class Bank. Account {. . . } public class Savings. Account extends Bank. Account {. . . } public class Checking. Account extends Bank. Account {. . . }
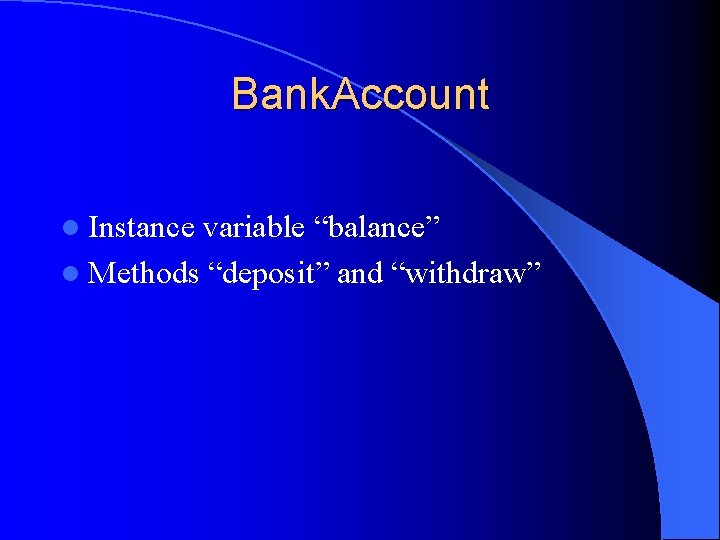
Bank. Account l Instance variable “balance” l Methods “deposit” and “withdraw”
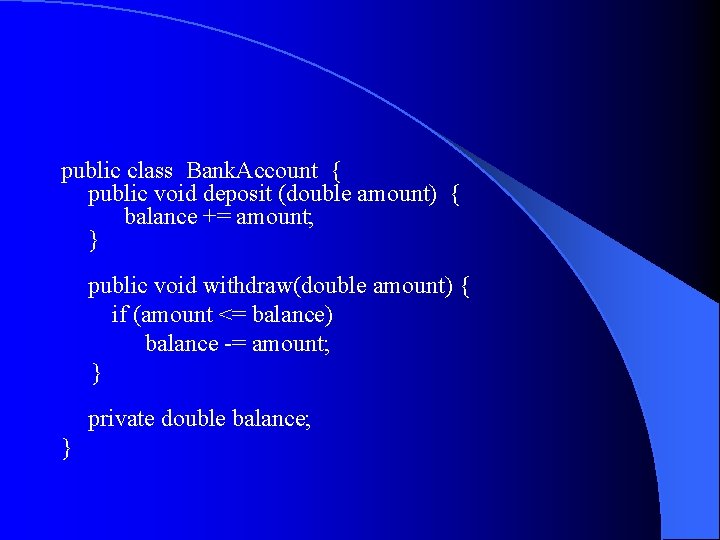
public class Bank. Account { public void deposit (double amount) { balance += amount; } public void withdraw(double amount) { if (amount <= balance) balance -= amount; } private double balance; }
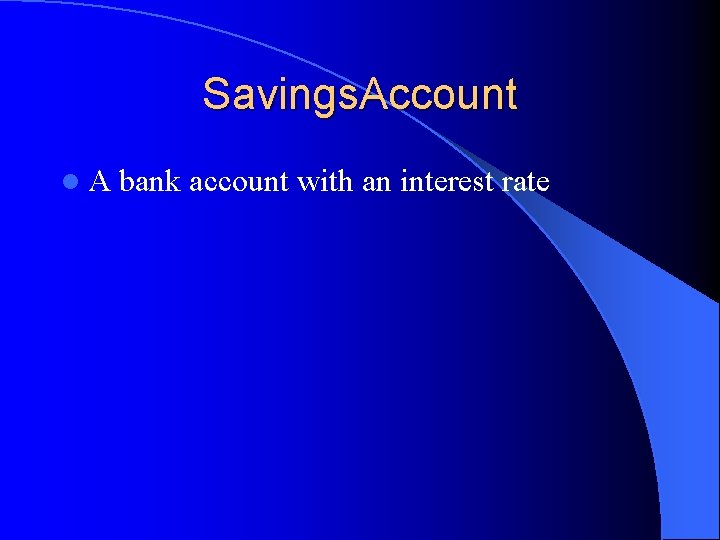
Savings. Account l. A bank account with an interest rate
