EENG 212 Algorithms and Data Structures ARRAYS IN
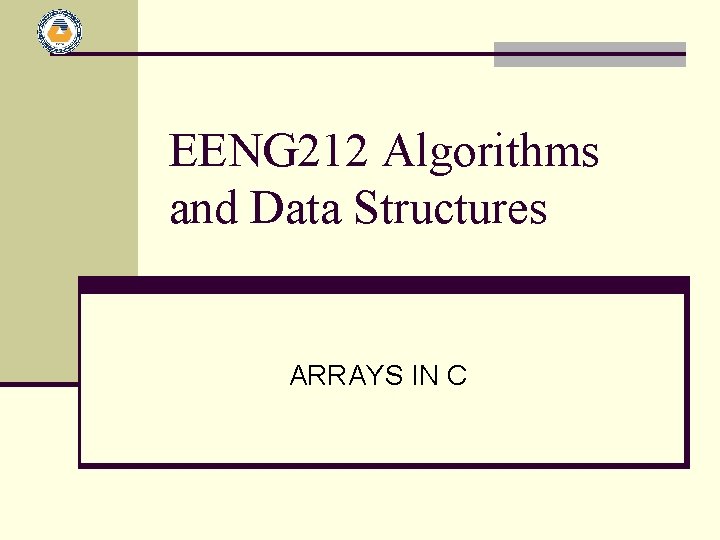
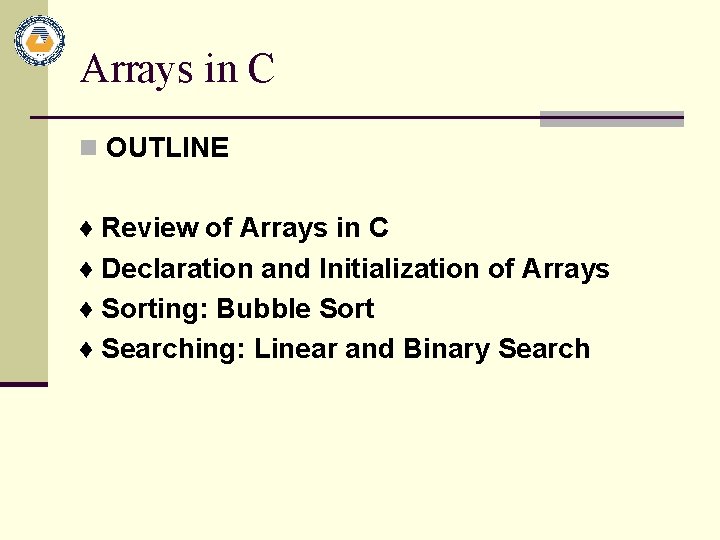
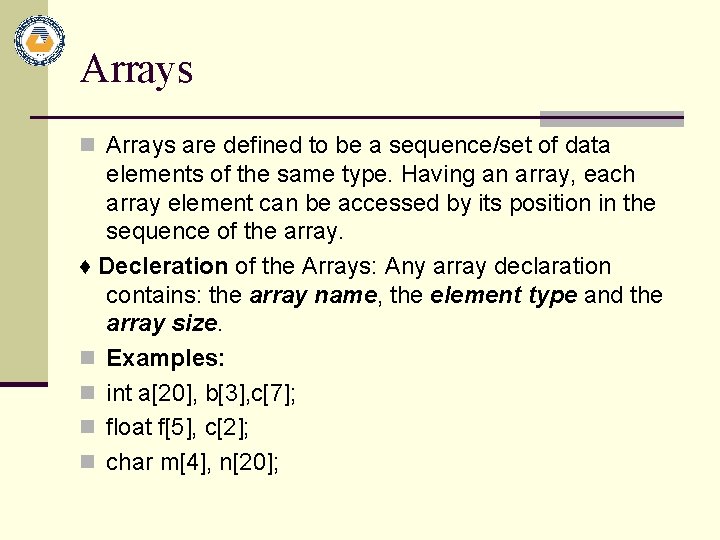
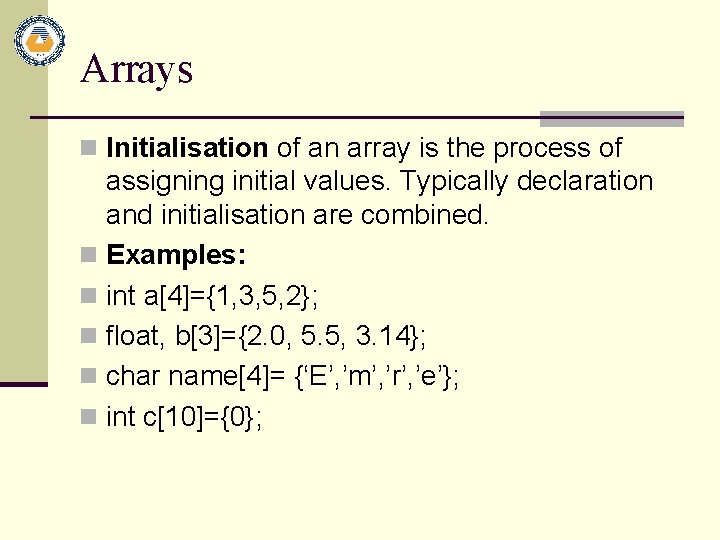
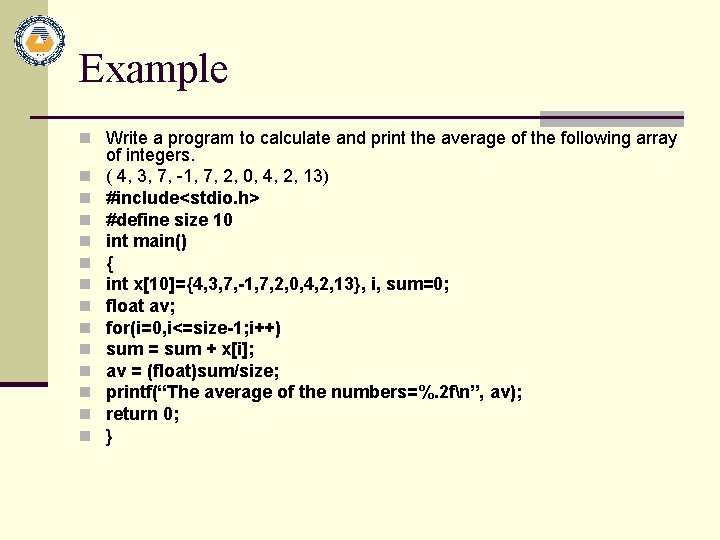
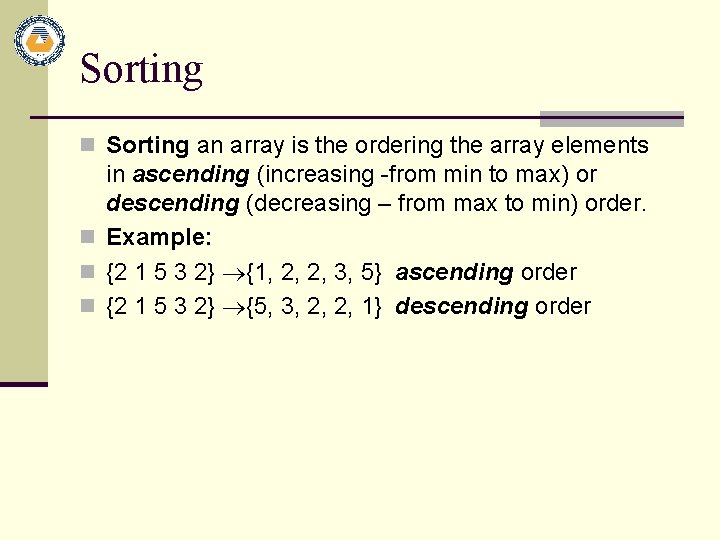
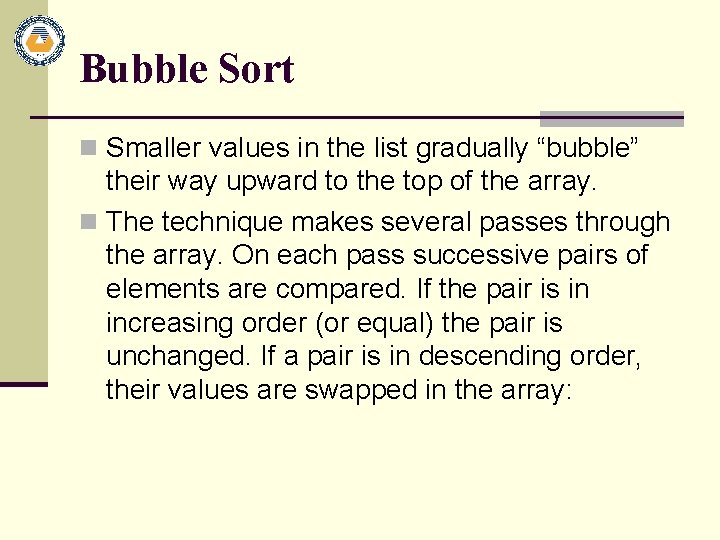
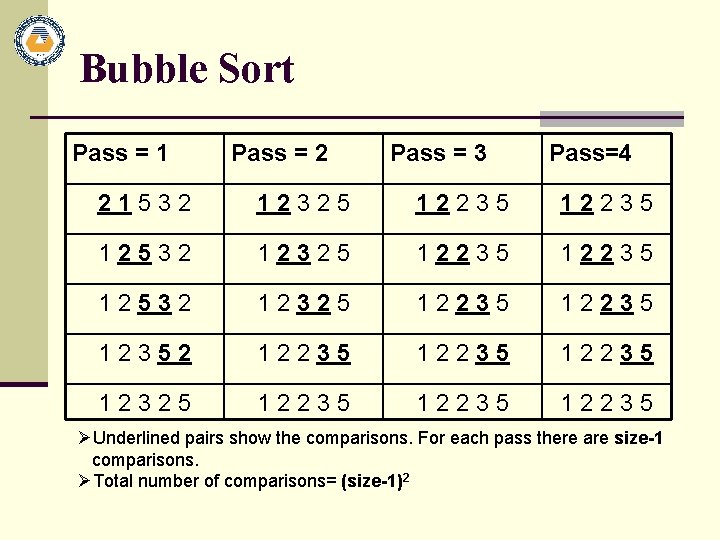
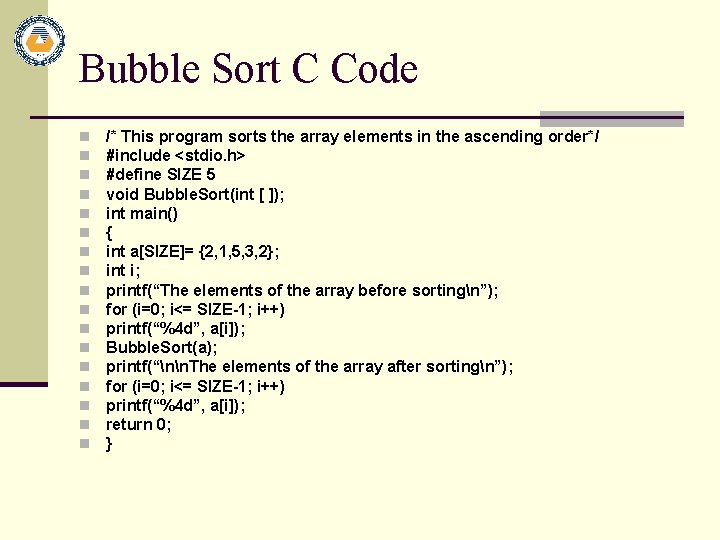
![void Bubble. Sort(int A[ ]) n n void Bubble. Sort(int A[ ]) { int void Bubble. Sort(int A[ ]) n n void Bubble. Sort(int A[ ]) { int](https://slidetodoc.com/presentation_image_h2/973928256c9983a775d5f5e8dc4b6be1/image-10.jpg)
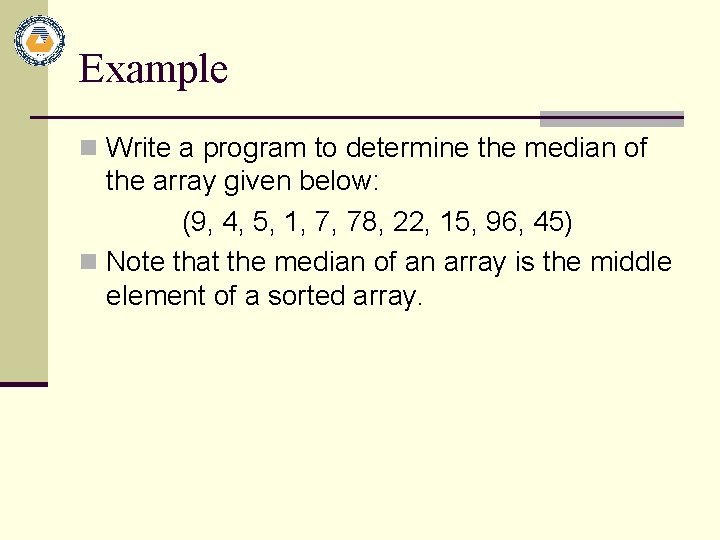
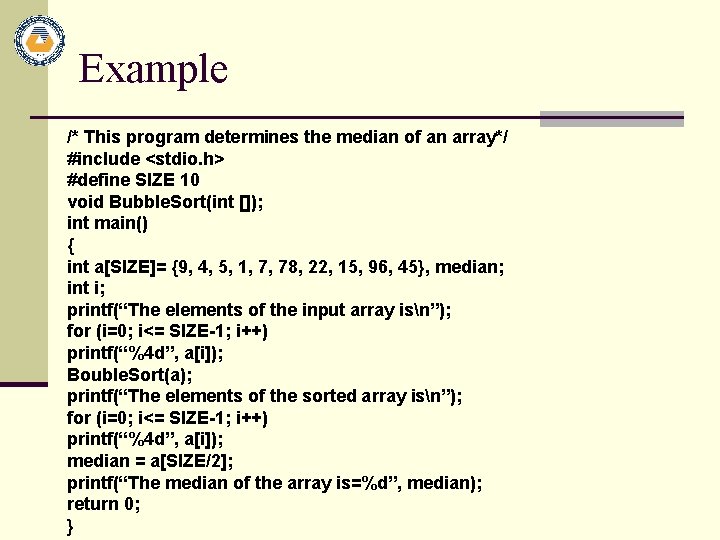
![Example void Bubble. Sort(int A[ ]) { int i, pass, hold; for (pass=1; pass<= Example void Bubble. Sort(int A[ ]) { int i, pass, hold; for (pass=1; pass<=](https://slidetodoc.com/presentation_image_h2/973928256c9983a775d5f5e8dc4b6be1/image-13.jpg)
