CS 212 Data Structures and Algorithms Trees Outline
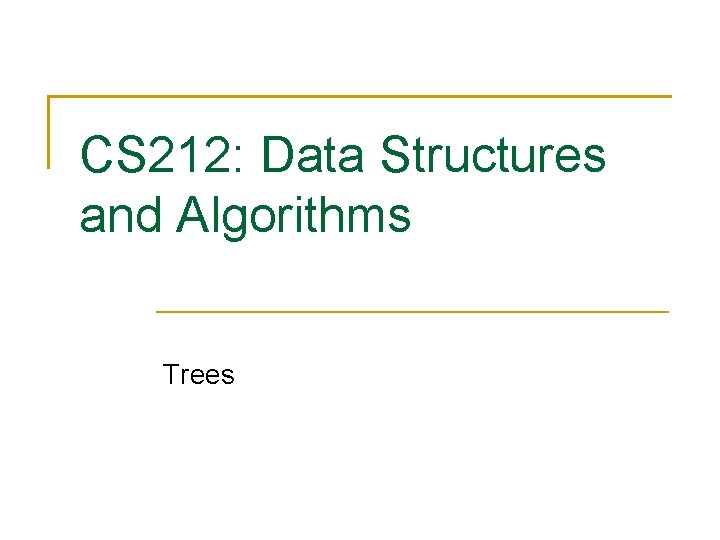
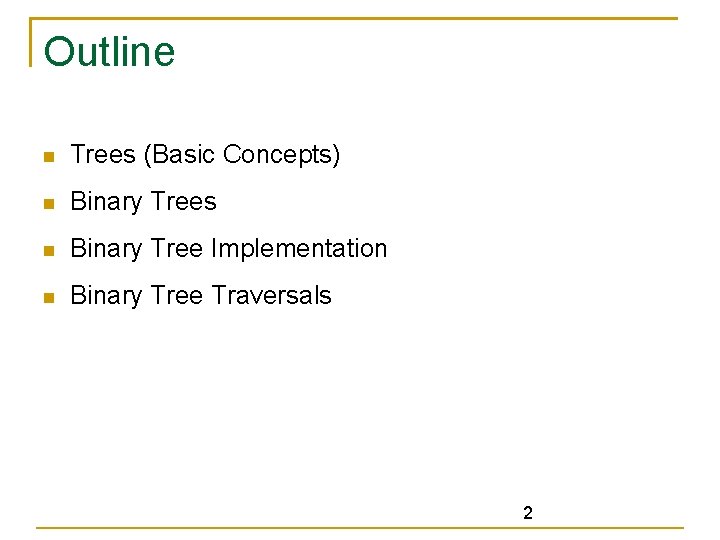
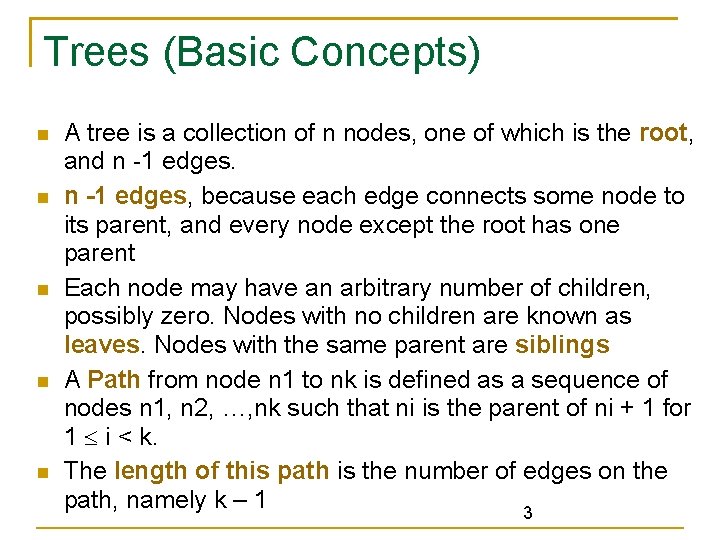
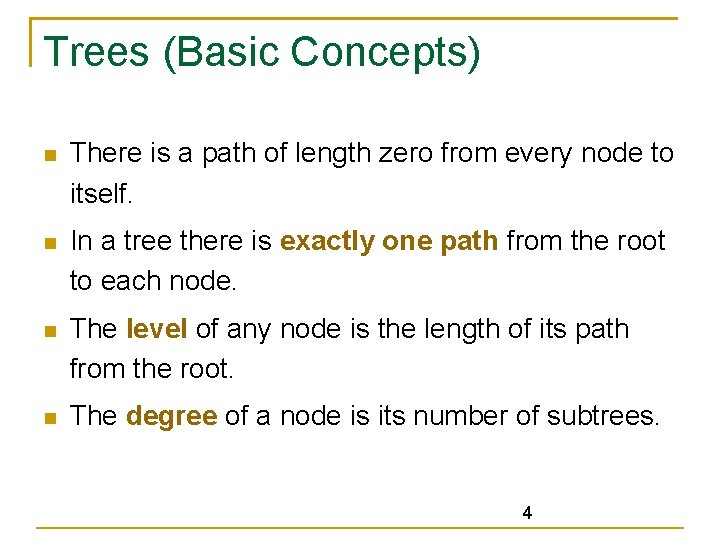
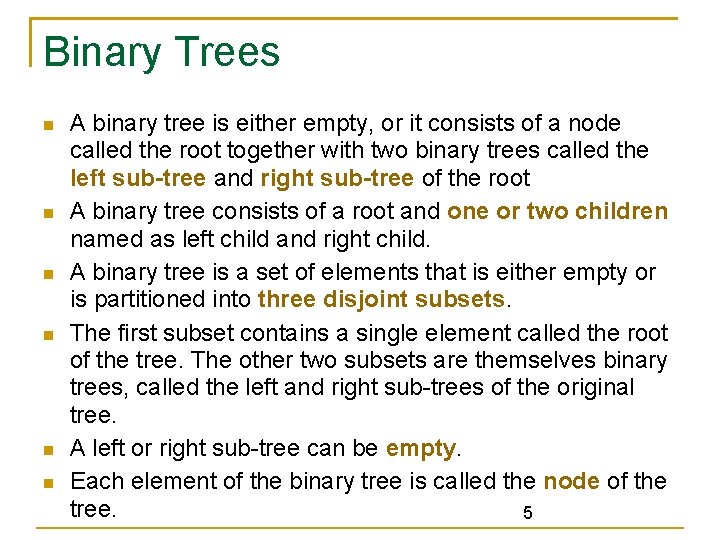
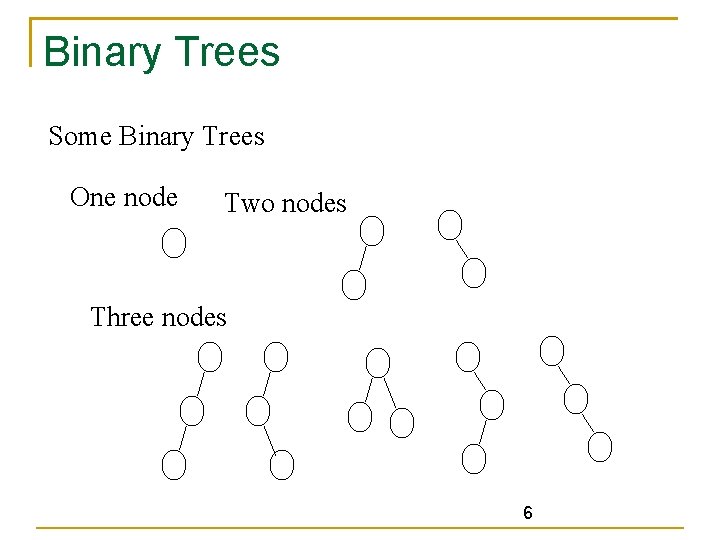
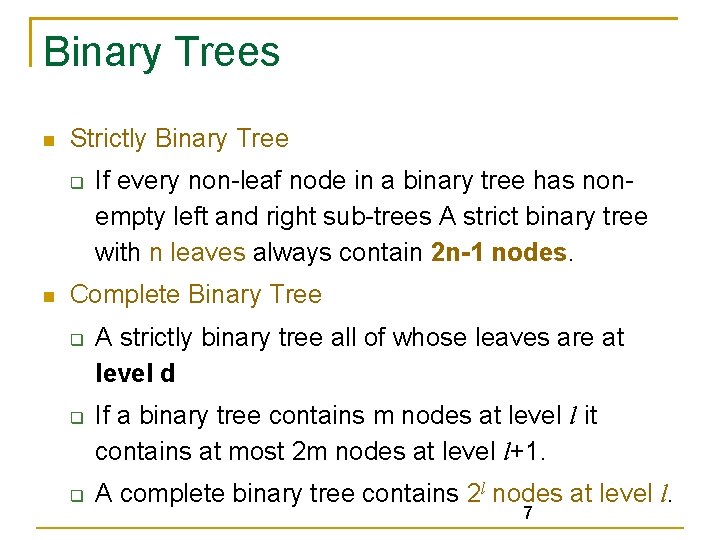
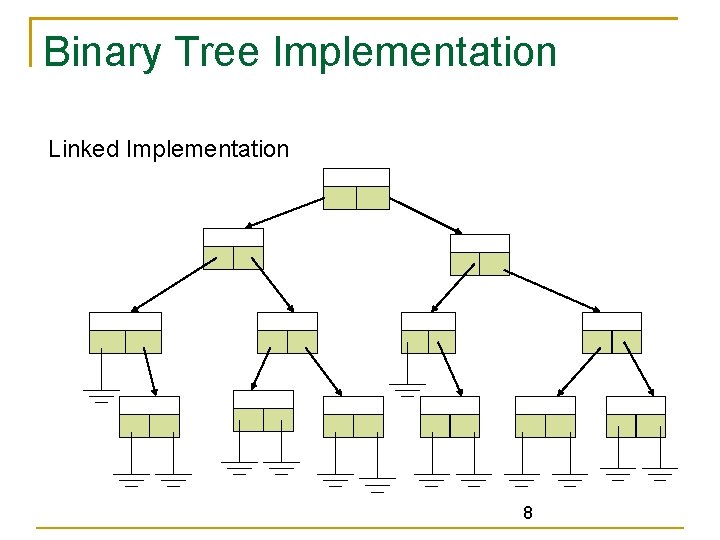
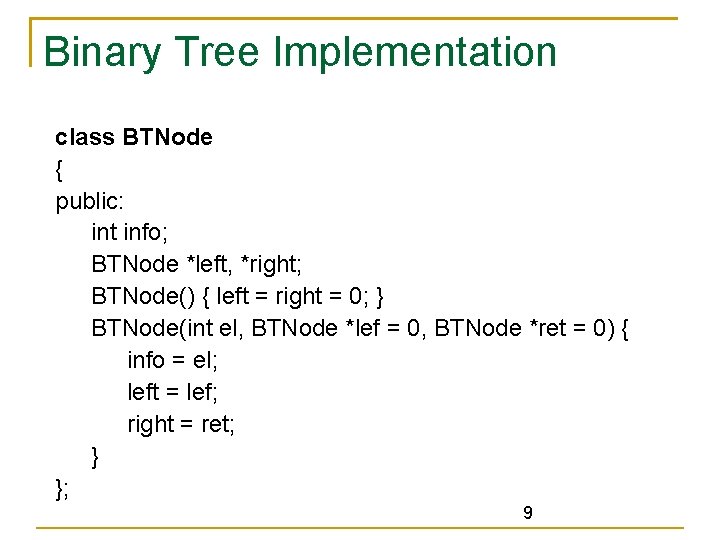
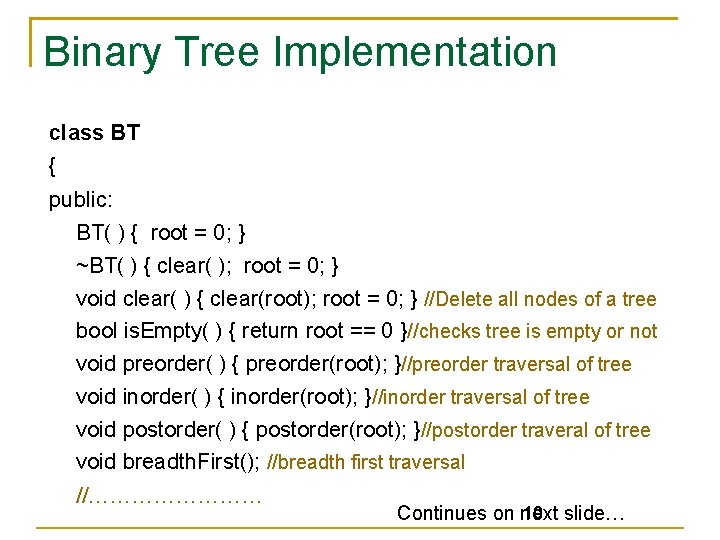
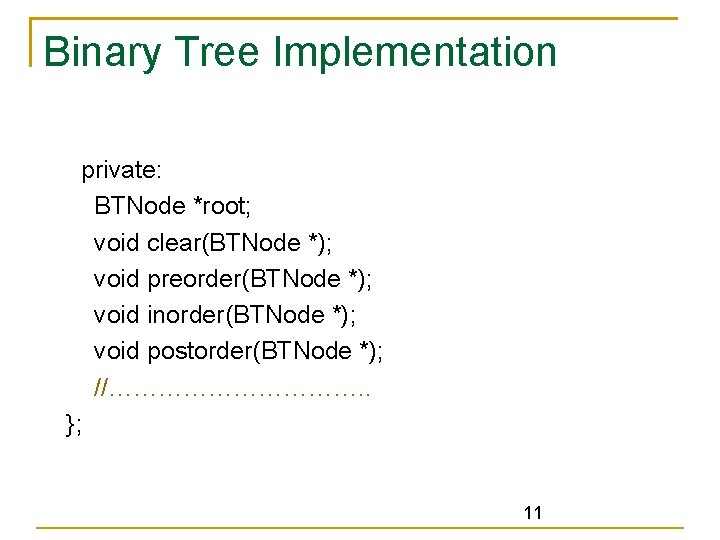
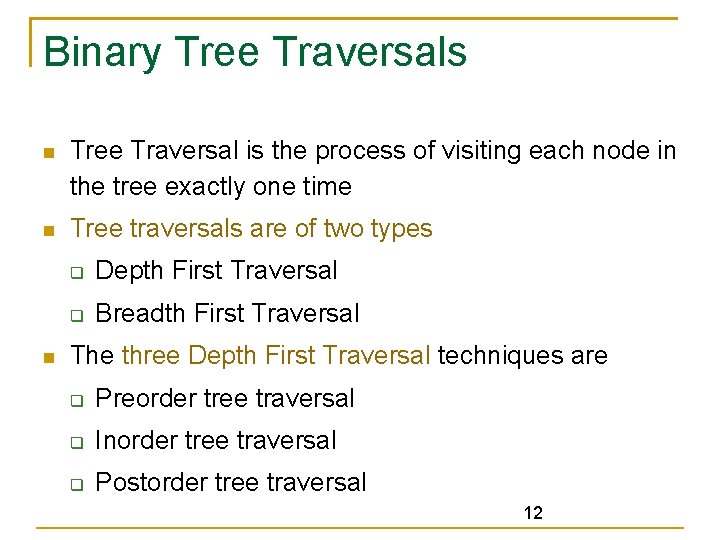
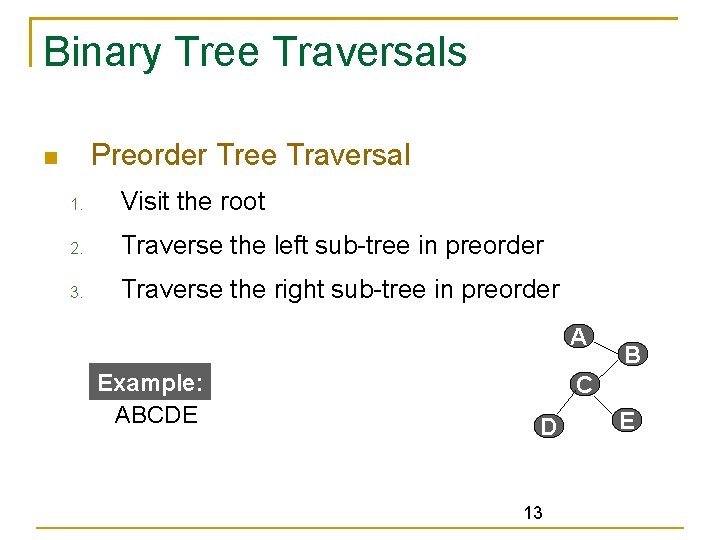
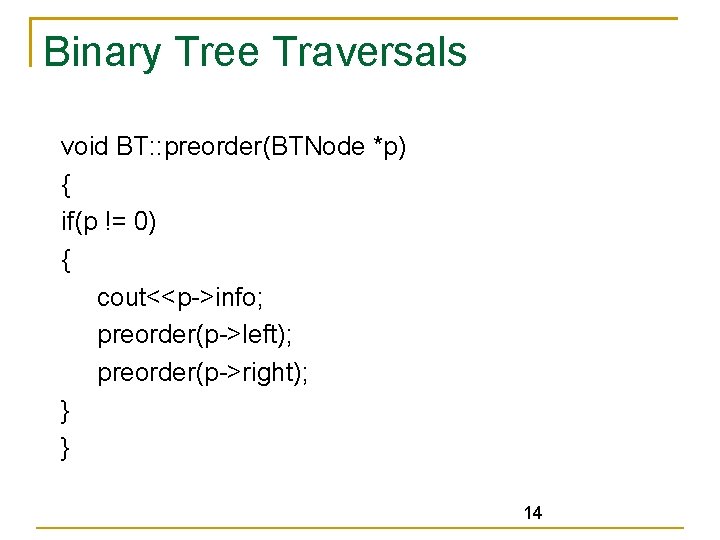
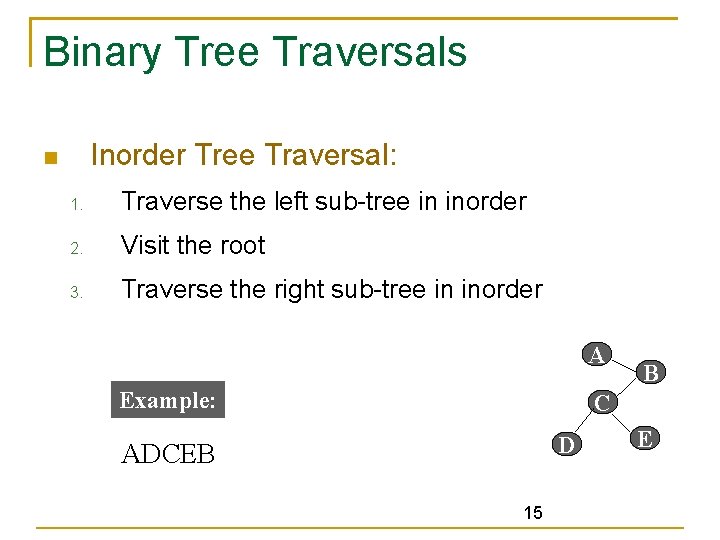
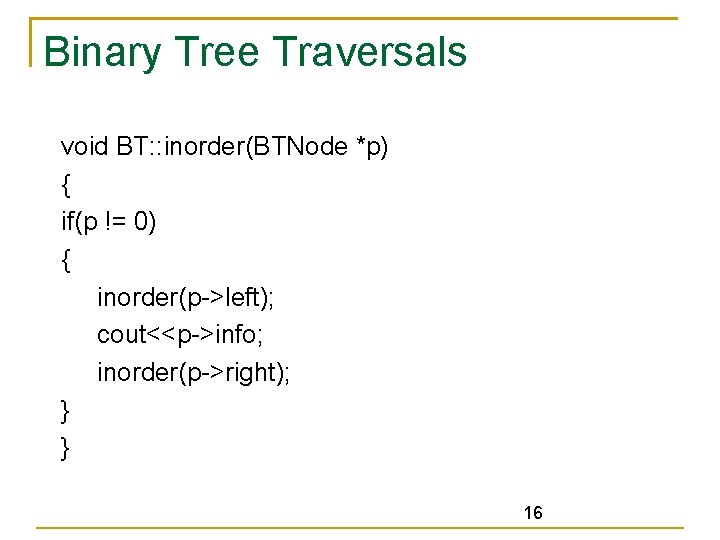
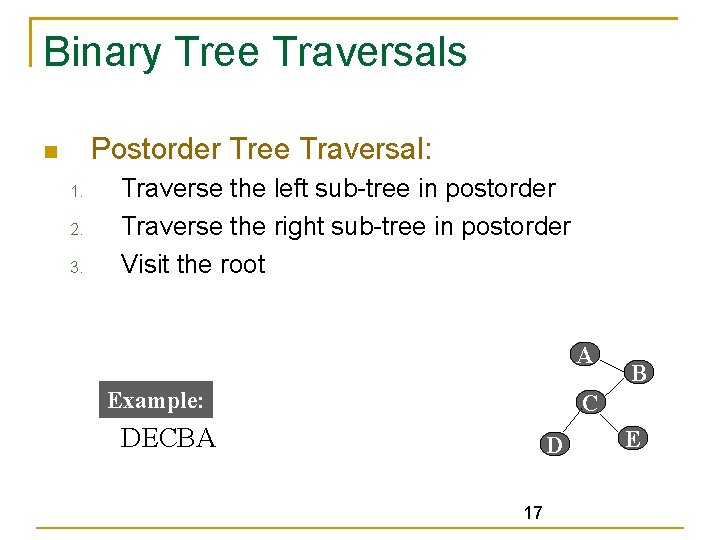
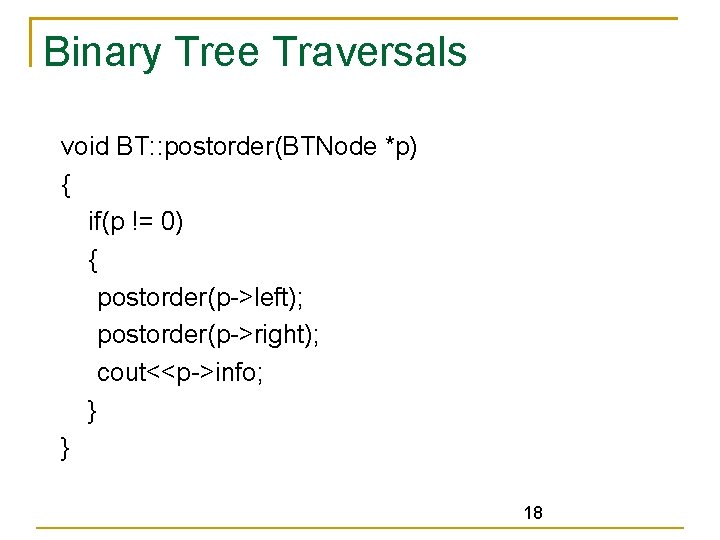
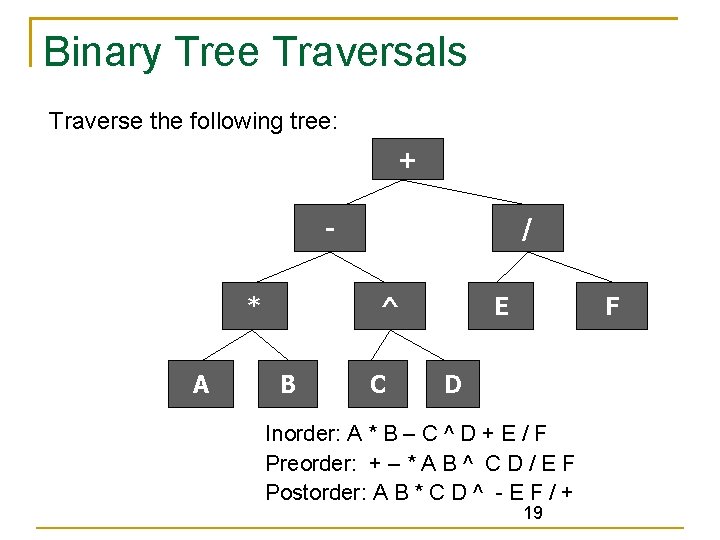
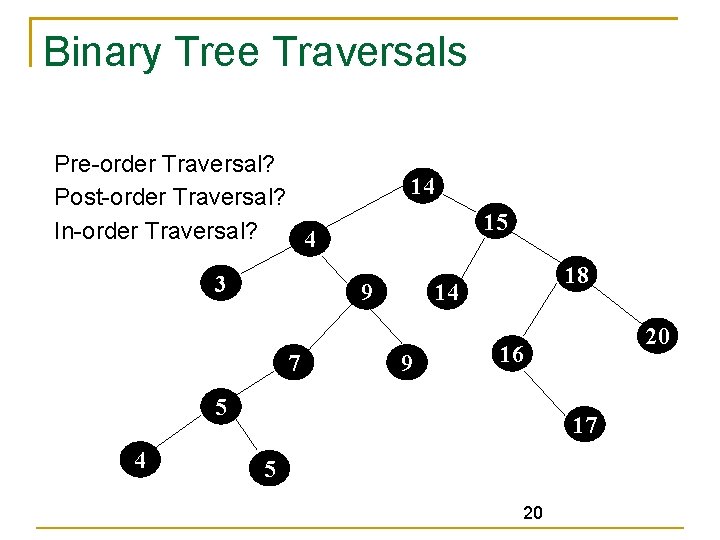
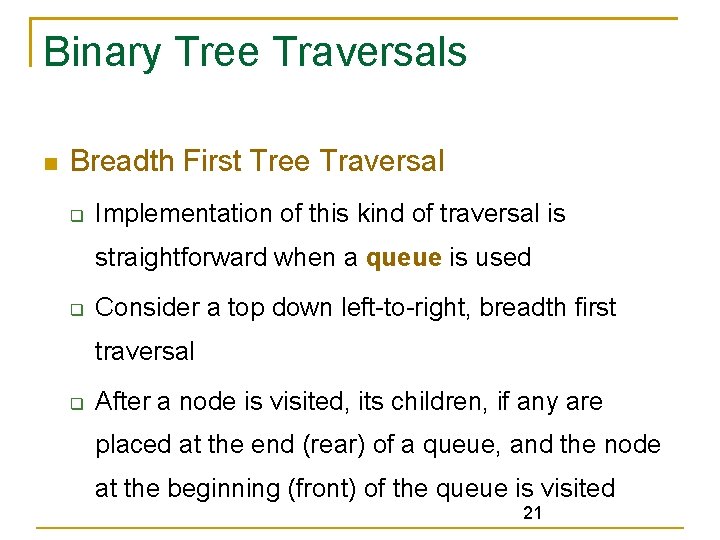
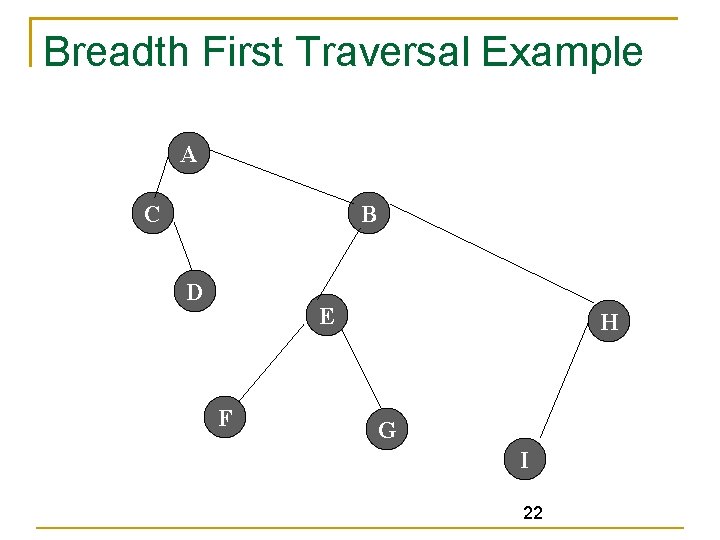
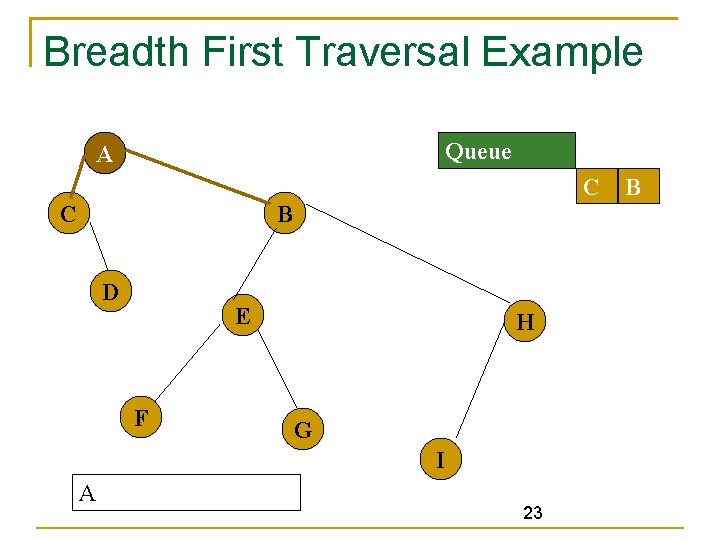
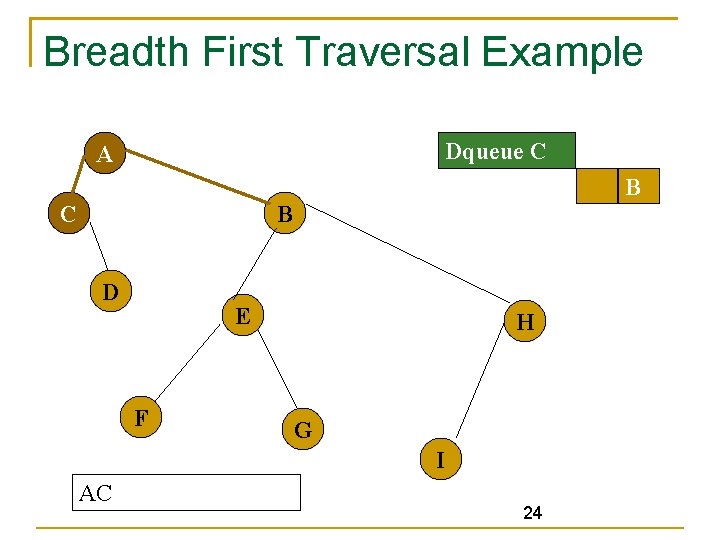
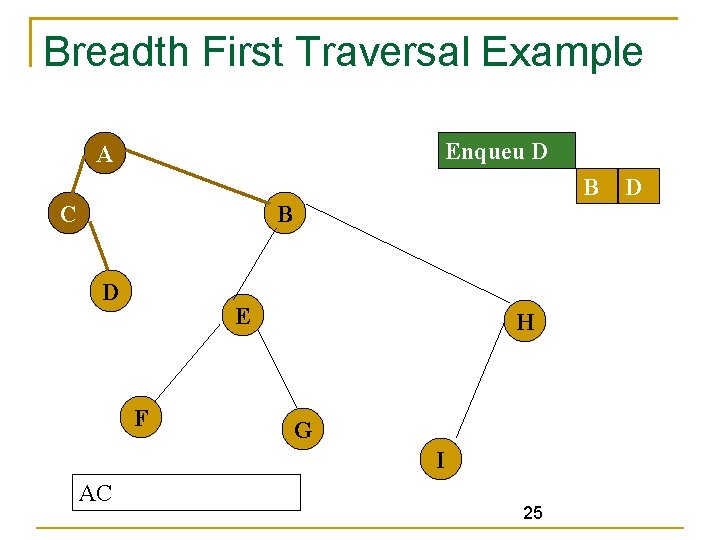
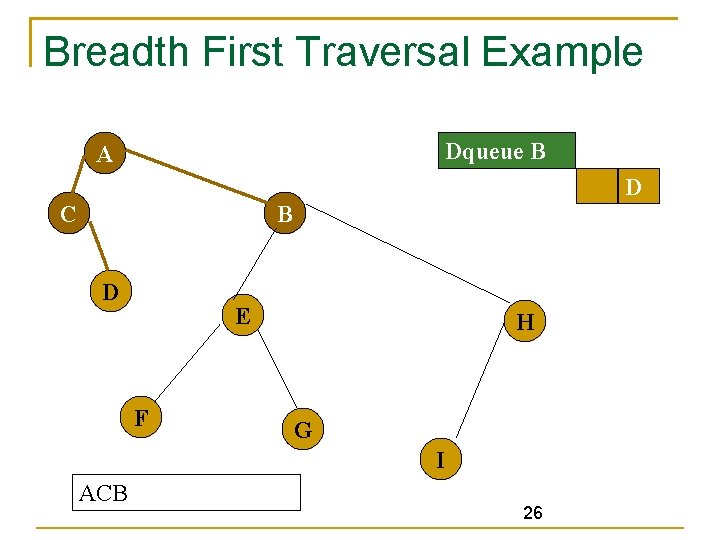
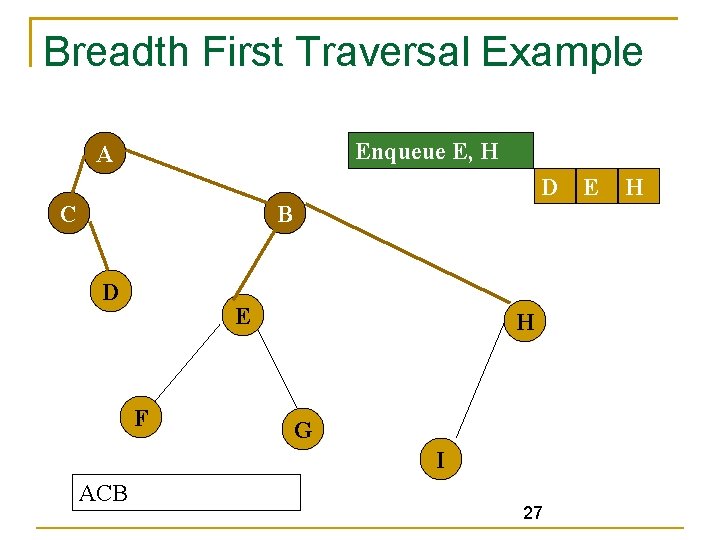
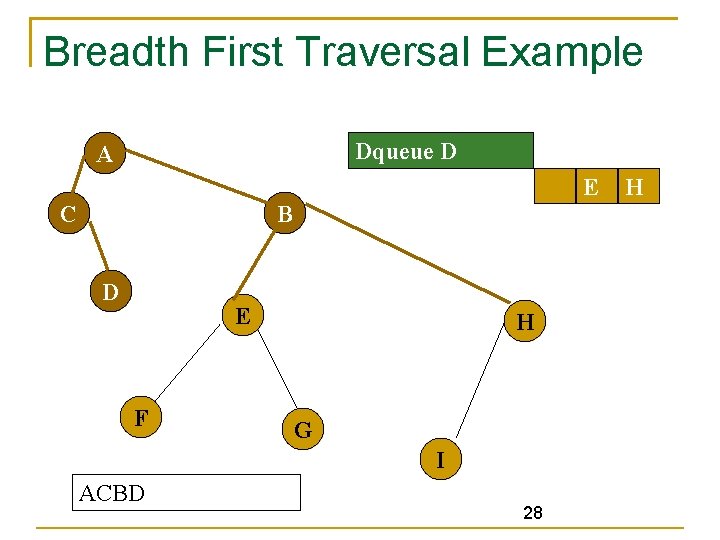
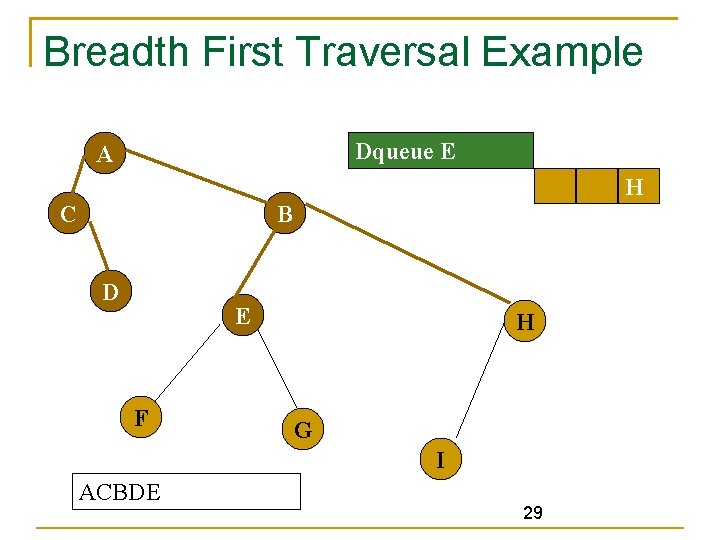
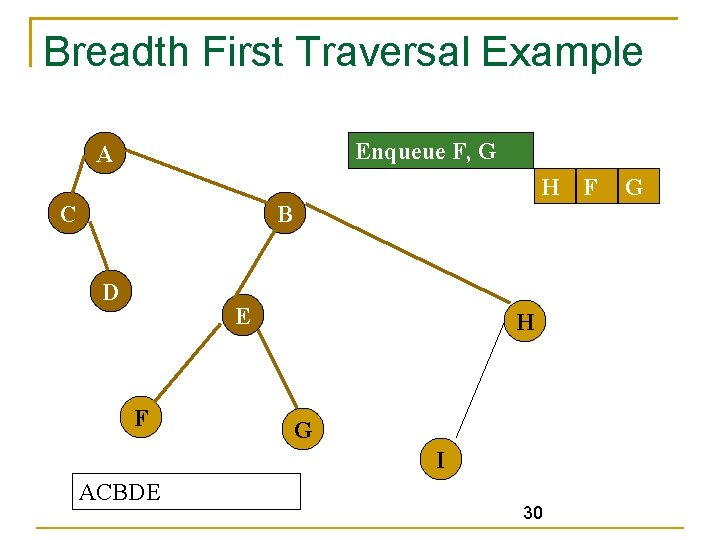
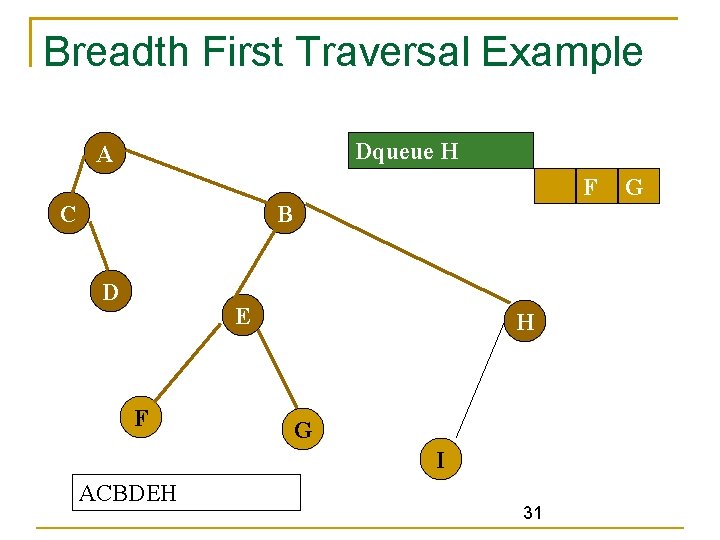
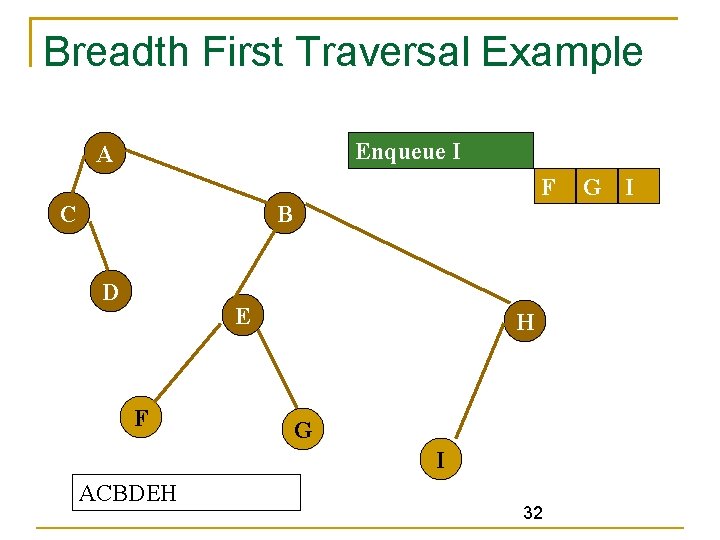
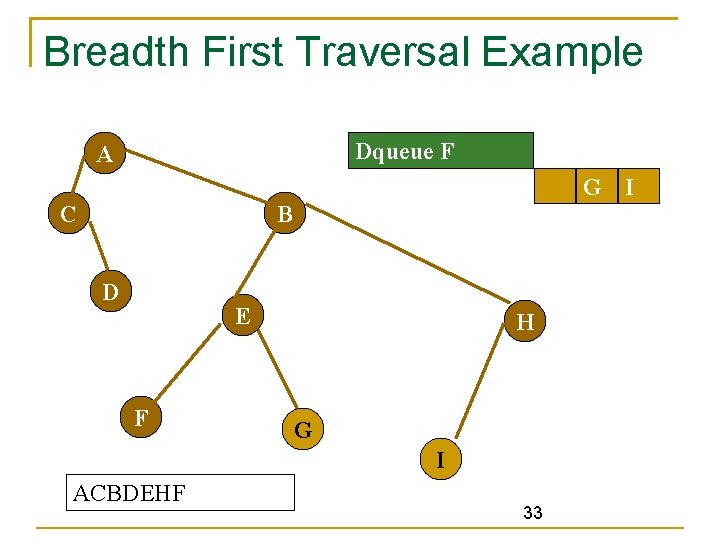
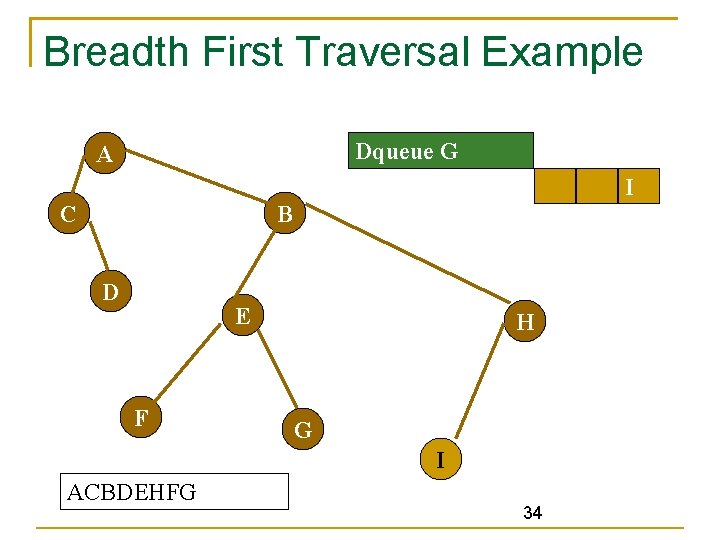
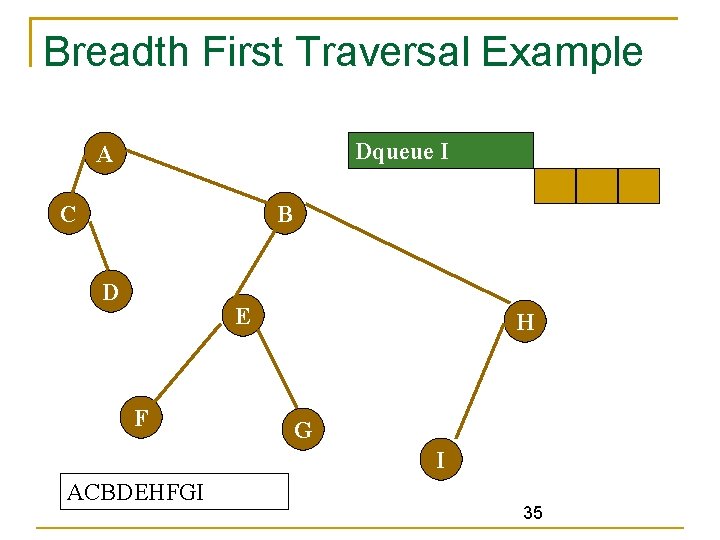
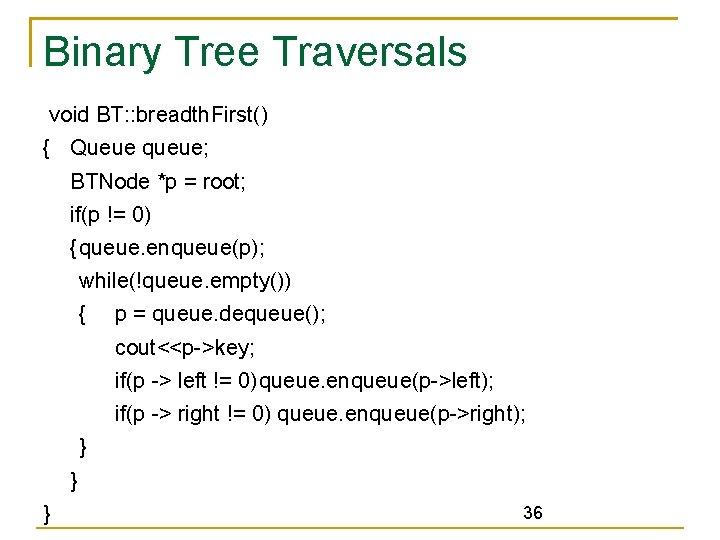
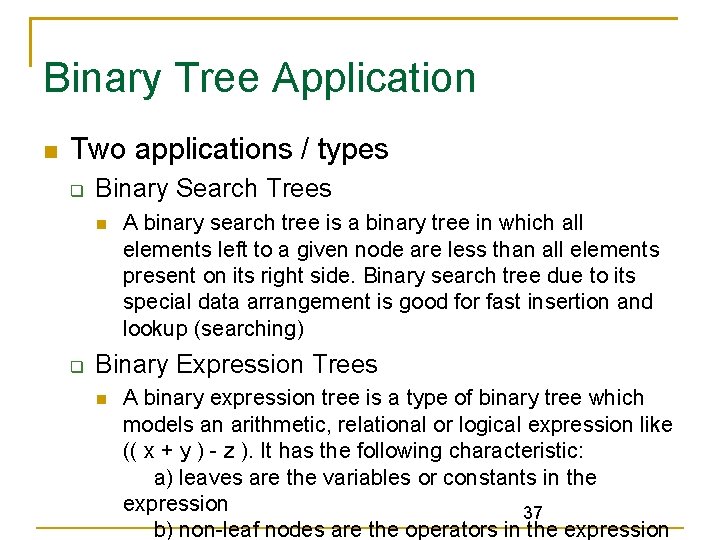