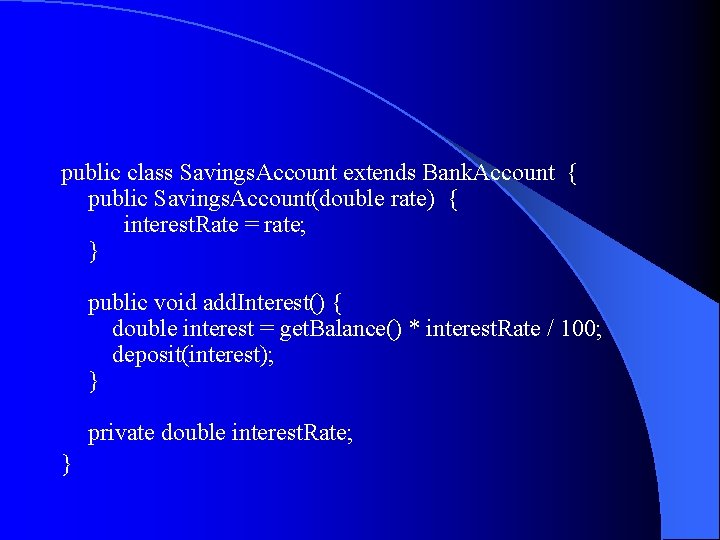
public class Savings. Account extends Bank. Account { public Savings. Account(double rate) { interest. Rate = rate; } public void add. Interest() { double interest = get. Balance() * interest. Rate / 100; deposit(interest); } private double interest. Rate; }
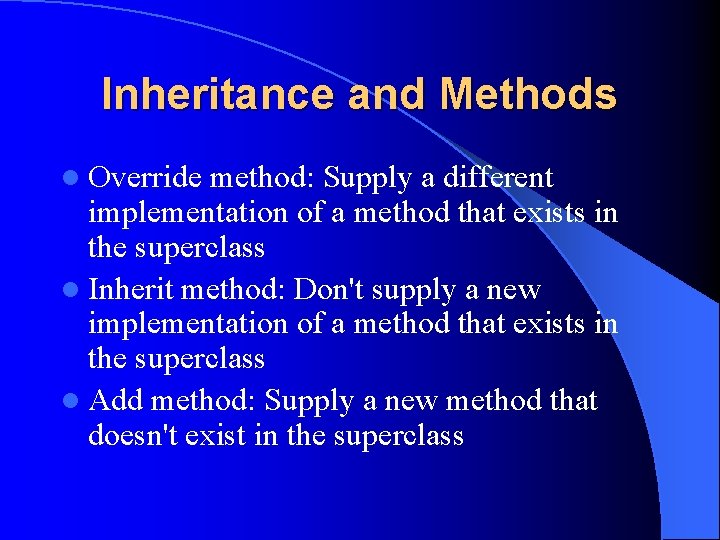
Inheritance and Methods l Override method: Supply a different implementation of a method that exists in the superclass l Inherit method: Don't supply a new implementation of a method that exists in the superclass l Add method: Supply a new method that doesn't exist in the superclass
