CS 212 Data Structures and Algorithms Lecture 4
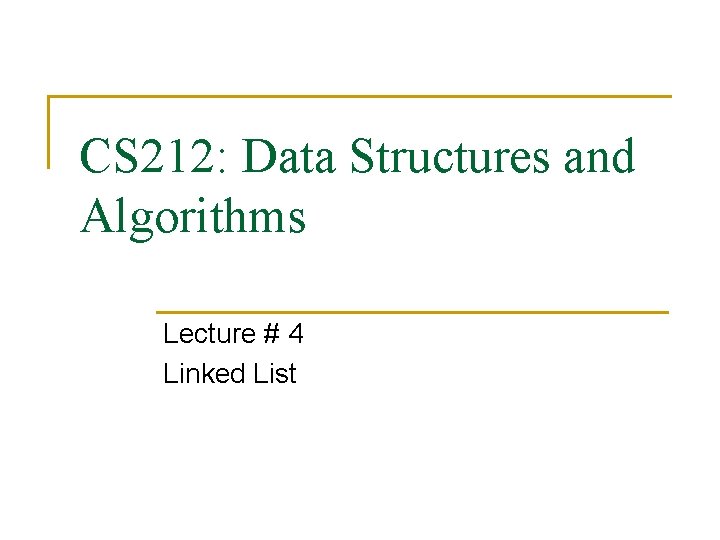
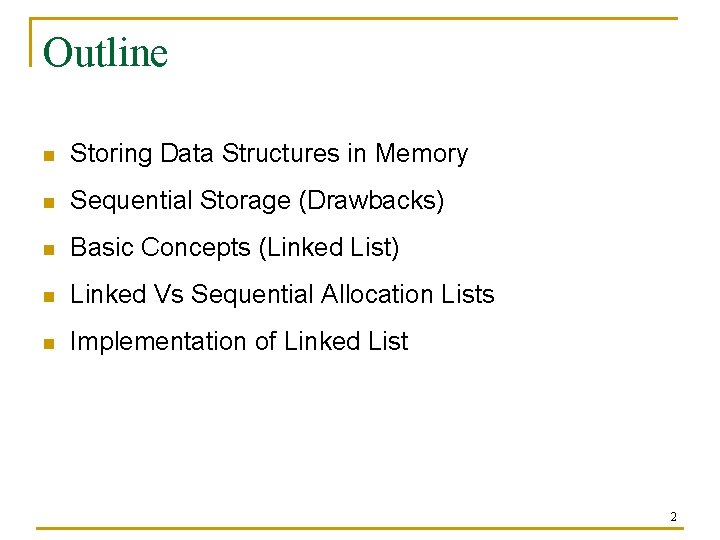
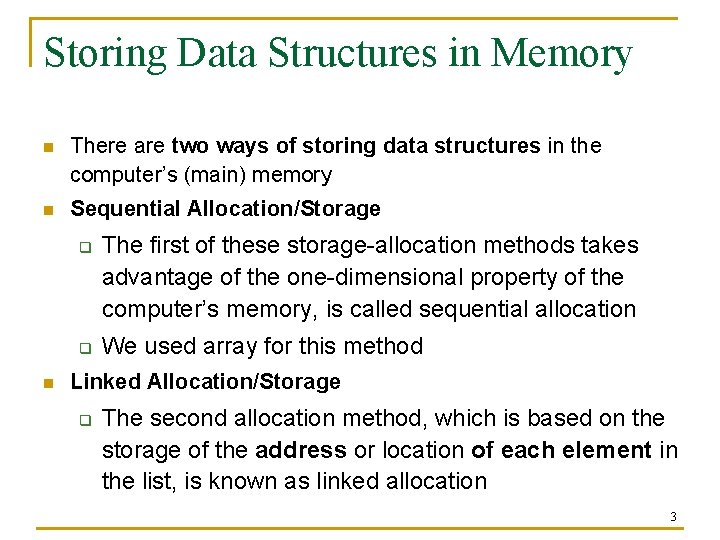
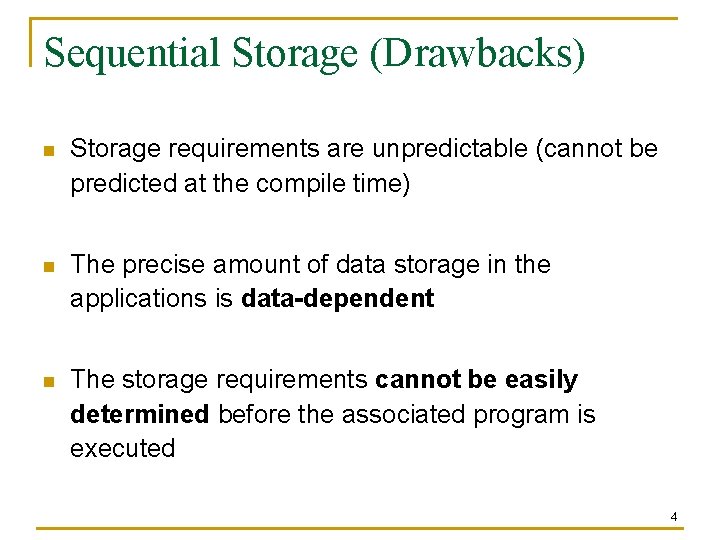
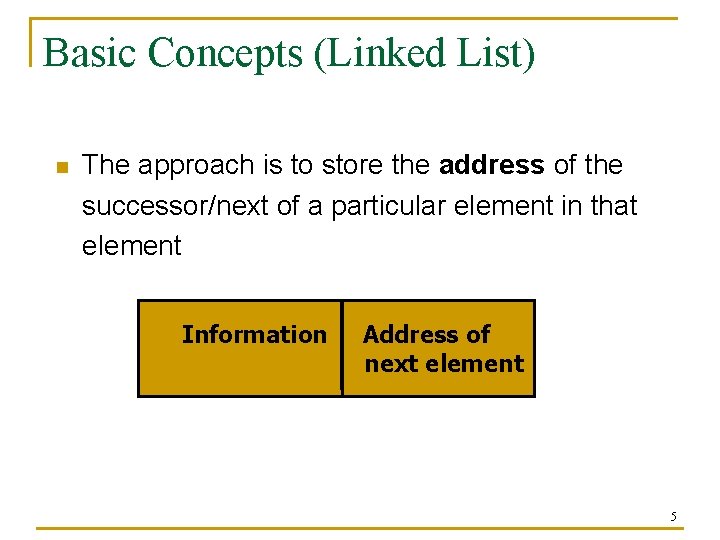
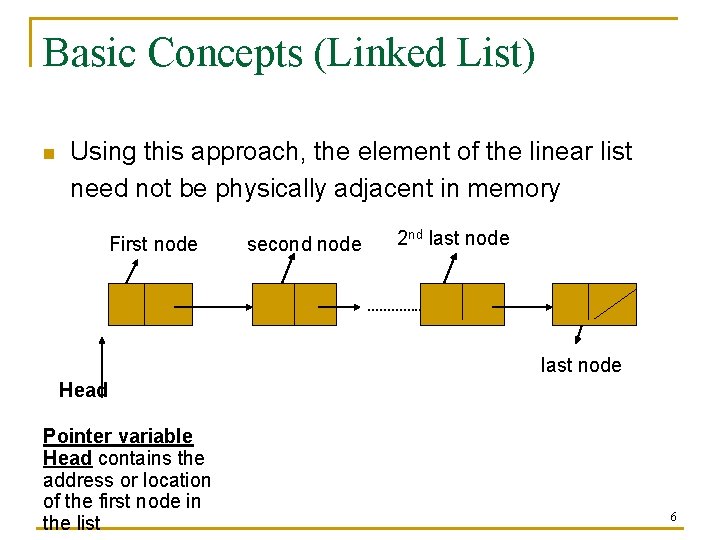
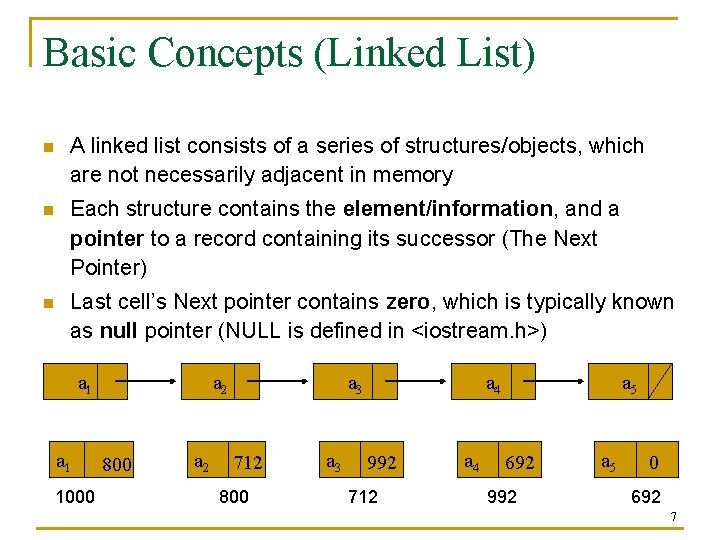
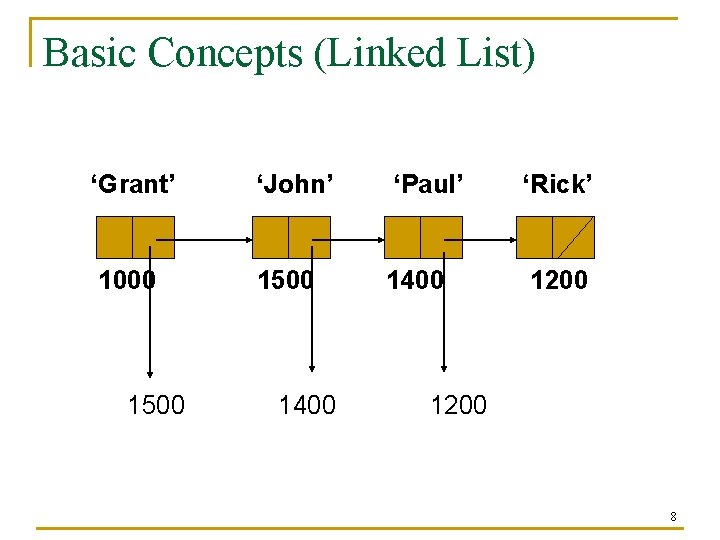
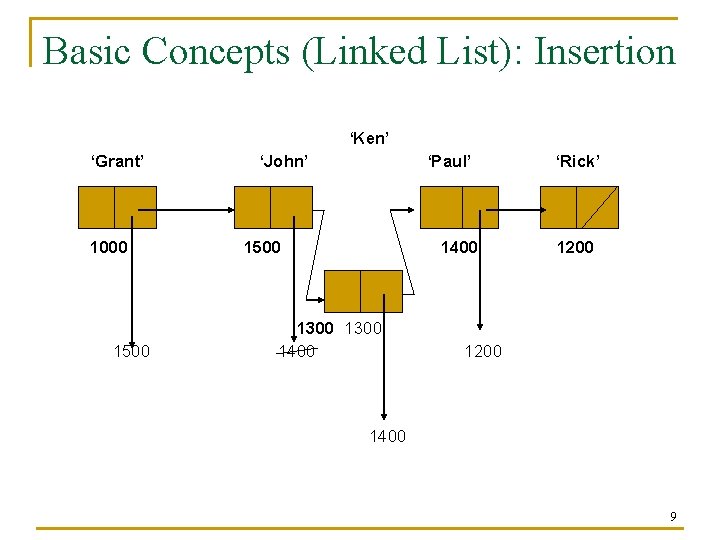
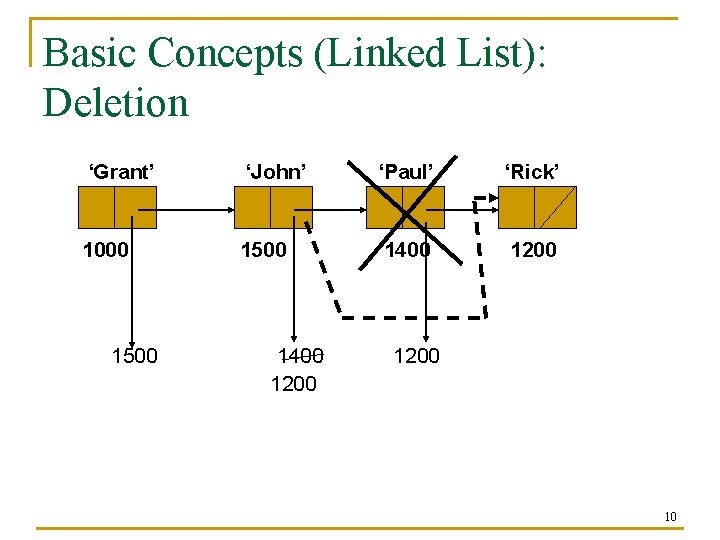
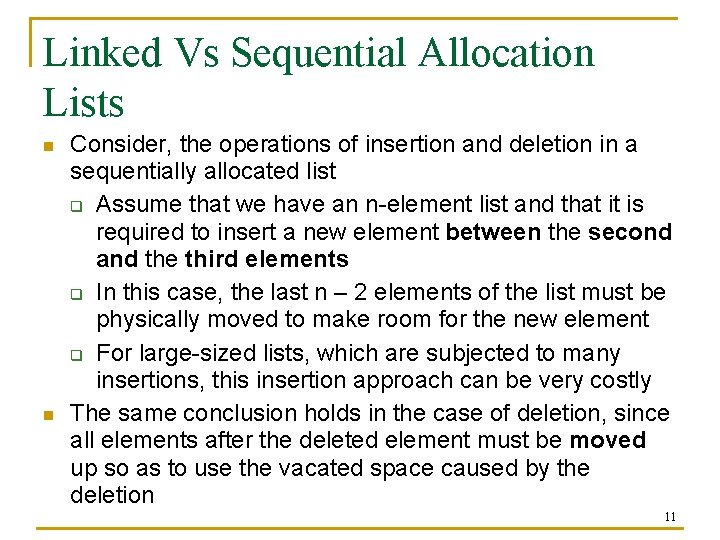
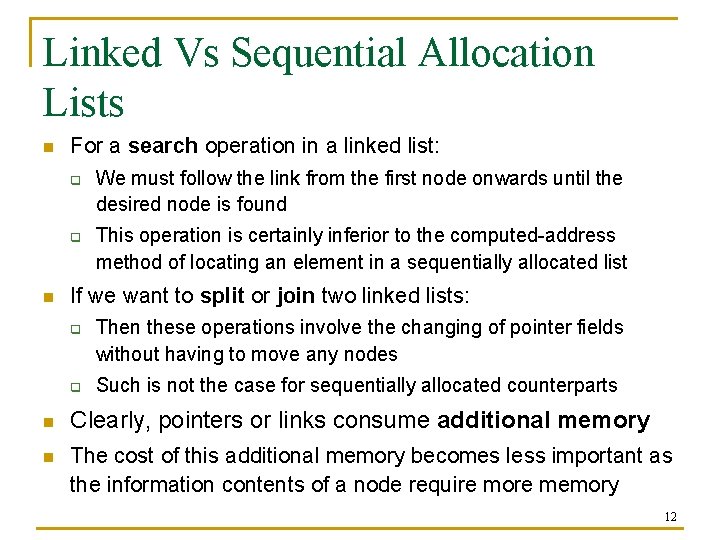
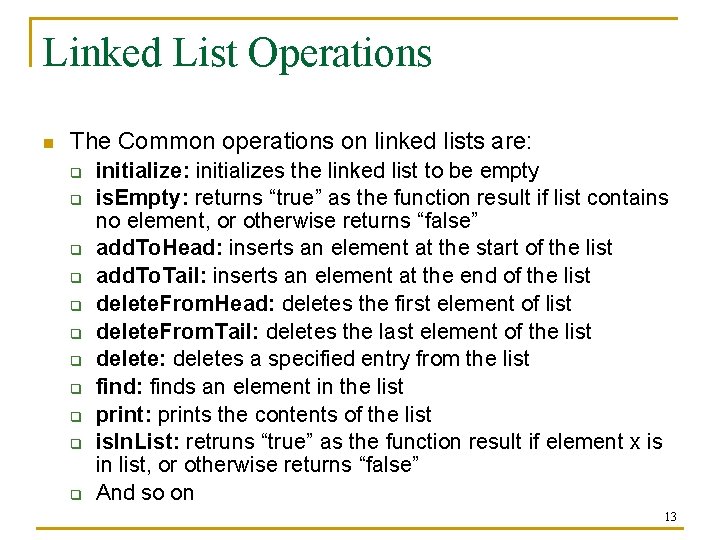
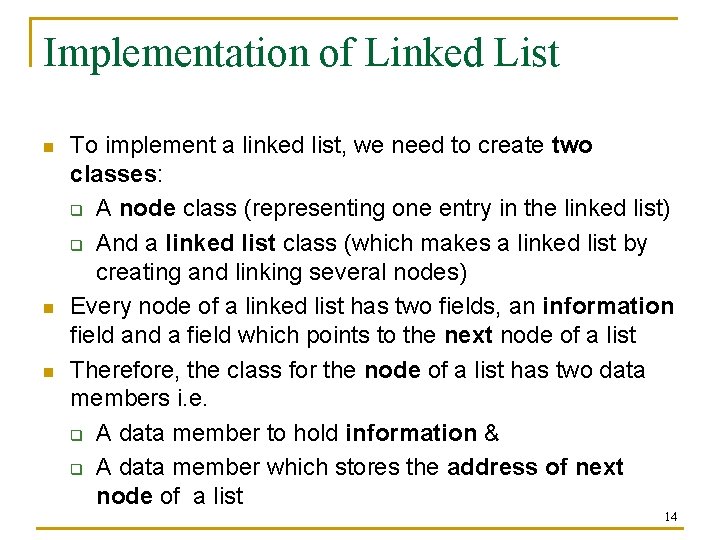
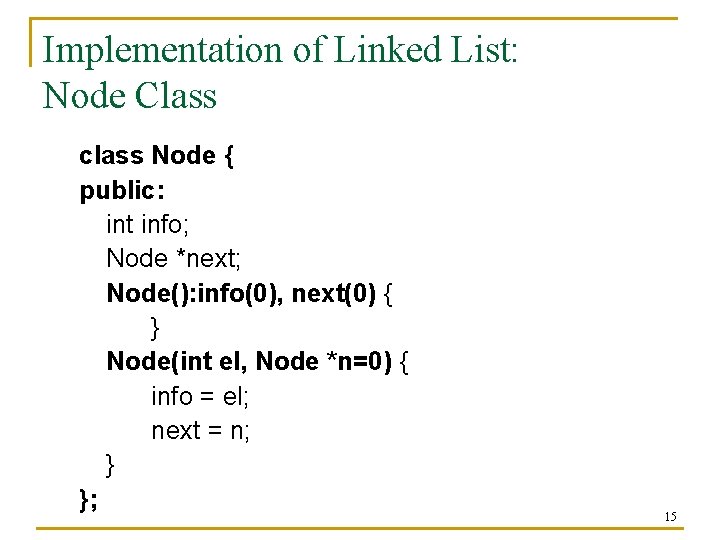
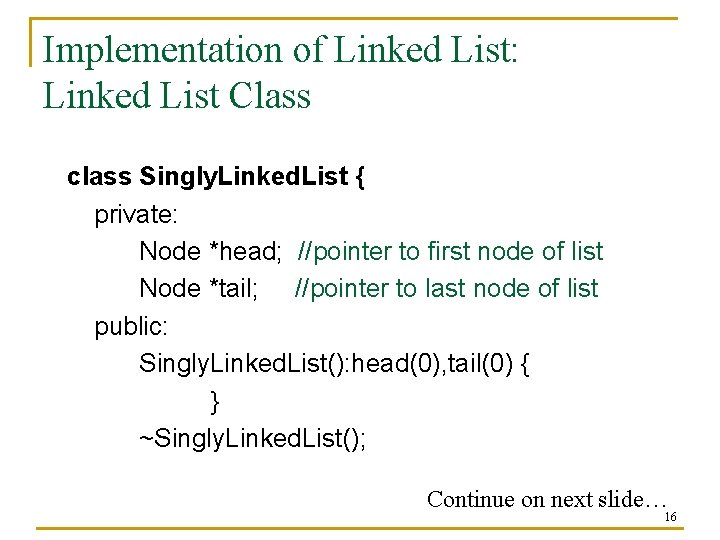
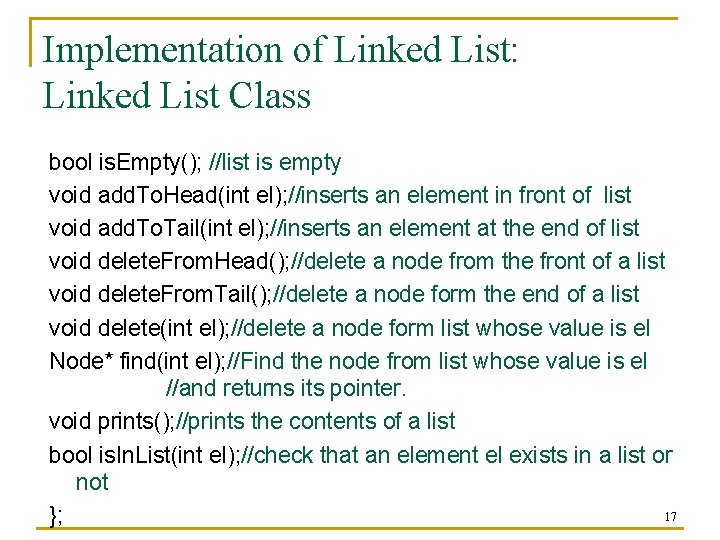
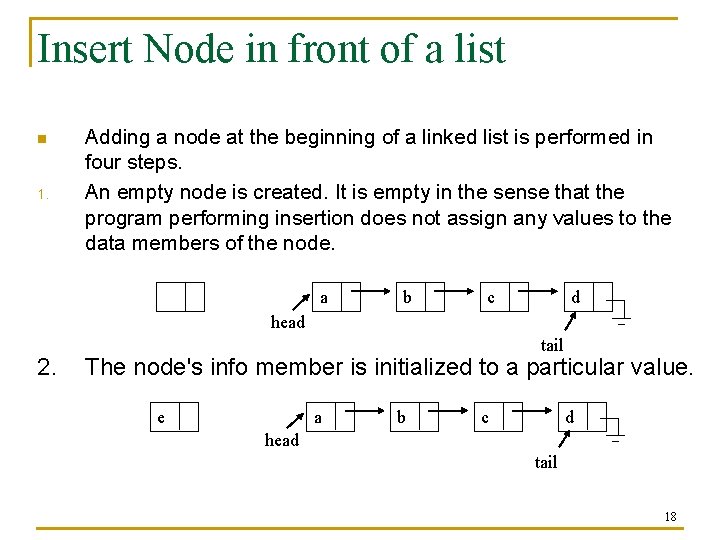
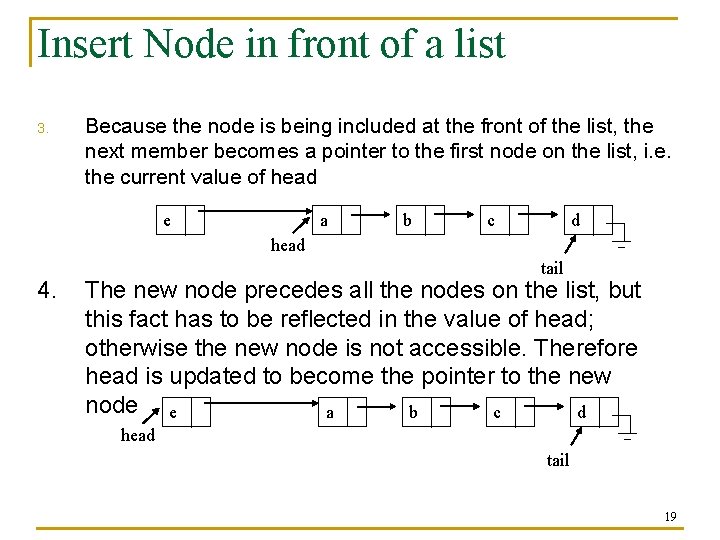
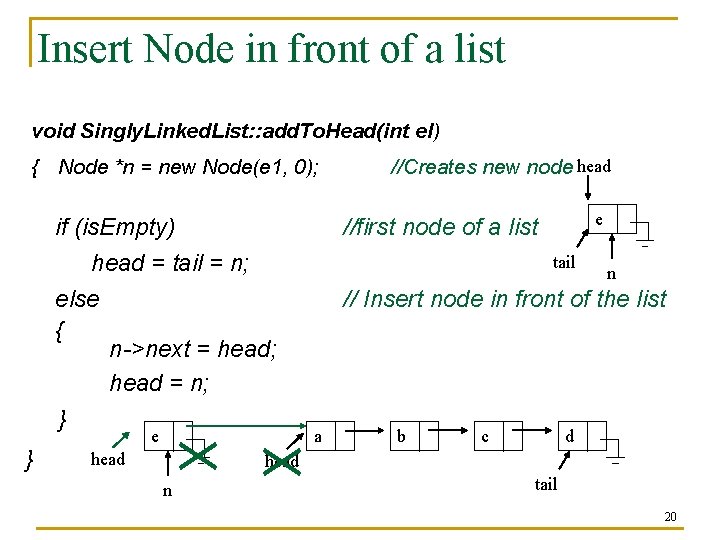
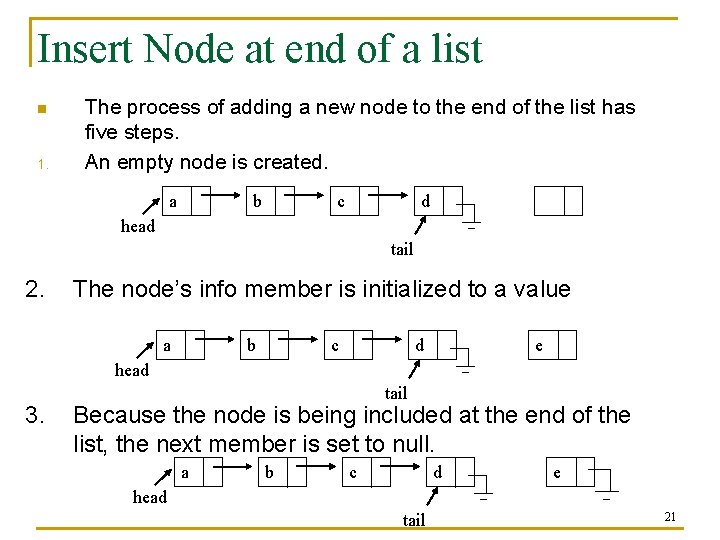
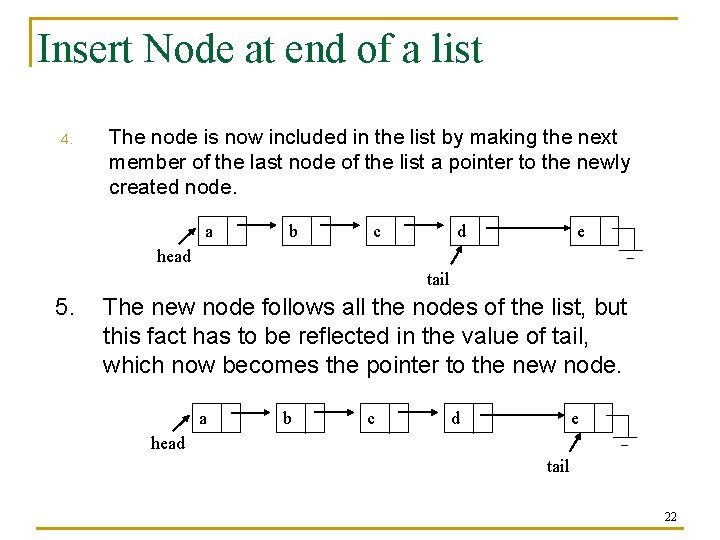
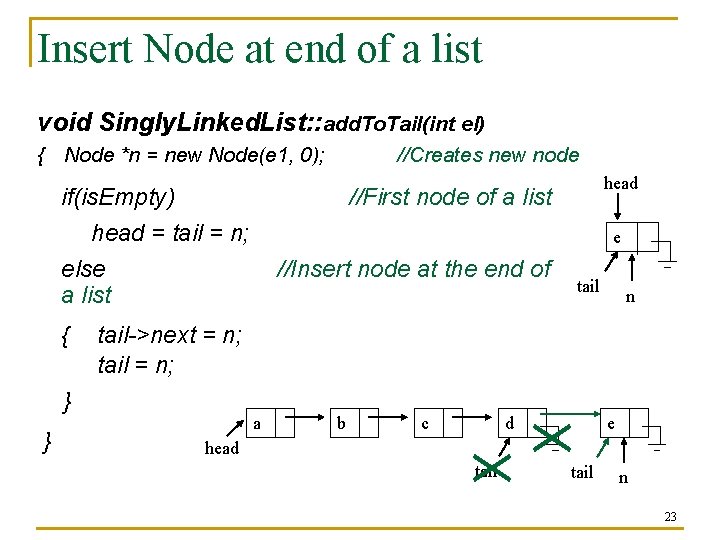
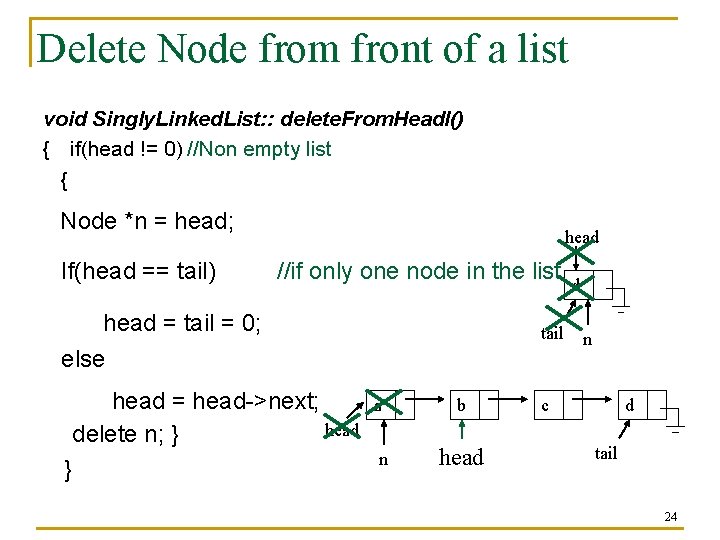
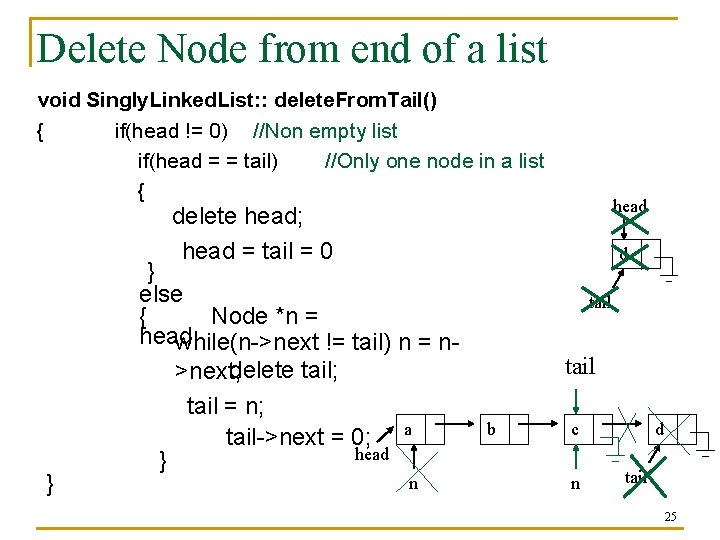
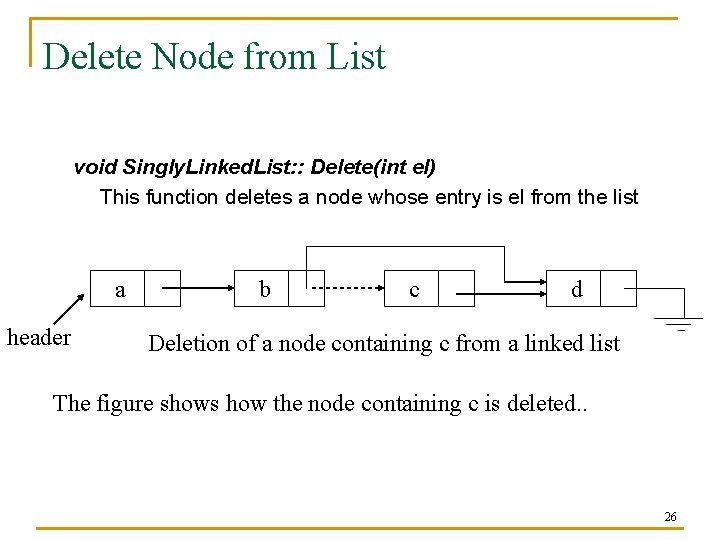
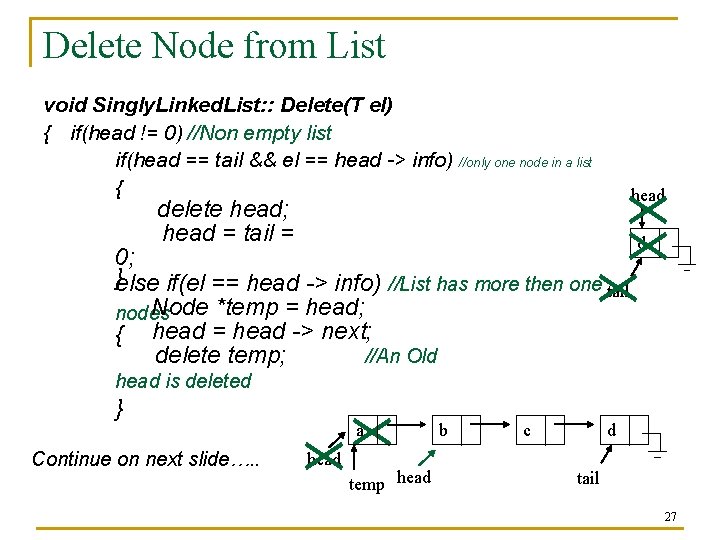
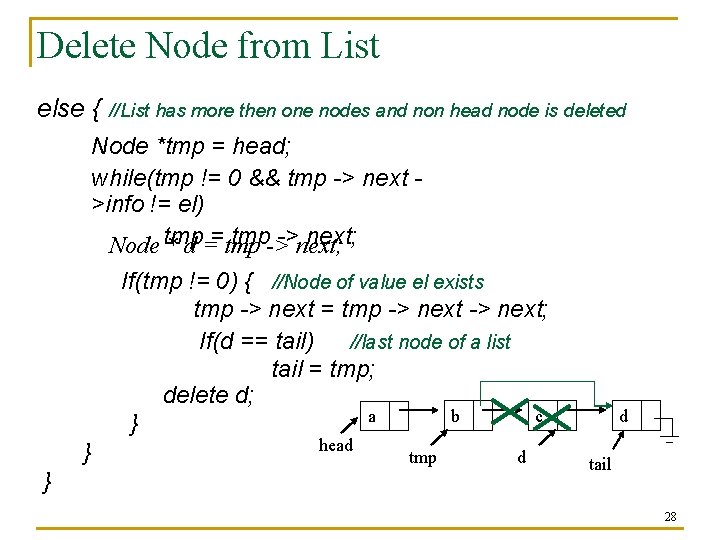
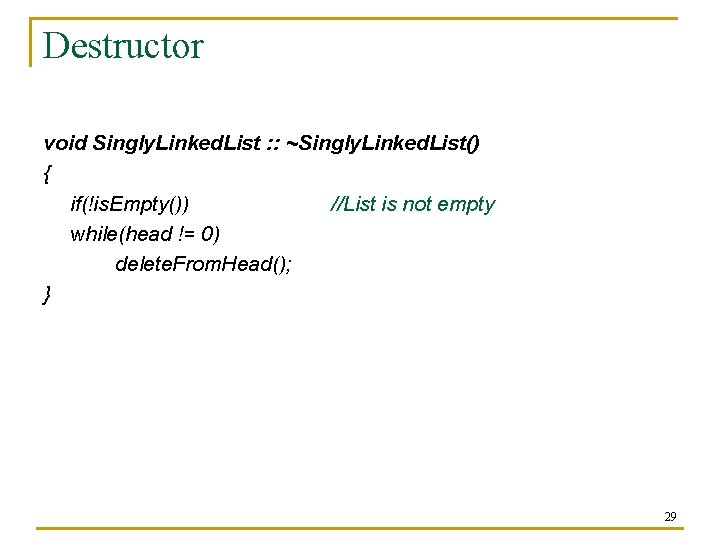
- Slides: 29
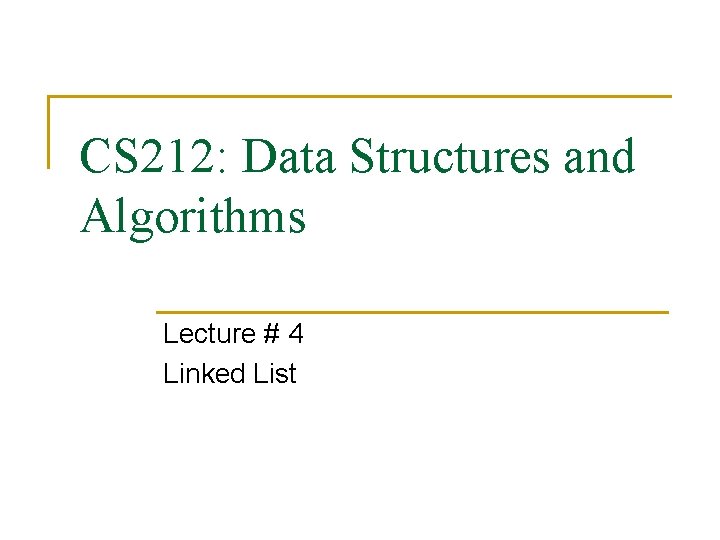
CS 212: Data Structures and Algorithms Lecture # 4 Linked List
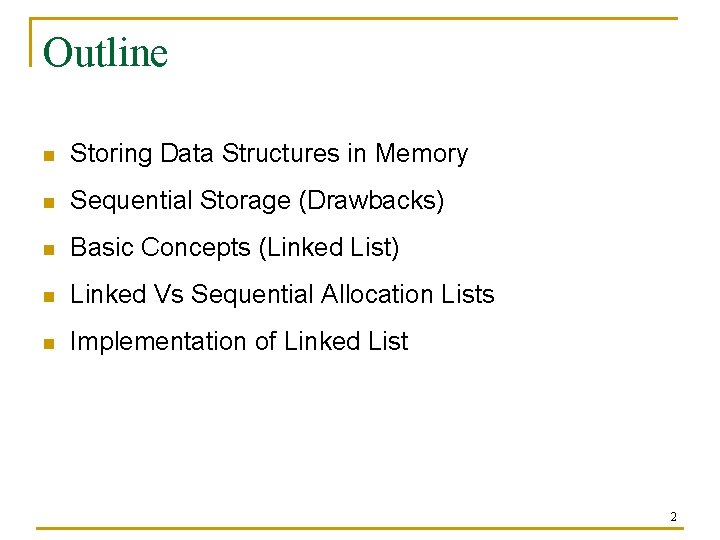
Outline n Storing Data Structures in Memory n Sequential Storage (Drawbacks) n Basic Concepts (Linked List) n Linked Vs Sequential Allocation Lists n Implementation of Linked List 2
