List Stack Queue 202137 1 List It is
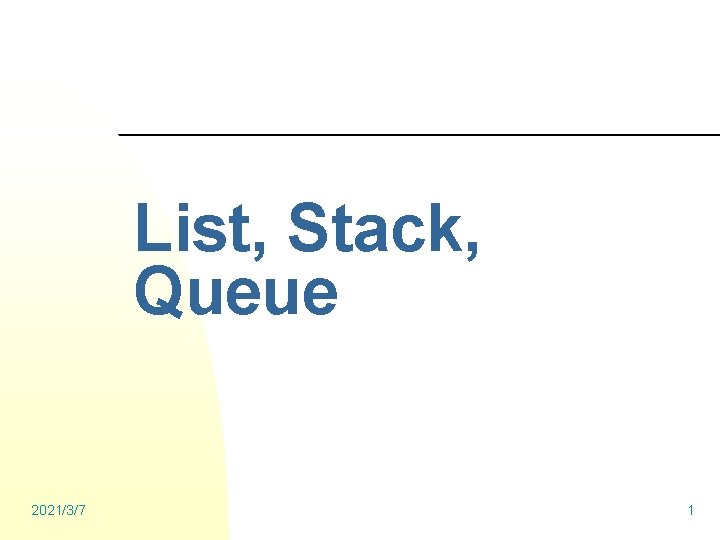
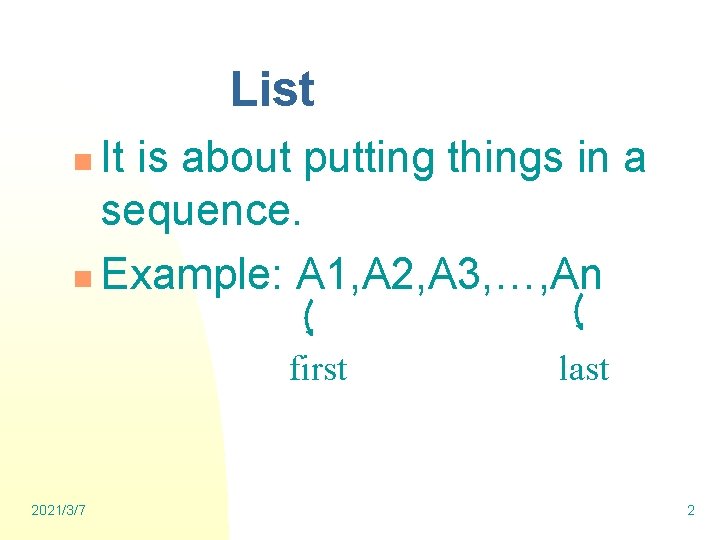
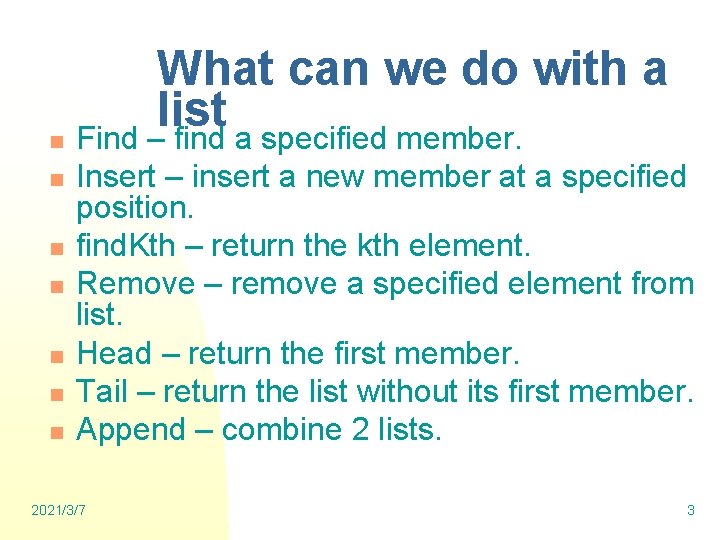
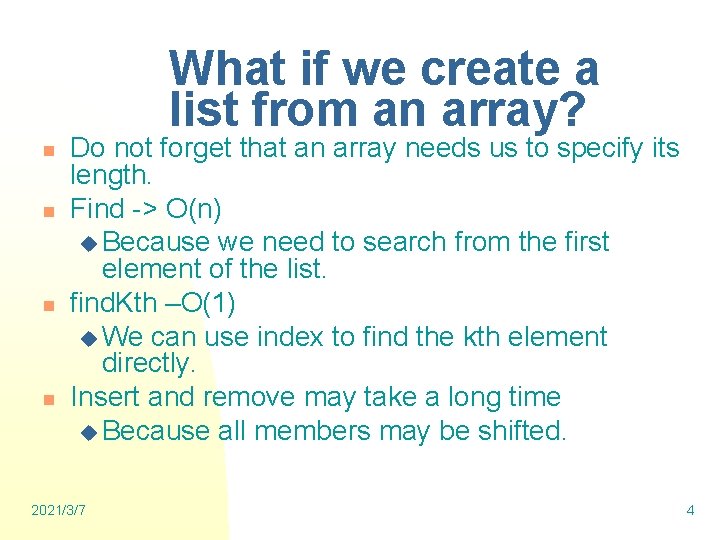
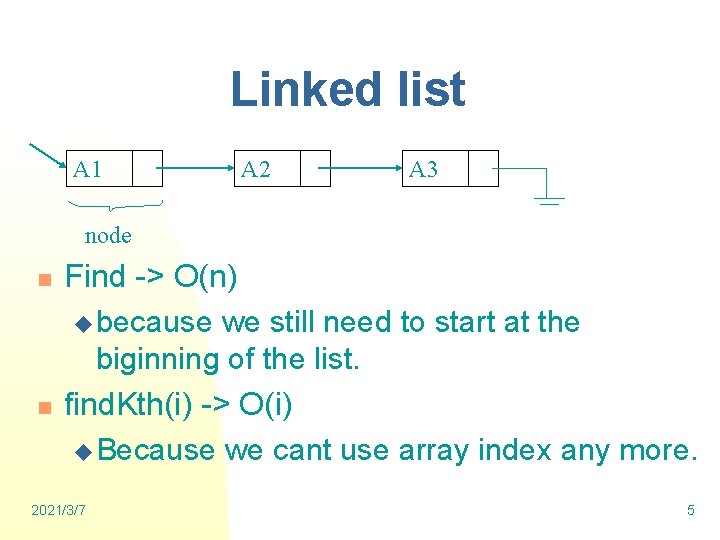
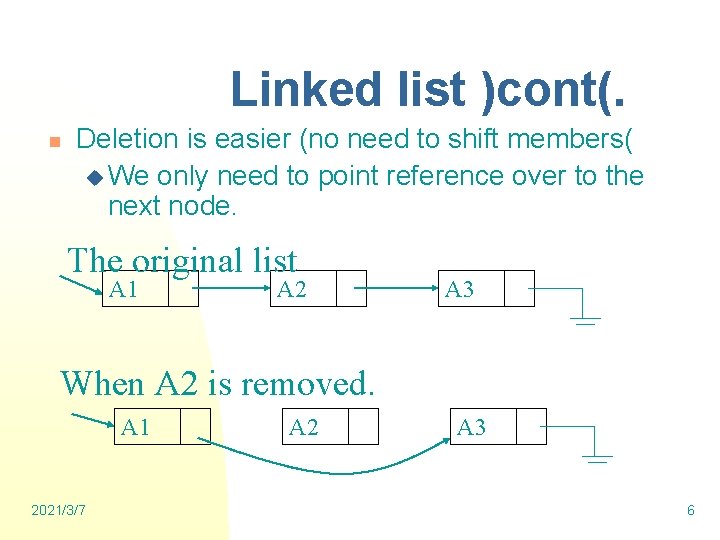
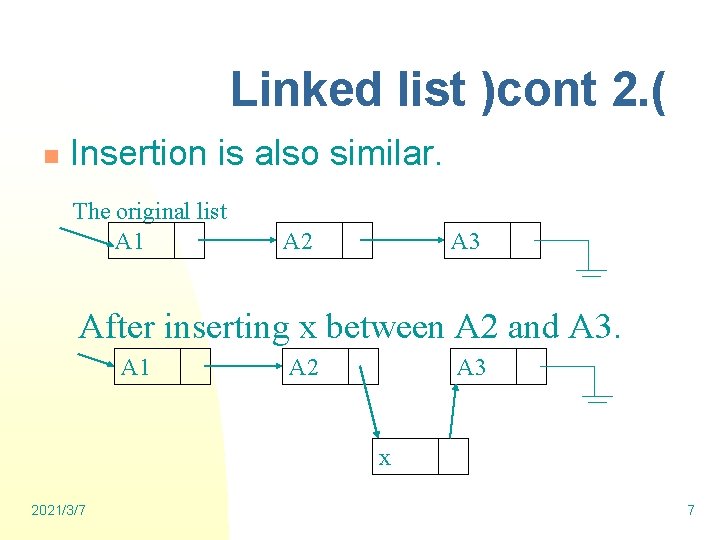
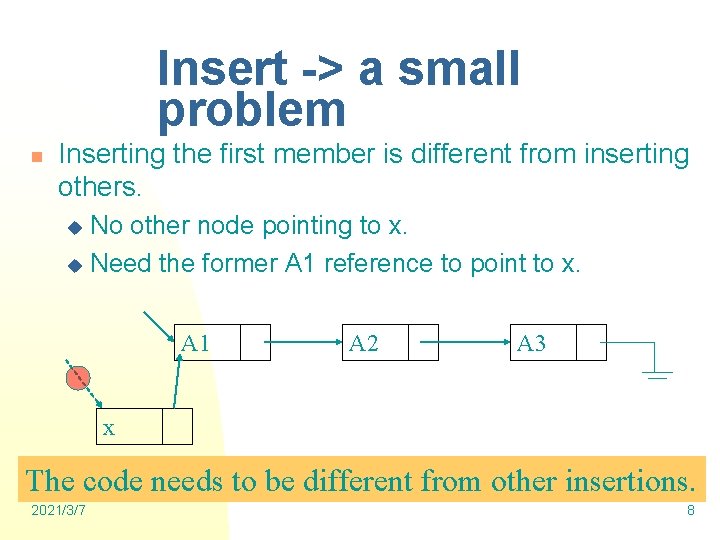
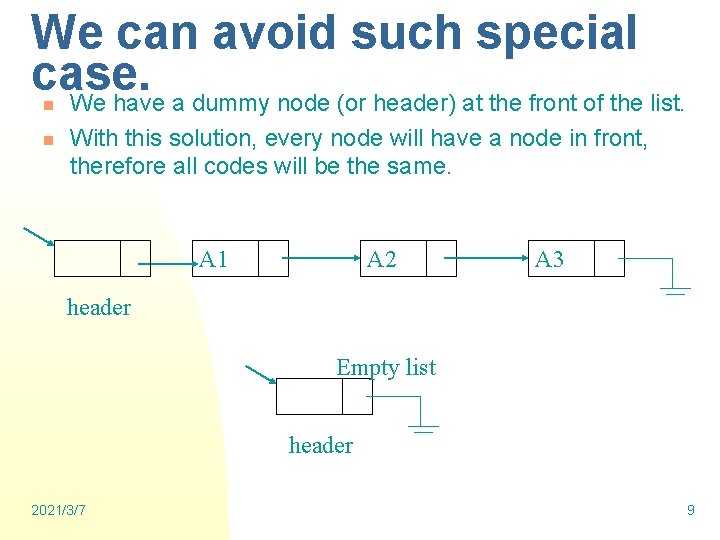
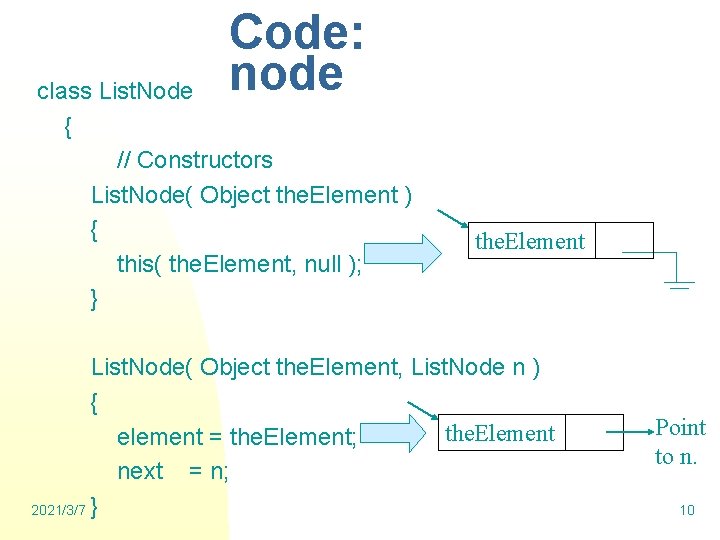
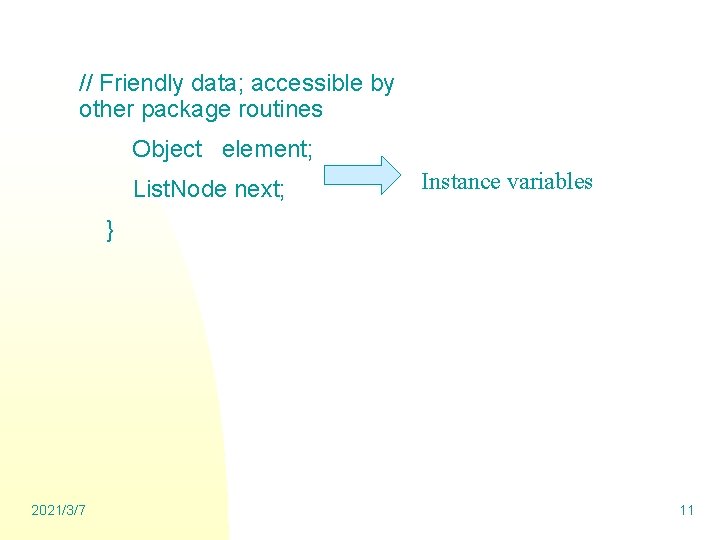
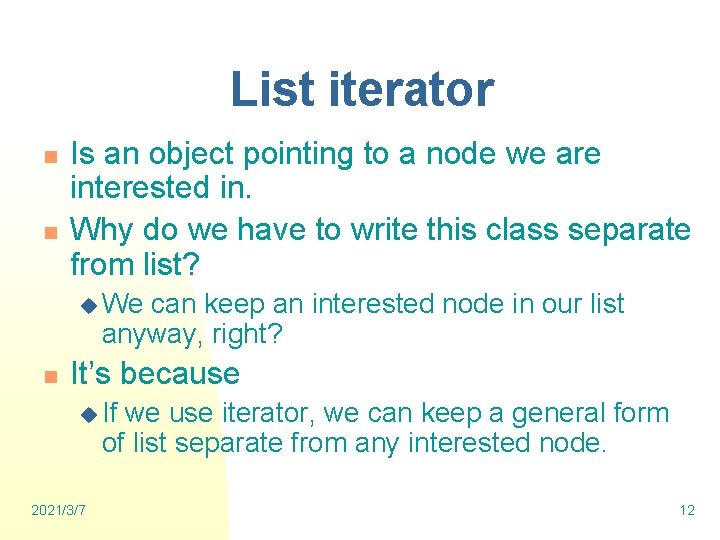
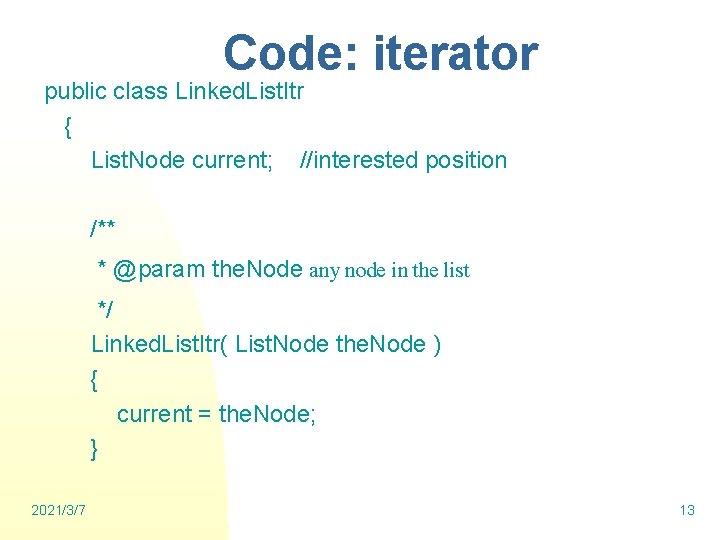
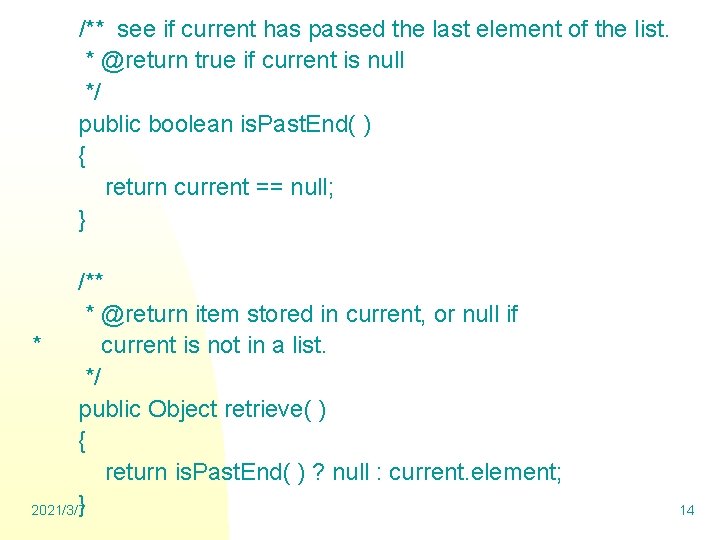
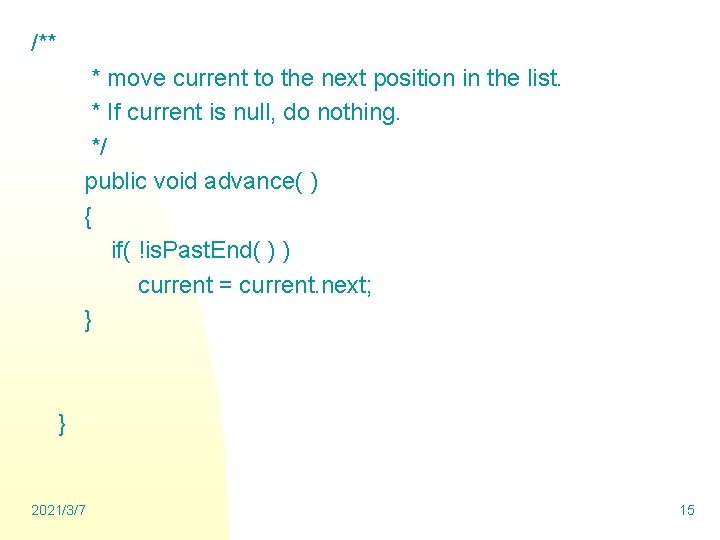
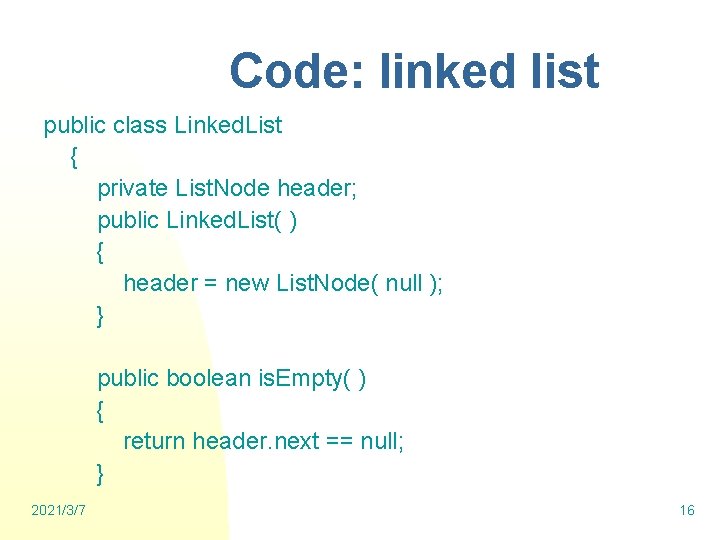
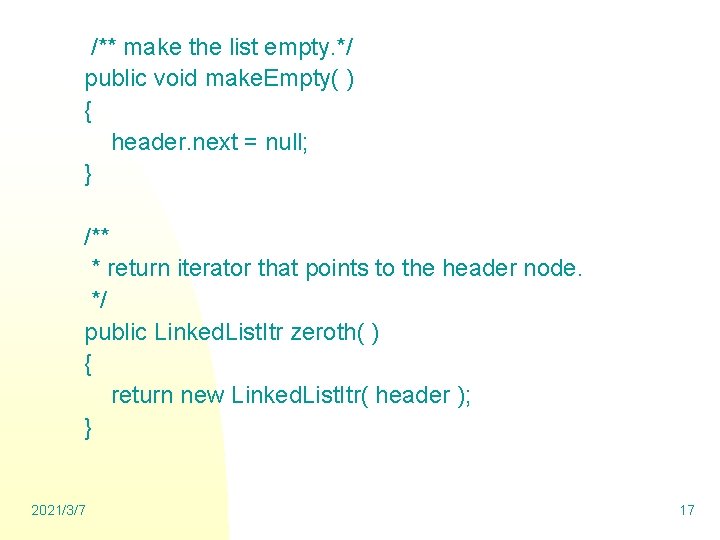
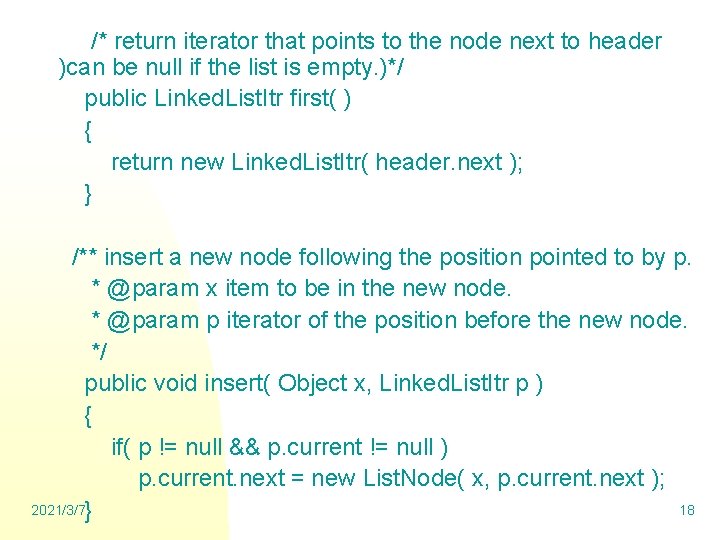
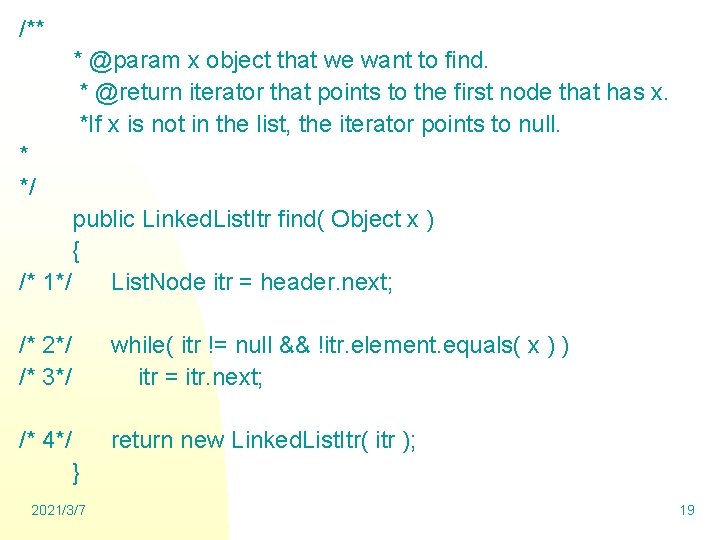
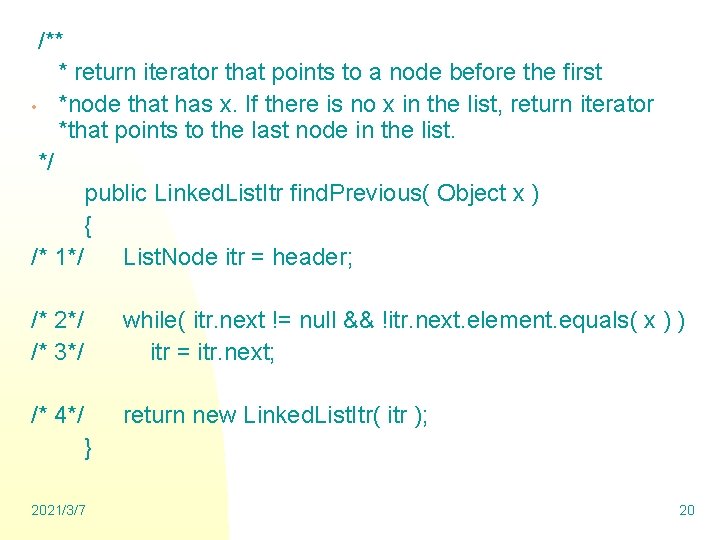
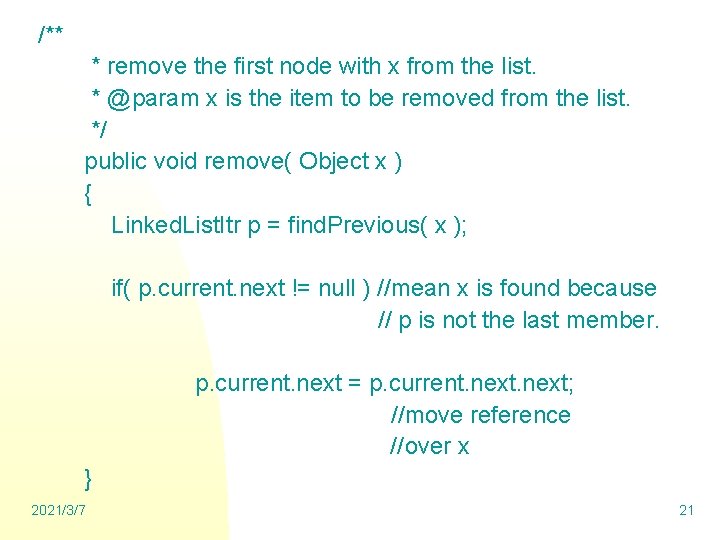
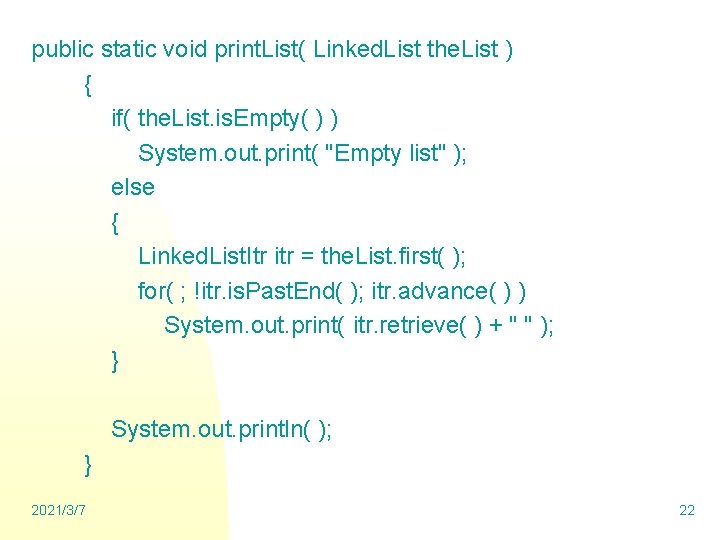
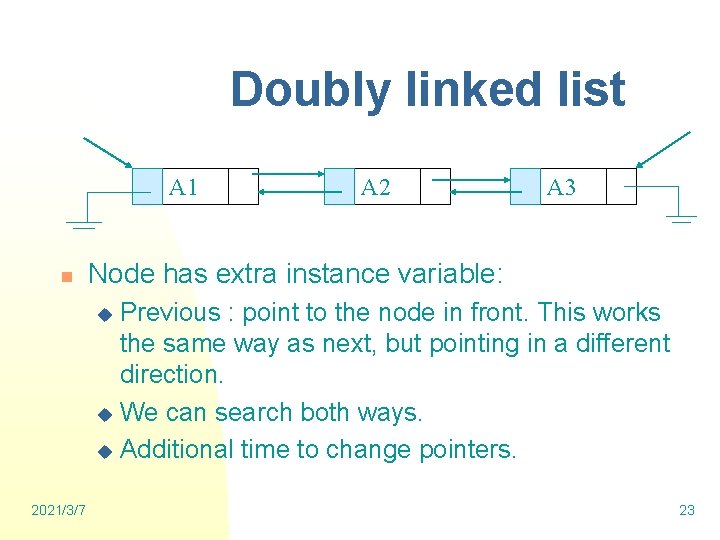
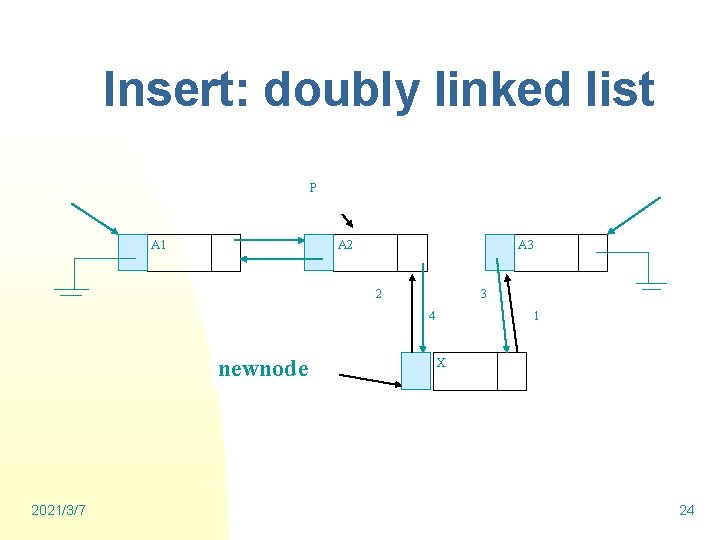
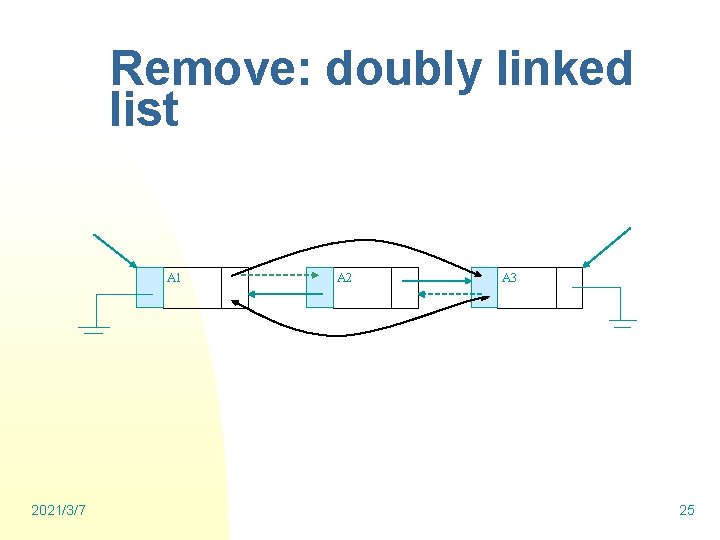
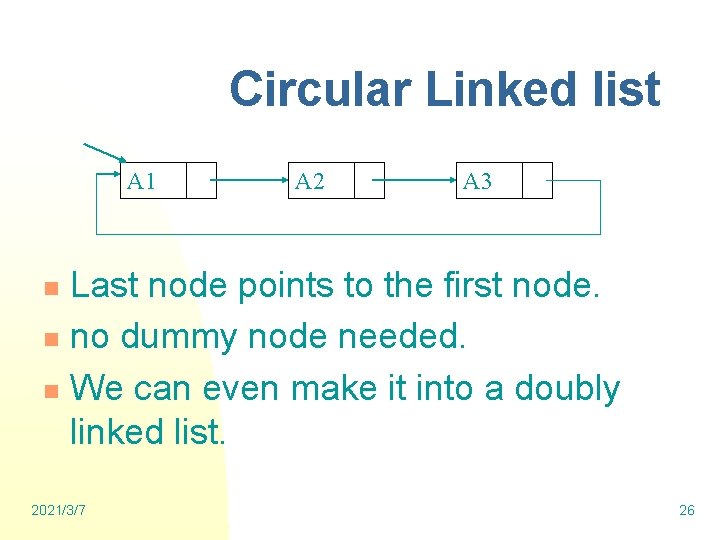
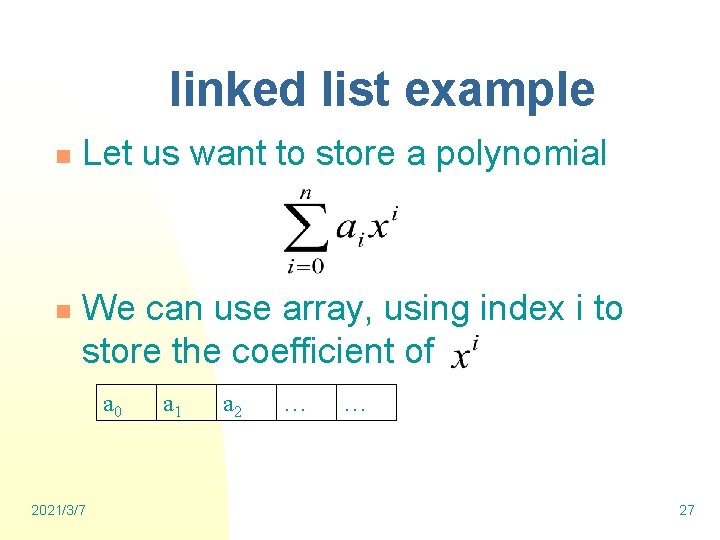
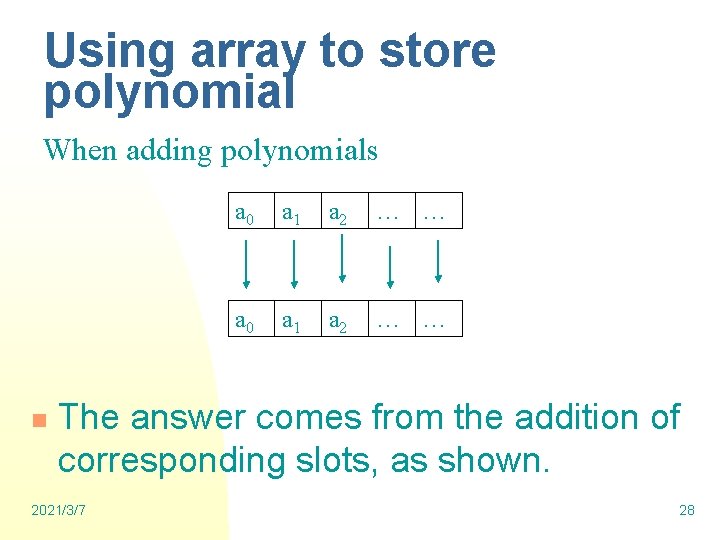
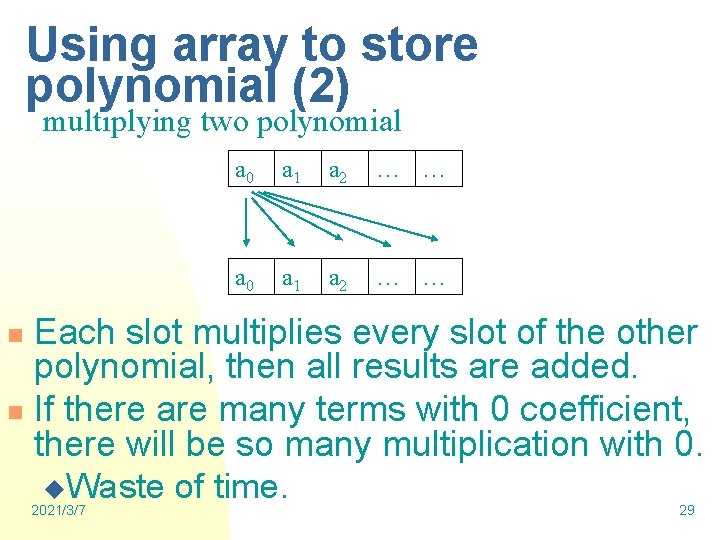
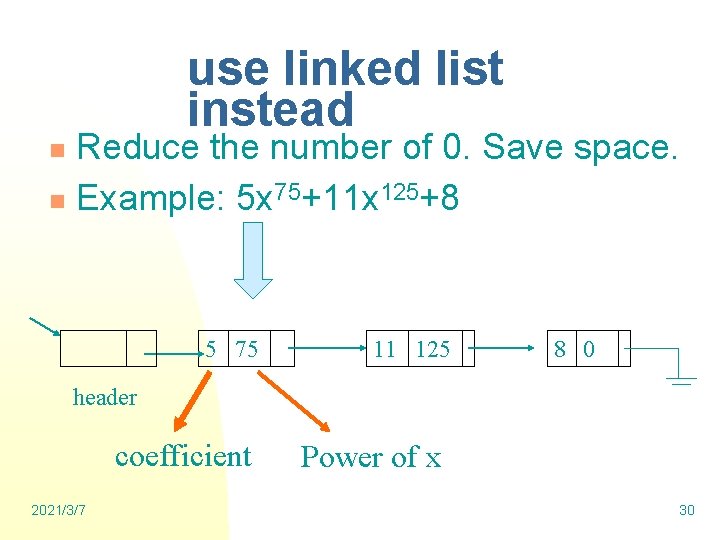
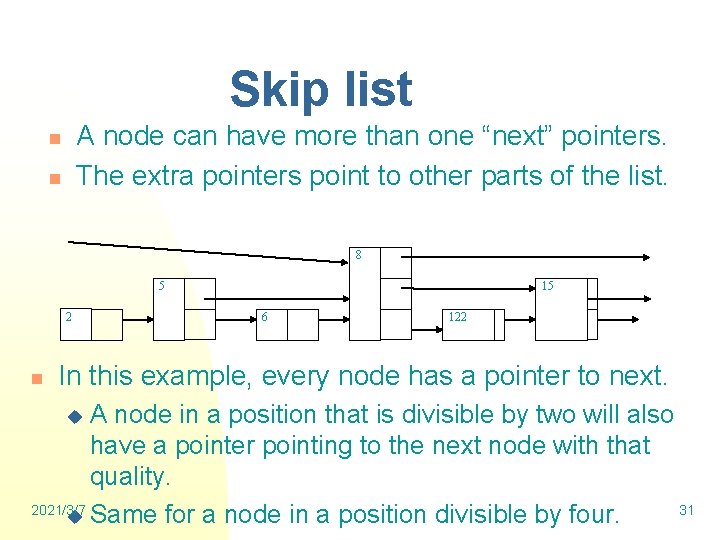
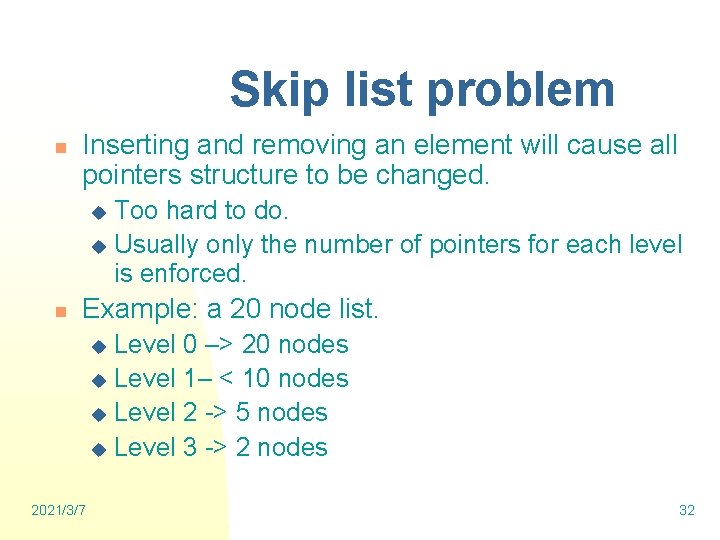
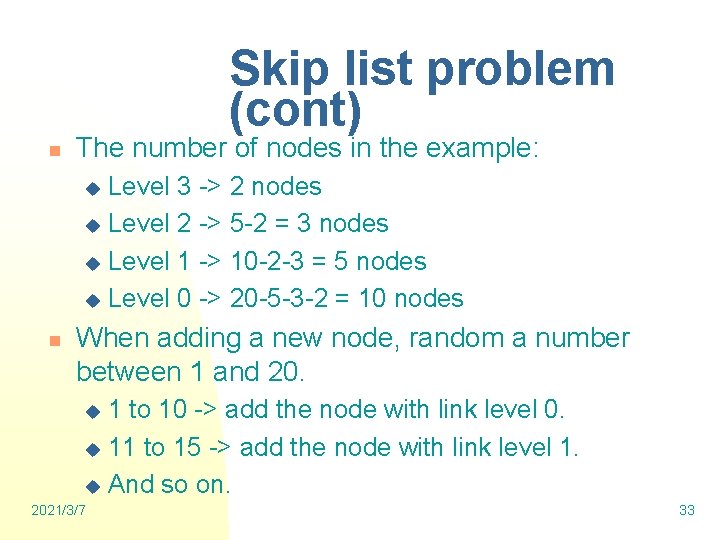
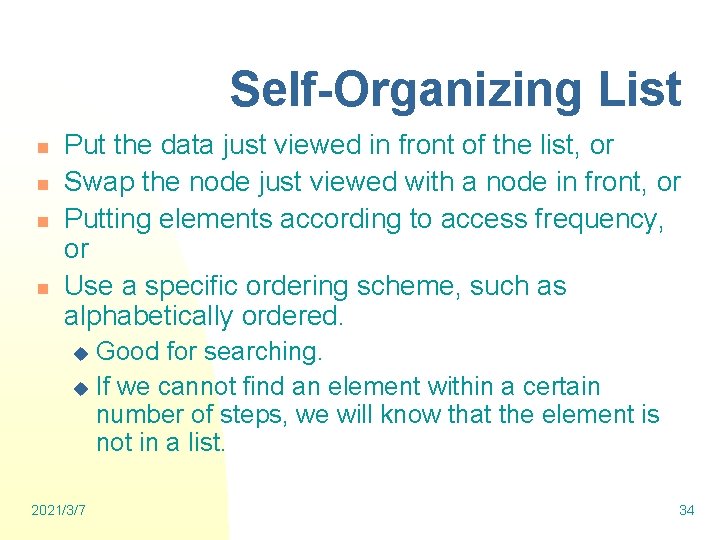
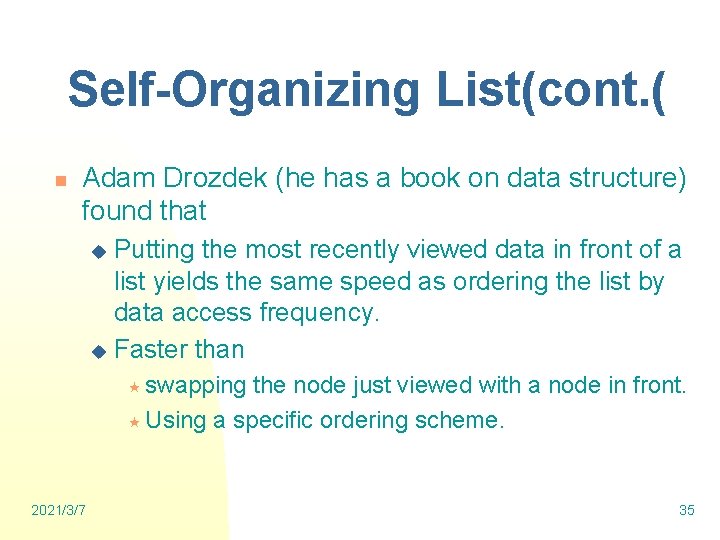
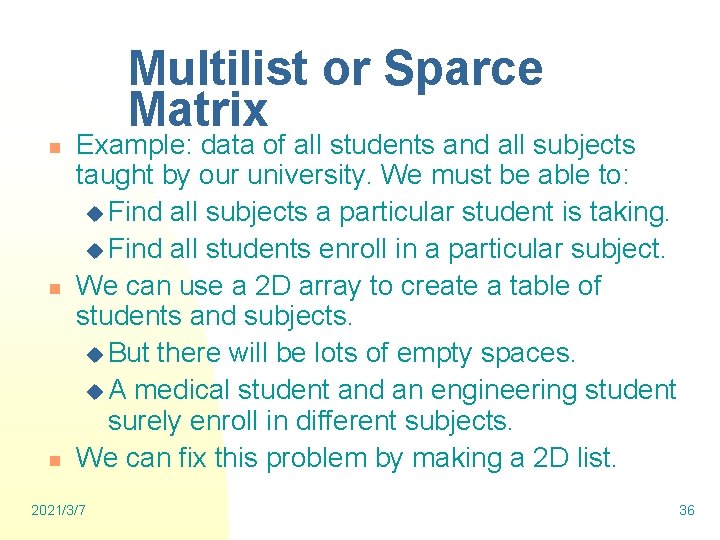
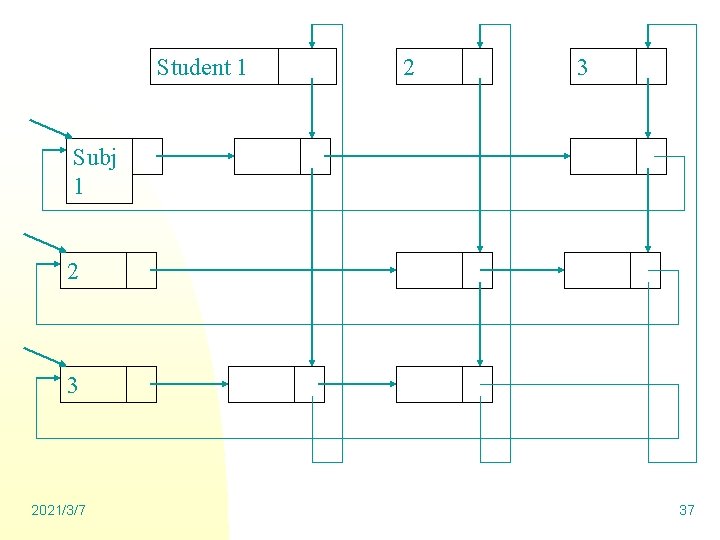
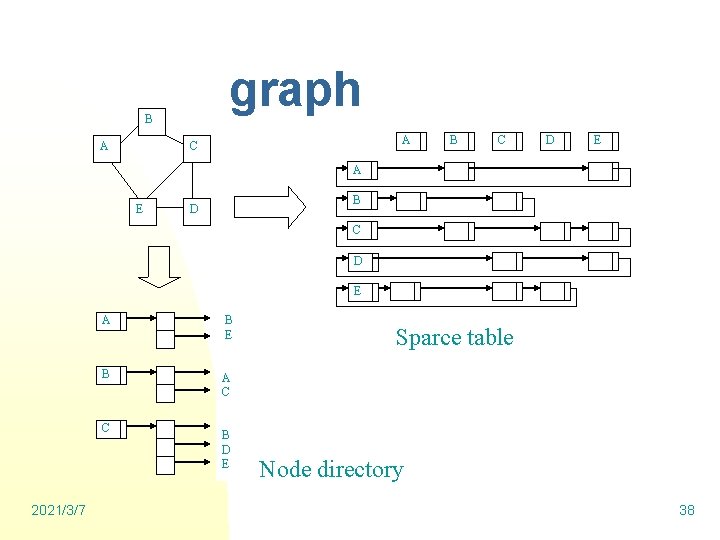
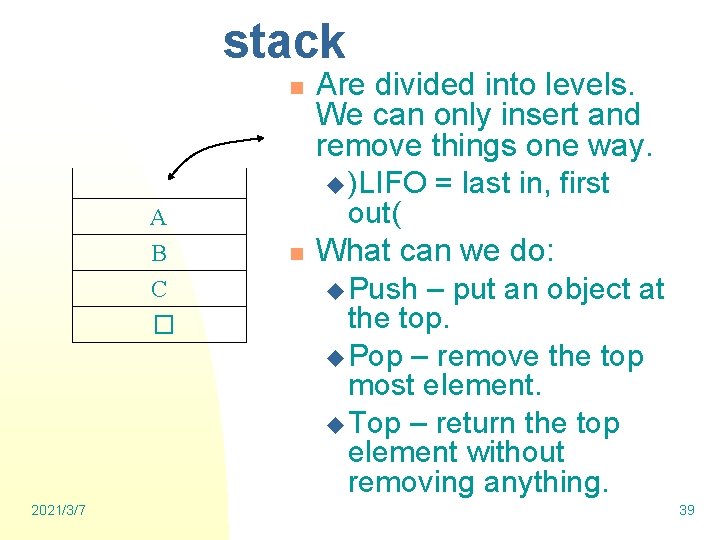
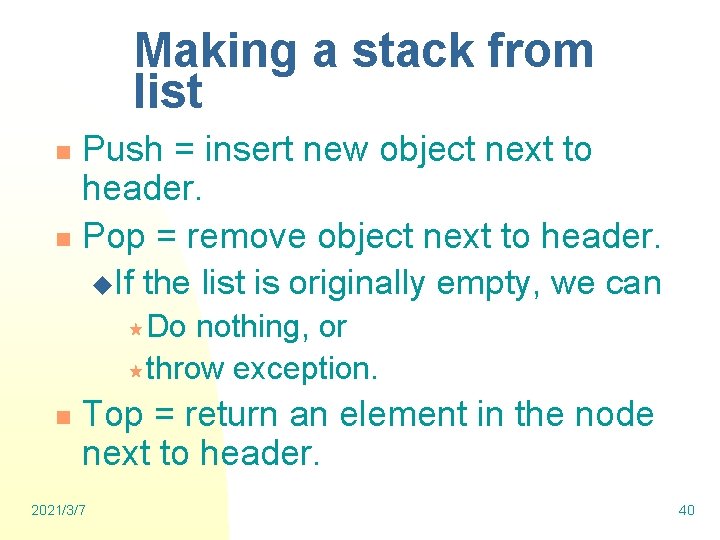
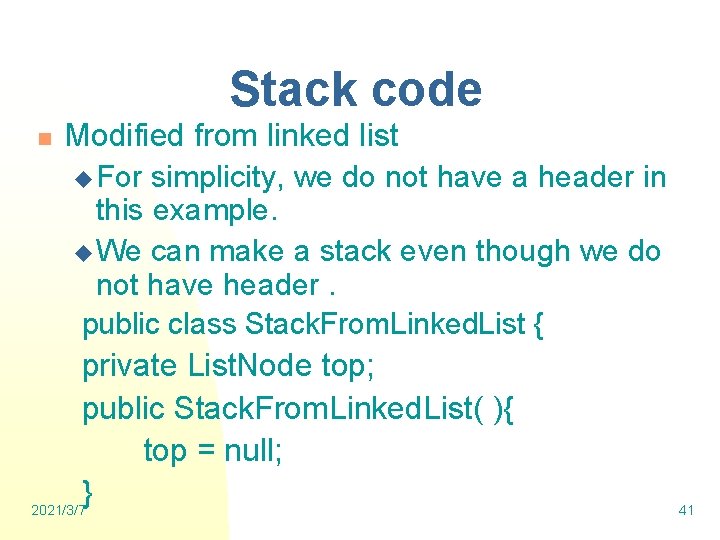
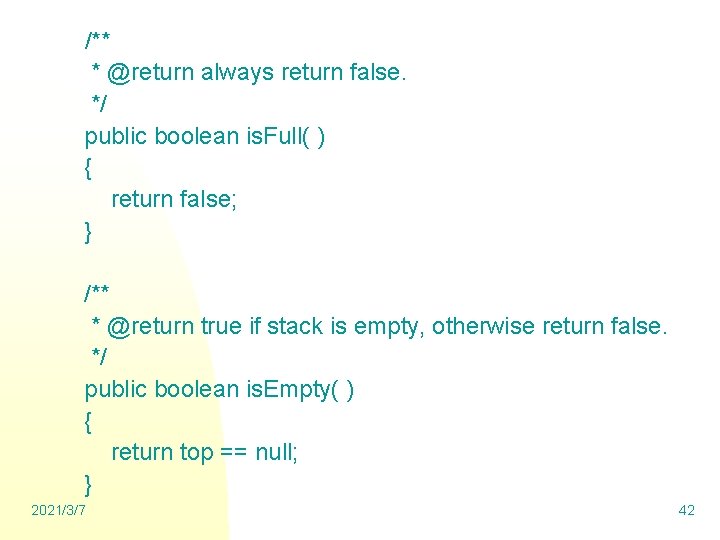
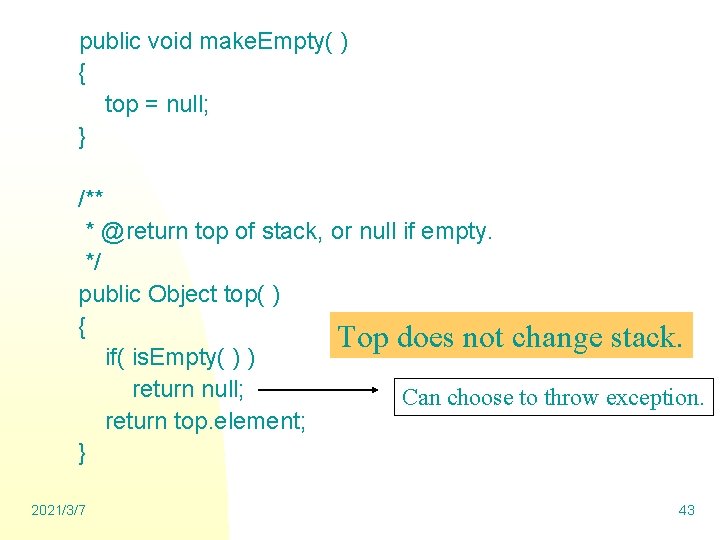
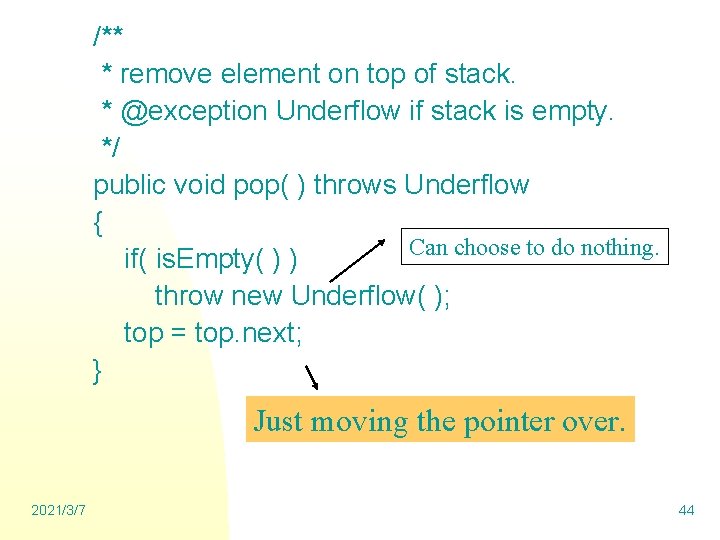
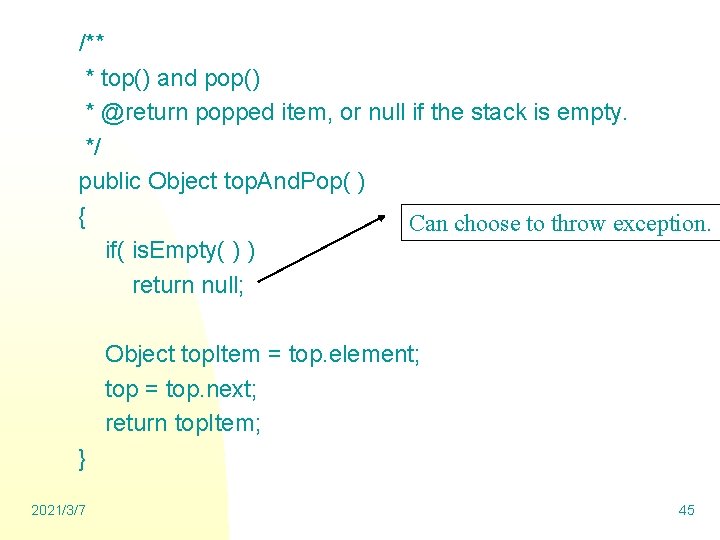
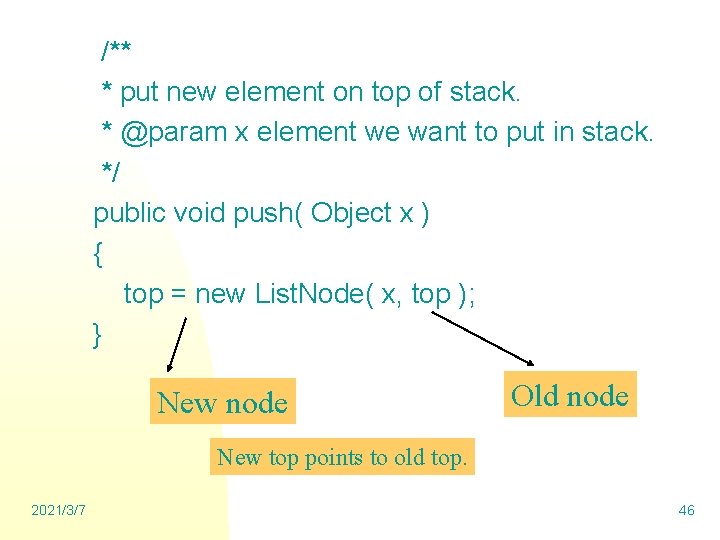
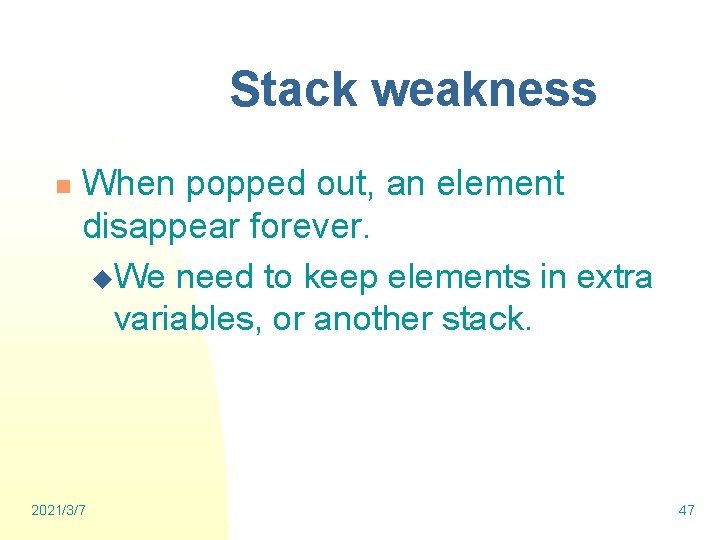
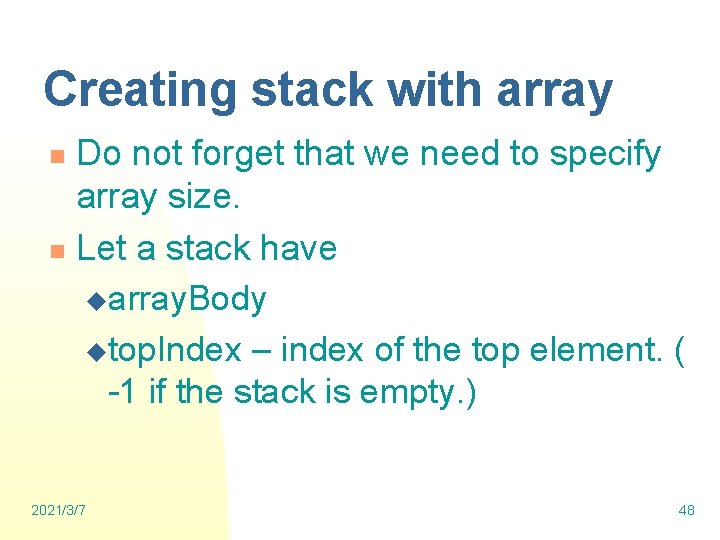
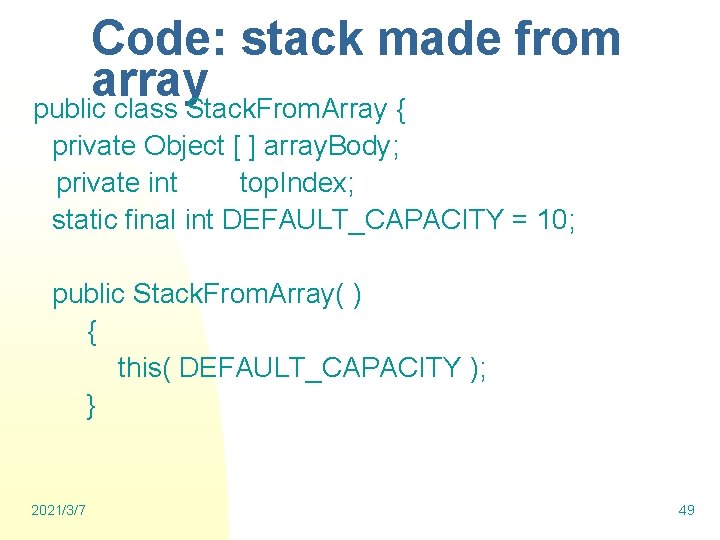
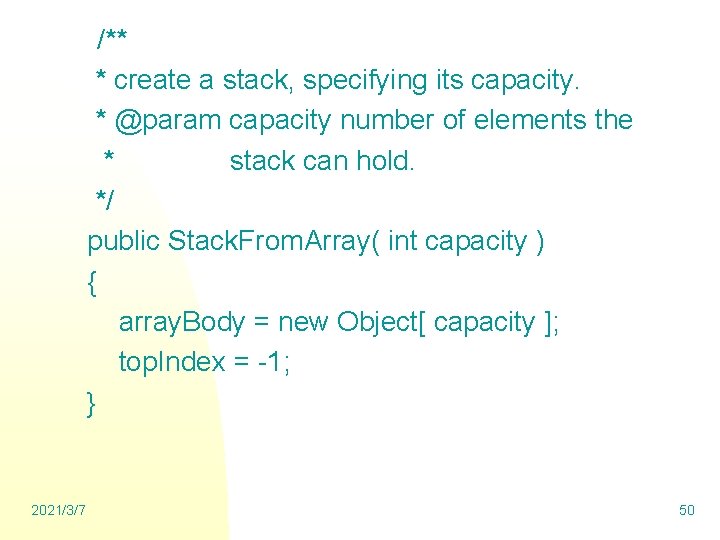
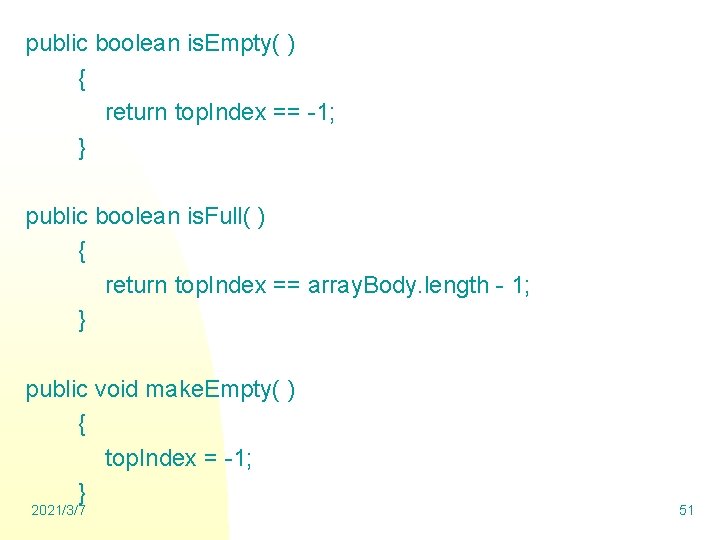
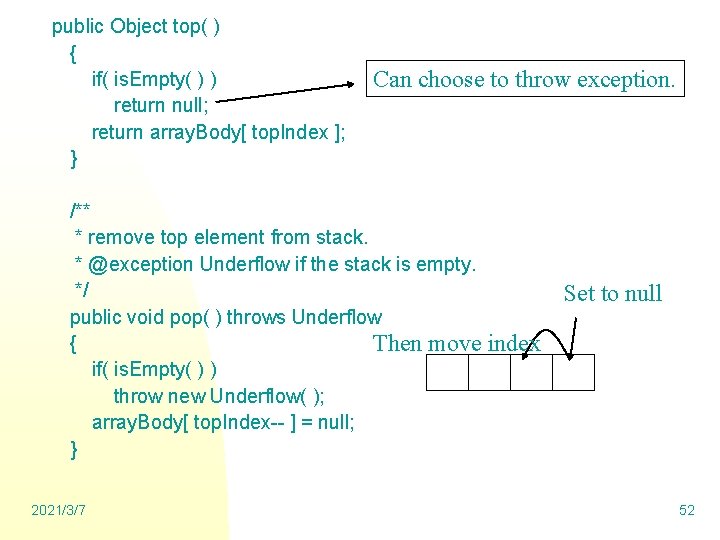
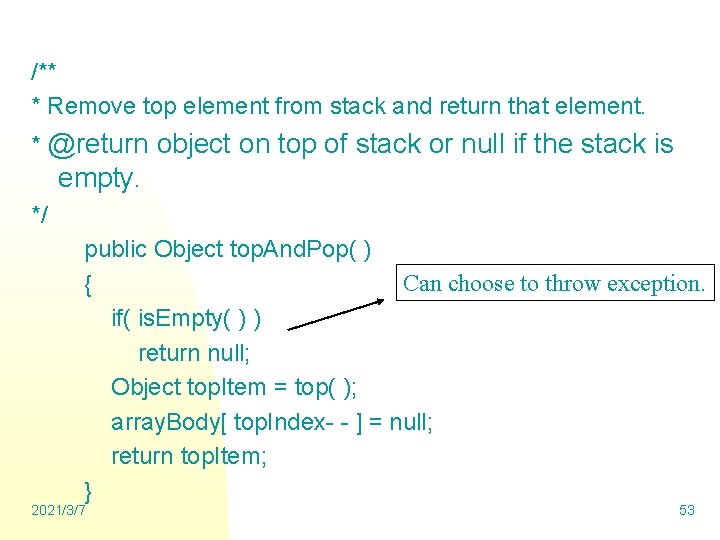
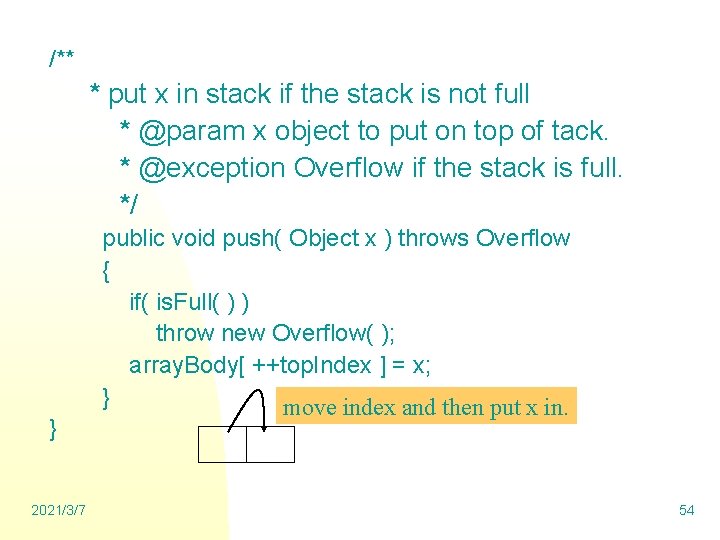
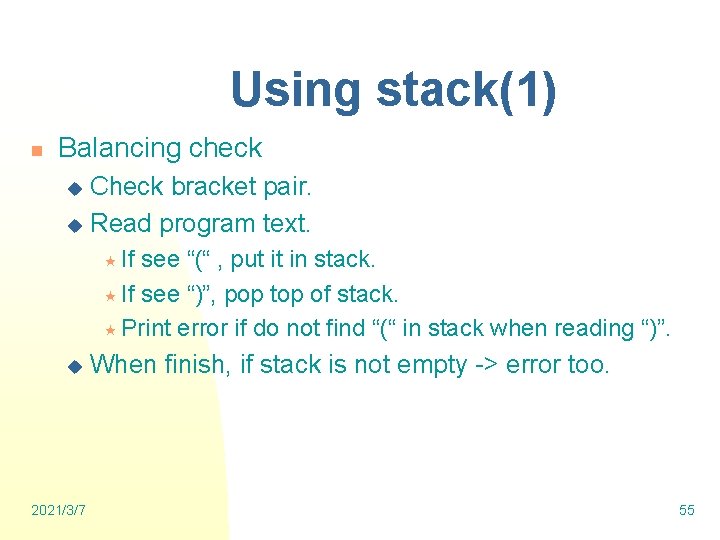
![Using stack(2) n Postfix expression (reverse polish) [7+(8*9)+5]*10 = 7 8 9 * 5 Using stack(2) n Postfix expression (reverse polish) [7+(8*9)+5]*10 = 7 8 9 * 5](https://slidetodoc.com/presentation_image_h/2ab4e1ea4ad3f8d8d7079ac0166ebdd9/image-56.jpg)
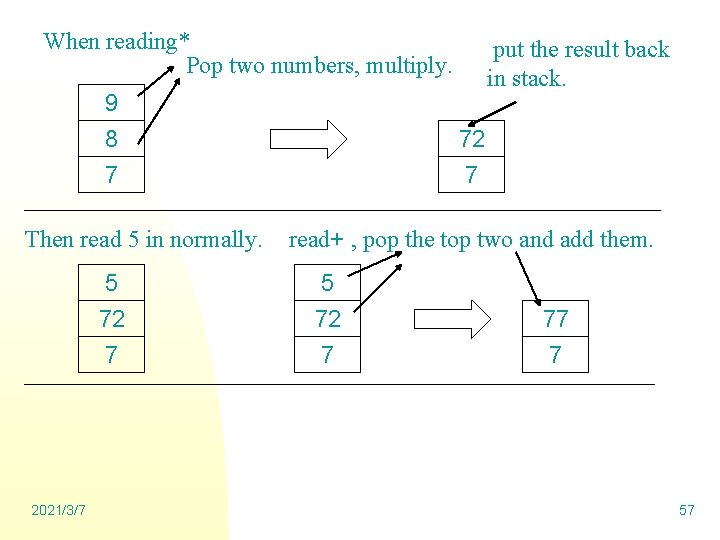
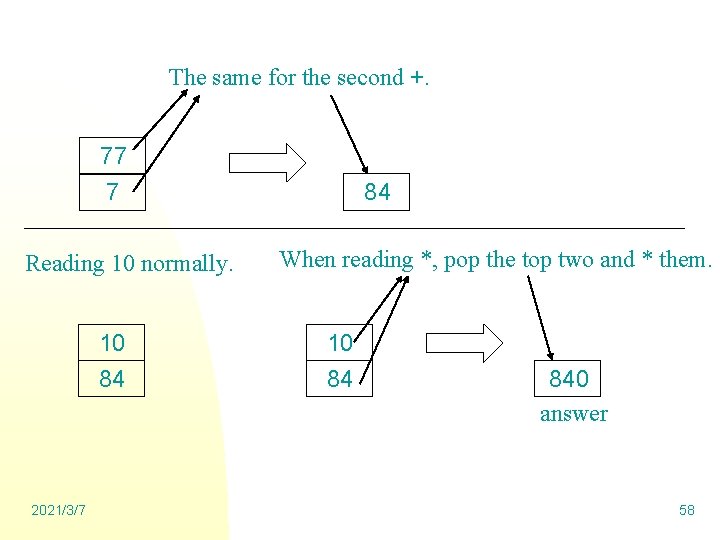
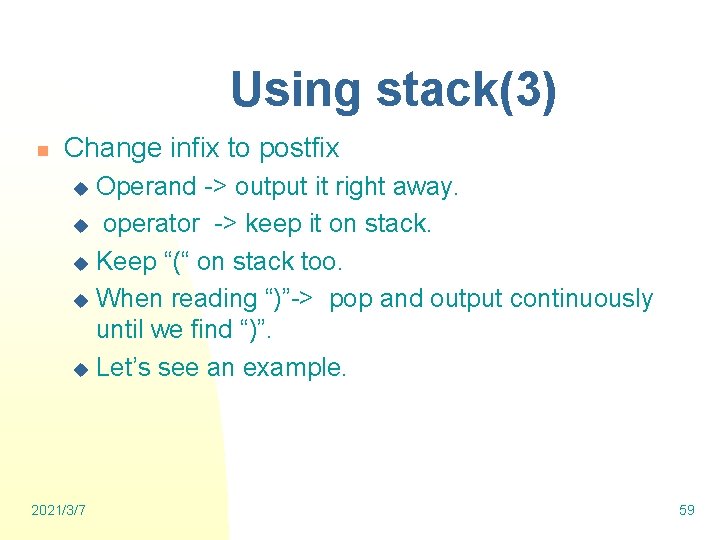
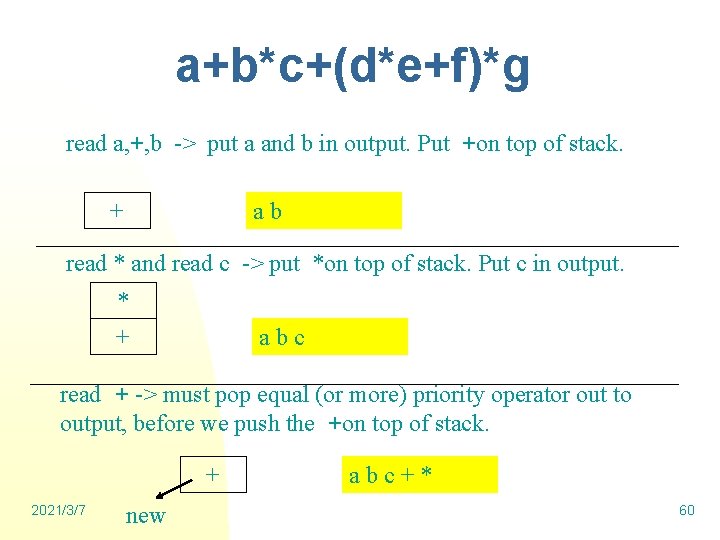
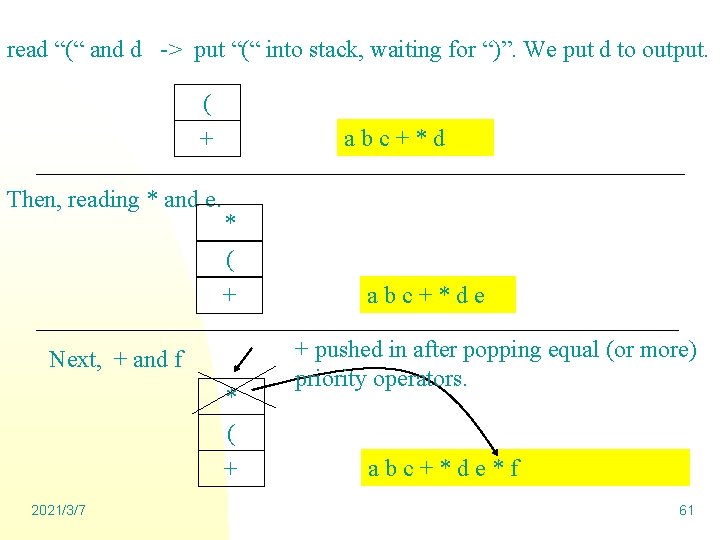
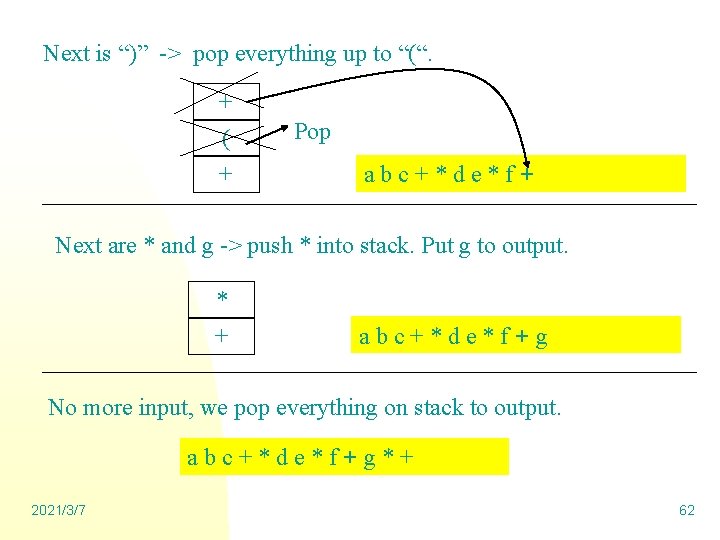
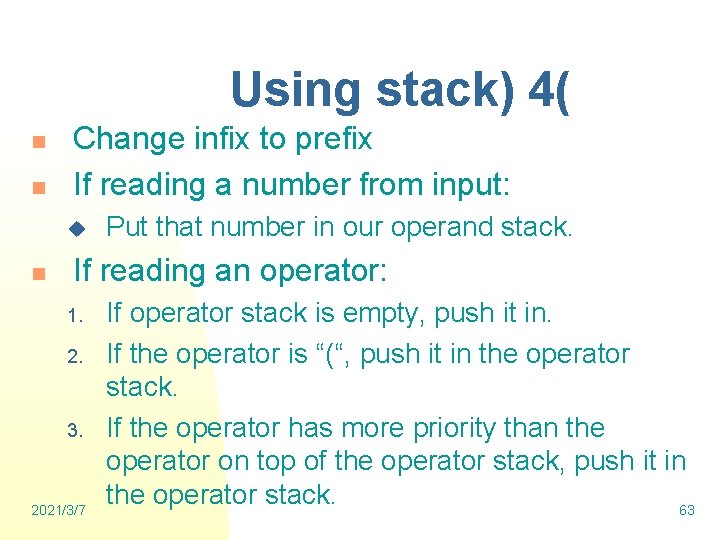
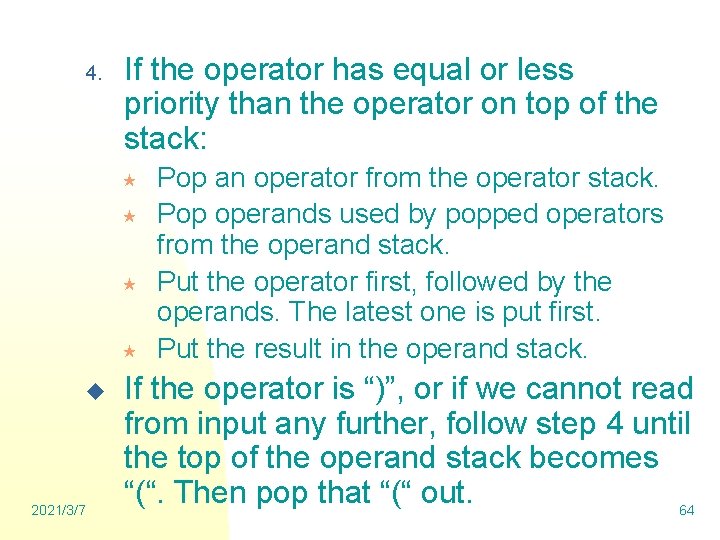
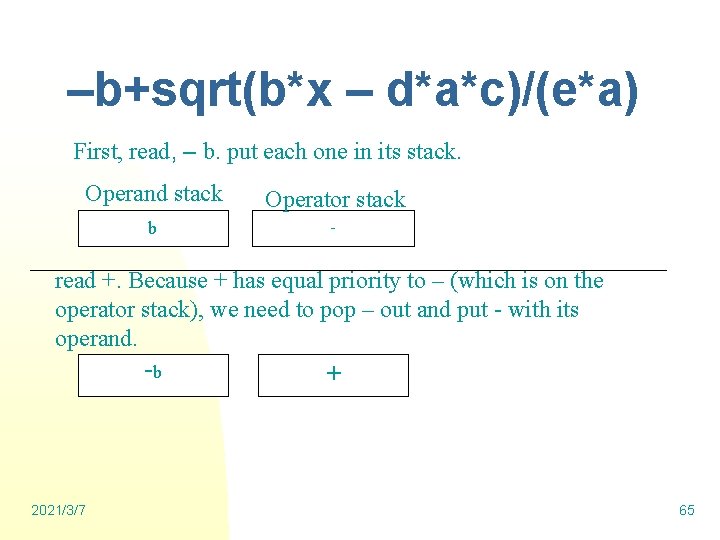
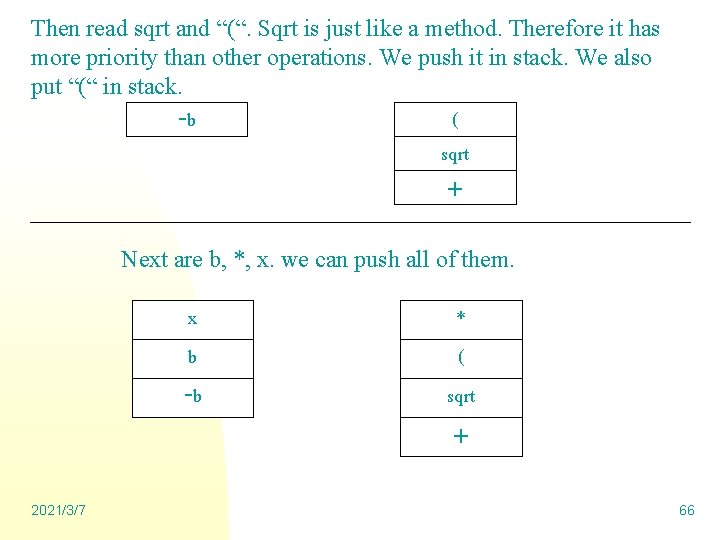
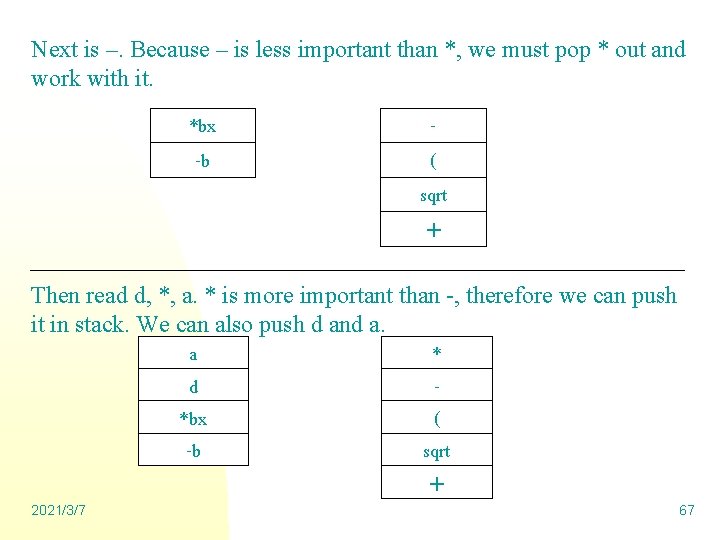
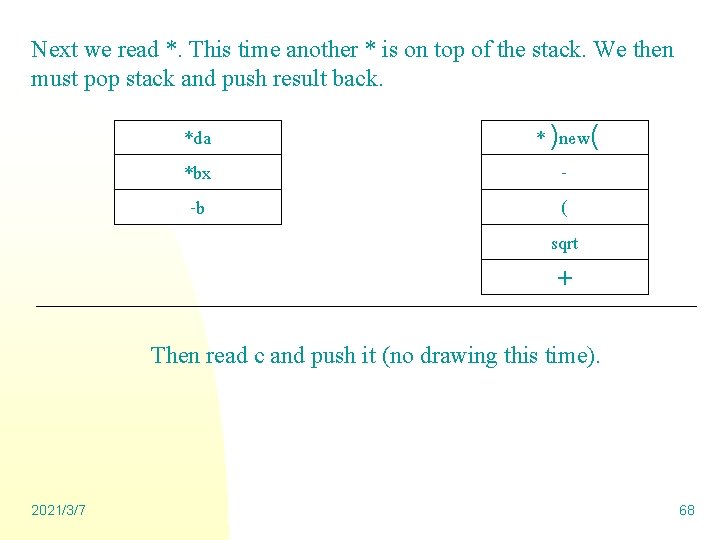
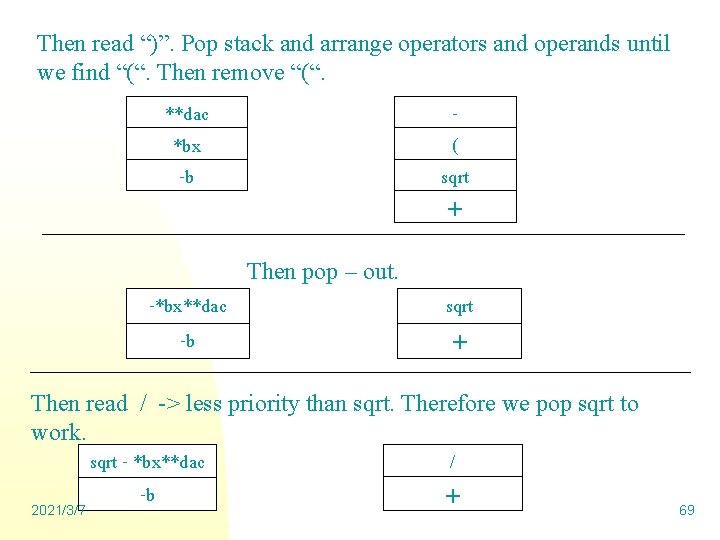
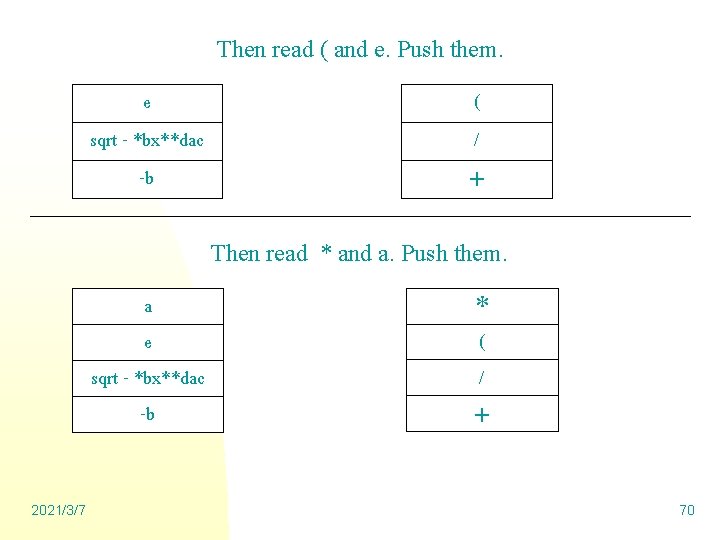
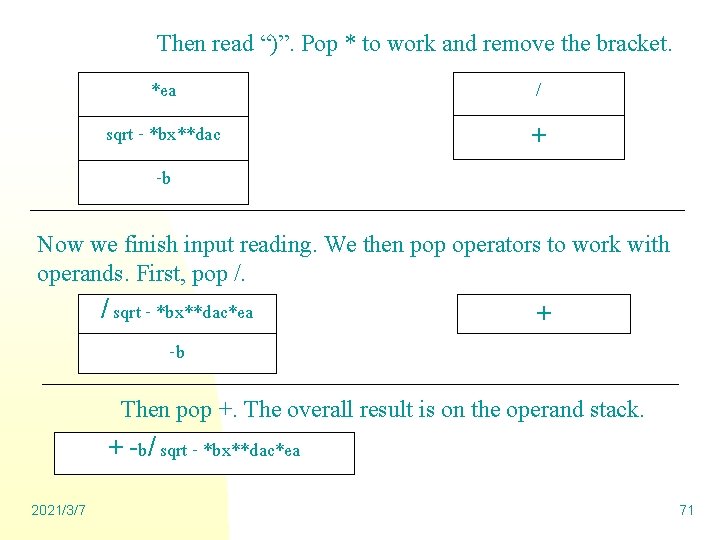
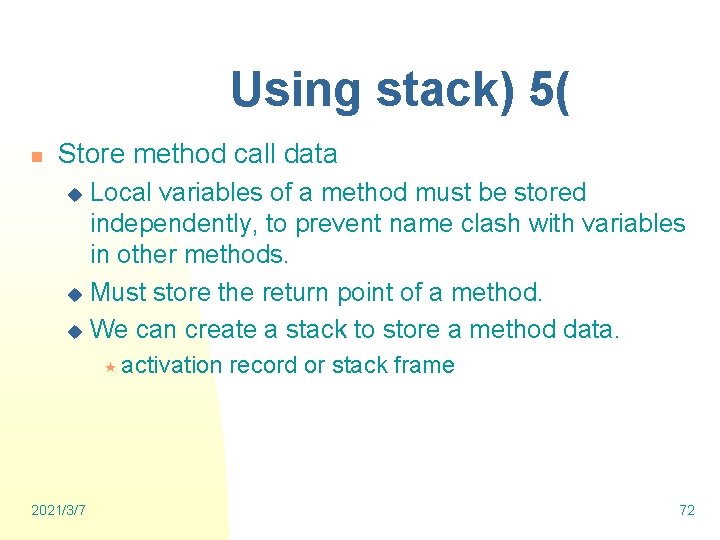
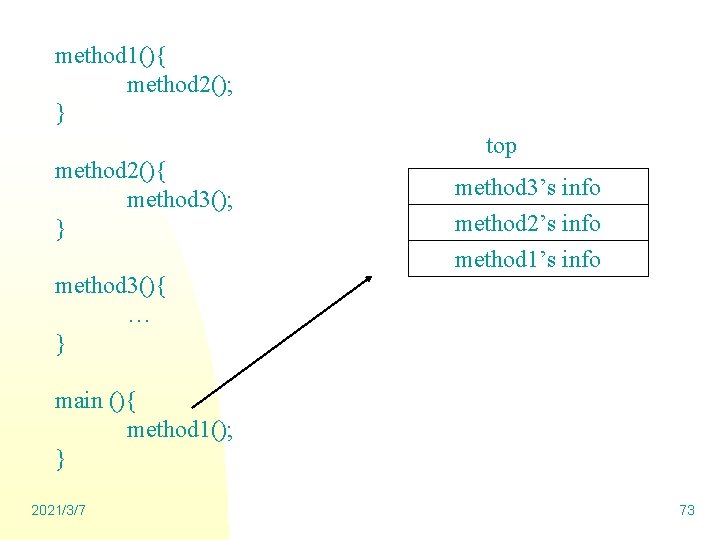
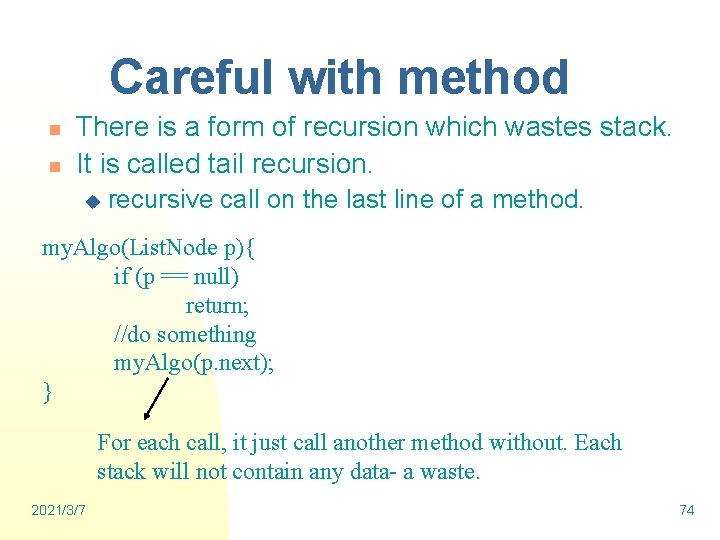
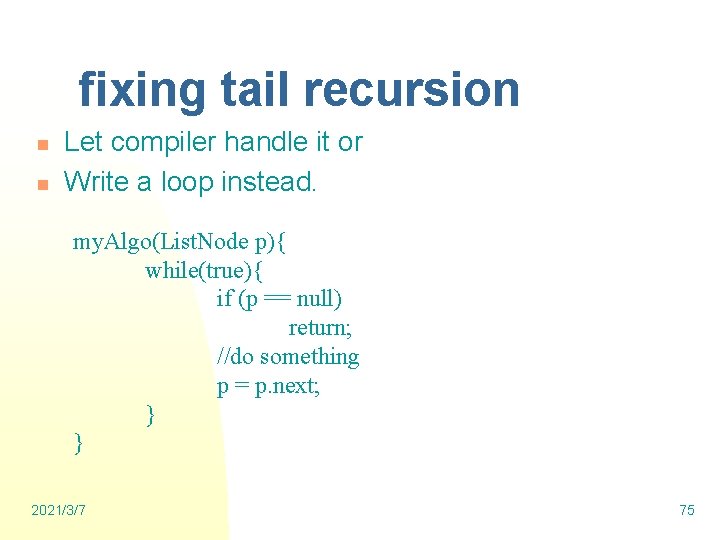
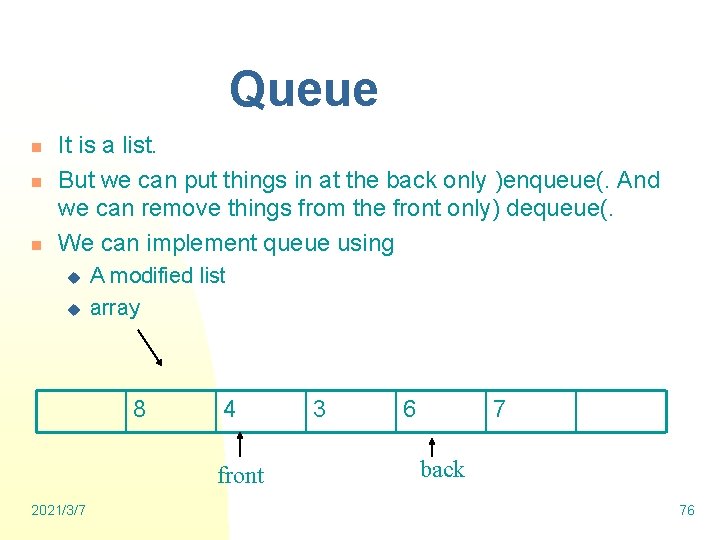
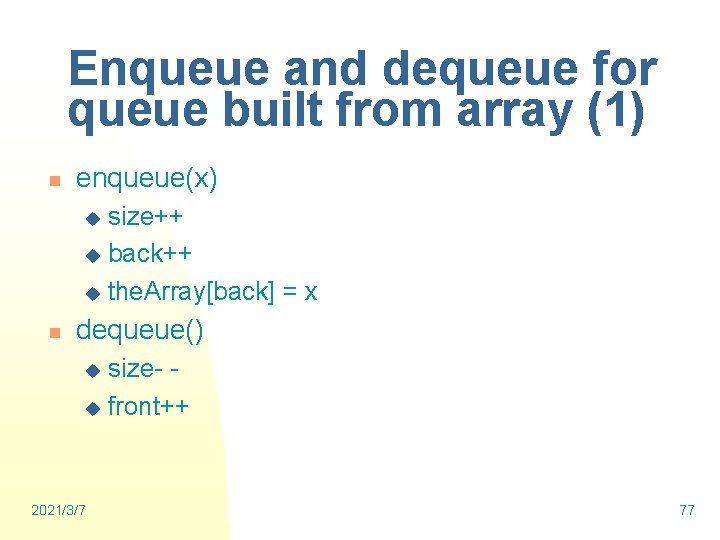
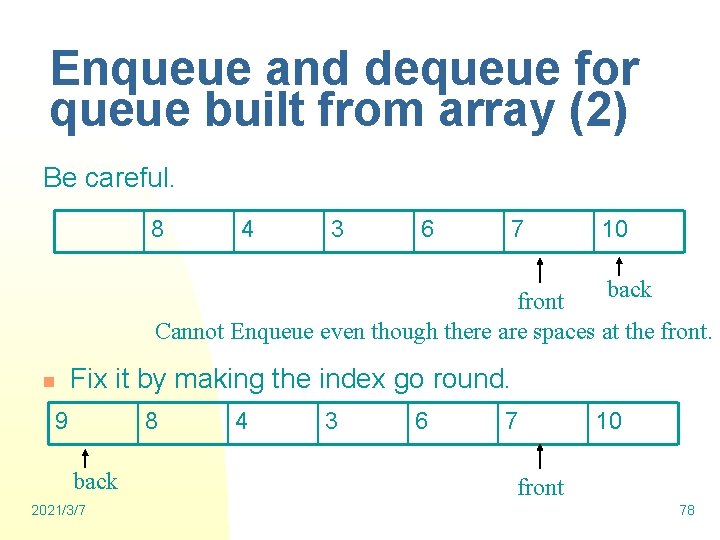
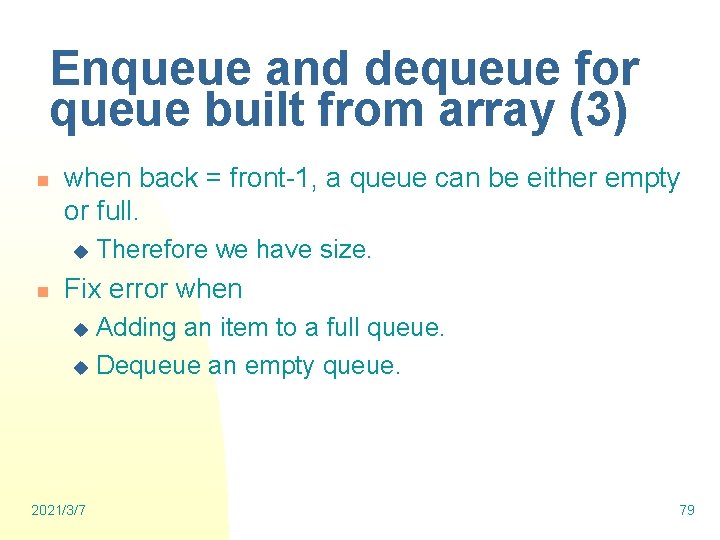
![public class Queue. Array{ private Object [ ] the. Array; private int size; private public class Queue. Array{ private Object [ ] the. Array; private int size; private](https://slidetodoc.com/presentation_image_h/2ab4e1ea4ad3f8d8d7079ac0166ebdd9/image-80.jpg)
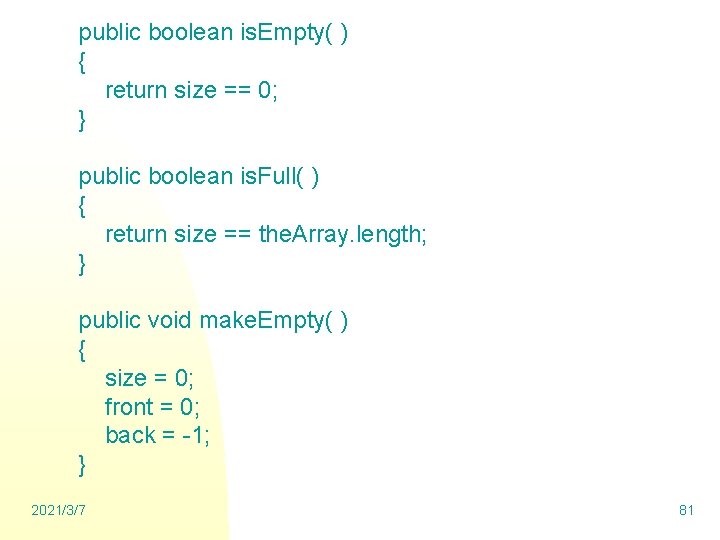
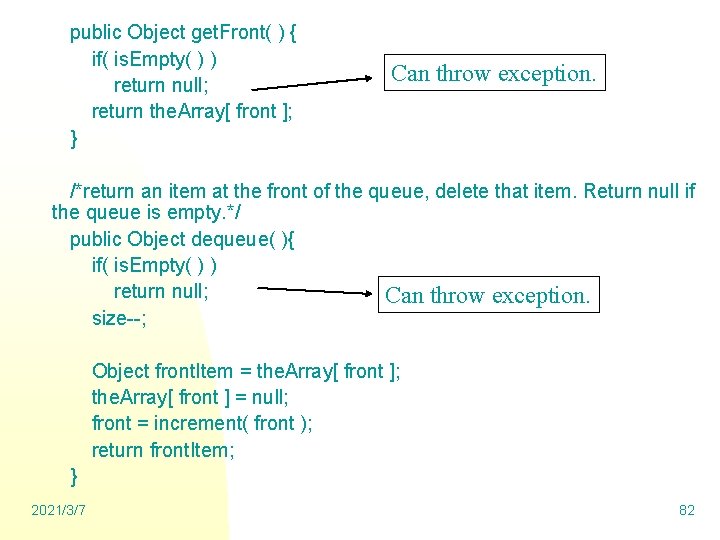
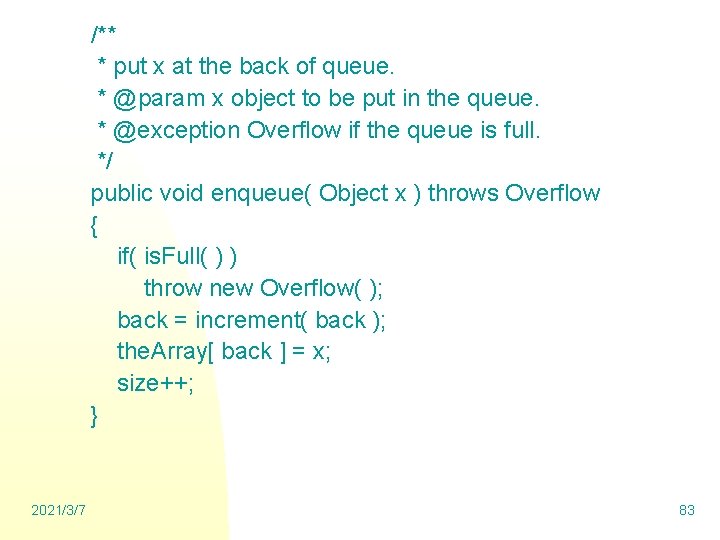
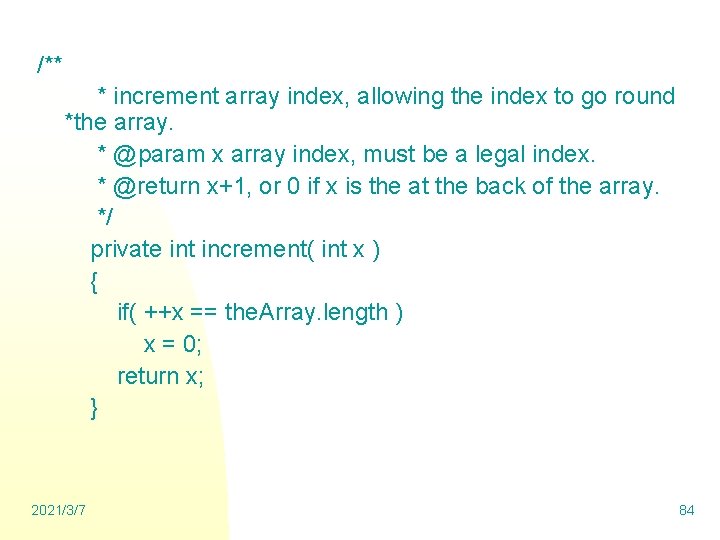
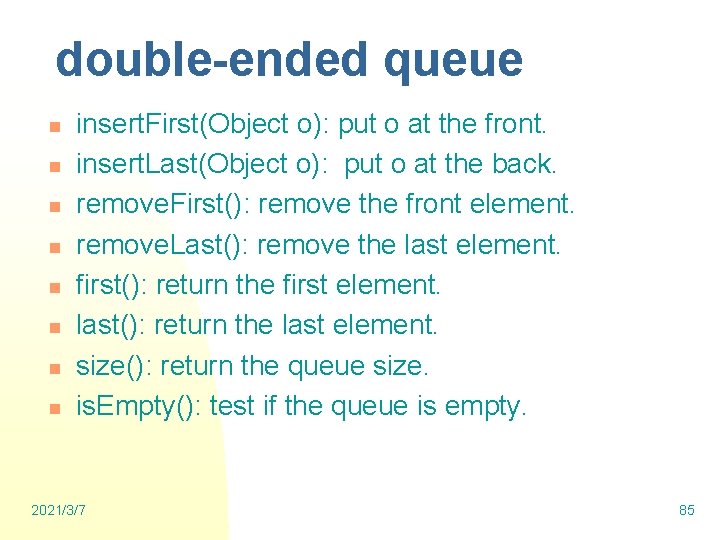
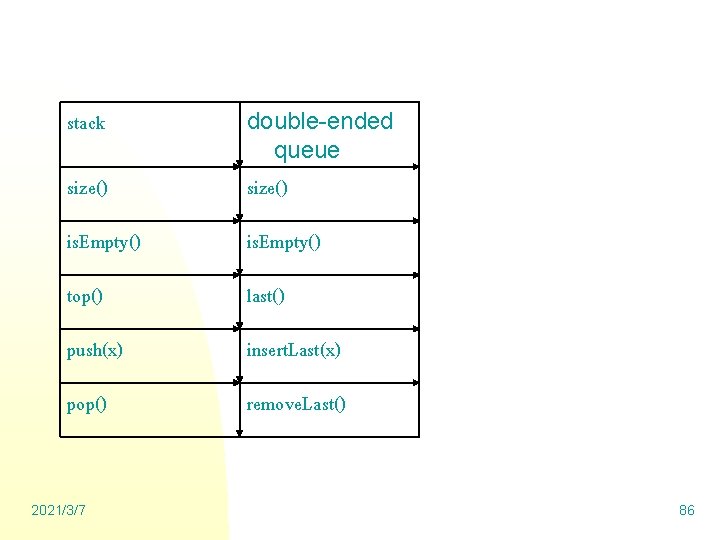
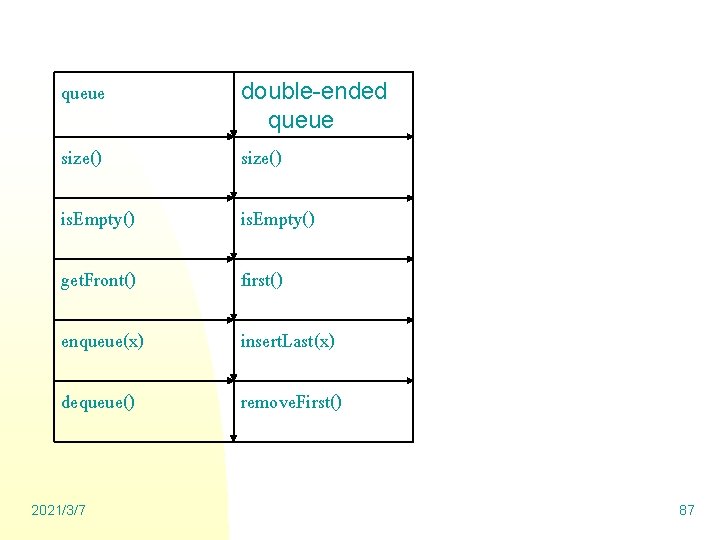