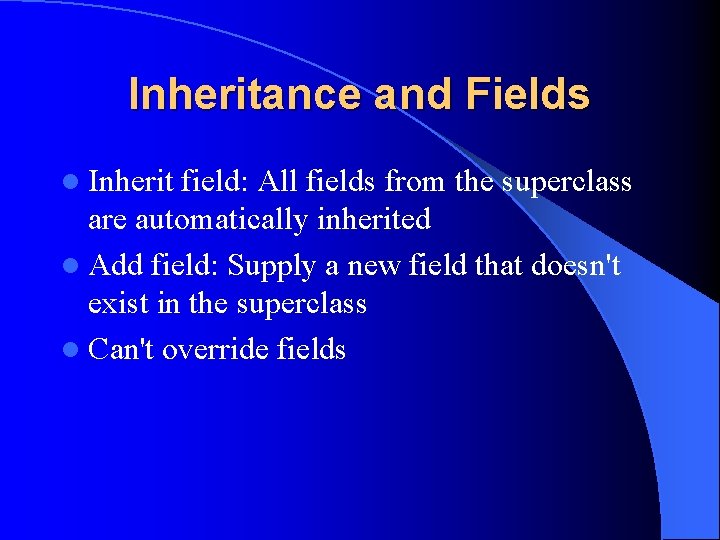
Inheritance and Fields l Inherit field: All fields from the superclass are automatically inherited l Add field: Supply a new field that doesn't exist in the superclass l Can't override fields
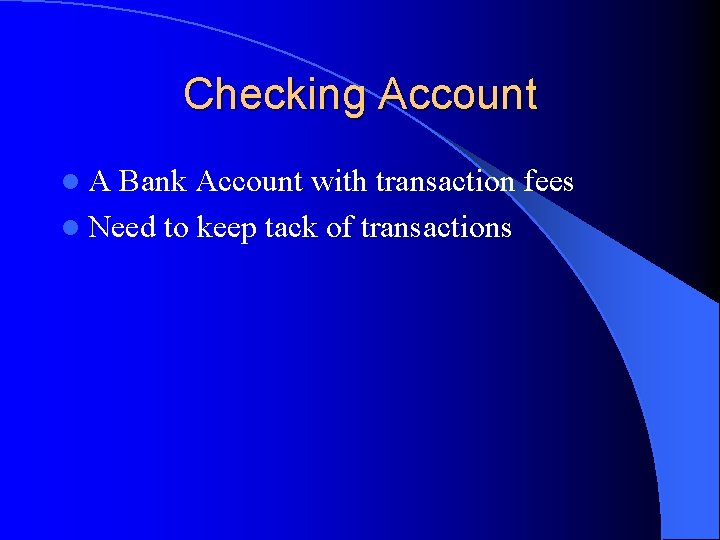
Checking Account l. A Bank Account with transaction fees l Need to keep tack of transactions
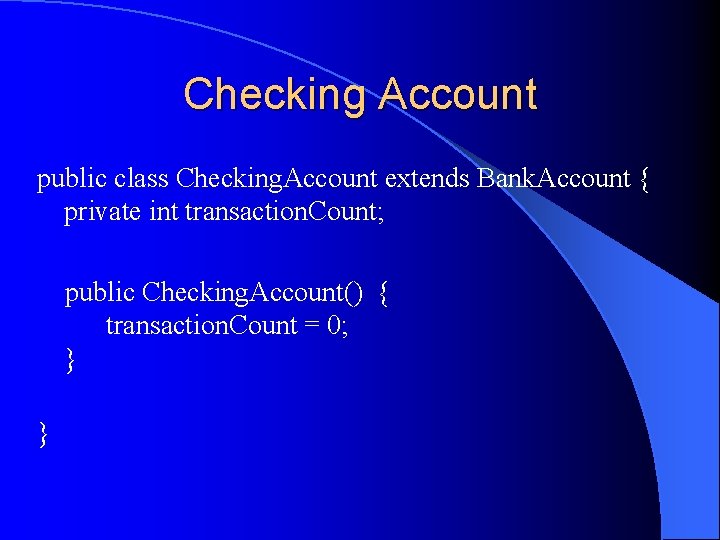
Checking Account public class Checking. Account extends Bank. Account { private int transaction. Count; public Checking. Account() { transaction. Count = 0; } }
