Data Structures and Algorithms Data Structure Lecture Queues
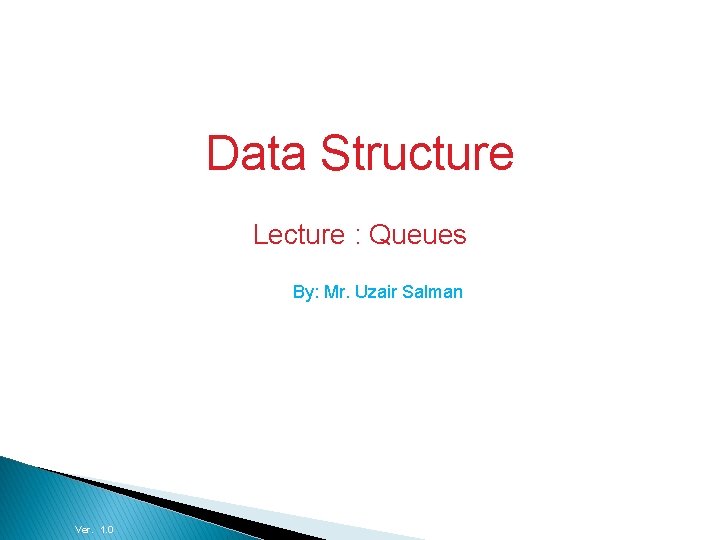
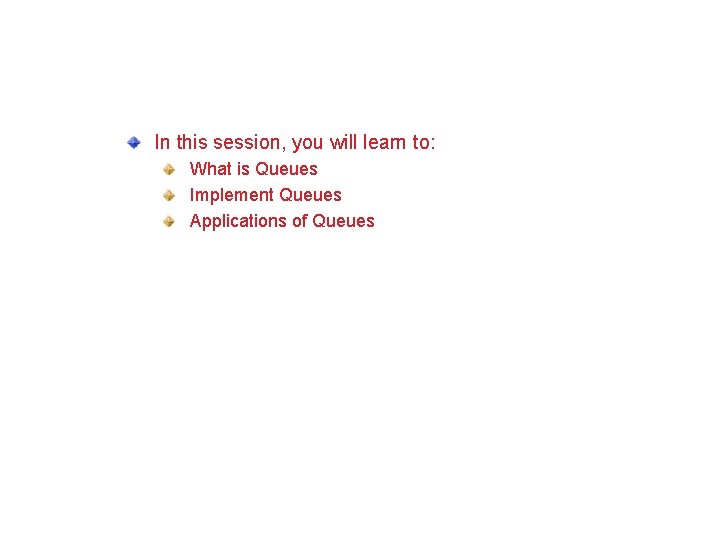
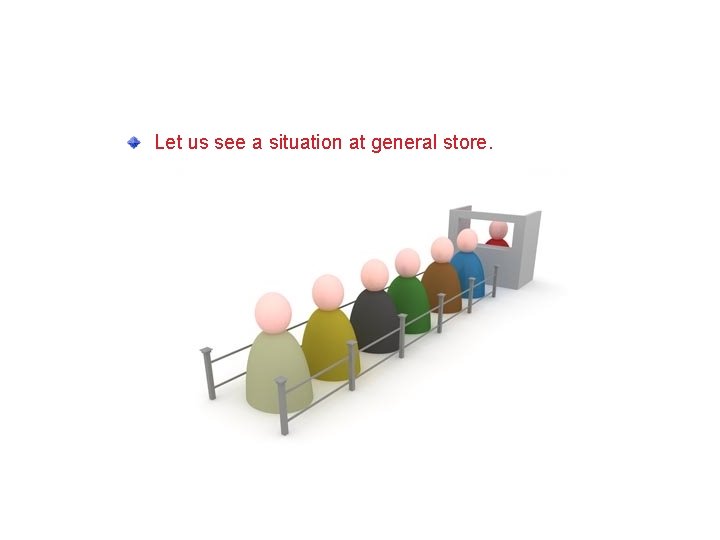
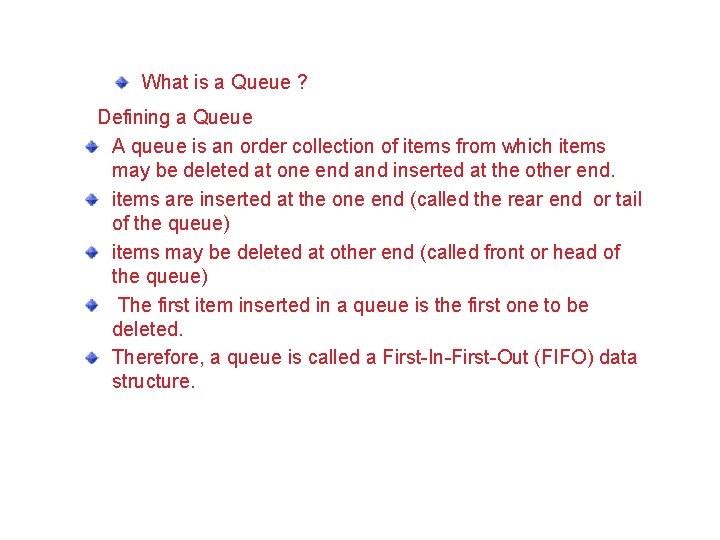
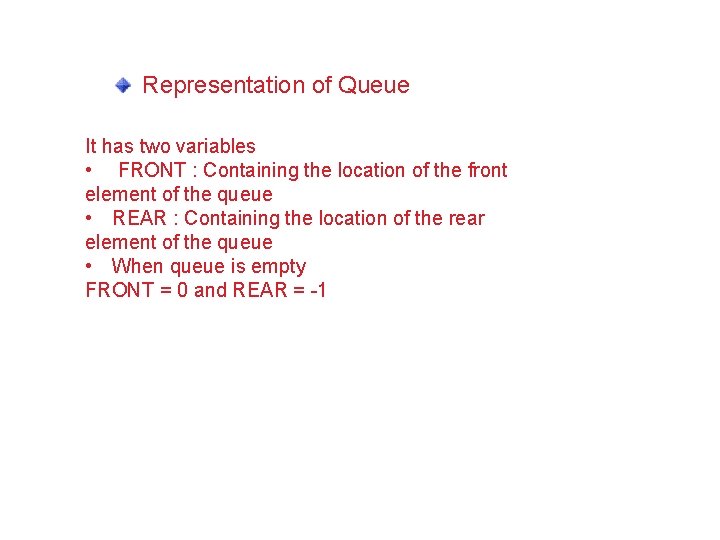
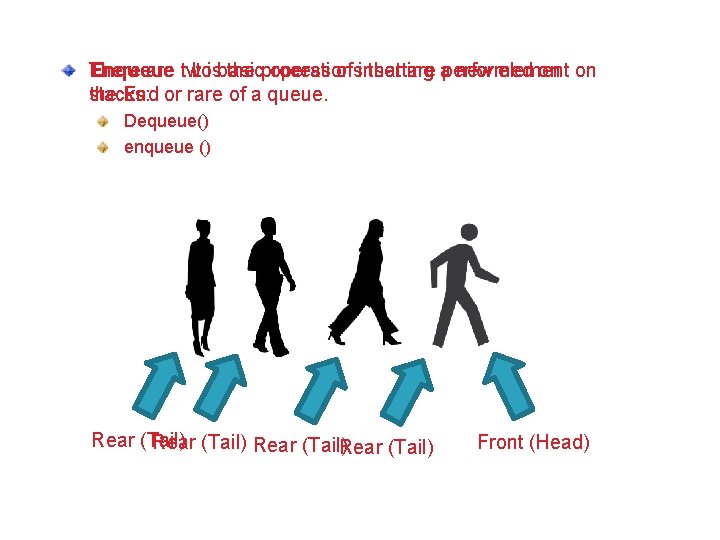
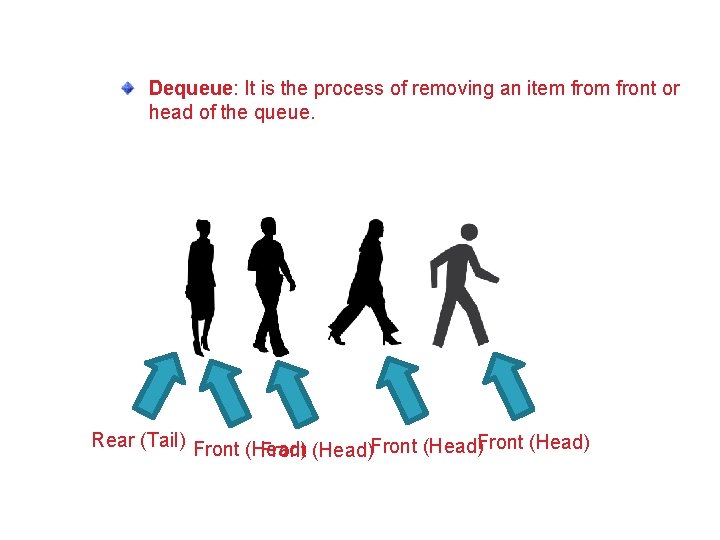
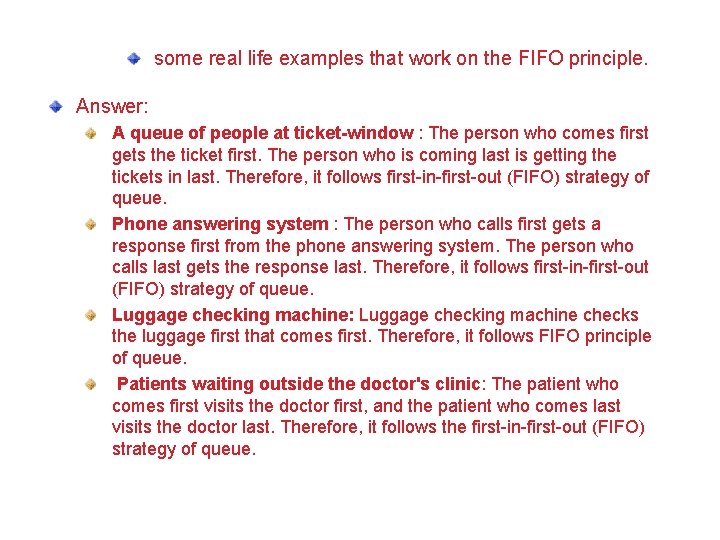
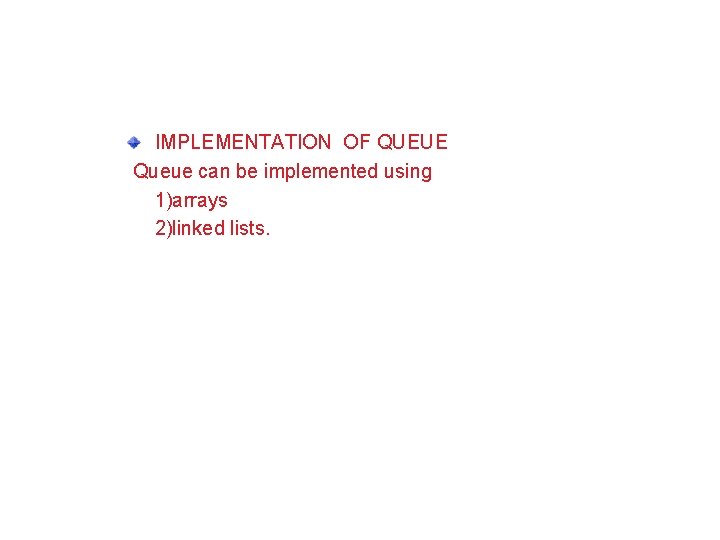
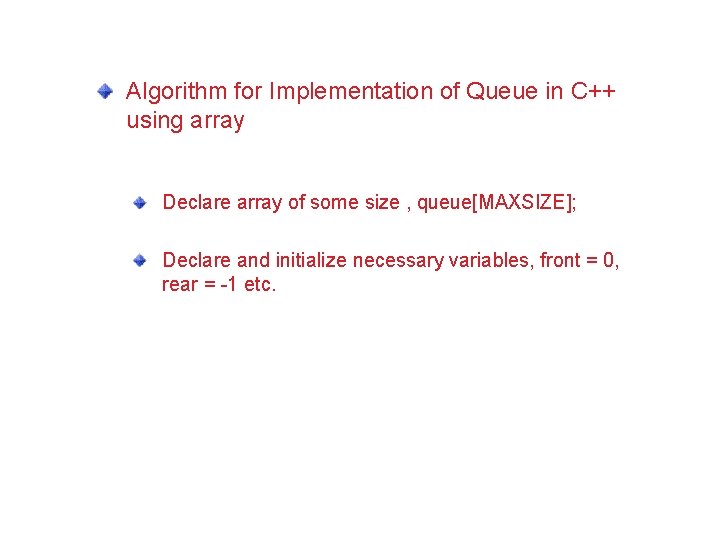
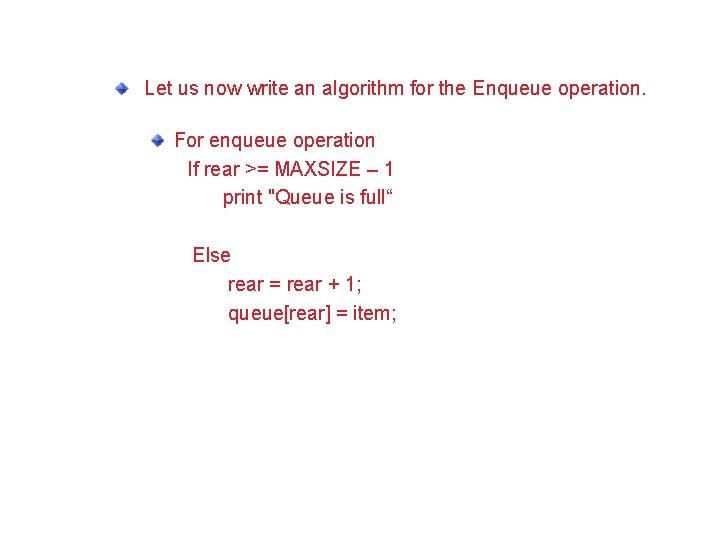
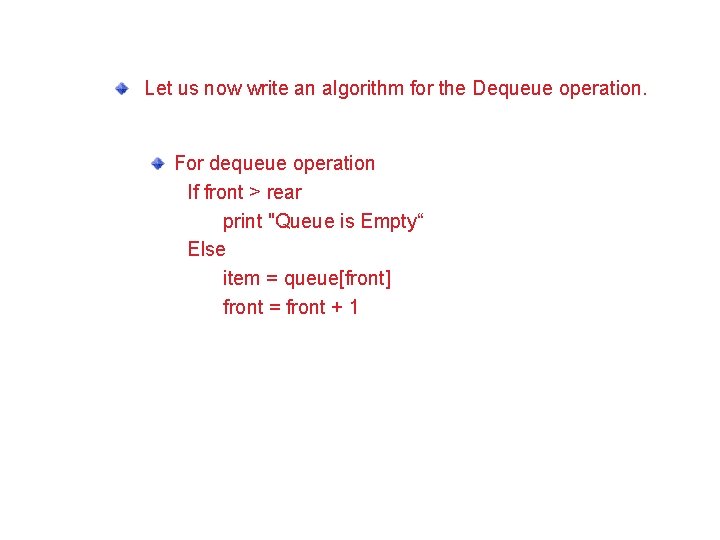
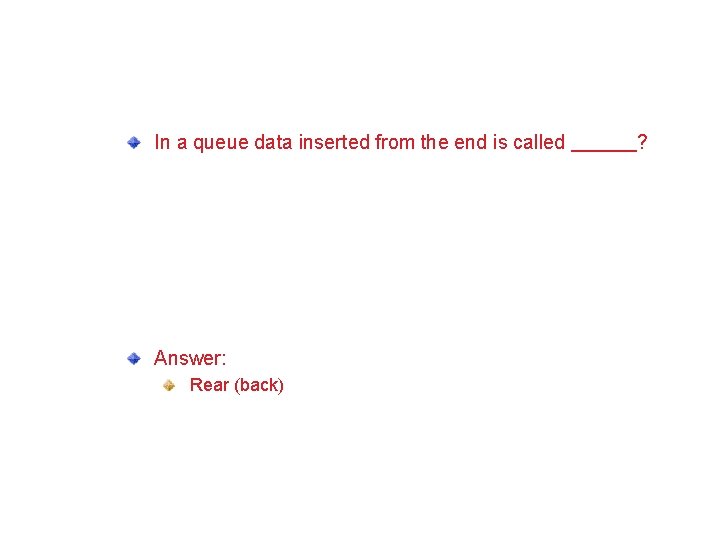
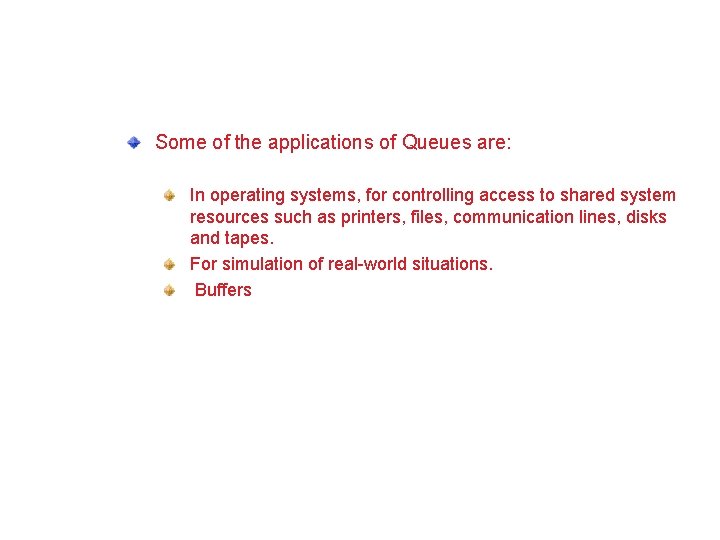
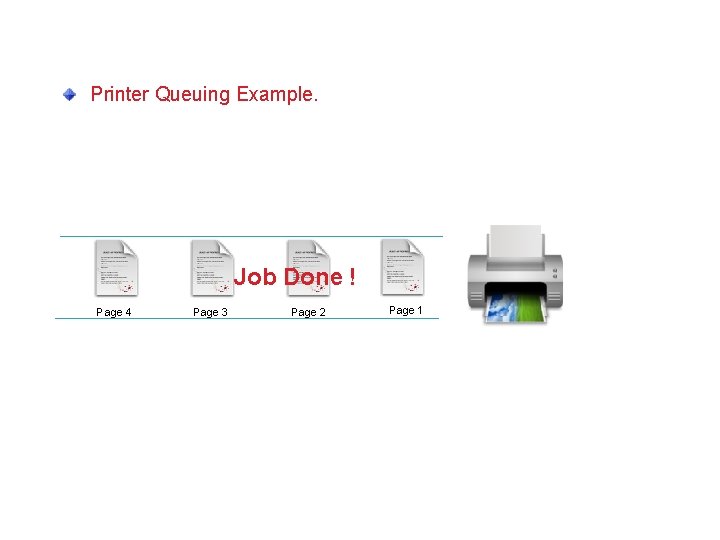
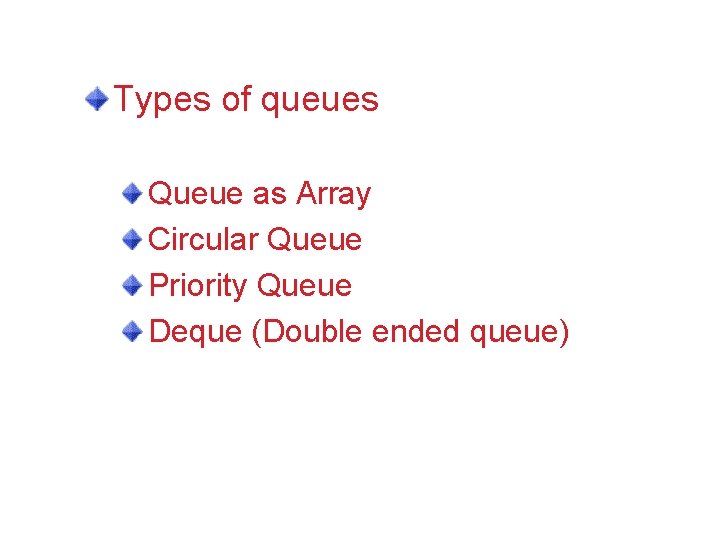
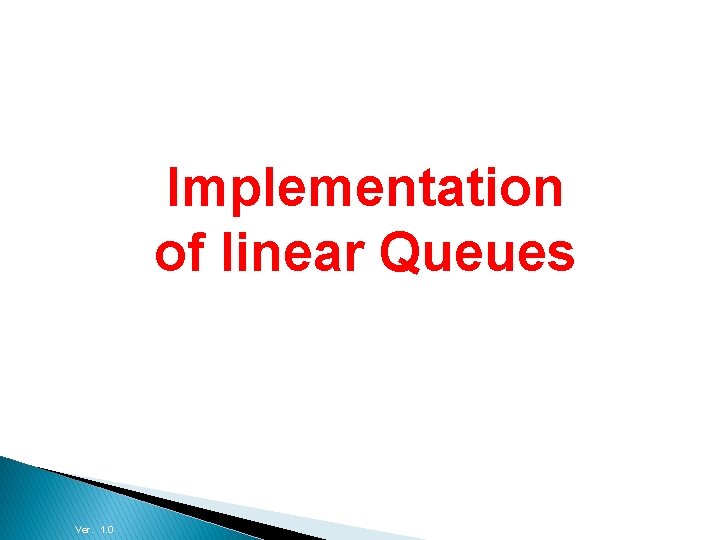
