Pointers to pointers multidimensional arrays Pointers to pointers
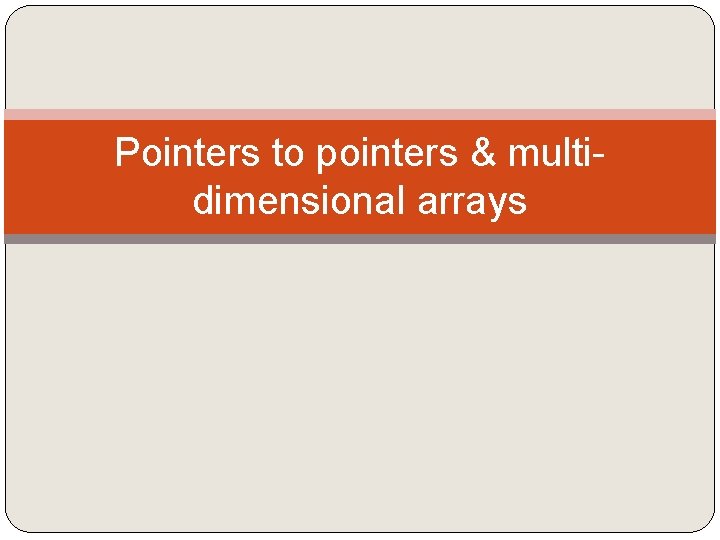
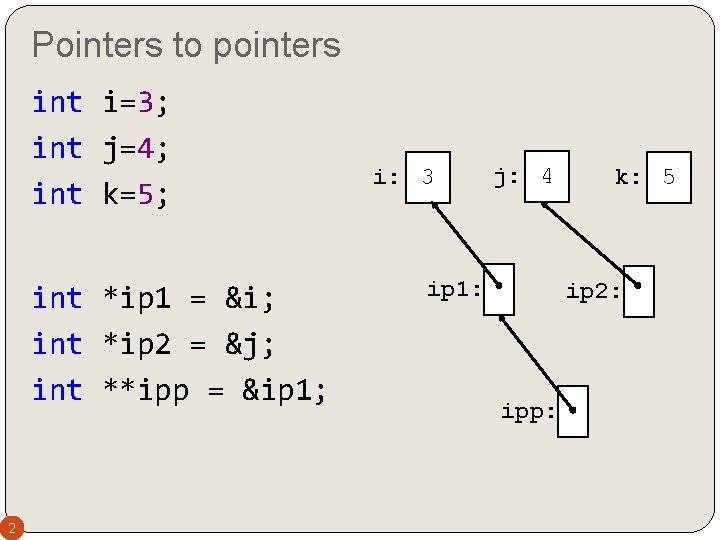
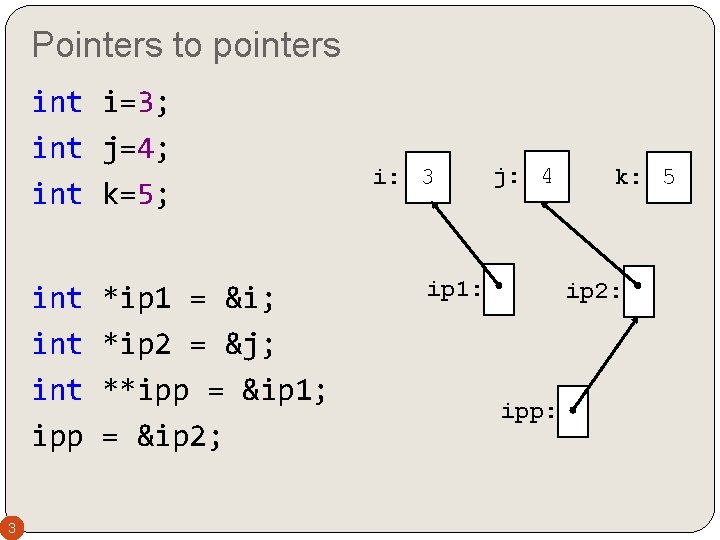
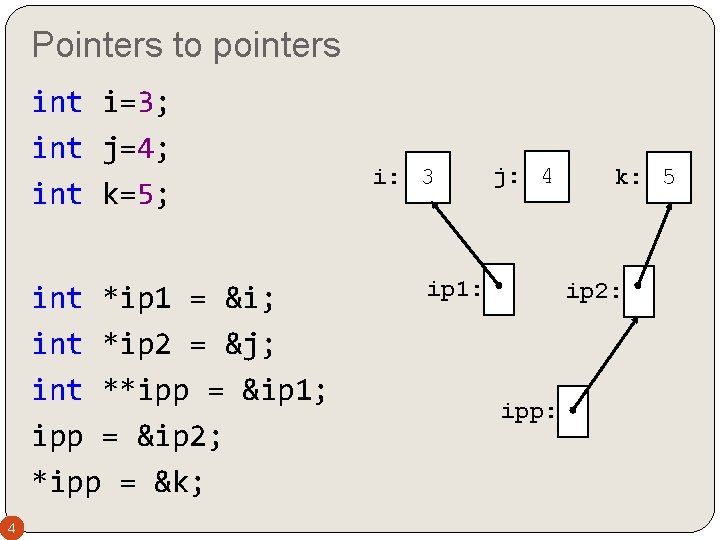
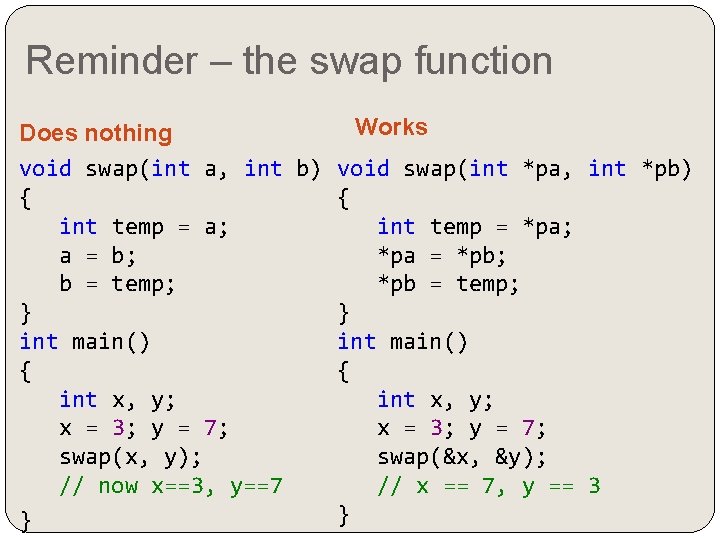
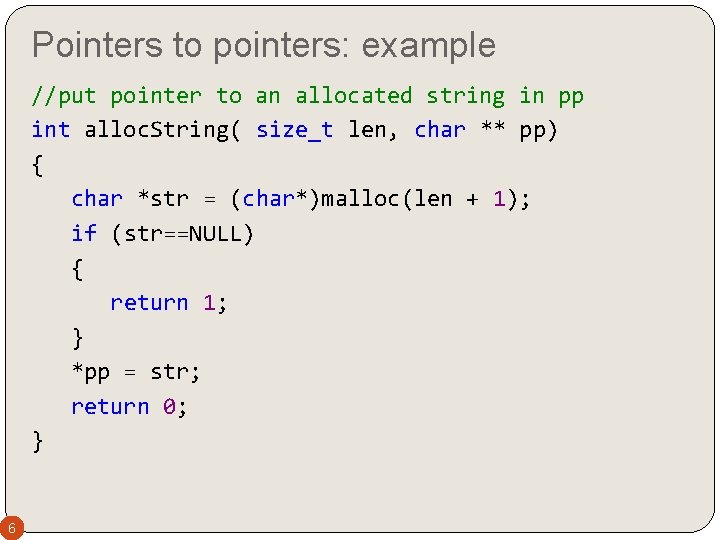
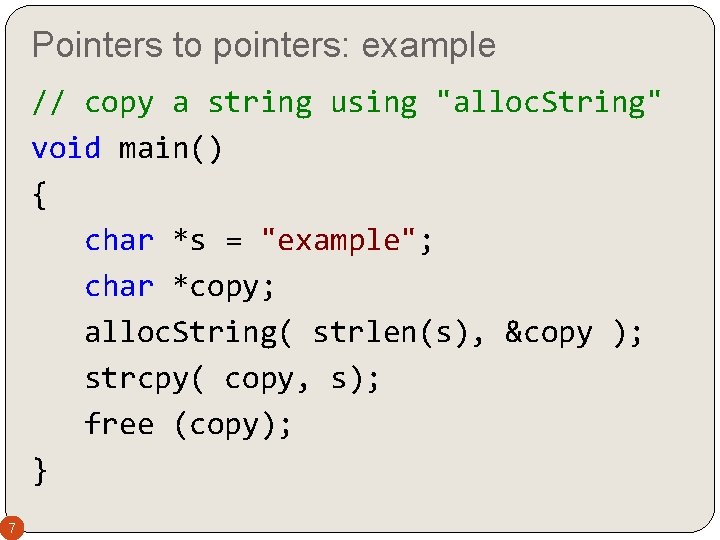
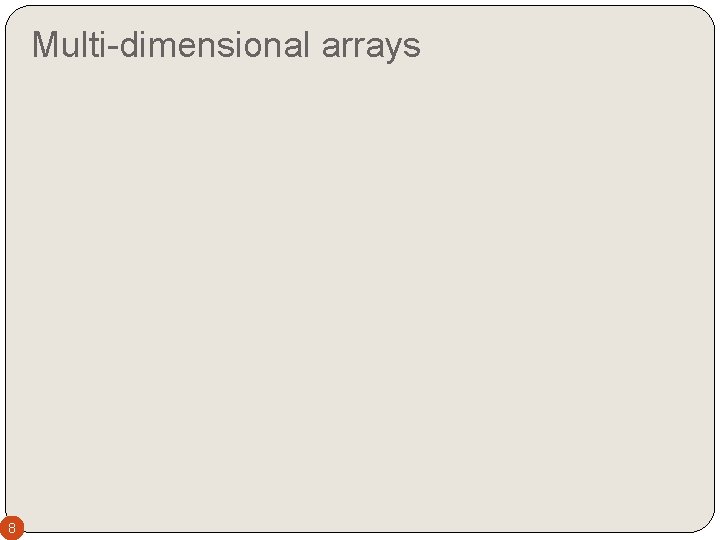
![Multi-dimensional arrays Can be created in few ways: 1. Automatically: int m[2][3]; // 2 Multi-dimensional arrays Can be created in few ways: 1. Automatically: int m[2][3]; // 2](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-9.jpg)
![Automatic multi-dimensional arrays #define R 2 #define C 3 int m[R][C]; int* m_ptr= &(m[0][0]); Automatic multi-dimensional arrays #define R 2 #define C 3 int m[R][C]; int* m_ptr= &(m[0][0]);](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-10.jpg)
![Automatic multi-dimensional arrays #define R 2 #define C 3 int m[R][C]; int* m_ptr; for Automatic multi-dimensional arrays #define R 2 #define C 3 int m[R][C]; int* m_ptr; for](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-11.jpg)
![Automatic multi-dimensional arrays int A[R][C]; Like a matrix A[0][0] A[0][1] … A[0][c-1] … … Automatic multi-dimensional arrays int A[R][C]; Like a matrix A[0][0] A[0][1] … A[0][c-1] … …](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-12.jpg)
![Semi-dynamic multi-dimensional arrays 2. Semi-dynamic: Define an array of pointers: int* m[5]; // allocates Semi-dynamic multi-dimensional arrays 2. Semi-dynamic: Define an array of pointers: int* m[5]; // allocates](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-13.jpg)
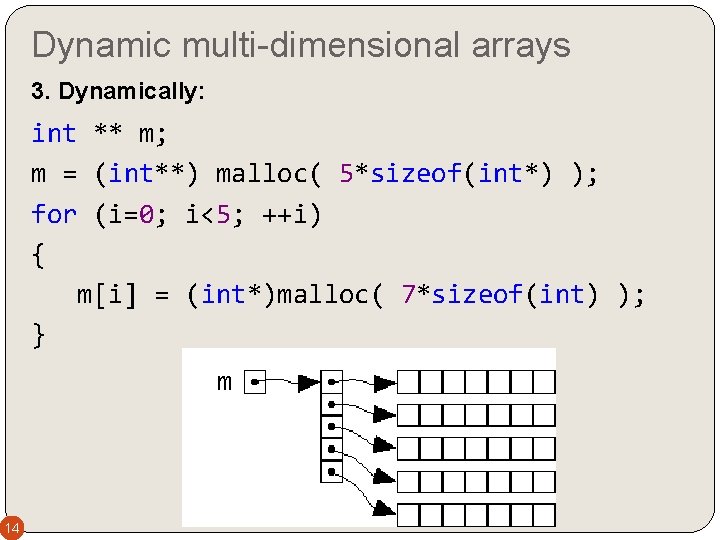
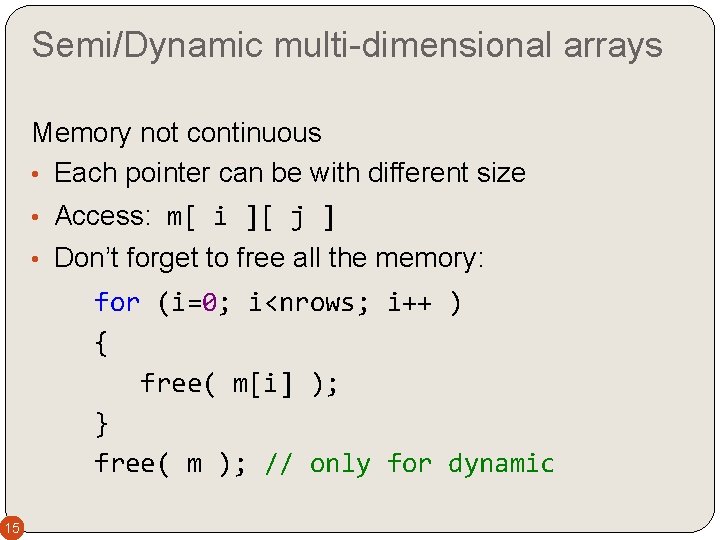
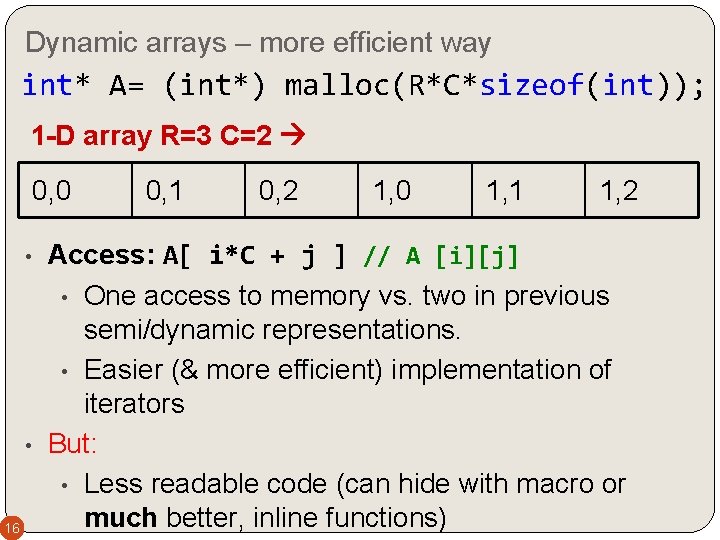
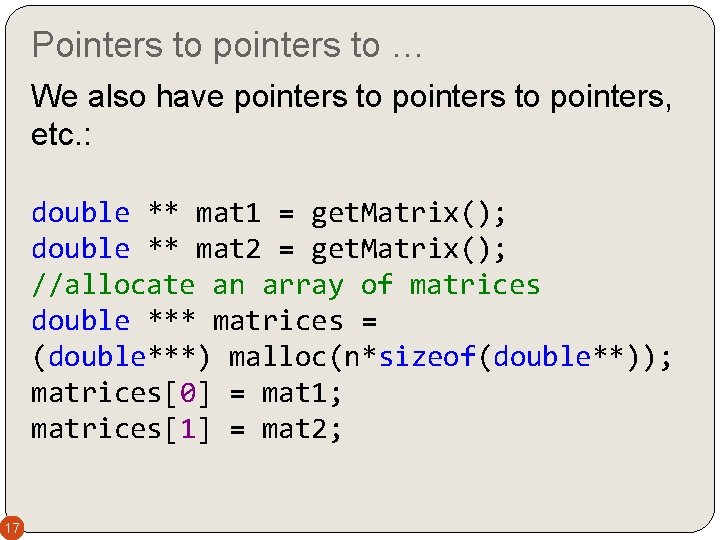
![Automatic multi-dimensional arrays as arguments to functions int x[5][7]; // 5 rows, 7 columns Automatic multi-dimensional arrays as arguments to functions int x[5][7]; // 5 rows, 7 columns](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-18.jpg)
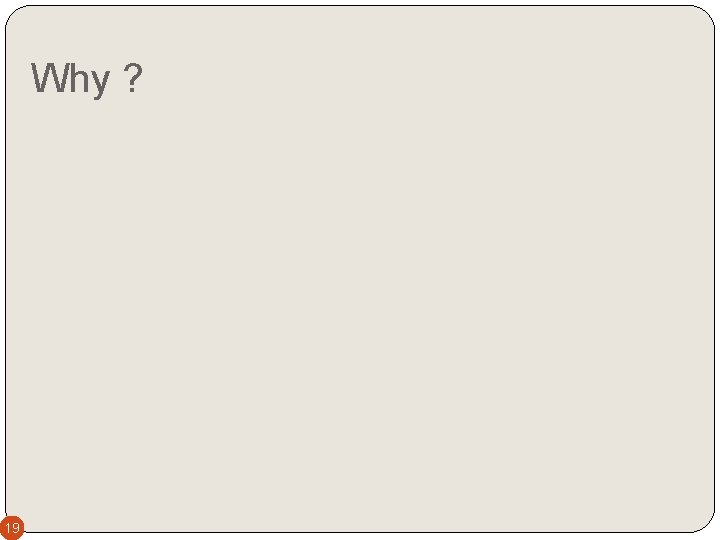