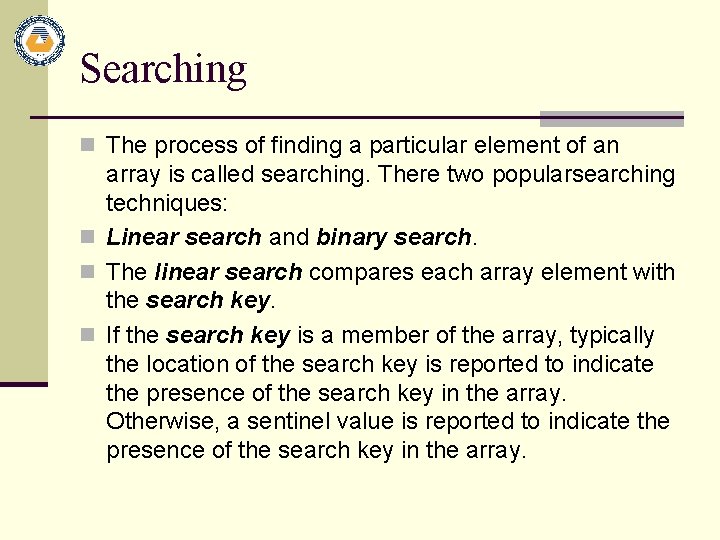
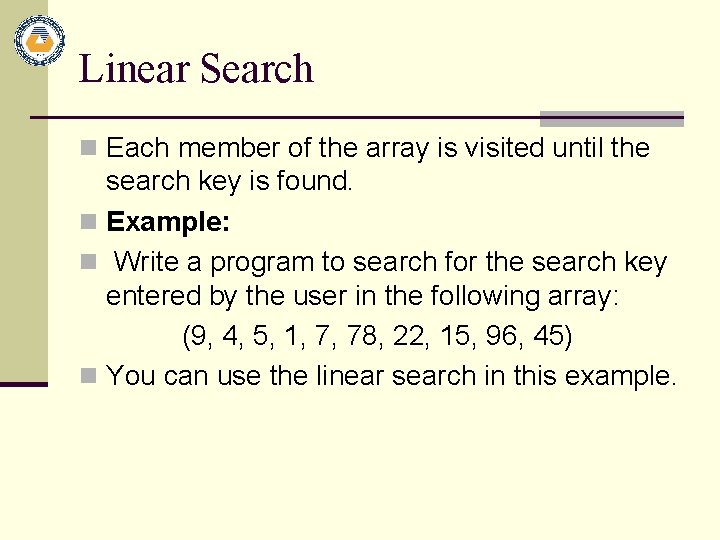
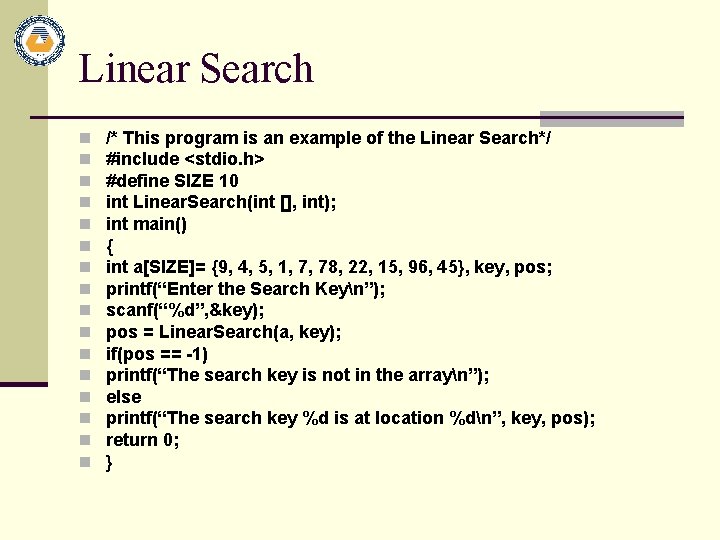
![int Linear. Search (int b[ ], int skey) { int i; for (i=0; i<= int Linear. Search (int b[ ], int skey) { int i; for (i=0; i<=](https://slidetodoc.com/presentation_image_h2/973928256c9983a775d5f5e8dc4b6be1/image-17.jpg)
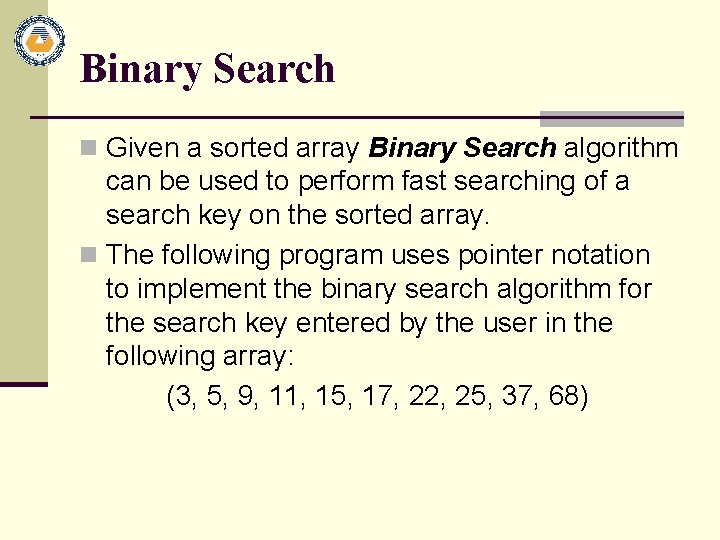
![Binary Search #include <stdio. h> #define SIZE 10 int Binary. Search(int [ ], int); Binary Search #include <stdio. h> #define SIZE 10 int Binary. Search(int [ ], int);](https://slidetodoc.com/presentation_image_h2/973928256c9983a775d5f5e8dc4b6be1/image-19.jpg)
![int Binary. Search (int A[], int skey) { int low=0, high=SIZE-1, middle; while(low <= int Binary. Search (int A[], int skey) { int low=0, high=SIZE-1, middle; while(low <=](https://slidetodoc.com/presentation_image_h2/973928256c9983a775d5f5e8dc4b6be1/image-20.jpg)