![Class queue { private: int size; int queue[size]; int front; int rear; public: queue() Class queue { private: int size; int queue[size]; int front; int rear; public: queue()](https://slidetodoc.com/presentation_image_h2/4b57158698b101b79a8dea92ff23dafa/image-18.jpg)
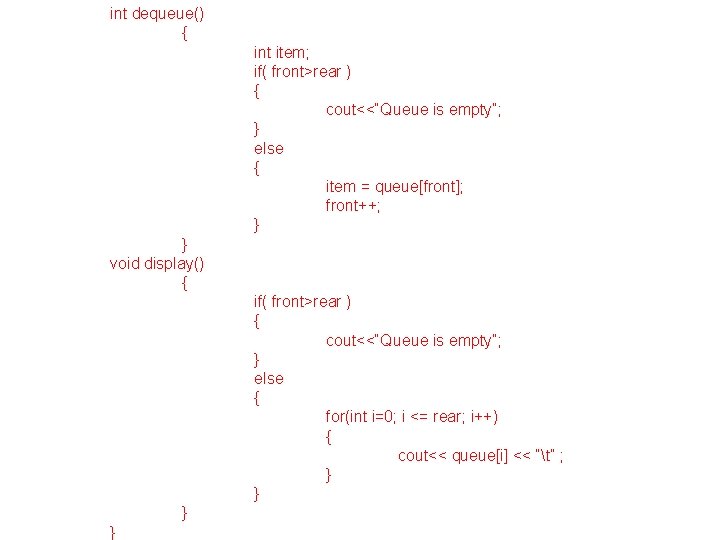
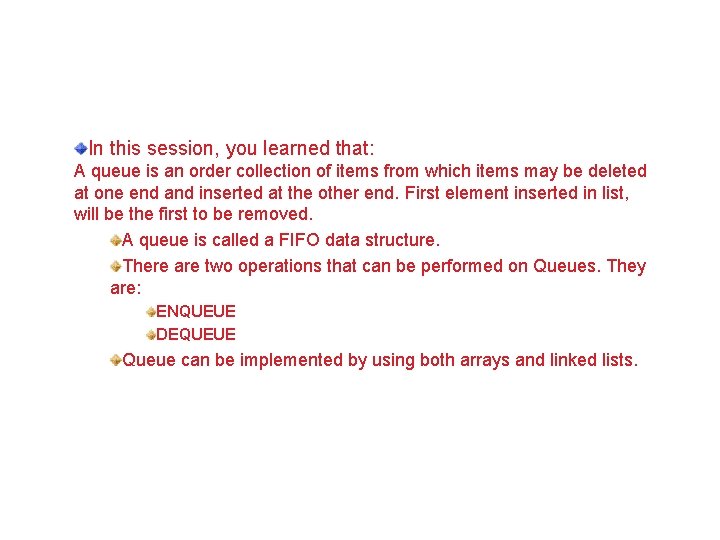
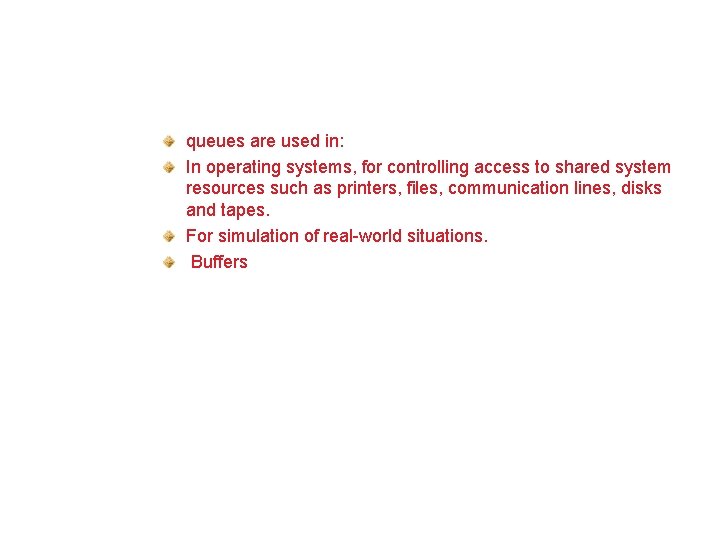
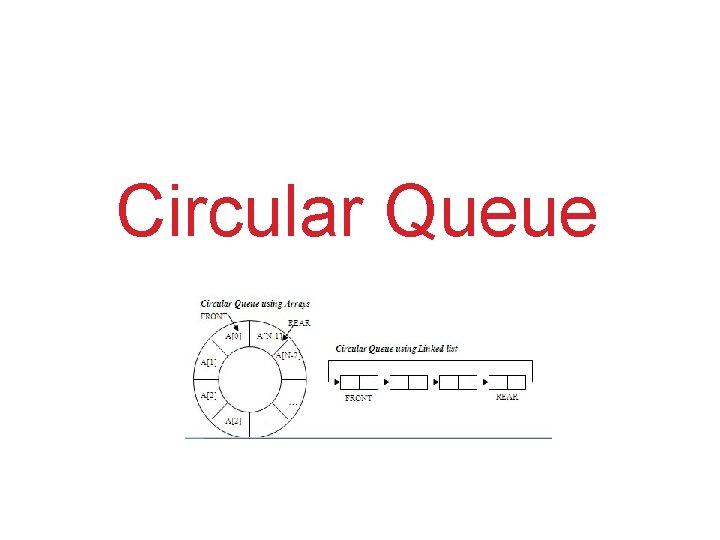
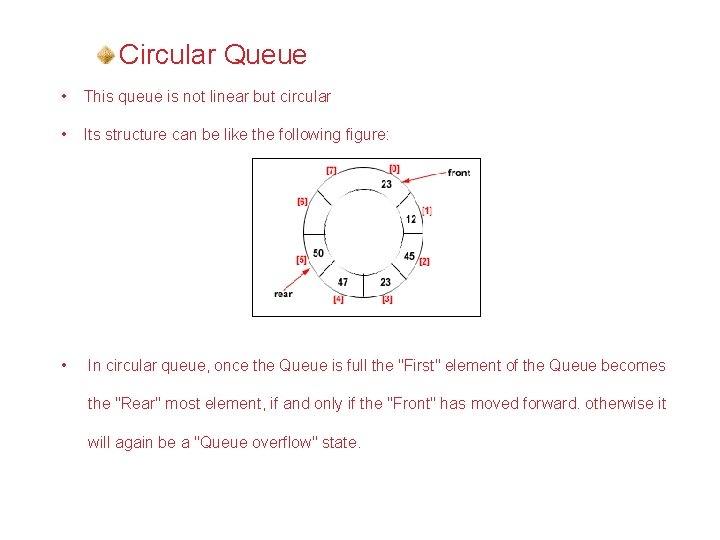
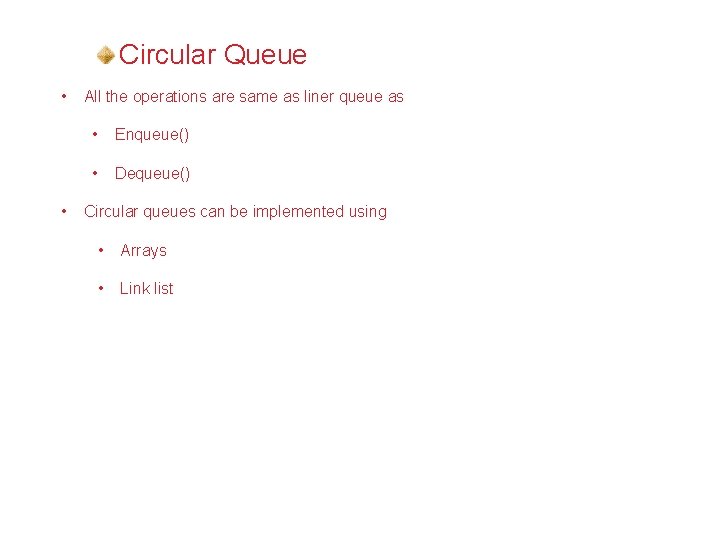
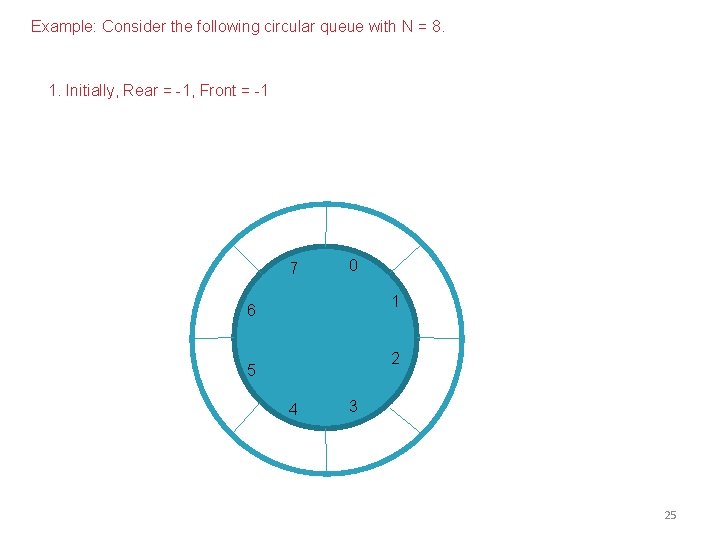
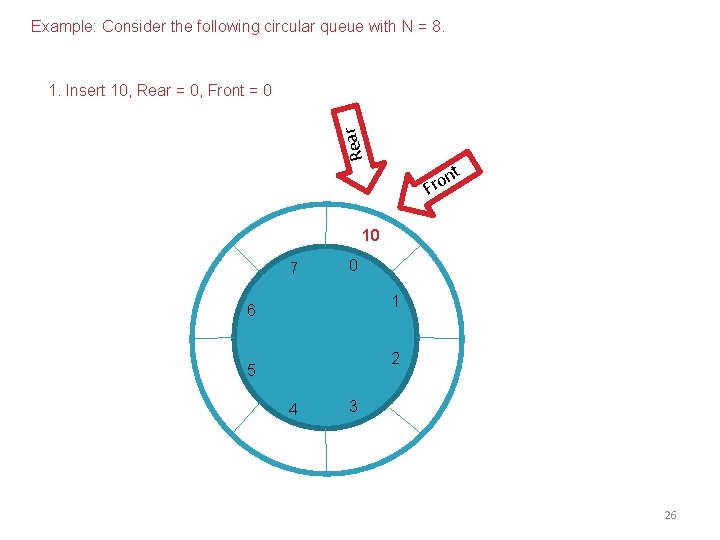
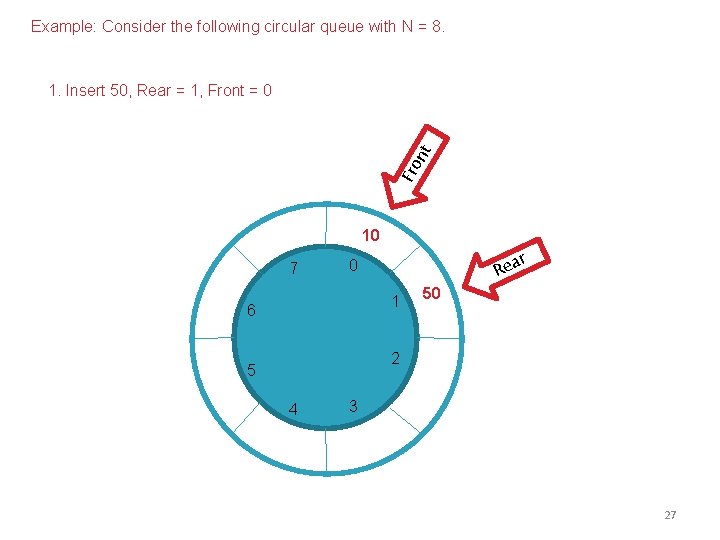
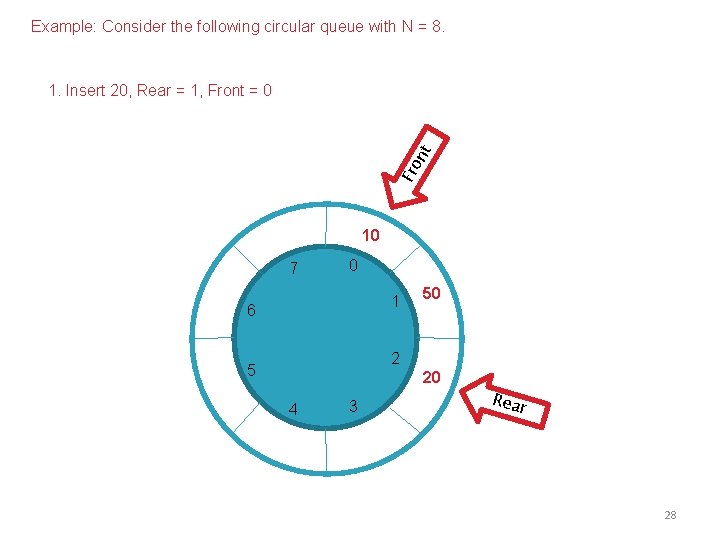
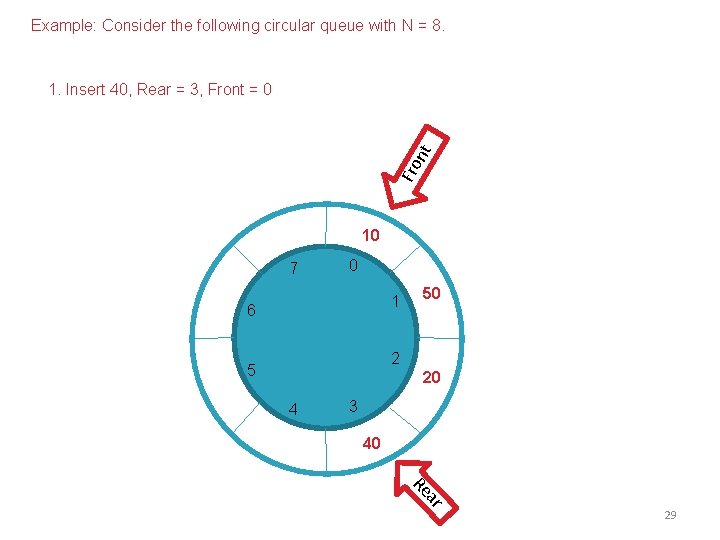
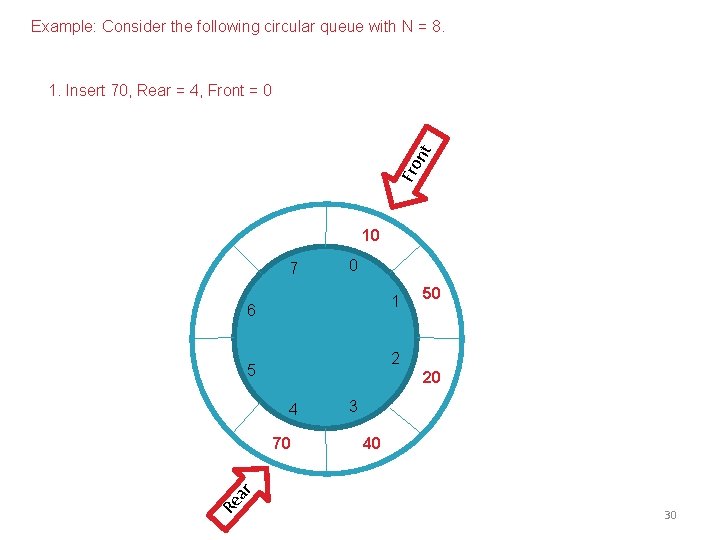
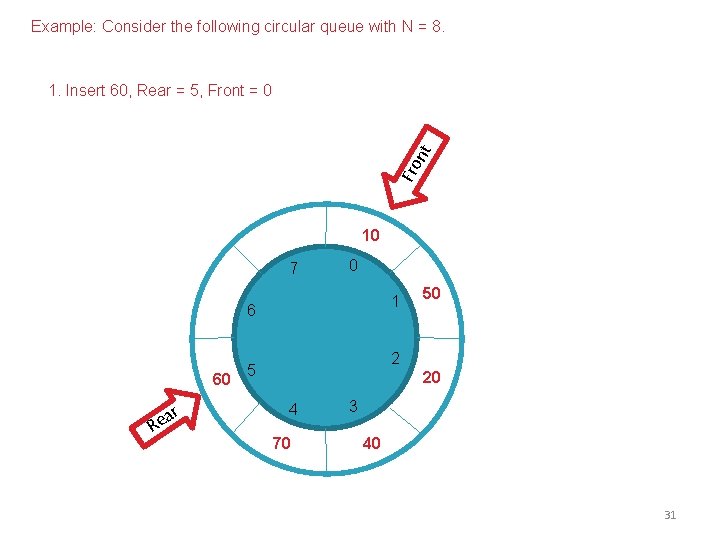
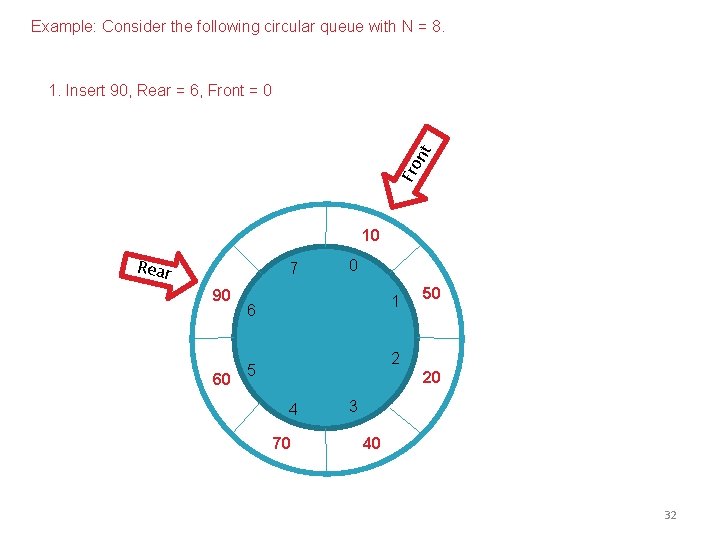
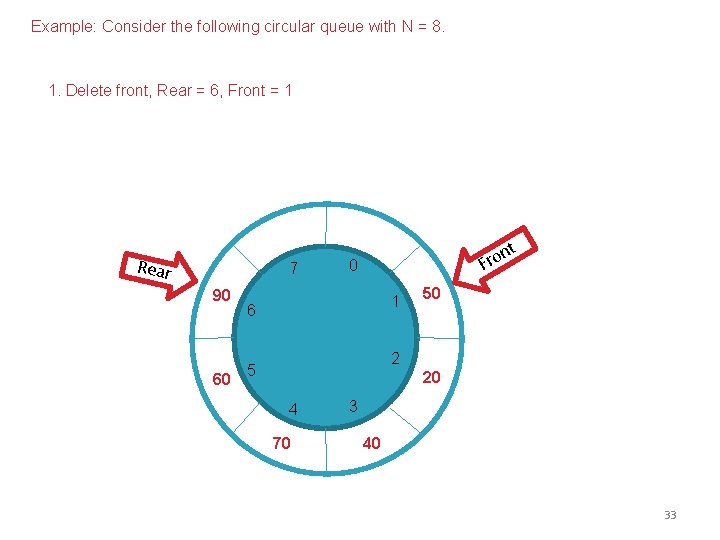
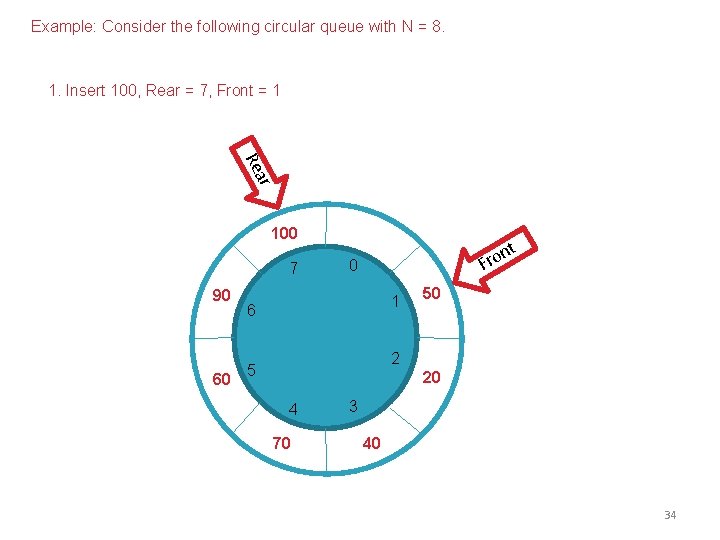
