Chapter2 Java Programming COMP417 GUI Components GUI COMPONENTS
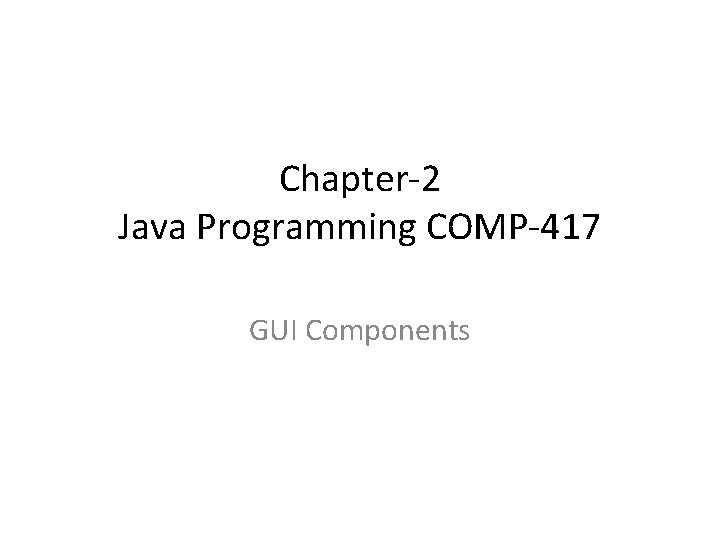
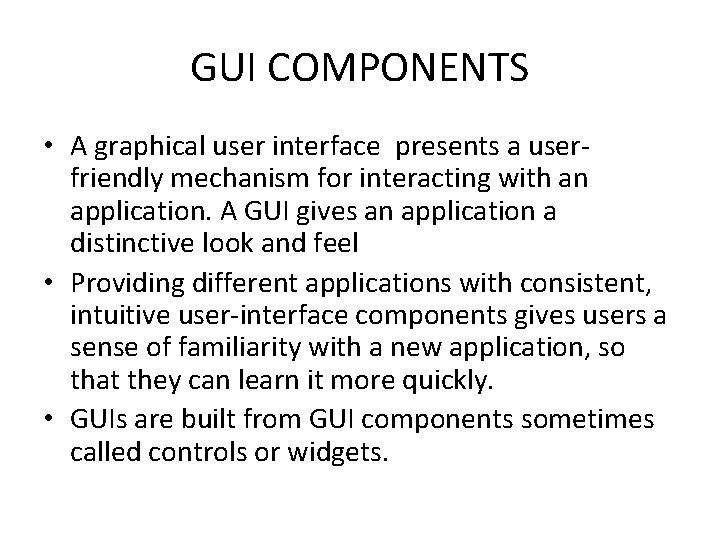
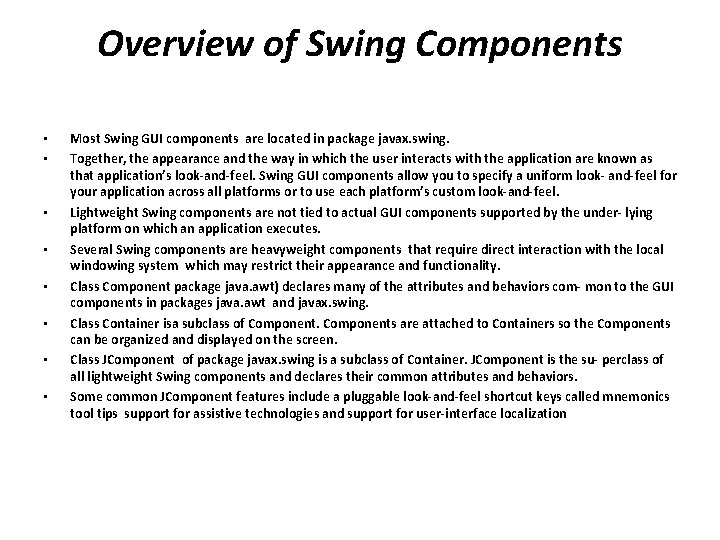
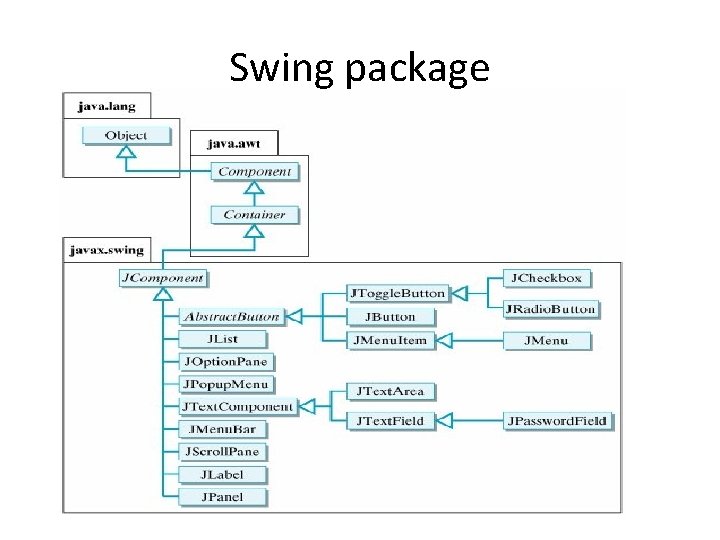
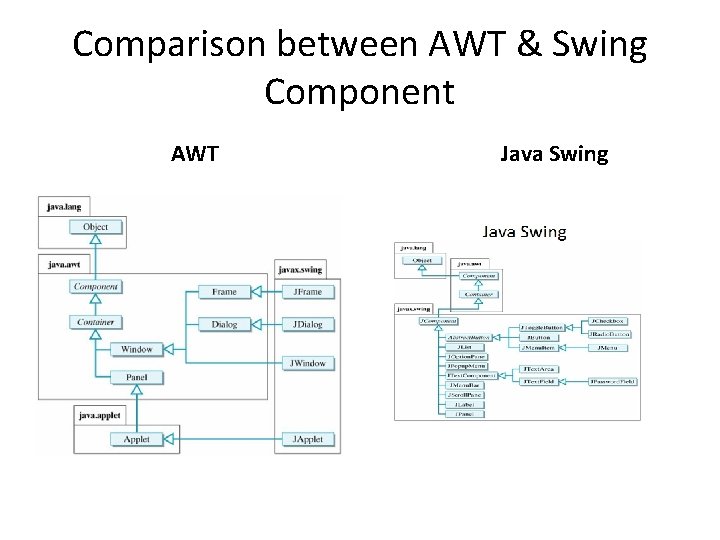
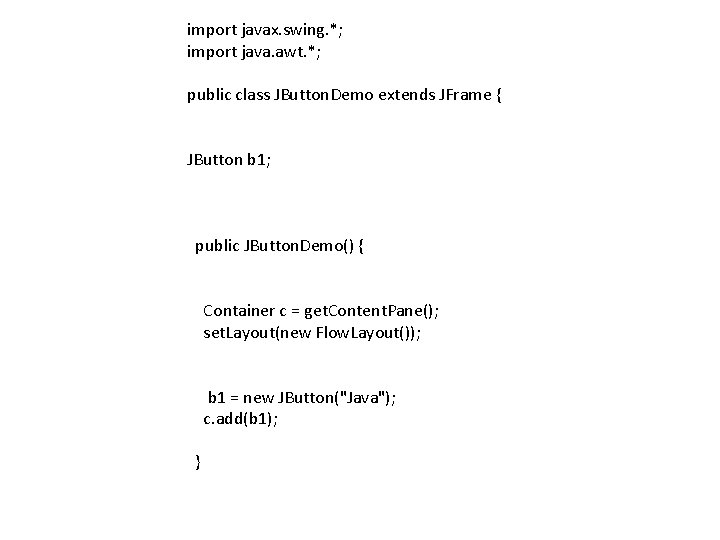
![public static void main(String[] args) { JButton. Demo b= new JButton. Demo(); b. set. public static void main(String[] args) { JButton. Demo b= new JButton. Demo(); b. set.](https://slidetodoc.com/presentation_image_h2/10d15b7efb15c98e75fb318ecd28e188/image-7.jpg)
