The Priority Queue ADT A priority queue is
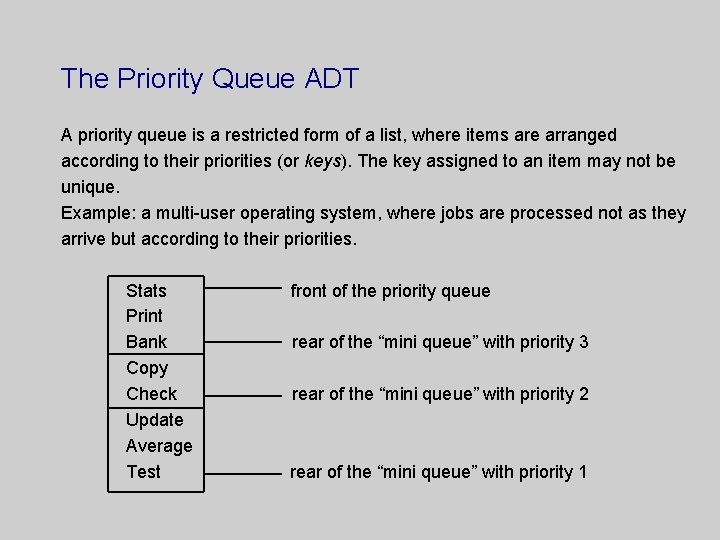
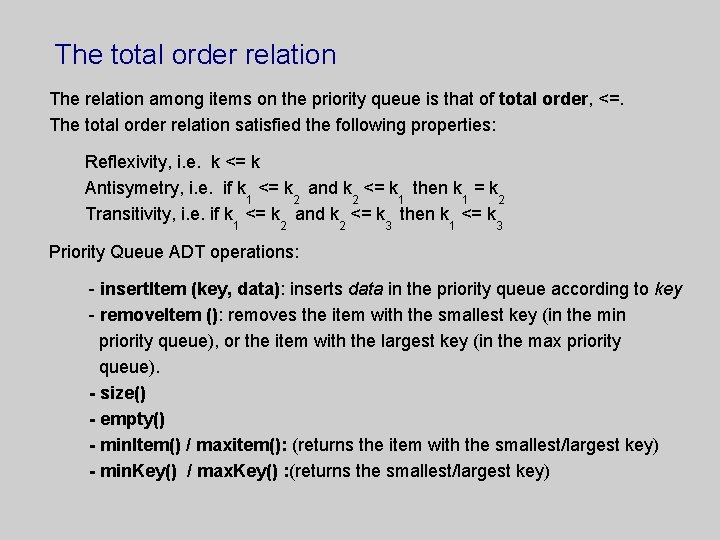
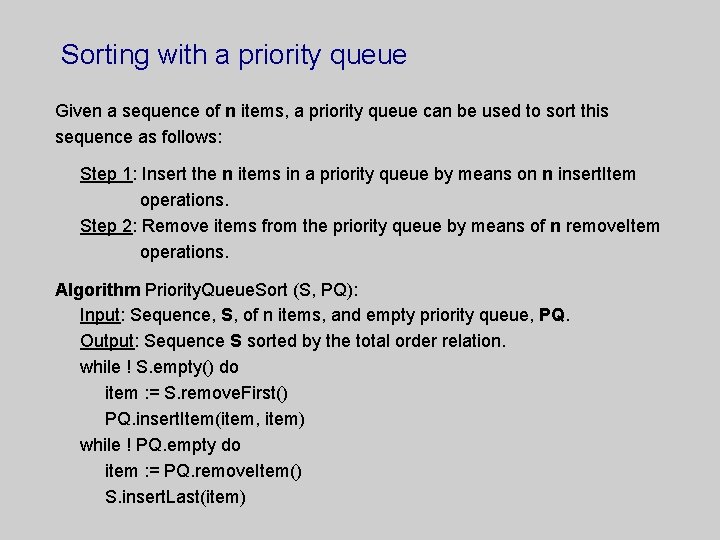
