Counting Sort A sort algorithm that is not
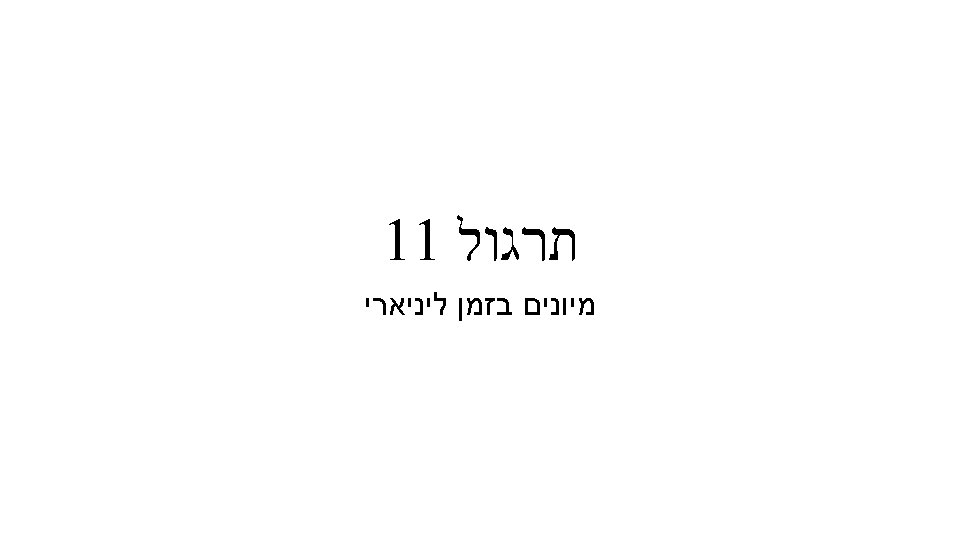
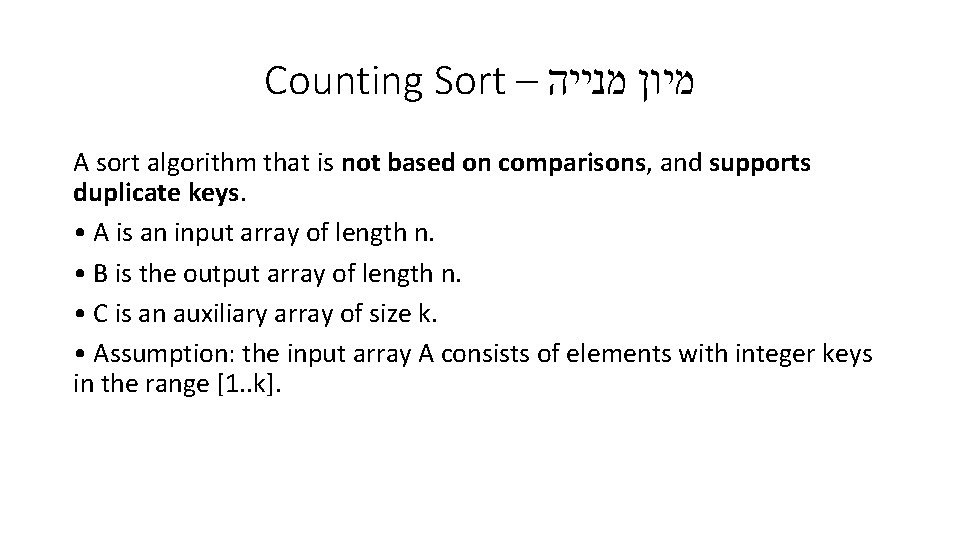
![Counting Sort for i ← 1 to k C[i] ← 0 for j ← Counting Sort for i ← 1 to k C[i] ← 0 for j ←](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-3.jpg)
![Counting Sort for i ← 1 to k C[i] ← 0 A 3 2 Counting Sort for i ← 1 to k C[i] ← 0 A 3 2](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-4.jpg)
![Counting Sort for j ← 1 to n C[A[j]] ← C[A[j]] + 1 // Counting Sort for j ← 1 to n C[A[j]] ← C[A[j]] + 1 //](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-5.jpg)
![Counting Sort for i ← 2 to k C[i] ← C[i] + C[i-1] // Counting Sort for i ← 2 to k C[i] ← C[i] + C[i-1] //](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-6.jpg)
![Counting Sort for i ← 2 to k C[i] ← C[i] + C[i-1] // Counting Sort for i ← 2 to k C[i] ← C[i] + C[i-1] //](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-7.jpg)
![Counting Sort for i ← 2 to k C[i] ← C[i] + C[i-1] // Counting Sort for i ← 2 to k C[i] ← C[i] + C[i-1] //](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-8.jpg)
![Counting Sort for i ← 2 to k C[i] ← C[i] + C[i-1] // Counting Sort for i ← 2 to k C[i] ← C[i] + C[i-1] //](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-9.jpg)
