Queue Stack implementations Array resizing Queue January 16
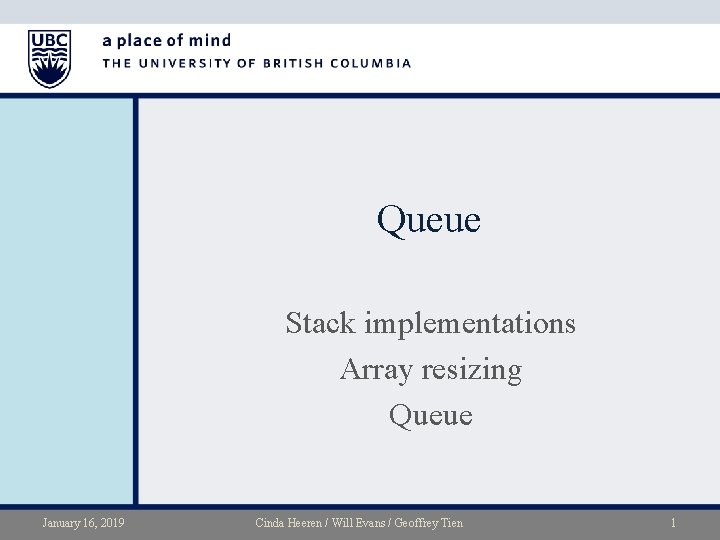
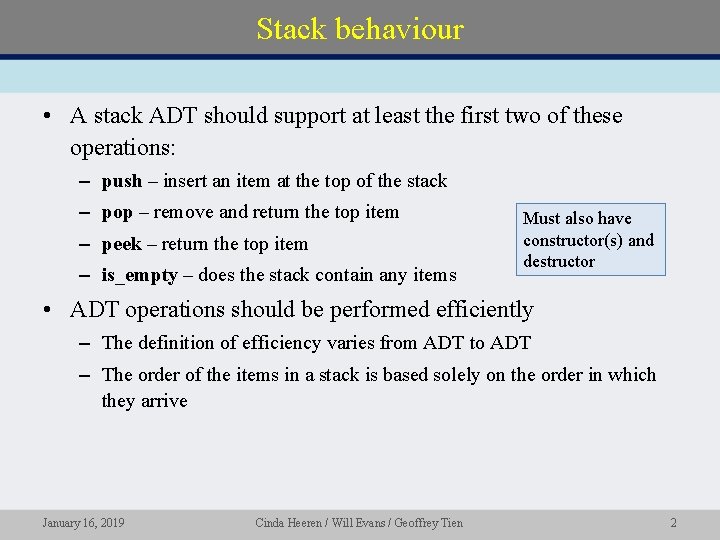
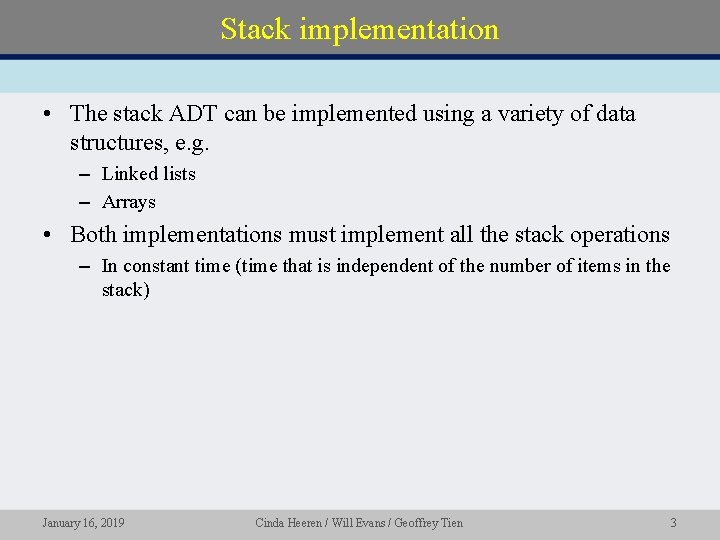
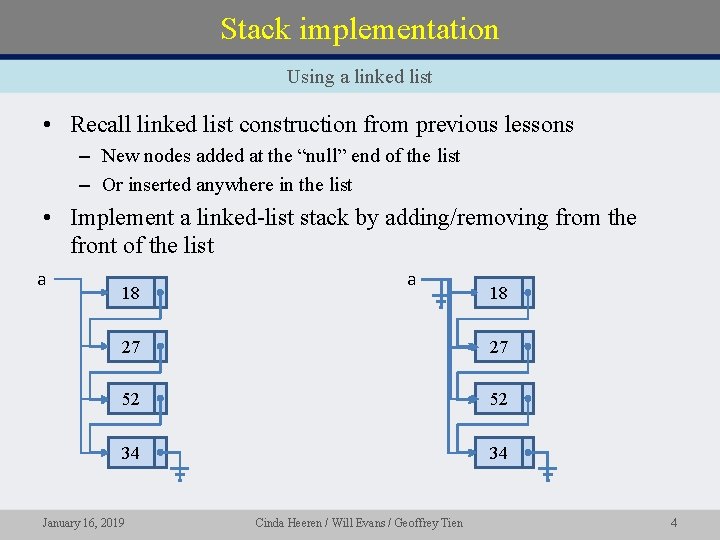
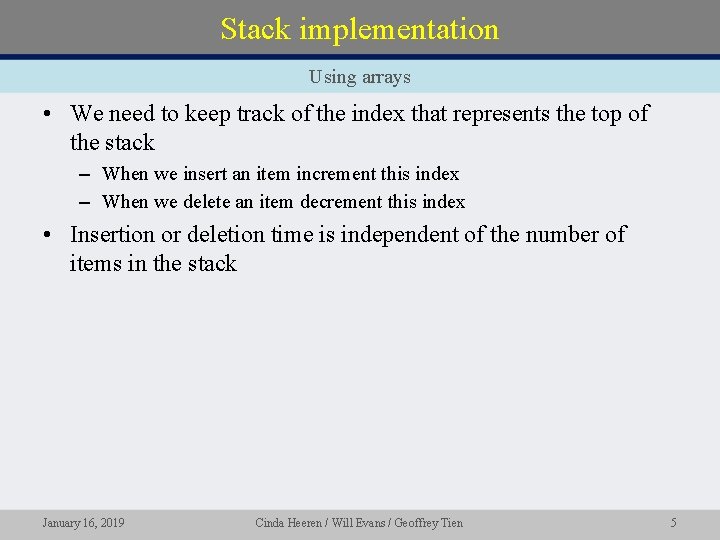
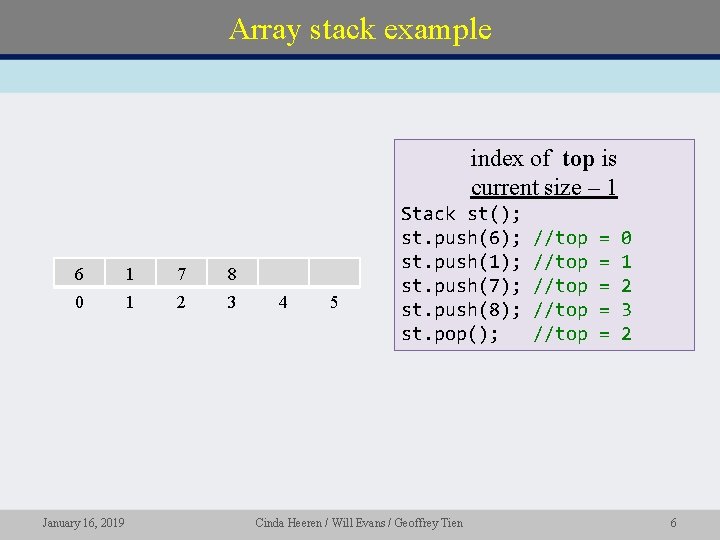
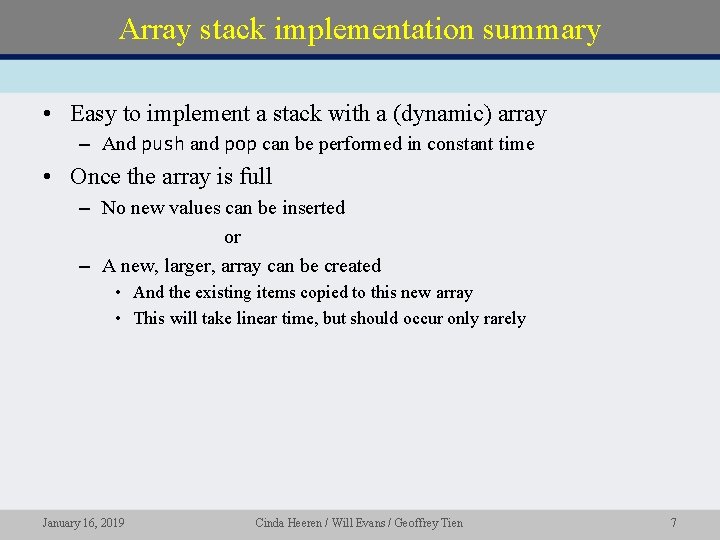
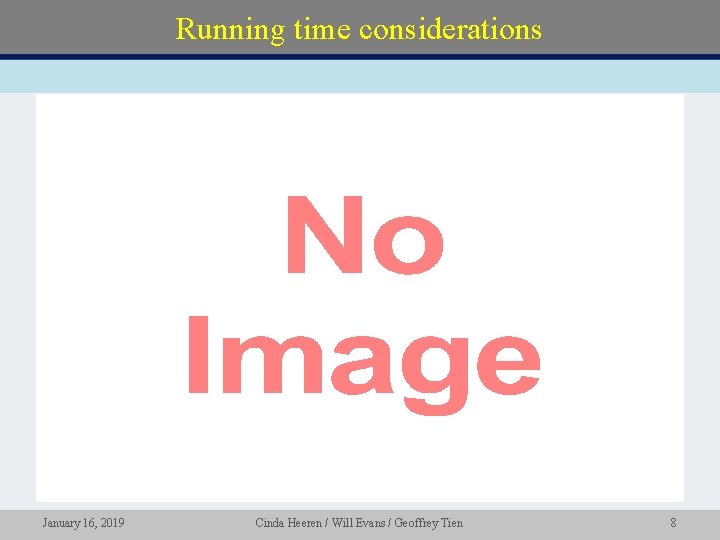
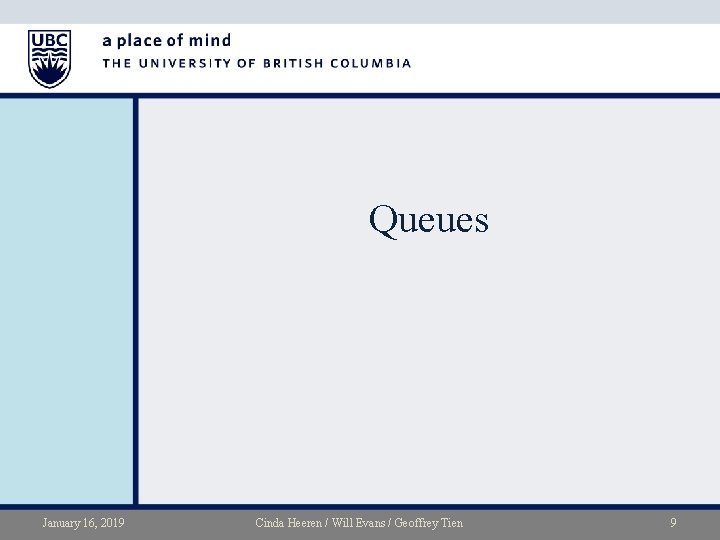
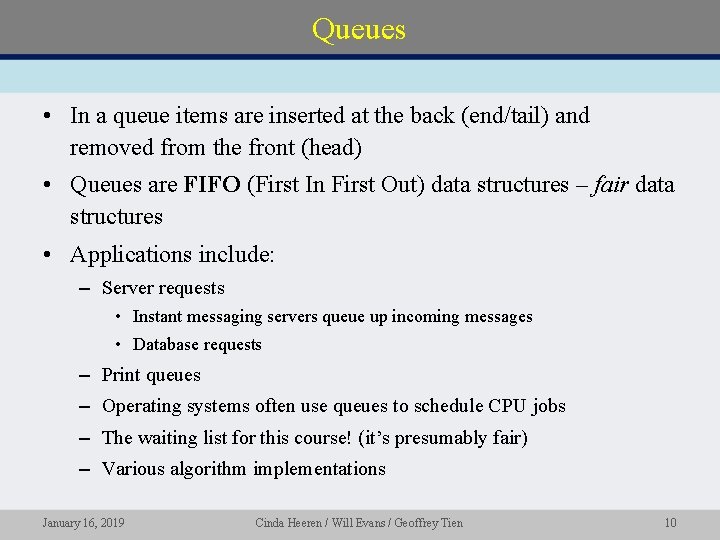
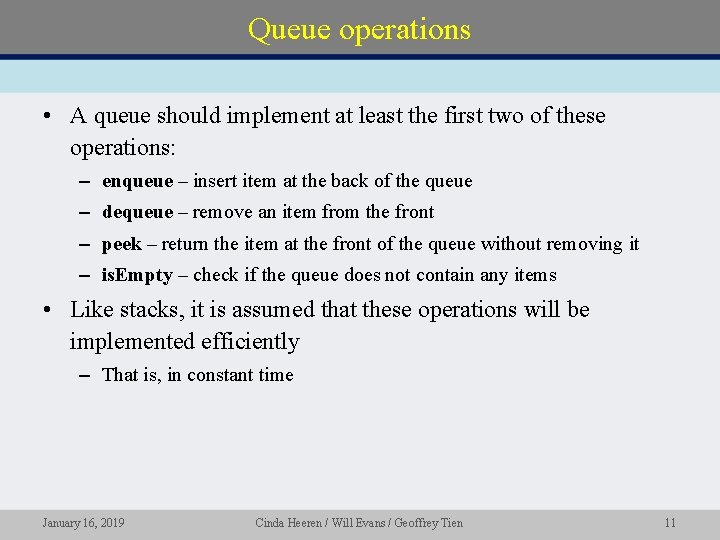
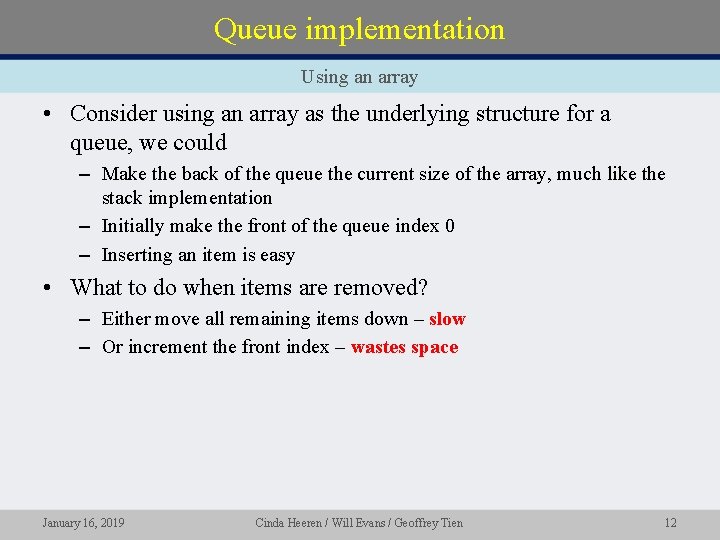
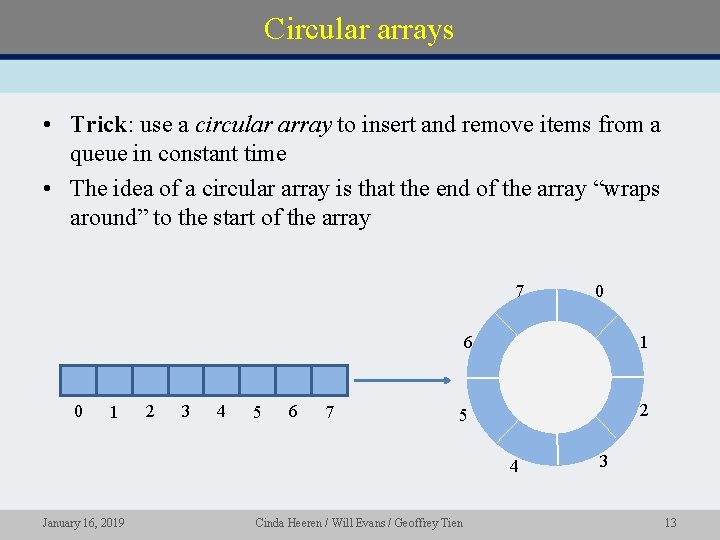
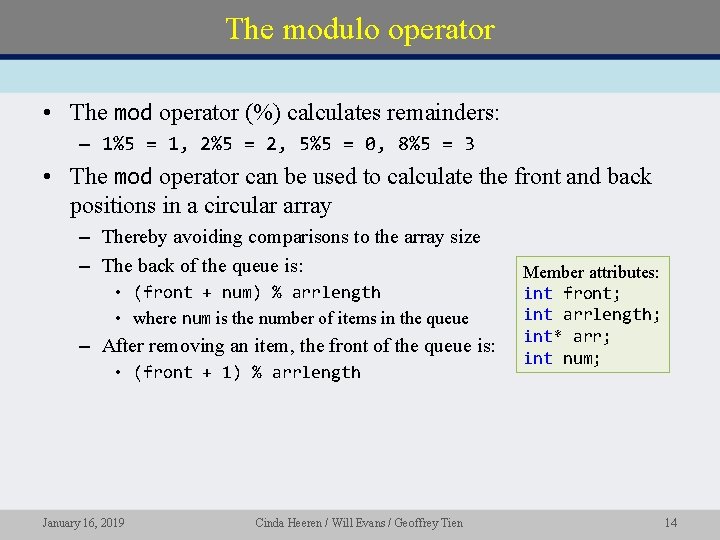
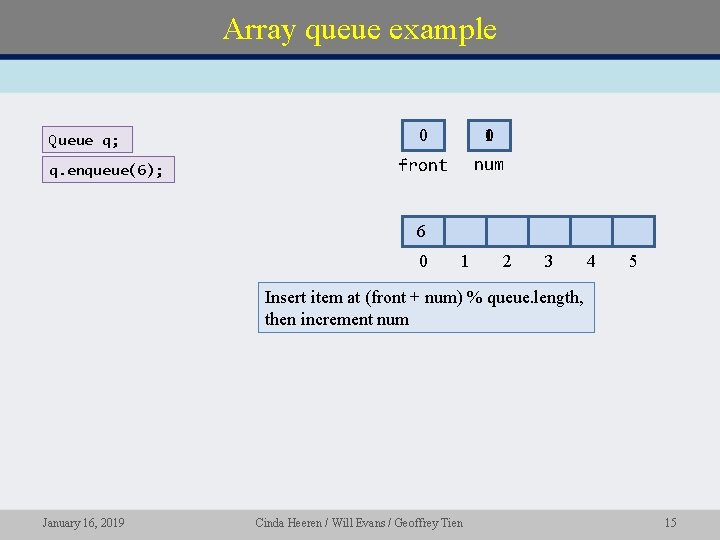
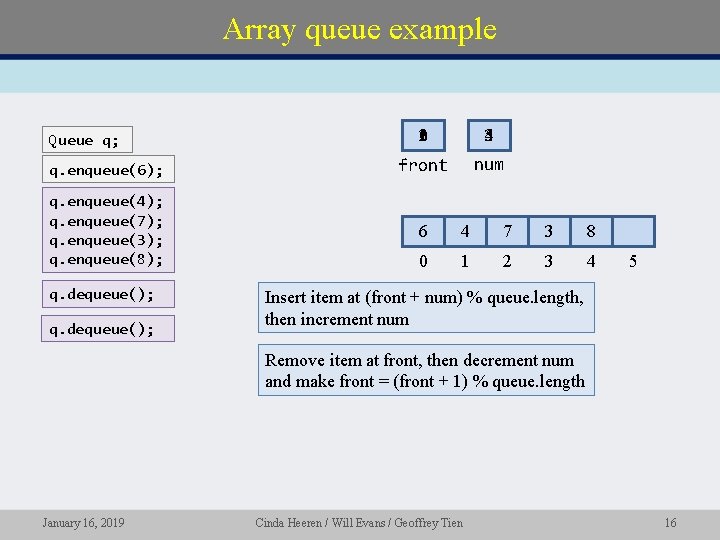
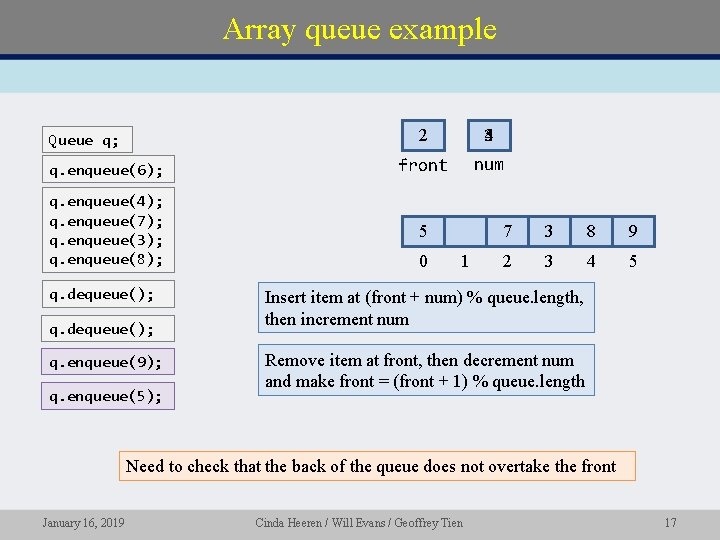
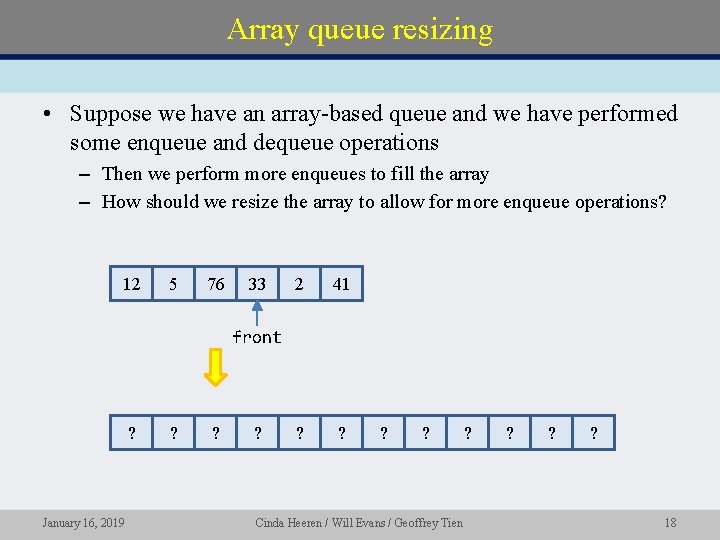
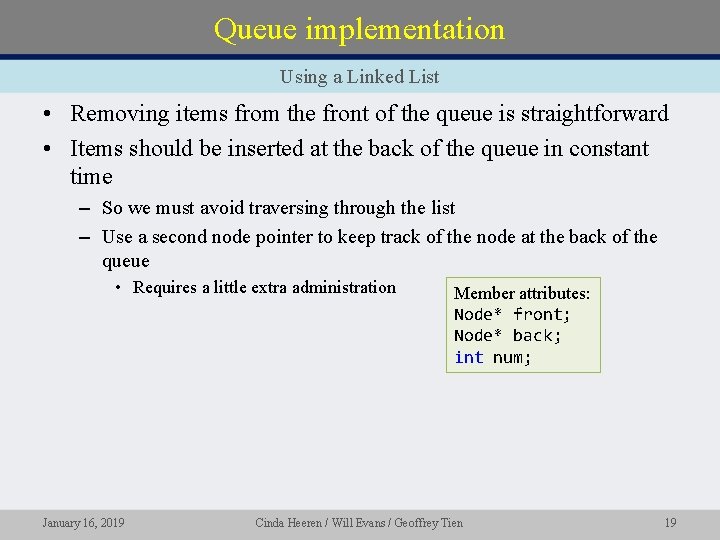
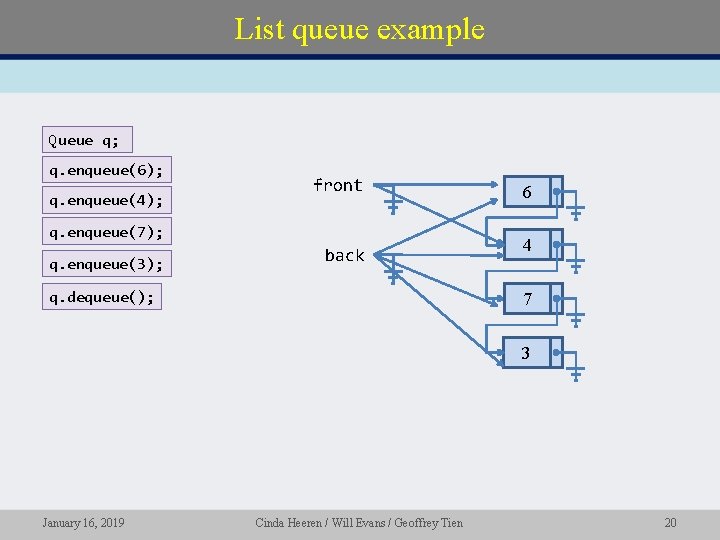
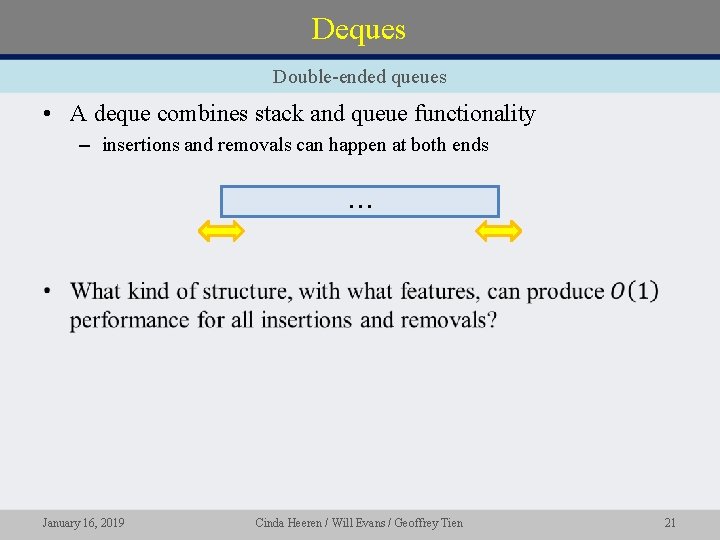
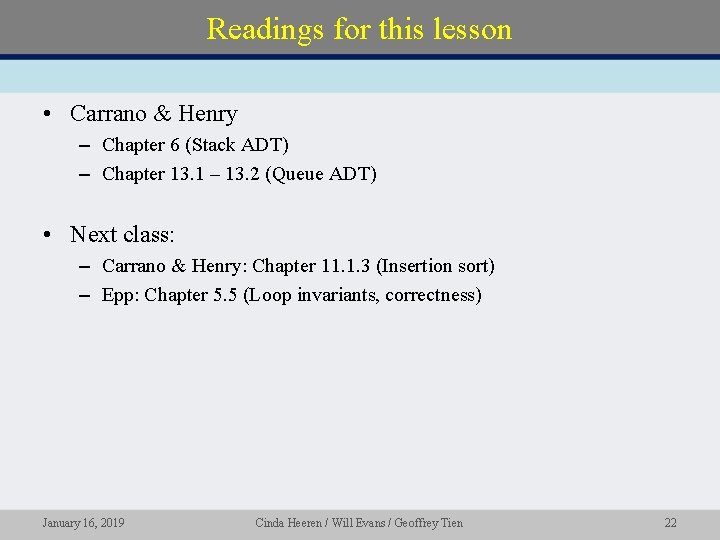
- Slides: 22
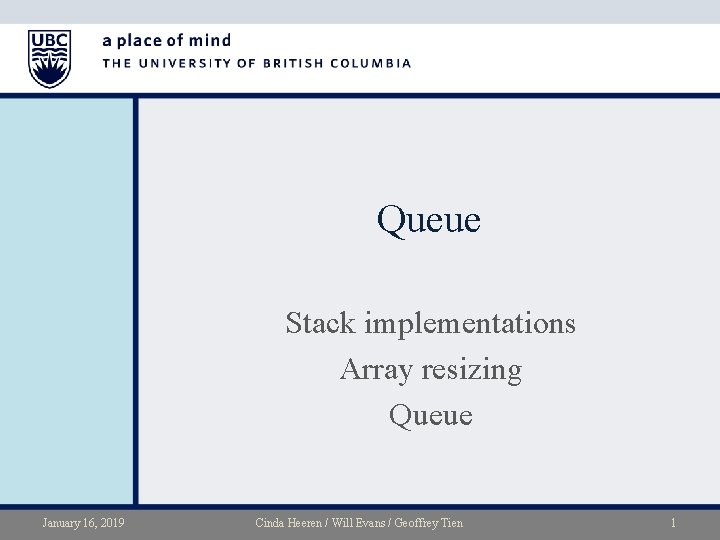
Queue Stack implementations Array resizing Queue January 16, 2019 Cinda Heeren / Will Evans / Geoffrey Tien 1
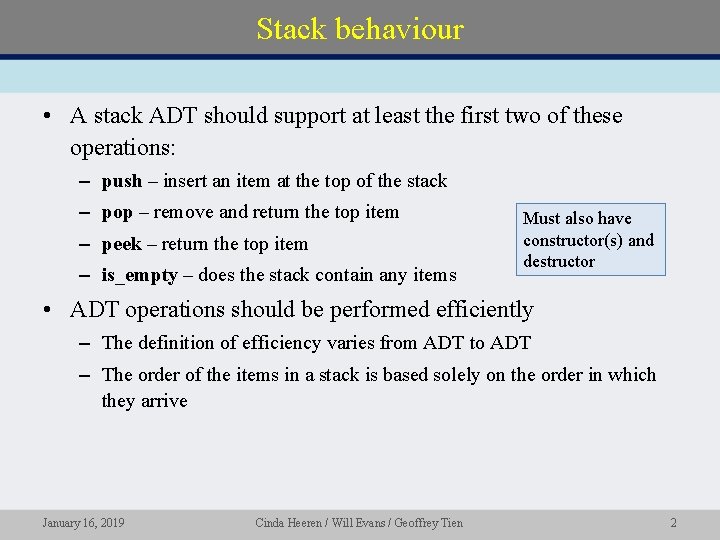
