Programming Graphical User Interface GUI Programming GUI with
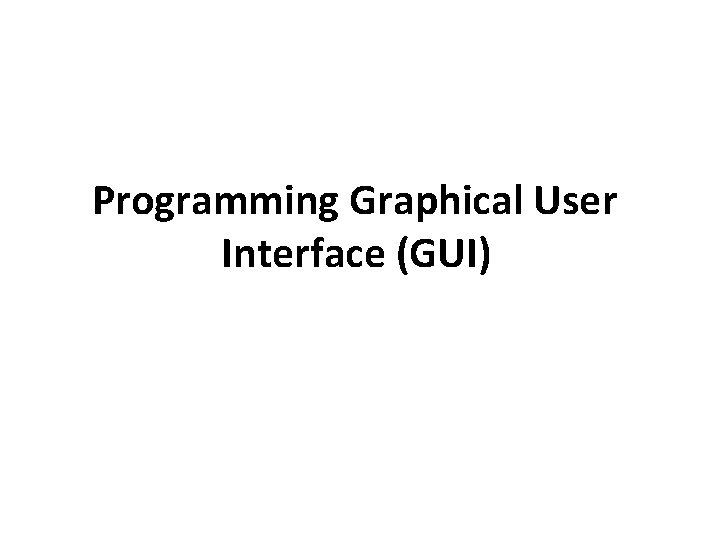
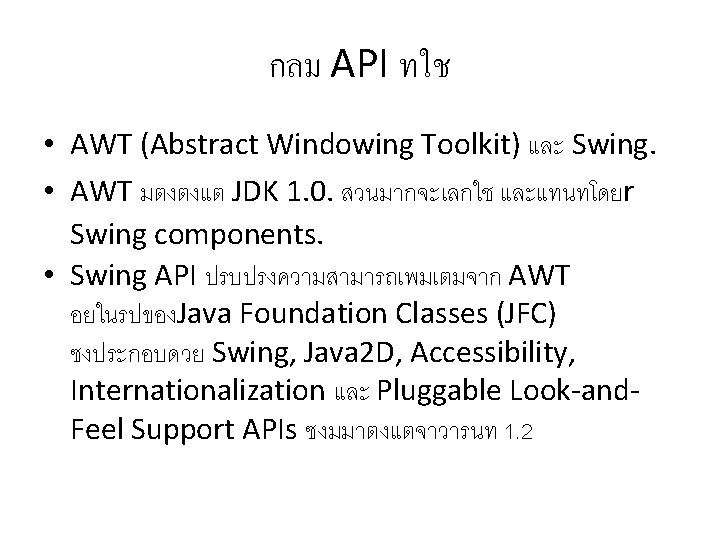
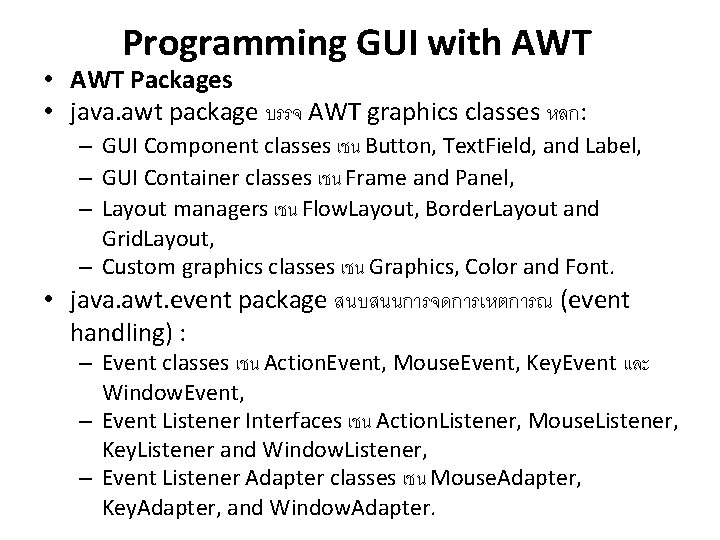
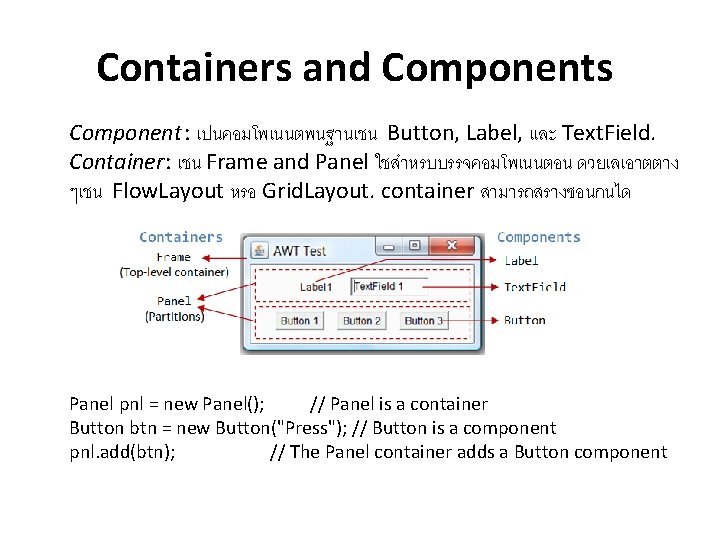
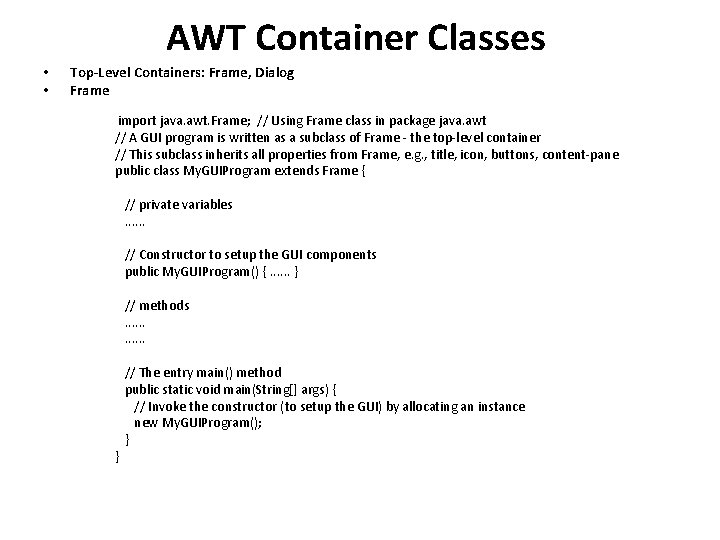
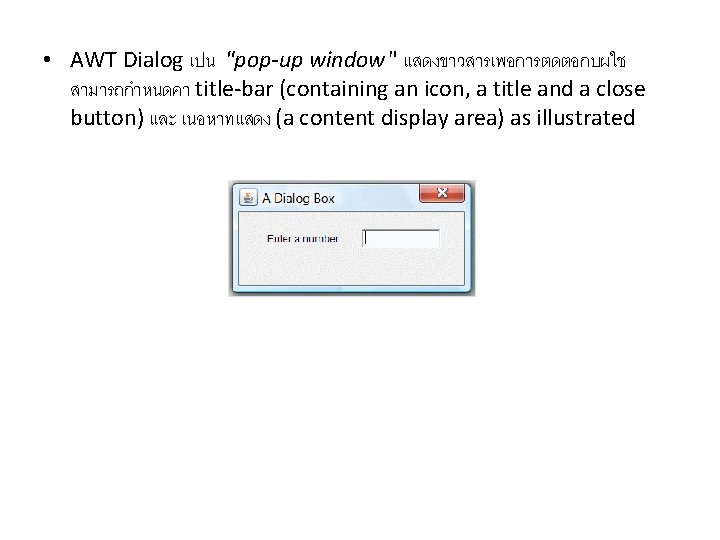
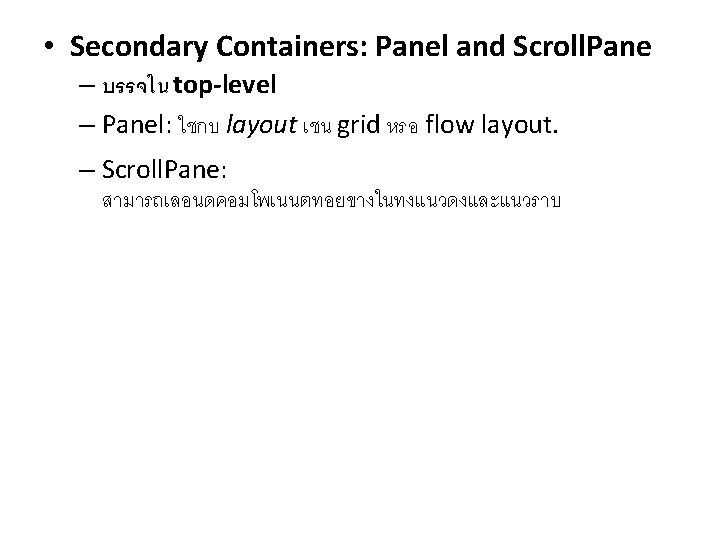
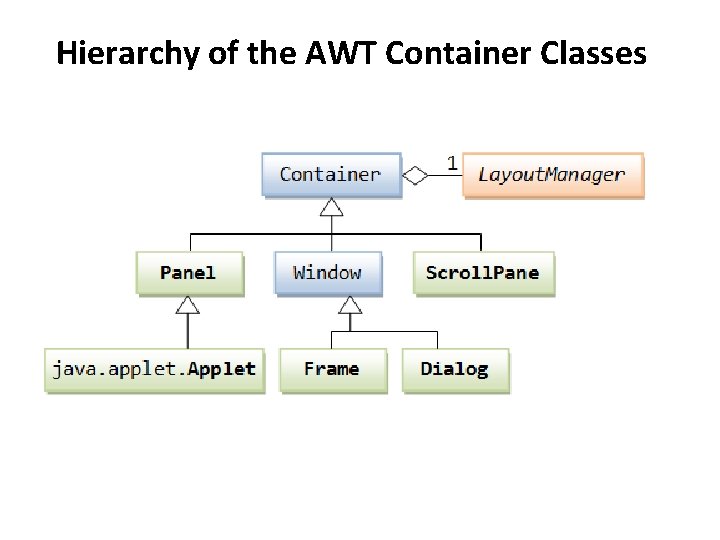
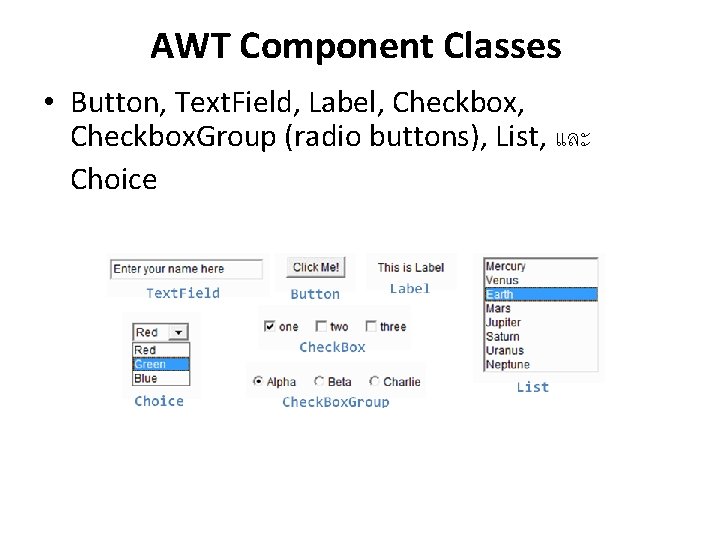
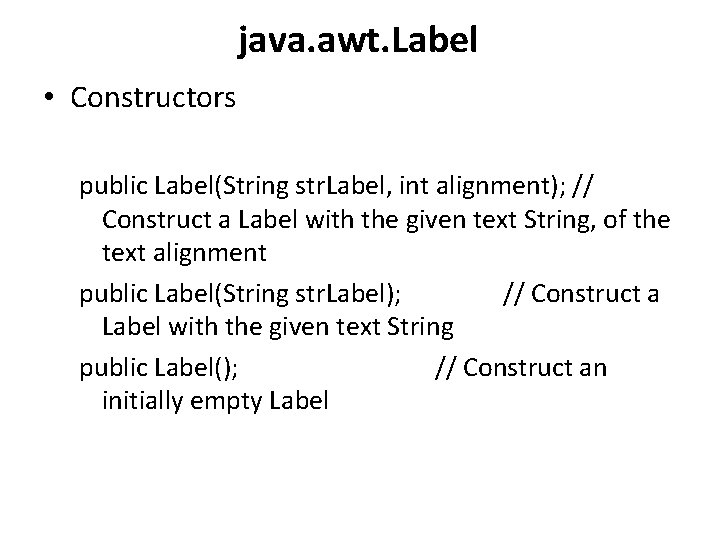
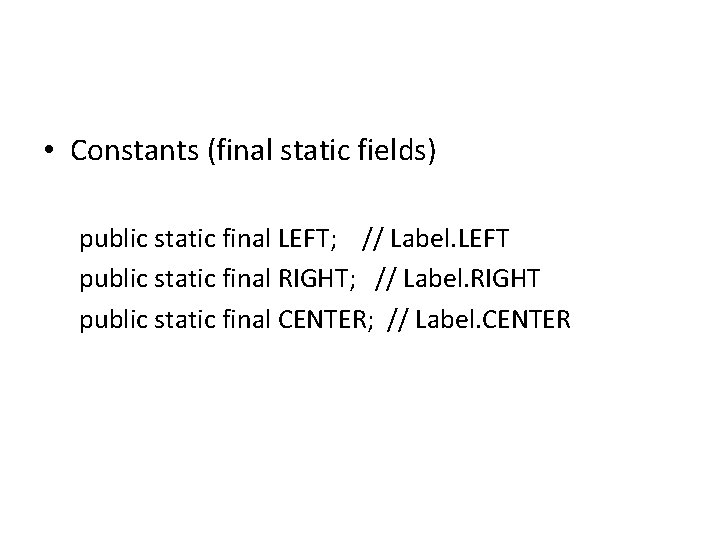
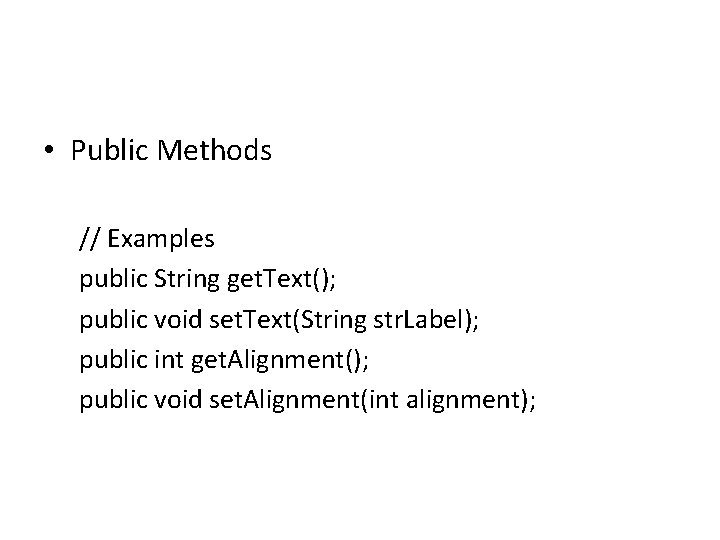