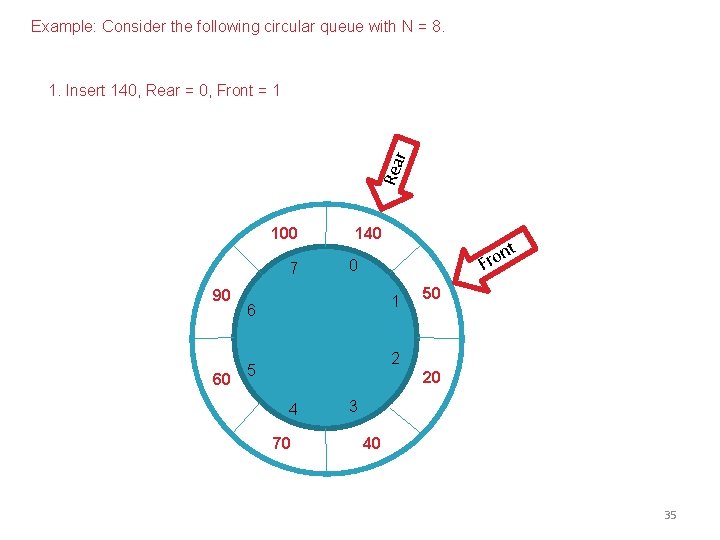
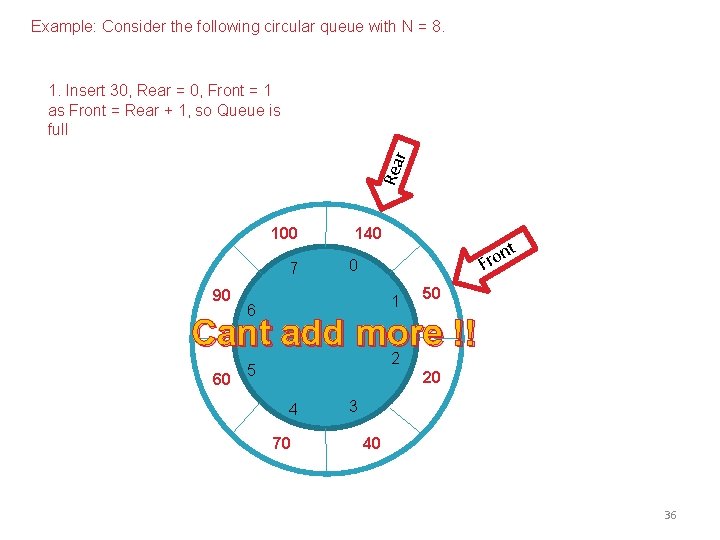
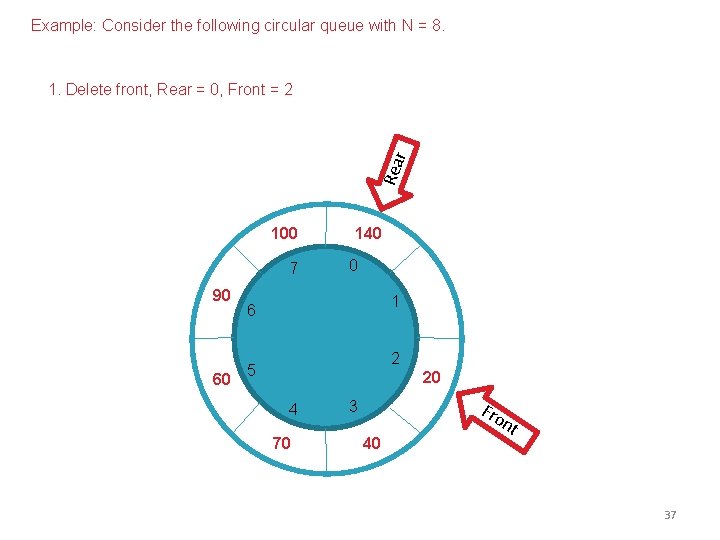
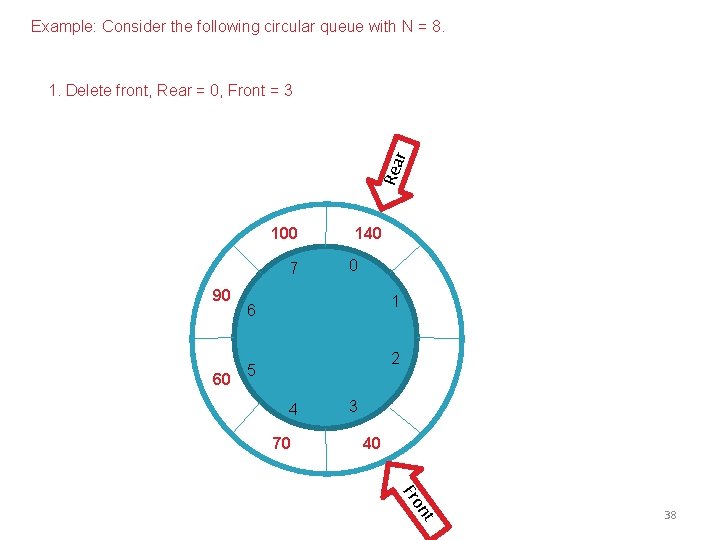
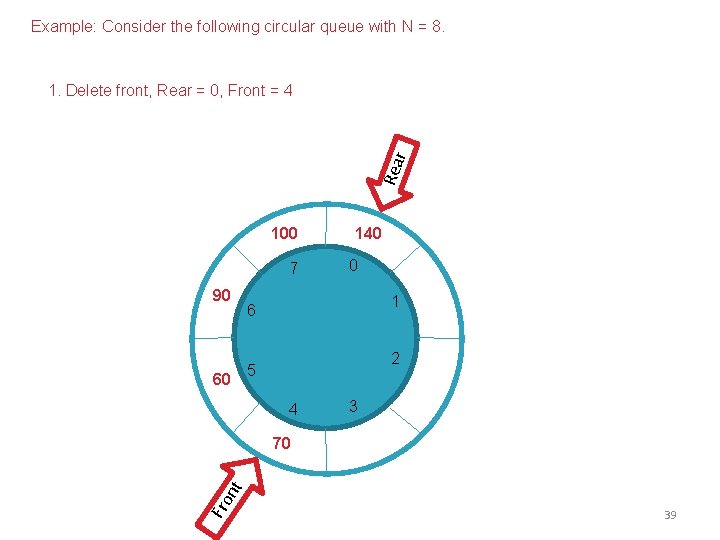
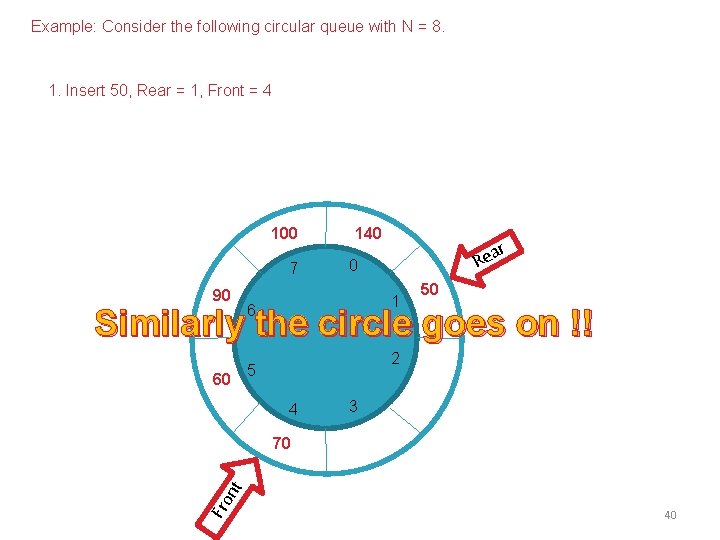
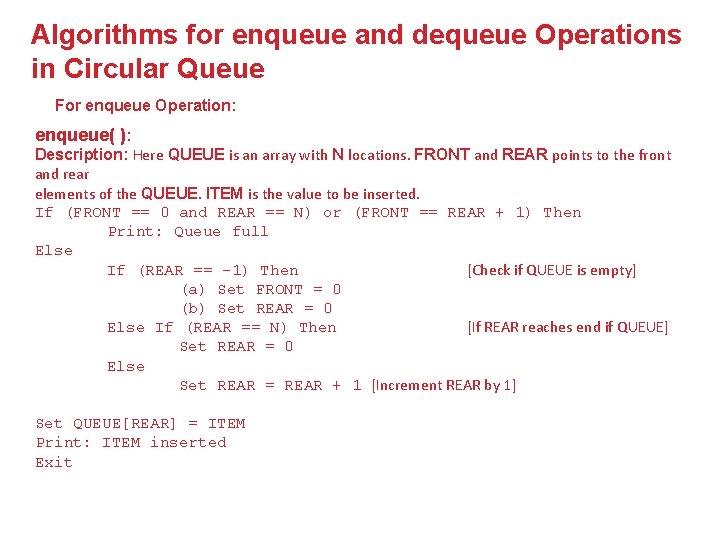
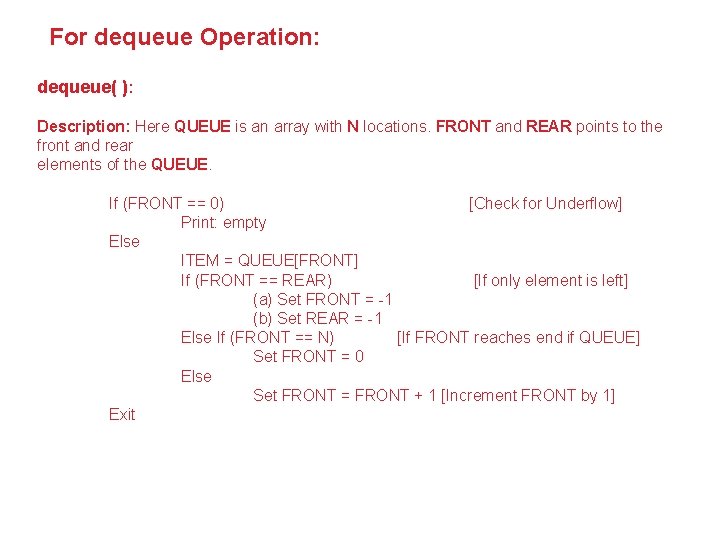
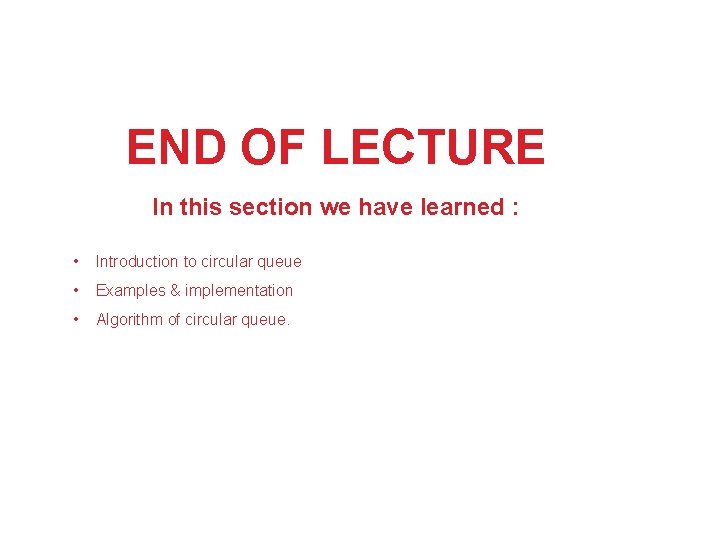
![Implementation of Circular Queues Class CQueue { private: int size; int queue[size]; int front; Implementation of Circular Queues Class CQueue { private: int size; int queue[size]; int front;](https://slidetodoc.com/presentation_image_h2/4b57158698b101b79a8dea92ff23dafa/image-44.jpg)
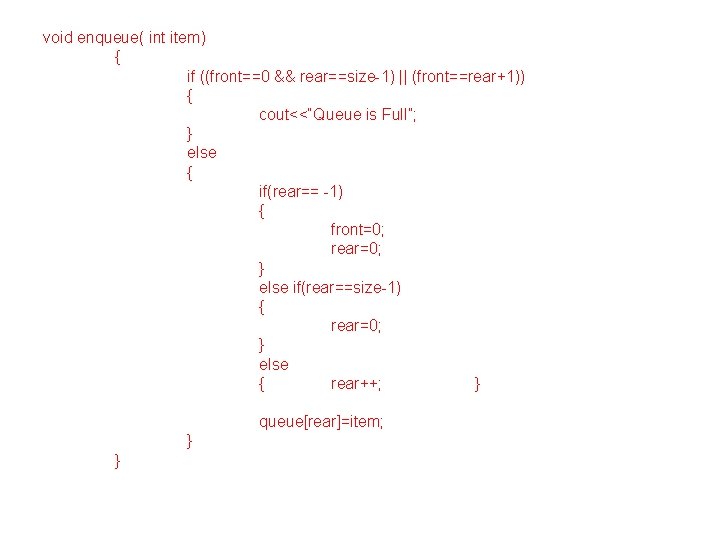
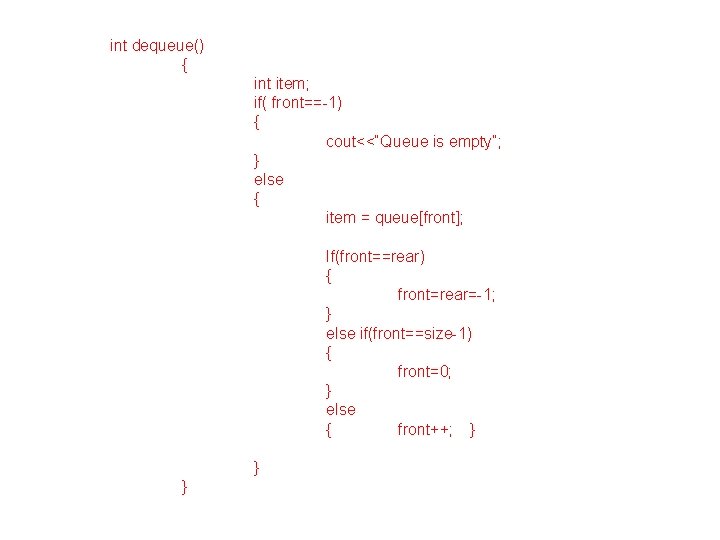
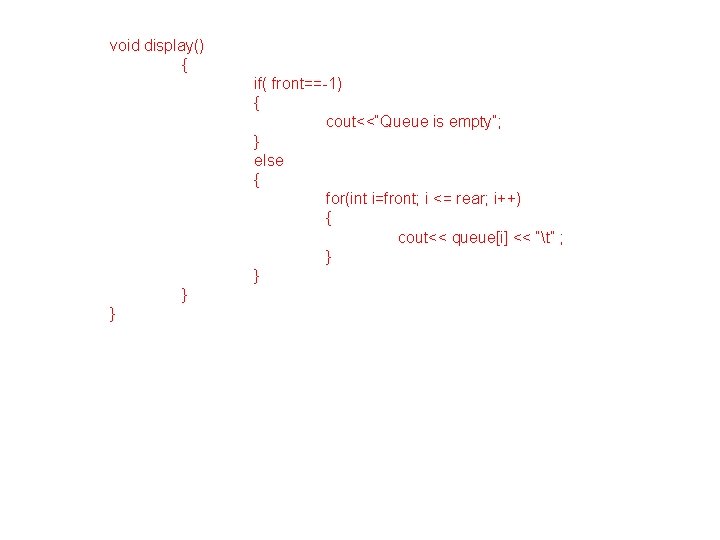
- Slides: 47
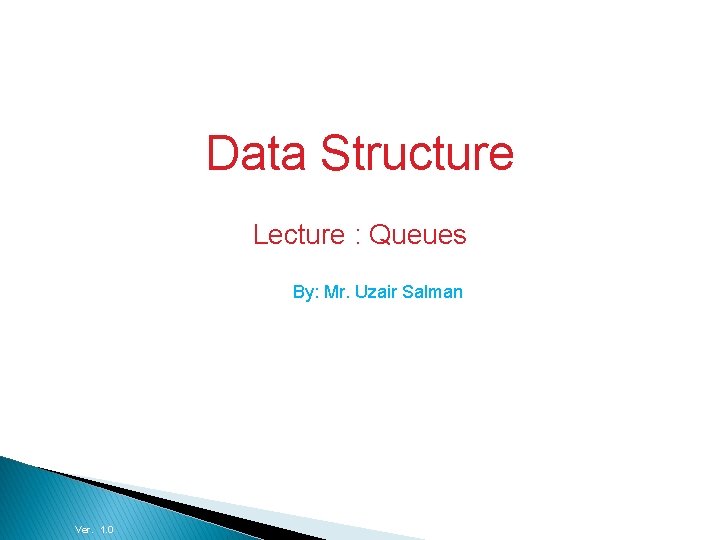