Stack behaviour • A stack ADT should support at least the first two of these operations: – push – insert an item at the top of the stack – pop – remove and return the top item – peek – return the top item – is_empty – does the stack contain any items Must also have constructor(s) and destructor • ADT operations should be performed efficiently – The definition of efficiency varies from ADT to ADT – The order of the items in a stack is based solely on the order in which they arrive January 16, 2019 Cinda Heeren / Will Evans / Geoffrey Tien 2
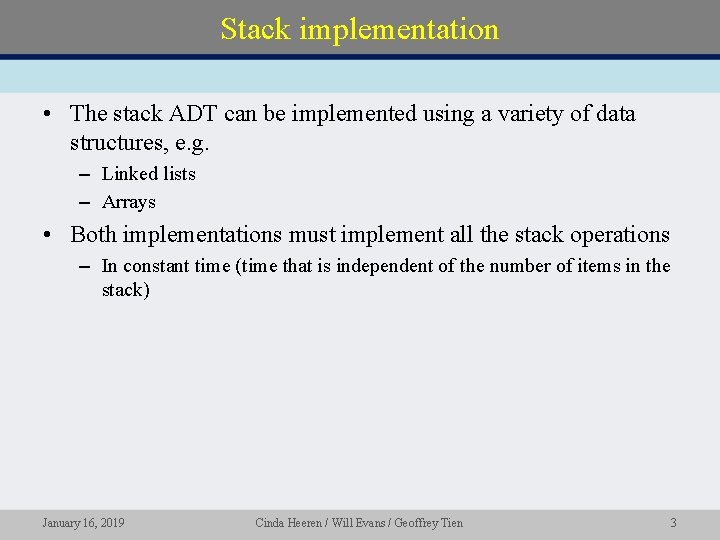
Stack implementation • The stack ADT can be implemented using a variety of data structures, e. g. – Linked lists – Arrays • Both implementations must implement all the stack operations – In constant time (time that is independent of the number of items in the stack) January 16, 2019 Cinda Heeren / Will Evans / Geoffrey Tien 3
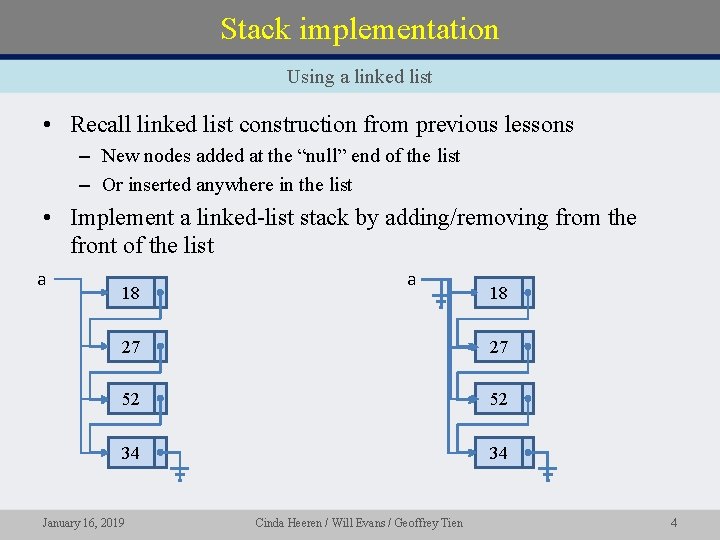
Stack implementation Using a linked list • Recall linked list construction from previous lessons – New nodes added at the “null” end of the list – Or inserted anywhere in the list • Implement a linked-list stack by adding/removing from the front of the list a 18 27 27 52 52 34 34 January 16, 2019 Cinda Heeren / Will Evans / Geoffrey Tien 4