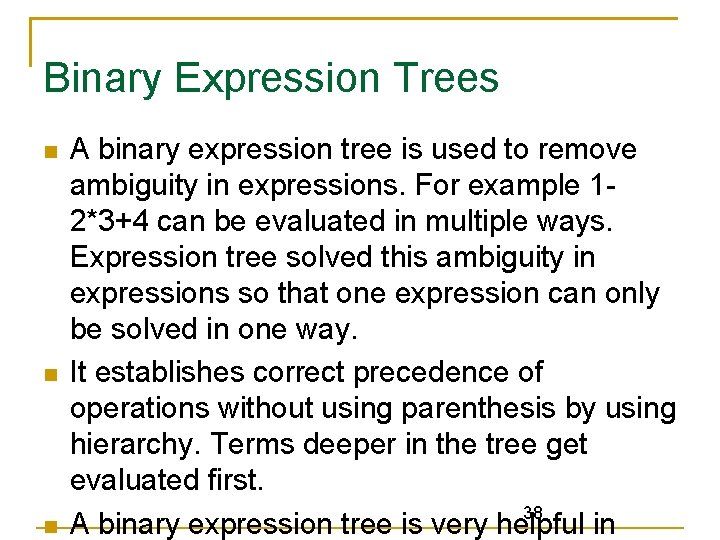
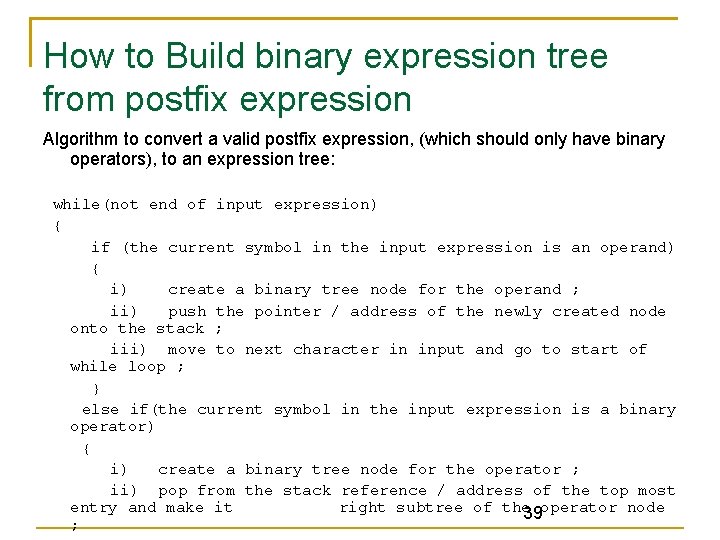
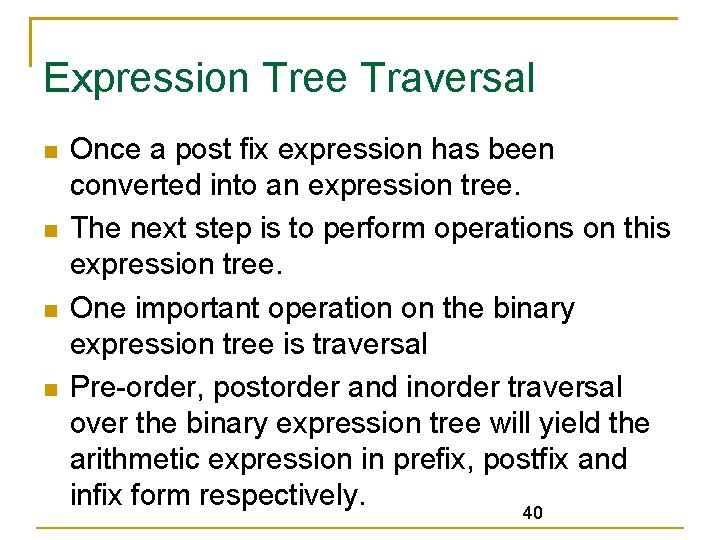
- Slides: 40
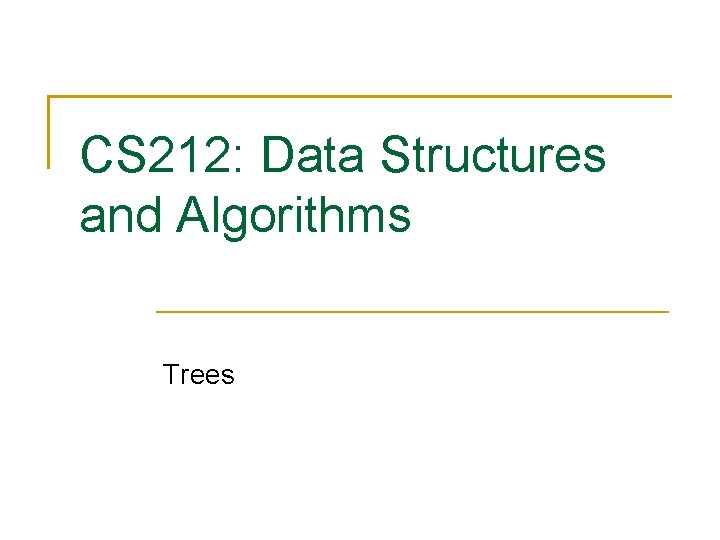
CS 212: Data Structures and Algorithms Trees
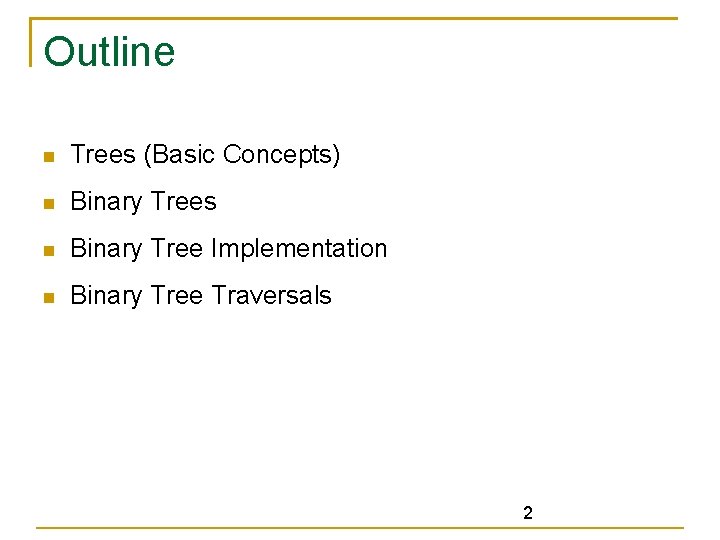
Outline Trees (Basic Concepts) Binary Trees Binary Tree Implementation Binary Tree Traversals 2
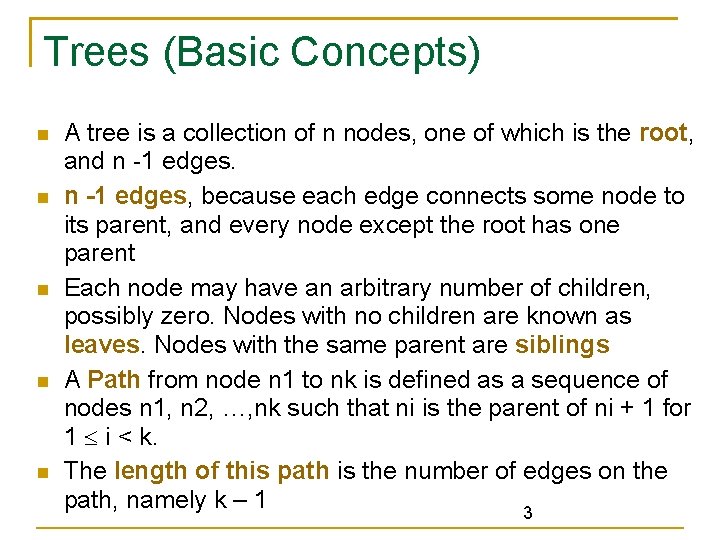
Trees (Basic Concepts) A tree is a collection of n nodes, one of which is the root, and n -1 edges, because each edge connects some node to its parent, and every node except the root has one parent Each node may have an arbitrary number of children, possibly zero. Nodes with no children are known as leaves. Nodes with the same parent are siblings A Path from node n 1 to nk is defined as a sequence of nodes n 1, n 2, …, nk such that ni is the parent of ni + 1 for 1 i < k. The length of this path is the number of edges on the path, namely k – 1 3
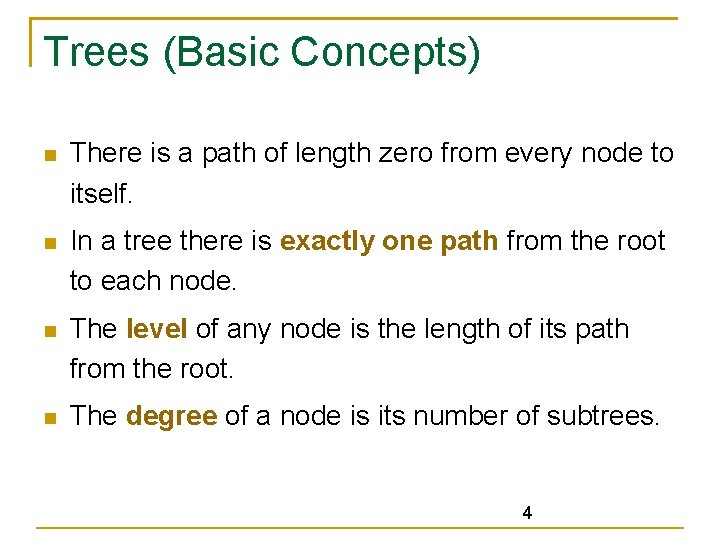
Trees (Basic Concepts) There is a path of length zero from every node to itself. In a tree there is exactly one path from the root to each node. The level of any node is the length of its path from the root. The degree of a node is its number of subtrees. 4
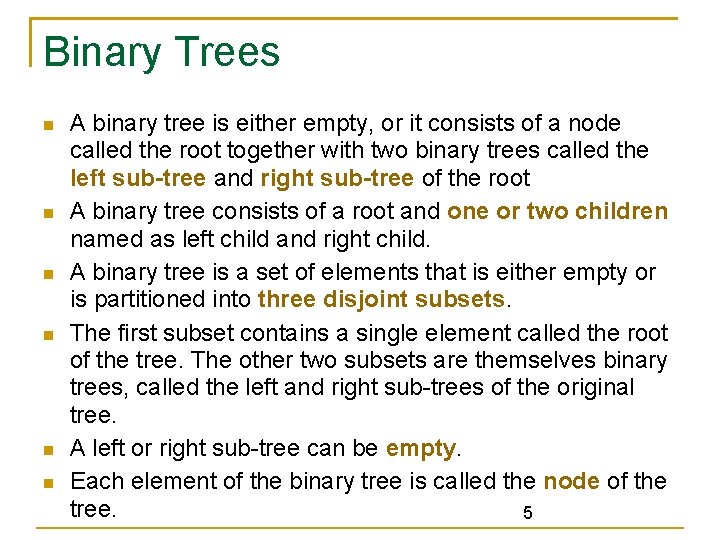
Binary Trees A binary tree is either empty, or it consists of a node called the root together with two binary trees called the left sub-tree and right sub-tree of the root A binary tree consists of a root and one or two children named as left child and right child. A binary tree is a set of elements