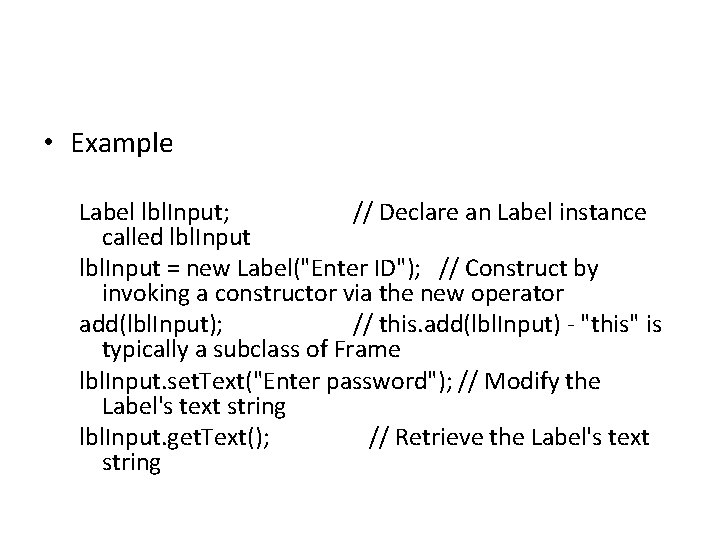
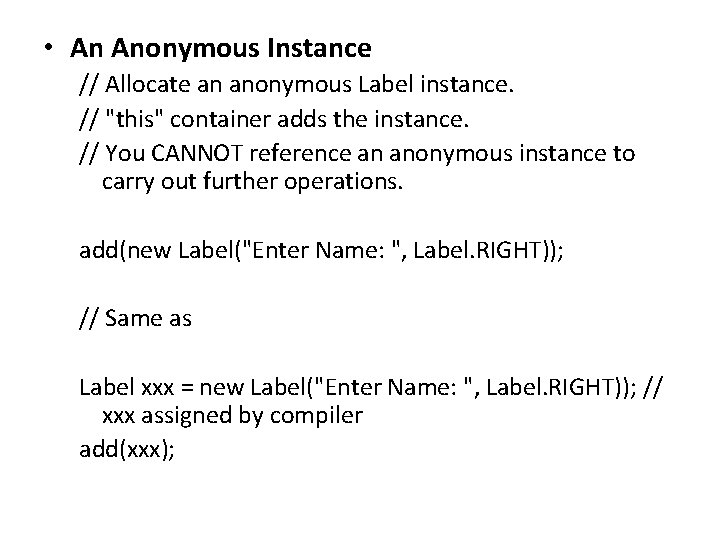
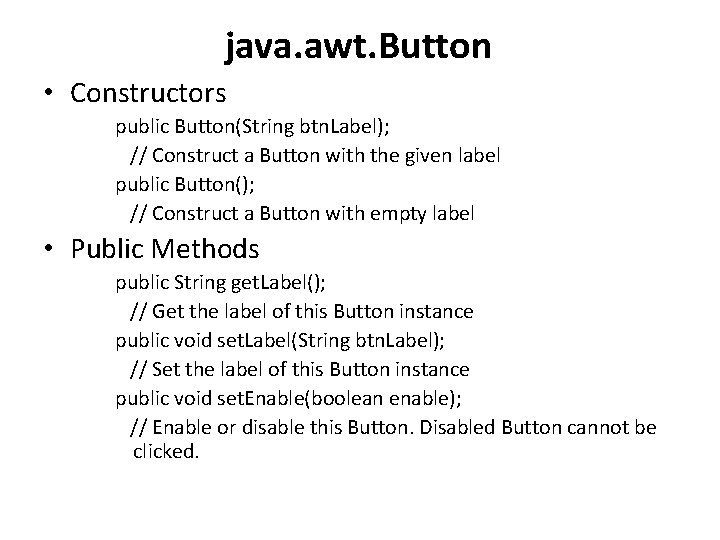
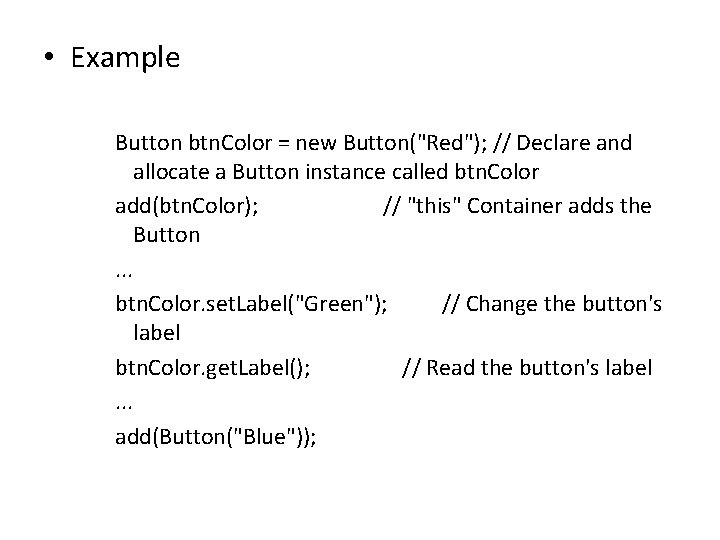
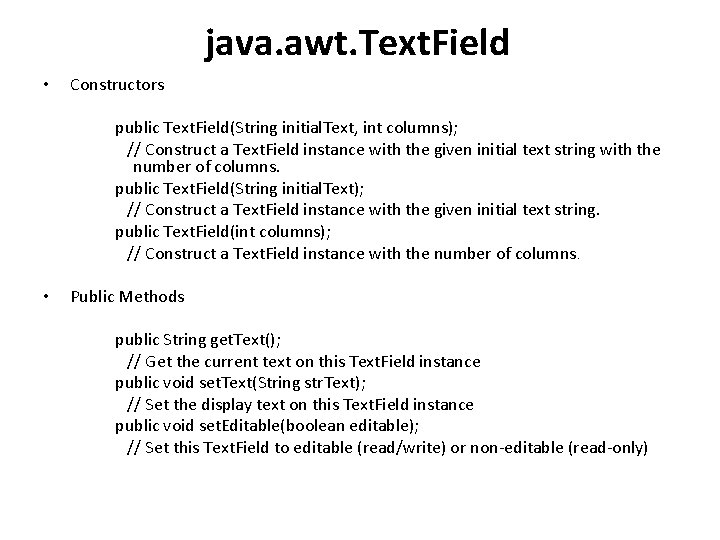
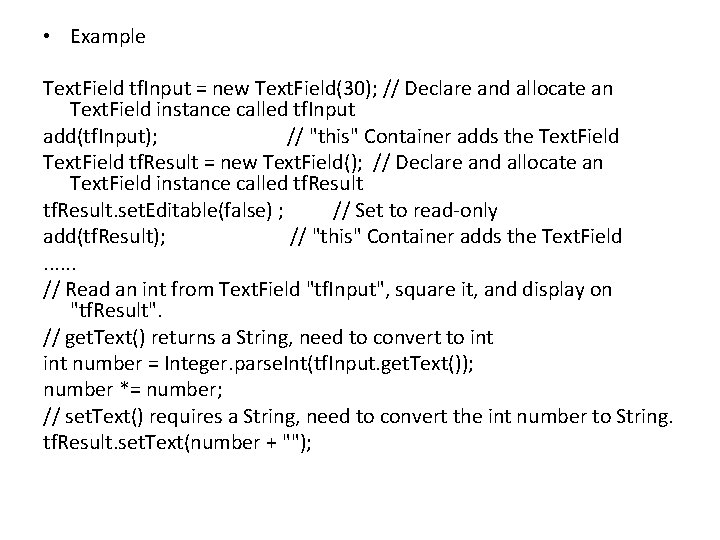
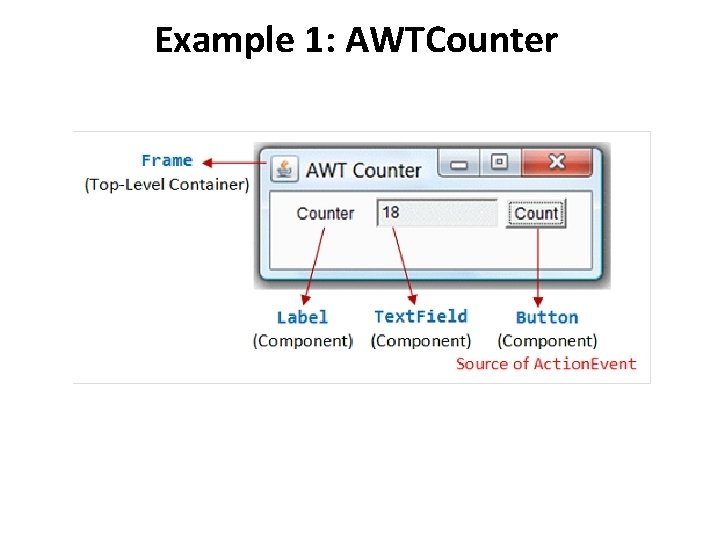
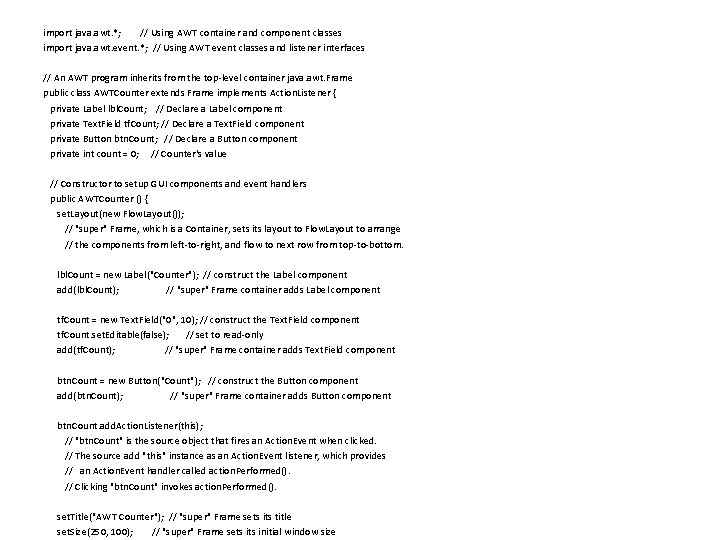
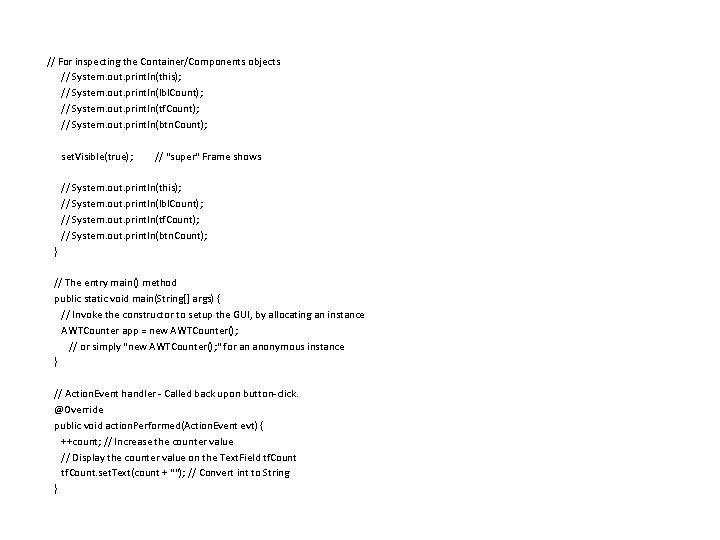
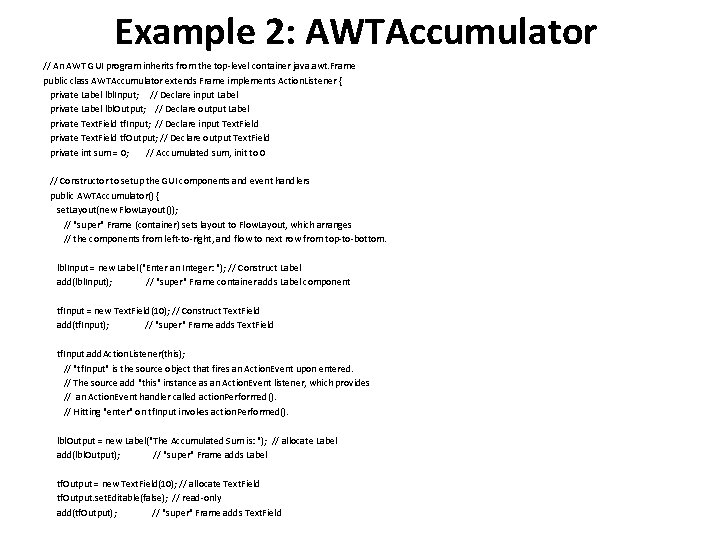
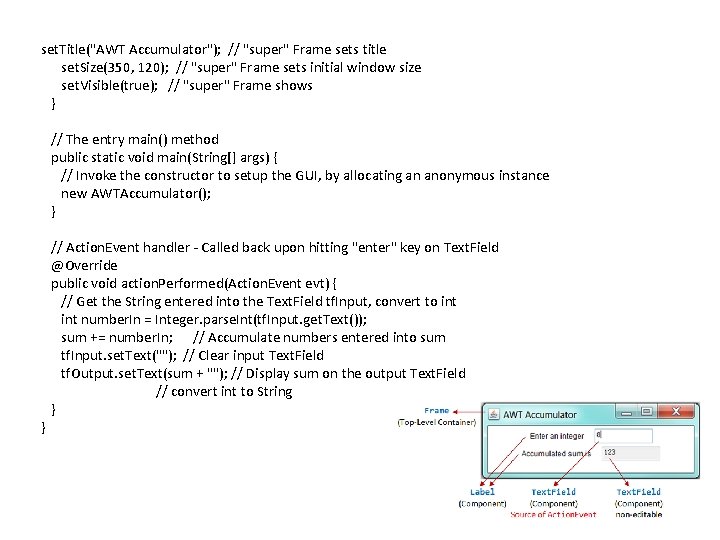
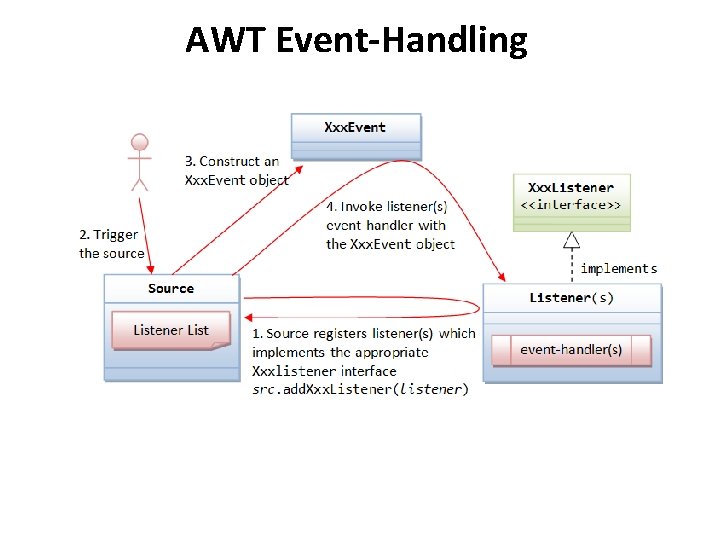
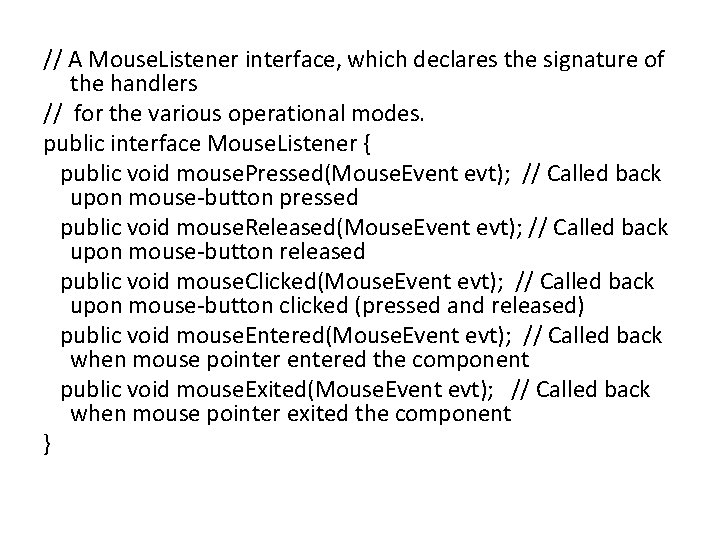
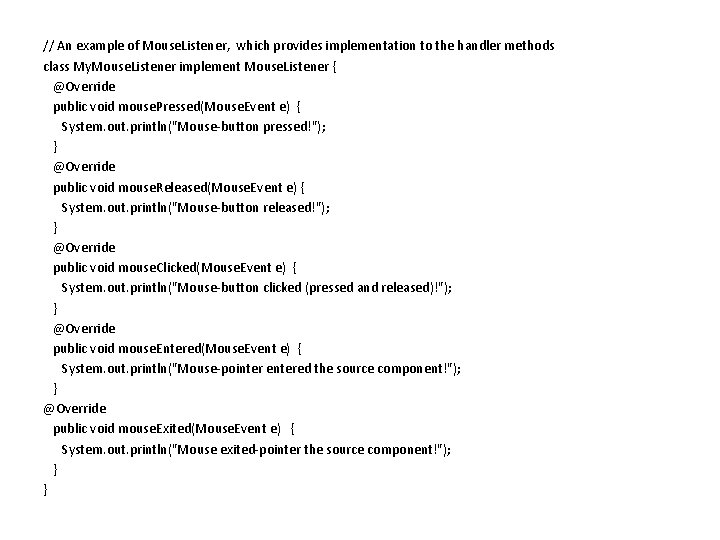
