Queue Deque and Priority Queue Implementations Chapter 11
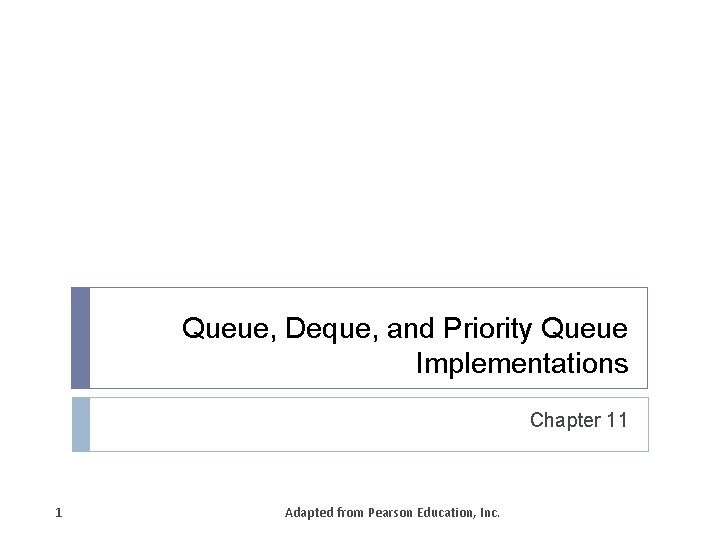
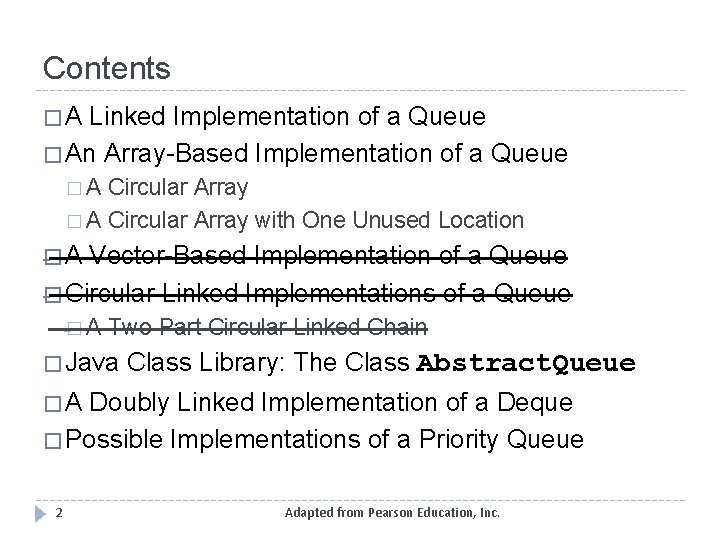
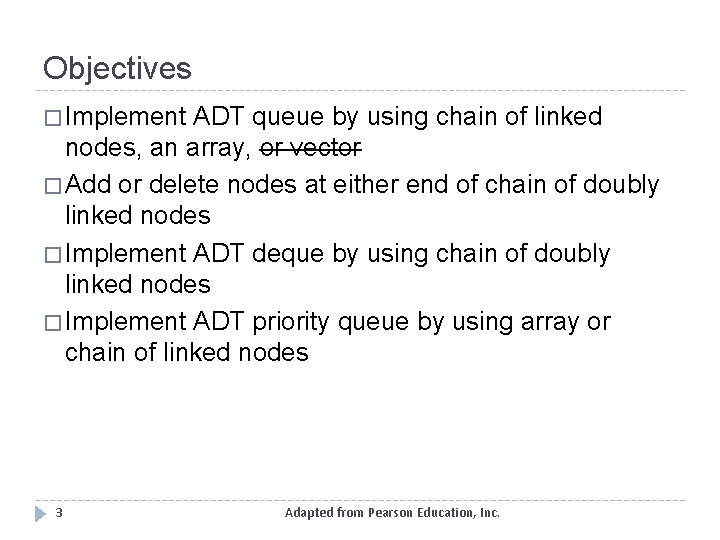
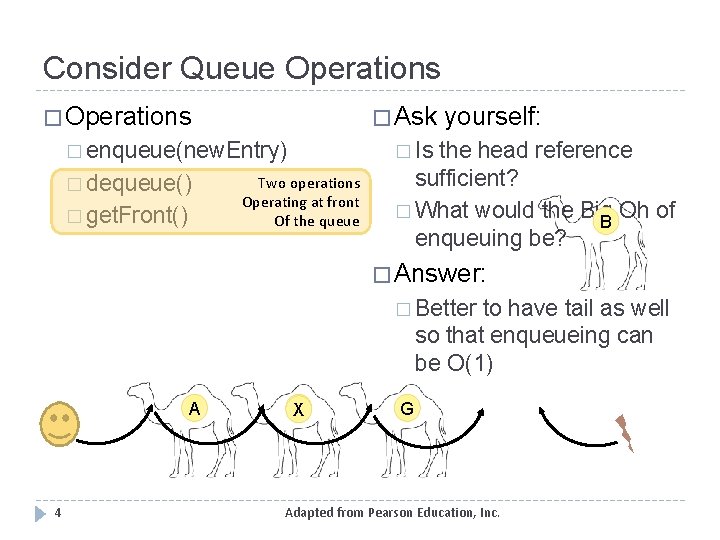
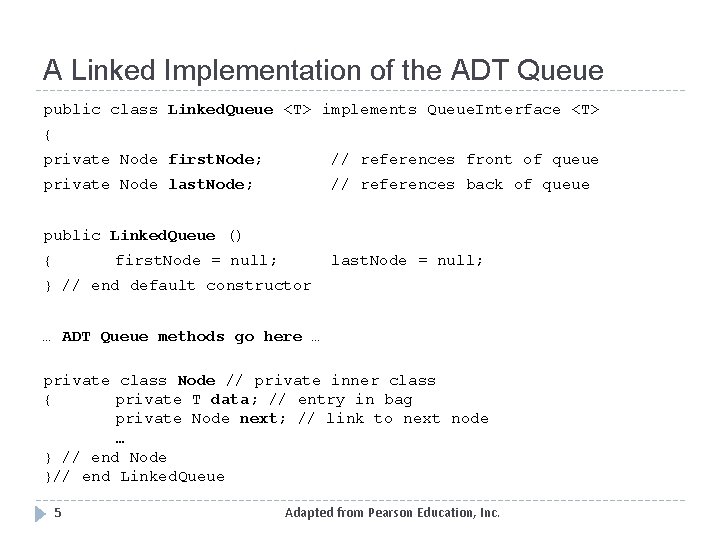
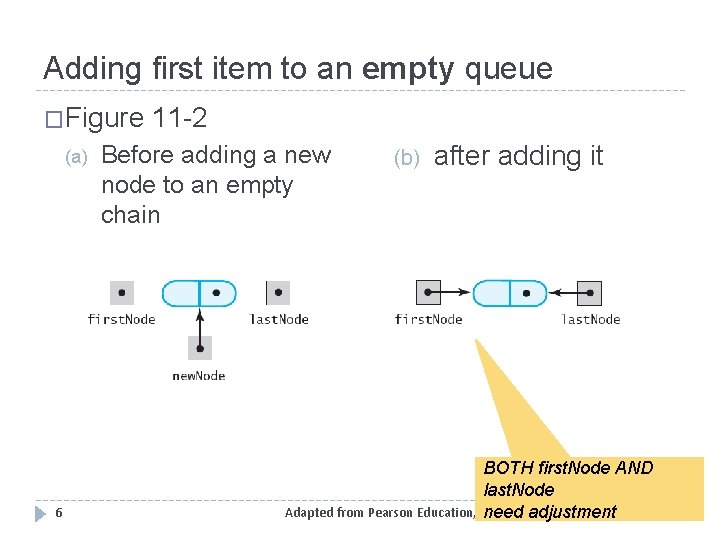
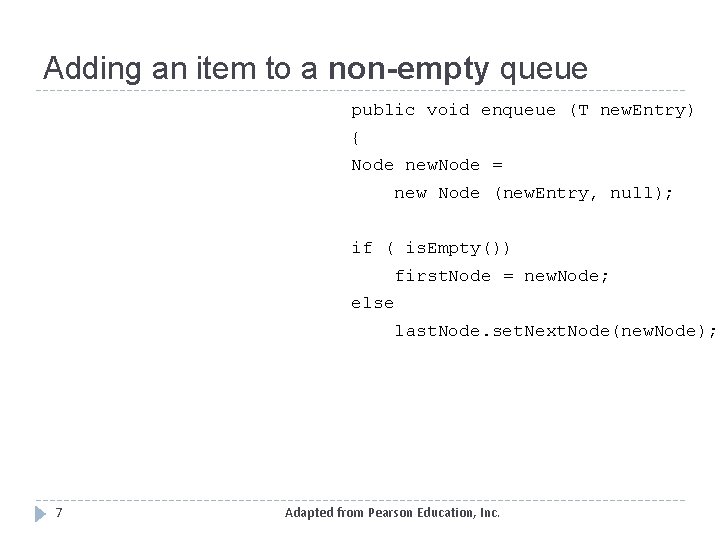
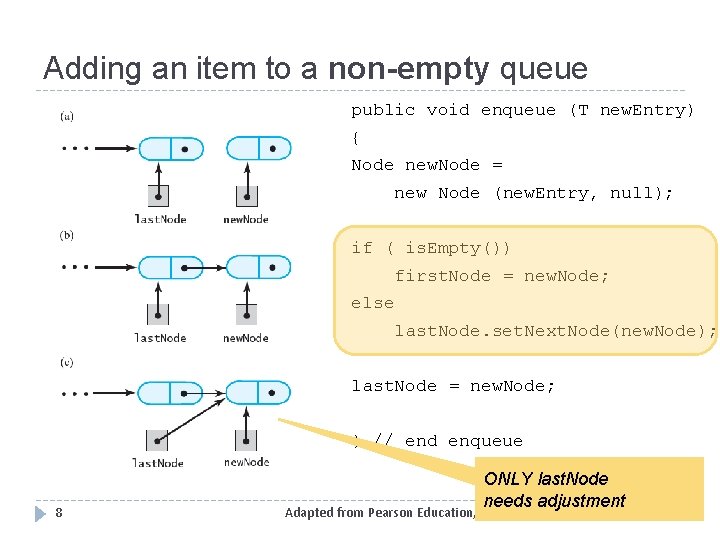
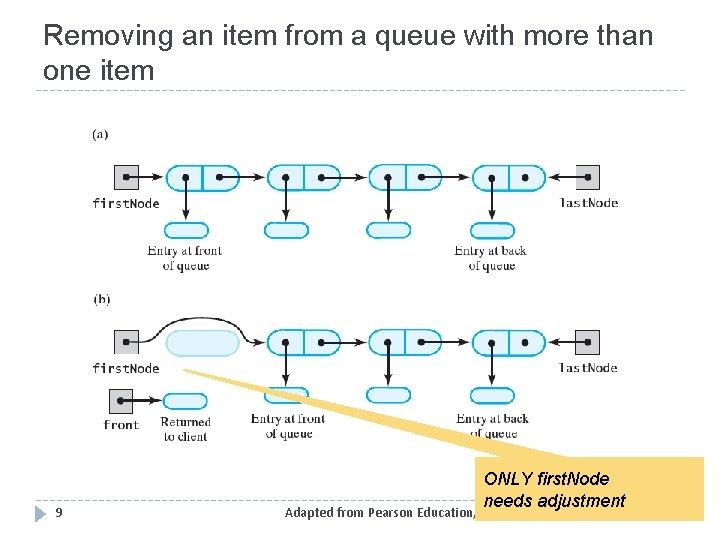
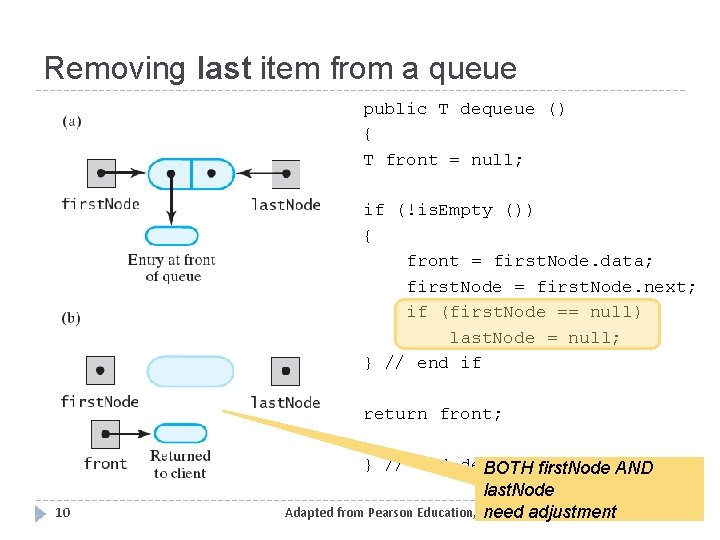
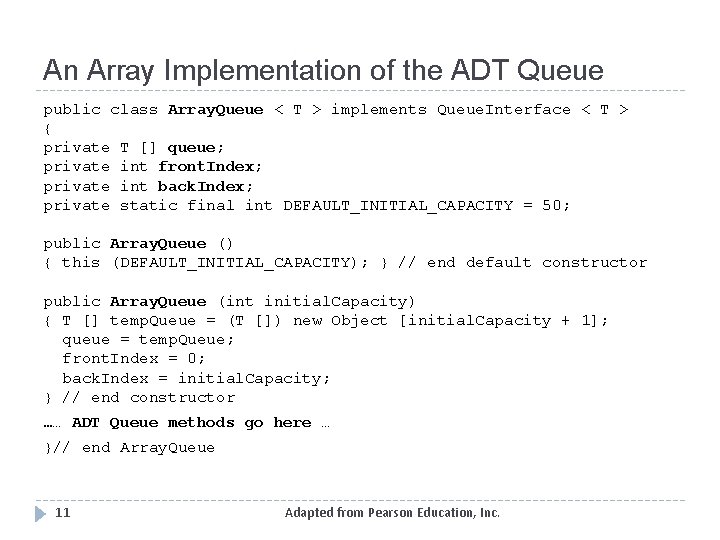
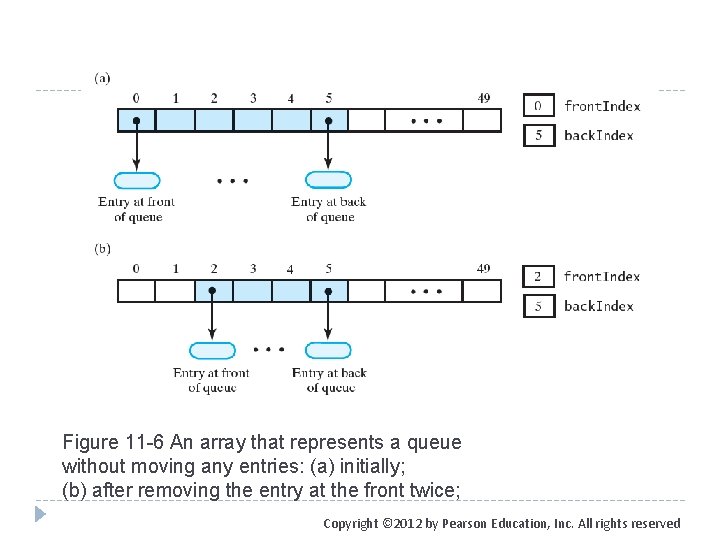
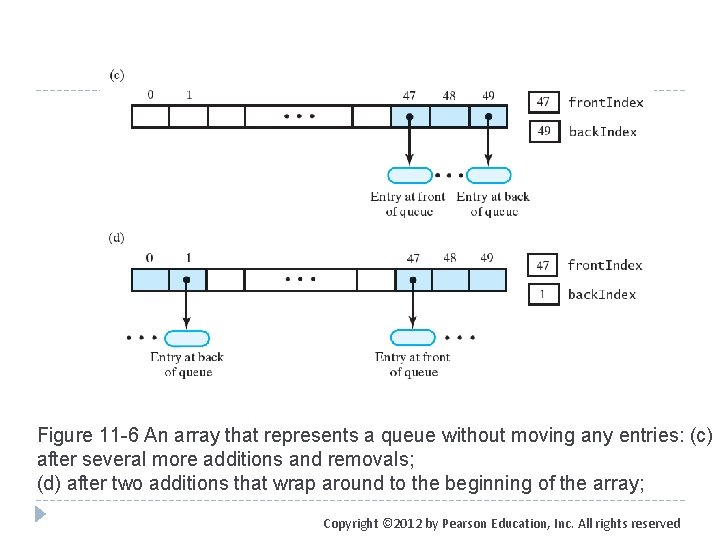
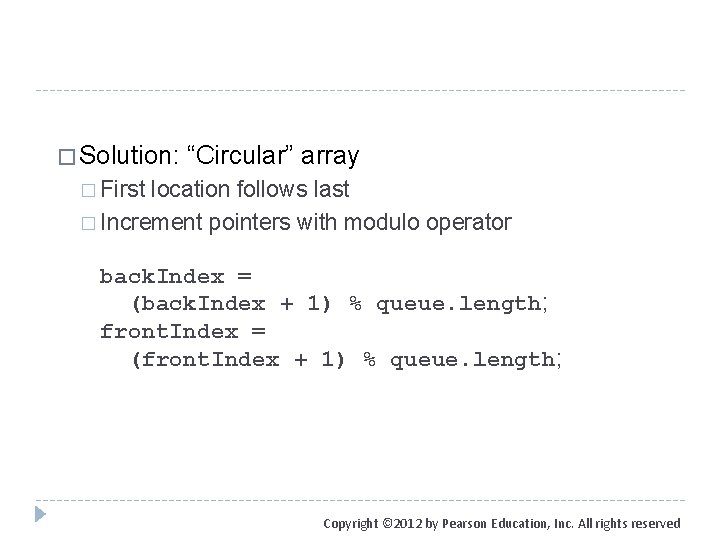
![An empty Queue [0] [4] [1] Front 0 Back 4 Enqueue at back incr An empty Queue [0] [4] [1] Front 0 Back 4 Enqueue at back incr](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-15.jpg)
![en. Queue A [0] A [4] [1] [3] [2] Front 0 Back 0 The en. Queue A [0] A [4] [1] [3] [2] Front 0 Back 0 The](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-16.jpg)
![en. Queue X [0] A [4] [1] X [3] [2] Front 0 Back 1 en. Queue X [0] A [4] [1] X [3] [2] Front 0 Back 1](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-17.jpg)
![en. Queue G, B, and Z – it’s full now [0] A [4] Z en. Queue G, B, and Z – it’s full now [0] A [4] Z](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-18.jpg)