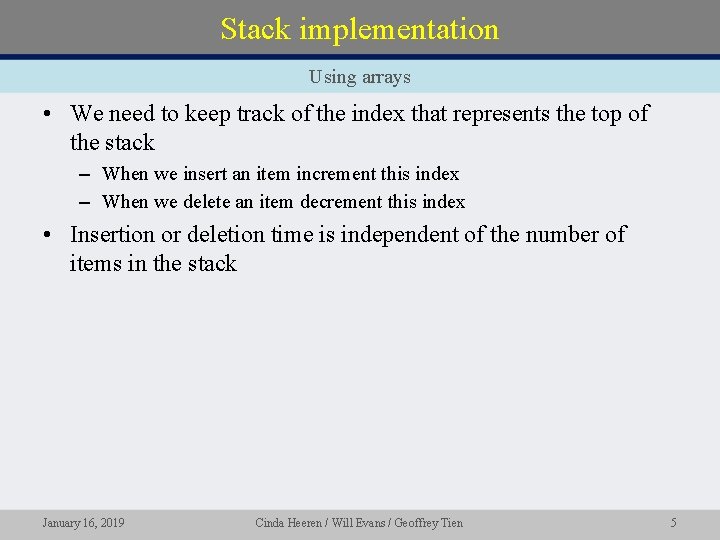
Stack implementation Using arrays • We need to keep track of the index that represents the top of the stack – When we insert an item increment this index – When we delete an item decrement this index • Insertion or deletion time is independent of the number of items in the stack January 16, 2019 Cinda Heeren / Will Evans / Geoffrey Tien 5
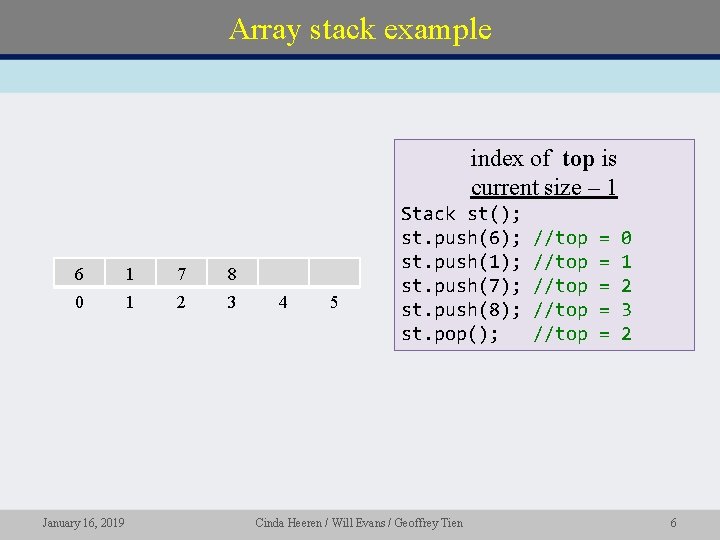
Array stack example index of top is current size – 1 6 1 7 8 0 1 2 3 January 16, 2019 4 5 Stack st(); st. push(6); st. push(1); st. push(7); st. push(8); st. pop(); Cinda Heeren / Will Evans / Geoffrey Tien //top //top = = = 0 1 2 3 2 6
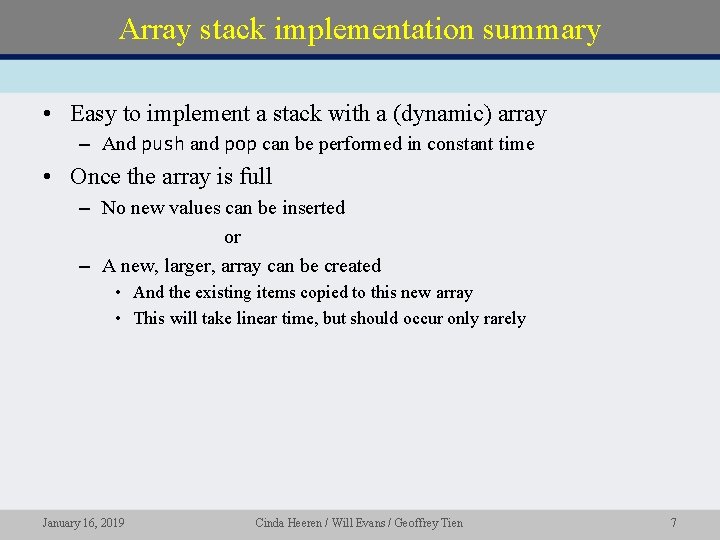
Array stack implementation summary • Easy to implement a stack with a (dynamic) array – And push and pop can be performed in constant time • Once the array is full – No new values can be inserted or – A new, larger, array can be created • And the existing items copied to this new array • This will take linear time, but should occur only rarely January 16, 2019 Cinda Heeren / Will Evans / Geoffrey Tien 7
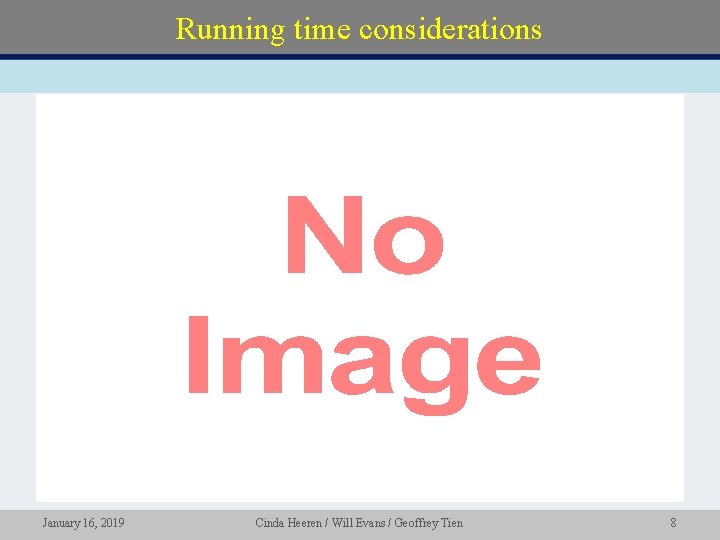
Running time considerations • January 16, 2019 Cinda Heeren / Will Evans / Geoffrey Tien 8
