Programming for Engineers in Python Lecture 11 GUI
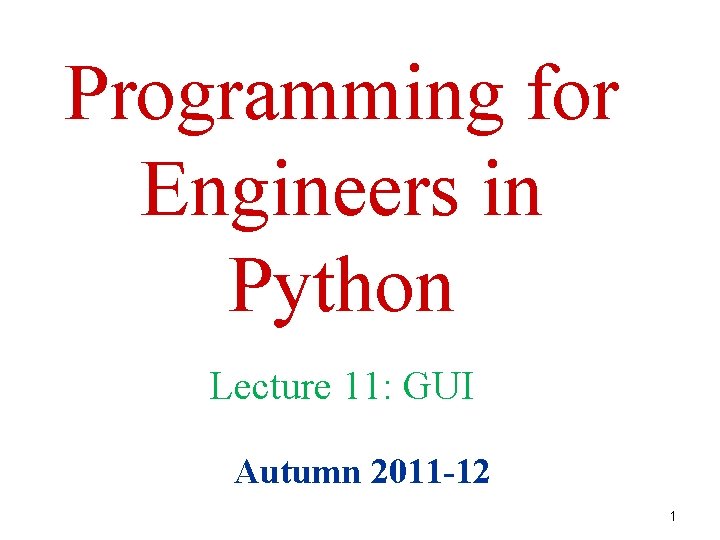
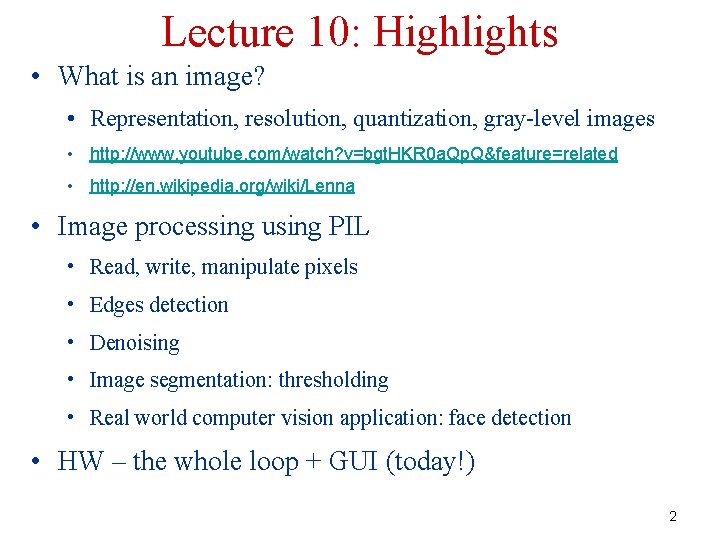
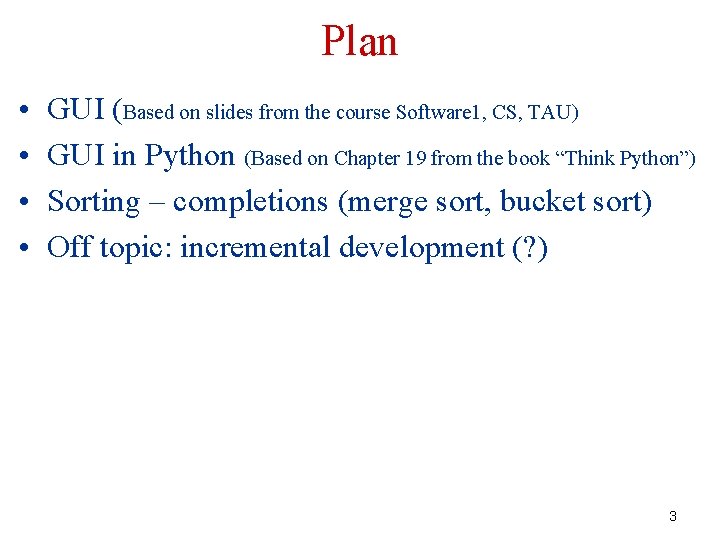
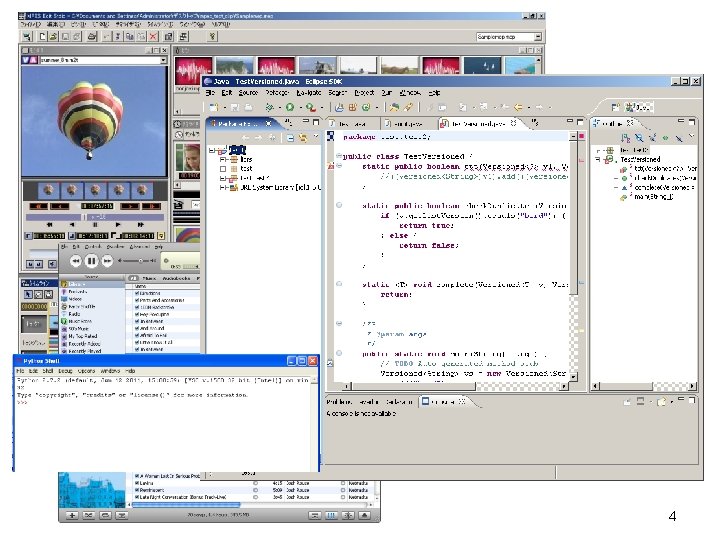
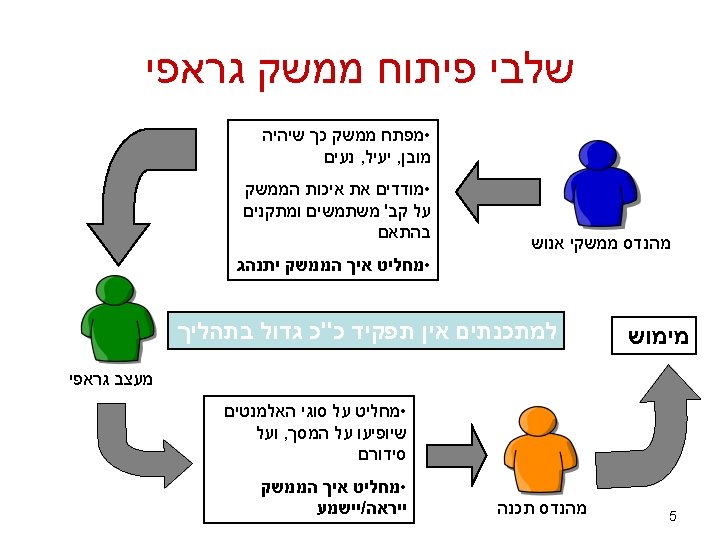
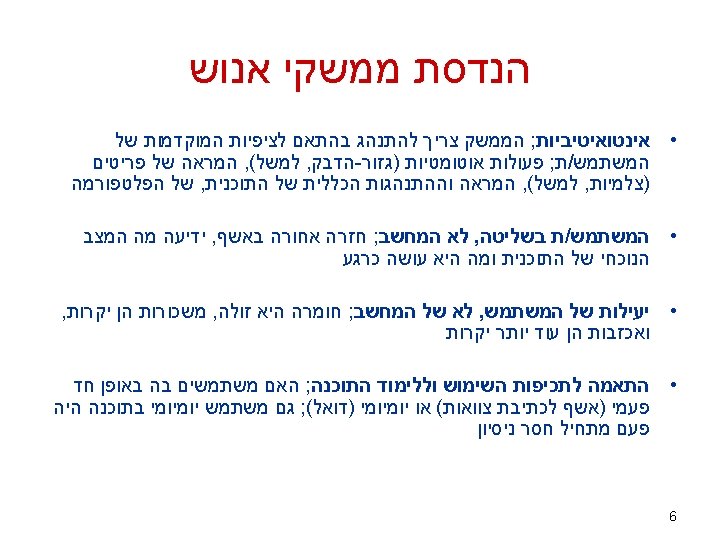
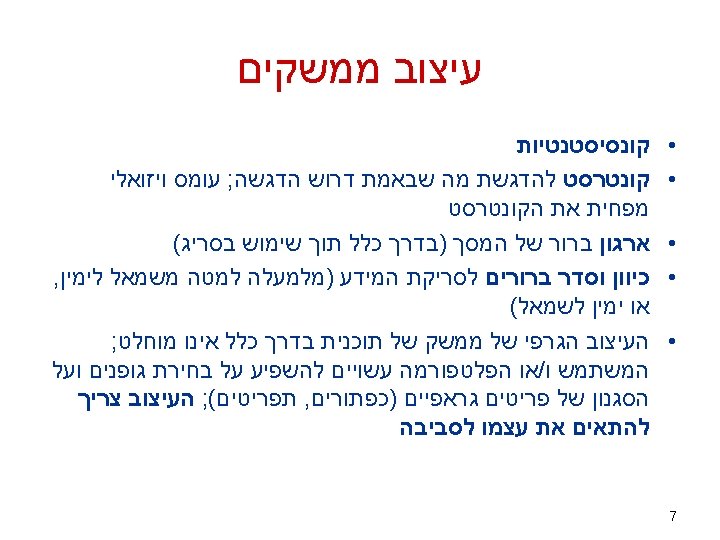
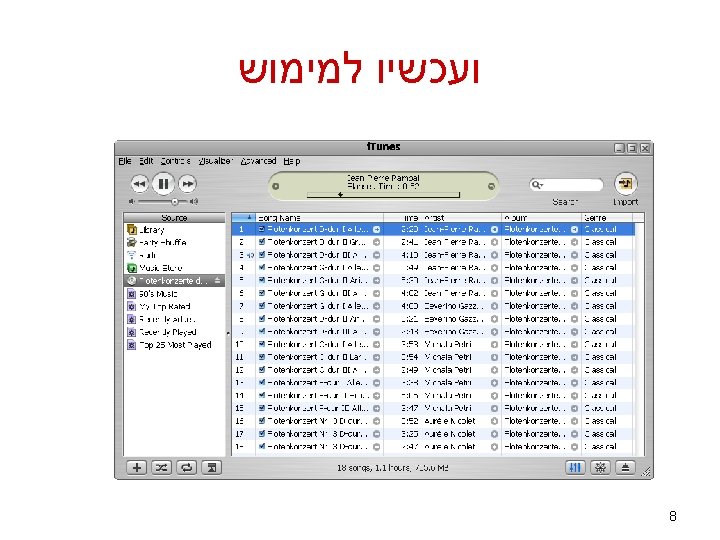
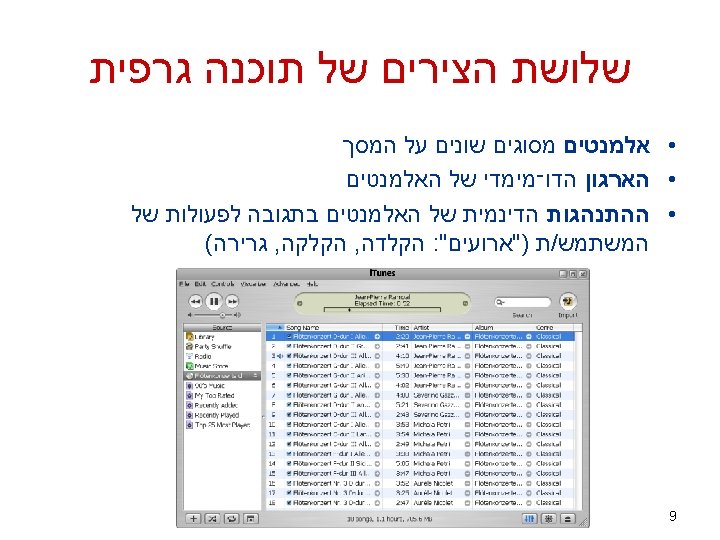
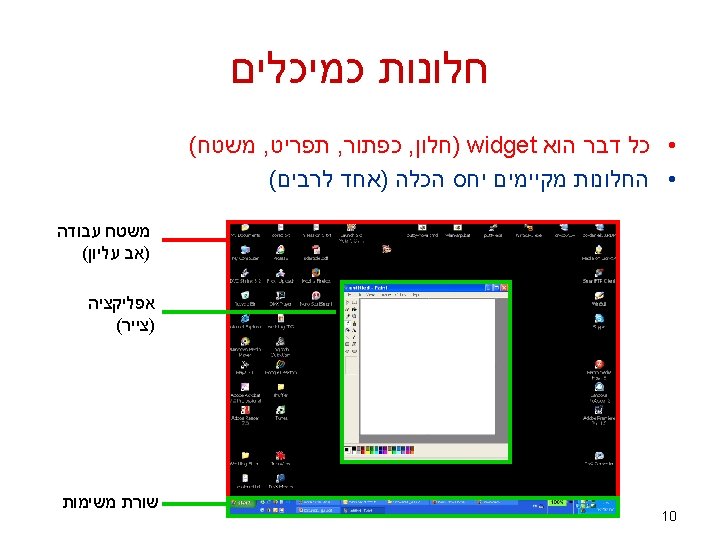
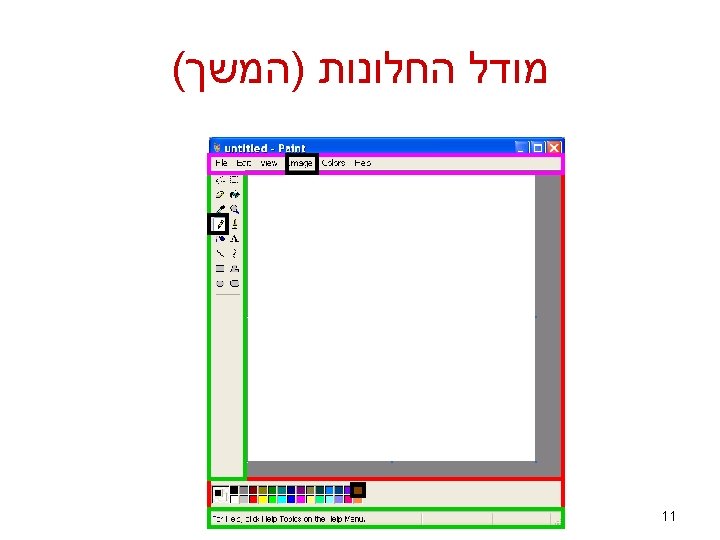
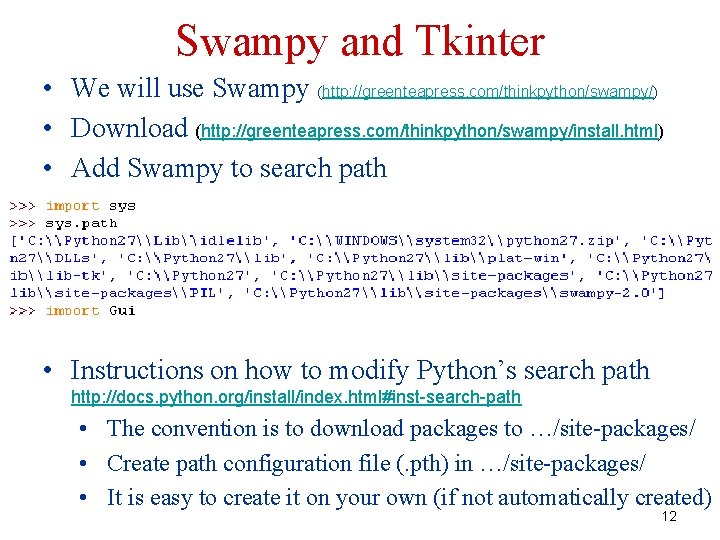
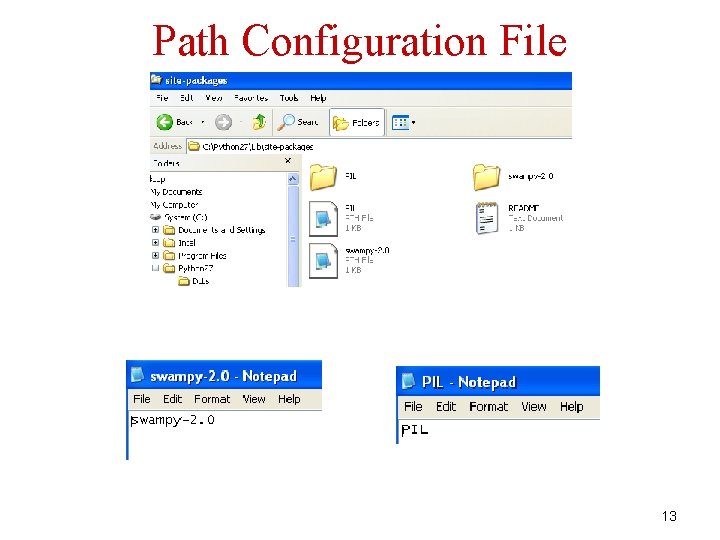
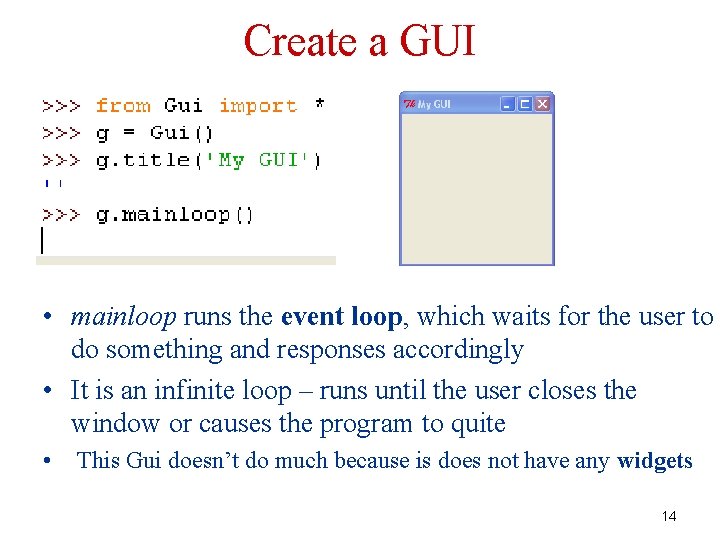
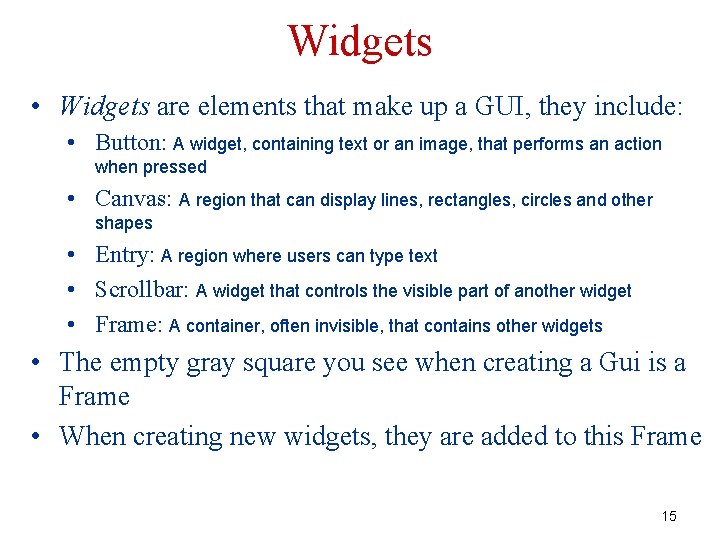
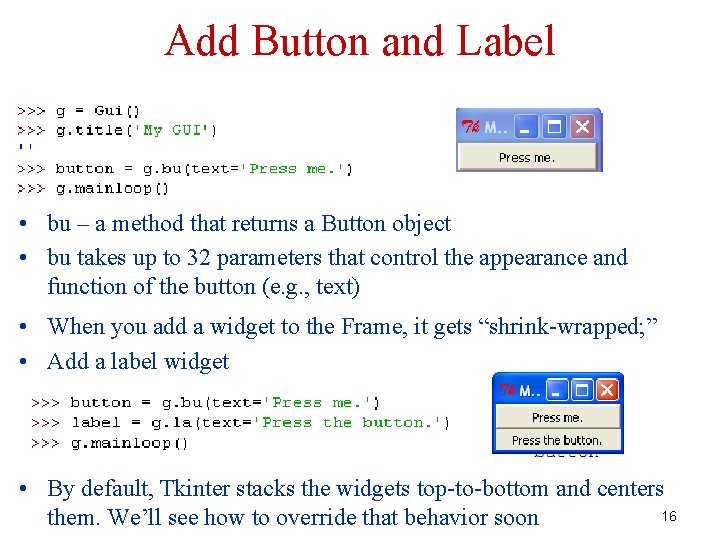
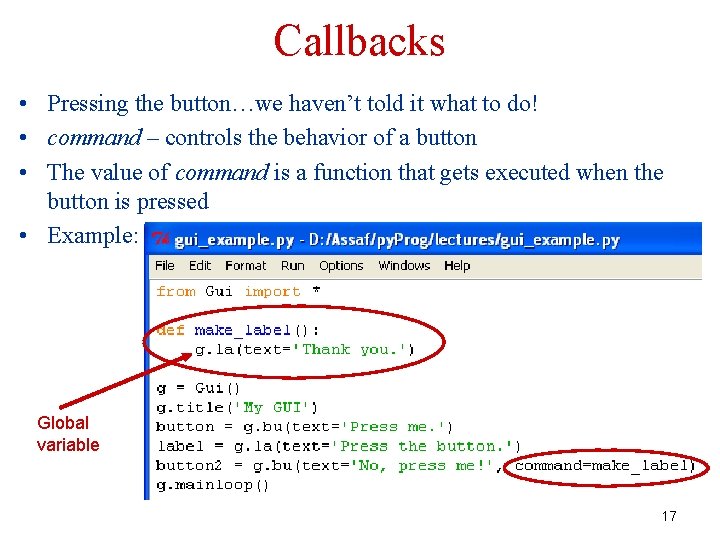
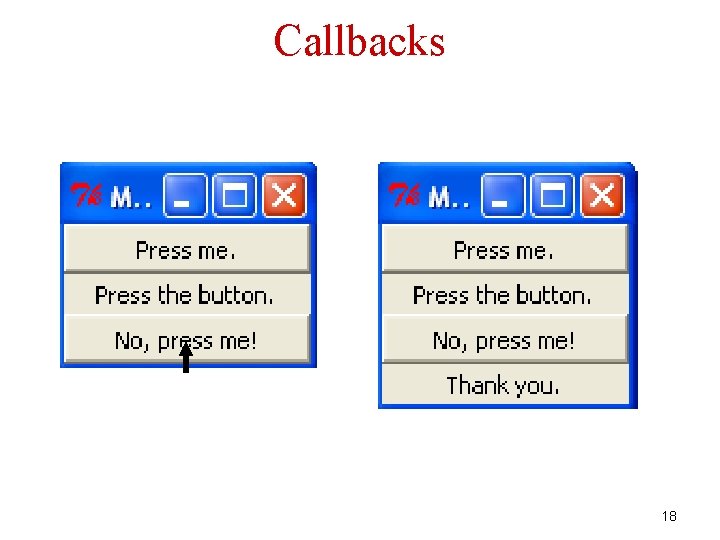
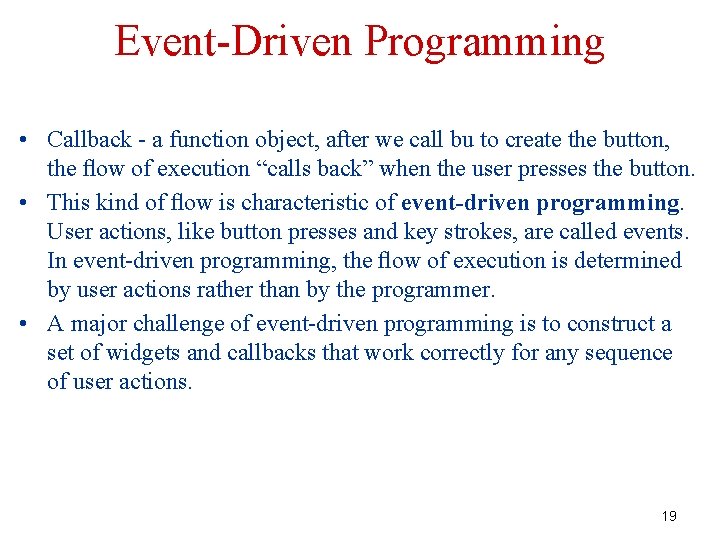
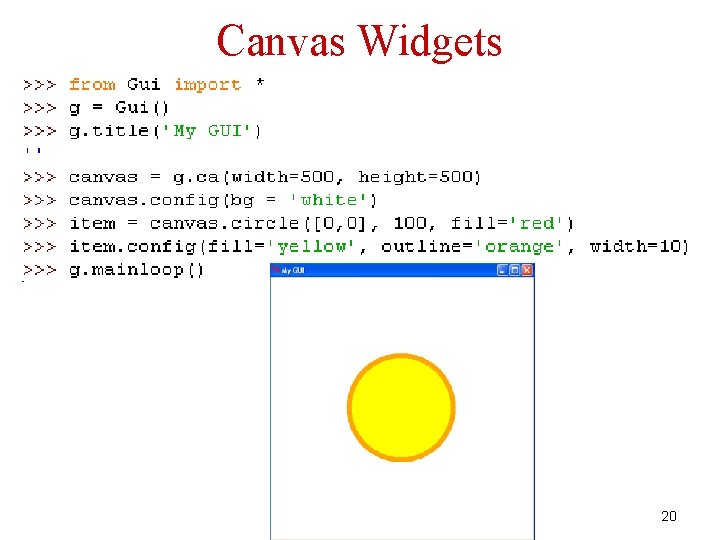
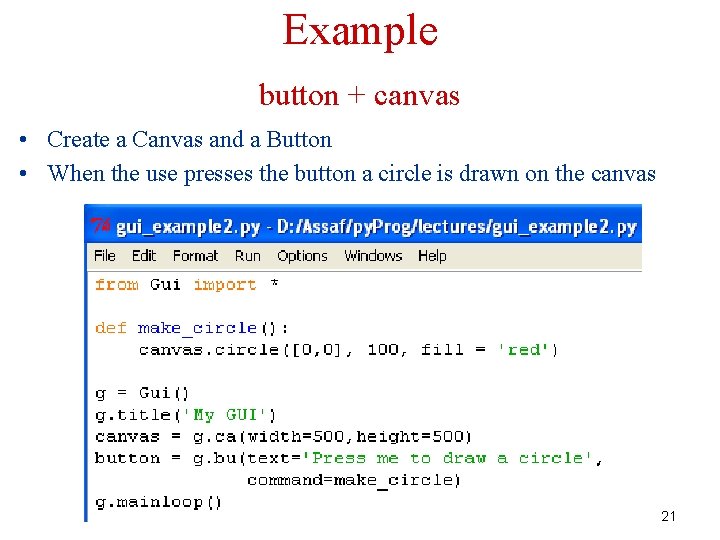
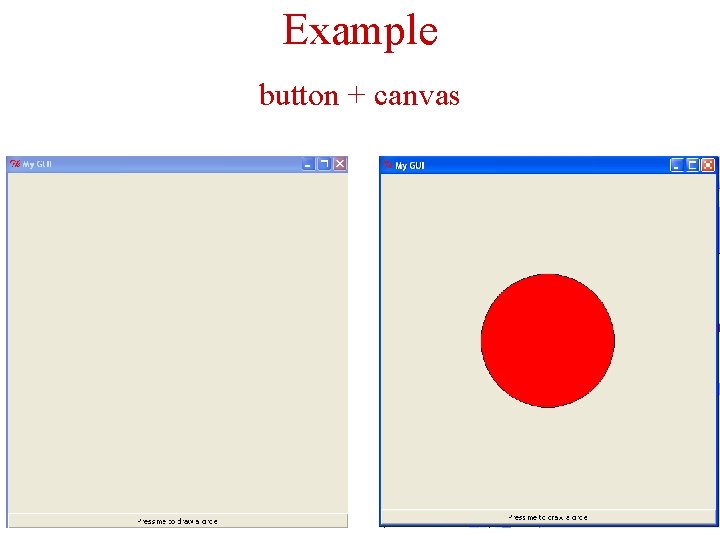
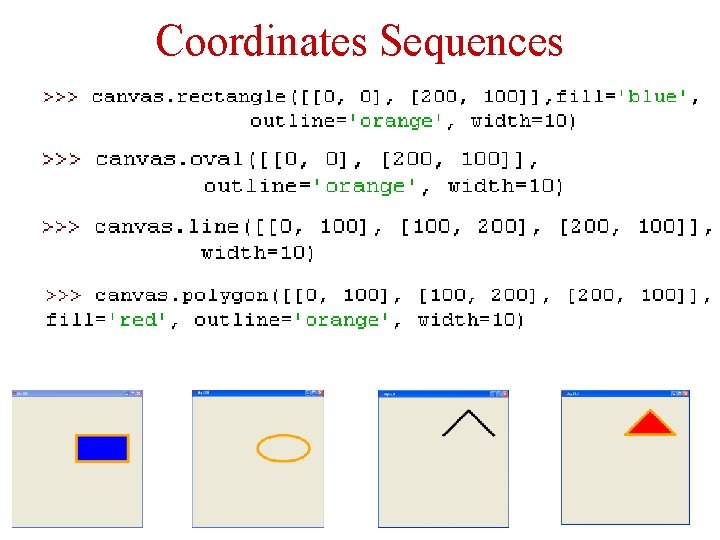
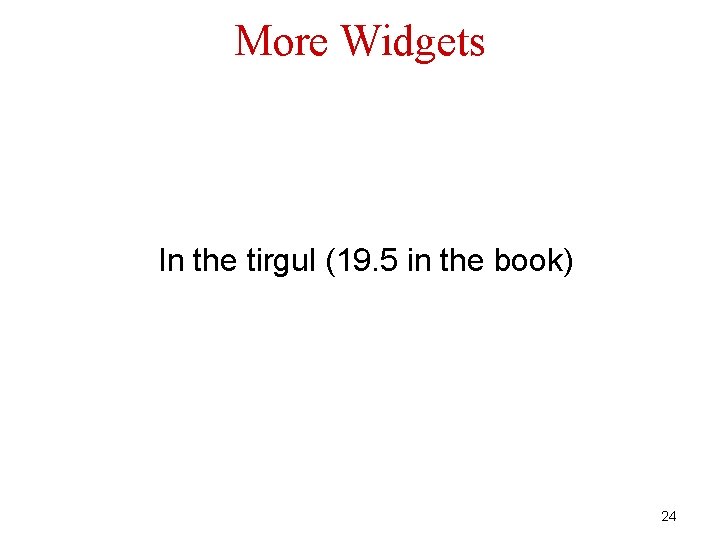
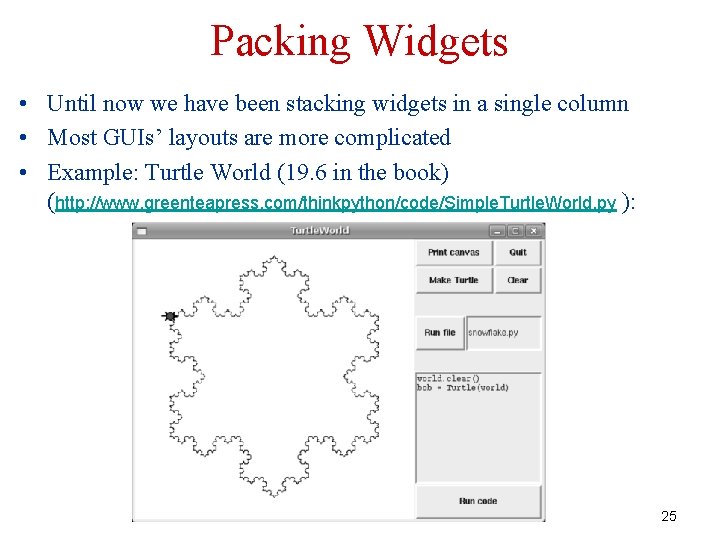
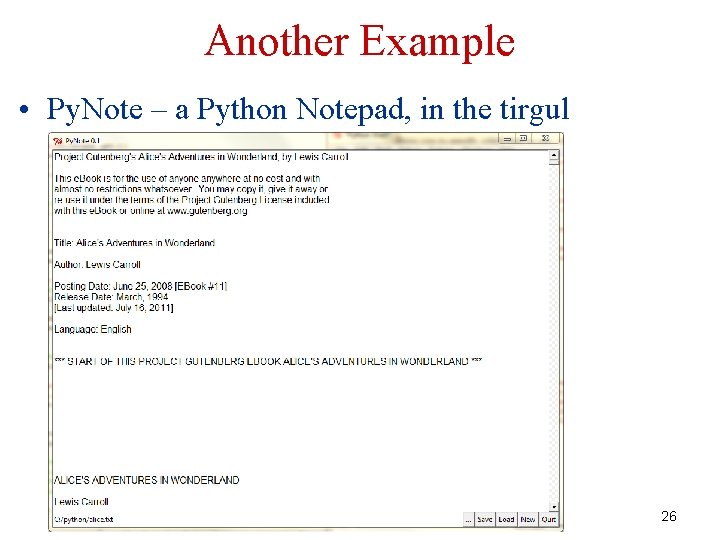
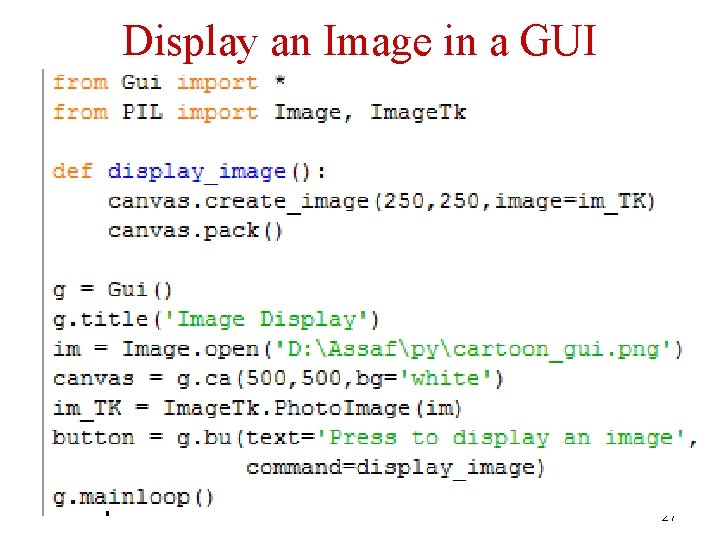
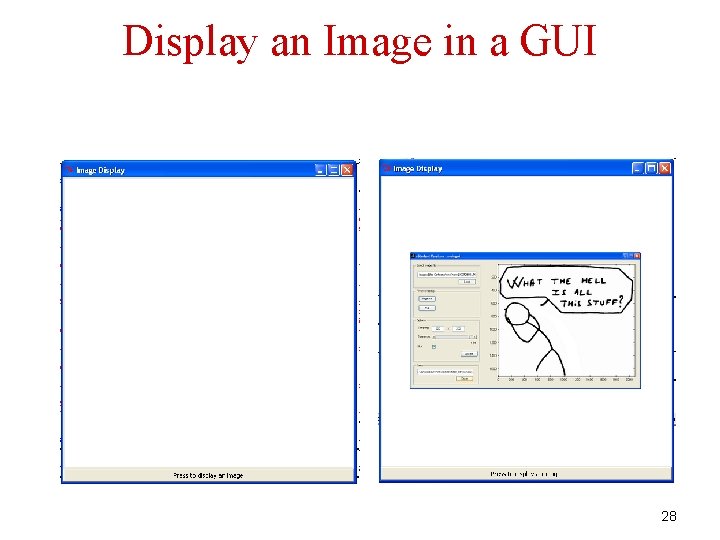