![de. Queue [0] [4] Z [1] X [3] B 19 [2] G Front 1 de. Queue [0] [4] Z [1] X [3] B 19 [2] G Front 1](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-19.jpg)
![de. Queue [0] [4] Z [1] Front 2 Back 4 Let’s enqueue 2 more: de. Queue [0] [4] Z [1] Front 2 Back 4 Let’s enqueue 2 more:](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-20.jpg)
![en. Queue W [0] W [4] Z [1] [3] B 21 [2] G Front en. Queue W [0] W [4] Z [1] [3] B 21 [2] G Front](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-21.jpg)
![en. Queue K – it’s full again [0] W [4] Z [1] K [3] en. Queue K – it’s full again [0] W [4] Z [1] K [3]](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-22.jpg)
![Start with an empty Queue again [0] [4] [1] One Location remains unused [3] Start with an empty Queue again [0] [4] [1] One Location remains unused [3]](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-23.jpg)
![en. Queue A [0] A [4] [1] One Location remains unused [3] [2] Front en. Queue A [0] A [4] [1] One Location remains unused [3] [2] Front](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-24.jpg)
![en. Queue X [0] A [4] [1] X One Location remains unused [3] [2] en. Queue X [0] A [4] [1] X One Location remains unused [3] [2]](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-25.jpg)
![en. Queue G, and B – it’s full now [0] A [4] [3] B en. Queue G, and B – it’s full now [0] A [4] [3] B](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-26.jpg)