![Pointers to arrays (of size X): int foo (char m[][20]); m is a pointer Pointers to arrays (of size X): int foo (char m[][20]); m is a pointer](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-20.jpg)
![Pointers to arrays (of size X): Explicit declaration: char (*arr_2 d)[20]; // arr_2 d Pointers to arrays (of size X): Explicit declaration: char (*arr_2 d)[20]; // arr_2 d](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-21.jpg)
![Pointers to arrays (of size X): char (*m)[5]; m 22 char *m[5]; Pointers to arrays (of size X): char (*m)[5]; m 22 char *m[5];](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-22.jpg)
![Pointers to arrays (of size X): char (*m)[5]; m After initialization: char arr[5]; m= Pointers to arrays (of size X): char (*m)[5]; m After initialization: char arr[5]; m=](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-23.jpg)
![Pointers to arrays (of size X): char (*m)[5]; sizeof (m) = sizeof(void*) sizeof (*m) Pointers to arrays (of size X): char (*m)[5]; sizeof (m) = sizeof(void*) sizeof (*m)](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-24.jpg)
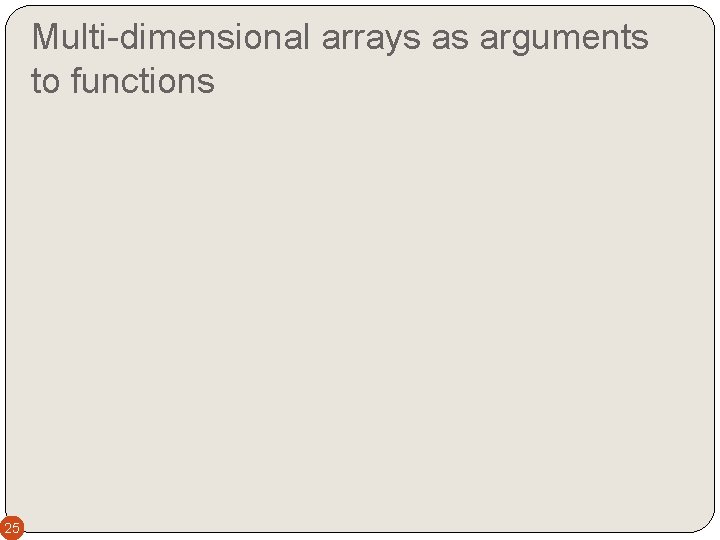
![Multi-dimensional arrays as arguments to functions void foo( int matrix[ROWS_NUM][COLS_NUM] ) { 26 Multi-dimensional arrays as arguments to functions void foo( int matrix[ROWS_NUM][COLS_NUM] ) { 26](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-26.jpg)
![Multi-dimensional arrays as arguments to functions void foo( int matrix[ROWS_NUM][COLS_NUM] ){ [ROWS_NUM] What is Multi-dimensional arrays as arguments to functions void foo( int matrix[ROWS_NUM][COLS_NUM] ){ [ROWS_NUM] What is](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-27.jpg)
![Multi-dimensional arrays as arguments to functions void foo( int (*matrix )[COLS_NUM] ) { (* Multi-dimensional arrays as arguments to functions void foo( int (*matrix )[COLS_NUM] ) { (*](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-28.jpg)
![Multi-dimensional arrays as arguments to functions void foo( int (*matrix)[COLS_NUM] ) {. . . Multi-dimensional arrays as arguments to functions void foo( int (*matrix)[COLS_NUM] ) {. . .](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-29.jpg)