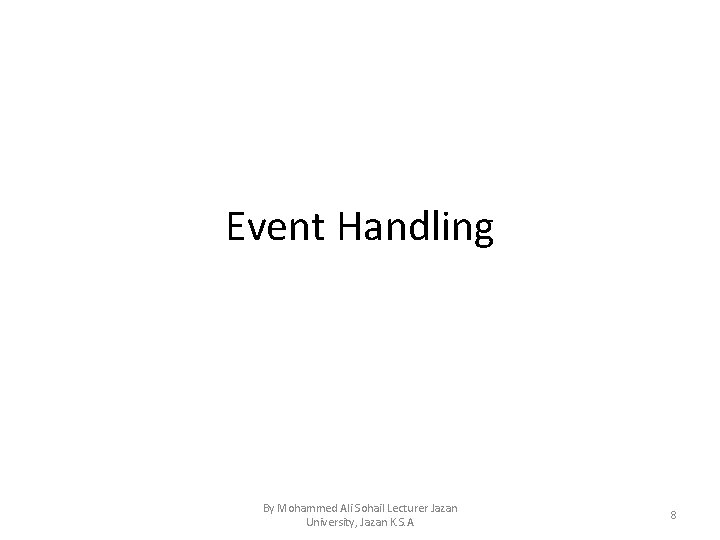
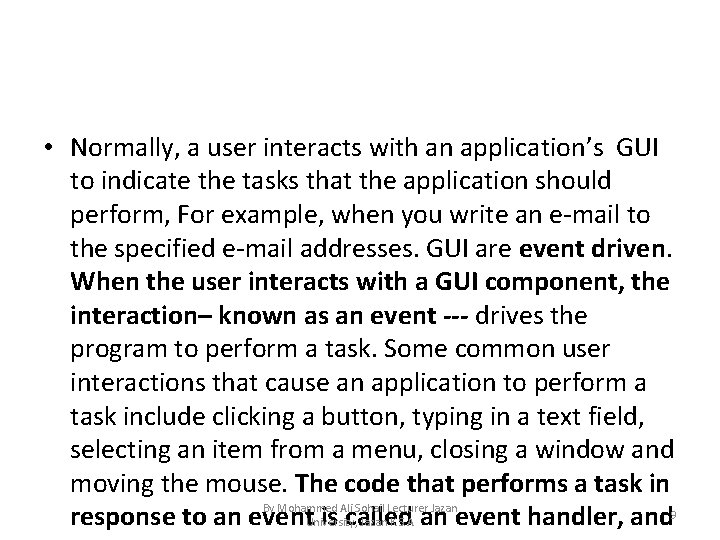
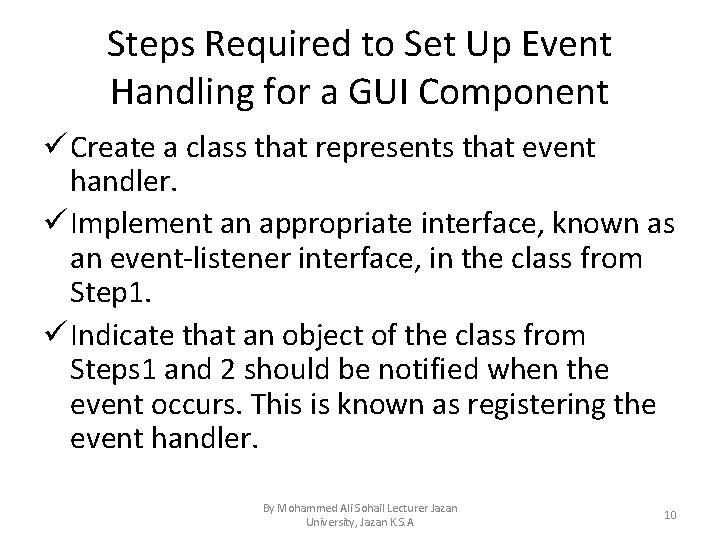
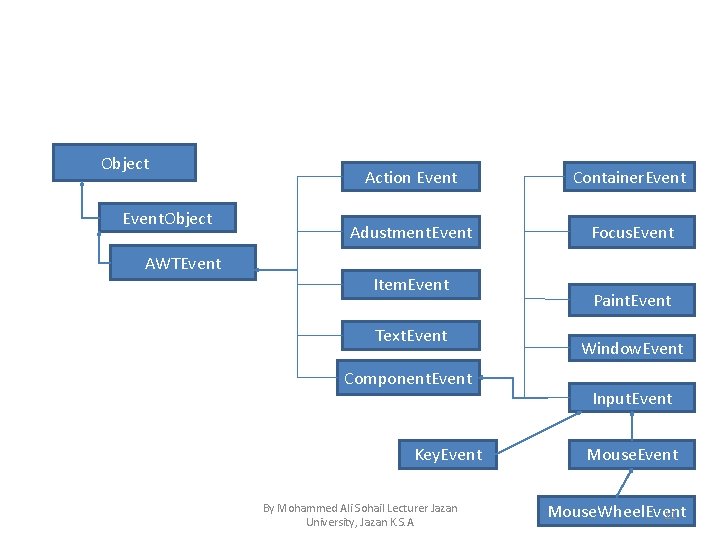
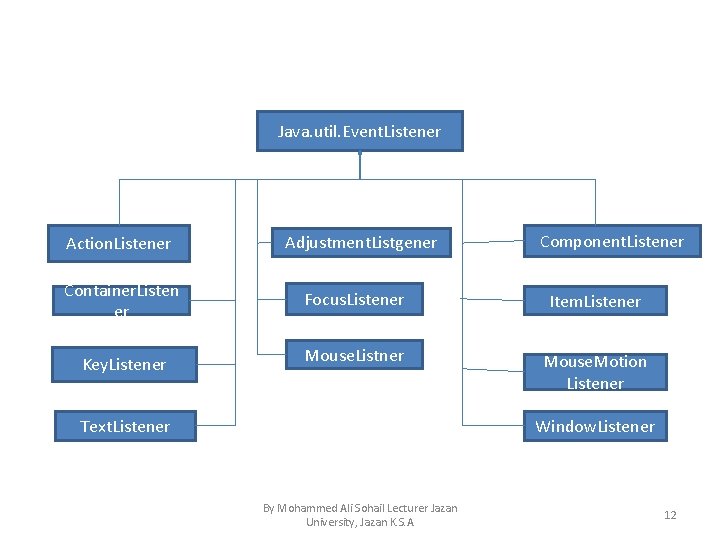
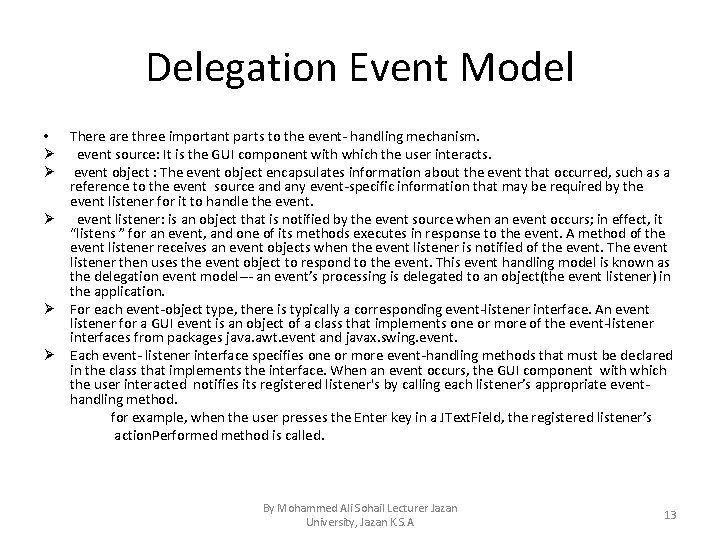
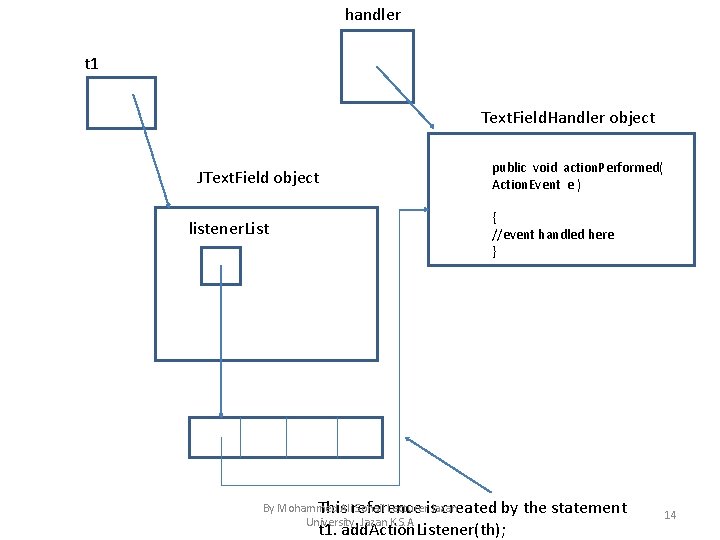
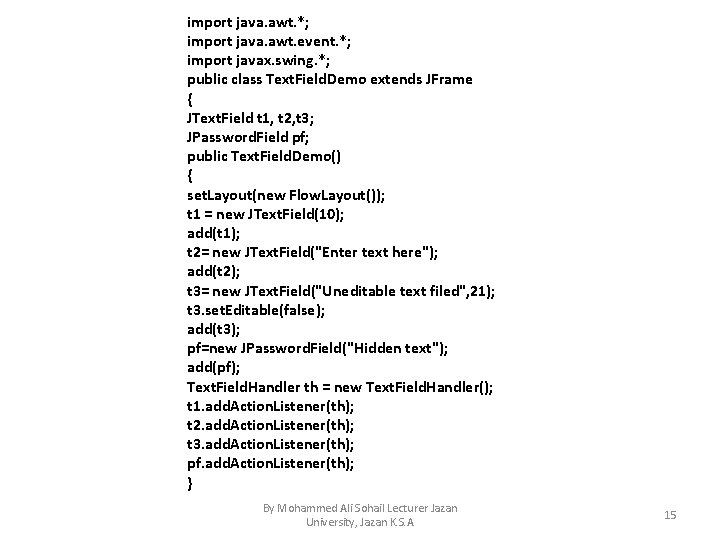
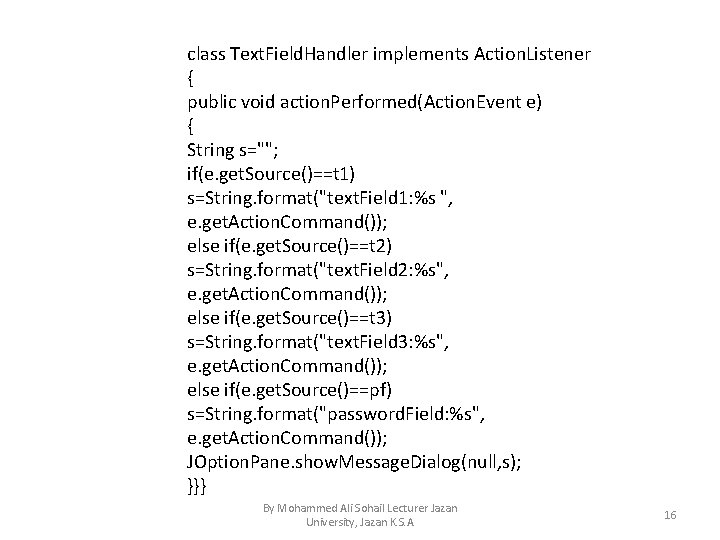
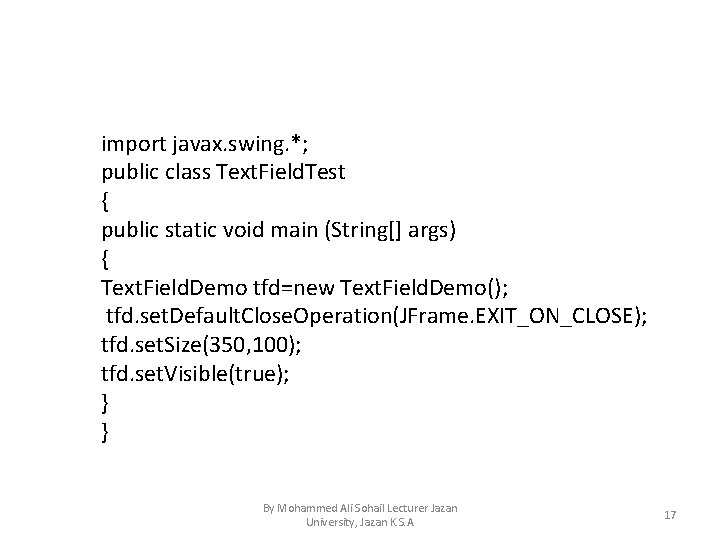
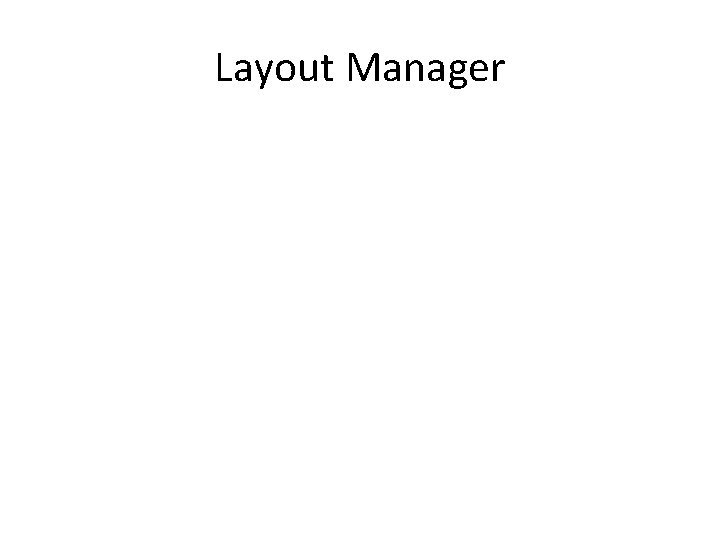