Data Structures and Algorithms Data Structure Lecture : Queues By: Mr. Uzair Salman Ver. 1. 0 Session 10
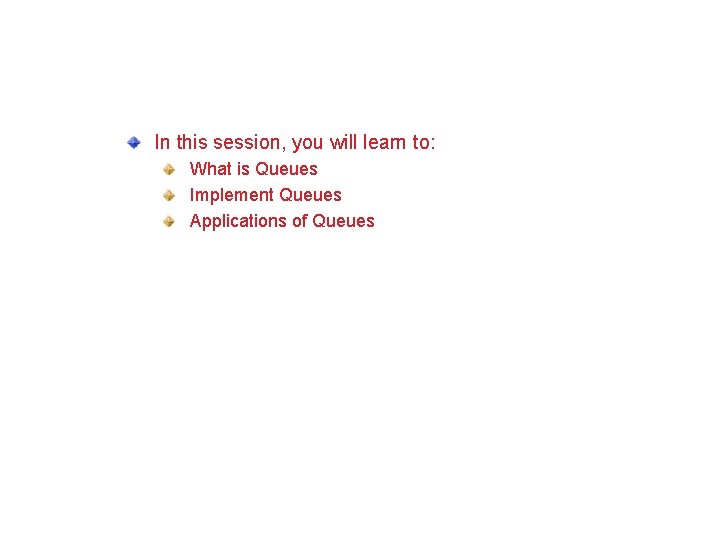
Objectives In this session, you will learn to: What is Queues Implement Queues Applications of Queues
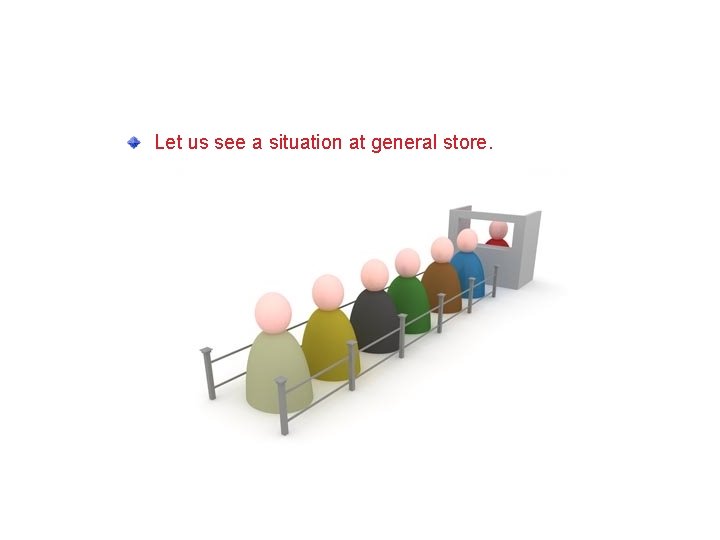
Stacks Let us see a situation at general store.
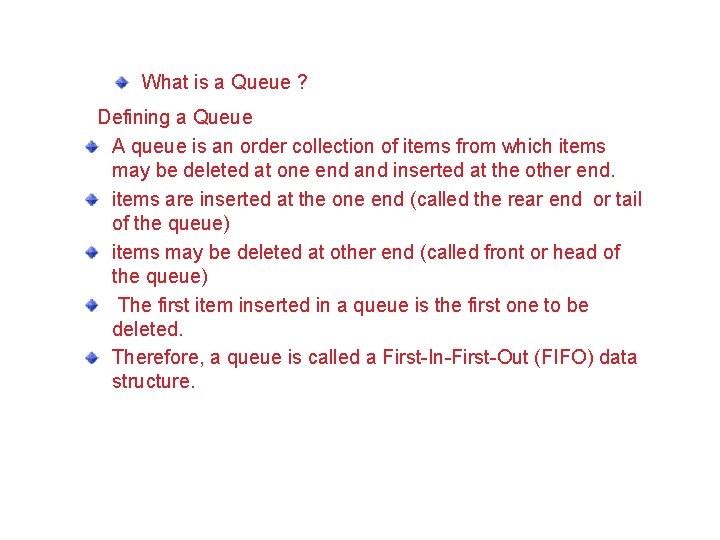
What is a Queue ? Defining a Queue A queue is an order collection of items from which items may be deleted at one end and inserted at the other end. items are inserted at the one end (called the rear end or tail of the queue) items may be deleted at other end (called front or head of the queue) The first item inserted in a queue is the first one to be deleted. Therefore, a queue is called a First-In-First-Out (FIFO) data structure.