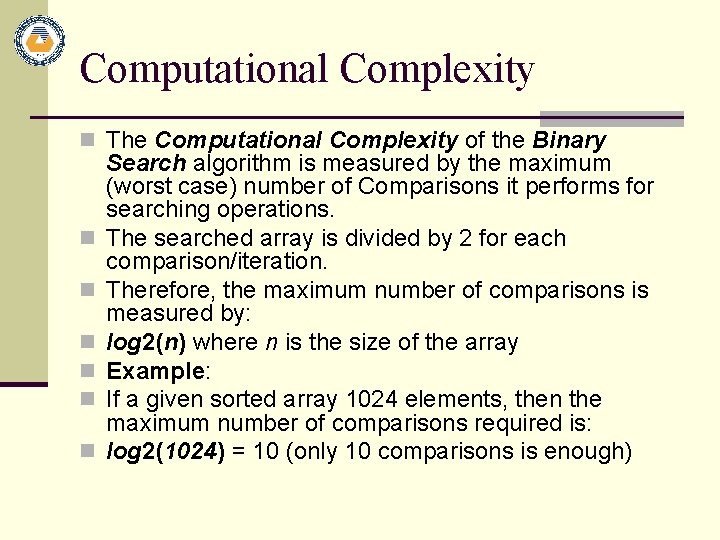
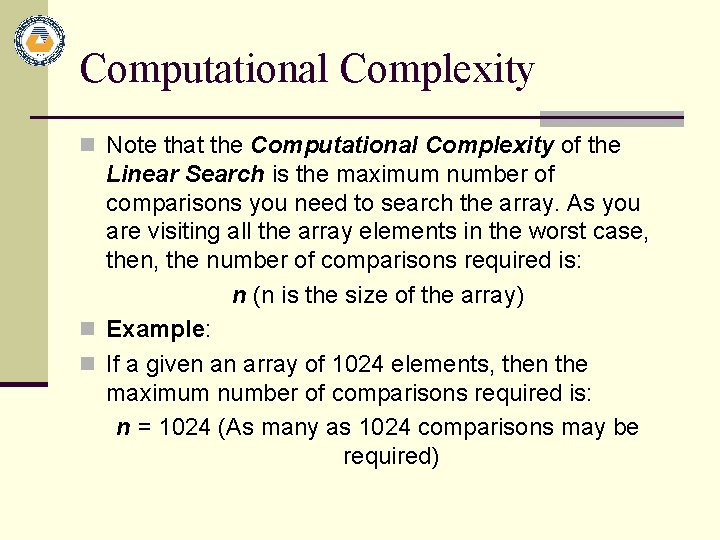
- Slides: 22
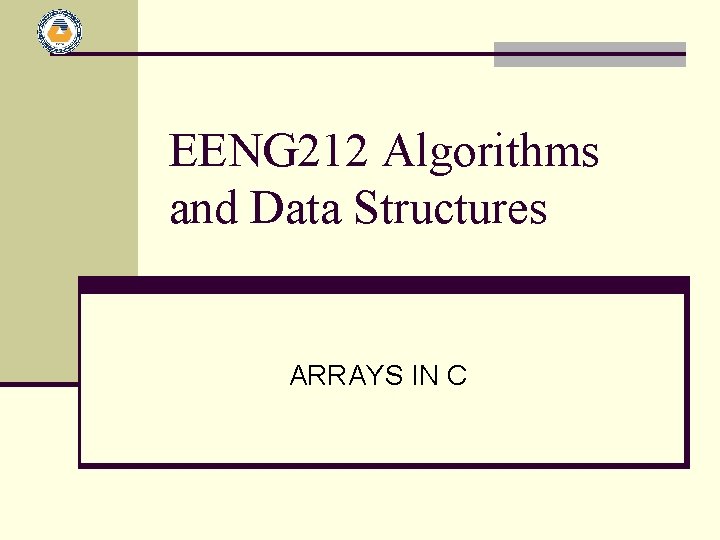
EENG 212 Algorithms and Data Structures ARRAYS IN C
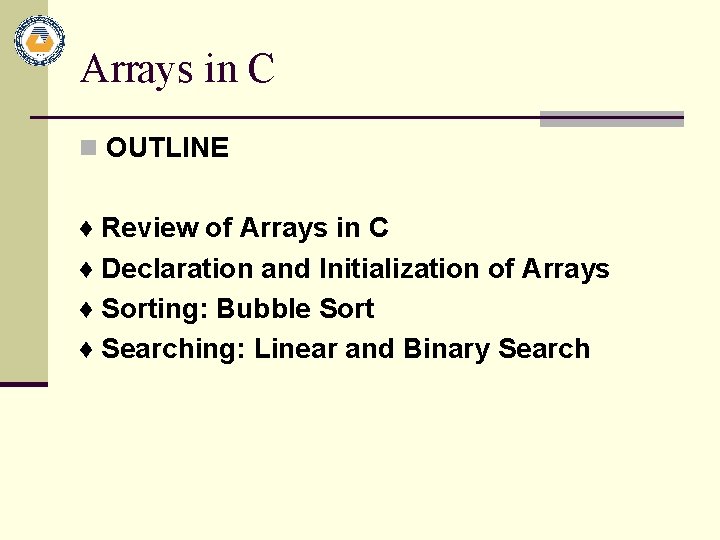
Arrays in C n OUTLINE ♦ Review of Arrays in C ♦ Declaration and Initialization of Arrays ♦ Sorting: Bubble Sort ♦ Searching: Linear and Binary Search
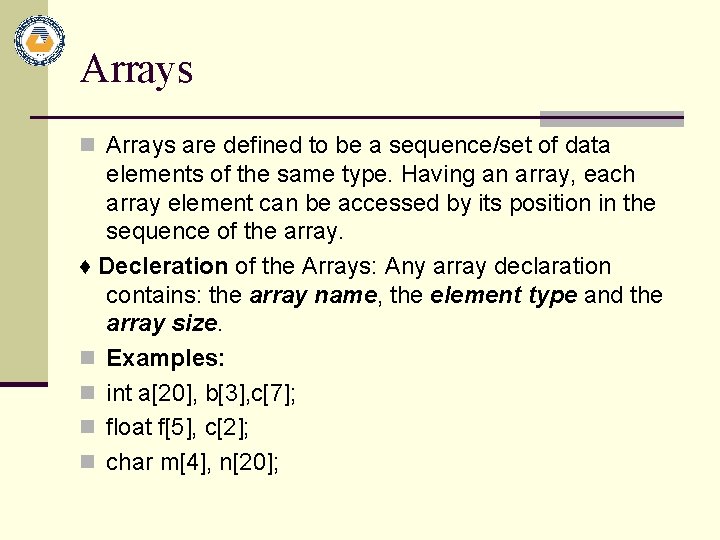
Arrays n Arrays are defined to be a sequence/set of data elements of the same type. Having an array, each array element can be accessed by its position in the sequence of the array. ♦ Decleration of the Arrays: Any array declaration contains: the array name, the element type and the array size. n Examples: n int a[20], b[3], c[7]; n float f[5], c[2]; n char m[4], n[20];
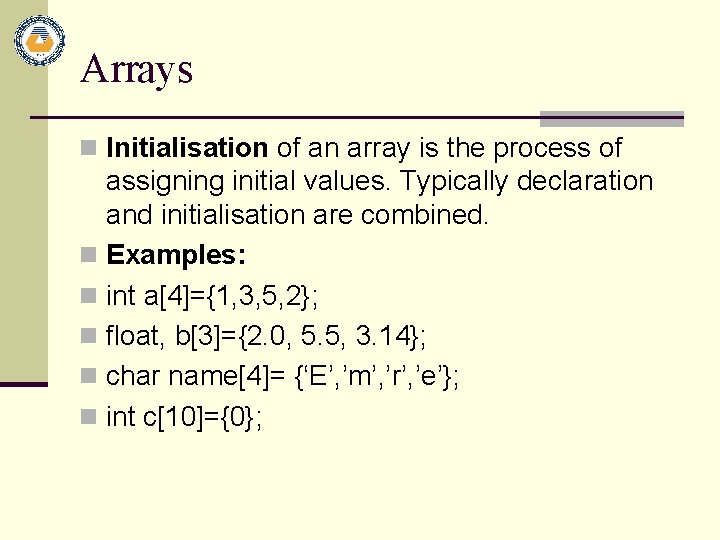