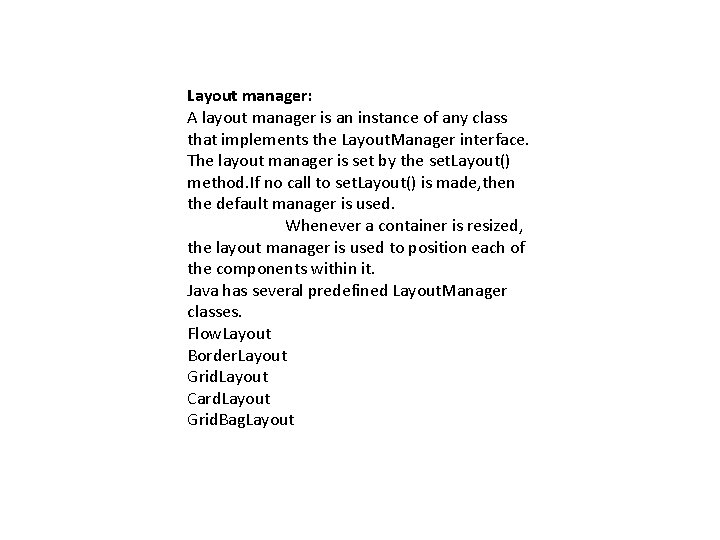
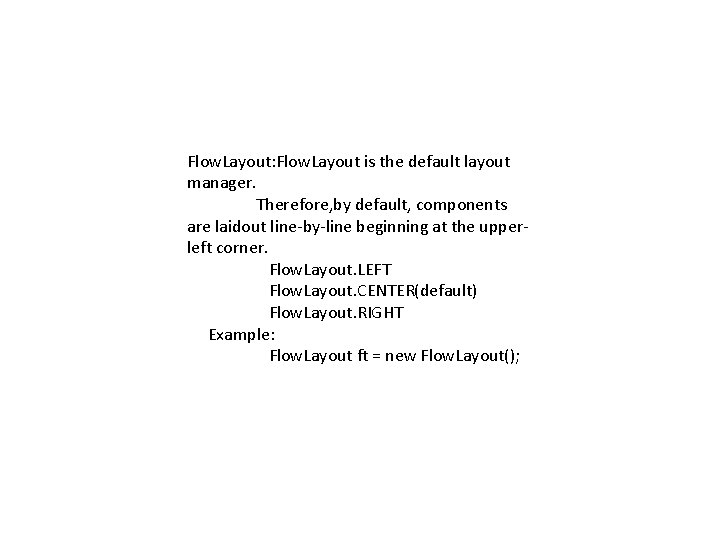
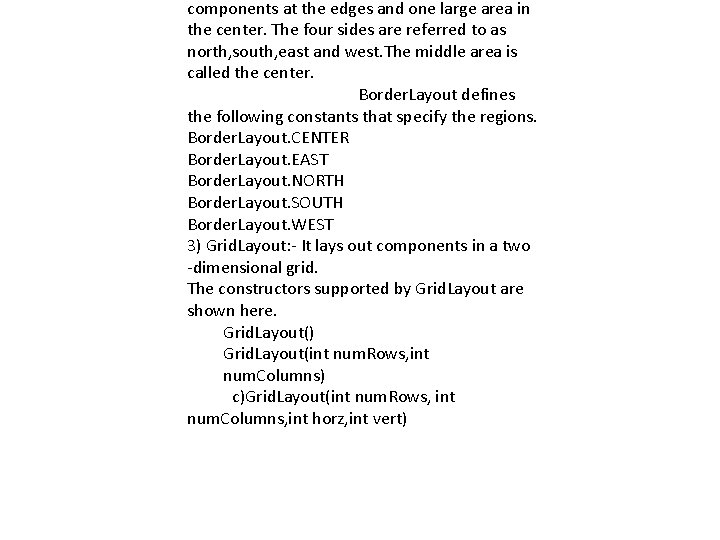
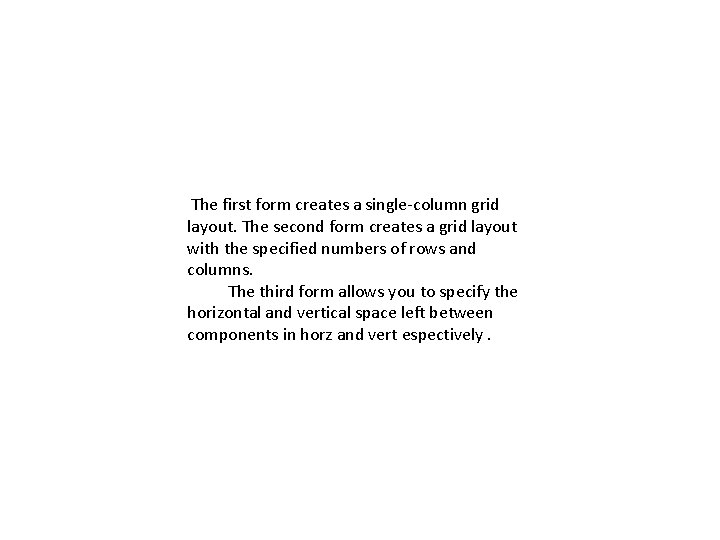
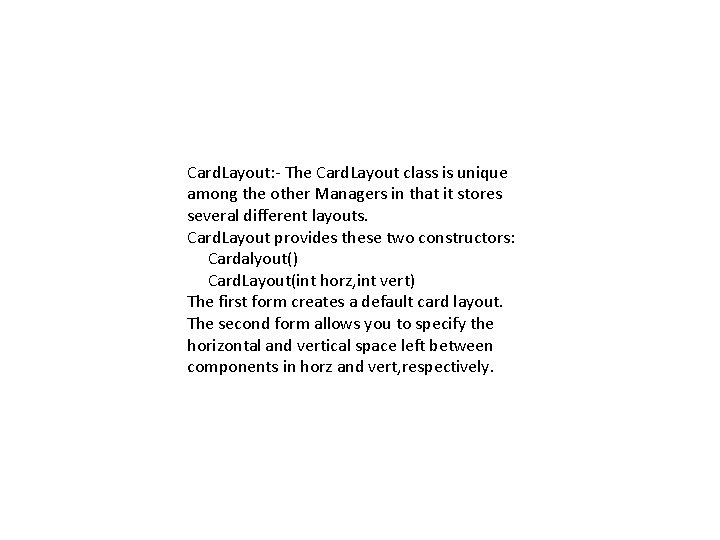
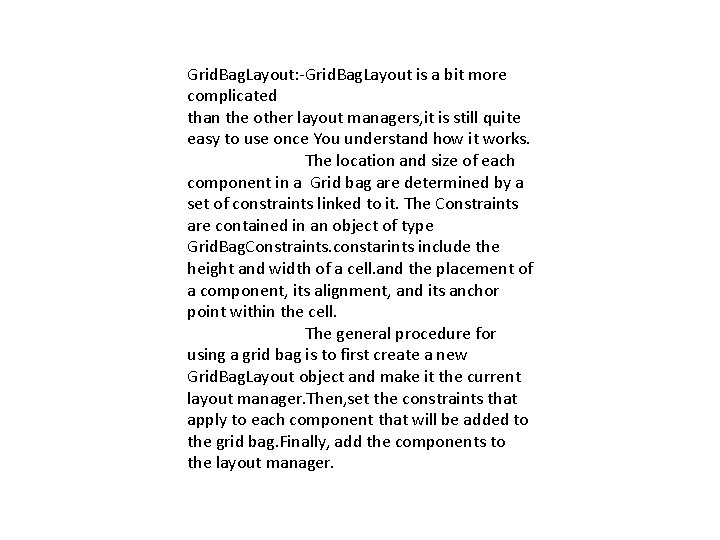
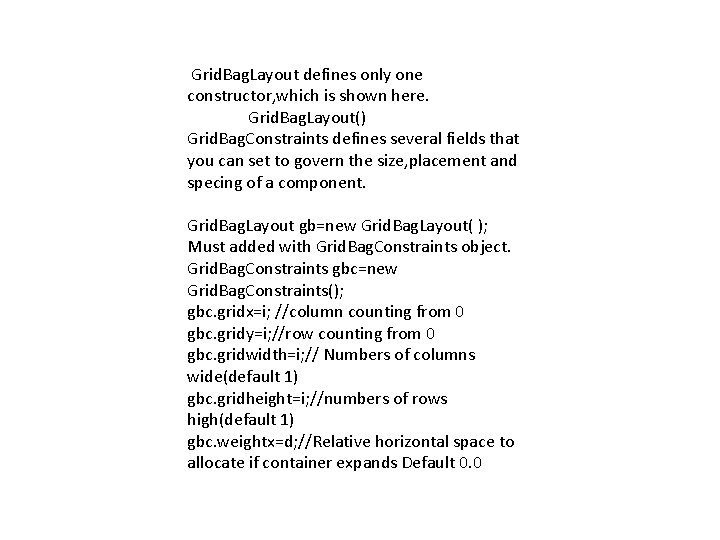
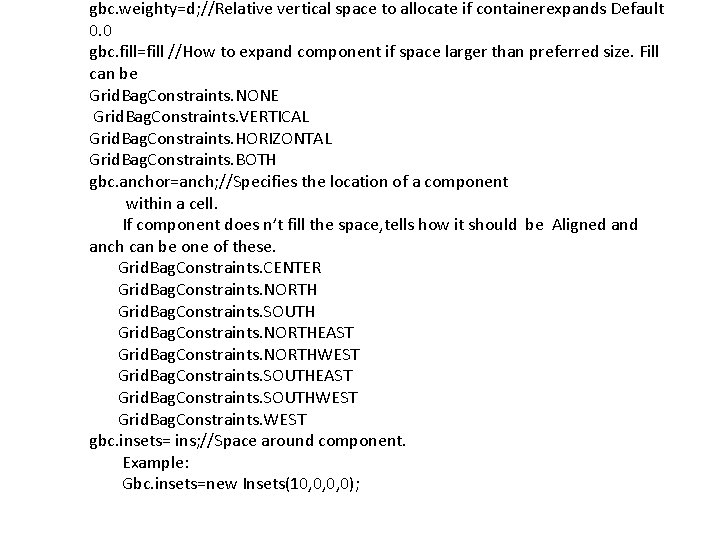
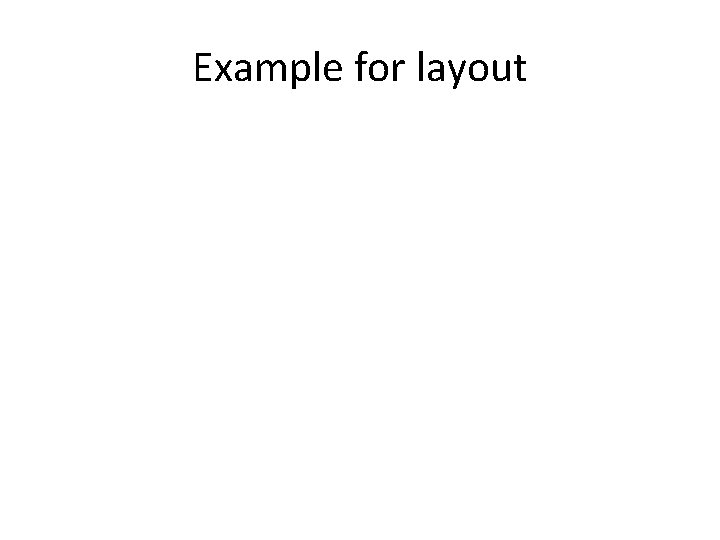
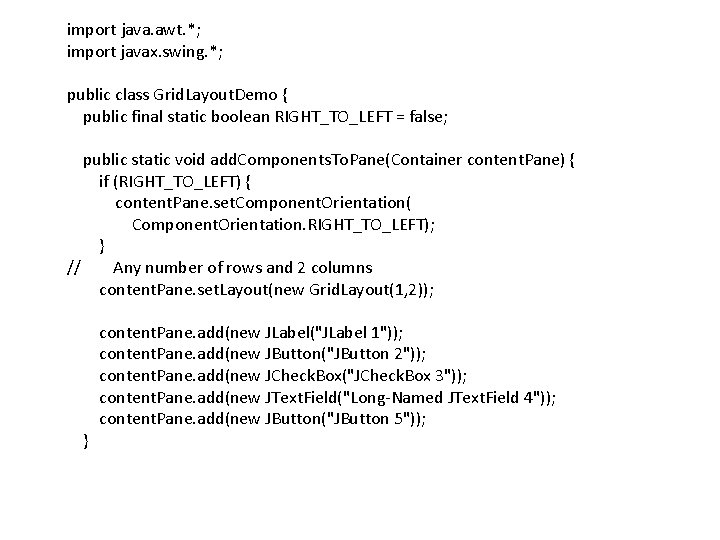
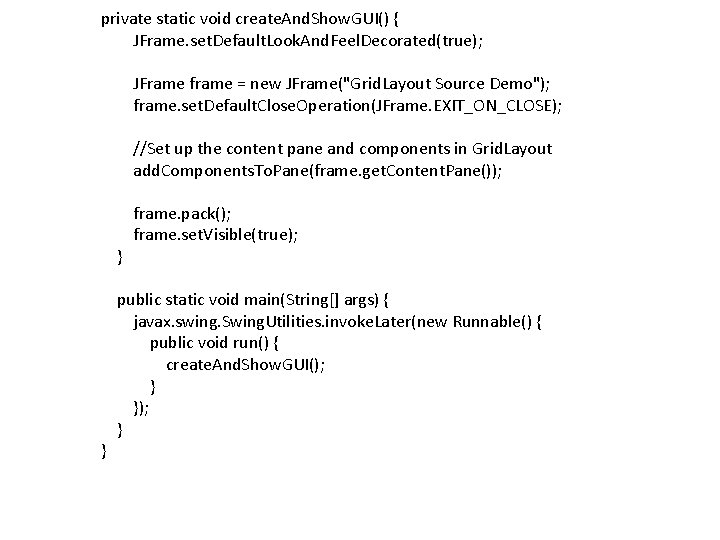
- Slides: 29
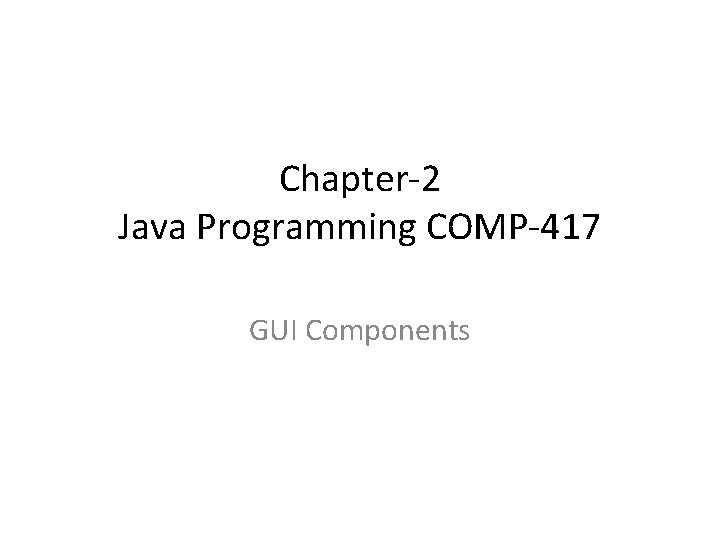