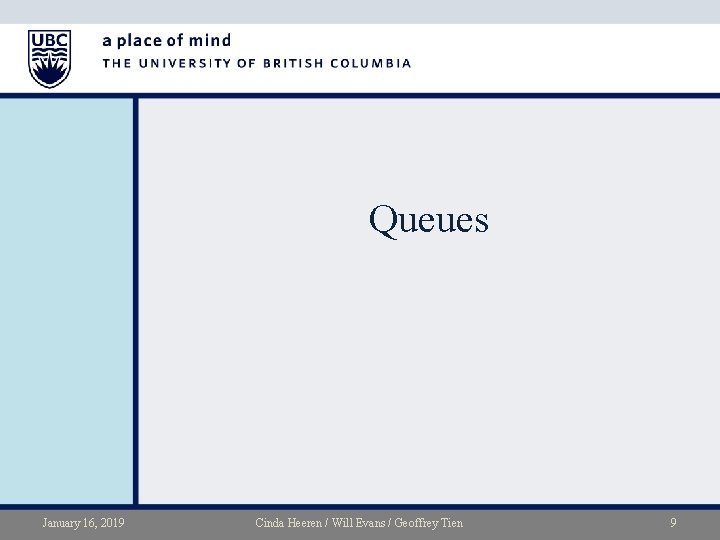
Queues January 16, 2019 Cinda Heeren / Will Evans / Geoffrey Tien 9
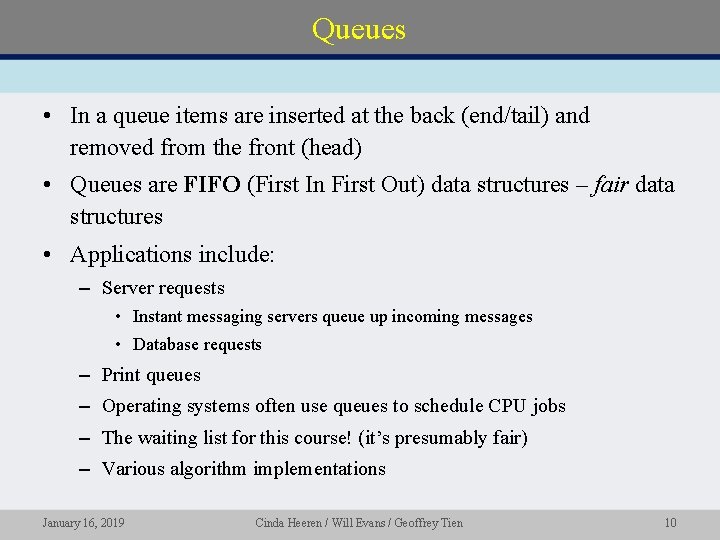
Queues • In a queue items are inserted at the back (end/tail) and removed from the front (head) • Queues are FIFO (First In First Out) data structures – fair data structures • Applications include: – Server requests • Instant messaging servers queue up incoming messages • Database requests – Print queues – Operating systems often use queues to schedule CPU jobs – The waiting list for this course! (it’s presumably fair) – Various algorithm implementations January 16, 2019 Cinda Heeren / Will Evans / Geoffrey Tien 10
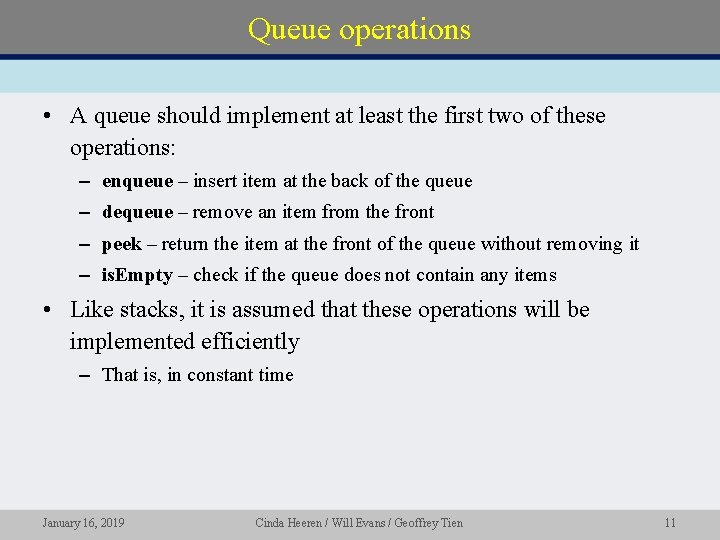
Queue operations • A queue should implement at least the first two of these operations: – enqueue – insert item at the back of the queue – dequeue – remove an item from the front – peek – return the item at the front of the queue without removing it – is. Empty – check if the queue does not contain any items • Like stacks, it is assumed that these operations will be implemented efficiently – That is, in constant time January 16, 2019 Cinda Heeren / Will Evans / Geoffrey Tien 11
