INFORMATICS PRACTICES PYTHON Python Programming Language Python is
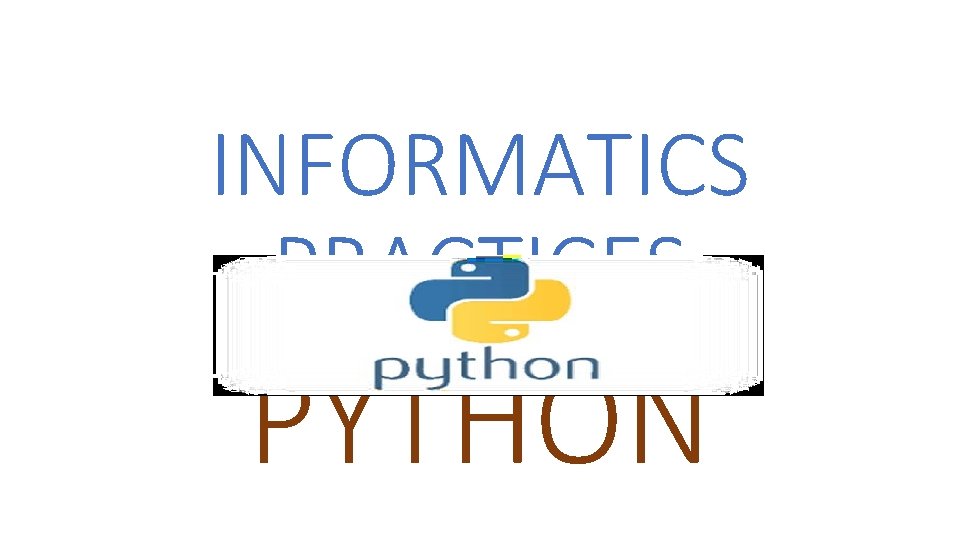
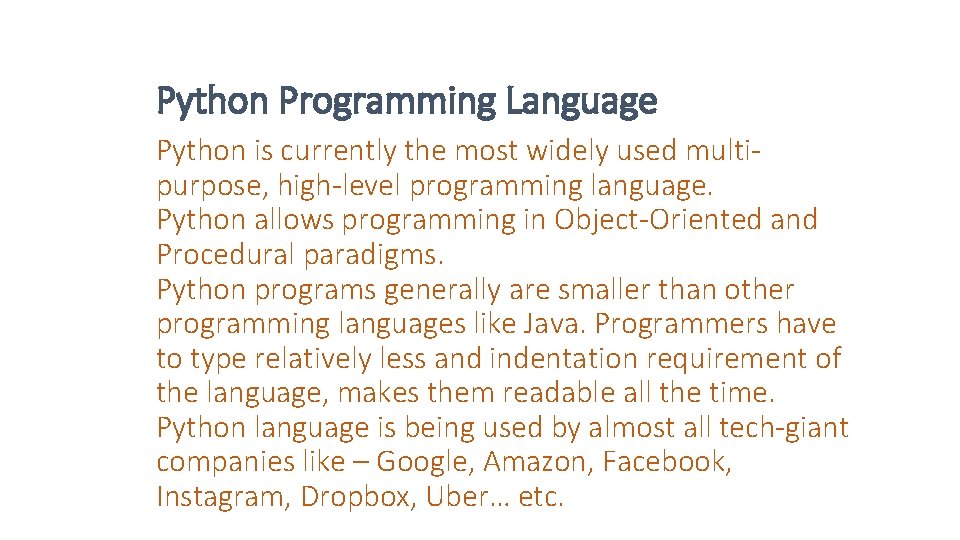
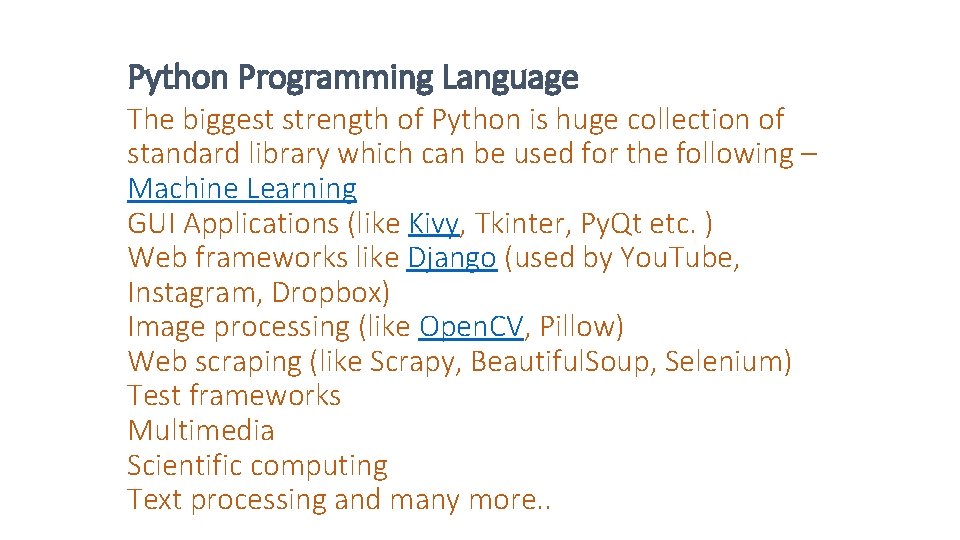
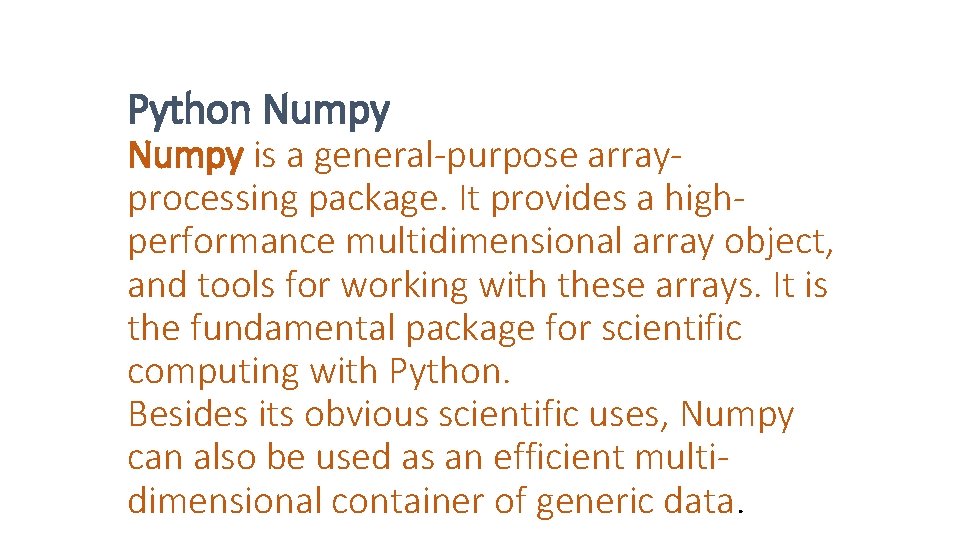
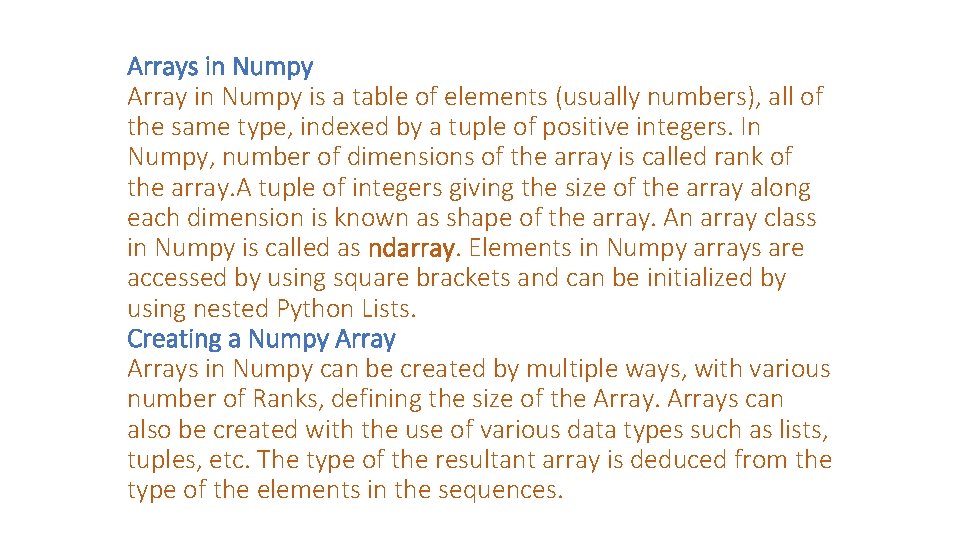
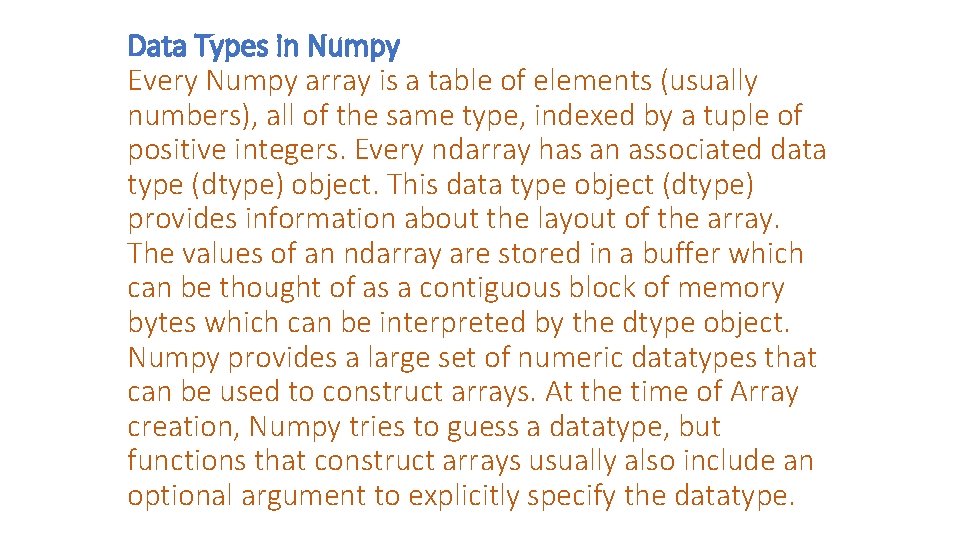
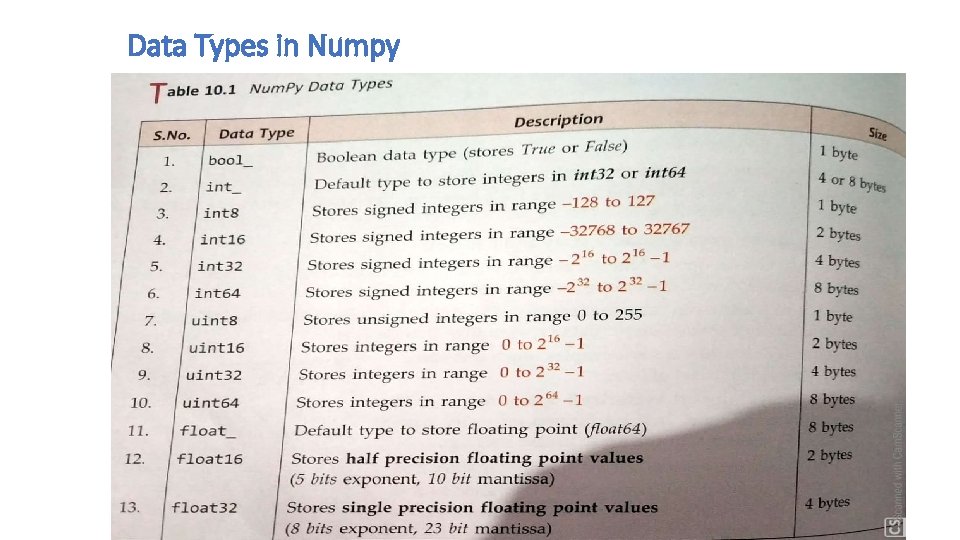
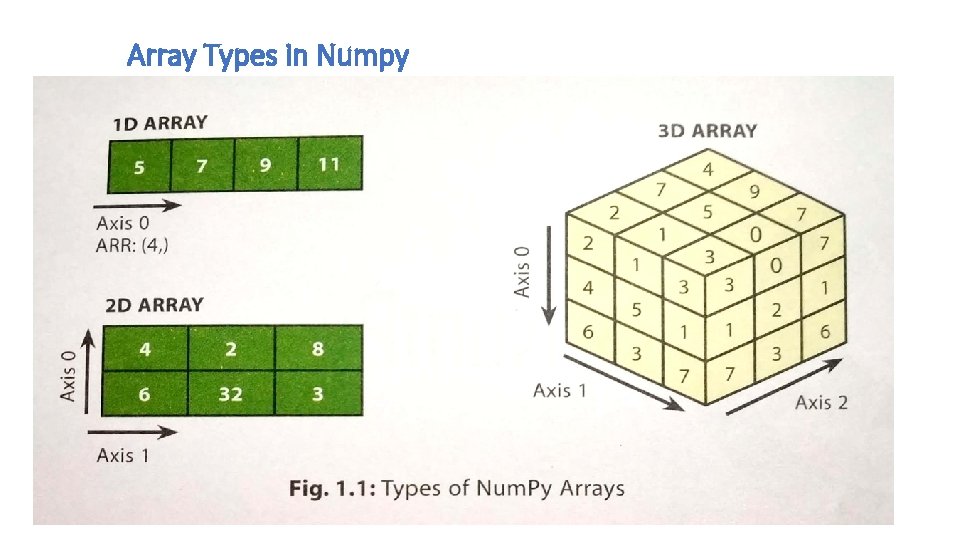
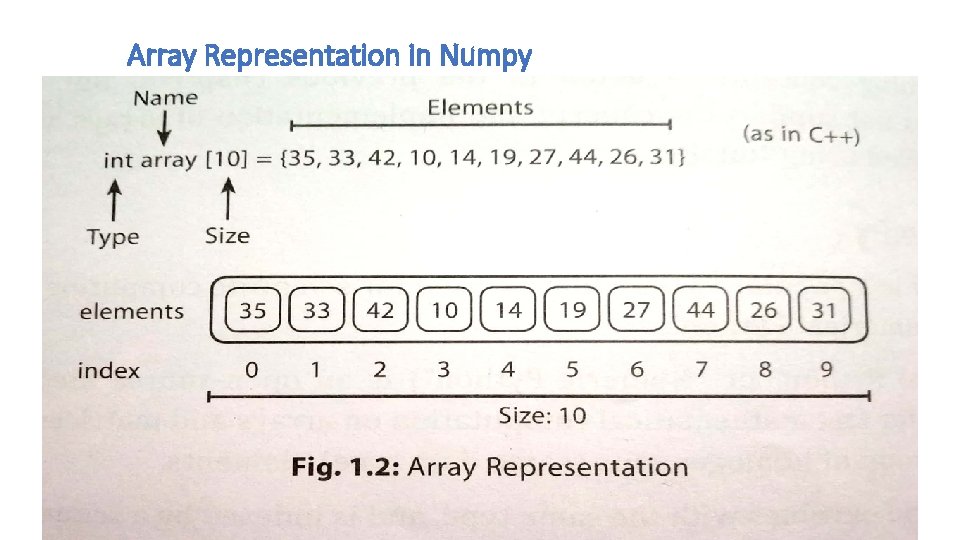
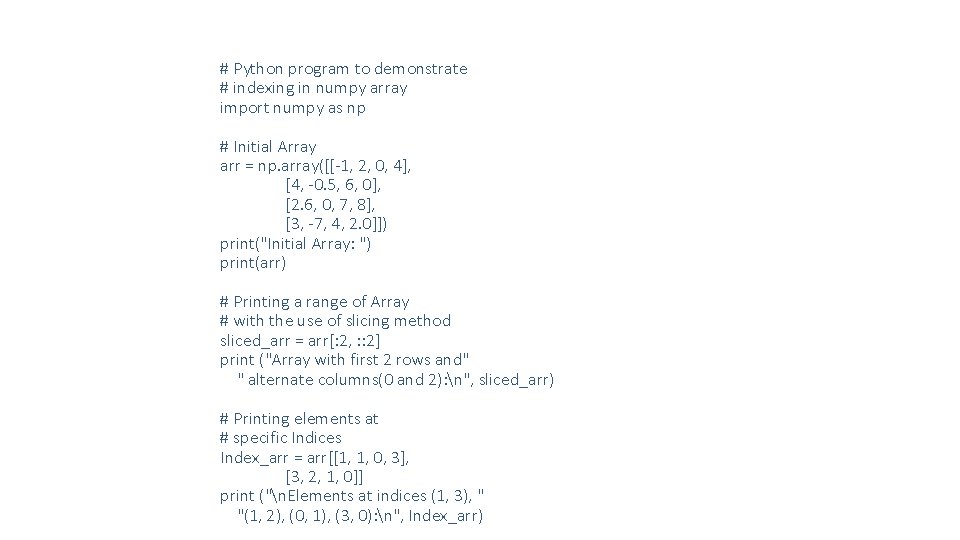
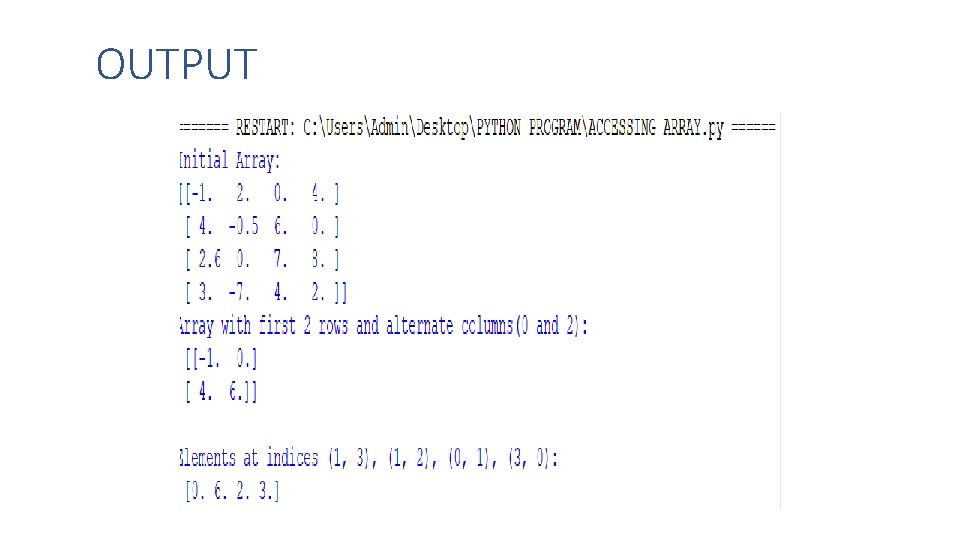
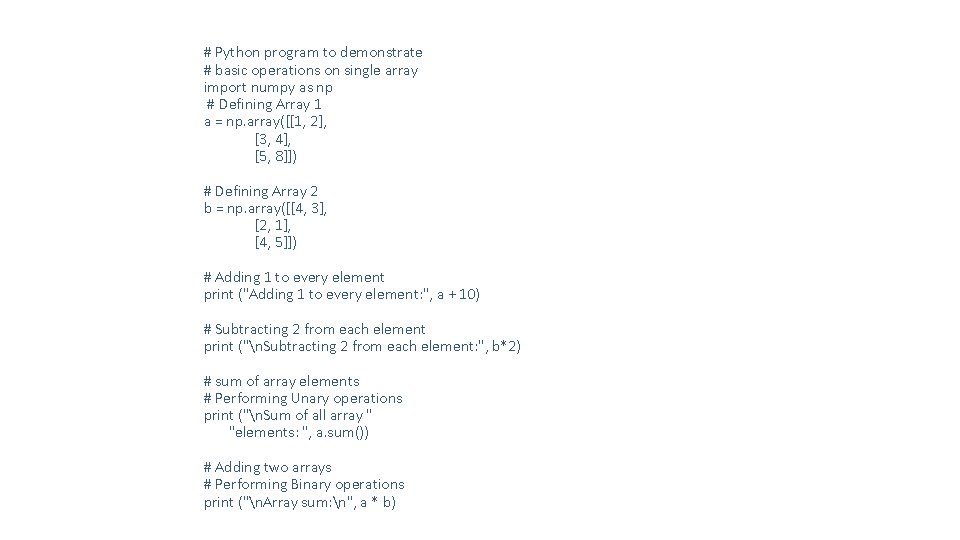
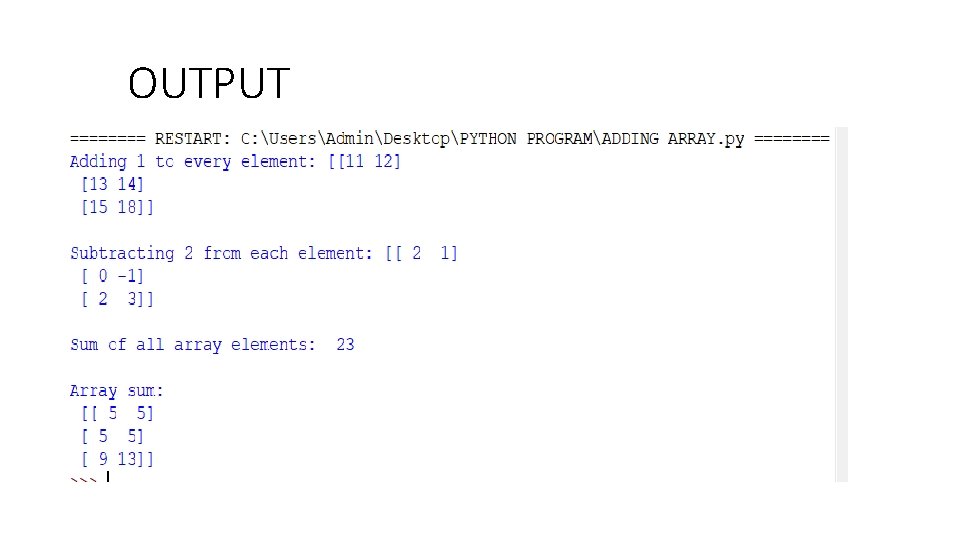
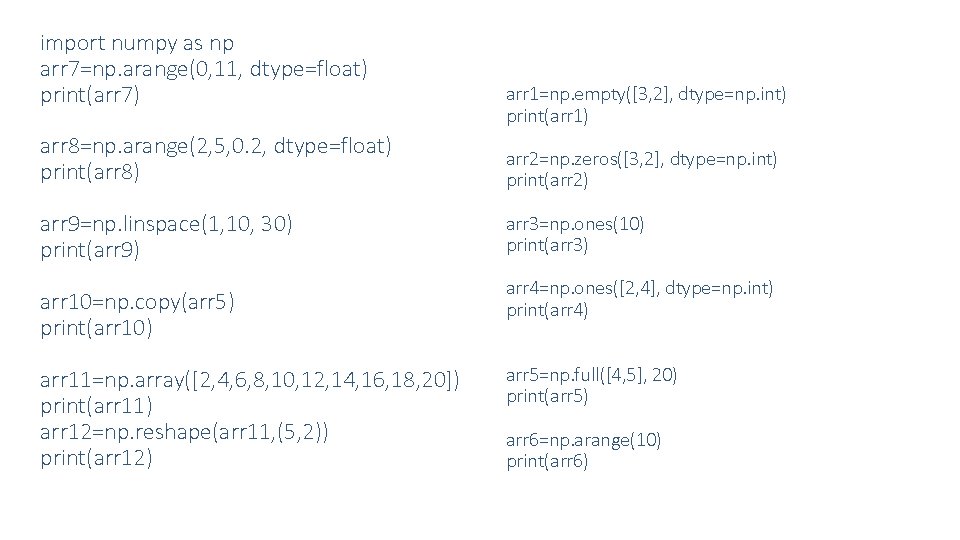