Chapter-2 Java Programming COMP-417 GUI Components
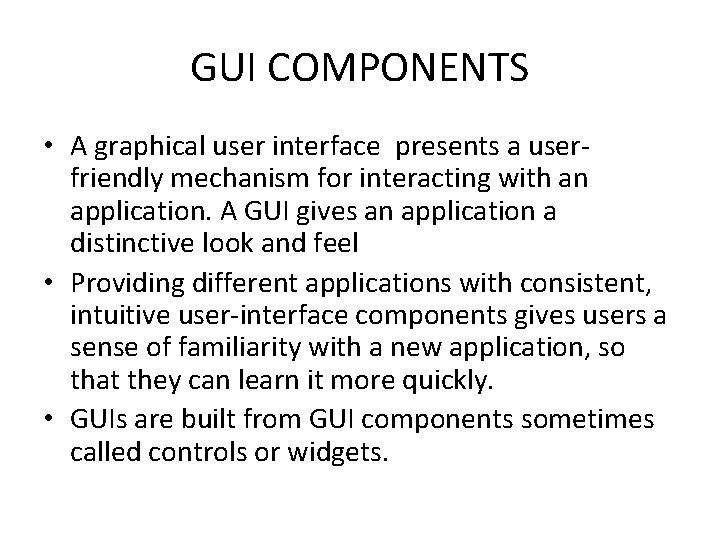
GUI COMPONENTS • A graphical user interface presents a userfriendly mechanism for interacting with an application. A GUI gives an application a distinctive look and feel • Providing different applications with consistent, intuitive user-interface components gives users a sense of familiarity with a new application, so that they can learn it more quickly. • GUIs are built from GUI components sometimes called controls or widgets.