that is either empty or is partitioned into three disjoint subsets. The first subset contains a single element called the root of the tree. The other two subsets are themselves binary trees, called the left and right sub-trees of the original tree. A left or right sub-tree can be empty. Each element of the binary tree is called the node of the tree. 5
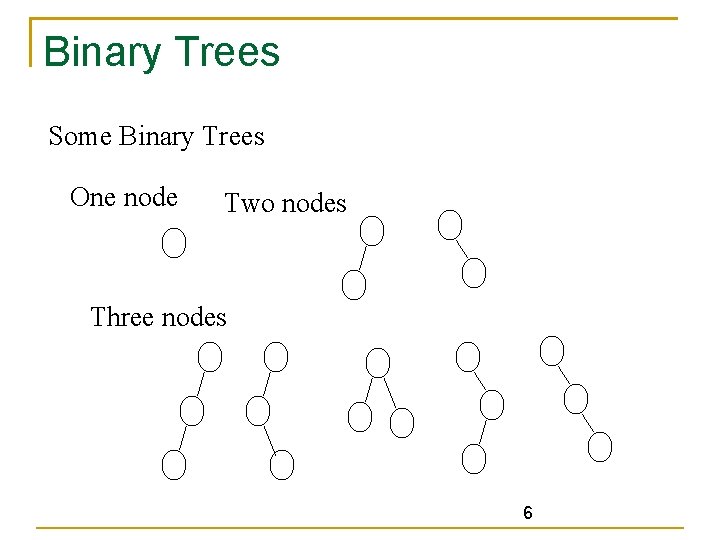
Binary Trees Some Binary Trees One node Two nodes Three nodes 6
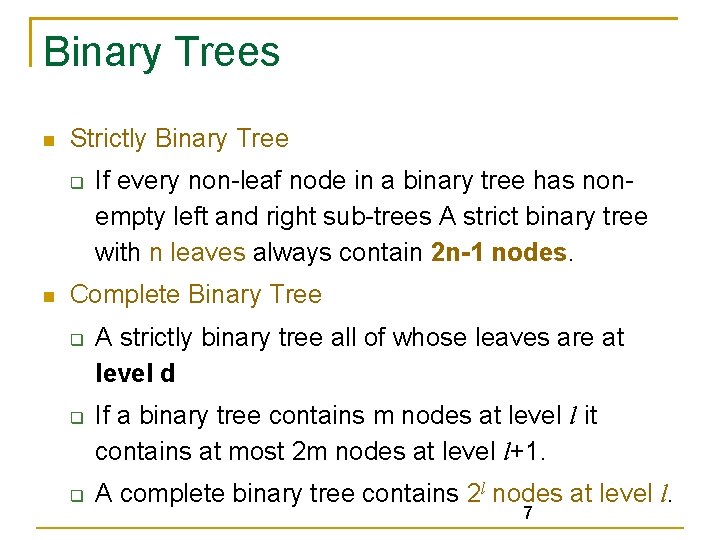
Binary Trees Strictly Binary Tree If every non-leaf node in a binary tree has nonempty left and right sub-trees A strict binary tree with n leaves always contain 2 n-1 nodes. Complete Binary Tree A strictly binary tree all of whose leaves are at level d If a binary tree contains m nodes at level l it contains at most 2 m nodes at level l+1. A complete binary tree contains 2 l nodes at level l. 7
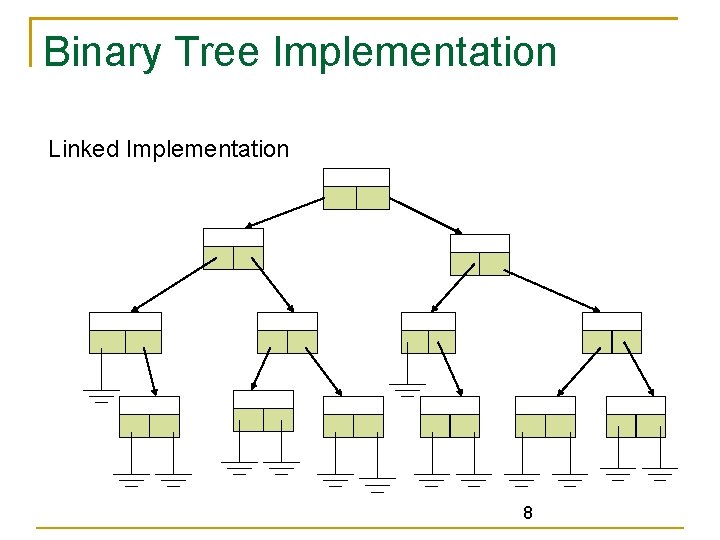
Binary Tree Implementation Linked Implementation 8
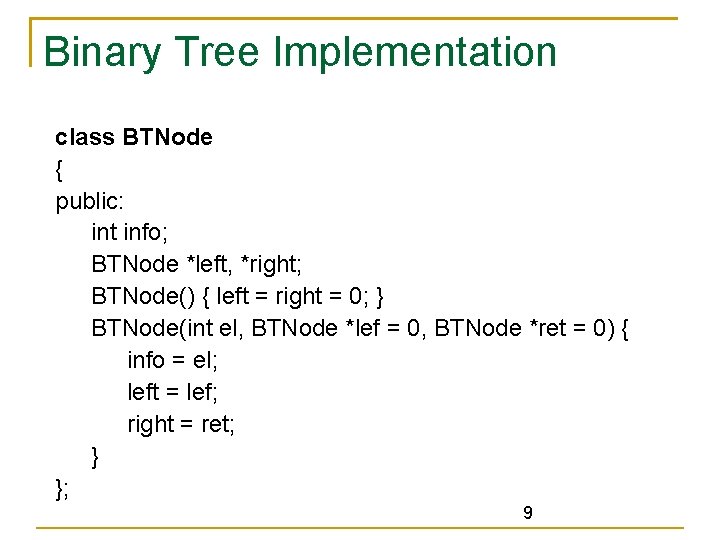
Binary Tree Implementation class BTNode { public: int info; BTNode *left, *right; BTNode() { left = right = 0; } BTNode(int el, BTNode *lef = 0, BTNode *ret = 0) { info = el; left = lef; right = ret; } }; 9
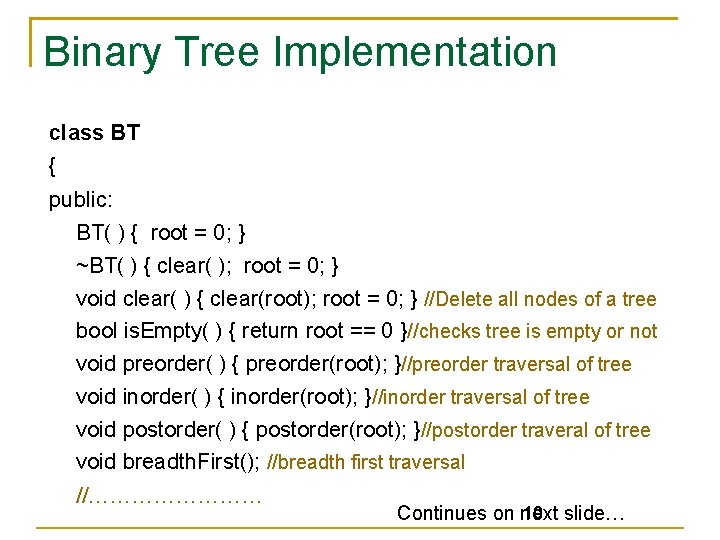
Binary Tree Implementation class BT { public: BT( ) { root = 0; } ~BT( ) { clear( ); root = 0; } void clear( ) { clear(root); root = 0; } //Delete all nodes of a tree bool is. Empty( ) { return root == 0 }//checks tree is empty or not void preorder( ) { preorder(root); }//preorder traversal of tree void inorder( ) { inorder(root); }//inorder traversal of tree void postorder( ) { postorder(root); }//postorder traveral of tree void breadth. First(); //breadth first traversal //………… 10 slide… Continues on next