Arrays n Initialisation of an array is the process of assigning initial values. Typically declaration and initialisation are combined. n Examples: n int a[4]={1, 3, 5, 2}; n float, b[3]={2. 0, 5. 5, 3. 14}; n char name[4]= {‘E’, ’m’, ’r’, ’e’}; n int c[10]={0};
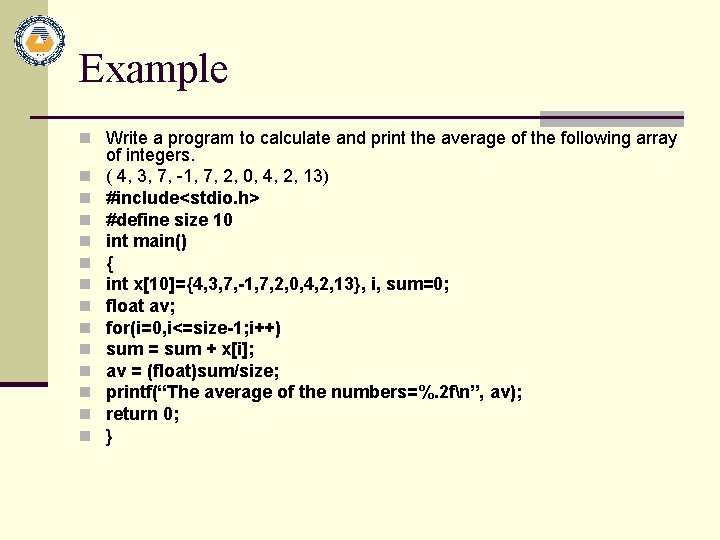
Example n Write a program to calculate and print the average of the following array n n n n of integers. ( 4, 3, 7, -1, 7, 2, 0, 4, 2, 13) #include<stdio. h> #define size 10 int main() { int x[10]={4, 3, 7, -1, 7, 2, 0, 4, 2, 13}, i, sum=0; float av; for(i=0, i<=size-1; i++) sum = sum + x[i]; av = (float)sum/size; printf(“The average of the numbers=%. 2 fn”, av); return 0; }
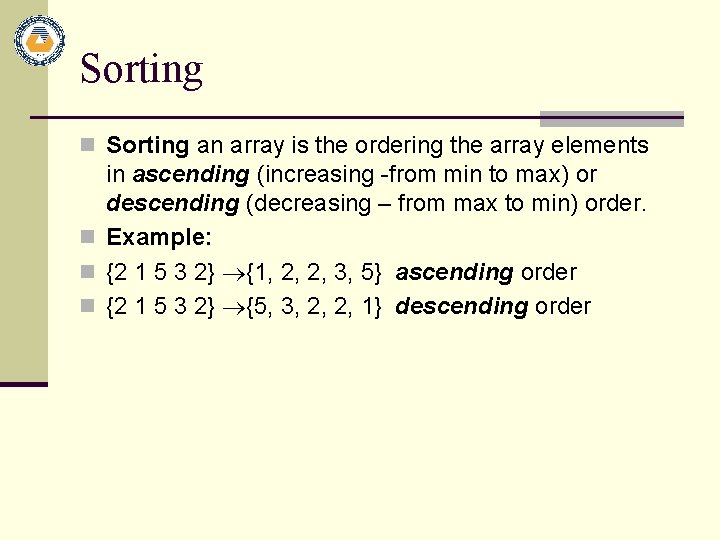
Sorting n Sorting an array is the ordering the array elements in ascending (increasing -from min to max) or descending (decreasing – from max to min) order. n Example: n {2 1 5 3 2} {1, 2, 2, 3, 5} ascending order n {2 1 5 3 2} {5, 3, 2, 2, 1} descending order