![Counting Sort for i ← 2 to k C[i] ← C[i] + C[i-1] // Counting Sort for i ← 2 to k C[i] ← C[i] + C[i-1] //](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-10.jpg)
![Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]] Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]]](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-11.jpg)
![Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]] Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]]](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-12.jpg)
![Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]] Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]]](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-13.jpg)
![Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]] Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]]](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-14.jpg)
![Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]] Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]]](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-15.jpg)
![Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]] Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]]](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-16.jpg)
![Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]] Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]]](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-17.jpg)
![Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]] Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]]](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-18.jpg)
![Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]] Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]]](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-19.jpg)
![Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]] Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]]](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-20.jpg)
![Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]] Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]]](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-21.jpg)
![Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]] Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]]](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-22.jpg)
![Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]] Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]]](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-23.jpg)
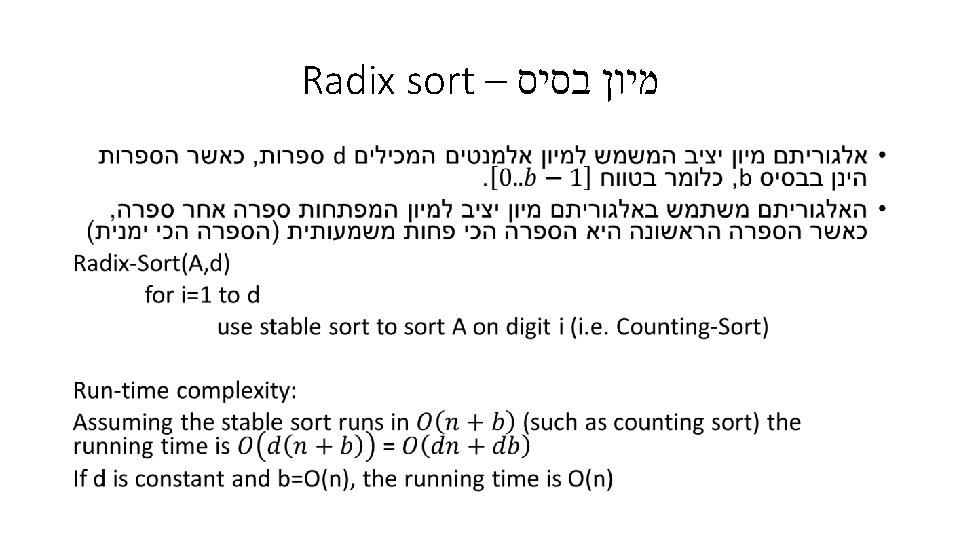
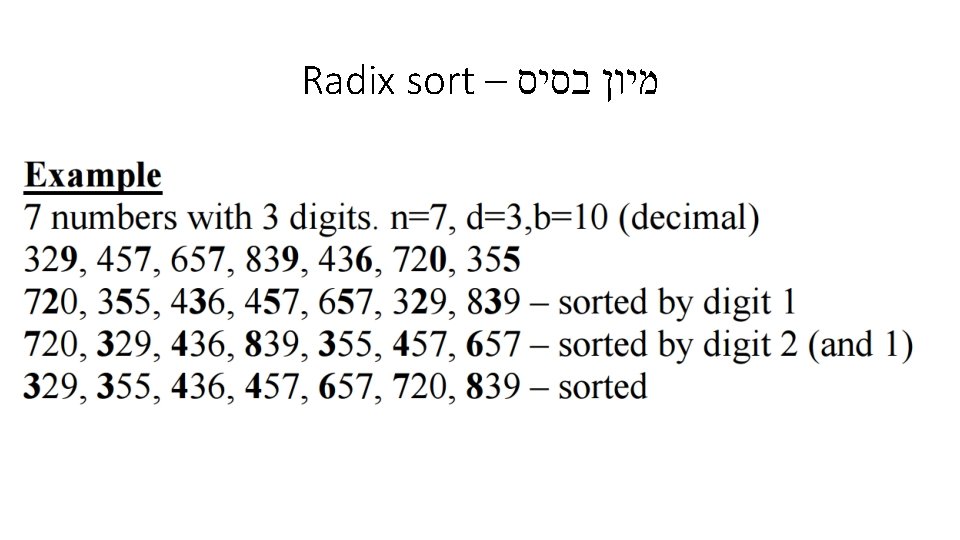
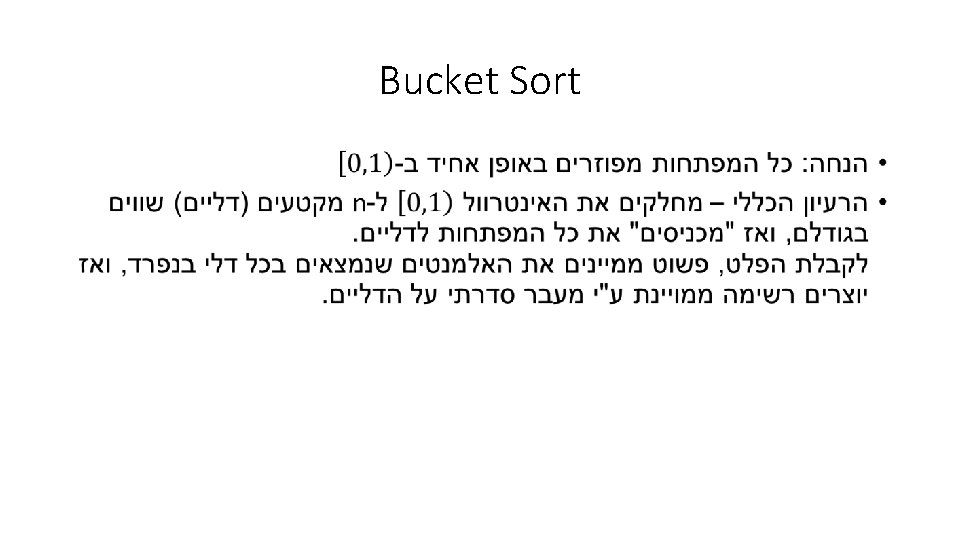
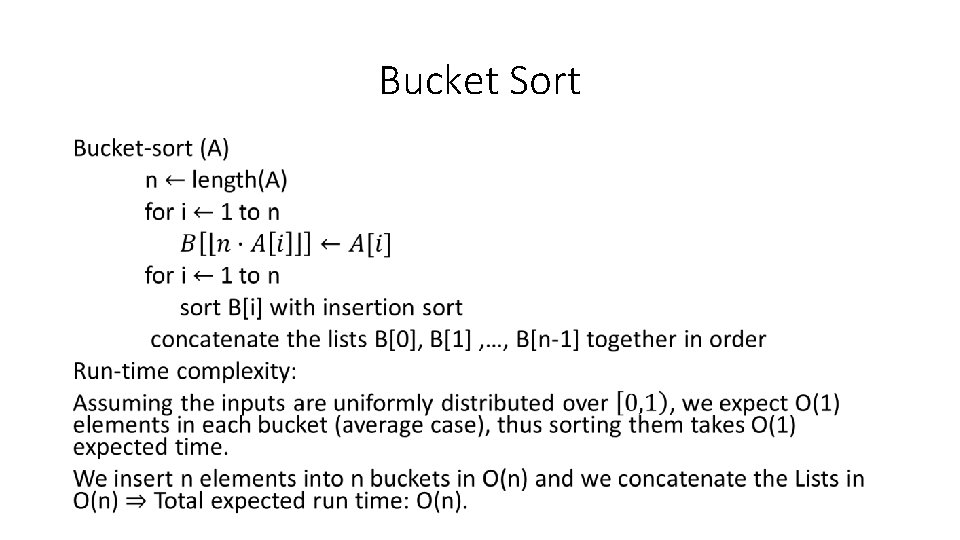