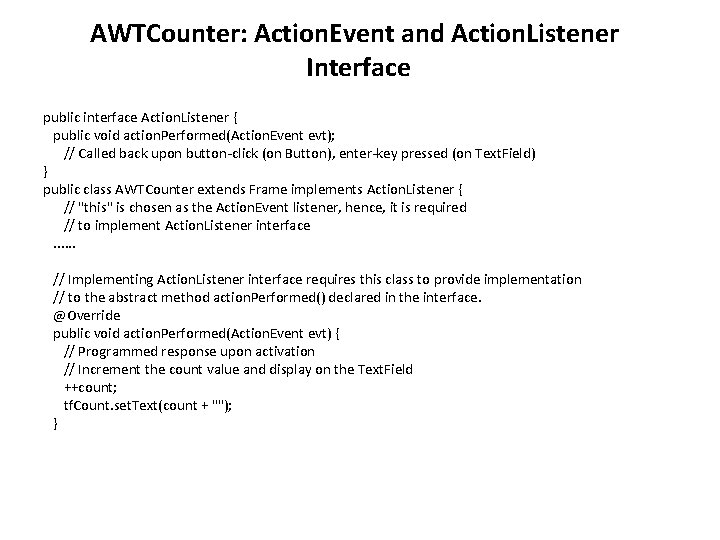
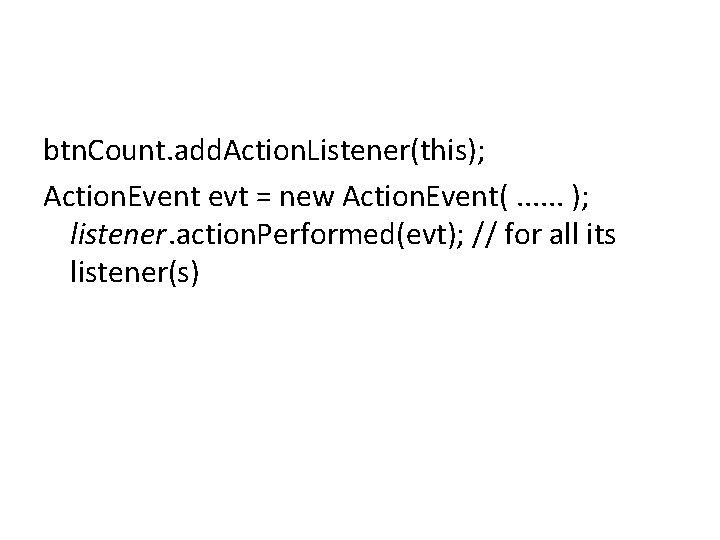
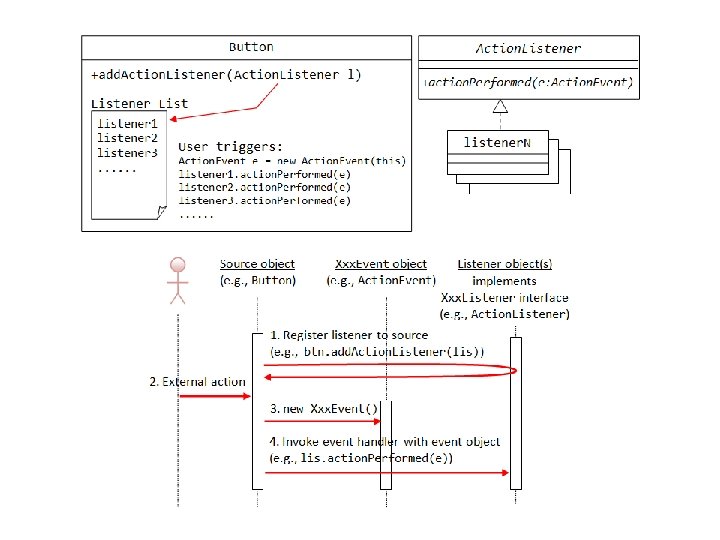
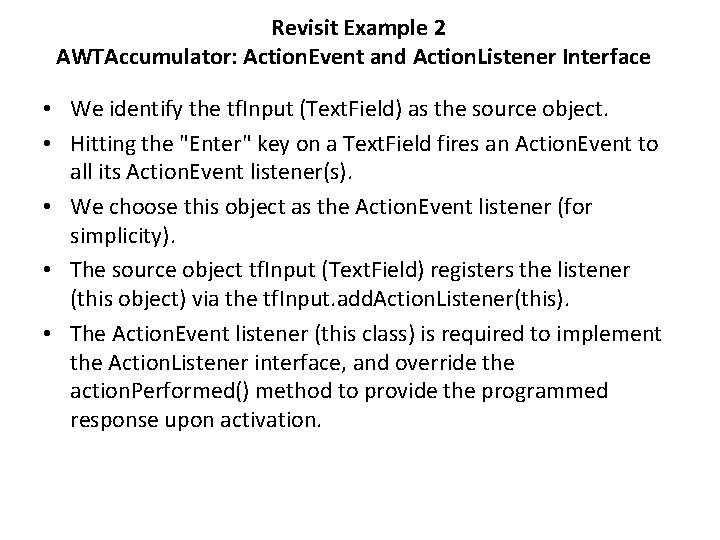
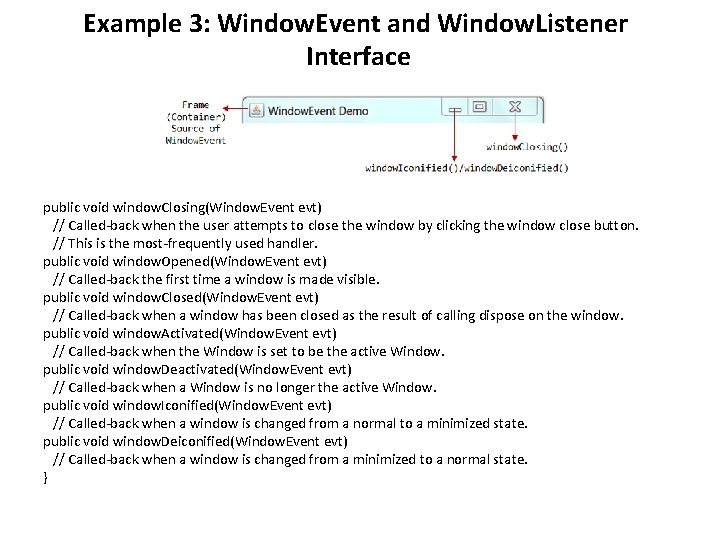
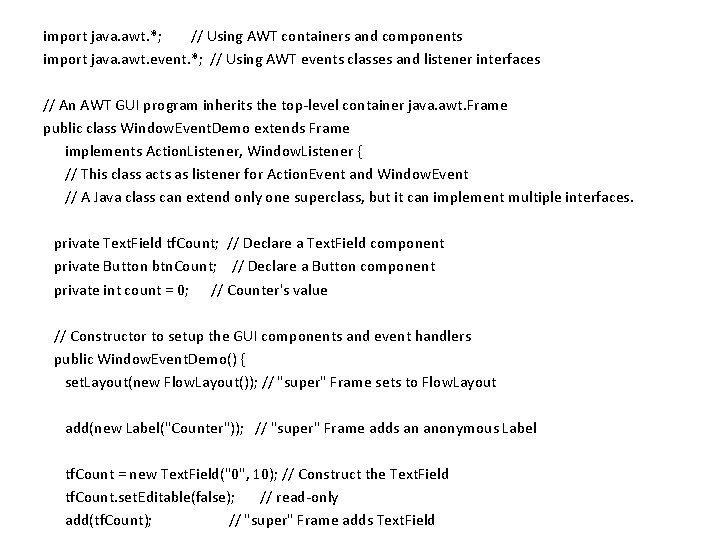
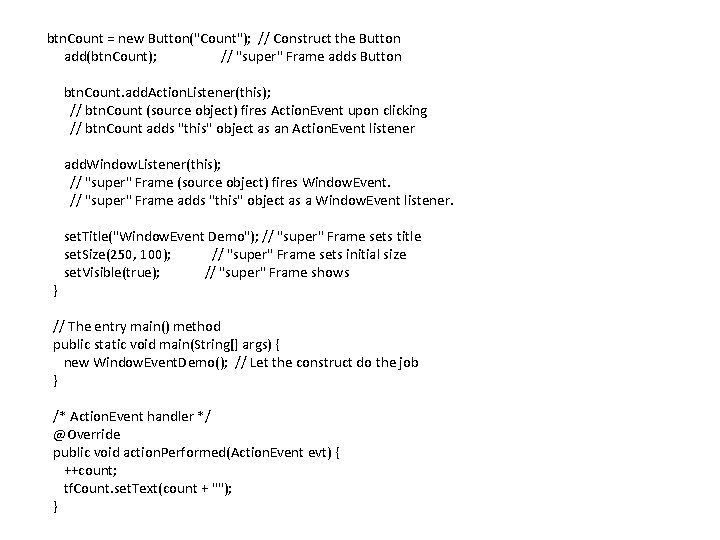
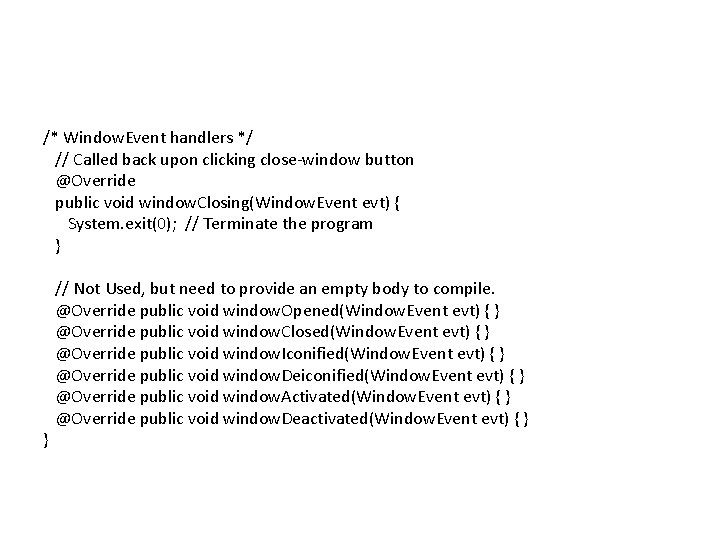
- Slides: 34
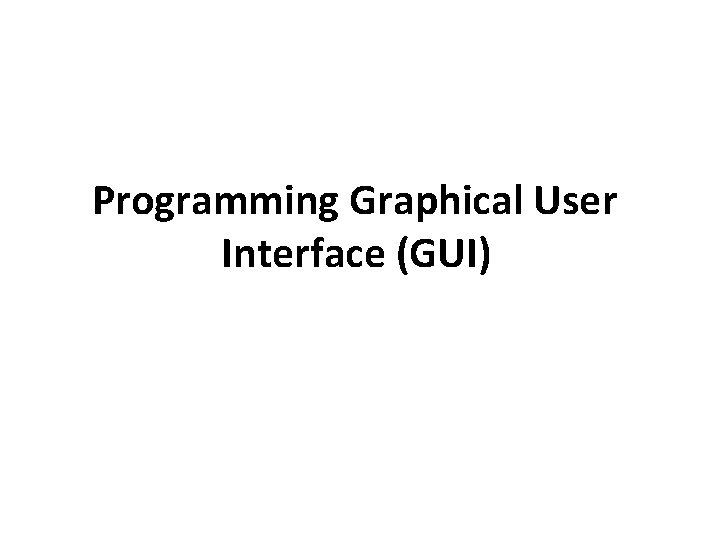
Programming Graphical User Interface (GUI)
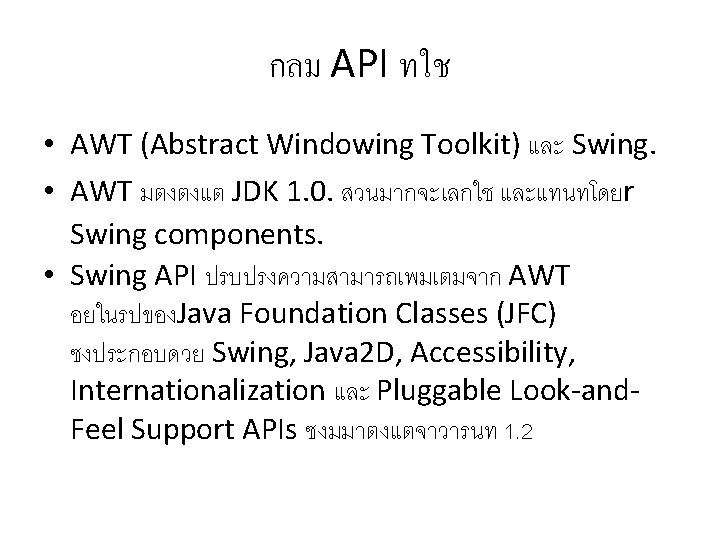
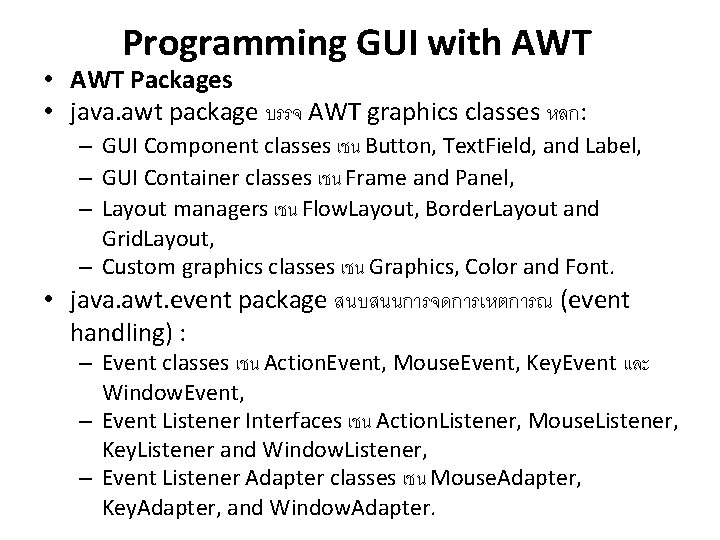
Programming GUI with AWT • AWT Packages • java. awt package บรรจ AWT graphics classes หลก: – GUI Component classes เชน Button, Text. Field, and Label, – GUI Container classes เชน Frame and Panel, – Layout managers เชน Flow. Layout, Border. Layout and Grid. Layout, – Custom graphics classes เชน Graphics, Color and Font. • java. awt. event package สนบสนนการจดการเหตการณ (event handling) : – Event classes เชน Action. Event, Mouse. Event, Key. Event และ Window. Event, – Event Listener Interfaces เชน Action. Listener, Mouse. Listener, Key. Listener and Window. Listener, – Event Listener Adapter classes เชน Mouse. Adapter, Key. Adapter, and Window. Adapter.