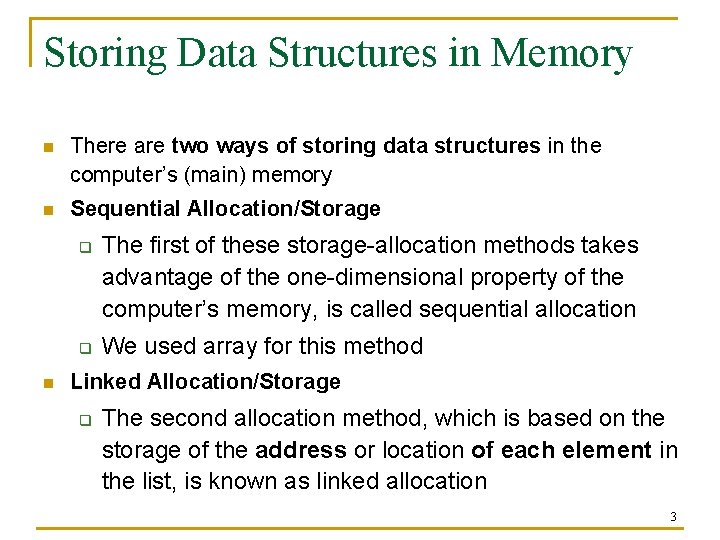
Storing Data Structures in Memory n There are two ways of storing data structures in the computer’s (main) memory n Sequential Allocation/Storage q q n The first of these storage-allocation methods takes advantage of the one-dimensional property of the computer’s memory, is called sequential allocation We used array for this method Linked Allocation/Storage q The second allocation method, which is based on the storage of the address or location of each element in the list, is known as linked allocation 3
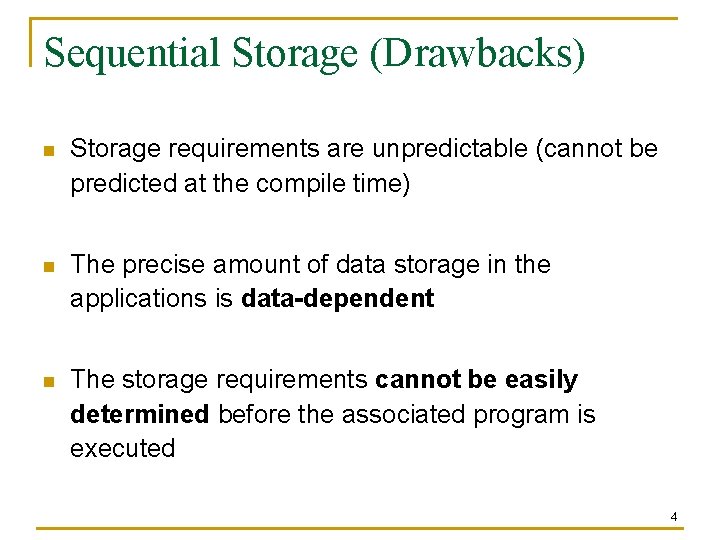
Sequential Storage (Drawbacks) n Storage requirements are unpredictable (cannot be predicted at the compile time) n The precise amount of data storage in the applications is data-dependent n The storage requirements cannot be easily determined before the associated program is executed 4
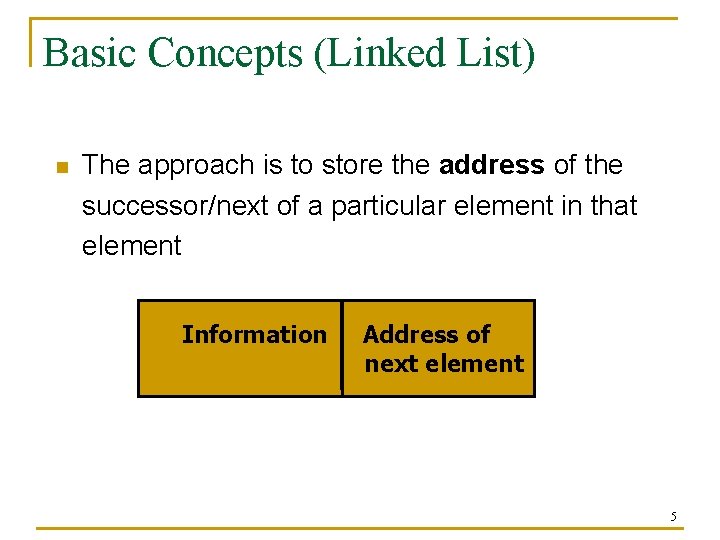
Basic Concepts (Linked List) n The approach is to store the address of the successor/next of a particular element in that element Information Address of next element 5
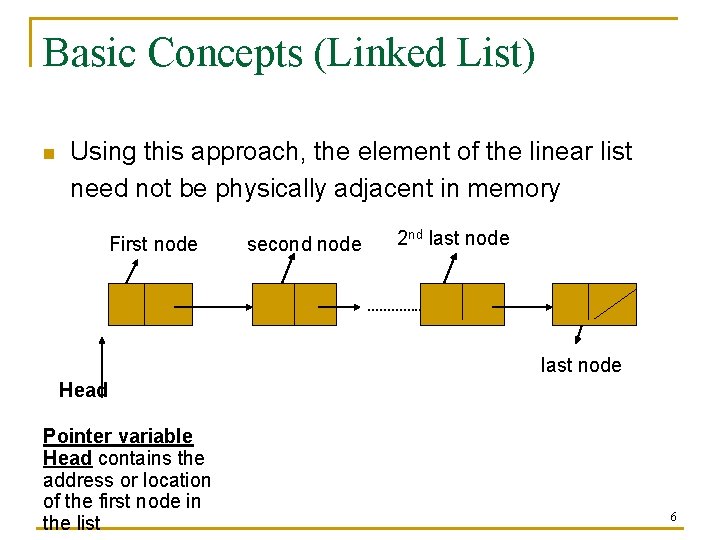
Basic Concepts (Linked List) n Using this approach, the element of the linear list need not be physically adjacent in memory First node second node 2 nd last node …………. . last node Head Pointer variable Head contains the address or location of the first node in the list 6
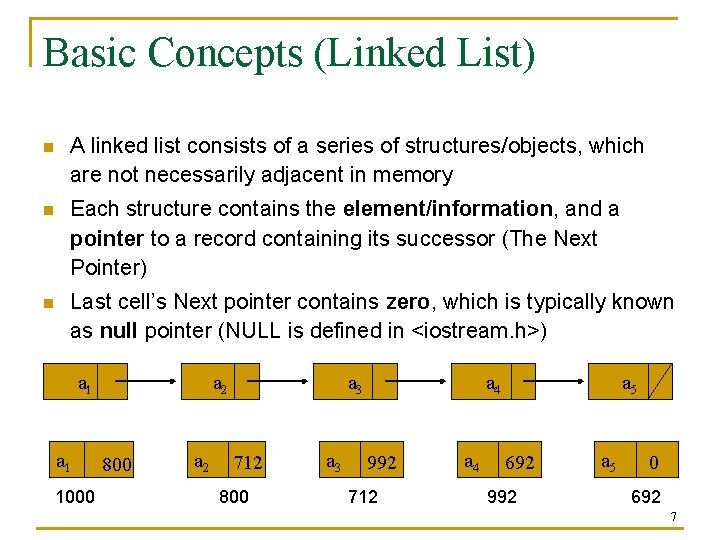