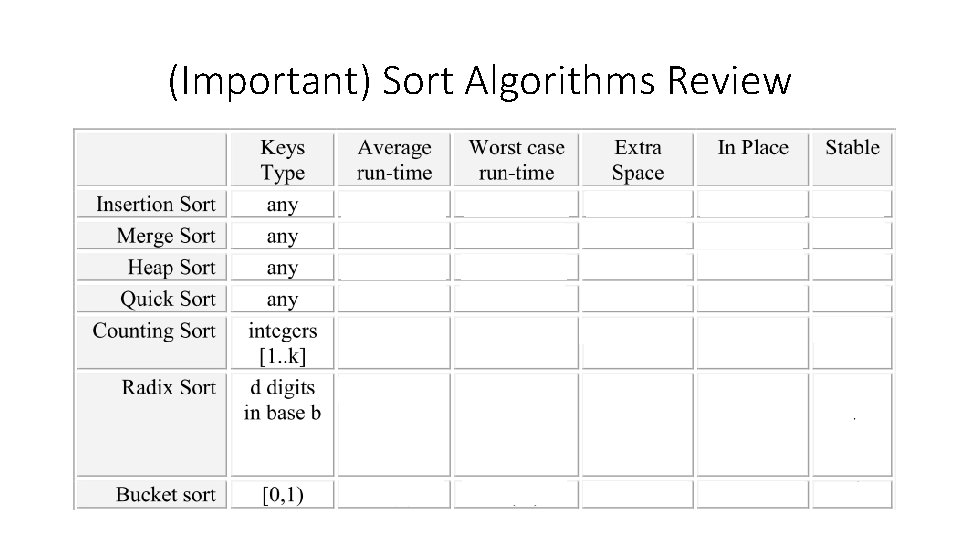
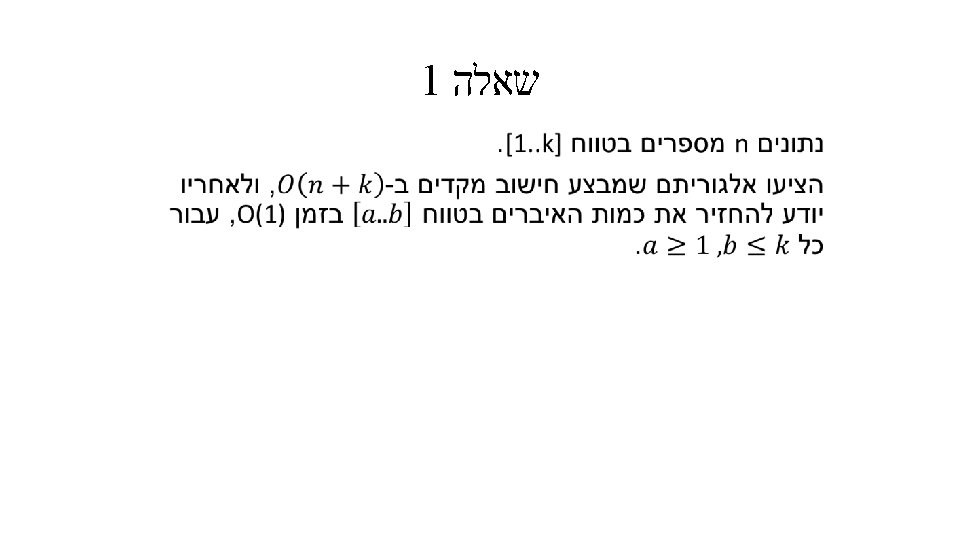
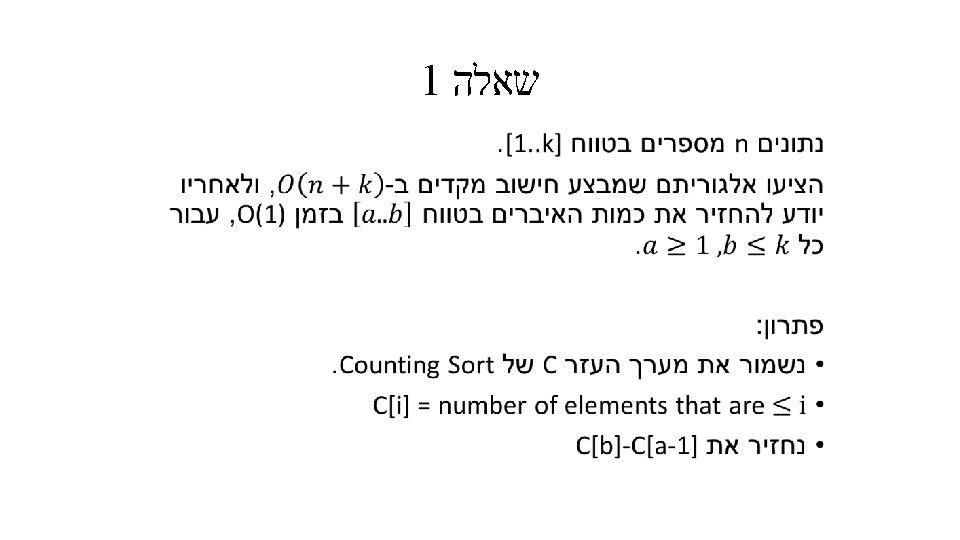
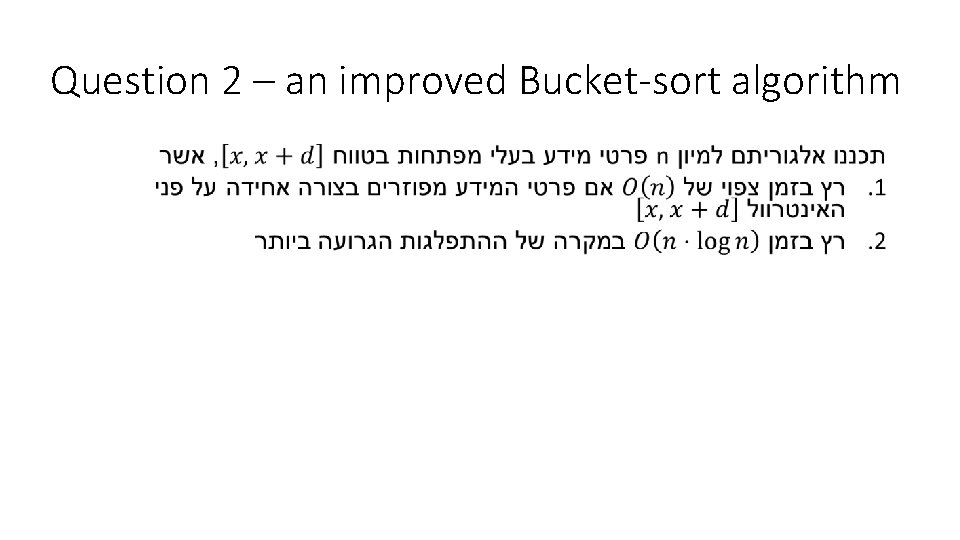
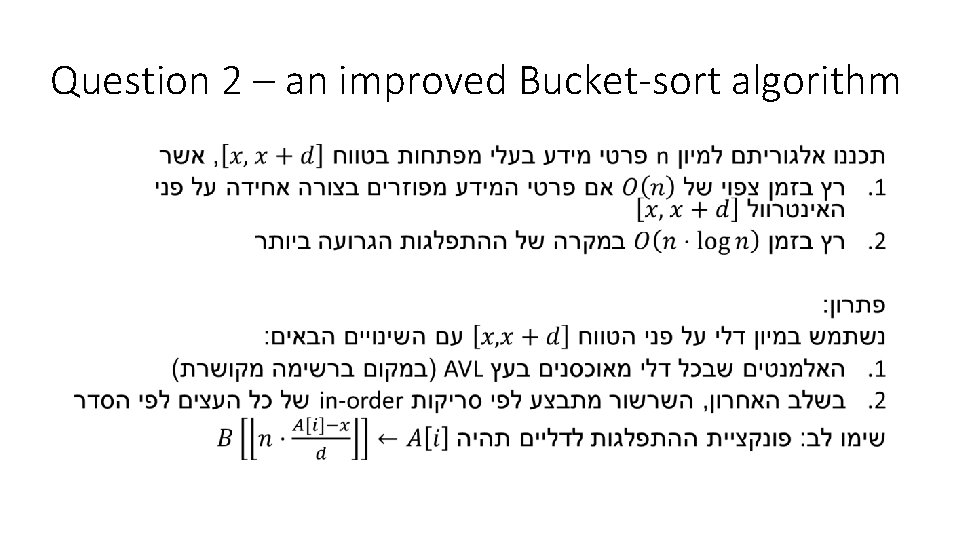
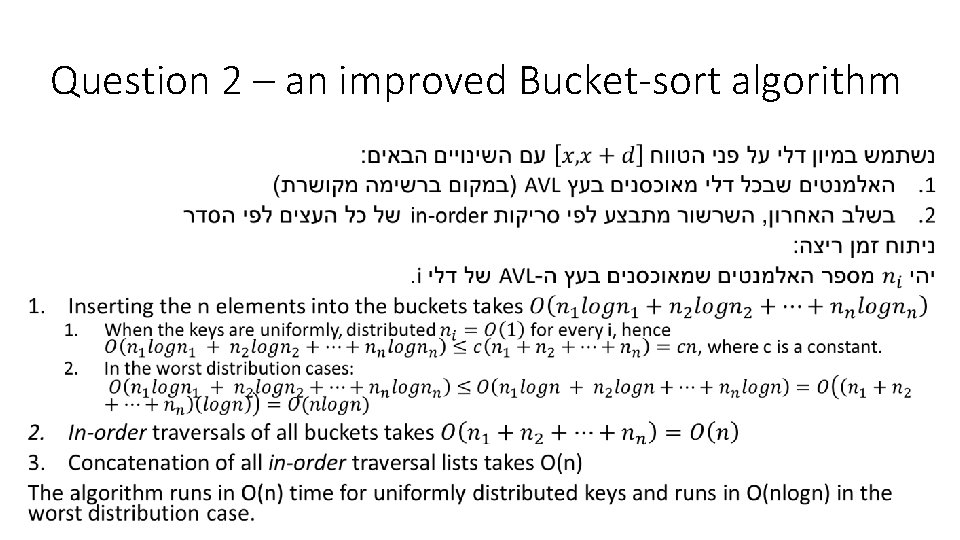
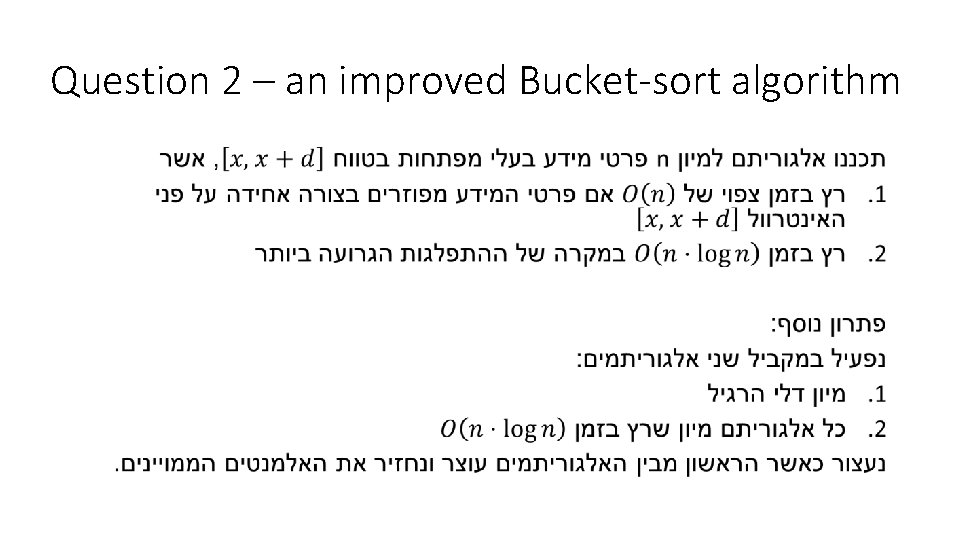
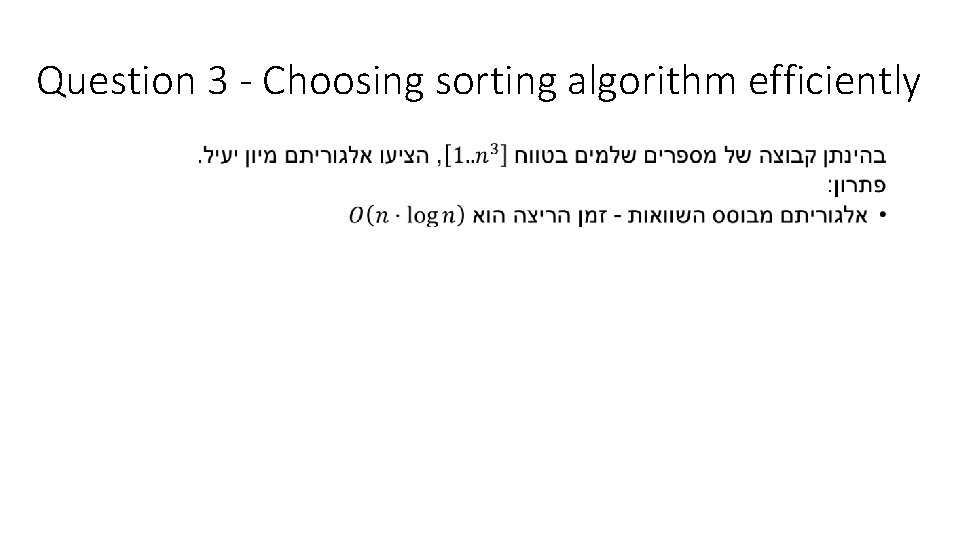
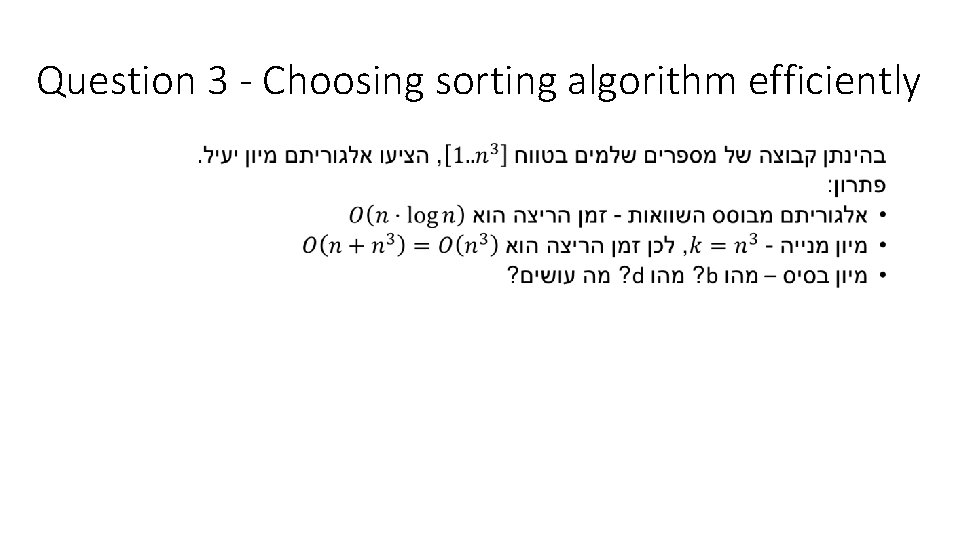
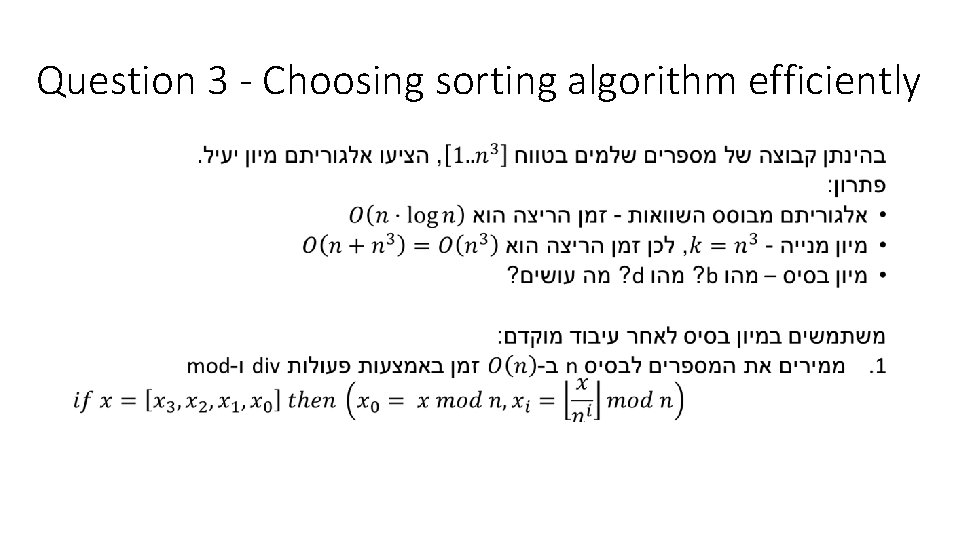
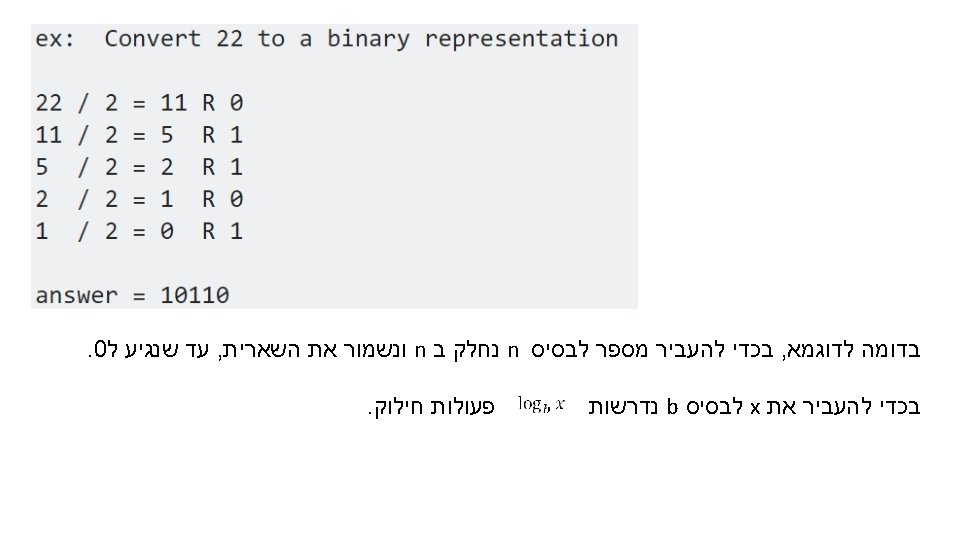
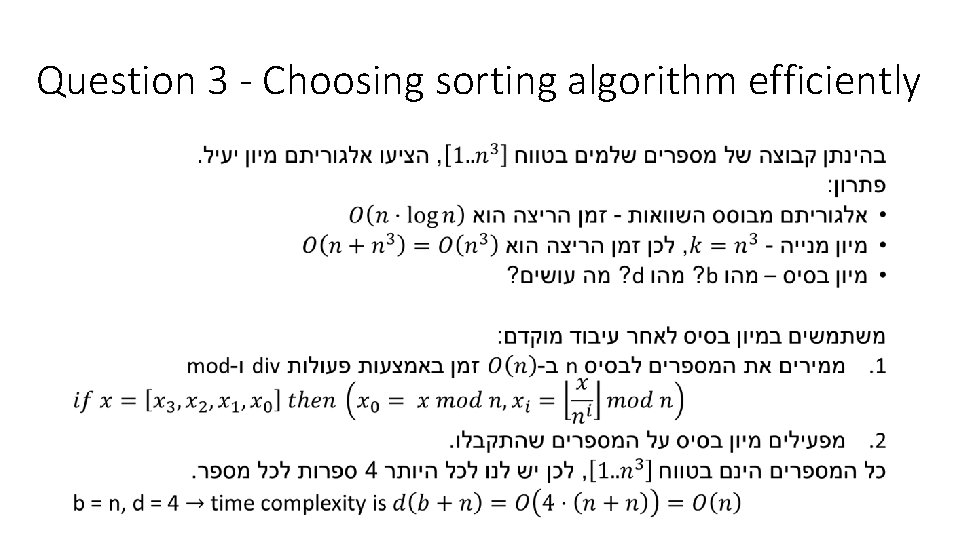
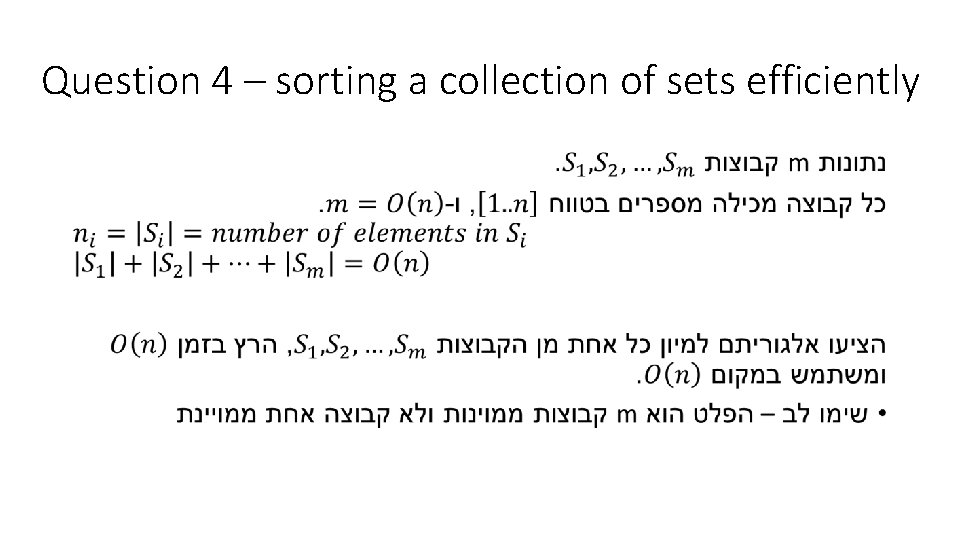
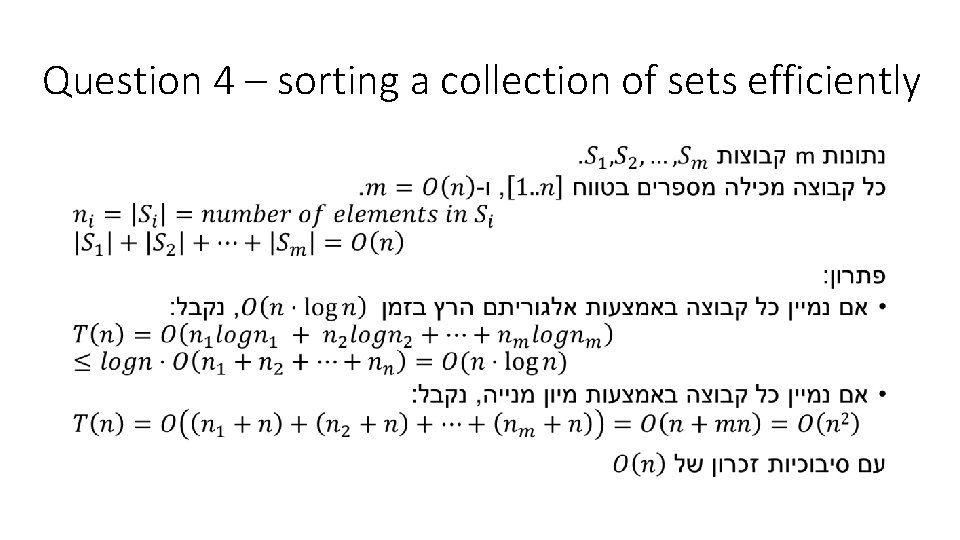
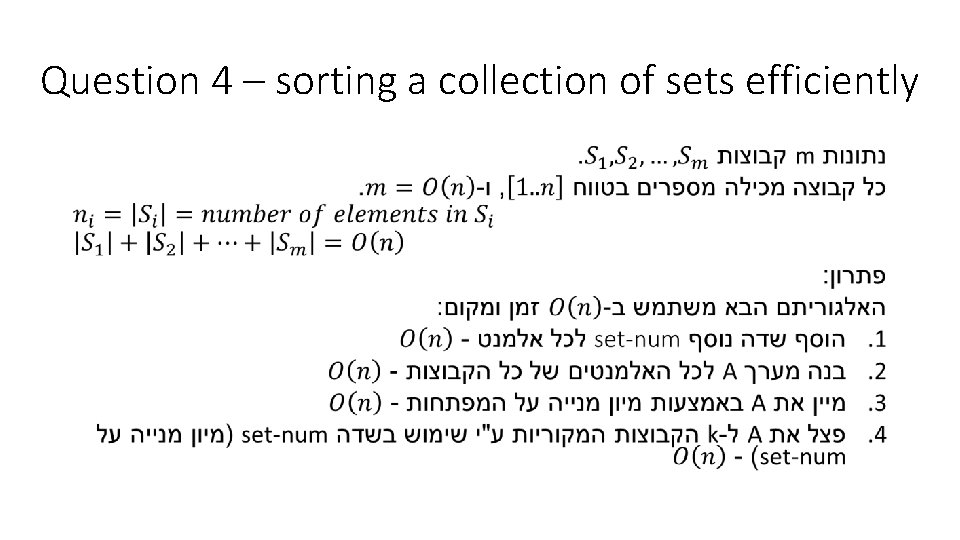
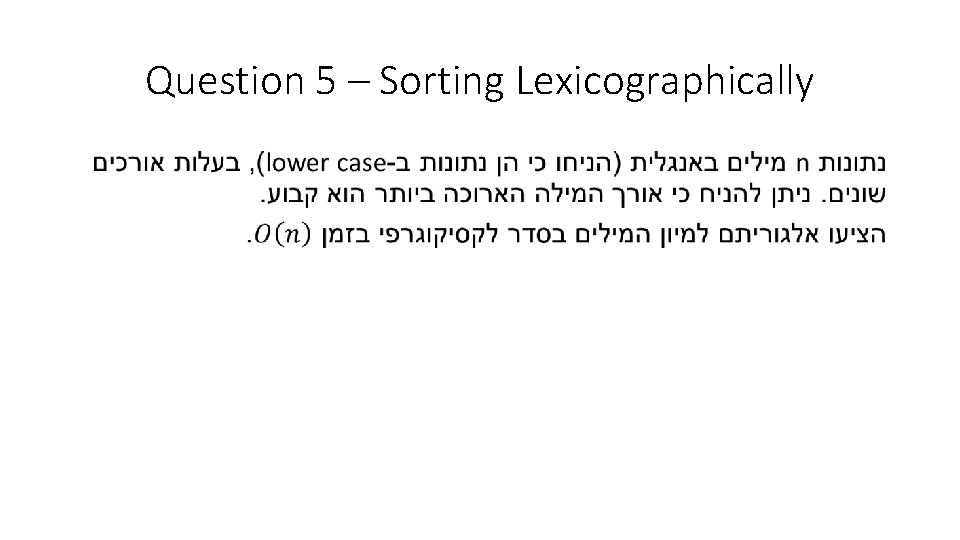
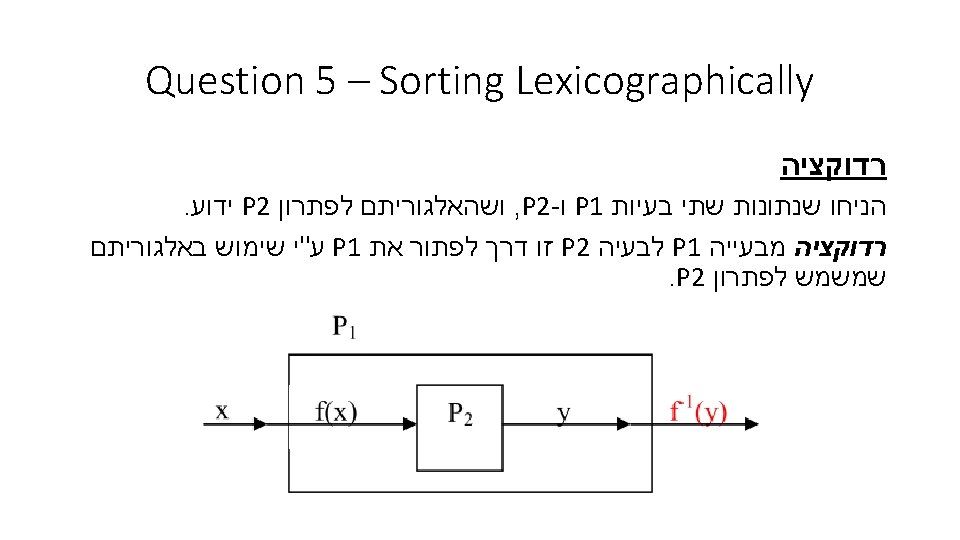
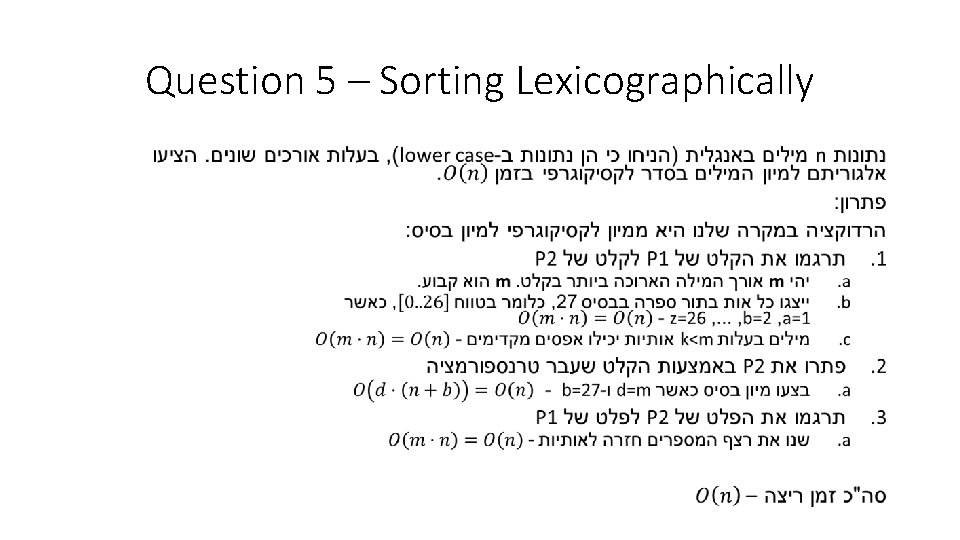
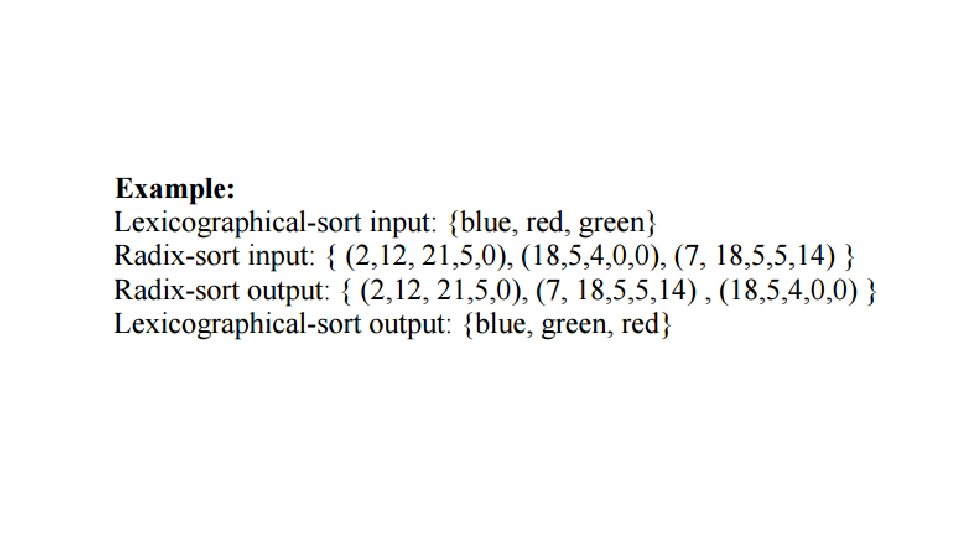
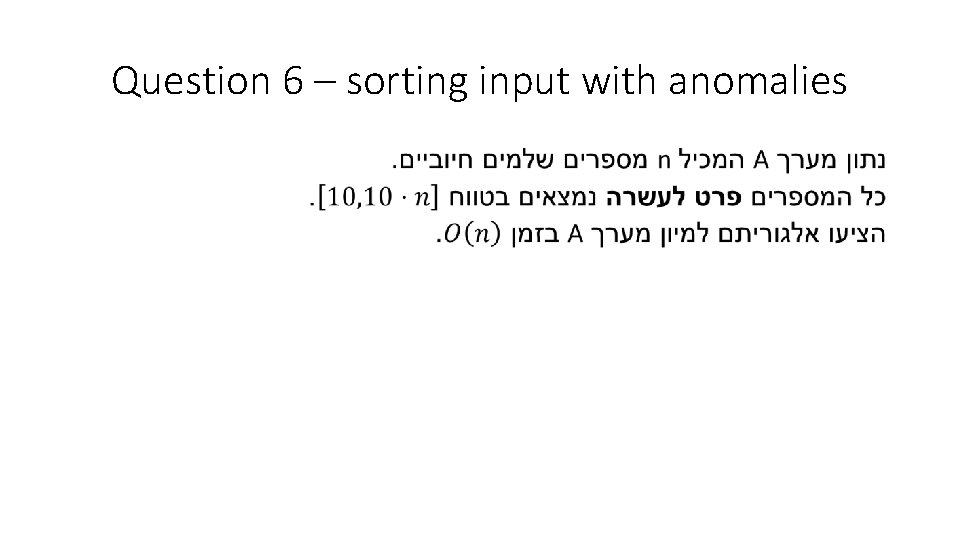
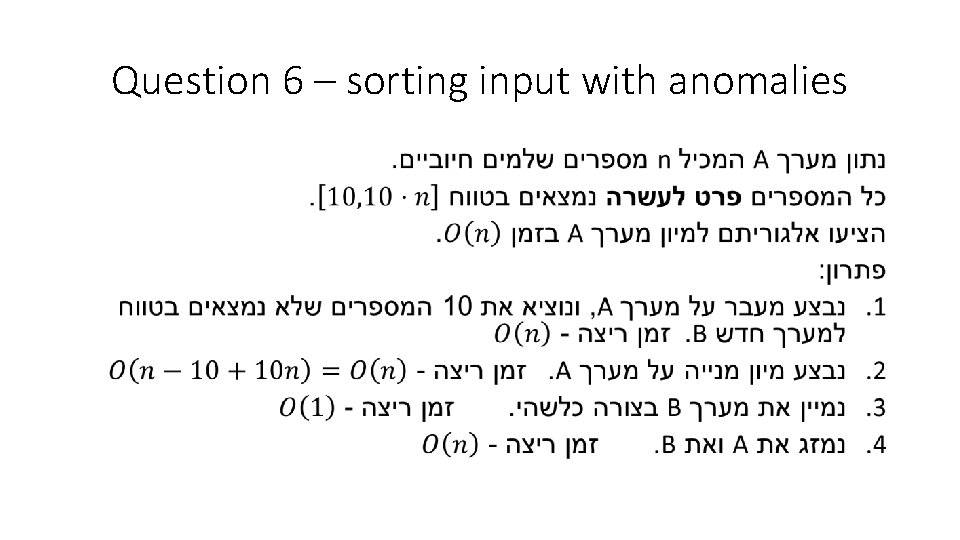
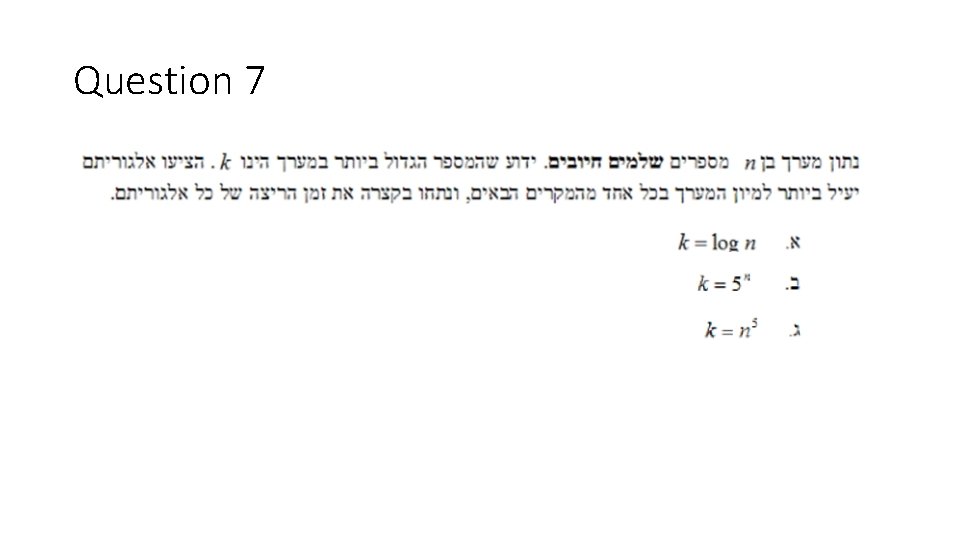
- Slides: 49
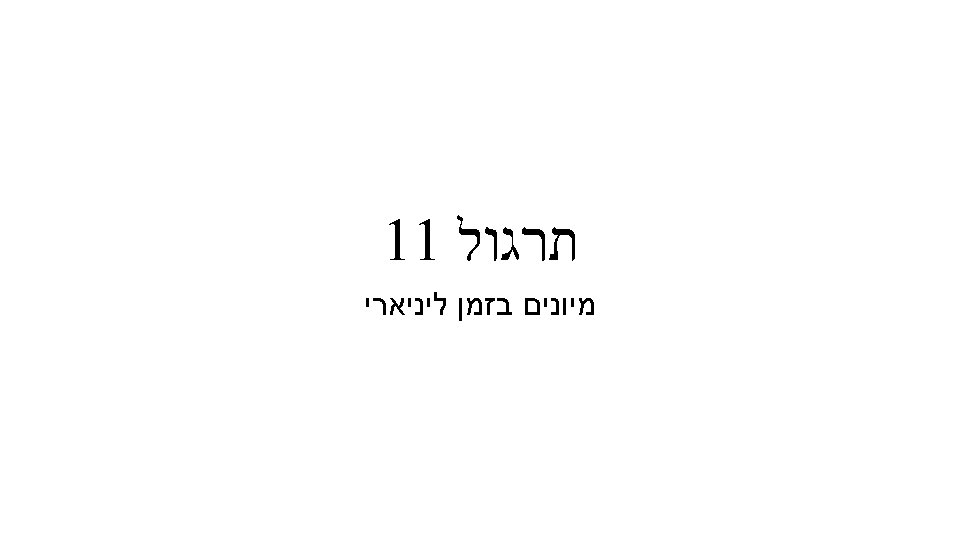
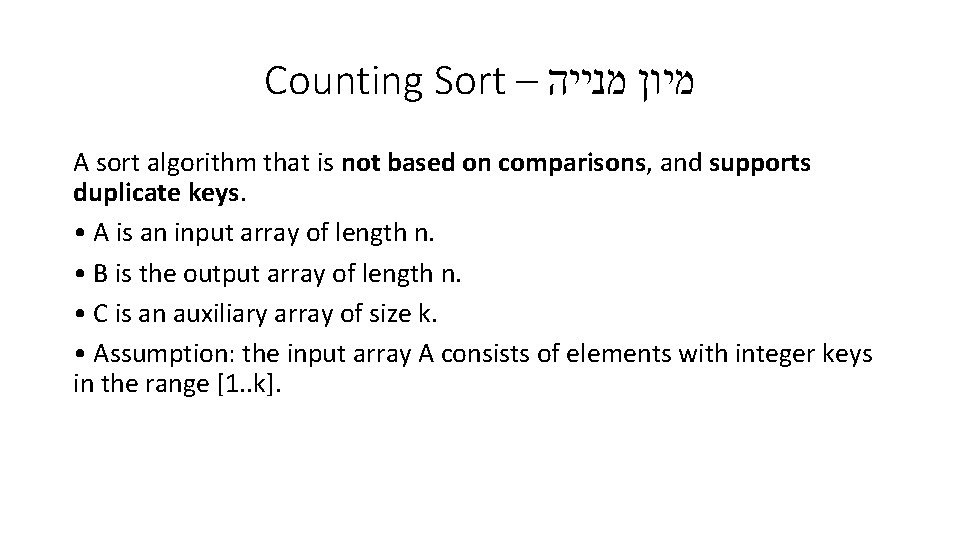
Counting Sort – מיון מנייה A sort algorithm that is not based on comparisons, and supports duplicate keys. • A is an input array of length n. • B is the output array of length n. • C is an auxiliary array of size k. • Assumption: the input array A consists of elements with integer keys in the range [1. . k].