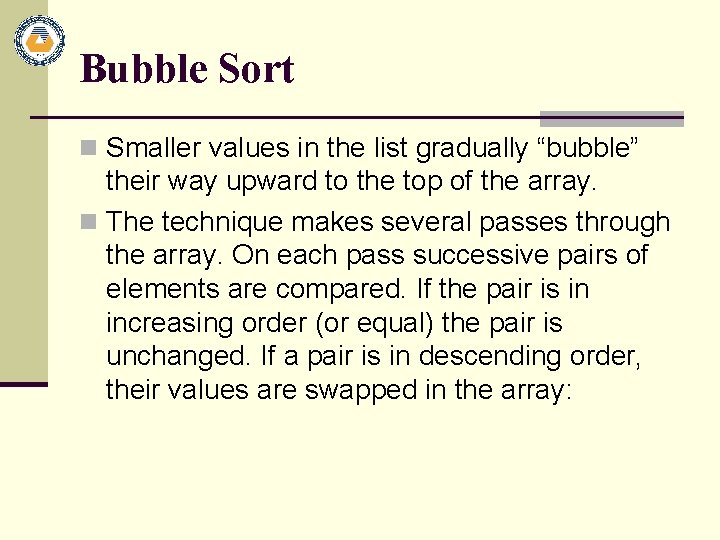
Bubble Sort n Smaller values in the list gradually “bubble” their way upward to the top of the array. n The technique makes several passes through the array. On each pass successive pairs of elements are compared. If the pair is in increasing order (or equal) the pair is unchanged. If a pair is in descending order, their values are swapped in the array:
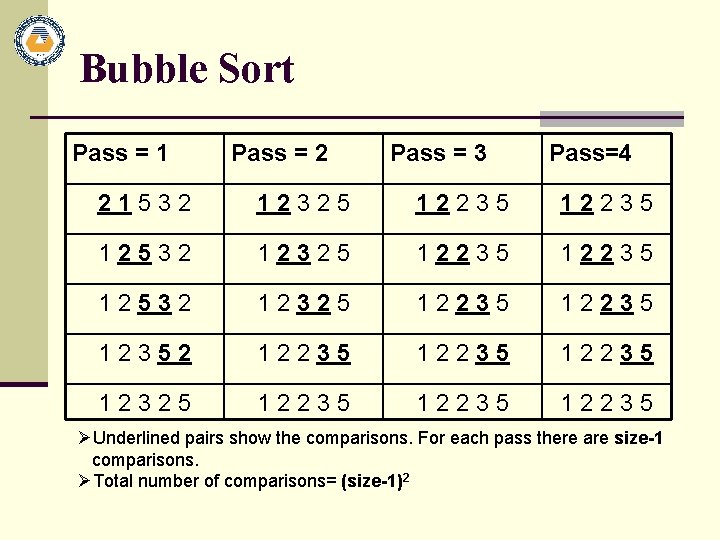
Bubble Sort Pass = 1 Pass = 2 Pass = 3 Pass=4 21532 12325 12235 12532 12325 12235 12352 12235 12325 12235 ØUnderlined pairs show the comparisons. For each pass there are size-1 comparisons. ØTotal number of comparisons= (size-1)2
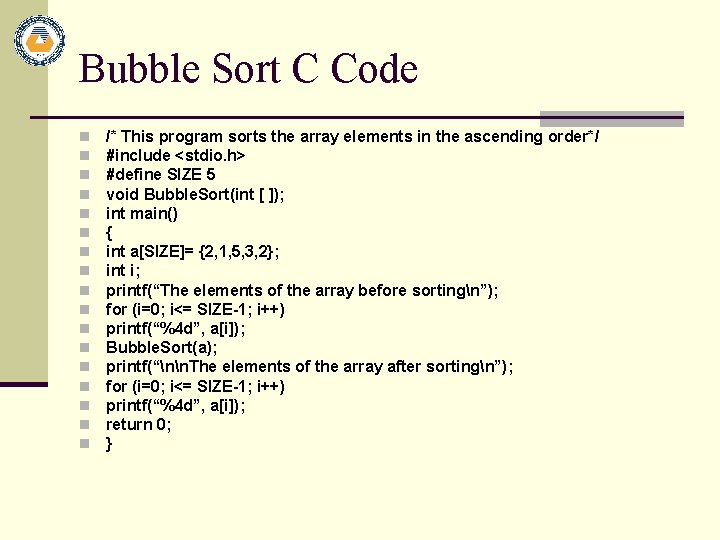
Bubble Sort C Code n n n n n /* This program sorts the array elements in the ascending order*/ #include <stdio. h> #define SIZE 5 void Bubble. Sort(int [ ]); int main() { int a[SIZE]= {2, 