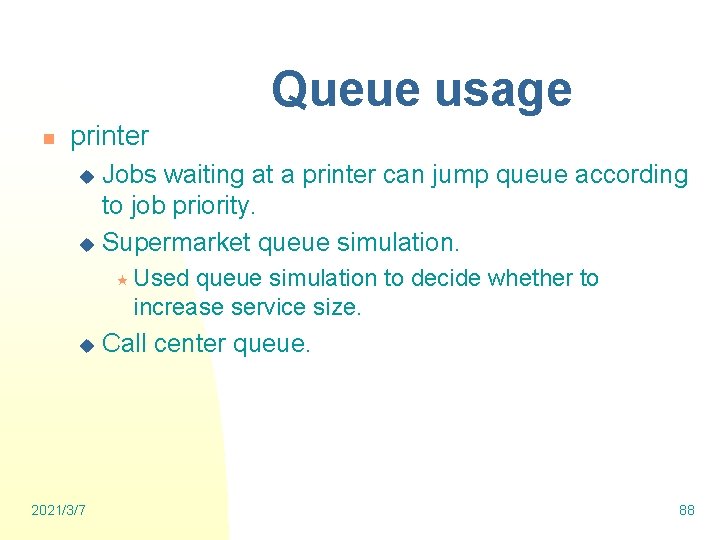
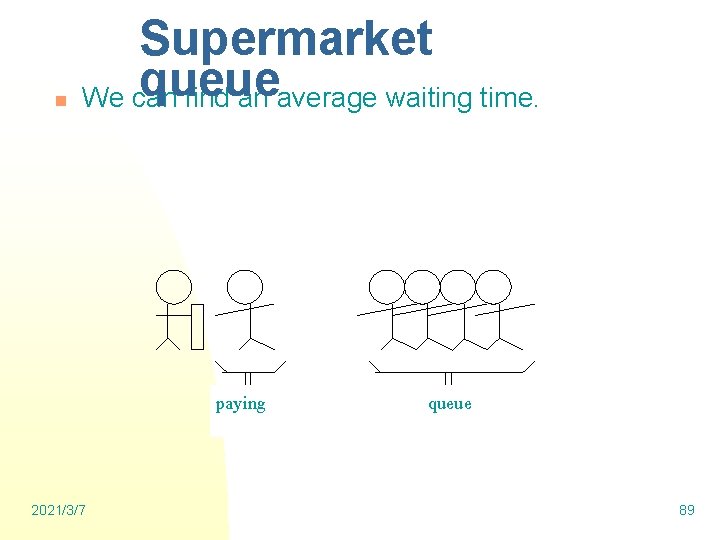
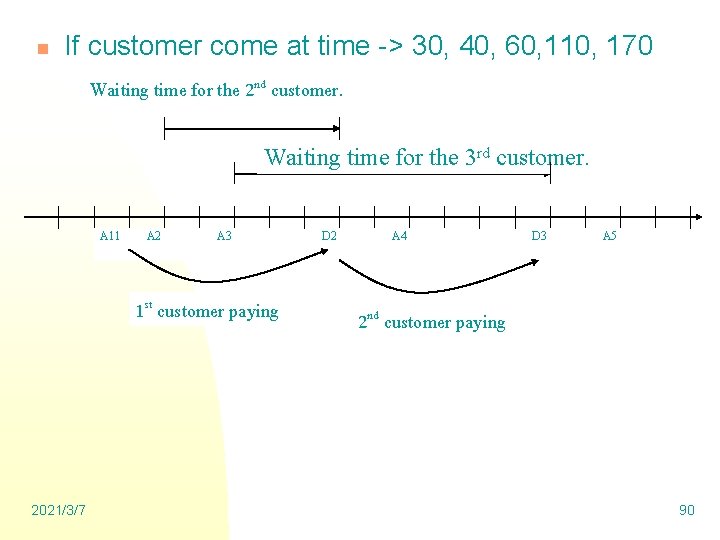
- Slides: 90
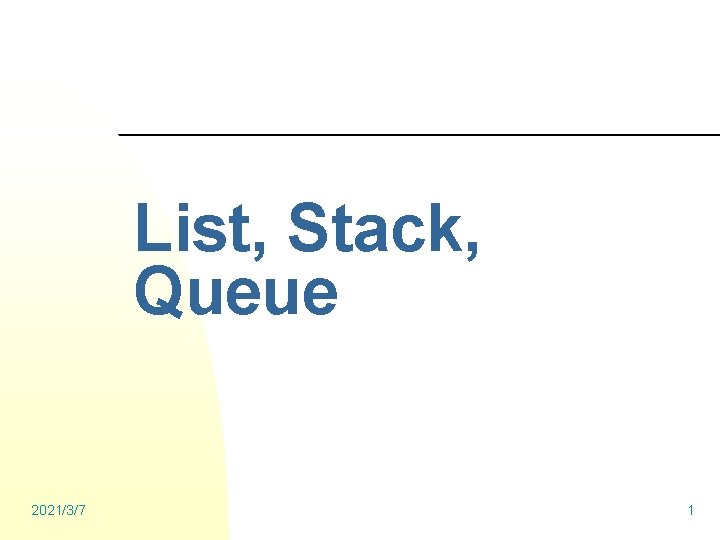
List, Stack, Queue 2021/3/7 1
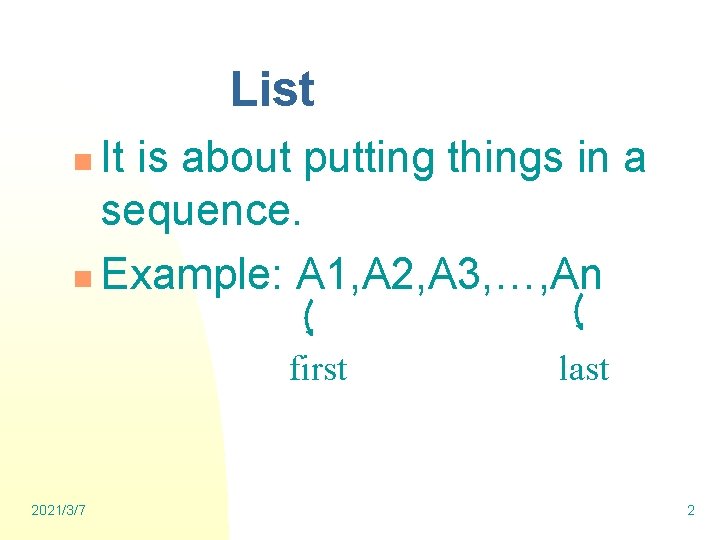
List It is about putting things in a sequence. n Example: A 1, A 2, A 3, …, An n first 2021/3/7 last 2
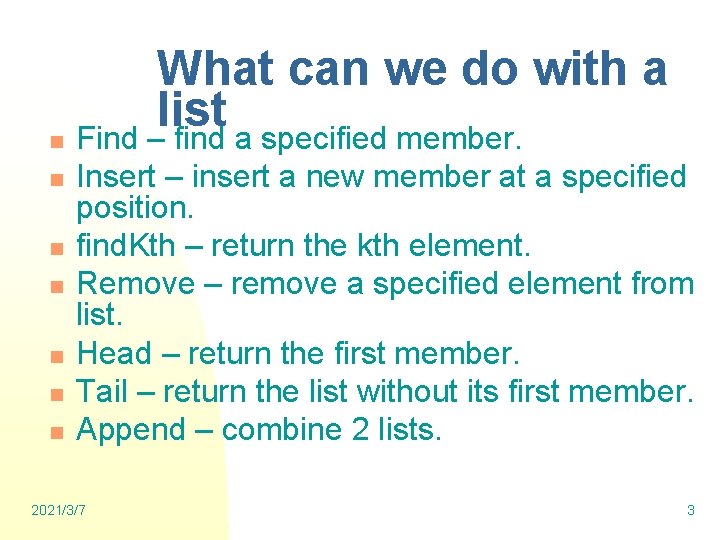
What can we do with a list n Find – find a specified member. n n n Insert – insert a new member at a specified position. find. Kth – return the kth element. Remove – remove a specified element from list. Head – return the first member. Tail – return the list without its first member. Append – combine 2 lists. 2021/3/7 3
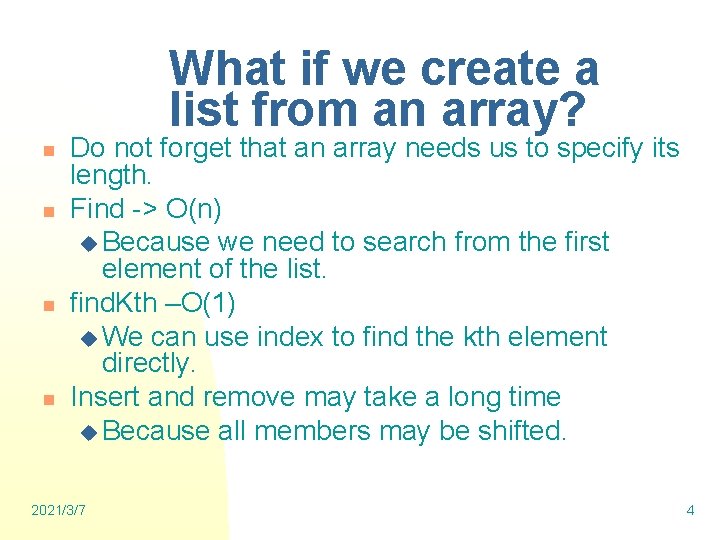
What if we create a list from an array? n n Do not forget that an array needs us to specify its length. Find -> O(n) u Because we need to search from the first element of the list. find. Kth –O(1) u We can use index to find the kth element directly. Insert and remove may take a long time u Because all members may be shifted. 2021/3/7 4
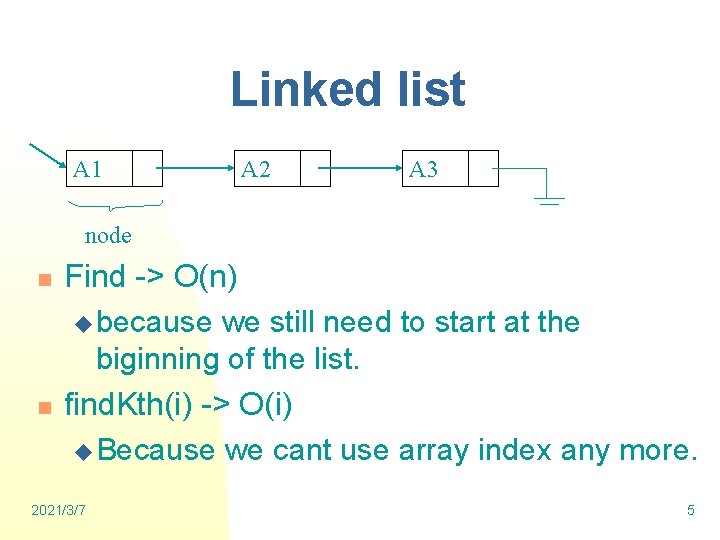
Linked list A 1 A 2 A 3 node n n Find -> O(n) u because we still need to start at the biginning of the list. find. Kth(i) -> O(i) u Because we cant use array index any more. 2021/3/7 5
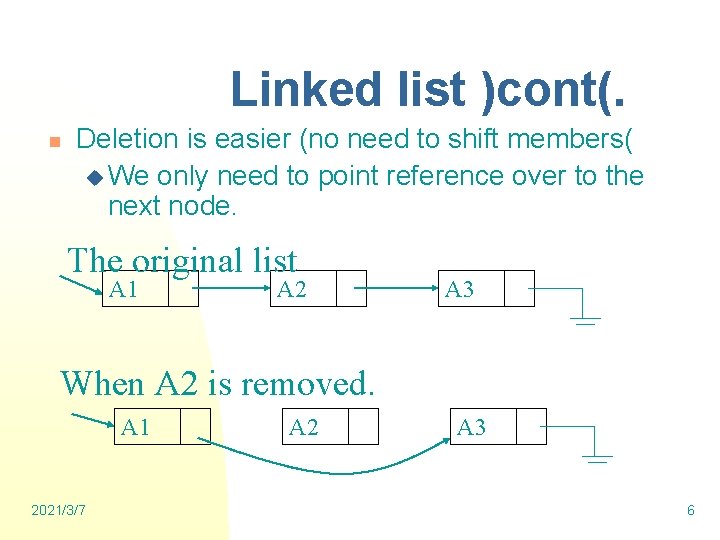
Linked list )cont(. n Deletion is easier (no need to shift members( u We only need to point reference over to the next node. The original list A 1 A 2 A 3 When A 2 is removed. A 1 2021/3/7 A 2 A 3 6
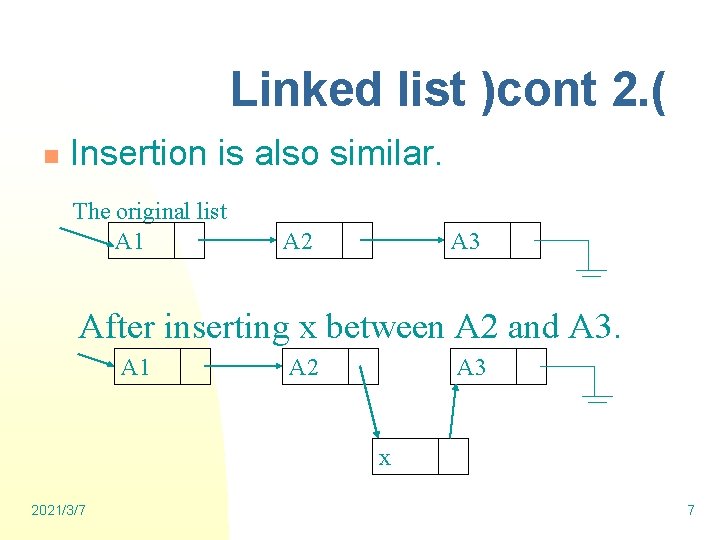
Linked list )cont 2. ( n Insertion is also similar. The original list A 1 A 2 A 3 After inserting x between A 2 and A 3. A 1 A 2 A 3 x 2021/3/7 7
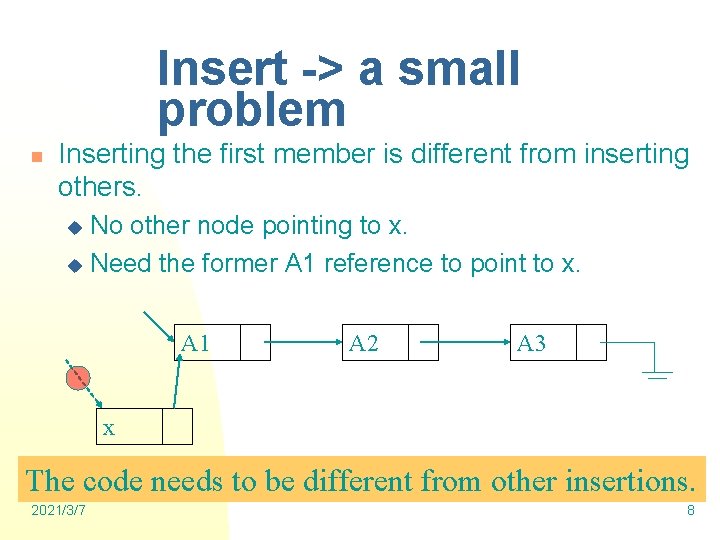
Insert -> a small problem n Inserting the first member is different from inserting others. No other node pointing to x. u Need the former A 1 reference to point to x. u A 1 A 2 A 3 x The code needs to be different from other insertions. 2021/3/7 8
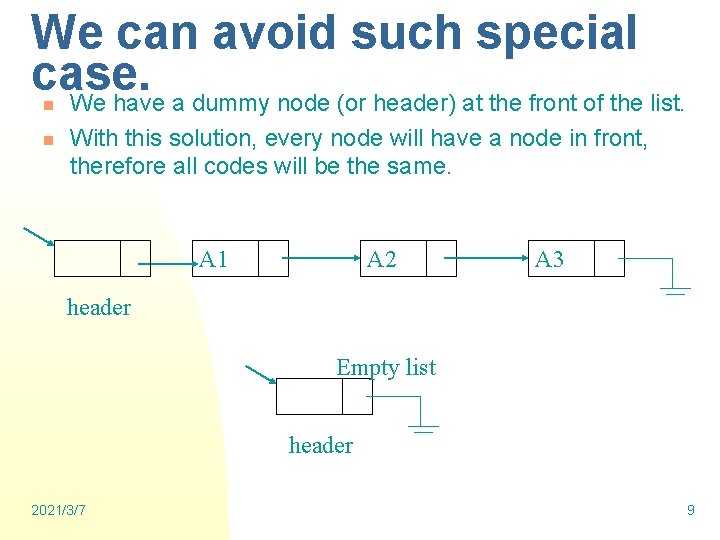
We can avoid such special case. We have a dummy node (or header) at the front of the list. n n With this solution, every node will have a node in front, therefore all codes will be the same. A 1 A 2 A 3 header Empty list header 2021/3/7 9
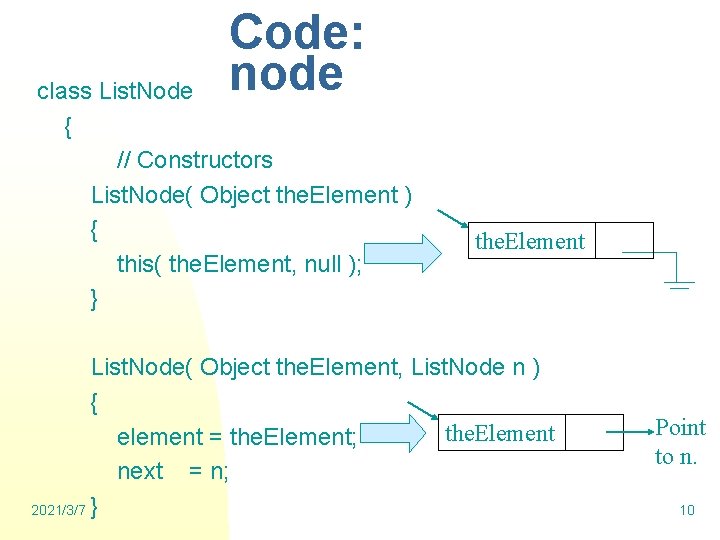
Code: node class List. Node { // Constructors List. Node( Object the. Element ) { this( the. Element, null ); } the. Element List. Node( Object the. Element, List. Node n ) { the. Element element = the. Element; next = n; 2021/3/7 } Point to n. 10
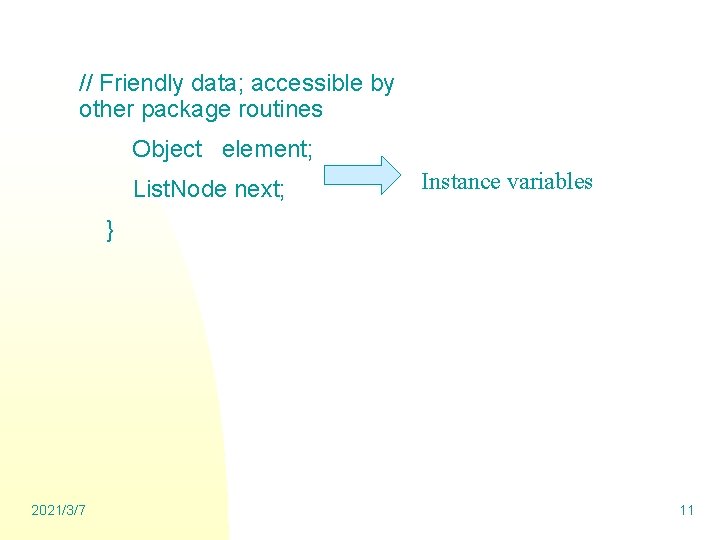
// Friendly data; accessible by other package routines Object element; List. Node next; Instance variables } 2021/3/7 11
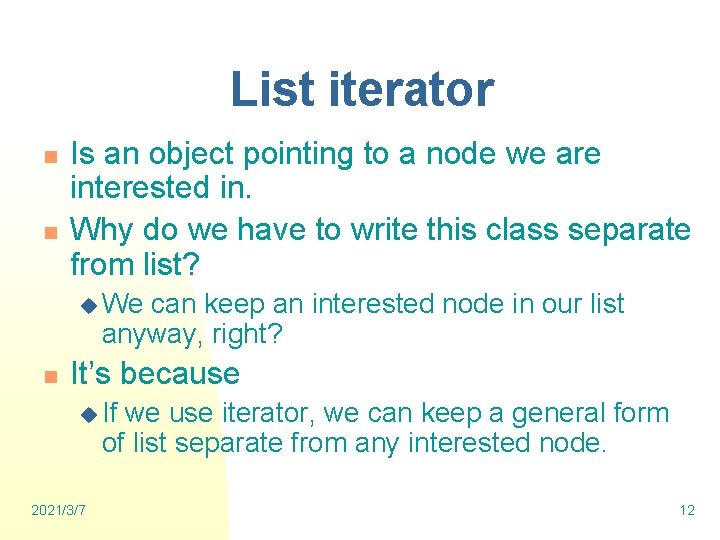
List iterator n n Is an object pointing to a node we are interested in. Why do we have to write this class separate from list? u We can keep an interested node in our list anyway, right? n It’s because u If we use iterator, we can keep a general form of list separate from any interested node. 2021/3/7 12
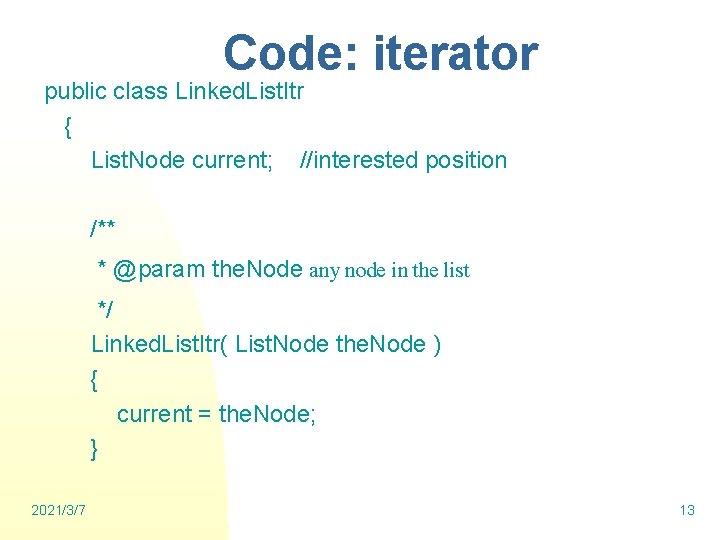
Code: iterator public class Linked. List. Itr { List. Node current; //interested position /** * @param the. Node any node in the list */ Linked. List. Itr( List. Node the. Node ) { current = the. Node; } 2021/3/7 13
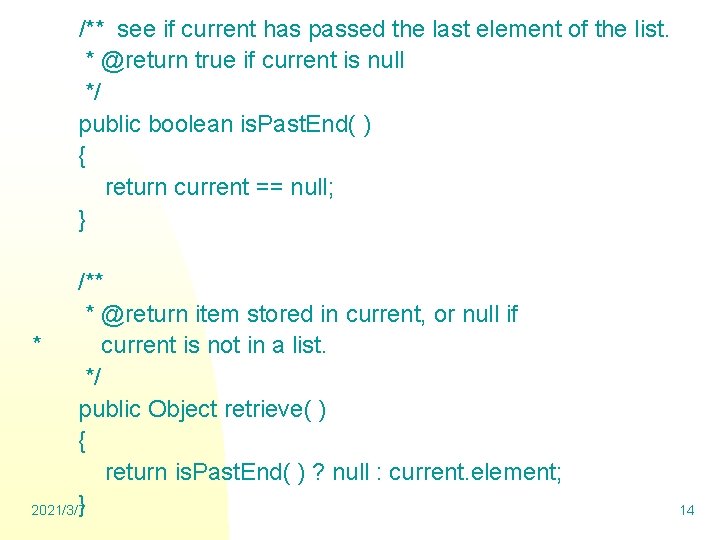
/** see if current has passed the last element of the list. * @return true if current is null */ public boolean is. Past. End( ) { return current == null; } /** * @return item stored in current, or null if * current is not in a list. */ public Object retrieve( ) { return is. Past. End( ) ? null : current. element; } 2021/3/7 14
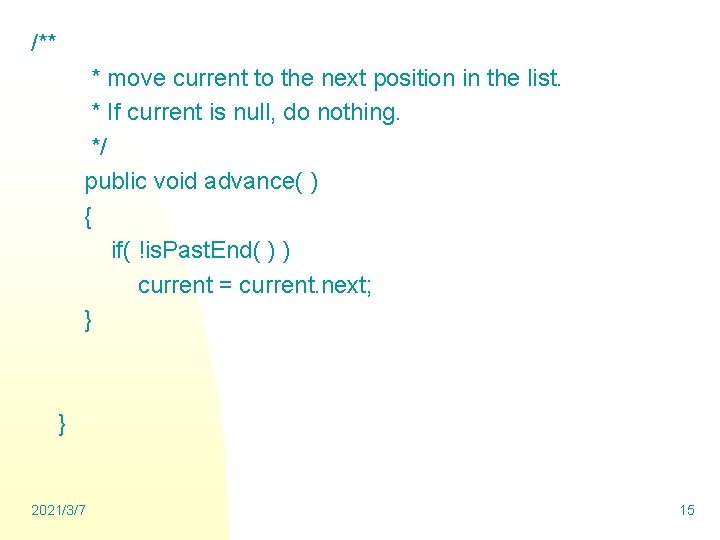
/** * move current to the next position in the list. * If current is null, do nothing. */ public void advance( ) { if( !is. Past. End( ) ) current = current. next; } } 2021/3/7 15
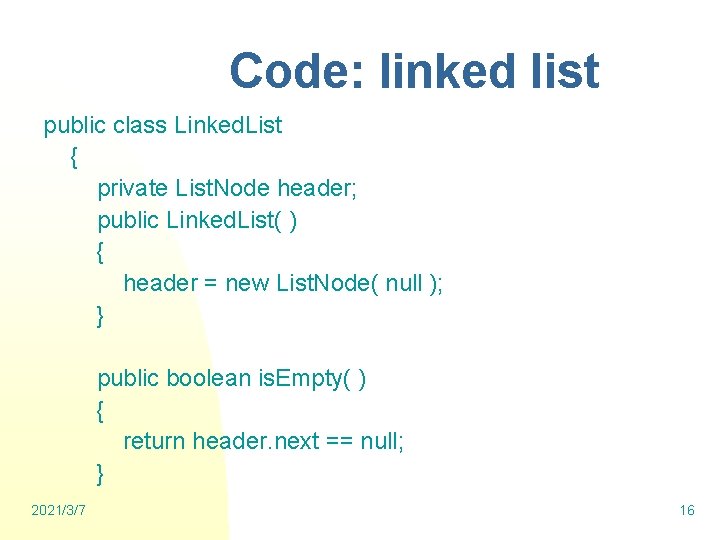