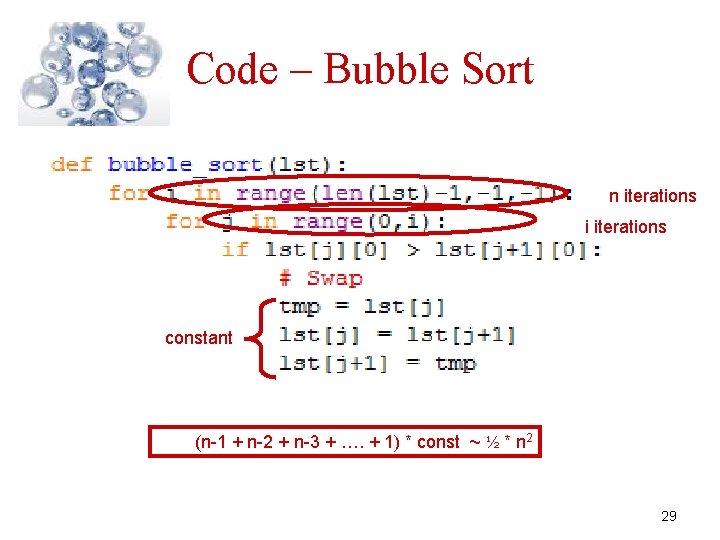
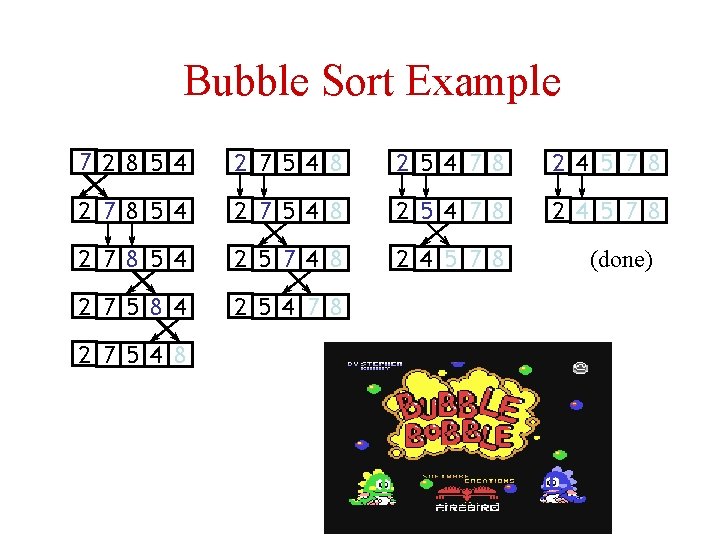
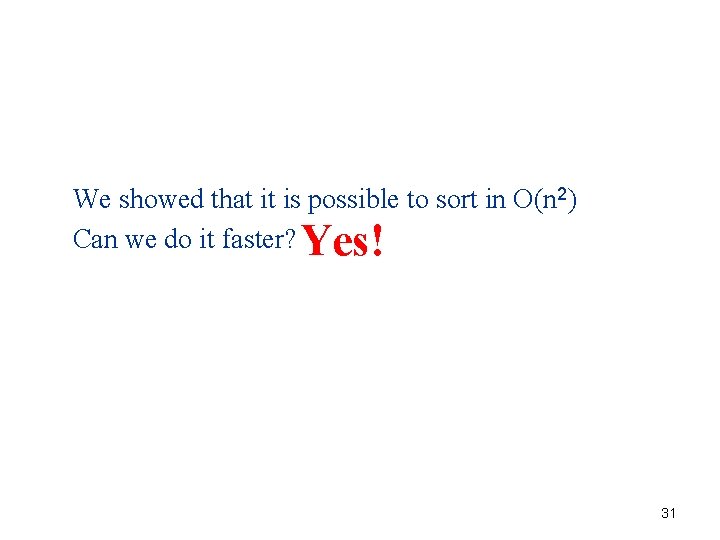
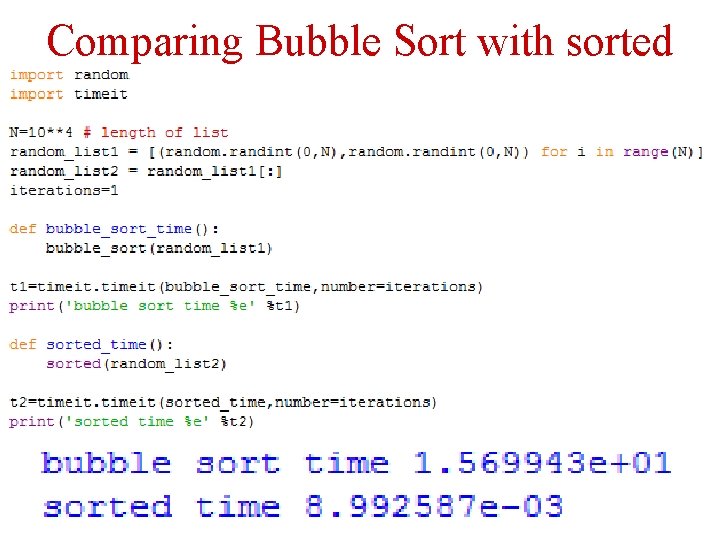
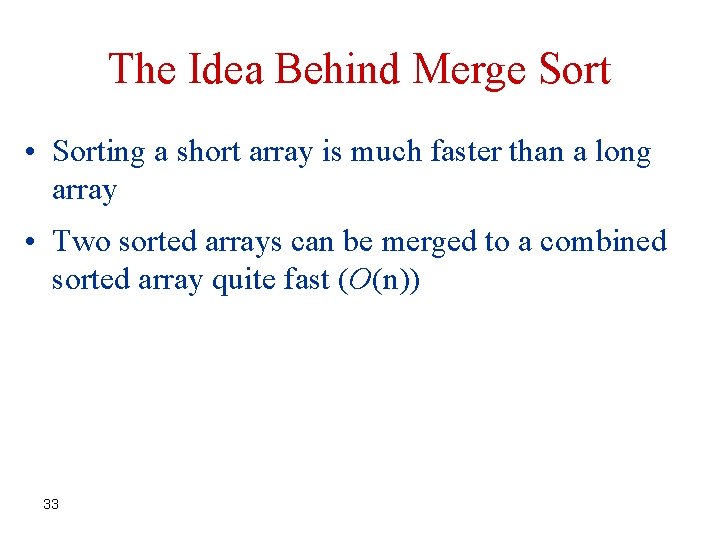
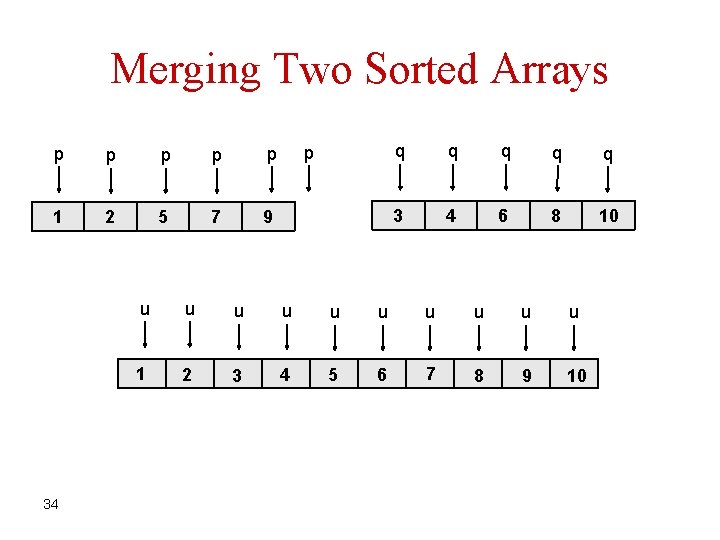
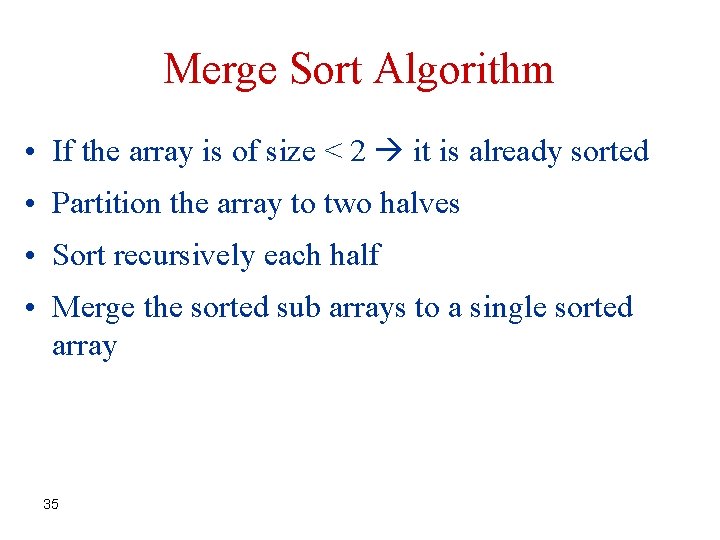
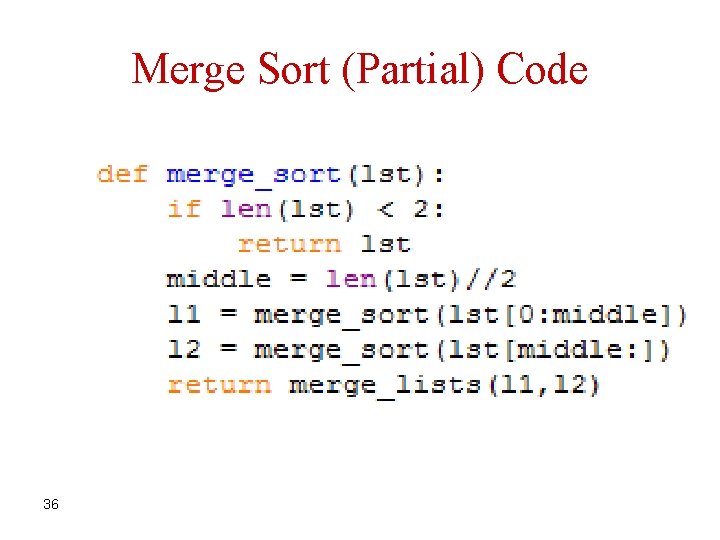
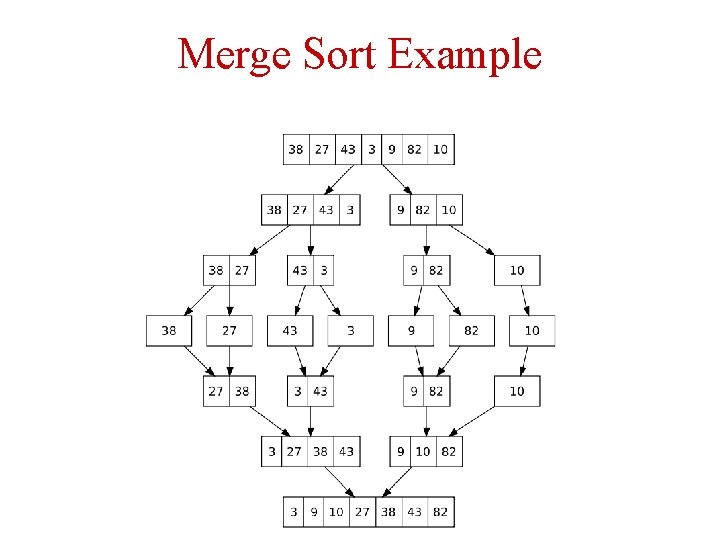
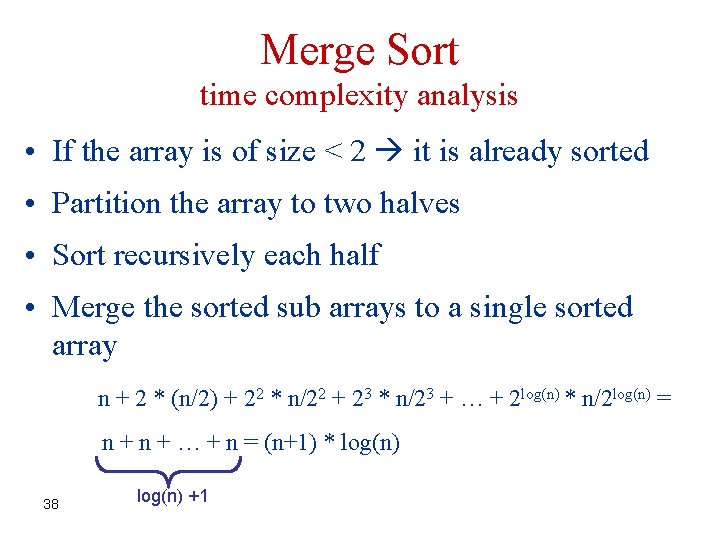
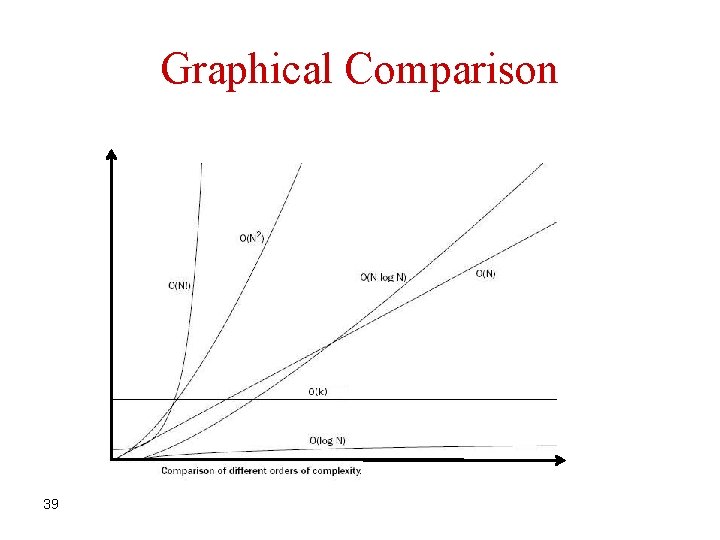
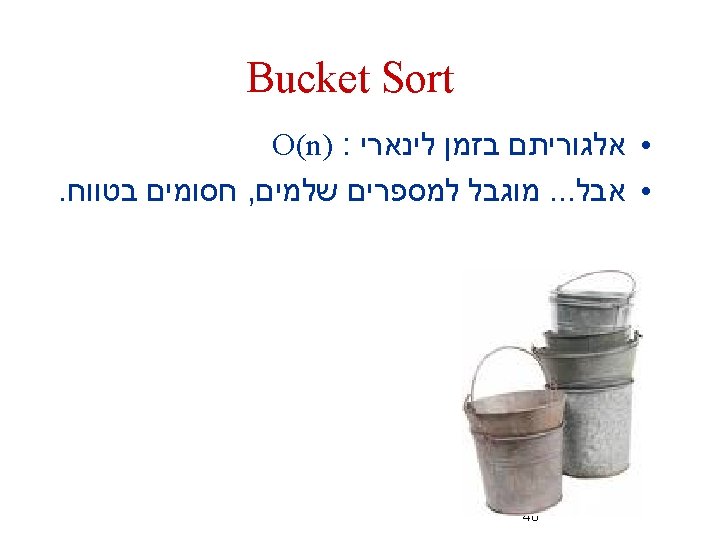
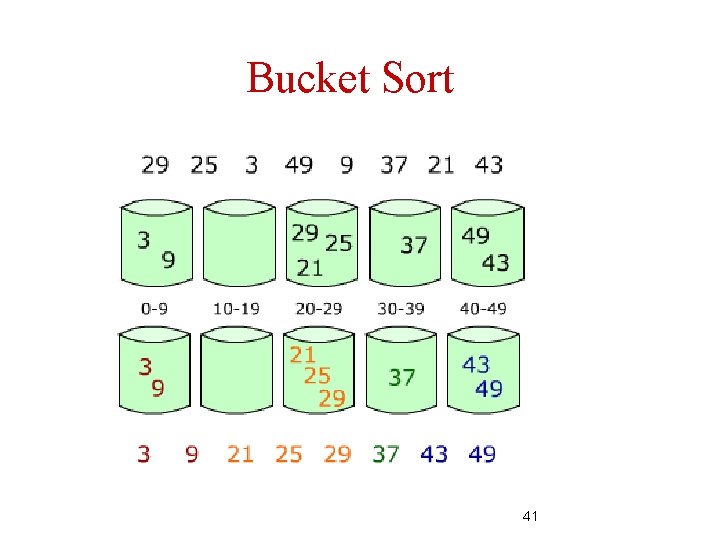
- Slides: 41
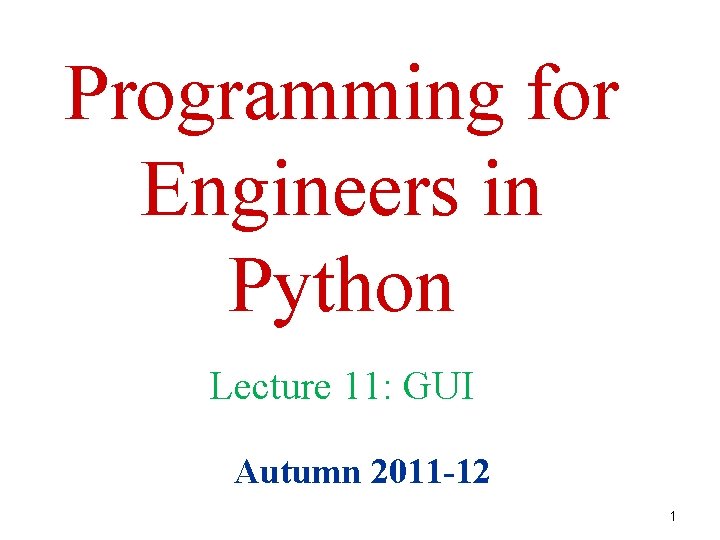
Programming for Engineers in Python Lecture 11: GUI Autumn 2011 -12 1
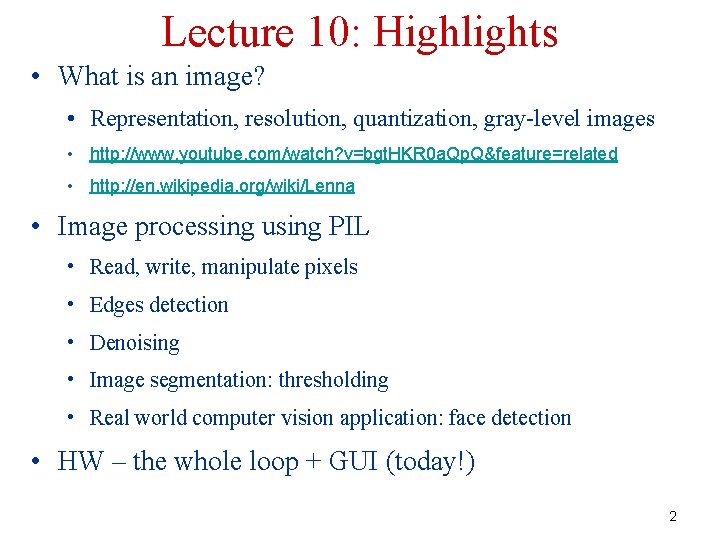
Lecture 10: Highlights • What is an image? • Representation, resolution, quantization, gray-level images • http: //www. youtube. com/watch? v=bgt. HKR 0 a. Qp. Q&feature=related • http: //en. wikipedia. org/wiki/Lenna • Image processing using PIL • Read, write, manipulate pixels • Edges detection • Denoising • Image segmentation: thresholding • Real world computer vision application: face detection • HW – the whole loop + GUI (today!) 2
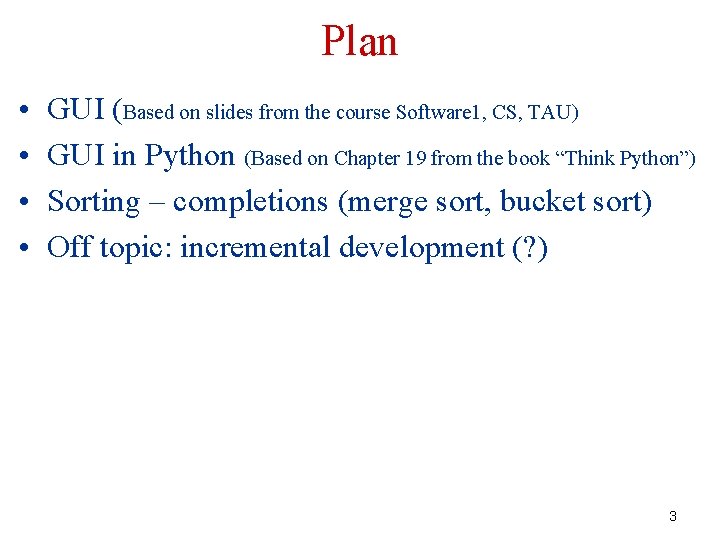