![de. Queue [0] [4] [3] B 27 [1] X One Location remains unused [2] de. Queue [0] [4] [3] B 27 [1] X One Location remains unused [2]](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-27.jpg)
![de. Queue [0] [4] [3] B 28 [1] One Location remains unused Front 2 de. Queue [0] [4] [3] B 28 [1] One Location remains unused Front 2](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-28.jpg)
![en. Queue W [0] [4] W [3] B 29 [1] One Location remains unused en. Queue W [0] [4] W [3] B 29 [1] One Location remains unused](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-29.jpg)
![en. Queue K [0] K [4] W [3] B 30 [1] One Location remains en. Queue K [0] K [4] W [3] B 30 [1] One Location remains](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-30.jpg)
![en. Queue Z – can’t do it… already full [0] K [4] W [3] en. Queue Z – can’t do it… already full [0] K [4] W [3]](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-31.jpg)
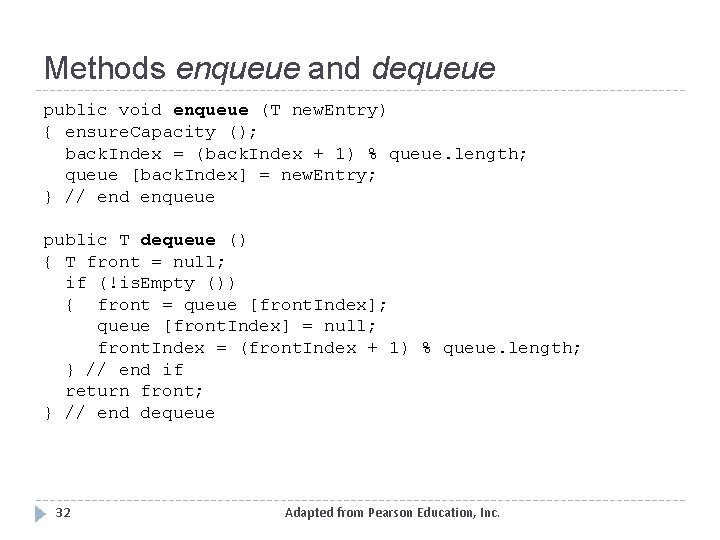
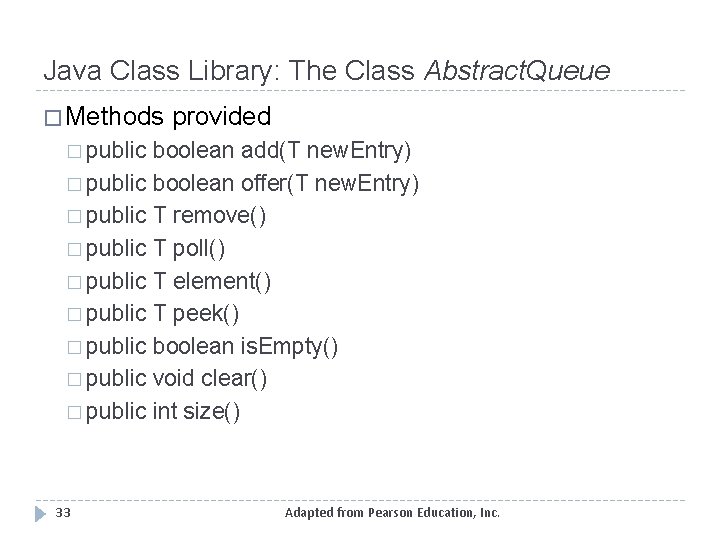
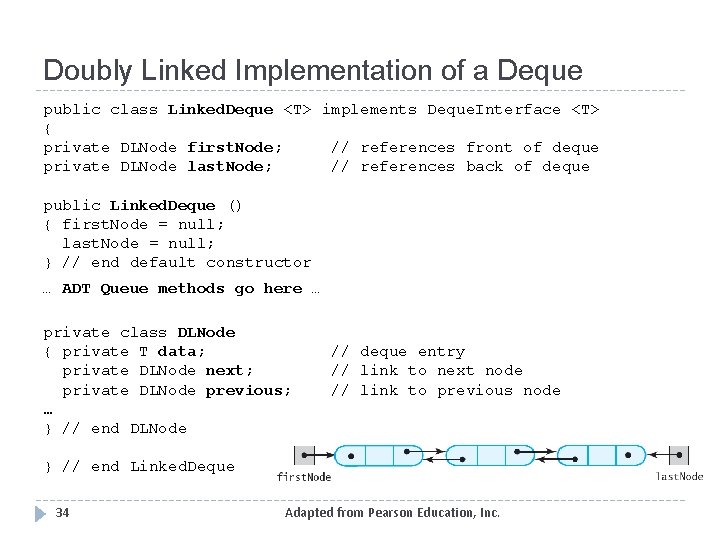
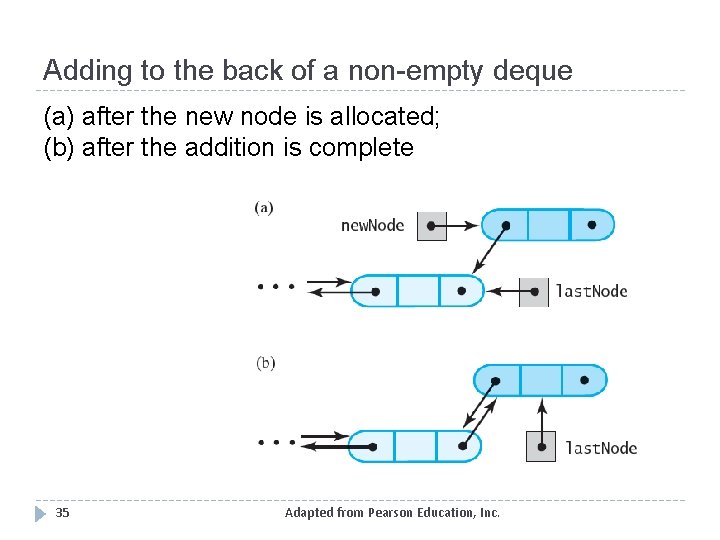
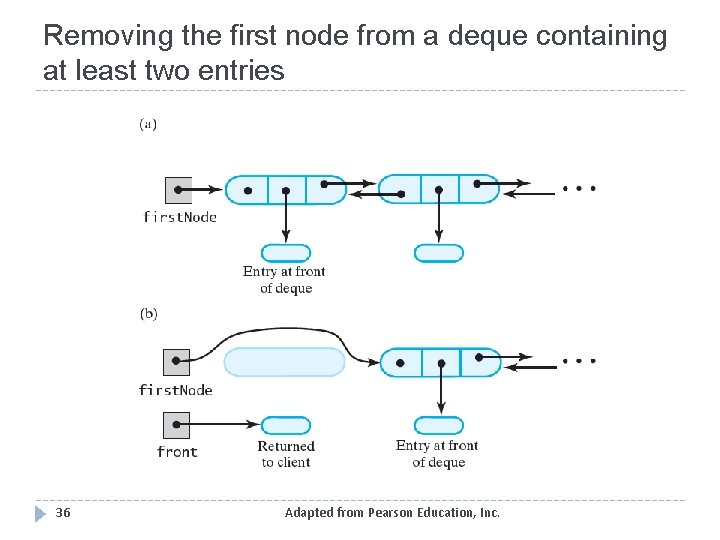
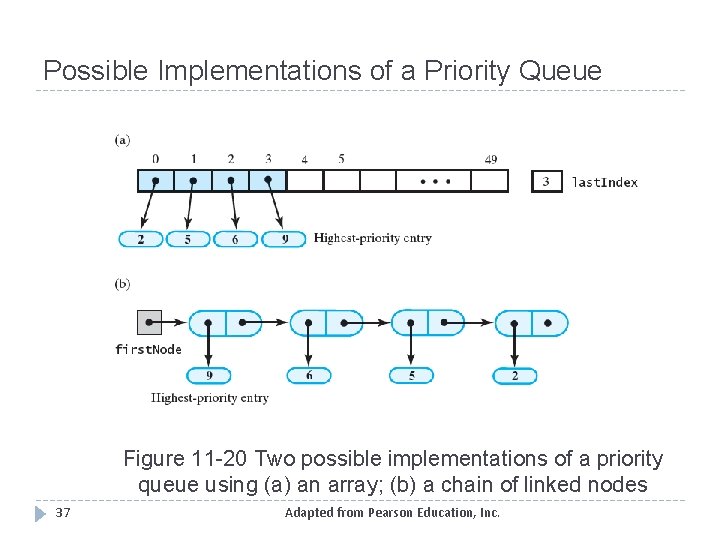
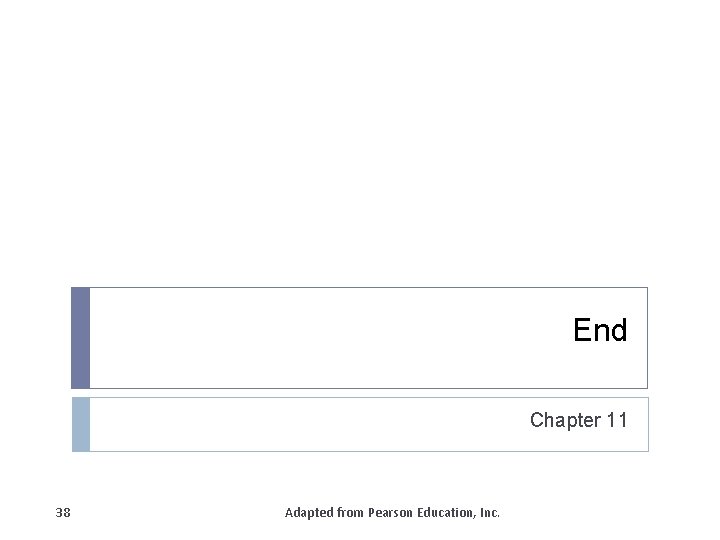
- Slides: 38
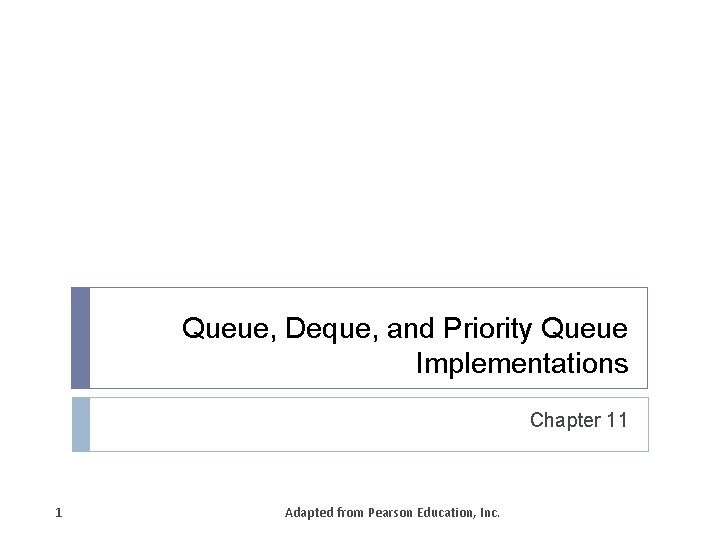
