GUI programming Part 1 Intro to GUI events
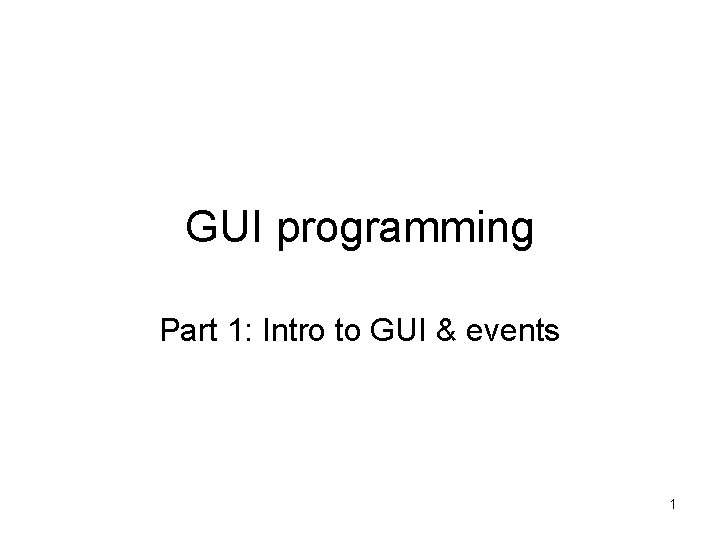
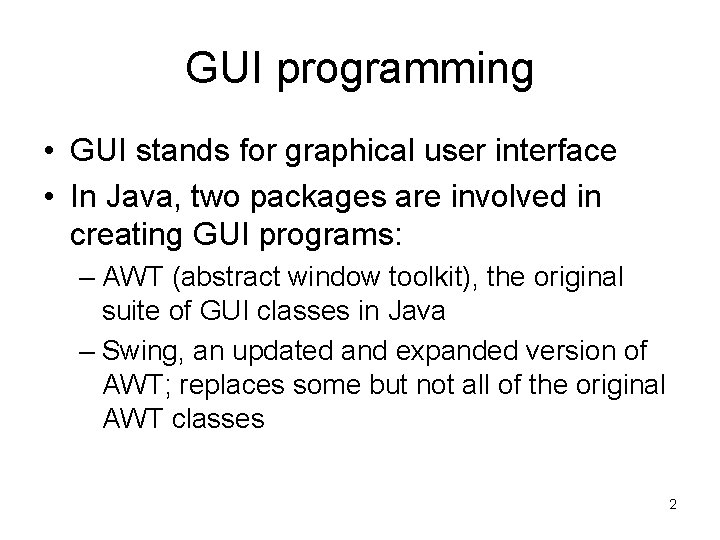
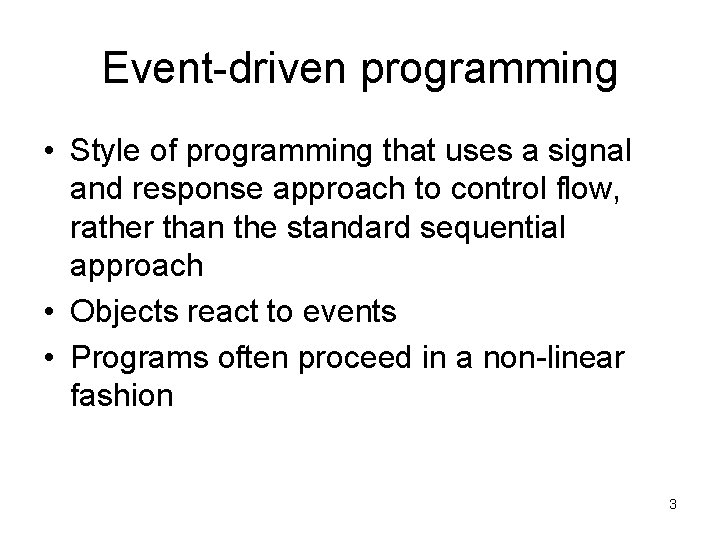
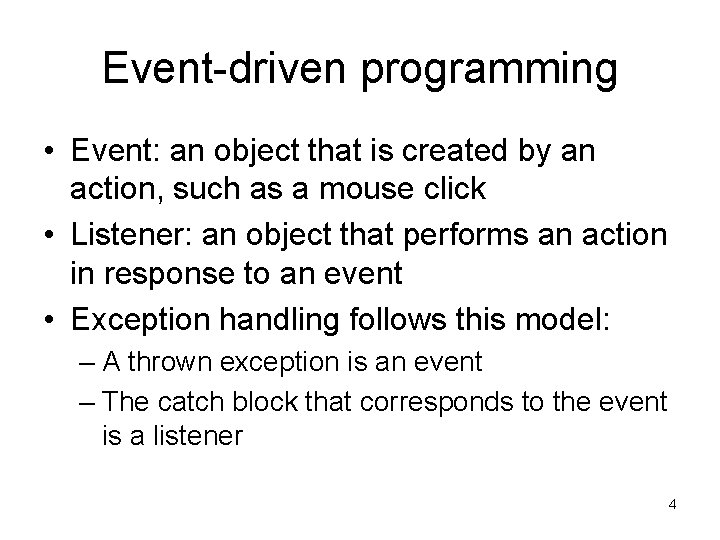
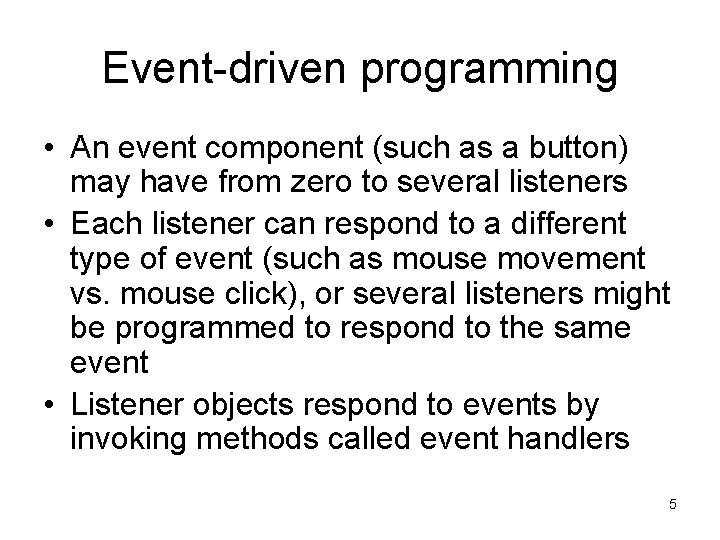
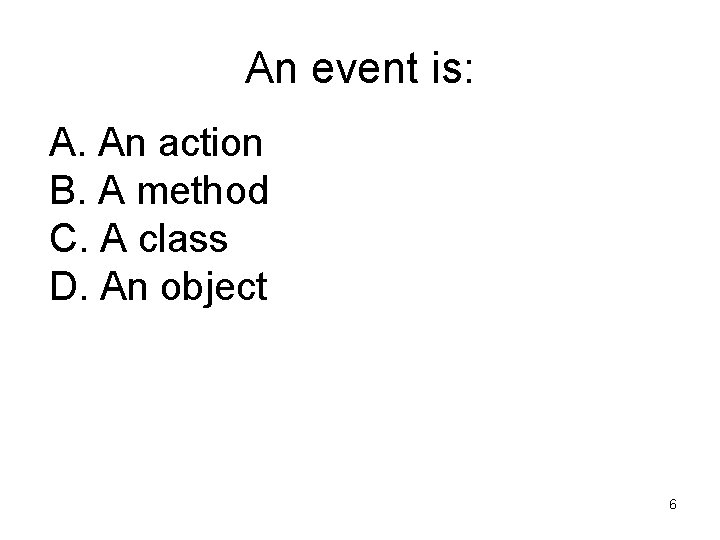
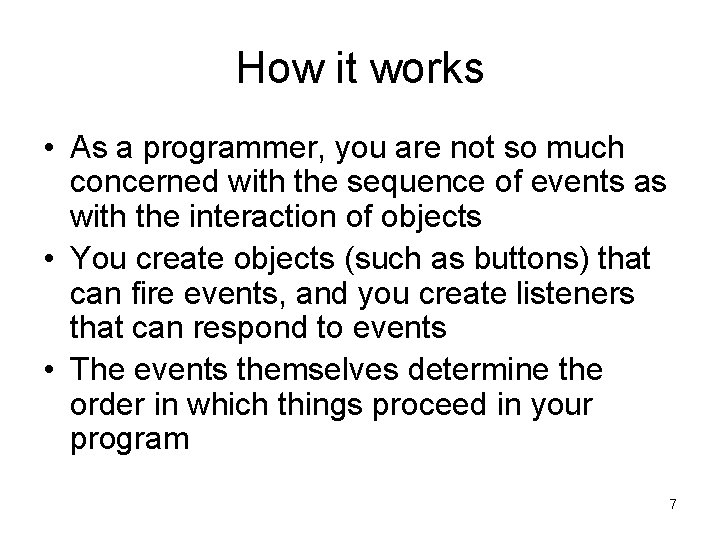
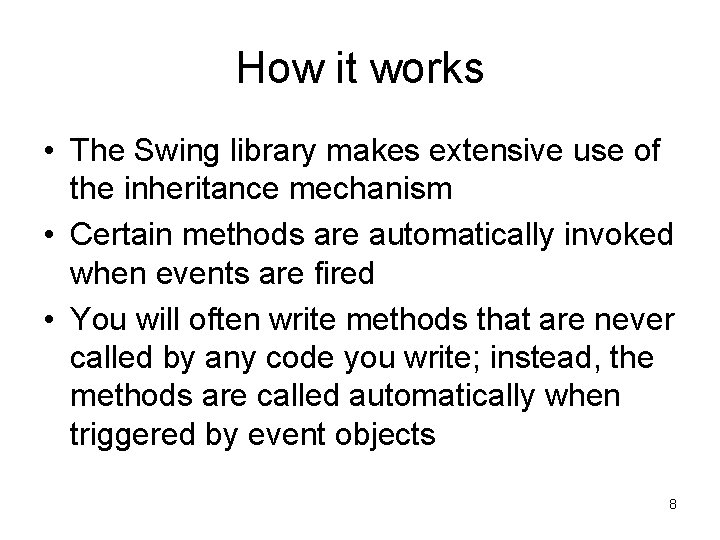
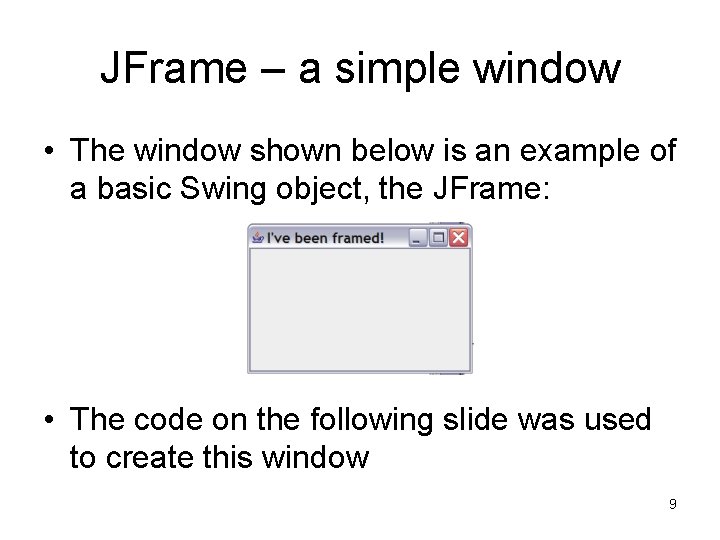
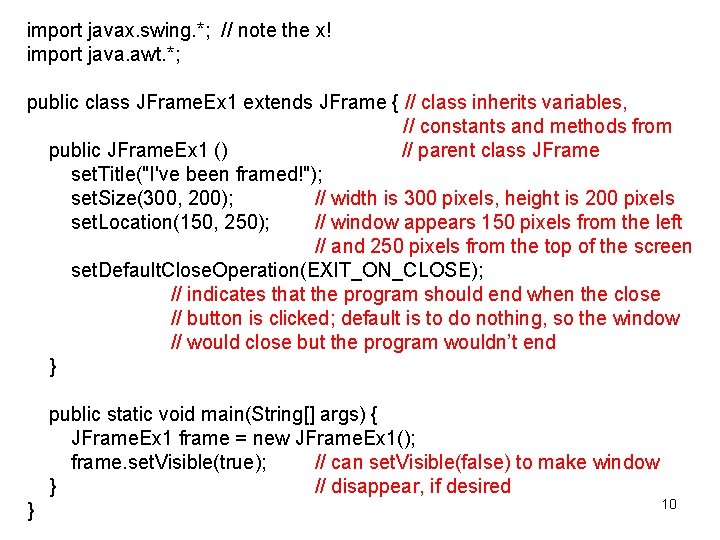
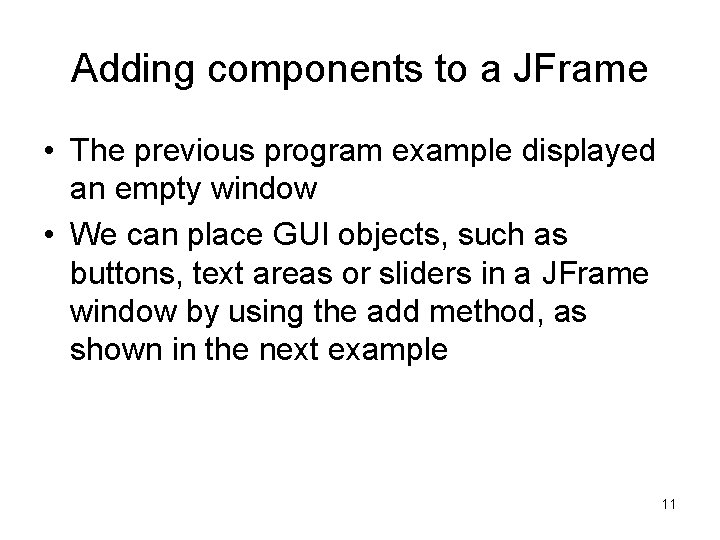
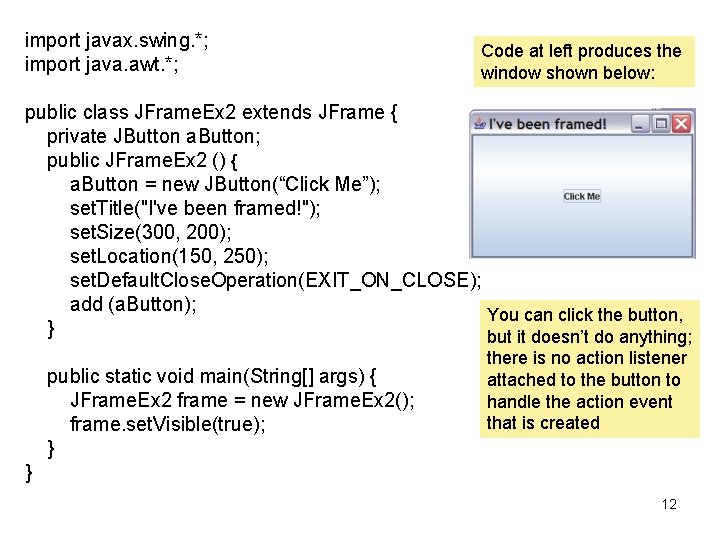
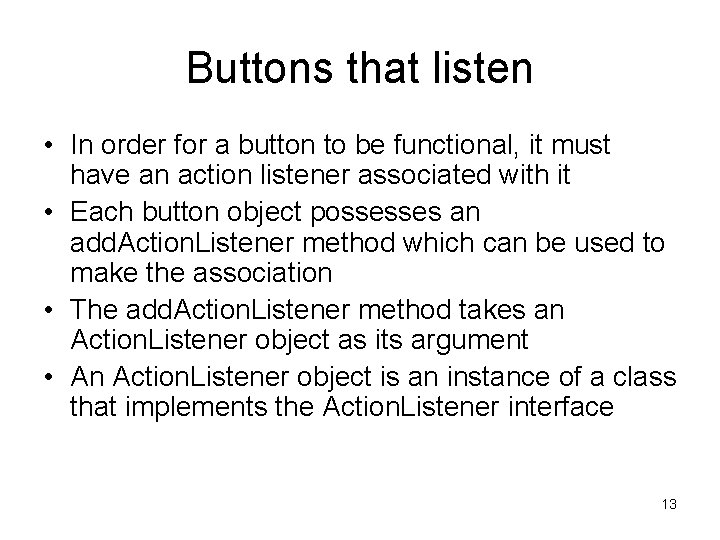
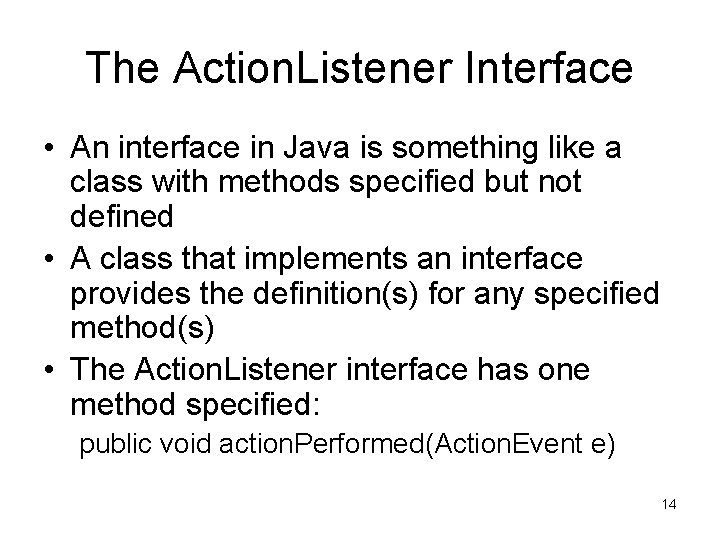
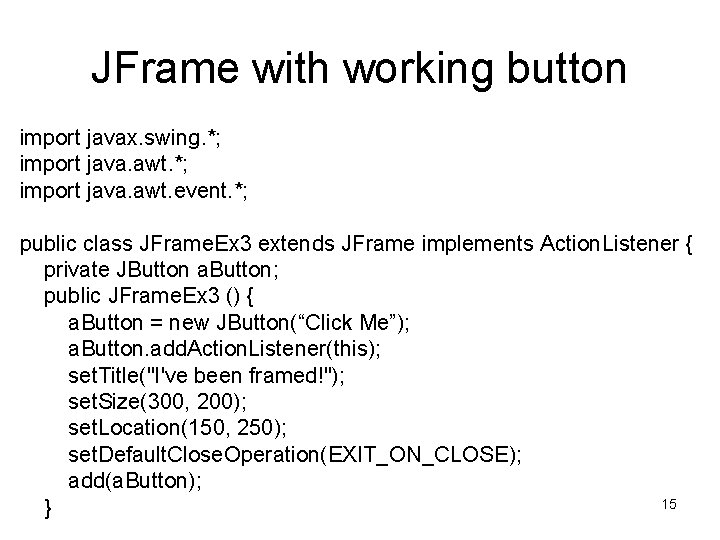
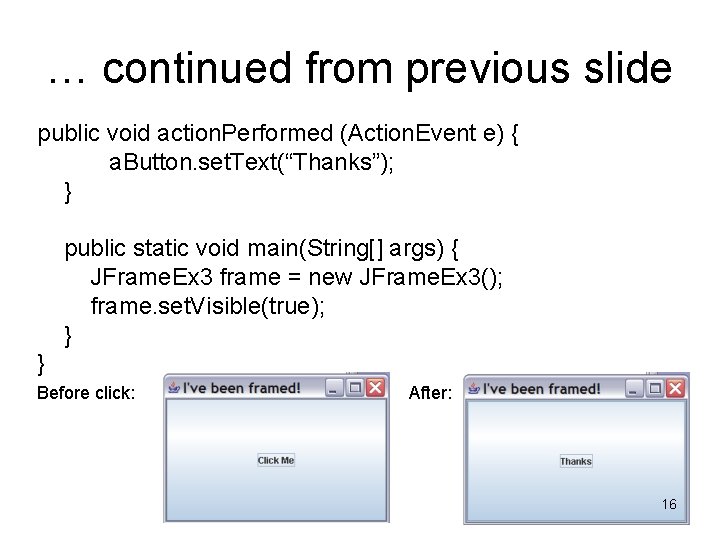
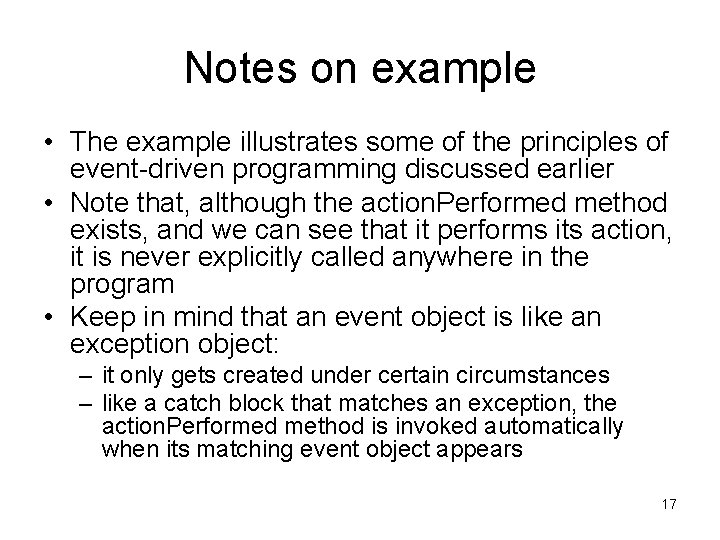
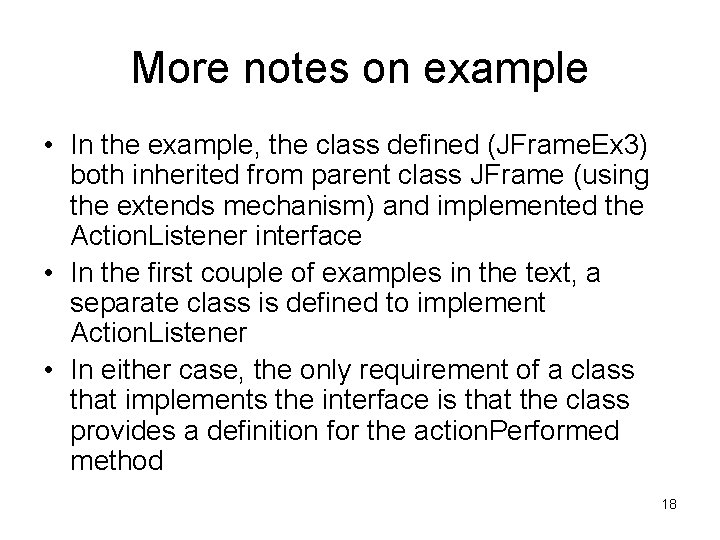
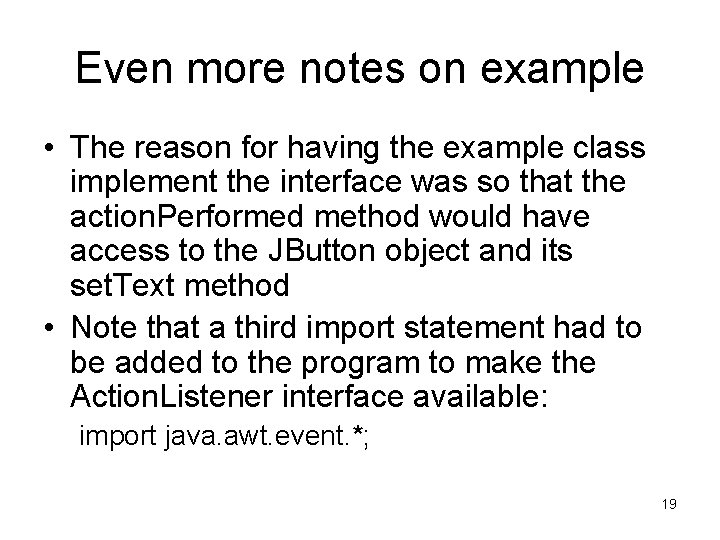
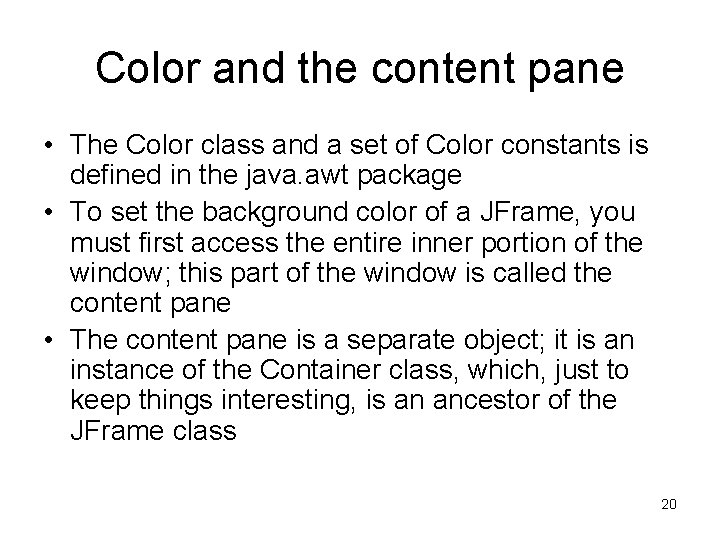
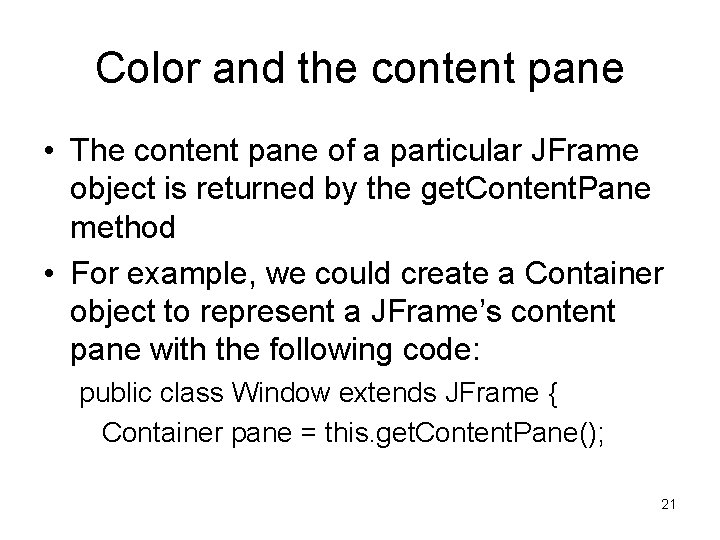
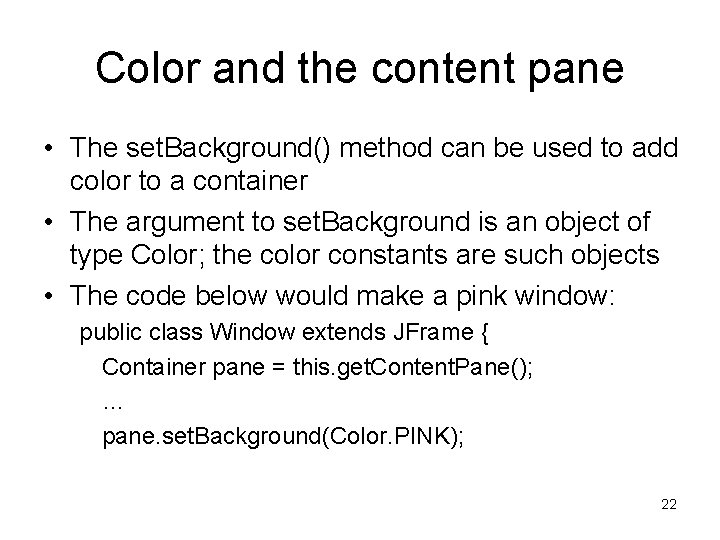
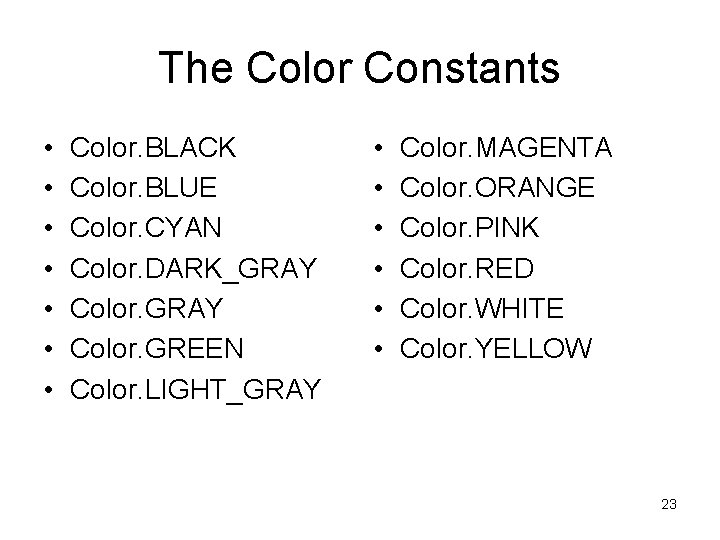
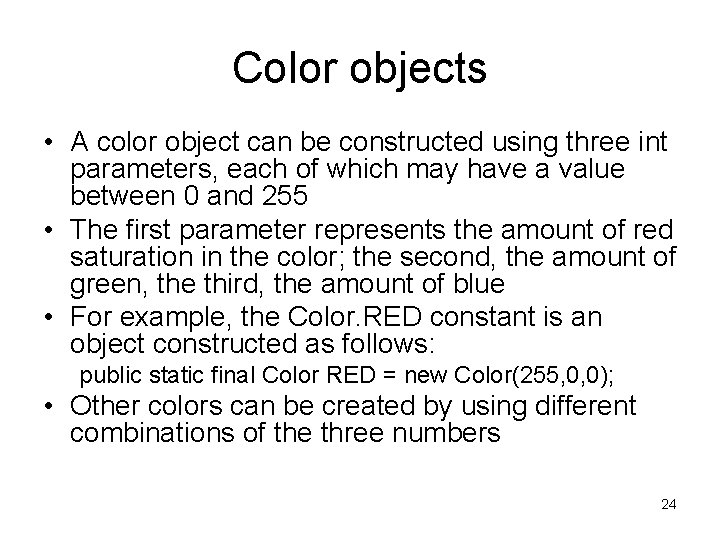
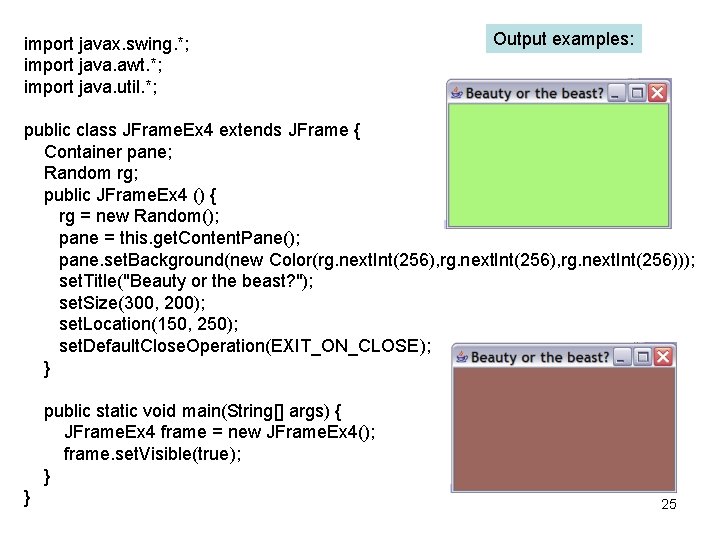
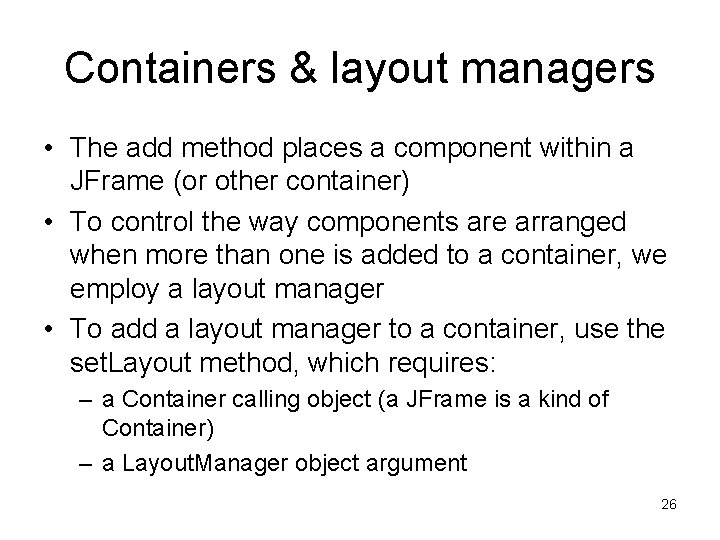
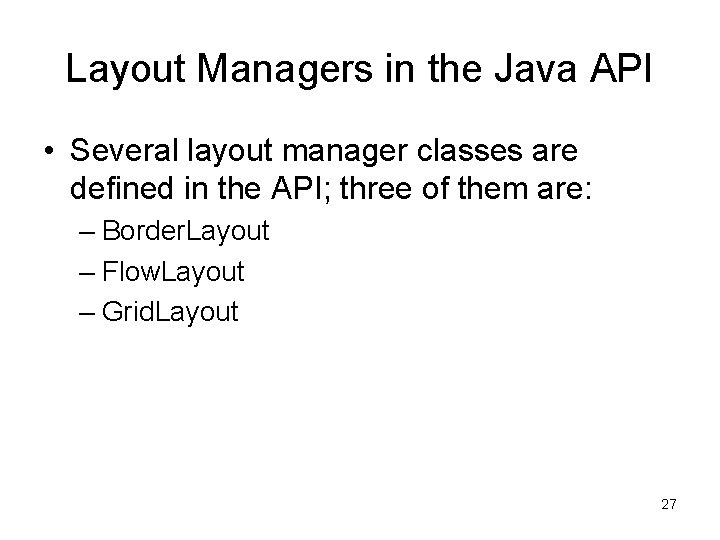
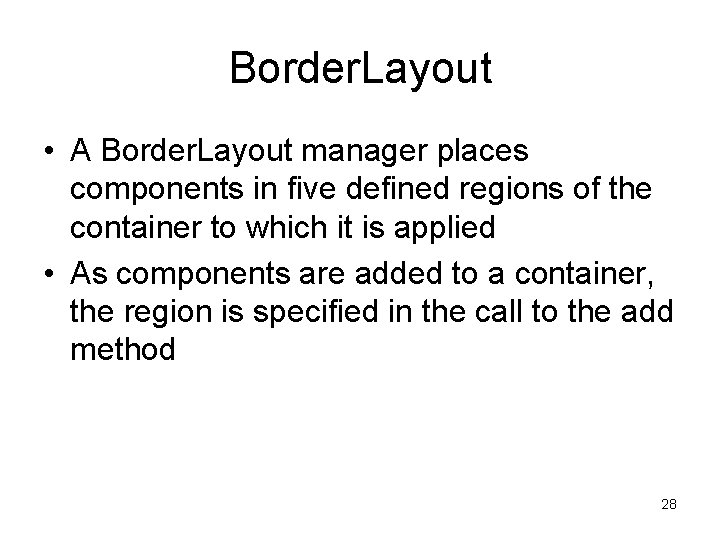
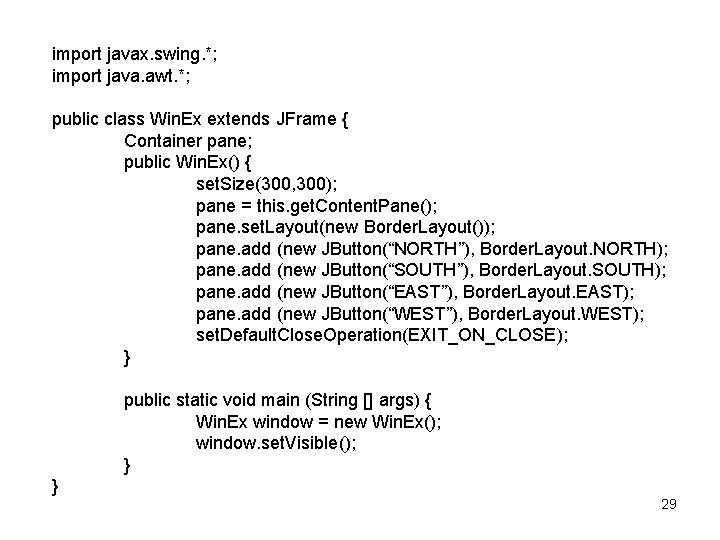
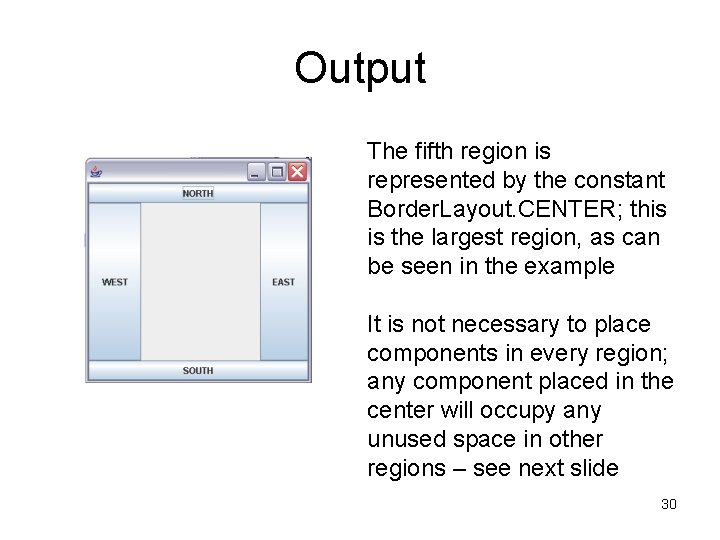
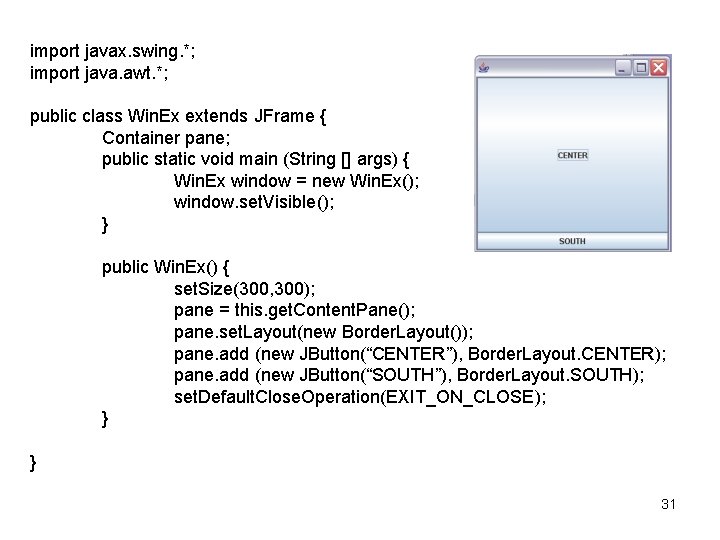
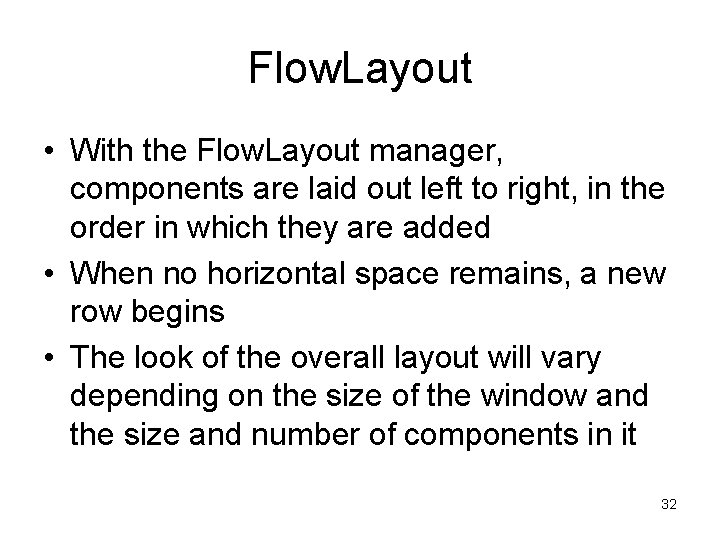
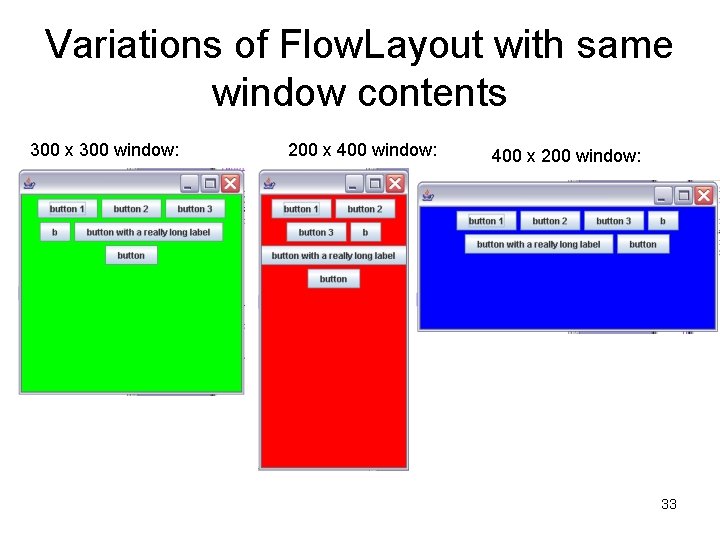
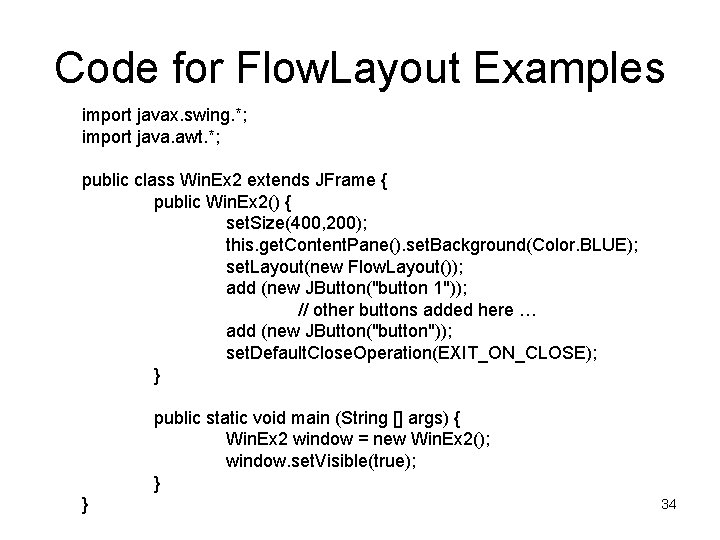
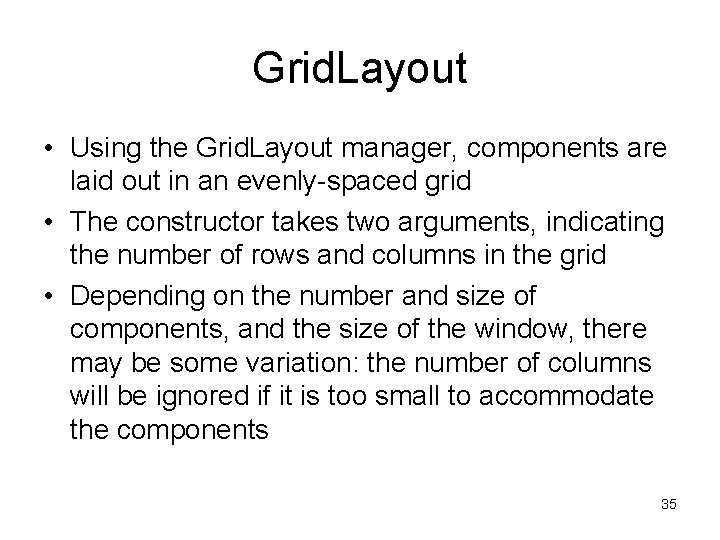
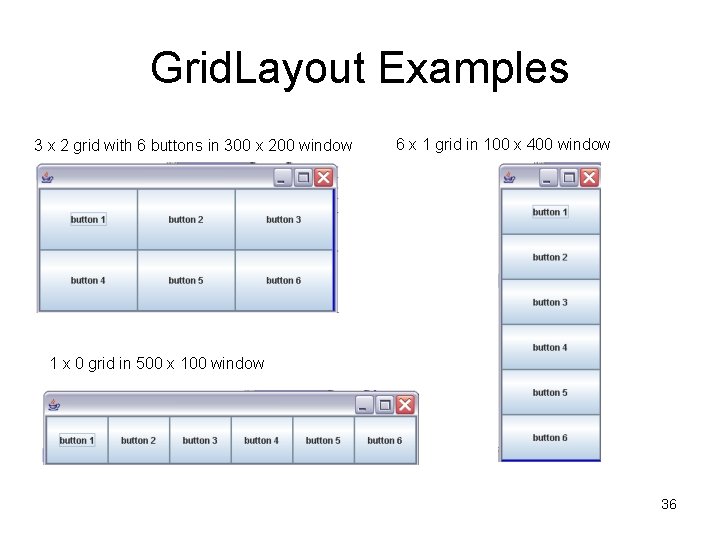
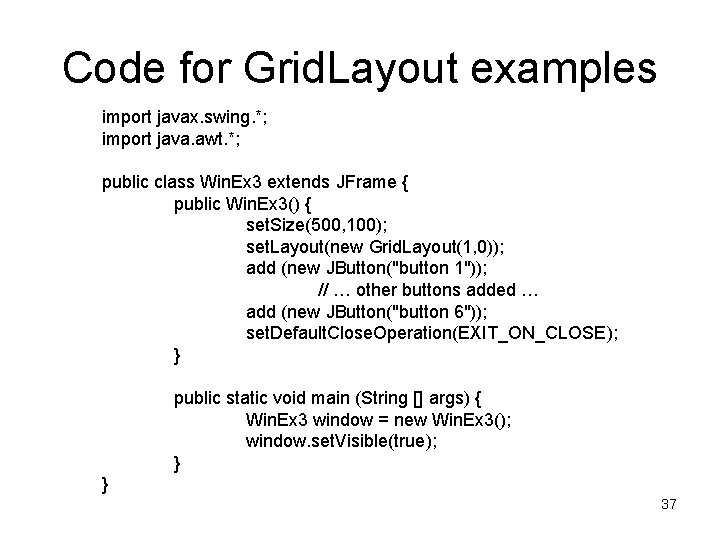
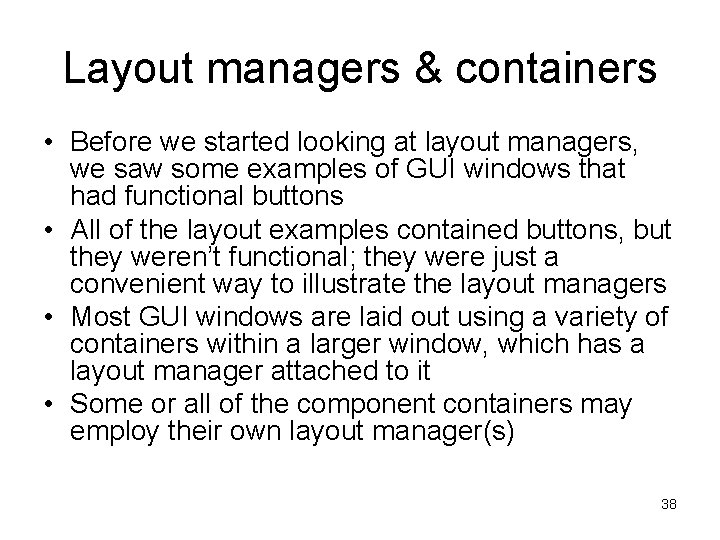
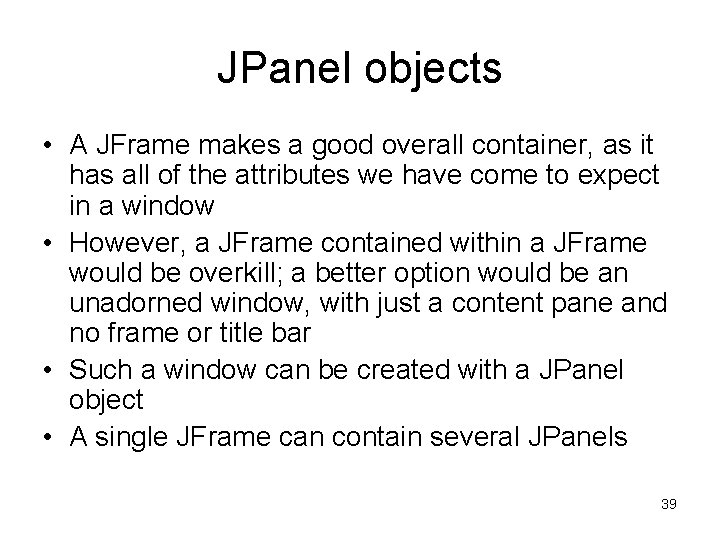
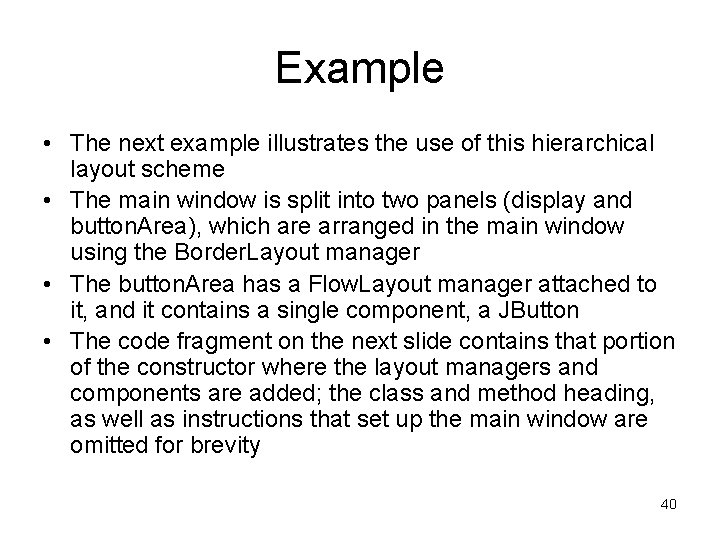
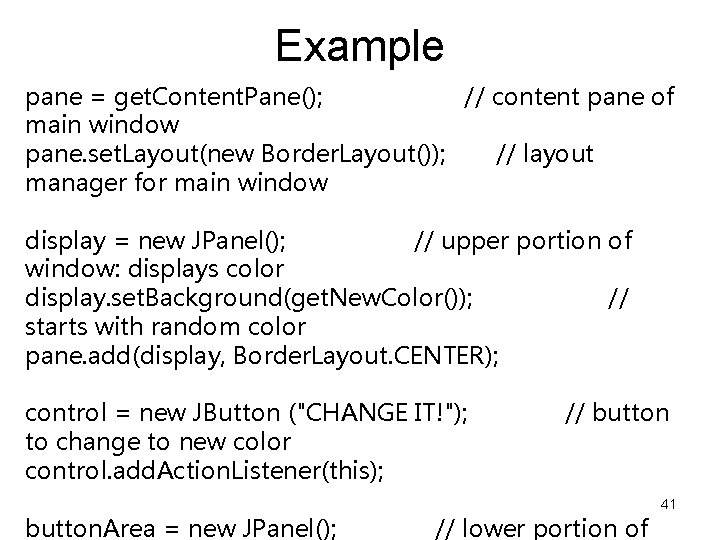
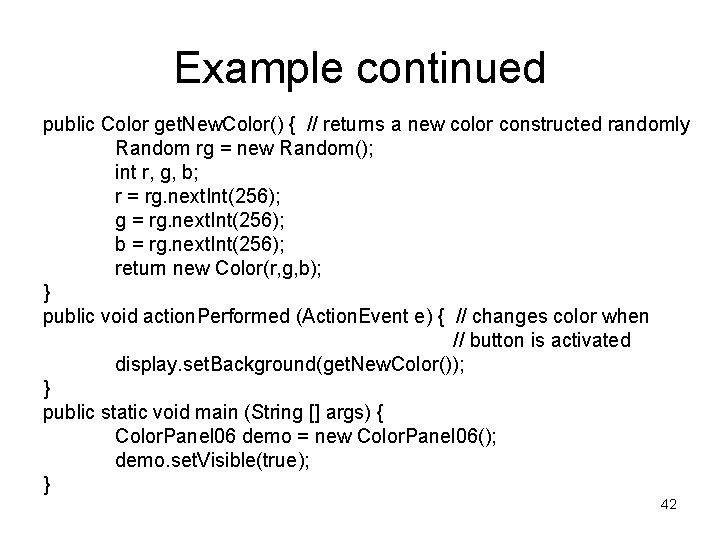
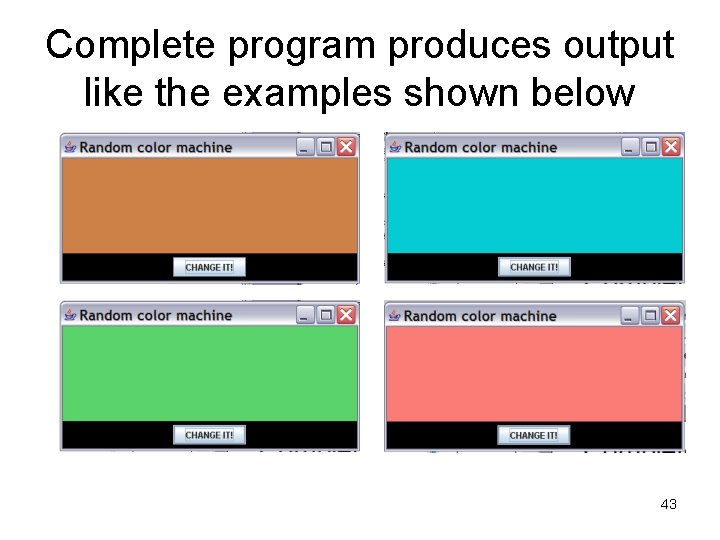
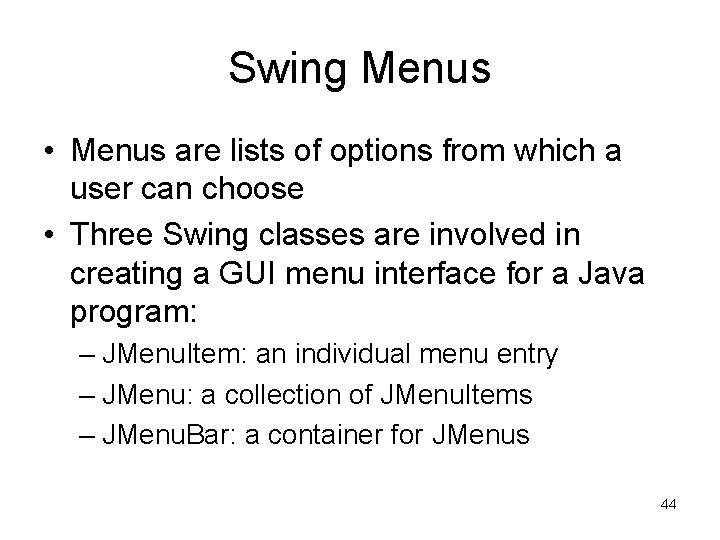
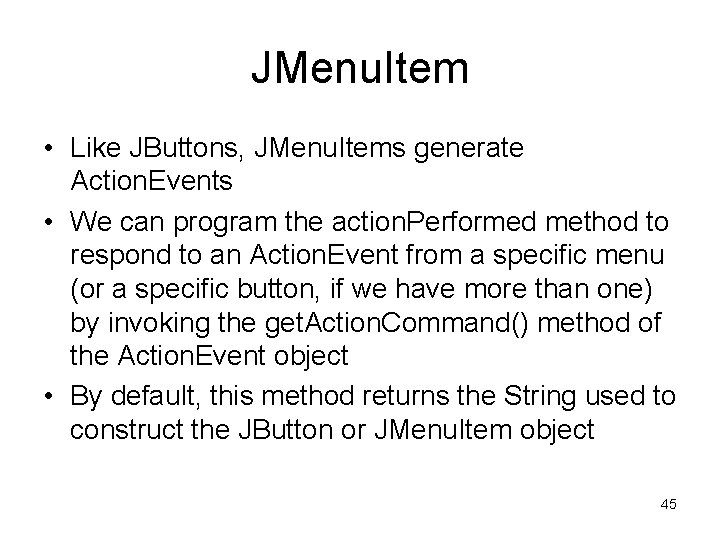
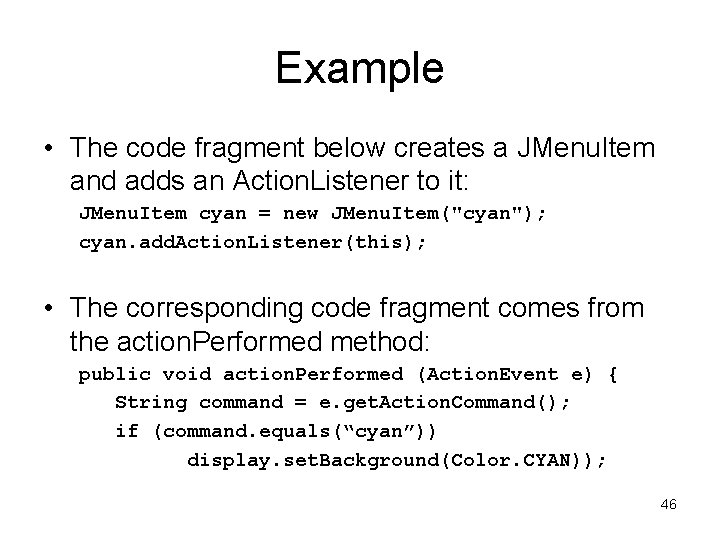
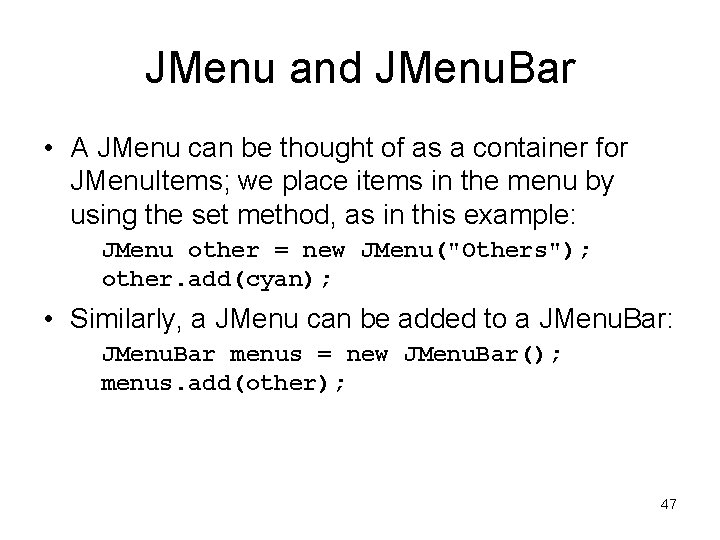
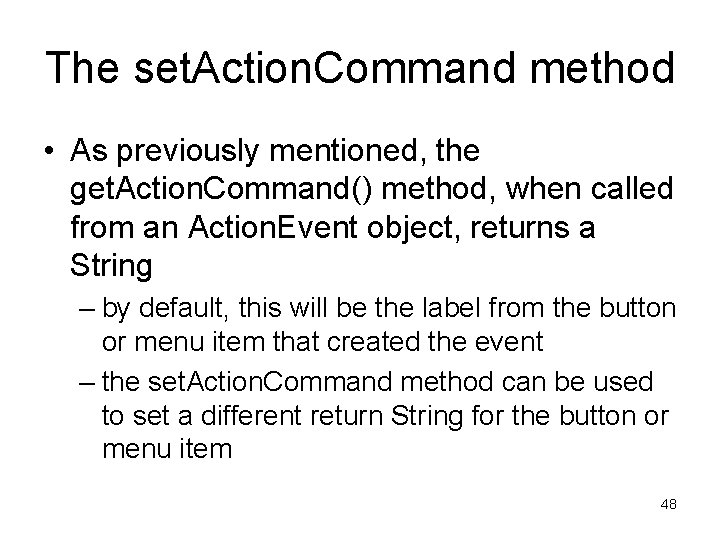
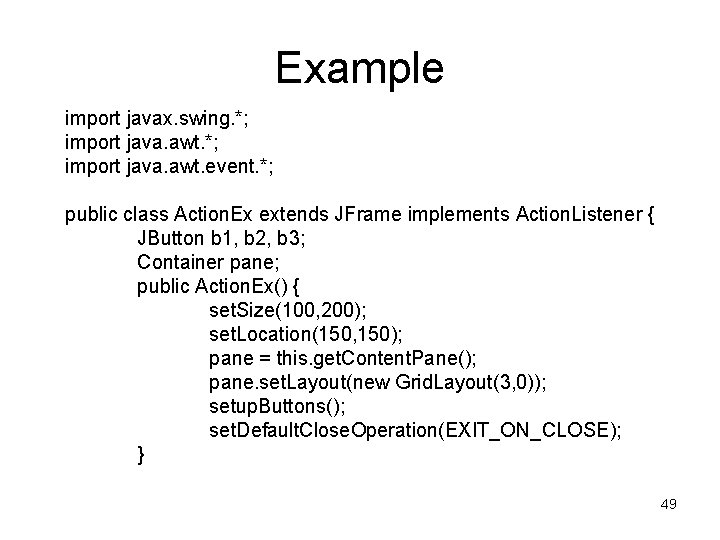
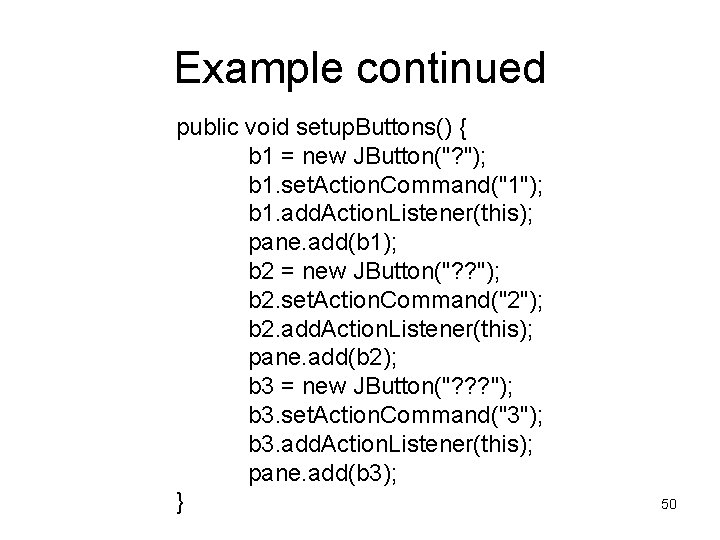
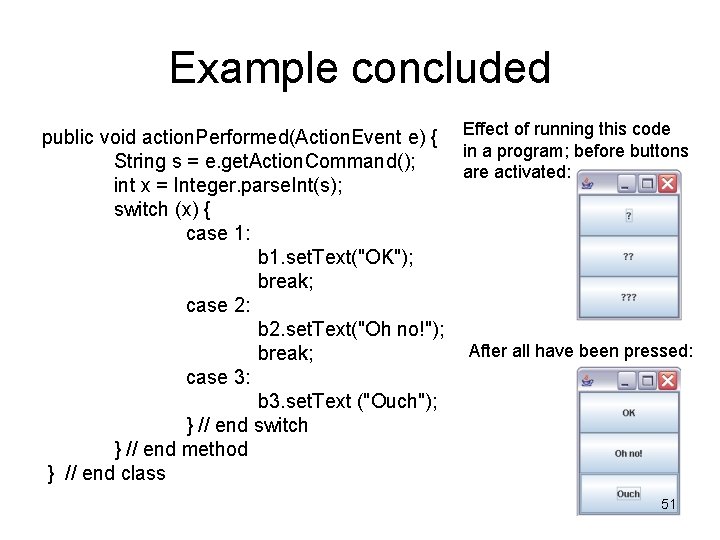
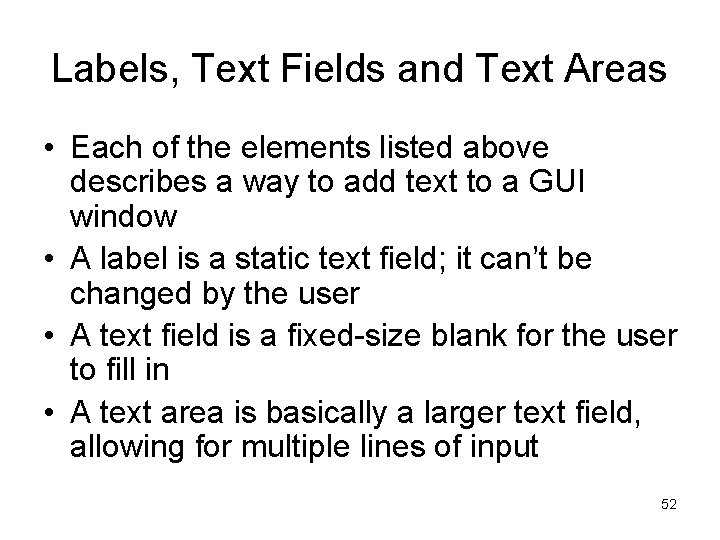
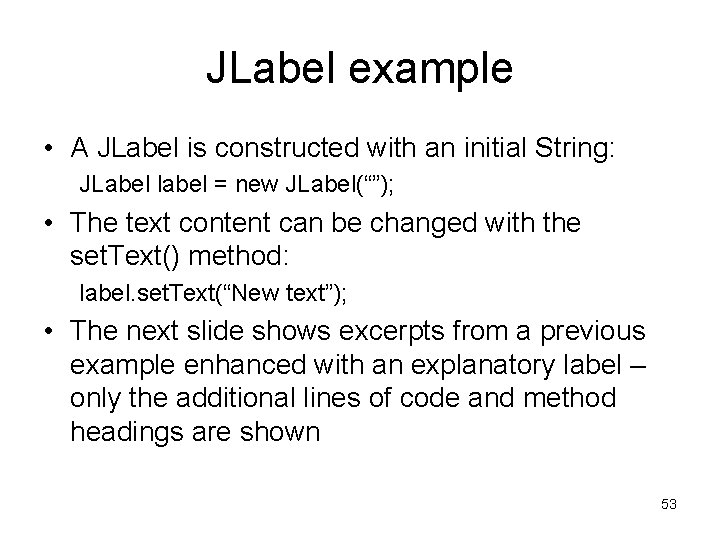
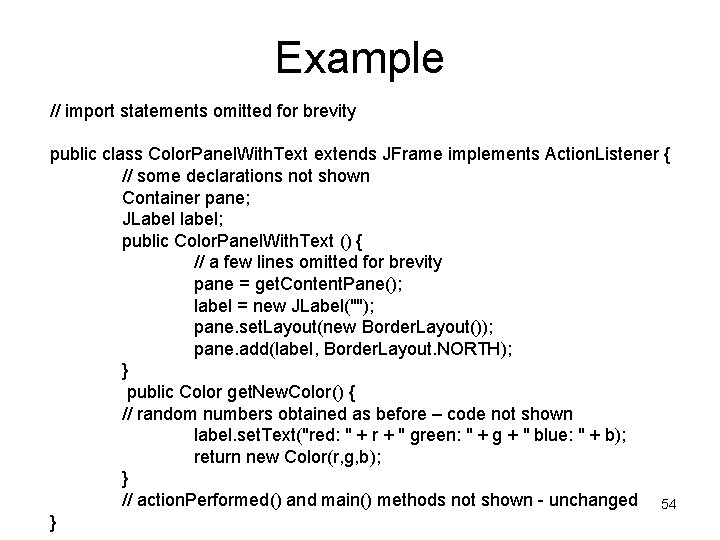
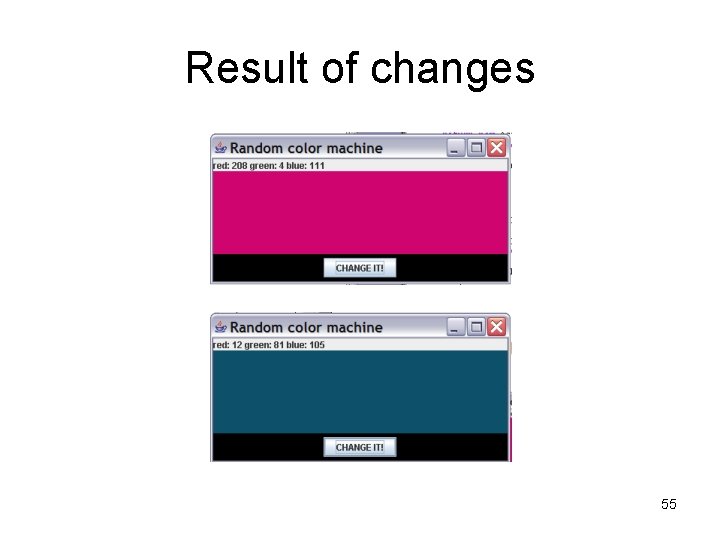
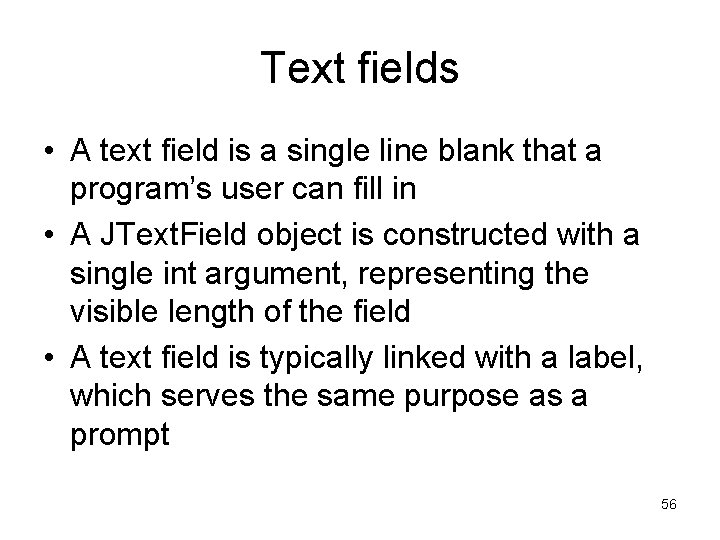
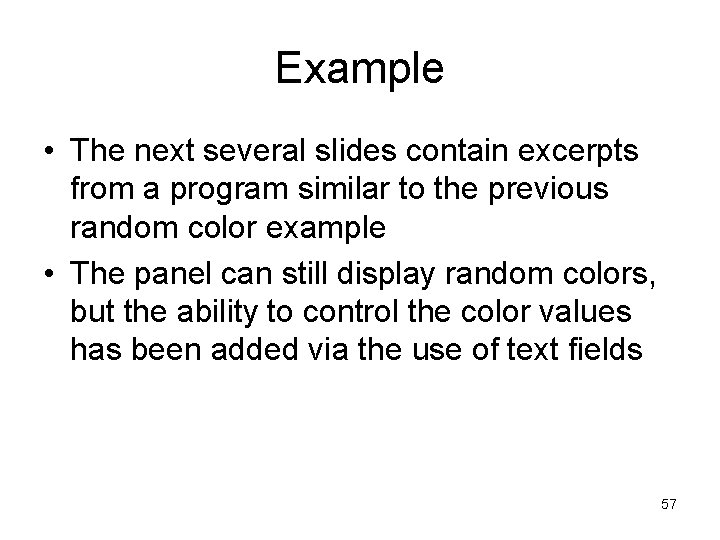
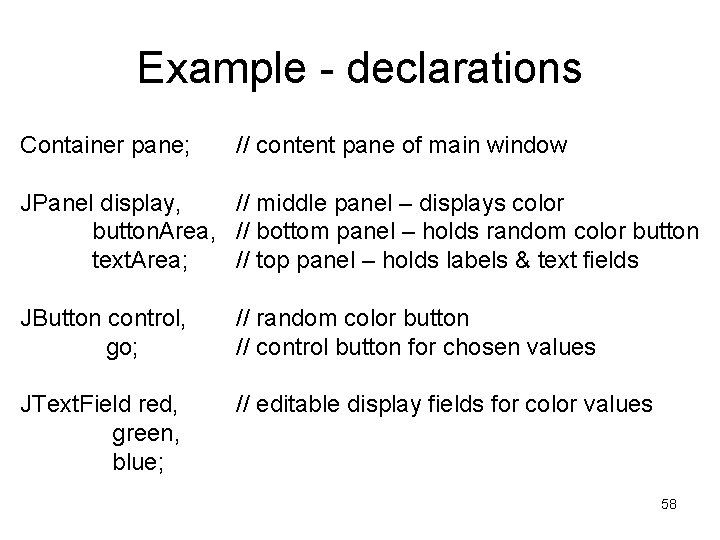
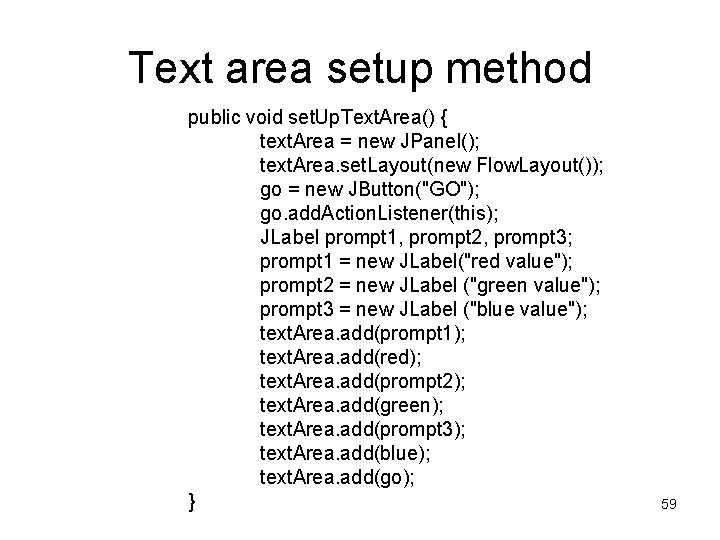
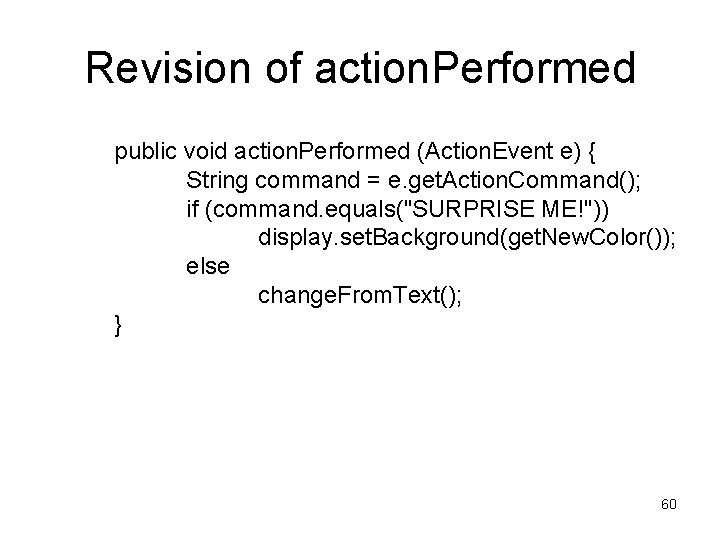
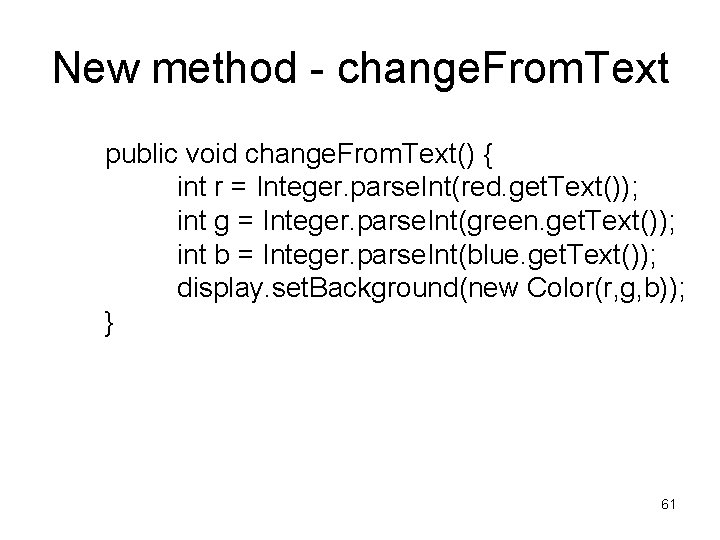
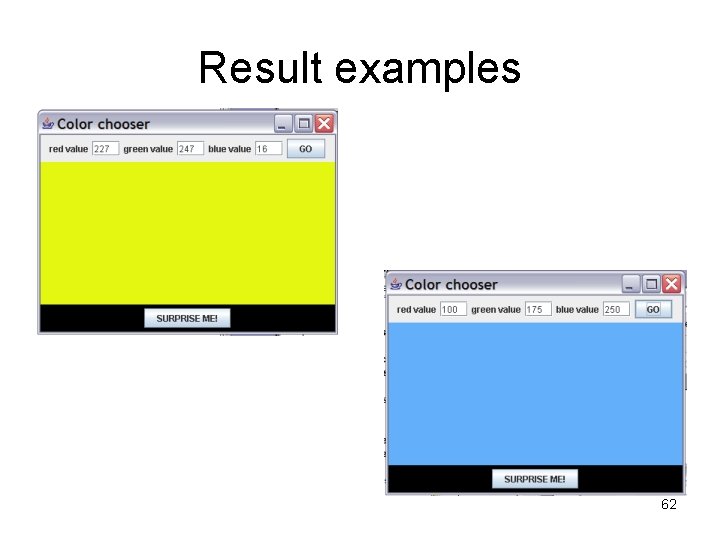
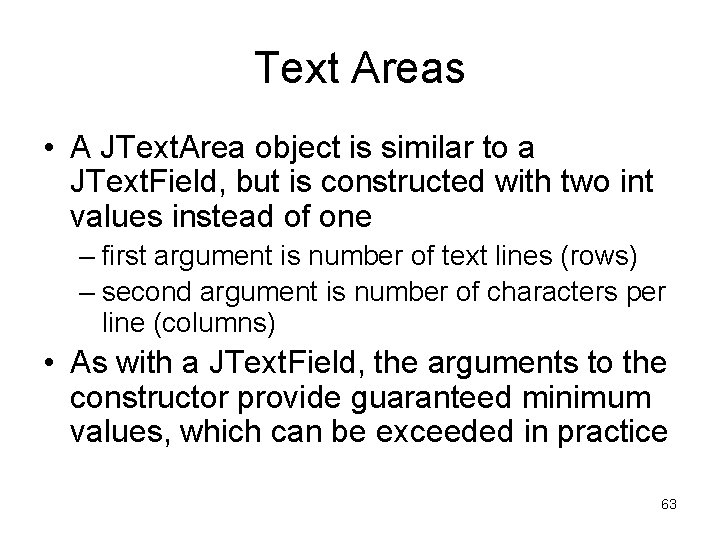
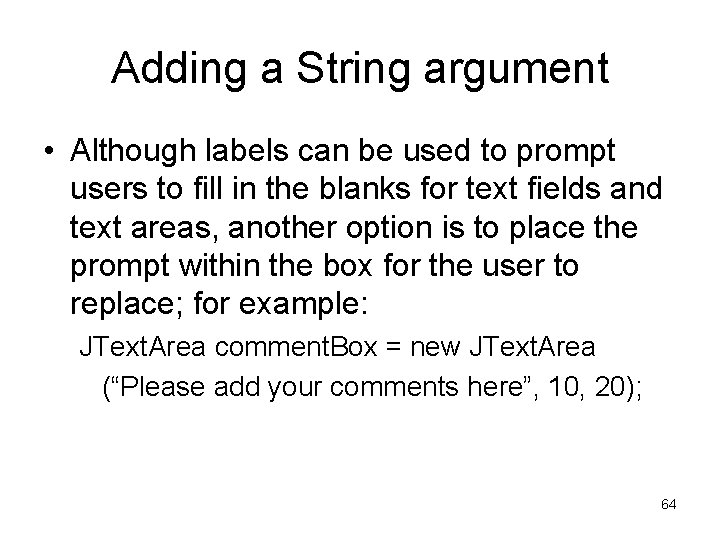
- Slides: 64
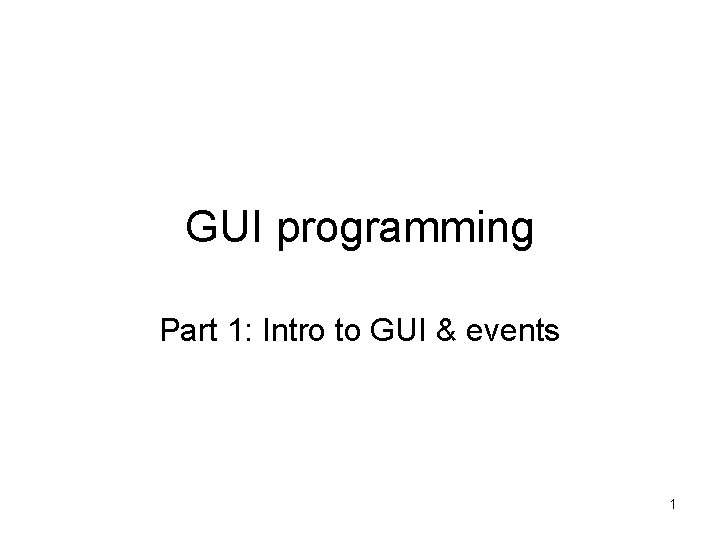
GUI programming Part 1: Intro to GUI & events 1
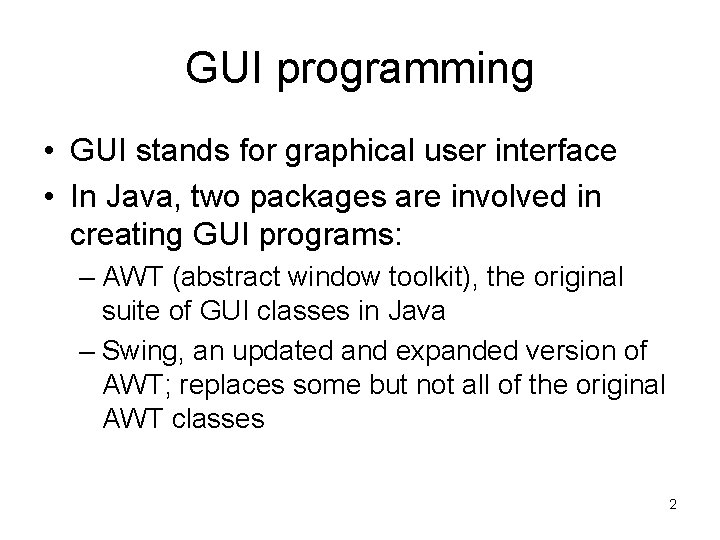
GUI programming • GUI stands for graphical user interface • In Java, two packages are involved in creating GUI programs: – AWT (abstract window toolkit), the original suite of GUI classes in Java – Swing, an updated and expanded version of AWT; replaces some but not all of the original AWT classes 2
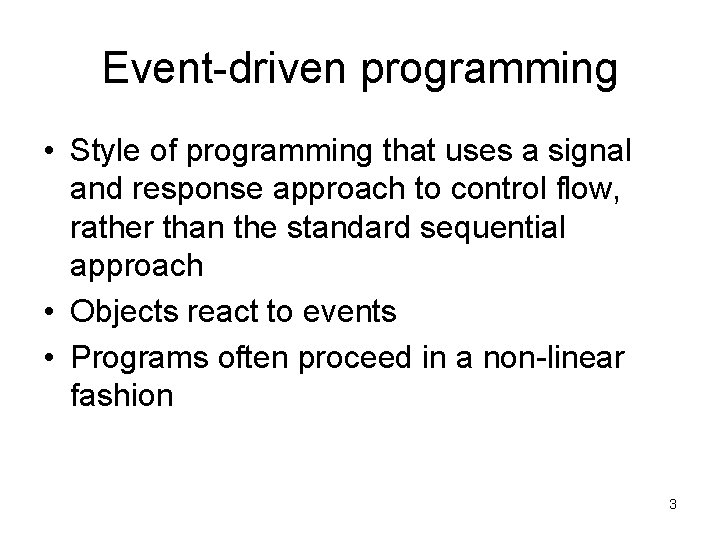
Event-driven programming • Style of programming that uses a signal and response approach to control flow, rather than the standard sequential approach • Objects react to events • Programs often proceed in a non-linear fashion 3
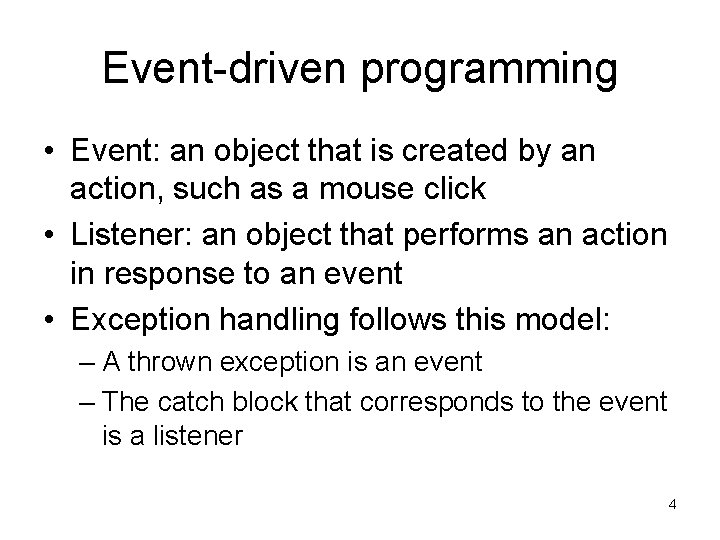
Event-driven programming • Event: an object that is created by an action, such as a mouse click • Listener: an object that performs an action in response to an event • Exception handling follows this model: – A thrown exception is an event – The catch block that corresponds to the event is a listener 4
