EENG 212 ALGORITHMS DATA STRUCTURES POINTERS EASTERN MEDITERRANEAN
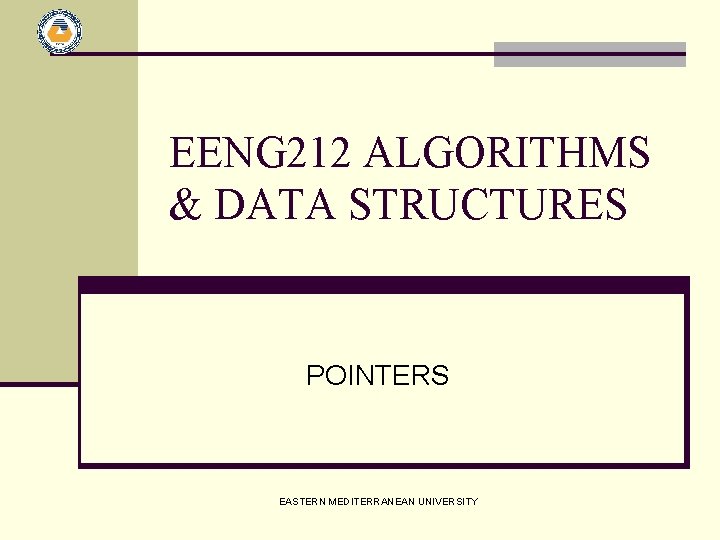
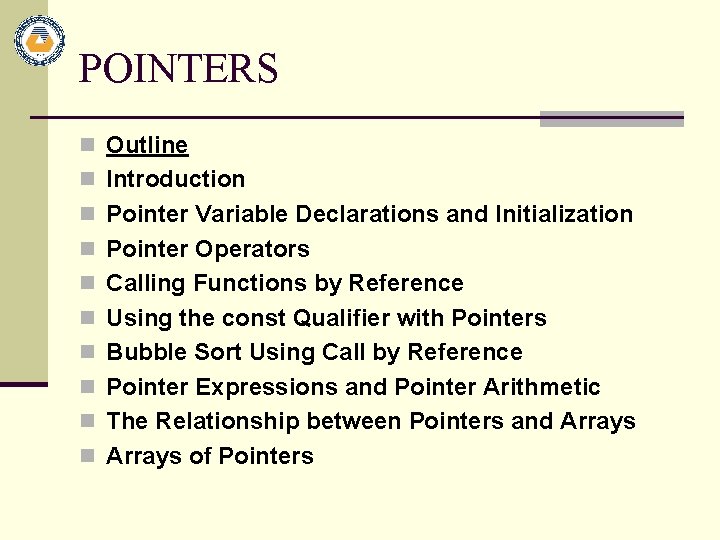
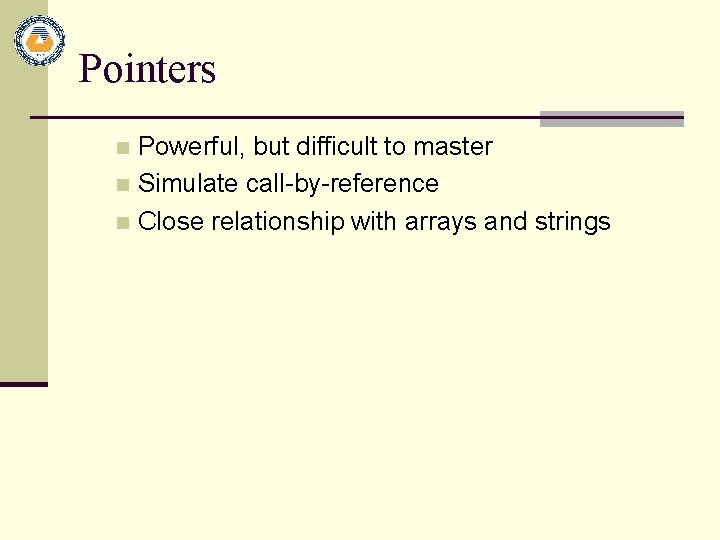
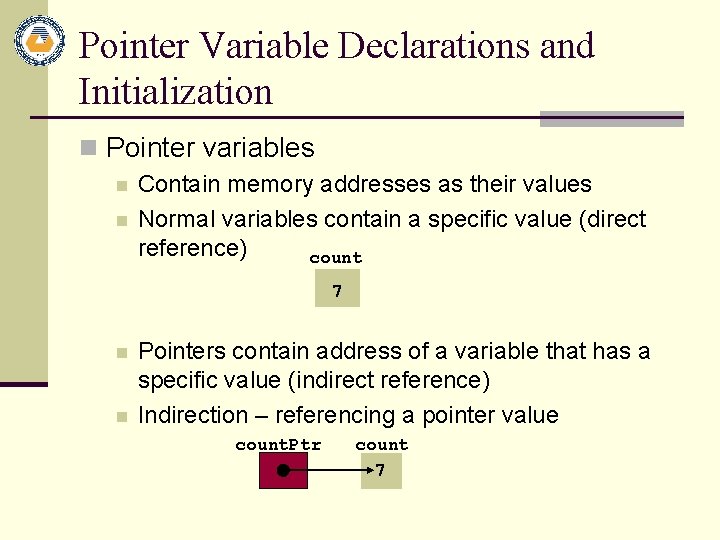
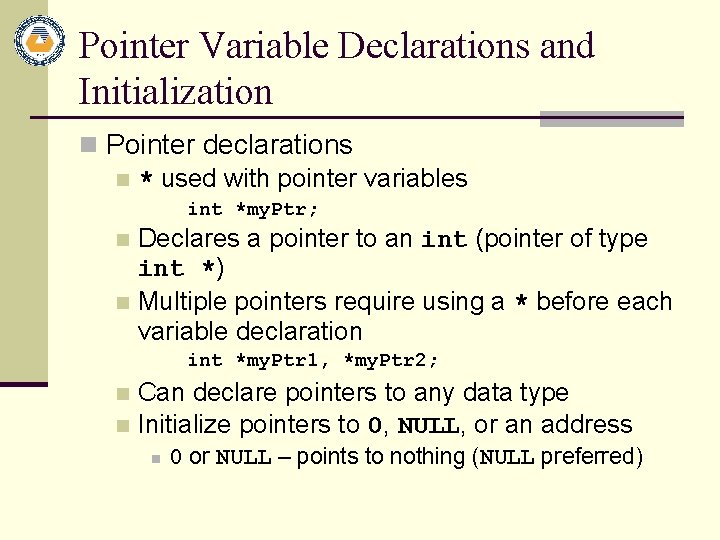
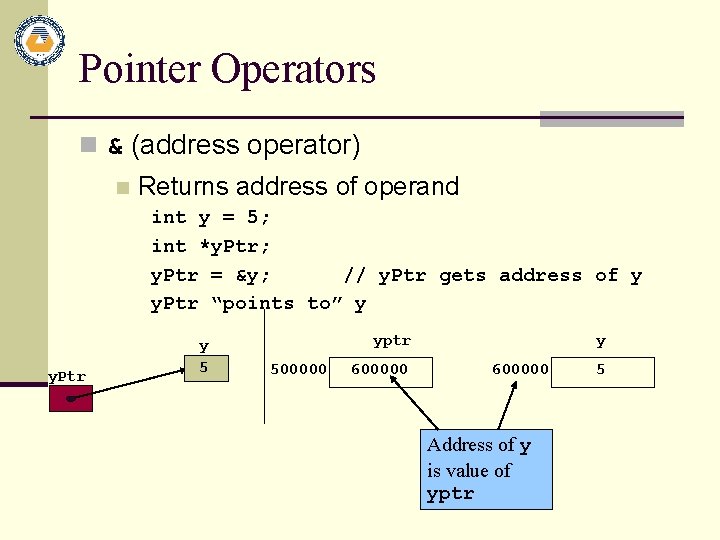
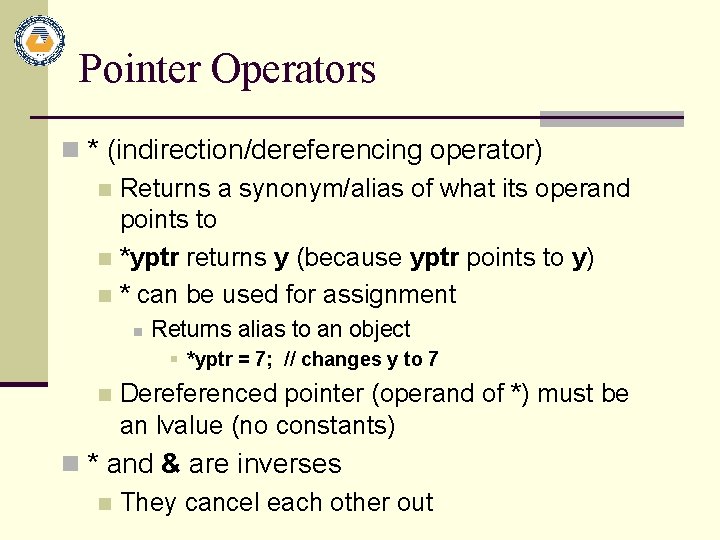
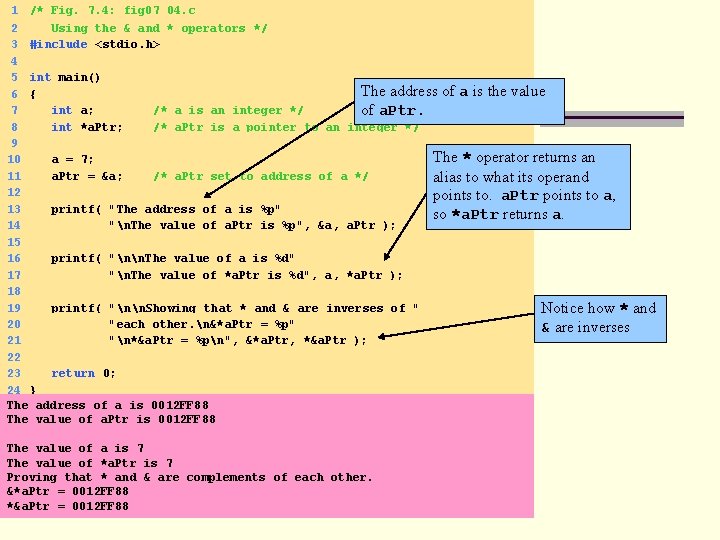
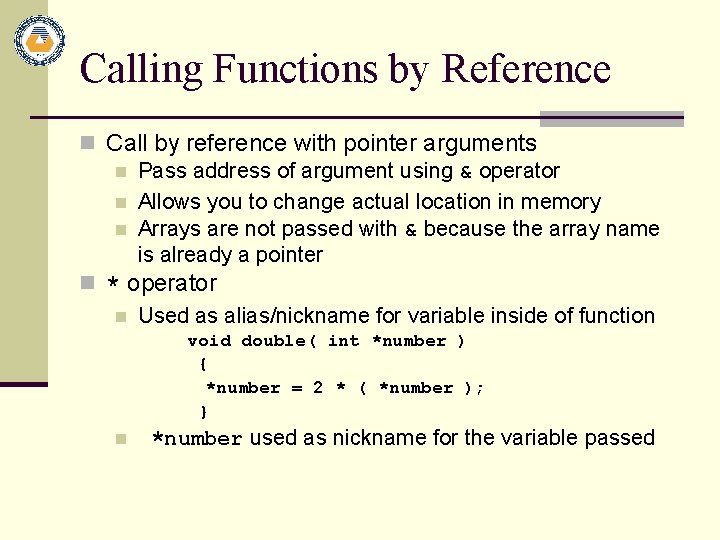
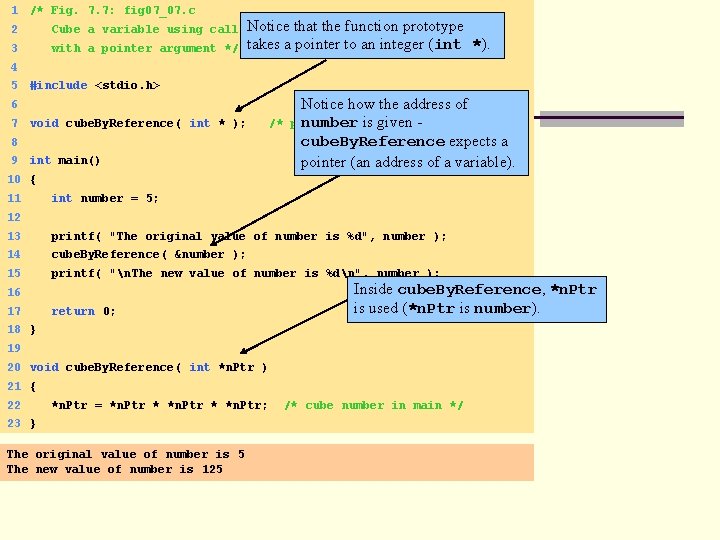
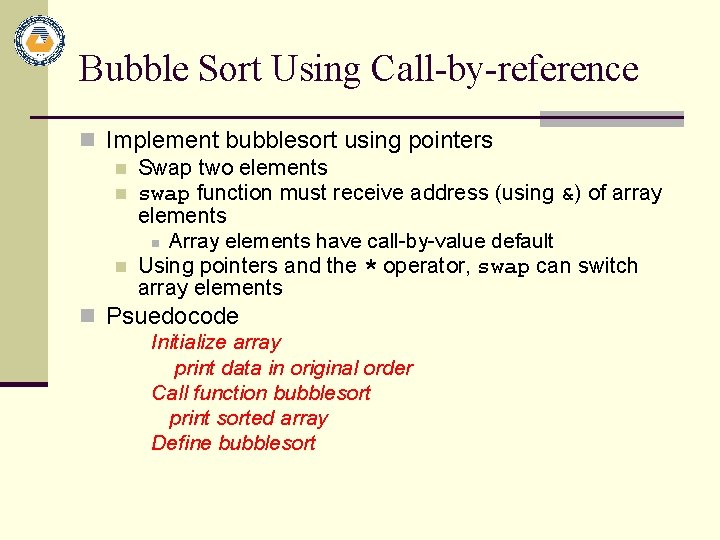
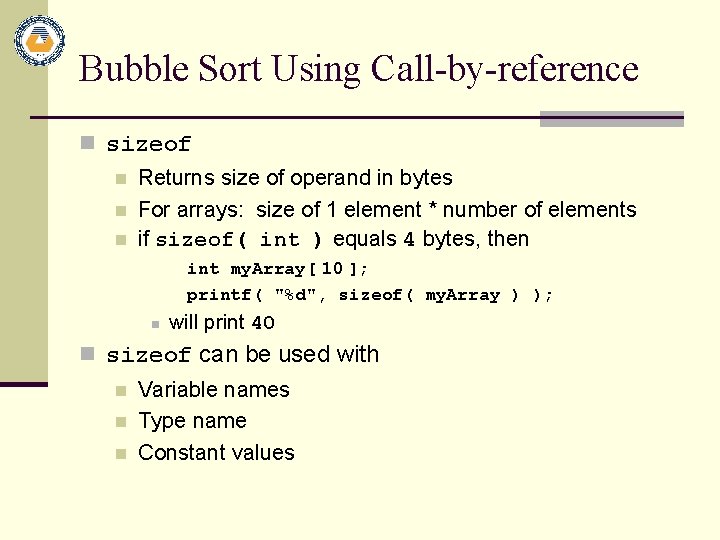
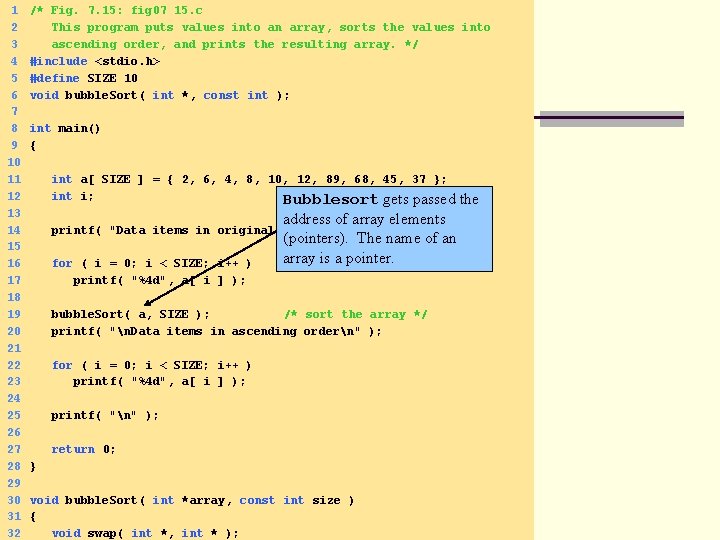
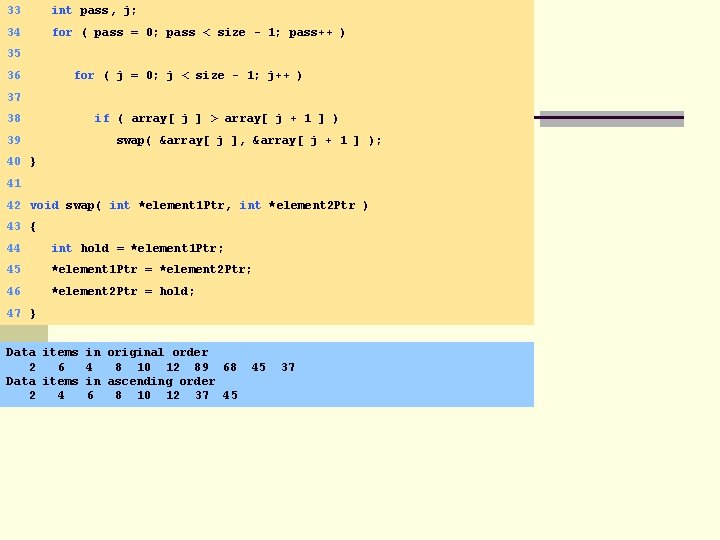
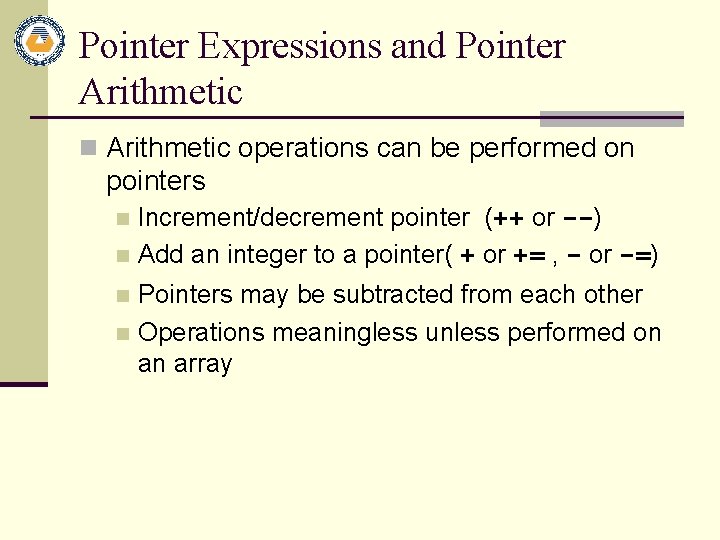
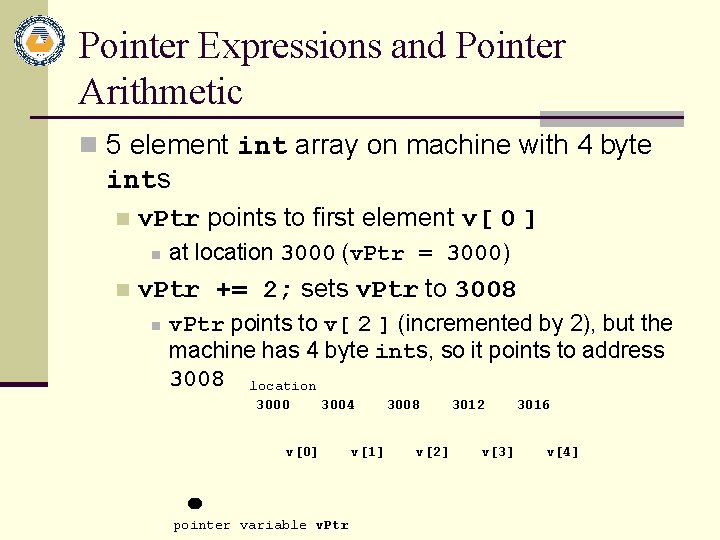
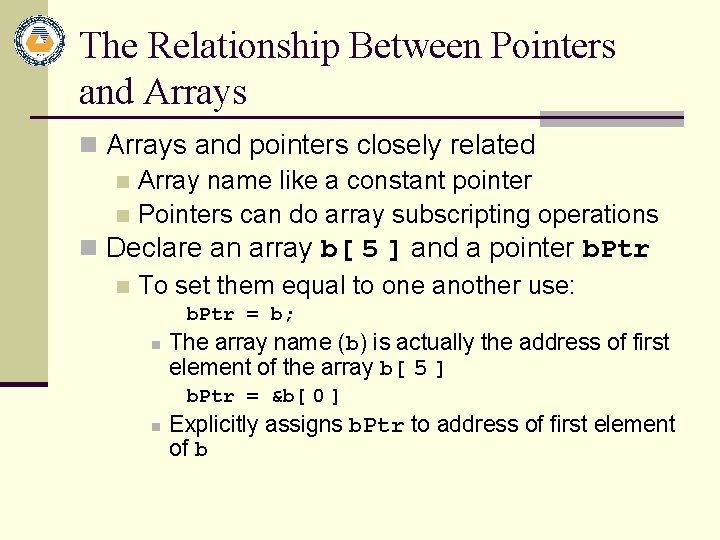
![The Relationship Between Pointers and Arrays n Element b[ 3 ] n Can be The Relationship Between Pointers and Arrays n Element b[ 3 ] n Can be](https://slidetodoc.com/presentation_image_h2/08f15475828dd8426a6b0b8799f69621/image-18.jpg)
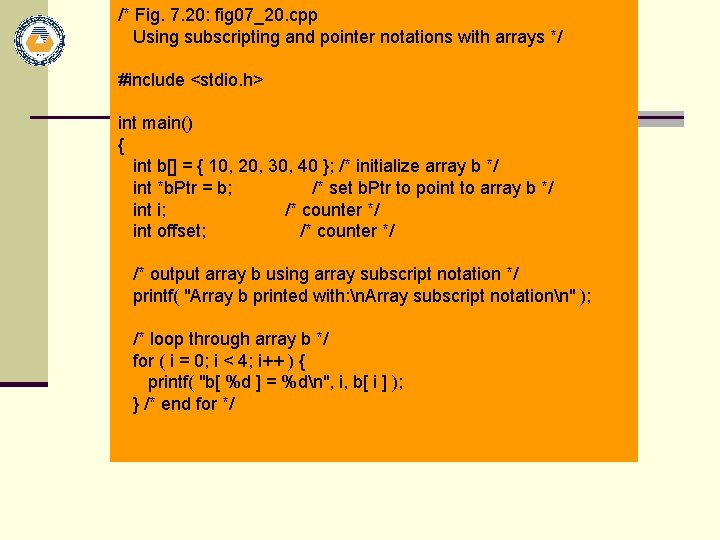
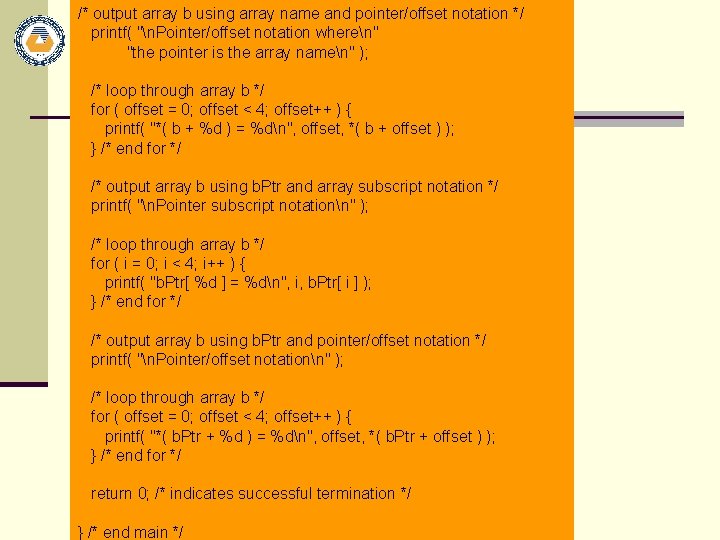
![Array b printed with: Array subscript notation b[ 0 ] = 10 b[ 1 Array b printed with: Array subscript notation b[ 0 ] = 10 b[ 1](https://slidetodoc.com/presentation_image_h2/08f15475828dd8426a6b0b8799f69621/image-21.jpg)
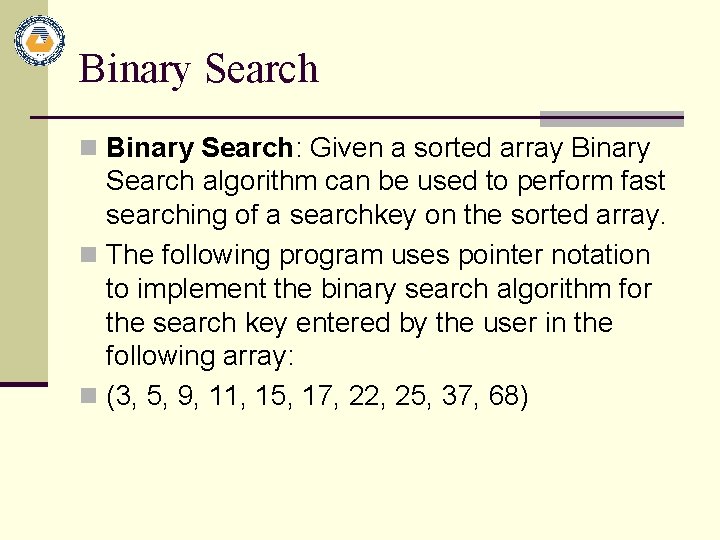
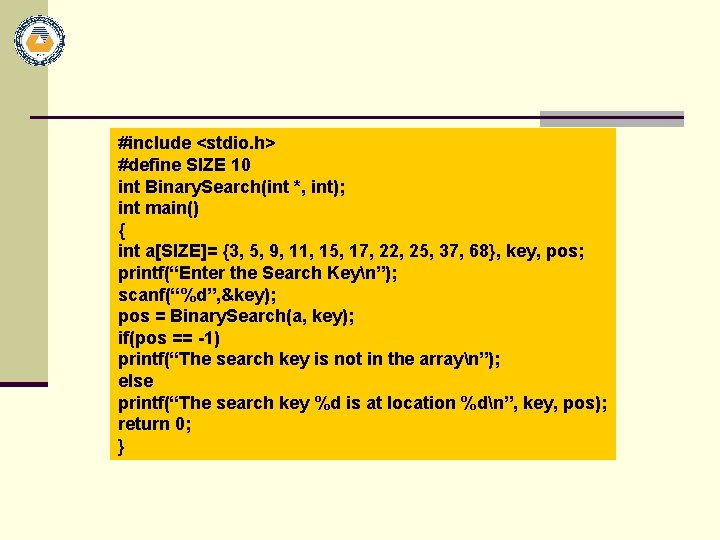
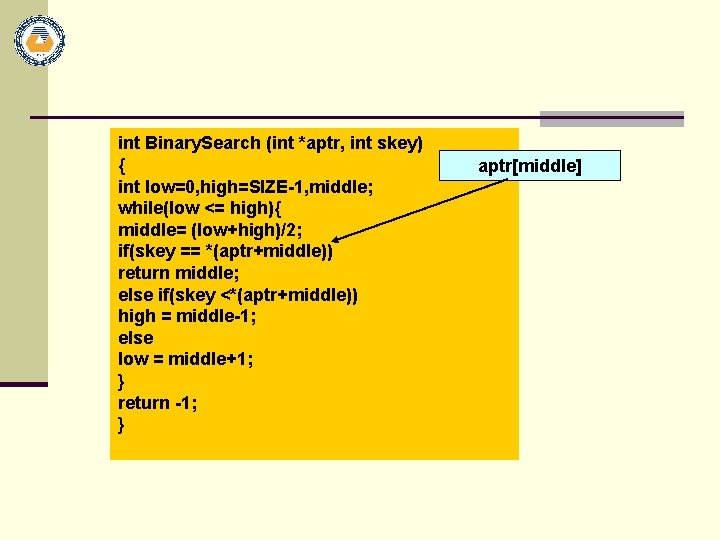
- Slides: 24
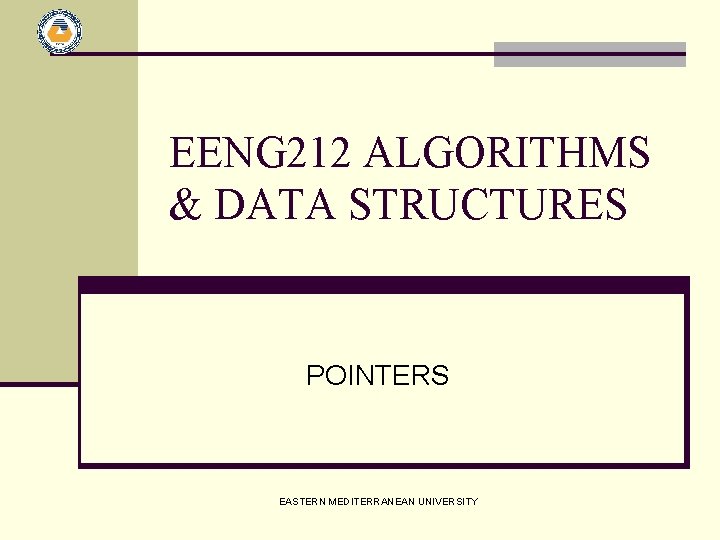
EENG 212 ALGORITHMS & DATA STRUCTURES POINTERS EASTERN MEDITERRANEAN UNIVERSITY
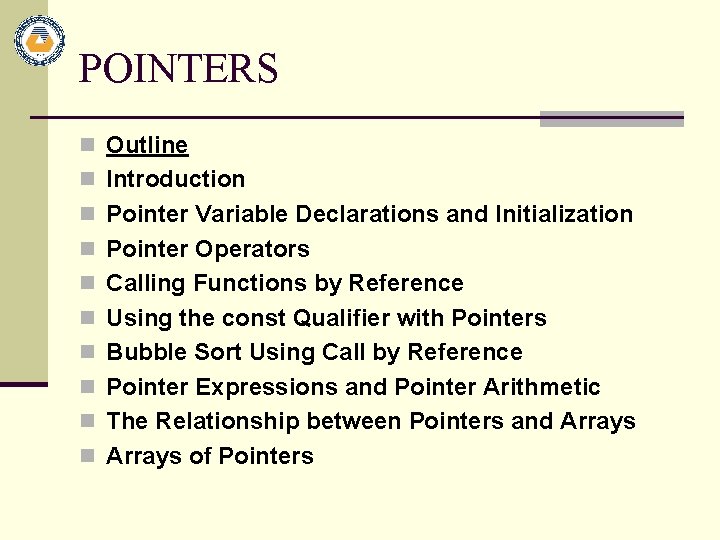