Queue, Deque, and Priority Queue Implementations Chapter 11 1 Adapted from Pearson Education, Inc.
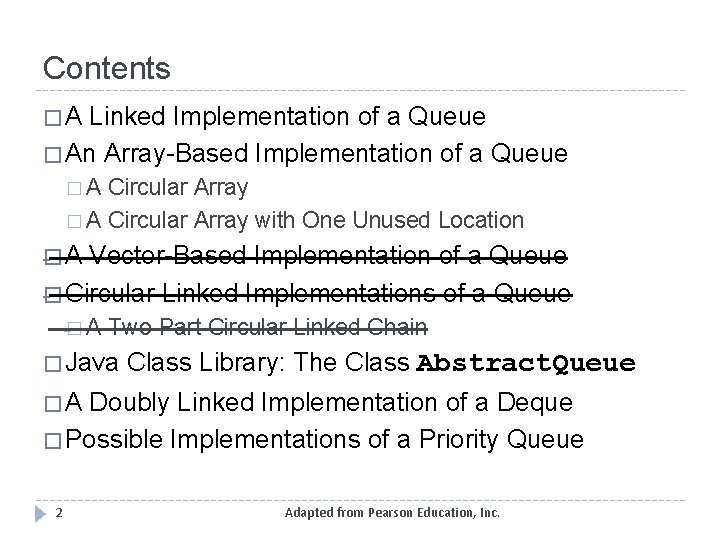
Contents �A Linked Implementation of a Queue � An Array-Based Implementation of a Queue �A Circular Array � A Circular Array with One Unused Location �A Vector-Based Implementation of a Queue � Circular Linked Implementations of a Queue �A Two-Part Circular Linked Chain � Java Class Library: The Class Abstract. Queue �A Doubly Linked Implementation of a Deque � Possible Implementations of a Priority Queue 2 Adapted from Pearson Education, Inc.
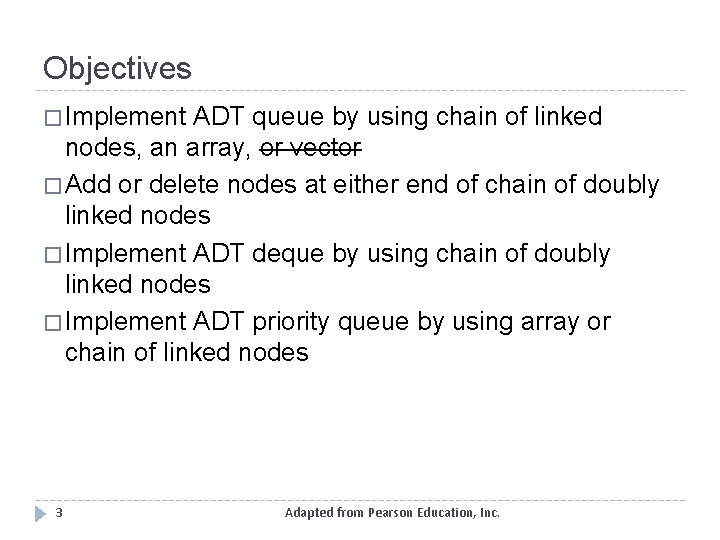
Objectives � Implement ADT queue by using chain of linked nodes, an array, or vector � Add or delete nodes at either end of chain of doubly linked nodes � Implement ADT deque by using chain of doubly linked nodes � Implement ADT priority queue by using array or chain of linked nodes 3 Adapted from Pearson Education, Inc.
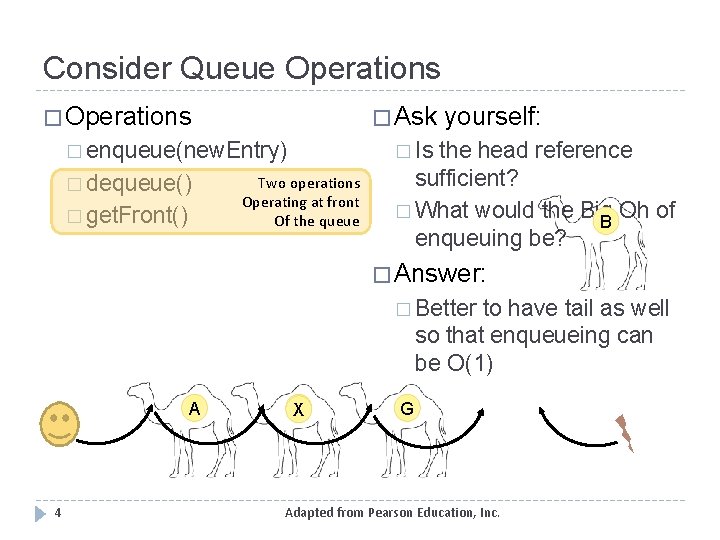
Consider Queue Operations � Ask � Operations � Is � enqueue(new. Entry) � dequeue() � get. Front() yourself: Two operations Operating at front Of the queue the head reference sufficient? � What would the Big Oh of B enqueuing be? � Answer: � Better to have tail as well so that enqueueing can be O(1) A 4 X G Adapted from Pearson Education, Inc.