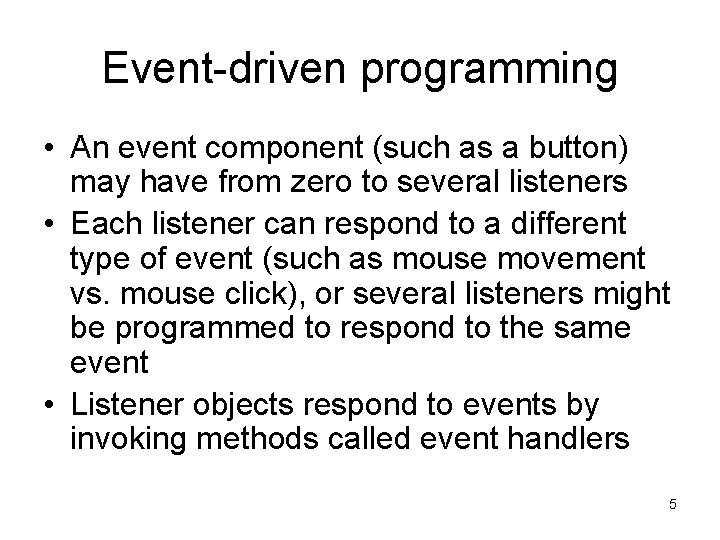
Event-driven programming • An event component (such as a button) may have from zero to several listeners • Each listener can respond to a different type of event (such as mouse movement vs. mouse click), or several listeners might be programmed to respond to the same event • Listener objects respond to events by invoking methods called event handlers 5
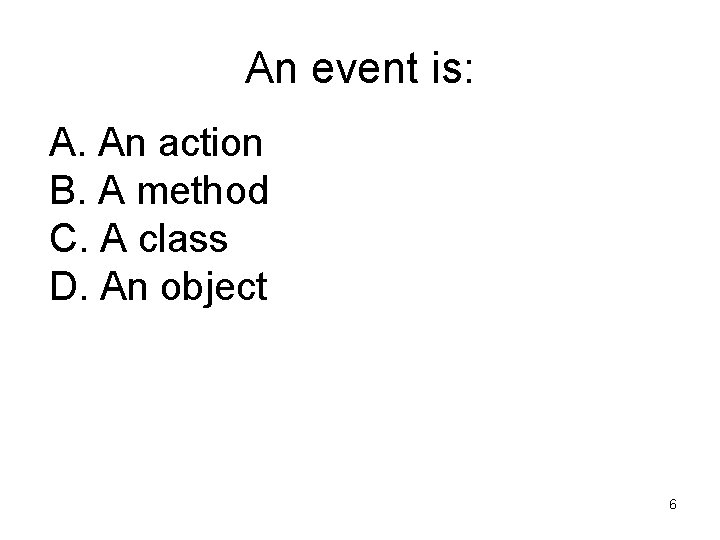
An event is: A. An action B. A method C. A class D. An object 6
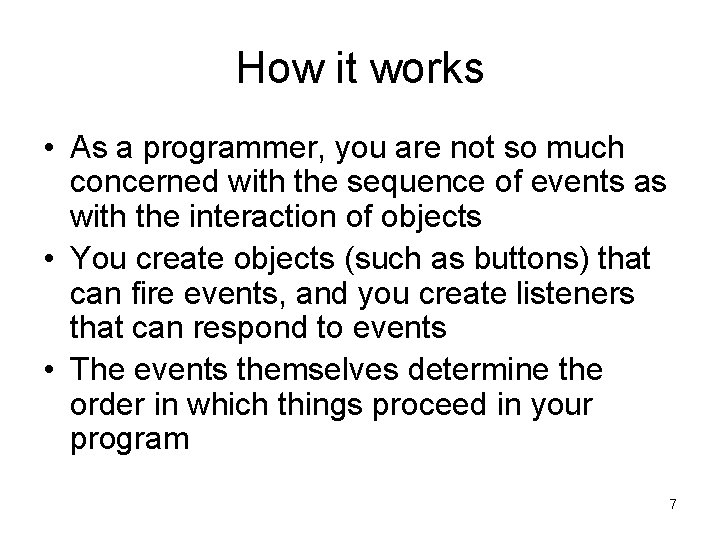
How it works • As a programmer, you are not so much concerned with the sequence of events as with the interaction of objects • You create objects (such as buttons) that can fire events, and you create listeners that can respond to events • The events themselves determine the order in which things proceed in your program 7
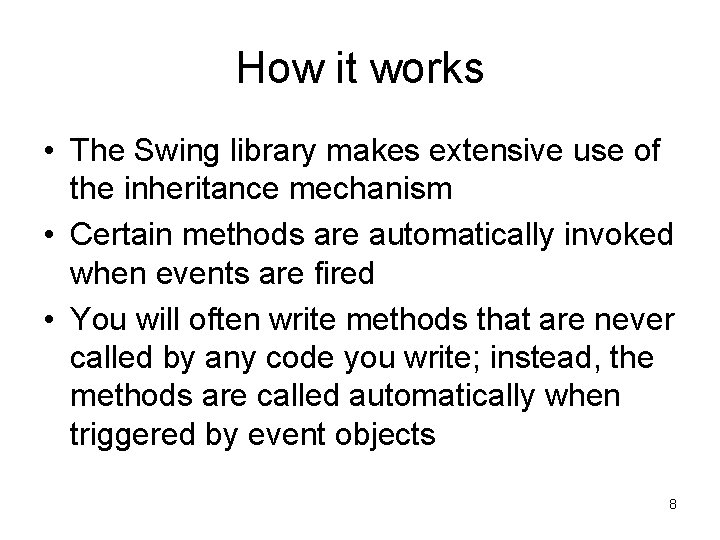
How it works • The Swing library makes extensive use of the inheritance mechanism • Certain methods are automatically invoked when events are fired • You will often write methods that are never called by any code you write; instead, the methods are called automatically when triggered by event objects 8
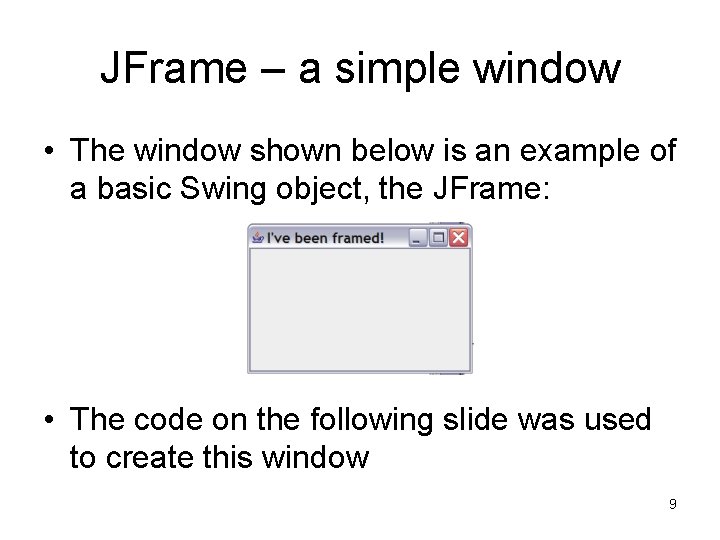
JFrame – a simple window • The window shown below is an example of a basic Swing object, the JFrame: • The code on the following slide was used to create this window 9
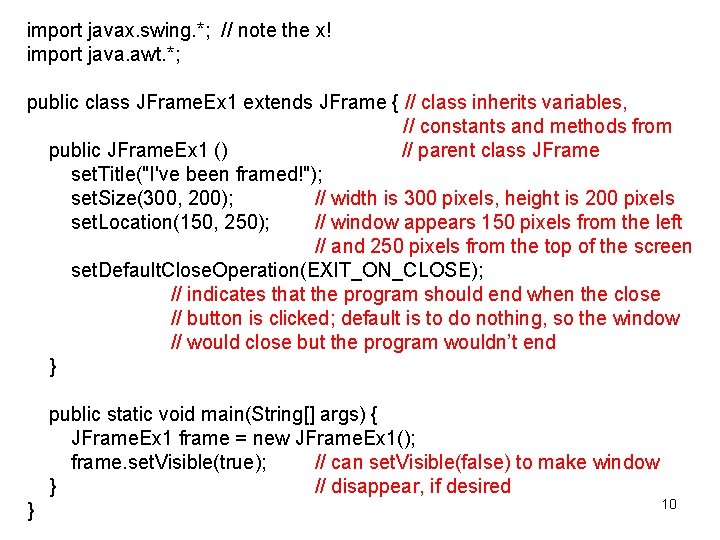
import javax. swing. *; // note the x! import java. awt. *; public class JFrame. Ex 1 extends JFrame { // class inherits variables, // constants and methods from public JFrame. Ex 1 () // parent class JFrame set. Title("I've been framed!"); set. Size(300, 200); // width is 300 pixels, height is 200 pixels set. Location(150, 250); // window appears 150 pixels from the left // and 250 pixels from the top of the screen set. Default. Close. Operation(EXIT_ON_CLOSE); // indicates that the program should end when the close // button is clicked; default is to do nothing, so the window // would close but the program wouldn’t end } public static void main(String[] args) { JFrame. Ex 1 frame = new JFrame. Ex 1(); frame. set. Visible(true); // can set. Visible(false) to make window } // disappear, if desired } 10
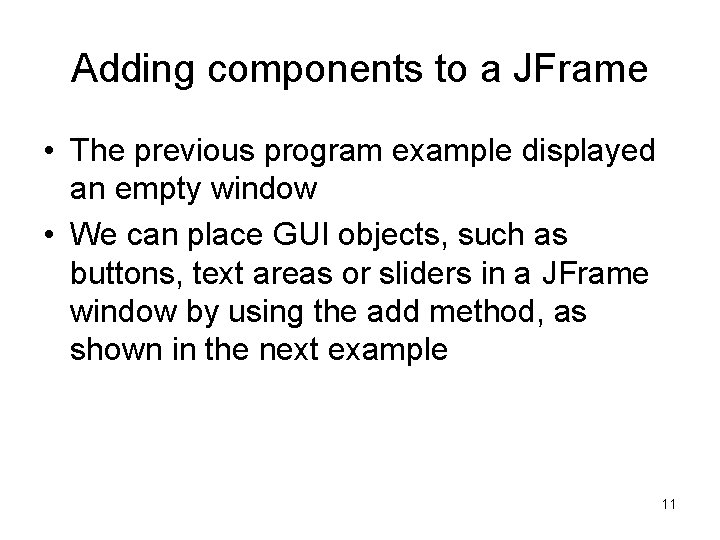
Adding components to a JFrame • The previous program example displayed an empty window • We can place GUI objects, such as buttons, text areas or sliders in a JFrame window by using the add method, as shown in the next example 11
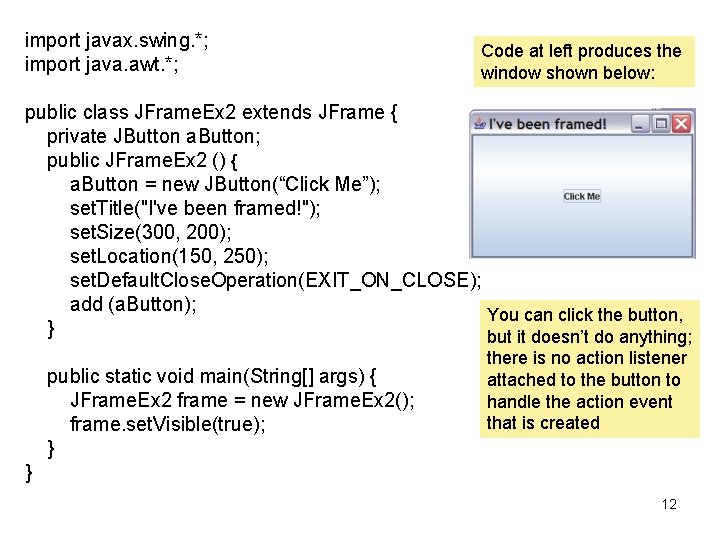
import javax. swing. *; import java. awt. *; Code at left produces the window shown below: public class JFrame. Ex 2 extends JFrame { private JButton a. Button; public JFrame. Ex 2 () { a. Button = new JButton(“Click Me”); set. Title("I've been framed!"); set. Size(300, 200); set. Location(150, 250); set. Default. Close. Operation(EXIT_ON_CLOSE); add (a. Button); You can click the button, } but it doesn’t do anything; public static void main(String[] args) { JFrame. Ex 2 frame = new JFrame. Ex 2(); frame. set. Visible(true); } there is no action listener attached to the button to handle the action event that is created } 12
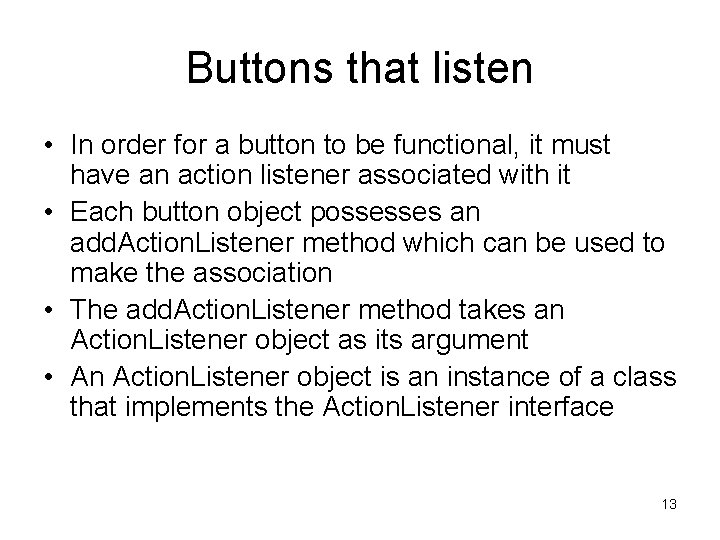
Buttons that listen • In order for a button to be functional, it must have an action listener associated with it • Each button object possesses an add. Action. Listener method which can be used to make the association • The add. Action. Listener method takes an Action. Listener object as its argument • An Action. Listener object is an instance of a class that implements the Action. Listener interface 13