Basic Concepts (Linked List) n A linked list consists of a series of structures/objects, which are not necessarily adjacent in memory n Each structure contains the element/information, and a pointer to a record containing its successor (The Next Pointer) n Last cell’s Next pointer contains zero, which is typically known as null pointer (NULL is defined in <iostream. h>) a 1 1000 a 2 800 a 2 a 3 712 800 a 3 a 4 992 712 a 4 a 5 692 992 a 5 0 692 7
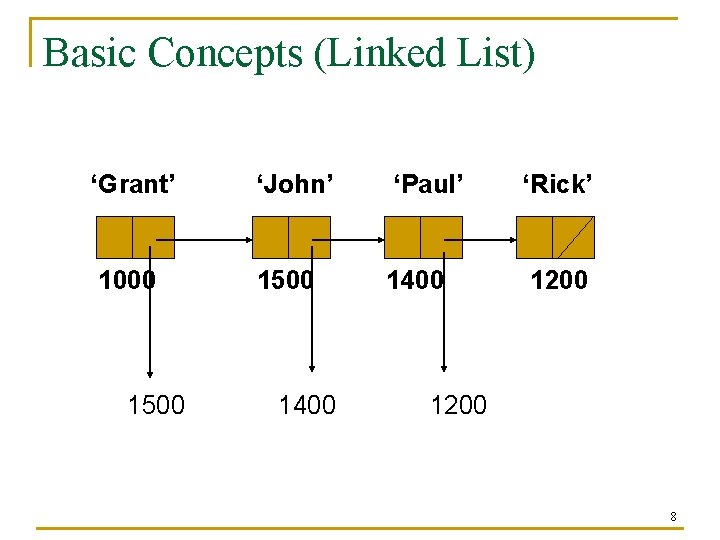
Basic Concepts (Linked List) ‘Grant’ ‘John’ ‘Paul’ ‘Rick’ 1000 1500 1400 1200 8
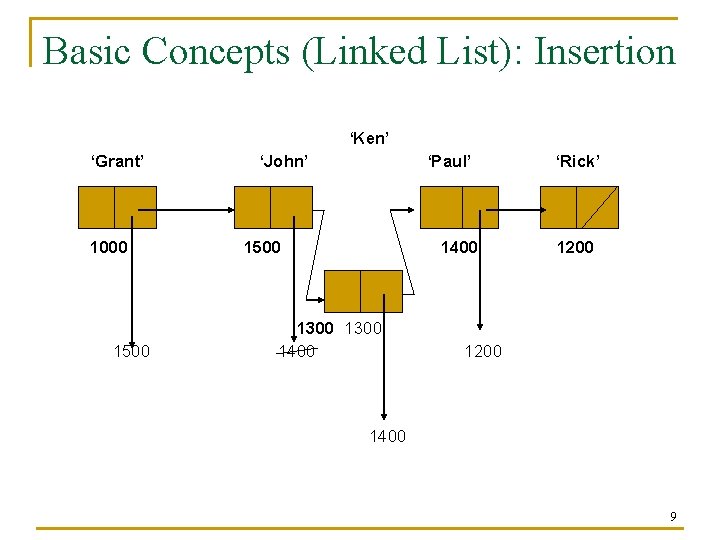
Basic Concepts (Linked List): Insertion ‘Ken’ ‘Grant’ 1000 1500 ‘John’ ‘Paul’ 1500 1400 1300 1400 ‘Rick’ 1200 1400 9
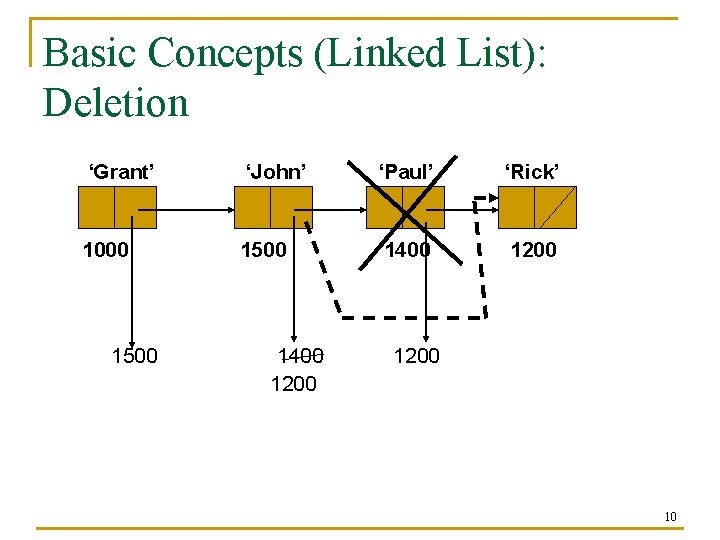
Basic Concepts (Linked List): Deletion ‘Grant’ ‘John’ ‘Paul’ ‘Rick’ 1000 1500 1400 1200 10
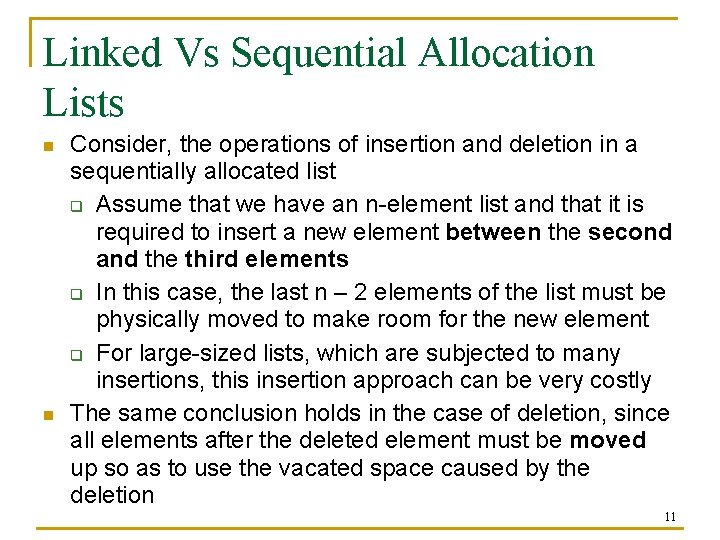
Linked Vs Sequential Allocation Lists n n Consider, the operations of insertion and deletion in a sequentially allocated list q Assume that we have an n-element list and that it is required to insert a new element between the second and the third elements q In this case, the last n – 2 elements of the list must be physically moved to make room for the new element q For large-sized lists, which are subjected to many insertions, this insertion approach can be very costly The same conclusion holds in the case of deletion, since all elements after the deleted element must be moved up so as to use the vacated space caused by the deletion 11