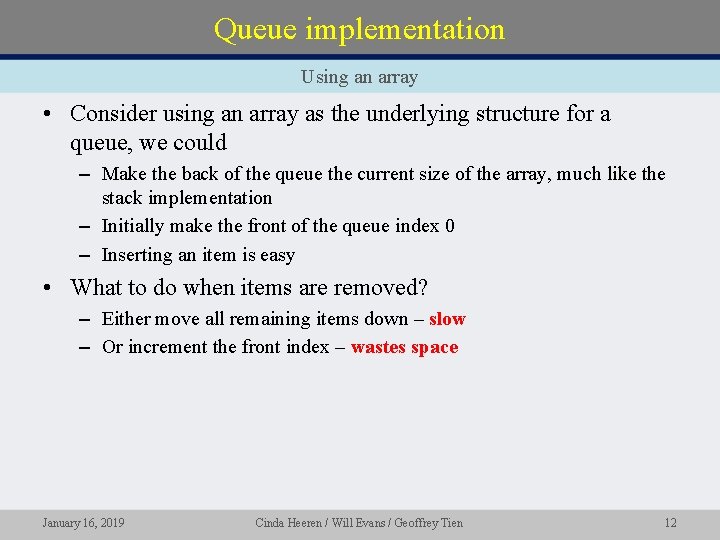
Queue implementation Using an array • Consider using an array as the underlying structure for a queue, we could – Make the back of the queue the current size of the array, much like the stack implementation – Initially make the front of the queue index 0 – Inserting an item is easy • What to do when items are removed? – Either move all remaining items down – slow – Or increment the front index – wastes space January 16, 2019 Cinda Heeren / Will Evans / Geoffrey Tien 12
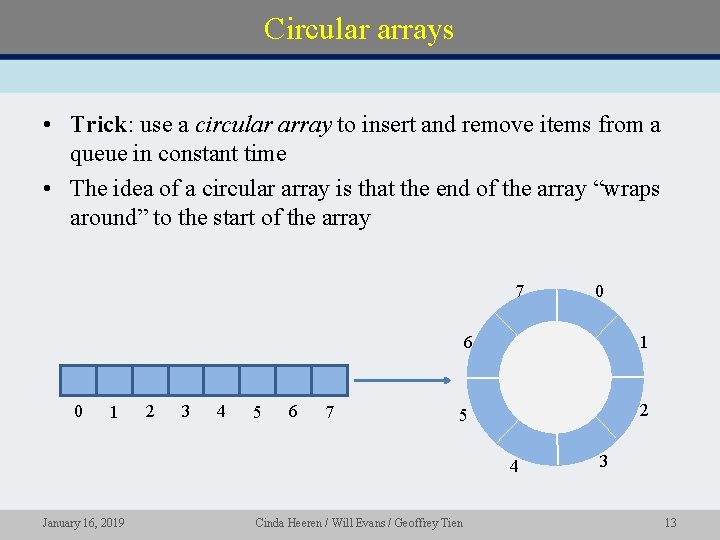
Circular arrays • Trick: use a circular array to insert and remove items from a queue in constant time • The idea of a circular array is that the end of the array “wraps around” to the start of the array 7 0 6 0 1 2 3 4 5 6 7 1 2 5 4 January 16, 2019 Cinda Heeren / Will Evans / Geoffrey Tien 3 13
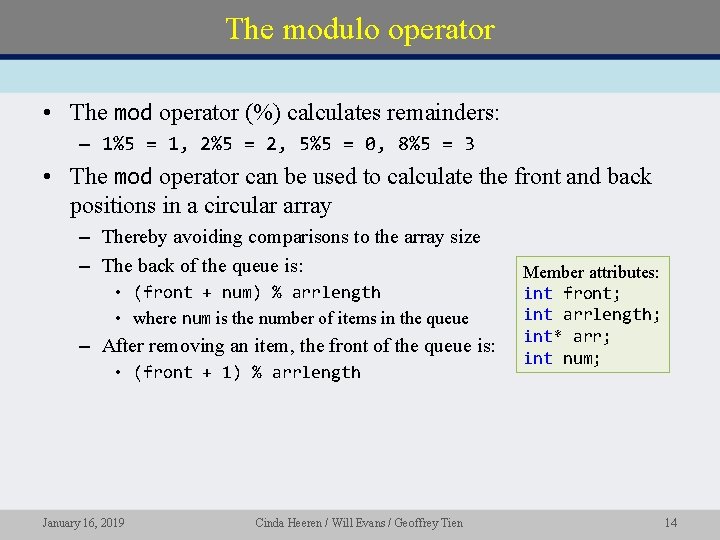
The modulo operator • The mod operator (%) calculates remainders: – 1%5 = 1, 2%5 = 2, 5%5 = 0, 8%5 = 3 • The mod operator can be used to calculate the front and back positions in a circular array – Thereby avoiding comparisons to the array size – The back of the queue is: • (front + num) % arrlength • where num is the number of items