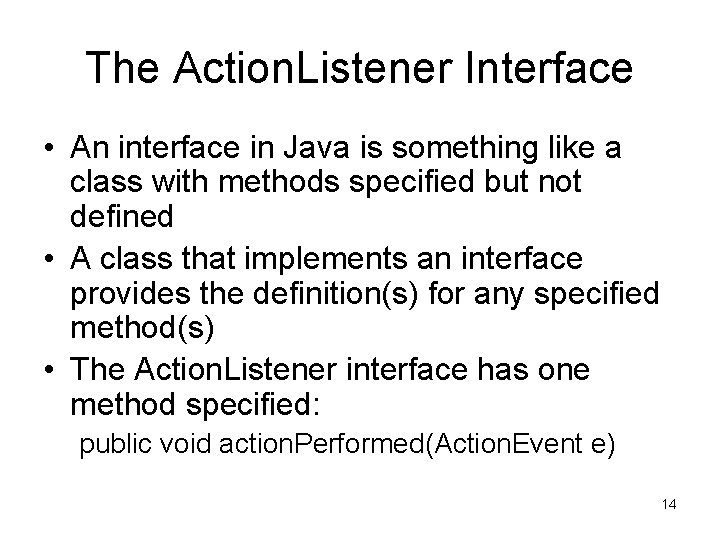
The Action. Listener Interface • An interface in Java is something like a class with methods specified but not defined • A class that implements an interface provides the definition(s) for any specified method(s) • The Action. Listener interface has one method specified: public void action. Performed(Action. Event e) 14
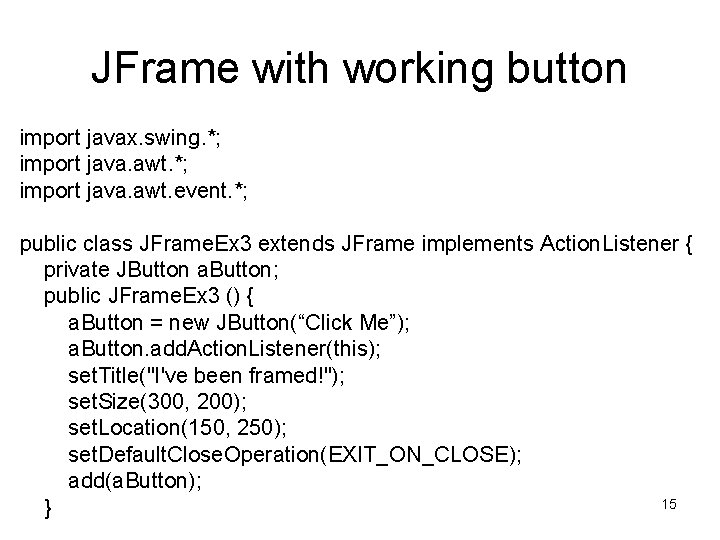
JFrame with working button import javax. swing. *; import java. awt. event. *; public class JFrame. Ex 3 extends JFrame implements Action. Listener { private JButton a. Button; public JFrame. Ex 3 () { a. Button = new JButton(“Click Me”); a. Button. add. Action. Listener(this); set. Title("I've been framed!"); set. Size(300, 200); set. Location(150, 250); set. Default. Close. Operation(EXIT_ON_CLOSE); add(a. Button); 15 }
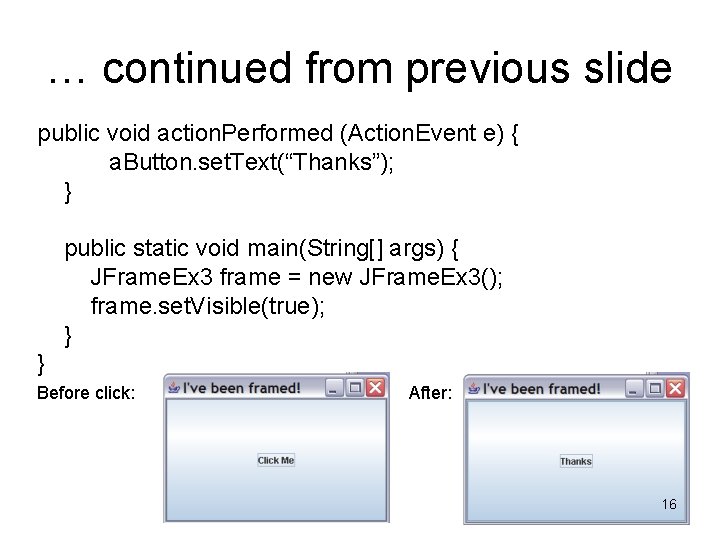
… continued from previous slide public void action. Performed (Action. Event e) { a. Button. set. Text(“Thanks”); } public static void main(String[] args) { JFrame. Ex 3 frame = new JFrame. Ex 3(); frame. set. Visible(true); } } Before click: After: 16
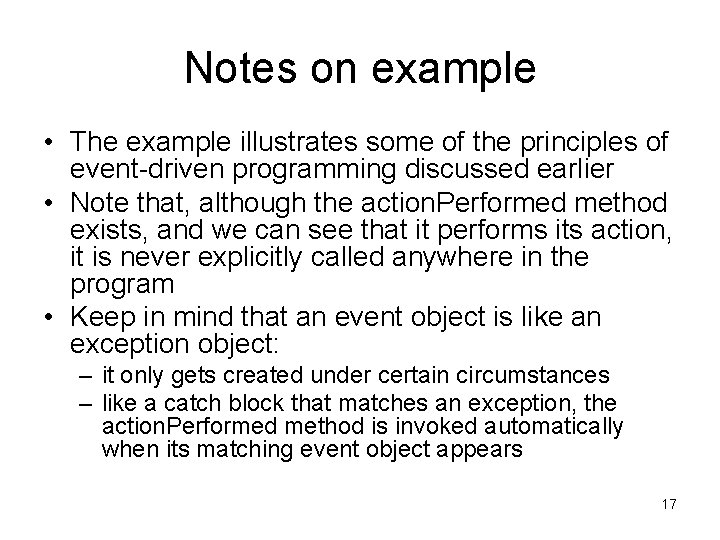
Notes on example • The example illustrates some of the principles of event-driven programming discussed earlier • Note that, although the action. Performed method exists, and we can see that it performs its action, it is never explicitly called anywhere in the program • Keep in mind that an event object is like an exception object: – it only gets created under certain circumstances – like a catch block that matches an exception, the action. Performed method is invoked automatically when its matching event object appears 17
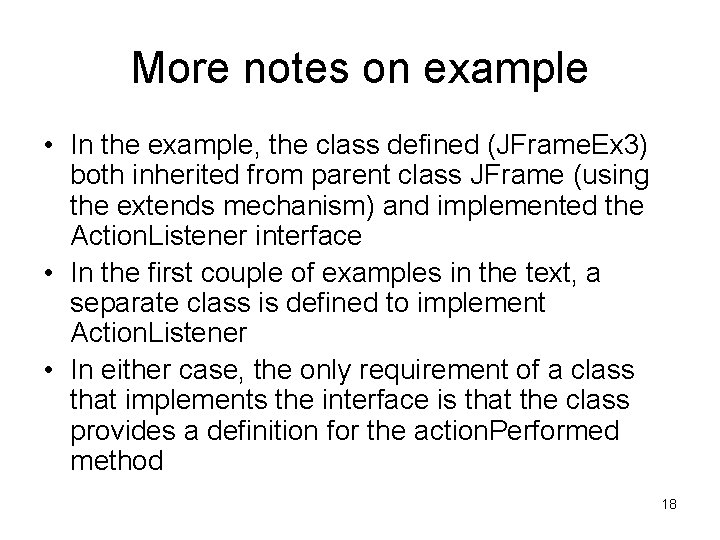
More notes on example • In the example, the class defined (JFrame. Ex 3) both inherited from parent class JFrame (using the extends mechanism) and implemented the Action. Listener interface • In the first couple of examples in the text, a separate class is defined to implement Action. Listener • In either case, the only requirement of a class that implements the interface is that the class provides a definition for the action. Performed method 18
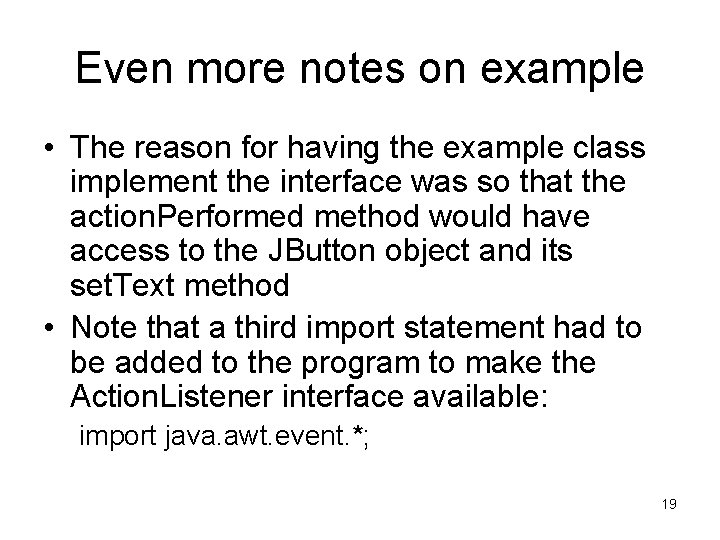
Even more notes on example • The reason for having the example class implement the interface was so that the action. Performed method would have access to the JButton object and its set. Text method • Note that a third import statement had to be added to the program to make the Action. Listener interface available: import java. awt. event. *; 19
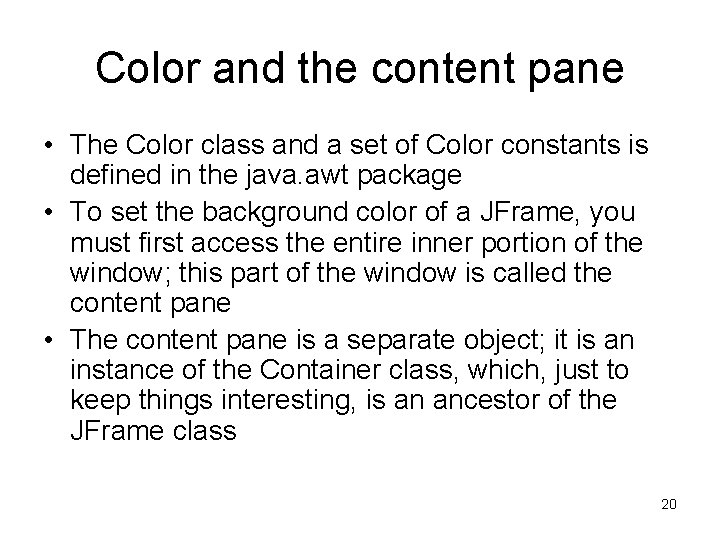
Color and the content pane • The Color class and a set of Color constants is defined in the java. awt package • To set the background color of a JFrame, you must first access the entire inner portion of the window; this part of the window is called the content pane • The content pane is a separate object; it is an instance of the Container class, which, just to keep things interesting, is an ancestor of the JFrame class 20
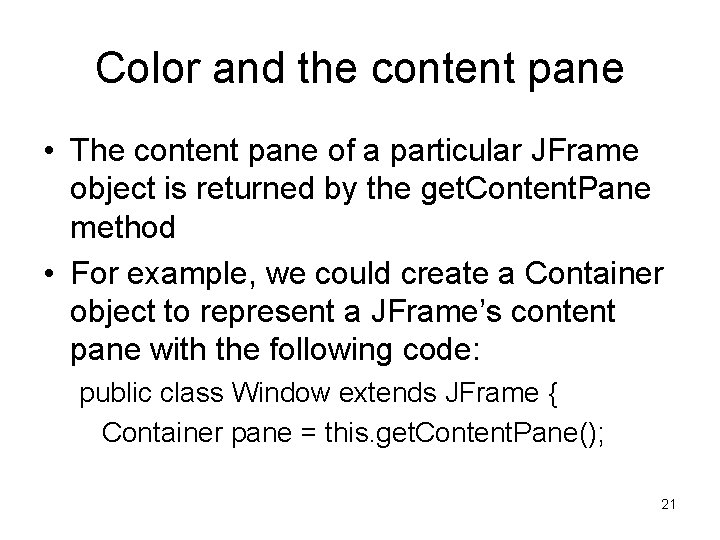
Color and the content pane • The content pane of a particular JFrame object is returned by the get. Content. Pane method • For example, we could create a Container object to represent a JFrame’s content pane with the following code: public class Window extends JFrame { Container pane = this. get. Content. Pane(); 21
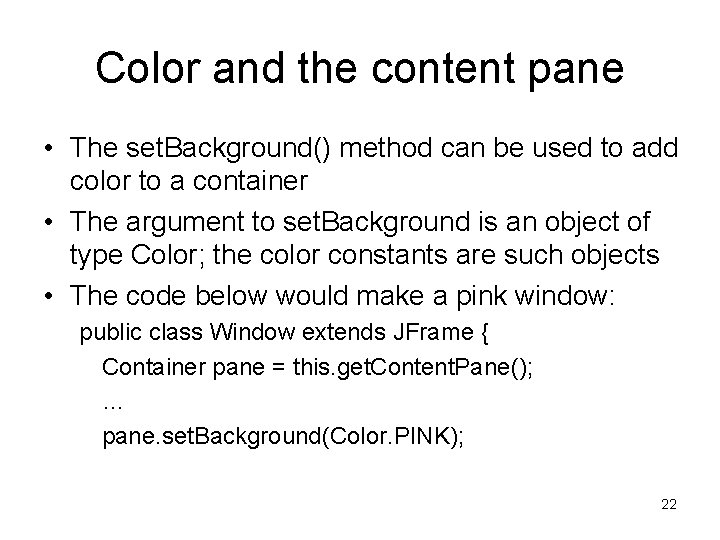