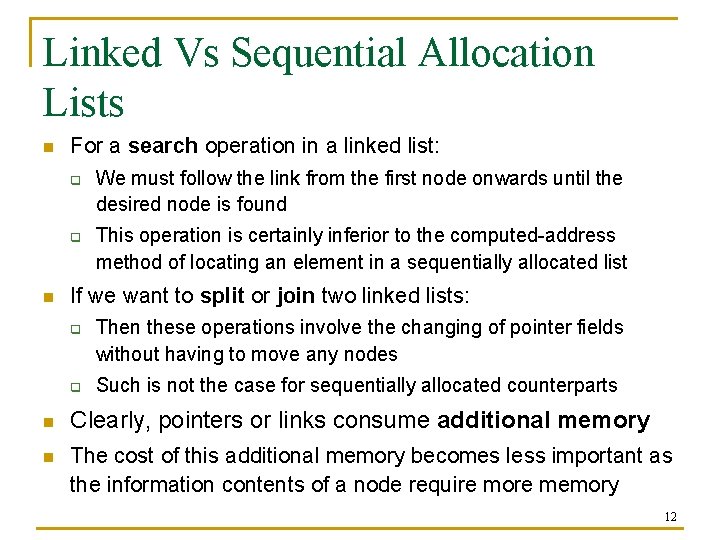
Linked Vs Sequential Allocation Lists n For a search operation in a linked list: q q n We must follow the link from the first node onwards until the desired node is found This operation is certainly inferior to the computed-address method of locating an element in a sequentially allocated list If we want to split or join two linked lists: q q Then these operations involve the changing of pointer fields without having to move any nodes Such is not the case for sequentially allocated counterparts n Clearly, pointers or links consume additional memory n The cost of this additional memory becomes less important as the information contents of a node require more memory 12
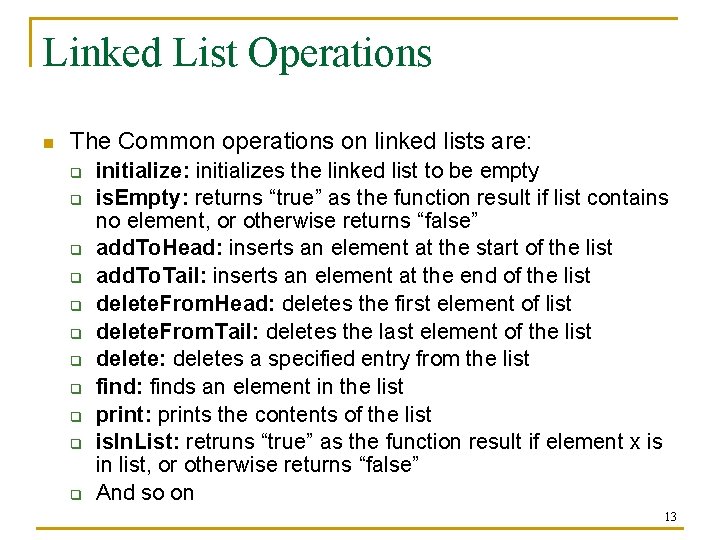
Linked List Operations n The Common operations on linked lists are: q q q initialize: initializes the linked list to be empty is. Empty: returns “true” as the function result if list contains no element, or otherwise returns “false” add. To. Head: inserts an element at the start of the list add. To. Tail: inserts an element at the end of the list delete. From. Head: deletes the first element of list delete. From. Tail: deletes the last element of the list delete: deletes a specified entry from the list find: finds an element in the list print: prints the contents of the list is. In. List: retruns “true” as the function result if element x is in list, or otherwise returns “false” And so on 13
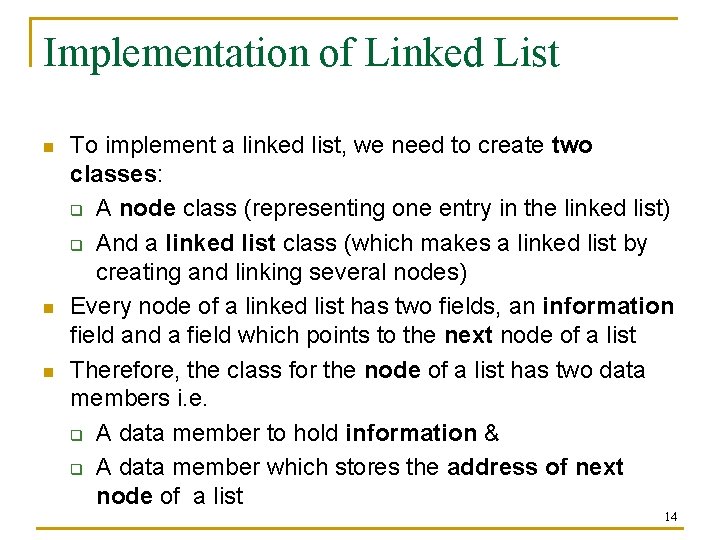