![Multi-dimensional arrays as arguments to functions void foo( int (*matrix)[COLS_NUM] ) {. . 30 Multi-dimensional arrays as arguments to functions void foo( int (*matrix)[COLS_NUM] ) {. . 30](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-30.jpg)
![Multi-dimensional arrays as arguments to functions void foo( int (*matrix)[COLS_NUM] ) {. . matrix[r][c] Multi-dimensional arrays as arguments to functions void foo( int (*matrix)[COLS_NUM] ) {. . matrix[r][c]](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-31.jpg)
![Multi-dimensional arrays as arguments to functions void foo( int (*matrix)[COLS_NUM] ) {. . . Multi-dimensional arrays as arguments to functions void foo( int (*matrix)[COLS_NUM] ) {. . .](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-32.jpg)
![Multi-dimensional arrays as arguments to functions void foo( int (*matrix)[COLS_NUM] ) {. . . Multi-dimensional arrays as arguments to functions void foo( int (*matrix)[COLS_NUM] ) {. . .](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-33.jpg)
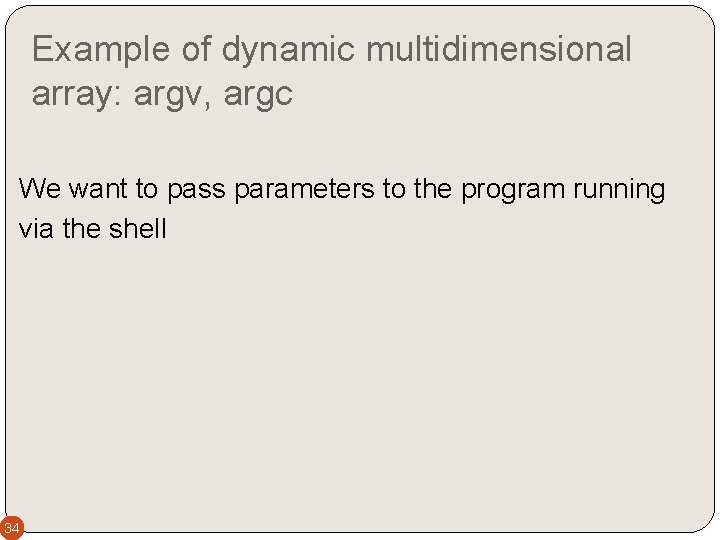
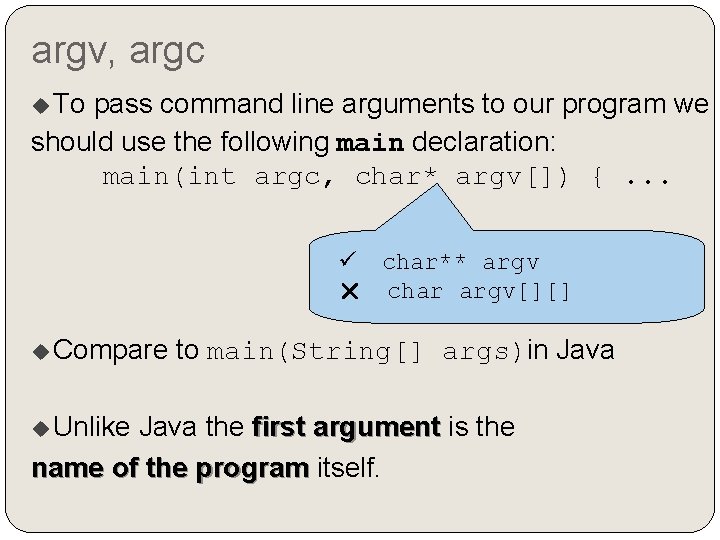
![argv & argc: example $ prog 1 –u danny –p 1234 argc == argv[0] argv & argc: example $ prog 1 –u danny –p 1234 argc == argv[0]](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-36.jpg)
- Slides: 36
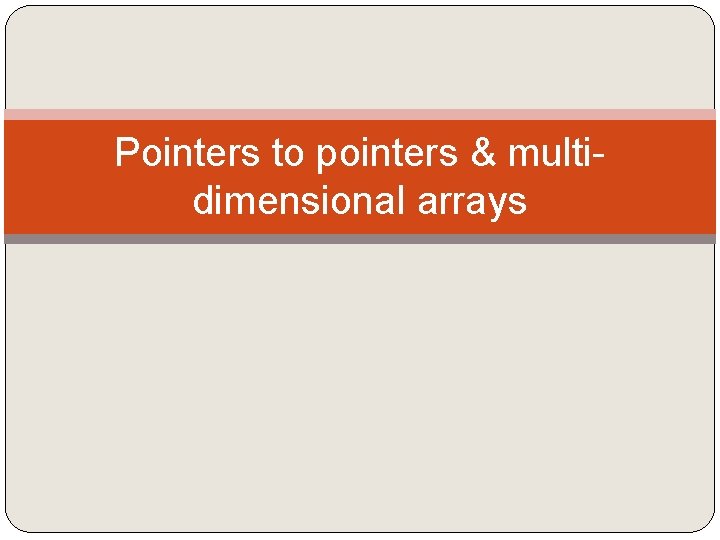
Pointers to pointers & multidimensional arrays
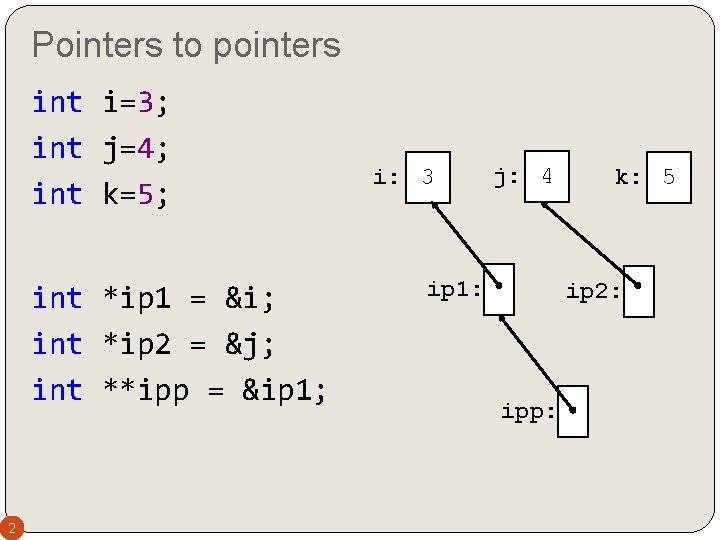
Pointers to pointers int i=3; int j=4; int k=5; int *ip 1 = &i; int *ip 2 = &j; int **ipp = &ip 1; 2 i: 3 j: 4 ip 1: k: 5 ip 2: ipp:
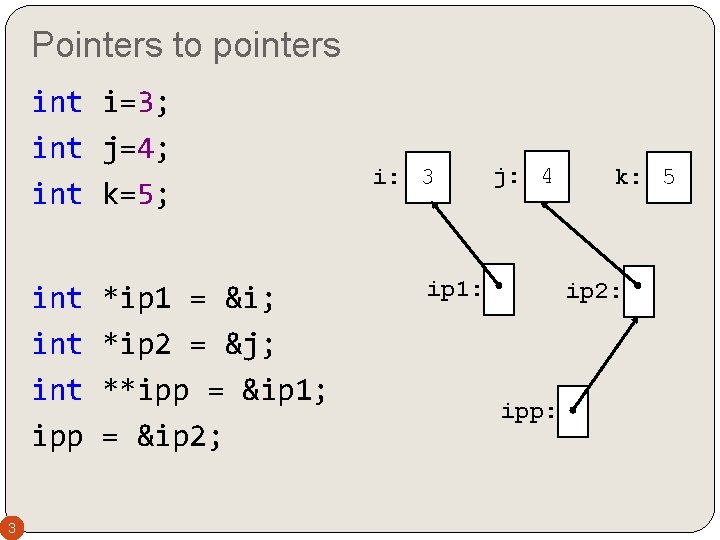
Pointers to pointers int i=3; int j=4; int k=5; int *ip 1 = &i; int *ip 2 = &j; int **ipp = &ip 1; ipp = &ip 2; 3 i: 3 j: 4 ip 1: k: 5 ip 2: ipp:
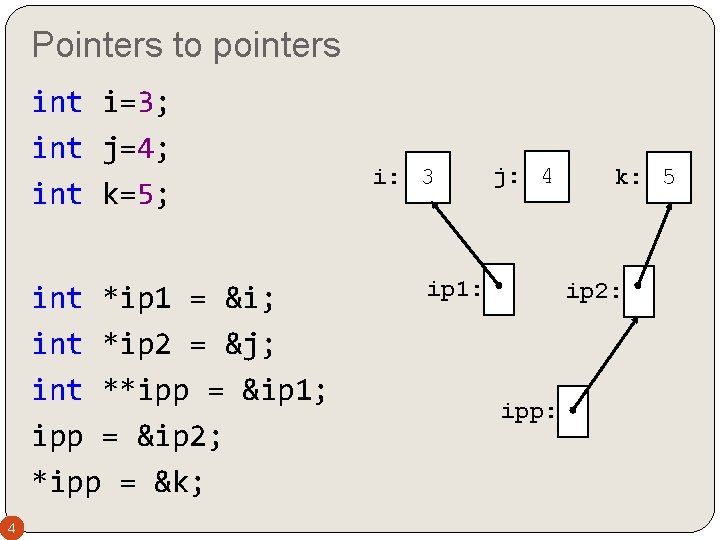
Pointers to pointers int i=3; int j=4; int k=5; int *ip 1 = &i; int *ip 2 = &j; int **ipp = &ip 1; ipp = &ip 2; *ipp = &k; 4 i: 3 j: 4 ip 1: k: 5 ip 2: ipp:
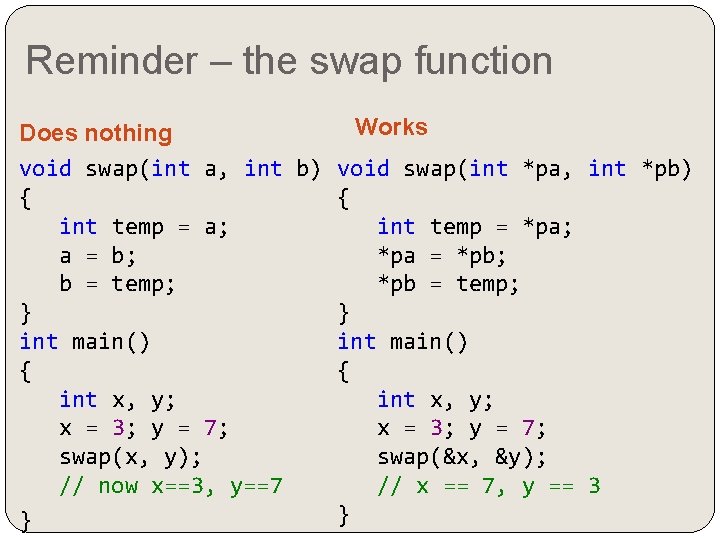