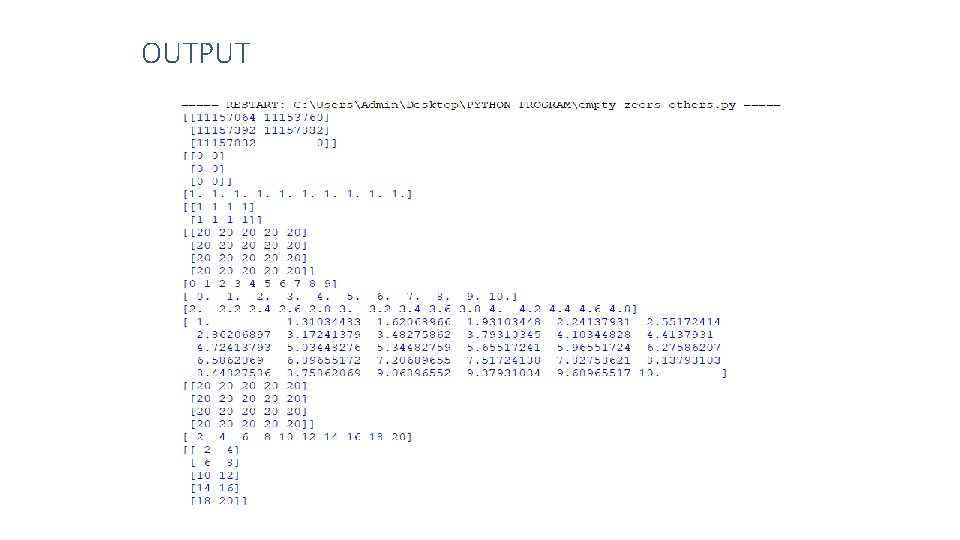
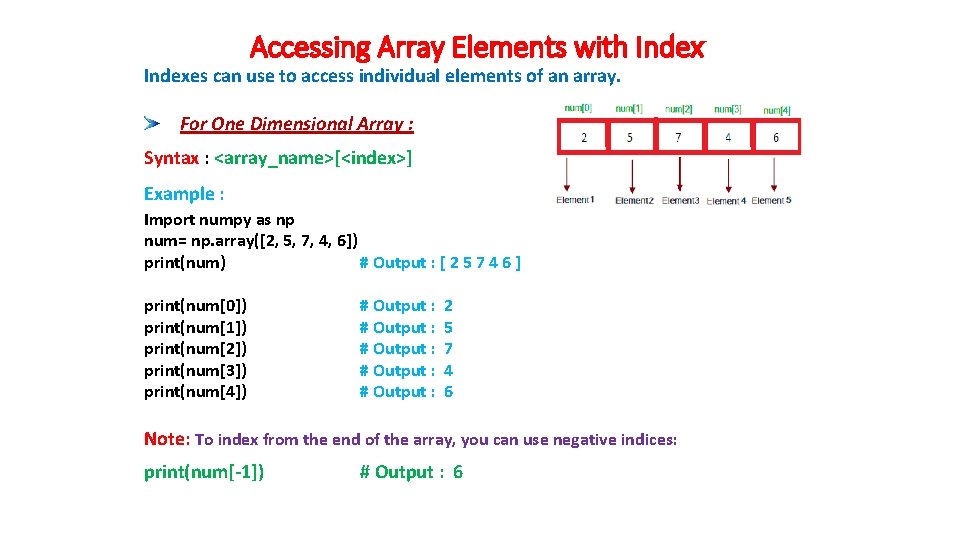
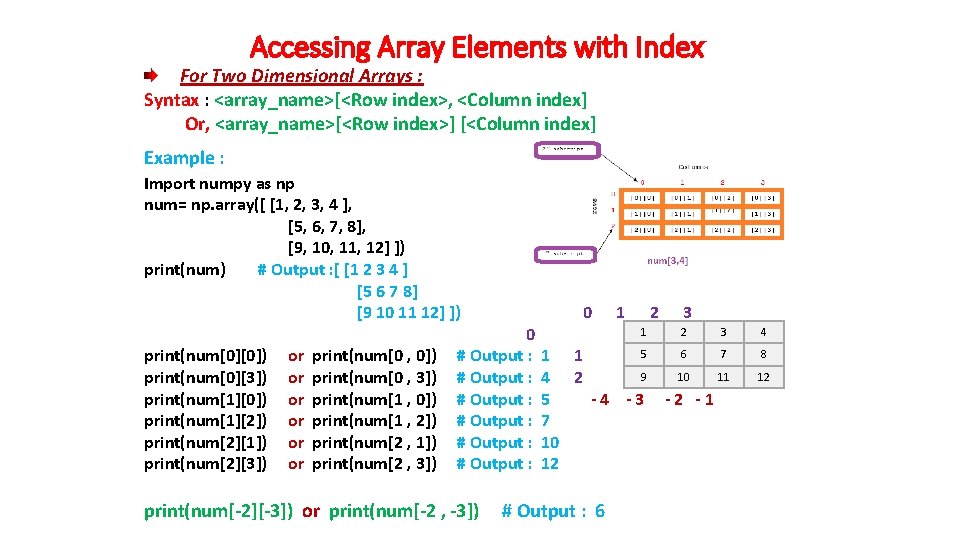
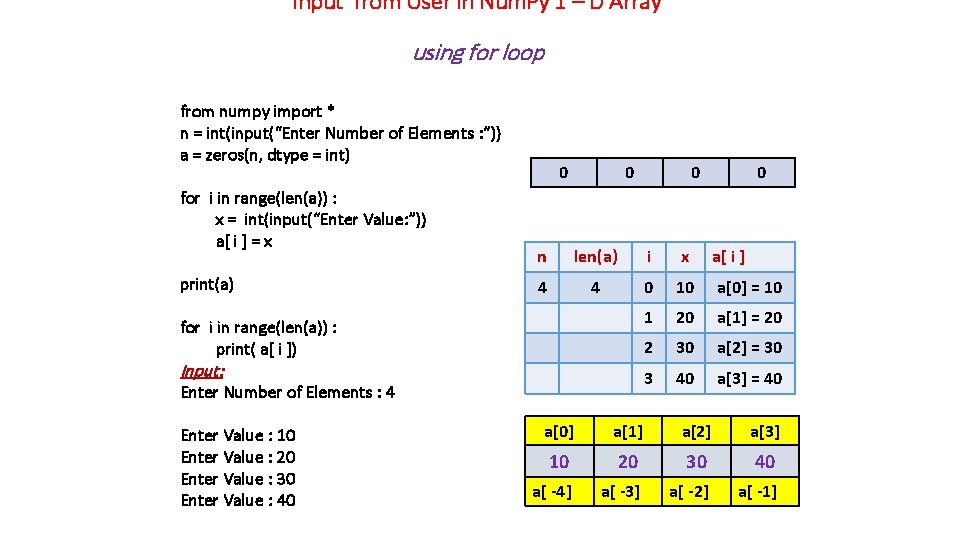
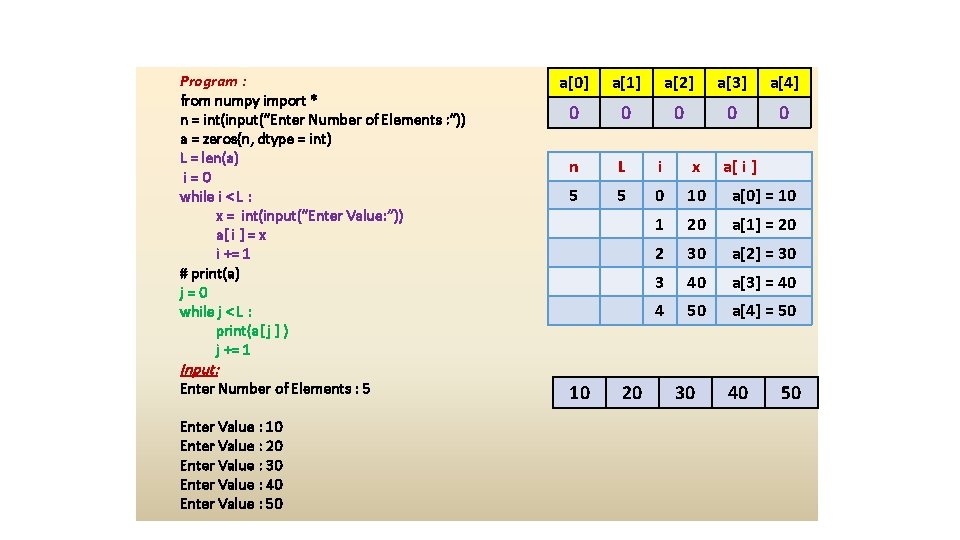
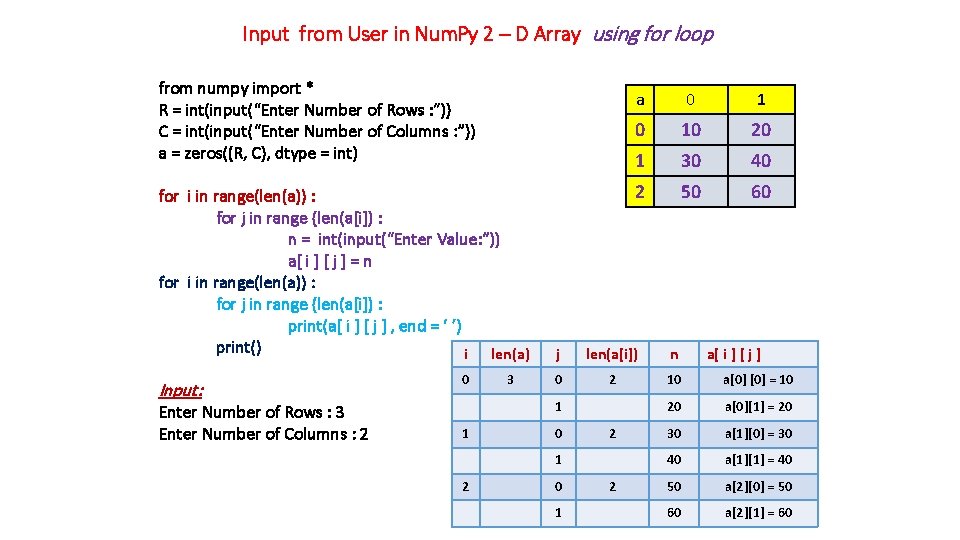
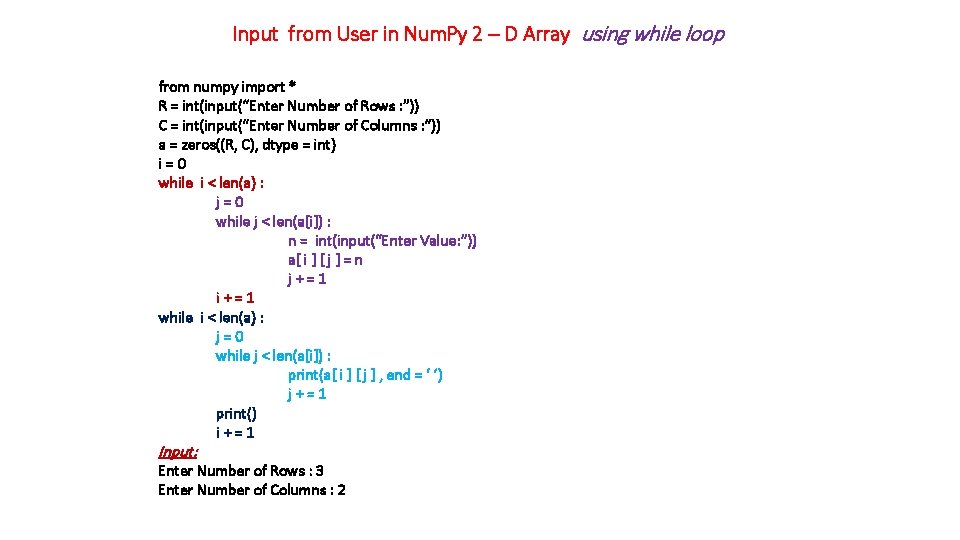
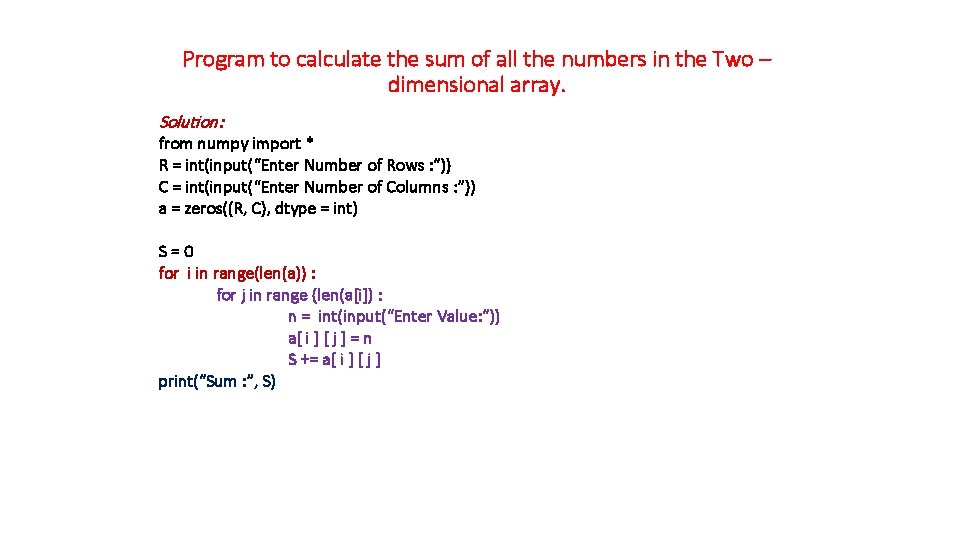
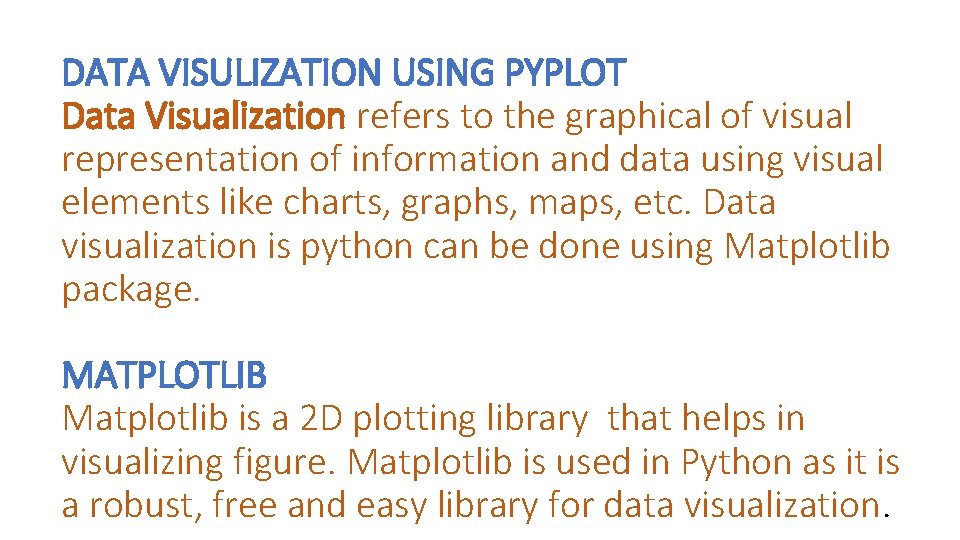
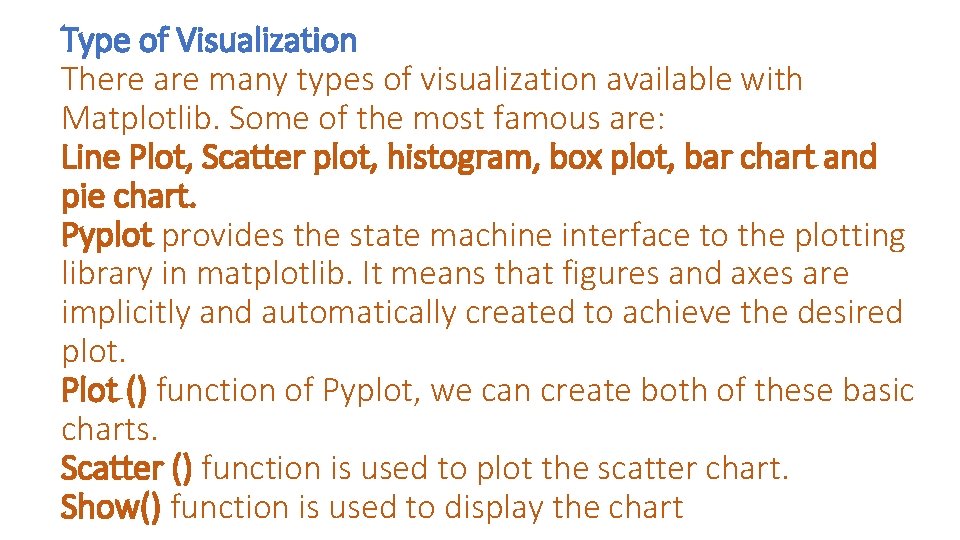
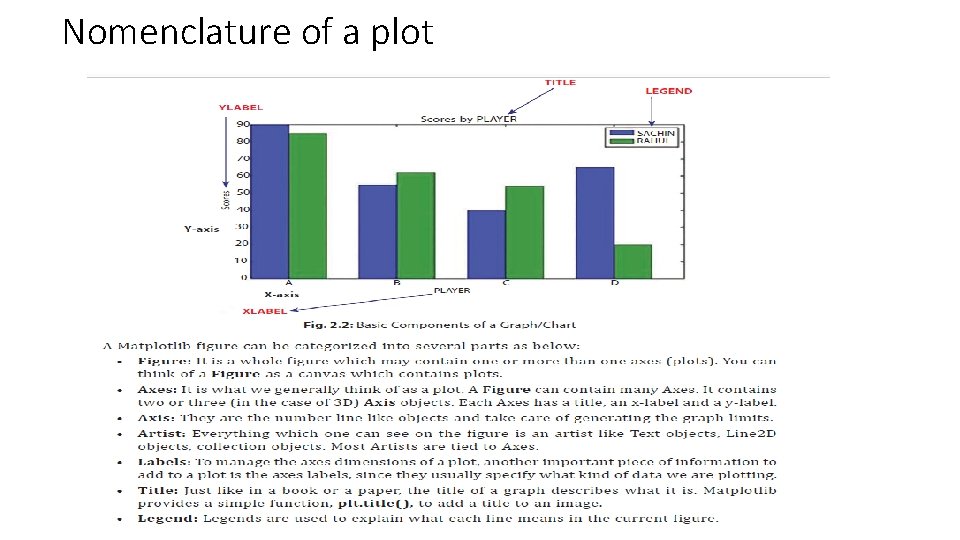
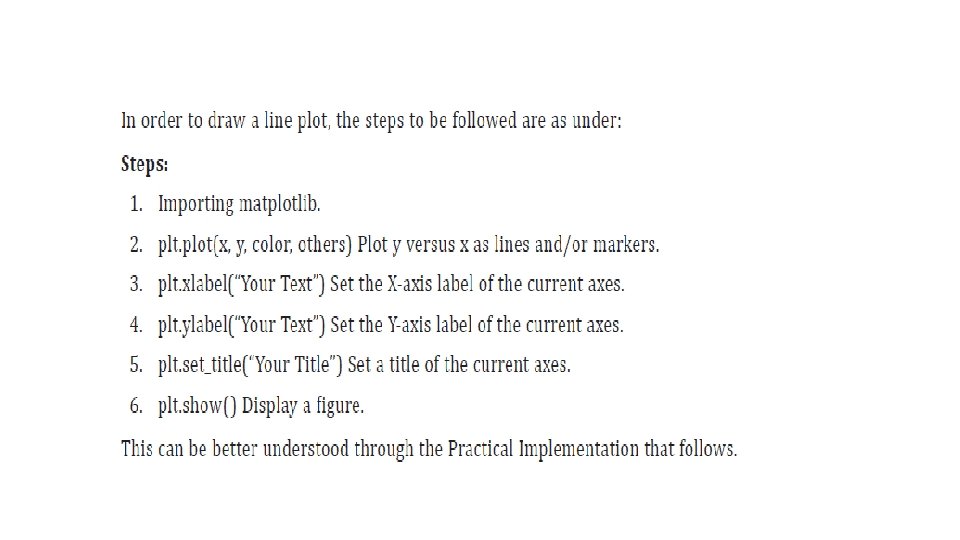
![import matplotlib. pyplot as plt import numpy as np #plt. plot([1, 2, 5, ], import matplotlib. pyplot as plt import numpy as np #plt. plot([1, 2, 5, ],](https://slidetodoc.com/presentation_image_h2/1c206adac322608f4bdd0363c604d790/image-27.jpg)