Implementation of Linked List n n n To implement a linked list, we need to create two classes: q A node class (representing one entry in the linked list) q And a linked list class (which makes a linked list by creating and linking several nodes) Every node of a linked list has two fields, an information field and a field which points to the next node of a list Therefore, the class for the node of a list has two data members i. e. q A data member to hold information & q A data member which stores the address of next node of a list 14
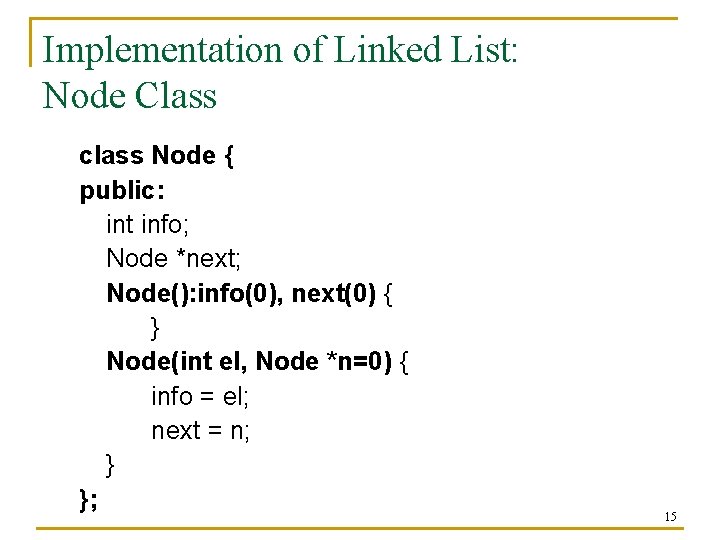
Implementation of Linked List: Node Class class Node { public: int info; Node *next; Node(): info(0), next(0) { } Node(int el, Node *n=0) { info = el; next = n; } }; 15
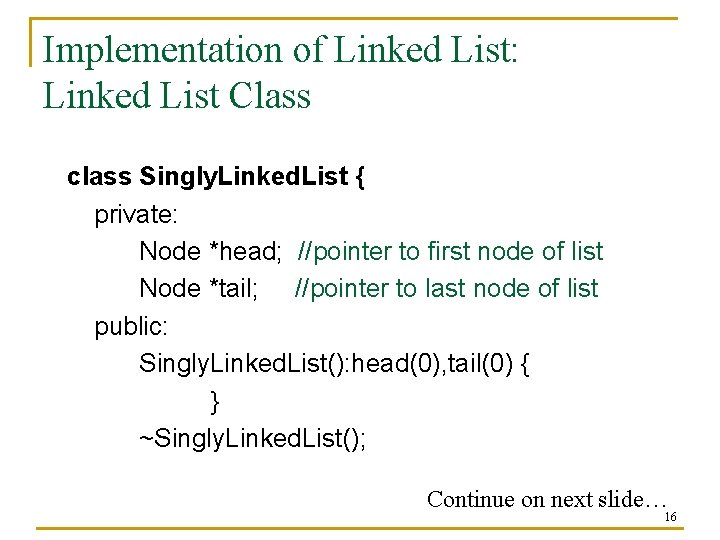
Implementation of Linked List: Linked List Class class Singly. Linked. List { private: Node *head; //pointer to first node of list Node *tail; //pointer to last node of list public: Singly. Linked. List(): head(0), tail(0) { } ~Singly. Linked. List(); Continue on next slide… 16
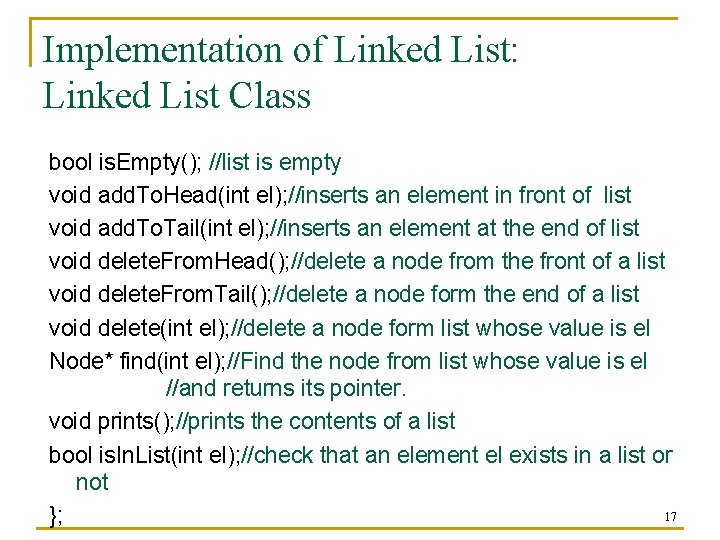
Implementation of Linked List: Linked List Class bool is. Empty(); //list is empty void add. To. Head(int el); //inserts an element in front of list void add. To. Tail(int el); //inserts an element at the end of list void delete. From. Head(); //delete a node from the front of a list void delete. From. Tail(); //delete a node form the end of a list void delete(int el); //delete a node form list whose value is el Node* find(int el); //Find the node from list whose value is el //and returns its pointer. void prints(); //prints the contents of a list bool is. In. List(int el); //check that an element el exists in a list or not 17 };