Color and the content pane • The set. Background() method can be used to add color to a container • The argument to set. Background is an object of type Color; the color constants are such objects • The code below would make a pink window: public class Window extends JFrame { Container pane = this. get. Content. Pane(); … pane. set. Background(Color. PINK); 22
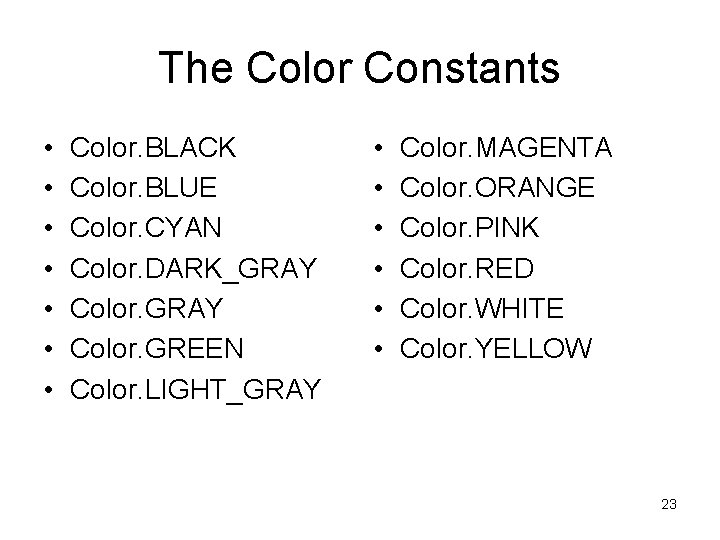
The Color Constants • • Color. BLACK Color. BLUE Color. CYAN Color. DARK_GRAY Color. GREEN Color. LIGHT_GRAY • • • Color. MAGENTA Color. ORANGE Color. PINK Color. RED Color. WHITE Color. YELLOW 23
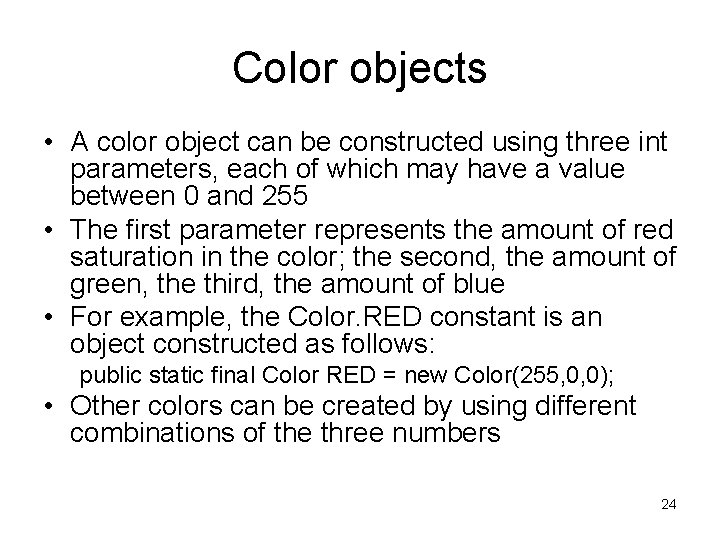
Color objects • A color object can be constructed using three int parameters, each of which may have a value between 0 and 255 • The first parameter represents the amount of red saturation in the color; the second, the amount of green, the third, the amount of blue • For example, the Color. RED constant is an object constructed as follows: public static final Color RED = new Color(255, 0, 0); • Other colors can be created by using different combinations of the three numbers 24
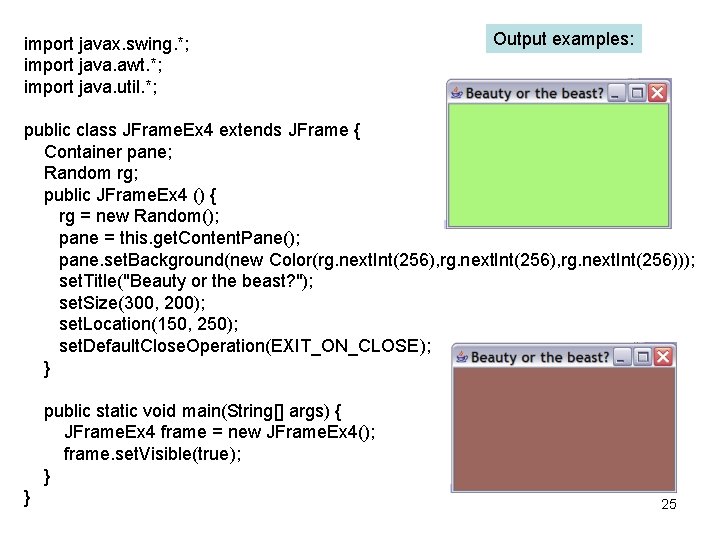
import javax. swing. *; import java. awt. *; import java. util. *; Output examples: public class JFrame. Ex 4 extends JFrame { Container pane; Random rg; public JFrame. Ex 4 () { rg = new Random(); pane = this. get. Content. Pane(); pane. set. Background(new Color(rg. next. Int(256), rg. next. Int(256))); set. Title("Beauty or the beast? "); set. Size(300, 200); set. Location(150, 250); set. Default. Close. Operation(EXIT_ON_CLOSE); } public static void main(String[] args) { JFrame. Ex 4 frame = new JFrame. Ex 4(); frame. set. Visible(true); } } 25
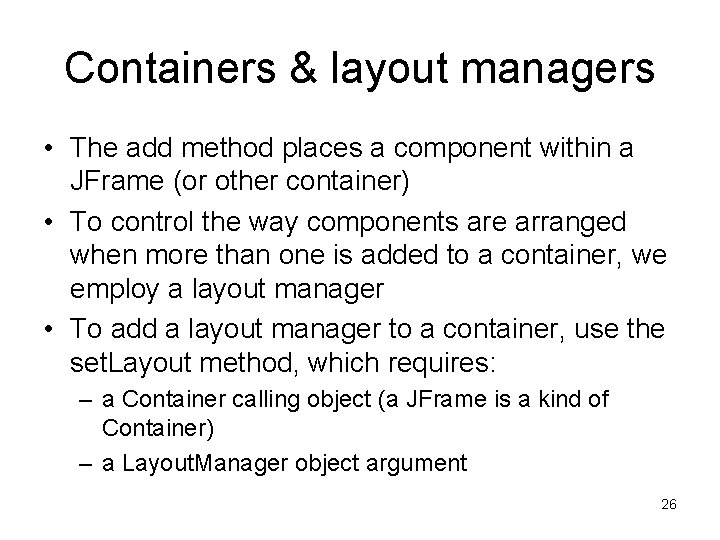
Containers & layout managers • The add method places a component within a JFrame (or other container) • To control the way components are arranged when more than one is added to a container, we employ a layout manager • To add a layout manager to a container, use the set. Layout method, which requires: – a Container calling object (a JFrame is a kind of Container) – a Layout. Manager object argument 26
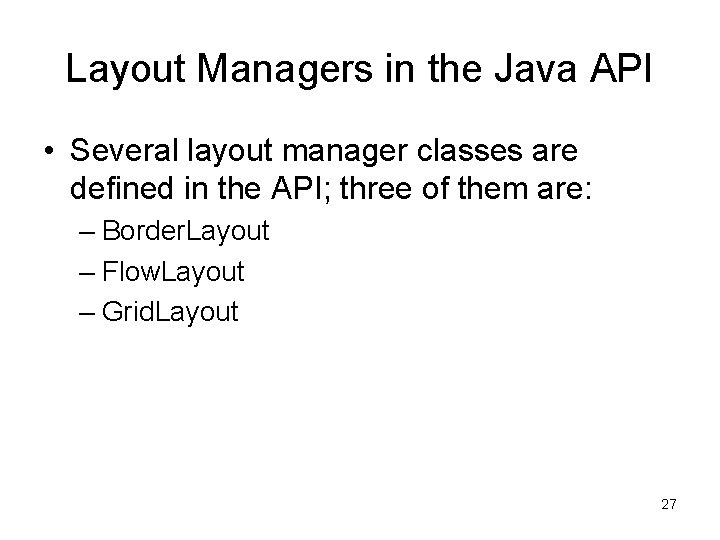
Layout Managers in the Java API • Several layout manager classes are defined in the API; three of them are: – Border. Layout – Flow. Layout – Grid. Layout 27
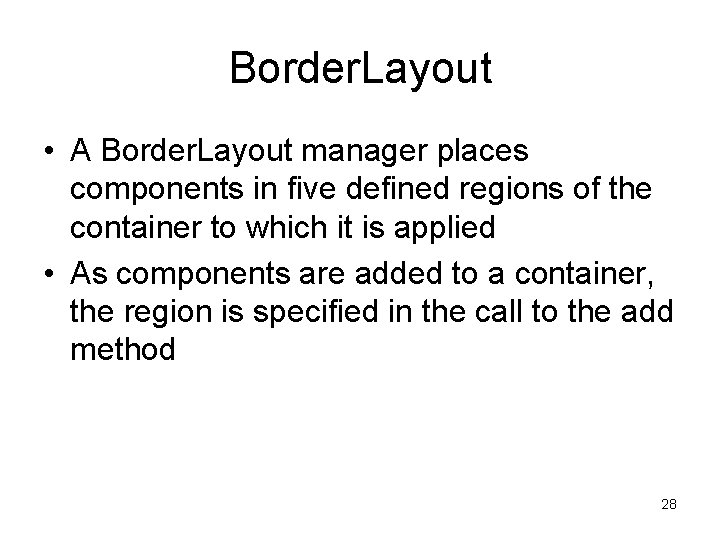
Border. Layout • A Border. Layout manager places components in five defined regions of the container to which it is applied • As components are added to a container, the region is specified in the call to the add method 28
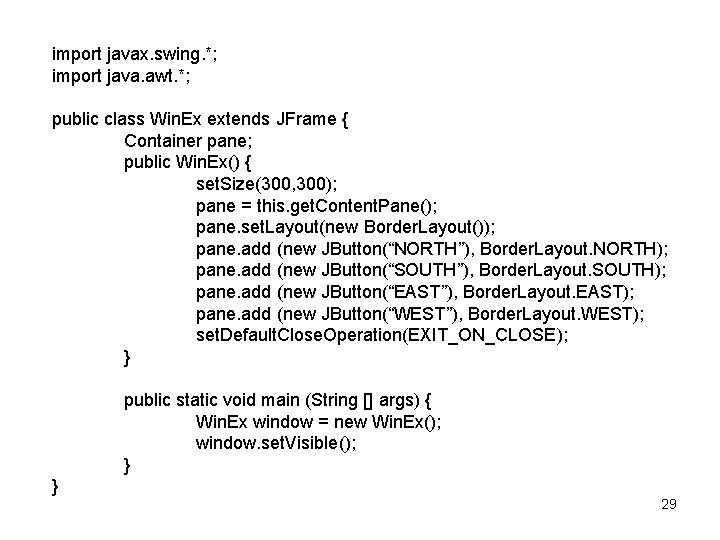
import javax. swing. *; import java. awt. *; public class Win. Ex extends JFrame { Container pane; public Win. Ex() { set. Size(300, 300); pane = this. get. Content. Pane(); pane. set. Layout(new Border. Layout()); pane. add (new JButton(“NORTH”), Border. Layout. NORTH); pane. add (new JButton(“SOUTH”), Border. Layout. SOUTH); pane. add (new JButton(“EAST”), Border. Layout. EAST); pane. add (new JButton(“WEST”), Border. Layout. WEST); set. Default. Close. Operation(EXIT_ON_CLOSE); } public static void main (String [] args) { Win. Ex window = new Win. Ex(); window. set. Visible(); } } 29
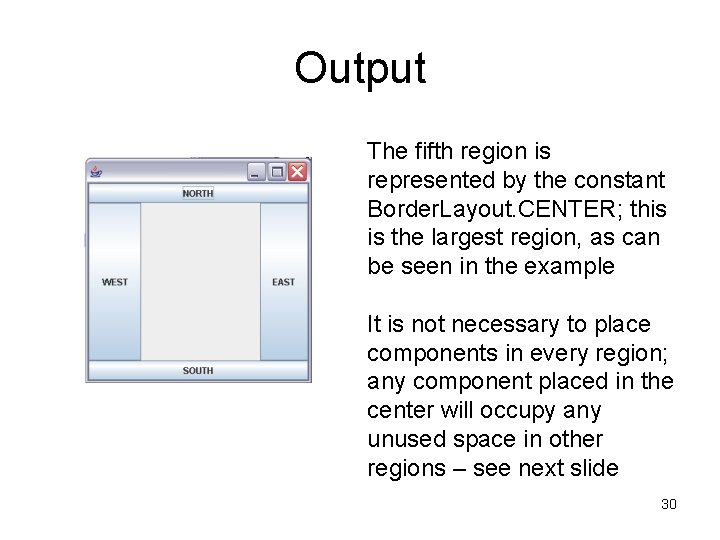
Output The fifth region is represented by the constant Border. Layout. CENTER; this is the largest region, as can be seen in the example It is not necessary to place components in every region; any component placed in the center will occupy any unused space in other regions – see next slide 30
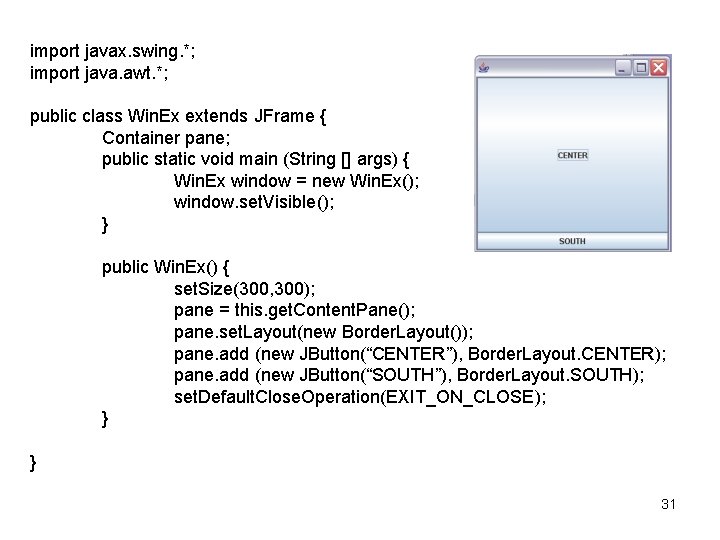