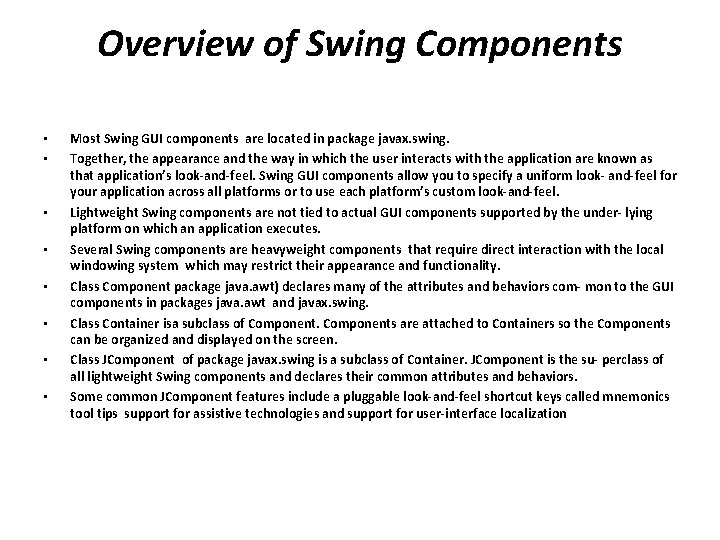
Overview of Swing Components • • Most Swing GUI components are located in package javax. swing. Together, the appearance and the way in which the user interacts with the application are known as that application’s look-and-feel. Swing GUI components allow you to specify a uniform look- and-feel for your application across all platforms or to use each platform’s custom look-and-feel. Lightweight Swing components are not tied to actual GUI components supported by the under- lying platform on which an application executes. Several Swing components are heavyweight components that require direct interaction with the local windowing system which may restrict their appearance and functionality. Class Component package java. awt) declares many of the attributes and behaviors com- mon to the GUI components in packages java. awt and javax. swing. Class Container isa subclass of Components are attached to Containers so the Components can be organized and displayed on the screen. Class JComponent of package javax. swing is a subclass of Container. JComponent is the su- perclass of all lightweight Swing components and declares their common attributes and behaviors. Some common JComponent features include a pluggable look-and-feel shortcut keys called mnemonics tool tips support for assistive technologies and support for user-interface localization
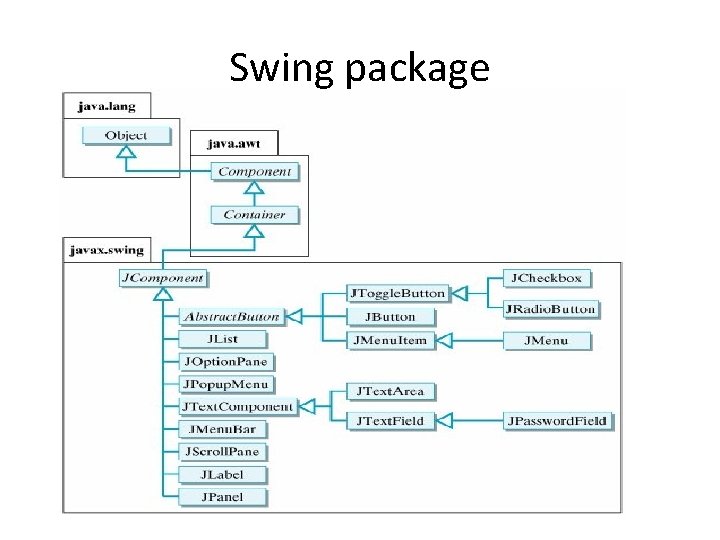
Swing package Overview of Swing Components
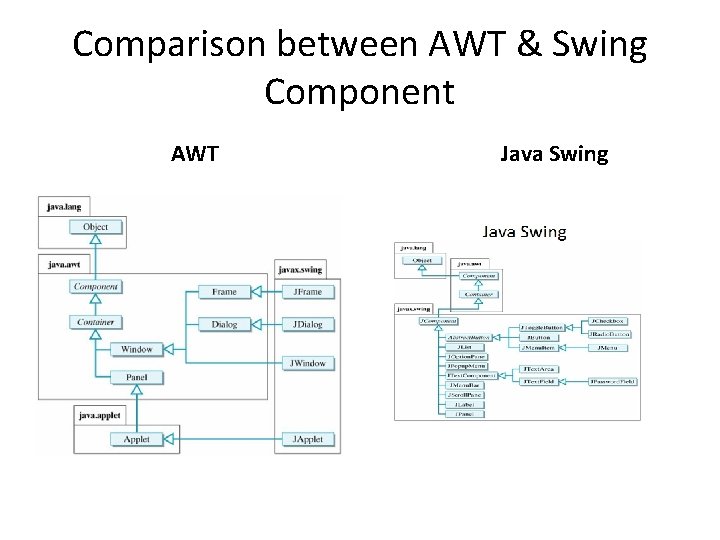
Comparison between AWT & Swing Component AWT Java Swing
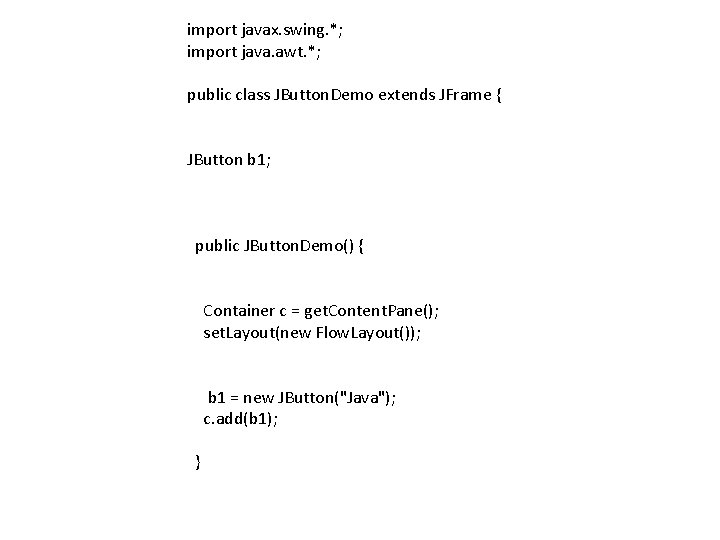
import javax. swing. *; import java. awt. *; public class JButton. Demo extends JFrame { JButton b 1; public JButton. Demo() { Container c = get. Content. Pane(); set. Layout(new Flow. Layout()); b 1 = new JButton("Java"); c. add(b 1); }
![public static void mainString args JButton Demo b new JButton Demo b set public static void main(String[] args) { JButton. Demo b= new JButton. Demo(); b. set.](https://slidetodoc.com/presentation_image_h2/10d15b7efb15c98e75fb318ecd28e188/image-7.jpg)
public static void main(String[] args) { JButton. Demo b= new JButton. Demo(); b. set. Size(275, 110); b. set. Visible(true); b. set. Default. Close. Operation(JFrame. EXIT_ON_CLOSE); } }
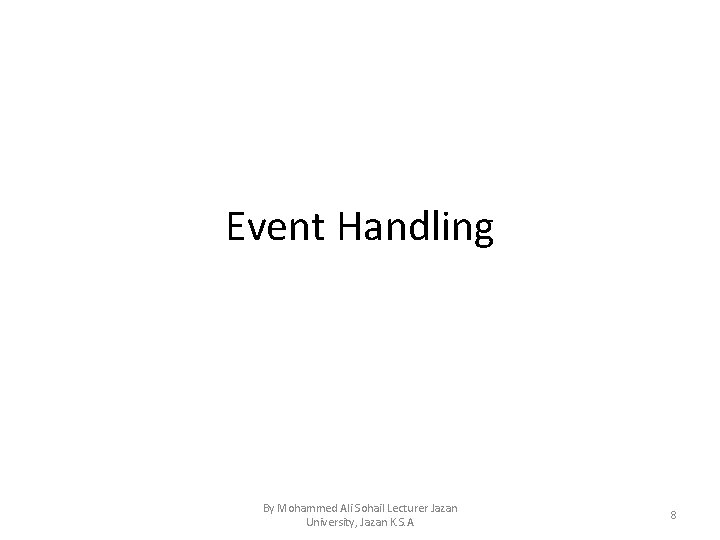
Event Handling By Mohammed Ali Sohail Lecturer Jazan University, Jazan K. S. A 8
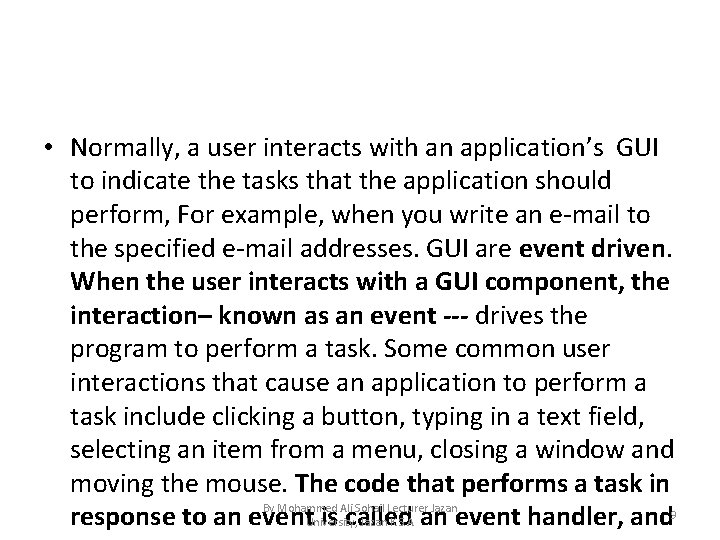
• Normally, a user interacts with an application’s GUI to indicate the tasks that the application should perform, For example, when you write an e-mail to the specified e-mail addresses. GUI are event driven. When the user interacts with a GUI component, the interaction– known as an event --- drives the program to perform a task. Some common user interactions that cause an application to perform a task include clicking a button, typing in a text field, selecting an item from a menu, closing a window and moving the mouse. The code that performs a task in By Mohammed Ali Sohail Lecturer Jazan 9 response to an event. University, is called Jazan K. S. A an event handler, and
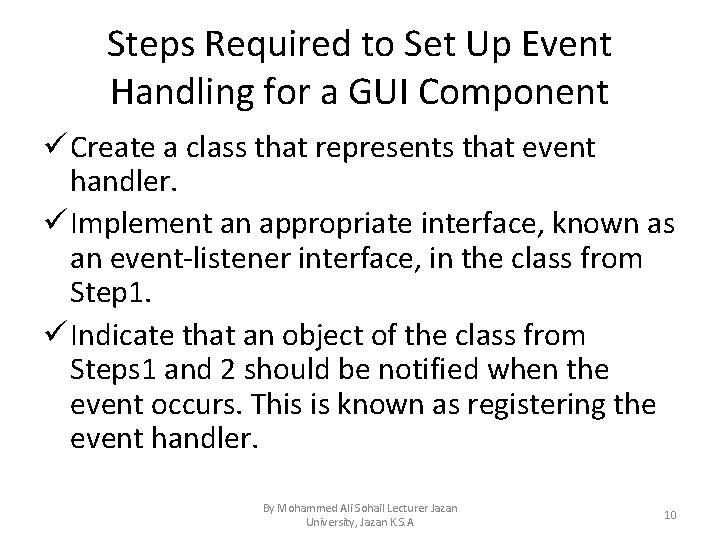
Steps Required to Set Up Event Handling for a GUI Component ü Create a class that represents that event handler. ü Implement an appropriate interface, known as an event-listener interface, in the class from Step 1. ü Indicate that an object of the class from Steps 1 and 2 should be notified when the event occurs. This is known as registering the event handler. By Mohammed Ali Sohail Lecturer Jazan University, Jazan K. S. A 10