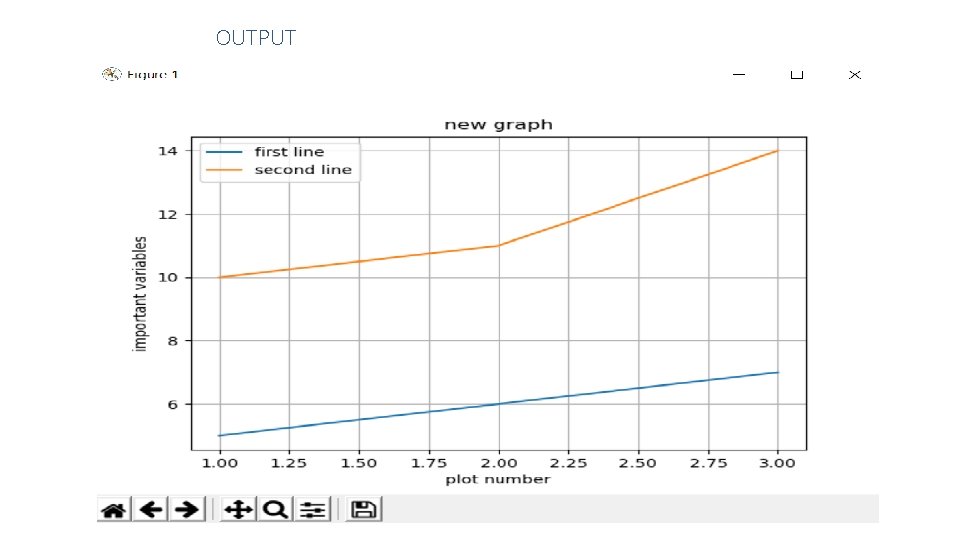
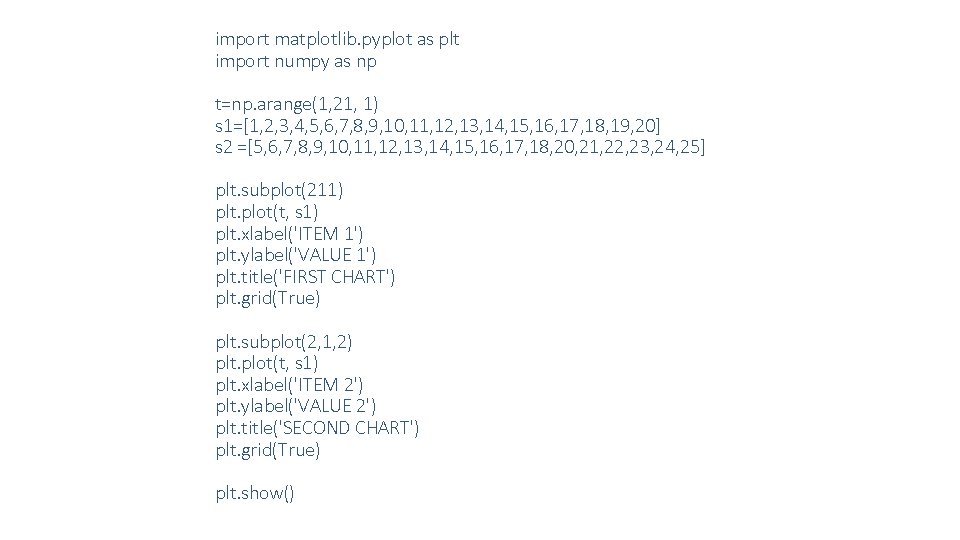
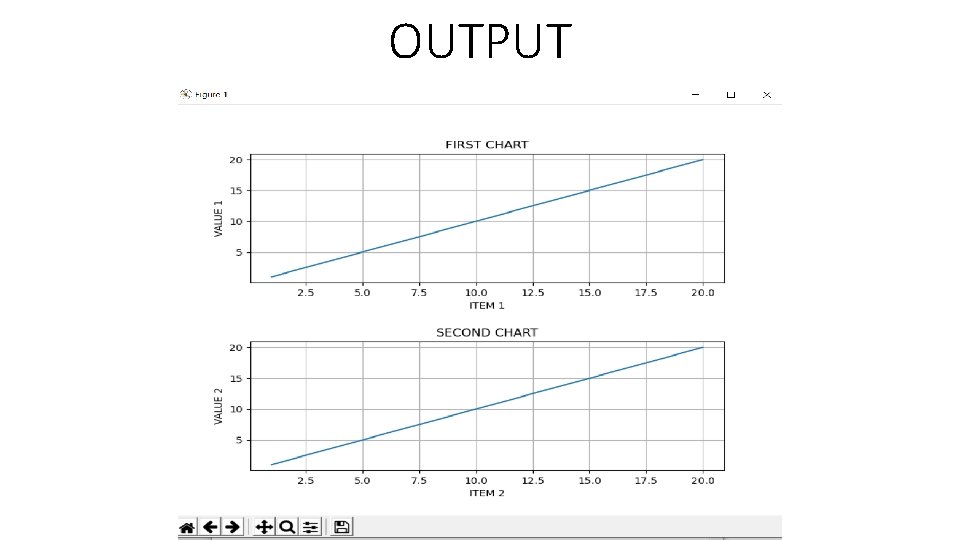
- Slides: 30
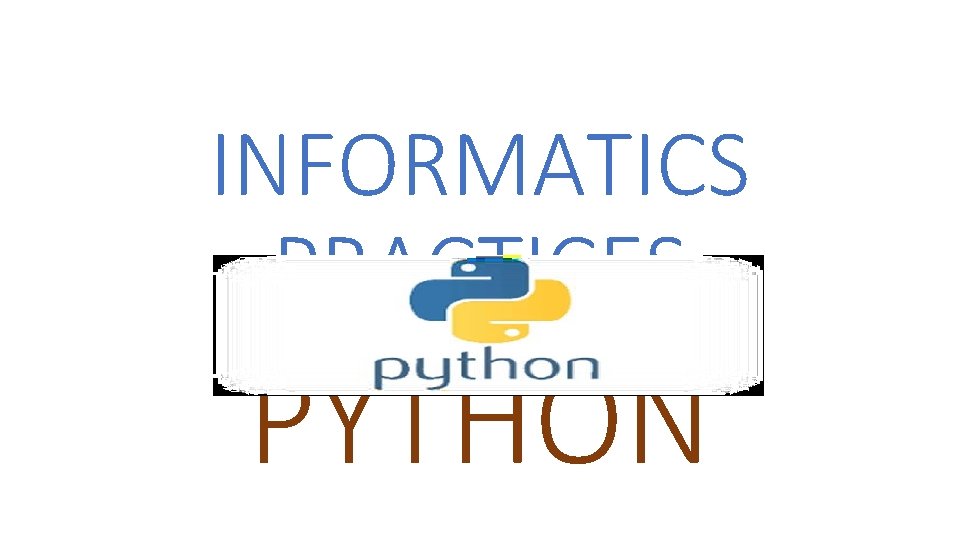
INFORMATICS PRACTICES PYTHON
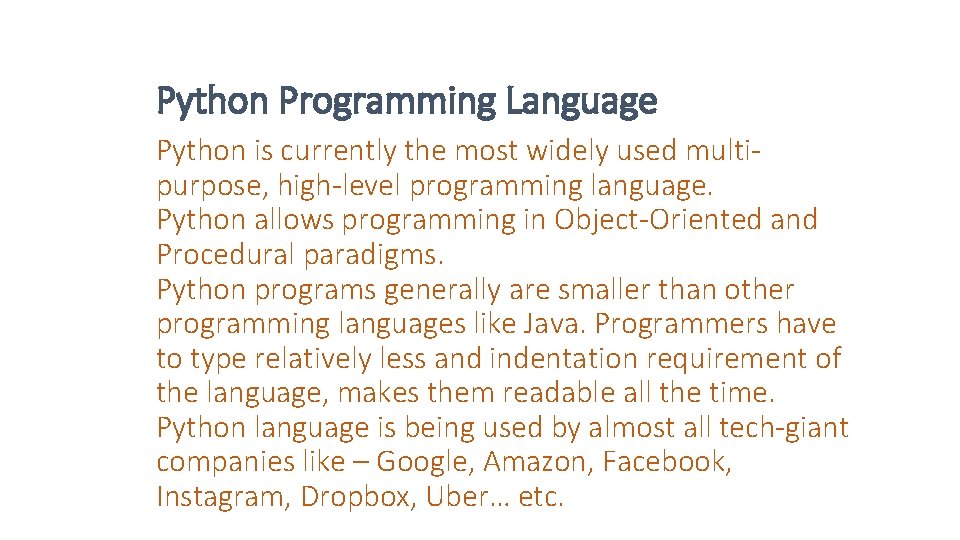
Python Programming Language Python is currently the most widely used multipurpose, high-level programming language. Python allows programming in Object-Oriented and Procedural paradigms. Python programs generally are smaller than other programming languages like Java. Programmers have to type relatively less and indentation requirement of the