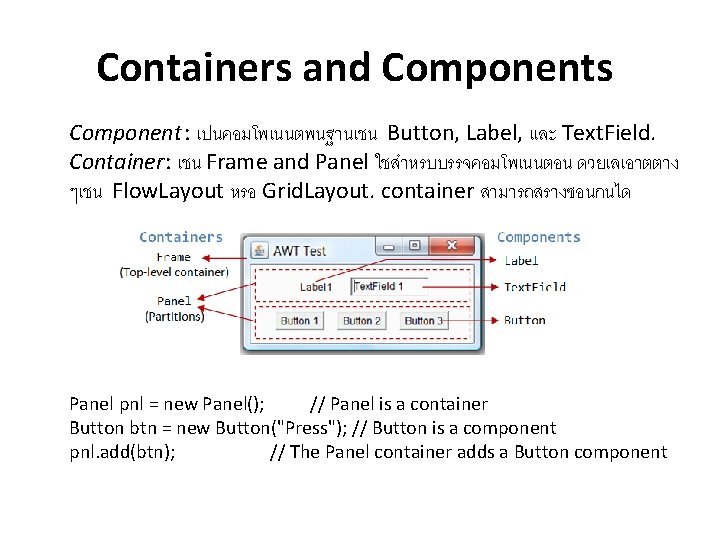
Containers and Components Component : เปนคอมโพเนนตพนฐานเชน Button, Label, และ Text. Field. Container: เชน Frame and Panel ใชสำหรบบรรจคอมโพเนนตอน ดวยเลเอาตตาง ๆเชน Flow. Layout หรอ Grid. Layout. container สามารถสรางซอนกนได Panel pnl = new Panel(); // Panel is a container Button btn = new Button("Press"); // Button is a component pnl. add(btn); // The Panel container adds a Button component
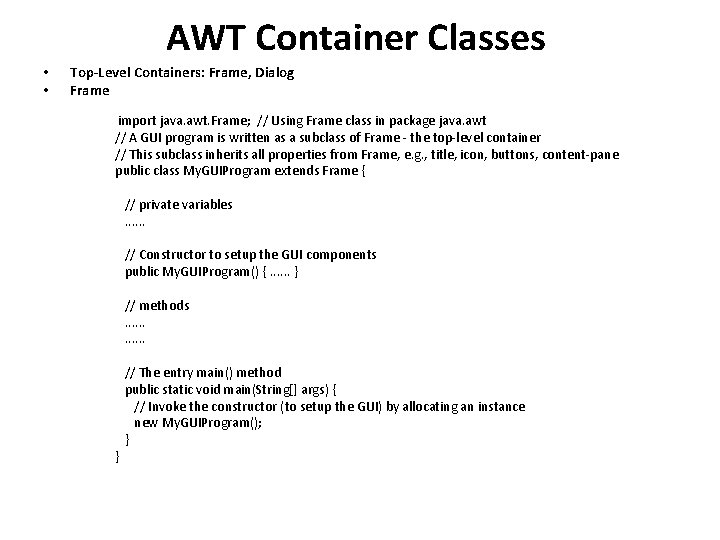
AWT Container Classes • • Top-Level Containers: Frame, Dialog Frame import java. awt. Frame; // Using Frame class in package java. awt // A GUI program is written as a subclass of Frame - the top-level container // This subclass inherits all properties from Frame, e. g. , title, icon, buttons, content-pane public class My. GUIProgram extends Frame { // private variables. . . // Constructor to setup the GUI components public My. GUIProgram() {. . . } // methods. . . } // The entry main() method public static void main(String[] args) { // Invoke the constructor (to setup the GUI) by allocating an instance new My. GUIProgram(); }
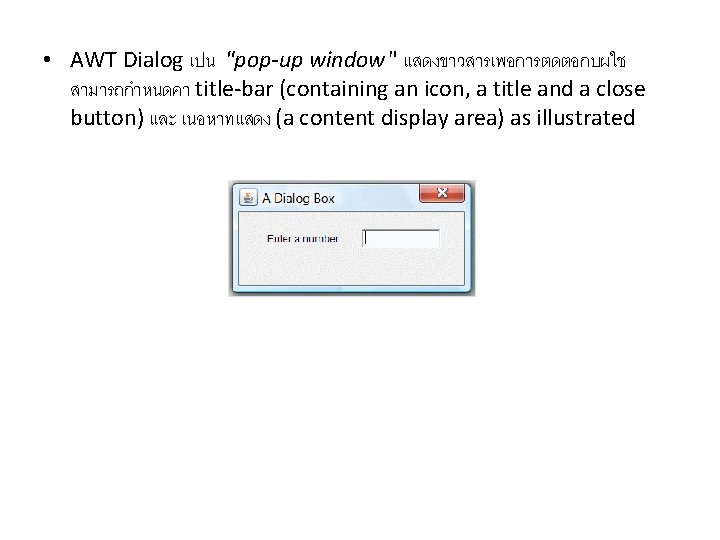
• AWT Dialog เปน "pop-up window " แสดงขาวสารเพอการตดตอกบผใช สามารถกำหนดคา title-bar (containing an icon, a title and a close button) และ เนอหาทแสดง (a content display area) as illustrated
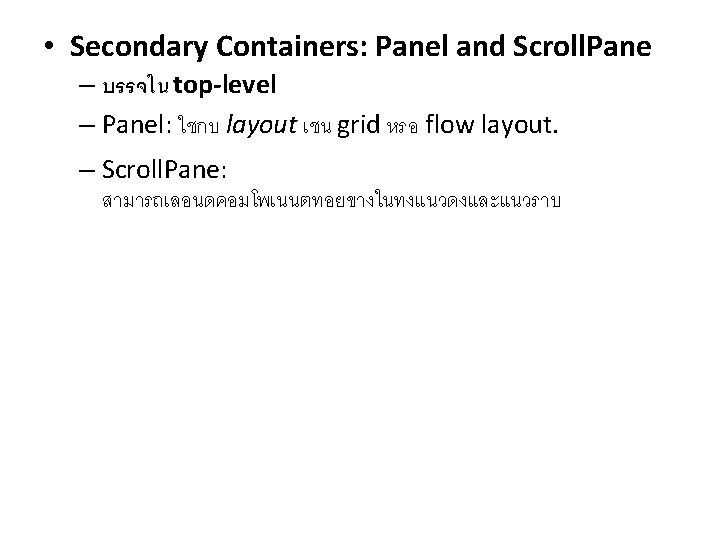
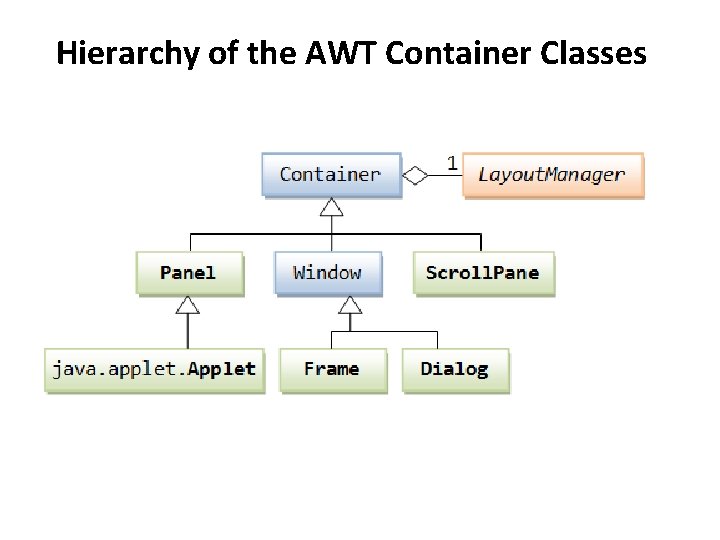
Hierarchy of the AWT Container Classes
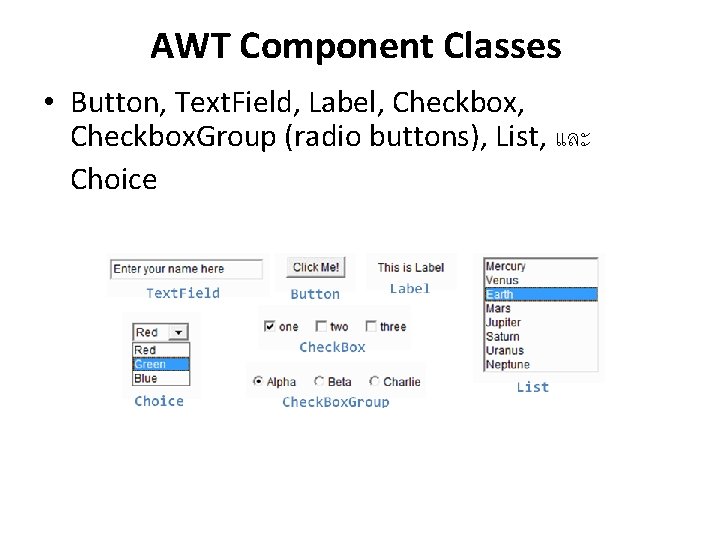
AWT Component Classes • Button, Text. Field, Label, Checkbox. Group (radio buttons), List, และ Choice
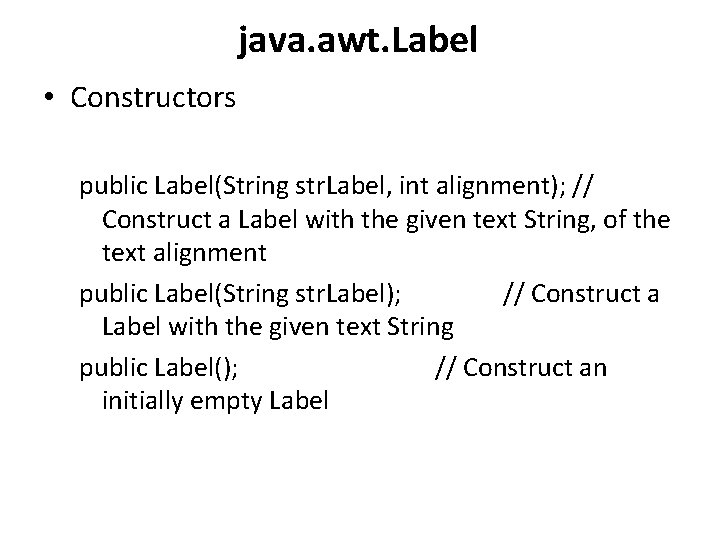
java. awt. Label • Constructors public Label(String str. Label, int alignment); // Construct a Label with the given text String, of the text alignment public Label(String str. Label); // Construct a Label with the given text String public Label(); // Construct an initially empty Label
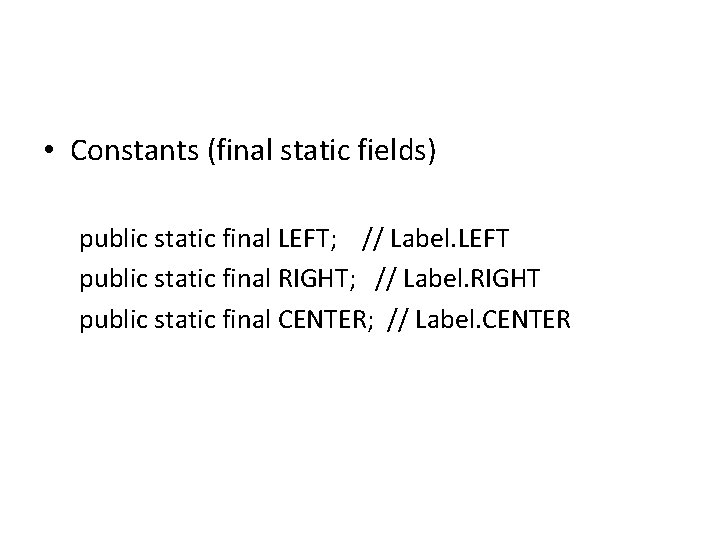
• Constants (final static fields) public static final LEFT; // Label. LEFT public static final RIGHT; // Label. RIGHT public static final CENTER; // Label. CENTER
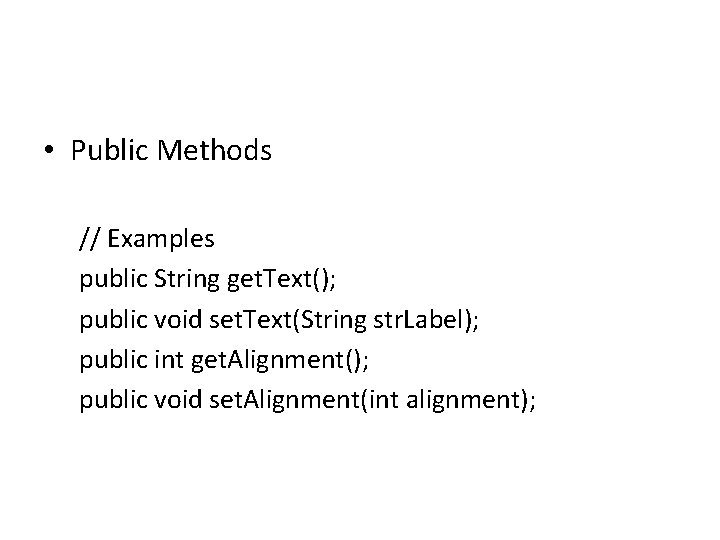
• Public Methods // Examples public String get. Text(); public void set. Text(String str. Label); public int get. Alignment(); public void set. Alignment(int alignment);
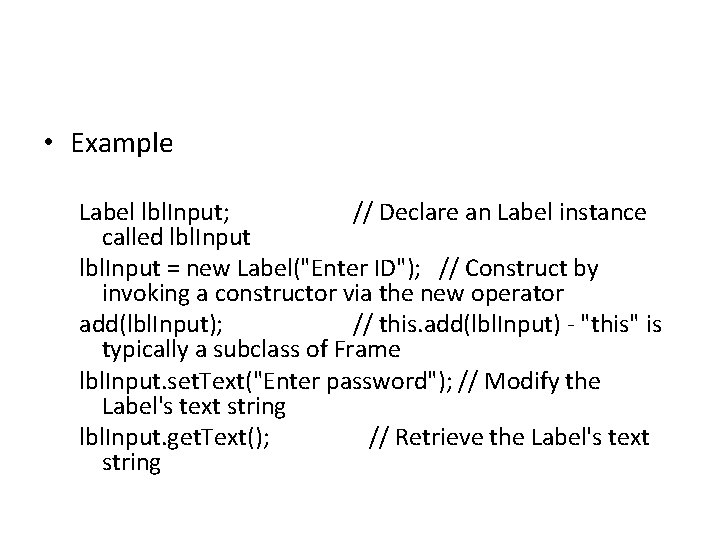
• Example Label lbl. Input; // Declare an Label instance called lbl. Input = new Label("Enter ID"); // Construct by invoking a constructor via the new operator add(lbl. Input); // this. add(lbl. Input) - "this" is typically a subclass of Frame lbl. Input. set. Text("Enter password"); // Modify the Label's text string lbl. Input. get. Text(); // Retrieve the Label's text string
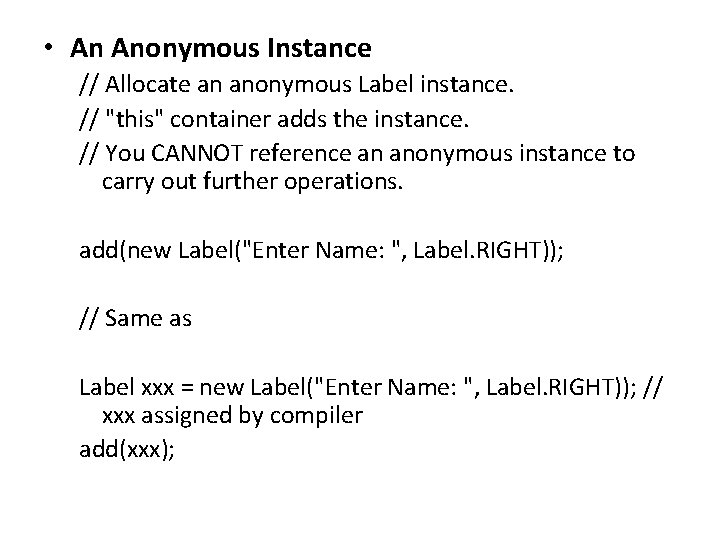
• An Anonymous Instance // Allocate an anonymous Label instance. // "this" container adds the instance. // You CANNOT reference an anonymous instance to carry out further operations. add(new Label("Enter Name: ", Label. RIGHT)); // Same as Label xxx = new Label("Enter Name: ", Label. RIGHT)); // xxx assigned by compiler add(xxx);
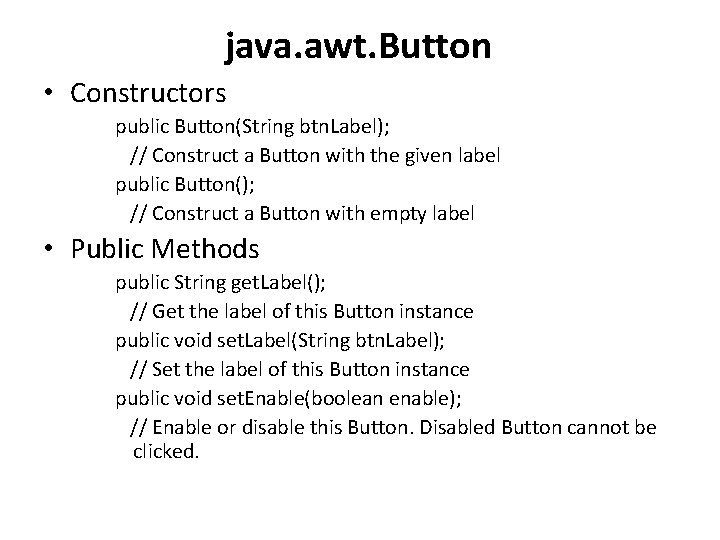
java. awt. Button • Constructors public Button(String btn. Label); // Construct a Button with the given label public Button(); // Construct a Button with empty label • Public Methods public String get. Label(); // Get the label of this Button instance public void set. Label(String btn. Label); // Set the label of this Button instance public void set. Enable(boolean enable); // Enable or disable this Button. Disabled Button cannot be clicked.