Plan • • GUI (Based on slides from the course Software 1, CS, TAU) GUI in Python (Based on Chapter 19 from the book “Think Python”) Sorting – completions (merge sort, bucket sort) Off topic: incremental development (? ) 3
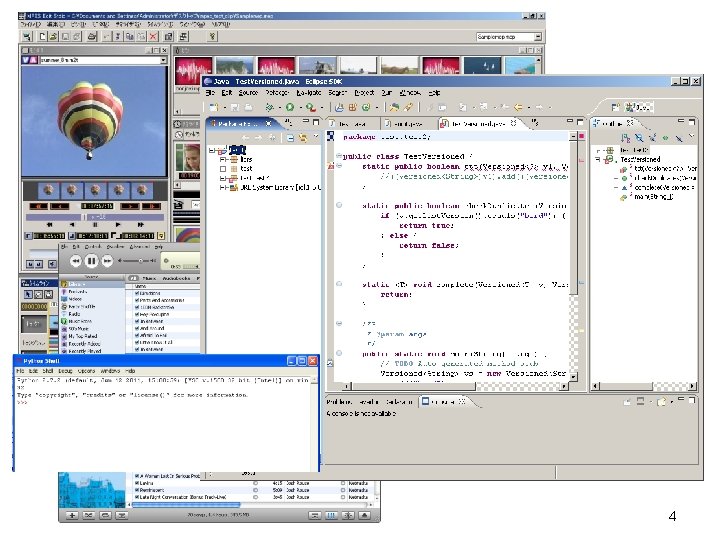
4
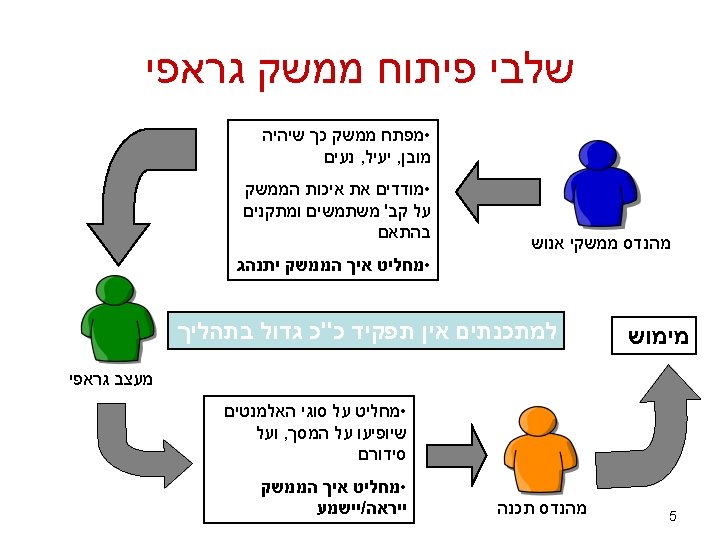
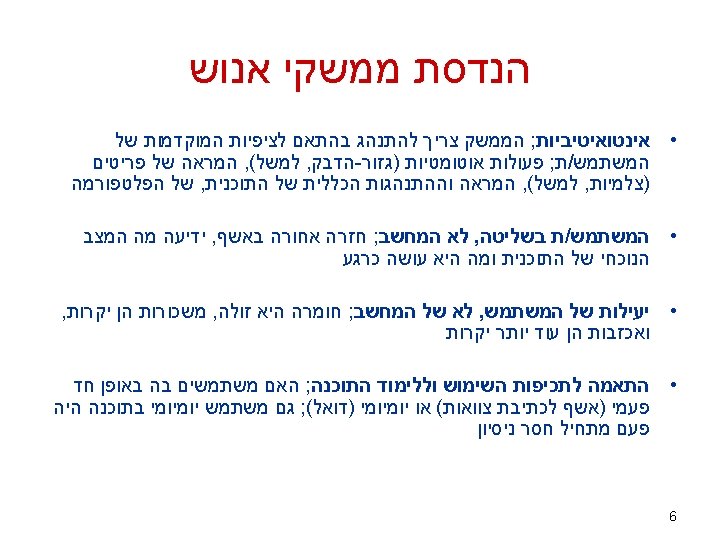
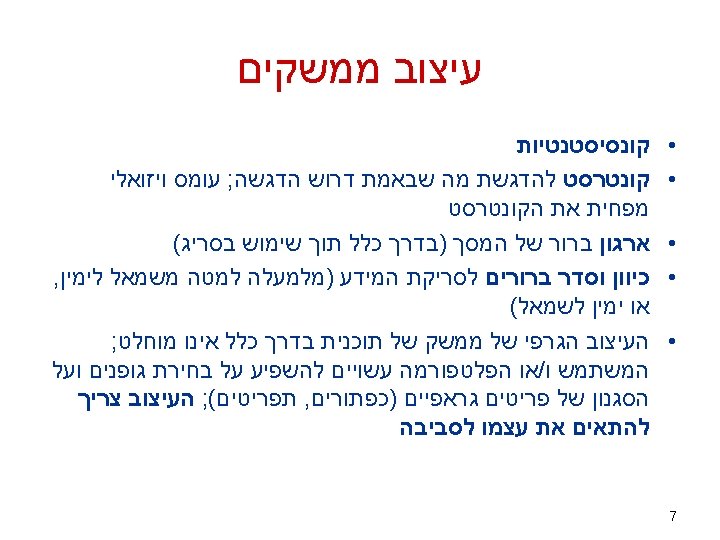
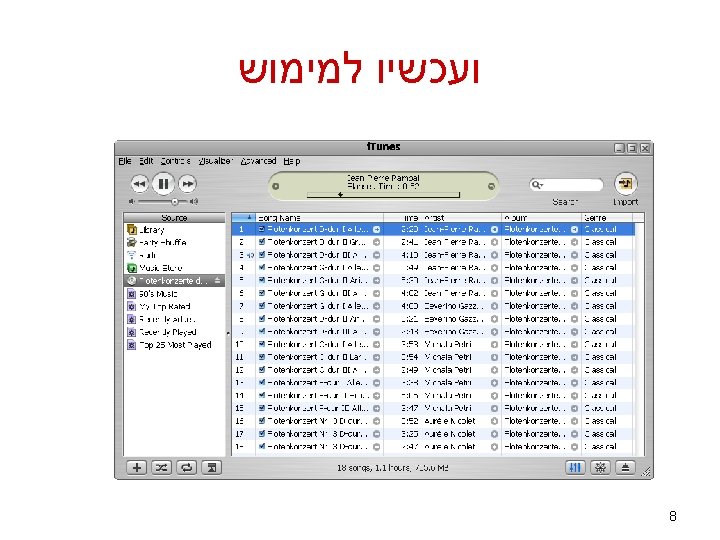
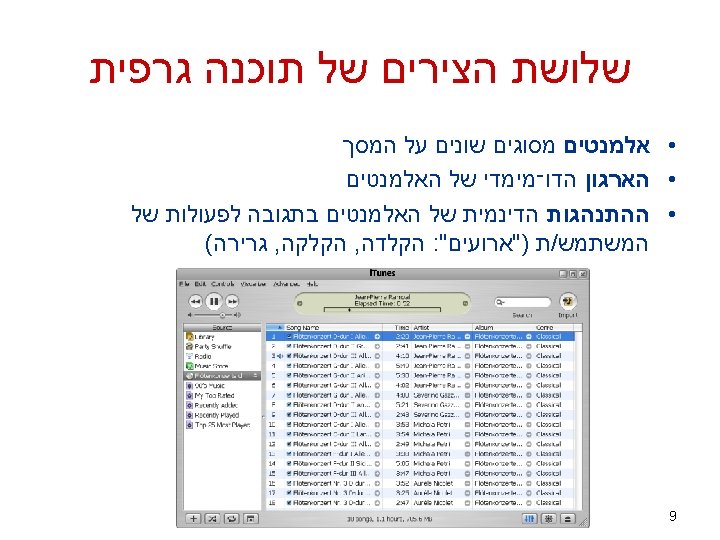
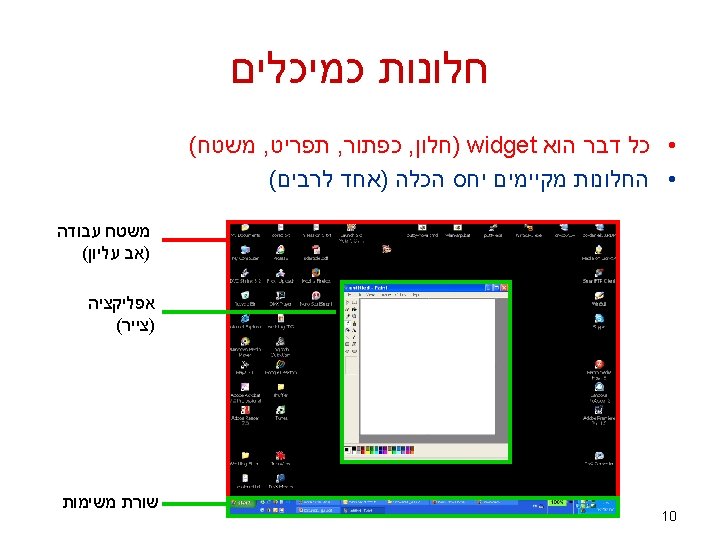
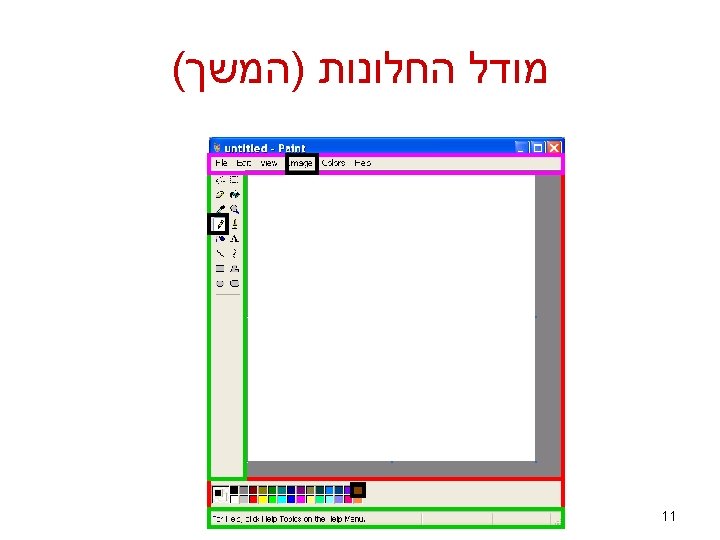
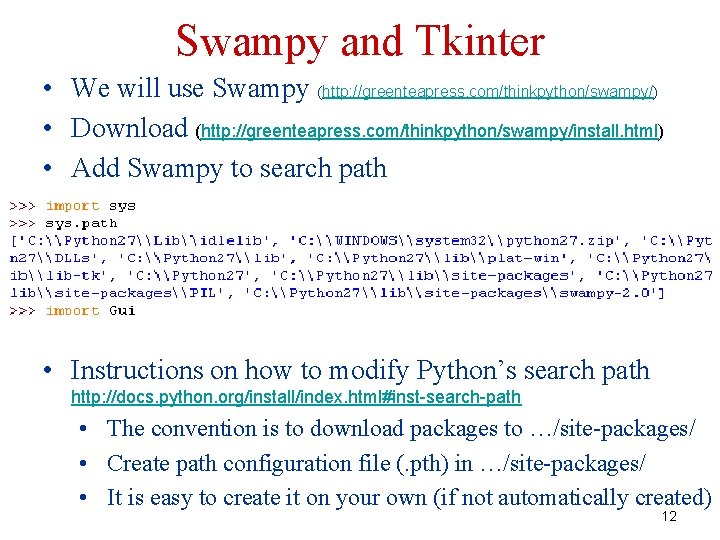
Swampy and Tkinter • We will use Swampy (http: //greenteapress. com/thinkpython/swampy/) • Download (http: //greenteapress. com/thinkpython/swampy/install. html) • Add Swampy to search path • Instructions on how to modify Python’s search path http: //docs. python. org/install/index. html#inst-search-path • The convention is to download packages to …/site-packages/ • Create path configuration file (. pth) in …/site-packages/ • It is easy to create it on your own (if not automatically created) 12
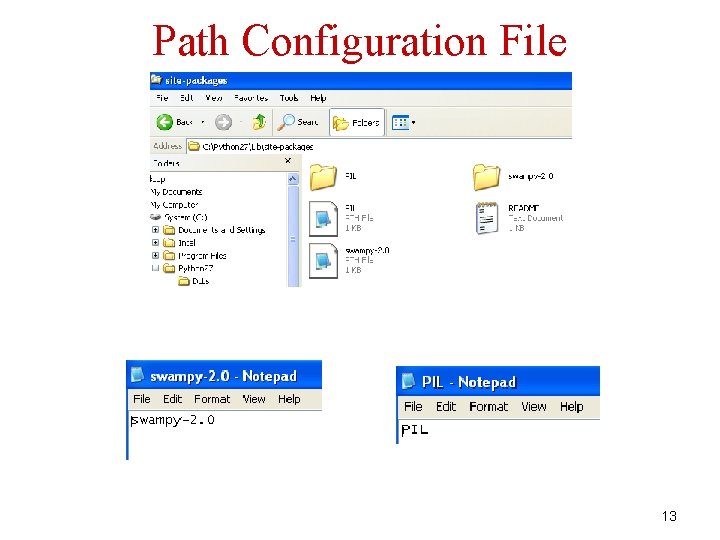
Path Configuration File 13
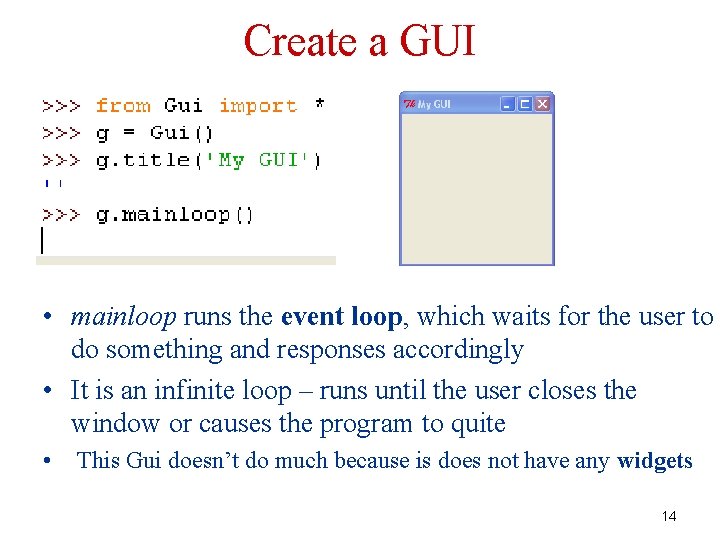