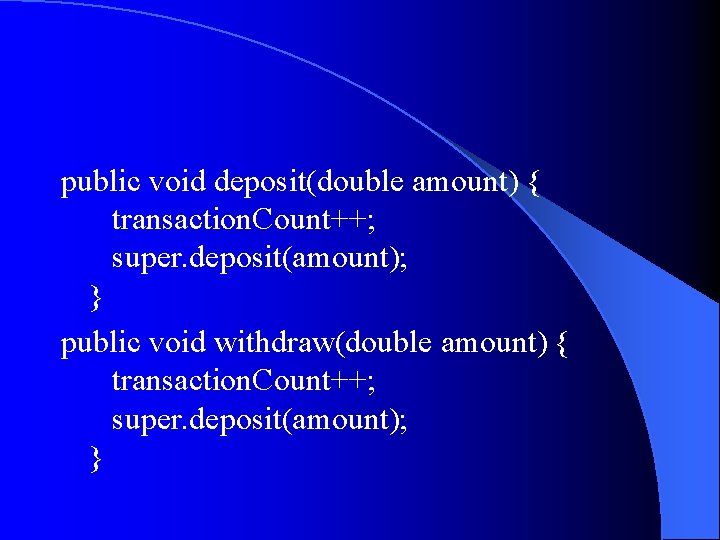
public void deposit(double amount) { transaction. Count++; super. deposit(amount); } public void withdraw(double amount) { transaction. Count++; super. deposit(amount); }
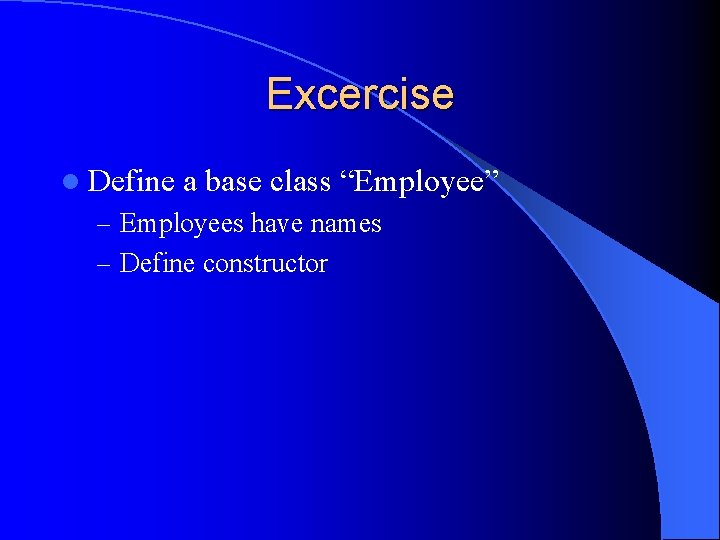
Excercise l Define a base class “Employee” – Employees have names – Define constructor
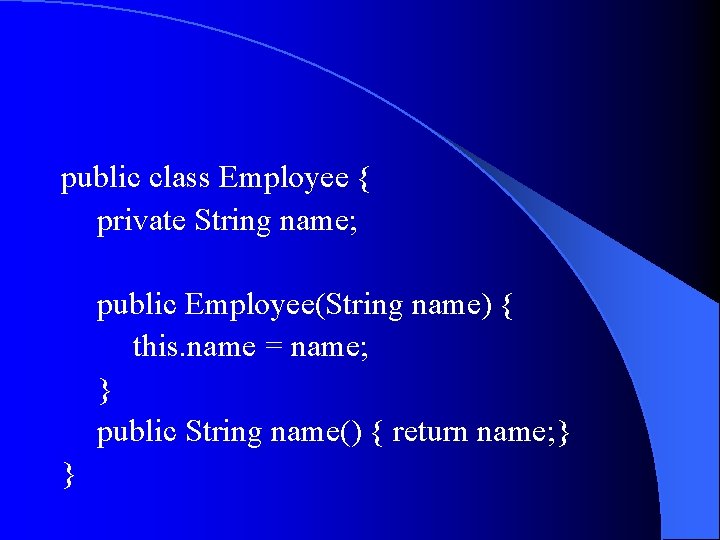
public class Employee { private String name; public Employee(String name) { this. name = name; } public String name() { return name; } }
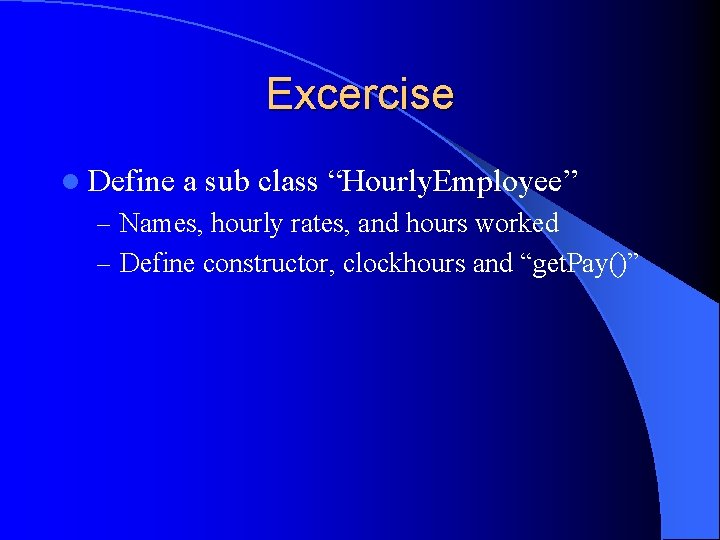