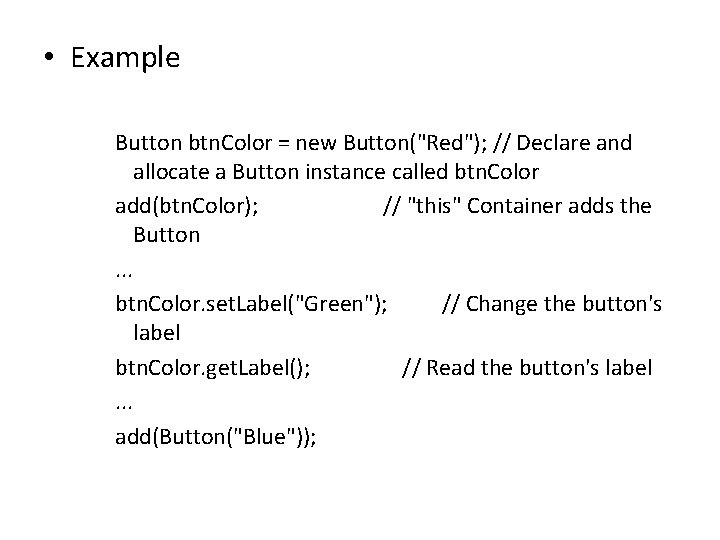
• Example Button btn. Color = new Button("Red"); // Declare and allocate a Button instance called btn. Color add(btn. Color); // "this" Container adds the Button. . . btn. Color. set. Label("Green"); // Change the button's label btn. Color. get. Label(); // Read the button's label. . . add(Button("Blue"));
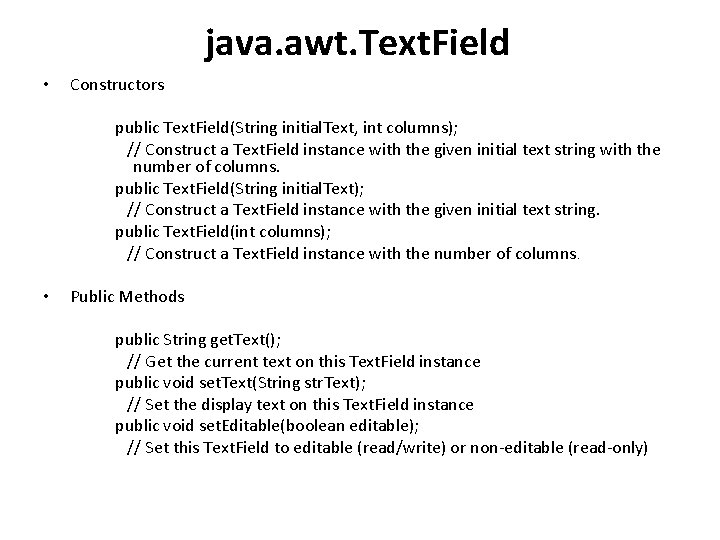
java. awt. Text. Field • Constructors public Text. Field(String initial. Text, int columns); // Construct a Text. Field instance with the given initial text string with the number of columns. public Text. Field(String initial. Text); // Construct a Text. Field instance with the given initial text string. public Text. Field(int columns); // Construct a Text. Field instance with the number of columns. • Public Methods public String get. Text(); // Get the current text on this Text. Field instance public void set. Text(String str. Text); // Set the display text on this Text. Field instance public void set. Editable(boolean editable); // Set this Text. Field to editable (read/write) or non-editable (read-only)
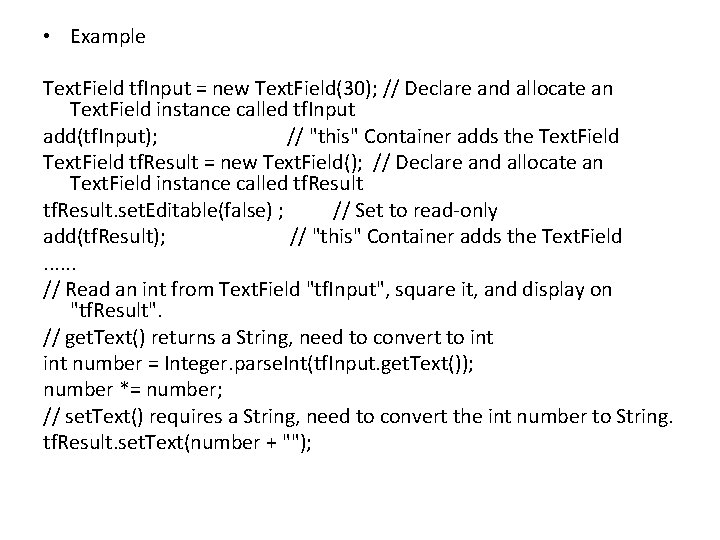
• Example Text. Field tf. Input = new Text. Field(30); // Declare and allocate an Text. Field instance called tf. Input add(tf. Input); // "this" Container adds the Text. Field tf. Result = new Text. Field(); // Declare and allocate an Text. Field instance called tf. Result. set. Editable(false) ; // Set to read-only add(tf. Result); // "this" Container adds the Text. Field. . . // Read an int from Text. Field "tf. Input", square it, and display on "tf. Result". // get. Text() returns a String, need to convert to int number = Integer. parse. Int(tf. Input. get. Text()); number *= number; // set. Text() requires a String, need to convert the int number to String. tf. Result. set. Text(number + "");
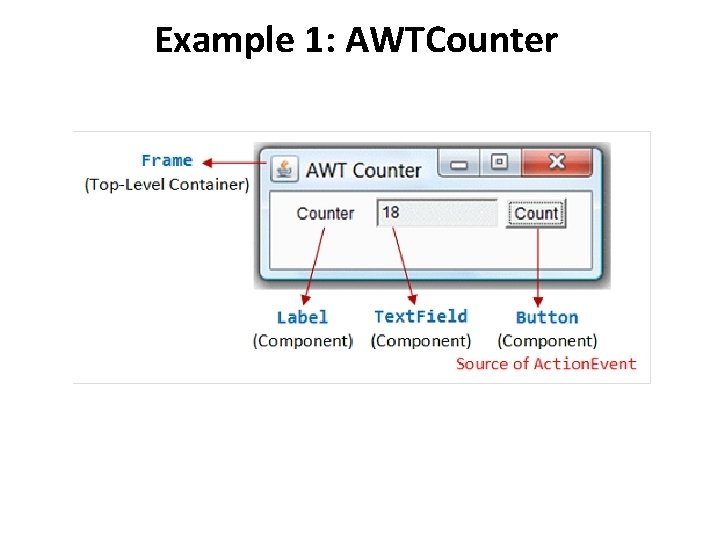
Example 1: AWTCounter
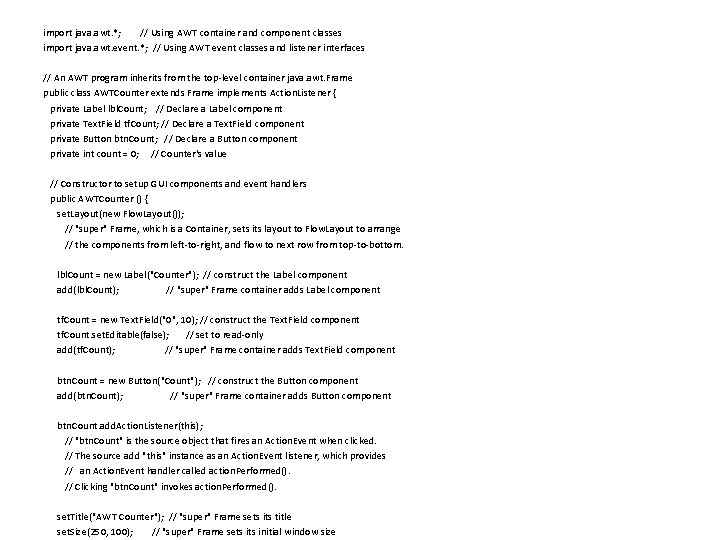
import java. awt. *; // Using AWT container and component classes import java. awt. event. *; // Using AWT event classes and listener interfaces // An AWT program inherits from the top-level container java. awt. Frame public class AWTCounter extends Frame implements Action. Listener { private Label lbl. Count; // Declare a Label component private Text. Field tf. Count; // Declare a Text. Field component private Button btn. Count; // Declare a Button component private int count = 0; // Counter's value // Constructor to setup GUI components and event handlers public AWTCounter () { set. Layout(new Flow. Layout()); // "super" Frame, which is a Container, sets its layout to Flow. Layout to arrange // the components from left-to-right, and flow to next row from top-to-bottom. lbl. Count = new Label("Counter"); // construct the Label component add(lbl. Count); // "super" Frame container adds Label component tf. Count = new Text. Field("0", 10); // construct the Text. Field component tf. Count. set. Editable(false); // set to read-only add(tf. Count); // "super" Frame container adds Text. Field component btn. Count = new Button("Count"); // construct the Button component add(btn. Count); // "super" Frame container adds Button component btn. Count. add. Action. Listener(this); // "btn. Count" is the source object that fires an Action. Event when clicked. // The source add "this" instance as an Action. Event listener, which provides // an Action. Event handler called action. Performed(). // Clicking "btn. Count" invokes action. Performed(). set. Title("AWT Counter"); // "super" Frame sets its title set. Size(250, 100); // "super" Frame sets initial window size
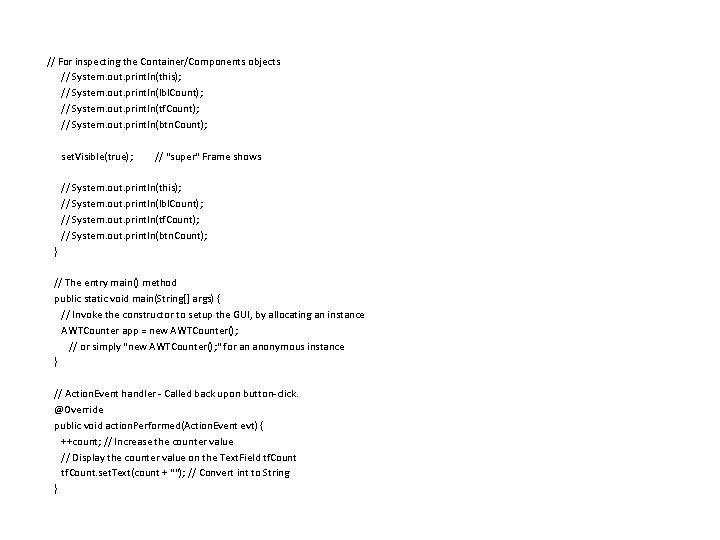
// For inspecting the Container/Components objects // System. out. println(this); // System. out. println(lbl. Count); // System. out. println(tf. Count); // System. out. println(btn. Count); set. Visible(true); // "super" Frame shows // System. out. println(this); // System. out. println(lbl. Count); // System. out. println(tf. Count); // System. out. println(btn. Count); } // The entry main() method public static void main(String[] args) { // Invoke the constructor to setup the GUI, by allocating an instance AWTCounter app = new AWTCounter(); // or simply "new AWTCounter(); " for an anonymous instance } // Action. Event handler - Called back upon button-click. @Override public void action. Performed(Action. Event evt) { ++count; // Increase the counter value // Display the counter value on the Text. Field tf. Count. set. Text(count + ""); // Convert int to String }
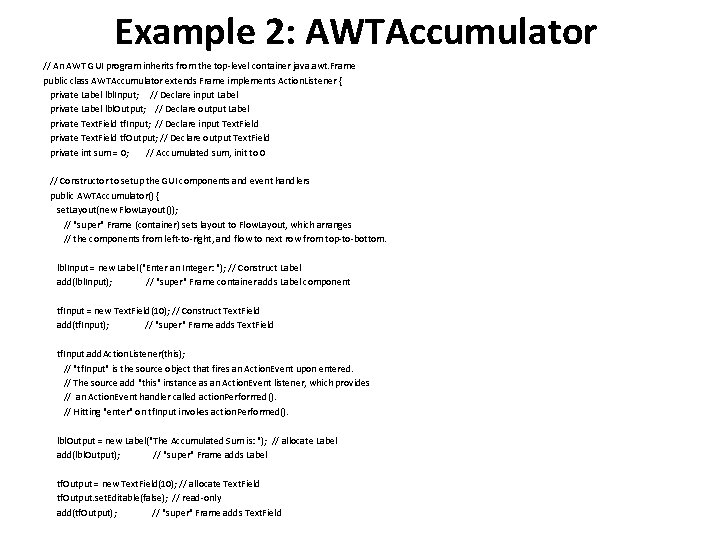
