GUI Programming with threads Java GUI programming and
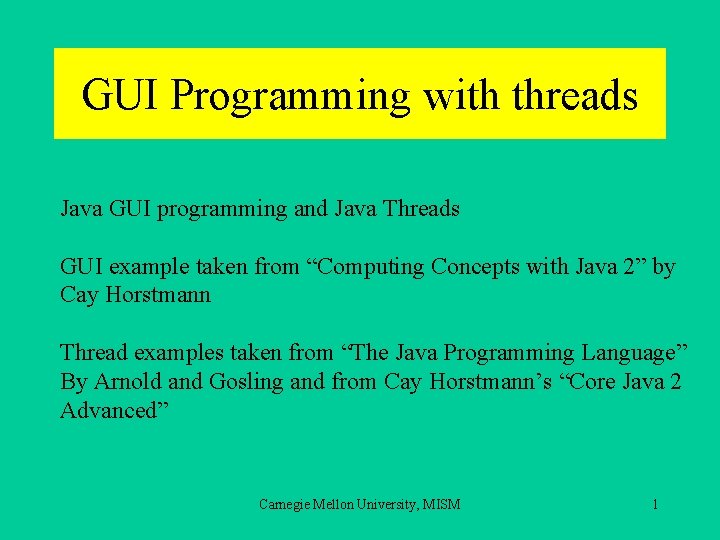
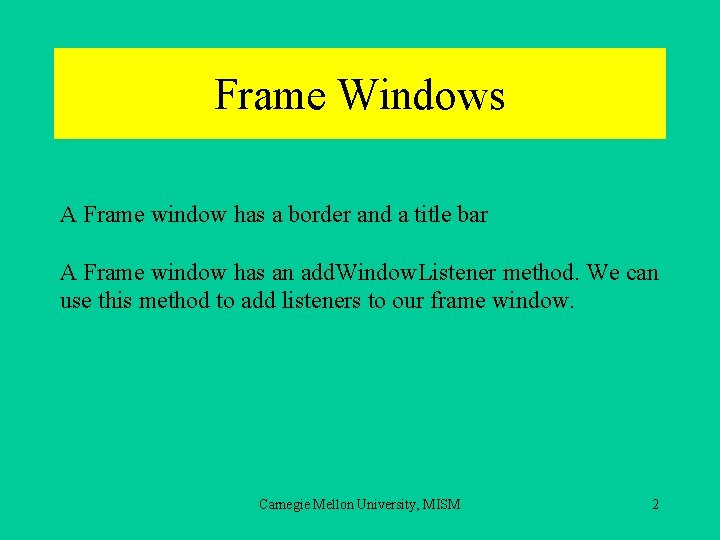
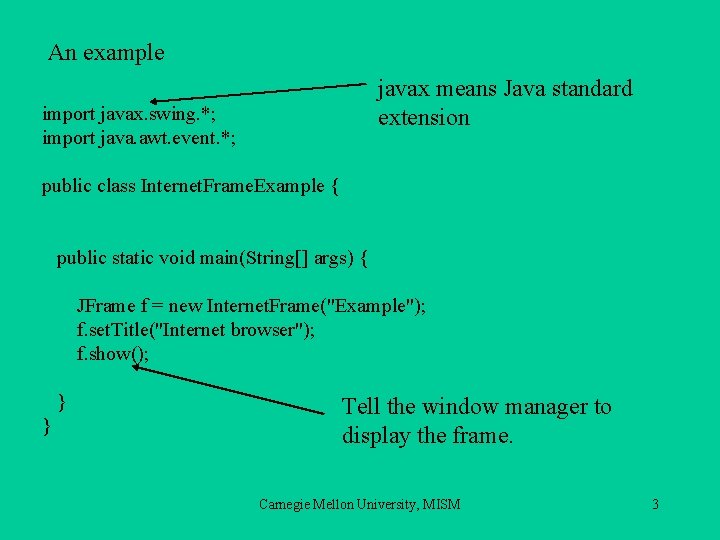
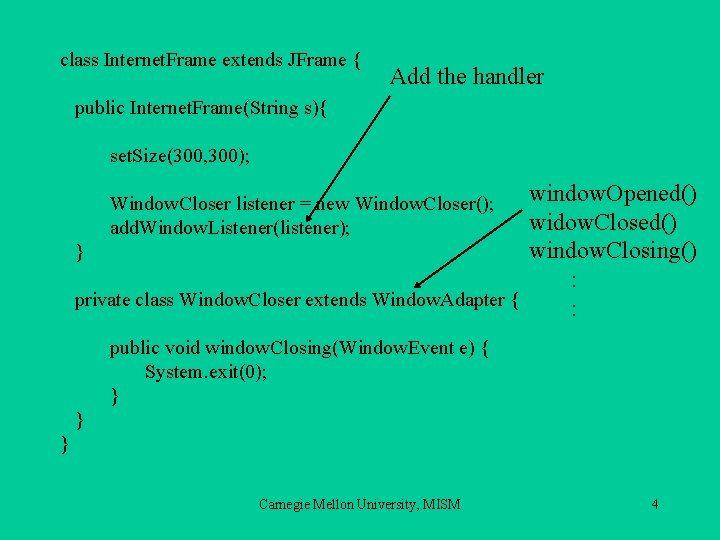
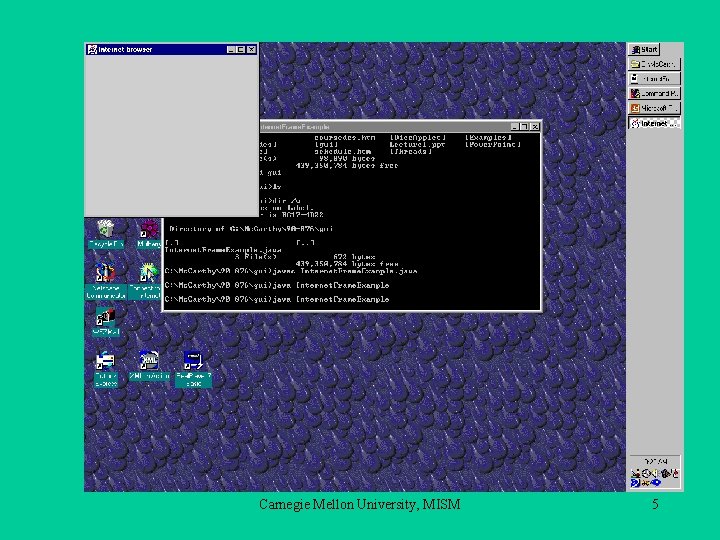
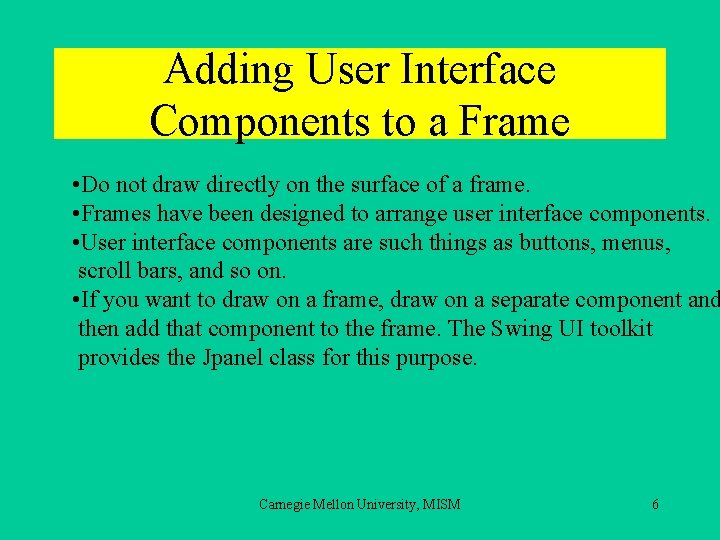
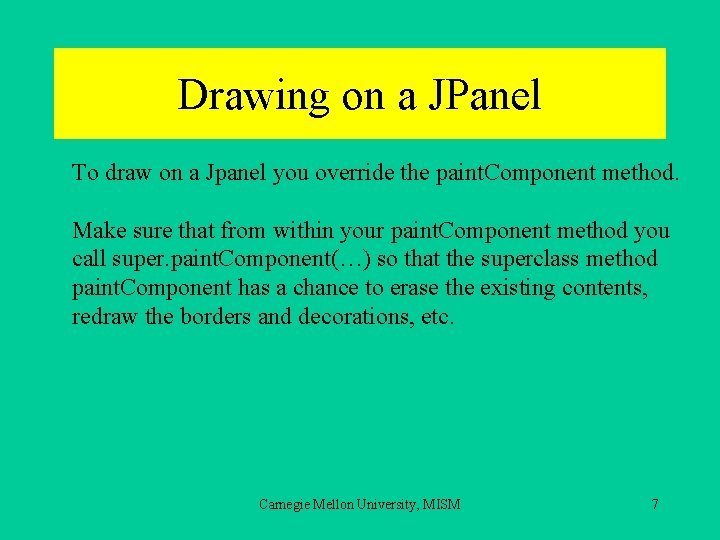
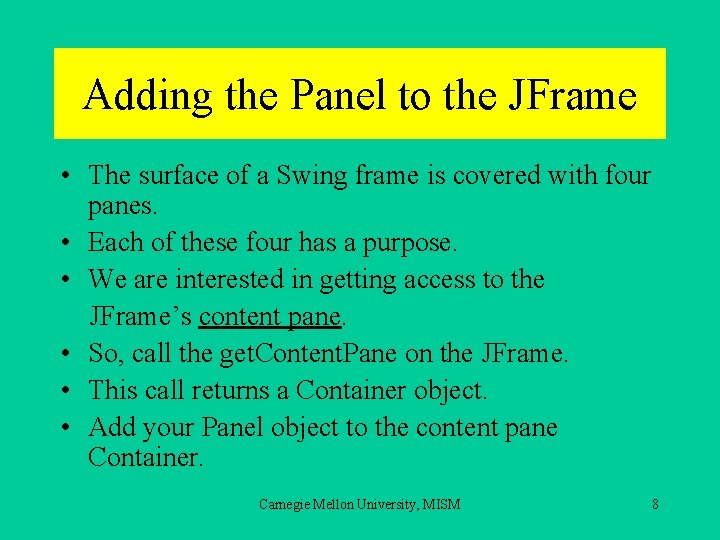
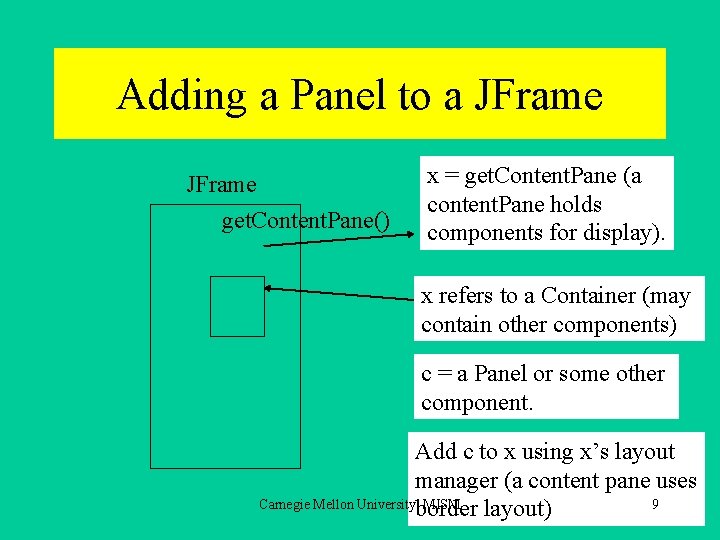
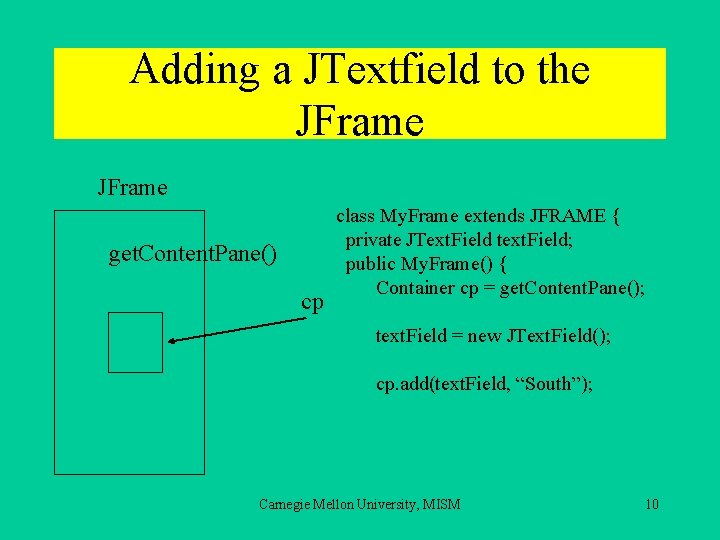
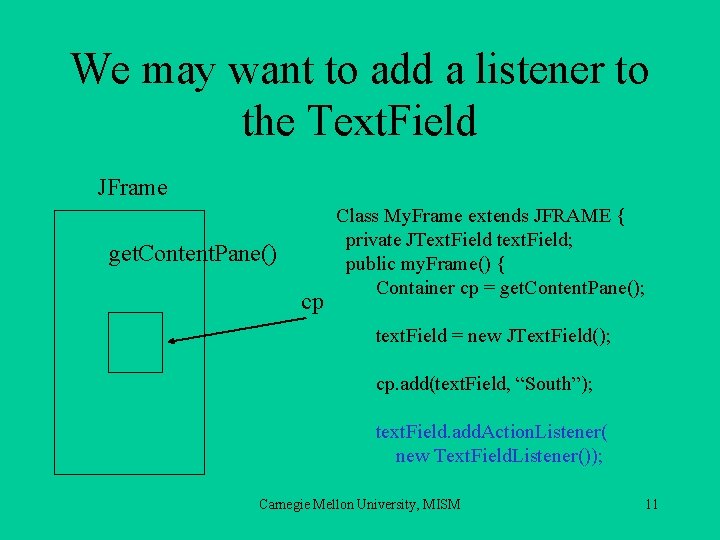
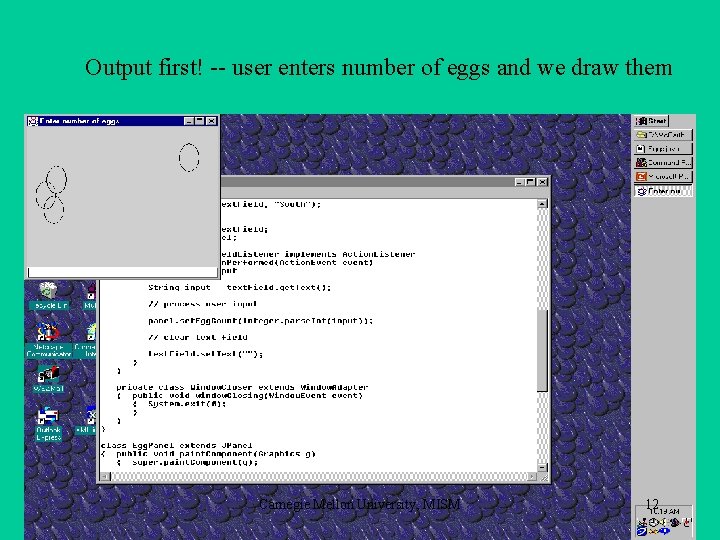
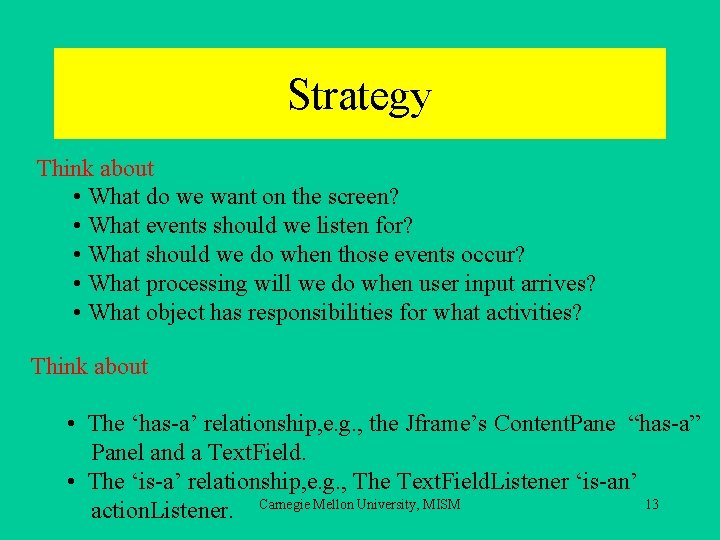
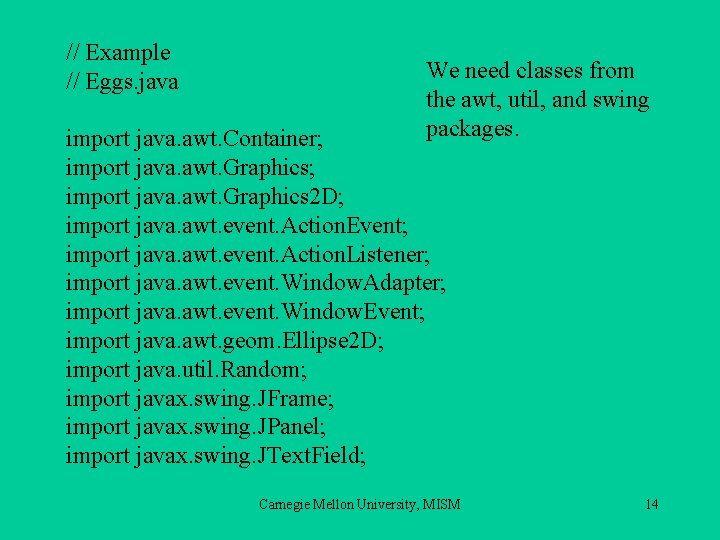
![public class Eggs { public static void main(String[] args) { Egg. Frame frame = public class Eggs { public static void main(String[] args) { Egg. Frame frame =](https://slidetodoc.com/presentation_image_h2/506a3c5628ee9ea5eaa4ba93c1b12c3c/image-15.jpg)
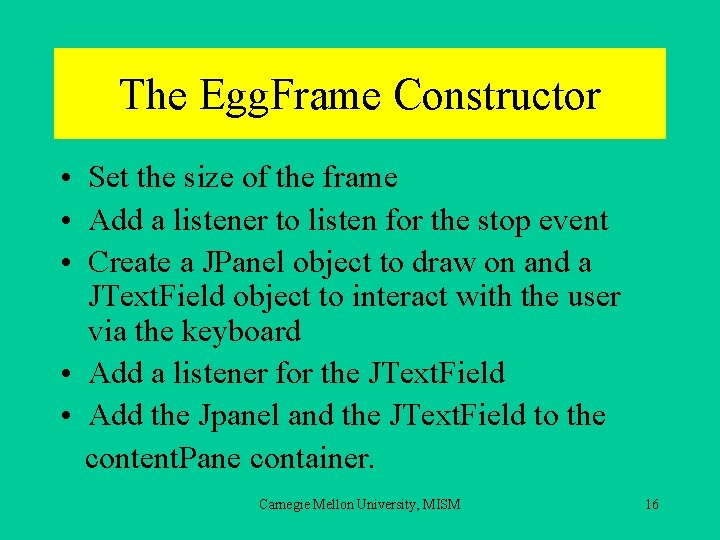
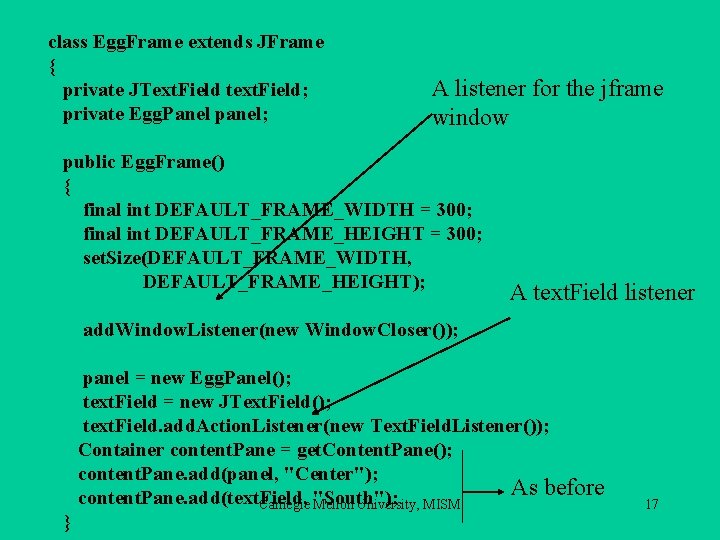
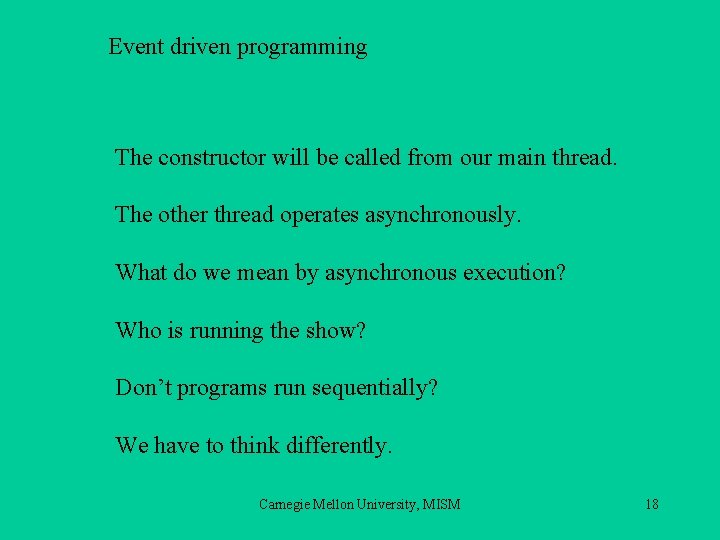
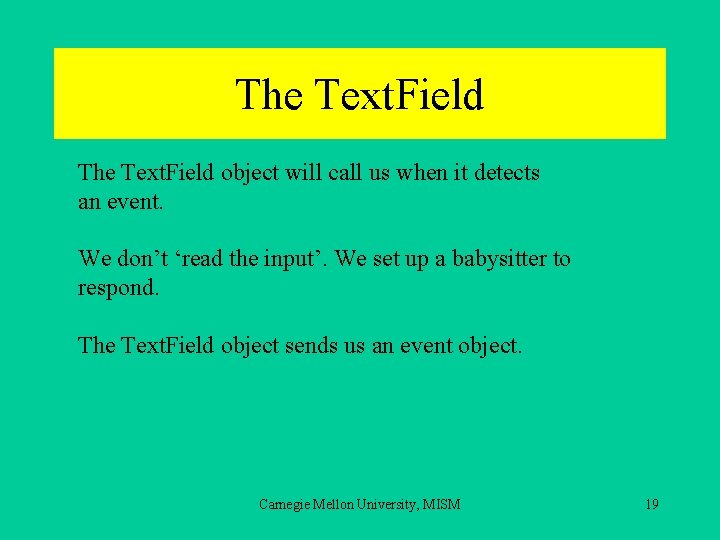
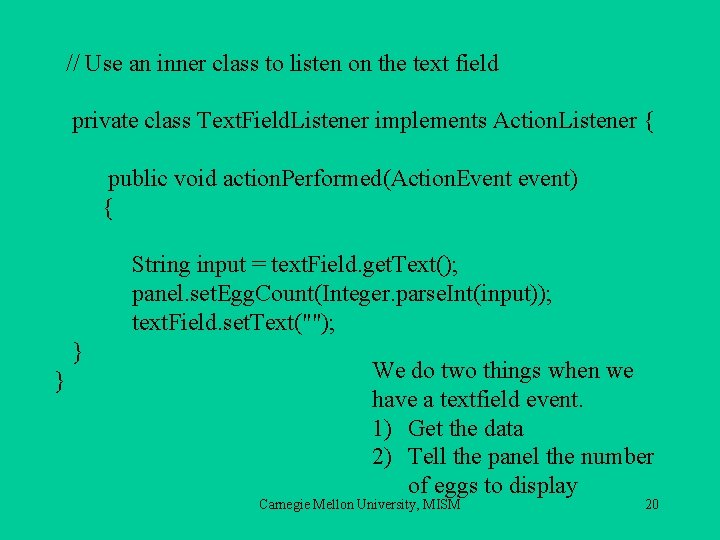
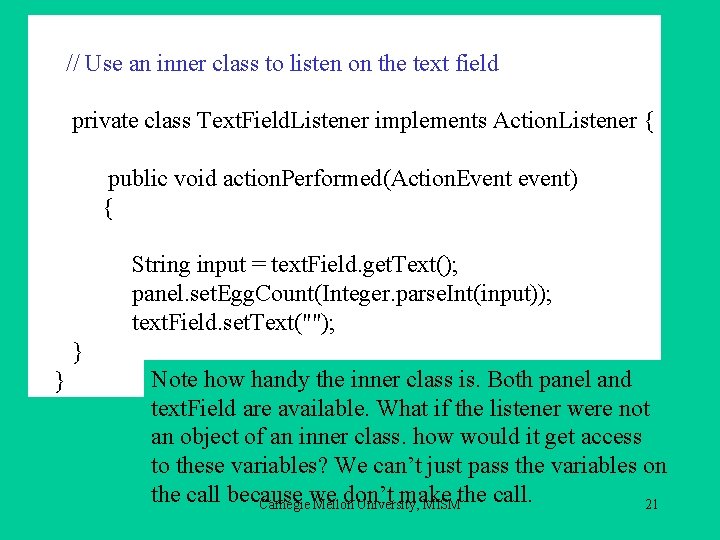
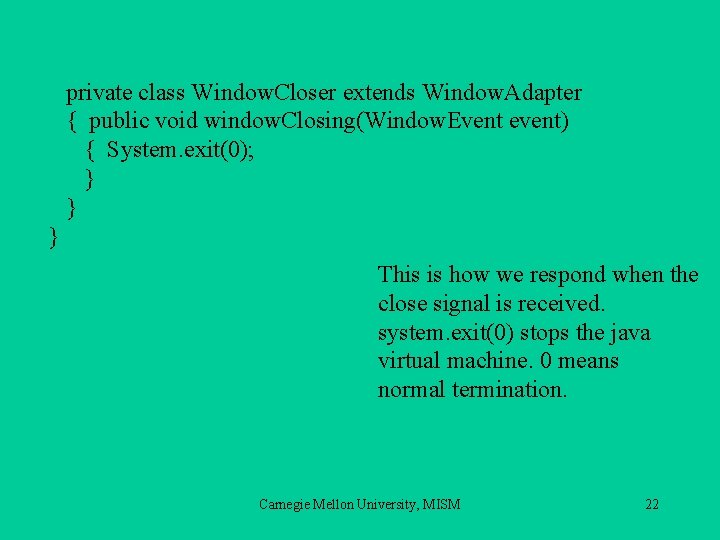
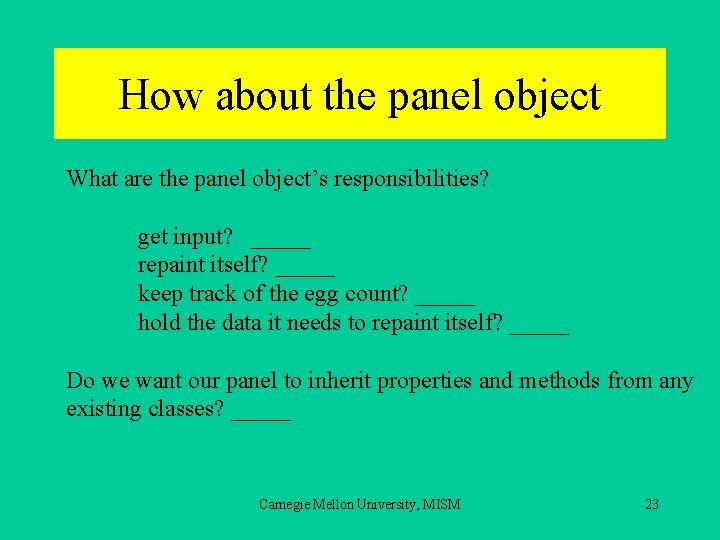
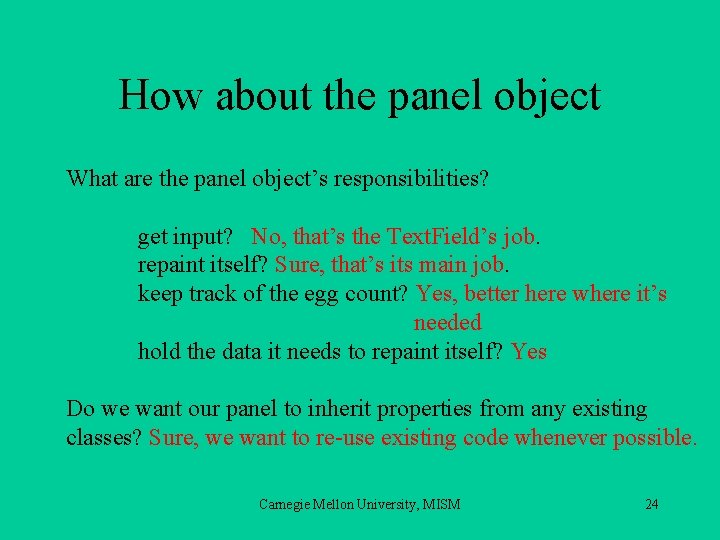
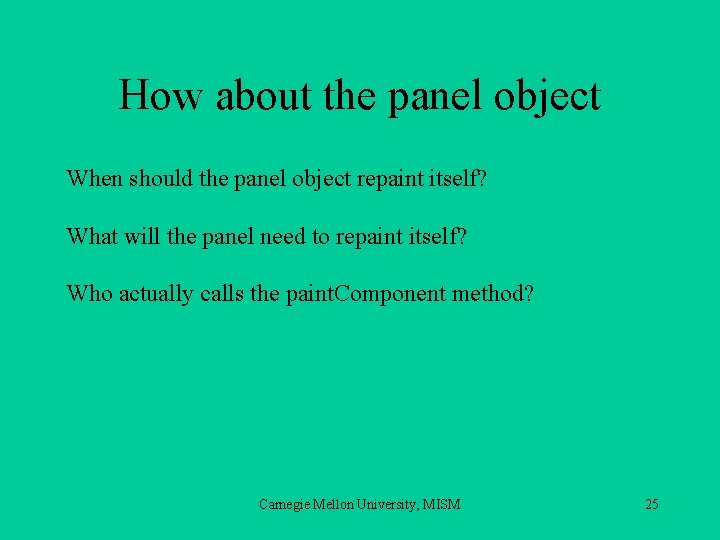
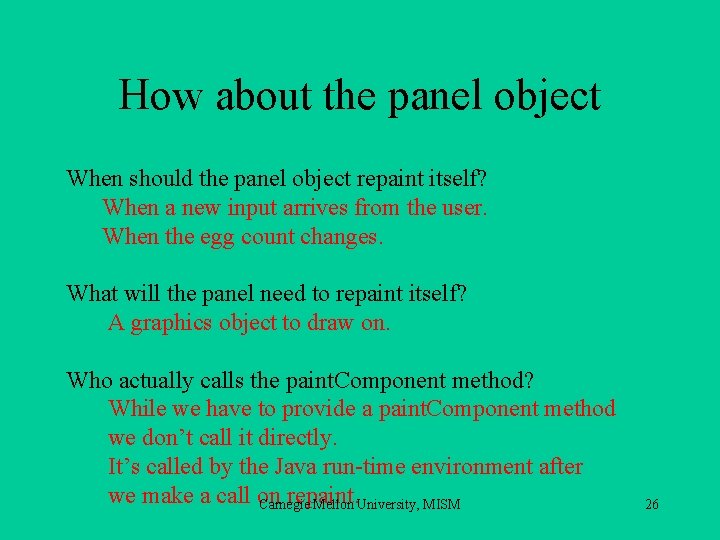
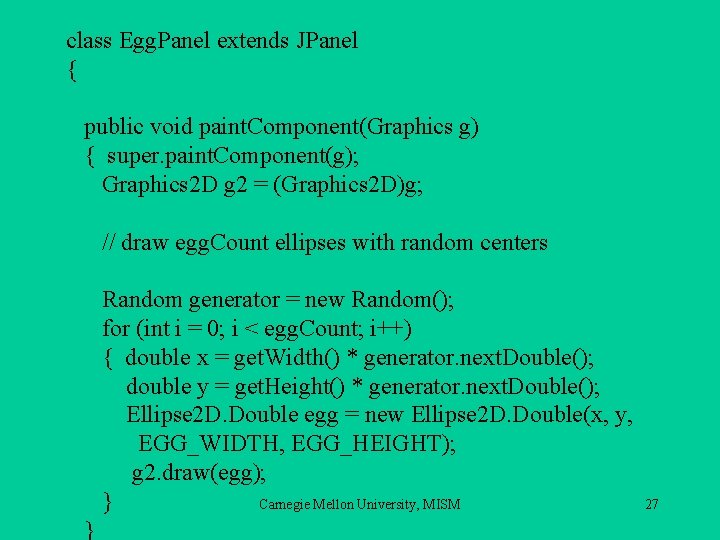
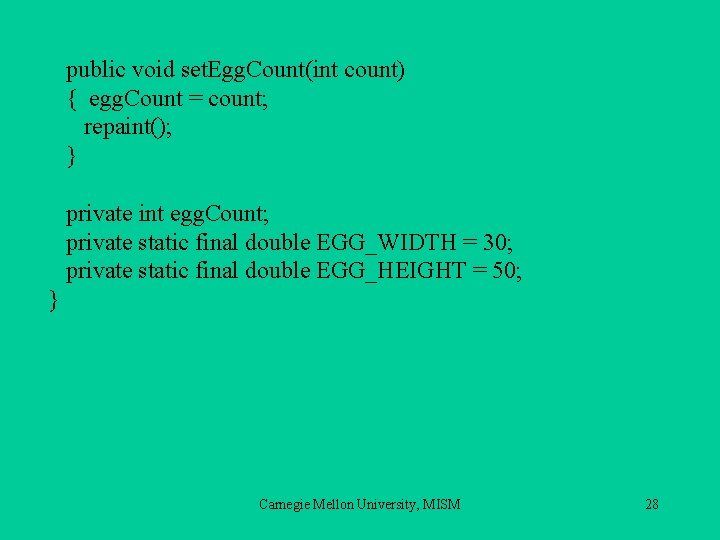
- Slides: 28
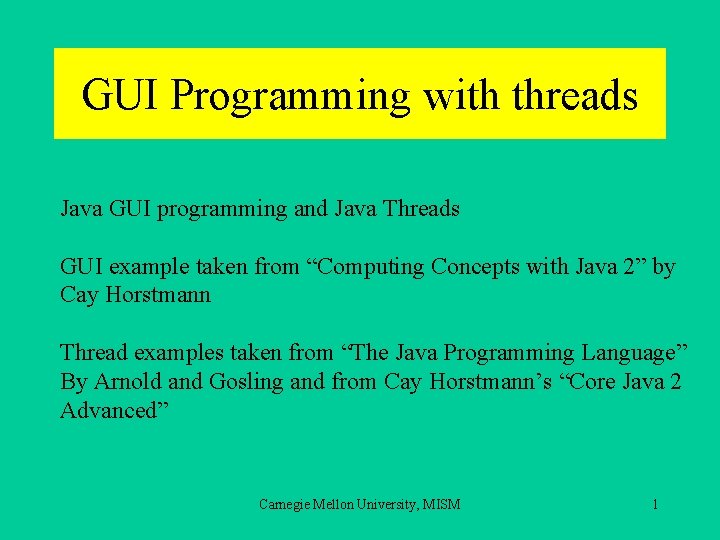
GUI Programming with threads Java GUI programming and Java Threads GUI example taken from “Computing Concepts with Java 2” by Cay Horstmann Thread examples taken from “The Java Programming Language” By Arnold and Gosling and from Cay Horstmann’s “Core Java 2 Advanced” Carnegie Mellon University, MISM 1