Excercise l Define a sub class “Hourly. Employee” – Names, hourly rates, and hours worked – Define constructor, clockhours and “get. Pay()”
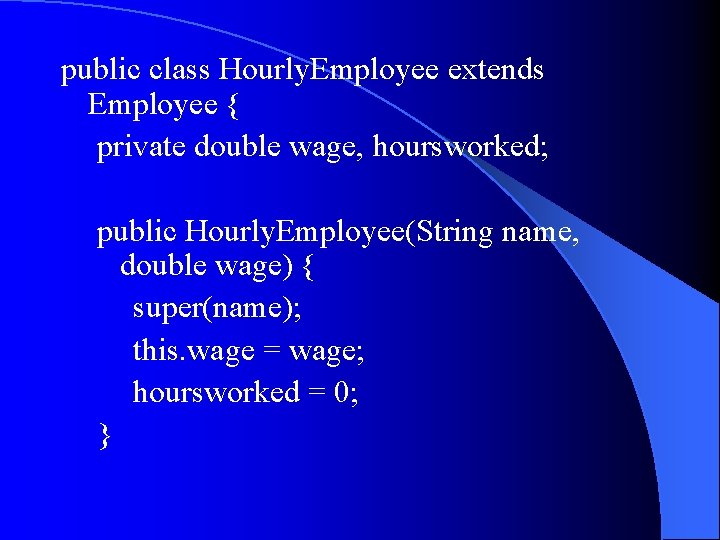
public class Hourly. Employee extends Employee { private double wage, hoursworked; public Hourly. Employee(String name, double wage) { super(name); this. wage = wage; hoursworked = 0; }
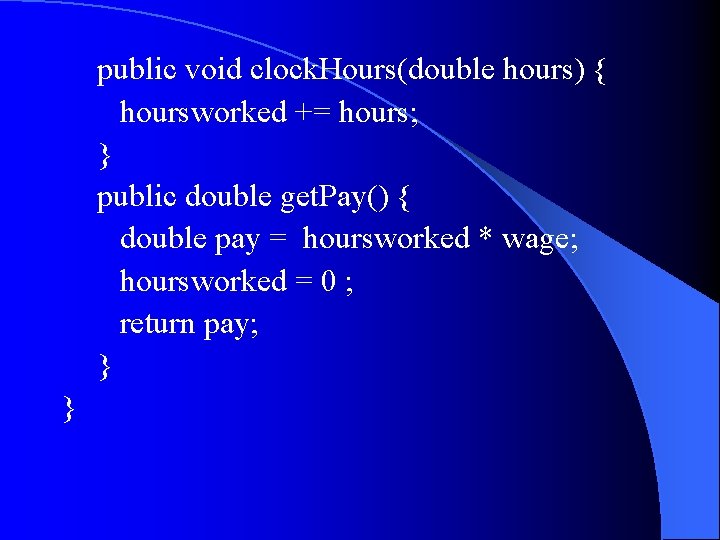
public void clock. Hours(double hours) { hoursworked += hours; } public double get. Pay() { double pay = hoursworked * wage; hoursworked = 0 ; return pay; } }
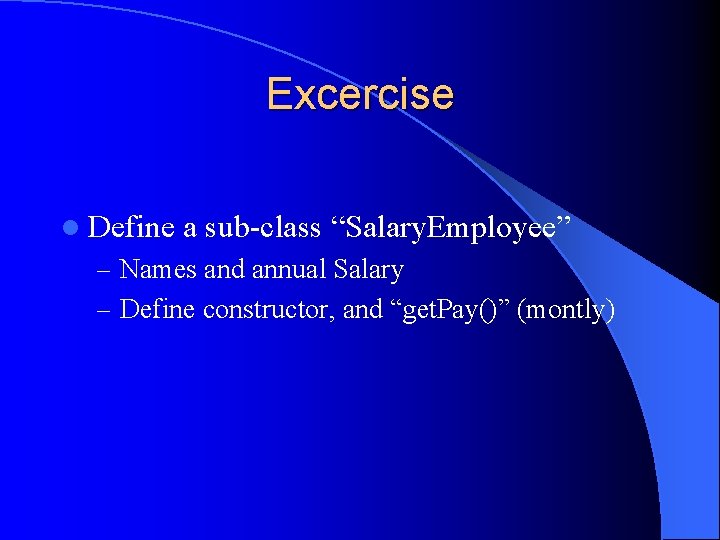