Create a GUI • mainloop runs the event loop, which waits for the user to do something and responses accordingly • It is an infinite loop – runs until the user closes the window or causes the program to quite • This Gui doesn’t do much because is does not have any widgets 14
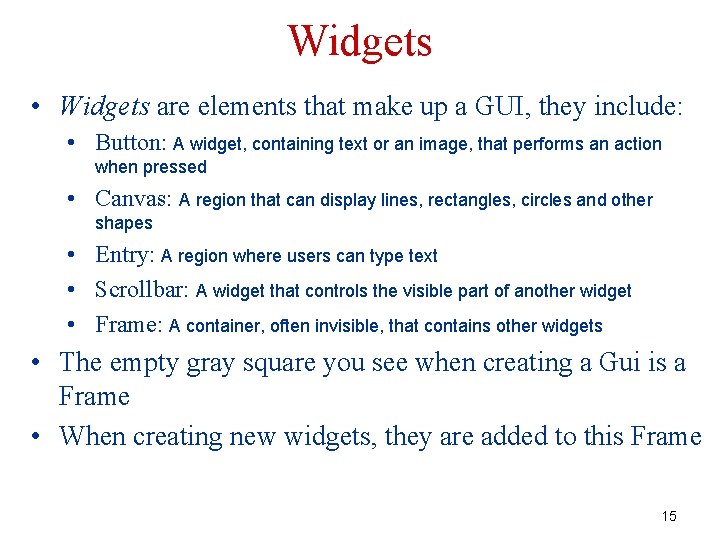
Widgets • Widgets are elements that make up a GUI, they include: • Button: A widget, containing text or an image, that performs an action when pressed • Canvas: A region that can display lines, rectangles, circles and other shapes • Entry: A region where users can type text • Scrollbar: A widget that controls the visible part of another widget • Frame: A container, often invisible, that contains other widgets • The empty gray square you see when creating a Gui is a Frame • When creating new widgets, they are added to this Frame 15
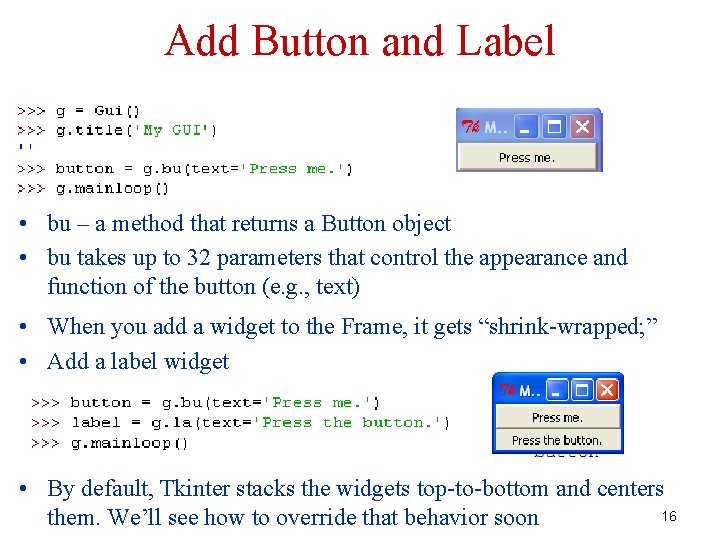
Add Button and Label • bu – a method that returns a Button object • bu takes up to 32 parameters that control the appearance and function of the button (e. g. , text) • When you add a widget to the Frame, it gets “shrink-wrapped; ” • Add a label widget • By default, Tkinter stacks the widgets top-to-bottom and centers 16 them. We’ll see how to override that behavior soon