language, makes them readable all the time. Python language is being used by almost all tech-giant companies like – Google, Amazon, Facebook, Instagram, Dropbox, Uber… etc.
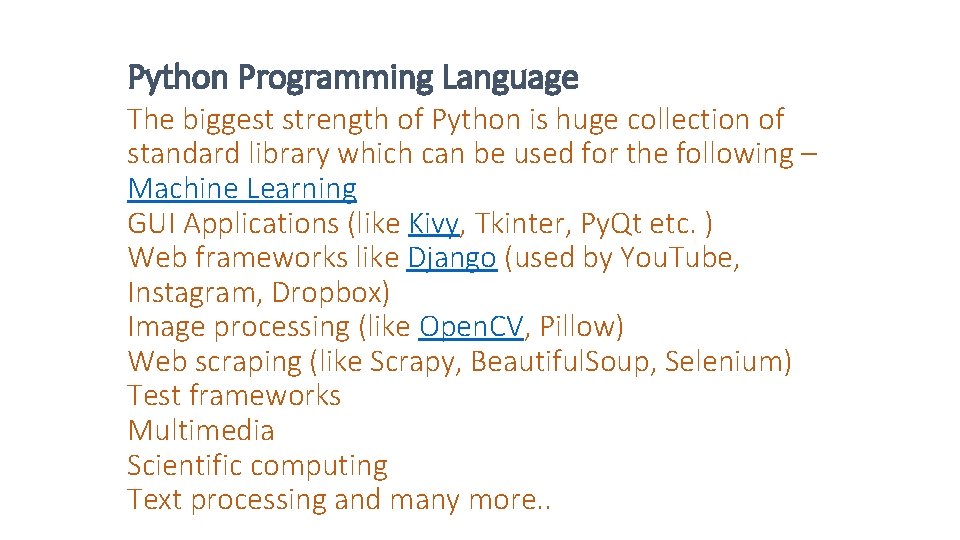
Python Programming Language The biggest strength of Python is huge collection of standard library which can be used for the following – Machine Learning GUI Applications (like Kivy, Tkinter, Py. Qt etc. ) Web frameworks like Django (used by You. Tube, Instagram, Dropbox) Image processing (like Open. CV, Pillow) Web scraping (like Scrapy, Beautiful. Soup, Selenium) Test frameworks Multimedia Scientific computing Text processing and many more. .