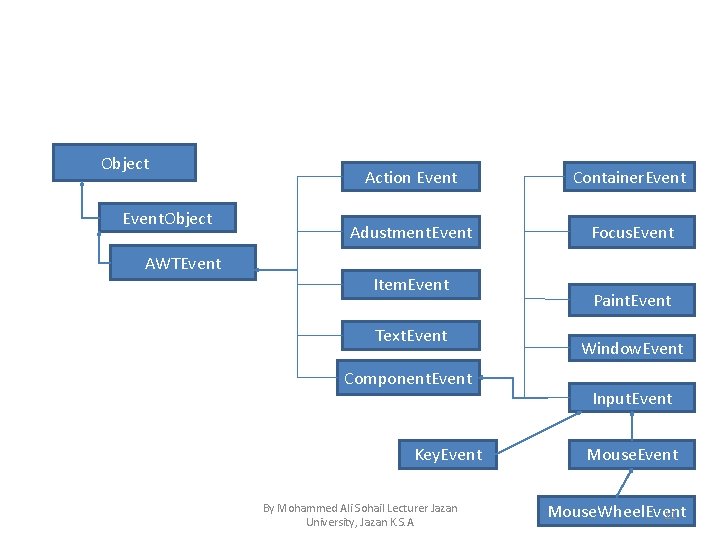
Object Event. Object AWTEvent Action Event Container. Event Adustment. Event Focus. Event Item. Event Text. Event Component. Event Key. Event By Mohammed Ali Sohail Lecturer Jazan University, Jazan K. S. A Paint. Event Window. Event Input. Event Mouse. Wheel. Event 11
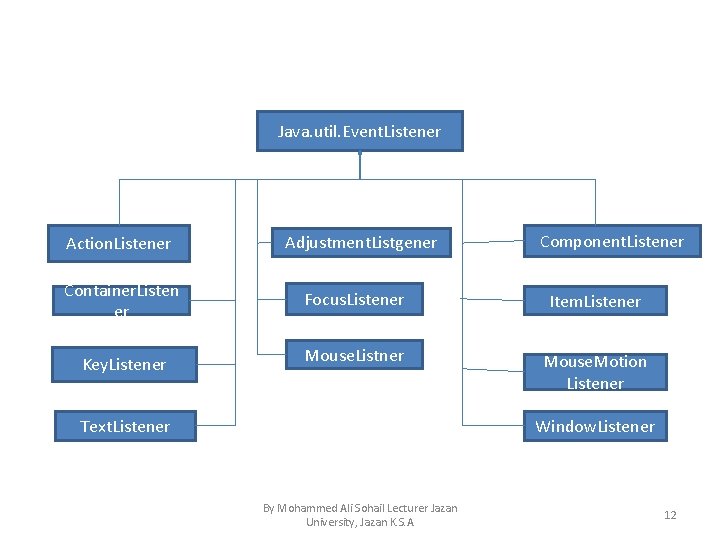
Java. util. Event. Listener Action. Listener Container. Listen er Key. Listener Adjustment. Listgener Component. Listener Focus. Listener Item. Listener Mouse. Listner Mouse. Motion Listener Text. Listener Window. Listener By Mohammed Ali Sohail Lecturer Jazan University, Jazan K. S. A 12
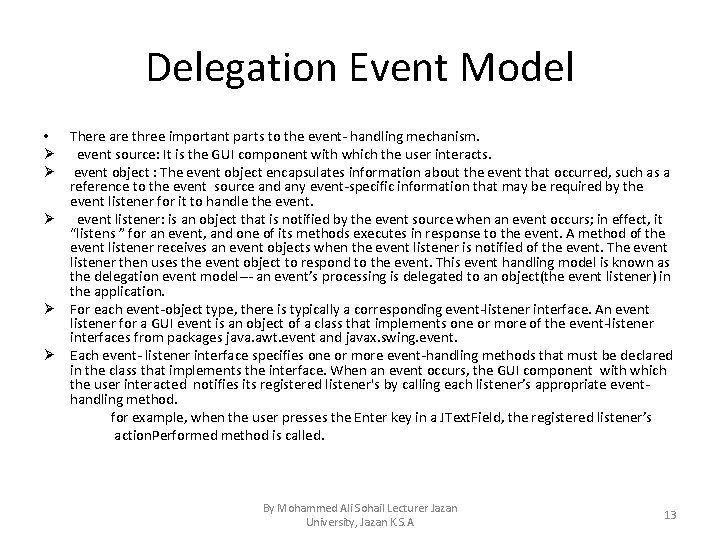
Delegation Event Model • There are three important parts to the event- handling mechanism. Ø event source: It is the GUI component with which the user interacts. Ø event object : The event object encapsulates information about the event that occurred, such as a reference to the event source and any event-specific information that may be required by the event listener for it to handle the event. Ø event listener: is an object that is notified by the event source when an event occurs; in effect, it “listens ” for an event, and one of its methods executes in response to the event. A method of the event listener receives an event objects when the event listener is notified of the event. The event listener then uses the event object to respond to the event. This event handling model is known as the delegation event model--- an event’s processing is delegated to an object(the event listener) in the application. Ø For each event-object type, there is typically a corresponding event-listener interface. An event listener for a GUI event is an object of a class that implements one or more of the event-listener interfaces from packages java. awt. event and javax. swing. event. Ø Each event- listener interface specifies one or more event-handling methods that must be declared in the class that implements the interface. When an event occurs, the GUI component with which the user interacted notifies its registered listener's by calling each listener’s appropriate eventhandling method. for example, when the user presses the Enter key in a JText. Field, the registered listener’s action. Performed method is called. By Mohammed Ali Sohail Lecturer Jazan University, Jazan K. S. A 13
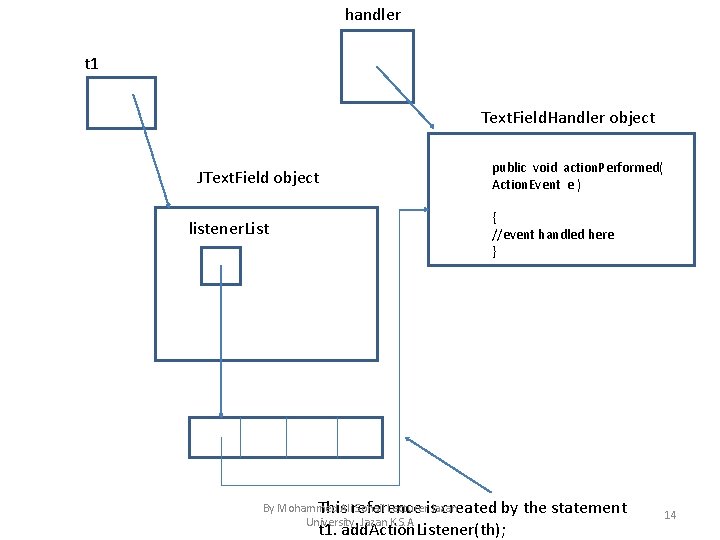
handler t 1 Text. Field. Handler object JText. Field object listener. List public void action. Performed( Action. Event e ) { //event handled here } By Mohammed Sohail Lectureris Jazan This. Alireference created University, Jazan K. S. A by the statement t 1. add. Action. Listener(th); 14
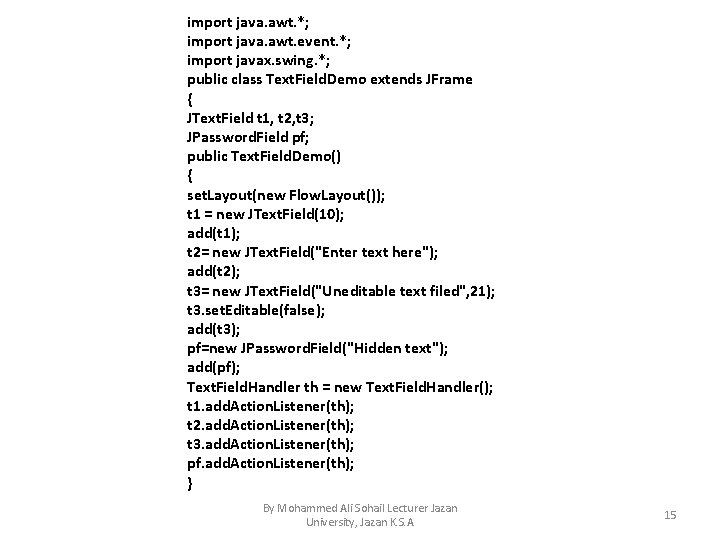
import java. awt. *; import java. awt. event. *; import javax. swing. *; public class Text. Field. Demo extends JFrame { JText. Field t 1, t 2, t 3; JPassword. Field pf; public Text. Field. Demo() { set. Layout(new Flow. Layout()); t 1 = new JText. Field(10); add(t 1); t 2= new JText. Field("Enter text here"); add(t 2); t 3= new JText. Field("Uneditable text filed", 21); t 3. set. Editable(false); add(t 3); pf=new JPassword. Field("Hidden text"); add(pf); Text. Field. Handler th = new Text. Field. Handler(); t 1. add. Action. Listener(th); t 2. add. Action. Listener(th); t 3. add. Action. Listener(th); pf. add. Action. Listener(th); } By Mohammed Ali Sohail Lecturer Jazan University, Jazan K. S. A 15