Reminder – the swap function Works Does nothing void swap(int a, int b) void swap(int *pa, int *pb) { { int temp = a; int temp = *pa; a = b; *pa = *pb; b = temp; *pb = temp; } } int main() { { int x, y; x = 3; y = 7; swap(x, y); swap(&x, &y); // now x==3, y==7 // x == 7, y == 3 } }
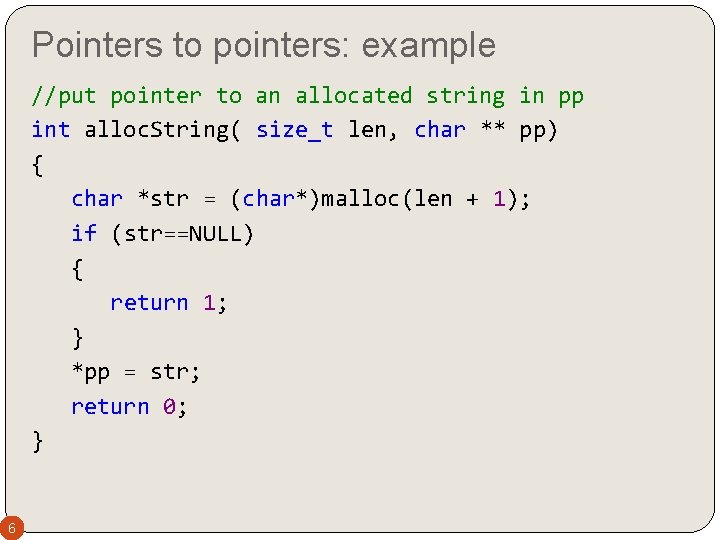
Pointers to pointers: example //put pointer to an allocated string in pp int alloc. String( size_t len, char ** pp) { char *str = (char*)malloc(len + 1); if (str==NULL) { return 1; } *pp = str; return 0; } 6
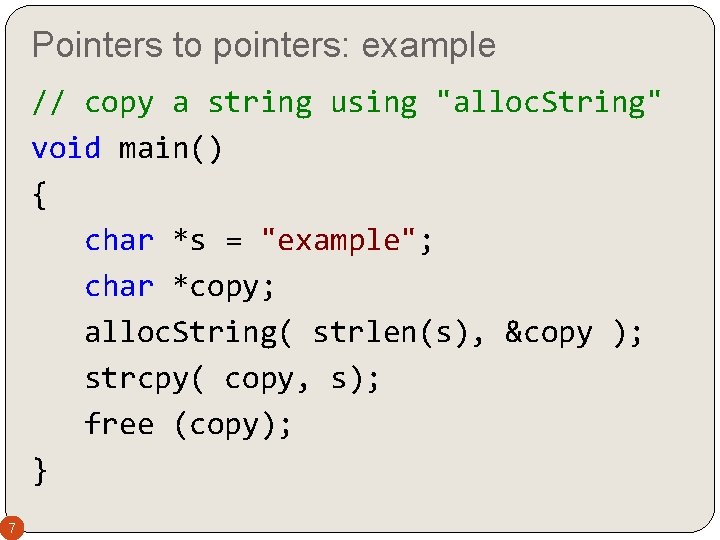
Pointers to pointers: example // copy a string using "alloc. String" void main() { char *s = "example"; char *copy; alloc. String( strlen(s), © ); strcpy( copy, s); free (copy); } 7
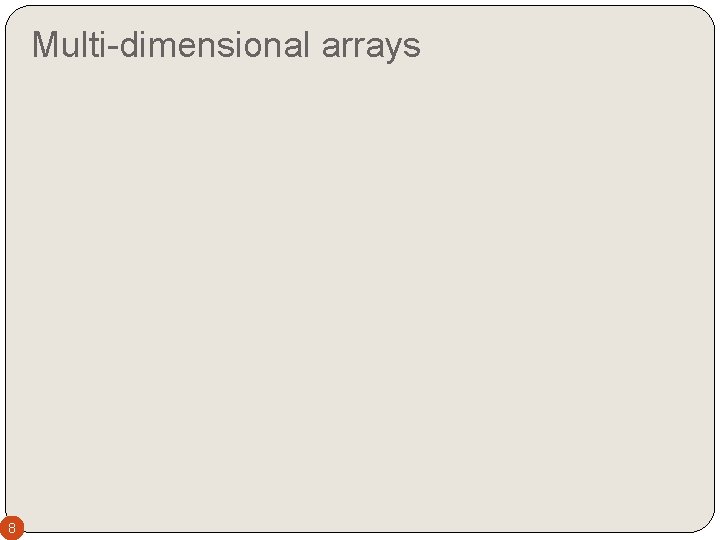
Multi-dimensional arrays 8
![Multidimensional arrays Can be created in few ways 1 Automatically int m23 2 Multi-dimensional arrays Can be created in few ways: 1. Automatically: int m[2][3]; // 2](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-9.jpg)
Multi-dimensional arrays Can be created in few ways: 1. Automatically: int m[2][3]; // 2 rows, 3 columns �continuous memory (divided to 2 blocks) �access: m[row][col] = 0; 9
![Automatic multidimensional arrays define R 2 define C 3 int mRC int mptr m00 Automatic multi-dimensional arrays #define R 2 #define C 3 int m[R][C]; int* m_ptr= &(m[0][0]);](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-10.jpg)
Automatic multi-dimensional arrays #define R 2 #define C 3 int m[R][C]; int* m_ptr= &(m[0][0]); size_t i; for (i=0; i<R*C; ++i) { printf(“%dn”, *(m_ptr++) ); } 10
![Automatic multidimensional arrays define R 2 define C 3 int mRC int mptr for Automatic multi-dimensional arrays #define R 2 #define C 3 int m[R][C]; int* m_ptr; for](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-11.jpg)
Automatic multi-dimensional arrays #define R 2 #define C 3 int m[R][C]; int* m_ptr; for (m_ptr= &(m[0][0]); m_ptr<=&(m[R-1][C-1]); ++m_ptr) { printf(“%dn”, *m_ptr ); 11 }
![Automatic multidimensional arrays int ARC Like a matrix A00 A01 A0c1 Automatic multi-dimensional arrays int A[R][C]; Like a matrix A[0][0] A[0][1] … A[0][c-1] … …](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-12.jpg)