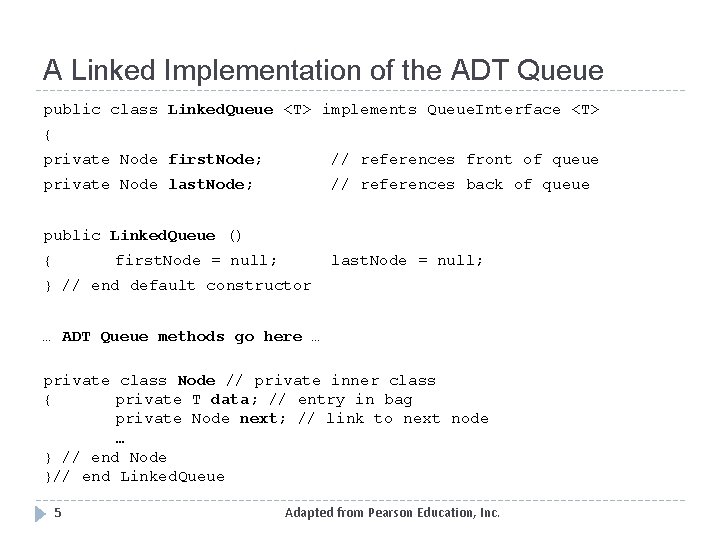
A Linked Implementation of the ADT Queue public class Linked. Queue <T> implements Queue. Interface <T> { private Node first. Node; // references front of queue private Node last. Node; // references back of queue public Linked. Queue () { first. Node = null; last. Node = null; } // end default constructor … ADT Queue methods go here … private class Node // private inner class { private T data; // entry in bag private Node next; // link to next node … } // end Node }// end Linked. Queue 5 Adapted from Pearson Education, Inc.
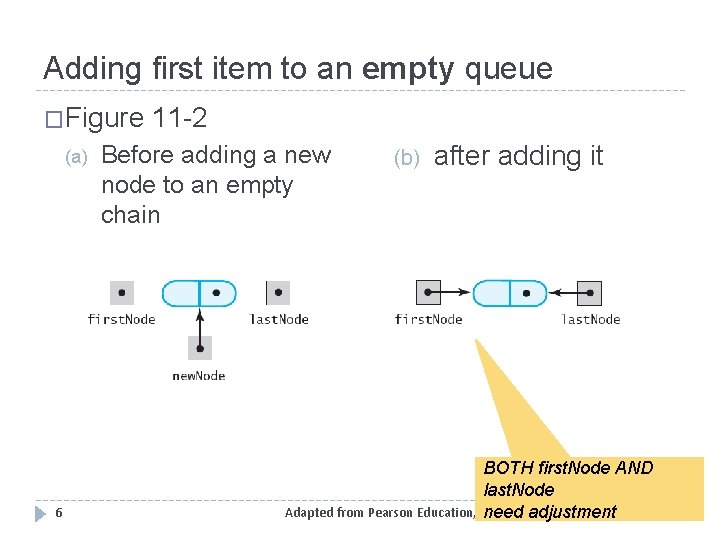
Adding first item to an empty queue �Figure (a) 6 11 -2 Before adding a new node to an empty chain (b) after adding it BOTH first. Node AND last. Node Adapted from Pearson Education, Inc. need adjustment
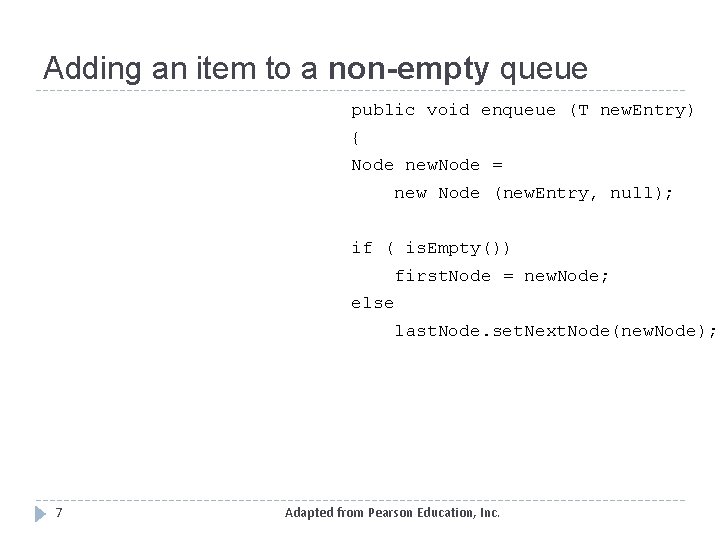
Adding an item to a non-empty queue public void enqueue (T new. Entry) { Node new. Node = new Node (new. Entry, null); if ( is. Empty()) first. Node = new. Node; else last. Node. set. Next. Node(new. Node); 7 Adapted from Pearson Education, Inc.
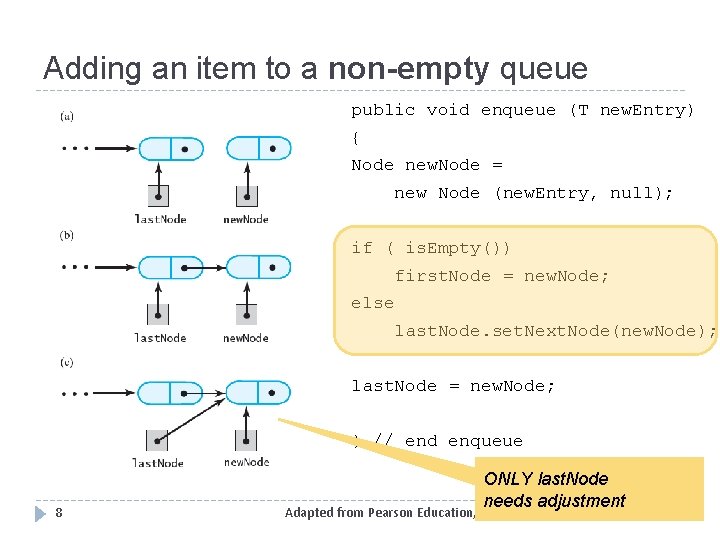
Adding an item to a non-empty queue public void enqueue (T new. Entry) { Node new. Node = new Node (new. Entry, null); if ( is. Empty()) first. Node = new. Node; else last. Node. set. Next. Node(new. Node); last. Node = new. Node; } // end enqueue 8 ONLY last. Node needs adjustment Adapted from Pearson Education, Inc.
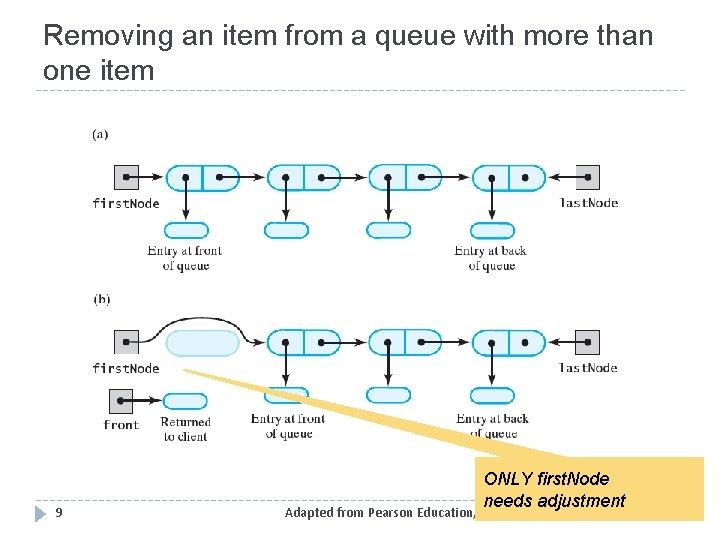
Removing an item from a queue with more than one item 9 ONLY first. Node needs adjustment Adapted from Pearson Education, Inc.