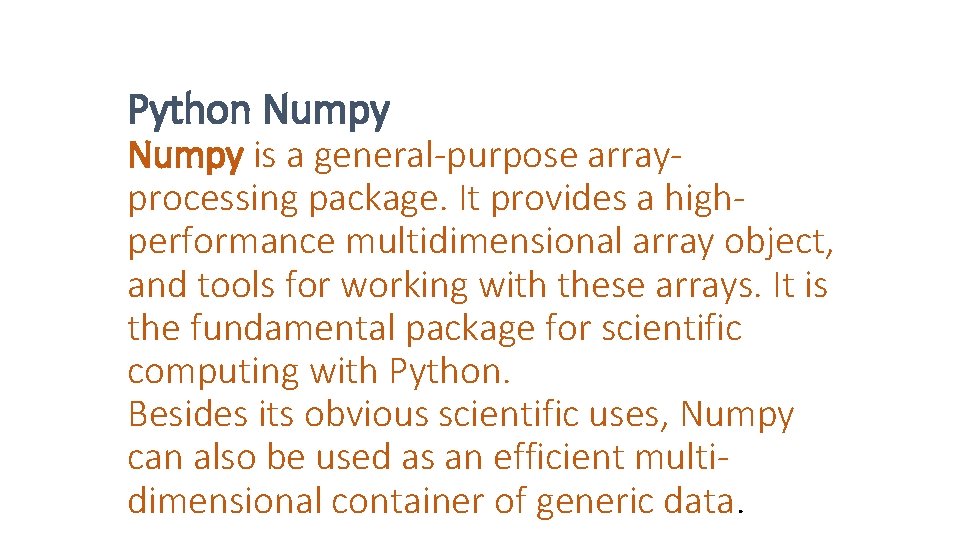
Python Numpy is a general-purpose arrayprocessing package. It provides a highperformance multidimensional array object, and tools for working with these arrays. It is the fundamental package for scientific computing with Python. Besides its obvious scientific uses, Numpy can also be used as an efficient multidimensional container of generic data.
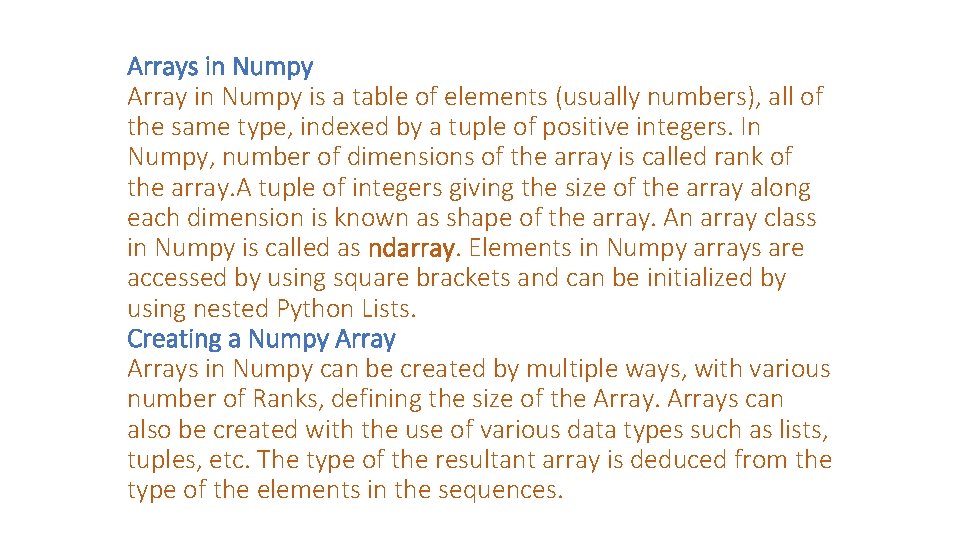
Arrays in Numpy Array in Numpy is a table of elements (usually numbers), all of the same type, indexed by a tuple of positive integers. In Numpy, number of dimensions of the array is called rank of the array. A tuple of integers giving the size of the array along each dimension is known as shape of the array. An array class in Numpy is called as ndarray. Elements in Numpy arrays are accessed by using square brackets and can be initialized by using nested Python Lists. Creating a Numpy Arrays in Numpy can be created by multiple ways, with various number of Ranks, defining the size of the Arrays can also be created with the use of various data types such as lists, tuples, etc. The type of the resultant array is deduced from the type of the elements in the sequences.
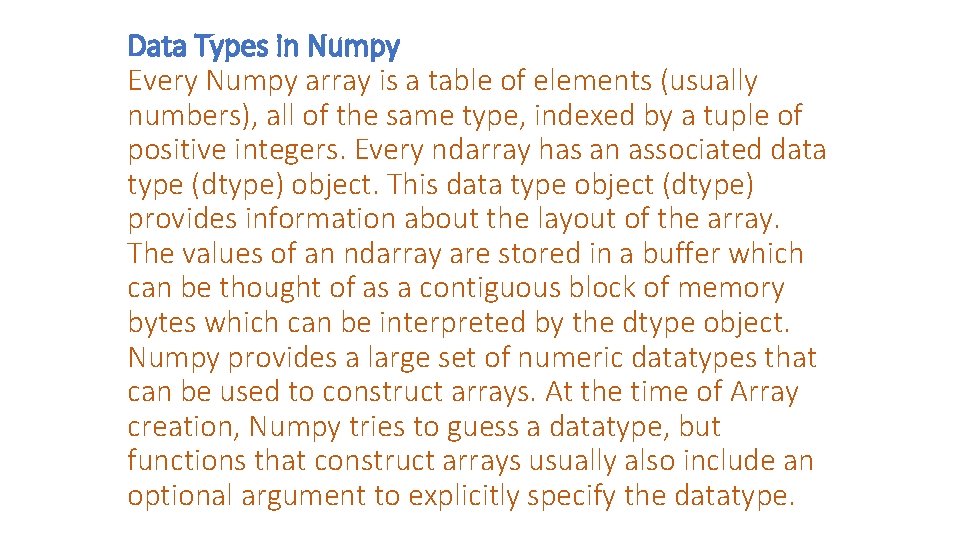
Data Types in Numpy Every Numpy array is a table of elements (usually numbers), all of the same type, indexed by a tuple of positive integers. Every ndarray has an associated data type (dtype) object. This data type object (dtype) provides information about the layout of the array. The values of an ndarray are stored in a buffer which can be thought of as a contiguous block of memory bytes which can be interpreted by the dtype object. Numpy provides a large set of numeric datatypes that can be used to construct arrays. At the time of Array creation, Numpy tries to guess a datatype, but functions that construct arrays usually also include an optional argument to explicitly specify the datatype.
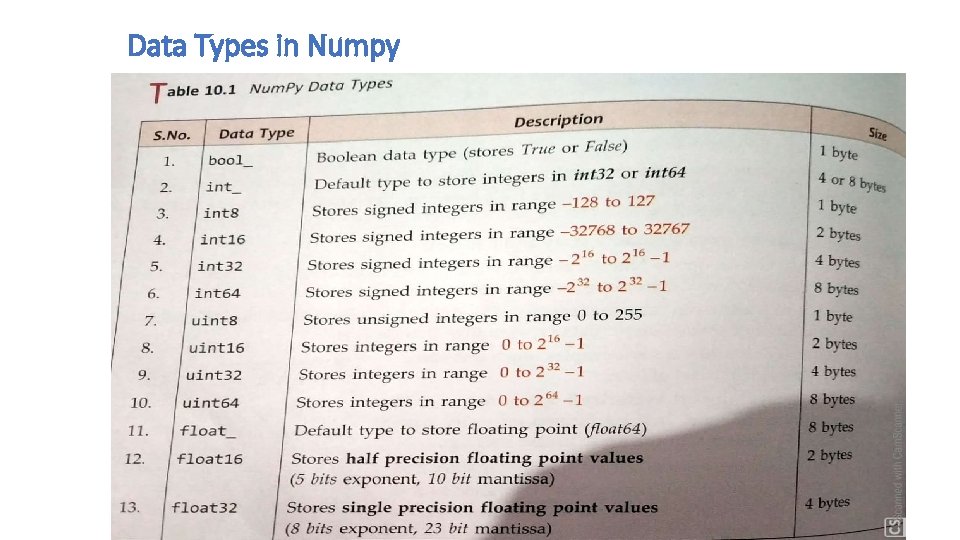
Data Types in Numpy
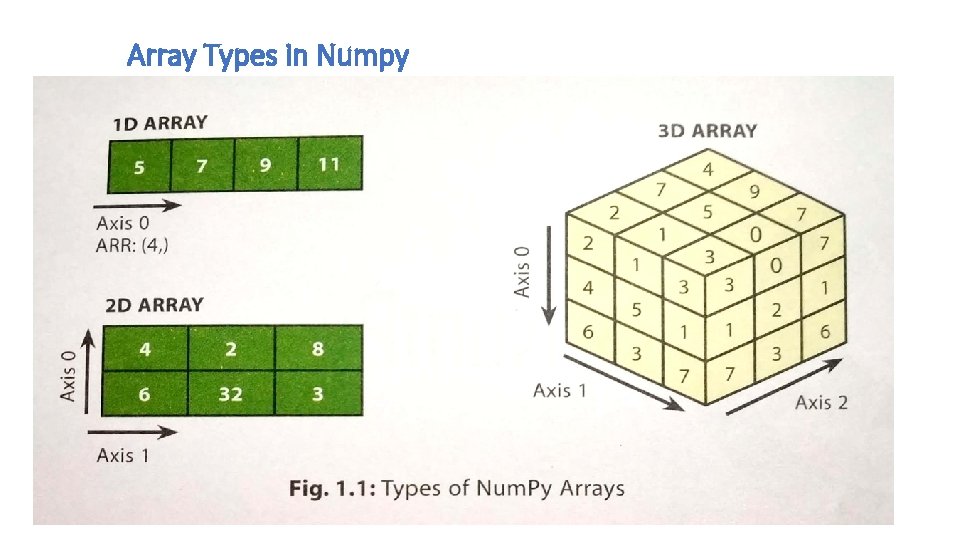
Array Types in Numpy
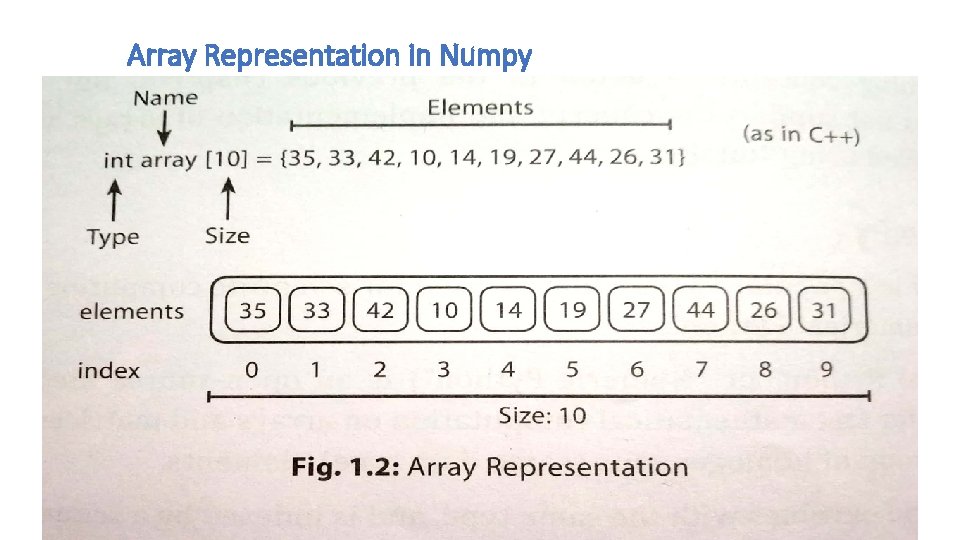
Array Representation in Numpy
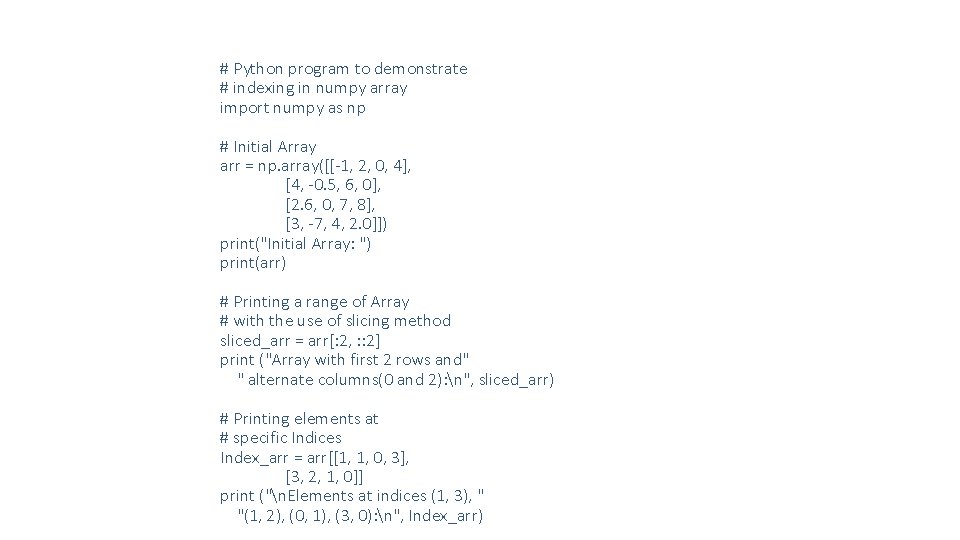
# Python program to demonstrate # indexing in numpy array import numpy as np # Initial Array arr = np. array([[-1, 2, 0, 4], [4, -0. 5, 6, 0], [2. 6, 0, 7, 8], [3, -7, 4, 2. 0]]) print("Initial Array: ") print(arr) # Printing a range of Array # with the use of slicing method sliced_arr = arr[: 2, : : 2] print ("Array with first 2 rows and" " alternate columns(0 and 2): n", sliced_arr) # Printing elements at # specific Indices Index_arr = arr[[1, 1, 0, 3], [3, 2, 1, 0]] print ("n. Elements at indices (1, 3), " "(1, 2), (0, 1), (3, 0): n", Index_arr)
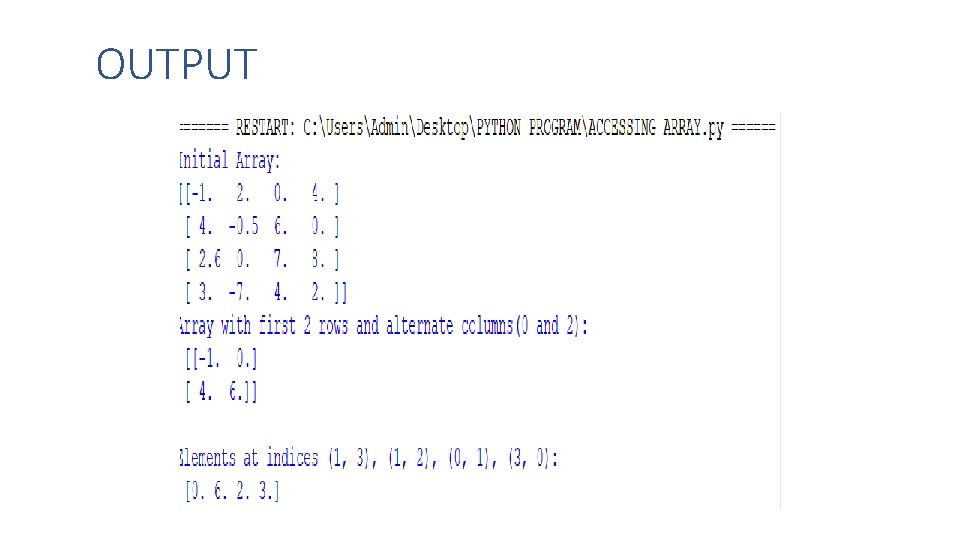
OUTPUT
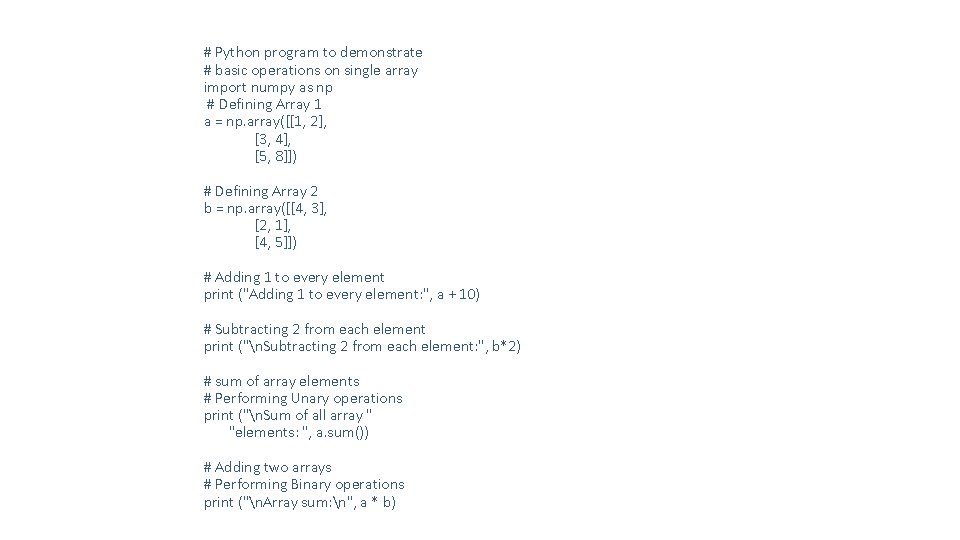