1, 5, 3, 2}; int i; printf(“The elements of the array before sortingn”); for (i=0; i<= SIZE-1; i++) printf(“%4 d”, a[i]); Bubble. Sort(a); printf(“nn. The elements of the array after sortingn”); for (i=0; i<= SIZE-1; i++) printf(“%4 d”, a[i]); return 0; }
![void Bubble Sortint A n n void Bubble Sortint A int void Bubble. Sort(int A[ ]) n n void Bubble. Sort(int A[ ]) { int](https://slidetodoc.com/presentation_image_h2/973928256c9983a775d5f5e8dc4b6be1/image-10.jpg)
void Bubble. Sort(int A[ ]) n n void Bubble. Sort(int A[ ]) { int i, pass, hold; for (pass=1; pass<= SIZE-1; pass++){ n n } n } for (i=0; i<= SIZE-2; i++) { if(A[i] >A[i+1]){ n hold =A[i]; n A[i]=A[i+1]; n A[i+1]=hold; n } }
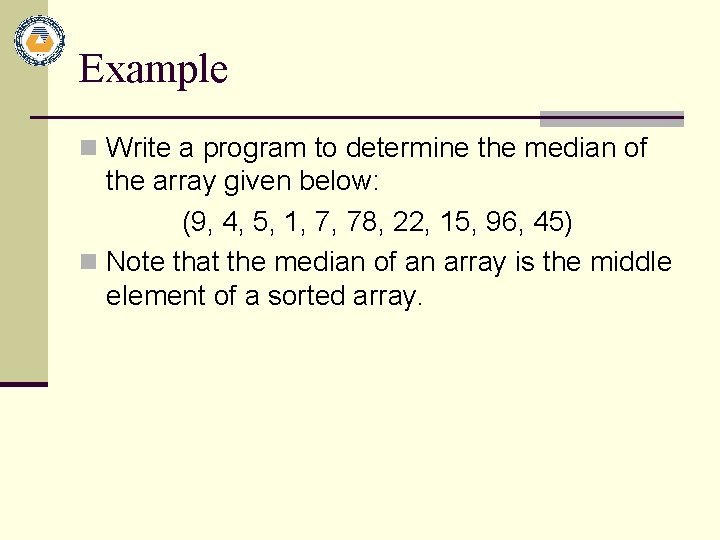
Example n Write a program to determine the median of the array given below: (9, 4, 5, 1, 7, 78, 22, 15, 96, 45) n Note that the median of an array is the middle element of a sorted array.