Code: linked list public class Linked. List { private List. Node header; public Linked. List( ) { header = new List. Node( null ); } public boolean is. Empty( ) { return header. next == null; } 2021/3/7 16
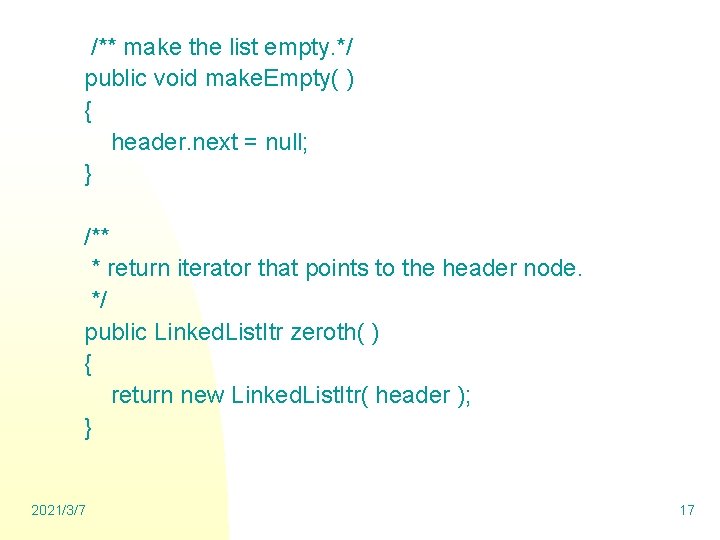
/** make the list empty. */ public void make. Empty( ) { header. next = null; } /** * return iterator that points to the header node. */ public Linked. List. Itr zeroth( ) { return new Linked. List. Itr( header ); } 2021/3/7 17
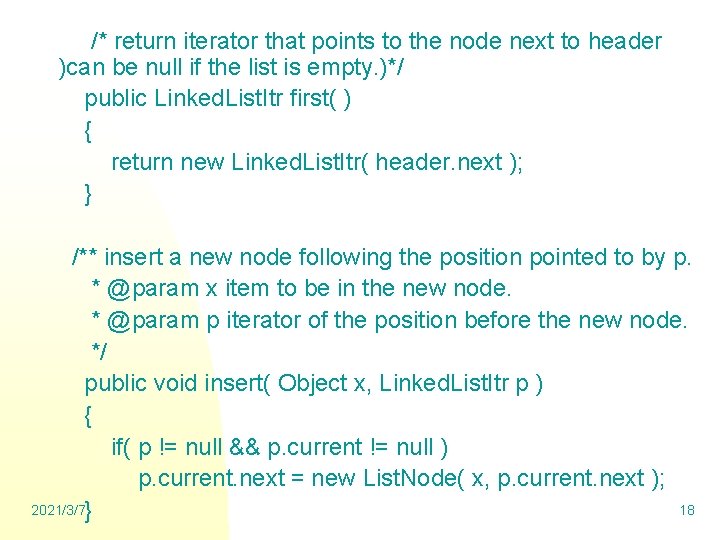
/* return iterator that points to the node next to header )can be null if the list is empty. )*/ public Linked. List. Itr first( ) { return new Linked. List. Itr( header. next ); } /** insert a new node following the position pointed to by p. * @param x item to be in the new node. * @param p iterator of the position before the new node. */ public void insert( Object x, Linked. List. Itr p ) { if( p != null && p. current != null ) p. current. next = new List. Node( x, p. current. next ); 2021/3/7} 18
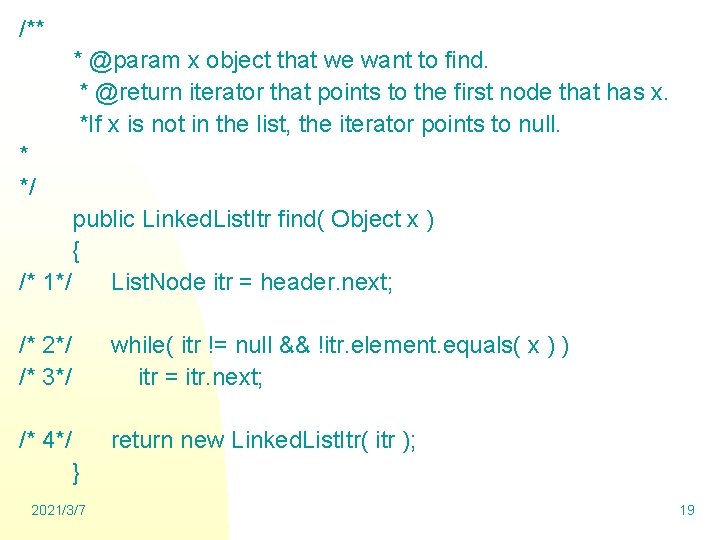
/** * @param x object that we want to find. * @return iterator that points to the first node that has x. *If x is not in the list, the iterator points to null. * */ public Linked. List. Itr find( Object x ) { /* 1*/ List. Node itr = header. next; /* 2*/ /* 3*/ while( itr != null && !itr. element. equals( x ) ) itr = itr. next; /* 4*/ return new Linked. List. Itr( itr ); } 2021/3/7 19
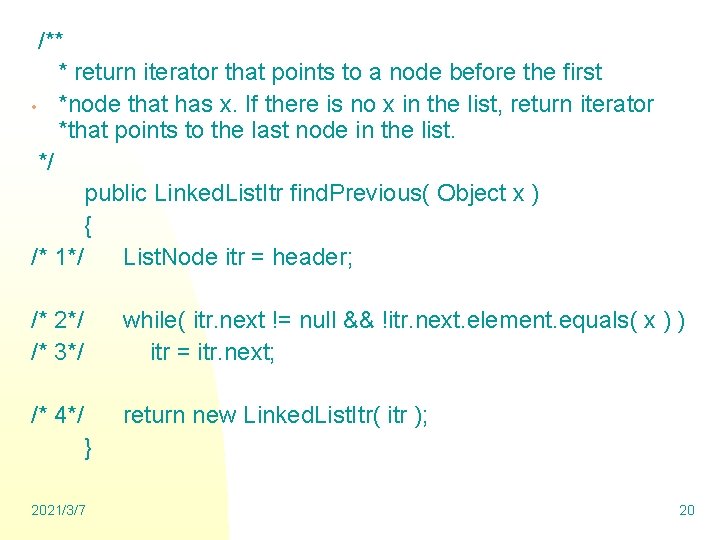
/** * return iterator that points to a node before the first • *node that has x. If there is no x in the list, return iterator *that points to the last node in the list. */ public Linked. List. Itr find. Previous( Object x ) { /* 1*/ List. Node itr = header; /* 2*/ /* 3*/ while( itr. next != null && !itr. next. element. equals( x ) ) itr = itr. next; /* 4*/ return new Linked. List. Itr( itr ); } 2021/3/7 20
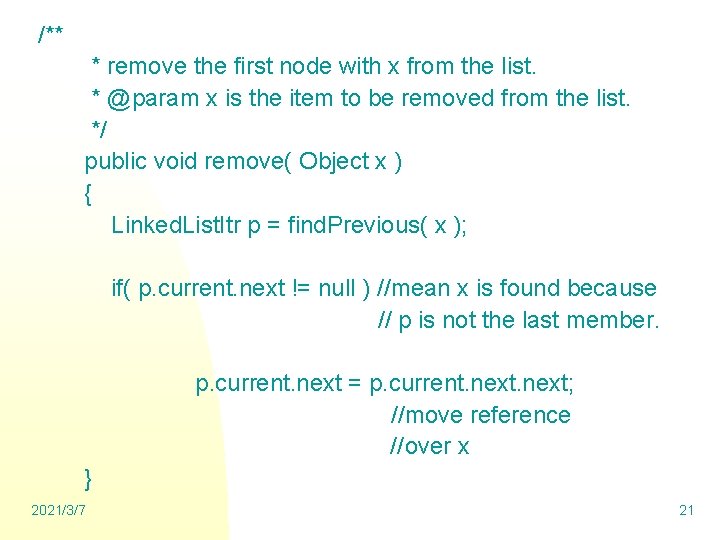
/** * remove the first node with x from the list. * @param x is the item to be removed from the list. */ public void remove( Object x ) { Linked. List. Itr p = find. Previous( x ); if( p. current. next != null ) //mean x is found because // p is not the last member. p. current. next = p. current. next; //move reference //over x } 2021/3/7 21
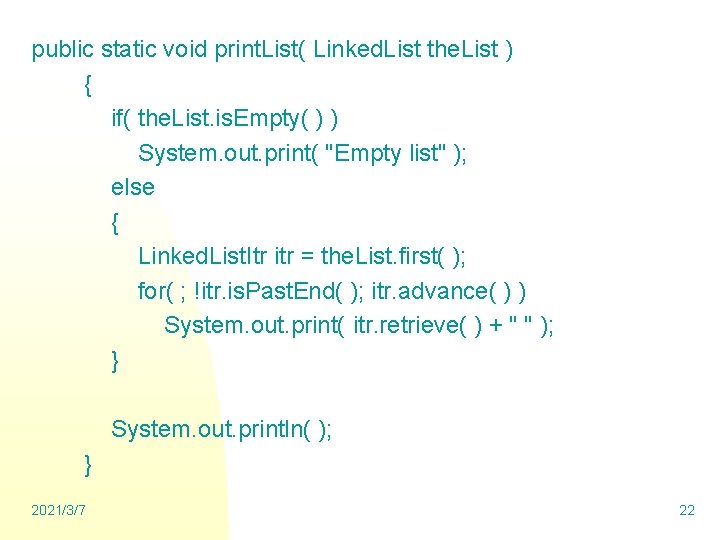
public static void print. List( Linked. List the. List ) { if( the. List. is. Empty( ) ) System. out. print( "Empty list" ); else { Linked. List. Itr itr = the. List. first( ); for( ; !itr. is. Past. End( ); itr. advance( ) ) System. out. print( itr. retrieve( ) + " " ); } System. out. println( ); } 2021/3/7 22
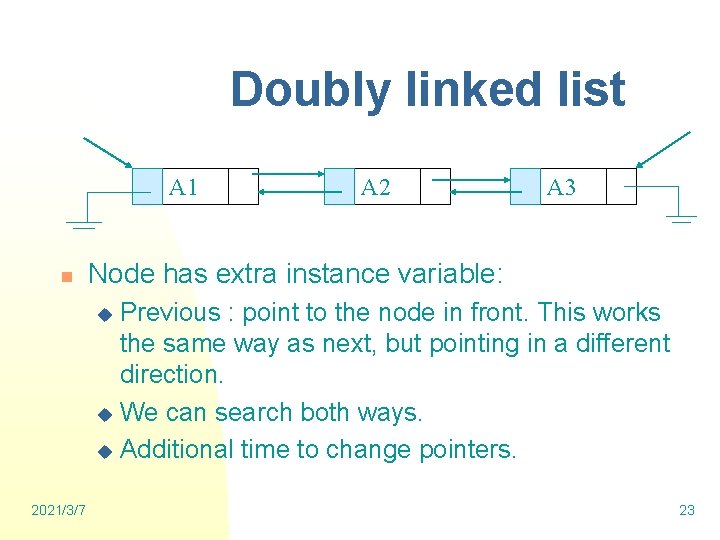
Doubly linked list A 1 n A 2 A 3 Node has extra instance variable: Previous : point to the node in front. This works the same way as next, but pointing in a different direction. u We can search both ways. u Additional time to change pointers. u 2021/3/7 23
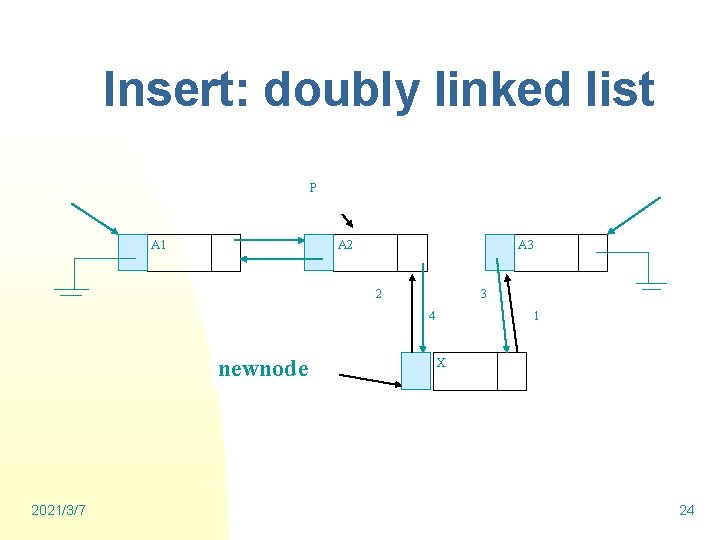
Insert: doubly linked list P A 1 A 2 A 3 2 3 4 newnode 2021/3/7 1 X 24
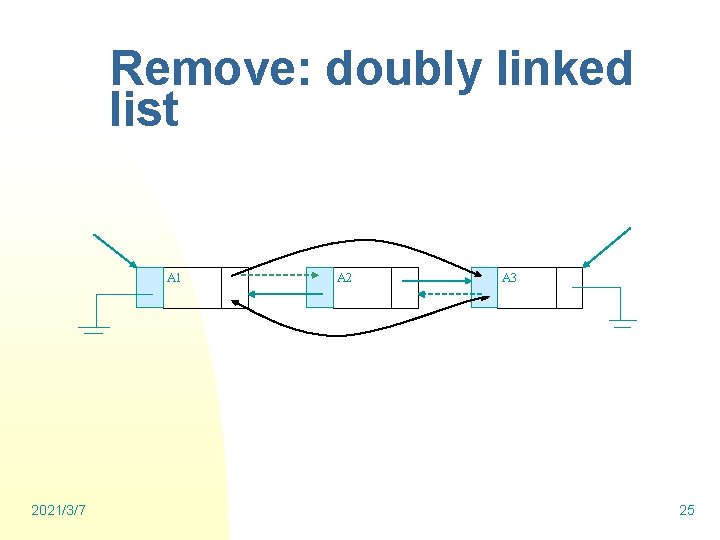
Remove: doubly linked list A 1 2021/3/7 A 2 A 3 25
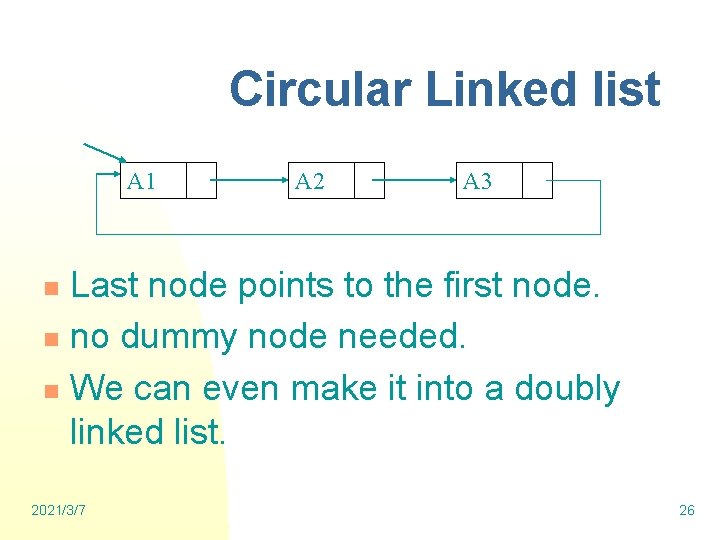
Circular Linked list A 1 A 2 A 3 Last node points to the first node. n no dummy node needed. n We can even make it into a doubly linked list. n 2021/3/7 26
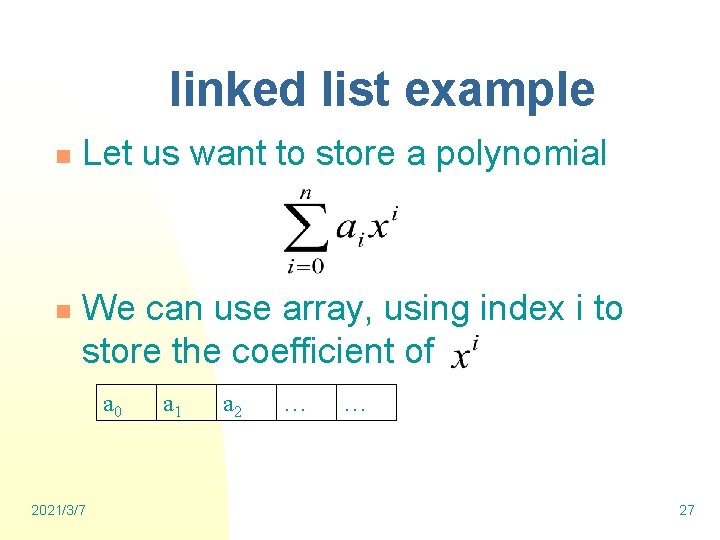
linked list example n n Let us want to store a polynomial We can use array, using index i to store the coefficient of a 0 2021/3/7 a 1 a 2 … … 27
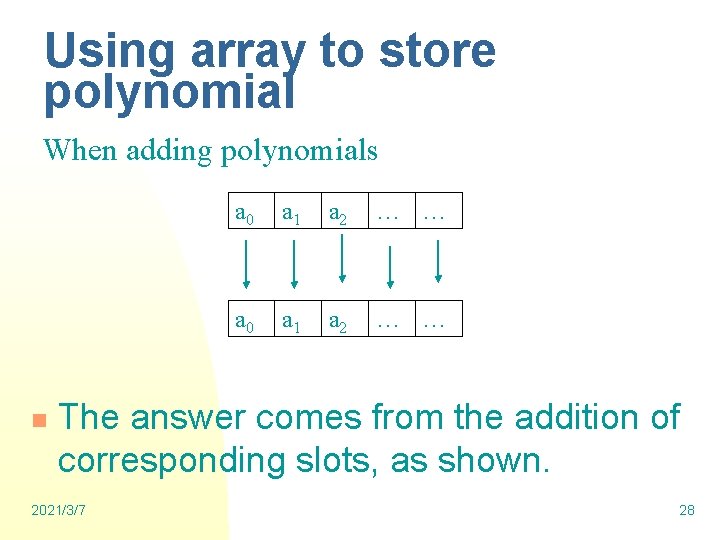
Using array to store polynomial When adding polynomials n a 0 a 1 a 2 … … The answer comes from the addition of corresponding slots, as shown. 2021/3/7 28
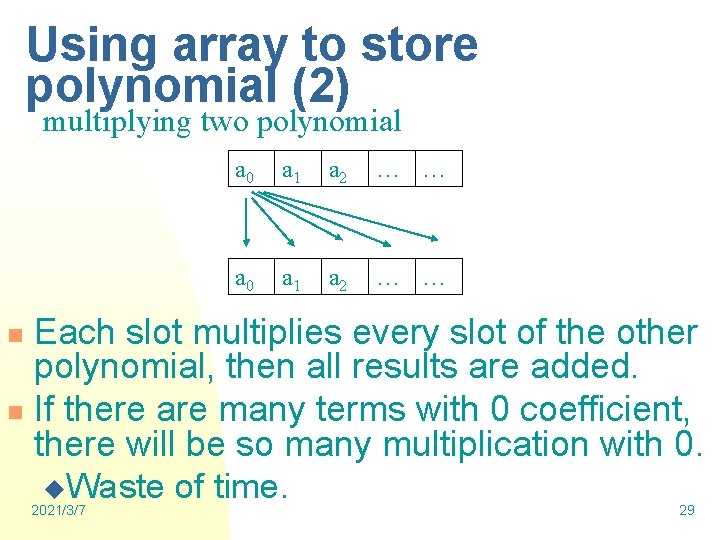
Using array to store polynomial (2) multiplying two polynomial a 0 a 1 a 2 … … Each slot multiplies every slot of the other polynomial, then all results are added. n If there are many terms with 0 coefficient, there will be so many multiplication with 0. u. Waste of time. n 2021/3/7 29