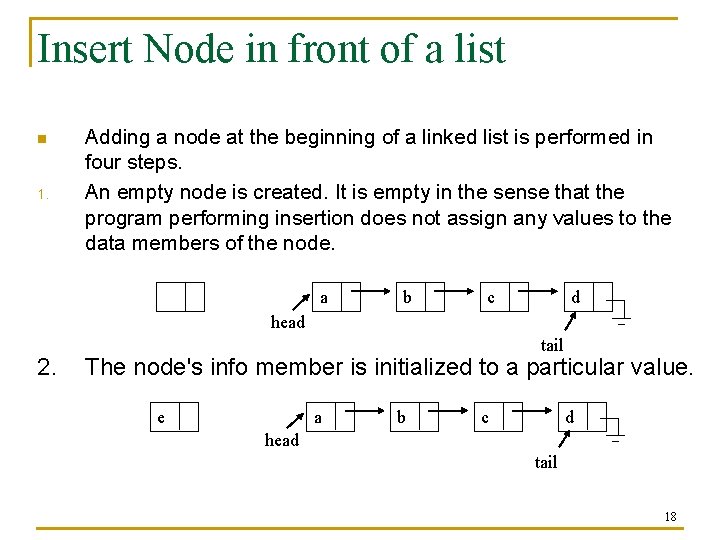
Insert Node in front of a list n 1. Adding a node at the beginning of a linked list is performed in four steps. An empty node is created. It is empty in the sense that the program performing insertion does not assign any values to the data members of the node. a b c d head 2. tail The node's info member is initialized to a particular value. e a b c d head tail 18
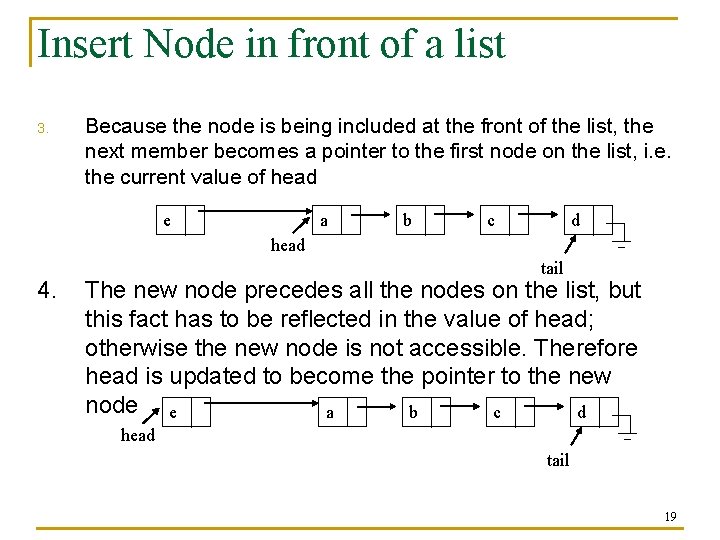
Insert Node in front of a list 3. Because the node is being included at the front of the list, the next member becomes a pointer to the first node on the list, i. e. the current value of head e a b c d head 4. tail The new node precedes all the nodes on the list, but this fact has to be reflected in the value of head; otherwise the new node is not accessible. Therefore head is updated to become the pointer to the new node e b c d a head tail 19
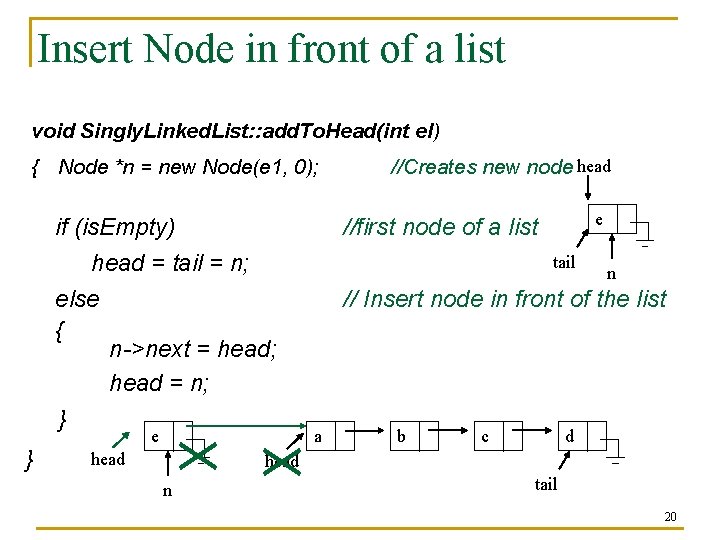
Insert Node in front of a list void Singly. Linked. List: : add. To. Head(int el) { Node *n = new Node(e 1, 0); e if (is. Empty) head = tail = n; //first node of a list else { // Insert node in front of the list tail n n->next = head; head = n; } } //Creates new node head e a head b c d head n tail 20
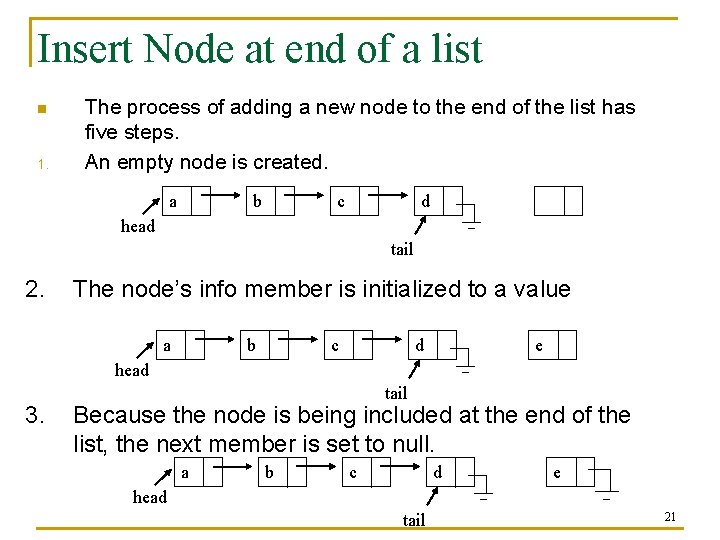