Example 2: AWTAccumulator // An AWT GUI program inherits from the top-level container java. awt. Frame public class AWTAccumulator extends Frame implements Action. Listener { private Label lbl. Input; // Declare input Label private Label lbl. Output; // Declare output Label private Text. Field tf. Input; // Declare input Text. Field private Text. Field tf. Output; // Declare output Text. Field private int sum = 0; // Accumulated sum, init to 0 // Constructor to setup the GUI components and event handlers public AWTAccumulator() { set. Layout(new Flow. Layout()); // "super" Frame (container) sets layout to Flow. Layout, which arranges // the components from left-to-right, and flow to next row from top-to-bottom. lbl. Input = new Label("Enter an Integer: "); // Construct Label add(lbl. Input); // "super" Frame container adds Label component tf. Input = new Text. Field(10); // Construct Text. Field add(tf. Input); // "super" Frame adds Text. Field tf. Input. add. Action. Listener(this); // "tf. Input" is the source object that fires an Action. Event upon entered. // The source add "this" instance as an Action. Event listener, which provides // an Action. Event handler called action. Performed(). // Hitting "enter" on tf. Input invokes action. Performed(). lbl. Output = new Label("The Accumulated Sum is: "); // allocate Label add(lbl. Output); // "super" Frame adds Label tf. Output = new Text. Field(10); // allocate Text. Field tf. Output. set. Editable(false); // read-only add(tf. Output); // "super" Frame adds Text. Field
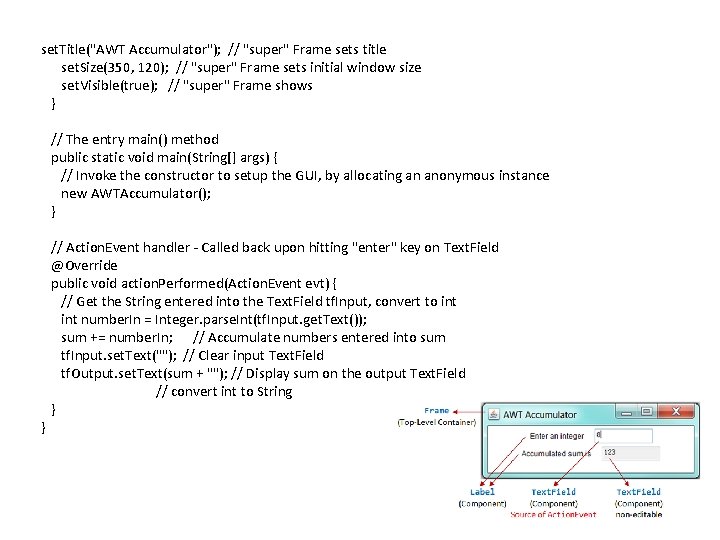
set. Title("AWT Accumulator"); // "super" Frame sets title set. Size(350, 120); // "super" Frame sets initial window size set. Visible(true); // "super" Frame shows } // The entry main() method public static void main(String[] args) { // Invoke the constructor to setup the GUI, by allocating an anonymous instance new AWTAccumulator(); } } // Action. Event handler - Called back upon hitting "enter" key on Text. Field @Override public void action. Performed(Action. Event evt) { // Get the String entered into the Text. Field tf. Input, convert to int number. In = Integer. parse. Int(tf. Input. get. Text()); sum += number. In; // Accumulate numbers entered into sum tf. Input. set. Text(""); // Clear input Text. Field tf. Output. set. Text(sum + ""); // Display sum on the output Text. Field // convert int to String }
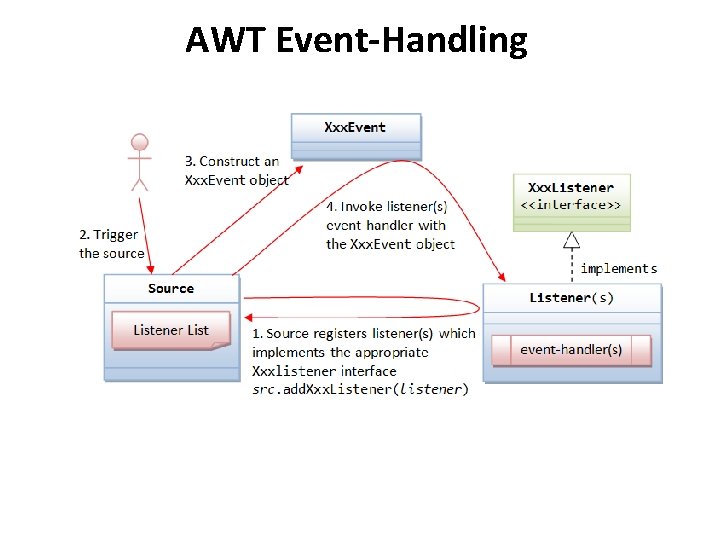
AWT Event-Handling
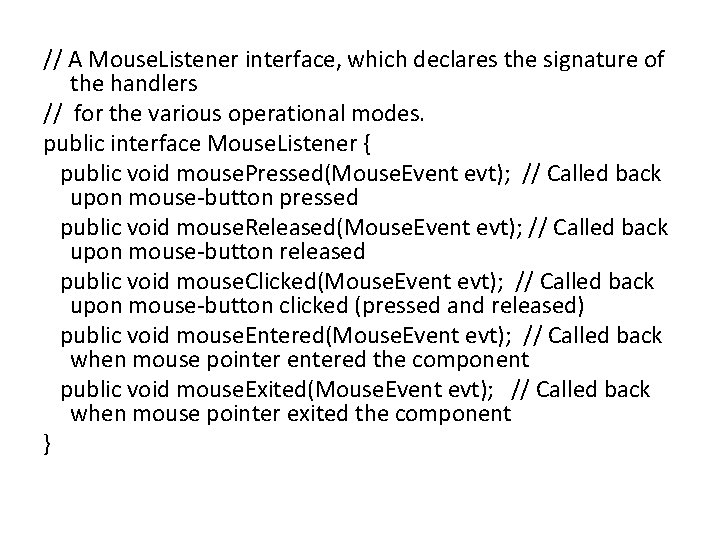
// A Mouse. Listener interface, which declares the signature of the handlers // for the various operational modes. public interface Mouse. Listener { public void mouse. Pressed(Mouse. Event evt); // Called back upon mouse-button pressed public void mouse. Released(Mouse. Event evt); // Called back upon mouse-button released public void mouse. Clicked(Mouse. Event evt); // Called back upon mouse-button clicked (pressed and released) public void mouse. Entered(Mouse. Event evt); // Called back when mouse pointer entered the component public void mouse. Exited(Mouse. Event evt); // Called back when mouse pointer exited the component }
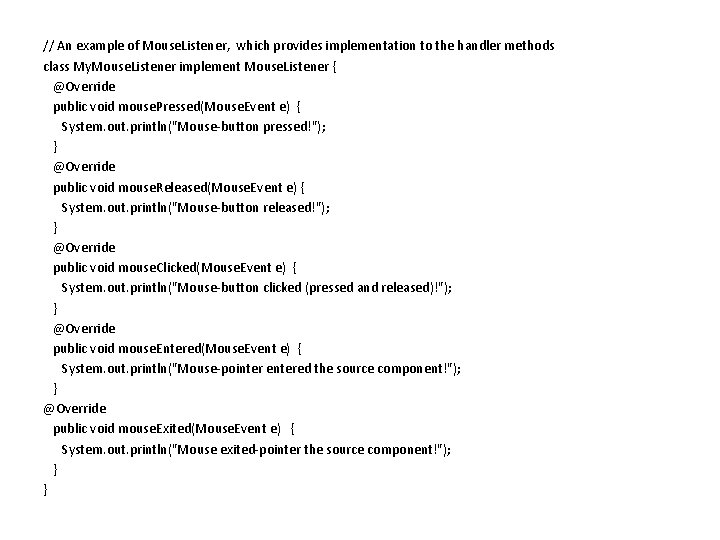
// An example of Mouse. Listener, which provides implementation to the handler methods class My. Mouse. Listener implement Mouse. Listener { @Override public void mouse. Pressed(Mouse. Event e) { System. out. println("Mouse-button pressed!"); } @Override public void mouse. Released(Mouse. Event e) { System. out. println("Mouse-button released!"); } @Override public void mouse. Clicked(Mouse. Event e) { System. out. println("Mouse-button clicked (pressed and released)!"); } @Override public void mouse. Entered(Mouse. Event e) { System. out. println("Mouse-pointer entered the source component!"); } @Override public void mouse. Exited(Mouse. Event e) { System. out. println("Mouse exited-pointer the source component!"); } }
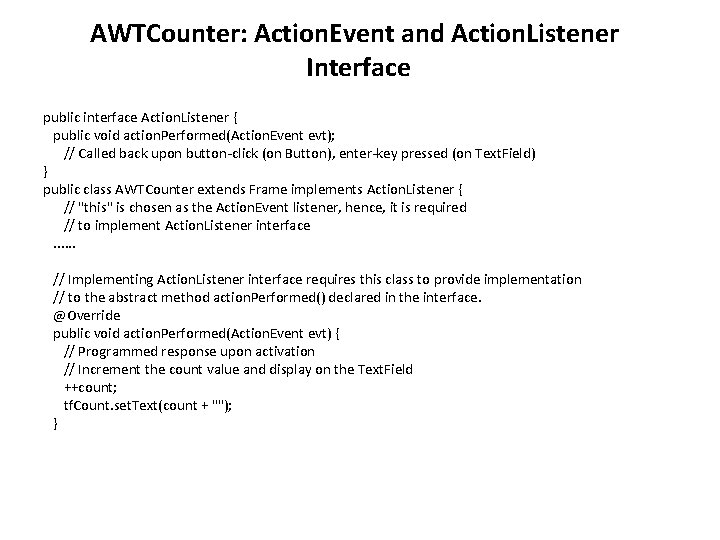
AWTCounter: Action. Event and Action. Listener Interface public interface Action. Listener { public void action. Performed(Action. Event evt); // Called back upon button-click (on Button), enter-key pressed (on Text. Field) } public class AWTCounter extends Frame implements Action. Listener { // "this" is chosen as the Action. Event listener, hence, it is required // to implement Action. Listener interface. . . // Implementing Action. Listener interface requires this class to provide implementation // to the abstract method action. Performed() declared in the interface. @Override public void action. Performed(Action. Event evt) { // Programmed response upon activation // Increment the count value and display on the Text. Field ++count; tf. Count. set. Text(count + ""); }
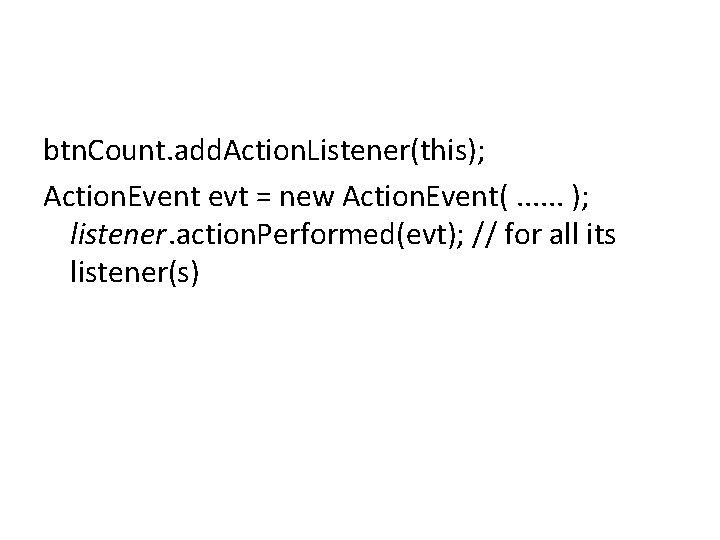
btn. Count. add. Action. Listener(this); Action. Event evt = new Action. Event(. . . ); listener. action. Performed(evt); // for all its listener(s)
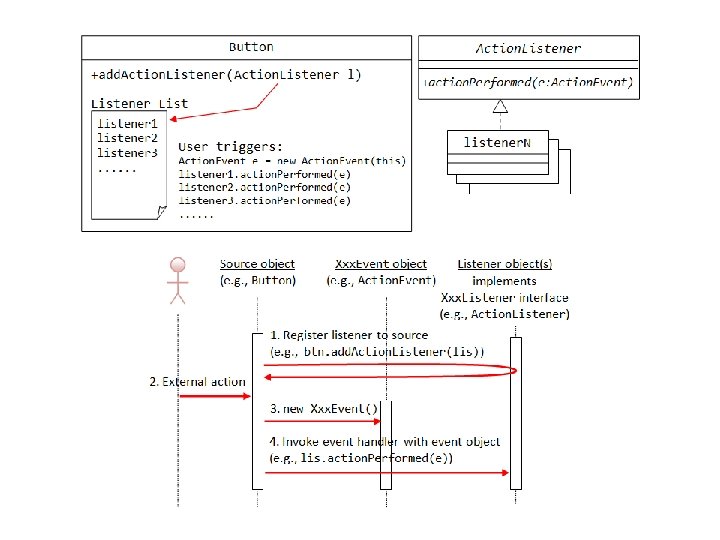
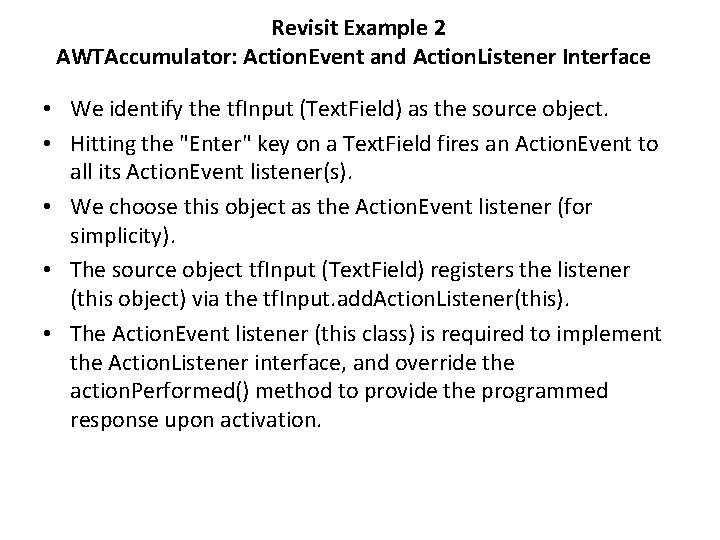
Revisit Example 2 AWTAccumulator: Action. Event and Action. Listener Interface • We identify the tf. Input (Text. Field) as the source object. • Hitting the "Enter" key on a Text. Field fires an Action. Event to all its Action. Event listener(s). • We choose this object as the Action. Event listener (for simplicity). • The source object tf. Input (Text. Field) registers the listener (this object) via the tf. Input. add. Action. Listener(this). • The Action. Event listener (this class) is required to implement the Action. Listener interface, and override the action. Performed() method to provide the programmed response upon activation.