POINTERS n Outline n Introduction n Pointer Variable Declarations and Initialization n Pointer Operators n Calling Functions by Reference n Using the const Qualifier with Pointers n Bubble Sort Using Call by Reference n Pointer Expressions and Pointer Arithmetic n The Relationship between Pointers and Arrays n Arrays of Pointers
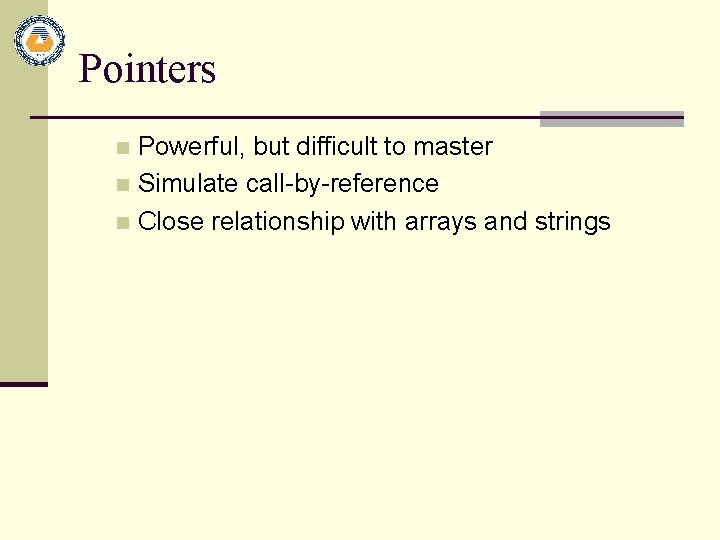
Pointers Powerful, but difficult to master n Simulate call-by-reference n Close relationship with arrays and strings n
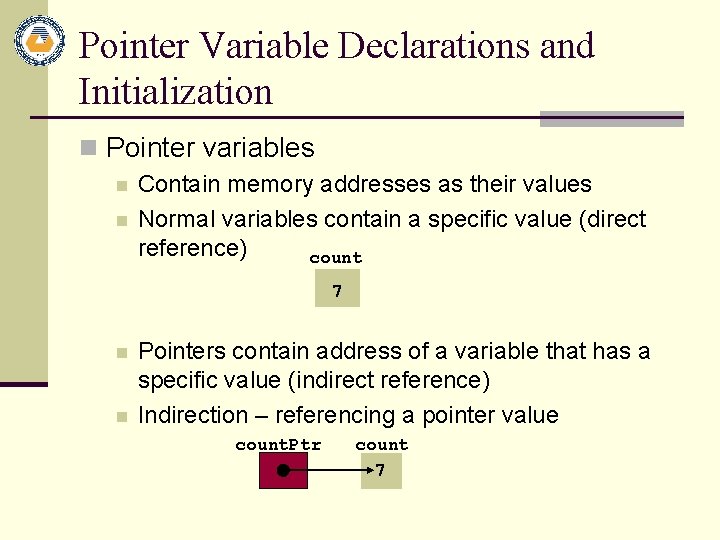
Pointer Variable Declarations and Initialization n Pointer variables n n Contain memory addresses as their values Normal variables contain a specific value (direct reference) count 7 n n Pointers contain address of a variable that has a specific value (indirect reference) Indirection – referencing a pointer value count. Ptr count 7
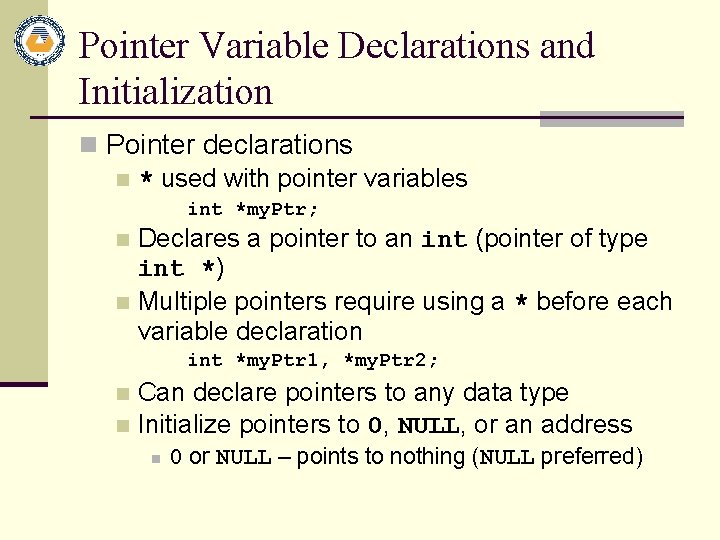
Pointer Variable Declarations and Initialization n Pointer declarations n * used with pointer variables int *my. Ptr; Declares a pointer to an int (pointer of type int *) n Multiple pointers require using a * before each variable declaration n int *my. Ptr 1, *my. Ptr 2; Can declare pointers to any data type n Initialize pointers to 0, NULL, or an address n n 0 or NULL – points to nothing (NULL preferred)
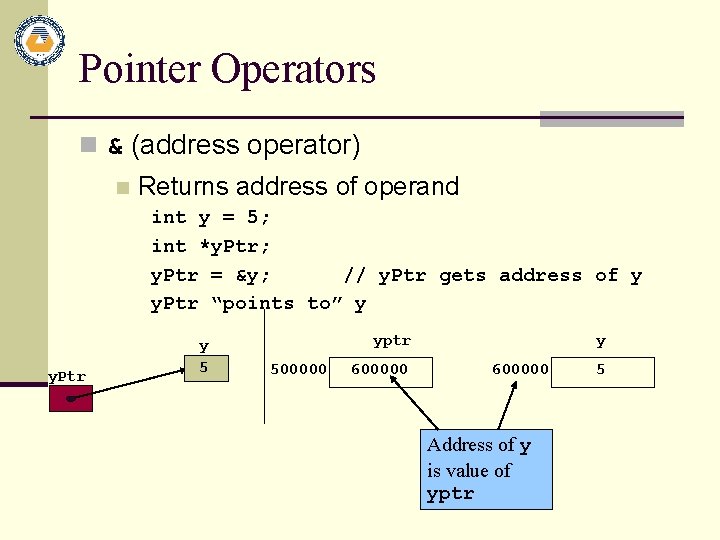
Pointer Operators n & (address operator) n Returns address of operand int y = 5; int *y. Ptr; y. Ptr = &y; // y. Ptr gets address of y y. Ptr “points to” y y. Ptr y 5 yptr 500000 600000 y 600000 Address of y is value of yptr 5
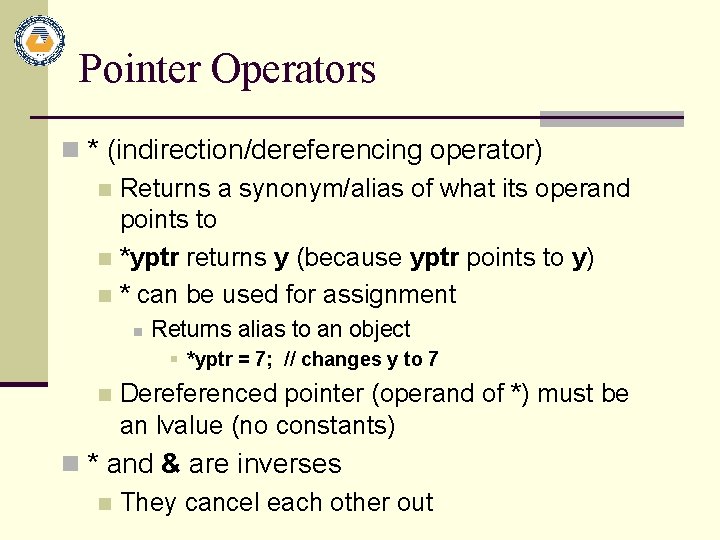
Pointer Operators n * (indirection/dereferencing operator) n Returns a synonym/alias of what its operand points to n *yptr returns y (because yptr points to y) n * can be used for assignment n Returns alias to an object § *yptr = 7; // changes y to 7 n Dereferenced pointer (operand of *) must be an lvalue (no constants) n * and & are inverses n They cancel each other out