![Counting Sort for i 1 to k Ci 0 for j Counting Sort for i ← 1 to k C[i] ← 0 for j ←](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-3.jpg)
Counting Sort for i ← 1 to k C[i] ← 0 for j ← 1 to n C[A[j]] ← C[A[j]] + 1 for i ← 2 to k C[i] ← C[i] + C[i-1] for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]] - 1 return B // Initialize auxiliary array C // C[i] = the number of appearances of i in A // C[i] = the number of elements in A that are ≤ i // Putting elements in their correct place in array B // Decreasing the amount of elements A[j] left
![Counting Sort for i 1 to k Ci 0 A 3 2 Counting Sort for i ← 1 to k C[i] ← 0 A 3 2](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-4.jpg)
Counting Sort for i ← 1 to k C[i] ← 0 A 3 2 3 C 0 0 0 1 3 1
![Counting Sort for j 1 to n CAj CAj 1 Counting Sort for j ← 1 to n C[A[j]] ← C[A[j]] + 1 //](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-5.jpg)
Counting Sort for j ← 1 to n C[A[j]] ← C[A[j]] + 1 // C[i] = the number of appearances of i in A A 3 2 3 C 2 1 3 1
![Counting Sort for i 2 to k Ci Ci Ci1 Counting Sort for i ← 2 to k C[i] ← C[i] + C[i-1] //](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-6.jpg)
Counting Sort for i ← 2 to k C[i] ← C[i] + C[i-1] // C[i] = the number of elements in A that are ≤ i A 3 2 3 C 2 1 3 1
![Counting Sort for i 2 to k Ci Ci Ci1 Counting Sort for i ← 2 to k C[i] ← C[i] + C[i-1] //](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-7.jpg)
Counting Sort for i ← 2 to k C[i] ← C[i] + C[i-1] // C[i] = the number of elements in A that are ≤ i A 3 2 3 C 2 1 3 i=2 1 3 1
![Counting Sort for i 2 to k Ci Ci Ci1 Counting Sort for i ← 2 to k C[i] ← C[i] + C[i-1] //](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-8.jpg)
Counting Sort for i ← 2 to k C[i] ← C[i] + C[i-1] // C[i] = the number of elements in A that are ≤ i A 3 2 3 C 2 3 3 i=2 1 3 1
![Counting Sort for i 2 to k Ci Ci Ci1 Counting Sort for i ← 2 to k C[i] ← C[i] + C[i-1] //](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-9.jpg)
Counting Sort for i ← 2 to k C[i] ← C[i] + C[i-1] // C[i] = the number of elements in A that are ≤ i A 3 2 3 C 2 3 3 i=3 1
![Counting Sort for i 2 to k Ci Ci Ci1 Counting Sort for i ← 2 to k C[i] ← C[i] + C[i-1] //](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-10.jpg)