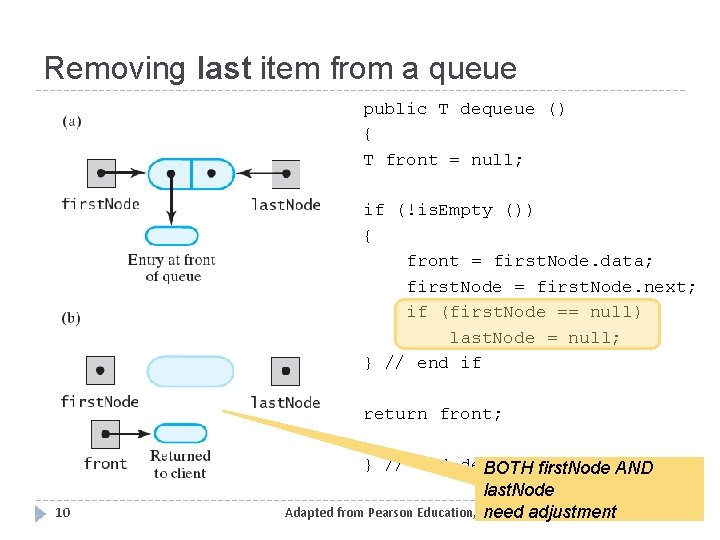
Removing last item from a queue public T dequeue () { T front = null; if (!is. Empty ()) { front = first. Node. data; first. Node = first. Node. next; if (first. Node == null) last. Node = null; } // end if return front; 10 } // end dequeue BOTH first. Node AND last. Node Adapted from Pearson Education, Inc. need adjustment
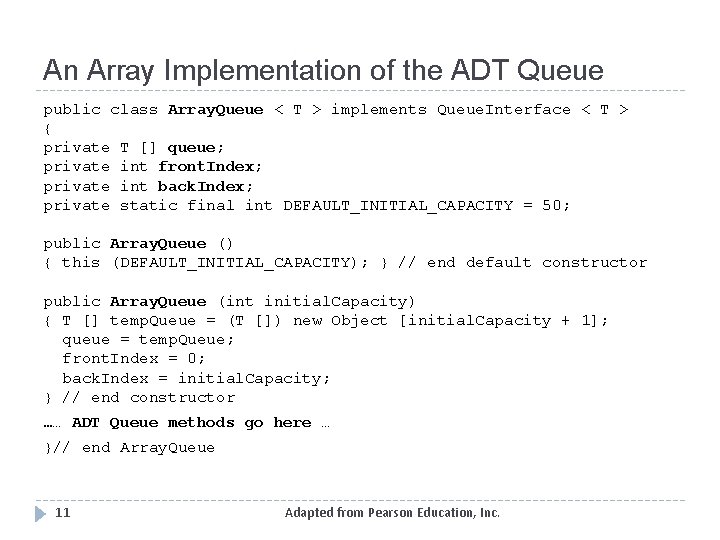
An Array Implementation of the ADT Queue public class Array. Queue < T > implements Queue. Interface < T > { private T [] queue; private int front. Index; private int back. Index; private static final int DEFAULT_INITIAL_CAPACITY = 50; public Array. Queue () { this (DEFAULT_INITIAL_CAPACITY); } // end default constructor public Array. Queue (int initial. Capacity) { T [] temp. Queue = (T []) new Object [initial. Capacity + 1]; queue = temp. Queue; front. Index = 0; back. Index = initial. Capacity; } // end constructor …… ADT Queue methods go here … }// end Array. Queue 11 Adapted from Pearson Education, Inc.
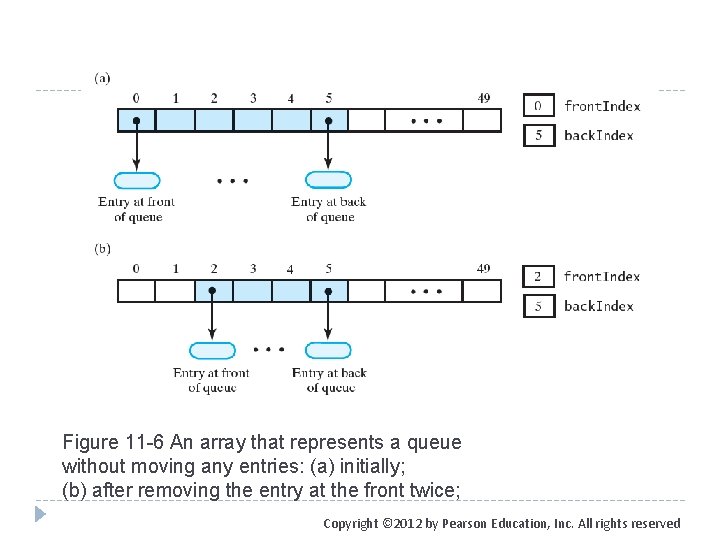
Figure 11 -6 An array that represents a queue without moving any entries: (a) initially; (b) after removing the entry at the front twice; Copyright © 2012 by Pearson Education, Inc. All rights reserved
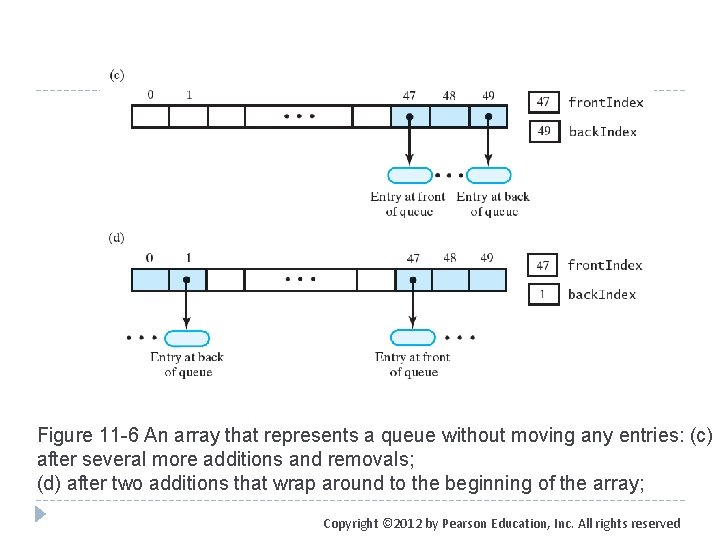
Figure 11 -6 An array that represents a queue without moving any entries: (c) after several more additions and removals; (d) after two additions that wrap around to the beginning of the array; Copyright © 2012 by Pearson Education, Inc. All rights reserved
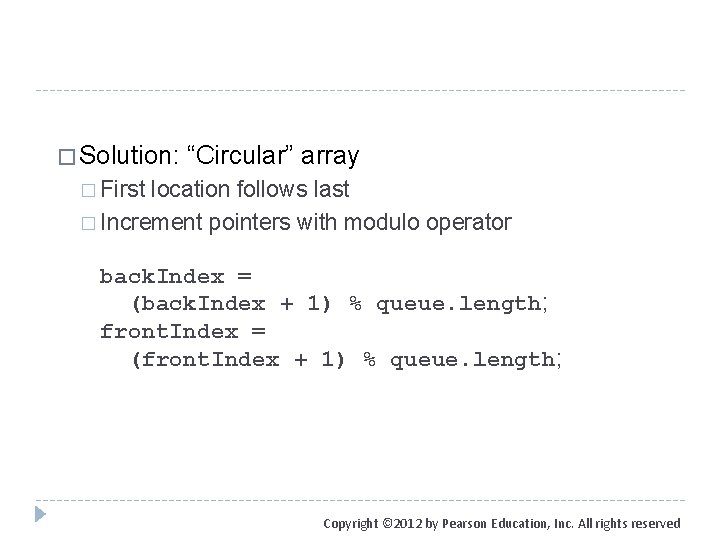
� Solution: “Circular” array � First location follows last � Increment pointers with modulo operator back. Index = (back. Index + 1) % queue. length; front. Index = (front. Index + 1) % queue. length; Copyright © 2012 by Pearson Education, Inc. All rights reserved
![An empty Queue 0 4 1 Front 0 Back 4 Enqueue at back incr An empty Queue [0] [4] [1] Front 0 Back 4 Enqueue at back incr](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-15.jpg)
An empty Queue [0] [4] [1] Front 0 Back 4 Enqueue at back incr back then store item [3] [2] The queue is EMPTY ( Back + 1 ) % size = ( 4 +1)% 5 = 0 == Front = 0 15 Adapted from Pearson Education, Inc.
![en Queue A 0 A 4 1 3 2 Front 0 Back 0 The en. Queue A [0] A [4] [1] [3] [2] Front 0 Back 0 The](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-16.jpg)
en. Queue A [0] A [4] [1] [3] [2] Front 0 Back 0 The queue is not EMPTY ( Back + 1 ) % size = ( 0 +1)% 5 = 1 =/= Front = 0 16 Adapted from Pearson Education, Inc.
![en Queue X 0 A 4 1 X 3 2 Front 0 Back 1 en. Queue X [0] A [4] [1] X [3] [2] Front 0 Back 1](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-17.jpg)
en. Queue X [0] A [4] [1] X [3] [2] Front 0 Back 1 The queue is not EMPTY ( Back + 1 ) % size = ( 1 +1)% 5 = 2 =/= Front = 0 17 Adapted from Pearson Education, Inc.