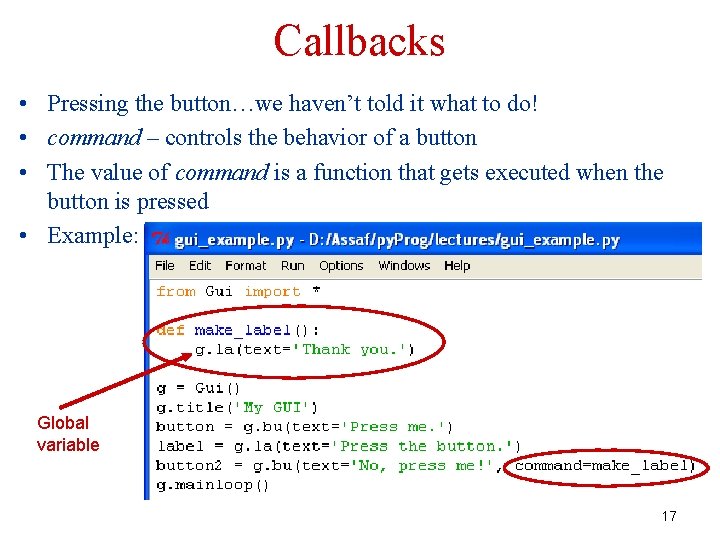
Callbacks • Pressing the button…we haven’t told it what to do! • command – controls the behavior of a button • The value of command is a function that gets executed when the button is pressed • Example: Global variable 17
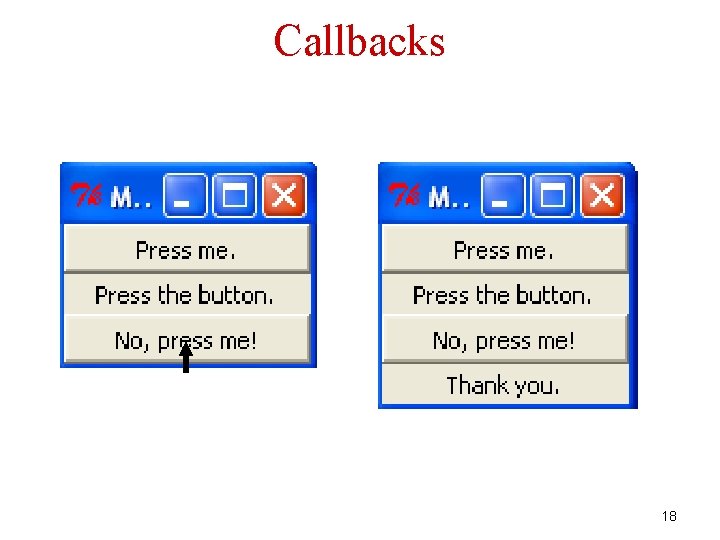
Callbacks 18
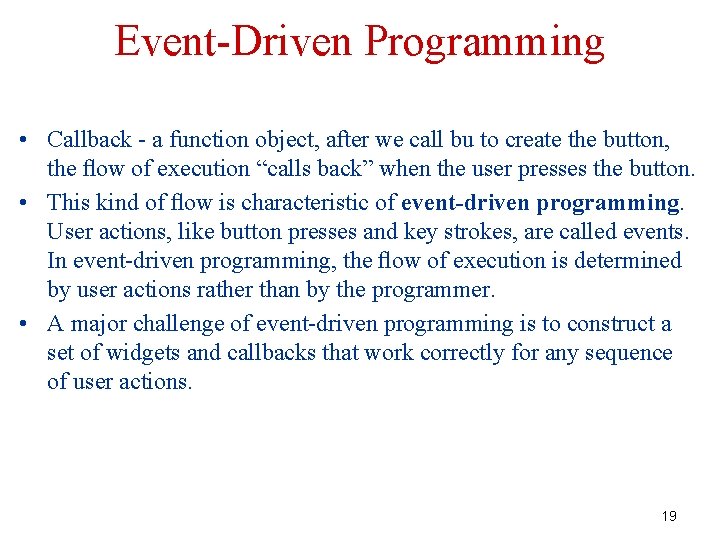
Event-Driven Programming • Callback - a function object, after we call bu to create the button, the flow of execution “calls back” when the user presses the button. • This kind of flow is characteristic of event-driven programming. User actions, like button presses and key strokes, are called events. In event-driven programming, the flow of execution is determined by user actions rather than by the programmer. • A major challenge of event-driven programming is to construct a set of widgets and callbacks that work correctly for any sequence of user actions. 19
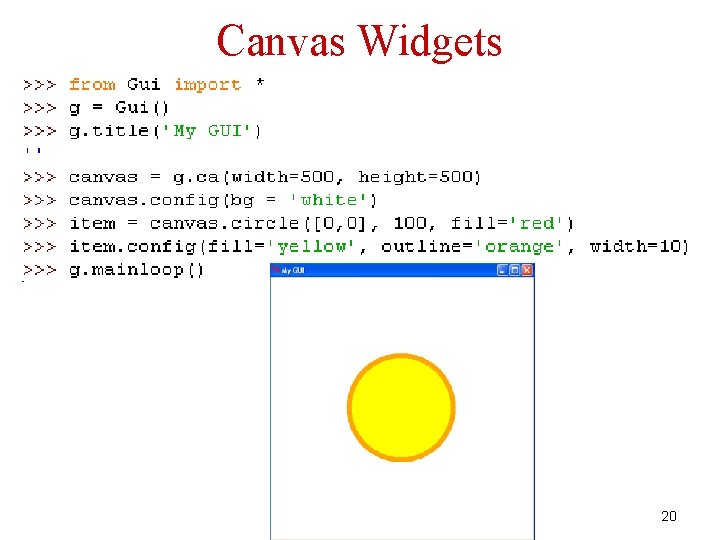
Canvas Widgets 20
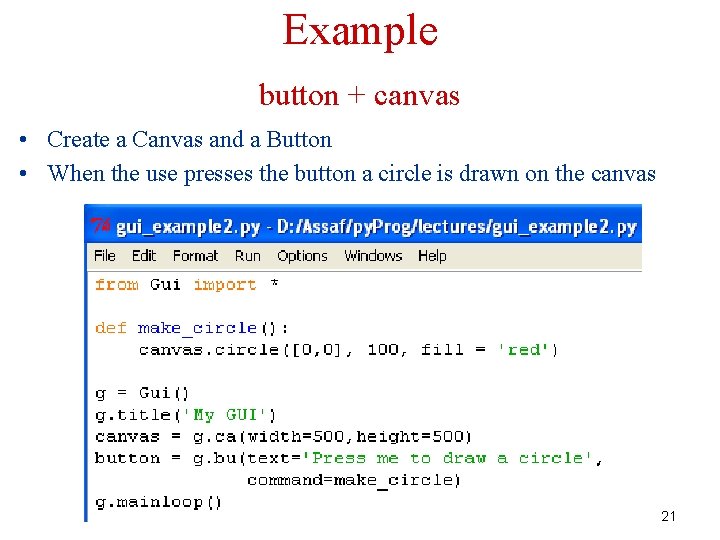
Example button + canvas • Create a Canvas and a Button • When the use presses the button a circle is drawn on the canvas 21
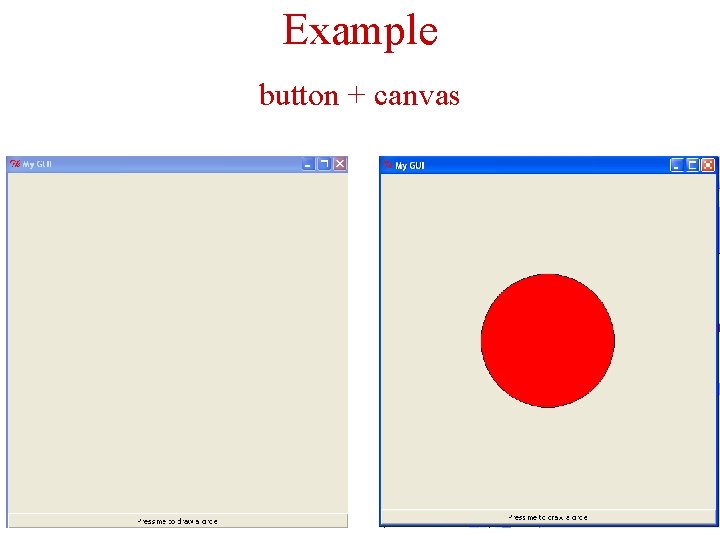