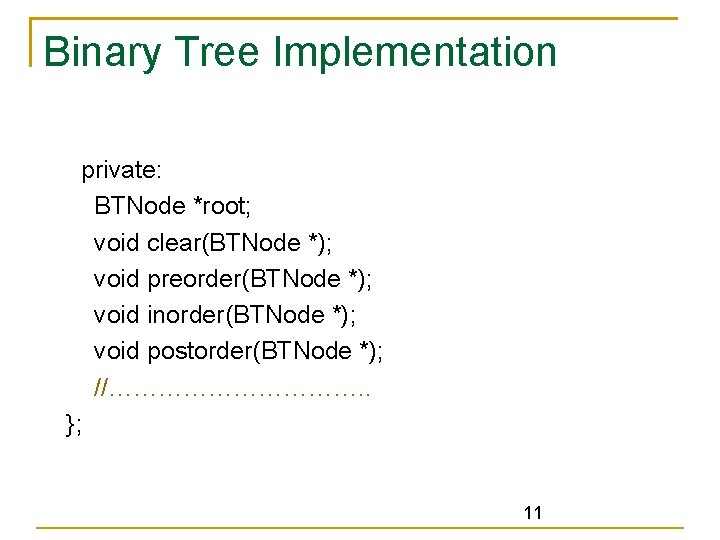
Binary Tree Implementation private: BTNode *root; void clear(BTNode *); void preorder(BTNode *); void inorder(BTNode *); void postorder(BTNode *); //……………. . }; 11
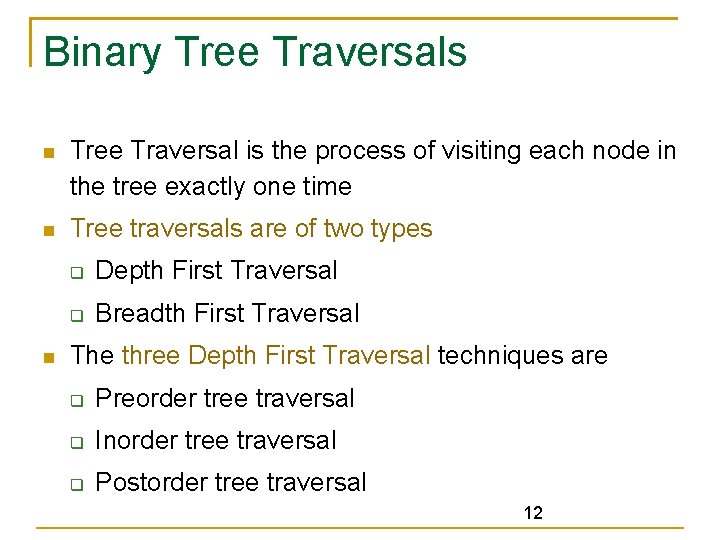
Binary Tree Traversals Tree Traversal is the process of visiting each node in the tree exactly one time Tree traversals are of two types Depth First Traversal Breadth First Traversal The three Depth First Traversal techniques are Preorder tree traversal Inorder tree traversal Postorder tree traversal 12
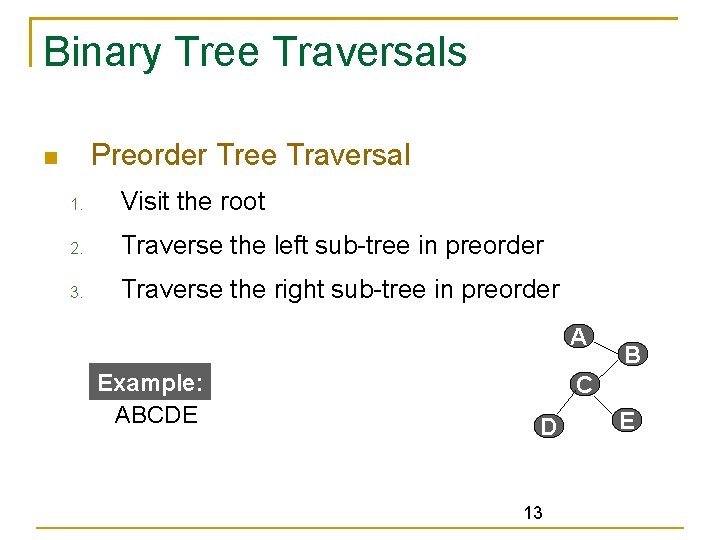
Binary Tree Traversals Preorder Tree Traversal 1. Visit the root 2. Traverse the left sub-tree in preorder 3. Traverse the right sub-tree in preorder A Example: ABCDE B C D 13 E
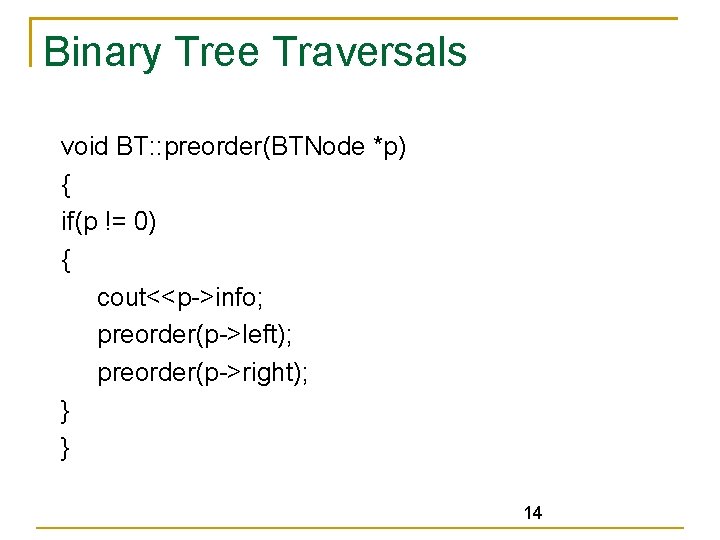
Binary Tree Traversals void BT: : preorder(BTNode *p) { if(p != 0) { cout<<p->info; preorder(p->left); preorder(p->right); } } 14
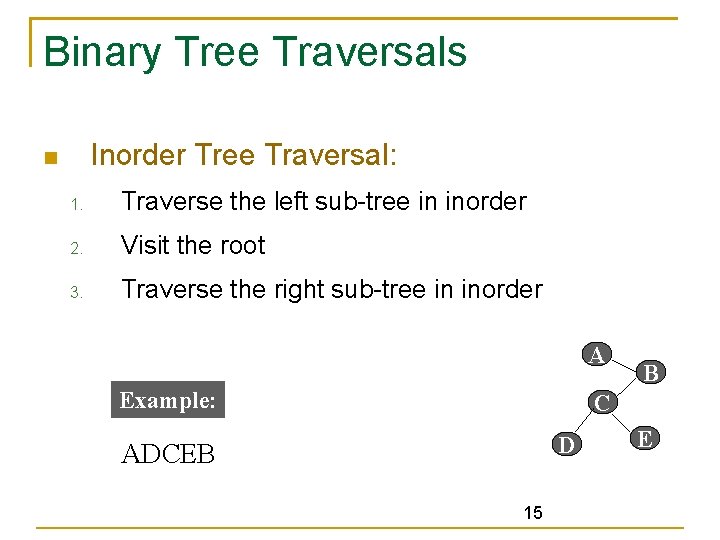
Binary Tree Traversals Inorder Tree Traversal: 1. Traverse the left sub-tree in inorder 2. Visit the root 3. Traverse the right sub-tree in inorder A Example: B C D ADCEB 15 E
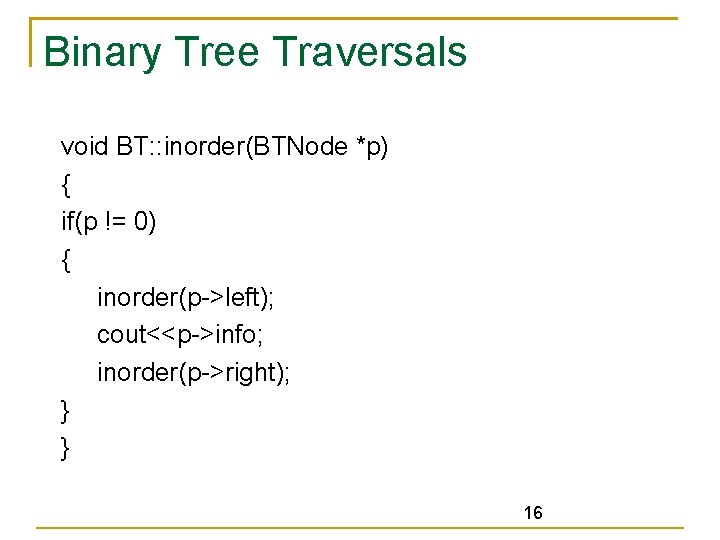
Binary Tree Traversals void BT: : inorder(BTNode *p) { if(p != 0) { inorder(p->left); cout<<p->info; inorder(p->right); } } 16
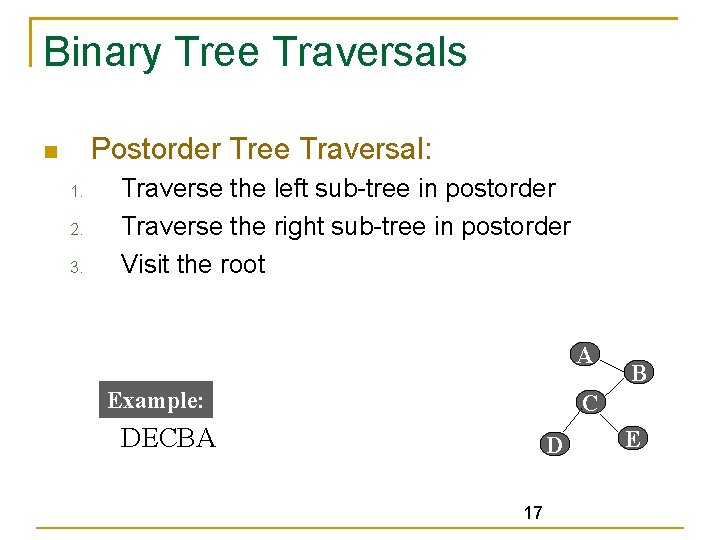