Automatic multi-dimensional arrays int A[R][C]; Like a matrix A[0][0] A[0][1] … A[0][c-1] … … A[i][0] A[i][1] … A[i][c-1] … … A[R-1][0] A[R-1][1] … A[R-1][C-1] Row-major ordering A[0] A 12 A[i] A A • • • [0] • • • [i] [C-1] [0] A[R-1] A A A • • • [i] • • • [R-1] [C-1] [0] [C-1] A+i*C*4 Starting address of A[i] A+(R-1)*C*4
![Semidynamic multidimensional arrays 2 Semidynamic Define an array of pointers int m5 allocates Semi-dynamic multi-dimensional arrays 2. Semi-dynamic: Define an array of pointers: int* m[5]; // allocates](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-13.jpg)
Semi-dynamic multi-dimensional arrays 2. Semi-dynamic: Define an array of pointers: int* m[5]; // allocates memory for 5 pointers for (i=0; i<5; ++i) { m[i] = (int*) malloc( 7*sizeof(int) ); // m[i] now points to a memory for 7 ints } 13
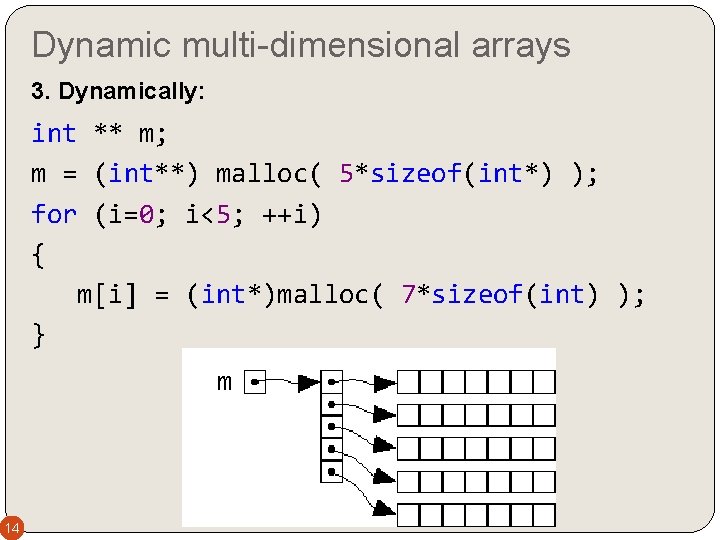
Dynamic multi-dimensional arrays 3. Dynamically: int ** m; m = (int**) malloc( 5*sizeof(int*) ); for (i=0; i<5; ++i) { m[i] = (int*)malloc( 7*sizeof(int) ); } m 14
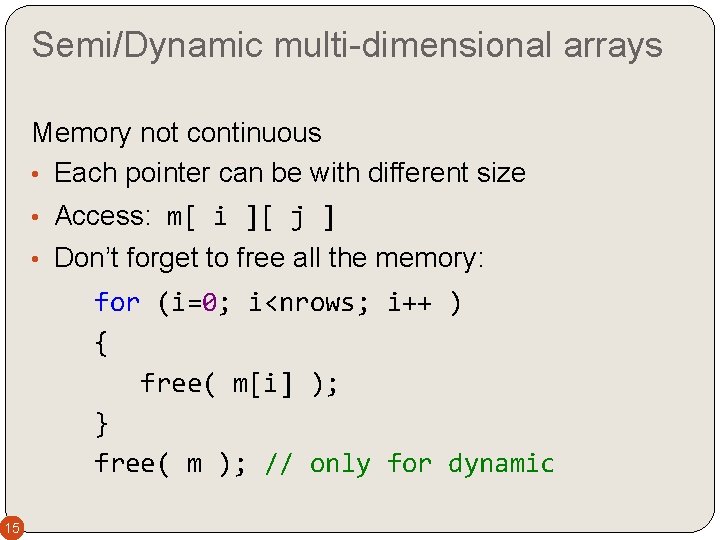
Semi/Dynamic multi-dimensional arrays Memory not continuous • Each pointer can be with different size • Access: m[ i ][ j ] • Don’t forget to free all the memory: for (i=0; i<nrows; i++ ) { free( m[i] ); } free( m ); // only for dynamic 15
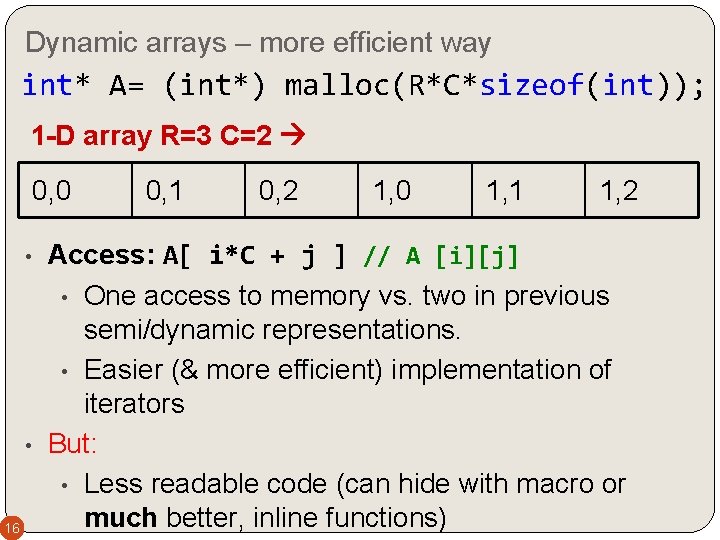
Dynamic arrays – more efficient way int* A= (int*) malloc(R*C*sizeof(int)); 1 -D array R=3 C=2 0, 0 • • 16 0, 1 0, 2 1, 0 1, 1 1, 2 Access: A[ i*C + j ] // A [i][j] • One access to memory vs. two in previous semi/dynamic representations. • Easier (& more efficient) implementation of iterators But: • Less readable code (can hide with macro or much better, inline functions)
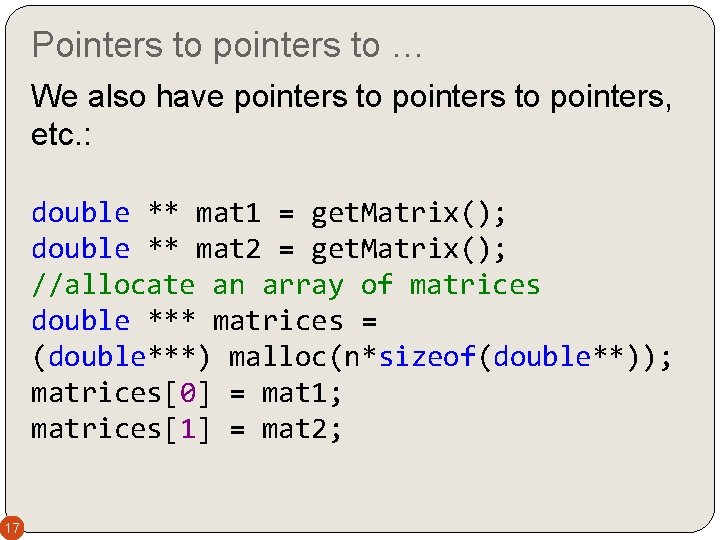
Pointers to pointers to … We also have pointers to pointers, etc. : double ** mat 1 = get. Matrix(); double ** mat 2 = get. Matrix(); //allocate an array of matrices double *** matrices = (double***) malloc(n*sizeof(double**)); matrices[0] = mat 1; matrices[1] = mat 2; 17
![Automatic multidimensional arrays as arguments to functions int x57 5 rows 7 columns Automatic multi-dimensional arrays as arguments to functions int x[5][7]; // 5 rows, 7 columns](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-18.jpg)