import javax. swing. *; import java. awt. *; public class Win. Ex extends JFrame { Container pane; public static void main (String [] args) { Win. Ex window = new Win. Ex(); window. set. Visible(); } public Win. Ex() { set. Size(300, 300); pane = this. get. Content. Pane(); pane. set. Layout(new Border. Layout()); pane. add (new JButton(“CENTER”), Border. Layout. CENTER); pane. add (new JButton(“SOUTH”), Border. Layout. SOUTH); set. Default. Close. Operation(EXIT_ON_CLOSE); } } 31
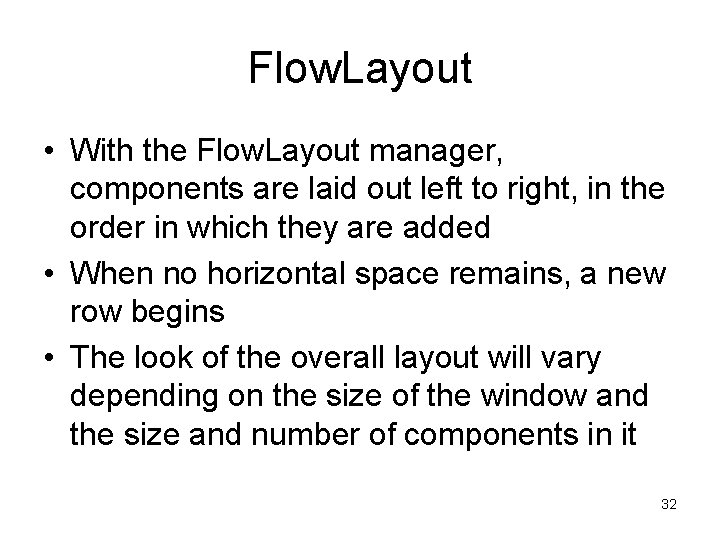
Flow. Layout • With the Flow. Layout manager, components are laid out left to right, in the order in which they are added • When no horizontal space remains, a new row begins • The look of the overall layout will vary depending on the size of the window and the size and number of components in it 32
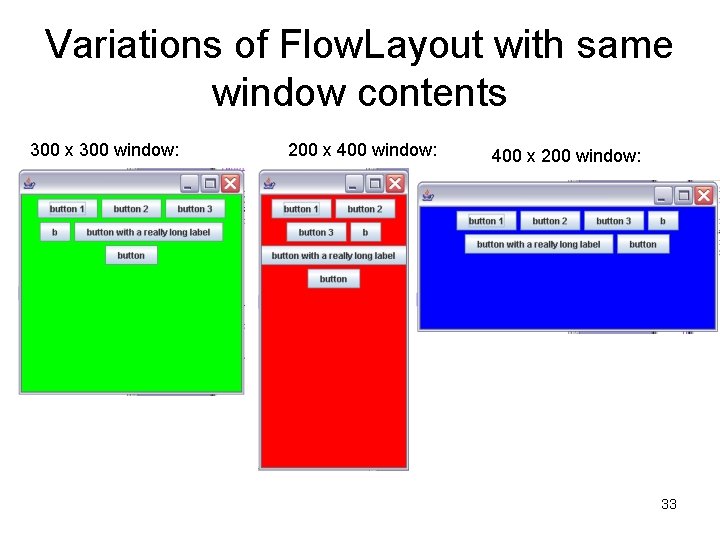
Variations of Flow. Layout with same window contents 300 x 300 window: 200 x 400 window: 400 x 200 window: 33
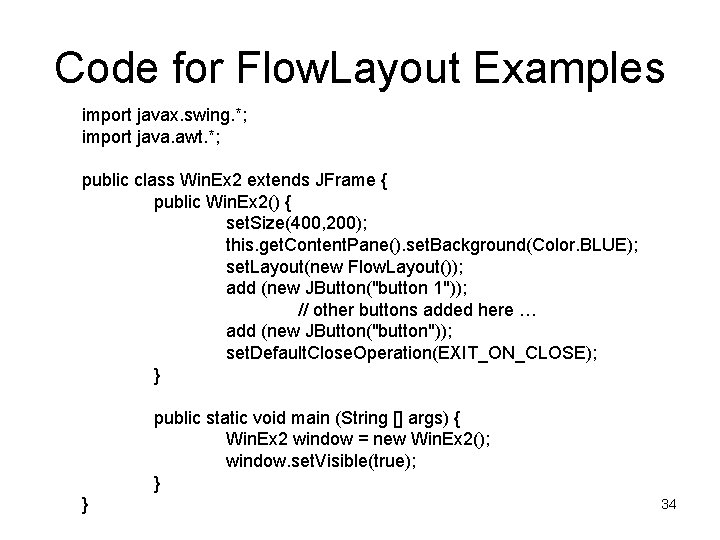
Code for Flow. Layout Examples import javax. swing. *; import java. awt. *; public class Win. Ex 2 extends JFrame { public Win. Ex 2() { set. Size(400, 200); this. get. Content. Pane(). set. Background(Color. BLUE); set. Layout(new Flow. Layout()); add (new JButton("button 1")); // other buttons added here … add (new JButton("button")); set. Default. Close. Operation(EXIT_ON_CLOSE); } public static void main (String [] args) { Win. Ex 2 window = new Win. Ex 2(); window. set. Visible(true); } } 34
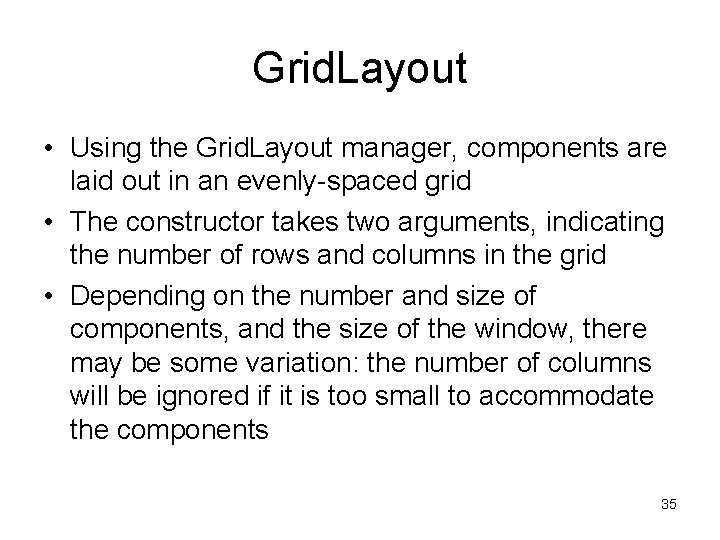
Grid. Layout • Using the Grid. Layout manager, components are laid out in an evenly-spaced grid • The constructor takes two arguments, indicating the number of rows and columns in the grid • Depending on the number and size of components, and the size of the window, there may be some variation: the number of columns will be ignored if it is too small to accommodate the components 35
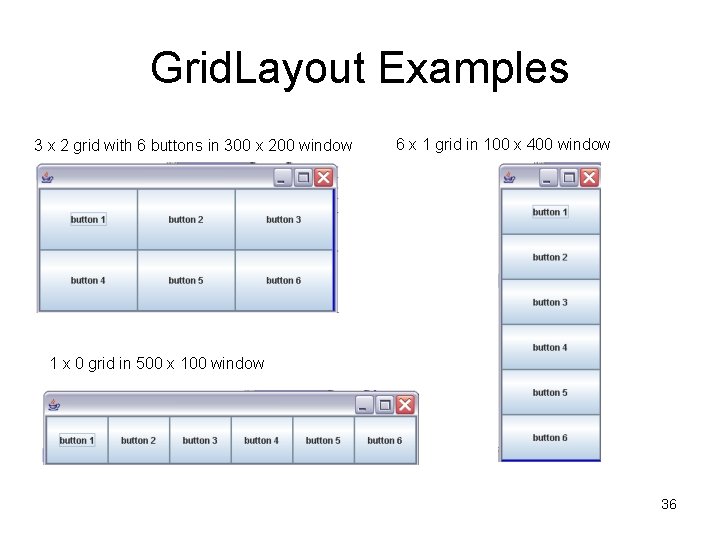
Grid. Layout Examples 3 x 2 grid with 6 buttons in 300 x 200 window 6 x 1 grid in 100 x 400 window 1 x 0 grid in 500 x 100 window 36
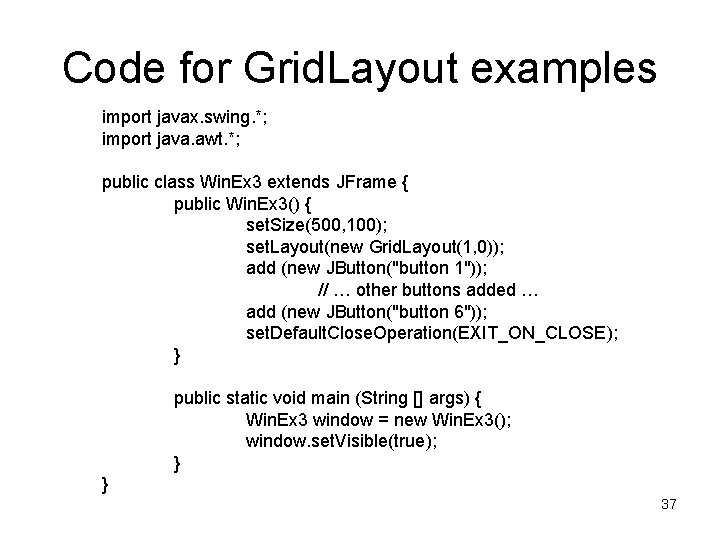
Code for Grid. Layout examples import javax. swing. *; import java. awt. *; public class Win. Ex 3 extends JFrame { public Win. Ex 3() { set. Size(500, 100); set. Layout(new Grid. Layout(1, 0)); add (new JButton("button 1")); // … other buttons added … add (new JButton("button 6")); set. Default. Close. Operation(EXIT_ON_CLOSE); } public static void main (String [] args) { Win. Ex 3 window = new Win. Ex 3(); window. set. Visible(true); } } 37
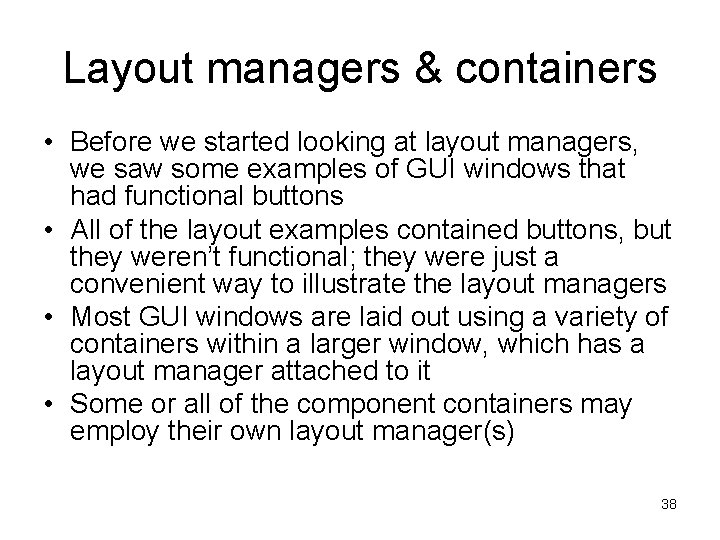
Layout managers & containers • Before we started looking at layout managers, we saw some examples of GUI windows that had functional buttons • All of the layout examples contained buttons, but they weren’t functional; they were just a convenient way to illustrate the layout managers • Most GUI windows are laid out using a variety of containers within a larger window, which has a layout manager attached to it • Some or all of the component containers may employ their own layout manager(s) 38
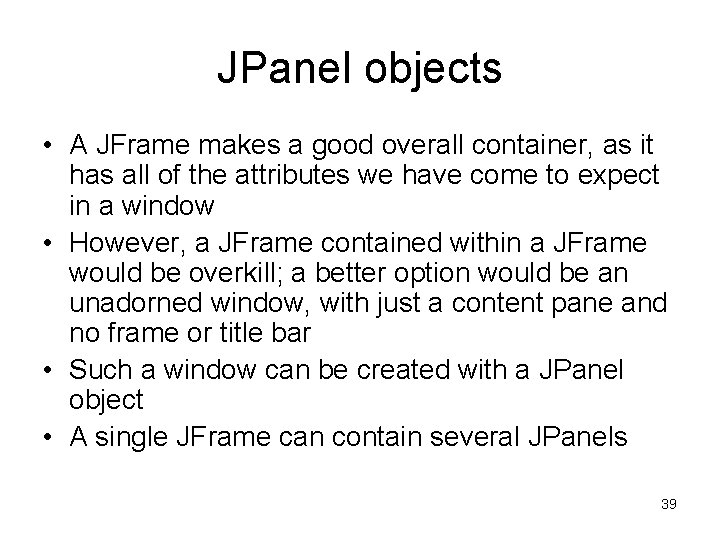
JPanel objects • A JFrame makes a good overall container, as it has all of the attributes we have come to expect in a window • However, a JFrame contained within a JFrame would be overkill; a better option would be an unadorned window, with just a content pane and no frame or title bar • Such a window can be created with a JPanel object • A single JFrame can contain several JPanels 39
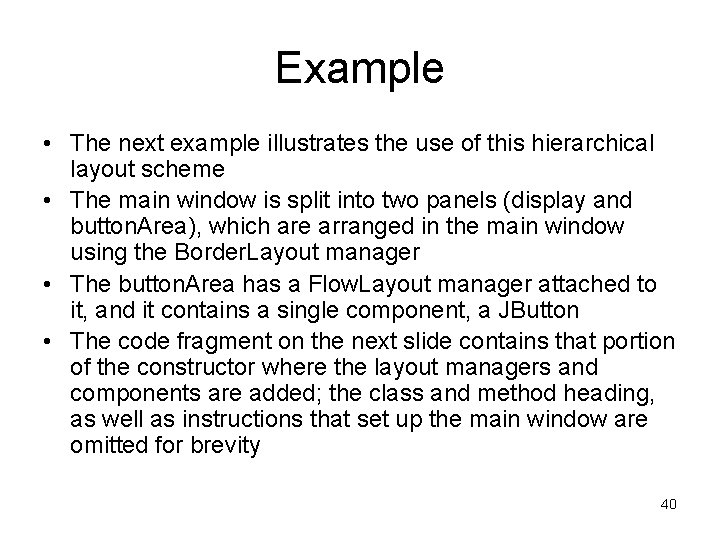