in the queue – After removing an item, the front of the queue is: • (front + 1) % arrlength January 16, 2019 Cinda Heeren / Will Evans / Geoffrey Tien Member attributes: int front; int arrlength; int* arr; int num; 14
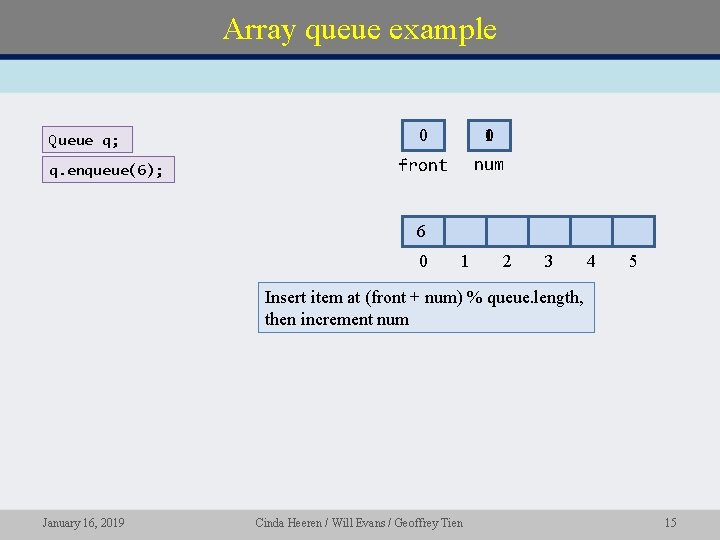
Array queue example Queue q; q. enqueue(6); 0 01 front num 6 0 1 2 3 4 5 Insert item at (front + num) % queue. length, then increment num January 16, 2019 Cinda Heeren / Will Evans / Geoffrey Tien 15
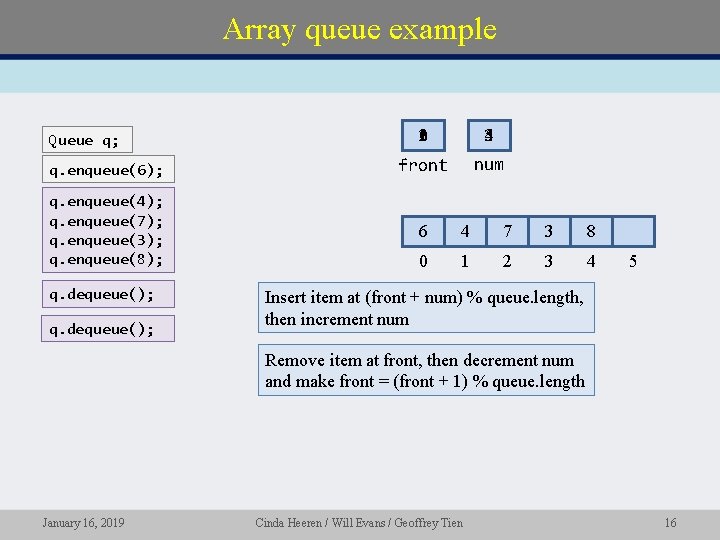
Array queue example 210 345 q. enqueue(6); front num q. enqueue(4); q. enqueue(7); q. enqueue(3); q. enqueue(8); 6 4 7 3 8 0 1 2 3 4 Queue q; q. dequeue(); 5 Insert item at (front + num) % queue. length, then increment num Remove item at front, then decrement num and make front = (front + 1) % queue. length January 16, 2019 Cinda Heeren / Will Evans / Geoffrey Tien 16
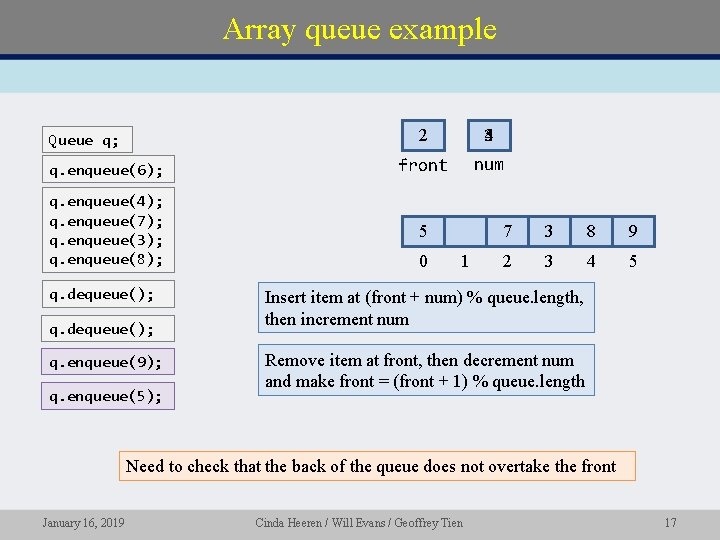
Array queue example 2 543 q. enqueue(6); front num q. enqueue(4); q. enqueue(7); q. enqueue(3); q. enqueue(8); 5 Queue q; q. dequeue(); q. enqueue(9); q. enqueue(5); 0 1 7 3 8 9 2 3 4 5 Insert item at (front + num) % queue. length, then increment num Remove item at front, then decrement num and make front = (front + 1) % queue. length Need to check that the back of the queue does not overtake the front January 16, 2019 Cinda Heeren / Will Evans / Geoffrey Tien 17