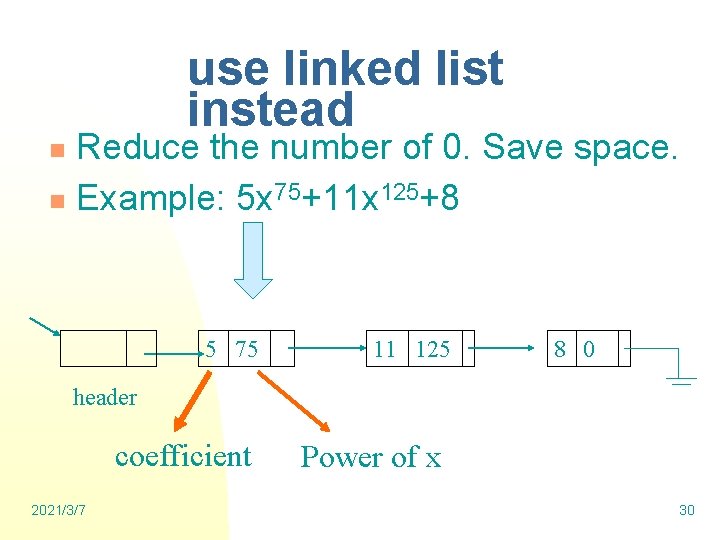
use linked list instead Reduce the number of 0. Save space. n Example: 5 x 75+11 x 125+8 n 5 75 11 125 8 0 header coefficient 2021/3/7 Power of x 30
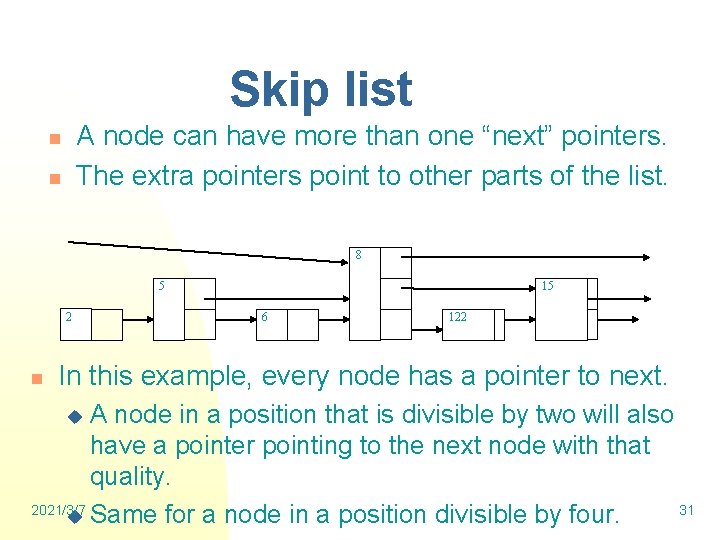
Skip list A node can have more than one “next” pointers. The extra pointers point to other parts of the list. n n 8 5 2 n 15 6 122 In this example, every node has a pointer to next. A node in a position that is divisible by two will also have a pointer pointing to the next node with that quality. 2021/3/7 31 u Same for a node in a position divisible by four. u
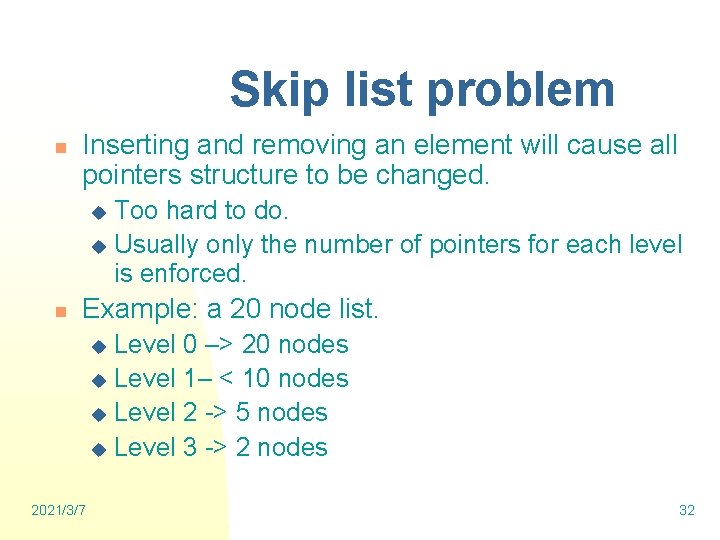
Skip list problem n Inserting and removing an element will cause all pointers structure to be changed. Too hard to do. u Usually only the number of pointers for each level is enforced. u n Example: a 20 node list. Level 0 –> 20 nodes u Level 1– < 10 nodes u Level 2 -> 5 nodes u Level 3 -> 2 nodes u 2021/3/7 32
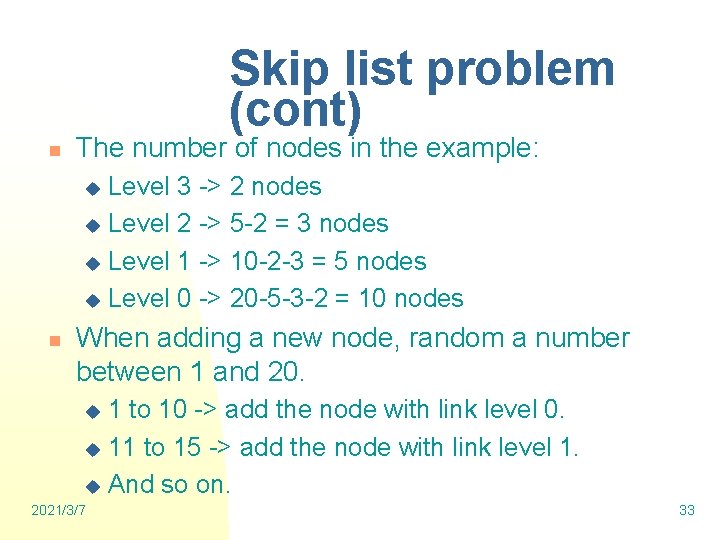
Skip list problem (cont) n The number of nodes in the example: Level 3 -> 2 nodes u Level 2 -> 5 -2 = 3 nodes u Level 1 -> 10 -2 -3 = 5 nodes u Level 0 -> 20 -5 -3 -2 = 10 nodes u n When adding a new node, random a number between 1 and 20. 1 to 10 -> add the node with link level 0. u 11 to 15 -> add the node with link level 1. u And so on. u 2021/3/7 33
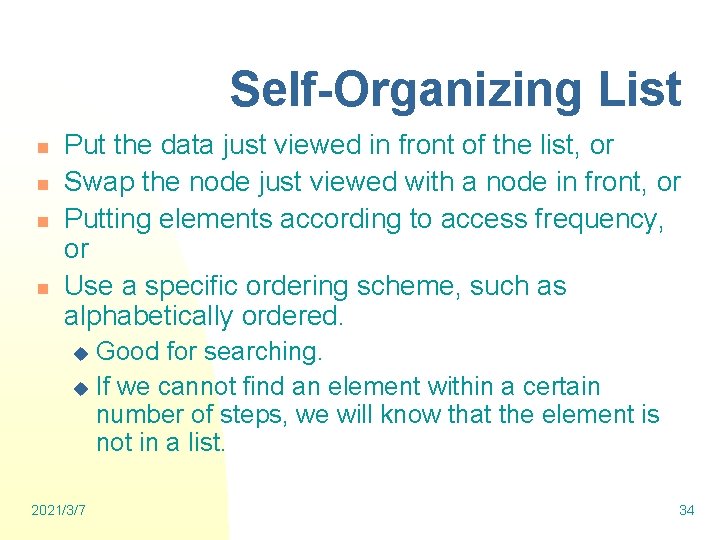
Self-Organizing List n n Put the data just viewed in front of the list, or Swap the node just viewed with a node in front, or Putting elements according to access frequency, or Use a specific ordering scheme, such as alphabetically ordered. Good for searching. u If we cannot find an element within a certain number of steps, we will know that the element is not in a list. u 2021/3/7 34
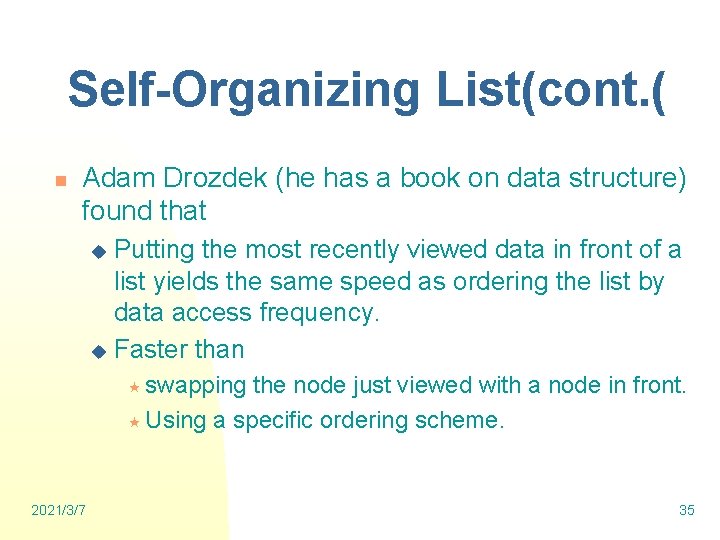
Self-Organizing List(cont. ( n Adam Drozdek (he has a book on data structure) found that Putting the most recently viewed data in front of a list yields the same speed as ordering the list by data access frequency. u Faster than u « swapping the node just viewed with a node in front. « Using a specific ordering scheme. 2021/3/7 35
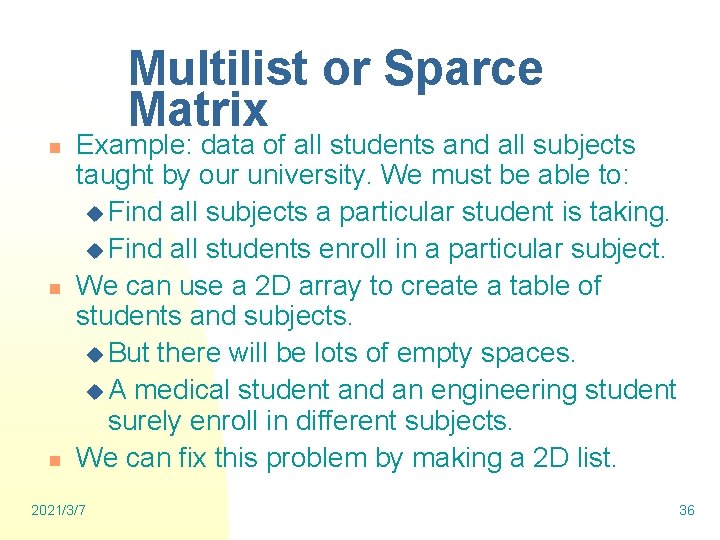
Multilist or Sparce Matrix n n n Example: data of all students and all subjects taught by our university. We must be able to: u Find all subjects a particular student is taking. u Find all students enroll in a particular subject. We can use a 2 D array to create a table of students and subjects. u But there will be lots of empty spaces. u A medical student and an engineering student surely enroll in different subjects. We can fix this problem by making a 2 D list. 2021/3/7 36
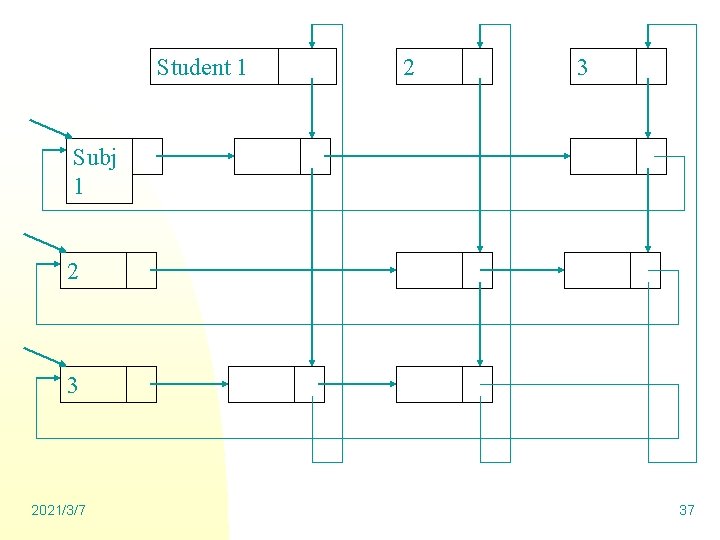
Student 1 2 3 Subj 1 2 3 2021/3/7 37
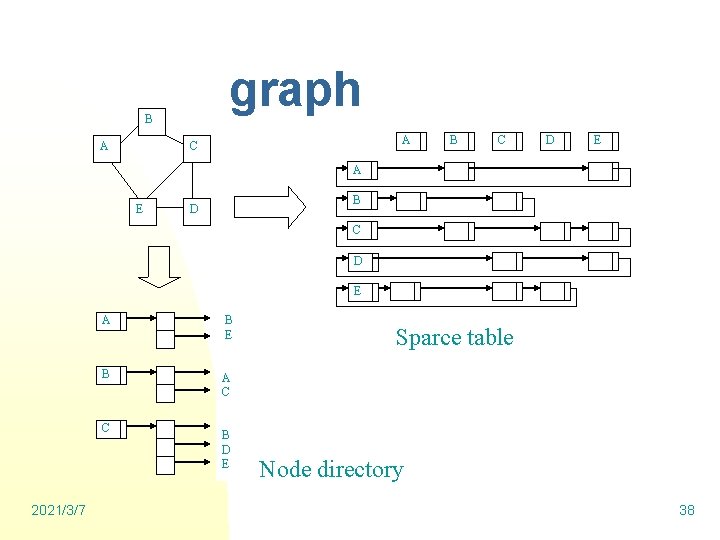
graph B A A C B C D E A E B D C D E A B E B A C C 2021/3/7 B D E Sparce table Node directory 38
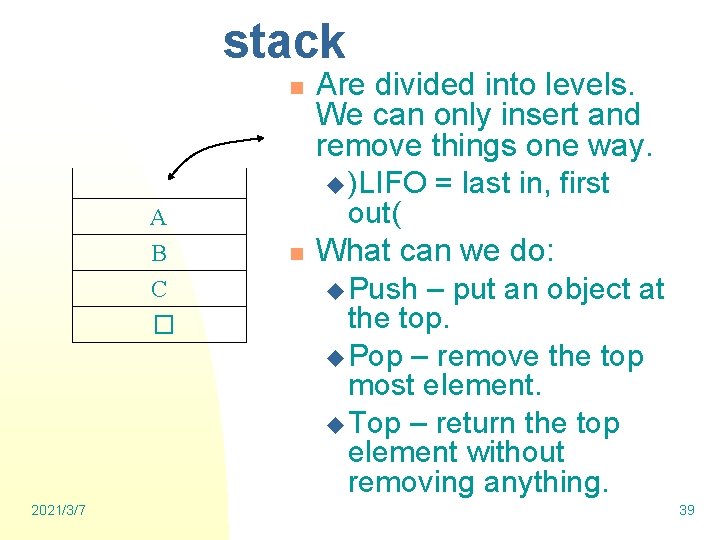
stack n A B C � 2021/3/7 n Are divided into levels. We can only insert and remove things one way. u )LIFO = last in, first out( What can we do: u Push – put an object at the top. u Pop – remove the top most element. u Top – return the top element without removing anything. 39
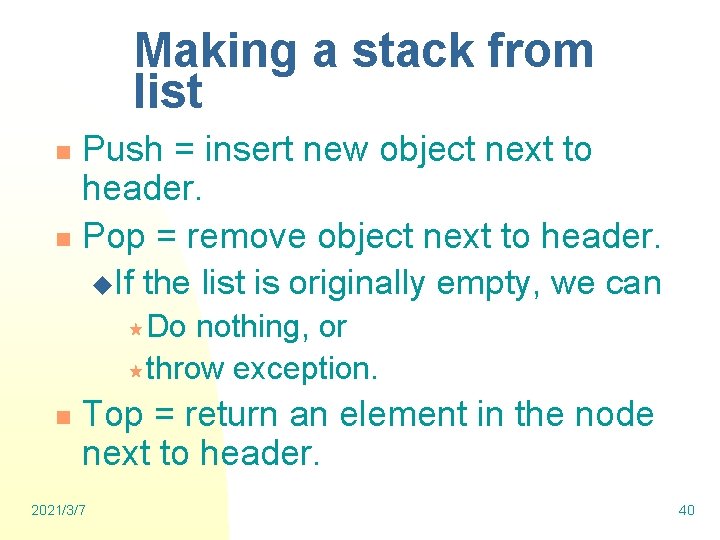
Making a stack from list Push = insert new object next to header. n Pop = remove object next to header. u. If the list is originally empty, we can n «Do nothing, or «throw exception. n Top = return an element in the node next to header. 2021/3/7 40
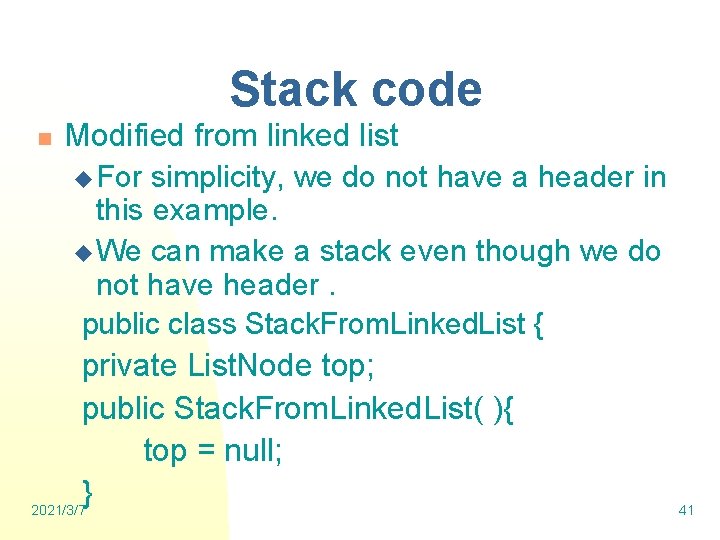
Stack code n Modified from linked list u For simplicity, we do not have a header in this example. u We can make a stack even though we do not have header. public class Stack. From. Linked. List { private List. Node top; public Stack. From. Linked. List( ){ top = null; } 2021/3/7 41
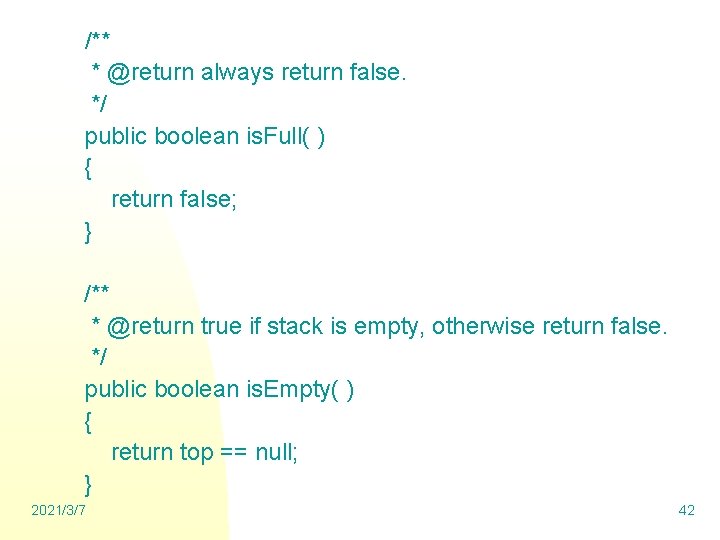
/** * @return always return false. */ public boolean is. Full( ) { return false; } /** * @return true if stack is empty, otherwise return false. */ public boolean is. Empty( ) { return top == null; } 2021/3/7 42