Automatic multi-dimensional arrays as arguments to functions int x[5][7]; // 5 rows, 7 columns When sending ‘x’ as an argument to a function, only the 1 st index can be omitted: • void func( int x[5][7] ) //ok • void func( int x[][] ) //does not compile • void func( int * x[] ) //something else • void func( int ** x ) //something else 18
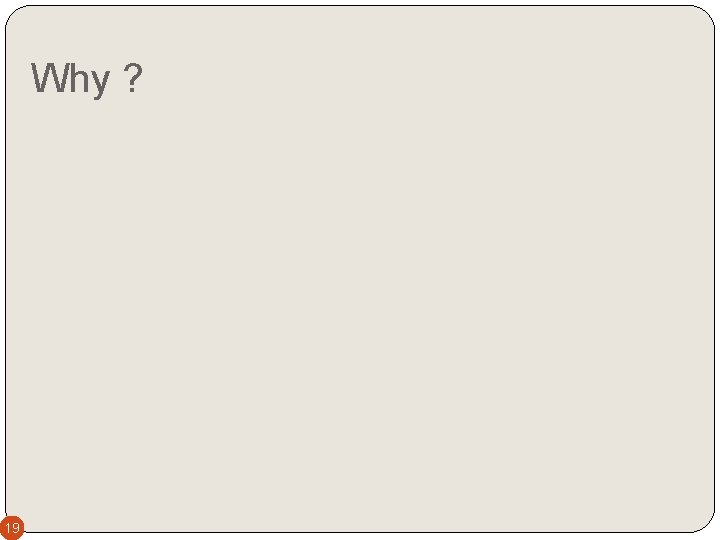
Why ? 19
![Pointers to arrays of size X int foo char m20 m is a pointer Pointers to arrays (of size X): int foo (char m[][20]); m is a pointer](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-20.jpg)
Pointers to arrays (of size X): int foo (char m[][20]); m is a pointer to an array of 20 chars. Therefore: sizeof (m) = sizeof (void*); sizeof (*m) = 20*sizeof (char); 20
![Pointers to arrays of size X Explicit declaration char arr2 d20 arr2 d Pointers to arrays (of size X): Explicit declaration: char (*arr_2 d)[20]; // arr_2 d](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-21.jpg)
Pointers to arrays (of size X): Explicit declaration: char (*arr_2 d)[20]; // arr_2 d is a pointer // not initialized Using typedef: typdef char arr_2 d_20[20]; arr_2 d_20 *p_arr_2 d; But: typdef char arr_2 d[][]; // comp. error 21
![Pointers to arrays of size X char m5 m 22 char m5 Pointers to arrays (of size X): char (*m)[5]; m 22 char *m[5];](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-22.jpg)
Pointers to arrays (of size X): char (*m)[5]; m 22 char *m[5];
![Pointers to arrays of size X char m5 m After initialization char arr5 m Pointers to arrays (of size X): char (*m)[5]; m After initialization: char arr[5]; m=](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-23.jpg)
Pointers to arrays (of size X): char (*m)[5]; m After initialization: char arr[5]; m= &arr; arr 23 char *m[5];
![Pointers to arrays of size X char m5 sizeof m sizeofvoid sizeof m Pointers to arrays (of size X): char (*m)[5]; sizeof (m) = sizeof(void*) sizeof (*m)](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-24.jpg)