Example • The next example illustrates the use of this hierarchical layout scheme • The main window is split into two panels (display and button. Area), which are arranged in the main window using the Border. Layout manager • The button. Area has a Flow. Layout manager attached to it, and it contains a single component, a JButton • The code fragment on the next slide contains that portion of the constructor where the layout managers and components are added; the class and method heading, as well as instructions that set up the main window are omitted for brevity 40
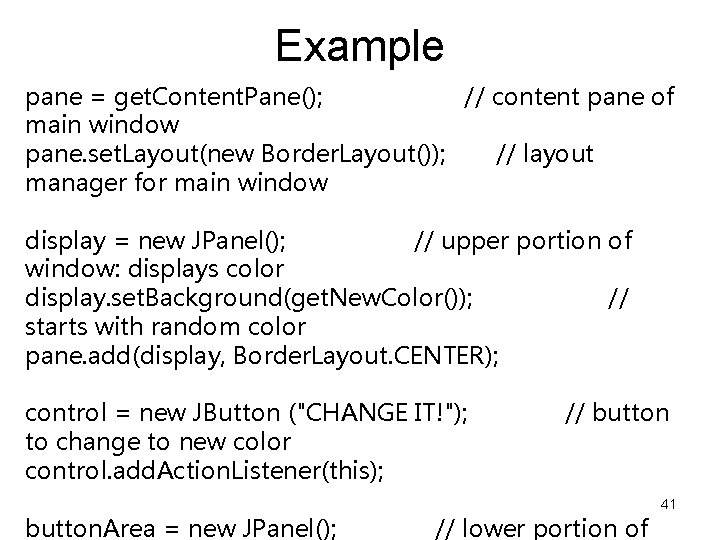
Example pane = get. Content. Pane(); // content pane of main window pane. set. Layout(new Border. Layout()); // layout manager for main window display = new JPanel(); // upper portion of window: displays color display. set. Background(get. New. Color()); // starts with random color pane. add(display, Border. Layout. CENTER); control = new JButton ("CHANGE IT!"); to change to new color control. add. Action. Listener(this); button. Area = new JPanel(); // button // lower portion of 41
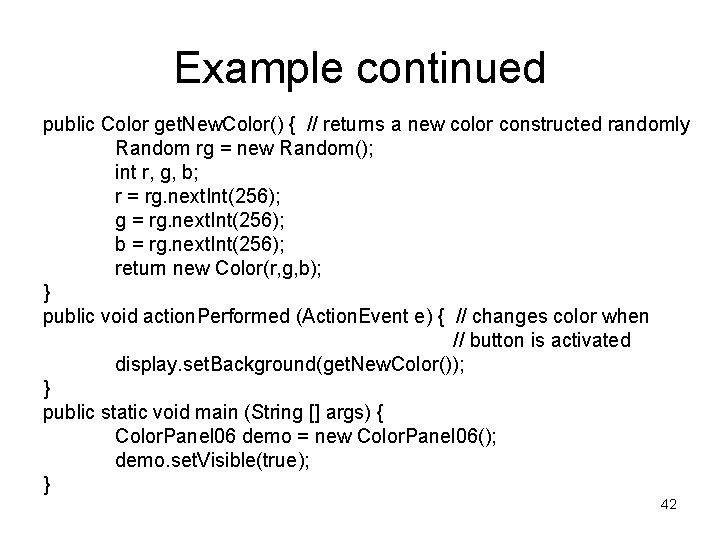
Example continued public Color get. New. Color() { // returns a new color constructed randomly Random rg = new Random(); int r, g, b; r = rg. next. Int(256); g = rg. next. Int(256); b = rg. next. Int(256); return new Color(r, g, b); } public void action. Performed (Action. Event e) { // changes color when // button is activated display. set. Background(get. New. Color()); } public static void main (String [] args) { Color. Panel 06 demo = new Color. Panel 06(); demo. set. Visible(true); } 42
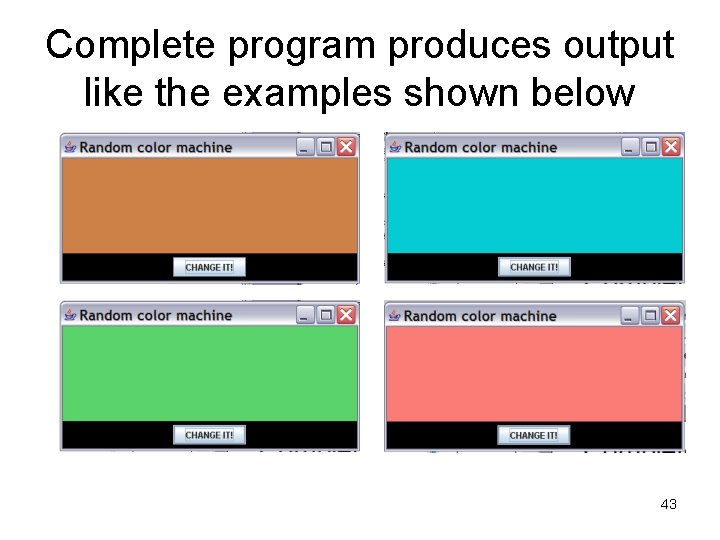
Complete program produces output like the examples shown below 43
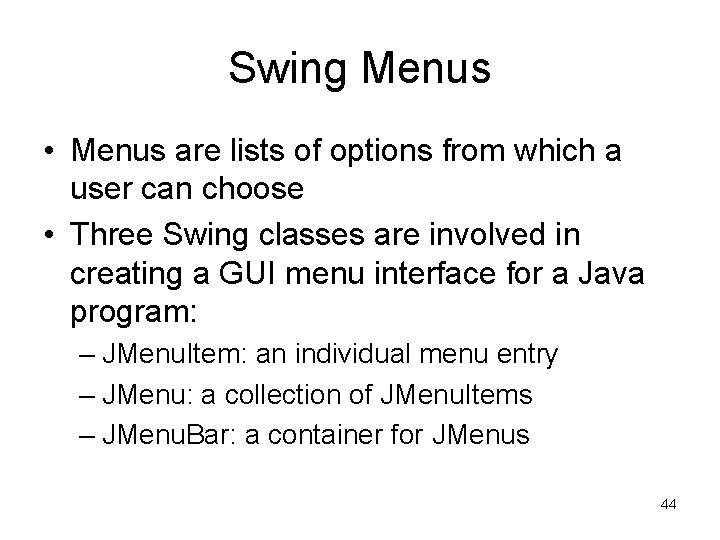
Swing Menus • Menus are lists of options from which a user can choose • Three Swing classes are involved in creating a GUI menu interface for a Java program: – JMenu. Item: an individual menu entry – JMenu: a collection of JMenu. Items – JMenu. Bar: a container for JMenus 44
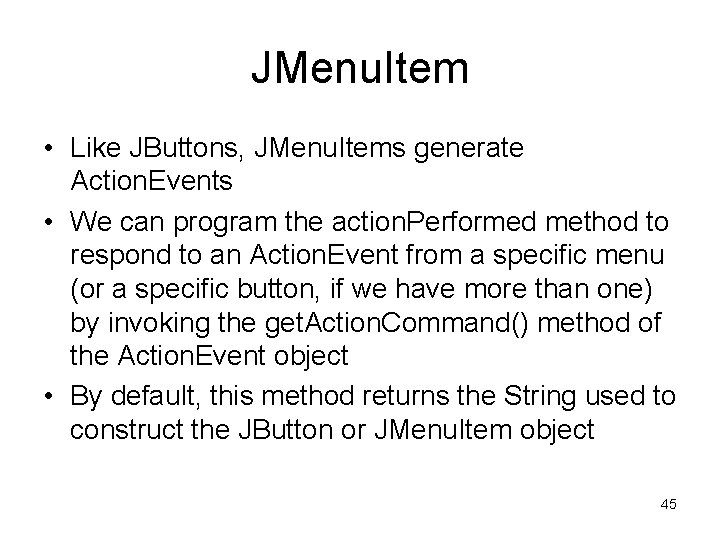
JMenu. Item • Like JButtons, JMenu. Items generate Action. Events • We can program the action. Performed method to respond to an Action. Event from a specific menu (or a specific button, if we have more than one) by invoking the get. Action. Command() method of the Action. Event object • By default, this method returns the String used to construct the JButton or JMenu. Item object 45
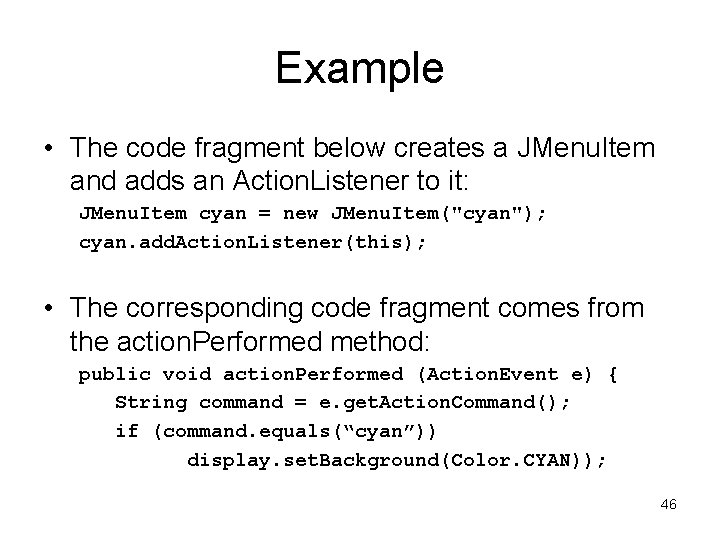
Example • The code fragment below creates a JMenu. Item and adds an Action. Listener to it: JMenu. Item cyan = new JMenu. Item("cyan"); cyan. add. Action. Listener(this); • The corresponding code fragment comes from the action. Performed method: public void action. Performed (Action. Event e) { String command = e. get. Action. Command(); if (command. equals(“cyan”)) display. set. Background(Color. CYAN)); 46
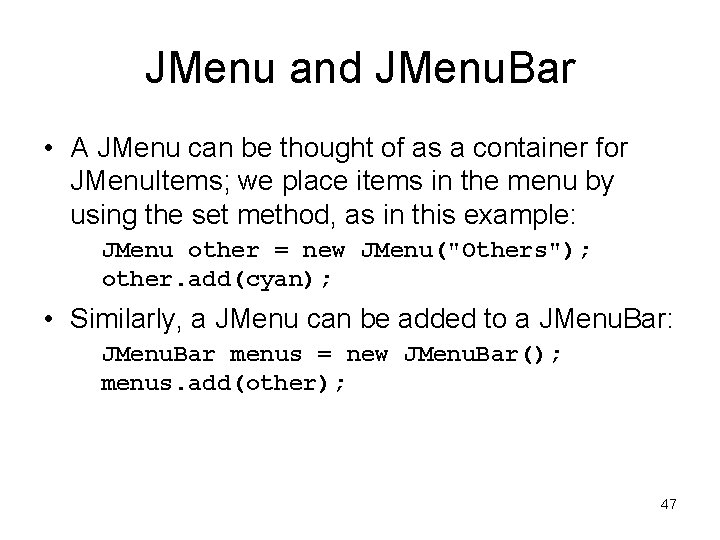
JMenu and JMenu. Bar • A JMenu can be thought of as a container for JMenu. Items; we place items in the menu by using the set method, as in this example: JMenu other = new JMenu("Others"); other. add(cyan); • Similarly, a JMenu can be added to a JMenu. Bar: JMenu. Bar menus = new JMenu. Bar(); menus. add(other); 47
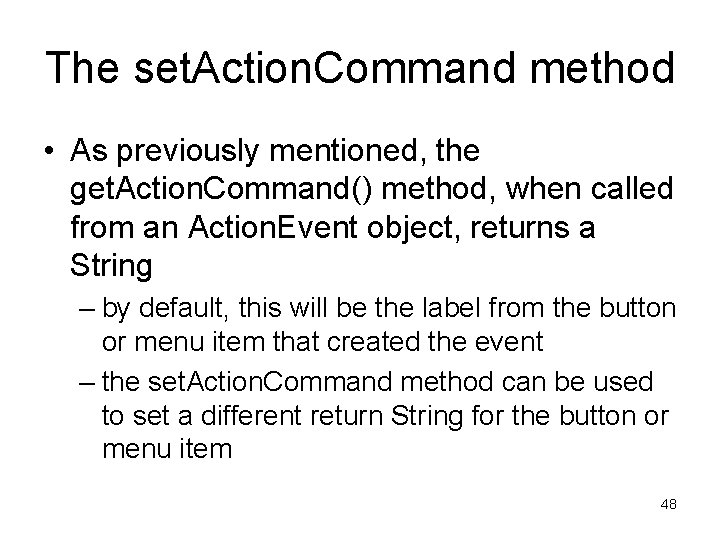
The set. Action. Command method • As previously mentioned, the get. Action. Command() method, when called from an Action. Event object, returns a String – by default, this will be the label from the button or menu item that created the event – the set. Action. Command method can be used to set a different return String for the button or menu item 48
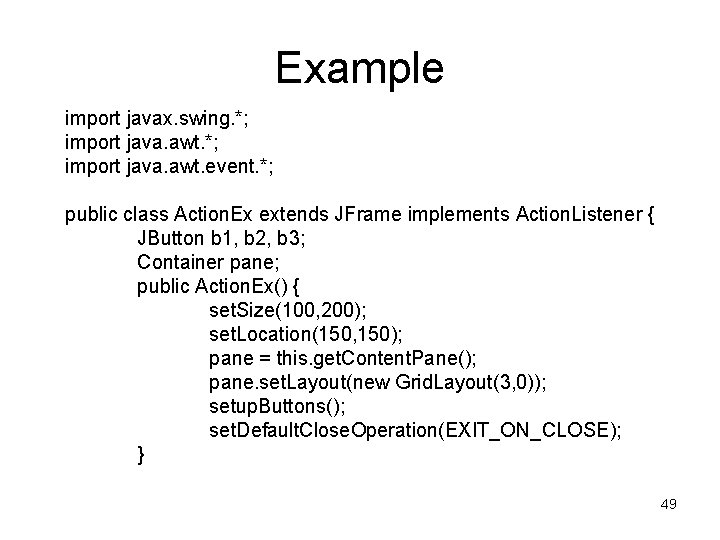
Example import javax. swing. *; import java. awt. event. *; public class Action. Ex extends JFrame implements Action. Listener { JButton b 1, b 2, b 3; Container pane; public Action. Ex() { set. Size(100, 200); set. Location(150, 150); pane = this. get. Content. Pane(); pane. set. Layout(new Grid. Layout(3, 0)); setup. Buttons(); set. Default. Close. Operation(EXIT_ON_CLOSE); } 49