Binary Tree Traversals Postorder Tree Traversal: 1. 2. 3. Traverse the left sub-tree in postorder Traverse the right sub-tree in postorder Visit the root A Example: B C DECBA D 17 E
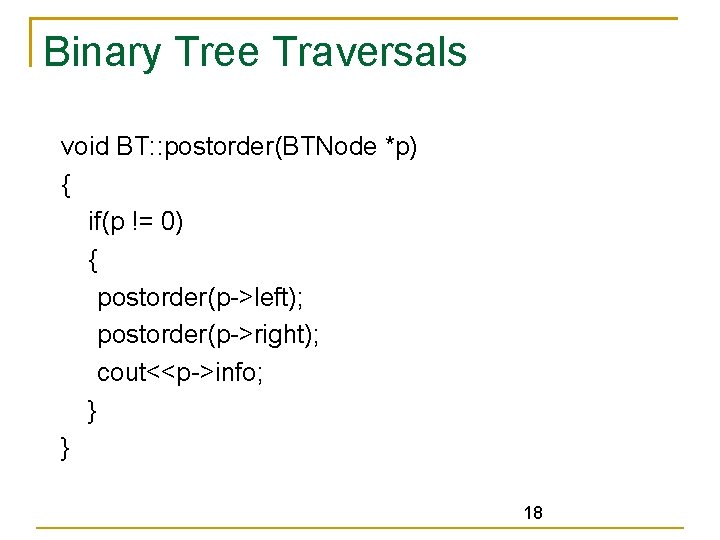
Binary Tree Traversals void BT: : postorder(BTNode *p) { if(p != 0) { postorder(p->left); postorder(p->right); cout<<p->info; } } 18
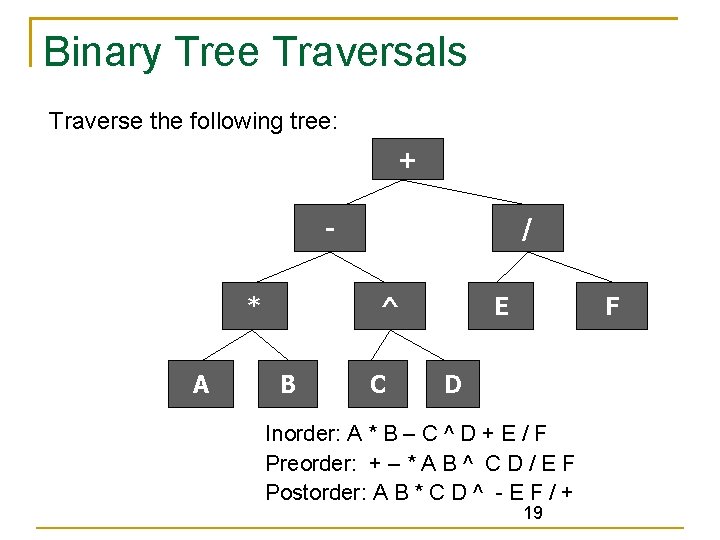
Binary Tree Traversals Traverse the following tree: + * A / ^ B C E F D Inorder: A * B – C ^ D + E / F Preorder: + – * A B ^ C D / E F Postorder: A B * C D ^ - E F / + 19
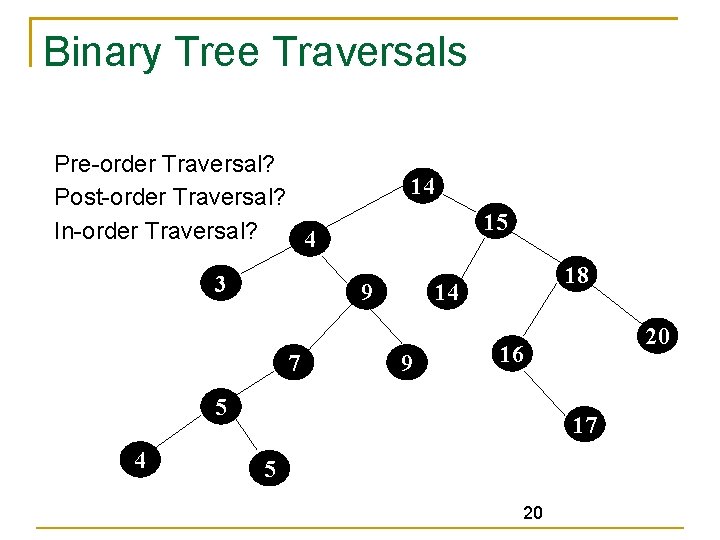
Binary Tree Traversals Traverse the following tree. Pre-order Traversal? Post-order Traversal? In-order Traversal? 4 3 14 15 9 7 18 14 9 16 5 4 20 17 5 20
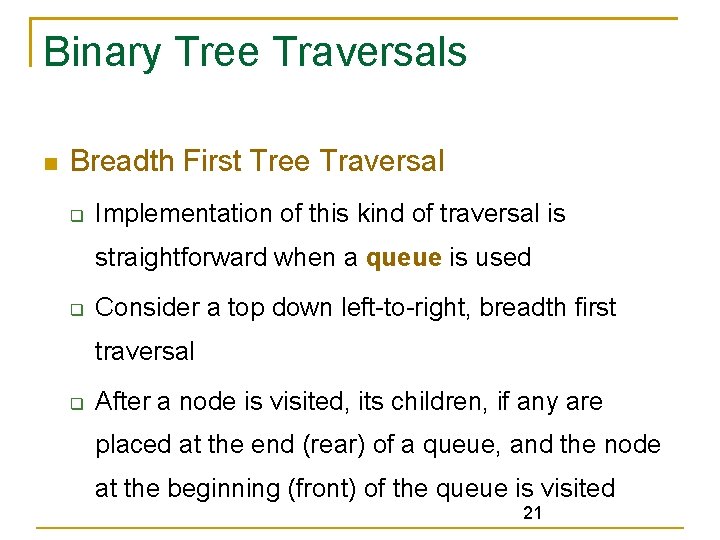
Binary Tree Traversals Breadth First Tree Traversal Implementation of this kind of traversal is straightforward when a queue is used Consider a top down left-to-right, breadth first traversal After a node is visited, its children, if any are placed at the end (rear) of a queue, and the node at the beginning (front) of the queue is visited 21
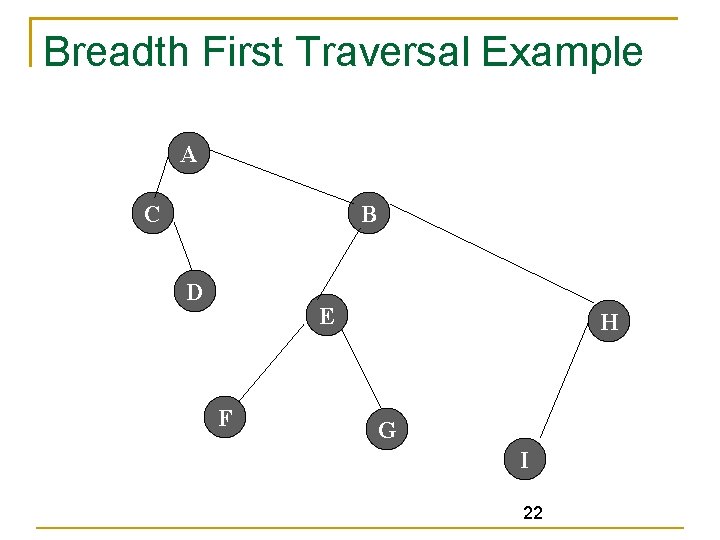
Breadth First Traversal Example A C B D E F H G I 22
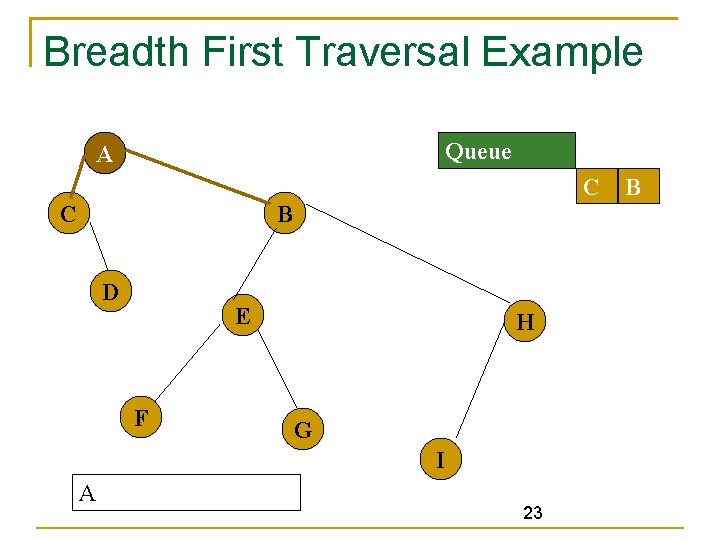
Breadth First Traversal Example Queue A C C B D E F H G I A 23 B
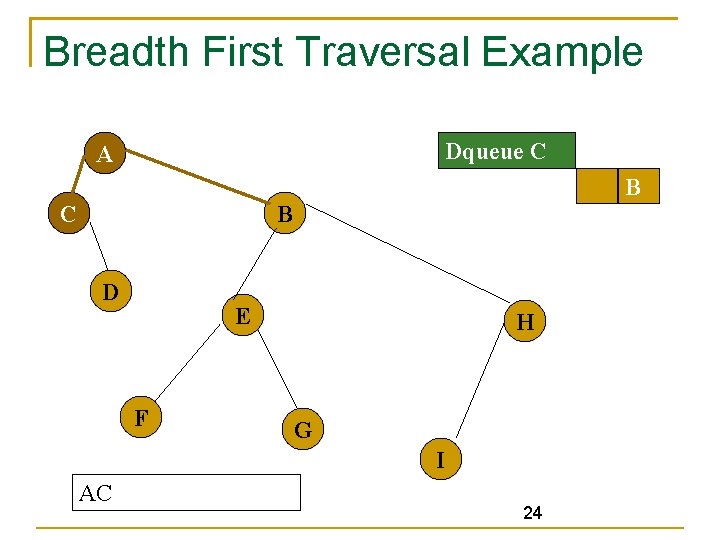
Breadth First Traversal Example Dqueue C A B C B D E F H G I AC 24
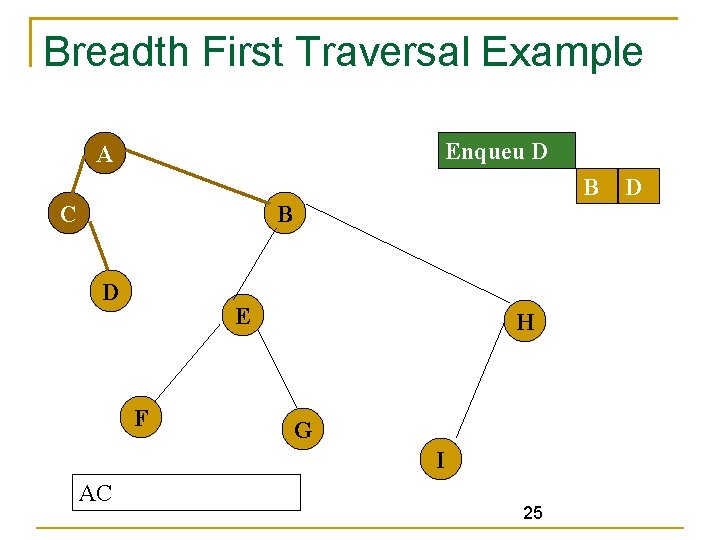
Breadth First Traversal Example Enqueu D A B C B D E F H G I AC 25 D