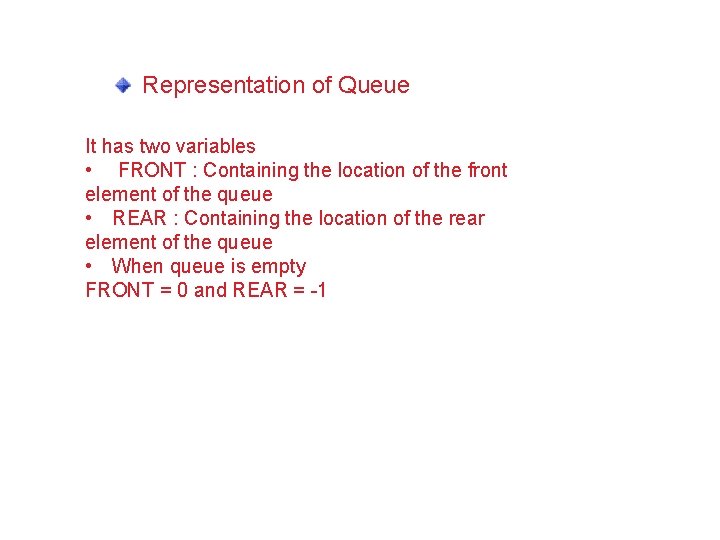
Representation of Queue It has two variables • FRONT : Containing the location of the front element of the queue • REAR : Containing the location of the rear element of the queue • When queue is empty FRONT = 0 and REAR = -1
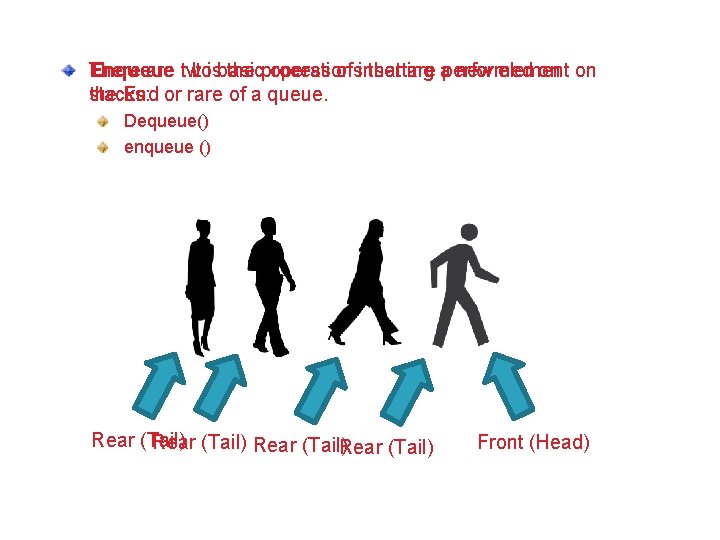
Enqueue It isbasic the process of inserting new element There are : two operations that are aperformed on on the End or rare of a queue. stacks: Dequeue() enqueue () Rear (Tail) Front (Head)
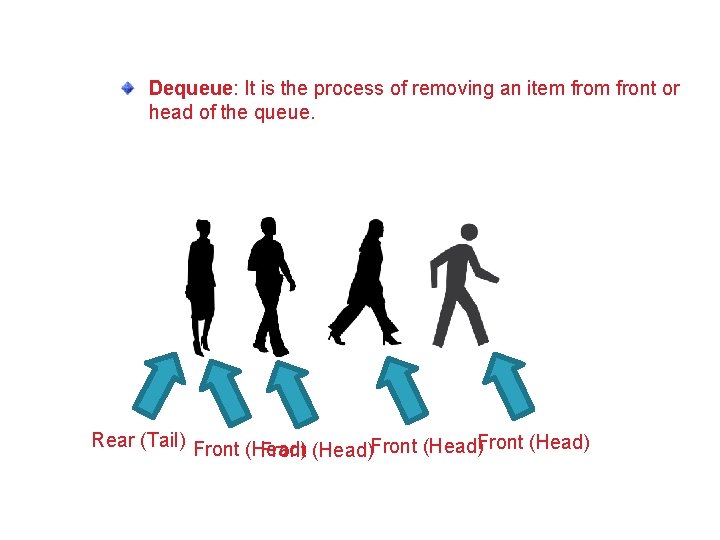
Dequeue: It is the process of removing an item front or head of the queue. Rear (Tail) Front (Head)Front (Head)
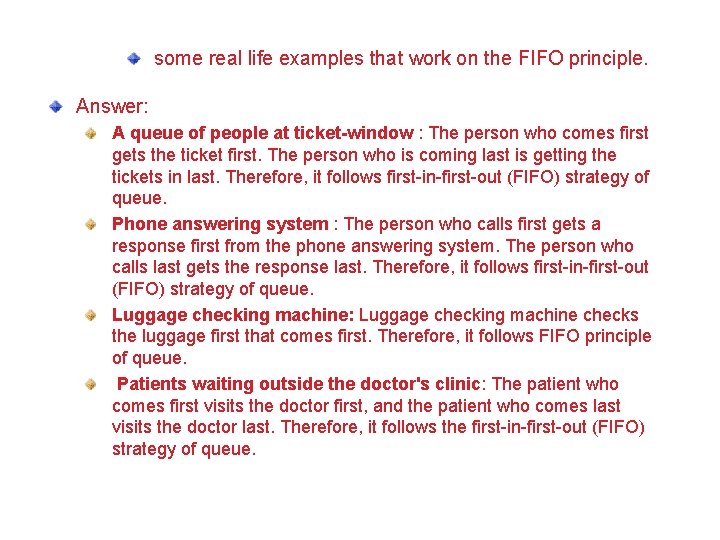
some real life examples that work on the FIFO principle. Answer: A queue of people at ticket-window : The person who comes first gets the ticket first. The person who is coming last is getting the tickets in last. Therefore, it follows first-in-first-out (FIFO) strategy of queue. Phone answering system : The person who calls first gets a response first from the phone answering system. The person who calls last gets the response last. Therefore, it follows first-in-first-out (FIFO) strategy of queue. Luggage checking machine: Luggage checking machine checks the luggage first that comes first. Therefore, it follows FIFO principle of queue. Patients waiting outside the doctor's clinic: The patient who comes first visits the doctor first, and the patient who comes last visits the doctor last. Therefore, it follows the first-in-first-out (FIFO) strategy of queue.
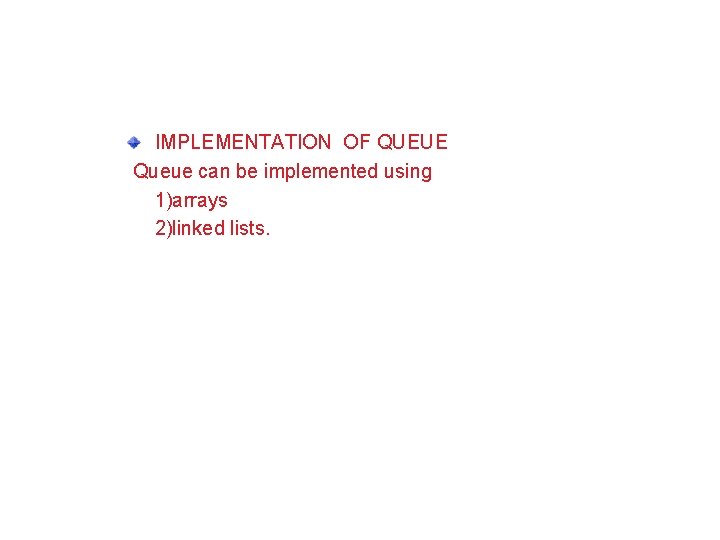
Implementing a Stack Using an Array IMPLEMENTATION OF QUEUE Queue can be implemented using 1)arrays 2)linked lists.
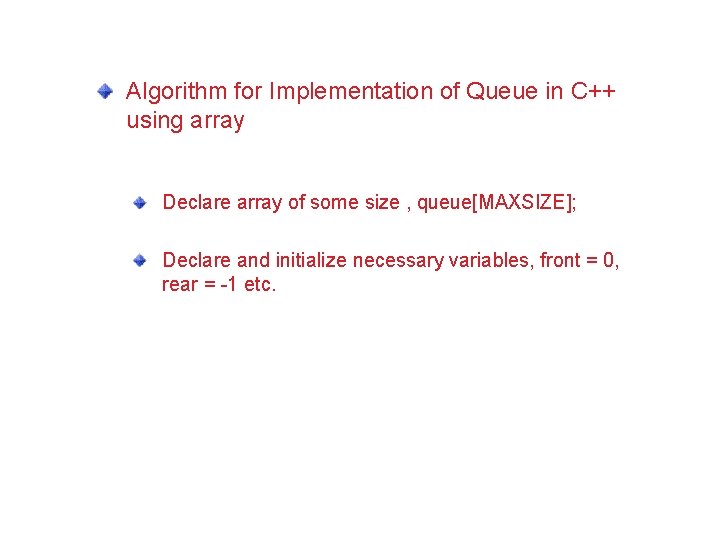
Algorithm for Implementation of Queue in C++ using array Declare array of some size , queue[MAXSIZE]; Declare and initialize necessary variables, front = 0, rear = -1 etc.
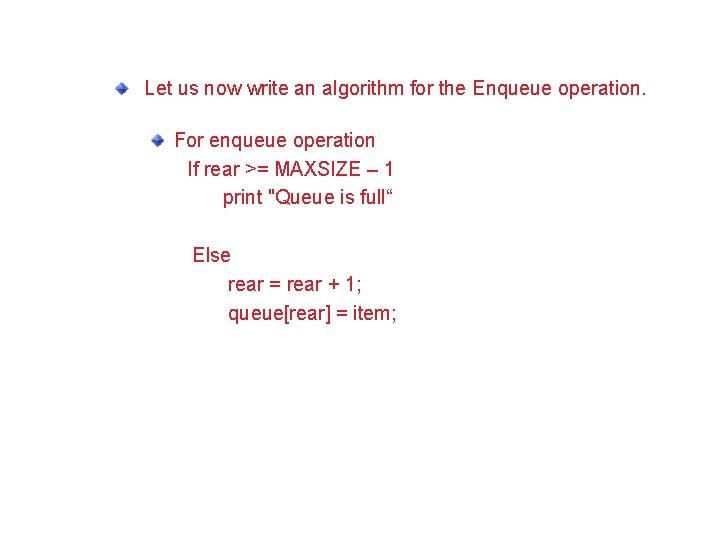
Implementing a Stack Using an Array (Contd. ) Let us now write an algorithm for the Enqueue operation. For enqueue operation If rear >= MAXSIZE – 1 print "Queue is full“ Else rear = rear + 1; queue[rear] = item;
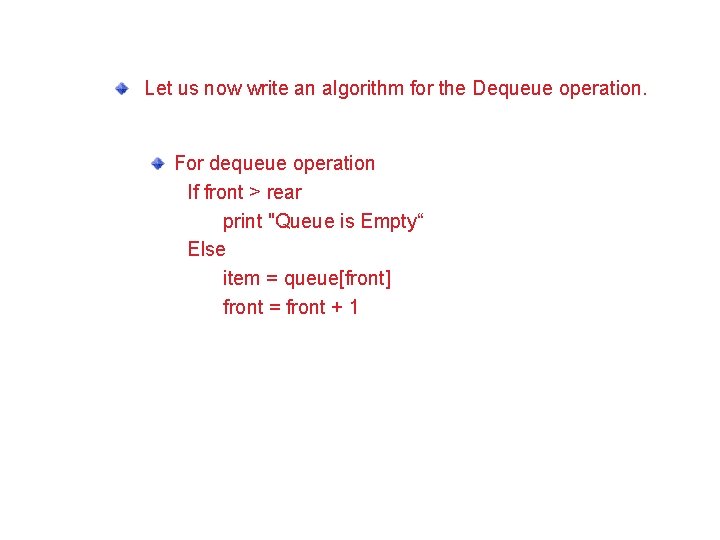
Implementing a Stack Using an Array (Contd. ) Let us now write an algorithm for the Dequeue operation. For dequeue operation If front > rear print "Queue is Empty“ Else item = queue[front] front = front + 1
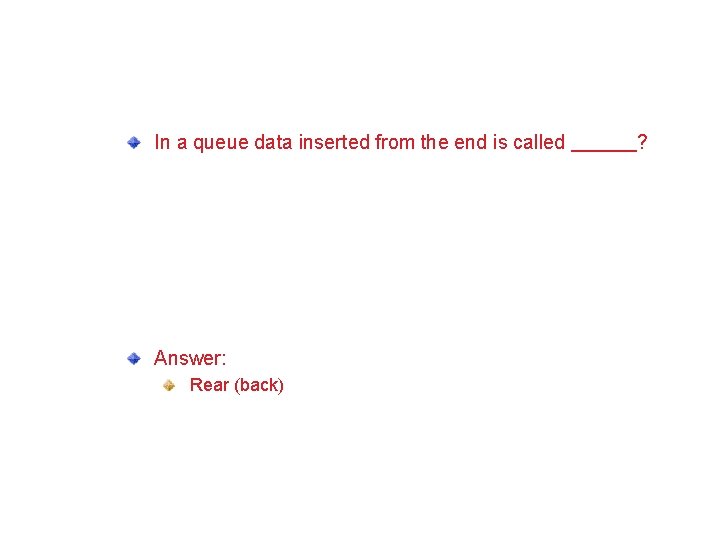
Just a minute In a queue data inserted from the end is called Answer: Rear (back) ?