![en Queue G B and Z its full now 0 A 4 Z en. Queue G, B, and Z – it’s full now [0] A [4] Z](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-18.jpg)
en. Queue G, B, and Z – it’s full now [0] A [4] Z [1] X Same As When Queue Was empty Front 0 Back 4 Dequeue from Front Serve front then incr front [3] B 18 [2] G The queue is FULL BUT ( Back + 1 ) % size = ( 4 +1)% 5 = 0 == Front = 0 Adapted from Pearson Education, Inc.
![de Queue 0 4 Z 1 X 3 B 19 2 G Front 1 de. Queue [0] [4] Z [1] X [3] B 19 [2] G Front 1](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-19.jpg)
de. Queue [0] [4] Z [1] X [3] B 19 [2] G Front 1 Back 4 The queue is not Empty And not full ( Back + 1 ) % size = ( 4 +1)% 5 = 0 =/= Front = 1 Adapted from Pearson Education, Inc.
![de Queue 0 4 Z 1 Front 2 Back 4 Lets enqueue 2 more de. Queue [0] [4] Z [1] Front 2 Back 4 Let’s enqueue 2 more:](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-20.jpg)
de. Queue [0] [4] Z [1] Front 2 Back 4 Let’s enqueue 2 more: W and K [3] B 20 [2] G The queue is not Empty ( Back + 1 ) % size = ( 4 +1)% 5 = 0 =/= Front = 2 Adapted from Pearson Education, Inc.
![en Queue W 0 W 4 Z 1 3 B 21 2 G Front en. Queue W [0] W [4] Z [1] [3] B 21 [2] G Front](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-21.jpg)
en. Queue W [0] W [4] Z [1] [3] B 21 [2] G Front 2 Back 0 The queue is not Empty ( Back + 1 ) % size = ( 0 +1)% 5 = 1 =/= Front = 2 Adapted from Pearson Education, Inc.
![en Queue K its full again 0 W 4 Z 1 K 3 en. Queue K – it’s full again [0] W [4] Z [1] K [3]](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-22.jpg)
en. Queue K – it’s full again [0] W [4] Z [1] K [3] B 22 [2] G Front 2 Back 1 How can we avoid the condition for empty and full being the same? The queue is FULL BUT ( Back + 1 ) % size = ( 1 +1)% 5 = 2 == Front = 2 Adapted from Pearson Education, Inc.
![Start with an empty Queue again 0 4 1 One Location remains unused 3 Start with an empty Queue again [0] [4] [1] One Location remains unused [3]](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-23.jpg)
Start with an empty Queue again [0] [4] [1] One Location remains unused [3] [2] Front 0 Back 4 The queue is EMPTY ( Back + 1 ) % size = ( 4 +1)% 5 = 0 == Front = 0 23 Adapted from Pearson Education, Inc.
![en Queue A 0 A 4 1 One Location remains unused 3 2 Front en. Queue A [0] A [4] [1] One Location remains unused [3] [2] Front](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-24.jpg)
en. Queue A [0] A [4] [1] One Location remains unused [3] [2] Front 0 Back 0 The queue is not EMPTY ( Back + 1 ) % size = ( 0 +1)% 5 = 1 =/= Front = 0 24 Adapted from Pearson Education, Inc.
![en Queue X 0 A 4 1 X One Location remains unused 3 2 en. Queue X [0] A [4] [1] X One Location remains unused [3] [2]](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-25.jpg)
en. Queue X [0] A [4] [1] X One Location remains unused [3] [2] Front 0 Back 1 The queue is not EMPTY ( Back + 1 ) % size = ( 1 +1)% 5 = 2 =/= Front = 1 25 Adapted from Pearson Education, Inc.
![en Queue G and B its full now 0 A 4 3 B en. Queue G, and B – it’s full now [0] A [4] [3] B](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-26.jpg)
en. Queue G, and B – it’s full now [0] A [4] [3] B 26 [1] X One Location remains unused [2] G Different From When Queue Was empty Front 0 Back 3 The queue is FULL ( Back + 2 ) % size = ( 3 +2)% 5 = 0 == Front = 0 Adapted from Pearson Education, Inc.
![de Queue 0 4 3 B 27 1 X One Location remains unused 2 de. Queue [0] [4] [3] B 27 [1] X One Location remains unused [2]](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-27.jpg)
de. Queue [0] [4] [3] B 27 [1] X One Location remains unused [2] G Front 1 Back 3 The queue is not Empty And not full ( Back + 2 ) % size = ( 3 +2)% 5 = 0 =/= Front = 1 Adapted from Pearson Education, Inc.
![de Queue 0 4 3 B 28 1 One Location remains unused Front 2 de. Queue [0] [4] [3] B 28 [1] One Location remains unused Front 2](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-28.jpg)
de. Queue [0] [4] [3] B 28 [1] One Location remains unused Front 2 Back 3 Let’s enqueue 2 more: W and K [2] G The queue is not Empty ( Back + 1 ) % size = ( 3 +1)% 5 = 4 =/= Front = 2 Adapted from Pearson Education, Inc.
![en Queue W 0 4 W 3 B 29 1 One Location remains unused en. Queue W [0] [4] W [3] B 29 [1] One Location remains unused](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-29.jpg)
en. Queue W [0] [4] W [3] B 29 [1] One Location remains unused [2] G Front 2 Back 4 The queue is not Empty ( Back + 1 ) % size = ( 4 +1)% 5 = 0 =/= Front = 2 Adapted from Pearson Education, Inc.
![en Queue K 0 K 4 W 3 B 30 1 One Location remains en. Queue K [0] K [4] W [3] B 30 [1] One Location remains](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-30.jpg)
en. Queue K [0] K [4] W [3] B 30 [1] One Location remains unused Front 2 Back 0 Let’s enqueue 1 more: Z [2] G The queue is not Empty ( Back + 1 ) % size = ( 0 +1)% 5 = 1 =/= Front = 2 Adapted from Pearson Education, Inc.
![en Queue Z cant do it already full 0 K 4 W 3 en. Queue Z – can’t do it… already full [0] K [4] W [3]](https://slidetodoc.com/presentation_image_h2/5e85e8daa1bcfd5d2a10867a40676b80/image-31.jpg)
en. Queue Z – can’t do it… already full [0] K [4] W [3] B 31 [1] One Location remains unused [2] G Front 2 Back 0 The queue is FULL ( Back + 2 ) % size = ( 0 +2)% 5 = 2 == Front = 2 Adapted from Pearson Education, Inc.
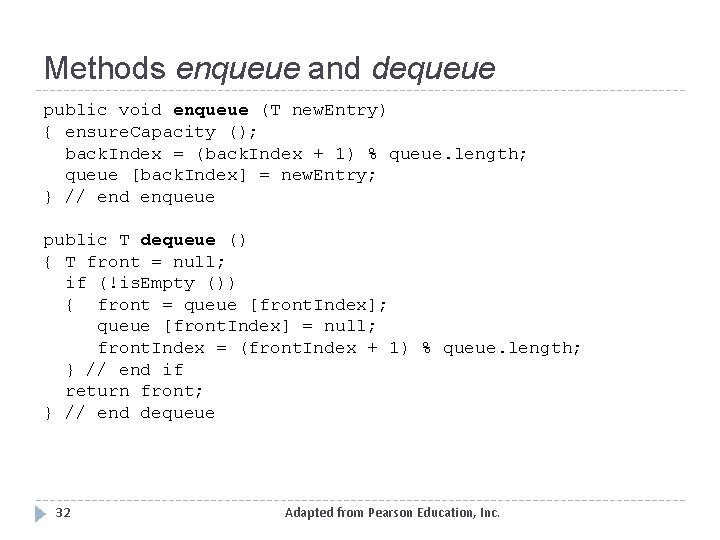
Methods enqueue and dequeue public void enqueue (T new. Entry) { ensure. Capacity (); back. Index = (back. Index + 1) % queue. length; queue [back. Index] = new. Entry; } // end enqueue public T dequeue () { T front = null; if (!is. Empty ()) { front = queue [front. Index]; queue [front. Index] = null; front. Index = (front. Index + 1) % queue. length; } // end if return front; } // end dequeue 32 Adapted from Pearson Education, Inc.
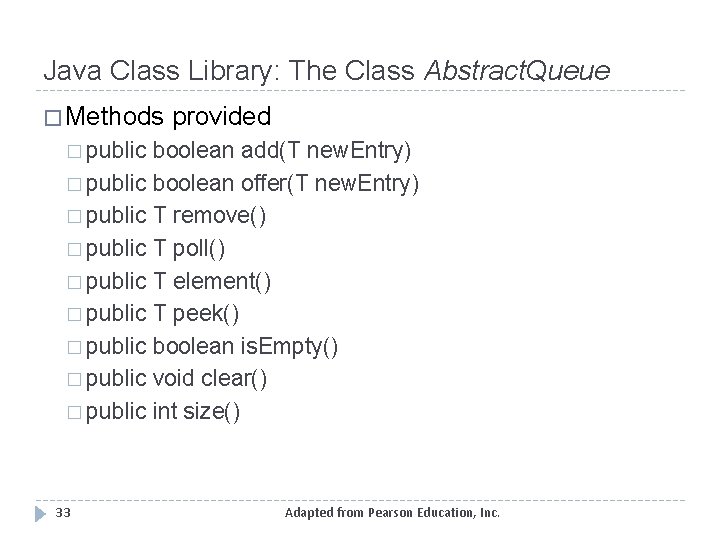
Java Class Library: The Class Abstract. Queue � Methods provided � public boolean add(T new. Entry) � public boolean offer(T new. Entry) � public T remove() � public T poll() � public T element() � public T peek() � public boolean is. Empty() � public void clear() � public int size() 33 Adapted from Pearson Education, Inc.