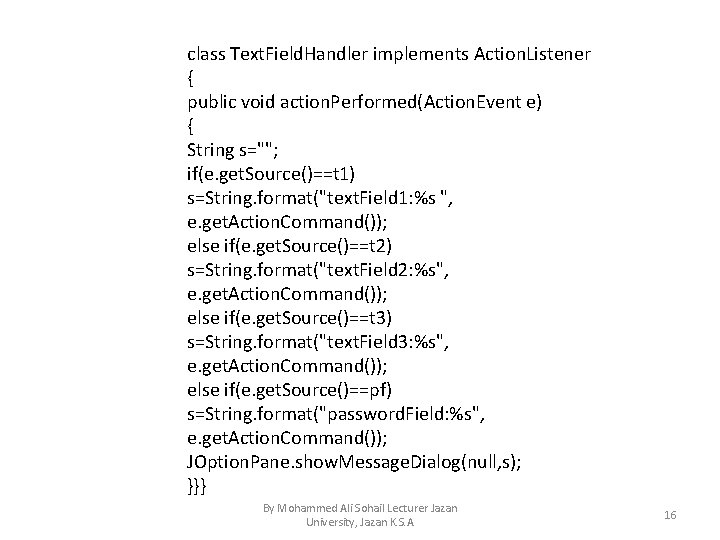
class Text. Field. Handler implements Action. Listener { public void action. Performed(Action. Event e) { String s=""; if(e. get. Source()==t 1) s=String. format("text. Field 1: %s ", e. get. Action. Command()); else if(e. get. Source()==t 2) s=String. format("text. Field 2: %s", e. get. Action. Command()); else if(e. get. Source()==t 3) s=String. format("text. Field 3: %s", e. get. Action. Command()); else if(e. get. Source()==pf) s=String. format("password. Field: %s", e. get. Action. Command()); JOption. Pane. show. Message. Dialog(null, s); }}} By Mohammed Ali Sohail Lecturer Jazan University, Jazan K. S. A 16
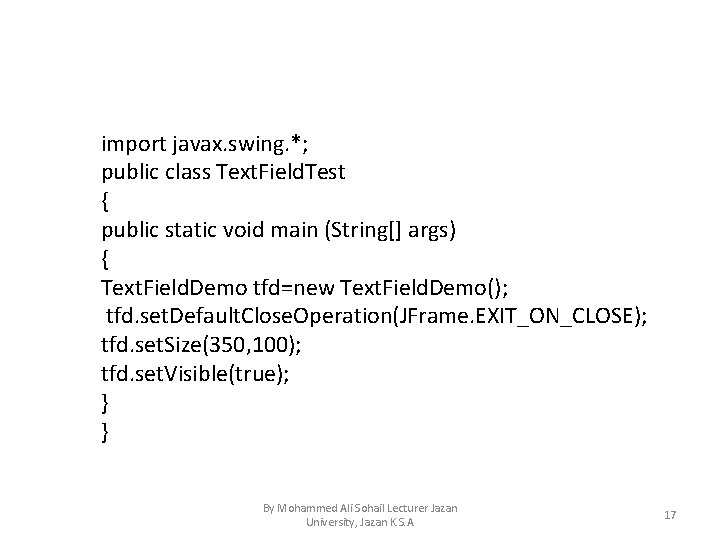
import javax. swing. *; public class Text. Field. Test { public static void main (String[] args) { Text. Field. Demo tfd=new Text. Field. Demo(); tfd. set. Default. Close. Operation(JFrame. EXIT_ON_CLOSE); tfd. set. Size(350, 100); tfd. set. Visible(true); } } By Mohammed Ali Sohail Lecturer Jazan University, Jazan K. S. A 17
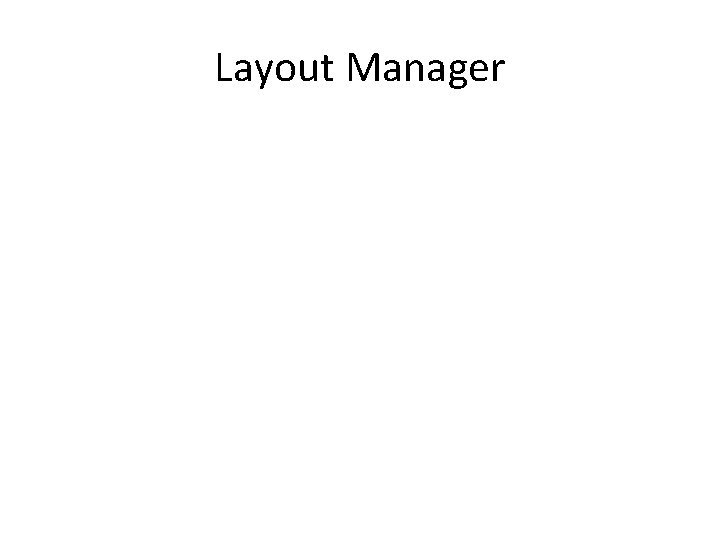
Layout Manager
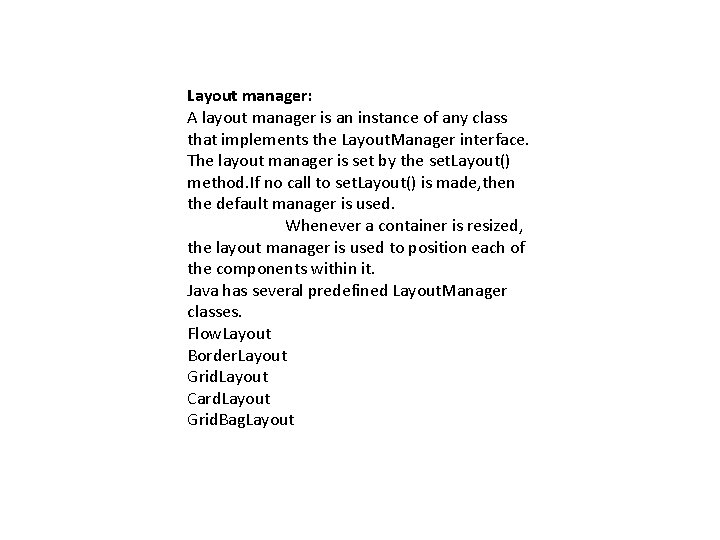
Layout manager: A layout manager is an instance of any class that implements the Layout. Manager interface. The layout manager is set by the set. Layout() method. If no call to set. Layout() is made, then the default manager is used. Whenever a container is resized, the layout manager is used to position each of the components within it. Java has several predefined Layout. Manager classes. Flow. Layout Border. Layout Grid. Layout Card. Layout Grid. Bag. Layout
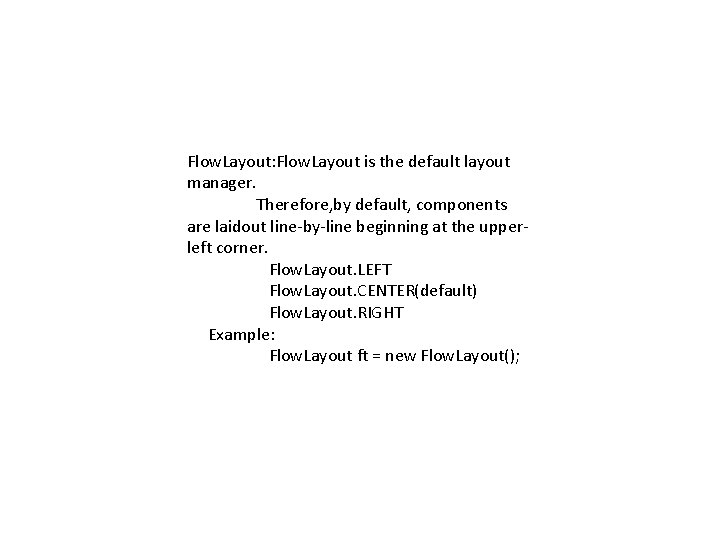
Flow. Layout: Flow. Layout is the default layout manager. Therefore, by default, components are laidout line-by-line beginning at the upperleft corner. Flow. Layout. LEFT Flow. Layout. CENTER(default) Flow. Layout. RIGHT Example: Flow. Layout ft = new Flow. Layout();
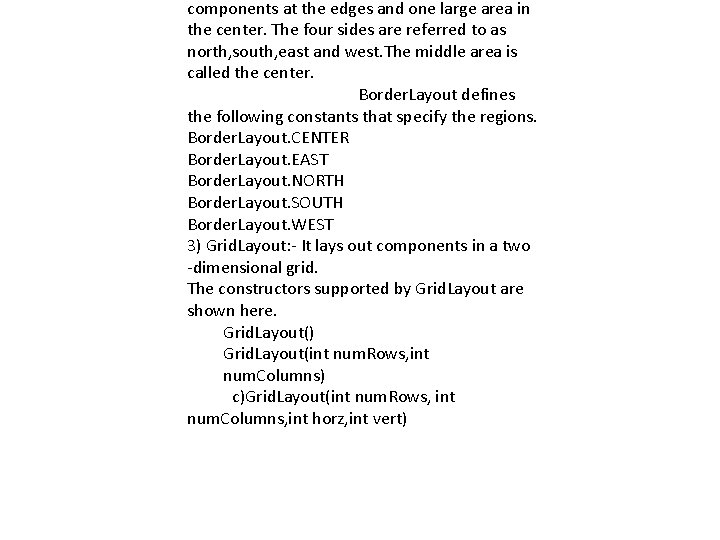
components at the edges and one large area in the center. The four sides are referred to as north, south, east and west. The middle area is called the center. Border. Layout defines the following constants that specify the regions. Border. Layout. CENTER Border. Layout. EAST Border. Layout. NORTH Border. Layout. SOUTH Border. Layout. WEST 3) Grid. Layout: - It lays out components in a two -dimensional grid. The constructors supported by Grid. Layout are shown here. Grid. Layout() Grid. Layout(int num. Rows, int num. Columns) c)Grid. Layout(int num. Rows, int num. Columns, int horz, int vert)
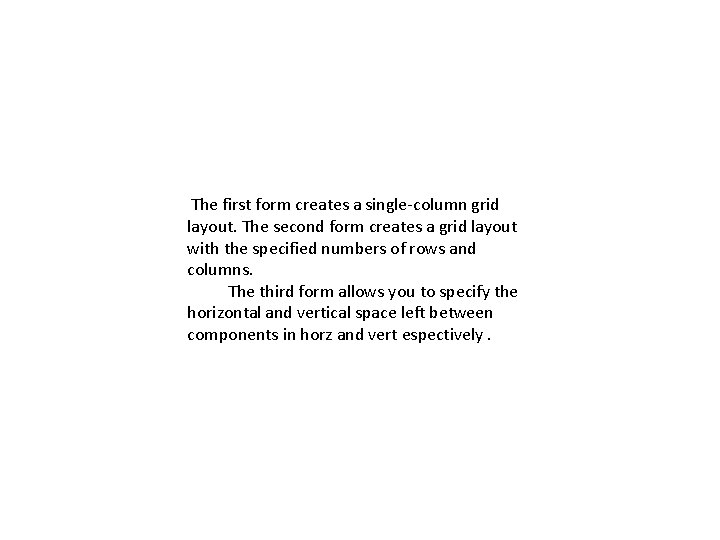
The first form creates a single-column grid layout. The second form creates a grid layout with the specified numbers of rows and columns. The third form allows you to specify the horizontal and vertical space left between components in horz and vert espectively.
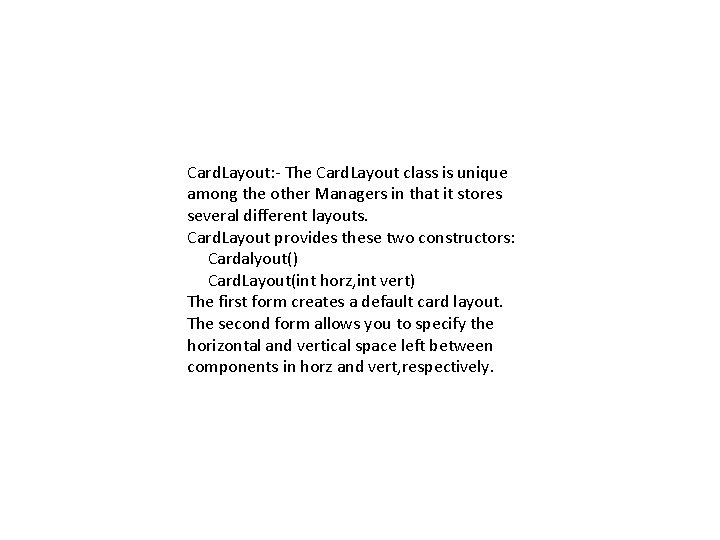
Card. Layout: - The Card. Layout class is unique among the other Managers in that it stores several different layouts. Card. Layout provides these two constructors: Cardalyout() Card. Layout(int horz, int vert) The first form creates a default card layout. The second form allows you to specify the horizontal and vertical space left between components in horz and vert, respectively.