Excercise l Define a sub-class “Salary. Employee” – Names and annual Salary – Define constructor, and “get. Pay()” (montly)
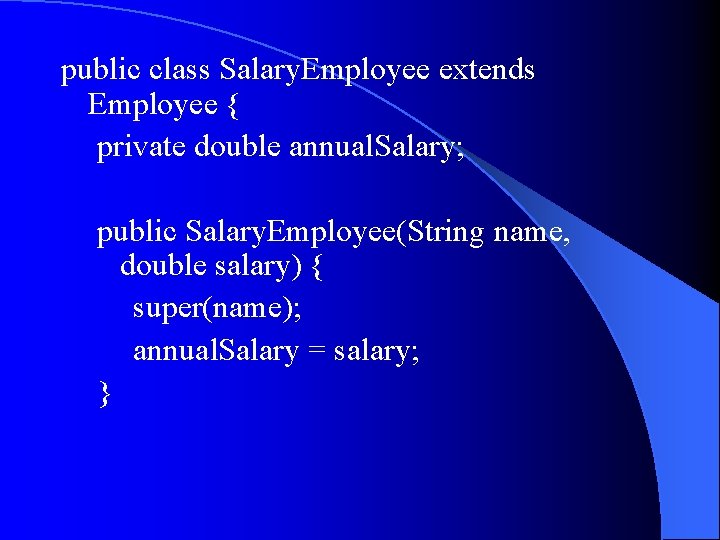
public class Salary. Employee extends Employee { private double annual. Salary; public Salary. Employee(String name, double salary) { super(name); annual. Salary = salary; }
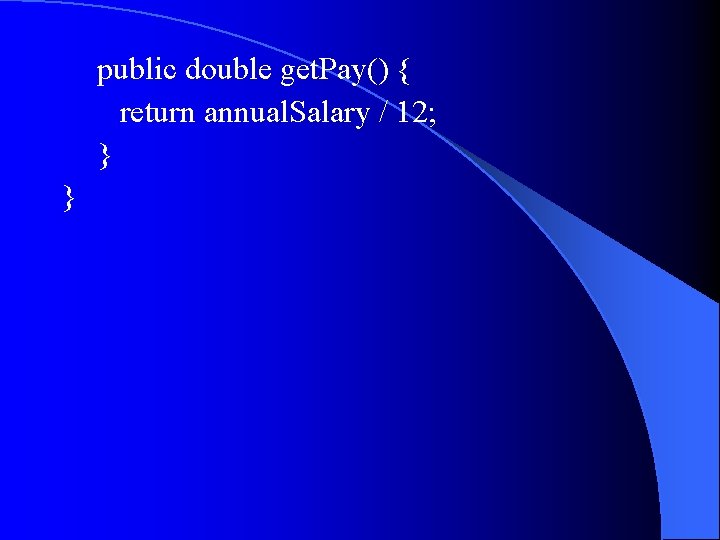
public double get. Pay() { return annual. Salary / 12; } }
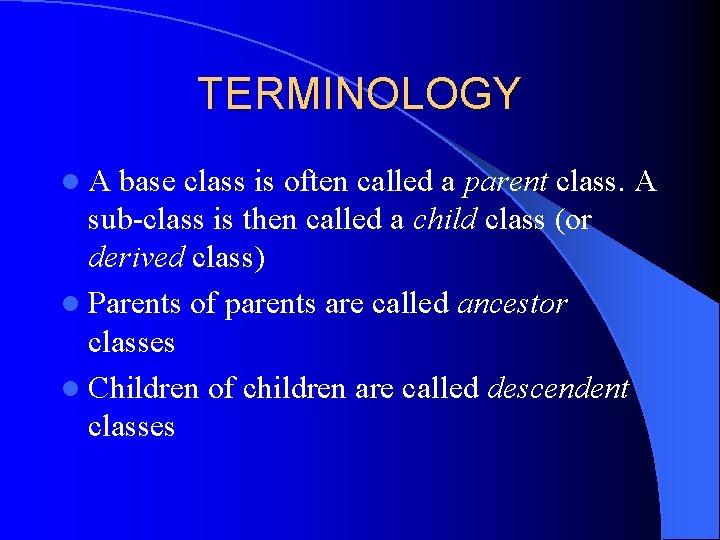
TERMINOLOGY l. A base class is often called a parent class. A sub-class is then called a child class (or derived class) l Parents of parents are called ancestor classes l Children of children are called descendent classes