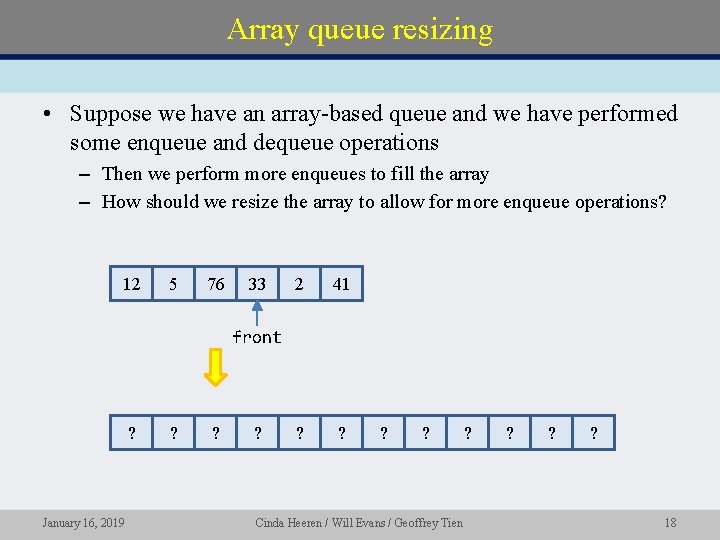
Array queue resizing • Suppose we have an array-based queue and we have performed some enqueue and dequeue operations – Then we perform more enqueues to fill the array – How should we resize the array to allow for more enqueue operations? 12 5 76 33 2 41 ? ? front ? January 16, 2019 ? ? ? Cinda Heeren / Will Evans / Geoffrey Tien ? ? 18
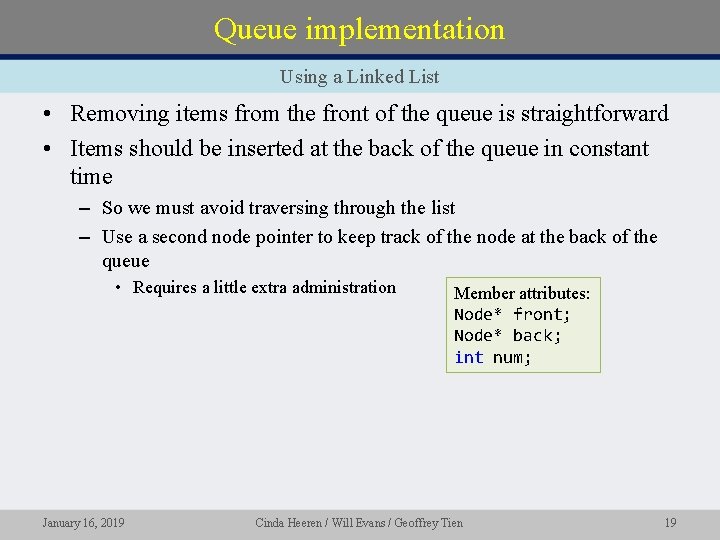
Queue implementation Using a Linked List • Removing items from the front of the queue is straightforward • Items should be inserted at the back of the queue in constant time – So we must avoid traversing through the list – Use a second node pointer to keep track of the node at the back of the queue • Requires a little extra administration January 16, 2019 Member attributes: Node* front; Node* back; int num; Cinda Heeren / Will Evans / Geoffrey Tien 19
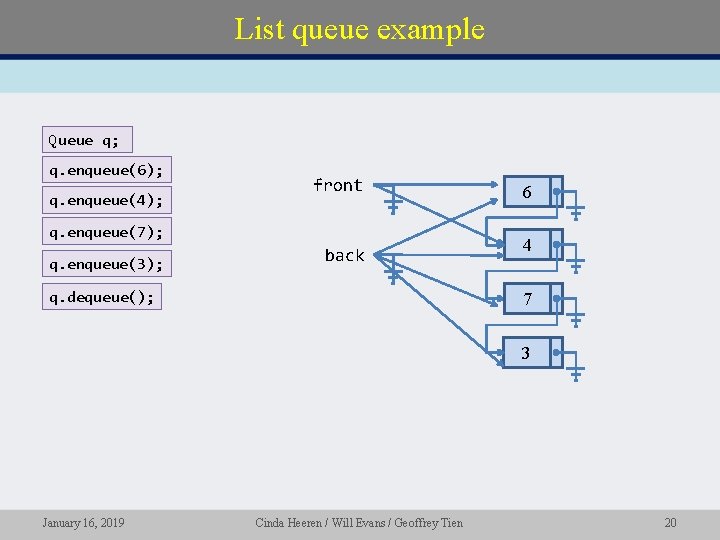
List queue example Queue q; q. enqueue(6); q. enqueue(4); front 6 back 4 q. enqueue(7); q. enqueue(3); q. dequeue(); 7 3 January 16, 2019 Cinda Heeren / Will Evans / Geoffrey Tien 20