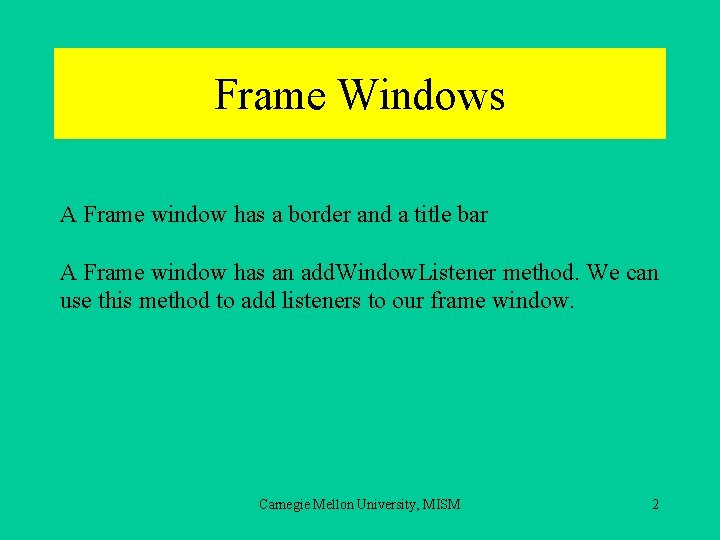
Frame Windows A Frame window has a border and a title bar A Frame window has an add. Window. Listener method. We can use this method to add listeners to our frame window. Carnegie Mellon University, MISM 2
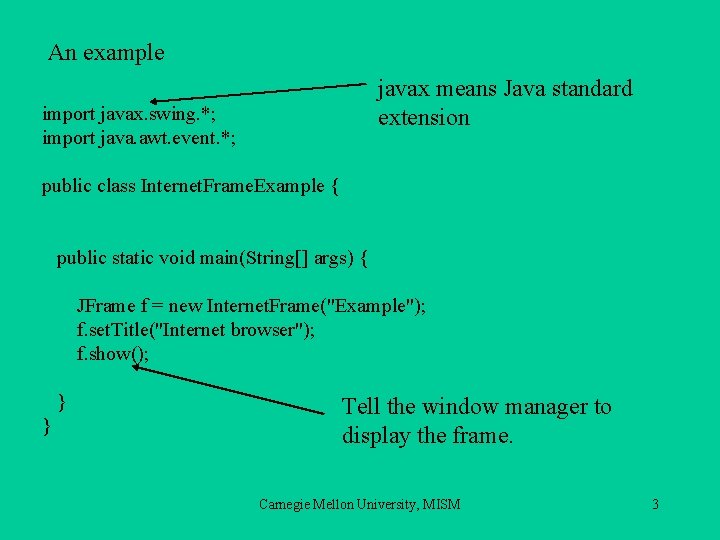
An example javax means Java standard extension import javax. swing. *; import java. awt. event. *; public class Internet. Frame. Example { public static void main(String[] args) { JFrame f = new Internet. Frame("Example"); f. set. Title("Internet browser"); f. show(); } } Tell the window manager to display the frame. Carnegie Mellon University, MISM 3
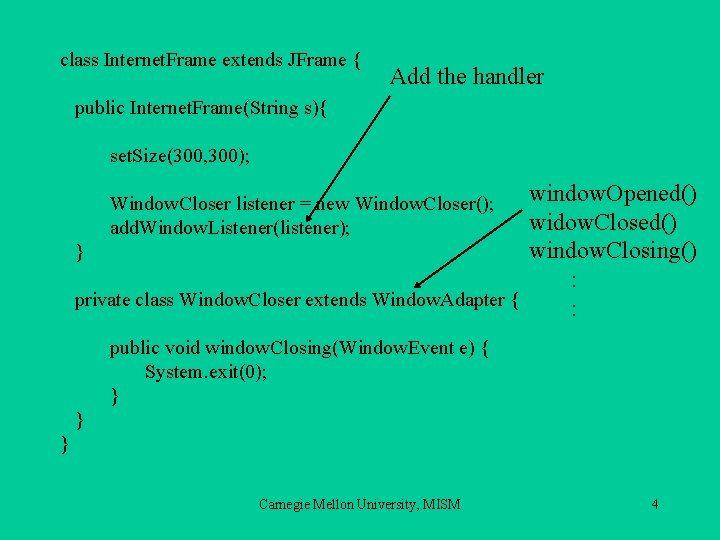
class Internet. Frame extends JFrame { Add the handler public Internet. Frame(String s){ set. Size(300, 300); window. Opened() widow. Closed() } window. Closing() : private class Window. Closer extends Window. Adapter { : Window. Closer listener = new Window. Closer(); add. Window. Listener(listener); public void window. Closing(Window. Event e) { System. exit(0); } } } Carnegie Mellon University, MISM 4
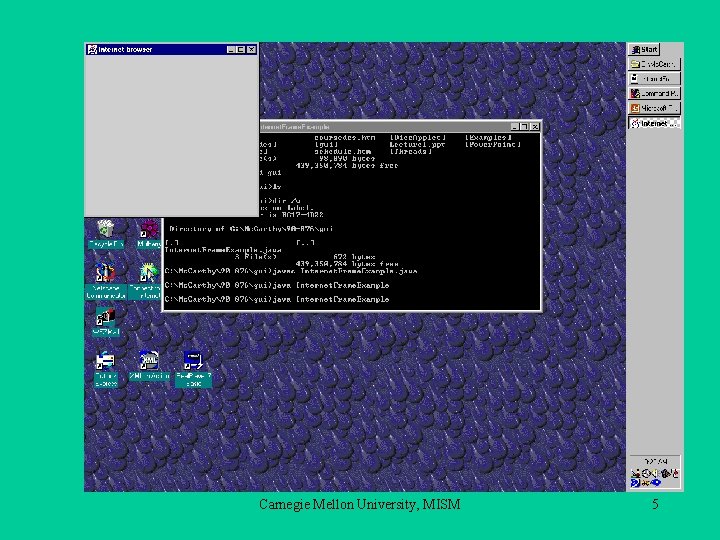
Carnegie Mellon University, MISM 5
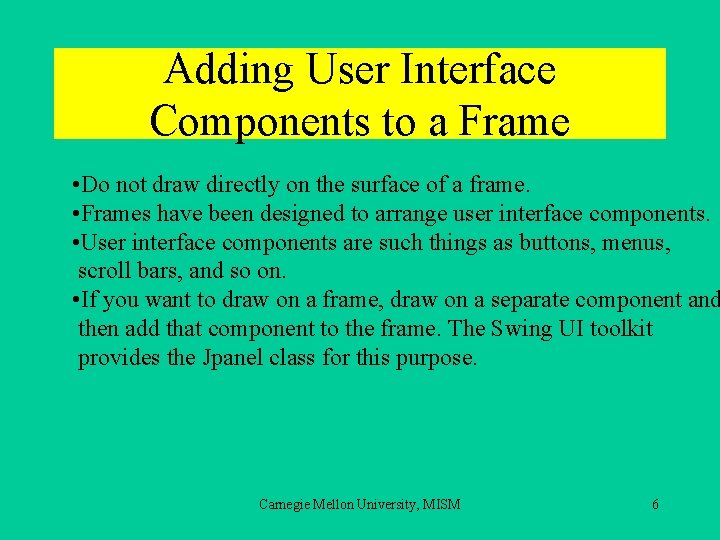
Adding User Interface Components to a Frame • Do not draw directly on the surface of a frame. • Frames have been designed to arrange user interface components. • User interface components are such things as buttons, menus, scroll bars, and so on. • If you want to draw on a frame, draw on a separate component and then add that component to the frame. The Swing UI toolkit provides the Jpanel class for this purpose. Carnegie Mellon University, MISM 6