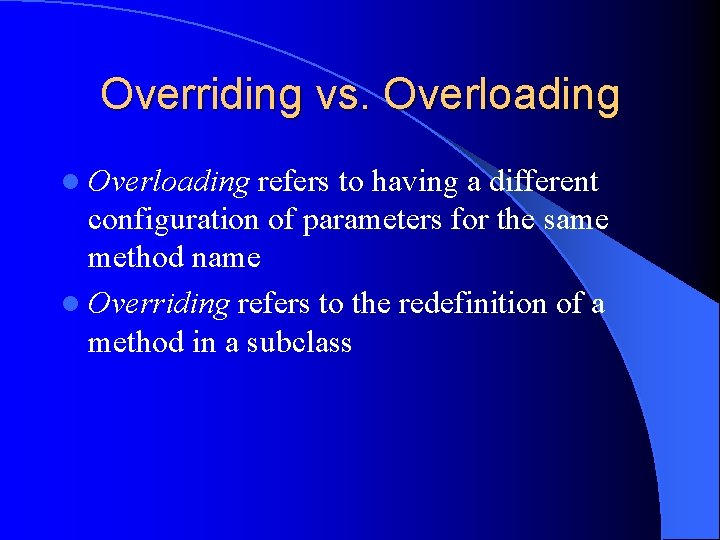
Overriding vs. Overloading l Overloading refers to having a different configuration of parameters for the same method name l Overriding refers to the redefinition of a method in a subclass
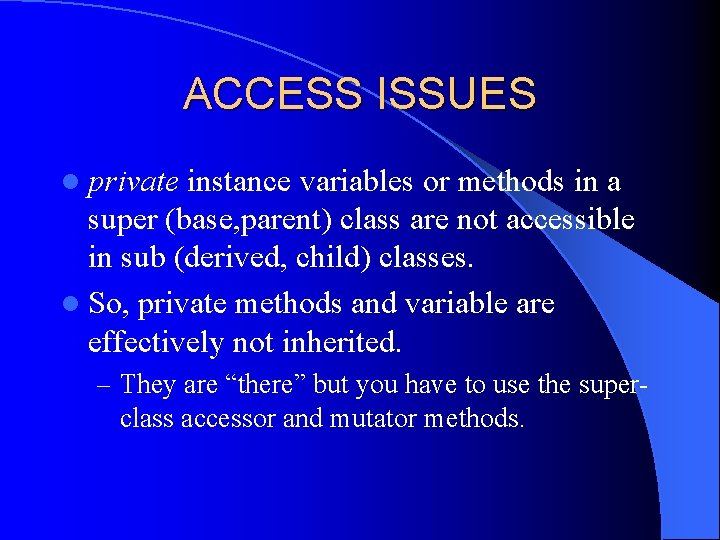
ACCESS ISSUES l private instance variables or methods in a super (base, parent) class are not accessible in sub (derived, child) classes. l So, private methods and variable are effectively not inherited. – They are “there” but you have to use the super- class accessor and mutator methods.