Example button + canvas 22
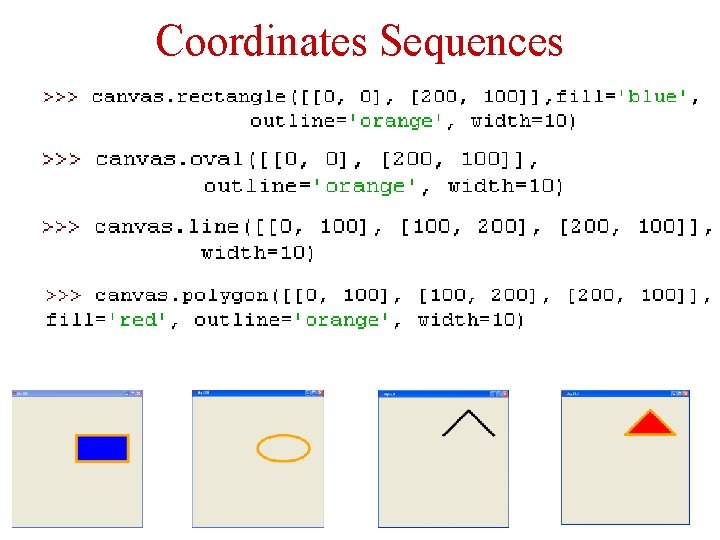
Coordinates Sequences 23
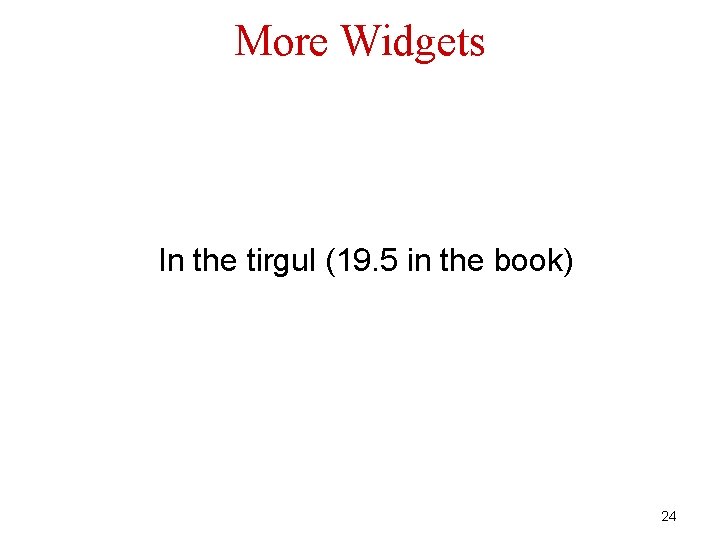
More Widgets In the tirgul (19. 5 in the book) 24
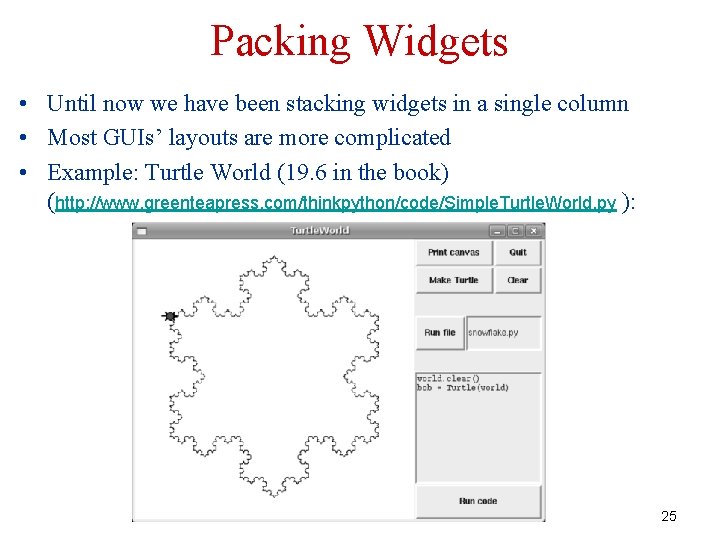
Packing Widgets • Until now we have been stacking widgets in a single column • Most GUIs’ layouts are more complicated • Example: Turtle World (19. 6 in the book) (http: //www. greenteapress. com/thinkpython/code/Simple. Turtle. World. py ): 25
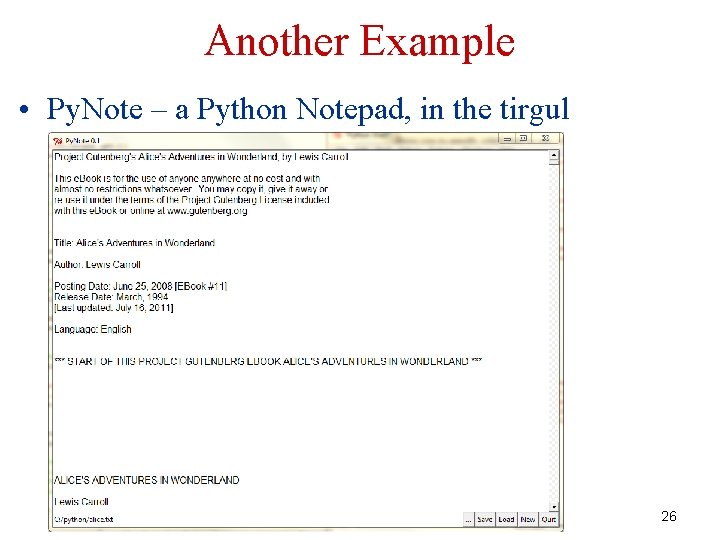
Another Example • Py. Note – a Python Notepad, in the tirgul 26
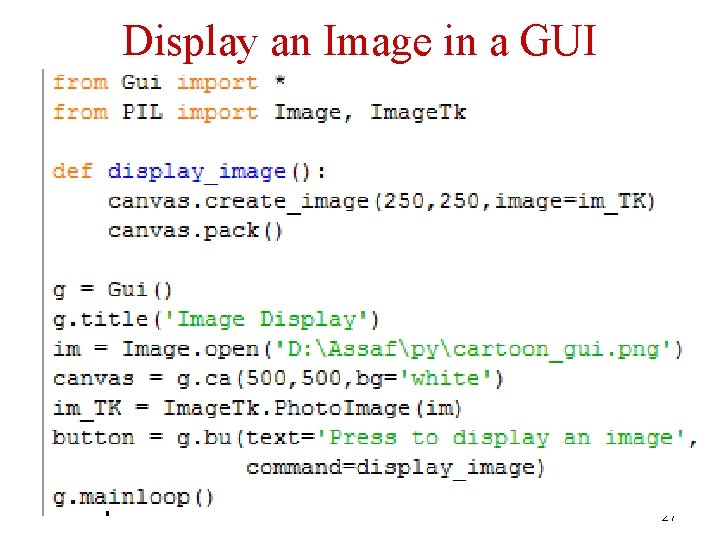
Display an Image in a GUI 27
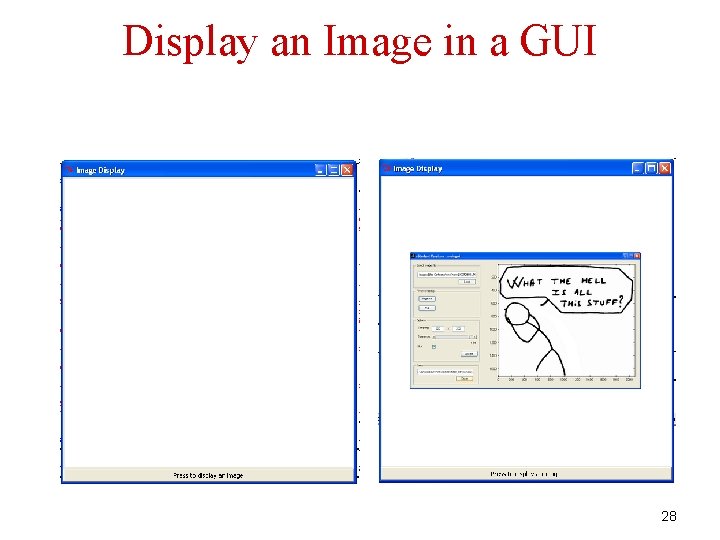
Display an Image in a GUI 28
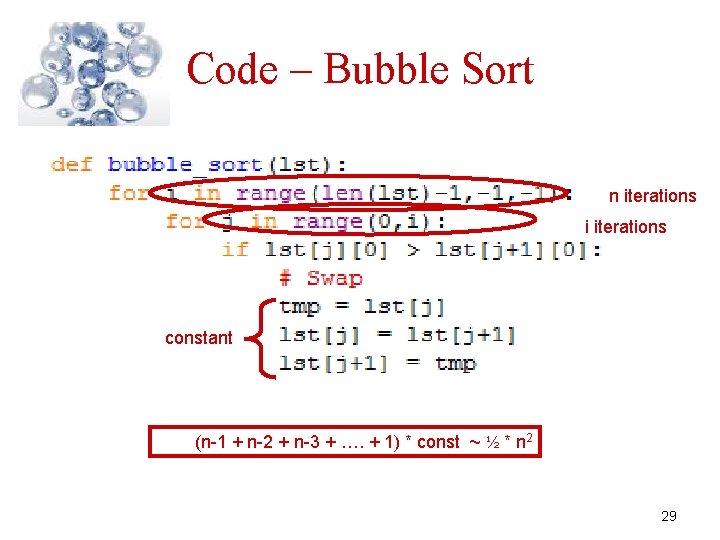
Code – Bubble Sort n iterations i iterations constant (n-1 + n-2 + n-3 + …. + 1) * const ~ ½ * n 2 29
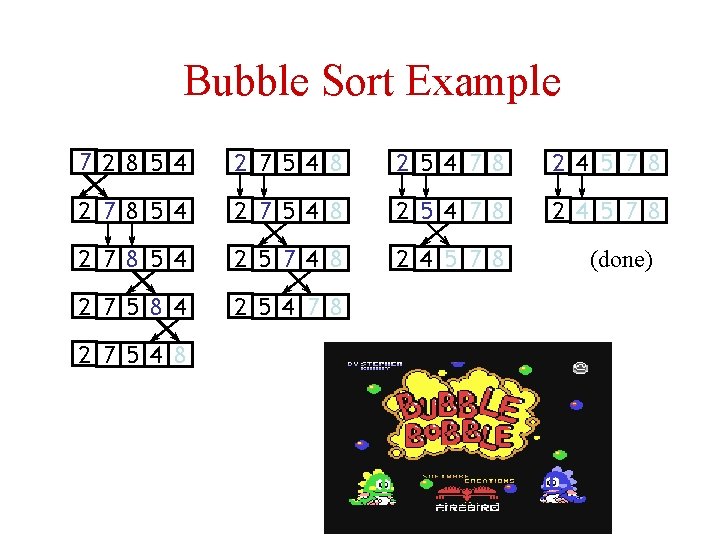
Bubble Sort Example 7 2 8 5 4 2 7 5 4 8 2 5 4 7 8 2 4 5 7 8 2 7 8 5 4 2 5 7 4 8 2 4 5 7 8 2 7 5 8 4 2 5 4 7 8 (done) 2 7 5 4 8 30
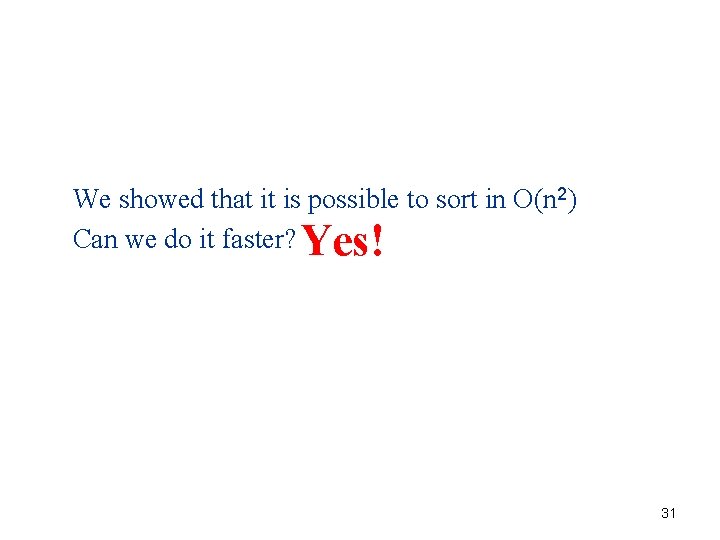
We showed that it is possible to sort in O(n 2) Can we do it faster? Yes! 31
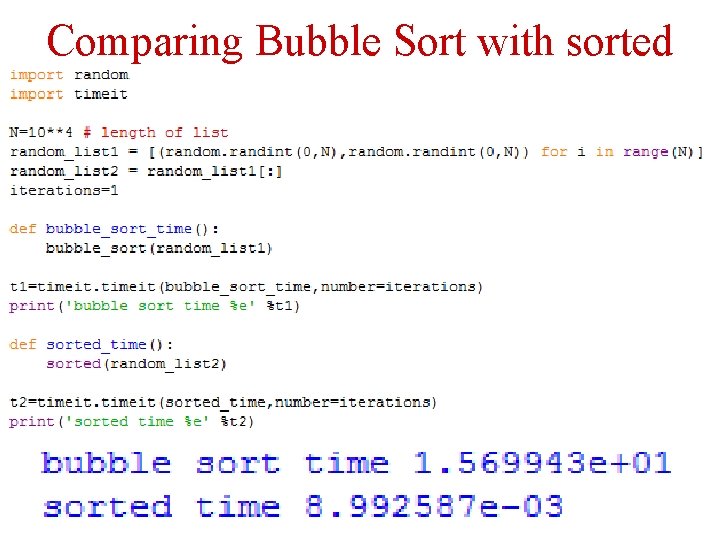
Comparing Bubble Sort with sorted 32