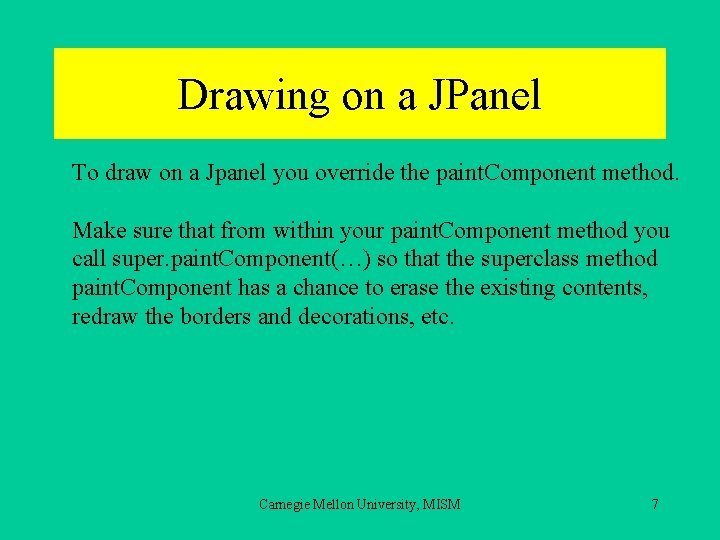
Drawing on a JPanel To draw on a Jpanel you override the paint. Component method. Make sure that from within your paint. Component method you call super. paint. Component(…) so that the superclass method paint. Component has a chance to erase the existing contents, redraw the borders and decorations, etc. Carnegie Mellon University, MISM 7
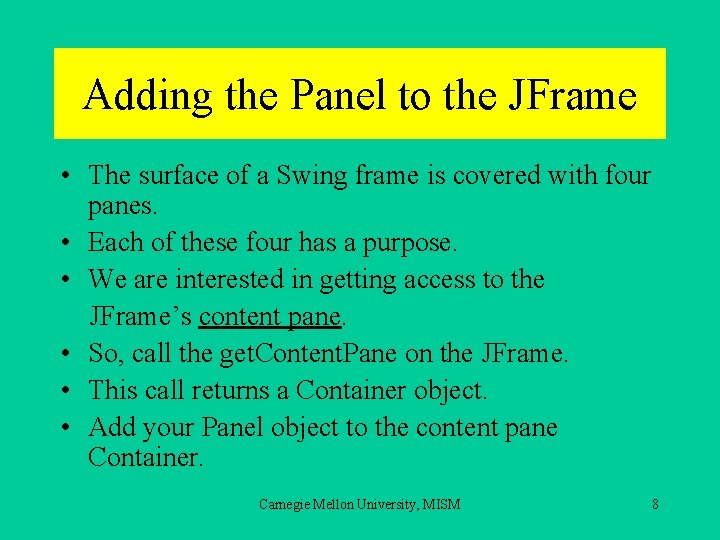
Adding the Panel to the JFrame • The surface of a Swing frame is covered with four panes. • Each of these four has a purpose. • We are interested in getting access to the JFrame’s content pane. • So, call the get. Content. Pane on the JFrame. • This call returns a Container object. • Add your Panel object to the content pane Container. Carnegie Mellon University, MISM 8
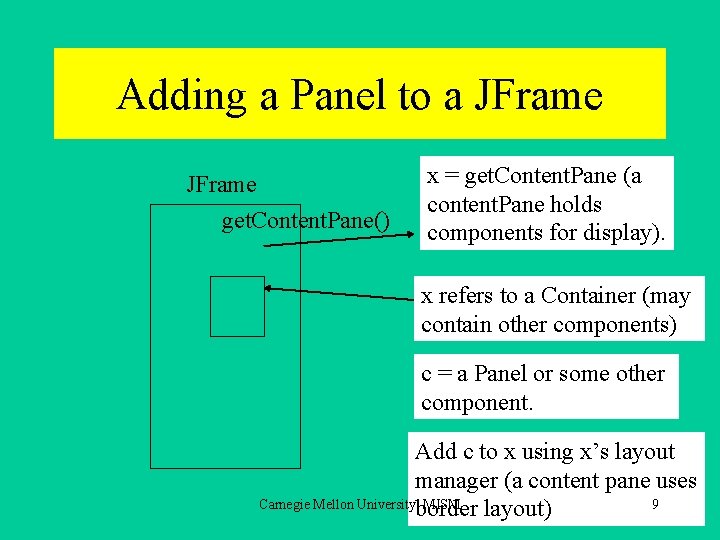
Adding a Panel to a JFrame get. Content. Pane() x = get. Content. Pane (a content. Pane holds components for display). x refers to a Container (may contain other components) c = a Panel or some other component. Add c to x using x’s layout manager (a content pane uses Carnegie Mellon University, MISM 9 border layout)
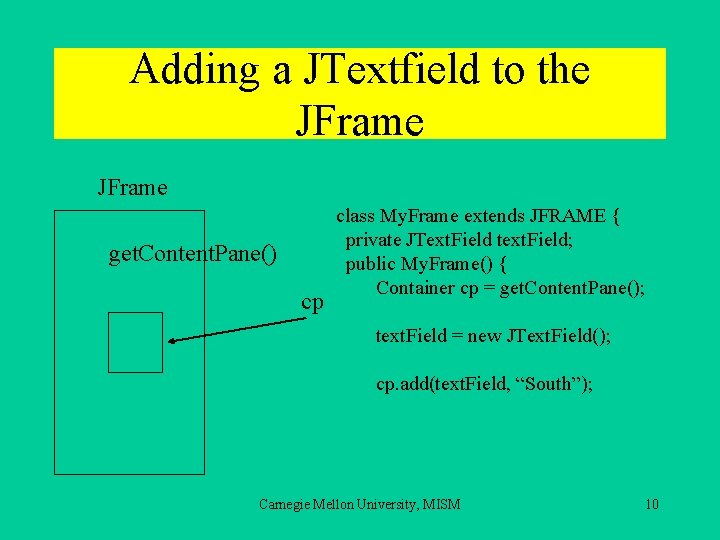
Adding a JTextfield to the JFrame get. Content. Pane() cp class My. Frame extends JFRAME { private JText. Field text. Field; public My. Frame() { Container cp = get. Content. Pane(); text. Field = new JText. Field(); cp. add(text. Field, “South”); Carnegie Mellon University, MISM 10