# Python program to demonstrate # basic operations on single array import numpy as np # Defining Array 1 a = np. array([[1, 2], [3, 4], [5, 8]]) # Defining Array 2 b = np. array([[4, 3], [2, 1], [4, 5]]) # Adding 1 to every element print ("Adding 1 to every element: ", a + 10) # Subtracting 2 from each element print ("n. Subtracting 2 from each element: ", b*2) # sum of array elements # Performing Unary operations print ("n. Sum of all array " "elements: ", a. sum()) # Adding two arrays # Performing Binary operations print ("n. Array sum: n", a * b)
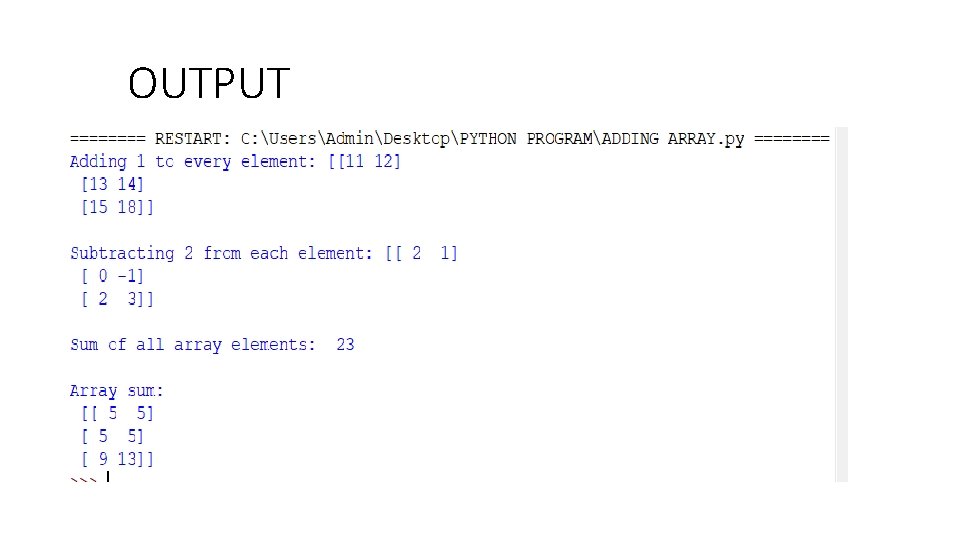
OUTPUT
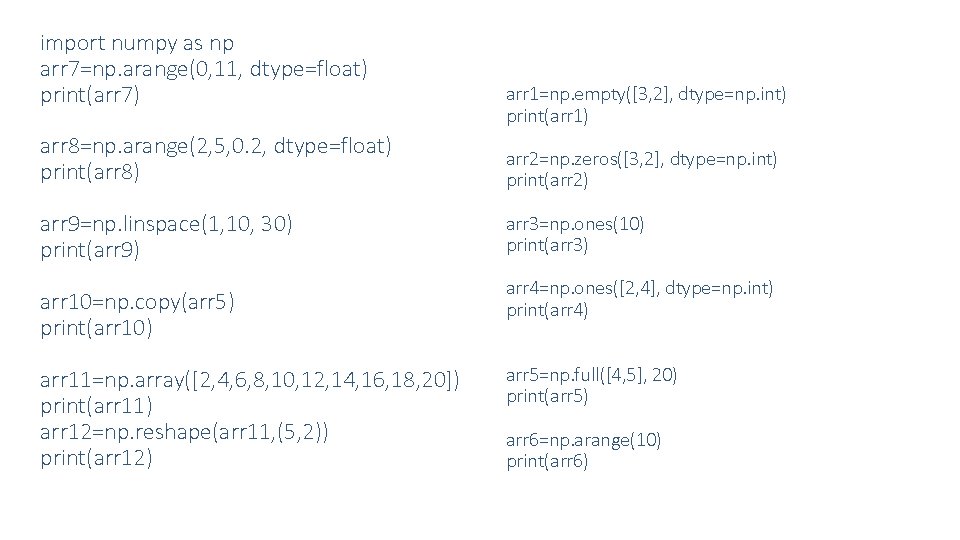
import numpy as np arr 7=np. arange(0, 11, dtype=float) print(arr 7) arr 1=np. empty([3, 2], dtype=np. int) print(arr 1) arr 8=np. arange(2, 5, 0. 2, dtype=float) print(arr 8) arr 2=np. zeros([3, 2], dtype=np. int) print(arr 2) arr 9=np. linspace(1, 10, 30) print(arr 9) arr 3=np. ones(10) print(arr 3) arr 10=np. copy(arr 5) print(arr 10) arr 11=np. array([2, 4, 6, 8, 10, 12, 14, 16, 18, 20]) print(arr 11) arr 12=np. reshape(arr 11, (5, 2)) print(arr 12) arr 4=np. ones([2, 4], dtype=np. int) print(arr 4) arr 5=np. full([4, 5], 20) print(arr 5) arr 6=np. arange(10) print(arr 6)
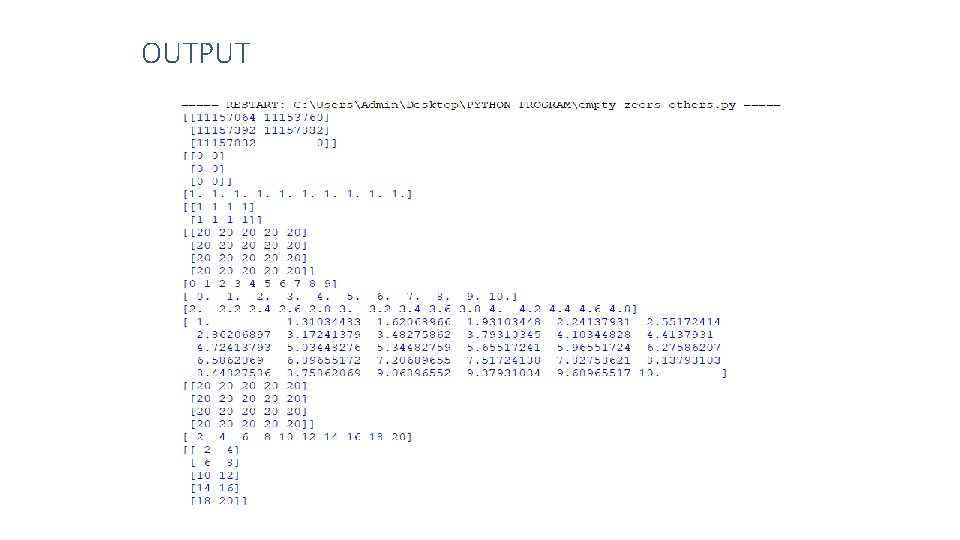
OUTPUT
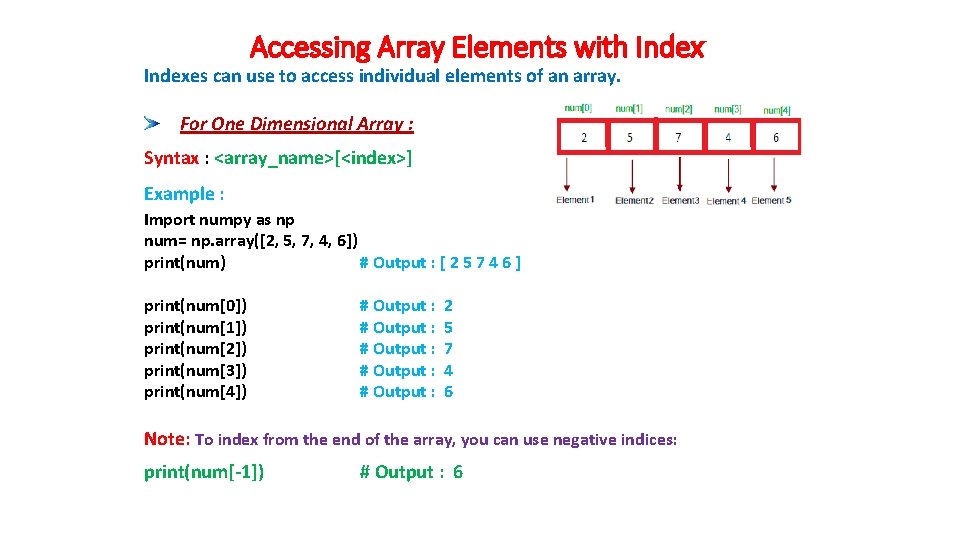
Accessing Array Elements with Indexes can use to access individual elements of an array. For One Dimensional Array : Syntax : <array_name>[<index>] Example : Import numpy as np num= np. array([2, 5, 7, 4, 6]) print(num) # Output : [ 2 5 7 4 6 ] print(num[0]) print(num[1]) print(num[2]) print(num[3]) print(num[4]) # Output : # Output : 2 5 7 4 6 Note: To index from the end of the array, you can use negative indices: print(num[-1]) # Output : 6
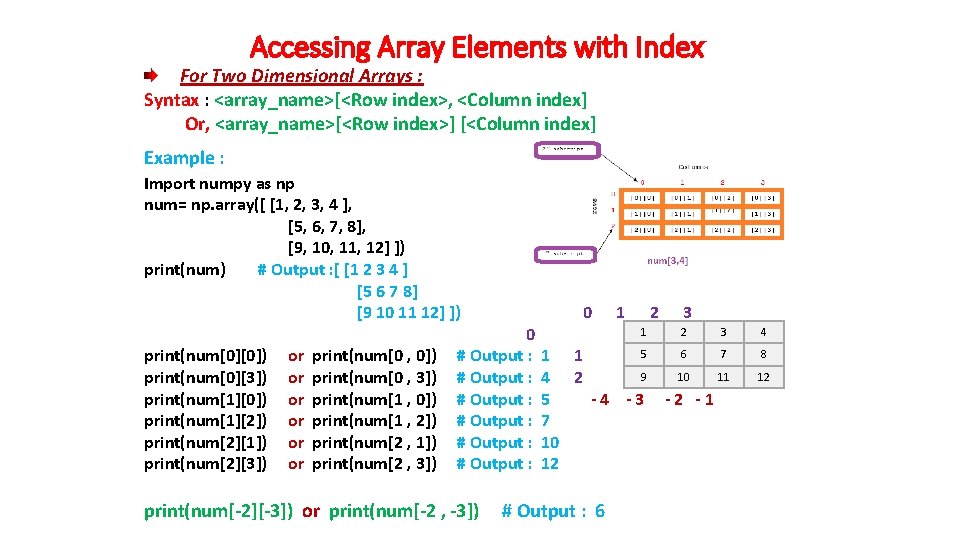
Accessing Array Elements with Index For Two Dimensional Arrays : Syntax : <array_name>[<Row index>, <Column index] Or, <array_name>[<Row index>] [<Column index] Example : Import numpy as np num= np. array([ [1, 2, 3, 4 ], [5, 6, 7, 8], [9, 10, 11, 12] ]) print(num) # Output : [ [1 2 3 4 ] [5 6 7 8] [9 10 11 12] ]) print(num[0][0]) print(num[0][3]) print(num[1][0]) print(num[1][2]) print(num[2][1]) print(num[2][3]) or or or print(num[0 , 0]) print(num[0 , 3]) print(num[1 , 0]) print(num[1 , 2]) print(num[2 , 1]) print(num[2 , 3]) 0 1 2 3 - 32 1 3 0 5 6 # Output : 1 1 -72 9 10 # Output : 4 2 -111 # Output : 5 -4 -3 -2 -1 # Output : 7 # Output : 10 # Output : 12 print(num[-2][-3]) or print(num[-2 , -3]) # Output : 6 4 8 12
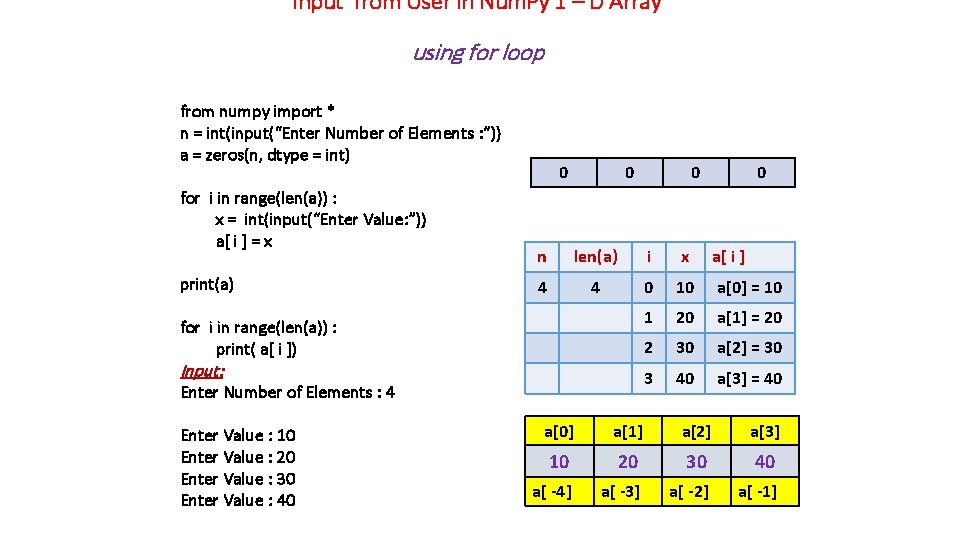
Input from User in Num. Py 1 – D Array using for loop from numpy import * n = int(input(“Enter Number of Elements : ”)) a = zeros(n, dtype = int) for i in range(len(a)) : x = int(input(“Enter Value: ”)) a[ i ] = x print(a) 0 0 0 n len(a) i x a[ i ] 4 4 0 10 a[0] = 10 1 20 a[1] = 20 2 30 a[2] = 30 3 40 a[3] = 40 for i in range(len(a)) : print( a[ i ]) Input: Enter Number of Elements : 4 Enter Value : 10 Enter Value : 20 Enter Value : 30 Enter Value : 40 0 a[0] a[1] a[2] a[3] 10 20 30 40 a[ -4] a[ -3] a[ -2] a[ -1]