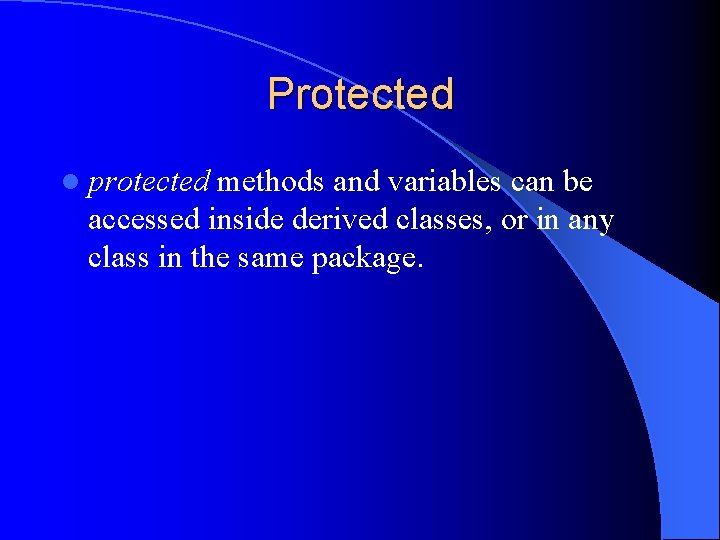
Protected l protected methods and variables can be accessed inside derived classes, or in any class in the same package.
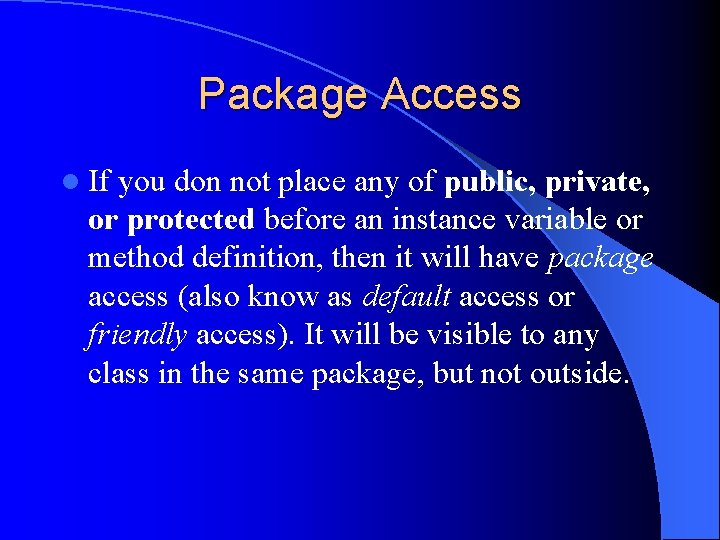
Package Access l If you don not place any of public, private, or protected before an instance variable or method definition, then it will have package access (also know as default access or friendly access). It will be visible to any class in the same package, but not outside.
Pre reading while reading and post reading activities
01:640:244 lecture notes - lecture 15: plat, idah, farad
Pg 171
Lei 171 de 2007
What do salir decir and venir have in common
Nonlinear pricing example
Ai 171
Ai 171
Meaning
171 nomreli mekteb
171 nomreli mekteb
Decret 171/2015
What is hazardous material
Cs 171 uci
171 in binary
171 sayli mekteb
171 nomreli mekteb
The fall of the house of usher pre reading activities
St. louis
Aims and objectives of teaching
Intensive reading characteristics
What is shared reading
According to gary goshgarian, critical reading is
Intensive reading and extensive reading
What is intensive reading
What is intensive reading
Mtved exemple
Pearson
Richard murray caltech
Sni 16-7061-2004
T. trimpe 2004 http //sciencespot.net/
Gaatn
2004 dress code
Sample of school grievance committee
Sworn statement for esales registration
Legge urbanistica regione campania 16/2004
Renbut abk
Perbedaan kurikulum 2004 dan 2006
2004 ford ranger 2.3 firing order