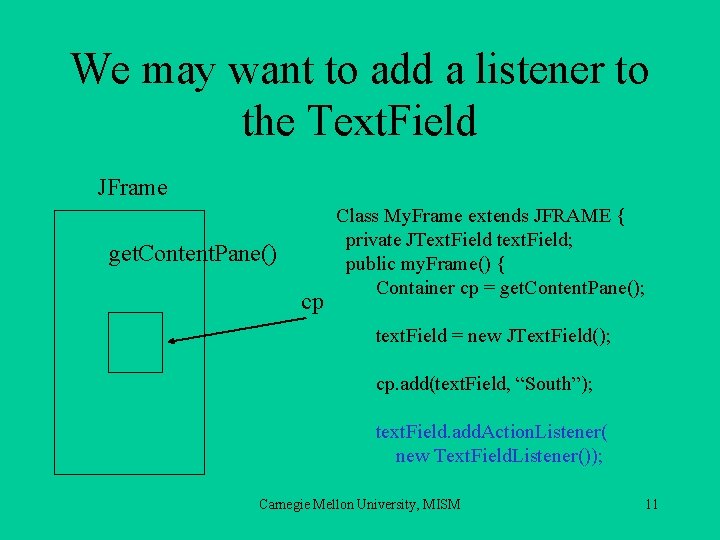
We may want to add a listener to the Text. Field JFrame get. Content. Pane() cp Class My. Frame extends JFRAME { private JText. Field text. Field; public my. Frame() { Container cp = get. Content. Pane(); text. Field = new JText. Field(); cp. add(text. Field, “South”); text. Field. add. Action. Listener( new Text. Field. Listener()); Carnegie Mellon University, MISM 11
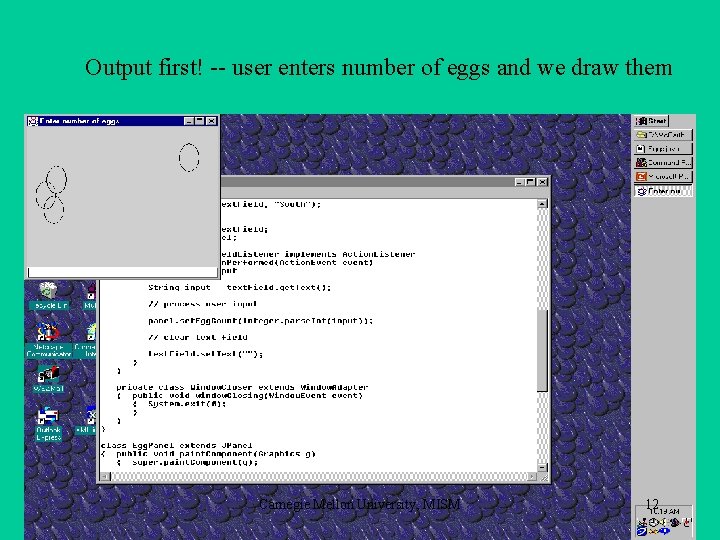
Output first! -- user enters number of eggs and we draw them Carnegie Mellon University, MISM 12
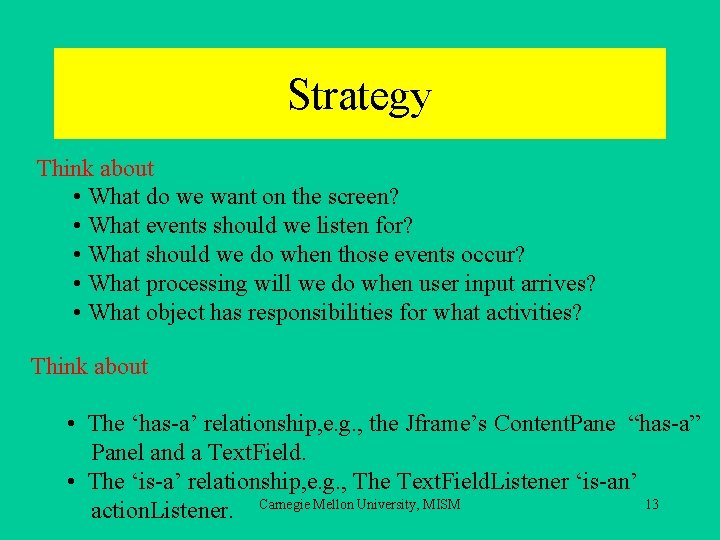
Strategy Think about • What do we want on the screen? • What events should we listen for? • What should we do when those events occur? • What processing will we do when user input arrives? • What object has responsibilities for what activities? Think about • The ‘has-a’ relationship, e. g. , the Jframe’s Content. Pane “has-a” Panel and a Text. Field. • The ‘is-a’ relationship, e. g. , The Text. Field. Listener ‘is-an’ 13 action. Listener. Carnegie Mellon University, MISM
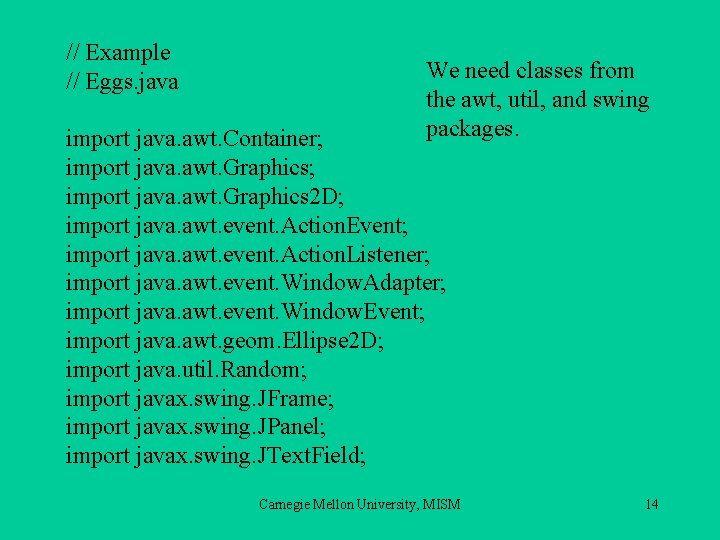
// Example // Eggs. java We need classes from the awt, util, and swing packages. import java. awt. Container; import java. awt. Graphics 2 D; import java. awt. event. Action. Event; import java. awt. event. Action. Listener; import java. awt. event. Window. Adapter; import java. awt. event. Window. Event; import java. awt. geom. Ellipse 2 D; import java. util. Random; import javax. swing. JFrame; import javax. swing. JPanel; import javax. swing. JText. Field; Carnegie Mellon University, MISM 14