Counting Sort for i ← 2 to k C[i] ← C[i] + C[i-1] // C[i] = the number of elements in A that are ≤ i A 3 2 3 C 2 3 6 i=3 1
![Counting Sort for j n downto 1 BCAj Aj CAj CAj Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]]](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-11.jpg)
Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]] – 1 A 3 2 3 C 2 3 6 B 1 3 1
![Counting Sort for j n downto 1 BCAj Aj CAj CAj Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]]](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-12.jpg)
Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]] – 1 j=6, A[6]=1, C[1]=2 A 3 2 3 C 2 3 6 B 1 3 1
![Counting Sort for j n downto 1 BCAj Aj CAj CAj Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]]](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-13.jpg)
Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]] – 1 j=6, A[6]=1, C[1]=2 A 3 2 3 C 1 3 6 B 1 1 3 1
![Counting Sort for j n downto 1 BCAj Aj CAj CAj Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]]](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-14.jpg)
Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]] – 1 j=5, A[5]=3, C[3]=6 A 3 2 3 C 1 3 6 B 1 1 3 1
![Counting Sort for j n downto 1 BCAj Aj CAj CAj Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]]](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-15.jpg)
Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]] – 1 j=5, A[5]=3, C[3]=6 A 3 2 3 C 1 3 5 B 1 1 3
![Counting Sort for j n downto 1 BCAj Aj CAj CAj Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]]](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-16.jpg)
Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]] – 1 j=4, A[4]=1, C[1]=1 A 3 2 3 C 1 3 5 B 1 1 3
![Counting Sort for j n downto 1 BCAj Aj CAj CAj Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]]](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-17.jpg)
Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]] – 1 j=4, A[4]=1, C[1]=1 A 3 2 3 C 0 3 5 B 1 1 1 3
![Counting Sort for j n downto 1 BCAj Aj CAj CAj Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]]](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-18.jpg)
Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]] – 1 j=3, A[3]=3, C[3]=5 A 3 2 3 C 0 3 5 B 1 1 1 3