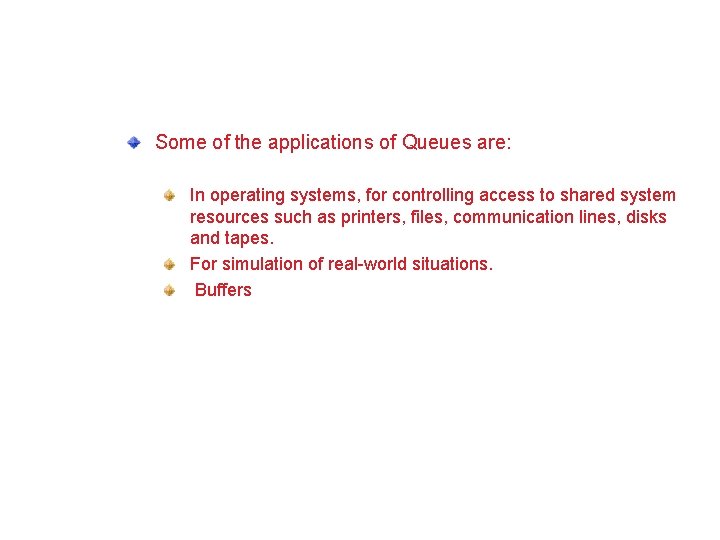
Applications of Stacks Some of the applications of Queues are: In operating systems, for controlling access to shared system resources such as printers, files, communication lines, disks and tapes. For simulation of real-world situations. Buffers
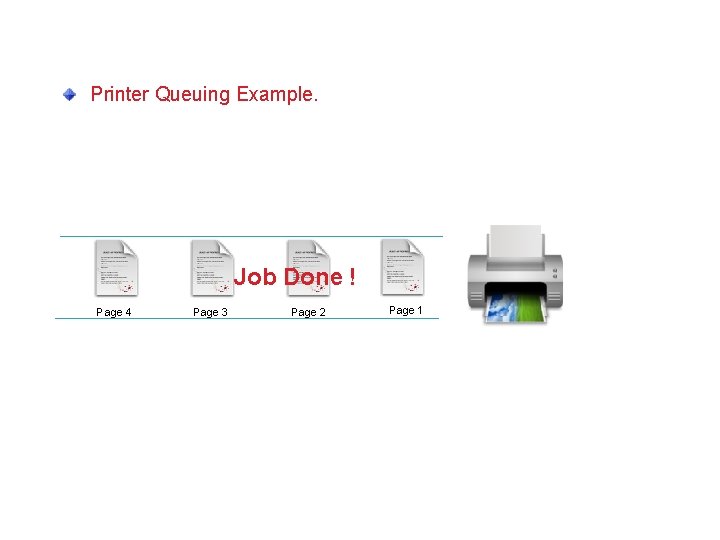
Printer Queuing Example. Job Done ! Page 4 Page 3 Page 2 Page 1
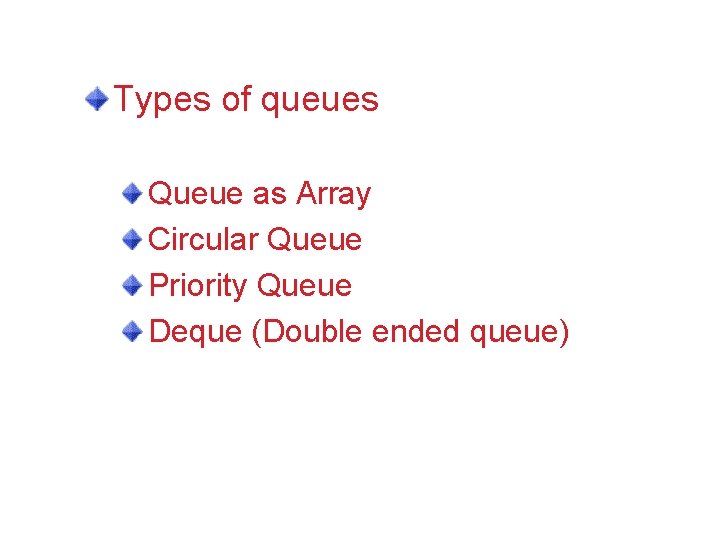
Types of queues Queue as Array Circular Queue Priority Queue Deque (Double ended queue)
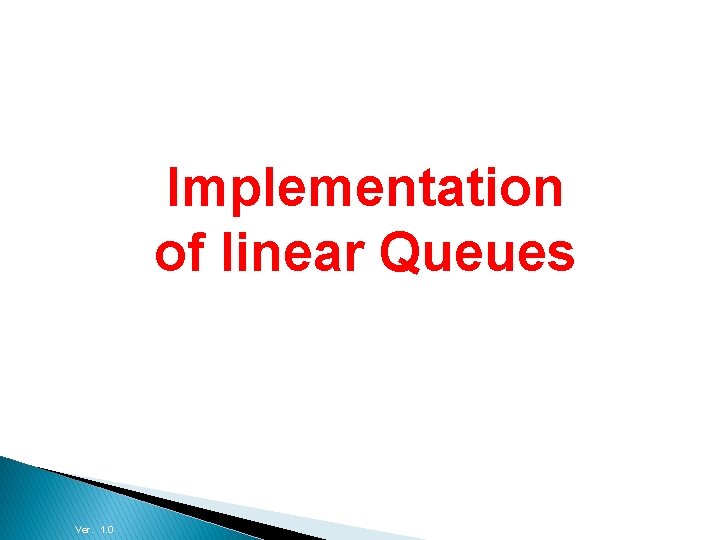
Data Structures and Algorithms Implementation of linear Queues Ver. 1. 0 Session 10
![Class queue private int size int queuesize int front int rear public queue Class queue { private: int size; int queue[size]; int front; int rear; public: queue()](https://slidetodoc.com/presentation_image_h2/4b57158698b101b79a8dea92ff23dafa/image-18.jpg)
Class queue { private: int size; int queue[size]; int front; int rear; public: queue() { size=10; front=0; rear= - 1; } void enqueue( int item) { if(rear= size-1) { cout<<“Queue is Full”; } else { rear++; queue[rear]=item; } }
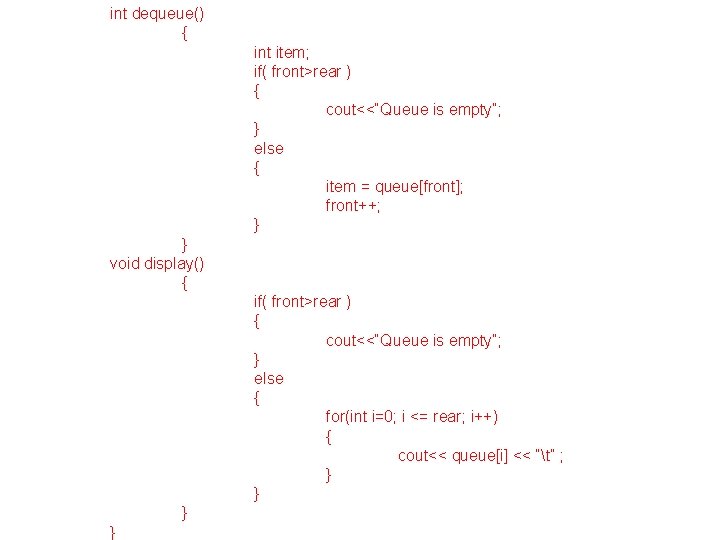
int dequeue() { int item; if( front>rear ) { cout<<“Queue is empty”; } else { item = queue[front]; front++; } } void display() { if( front>rear ) { cout<<“Queue is empty”; } else { for(int i=0; i <= rear; i++) { cout<< queue[i] << “t” ; } }
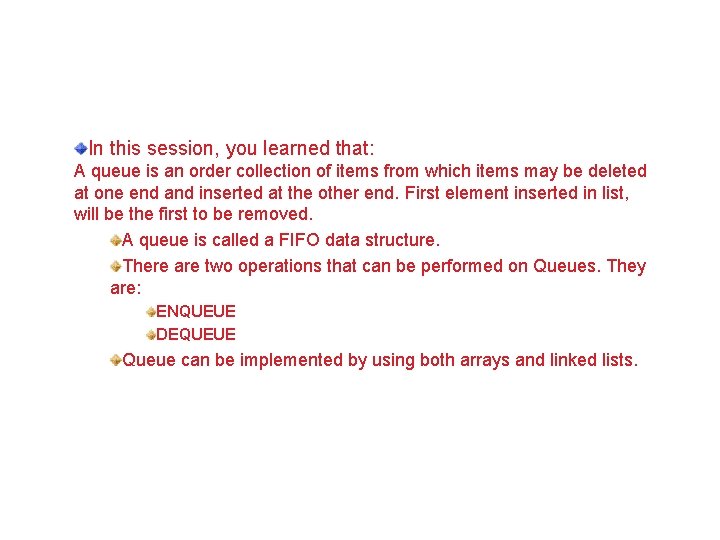
In this session, you learned that: A queue is an order collection of items from which items may be deleted at one end and inserted at the other end. First element inserted in list, will be the first to be removed. A queue is called a FIFO data structure. There are two operations that can be performed on Queues. They are: ENQUEUE DEQUEUE Queue can be implemented by using both arrays and linked lists.
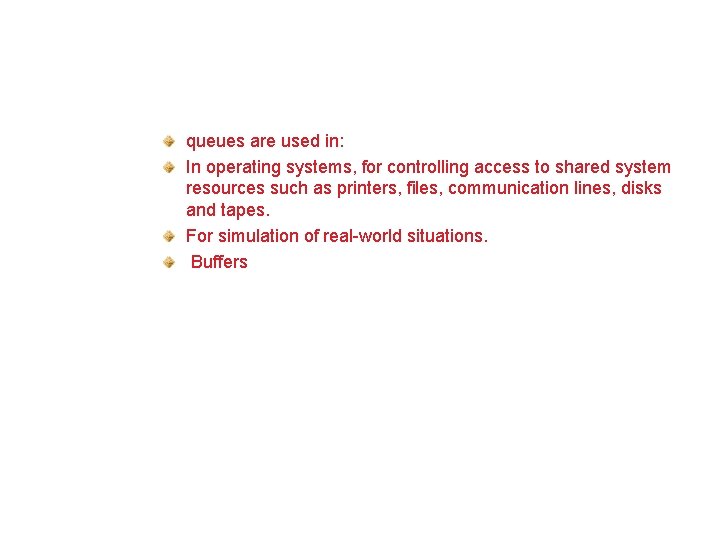
Summary (Contd. ) queues are used in: In operating systems, for controlling access to shared system resources such as printers, files, communication lines, disks and tapes. For simulation of real-world situations. Buffers
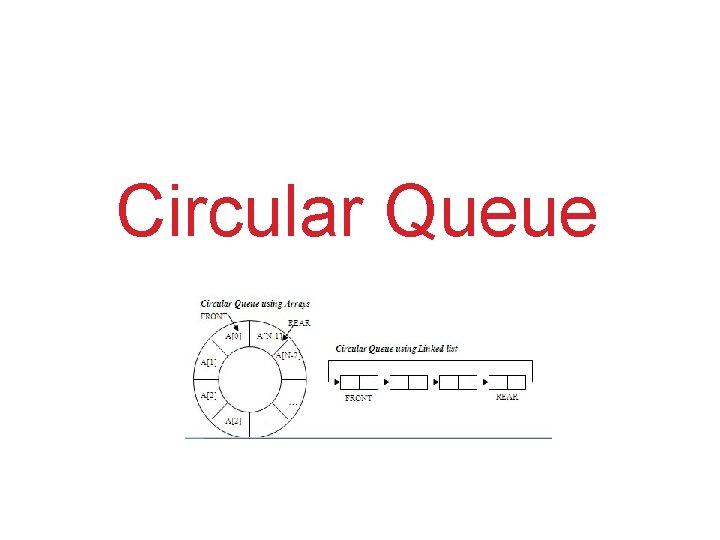
Summary (Contd. ) Circular Queue
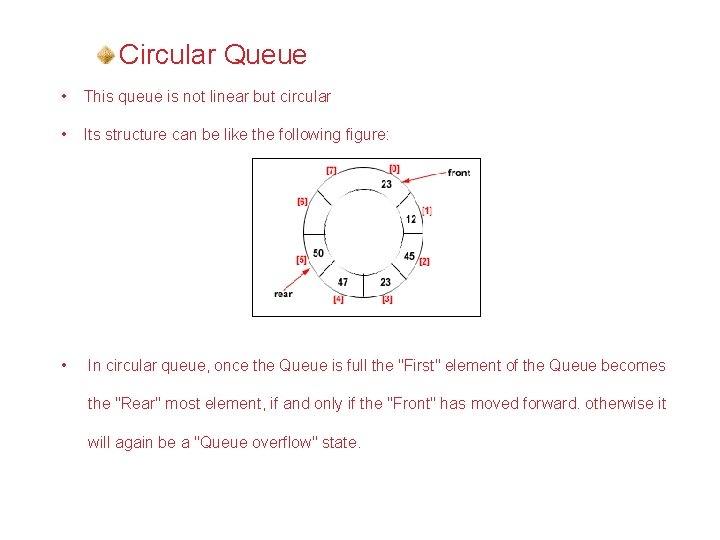
Circular Queue Summary (Contd. ) • This queue is not linear but circular • Its structure can be like the following figure: • In circular queue, once the Queue is full the "First" element of the Queue becomes the "Rear" most element, if and only if the "Front" has moved forward. otherwise it will again be a "Queue overflow" state.