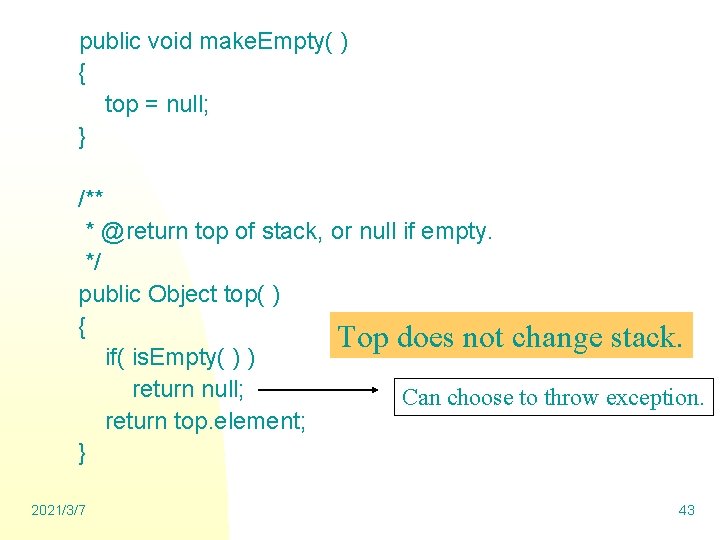
public void make. Empty( ) { top = null; } /** * @return top of stack, or null if empty. */ public Object top( ) { Top does not change stack. if( is. Empty( ) ) return null; Can choose to throw exception. return top. element; } 2021/3/7 43
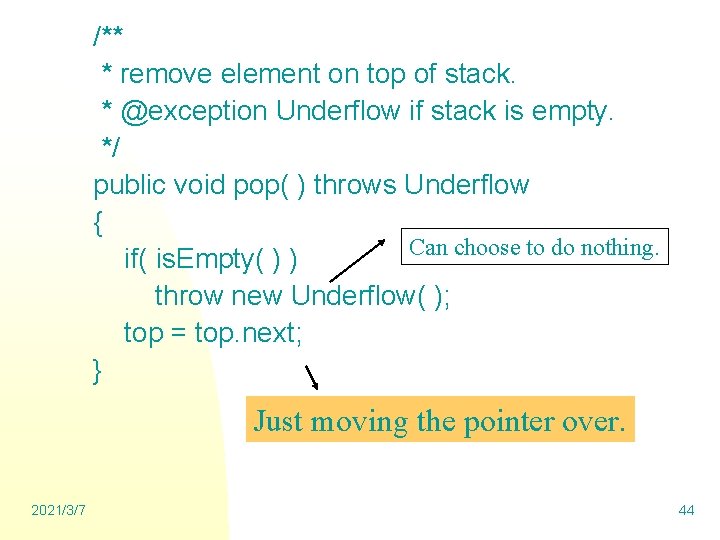
/** * remove element on top of stack. * @exception Underflow if stack is empty. */ public void pop( ) throws Underflow { Can choose to do nothing. if( is. Empty( ) ) throw new Underflow( ); top = top. next; } Just moving the pointer over. 2021/3/7 44
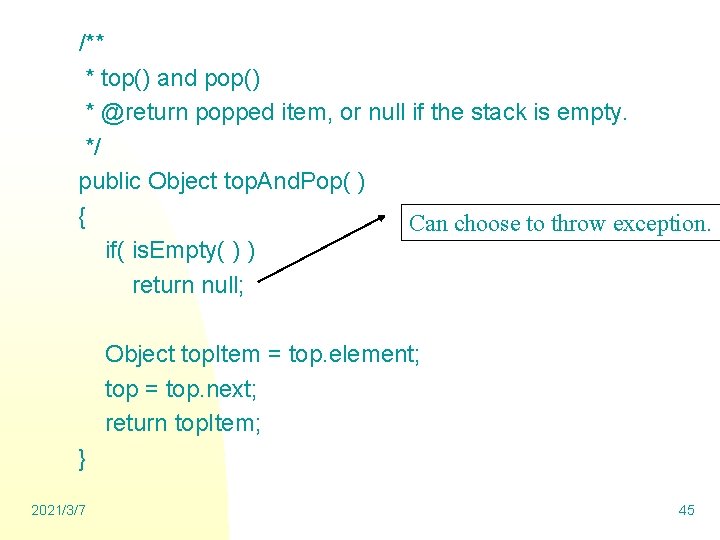
/** * top() and pop() * @return popped item, or null if the stack is empty. */ public Object top. And. Pop( ) { Can choose to throw exception. if( is. Empty( ) ) return null; Object top. Item = top. element; top = top. next; return top. Item; } 2021/3/7 45
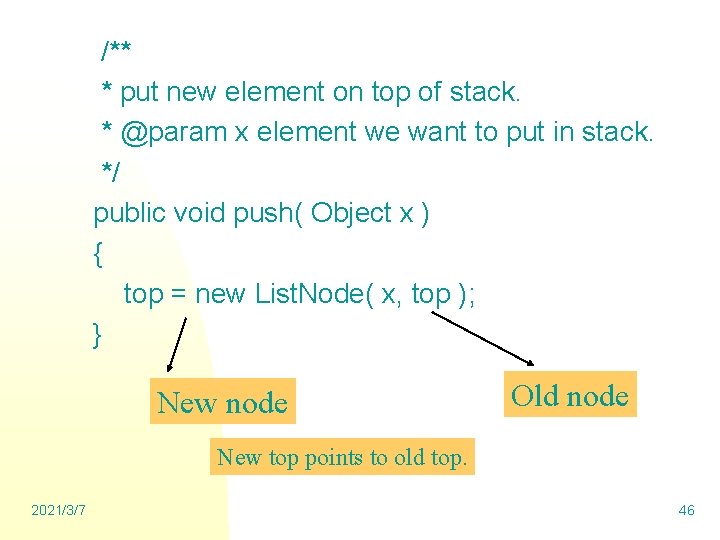
/** * put new element on top of stack. * @param x element we want to put in stack. */ public void push( Object x ) { top = new List. Node( x, top ); } New node Old node New top points to old top. 2021/3/7 46
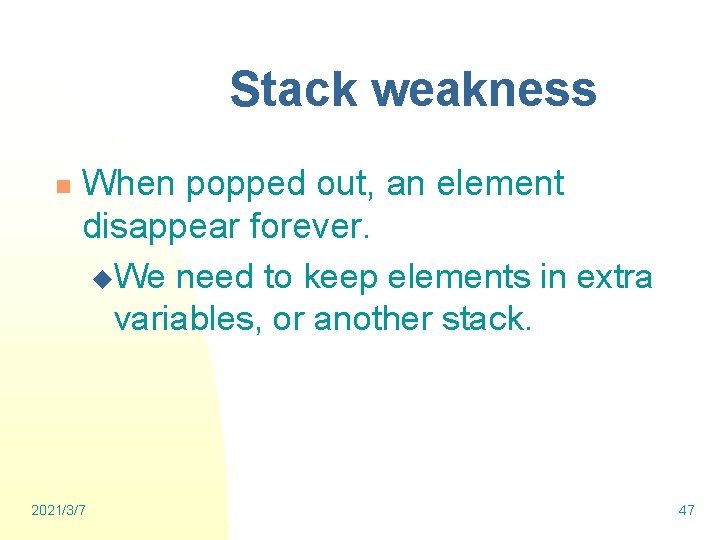
Stack weakness n When popped out, an element disappear forever. u. We need to keep elements in extra variables, or another stack. 2021/3/7 47
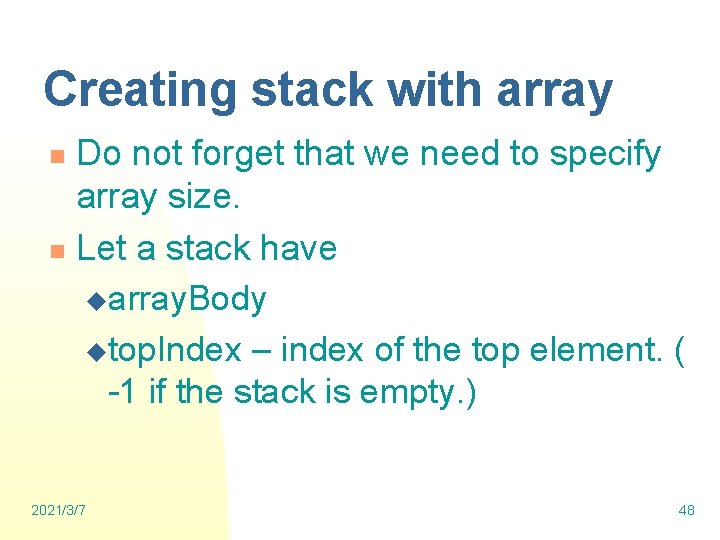
Creating stack with array Do not forget that we need to specify array size. n Let a stack have uarray. Body utop. Index – index of the top element. ( -1 if the stack is empty. ) n 2021/3/7 48
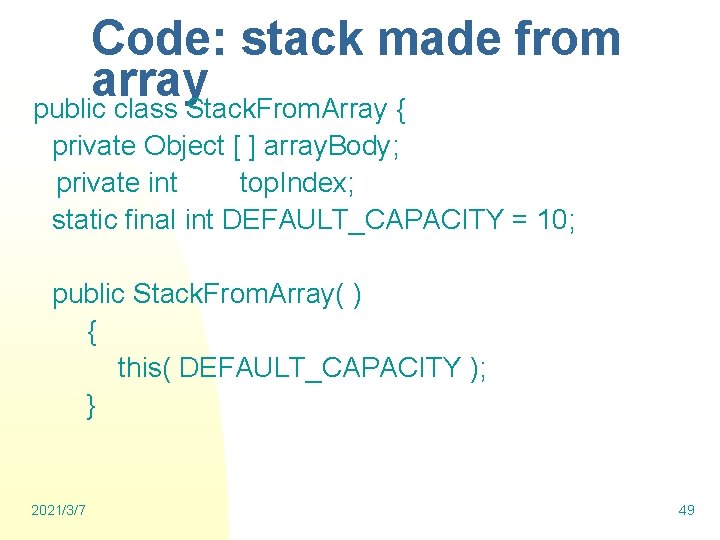
Code: stack made from array public class Stack. From. Array { private Object [ ] array. Body; private int top. Index; static final int DEFAULT_CAPACITY = 10; public Stack. From. Array( ) { this( DEFAULT_CAPACITY ); } 2021/3/7 49
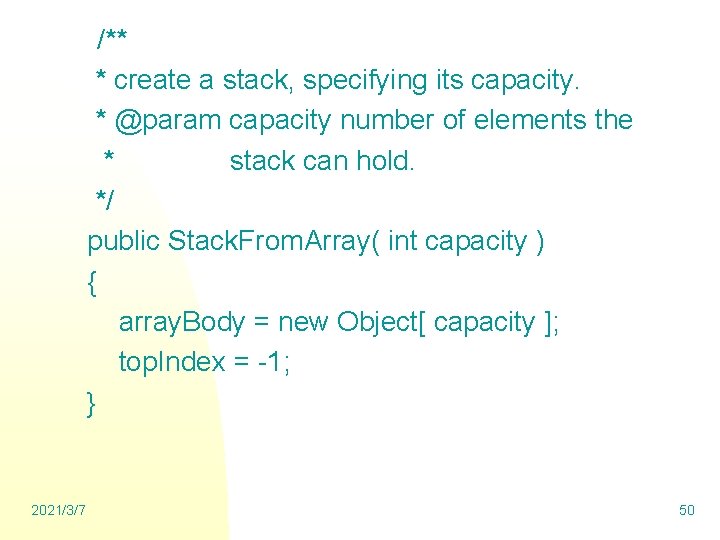
/** * create a stack, specifying its capacity. * @param capacity number of elements the * stack can hold. */ public Stack. From. Array( int capacity ) { array. Body = new Object[ capacity ]; top. Index = -1; } 2021/3/7 50
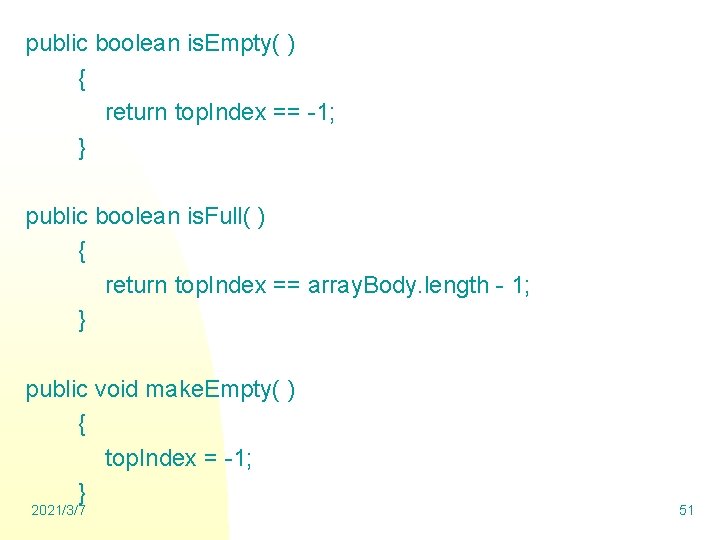
public boolean is. Empty( ) { return top. Index == -1; } public boolean is. Full( ) { return top. Index == array. Body. length - 1; } public void make. Empty( ) { top. Index = -1; } 2021/3/7 51
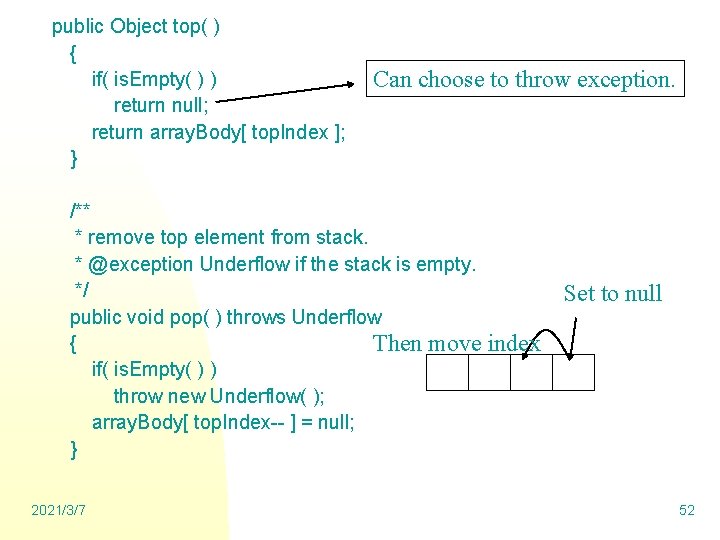
public Object top( ) { if( is. Empty( ) ) return null; return array. Body[ top. Index ]; } Can choose to throw exception. /** * remove top element from stack. * @exception Underflow if the stack is empty. */ public void pop( ) throws Underflow { Then move if( is. Empty( ) ) throw new Underflow( ); array. Body[ top. Index-- ] = null; } 2021/3/7 Set to null index 52
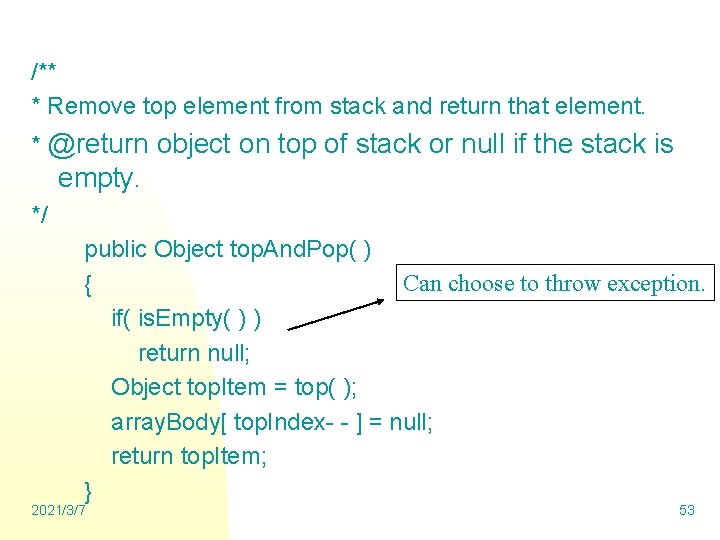
/** * Remove top element from stack and return that element. * @return object on top of stack or null if the stack is empty. */ public Object top. And. Pop( ) Can choose to throw exception. { if( is. Empty( ) ) return null; Object top. Item = top( ); array. Body[ top. Index- - ] = null; return top. Item; } 2021/3/7 53
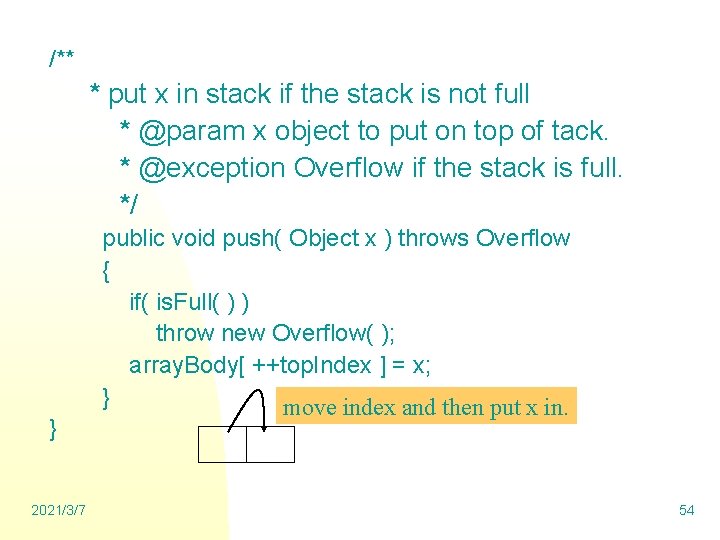
/** * put x in stack if the stack is not full * @param x object to put on top of tack. * @exception Overflow if the stack is full. */ } 2021/3/7 public void push( Object x ) throws Overflow { if( is. Full( ) ) throw new Overflow( ); array. Body[ ++top. Index ] = x; } move index and then put x in. 54
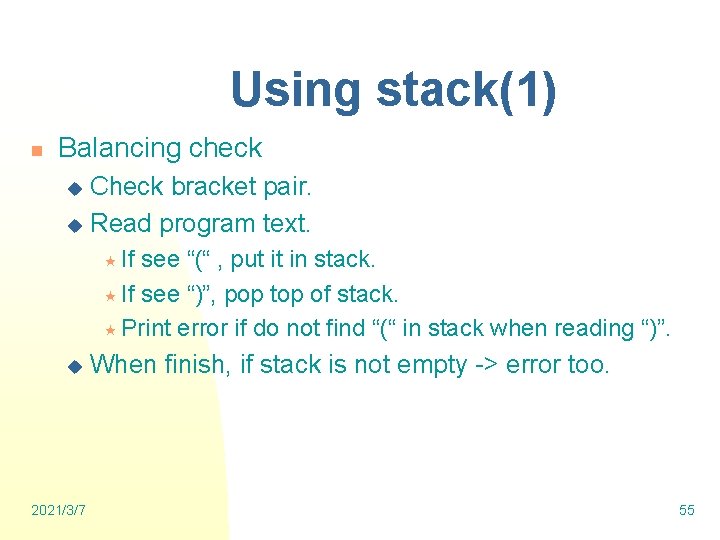
Using stack(1) n Balancing check Check bracket pair. u Read program text. u « If see “(“ , put it in stack. « If see “)”, pop top of stack. « Print error if do not find “(“ in stack when reading “)”. u 2021/3/7 When finish, if stack is not empty -> error too. 55
![Using stack2 n Postfix expression reverse polish 789510 7 8 9 5 Using stack(2) n Postfix expression (reverse polish) [7+(8*9)+5]*10 = 7 8 9 * 5](https://slidetodoc.com/presentation_image_h/2ab4e1ea4ad3f8d8d7079ac0166ebdd9/image-56.jpg)