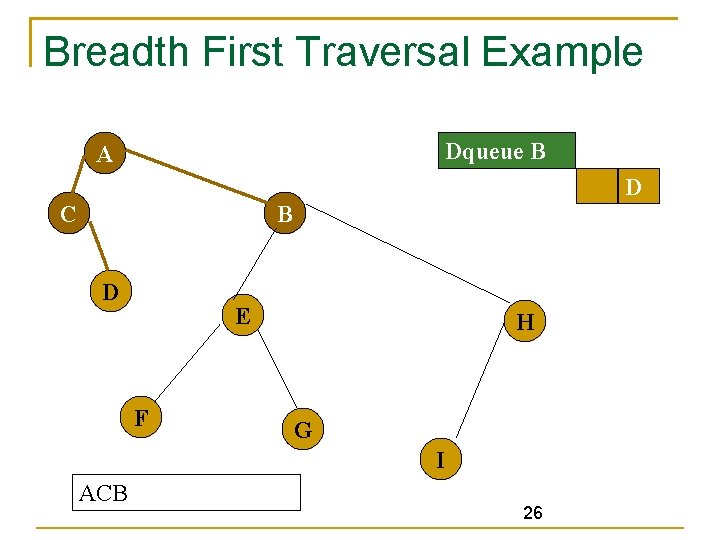
Breadth First Traversal Example Dqueue B A D C B D E F H G I ACB 26
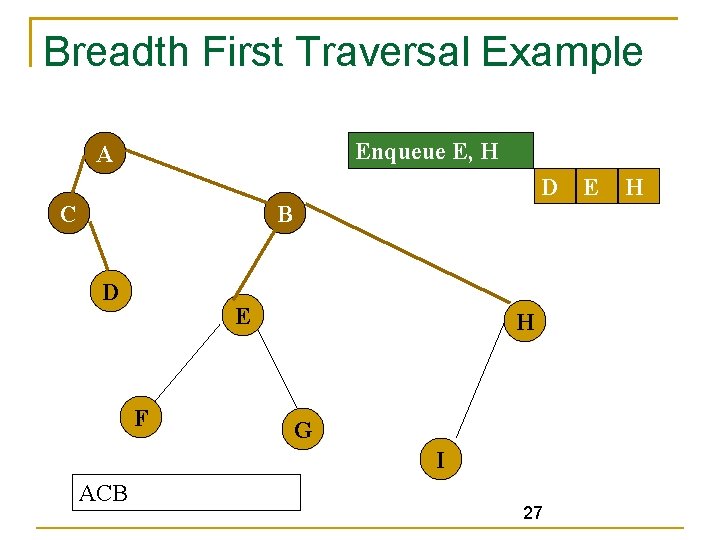
Breadth First Traversal Example Enqueue E, H A D C B D E F H G I ACB 27 E H
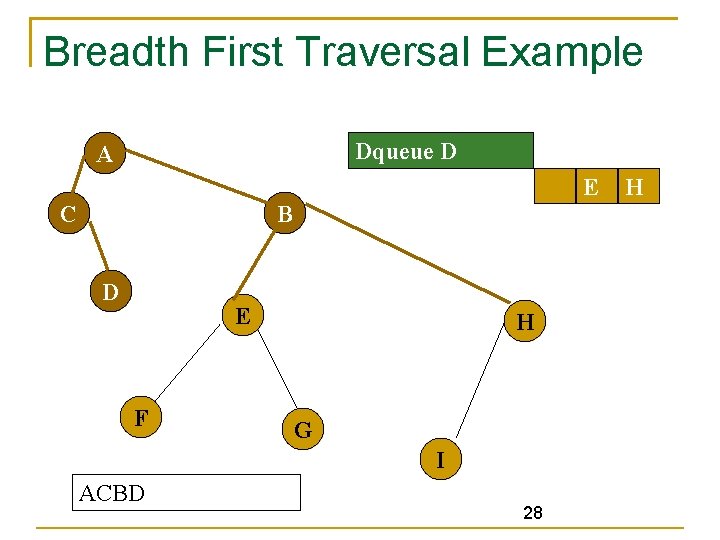
Breadth First Traversal Example Dqueue D A E C B D E F H G I ACBD 28 H
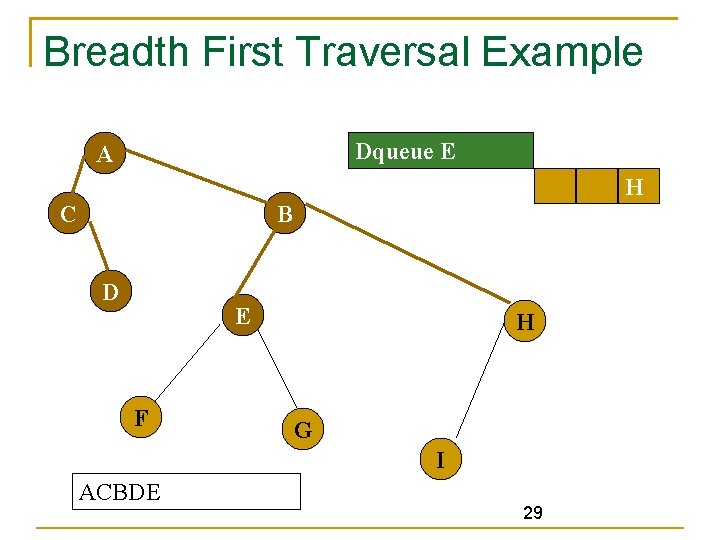
Breadth First Traversal Example Dqueue E A H C B D E F H G I ACBDE 29
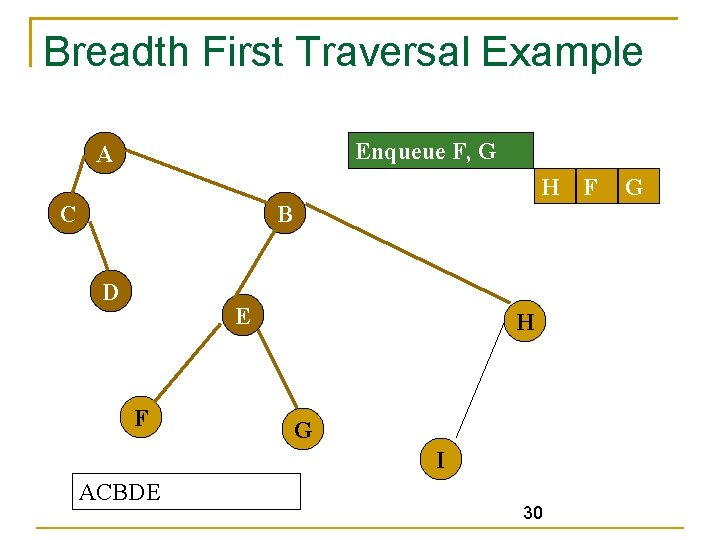
Breadth First Traversal Example Enqueue F, G A H F C B D E F H G I ACBDE 30 G
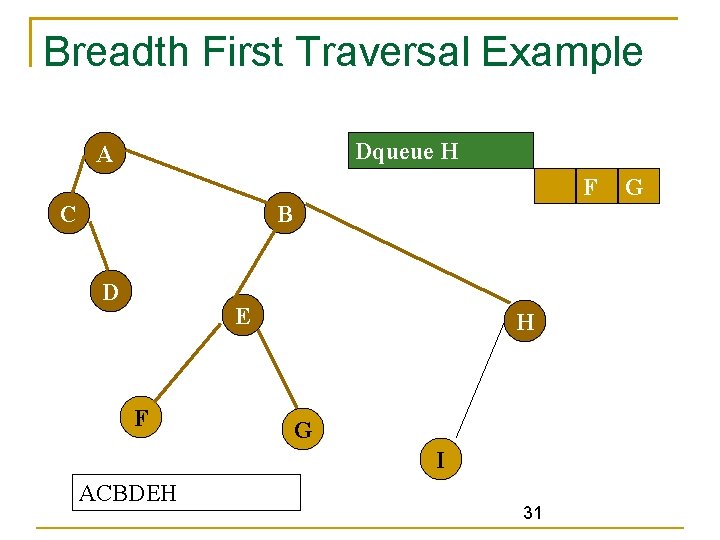
Breadth First Traversal Example Dqueue H A F C B D E F H G I ACBDEH 31 G
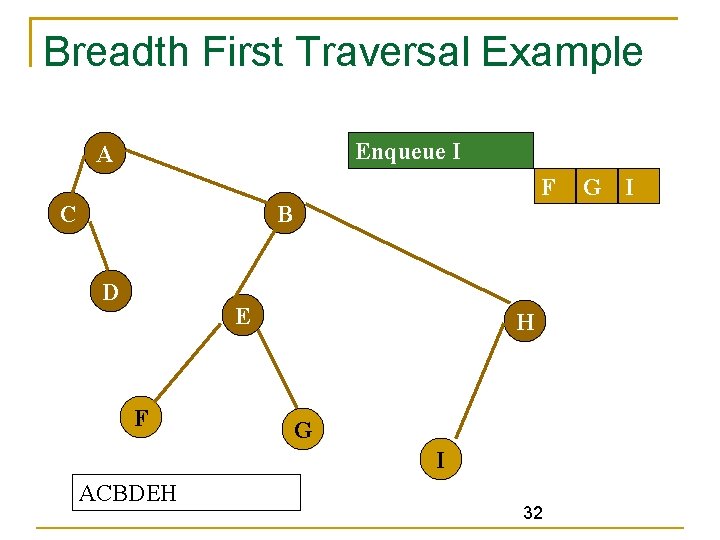
Breadth First Traversal Example Enqueue I A F C B D E F H G I ACBDEH 32 G I
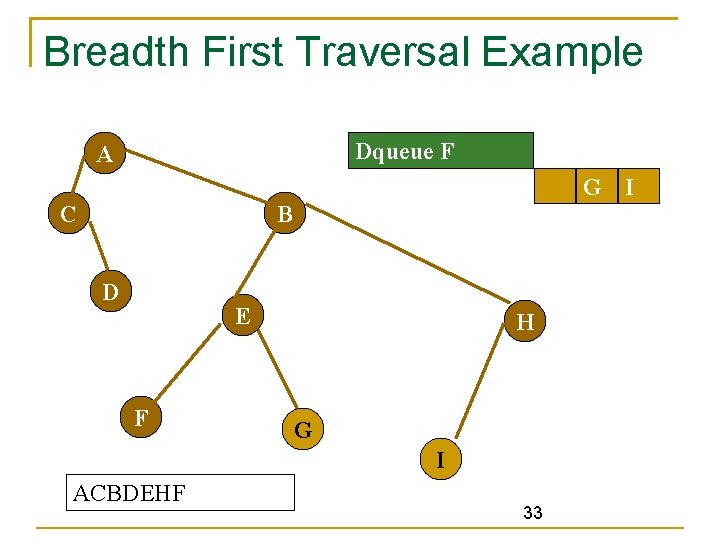
Breadth First Traversal Example Dqueue F A G I C B D E F H G I ACBDEHF 33
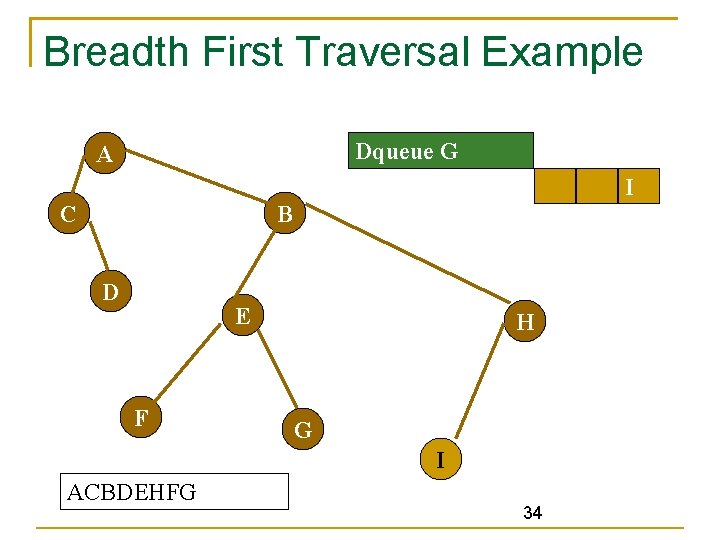
Breadth First Traversal Example Dqueue G A I C B D E F H G I ACBDEHFG 34
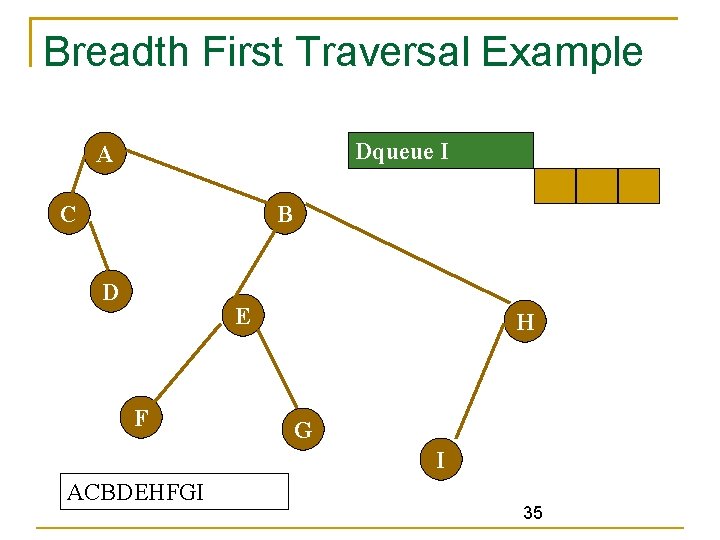
Breadth First Traversal Example Dqueue I A C B D E F H G I ACBDEHFGI 35
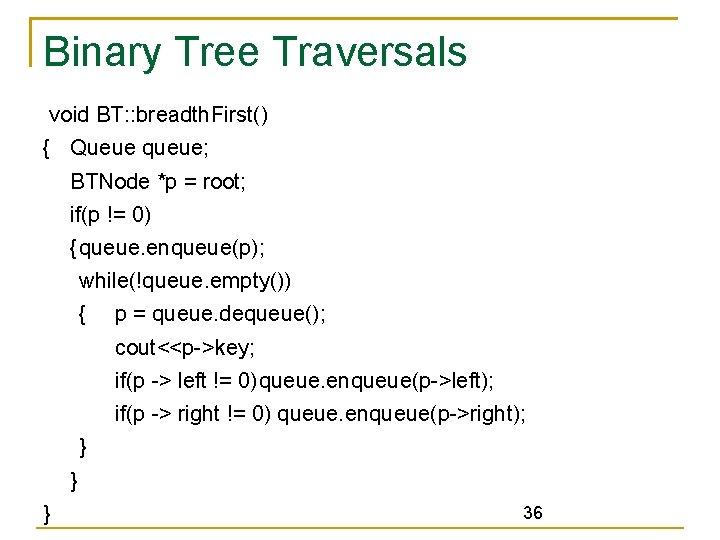
Binary Tree Traversals void BT: : breadth. First() { Queue queue; BTNode *p = root; if(p != 0) {queue. enqueue(p); while(!queue. empty()) { p = queue. dequeue(); cout<<p->key; if(p -> left != 0)queue. enqueue(p->left); if(p -> right != 0) queue. enqueue(p->right); } } } 36
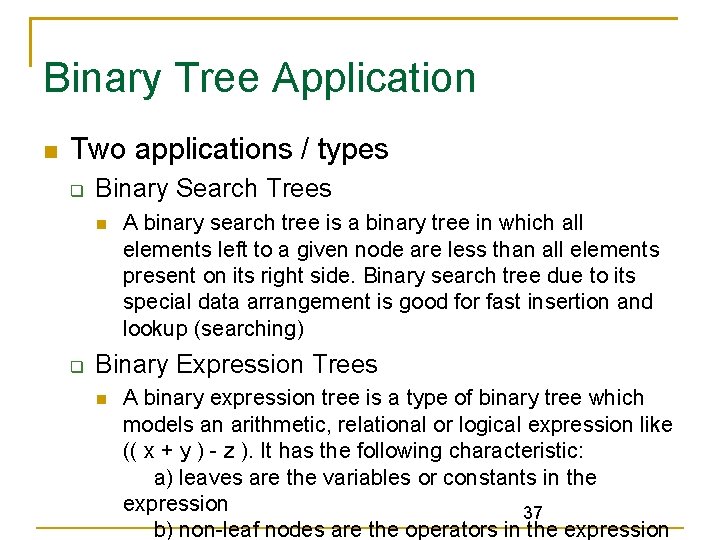