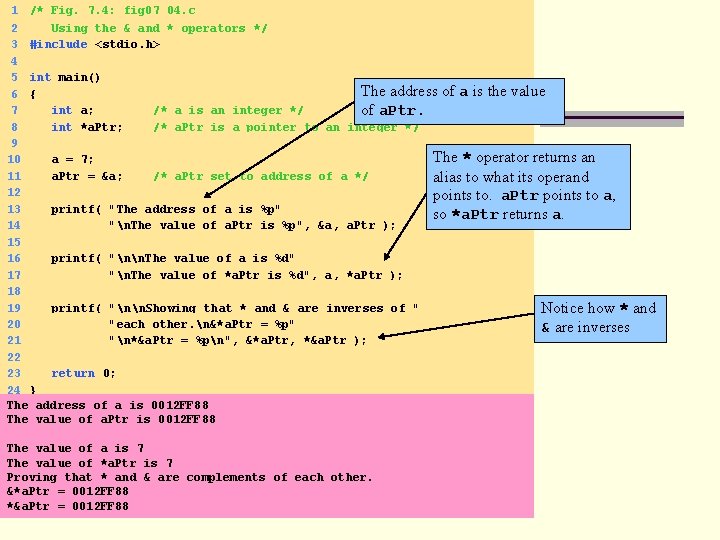
1 /* Fig. 7. 4: fig 07_04. c 2 Using the & and * operators */ 3 #include <stdio. h> 4 5 int main() The address of a is the value 6 { 7 int a; /* a is an integer */ of a. Ptr. 8 int *a. Ptr; /* a. Ptr is a pointer to an integer */ 9 The * operator returns an 10 a = 7; 11 a. Ptr = &a; /* a. Ptr set to address of a */ alias to what its operand 12 points to. a. Ptr points to a, 13 printf( "The address of a is %p" so *a. Ptr returns a. 14 "n. The value of a. Ptr is %p", &a, a. Ptr ); 15 16 printf( "nn. The value of a is %d" 17 "n. The value of *a. Ptr is %d", a, *a. Ptr ); 18 19 printf( "nn. Showing that * and & are inverses of " Notice how * and 20 "each other. n&*a. Ptr = %p" & are inverses 21 "n*&a. Ptr = %pn", &*a. Ptr, *&a. Ptr ); 22 23 return 0; 24 } The address of a is 0012 FF 88 The value of a. Ptr is 0012 FF 88 The value of a is 7 The value of *a. Ptr is 7 Proving that * and & are complements of each other. &*a. Ptr = 0012 FF 88 *&a. Ptr = 0012 FF 88
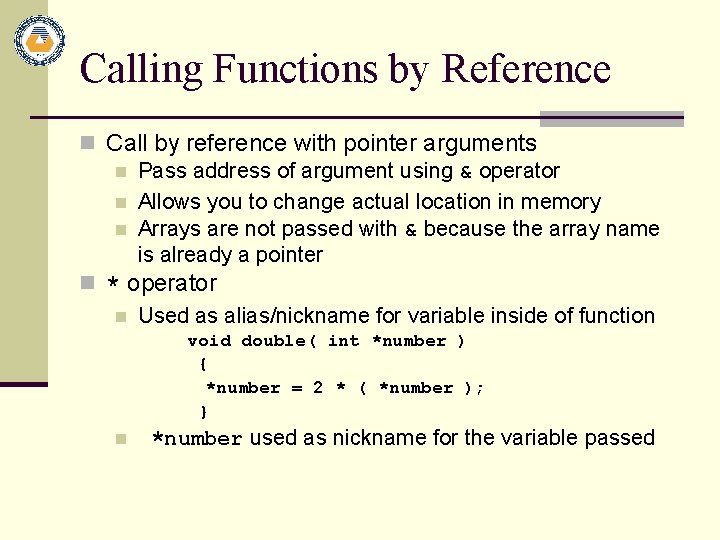
Calling Functions by Reference n Call by reference with pointer arguments n Pass address of argument using & operator n Allows you to change actual location in memory n Arrays are not passed with & because the array name is already a pointer n * operator n Used as alias/nickname for variable inside of function void double( int *number ) { *number = 2 * ( *number ); } n *number used as nickname for the variable passed
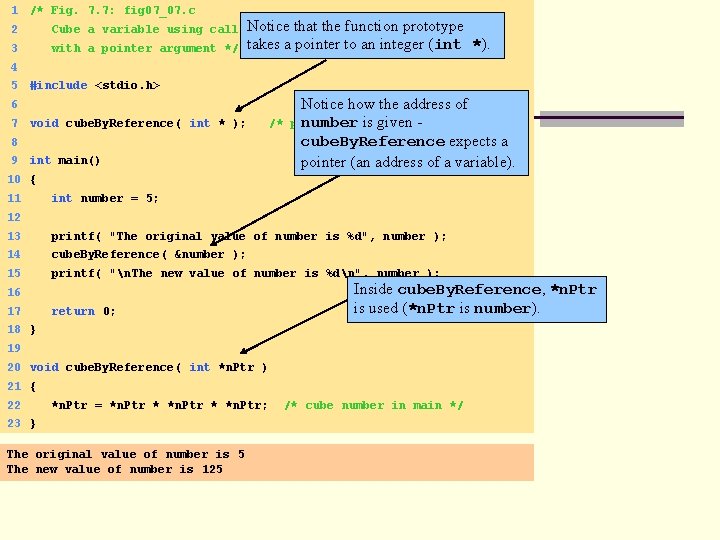