![public class Eggs public static void mainString args Egg Frame frame public class Eggs { public static void main(String[] args) { Egg. Frame frame =](https://slidetodoc.com/presentation_image_h2/506a3c5628ee9ea5eaa4ba93c1b12c3c/image-15.jpg)
public class Eggs { public static void main(String[] args) { Egg. Frame frame = new Egg. Frame(); frame. set. Title("Enter number of eggs"); frame. show(); } } This thread is done after creating a frame and starting up the frame thread. A frame now exists and is running. Carnegie Mellon University, MISM 15
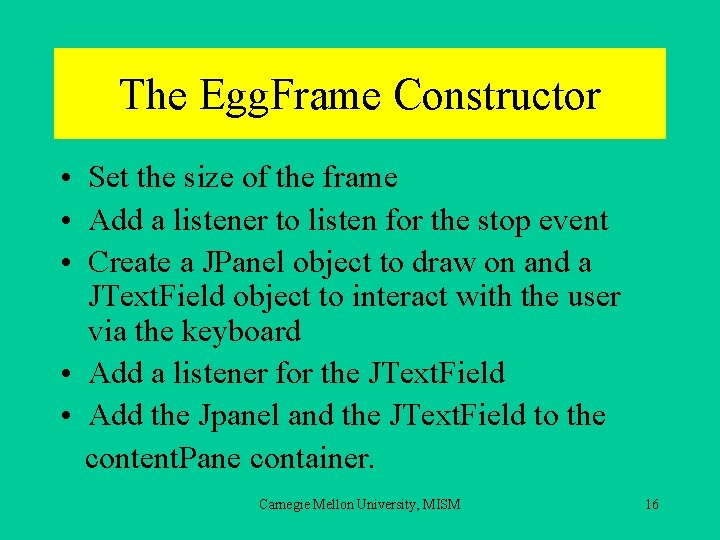
The Egg. Frame Constructor • Set the size of the frame • Add a listener to listen for the stop event • Create a JPanel object to draw on and a JText. Field object to interact with the user via the keyboard • Add a listener for the JText. Field • Add the Jpanel and the JText. Field to the content. Pane container. Carnegie Mellon University, MISM 16
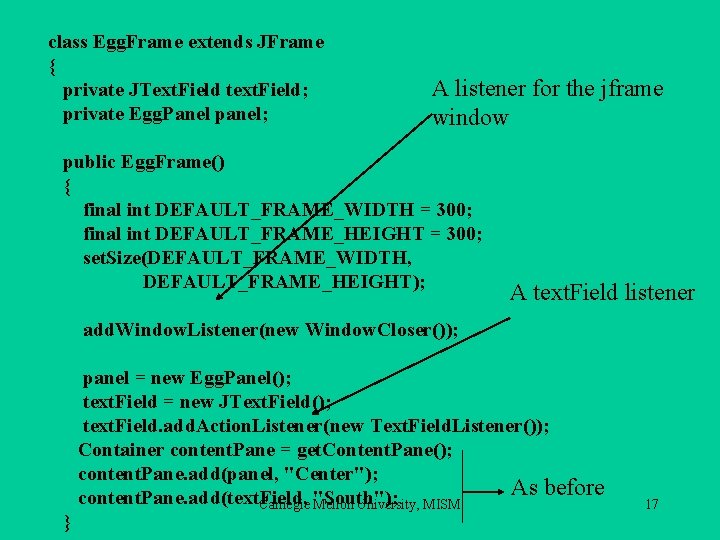
class Egg. Frame extends JFrame { private JText. Field text. Field; private Egg. Panel panel; A listener for the jframe window public Egg. Frame() { final int DEFAULT_FRAME_WIDTH = 300; final int DEFAULT_FRAME_HEIGHT = 300; set. Size(DEFAULT_FRAME_WIDTH, DEFAULT_FRAME_HEIGHT); A text. Field listener add. Window. Listener(new Window. Closer()); } panel = new Egg. Panel(); text. Field = new JText. Field(); text. Field. add. Action. Listener(new Text. Field. Listener()); Container content. Pane = get. Content. Pane(); content. Pane. add(panel, "Center"); As before content. Pane. add(text. Field, "South"); Carnegie Mellon University, MISM 17
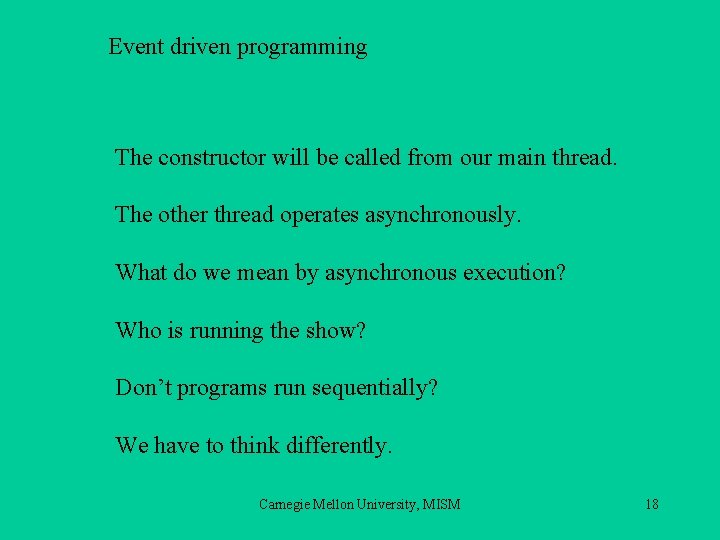