Binary Tree Application Two applications / types Binary Search Trees A binary search tree is a binary tree in which all elements left to a given node are less than all elements present on its right side. Binary search tree due to its special data arrangement is good for fast insertion and lookup (searching) Binary Expression Trees A binary expression tree is a type of binary tree which models an arithmetic, relational or logical expression like (( x + y ) - z ). It has the following characteristic: a) leaves are the variables or constants in the expression 37 b) non-leaf nodes are the operators in the expression
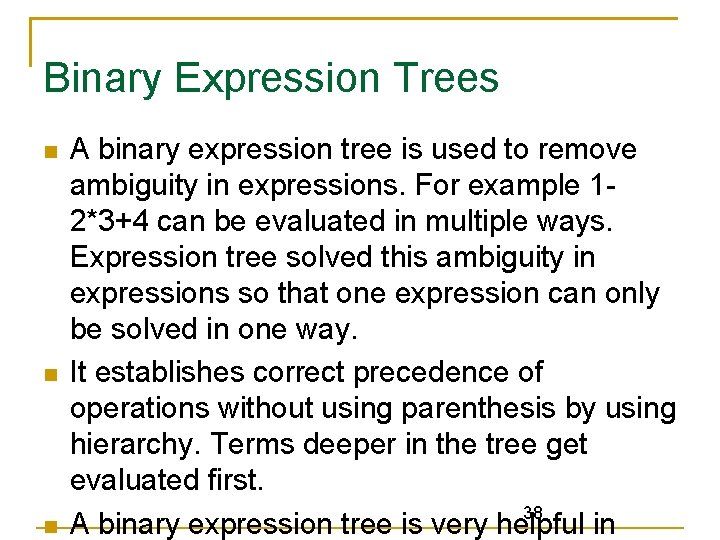
Binary Expression Trees A binary expression tree is used to remove ambiguity in expressions. For example 12*3+4 can be evaluated in multiple ways. Expression tree solved this ambiguity in expressions so that one expression can only be solved in one way. It establishes correct precedence of operations without using parenthesis by using hierarchy. Terms deeper in the tree get evaluated first. 38 A binary expression tree is very helpful in
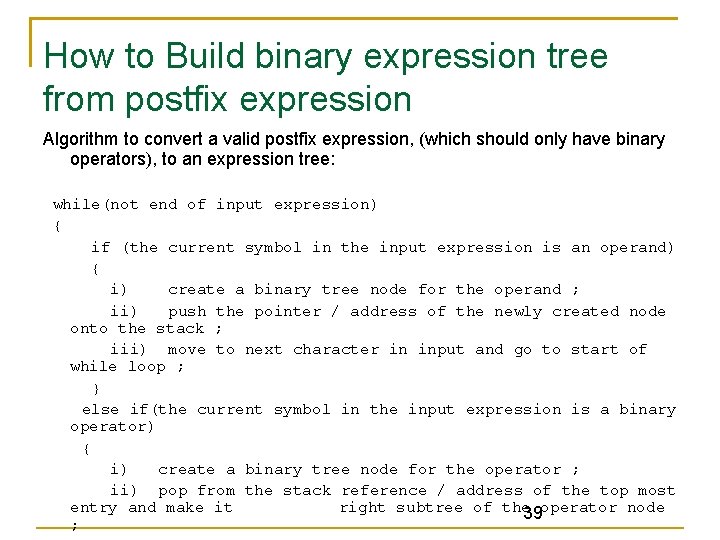
How to Build binary expression tree from postfix expression Algorithm to convert a valid postfix expression, (which should only have binary operators), to an expression tree: while(not end of input expression) { if (the current symbol in the input expression is an operand) { i) create a binary tree node for the operand ; ii) push the pointer / address of the newly created node onto the stack ; iii) move to next character in input and go to start of while loop ; } else if(the current symbol in the input expression is a binary operator) { i) create a binary tree node for the operator ; ii) pop from the stack reference / address of the top most entry and make it right subtree of the 39 operator node ;