1 /* Fig. 7. 7: fig 07_07. c 2 Notice that the Cube a variable using call-by-reference function prototype takes a pointer to an integer (int *). 3 with a pointer argument */ 4 5 #include <stdio. h> 6 7 void cube. By. Reference( int * ); 8 9 int main() /* Notice how the address of prototype */given number is cube. By. Reference expects a pointer (an address of a variable). 10 { 11 int number = 5; 12 13 printf( "The original value of number is %d", number ); 14 cube. By. Reference( &number ); 15 printf( "n. The new value of number is %dn", number ); 16 17 return 0; Inside cube. By. Reference, *n. Ptr is used (*n. Ptr is number). 18 } 19 20 void cube. By. Reference( int *n. Ptr ) 21 { 22 *n. Ptr = *n. Ptr * *n. Ptr; 23 } The original value of number is 5 The new value of number is 125 /* cube number in main */
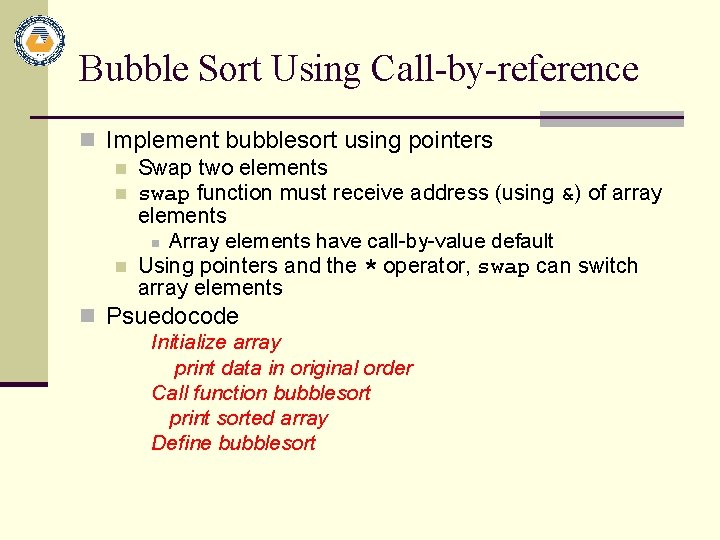
Bubble Sort Using Call-by-reference n Implement bubblesort using pointers n Swap two elements n swap function must receive address (using &) of array elements n Array elements have call-by-value default n Using pointers and the * operator, swap can switch array elements n Psuedocode Initialize array print data in original order Call function bubblesort print sorted array Define bubblesort
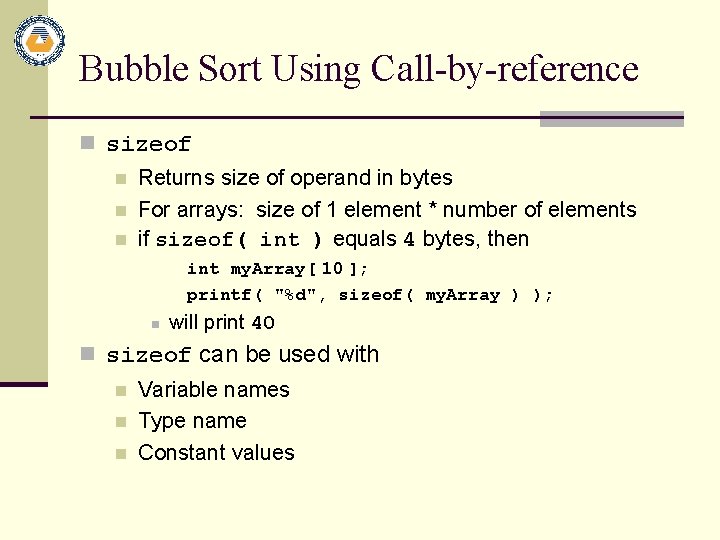
Bubble Sort Using Call-by-reference n sizeof n n n Returns size of operand in bytes For arrays: size of 1 element * number of elements if sizeof( int ) equals 4 bytes, then int my. Array[ 10 ]; printf( "%d", sizeof( my. Array ) ); n will print 40 n sizeof can be used with n n n Variable names Type name Constant values
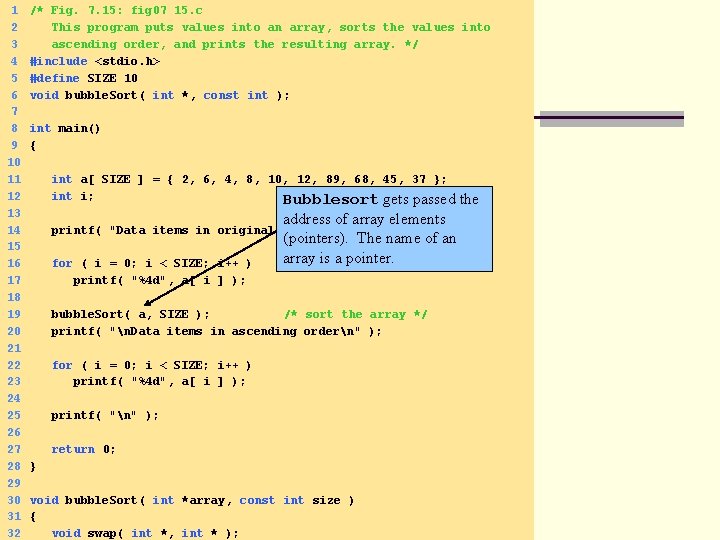