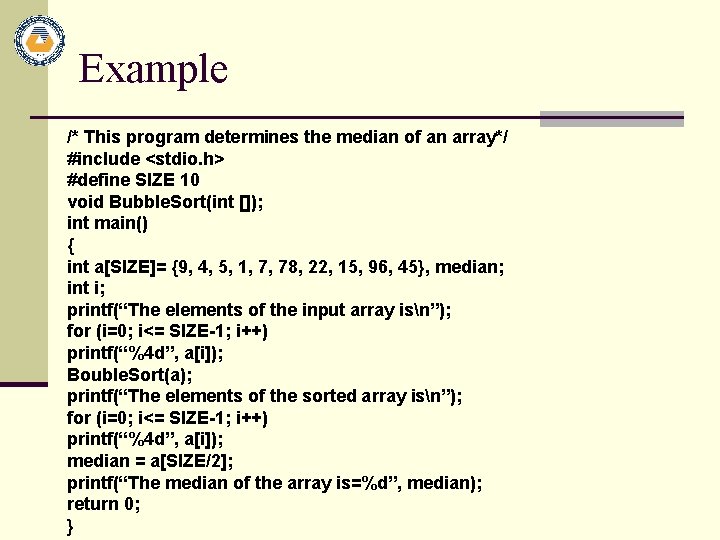
Example /* This program determines the median of an array*/ #include <stdio. h> #define SIZE 10 void Bubble. Sort(int []); int main() { int a[SIZE]= {9, 4, 5, 1, 7, 78, 22, 15, 96, 45}, median; int i; printf(“The elements of the input array isn”); for (i=0; i<= SIZE-1; i++) printf(“%4 d”, a[i]); Bouble. Sort(a); printf(“The elements of the sorted array isn”); for (i=0; i<= SIZE-1; i++) printf(“%4 d”, a[i]); median = a[SIZE/2]; printf(“The median of the array is=%d”, median); return 0; }
![Example void Bubble Sortint A int i pass hold for pass1 pass Example void Bubble. Sort(int A[ ]) { int i, pass, hold; for (pass=1; pass<=](https://slidetodoc.com/presentation_image_h2/973928256c9983a775d5f5e8dc4b6be1/image-13.jpg)
Example void Bubble. Sort(int A[ ]) { int i, pass, hold; for (pass=1; pass<= SIZE-1; pass++){ for (i=0; i<= SIZE-2; i++) { if(A[i] >A[i+1]){ hold =A[i]; A[i]=A[i+1]; A[i+1]=hold; } }
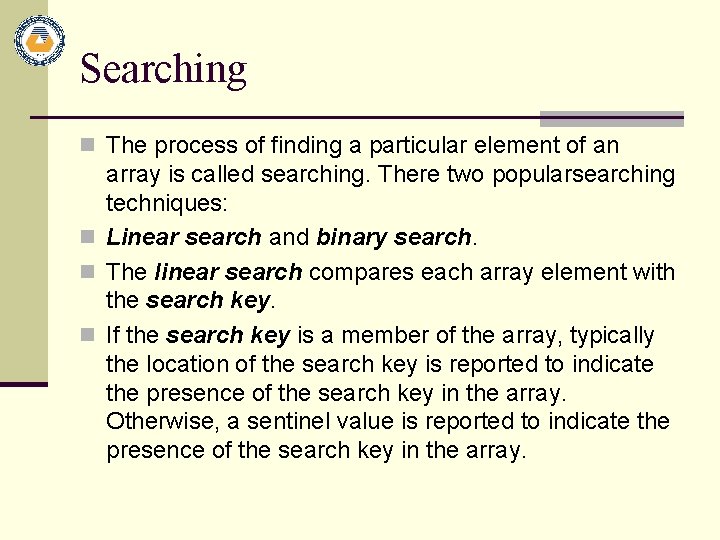
Searching n The process of finding a particular element of an array is called searching. There two popularsearching techniques: n Linear search and binary search. n The linear search compares each array element with the search key. n If the search key is a member of the array, typically the location of the search key is reported to indicate the presence of the search key in the array. Otherwise, a sentinel value is reported to indicate the presence of the search key in the array.
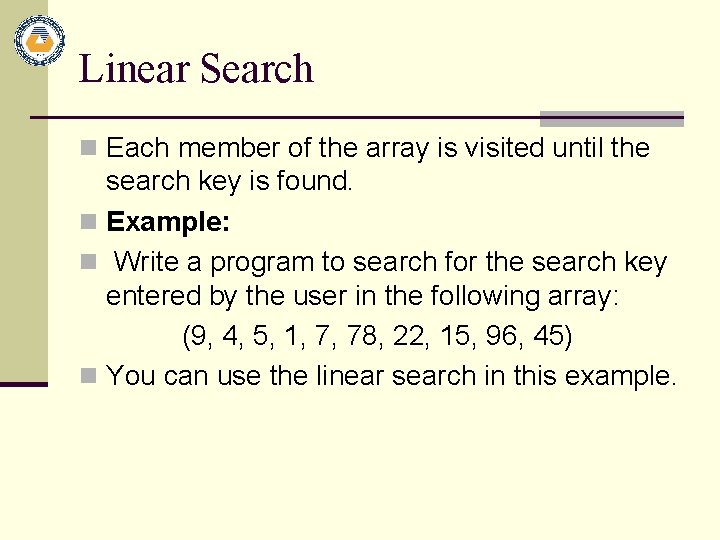
Linear Search n Each member of the array is visited until the search key is found. n Example: n Write a program to search for the search key entered by the user in the following array: (9, 4, 5, 1, 7, 78, 22, 15, 96, 45) n You can use the linear search in this example.
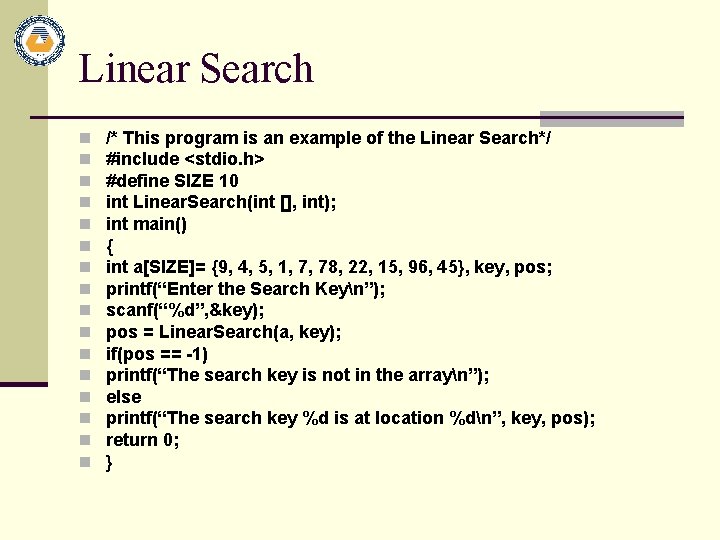
Linear Search n n n n /* This program is an example of the Linear Search*/ #include <stdio. h> #define SIZE 10 int Linear. Search(int [], int); int main() { int a[SIZE]= {9, 4, 5, 1, 7, 78, 22, 15, 96, 45}, key, pos; printf(“Enter the Search Keyn”); scanf(“%d”, &key); pos = Linear. Search(a, key); if(pos == -1) printf(“The search key is not in the arrayn”); else printf(“The search key %d is at location %dn”, key, pos); return 0; }
![int Linear Search int b int skey int i for i0 i int Linear. Search (int b[ ], int skey) { int i; for (i=0; i<=](https://slidetodoc.com/presentation_image_h2/973928256c9983a775d5f5e8dc4b6be1/image-17.jpg)
int Linear. Search (int b[ ], int skey) { int i; for (i=0; i<= SIZE-1; i++) if(b[i] == skey) return i; return -1; }
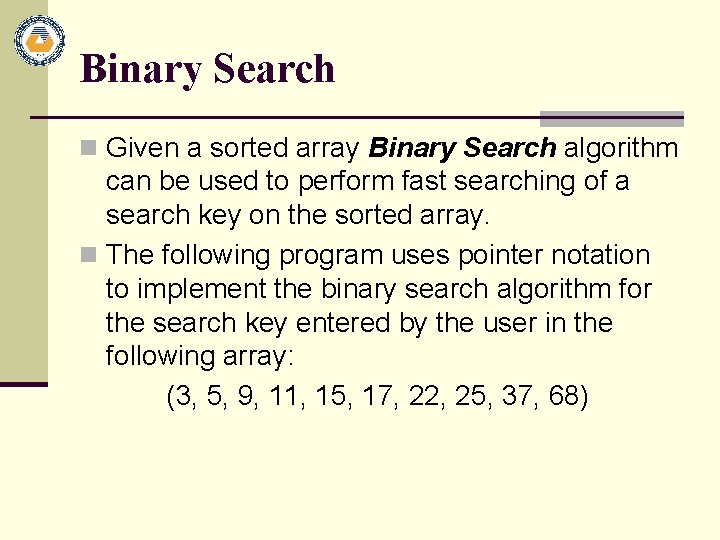
Binary Search n Given a sorted array Binary Search algorithm can be used to perform fast searching of a search key on the sorted array. n The following program uses pointer notation to implement the binary search algorithm for the search key entered by the user in the following array: (3, 5, 9, 11, 15, 17, 22, 25, 37, 68)