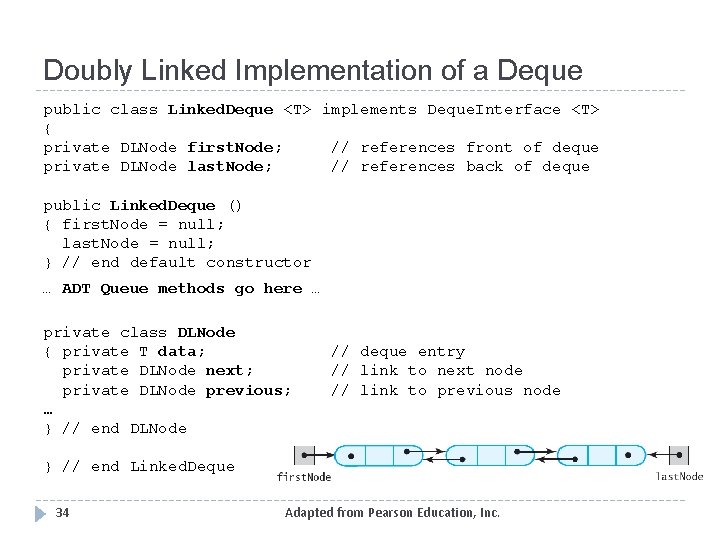
Doubly Linked Implementation of a Deque public class Linked. Deque <T> implements Deque. Interface <T> { private DLNode first. Node; // references front of deque private DLNode last. Node; // references back of deque public Linked. Deque () { first. Node = null; last. Node = null; } // end default constructor … ADT Queue methods go here … private class DLNode { private T data; private DLNode next; private DLNode previous; … } // end DLNode // deque entry // link to next node // link to previous node } // end Linked. Deque 34 Adapted from Pearson Education, Inc.
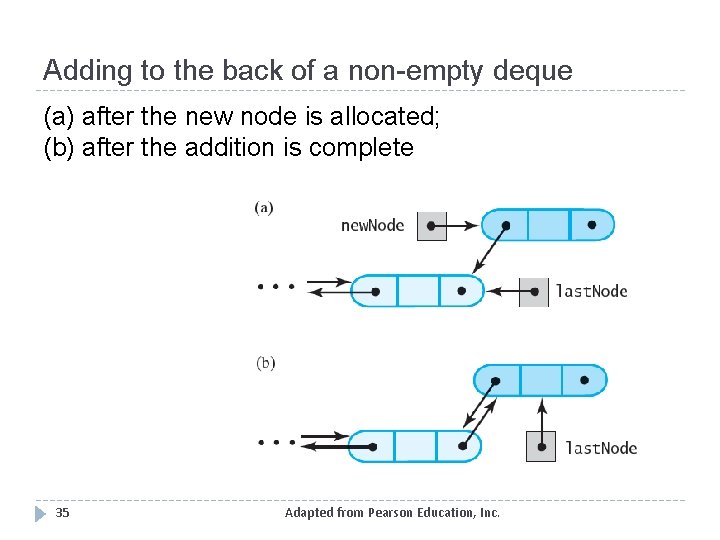
Adding to the back of a non-empty deque (a) after the new node is allocated; (b) after the addition is complete 35 Adapted from Pearson Education, Inc.
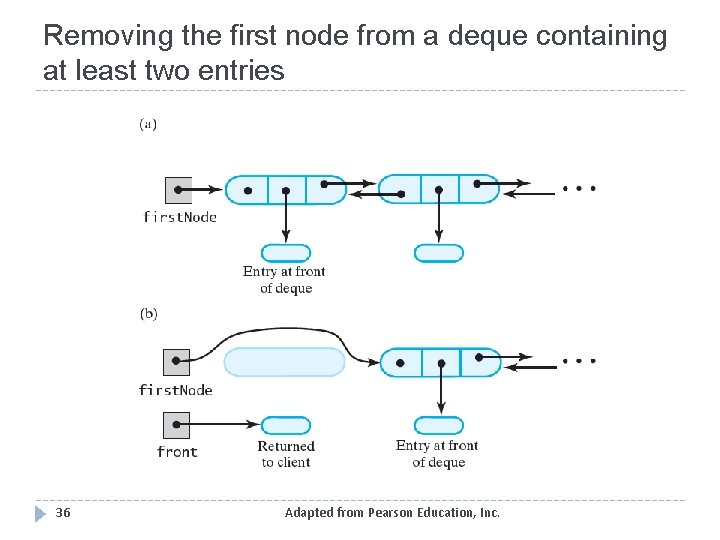
Removing the first node from a deque containing at least two entries 36 Adapted from Pearson Education, Inc.
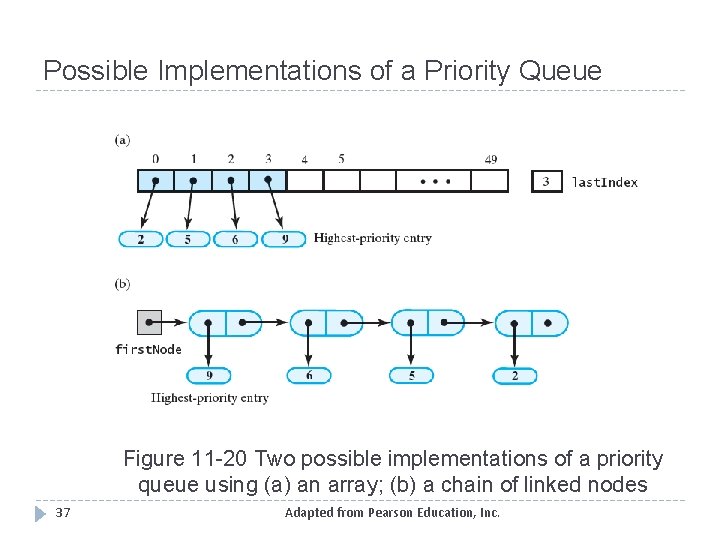
Possible Implementations of a Priority Queue Figure 11 -20 Two possible implementations of a priority queue using (a) an array; (b) a chain of linked nodes 37 Adapted from Pearson Education, Inc.
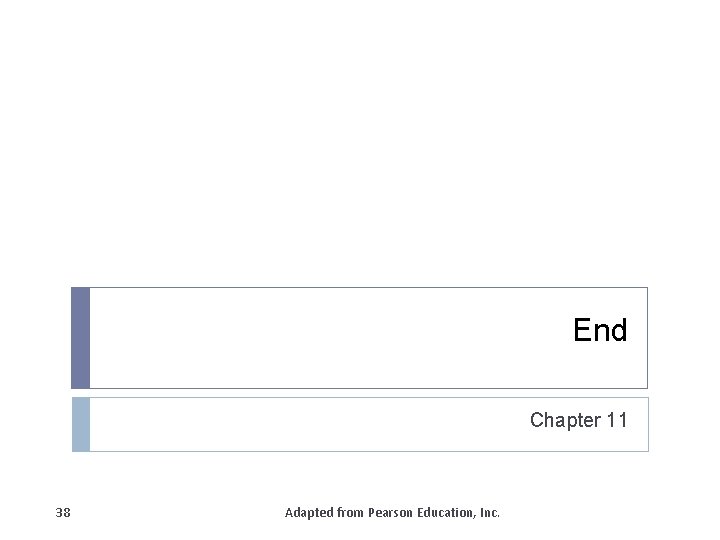
End Chapter 11 38 Adapted from Pearson Education, Inc.
Deque and priority queue
Output restricted deque
Burman's priority list gives priority to
Priority mail vs priority mail express
Deque salman
Deque salman
In a rootisharraystack, a call to get(20) will return
Deque falls
Min-priority queue
Priority queue abstract data type
Insert element in priority queue
Priority queue using heap
Double-ended priority queue
Priority queue doubly linked list
Apache kafka message queue
Priority queue order
Adaptable priority queue java
Adaptable priority queues
Priority queue lower bound
Transform and conquer technique
Operasi dasar pada queue
The queue summary
Priority queue animation
Smalltalk example
What are the common standard ethernet implementations?
Ethics in security management
Chapter 3 queue
Use case priority matrix for system
Use case ranking and priority matrix
Use case ranking and priority matrix images
Use case ranking and priority matrix
Use case ranking and priority matrix images
Use case ranking and priority matrix images
Priority of sendai framework
Priority inheritance
Stakeholder priority matrix
Sendai framework guiding principles
Verilog operator
Hit another home run strategy