Event driven programming The constructor will be called from our main thread. The other thread operates asynchronously. What do we mean by asynchronous execution? Who is running the show? Don’t programs run sequentially? We have to think differently. Carnegie Mellon University, MISM 18
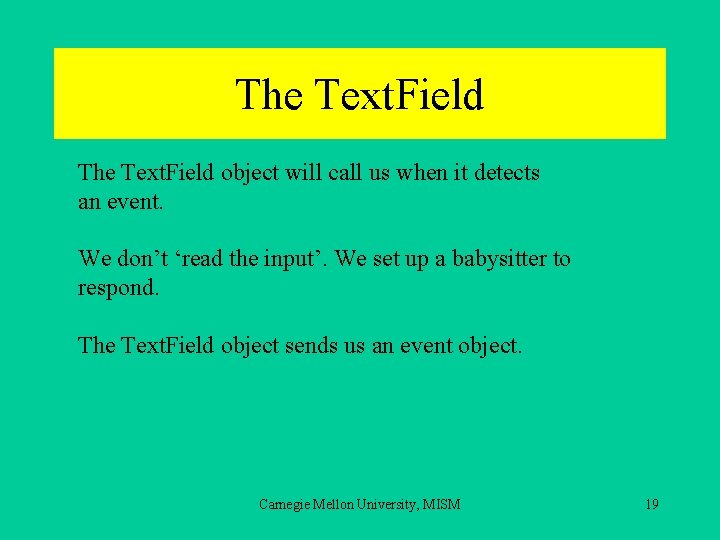
The Text. Field object will call us when it detects an event. We don’t ‘read the input’. We set up a babysitter to respond. The Text. Field object sends us an event object. Carnegie Mellon University, MISM 19
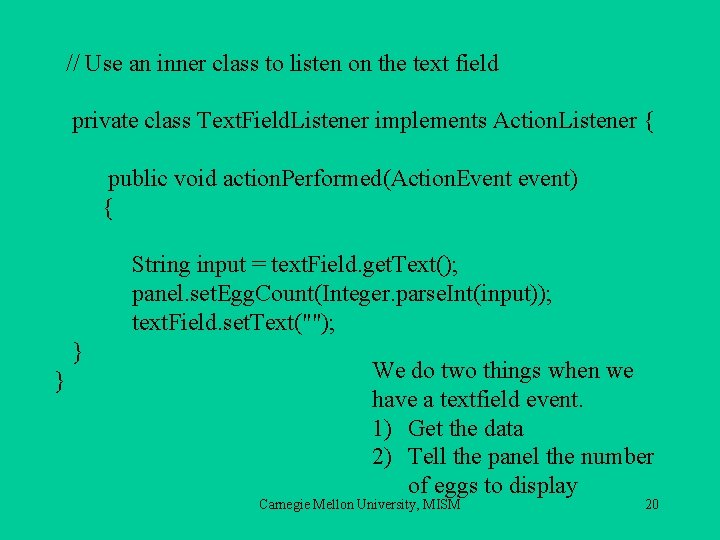
// Use an inner class to listen on the text field private class Text. Field. Listener implements Action. Listener { public void action. Performed(Action. Event event) { String input = text. Field. get. Text(); panel. set. Egg. Count(Integer. parse. Int(input)); text. Field. set. Text(""); } } We do two things when we have a textfield event. 1) Get the data 2) Tell the panel the number of eggs to display Carnegie Mellon University, MISM 20
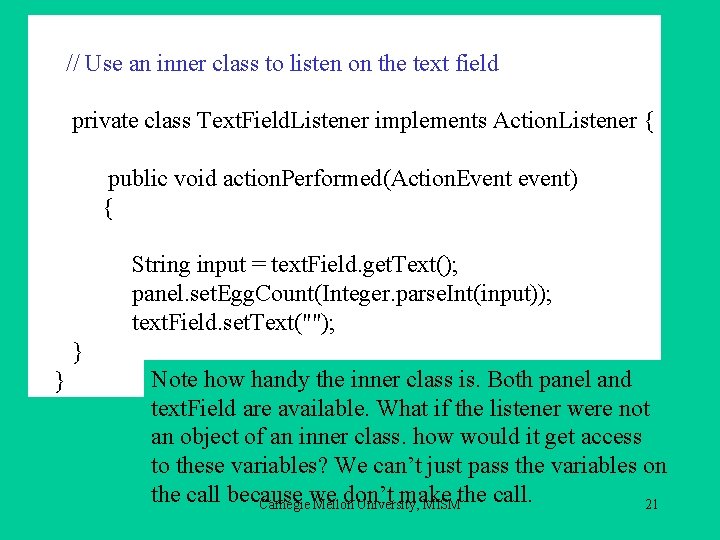
// Use an inner class to listen on the text field private class Text. Field. Listener implements Action. Listener { public void action. Performed(Action. Event event) { String input = text. Field. get. Text(); panel. set. Egg. Count(Integer. parse. Int(input)); text. Field. set. Text(""); } } Note how handy the inner class is. Both panel and text. Field are available. What if the listener were not an object of an inner class. how would it get access to these variables? We can’t just pass the variables on the call because we don’t make the call. Carnegie Mellon University, MISM 21