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 /* Fig. 7. 15: fig 07_15. c This program puts values into an array, sorts the values into ascending order, and prints the resulting array. */ #include <stdio. h> #define SIZE 10 void bubble. Sort( int *, const int ); int main() { int a[ SIZE ] = { 2, 6, 4, 8, 10, 12, 89, 68, 45, 37 }; int i; Bubblesort gets passed address of array elements (pointers). The name of an array is a pointer. printf( "Data items in original ordern" ); for ( i = 0; i < SIZE; i++ ) printf( "%4 d", a[ i ] ); bubble. Sort( a, SIZE ); /* sort the array */ printf( "n. Data items in ascending ordern" ); for ( i = 0; i < SIZE; i++ ) printf( "%4 d", a[ i ] ); printf( "n" ); return 0; } void bubble. Sort( int *array, const int size ) { void swap( int *, int * ); the
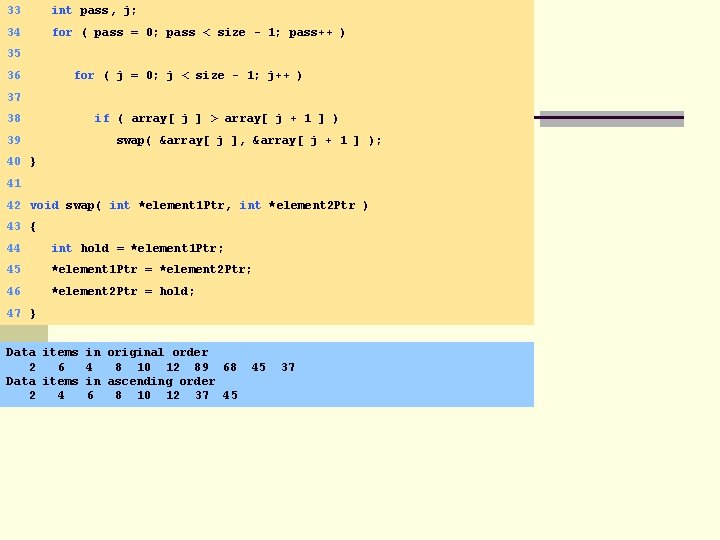
33 int pass, j; 34 for ( pass = 0; pass < size - 1; pass++ ) 35 36 for ( j = 0; j < size - 1; j++ ) 37 38 39 if ( array[ j ] > array[ j + 1 ] ) swap( &array[ j ], &array[ j + 1 ] ); 40 } 41 42 void swap( int *element 1 Ptr, int *element 2 Ptr ) 43 { 44 int hold = *element 1 Ptr; 45 *element 1 Ptr = *element 2 Ptr; 46 *element 2 Ptr = hold; 47 } Data items in original order 2 6 4 8 10 12 89 68 Data items in ascending order 2 4 6 8 10 12 37 45 45 37
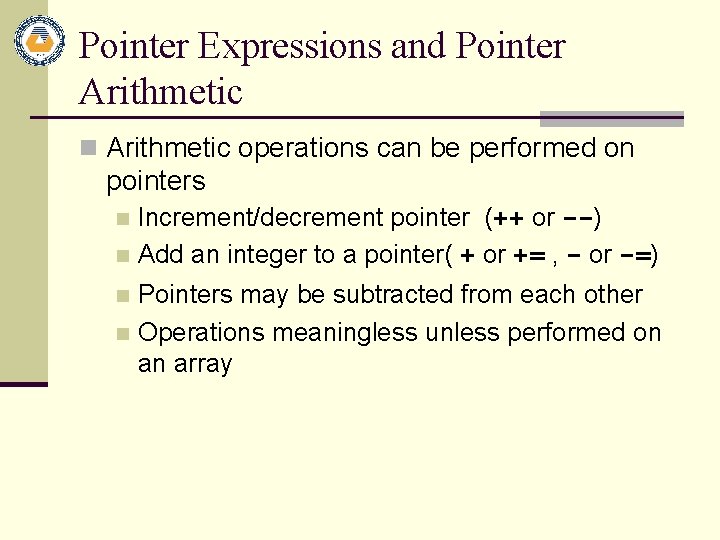
Pointer Expressions and Pointer Arithmetic n Arithmetic operations can be performed on pointers Increment/decrement pointer (++ or --) n Add an integer to a pointer( + or += , - or -=) n Pointers may be subtracted from each other n Operations meaningless unless performed on an array n
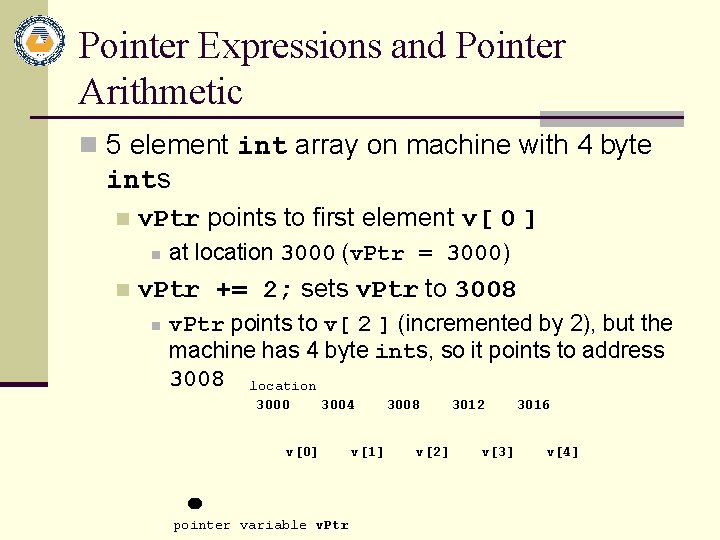