Insert Node at end of a list n 1. The process of adding a new node to the end of the list has five steps. An empty node is created. a b c d head tail 2. The node’s info member is initialized to a value a b c d e head 3. tail Because the node is being included at the end of the list, the next member is set to null. a b c d e head tail 21
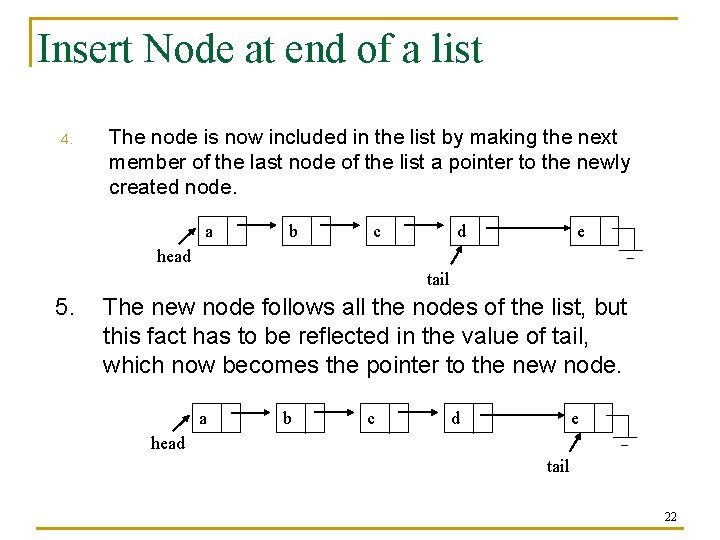
Insert Node at end of a list 4. The node is now included in the list by making the next member of the last node of the list a pointer to the newly created node. a b c e d head tail 5. The new node follows all the nodes of the list, but this fact has to be reflected in the value of tail, which now becomes the pointer to the new node. a b c d e head tail 22
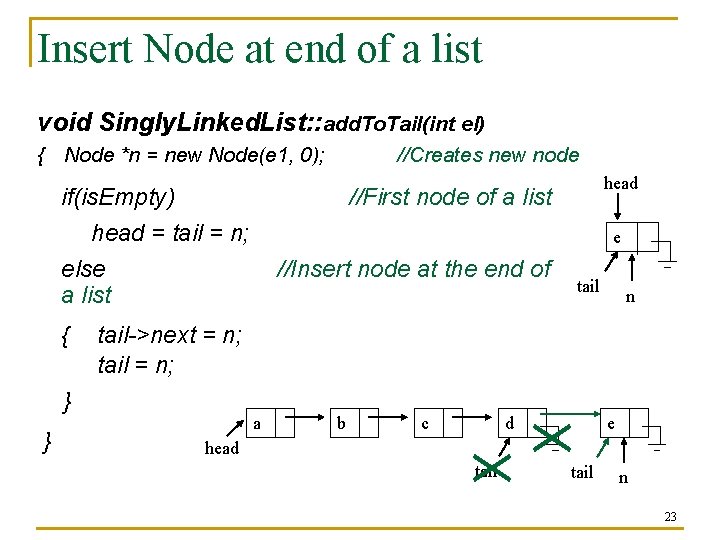
Insert Node at end of a list void Singly. Linked. List: : add. To. Tail(int el) { Node *n = new Node(e 1, 0); if(is. Empty) head = tail = n; else a list { head //First node of a list e //Insert node at the end of tail n tail->next = n; tail = n; } } //Creates new node a b c d e head tail n 23
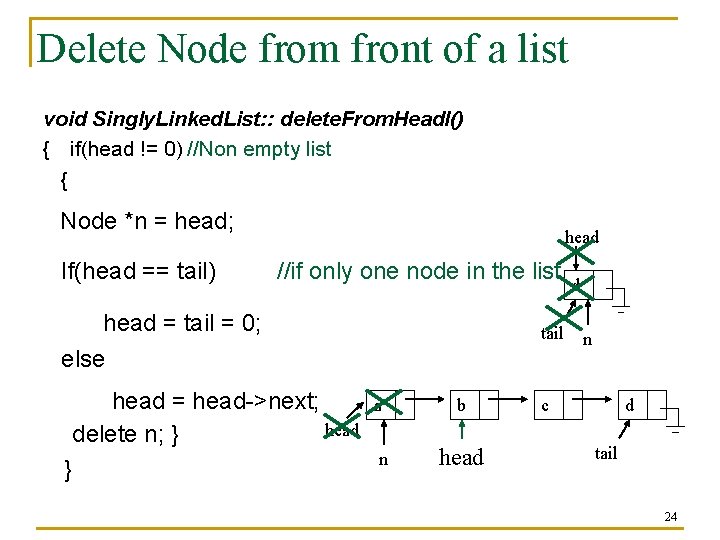
Delete Node from front of a list void Singly. Linked. List: : delete. From. Headl() { if(head != 0) //Non empty list { Node *n = head; If(head == tail) head //if only one node in the list head = tail = 0; else head = head->next; head delete n; } } tail a n b head d n c d tail 24
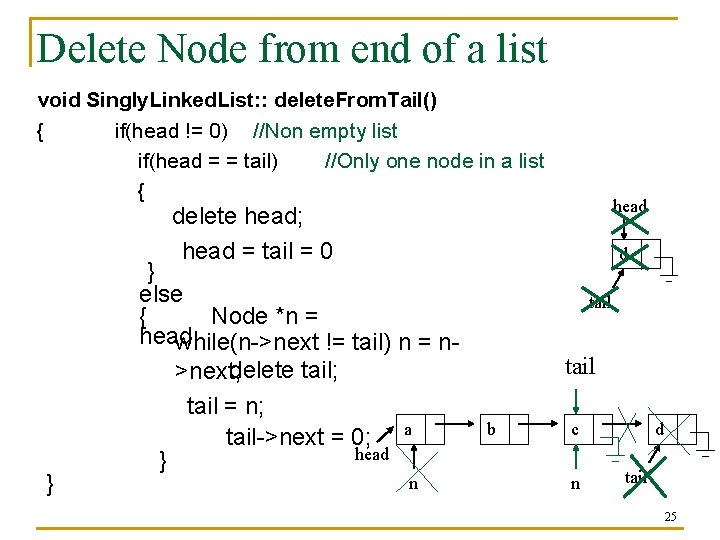