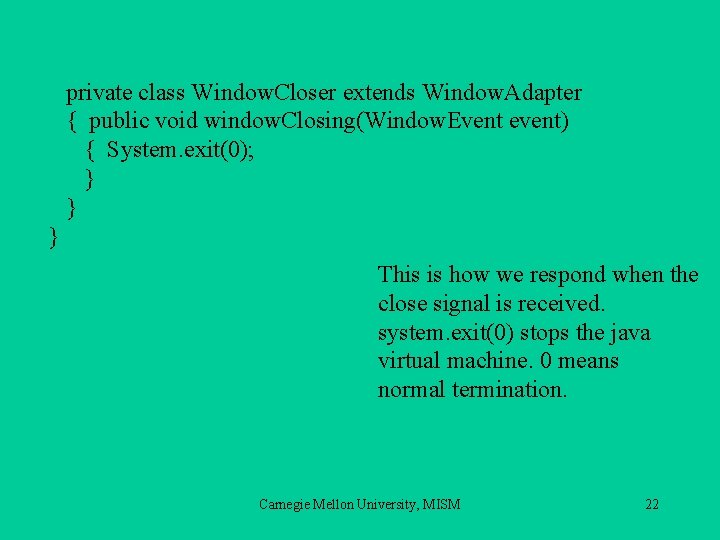
private class Window. Closer extends Window. Adapter { public void window. Closing(Window. Event event) { System. exit(0); } } } This is how we respond when the close signal is received. system. exit(0) stops the java virtual machine. 0 means normal termination. Carnegie Mellon University, MISM 22
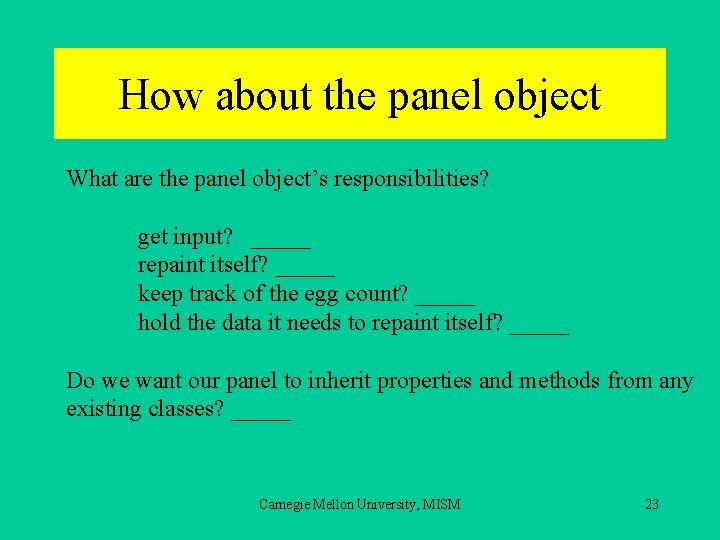
How about the panel object What are the panel object’s responsibilities? get input? _____ repaint itself? _____ keep track of the egg count? _____ hold the data it needs to repaint itself? _____ Do we want our panel to inherit properties and methods from any existing classes? _____ Carnegie Mellon University, MISM 23
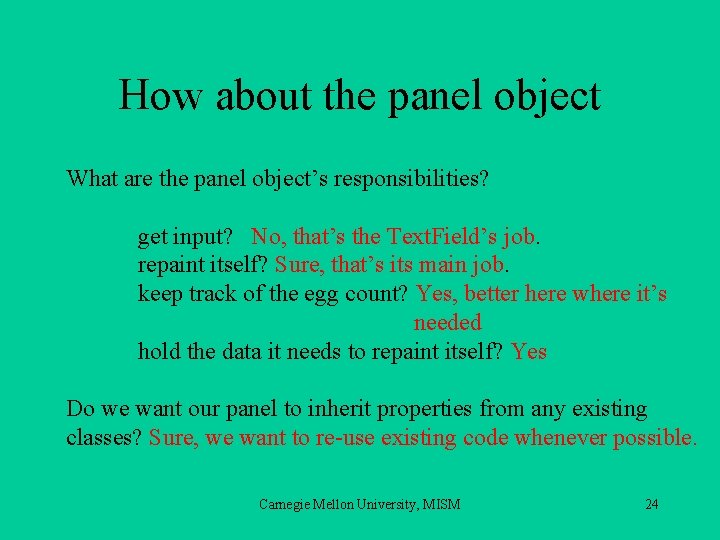
How about the panel object What are the panel object’s responsibilities? get input? No, that’s the Text. Field’s job. repaint itself? Sure, that’s its main job. keep track of the egg count? Yes, better here where it’s needed hold the data it needs to repaint itself? Yes Do we want our panel to inherit properties from any existing classes? Sure, we want to re-use existing code whenever possible. Carnegie Mellon University, MISM 24
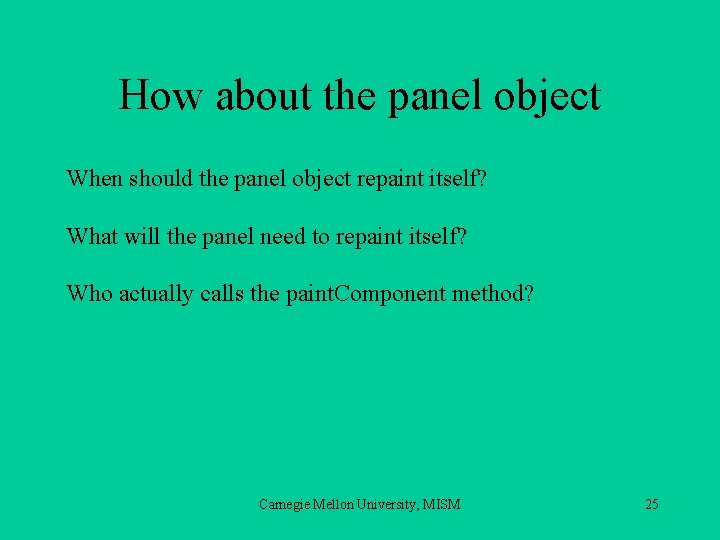
How about the panel object When should the panel object repaint itself? What will the panel need to repaint itself? Who actually calls the paint. Component method? Carnegie Mellon University, MISM 25
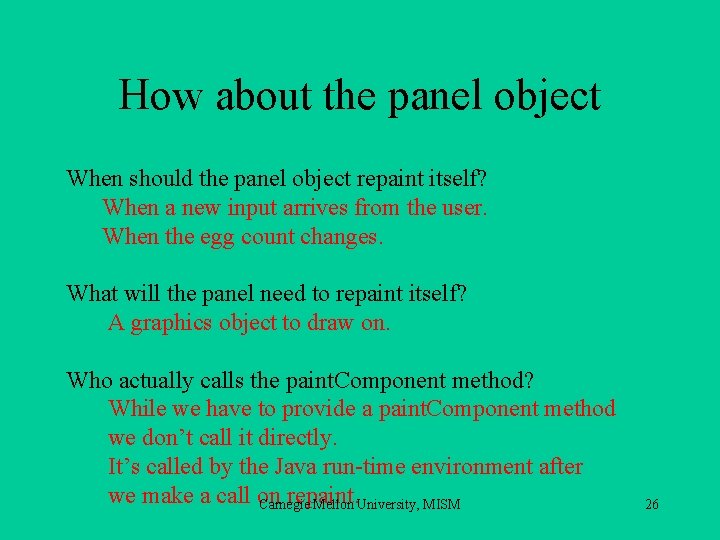