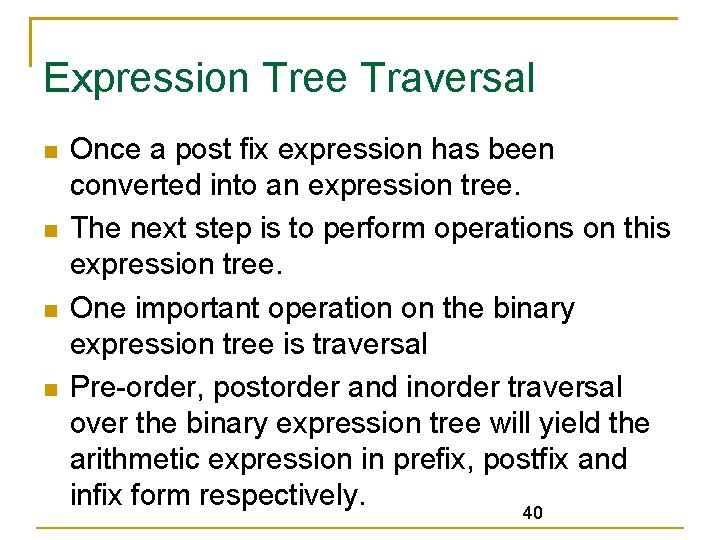
Expression Tree Traversal Once a post fix expression has been converted into an expression tree. The next step is to perform operations on this expression tree. One important operation on the binary expression tree is traversal Pre-order, postorder and inorder traversal over the binary expression tree will yield the arithmetic expression in prefix, postfix and infix form respectively. 40
Professor ajit diwan
Cos423
Data structures and algorithms tutorial
Information retrieval data structures and algorithms
Data structures and algorithms bits pilani
Ajit diwan iit bombay
Data structures and algorithms
Data structures and algorithms
Waterloo data structures and algorithms
Information retrieval data structures and algorithms
Data structures and algorithms
Algorithms + data structures = programs
Homologous structures definition
Sandwich quotes examples
Q=vc physics
Physics 212 gradebook
Pese 212
Nkb 212 sungguh inginkah engkau lakukan
Ienf 212
Et 212
Et 212
12345 6789 10
Archimedes ( arşimet) (mö 287–212 )
287 bc
Phys 212 equation sheet
212 instalaciones tecnicas ejemplos
Attiny 212
Có 3 thùng dầu mỗi thùng chứa 125l tóm tắt
Grafik hubungan suhu dan kalor
Naca 63-212
Tipos de arco para aislamiento absoluto
Cls 212
Naca 4421
Disadvantages of fermentation
Cmpe 212
212
212
Cls 212
Ps 212 midtown west
Ables curriculum
Garra de arquímedes