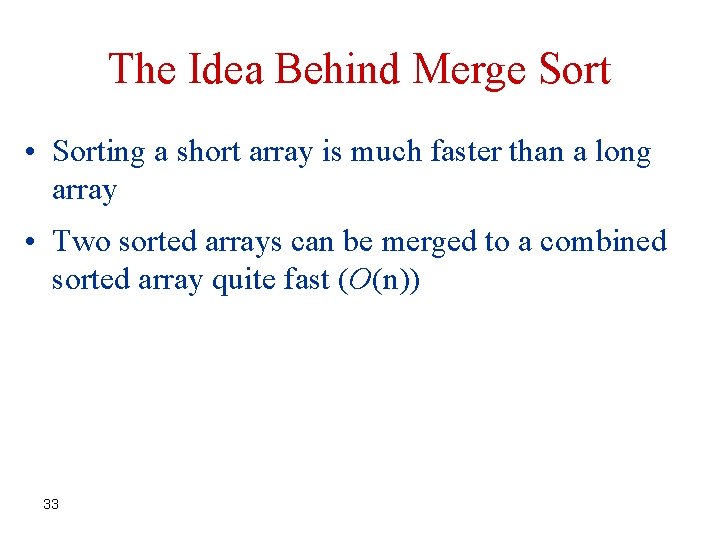
The Idea Behind Merge Sort • Sorting a short array is much faster than a long array • Two sorted arrays can be merged to a combined sorted array quite fast (O(n)) 33
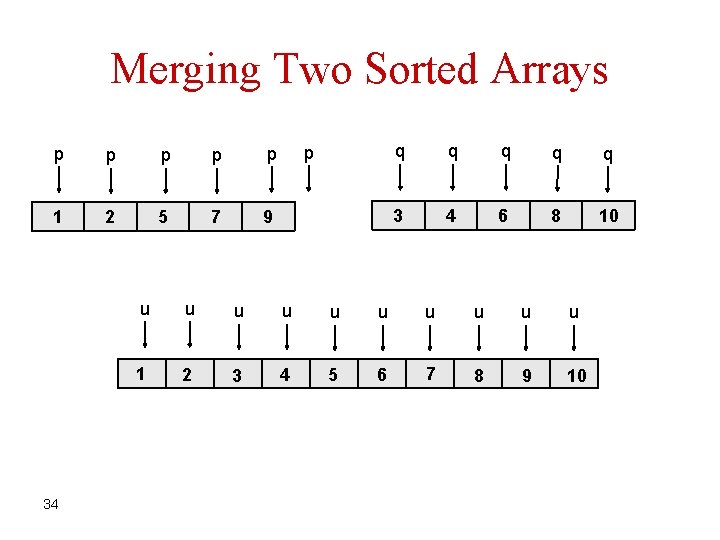
Merging Two Sorted Arrays p p p 1 2 5 7 9 34 p q q q 3 4 6 8 10 u u u u u 1 2 3 4 5 6 7 8 9 10
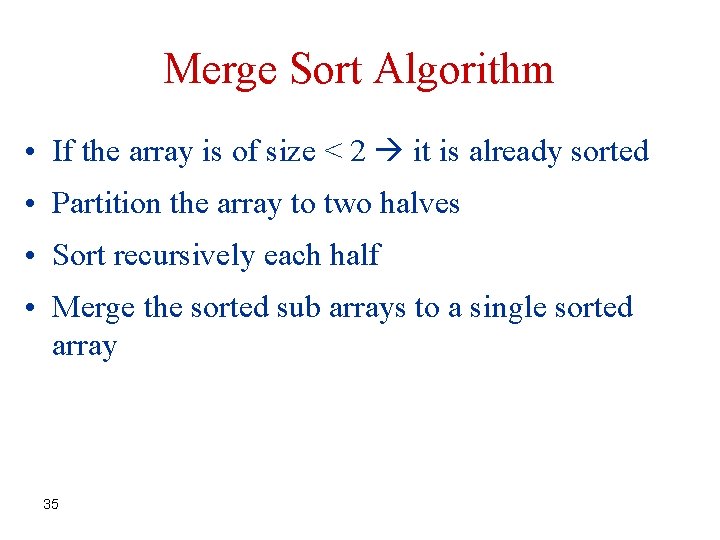
Merge Sort Algorithm • If the array is of size < 2 it is already sorted • Partition the array to two halves • Sort recursively each half • Merge the sorted sub arrays to a single sorted array 35
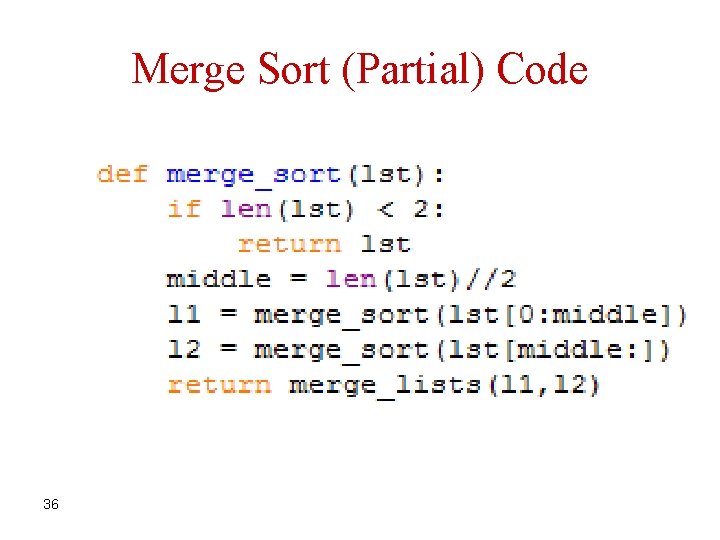
Merge Sort (Partial) Code 36
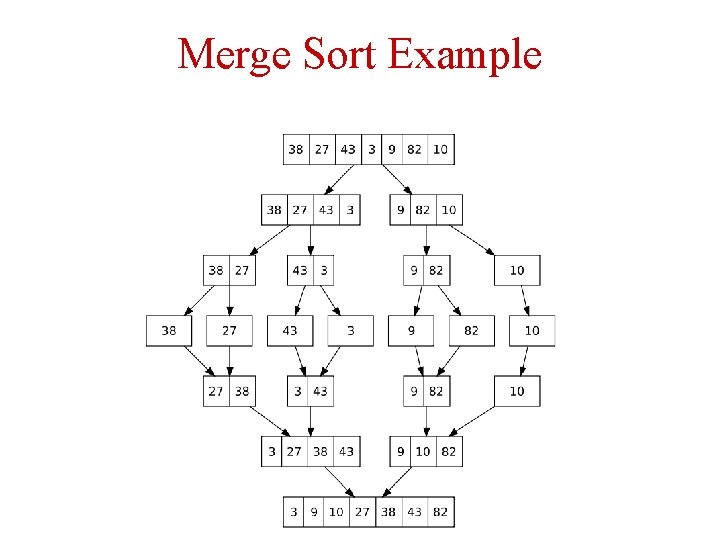
Merge Sort Example 37
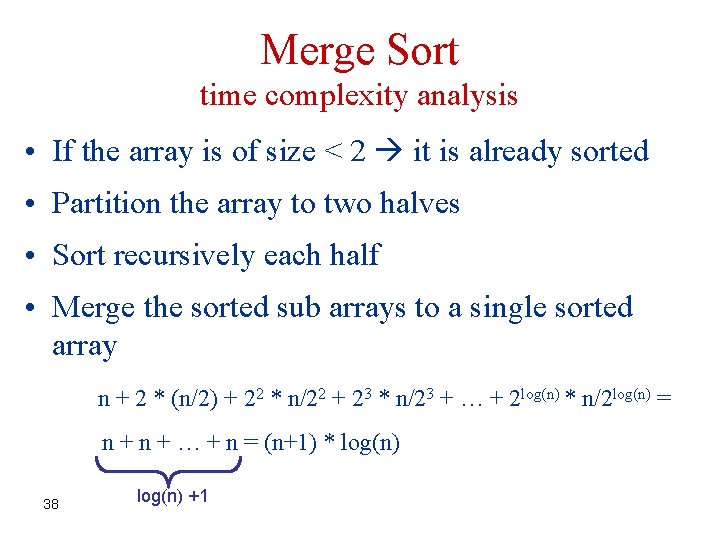
Merge Sort time complexity analysis • If the array is of size < 2 it is already sorted • Partition the array to two halves • Sort recursively each half • Merge the sorted sub arrays to a single sorted array n + 2 * (n/2) + 22 * n/22 + 23 * n/23 + … + 2 log(n) * n/2 log(n) = n + … + n = (n+1) * log(n) 38 log(n) +1
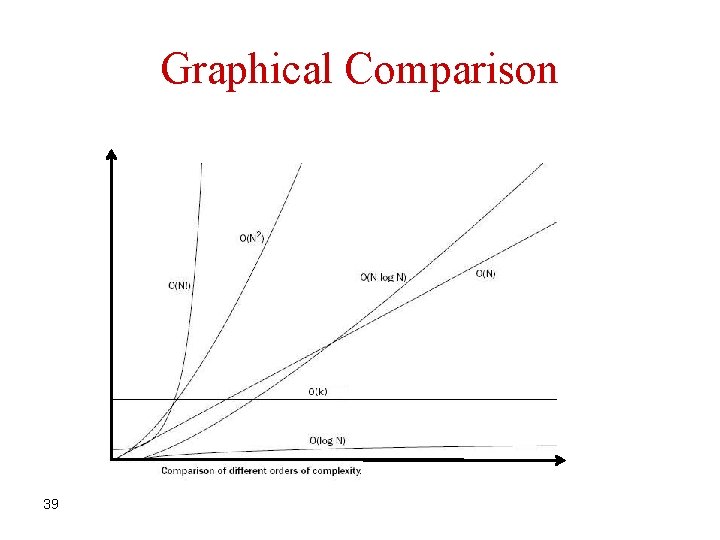
Graphical Comparison 39
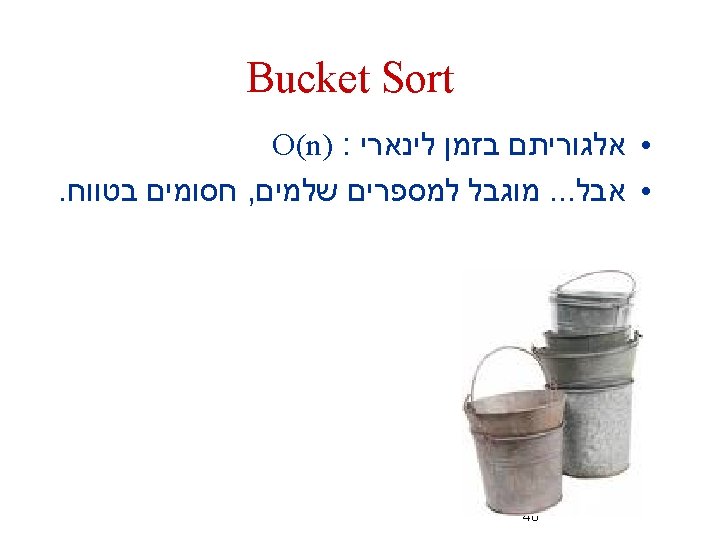