Using stack(2) n Postfix expression (reverse polish) [7+(8*9)+5]*10 = 7 8 9 * 5 + + 10 * u We can use stack to evaluate a postfix expression: u « Read a number -> push to stack. « Read an operator -> pop numbers in the stack and use the operator on the numbers. 9 8 7 2021/3/7 Example: reading the first three numbers. 56
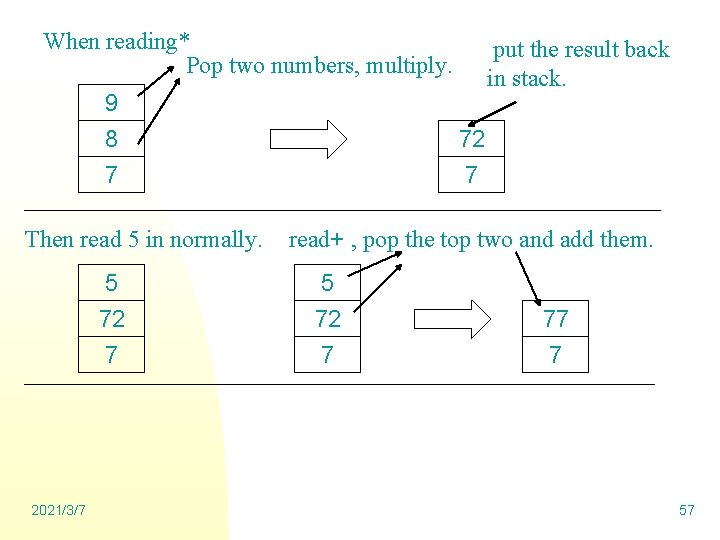
When reading* Pop two numbers, multiply. 9 8 72 7 7 Then read 5 in normally. 5 72 7 2021/3/7 put the result back in stack. read+ , pop the top two and add them. 5 72 7 77 7 57
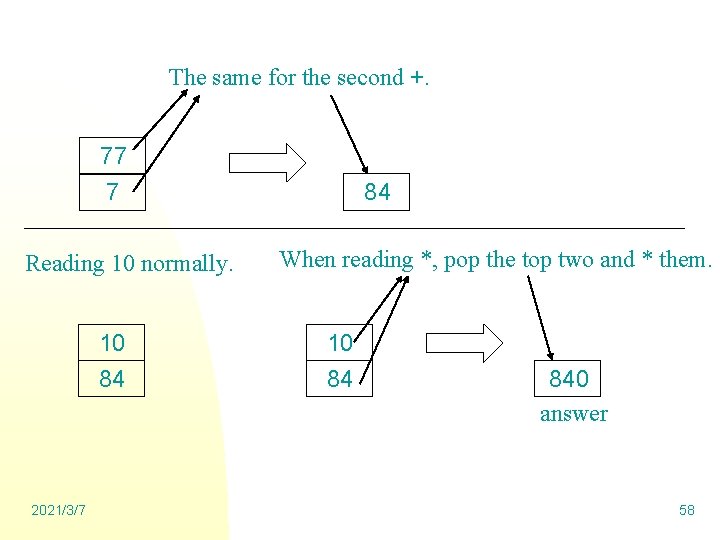
The same for the second +. 77 7 Reading 10 normally. 10 84 2021/3/7 84 When reading *, pop the top two and * them. 10 84 840 answer 58
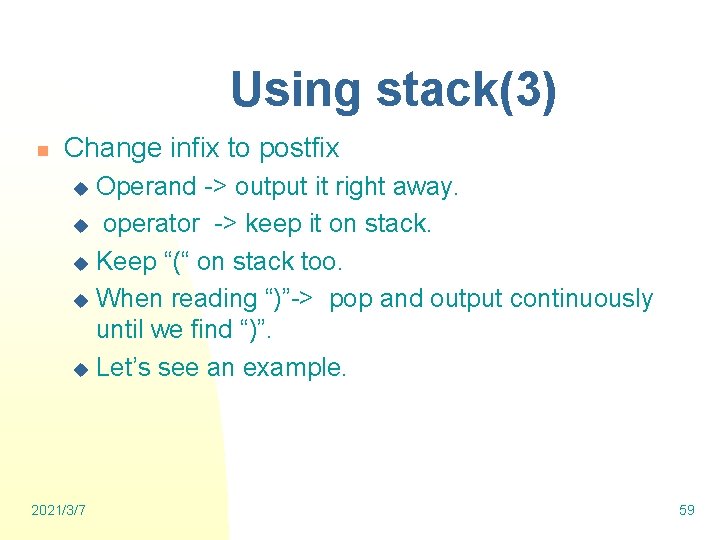
Using stack(3) n Change infix to postfix Operand -> output it right away. u operator -> keep it on stack. u Keep “(“ on stack too. u When reading “)”-> pop and output continuously until we find “)”. u Let’s see an example. u 2021/3/7 59
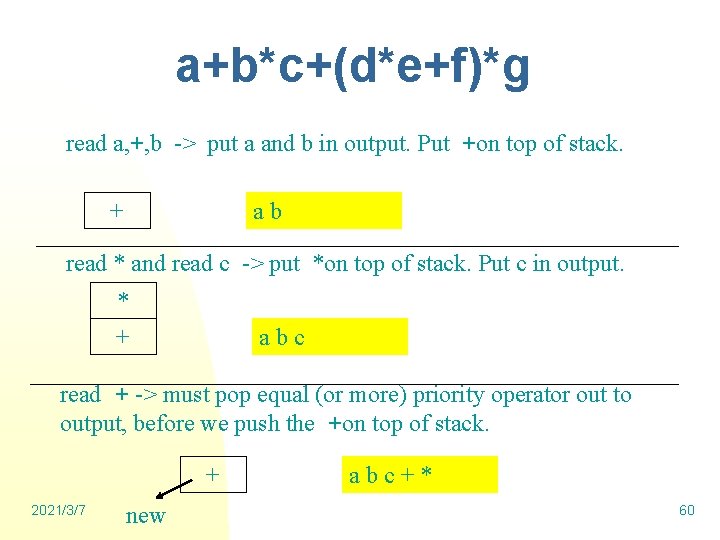
a+b*c+(d*e+f)*g read a, +, b -> put a and b in output. Put +on top of stack. + ab read * and read c -> put *on top of stack. Put c in output. * + abc read + -> must pop equal (or more) priority operator out to output, before we push the +on top of stack. + 2021/3/7 new abc+* 60
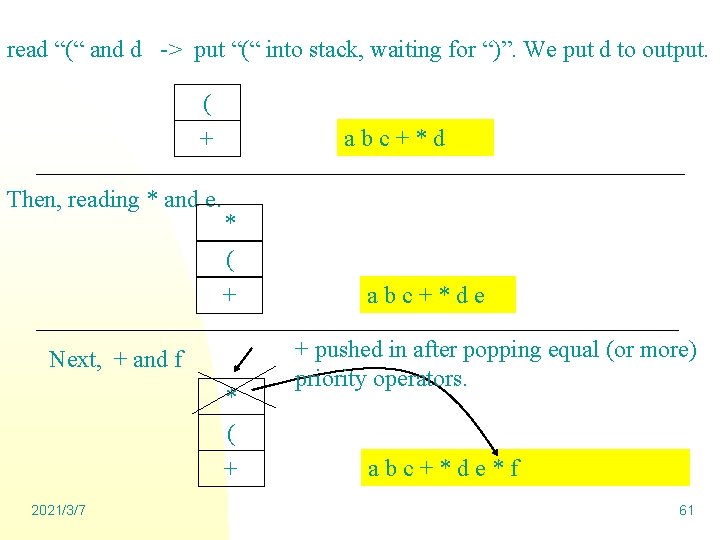
read “(“ and d -> put “(“ into stack, waiting for “)”. We put d to output. ( + Then, reading * and e. abc+*d * ( + Next, + and f * ( + 2021/3/7 abc+*de + pushed in after popping equal (or more) priority operators. abc+*de*f 61
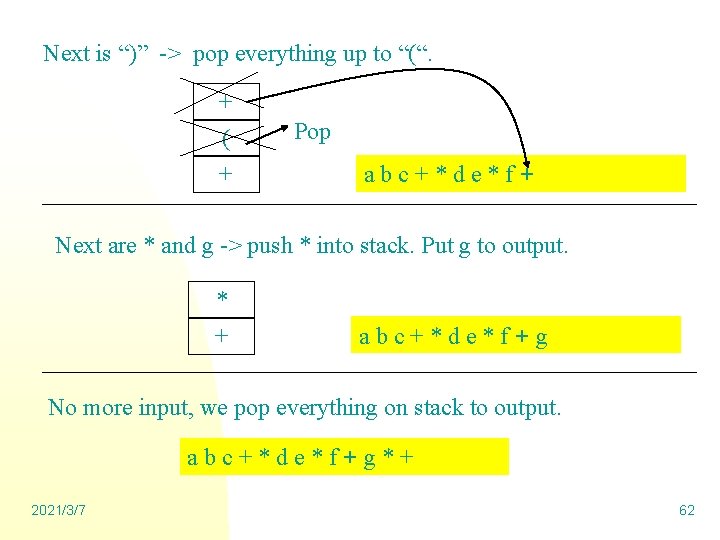
Next is “)” -> pop everything up to “(“. + ( + Pop abc+*de*f+ Next are * and g -> push * into stack. Put g to output. * + abc+*de*f+g No more input, we pop everything on stack to output. abc+*de*f+g*+ 2021/3/7 62
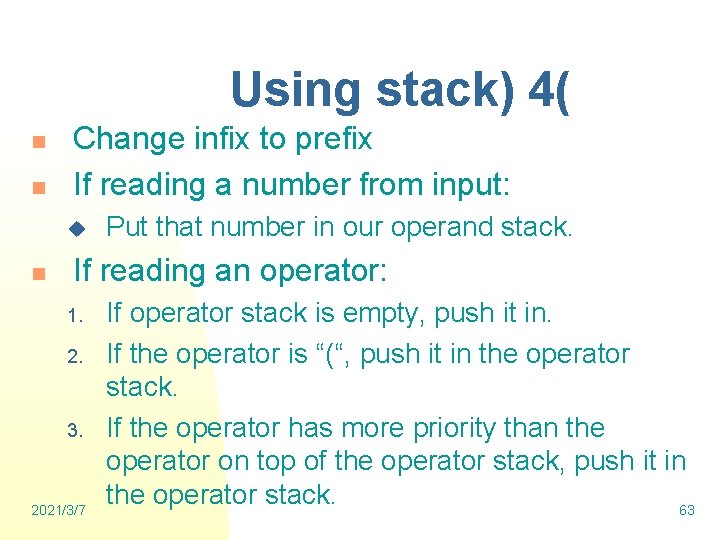
Using stack) 4( n n Change infix to prefix If reading a number from input: u n Put that number in our operand stack. If reading an operator: 1. 2. 3. 2021/3/7 If operator stack is empty, push it in. If the operator is “(“, push it in the operator stack. If the operator has more priority than the operator on top of the operator stack, push it in the operator stack. 63
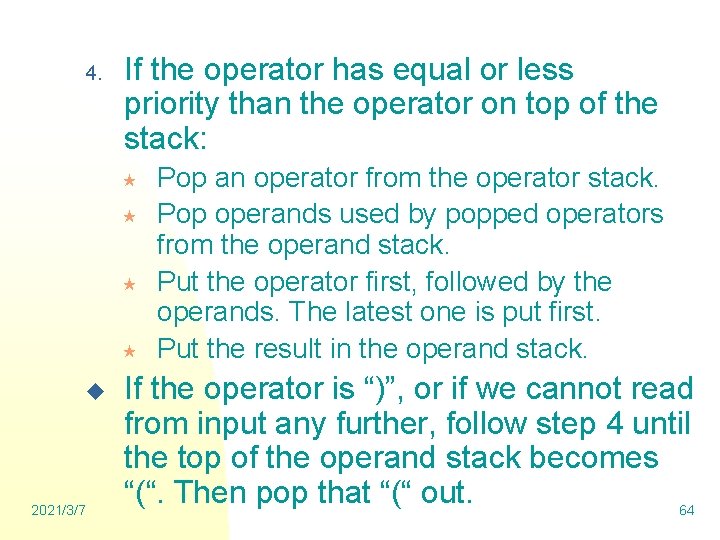
4. If the operator has equal or less priority than the operator on top of the stack: « « u 2021/3/7 Pop an operator from the operator stack. Pop operands used by popped operators from the operand stack. Put the operator first, followed by the operands. The latest one is put first. Put the result in the operand stack. If the operator is “)”, or if we cannot read from input any further, follow step 4 until the top of the operand stack becomes “(“. Then pop that “(“ out. 64
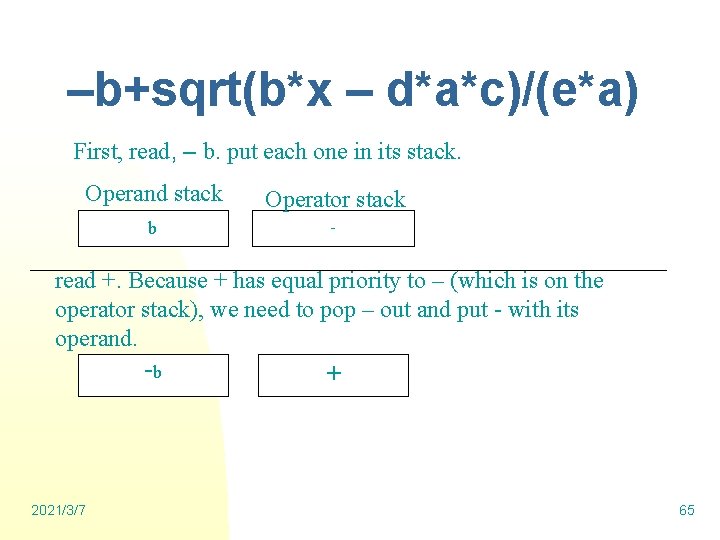
–b+sqrt(b*x – d*a*c)/(e*a) First, read, – b. put each one in its stack. Operand stack b Operator stack - read +. Because + has equal priority to – (which is on the operator stack), we need to pop – out and put - with its operand. -b 2021/3/7 + 65
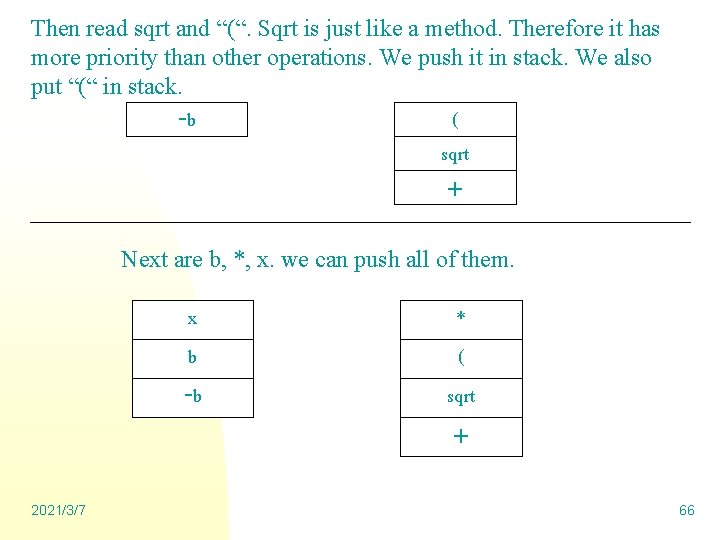
Then read sqrt and “(“. Sqrt is just like a method. Therefore it has more priority than other operations. We push it in stack. We also put “(“ in stack. -b ( sqrt + Next are b, *, x. we can push all of them. x b -b * ( sqrt + 2021/3/7 66
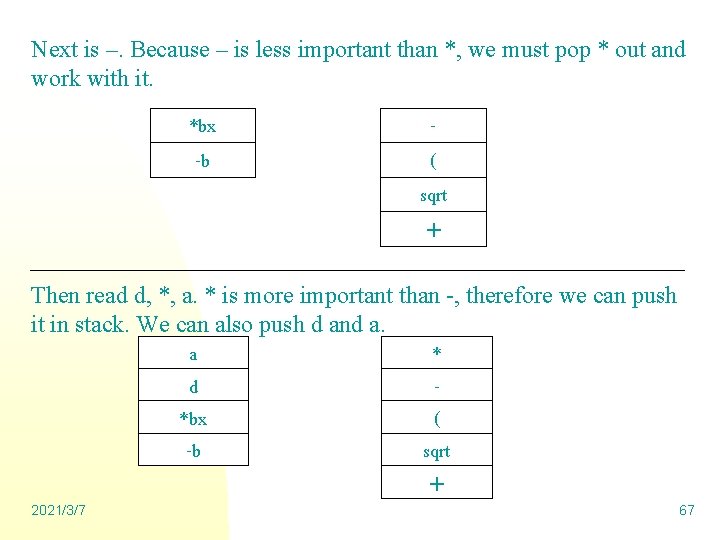
Next is –. Because – is less important than *, we must pop * out and work with it. *bx -b ( sqrt + Then read d, *, a. * is more important than -, therefore we can push it in stack. We can also push d and a. a d *bx -b 2021/3/7 * ( sqrt + 67
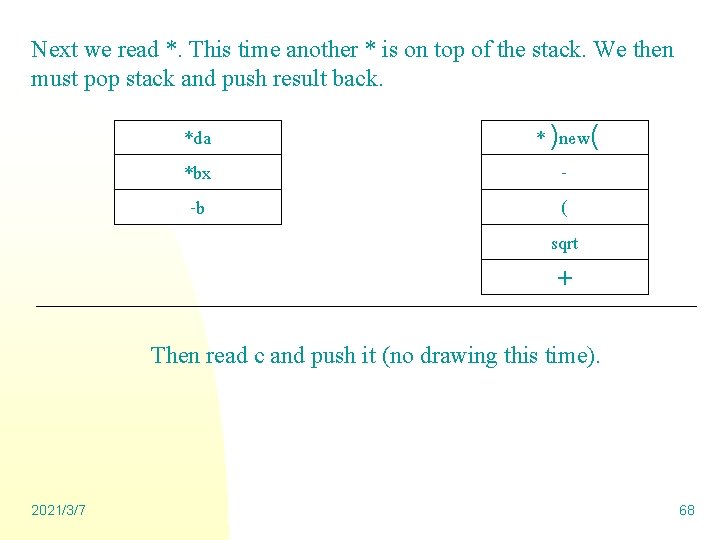
Next we read *. This time another * is on top of the stack. We then must pop stack and push result back. *da *bx -b * )new( ( sqrt + Then read c and push it (no drawing this time). 2021/3/7 68
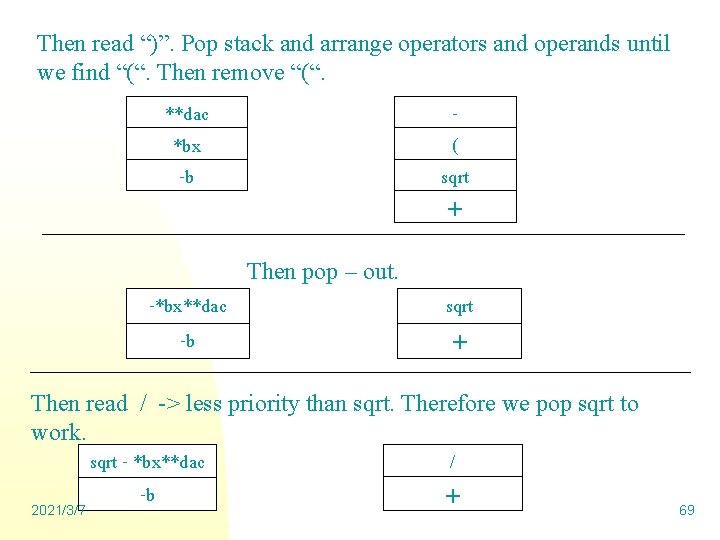
Then read “)”. Pop stack and arrange operators and operands until we find “(“. Then remove “(“. **dac *bx -b ( sqrt + Then pop – out. -*bx**dac -b sqrt + Then read / -> less priority than sqrt. Therefore we pop sqrt to work. 2021/3/7 sqrt - *bx**dac -b / + 69