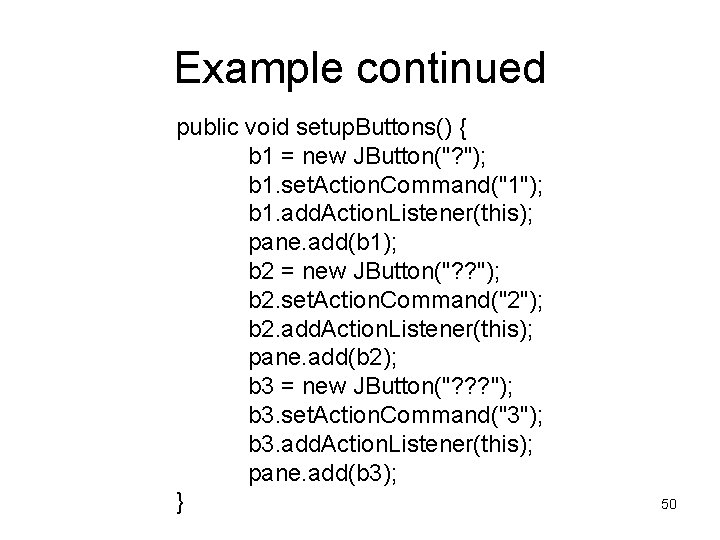
Example continued public void setup. Buttons() { b 1 = new JButton("? "); b 1. set. Action. Command("1"); b 1. add. Action. Listener(this); pane. add(b 1); b 2 = new JButton("? ? "); b 2. set. Action. Command("2"); b 2. add. Action. Listener(this); pane. add(b 2); b 3 = new JButton("? ? ? "); b 3. set. Action. Command("3"); b 3. add. Action. Listener(this); pane. add(b 3); } 50
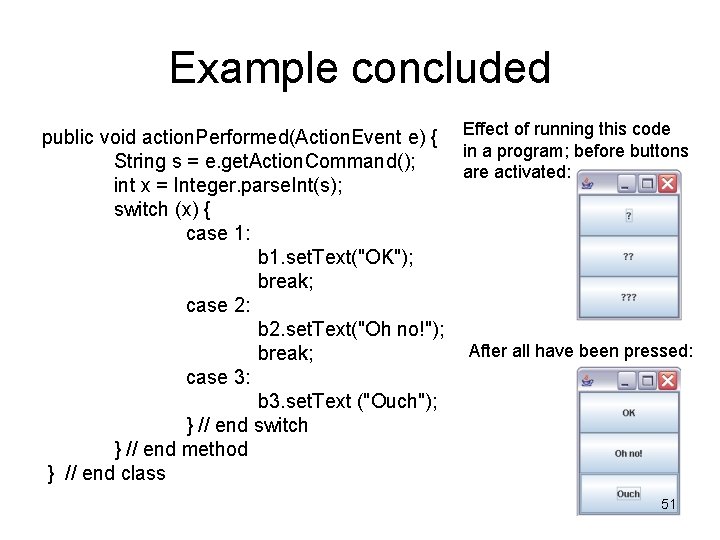
Example concluded public void action. Performed(Action. Event e) { Effect of running this code in a program; before buttons String s = e. get. Action. Command(); are activated: int x = Integer. parse. Int(s); switch (x) { case 1: b 1. set. Text("OK"); break; case 2: b 2. set. Text("Oh no!"); After all have been pressed: break; case 3: b 3. set. Text ("Ouch"); } // end switch } // end method } // end class 51
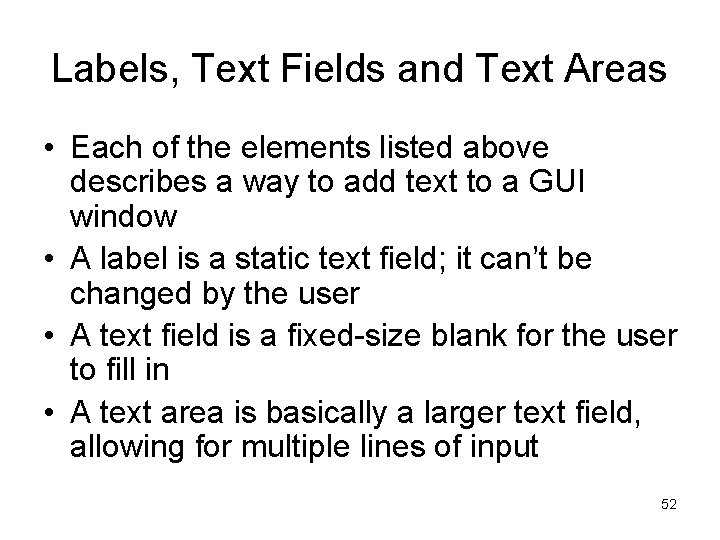
Labels, Text Fields and Text Areas • Each of the elements listed above describes a way to add text to a GUI window • A label is a static text field; it can’t be changed by the user • A text field is a fixed-size blank for the user to fill in • A text area is basically a larger text field, allowing for multiple lines of input 52
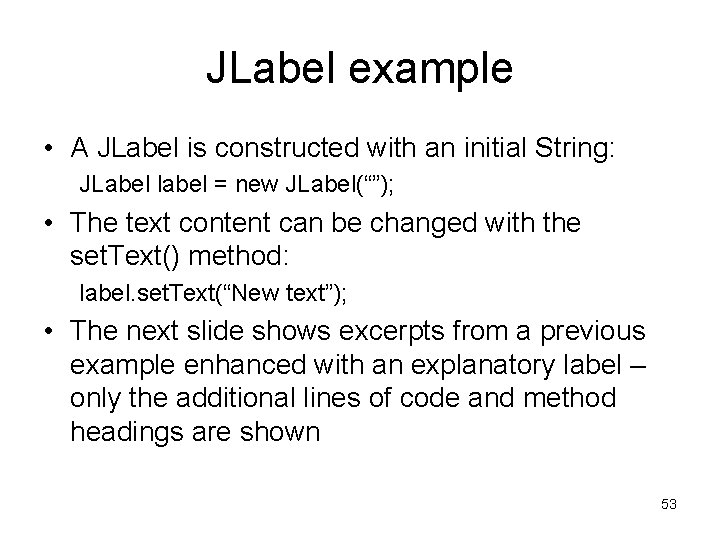
JLabel example • A JLabel is constructed with an initial String: JLabel label = new JLabel(“”); • The text content can be changed with the set. Text() method: label. set. Text(“New text”); • The next slide shows excerpts from a previous example enhanced with an explanatory label – only the additional lines of code and method headings are shown 53
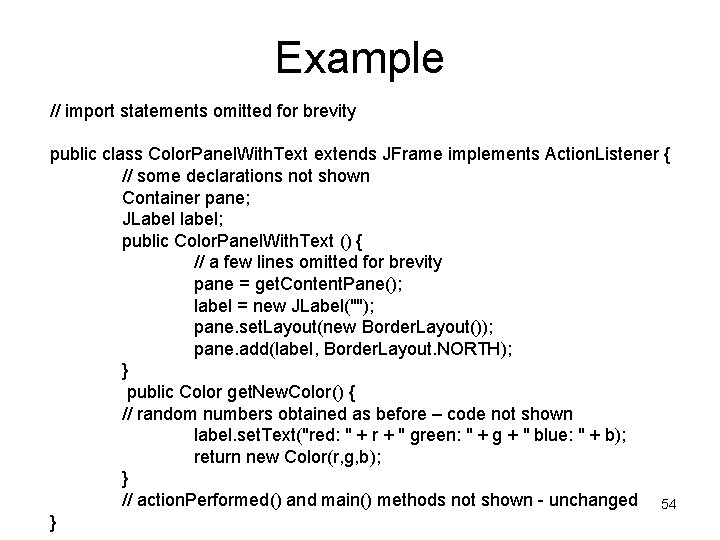
Example // import statements omitted for brevity public class Color. Panel. With. Text extends JFrame implements Action. Listener { // some declarations not shown Container pane; JLabel label; public Color. Panel. With. Text () { // a few lines omitted for brevity pane = get. Content. Pane(); label = new JLabel(""); pane. set. Layout(new Border. Layout()); pane. add(label, Border. Layout. NORTH); } public Color get. New. Color() { // random numbers obtained as before – code not shown label. set. Text("red: " + r + " green: " + g + " blue: " + b); return new Color(r, g, b); } // action. Performed() and main() methods not shown - unchanged 54 }
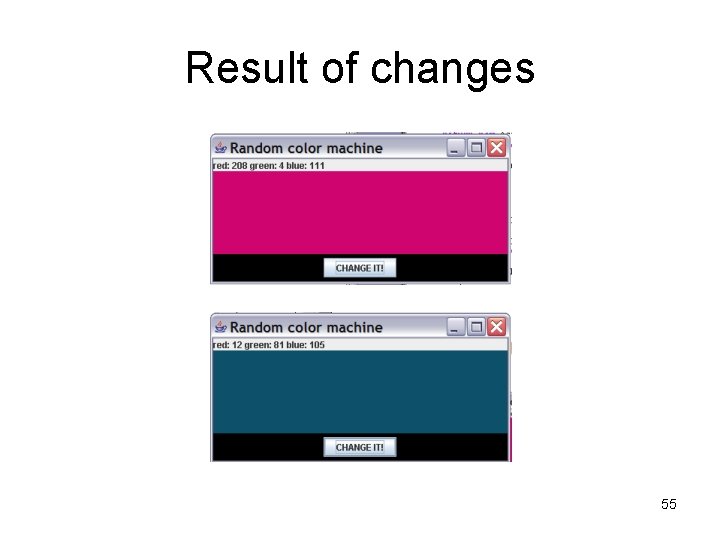
Result of changes 55
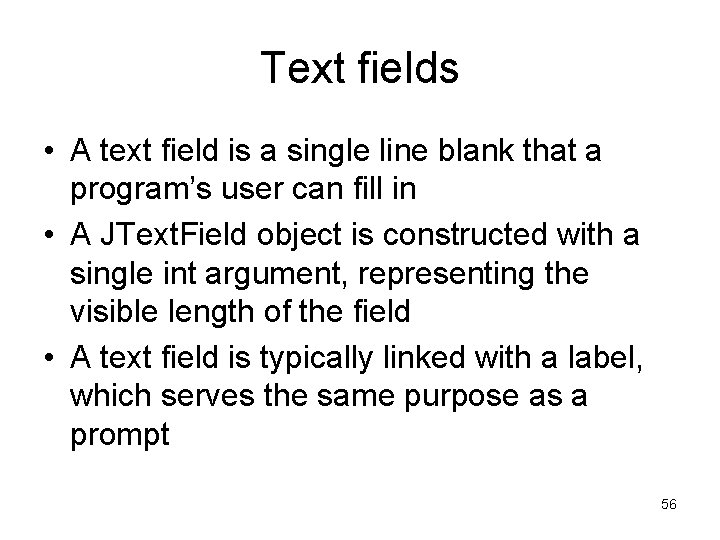
Text fields • A text field is a single line blank that a program’s user can fill in • A JText. Field object is constructed with a single int argument, representing the visible length of the field • A text field is typically linked with a label, which serves the same purpose as a prompt 56
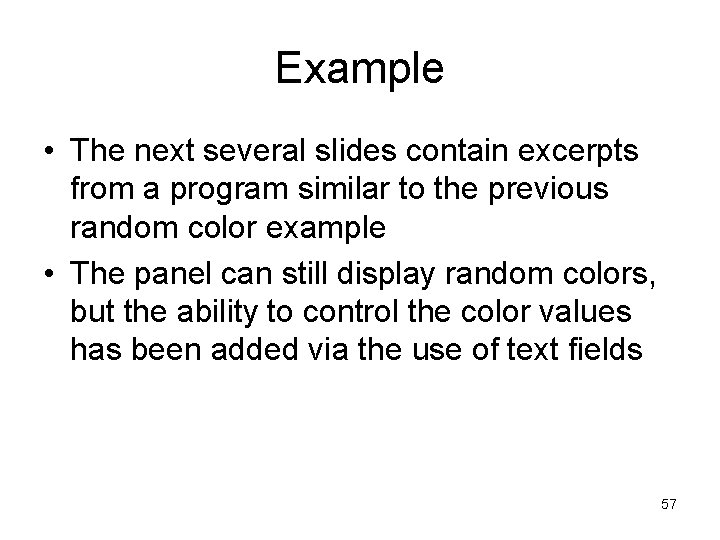
Example • The next several slides contain excerpts from a program similar to the previous random color example • The panel can still display random colors, but the ability to control the color values has been added via the use of text fields 57
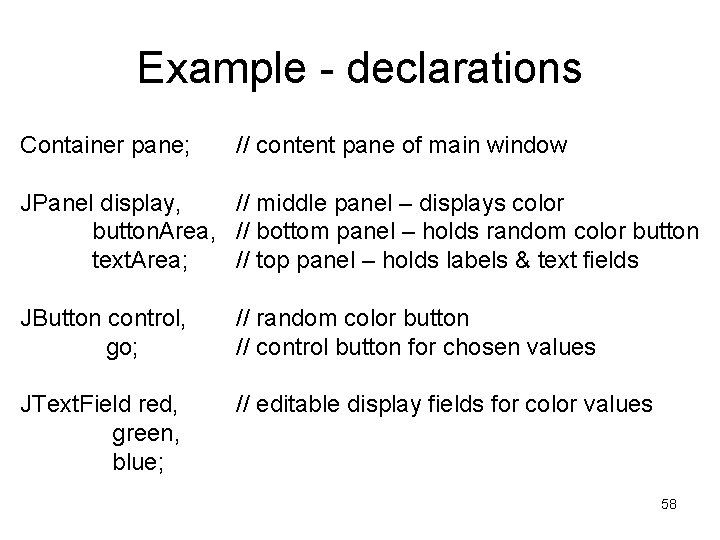
Example - declarations Container pane; // content pane of main window JPanel display, // middle panel – displays color button. Area, // bottom panel – holds random color button text. Area; // top panel – holds labels & text fields JButton control, go; // random color button // control button for chosen values JText. Field red, green, blue; // editable display fields for color values 58
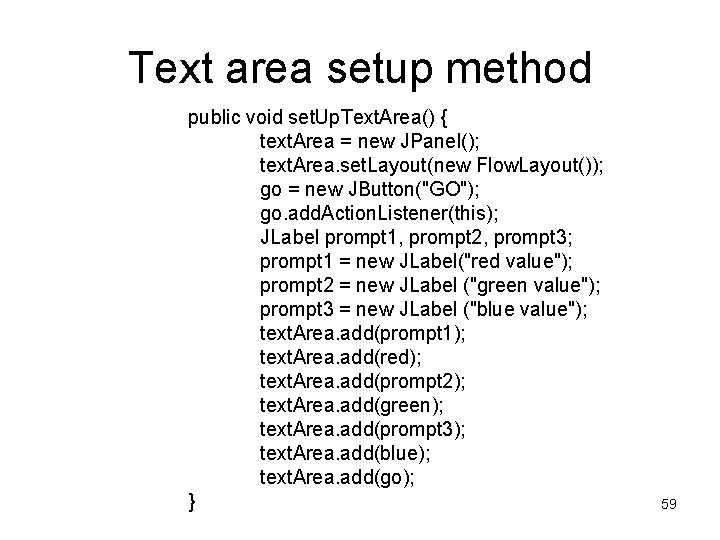