![Counting Sort for j n downto 1 BCAj Aj CAj CAj Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]]](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-19.jpg)
Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]] – 1 j=3, A[3]=3, C[3]=5 A 3 2 3 C 0 3 4 B 1 1 1 3 3
![Counting Sort for j n downto 1 BCAj Aj CAj CAj Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]]](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-20.jpg)
Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]] – 1 j=2, A[2]=2, C[2]=3 A 3 2 3 C 0 3 4 B 1 1 1 3 3
![Counting Sort for j n downto 1 BCAj Aj CAj CAj Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]]](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-21.jpg)
Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]] – 1 j=2, A[2]=2, C[2]=3 A 3 2 3 C 0 2 4 B 1 1 2 1 3 3
![Counting Sort for j n downto 1 BCAj Aj CAj CAj Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]]](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-22.jpg)
Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]] – 1 j=1, A[1]=3, C[3]=4 A 3 2 3 C 0 2 4 B 1 1 2 1 3 3
![Counting Sort for j n downto 1 BCAj Aj CAj CAj Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]]](https://slidetodoc.com/presentation_image/57a890b2bcbe2dd350a623e36f77e583/image-23.jpg)
Counting Sort for j ← n downto 1 B[C[A[j]]] ← A[j] C[A[j]] ← C[A[j]] – 1 j=1, A[1]=3, C[3]=4 A 3 2 3 C 0 2 3 B 1 1 2 1 3 3 3
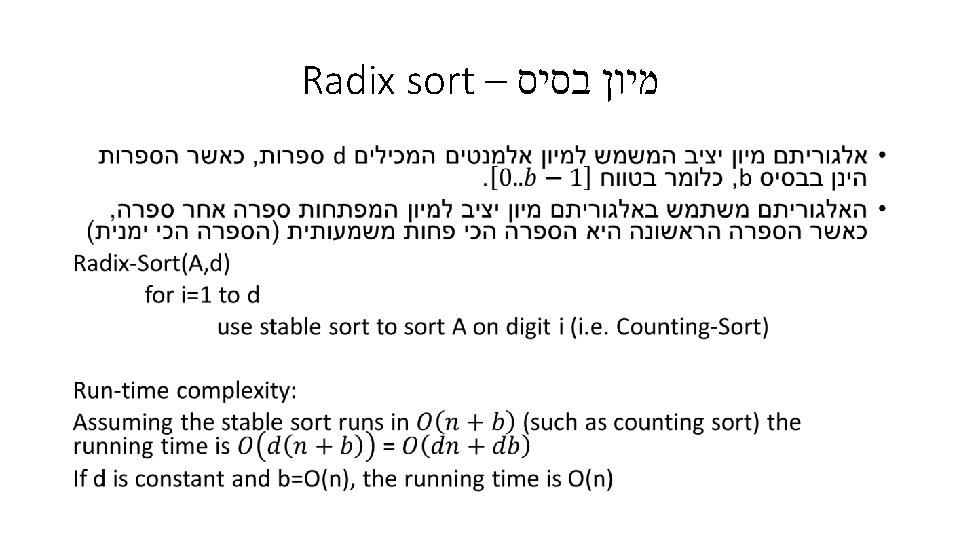
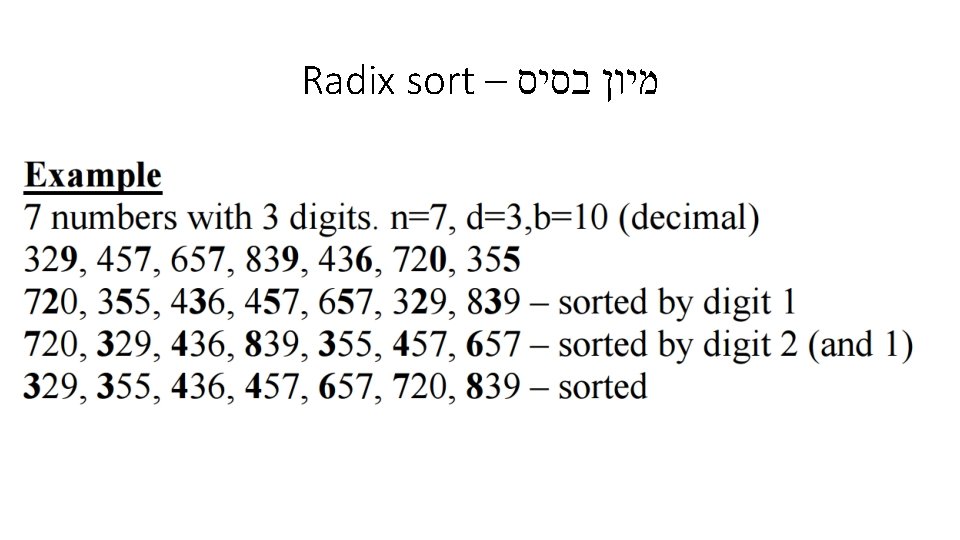
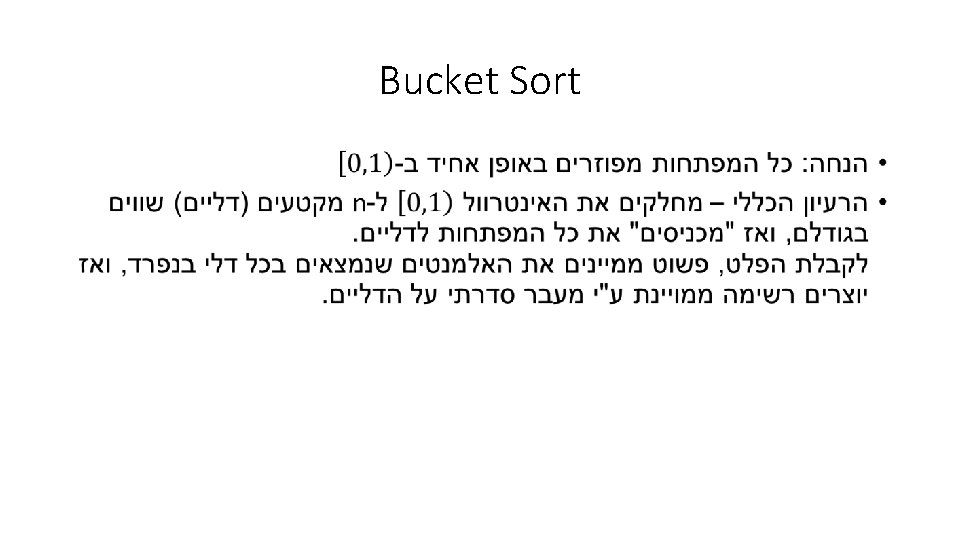
Bucket Sort •
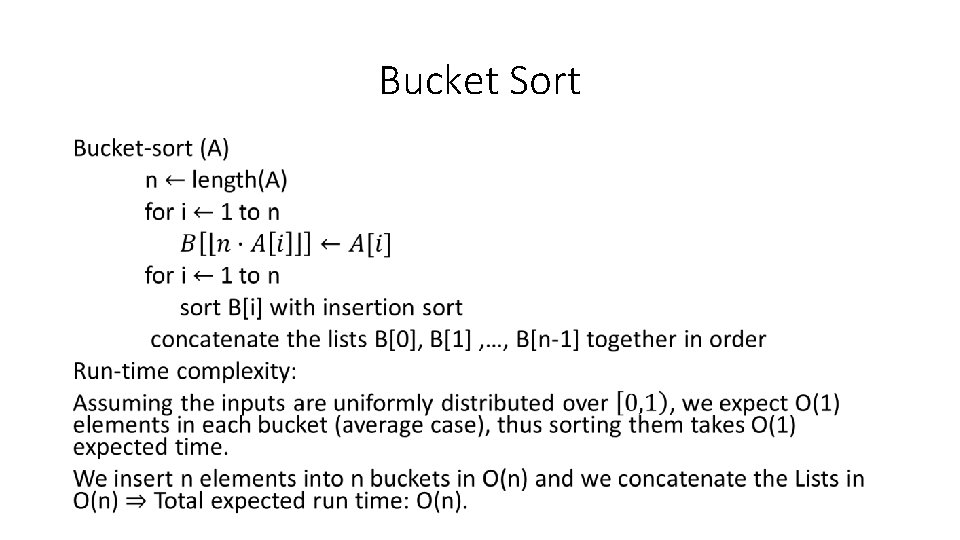
Bucket Sort •
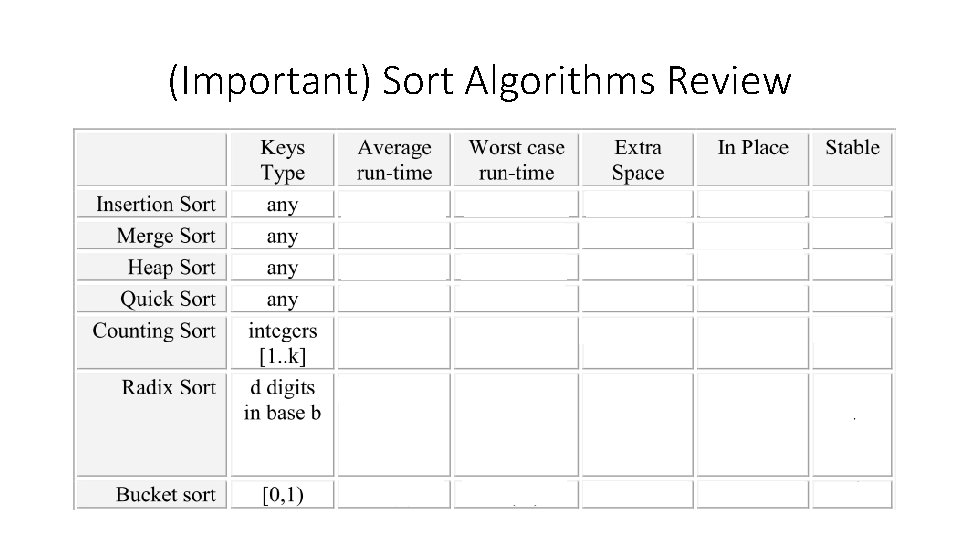
(Important) Sort Algorithms Review
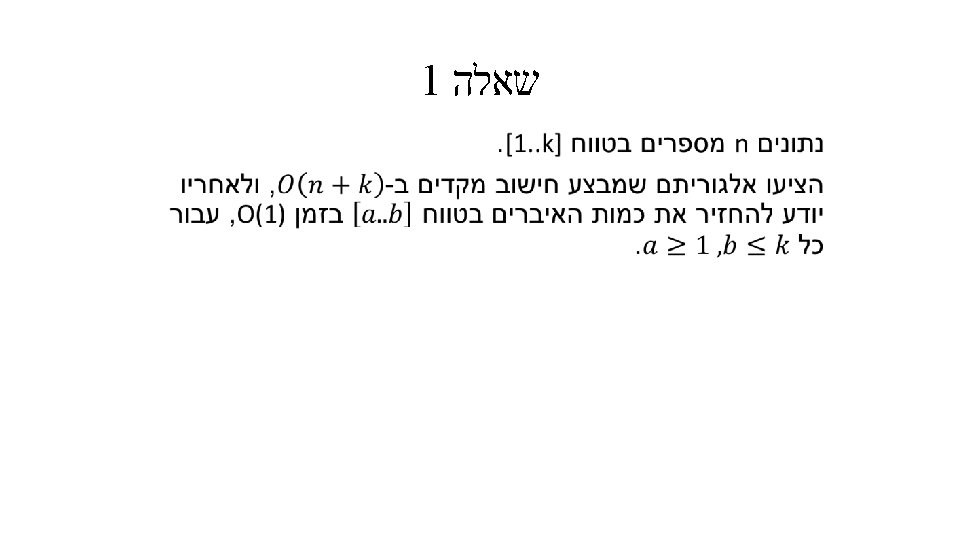
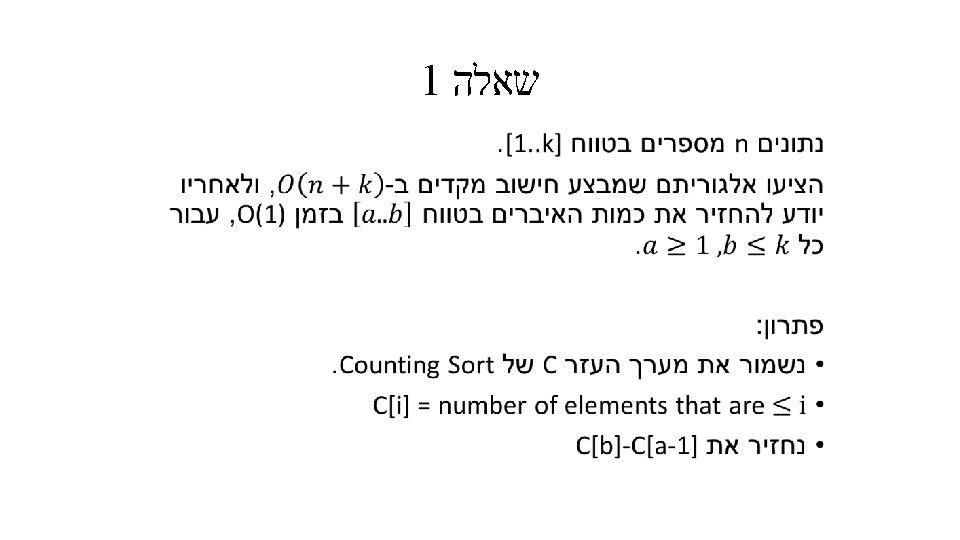
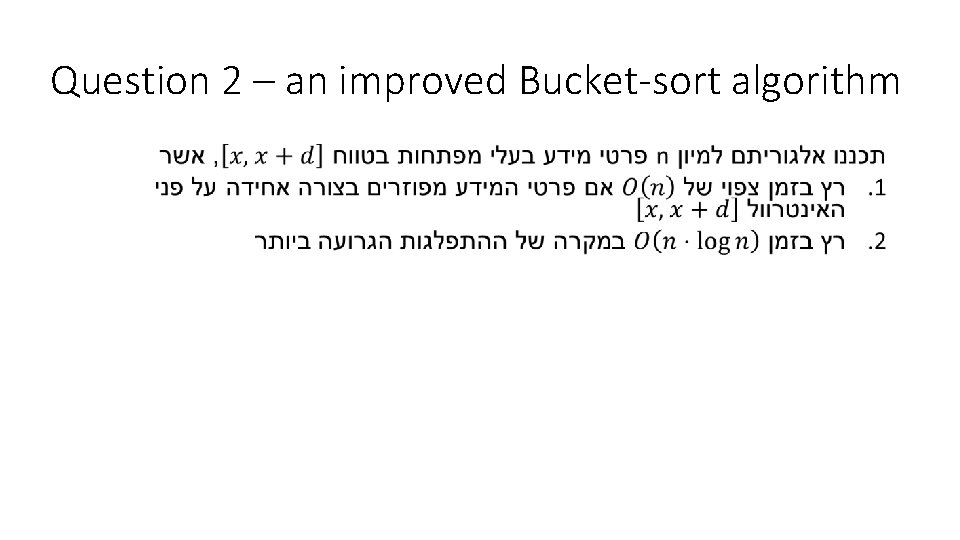
Question 2 – an improved Bucket-sort algorithm •
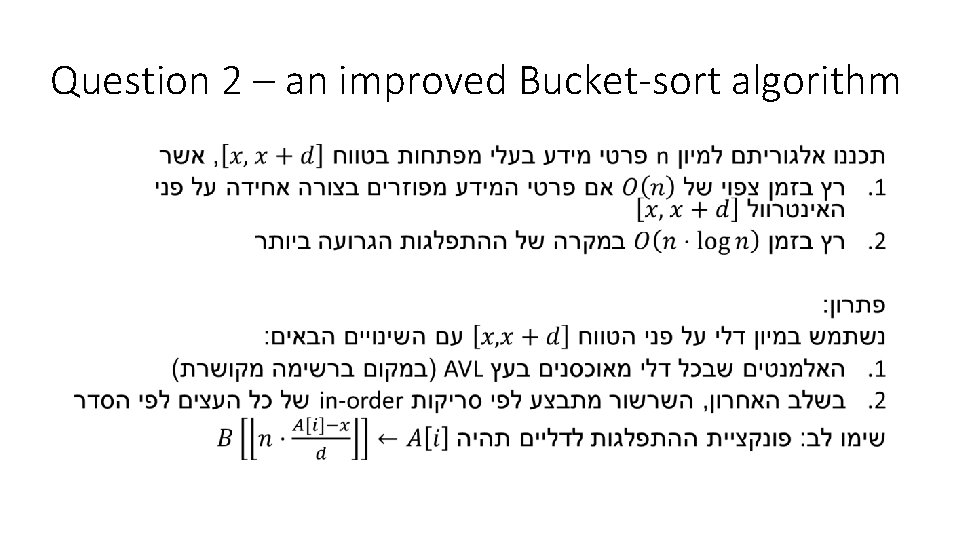
Question 2 – an improved Bucket-sort algorithm •
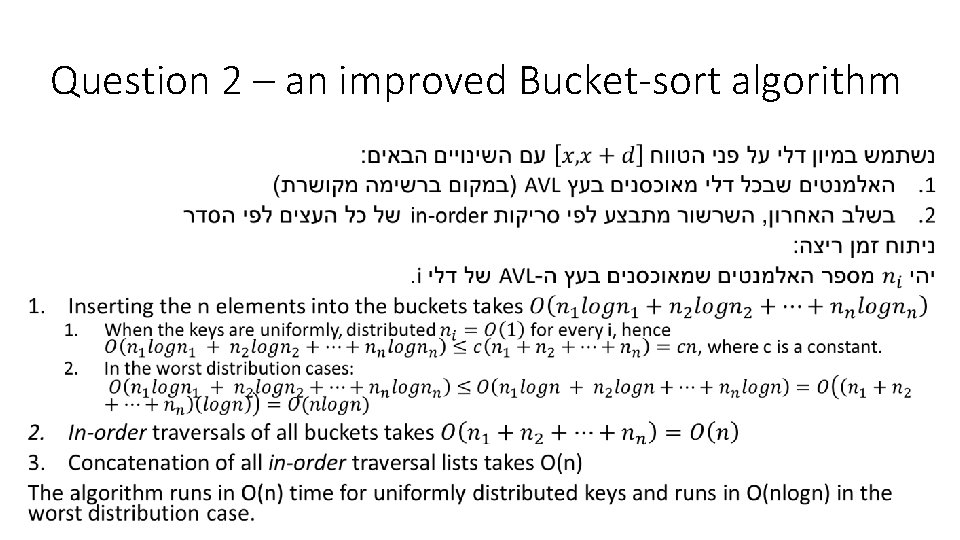
Question 2 – an improved Bucket-sort algorithm •
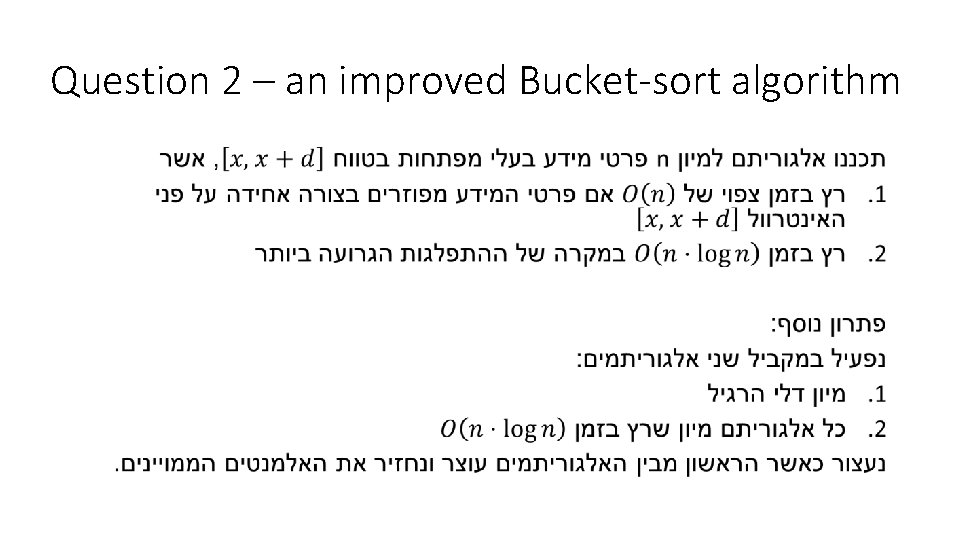
Question 2 – an improved Bucket-sort algorithm •
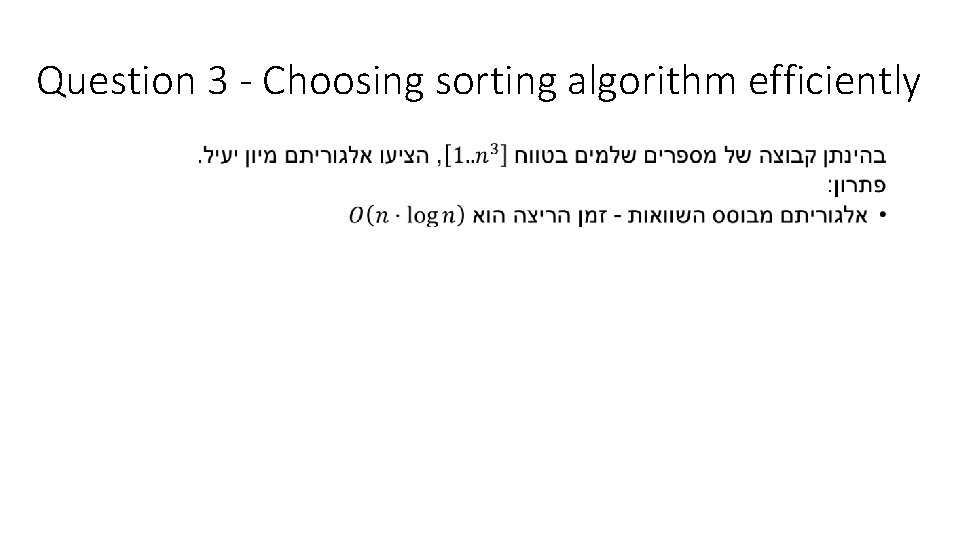
Question 3 - Choosing sorting algorithm efficiently •
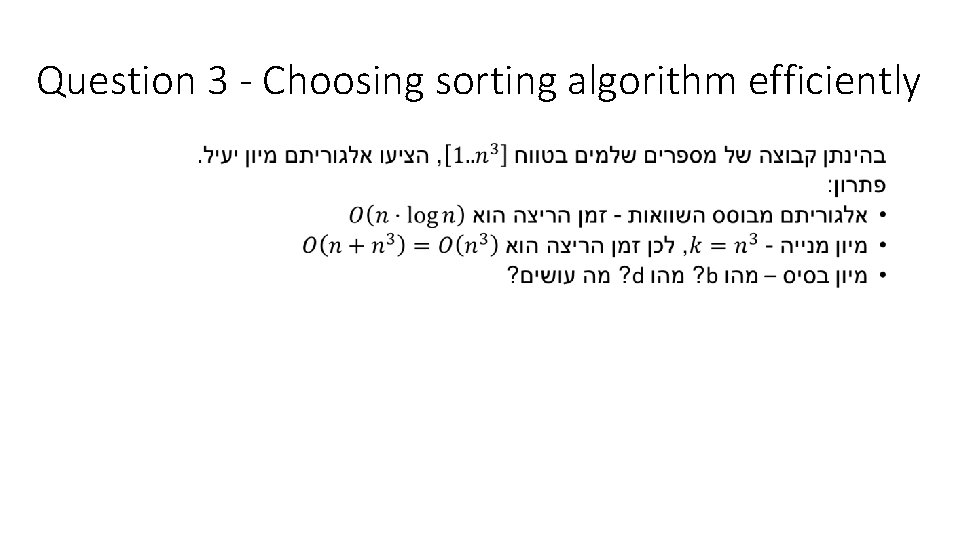
Question 3 - Choosing sorting algorithm efficiently •
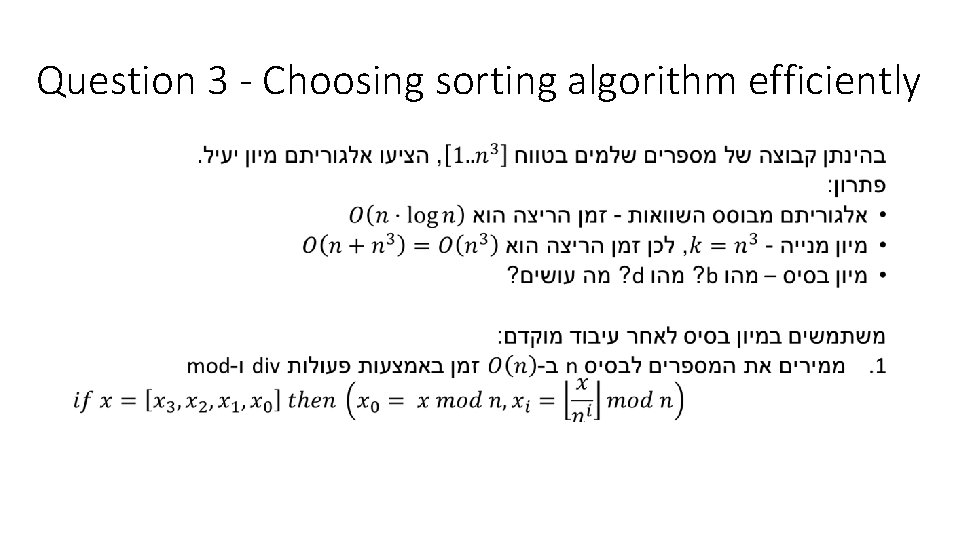