Pointer Expressions and Pointer Arithmetic n 5 element int array on machine with 4 byte ints n v. Ptr points to first element v[ 0 ] n n at location 3000 (v. Ptr = 3000) v. Ptr += 2; sets v. Ptr to 3008 n v. Ptr points to v[ 2 ] (incremented by 2), but the machine has 4 byte ints, so it points to address 3008 location 3000 3004 v[0] pointer variable v. Ptr v[1] 3008 v[2] 3012 v[3] 3016 v[4]
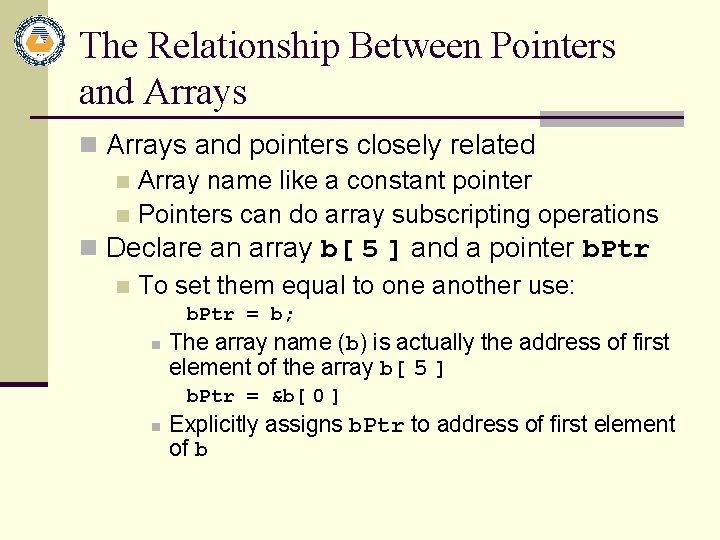
The Relationship Between Pointers and Arrays n Arrays and pointers closely related n Array name like a constant pointer n Pointers can do array subscripting operations n Declare an array b[ 5 ] and a pointer b. Ptr n To set them equal to one another use: b. Ptr = b; n The array name (b) is actually the address of first element of the array b[ 5 ] b. Ptr = &b[ 0 ] n Explicitly assigns b. Ptr to address of first element of b
![The Relationship Between Pointers and Arrays n Element b 3 n Can be The Relationship Between Pointers and Arrays n Element b[ 3 ] n Can be](https://slidetodoc.com/presentation_image_h2/08f15475828dd8426a6b0b8799f69621/image-18.jpg)
The Relationship Between Pointers and Arrays n Element b[ 3 ] n Can be accessed by *( b. Ptr + 3 ) § Where n is the offset. Called pointer/offset notation n Can be accessed by bptr[ 3 ] § Called pointer/subscript notation § b. Ptr[ 3 ] same as b[ 3 ] n Can be accessed by performing pointer arithmetic on the array itself *( b + 3 )
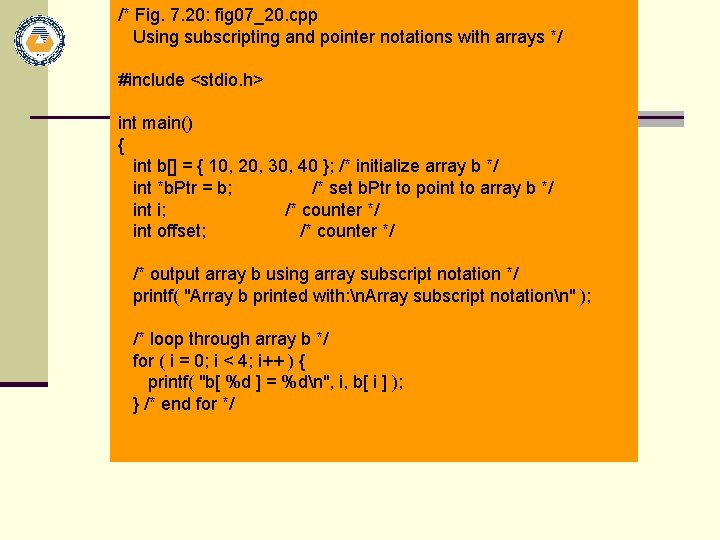
/* Fig. 7. 20: fig 07_20. cpp Using subscripting and pointer notations with arrays */ #include <stdio. h> int main() { int b[] = { 10, 20, 30, 40 }; /* initialize array b */ int *b. Ptr = b; /* set b. Ptr to point to array b */ int i; /* counter */ int offset; /* counter */ /* output array b using array subscript notation */ printf( "Array b printed with: n. Array subscript notationn" ); /* loop through array b */ for ( i = 0; i < 4; i++ ) { printf( "b[ %d ] = %dn", i, b[ i ] ); } /* end for */