Pointers to arrays (of size X): char (*m)[5]; sizeof (m) = sizeof(void*) sizeof (*m) = 5*sizeof(char) 24 char *m[5]; sizeof (m) = 5*sizeof (char*) = 5*sizeof (void*) sizeof (*m) = sizeof (char*)= sizeof (void*)
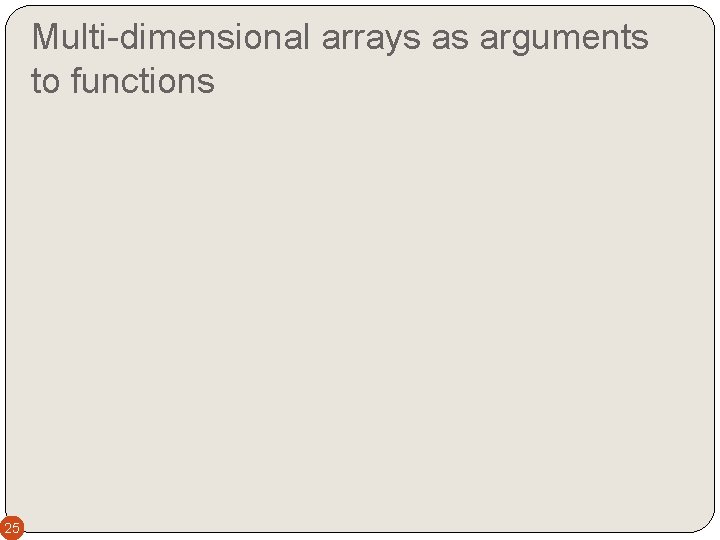
Multi-dimensional arrays as arguments to functions 25
![Multidimensional arrays as arguments to functions void foo int matrixROWSNUMCOLSNUM 26 Multi-dimensional arrays as arguments to functions void foo( int matrix[ROWS_NUM][COLS_NUM] ) { 26](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-26.jpg)
Multi-dimensional arrays as arguments to functions void foo( int matrix[ROWS_NUM][COLS_NUM] ) { 26
![Multidimensional arrays as arguments to functions void foo int matrixROWSNUMCOLSNUM ROWSNUM What is Multi-dimensional arrays as arguments to functions void foo( int matrix[ROWS_NUM][COLS_NUM] ){ [ROWS_NUM] What is](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-27.jpg)
Multi-dimensional arrays as arguments to functions void foo( int matrix[ROWS_NUM][COLS_NUM] ){ [ROWS_NUM] What is really sent? 27
![Multidimensional arrays as arguments to functions void foo int matrix COLSNUM Multi-dimensional arrays as arguments to functions void foo( int (*matrix )[COLS_NUM] ) { (*](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-28.jpg)
Multi-dimensional arrays as arguments to functions void foo( int (*matrix )[COLS_NUM] ) { (* pointer to an array of COLS_NUM ints! 28
![Multidimensional arrays as arguments to functions void foo int matrixCOLSNUM Multi-dimensional arrays as arguments to functions void foo( int (*matrix)[COLS_NUM] ) {. . .](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-29.jpg)
Multi-dimensional arrays as arguments to functions void foo( int (*matrix)[COLS_NUM] ) {. . . 29 matrix[r][c]
![Multidimensional arrays as arguments to functions void foo int matrixCOLSNUM 30 Multi-dimensional arrays as arguments to functions void foo( int (*matrix)[COLS_NUM] ) {. . 30](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-30.jpg)
Multi-dimensional arrays as arguments to functions void foo( int (*matrix)[COLS_NUM] ) {. . 30 matrix[r][c] (*(matrix+r))[c]