![Binary Search include stdio h define SIZE 10 int Binary Searchint int Binary Search #include <stdio. h> #define SIZE 10 int Binary. Search(int [ ], int);](https://slidetodoc.com/presentation_image_h2/973928256c9983a775d5f5e8dc4b6be1/image-19.jpg)
Binary Search #include <stdio. h> #define SIZE 10 int Binary. Search(int [ ], int); int main() { int a[SIZE]= {3, 5, 9, 11, 15, 17, 22, 25, 37, 68}, key, pos; printf(“Enter the Search Keyn”); scanf(“%d”, &key); pos = Binary. Search(a, key); if(pos == -1) printf(“The search key is not in the arrayn”); else printf(“The search key %d is at location %dn”, key, pos); return 0; }
![int Binary Search int A int skey int low0 highSIZE1 middle whilelow int Binary. Search (int A[], int skey) { int low=0, high=SIZE-1, middle; while(low <=](https://slidetodoc.com/presentation_image_h2/973928256c9983a775d5f5e8dc4b6be1/image-20.jpg)
int Binary. Search (int A[], int skey) { int low=0, high=SIZE-1, middle; while(low <= high){ middle= (low+high)/2; if(skey == A[middle]) return middle; else if(skey <A[middle]) high = middle-1; else low = middle+1; } return -1; }
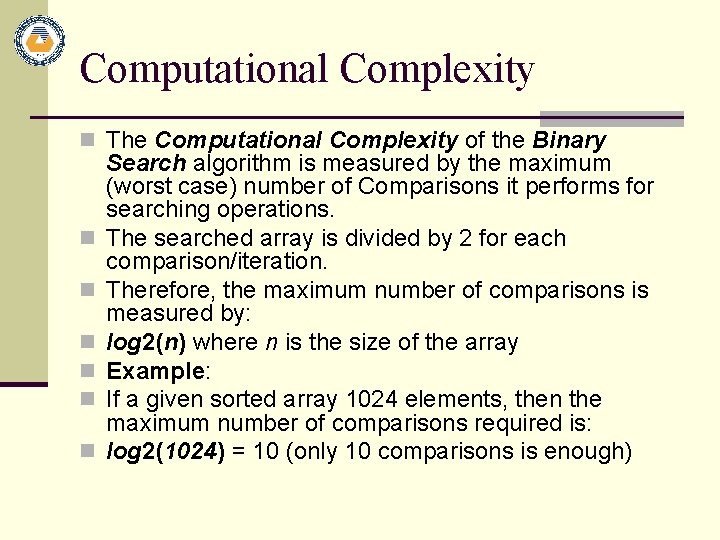
Computational Complexity n The Computational Complexity of the Binary n n n Search algorithm is measured by the maximum (worst case) number of Comparisons it performs for searching operations. The searched array is divided by 2 for each comparison/iteration. Therefore, the maximum number of comparisons is measured by: log 2(n) where n is the size of the array Example: If a given sorted array 1024 elements, then the maximum number of comparisons required is: log 2(1024) = 10 (only 10 comparisons is enough)
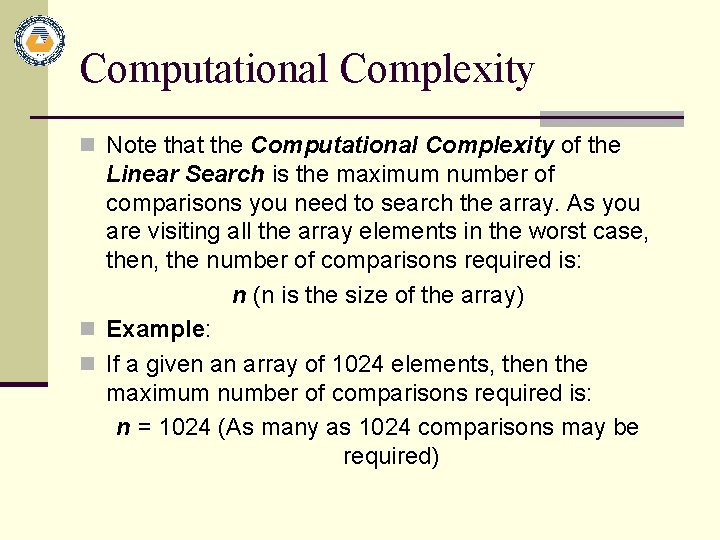
Computational Complexity n Note that the Computational Complexity of the Linear Search is the maximum number of comparisons you need to search the array. As you are visiting all the array elements in the worst case, then, the number of comparisons required is: n (n is the size of the array) n Example: n If a given an array of 1024 elements, then the maximum number of comparisons required is: n = 1024 (As many as 1024 comparisons may be required)
Data structures and algorithms iit bombay
Cos 423 princeton
Data structures and algorithms tutorial
Information retrieval data structures and algorithms
Data structures and algorithms bits pilani
Ajit diwan iit bombay
Data structures and algorithms
Data structures and algorithms
Ian munro waterloo
Information retrieval data structures and algorithms
Data structures and algorithms
Algorithms + data structures = programs
Advantages and disadvantages of array over linked list
Parallel arrays in c
Analogous structures
Dynamic arrays and amortized analysis
Searching and sorting arrays in c++
Array of arrays c++
Parallel arrays
Partially filled arrays
Parallel arrays
Why do we need arrays?
Variables unidimensionales ejemplos