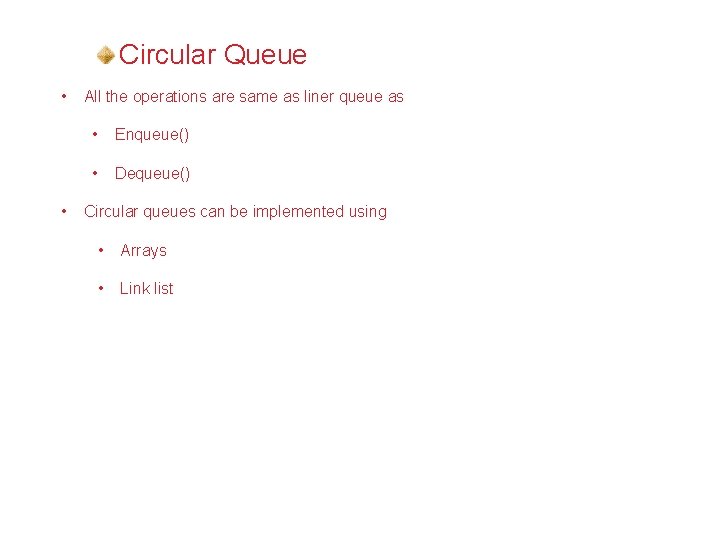
Circular Queue Summary (Contd. ) • • All the operations are same as liner queue as • Enqueue() • Dequeue() Circular queues can be implemented using • Arrays • Link list
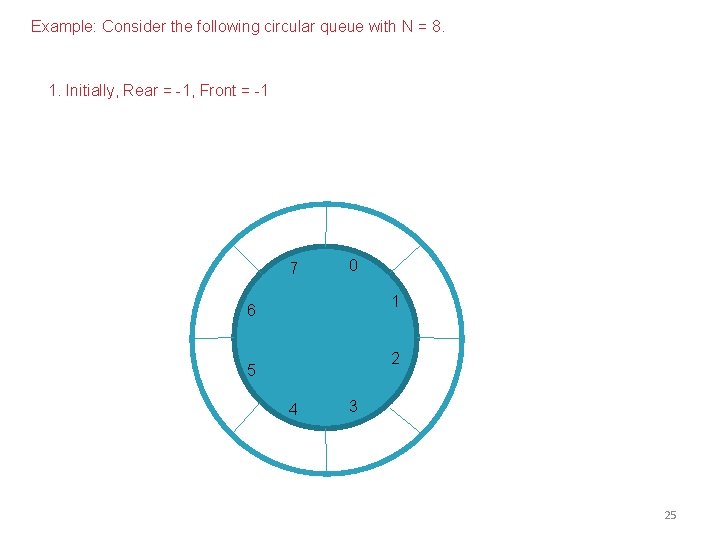
Example: Consider the following circular queue with N = 8. 1. Initially, Rear = -1, Front = -1 7 0 1 6 2 5 4 3 25
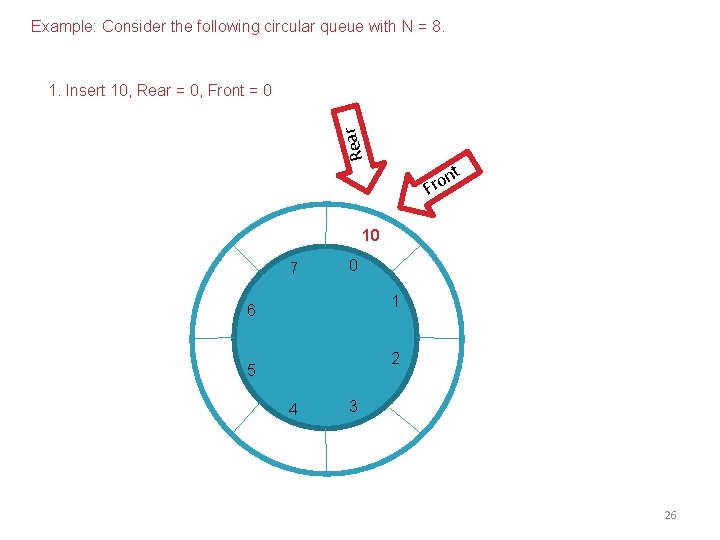
Example: Consider the following circular queue with N = 8. Rear 1. Insert 10, Rear = 0, Front = 0 nt Fro 10 7 0 1 6 2 5 4 3 26
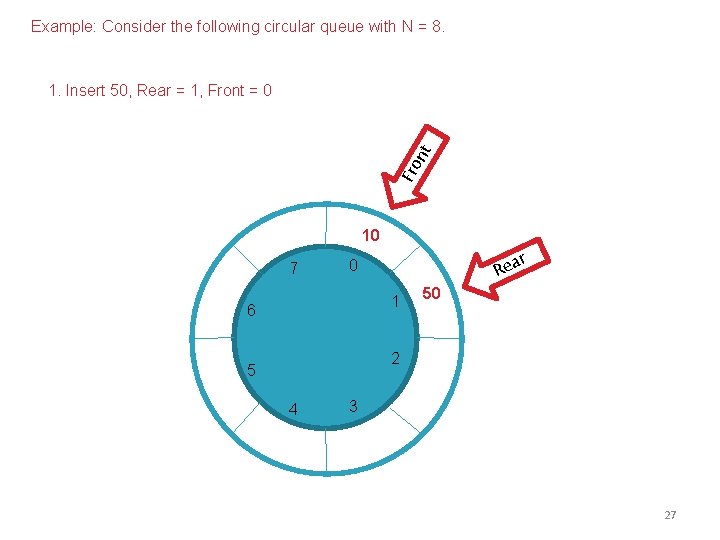
Example: Consider the following circular queue with N = 8. Fro nt 1. Insert 50, Rear = 1, Front = 0 10 7 ar Re 0 1 6 50 2 5 4 3 27
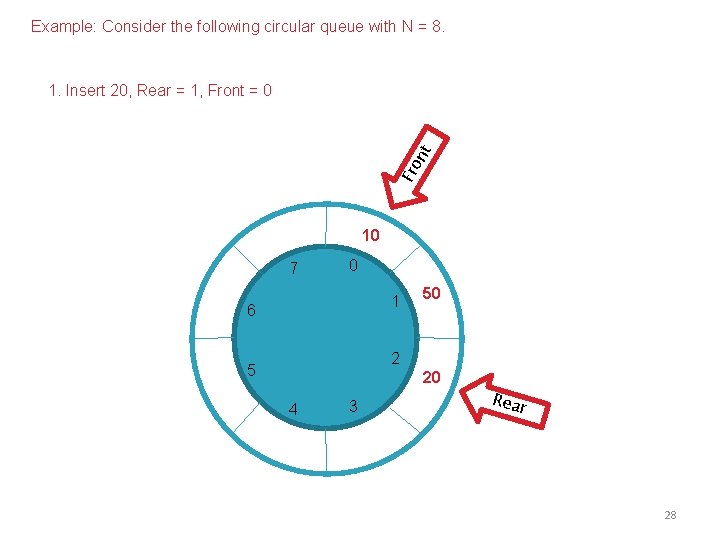
Example: Consider the following circular queue with N = 8. Fro nt 1. Insert 20, Rear = 1, Front = 0 10 7 0 1 6 50 2 5 20 4 3 Rear 28
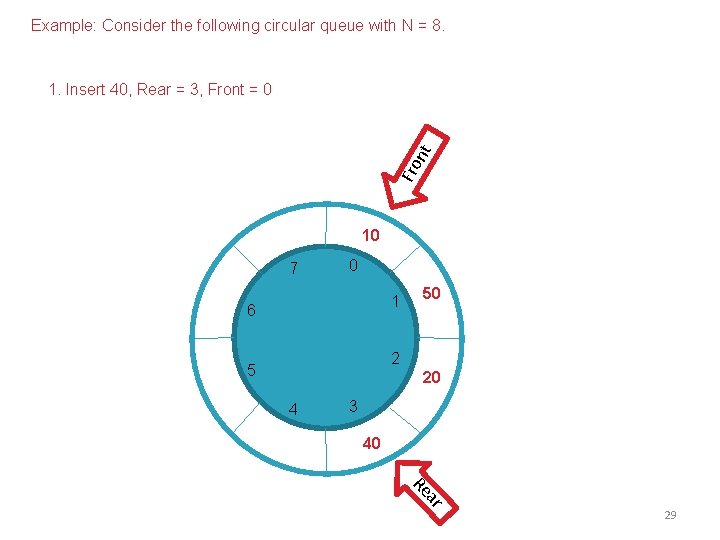
Example: Consider the following circular queue with N = 8. Fro nt 1. Insert 40, Rear = 3, Front = 0 10 7 0 1 6 50 2 5 20 4 3 40 ar Re 29
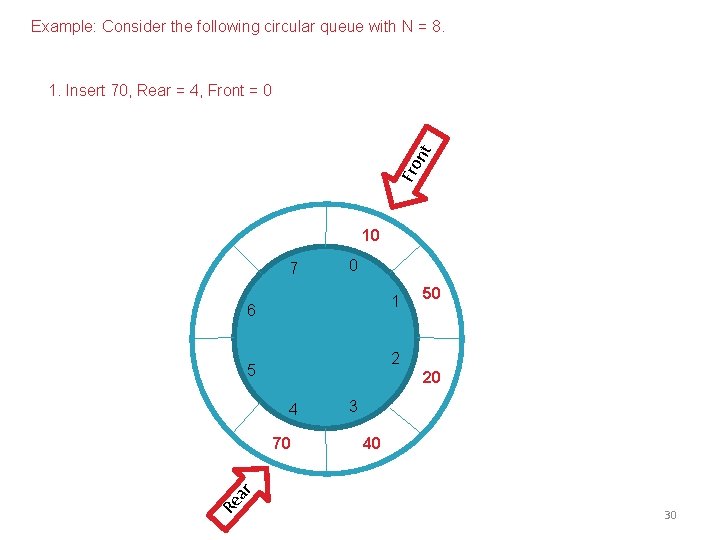
Example: Consider the following circular queue with N = 8. Fro nt 1. Insert 70, Rear = 4, Front = 0 10 7 0 1 6 50 2 5 20 4 40 Re ar 70 3 30
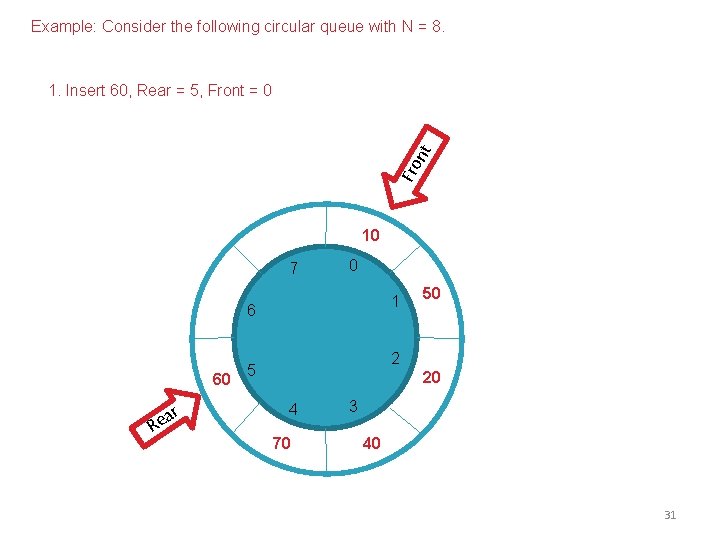
Example: Consider the following circular queue with N = 8. Fro nt 1. Insert 60, Rear = 5, Front = 0 10 7 0 1 6 60 r a Re 50 2 5 20 4 70 3 40 31
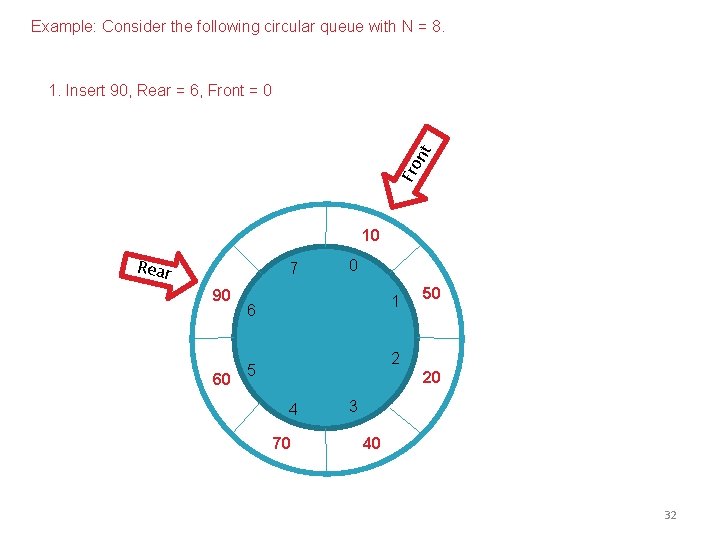
Example: Consider the following circular queue with N = 8. Fro nt 1. Insert 90, Rear = 6, Front = 0 10 Rear 7 90 60 0 1 6 50 2 5 20 4 70 3 40 32
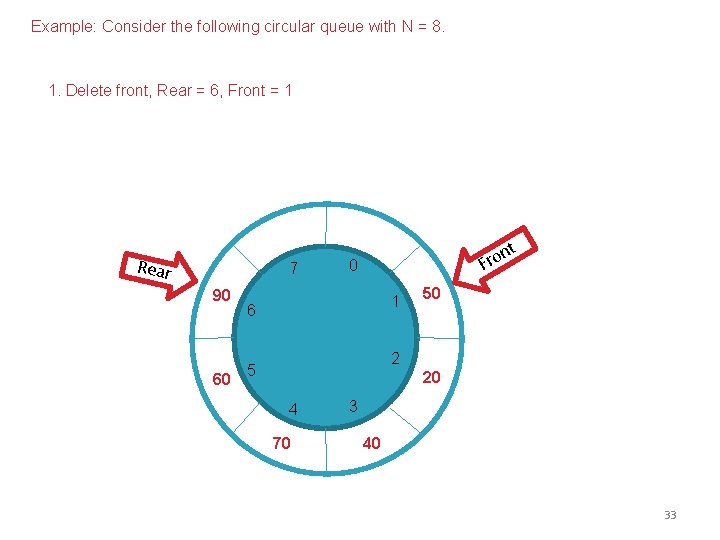
Example: Consider the following circular queue with N = 8. 1. Delete front, Rear = 6, Front = 1 Rear 7 90 60 nt Fro 0 1 6 50 2 5 20 4 70 3 40 33
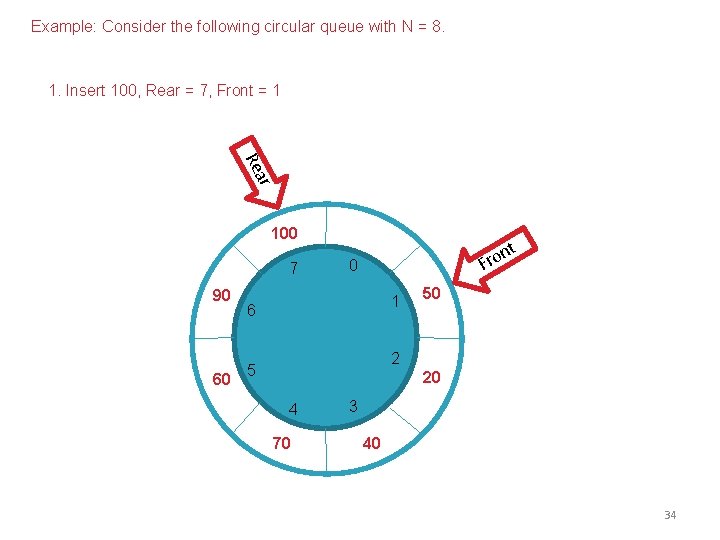
Example: Consider the following circular queue with N = 8. 1. Insert 100, Rear = 7, Front = 1 ar Re 100 7 90 60 nt Fro 0 1 6 50 2 5 20 4 70 3 40 34
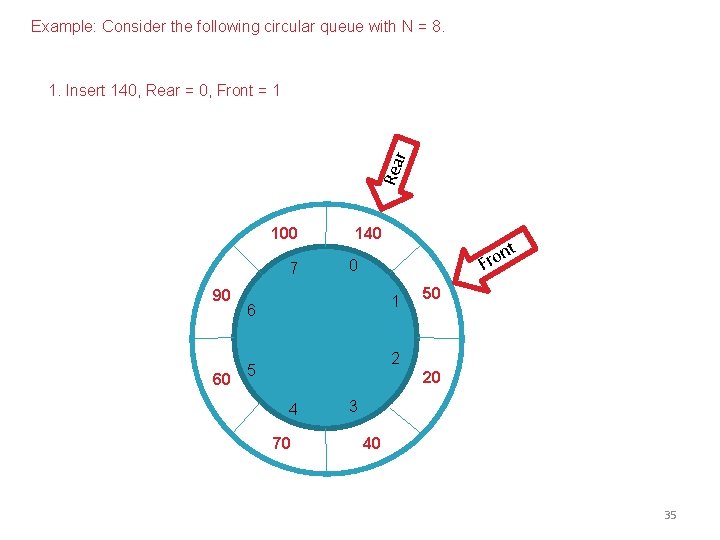
Example: Consider the following circular queue with N = 8. Rea r 1. Insert 140, Rear = 0, Front = 1 100 7 90 60 140 nt Fro 0 1 6 50 2 5 20 4 70 3 40 35
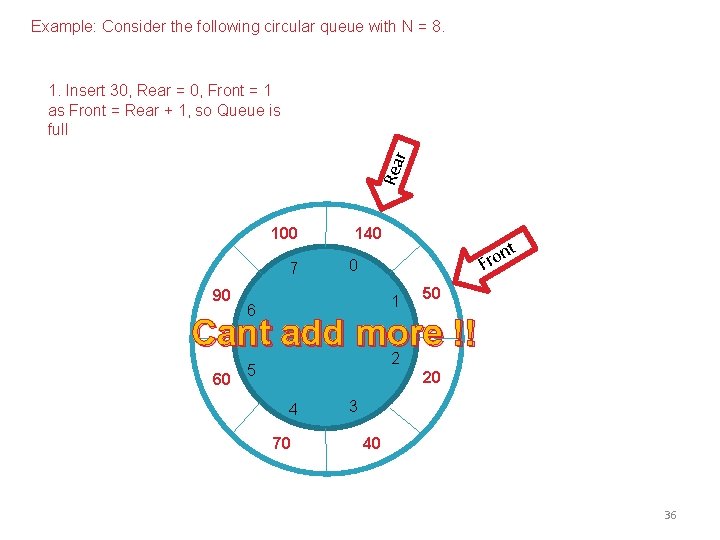
Example: Consider the following circular queue with N = 8. Rea r 1. Insert 30, Rear = 0, Front = 1 as Front = Rear + 1, so Queue is full 100 7 90 140 nt Fro 0 1 6 50 Cant add more !! 2 60 5 20 4 70 3 40 36
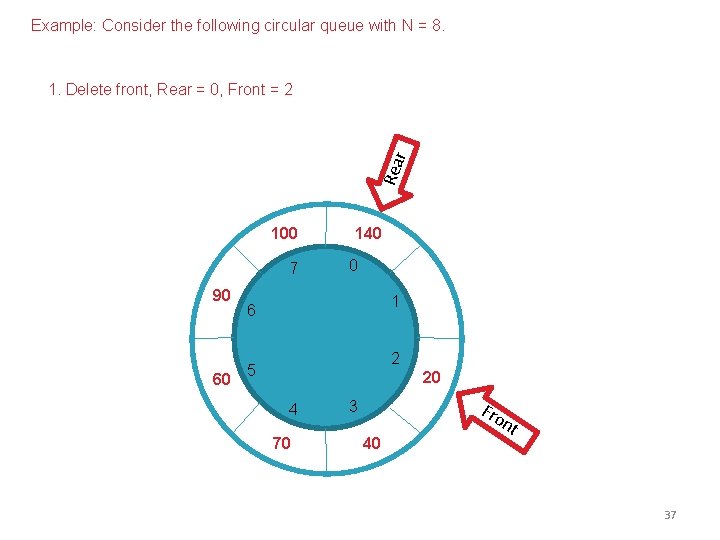
Example: Consider the following circular queue with N = 8. Rea r 1. Delete front, Rear = 0, Front = 2 100 7 90 60 140 0 1 6 2 5 20 4 70 Fr o 3 40 nt 37
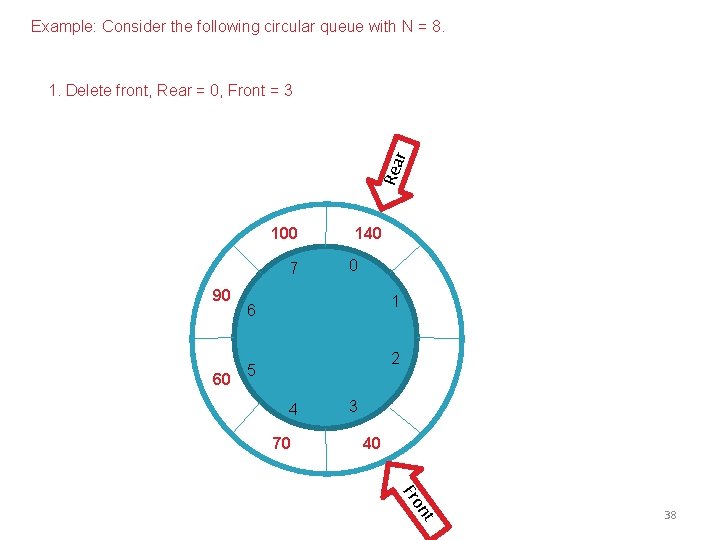
Example: Consider the following circular queue with N = 8. Rea r 1. Delete front, Rear = 0, Front = 3 100 7 90 60 140 0 1 6 2 5 4 70 3 40 o Fr nt 38
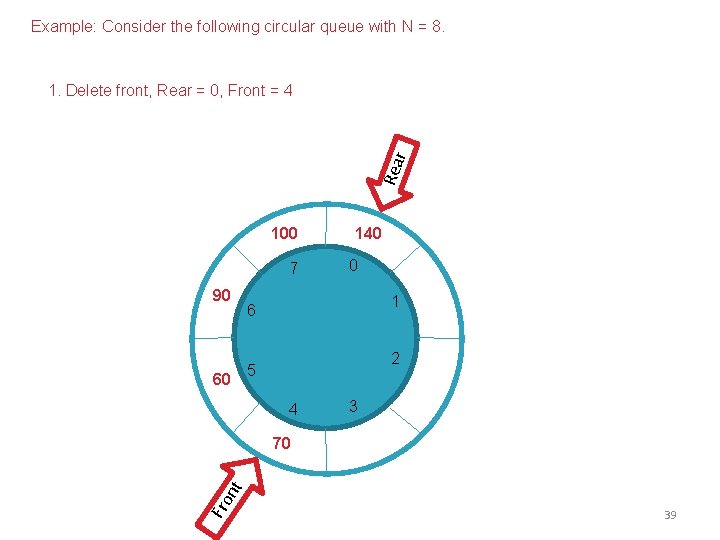
Example: Consider the following circular queue with N = 8. Rea r 1. Delete front, Rear = 0, Front = 4 100 7 90 60 140 0 1 6 2 5 4 3 Fr on t 70 39
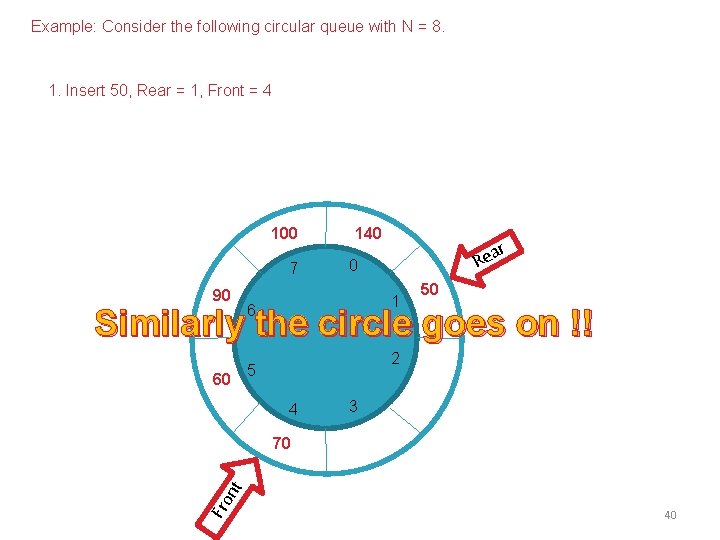
Example: Consider the following circular queue with N = 8. 1. Insert 50, Rear = 1, Front = 4 100 7 90 140 r a Re 0 1 6 50 Similarly the circle goes on !! 60 2 5 4 3 Fr on t 70 40
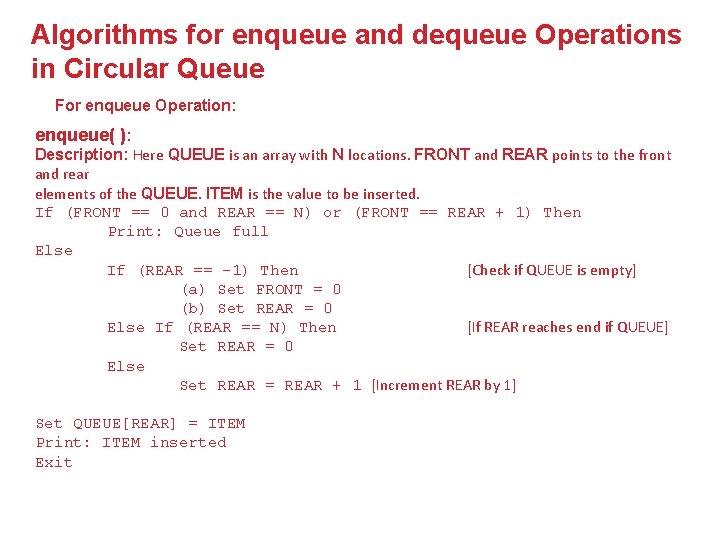
Algorithms for enqueue and dequeue Operations in Circular Queue For enqueue Operation: enqueue( ): Description: Here QUEUE is an array with N locations. FRONT and REAR points to the front and rear elements of the QUEUE. ITEM is the value to be inserted. If (FRONT == 0 and REAR == N) or (FRONT == REAR + 1) Then Print: Queue full Else If (REAR == -1) Then [Check if QUEUE is empty] (a) Set FRONT = 0 (b) Set REAR = 0 Else If (REAR == N) Then [If REAR reaches end if QUEUE] Set REAR = 0 Else Set REAR = REAR + 1 [Increment REAR by 1] Set QUEUE[REAR] = ITEM Print: ITEM inserted Exit