Question 3 - Choosing sorting algorithm efficiently •
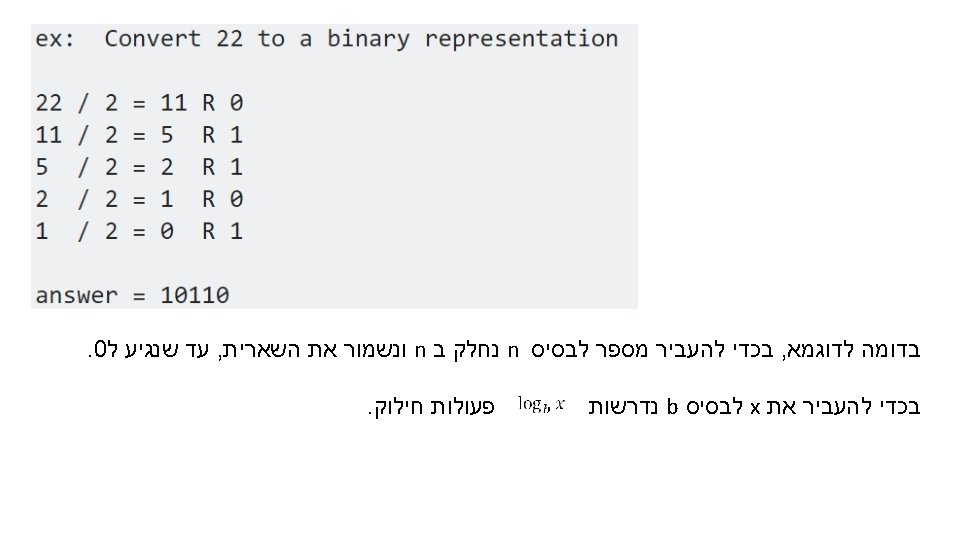
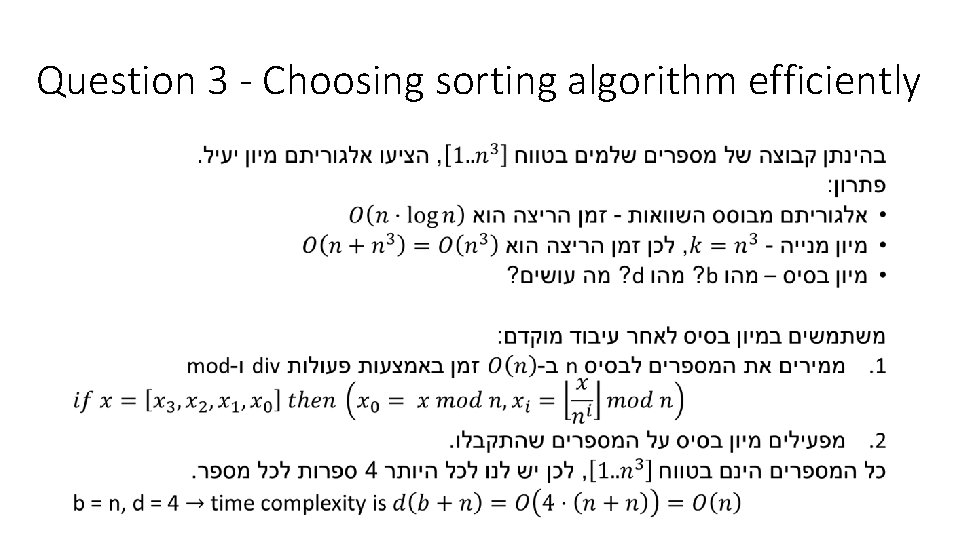
Question 3 - Choosing sorting algorithm efficiently •
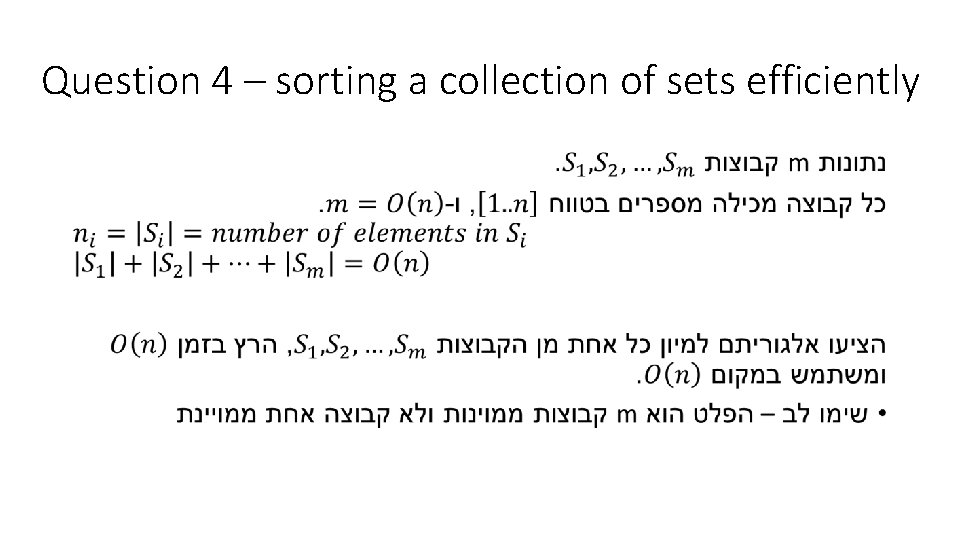
Question 4 – sorting a collection of sets efficiently •
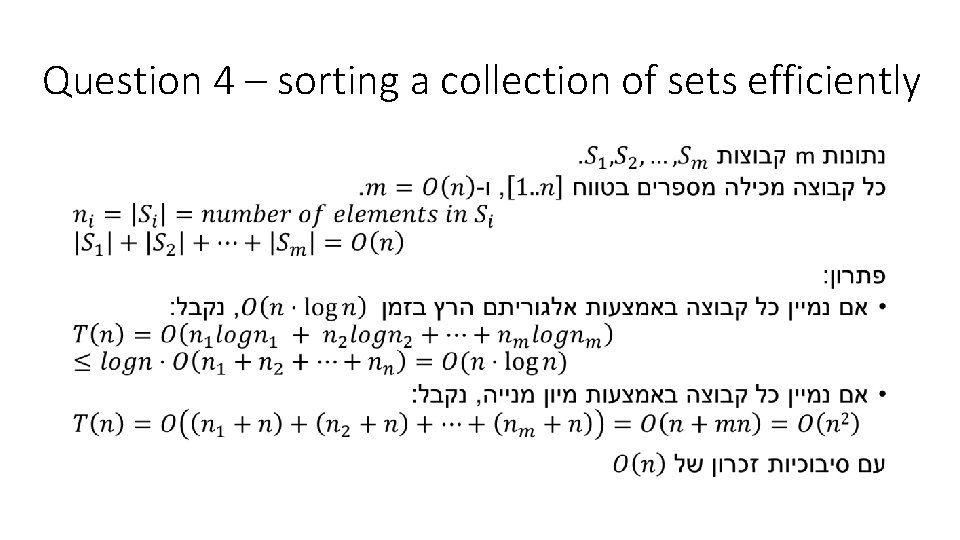
Question 4 – sorting a collection of sets efficiently •
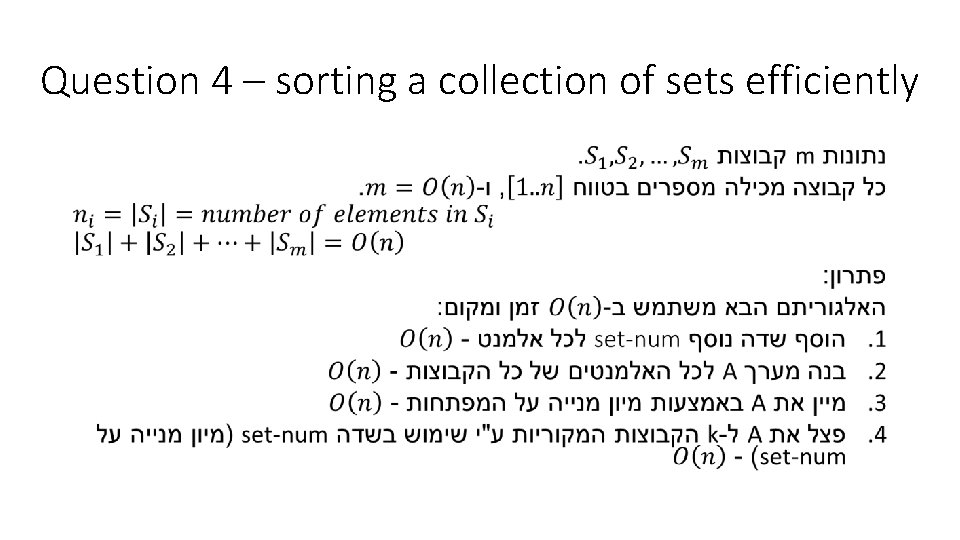
Question 4 – sorting a collection of sets efficiently •
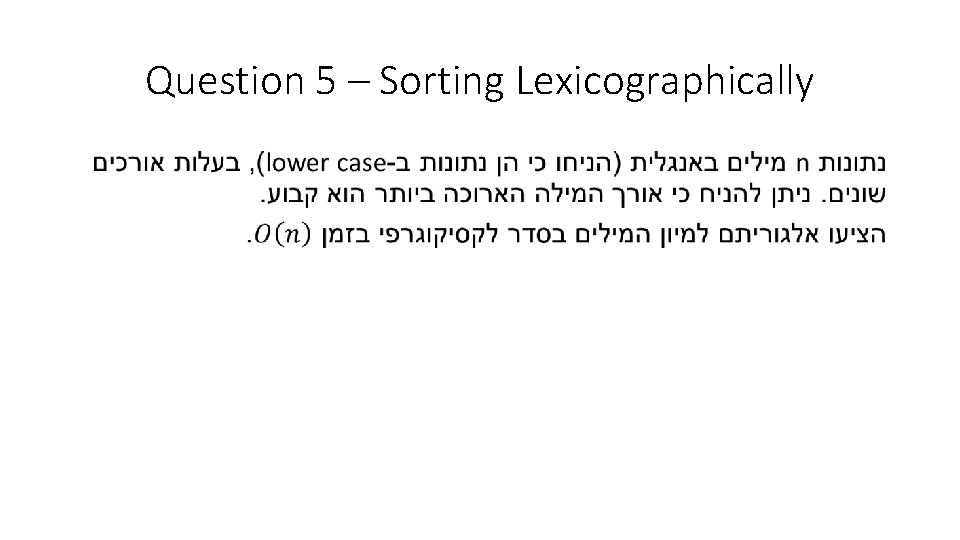
Question 5 – Sorting Lexicographically •
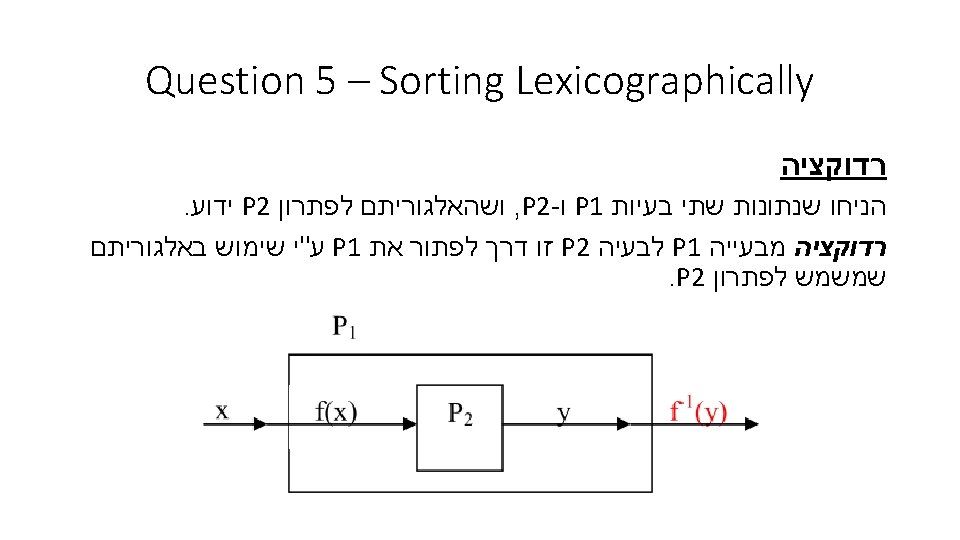
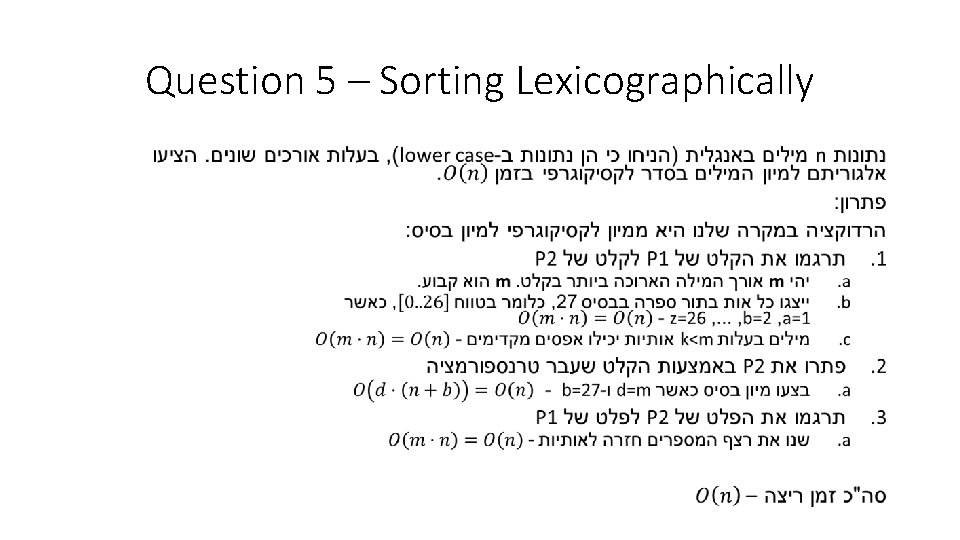
Question 5 – Sorting Lexicographically •
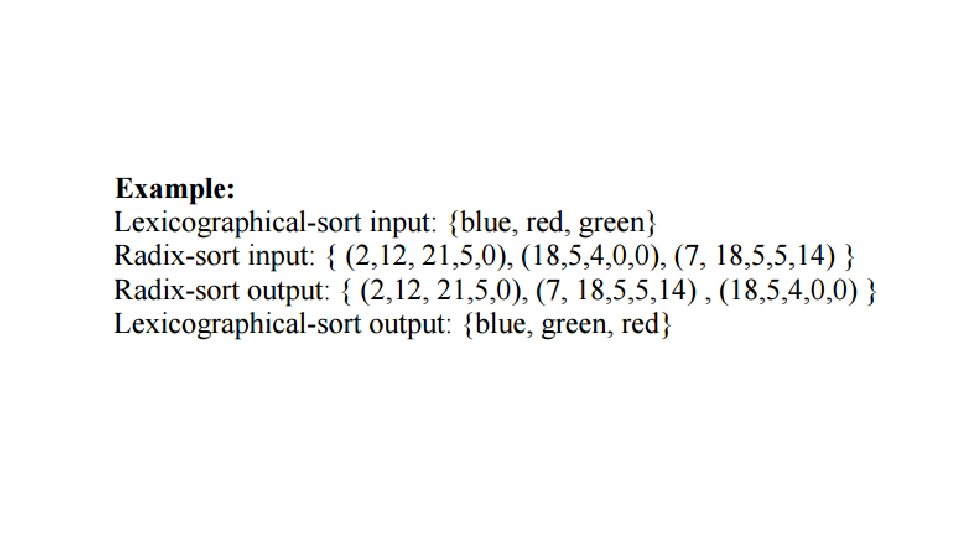
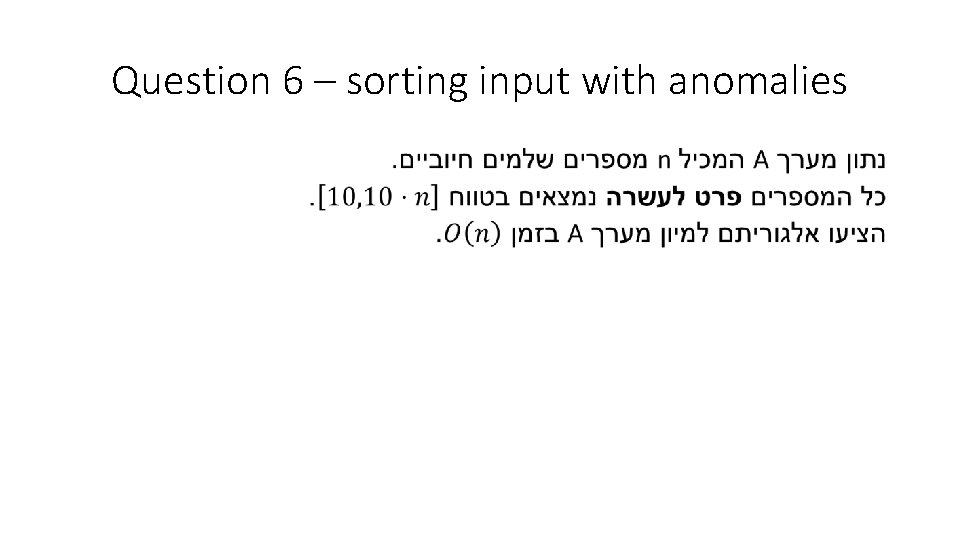
Question 6 – sorting input with anomalies •
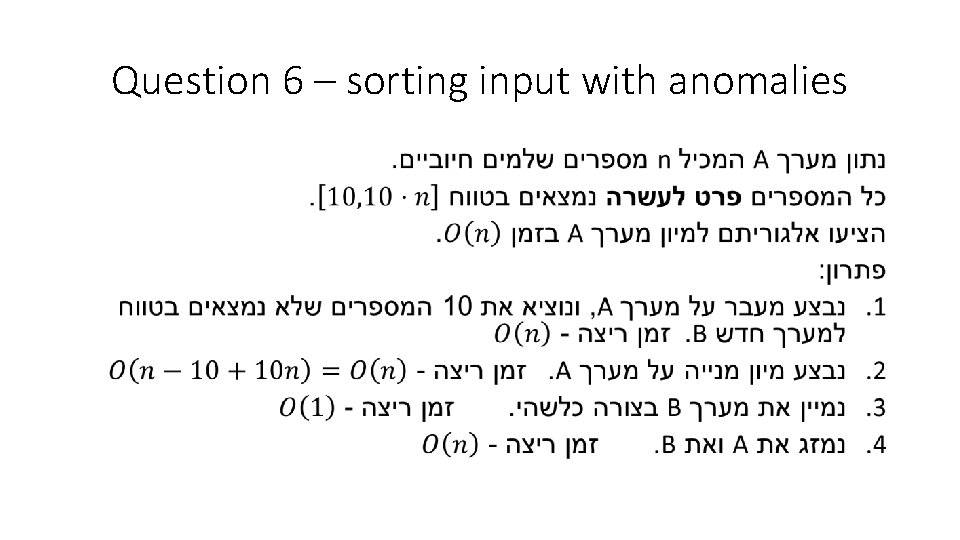
Question 6 – sorting input with anomalies •
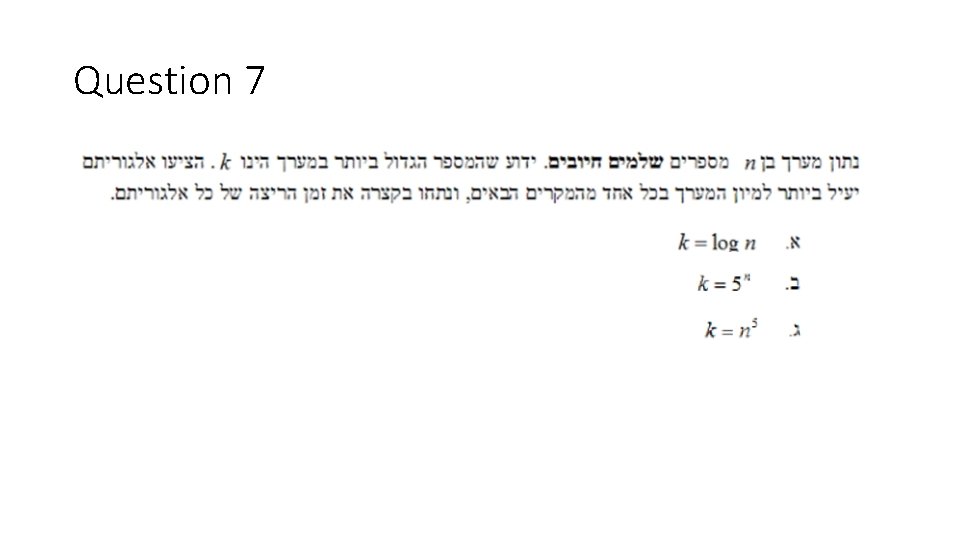
Question 7
Who invented selection sort
Phân độ lown
Block nhĩ thất độ 3
Thơ thất ngôn tứ tuyệt đường luật
Thơ thất ngôn tứ tuyệt đường luật
Walmart thất bại ở nhật
Tìm độ lớn thật của tam giác abc
Con hãy đưa tay khi thấy người vấp ngã
Tôn thất thuyết là ai
Gây tê cơ vuông thắt lưng
Sau thất bại ở hồ điển triệt
Bubble sort parallel programming
Radix sort animation
Approximate counting algorithm
Sadlier level d unit 1 synonyms
Bubble sort vs selection sort
Topological sort can be implemented by?
Sorting pseudocode
Difference between selection sort and bubble sort
Nnn sort
Bubble sort vs selection sort
Quick sort merge sort
Quick sort merge sort
Radix bucket sort
Topological sort kahn's algorithm
Radix sort algorithm
Library sorting method
Insertion sort flowchart in c
Quick sort algorithm
Topological sort algorithm
Search sort algorithm
Bubble sort algorithm pseudocode
Search sort algorithm
Quick sort algorithm with example
Bad sort algorithm
Simplest sort algorithm
A* vs ao*
Sweep line codeforces
If you're not confused you're not paying attention
Informal and casual
Attention is not not explanation
Not too narrow not too deep
Q then p
Just about right scale
Love is not all it is not meat nor drink
Ears that hear and eyes that see
Pp sit
Quotes about measurement and improvement
We will not be moved you're standing with us
Not a rustling leaf, not a bird in flight