Delete Node from end of a list void Singly. Linked. List: : delete. From. Tail() { if(head != 0) //Non empty list if(head = = tail) //Only one node in a list { head delete head; head = tail = 0 } } else { Node *n = head; while(n->next != tail) n = n>next; delete tail; tail = n; tail->next = 0; a head } n d tail b c n d tail 25
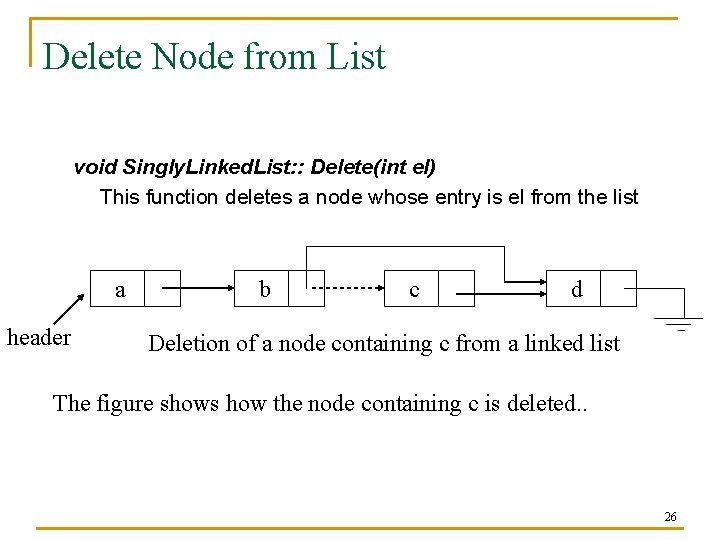
Delete Node from List void Singly. Linked. List: : Delete(int el) This function deletes a node whose entry is el from the list a header b c d Deletion of a node containing c from a linked list The figure shows how the node containing c is deleted. . 26
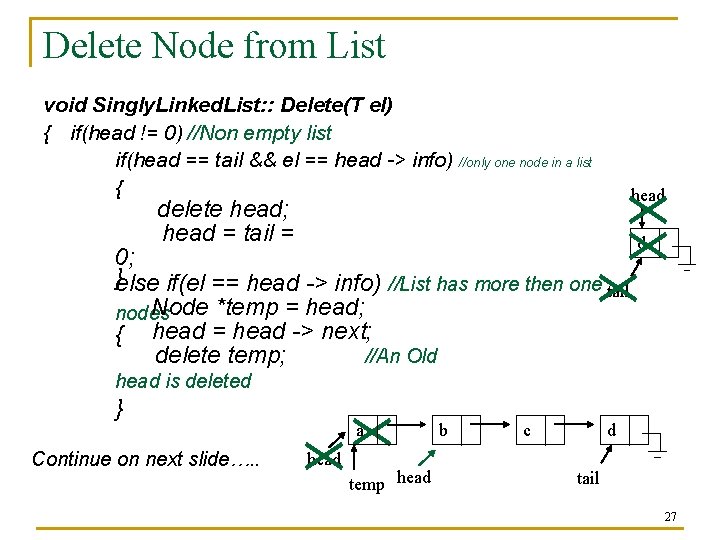
Delete Node from List void Singly. Linked. List: : Delete(T el) { if(head != 0) //Non empty list if(head == tail && el == head -> info) //only one node in a list { head delete head; head = tail = 0; }else if(el == head -> info) //List has more then one tail Node *temp = head; nodes { head = head -> next; delete temp; //An Old d head is deleted } Continue on next slide…. . a head temp head b c d tail 27
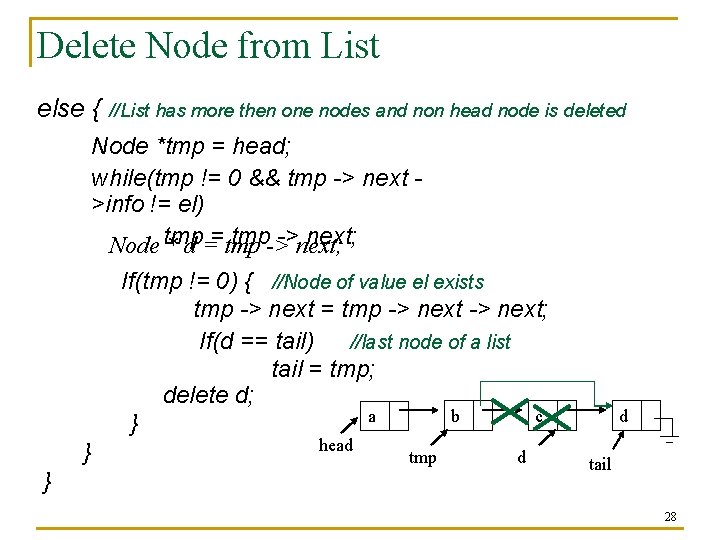
Delete Node from List else { //List has more then one nodes and non head node is deleted Node *tmp = head; while(tmp != 0 && tmp -> next >info != el) tmp-> ->next; Node tmp * d ==tmp If(tmp != 0) { //Node of value el exists tmp -> next = tmp -> next; If(d == tail) //last node of a list tail = tmp; delete d; b c a } } } head tmp d d tail 28
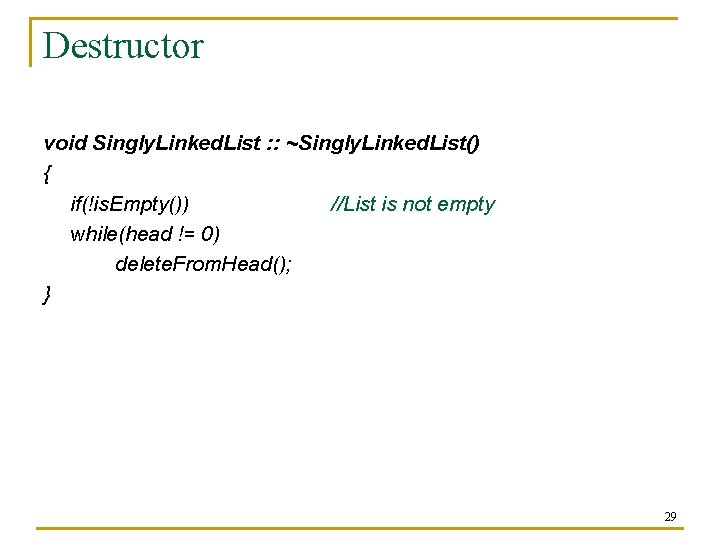
Destructor void Singly. Linked. List : : ~Singly. Linked. List() { if(!is. Empty()) //List is not empty while(head != 0) delete. From. Head(); } 29