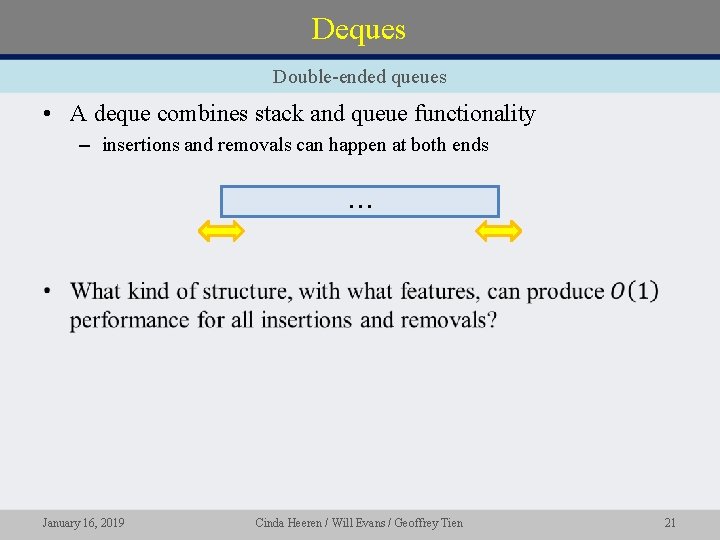
Deques Double-ended queues • A deque combines stack and queue functionality – insertions and removals can happen at both ends. . . January 16, 2019 Cinda Heeren / Will Evans / Geoffrey Tien 21
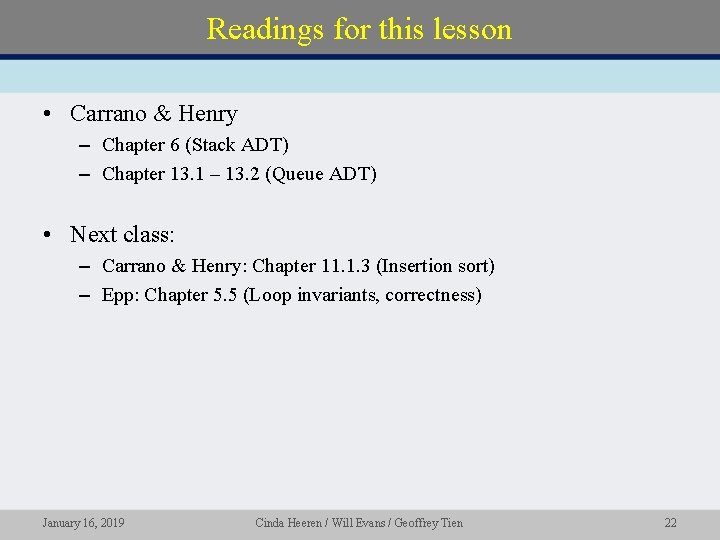
Readings for this lesson • Carrano & Henry – Chapter 6 (Stack ADT) – Chapter 13. 1 – 13. 2 (Queue ADT) • Next class: – Carrano & Henry: Chapter 11. 1. 3 (Insertion sort) – Epp: Chapter 5. 5 (Loop invariants, correctness) January 16, 2019 Cinda Heeren / Will Evans / Geoffrey Tien 22
Origen del downsizing
Resizing rh
Contoh soal stack dan jawabannya
Stack smashing vs buffer overflow
Stack characteristics
Python stack and queue
Stack dan queue
Contoh program stack
Struktur data queue
Contoh algoritma stack
Palindrome c++
Double ended queue in data structure
With erp implementations why would an auditorget involved
Smalltalk programming language
What are the common standard ethernet implementations?
Deque list
Implementasi queue dengan array
Jagged array vs multidimensional array
Sparse array adalah
Associative array vs indexed array
Contoh program array 1 dan 2 dimensi
North bridge south bridge
Broadside vs endfire