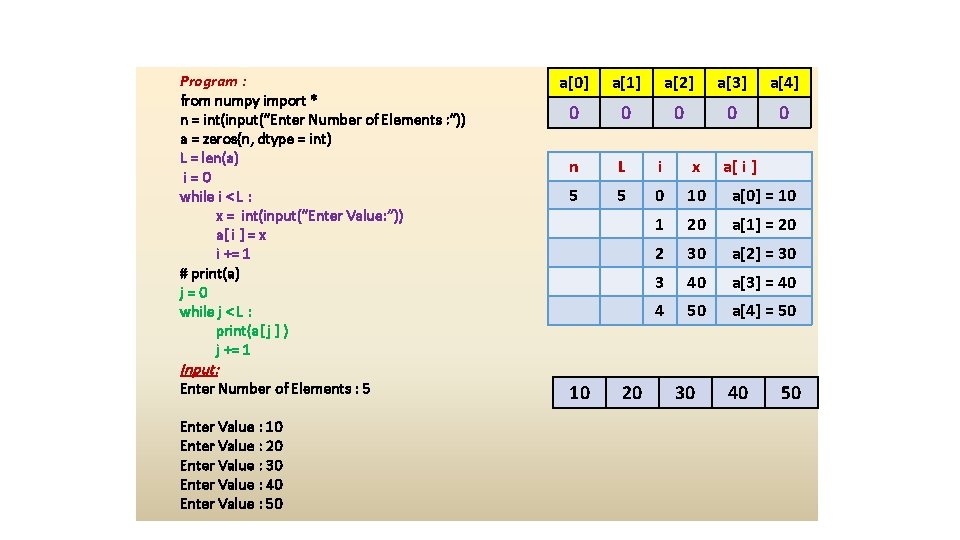
Program : from numpy import * n = int(input(“Enter Number of Elements : ”)) a = zeros(n, dtype = int) L = len(a) i=0 while i < L : x = int(input(“Enter Value: ”)) a[ i ] = x i += 1 # print(a) j=0 while j < L : print(a[ j ] ) j += 1 a[0] a[1] a[2] a[3] a[4] 0 0 0 n L i x 5 5 0 10 a[0] = 10 1 20 a[1] = 20 2 30 a[2] = 30 3 40 a[3] = 40 4 50 a[4] = 50 a[ i ] Input: Enter Number of Elements : 5 Enter Value : 10 Enter Value : 20 Enter Value : 30 Enter Value : 40 Enter Value : 50 10 20 30 40 50
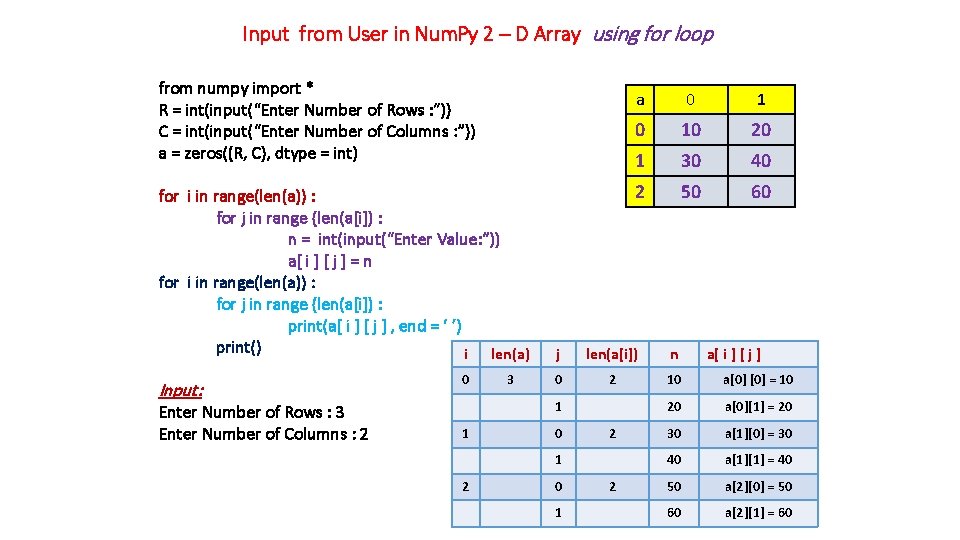
Input from User in Num. Py 2 – D Array using for loop from numpy import * R = int(input(“Enter Number of Rows : ”)) C = int(input(“Enter Number of Columns : ”)) a = zeros((R, C), dtype = int) for i in range(len(a)) : for j in range (len(a[i]) : n = int(input(“Enter Value: ”)) a[ i ] [ j ] = n for i in range(len(a)) : for j in range (len(a[i]) : print(a[ i ] [ j ] , end = ‘ ‘) print() i len(a) Input: Enter Number of Rows : 3 Enter Number of Columns : 2 0 3 1 0 10 20 1 30 40 2 50 60 len(a[i]) n 0 2 10 a[0] = 10 20 a[0][1] = 20 30 a[1][0] = 30 40 a[1][1] = 40 50 a[2][0] = 50 60 a[2][1] = 60 0 2 1 2 0 j 1 1 a 0 1 2 a[ i ] [ j ]
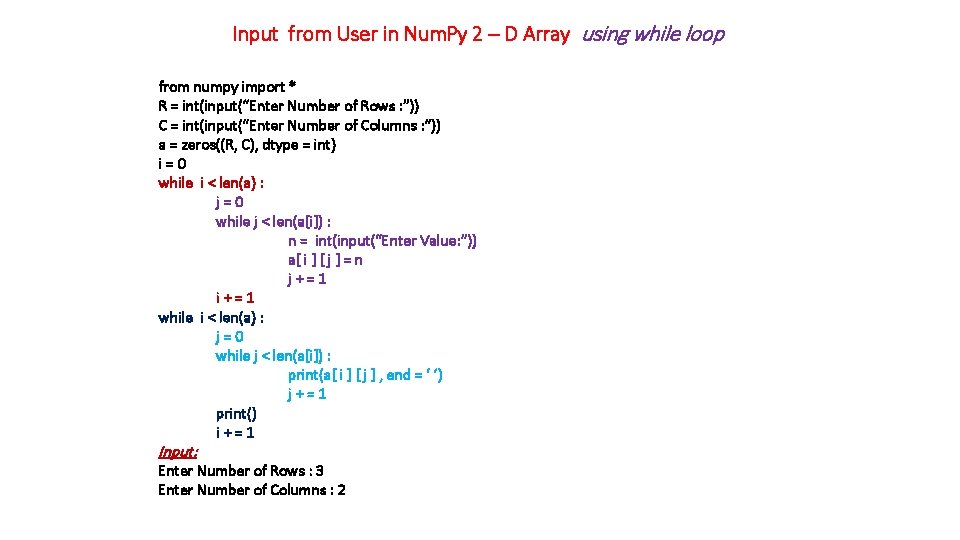
Input from User in Num. Py 2 – D Array using while loop from numpy import * R = int(input(“Enter Number of Rows : ”)) C = int(input(“Enter Number of Columns : ”)) a = zeros((R, C), dtype = int) i=0 while i < len(a) : j=0 while j < len(a[i]) : n = int(input(“Enter Value: ”)) a[ i ] [ j ] = n j+=1 i+=1 while i < len(a) : j=0 while j < len(a[i]) : print(a[ i ] [ j ] , end = ‘ ‘) j+=1 print() i+=1 Input: Enter Number of Rows : 3 Enter Number of Columns : 2
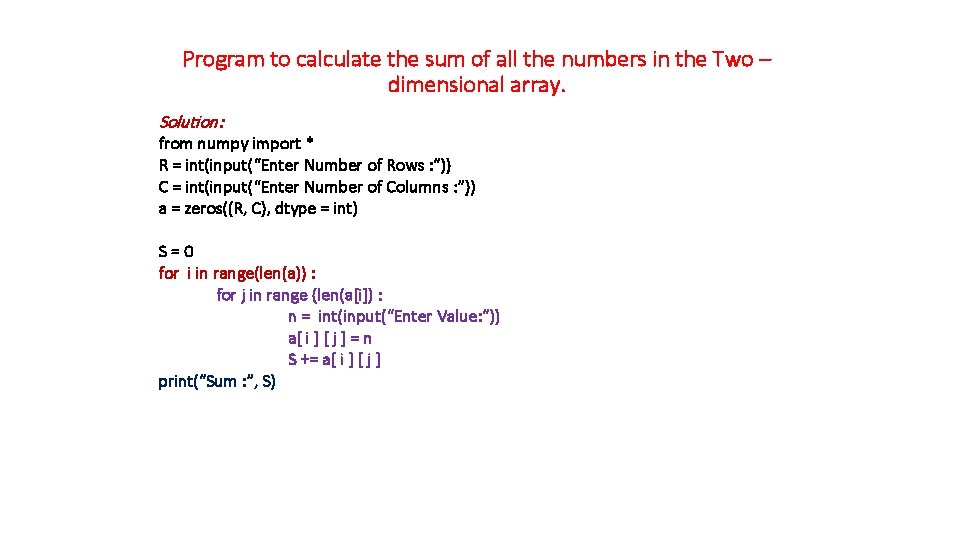
Program to calculate the sum of all the numbers in the Two – dimensional array. Solution: from numpy import * R = int(input(“Enter Number of Rows : ”)) C = int(input(“Enter Number of Columns : ”)) a = zeros((R, C), dtype = int) S=0 for i in range(len(a)) : for j in range (len(a[i]) : n = int(input(“Enter Value: ”)) a[ i ] [ j ] = n S += a[ i ] [ j ] print(“Sum : ”, S)
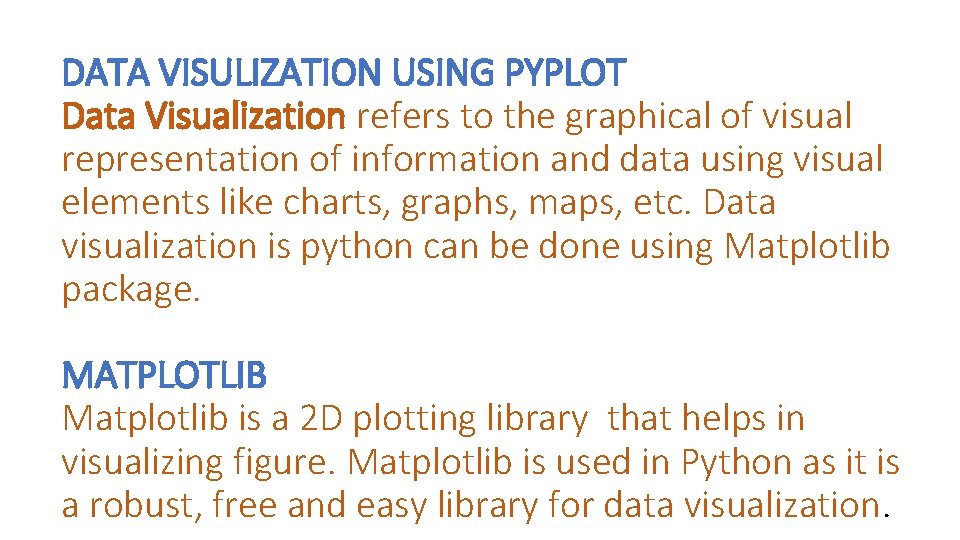
DATA VISULIZATION USING PYPLOT Data Visualization refers to the graphical of visual representation of information and data using visual elements like charts, graphs, maps, etc. Data visualization is python can be done using Matplotlib package. MATPLOTLIB Matplotlib is a 2 D plotting library that helps in visualizing figure. Matplotlib is used in Python as it is a robust, free and easy library for data visualization.