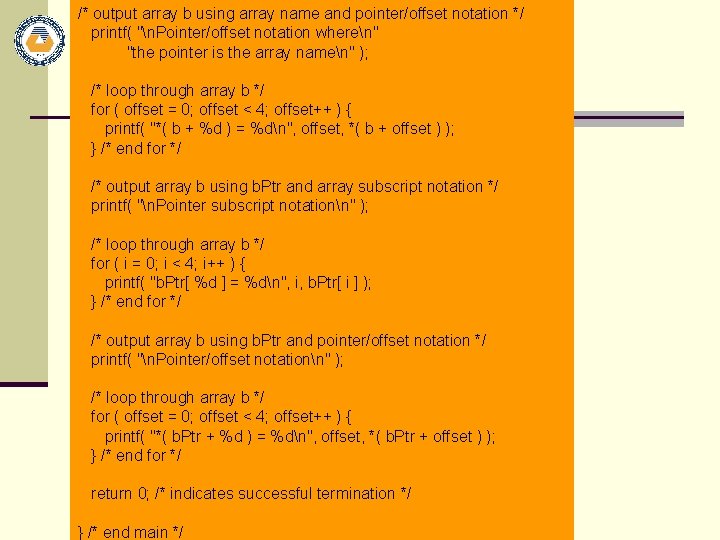
/* output array b using array name and pointer/offset notation */ printf( "n. Pointer/offset notation wheren" "the pointer is the array namen" ); /* loop through array b */ for ( offset = 0; offset < 4; offset++ ) { printf( "*( b + %d ) = %dn", offset, *( b + offset ) ); } /* end for */ /* output array b using b. Ptr and array subscript notation */ printf( "n. Pointer subscript notationn" ); /* loop through array b */ for ( i = 0; i < 4; i++ ) { printf( "b. Ptr[ %d ] = %dn", i, b. Ptr[ i ] ); } /* end for */ /* output array b using b. Ptr and pointer/offset notation */ printf( "n. Pointer/offset notationn" ); /* loop through array b */ for ( offset = 0; offset < 4; offset++ ) { printf( "*( b. Ptr + %d ) = %dn", offset, *( b. Ptr + offset ) ); } /* end for */ return 0; /* indicates successful termination */ } /* end main */
![Array b printed with Array subscript notation b 0 10 b 1 Array b printed with: Array subscript notation b[ 0 ] = 10 b[ 1](https://slidetodoc.com/presentation_image_h2/08f15475828dd8426a6b0b8799f69621/image-21.jpg)
Array b printed with: Array subscript notation b[ 0 ] = 10 b[ 1 ] = 20 b[ 2 ] = 30 b[ 3 ] = 40 Pointer/offset notation where the pointer is the array name *( b + 0 ) = 10 *( b + 1 ) = 20 *( b + 2 ) = 30 *( b + 3 ) = 40 Pointer subscript notation b. Ptr[ 0 ] = 10 b. Ptr[ 1 ] = 20 b. Ptr[ 2 ] = 30 b. Ptr[ 3 ] = 40 Pointer/offset notation *( b. Ptr + 0 ) = 10 *( b. Ptr + 1 ) = 20 *( b. Ptr + 2 ) = 30 *( b. Ptr + 3 ) = 40 Press any key to continue
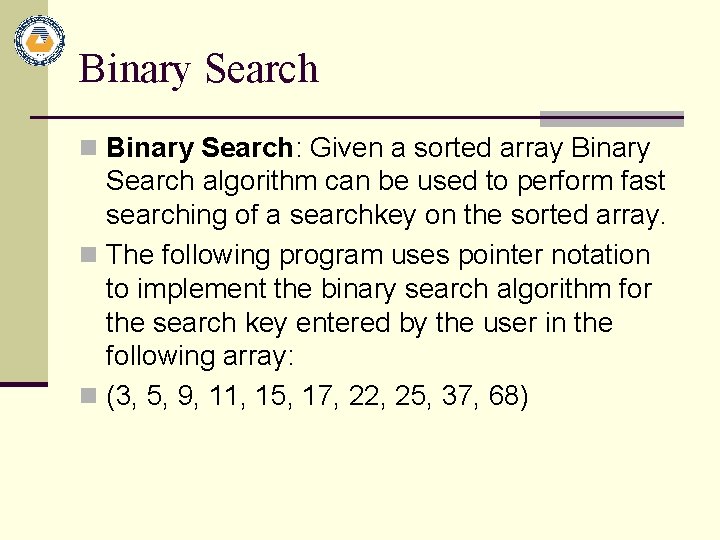