![Multidimensional arrays as arguments to functions void foo int matrixCOLSNUM matrixrc Multi-dimensional arrays as arguments to functions void foo( int (*matrix)[COLS_NUM] ) {. . matrix[r][c]](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-31.jpg)
Multi-dimensional arrays as arguments to functions void foo( int (*matrix)[COLS_NUM] ) {. . matrix[r][c] (*(matrix+r))[c] matrix is a pointer to int[COLS_NUM]. addition is done in this units. This is why COLS_NUM is needed! 31
![Multidimensional arrays as arguments to functions void foo int matrixCOLSNUM Multi-dimensional arrays as arguments to functions void foo( int (*matrix)[COLS_NUM] ) {. . .](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-32.jpg)
Multi-dimensional arrays as arguments to functions void foo( int (*matrix)[COLS_NUM] ) {. . . matrix[r][c]. . . (*(matrix+r))[c]. . . *((*(matrix+r)) + c) 32
![Multidimensional arrays as arguments to functions void foo int matrixCOLSNUM Multi-dimensional arrays as arguments to functions void foo( int (*matrix)[COLS_NUM] ) {. . .](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-33.jpg)
Multi-dimensional arrays as arguments to functions void foo( int (*matrix)[COLS_NUM] ) {. . . matrix[r][c]. . . (*(matrix+r))[c]. . . *((*(matrix+r)) + c) == *(matrix+r*COLS_NUM+c) The compiler is probably optimizing the internal computation to this. 33
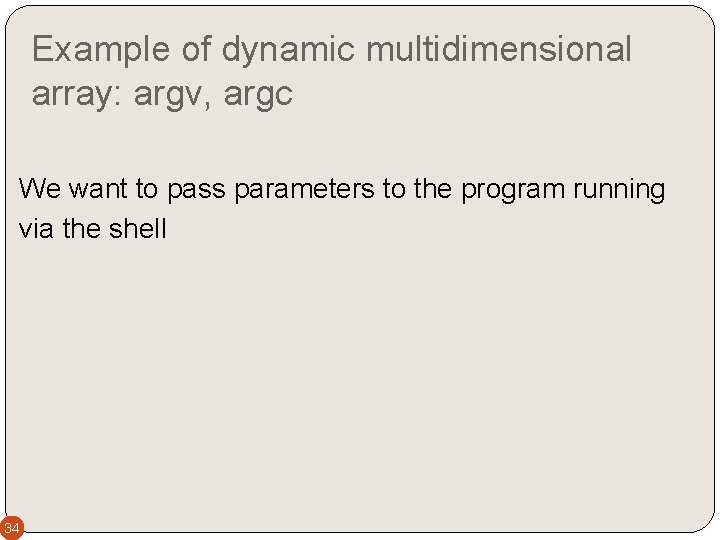
Example of dynamic multidimensional array: argv, argc We want to pass parameters to the program running via the shell 34
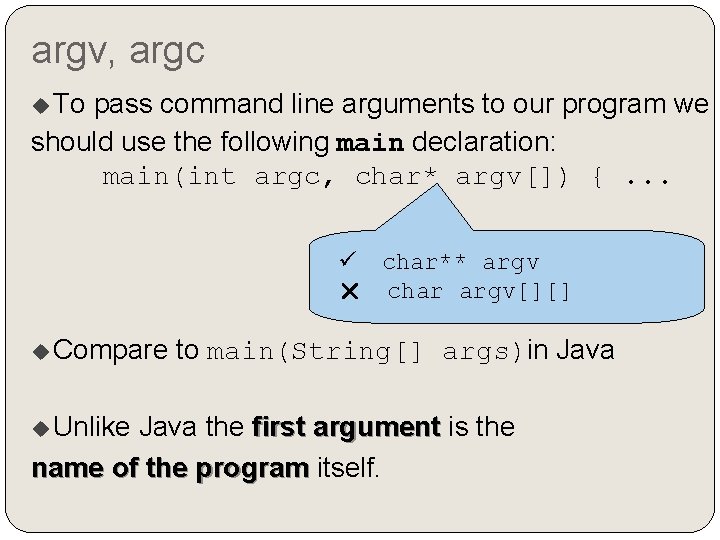
argv, argc To pass command line arguments to our program we should use the following main declaration: main(int argc, char* argv[]) {. . . char** argv char argv[][] Compare Unlike to main(String[] args)in Java the first argument is the name of the program itself.
![argv argc example prog 1 u danny p 1234 argc argv0 argv & argc: example $ prog 1 –u danny –p 1234 argc == argv[0]](https://slidetodoc.com/presentation_image_h/7794408fde5b24d94cf1b04931032720/image-36.jpg)
argv & argc: example $ prog 1 –u danny –p 1234 argc == argv[0] argv[1]. . . argv[4] 5 == “prog 1” == “-u” == “ 1234” Always: argv[argc] == NULL (redundant since we are also given argc)