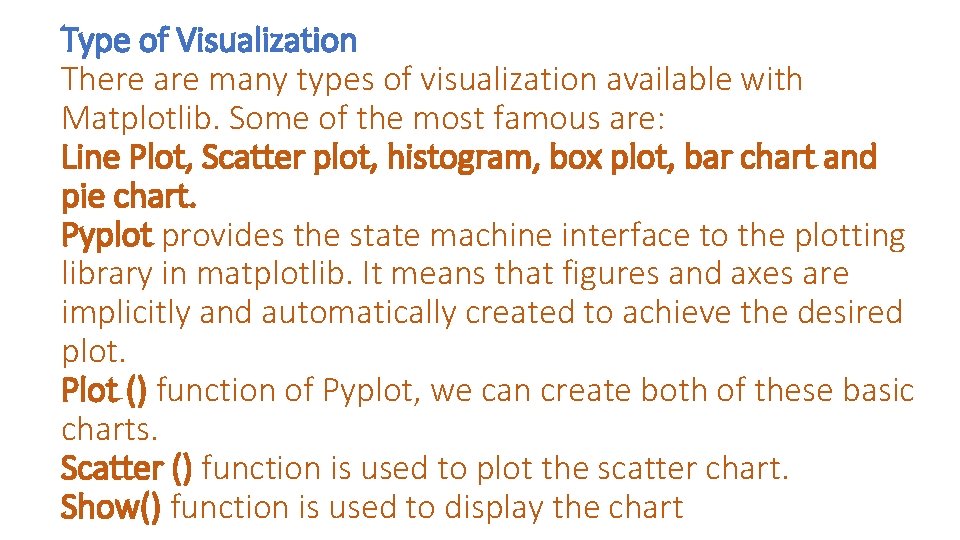
Type of Visualization There are many types of visualization available with Matplotlib. Some of the most famous are: Line Plot, Scatter plot, histogram, box plot, bar chart and pie chart. Pyplot provides the state machine interface to the plotting library in matplotlib. It means that figures and axes are implicitly and automatically created to achieve the desired plot. Plot () function of Pyplot, we can create both of these basic charts. Scatter () function is used to plot the scatter chart. Show() function is used to display the chart
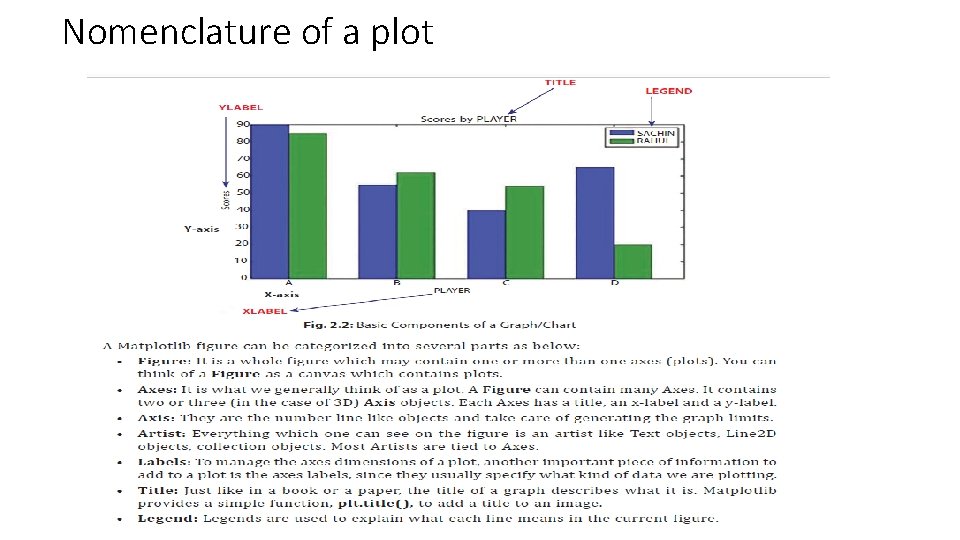
Nomenclature of a plot
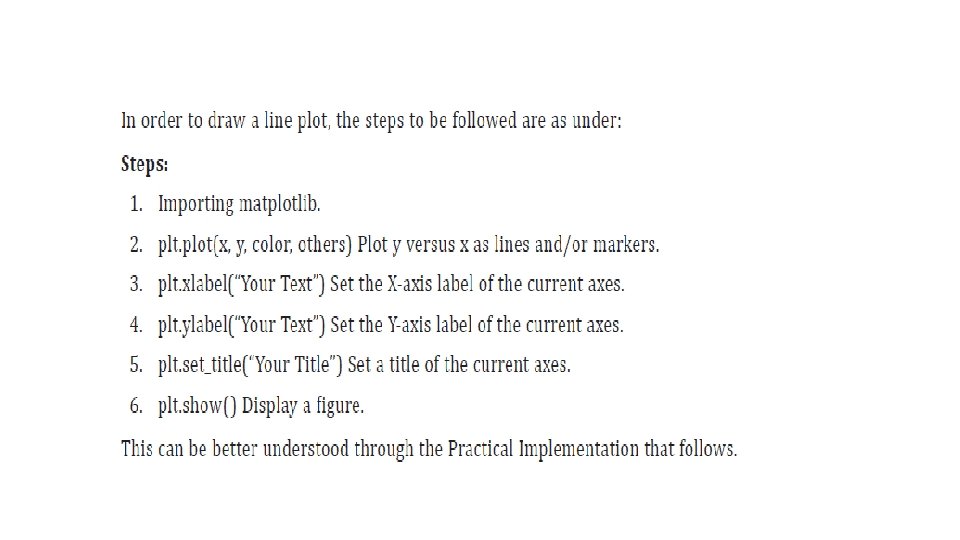
![import matplotlib pyplot as plt import numpy as np plt plot1 2 5 import matplotlib. pyplot as plt import numpy as np #plt. plot([1, 2, 5, ],](https://slidetodoc.com/presentation_image_h2/1c206adac322608f4bdd0363c604d790/image-27.jpg)
import matplotlib. pyplot as plt import numpy as np #plt. plot([1, 2, 5, ], [2, 1, 6]) #plt. show() x=[1, 2, 3] y=[5, 6, 7] plt. plot(x, y, label='first line') x 2=[1, 2, 3] y 2=[10, 11, 14] plt. plot(x 2, y 2, label='second line') plt. xlabel('plot number') plt. ylabel('important variables') plt. title('new graph') plt. legend() plt. grid(True) plt. show()
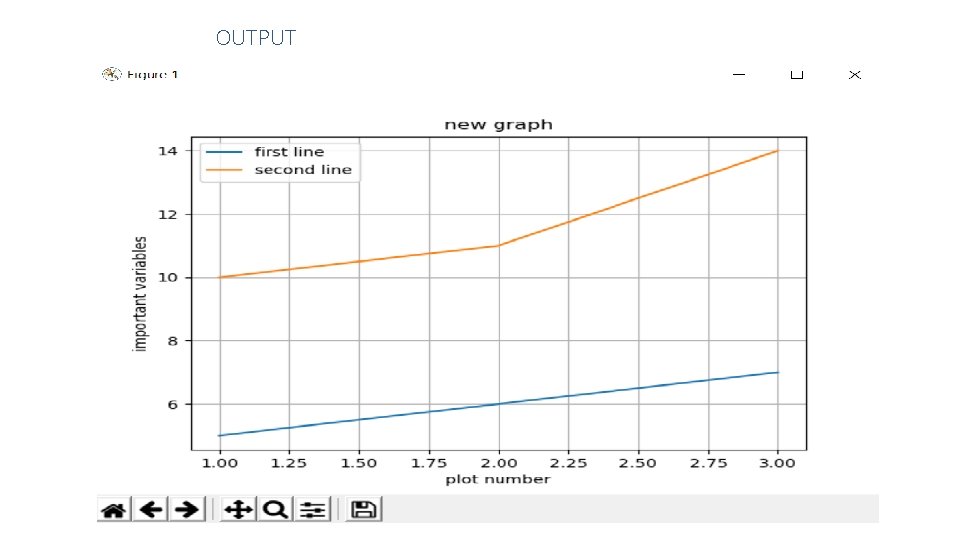
OUTPUT
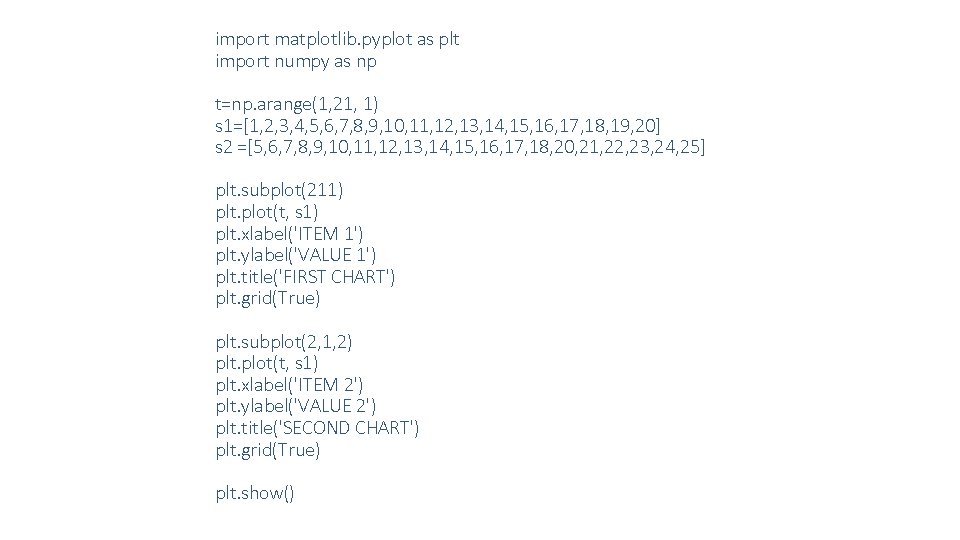
import matplotlib. pyplot as plt import numpy as np t=np. arange(1, 21, 1) s 1=[1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20] s 2 =[5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 20, 21, 22, 23, 24, 25] plt. subplot(211) plt. plot(t, s 1) plt. xlabel('ITEM 1') plt. ylabel('VALUE 1') plt. title('FIRST CHART') plt. grid(True) plt. subplot(2, 1, 2) plt. plot(t, s 1) plt. xlabel('ITEM 2') plt. ylabel('VALUE 2') plt. title('SECOND CHART') plt. grid(True) plt. show()
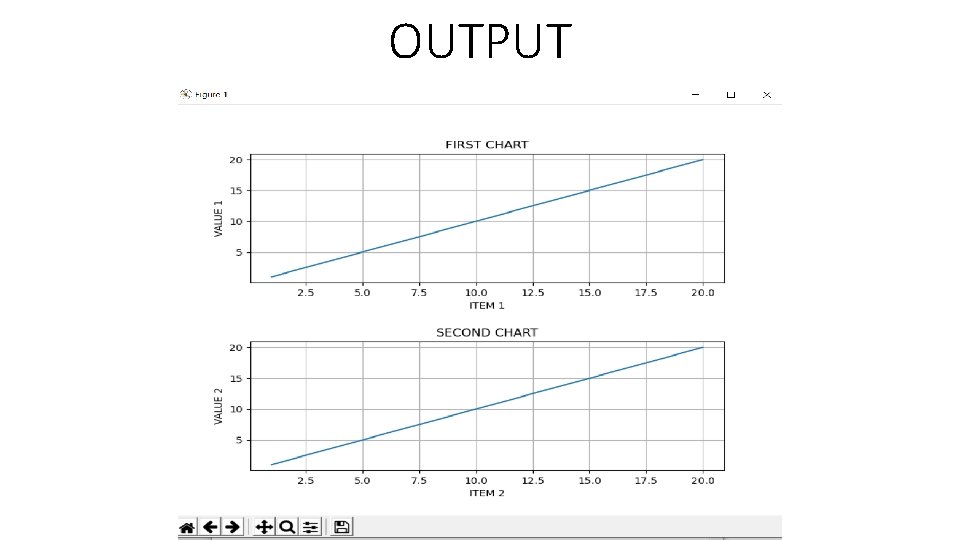
OUTPUT
Python for informatics: exploring information
Python for informatics: exploring information
Python for informatics: exploring information
Python for informatics
Python for informatics: exploring information
C coding standards best practices
Hdl language
Perbedaan linear programming dan integer programming
Greedy vs dynamic
What is system program
Linear vs integer programming
Perbedaan linear programming dan integer programming
Language assessment: principles and classroom practices
Summative evaluation
Observational health data sciences and informatics
Nursing informatics and healthcare policy
Introduction to medical informatics
Informatics 43 uci
Informatics 43 uci
Supply chain informatics
Metastructures of nursing informatics
Supply chain informatics
Medical informatics definition
Health informatics
Medical automation systems
Nursing informatics theories, models and frameworks
Belarusian university of informatics and radioelectronics
What is pharmacy
Cybernetics statistics and economic informatics
How to disconnect from society
Chapter 26 documentation and informatics