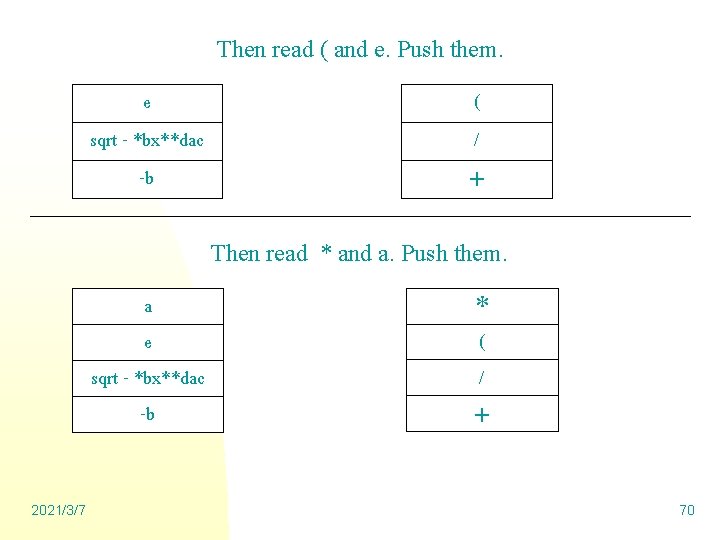
Then read ( and e. Push them. e sqrt - *bx**dac -b ( / + Then read * and a. Push them. a e sqrt - *bx**dac -b 2021/3/7 * ( / + 70
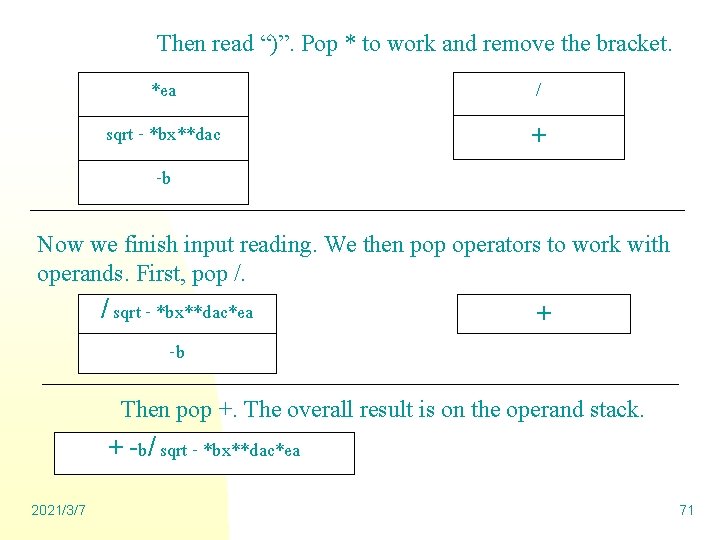
Then read “)”. Pop * to work and remove the bracket. *ea / sqrt - *bx**dac + -b Now we finish input reading. We then pop operators to work with operands. First, pop /. / sqrt - *bx**dac*ea -b + Then pop +. The overall result is on the operand stack. + -b/ sqrt - *bx**dac*ea 2021/3/7 71
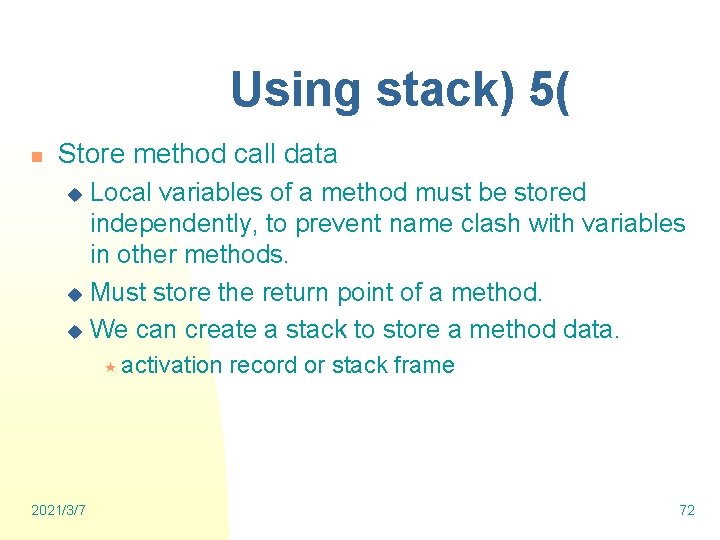
Using stack) 5( n Store method call data Local variables of a method must be stored independently, to prevent name clash with variables in other methods. u Must store the return point of a method. u We can create a stack to store a method data. u « activation 2021/3/7 record or stack frame 72
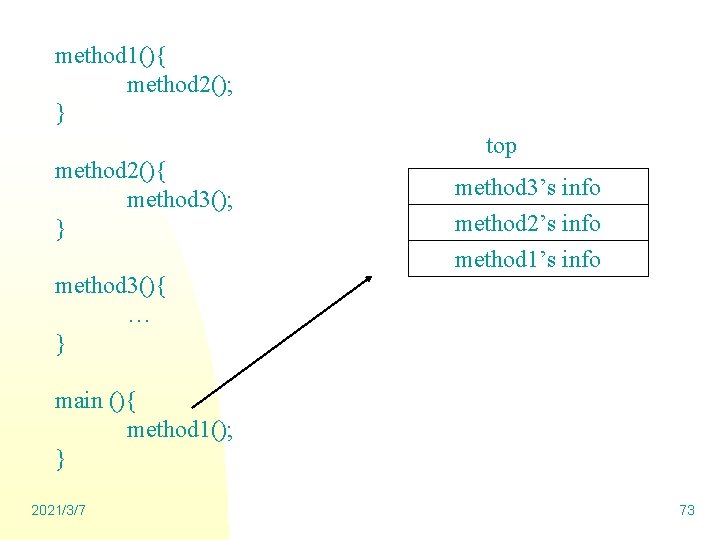
method 1(){ method 2(); } method 2(){ method 3(); } method 3(){ … } top method 3’s info method 2’s info method 1’s info main (){ method 1(); } 2021/3/7 73
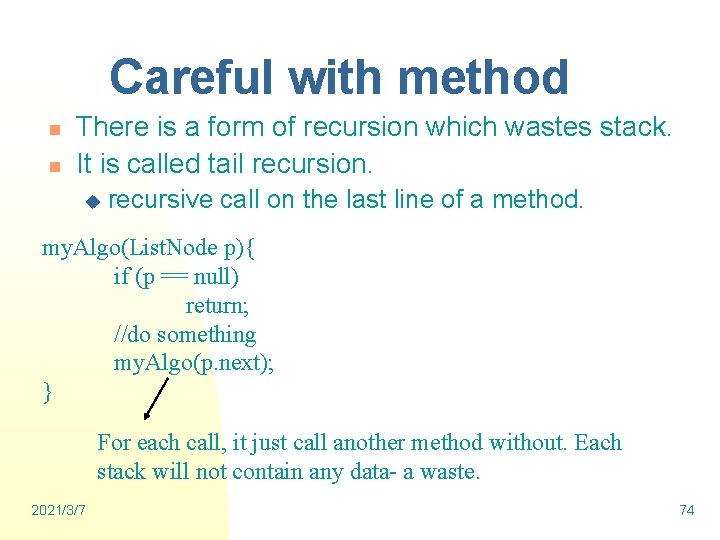
Careful with method n n There is a form of recursion which wastes stack. It is called tail recursion. u recursive call on the last line of a method. my. Algo(List. Node p){ if (p == null) return; //do something my. Algo(p. next); } For each call, it just call another method without. Each stack will not contain any data- a waste. 2021/3/7 74
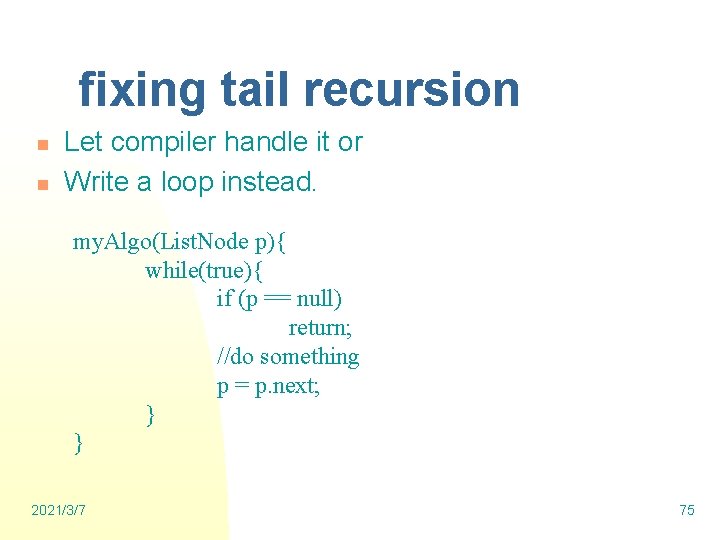
fixing tail recursion n n Let compiler handle it or Write a loop instead. my. Algo(List. Node p){ while(true){ if (p == null) return; //do something p = p. next; } } 2021/3/7 75
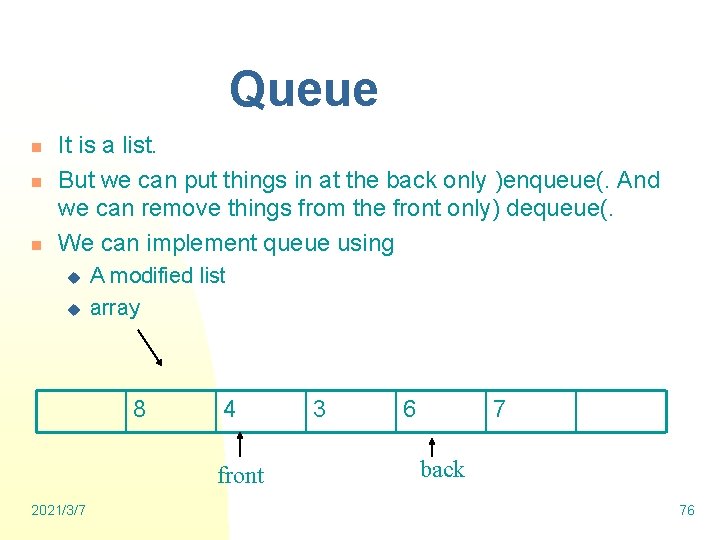
Queue n n n It is a list. But we can put things in at the back only )enqueue(. And we can remove things from the front only) dequeue(. We can implement queue using u u A modified list array 8 4 front 2021/3/7 3 6 7 back 76
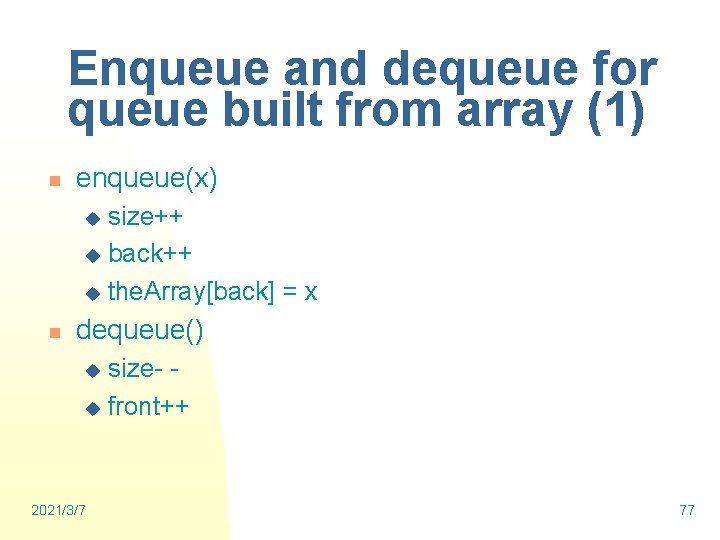
Enqueue and dequeue for queue built from array (1) n enqueue(x) size++ u back++ u the. Array[back] = x u n dequeue() size- u front++ u 2021/3/7 77
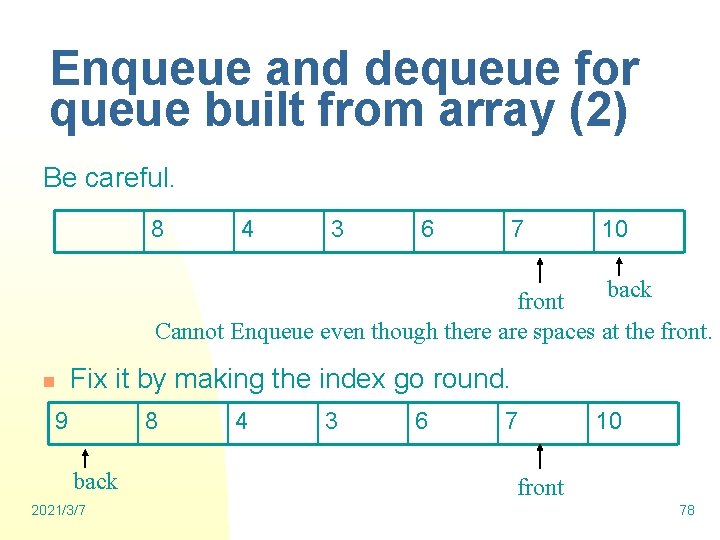
Enqueue and dequeue for queue built from array (2) Be careful. 8 4 3 6 7 10 back front Cannot Enqueue even though there are spaces at the front. n Fix it by making the index go round. 9 8 back 2021/3/7 4 3 6 7 10 front 78
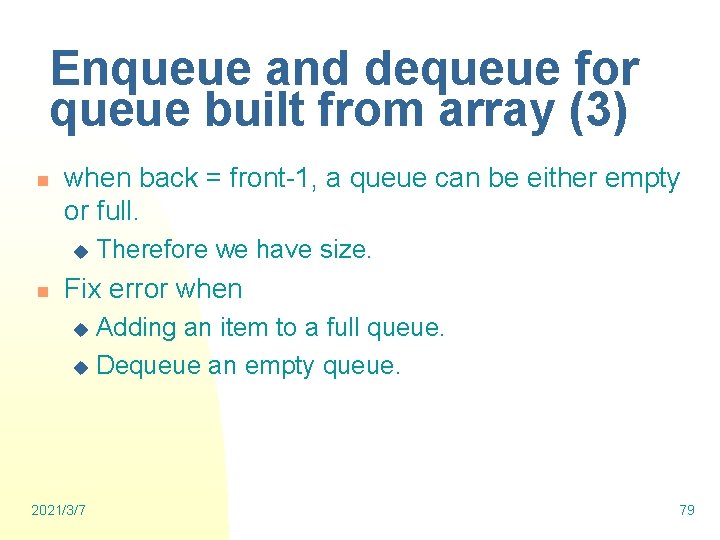
Enqueue and dequeue for queue built from array (3) n when back = front-1, a queue can be either empty or full. u n Therefore we have size. Fix error when Adding an item to a full queue. u Dequeue an empty queue. u 2021/3/7 79
![public class Queue Array private Object the Array private int size private public class Queue. Array{ private Object [ ] the. Array; private int size; private](https://slidetodoc.com/presentation_image_h/2ab4e1ea4ad3f8d8d7079ac0166ebdd9/image-80.jpg)
public class Queue. Array{ private Object [ ] the. Array; private int size; private int front; private int back; static final int DEFAULT_CAPACITY = 10; public Queue. Array( ) { this( DEFAULT_CAPACITY ); } public Queue. Array( int capacity ) { the. Array = new Object[ capacity ]; make. Empty( ); } 2021/3/7 80
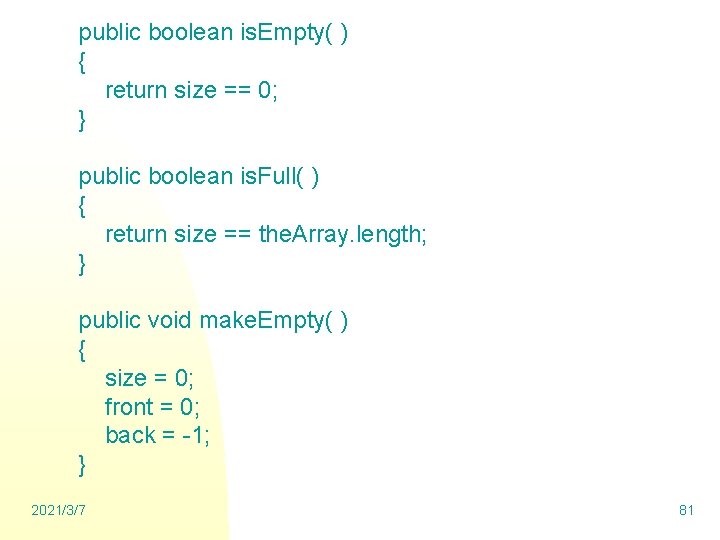
public boolean is. Empty( ) { return size == 0; } public boolean is. Full( ) { return size == the. Array. length; } public void make. Empty( ) { size = 0; front = 0; back = -1; } 2021/3/7 81
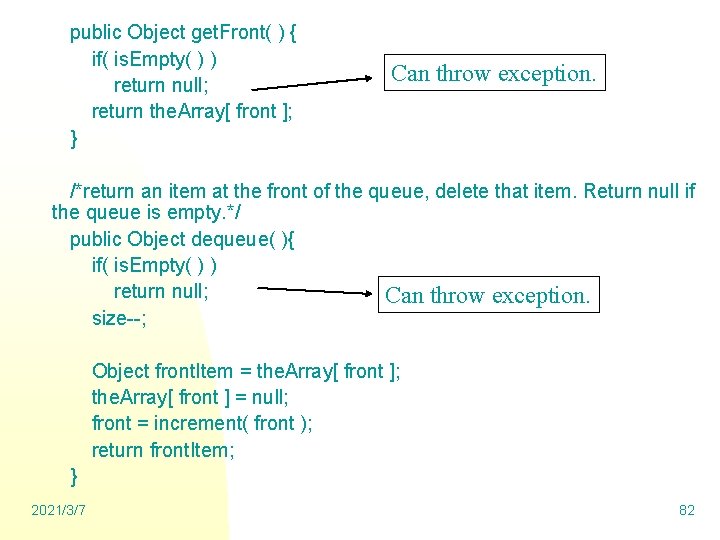
public Object get. Front( ) { if( is. Empty( ) ) return null; return the. Array[ front ]; } Can throw exception. /*return an item at the front of the queue, delete that item. Return null if the queue is empty. */ public Object dequeue( ){ if( is. Empty( ) ) return null; Can throw exception. size--; Object front. Item = the. Array[ front ]; the. Array[ front ] = null; front = increment( front ); return front. Item; } 2021/3/7 82
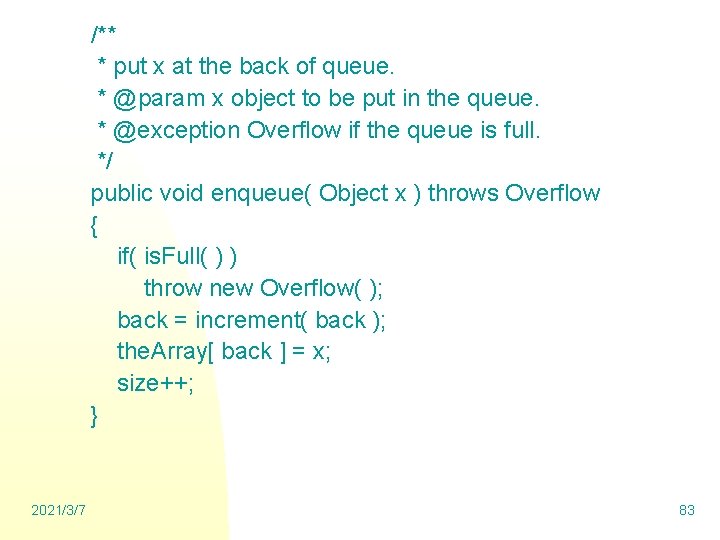