Binary Search n Binary Search: Given a sorted array Binary Search algorithm can be used to perform fast searching of a searchkey on the sorted array. n The following program uses pointer notation to implement the binary search algorithm for the search key entered by the user in the following array: n (3, 5, 9, 11, 15, 17, 22, 25, 37, 68)
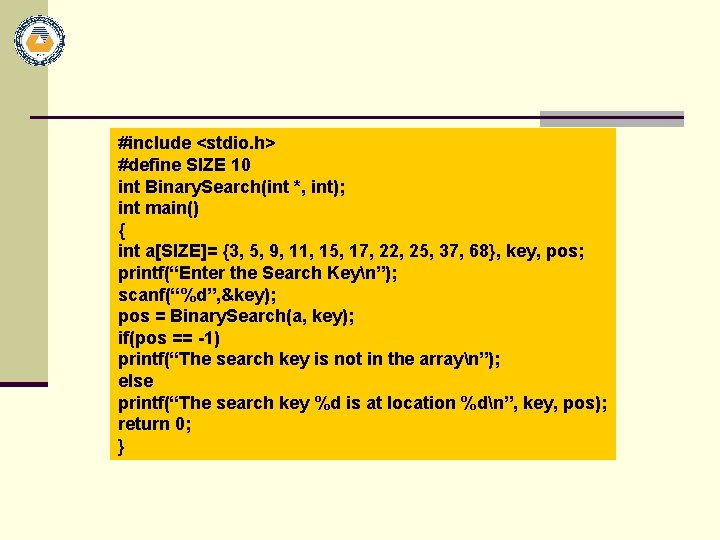
#include <stdio. h> #define SIZE 10 int Binary. Search(int *, int); int main() { int a[SIZE]= {3, 5, 9, 11, 15, 17, 22, 25, 37, 68}, key, pos; printf(“Enter the Search Keyn”); scanf(“%d”, &key); pos = Binary. Search(a, key); if(pos == -1) printf(“The search key is not in the arrayn”); else printf(“The search key %d is at location %dn”, key, pos); return 0; }
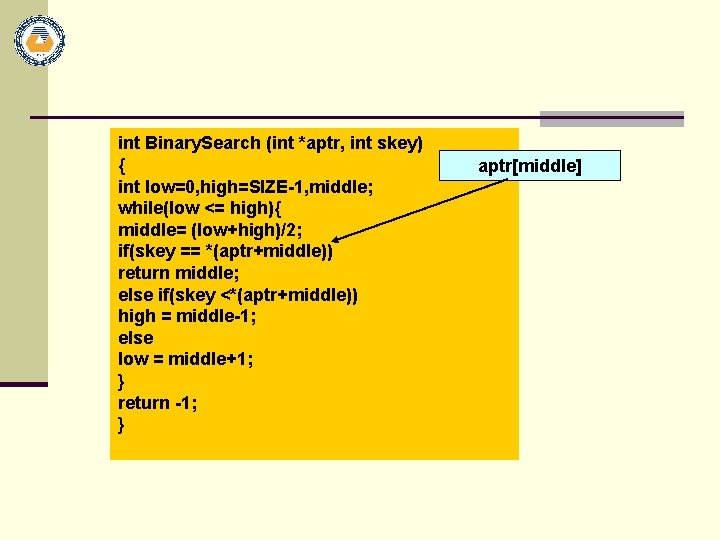
int Binary. Search (int *aptr, int skey) { int low=0, high=SIZE-1, middle; while(low <= high){ middle= (low+high)/2; if(skey == *(aptr+middle)) return middle; else if(skey <*(aptr+middle)) high = middle-1; else low = middle+1; } return -1; } aptr[middle]
Chapter 16 eastern mediterranean answers
Data structures and algorithms iit bombay
Princeton data structures and algorithms
Data structures and algorithms tutorial
Information retrieval data structures and algorithms
Data structures and algorithms bits pilani
Ajit diwan iit bombay
Algorithms + data structures = programs
Data structures and algorithms
Data structures and algorithms
Waterloo data structures and algorithms
Signature file structure in information retrieval system
Data structures and algorithms
Homology
Q=vc physics
Physics 212 gradebook
Pese 212
Nkb 212 sungguh inginkah engkau lakukan
Ienf-212
Et 212
Et 212
Arm reach 212 cm on tiptoes
Archimedes ( arşimet) (mö 287–212 )
287-212
Phys 212 equation sheet