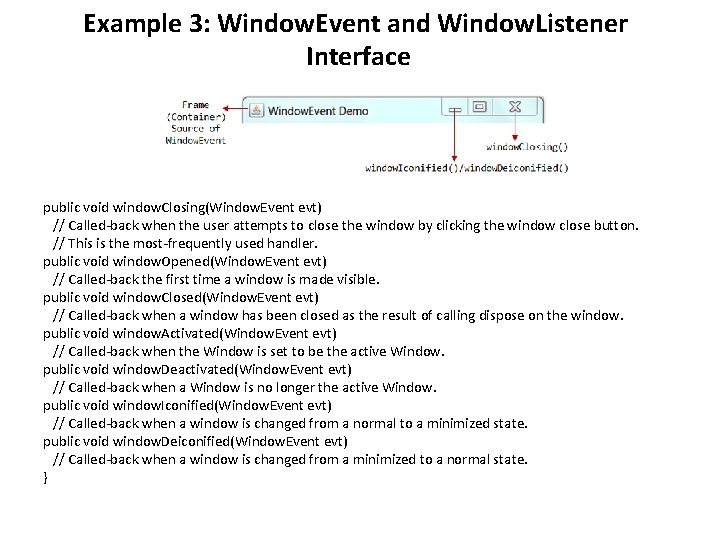
Example 3: Window. Event and Window. Listener Interface public void window. Closing(Window. Event evt) // Called-back when the user attempts to close the window by clicking the window close button. // This is the most-frequently used handler. public void window. Opened(Window. Event evt) // Called-back the first time a window is made visible. public void window. Closed(Window. Event evt) // Called-back when a window has been closed as the result of calling dispose on the window. public void window. Activated(Window. Event evt) // Called-back when the Window is set to be the active Window. public void window. Deactivated(Window. Event evt) // Called-back when a Window is no longer the active Window. public void window. Iconified(Window. Event evt) // Called-back when a window is changed from a normal to a minimized state. public void window. Deiconified(Window. Event evt) // Called-back when a window is changed from a minimized to a normal state. }
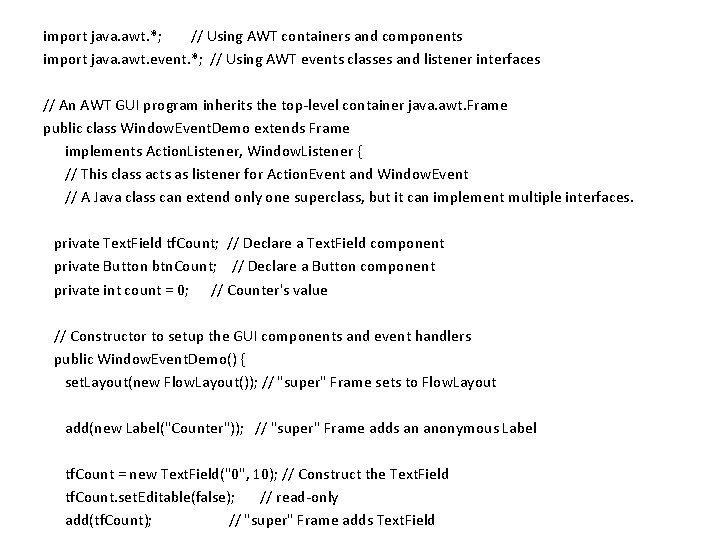
import java. awt. *; // Using AWT containers and components import java. awt. event. *; // Using AWT events classes and listener interfaces // An AWT GUI program inherits the top-level container java. awt. Frame public class Window. Event. Demo extends Frame implements Action. Listener, Window. Listener { // This class acts as listener for Action. Event and Window. Event // A Java class can extend only one superclass, but it can implement multiple interfaces. private Text. Field tf. Count; // Declare a Text. Field component private Button btn. Count; // Declare a Button component private int count = 0; // Counter's value // Constructor to setup the GUI components and event handlers public Window. Event. Demo() { set. Layout(new Flow. Layout()); // "super" Frame sets to Flow. Layout add(new Label("Counter")); // "super" Frame adds an anonymous Label tf. Count = new Text. Field("0", 10); // Construct the Text. Field tf. Count. set. Editable(false); // read-only add(tf. Count); // "super" Frame adds Text. Field
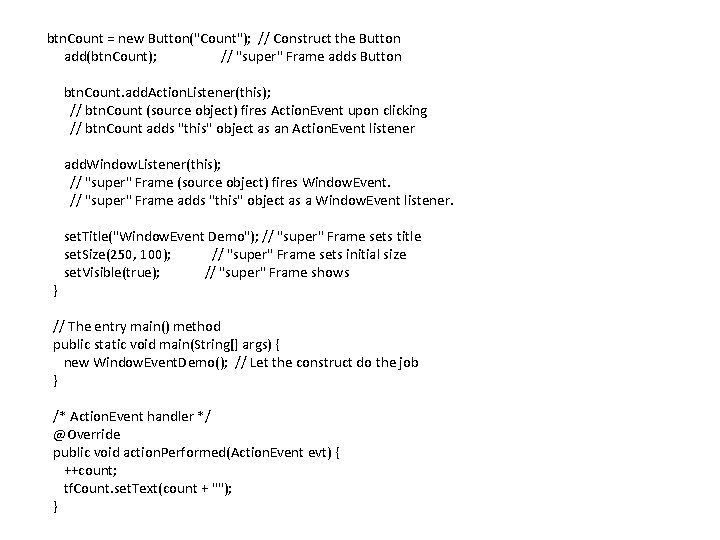
btn. Count = new Button("Count"); // Construct the Button add(btn. Count); // "super" Frame adds Button btn. Count. add. Action. Listener(this); // btn. Count (source object) fires Action. Event upon clicking // btn. Count adds "this" object as an Action. Event listener add. Window. Listener(this); // "super" Frame (source object) fires Window. Event. // "super" Frame adds "this" object as a Window. Event listener. } set. Title("Window. Event Demo"); // "super" Frame sets title set. Size(250, 100); // "super" Frame sets initial size set. Visible(true); // "super" Frame shows // The entry main() method public static void main(String[] args) { new Window. Event. Demo(); // Let the construct do the job } /* Action. Event handler */ @Override public void action. Performed(Action. Event evt) { ++count; tf. Count. set. Text(count + ""); }
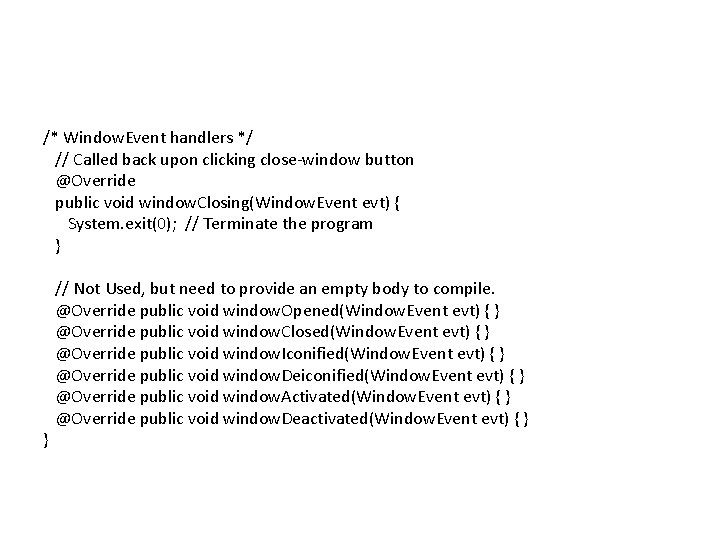
/* Window. Event handlers */ // Called back upon clicking close-window button @Override public void window. Closing(Window. Event evt) { System. exit(0); // Terminate the program } } // Not Used, but need to provide an empty body to compile. @Override public void window. Opened(Window. Event evt) { } @Override public void window. Closed(Window. Event evt) { } @Override public void window. Iconified(Window. Event evt) { } @Override public void window. Deiconified(Window. Event evt) { } @Override public void window. Activated(Window. Event evt) { } @Override public void window. Deactivated(Window. Event evt) { }
Characteristics of graphical user interface
Basic principle of input design
User interface history
Unix gui
Graphical user interface in data structures
Graphical user interface design principles
Write the characteristics of web interface
Components of graphical user interface
Function of graphical user interface
Graphical user interface testing
Graphical user interface testing
Characteristics of web user interface
Java user interface
Programming graphical user interfaces in r
Gui toolkits
Grafika bmp
Idscenter
Metasploit gui kali
Gdi graphics device interface
Ge gi gue gui güe güi
Linux gui development tools
Interface between user and kernel
Linear programming model formulation and graphical solution
Linear programming graphical calculator
Lp model formulation
Linear programming model formulation and graphical solution
Flair furniture company linear programming
Lp model formulation example
Fluidity in hci
Office interface vs industrial interface
Interface in interface java
Interface------------ an interface *
Recoverability in user interface design
Perancangan antar muka (user interface)
Character user interface