/** * put x at the back of queue. * @param x object to be put in the queue. * @exception Overflow if the queue is full. */ public void enqueue( Object x ) throws Overflow { if( is. Full( ) ) throw new Overflow( ); back = increment( back ); the. Array[ back ] = x; size++; } 2021/3/7 83
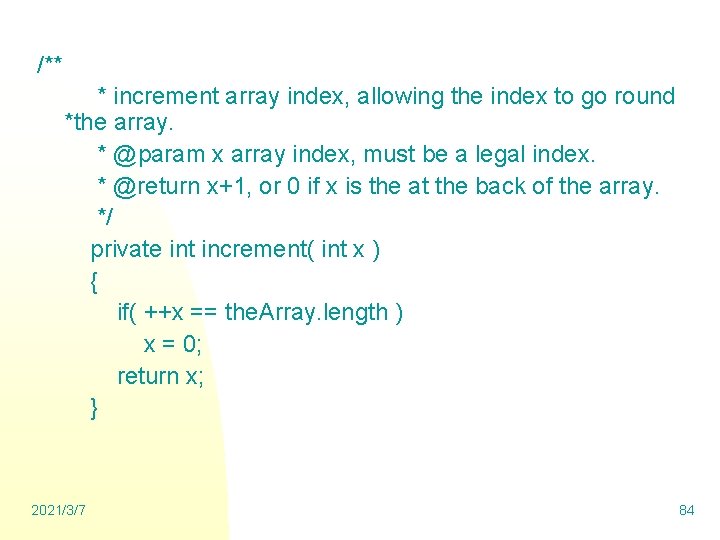
/** * increment array index, allowing the index to go round *the array. * @param x array index, must be a legal index. * @return x+1, or 0 if x is the at the back of the array. */ private int increment( int x ) { if( ++x == the. Array. length ) x = 0; return x; } 2021/3/7 84
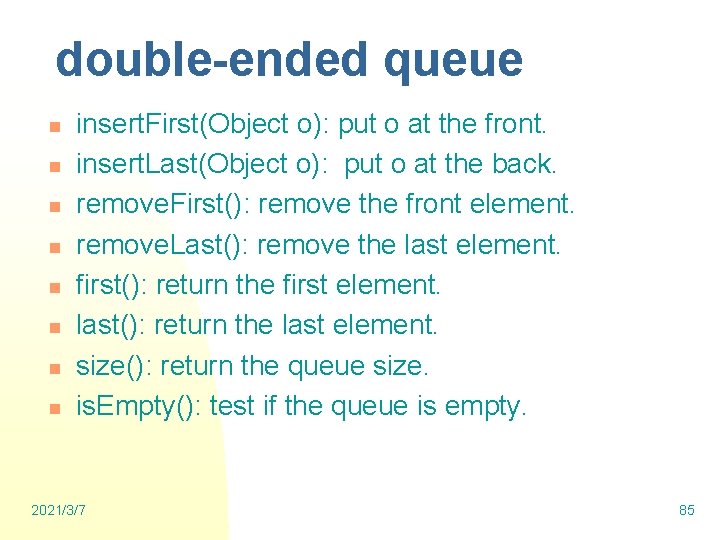
double-ended queue n n n n insert. First(Object o): put o at the front. insert. Last(Object o): put o at the back. remove. First(): remove the front element. remove. Last(): remove the last element. first(): return the first element. last(): return the last element. size(): return the queue size. is. Empty(): test if the queue is empty. 2021/3/7 85
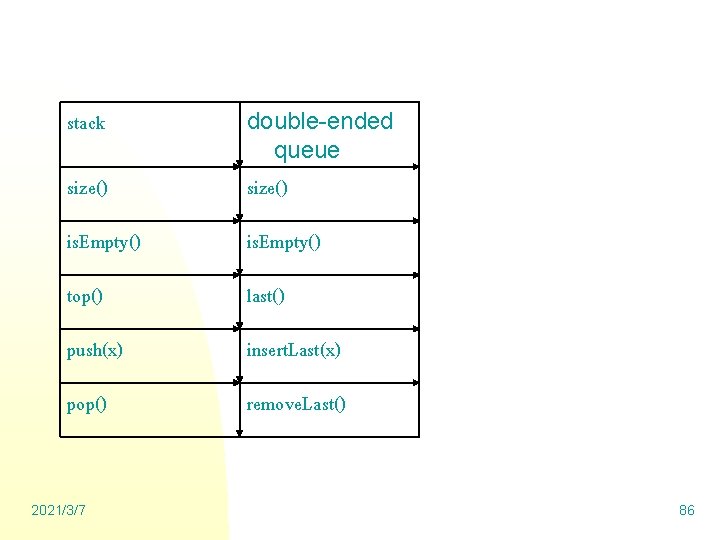
stack double-ended queue size() is. Empty() top() last() push(x) insert. Last(x) pop() remove. Last() 2021/3/7 86
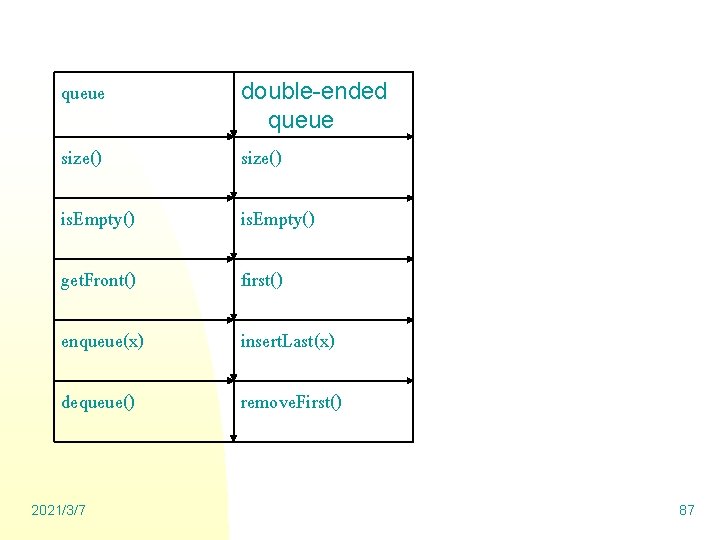
queue double-ended queue size() is. Empty() get. Front() first() enqueue(x) insert. Last(x) dequeue() remove. First() 2021/3/7 87
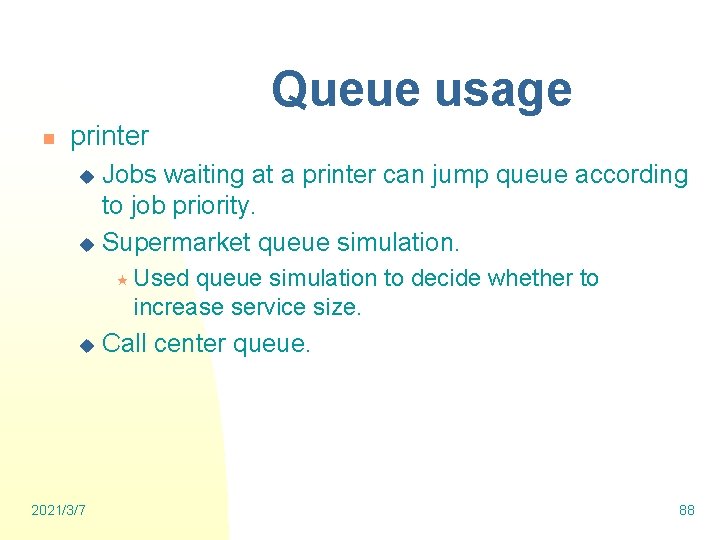
Queue usage n printer Jobs waiting at a printer can jump queue according to job priority. u Supermarket queue simulation. u « Used queue simulation to decide whether to increase service size. u 2021/3/7 Call center queue. 88
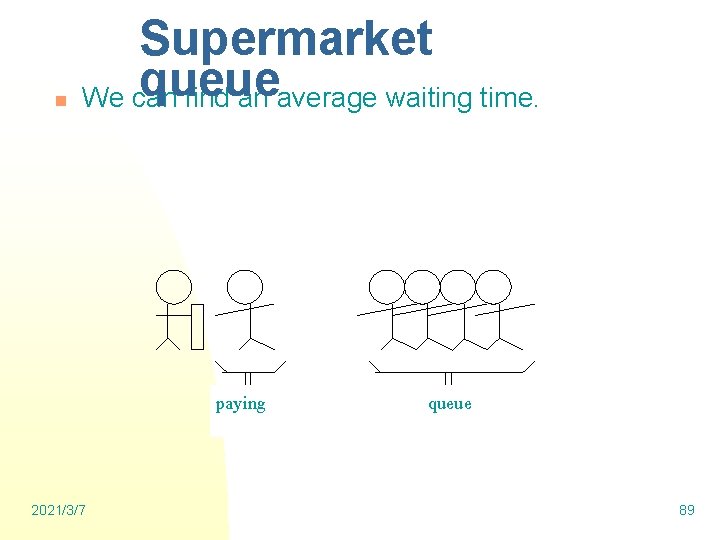
n Supermarket queue We can find an average waiting time. paying 2021/3/7 queue 89
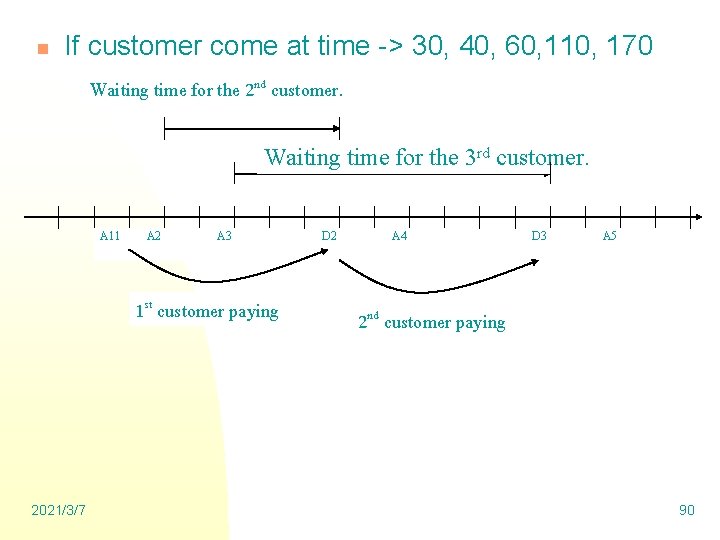
n If customer come at time -> 30, 40, 60, 110, 170 Waiting time for the 2 nd customer. Waiting time for the 3 rd customer. A 11 A 2 A 3 1 st customer paying 2021/3/7 D 2 A 4 D 3 A 5 2 nd customer paying 90
Difference between simple queue and circular queue
Stack dan queue
Python stack and queue
Stack
Pengertian stack dan queue
Queue stack c++
Python queue clear
Stack pointer is a
Stack=[] digunakan untuk memebuat stack dengan
Stack smashing vs buffer overflow
Difference between linked list and queue
Priority queue linked list python
Operasi dalam queue
Jika noel(create(q)) adalah 0, maka front(create(q)) adalah
Queue/ antraian = ordered list
Replay queue length exchange 2010
Applications of queue
If the character d c b a are placed in a queue
M m 1 queue
Message queues in unix
Miningph
Chapter 3 queue
A schoolyard contains only bicycles and 4 wheeled wagons
Priority queue order
Pushx
The queue summary
Sonic mq
Mmmm meaning
Sjf cpu scheduling
Acting queue
Chinese old hairstyle
Priority queue lower bound
Type abstrait de données
Queue abstract data type
Multilevel queue scheduling example with gantt chart
Queue data structure
Gadi queue limits
Queue time trivia
Brodal queue
Chp 4
Queue front rear
Pengertian queue
Insert element in priority queue
Queue waiting time
Consider the linear queue with rear =4
Adaptable priority queue java
Queue in data structure
Mm1 formulas
Adam queue
Delay queue java
Vxworks message queue
Homonyms of sixty minutes
Gpu queue
Kafka priority queue
Mm1 queue formulas
Priority queue animation
Event queue pattern
Deque and priority queue
Twolock
Fngmhy.com
Queue adt
Nyiso queue
Queue d'aronde
Multilevel feedback queue
M m 1 queue
Flip queue size
Queues definition
Representation of queues
Amadeus queue management
The bus stop queue answers
Binomial queue
Microsoft ignite dc
Priority queue using heap
Queue waiting time
Ipc
Binomial queue example
Queue front rear
Queue adt
Adaptable priority queue java
Bus queue shelters
Materi queue struktur data
Multiple processor scheduling in os
Queue management system
Queue in data structure example
Queue waiting time
Adaptive virtual queue management algorithm
Function queue scheduling architecture
Voxtron pricing
Pengertian queue
Queue adt
Types of priority queue