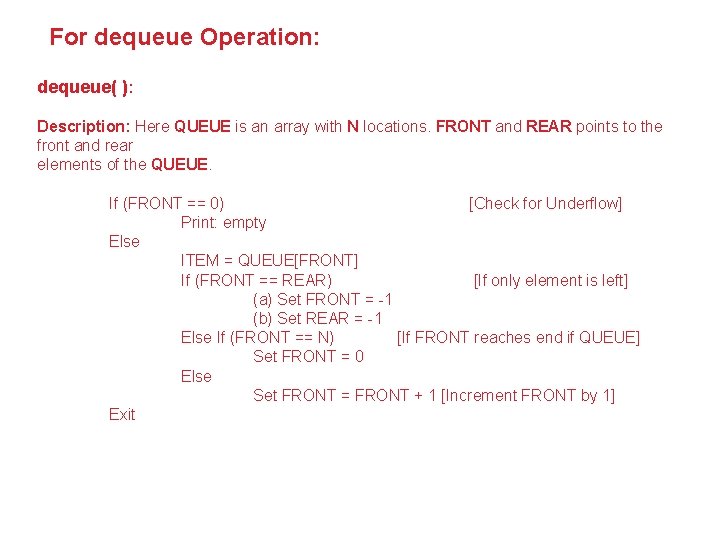
For dequeue Operation: dequeue( ): Description: Here QUEUE is an array with N locations. FRONT and REAR points to the front and rear elements of the QUEUE. If (FRONT == 0) [Check for Underflow] Print: empty Else ITEM = QUEUE[FRONT] If (FRONT == REAR) [If only element is left] (a) Set FRONT = -1 (b) Set REAR = -1 Else If (FRONT == N) [If FRONT reaches end if QUEUE] Set FRONT = 0 Else Set FRONT = FRONT + 1 [Increment FRONT by 1] Exit
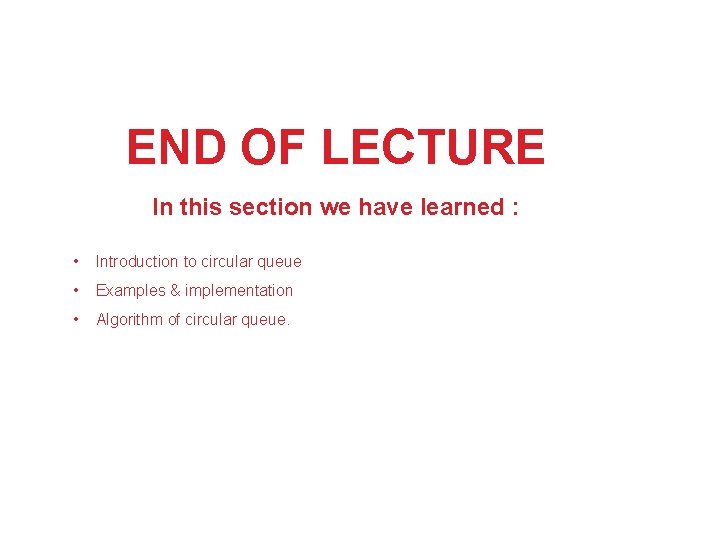
END OF LECTURE In this section we have learned : • Introduction to circular queue • Examples & implementation • Algorithm of circular queue.
![Implementation of Circular Queues Class CQueue private int size int queuesize int front Implementation of Circular Queues Class CQueue { private: int size; int queue[size]; int front;](https://slidetodoc.com/presentation_image_h2/4b57158698b101b79a8dea92ff23dafa/image-44.jpg)
Implementation of Circular Queues Class CQueue { private: int size; int queue[size]; int front; int rear; public: queue() { size=10; front= -1; rear= - 1; }
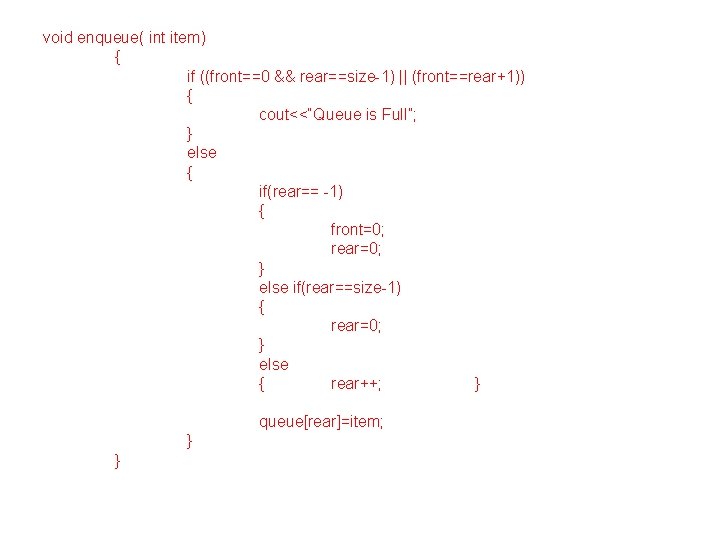
void enqueue( int item) { if ((front==0 && rear==size-1) || (front==rear+1)) { cout<<“Queue is Full”; } else { if(rear== -1) { front=0; rear=0; } else if(rear==size-1) { rear=0; } else { rear++; } queue[rear]=item; } }
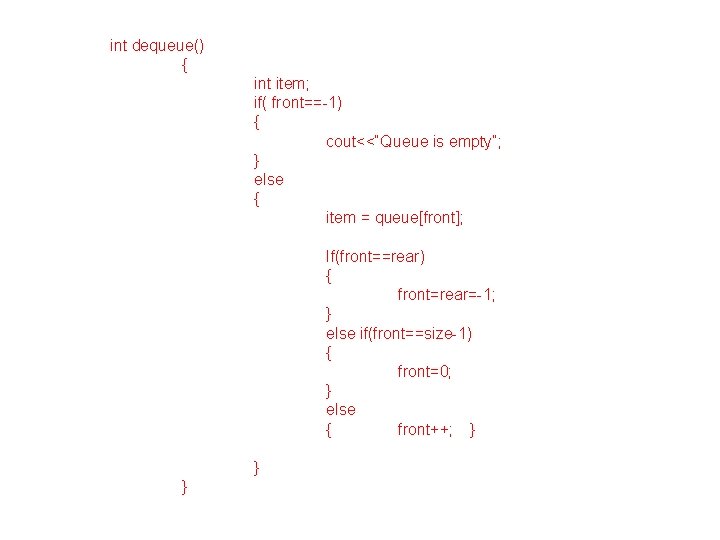
int dequeue() { int item; if( front==-1) { cout<<“Queue is empty”; } else { item = queue[front]; If(front==rear) { front=rear=-1; } else if(front==size-1) { front=0; } else { front++; } } }
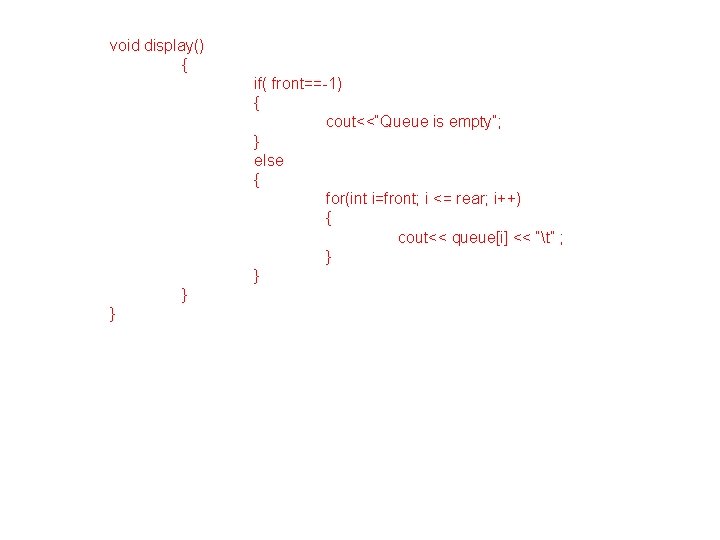
void display() { if( front==-1) { cout<<“Queue is empty”; } else { for(int i=front; i <= rear; i++) { cout<< queue[i] << “t” ; } }
Operasi yang tidak ada pada antrian (queue) adalah
Ajit diwan iit bombay
Kevin wayne princeton
Data structures and algorithms tutorial
Information retrieval data structures and algorithms
Data structures and algorithms bits pilani
Data structures and algorithms iit bombay
Data structures and algorithms
Data structures and algorithms
Ian munro waterloo
Signature file structure in information retrieval system
Data structures and algorithms
Algorithms + data structures = programs
Analysis of algorithms lecture notes
Introduction to algorithms lecture notes
Cqueue
Java stacks and queues
Exercises on stacks and queues
Java stacks and queues
Queue quiz
Queue representation
Message queues in unix
Adaptable priority queues
Rtos mailbox
Applications of priority queues
Queue head tail
Mgh
01:640:244 lecture notes - lecture 15: plat, idah, farad
Homologous
Static and dynamic queue in data structure
Stream data model
Conditional macro expansion example
Assembler data structures
Data structures and abstractions with java
Adts, data structures, and problem solving with c++
Data structures and algorithm
Ephemeral data structure
Computational thinking algorithms and programming
1001 design
Association analysis: basic concepts and algorithms
Fftooo
Algorithms for select and join operations
Algorithms and flowcharts
Undecidable problems and unreasonable time algorithms.
Cluster analysis basic concepts and algorithms
Randomized algorithms and probabilistic analysis
Introduction of design and analysis of algorithms
Algorithms for query processing and optimization