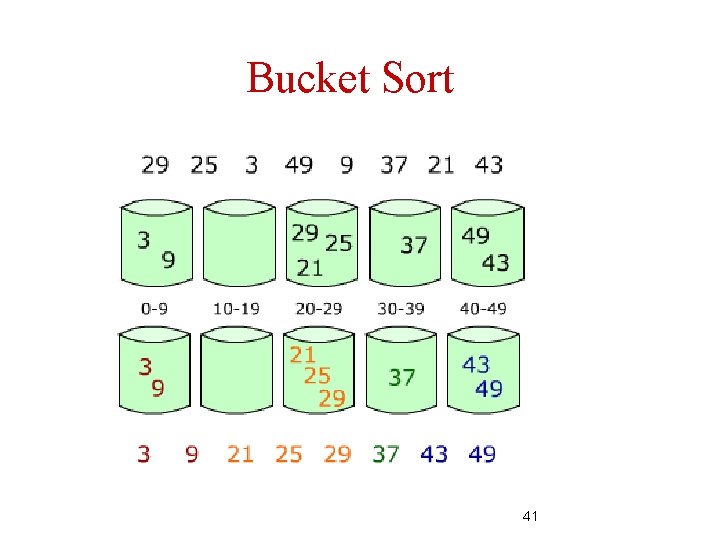
Bucket Sort 41
Rapid gui programming with python and qt
Ge gi gue gui güe güi
Introduction to matlab for engineers
Python coding utf 8
Python user interface design
Interface python 3
Ruby gui toolkit
Qt framework
01:640:244 lecture notes - lecture 15: plat, idah, farad
C data types with examples
Procedural python
Python chapter 5 programming exercises
Second order cone programming python
Python programming in context
Constraint programming python
Python programming in context
Cgi python
Audiolab python
Python programming
Programming essentials in python
Python blackjack oop
Python programming an introduction to computer science
Perbedaan linear programming dan integer programming
Greedy programming vs dynamic programming
Windows 10 system programming, part 1
Linear vs integer programming
Definisi linear
Formuö
Typiska novell drag
Nationell inriktning för artificiell intelligens
Vad står k.r.å.k.a.n för
Shingelfrisyren
En lathund för arbete med kontinuitetshantering
Adressändring ideell förening
Tidbok
Anatomi organ reproduksi
Vad är densitet
Datorkunskap för nybörjare
Stig kerman
Att skriva debattartikel
Autokratiskt ledarskap
Nyckelkompetenser för livslångt lärande