How about the panel object When should the panel object repaint itself? When a new input arrives from the user. When the egg count changes. What will the panel need to repaint itself? A graphics object to draw on. Who actually calls the paint. Component method? While we have to provide a paint. Component method we don’t call it directly. It’s called by the Java run-time environment after we make a call on repaint. Carnegie Mellon University, MISM 26
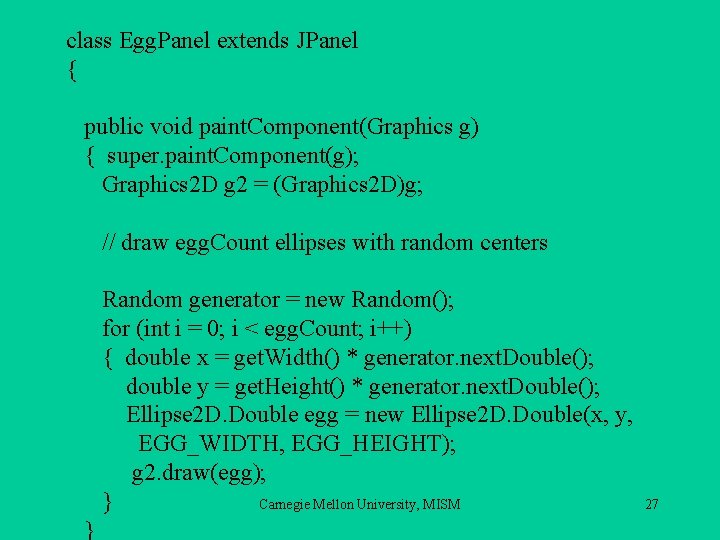
class Egg. Panel extends JPanel { public void paint. Component(Graphics g) { super. paint. Component(g); Graphics 2 D g 2 = (Graphics 2 D)g; // draw egg. Count ellipses with random centers Random generator = new Random(); for (int i = 0; i < egg. Count; i++) { double x = get. Width() * generator. next. Double(); double y = get. Height() * generator. next. Double(); Ellipse 2 D. Double egg = new Ellipse 2 D. Double(x, y, EGG_WIDTH, EGG_HEIGHT); g 2. draw(egg); } Carnegie Mellon University, MISM } 27
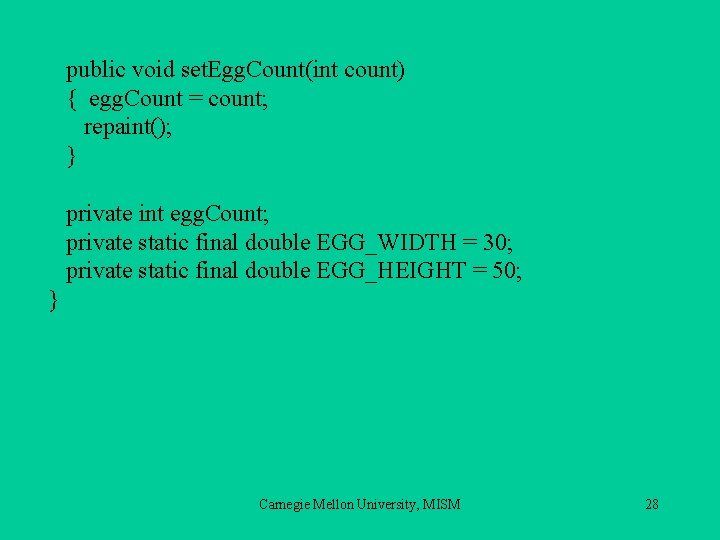
public void set. Egg. Count(int count) { egg. Count = count; repaint(); } private int egg. Count; private static final double EGG_WIDTH = 30; private static final double EGG_HEIGHT = 50; } Carnegie Mellon University, MISM 28
Shared memory in java
Threads java
Threads em java
Ge gi gue gui güe güi
Common gui event types and listener interfaces in java
Rapid gui programming with python and qt
Java gui libraries
Java gui awt
Java gui basics
Gui programmierung java
Picture of java
Java gui thread
Java event handler example
Java gui event handling
Ruby gui toolkit
Java gui for r
I gui
Java gui design
Awt gui
Jframe animation
Graphical user interface history
Java gui fx
Java gui basics
Gui programmierung java
Swing form
Java gui
Java gui basics
Java swing responsive
The awt toolkit window