Text area setup method public void set. Up. Text. Area() { text. Area = new JPanel(); text. Area. set. Layout(new Flow. Layout()); go = new JButton("GO"); go. add. Action. Listener(this); JLabel prompt 1, prompt 2, prompt 3; prompt 1 = new JLabel("red value"); prompt 2 = new JLabel ("green value"); prompt 3 = new JLabel ("blue value"); text. Area. add(prompt 1); text. Area. add(red); text. Area. add(prompt 2); text. Area. add(green); text. Area. add(prompt 3); text. Area. add(blue); text. Area. add(go); } 59
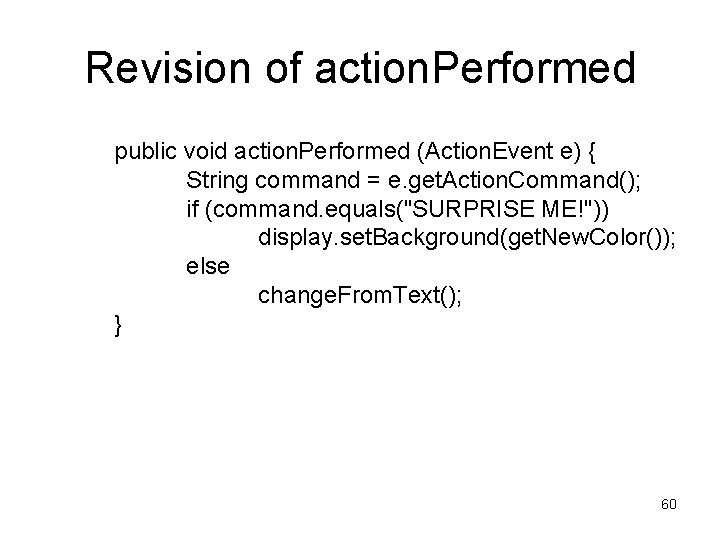
Revision of action. Performed public void action. Performed (Action. Event e) { String command = e. get. Action. Command(); if (command. equals("SURPRISE ME!")) display. set. Background(get. New. Color()); else change. From. Text(); } 60
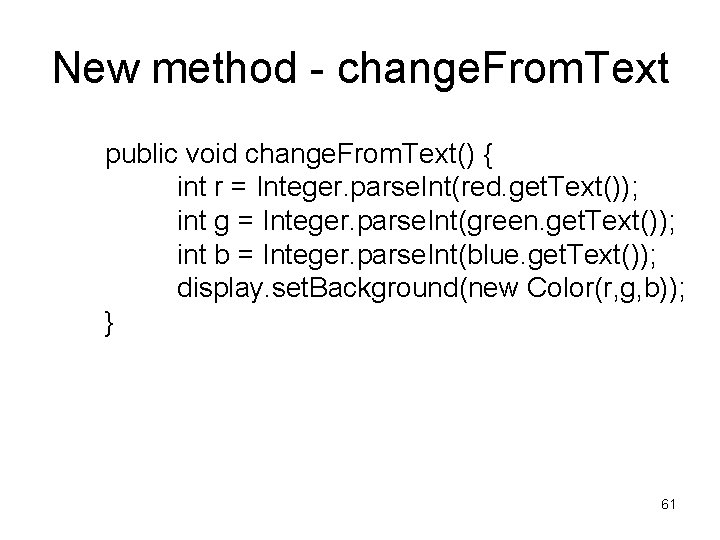
New method - change. From. Text public void change. From. Text() { int r = Integer. parse. Int(red. get. Text()); int g = Integer. parse. Int(green. get. Text()); int b = Integer. parse. Int(blue. get. Text()); display. set. Background(new Color(r, g, b)); } 61
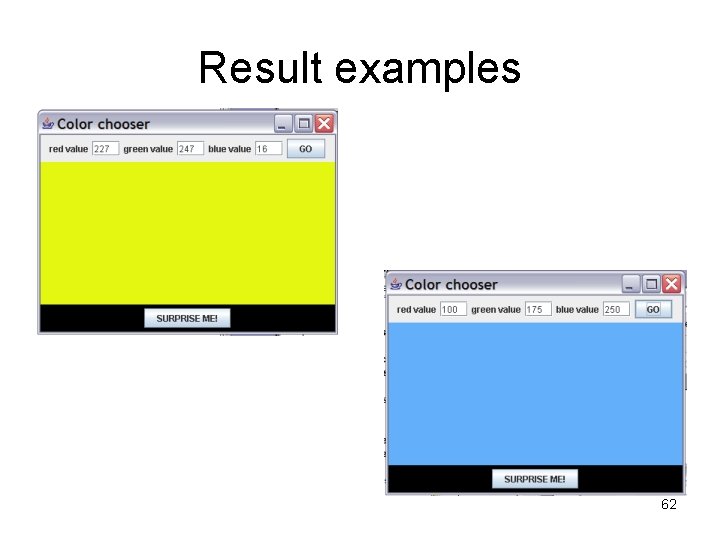
Result examples 62
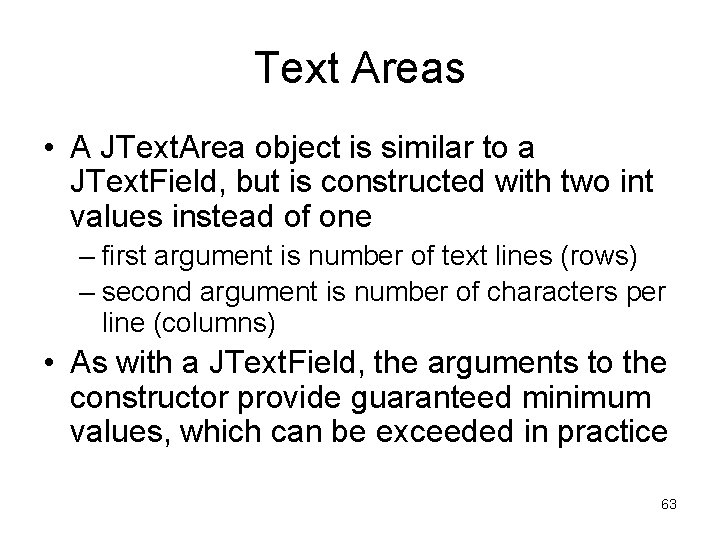
Text Areas • A JText. Area object is similar to a JText. Field, but is constructed with two int values instead of one – first argument is number of text lines (rows) – second argument is number of characters per line (columns) • As with a JText. Field, the arguments to the constructor provide guaranteed minimum values, which can be exceeded in practice 63
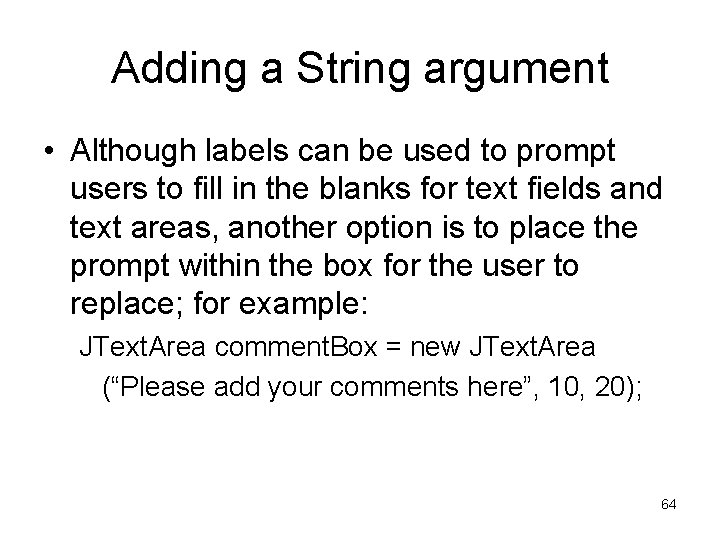
Adding a String argument • Although labels can be used to prompt users to fill in the blanks for text fields and text areas, another option is to place the prompt within the box for the user to replace; for example: JText. Area comment. Box = new JText. Area (“Please add your comments here”, 10, 20); 64
Ge gi gue gui güe güi
Common gui event types and listener interfaces in java
Mutually exclusive vs non mutually exclusive
Ruby gui toolkit
Linux gui development tools
Rapid gui programming with python and qt
Mouse events in windows programming
Perbedaan linear programming dan integer programming
Greedy vs dynamic programming
What is system programing
Integer programming vs linear programming
Programing adalah
Part programming
Linux kernel programming part 2
Addition symbol
Part to part ratio definition
Part part whole
Define technical description
Bar counter parts
The phase of the moon you see depends on ______.
Minitab adalah
Thematic paragraph example
Introduction victor hugo les contemplations
Types of hooks for persuasive essays
Where is the hook located in the introduction paragraph
Introduction to comparative essay
Introduction body conclusion
Huckleberry finn intro
Intro to matter
Sports product advertisement
Venus omkrets
Introduction body conclusion example
Reverse engineering basics
Example of introduction title
Four parts of research paper
Organic chemistry chapter 1
Intro to offensive security
Utvecklingssamtal engelska
Materialgruppenmanagement
Lord of the flies intro
Leq intro paragraph
Exemple de paragraphe argumentatif
Introduction paragraph examples
Andrew ng introduction to machine learning
Intro to verilog
Var_dump in php
Ifs managers examples
Intro to hadoop
Example of introduction paragraph
Introduction
Kfc profile
Essay introduction body conclusion
Intro to vlsi
Intro to vectors
Orphan graphic design
Define stagecraft
Intropy
Adding and subtracting polynomials
Intro to mis
Dns in computer networks
Chapter 10 marketing answer key
Whats an intro paragraph
Funnel introduction paragraph
Random forest intro
How to start an introduction