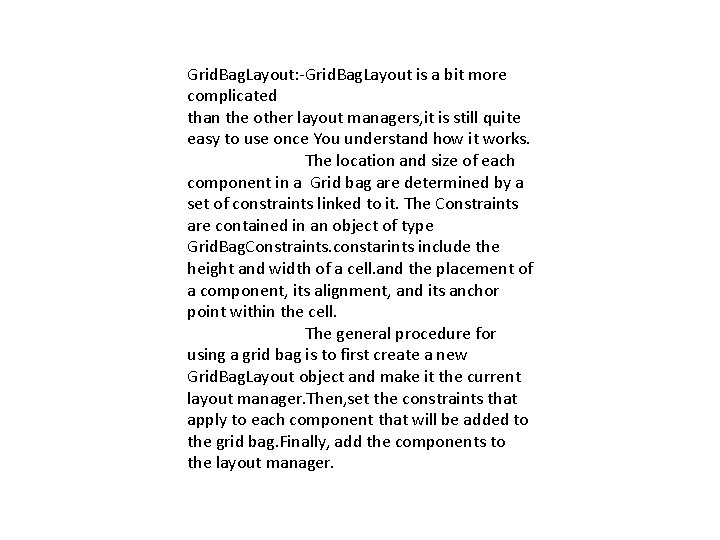
Grid. Bag. Layout: -Grid. Bag. Layout is a bit more complicated than the other layout managers, it is still quite easy to use once You understand how it works. The location and size of each component in a Grid bag are determined by a set of constraints linked to it. The Constraints are contained in an object of type Grid. Bag. Constraints. constarints include the height and width of a cell. and the placement of a component, its alignment, and its anchor point within the cell. The general procedure for using a grid bag is to first create a new Grid. Bag. Layout object and make it the current layout manager. Then, set the constraints that apply to each component that will be added to the grid bag. Finally, add the components to the layout manager.
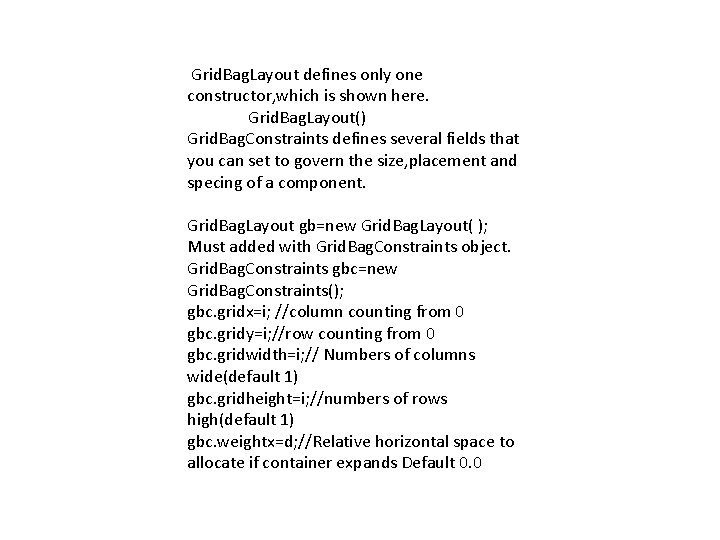
Grid. Bag. Layout defines only one constructor, which is shown here. Grid. Bag. Layout() Grid. Bag. Constraints defines several fields that you can set to govern the size, placement and specing of a component. Grid. Bag. Layout gb=new Grid. Bag. Layout( ); Must added with Grid. Bag. Constraints object. Grid. Bag. Constraints gbc=new Grid. Bag. Constraints(); gbc. gridx=i; //column counting from 0 gbc. gridy=i; //row counting from 0 gbc. gridwidth=i; // Numbers of columns wide(default 1) gbc. gridheight=i; //numbers of rows high(default 1) gbc. weightx=d; //Relative horizontal space to allocate if container expands Default 0. 0
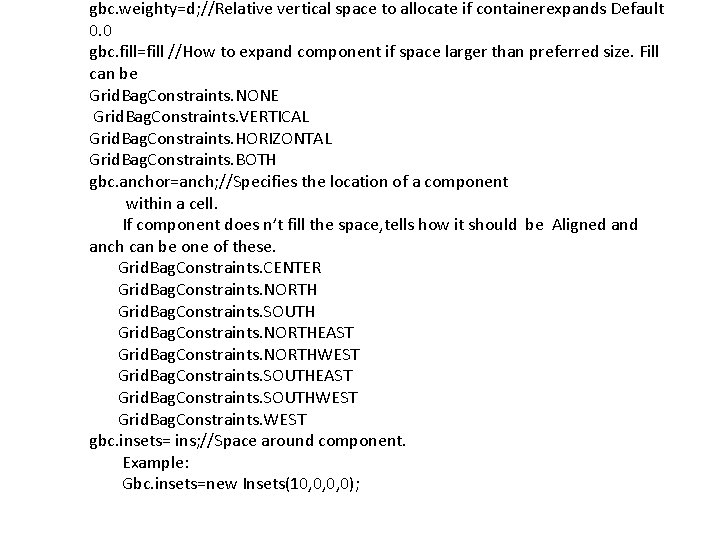
gbc. weighty=d; //Relative vertical space to allocate if containerexpands Default 0. 0 gbc. fill=fill //How to expand component if space larger than preferred size. Fill can be Grid. Bag. Constraints. NONE Grid. Bag. Constraints. VERTICAL Grid. Bag. Constraints. HORIZONTAL Grid. Bag. Constraints. BOTH gbc. anchor=anch; //Specifies the location of a component within a cell. If component does n’t fill the space, tells how it should be Aligned anch can be one of these. Grid. Bag. Constraints. CENTER Grid. Bag. Constraints. NORTH Grid. Bag. Constraints. SOUTH Grid. Bag. Constraints. NORTHEAST Grid. Bag. Constraints. NORTHWEST Grid. Bag. Constraints. SOUTHEAST Grid. Bag. Constraints. SOUTHWEST Grid. Bag. Constraints. WEST gbc. insets= ins; //Space around component. Example: Gbc. insets=new Insets(10, 0, 0, 0);
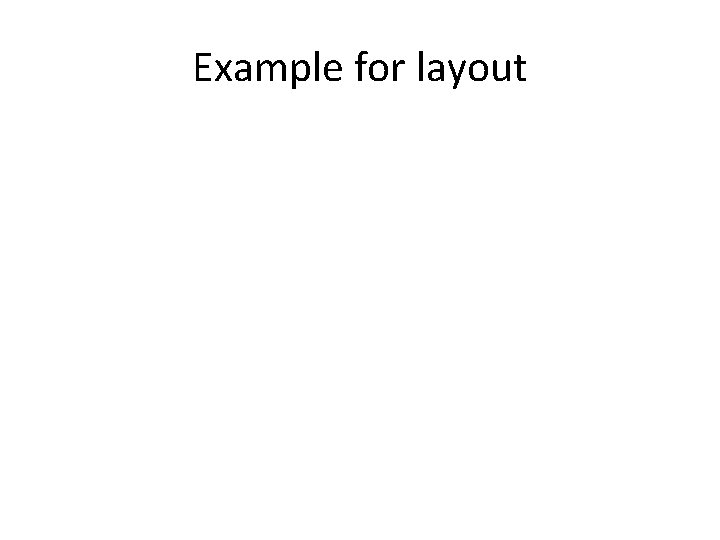
Example for layout
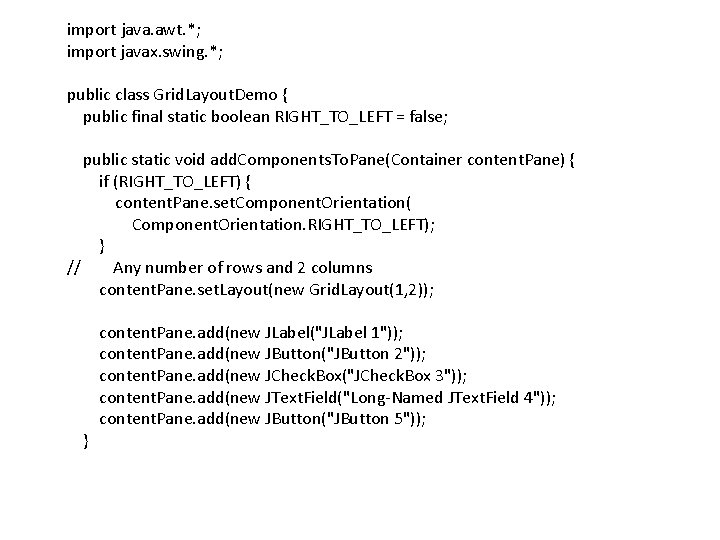
import java. awt. *; import javax. swing. *; public class Grid. Layout. Demo { public final static boolean RIGHT_TO_LEFT = false; public static void add. Components. To. Pane(Container content. Pane) { if (RIGHT_TO_LEFT) { content. Pane. set. Component. Orientation( Component. Orientation. RIGHT_TO_LEFT); } // Any number of rows and 2 columns content. Pane. set. Layout(new Grid. Layout(1, 2)); } content. Pane. add(new JLabel("JLabel 1")); content. Pane. add(new JButton("JButton 2")); content. Pane. add(new JCheck. Box("JCheck. Box 3")); content. Pane. add(new JText. Field("Long-Named JText. Field 4")); content. Pane. add(new JButton("JButton 5"));
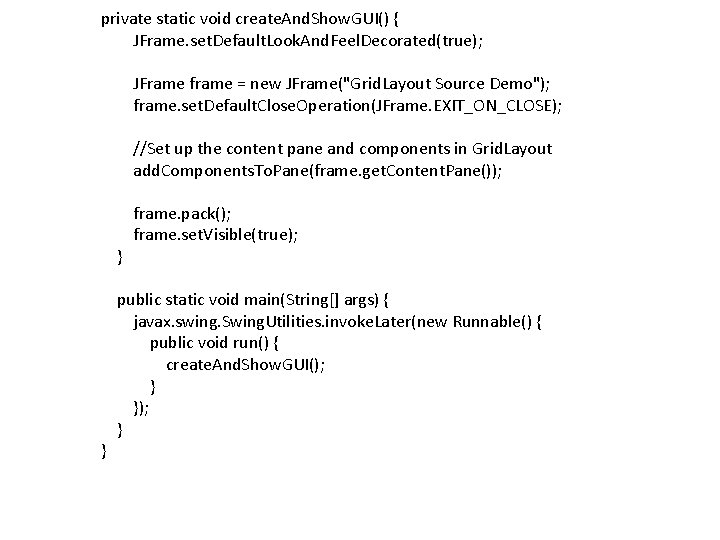
private static void create. And. Show. GUI() { JFrame. set. Default. Look. And. Feel. Decorated(true); JFrame frame = new JFrame("Grid. Layout Source Demo"); frame. set. Default. Close. Operation(JFrame. EXIT_ON_CLOSE); //Set up the content pane and components in Grid. Layout add. Components. To. Pane(frame. get. Content. Pane()); } } frame. pack(); frame. set. Visible(true); public static void main(String[] args) { javax. swing. Swing. Utilities. invoke. Later(new Runnable() { public void run() { create. And. Show. GUI(); } }); }