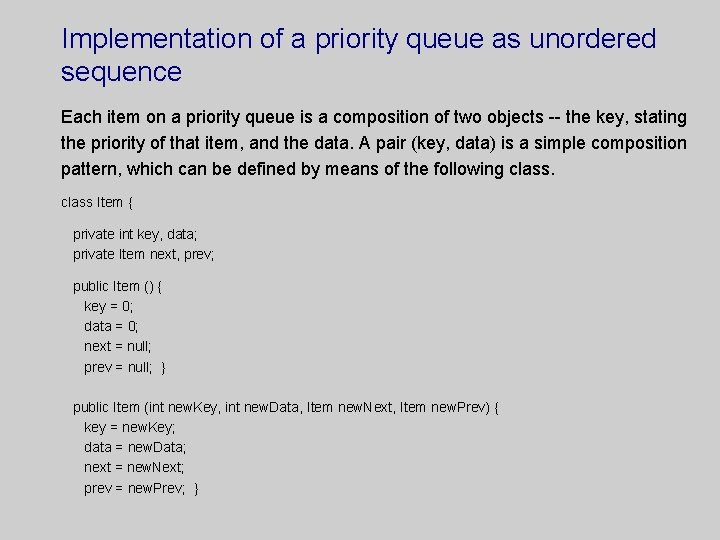
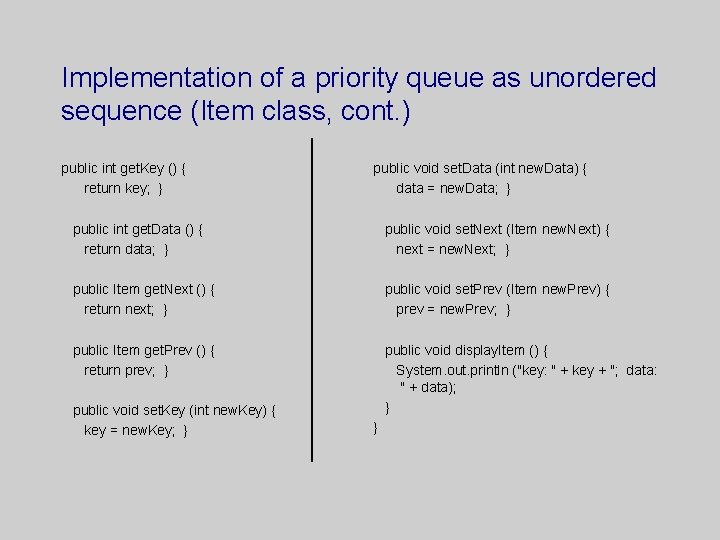
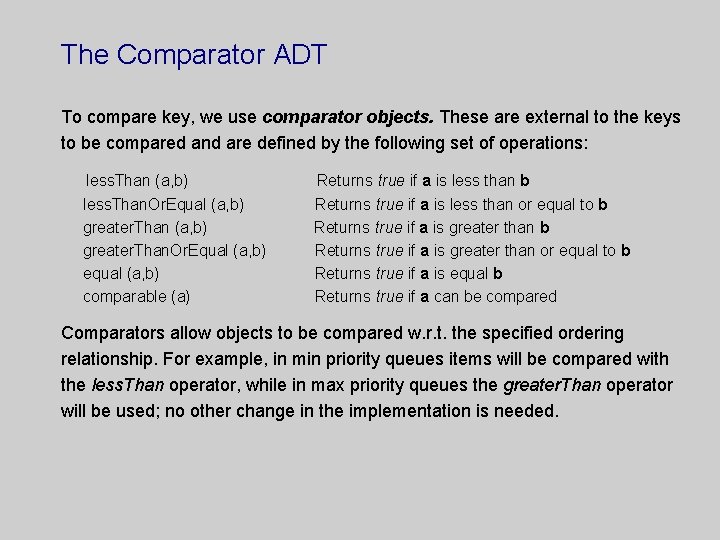
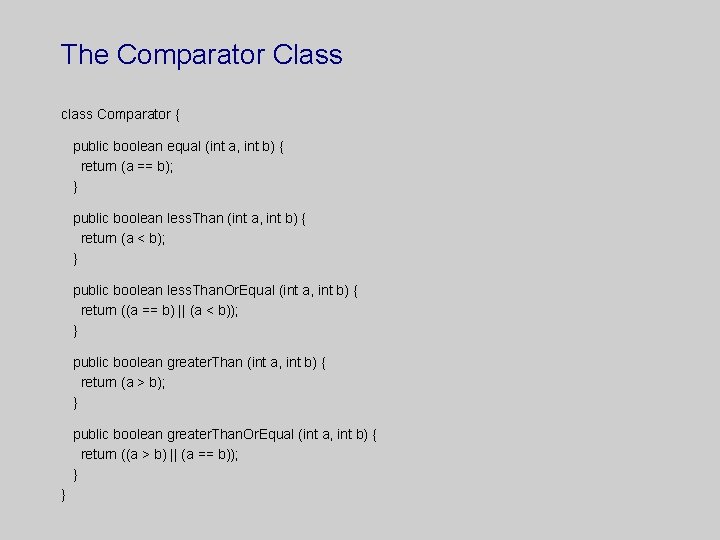
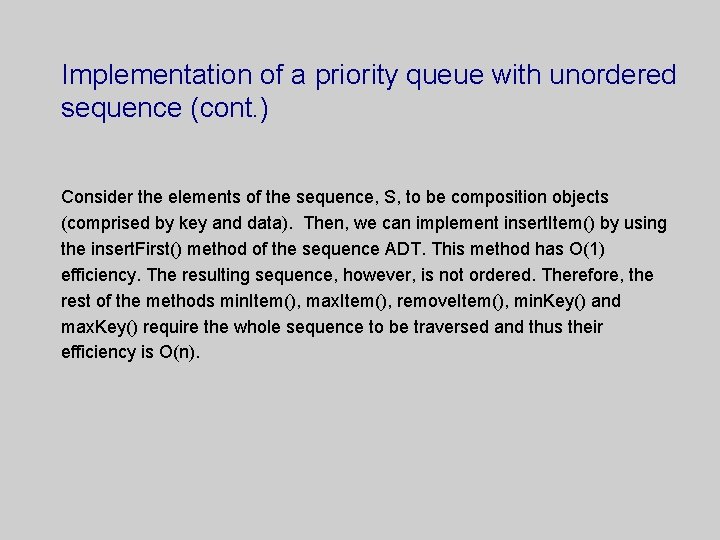
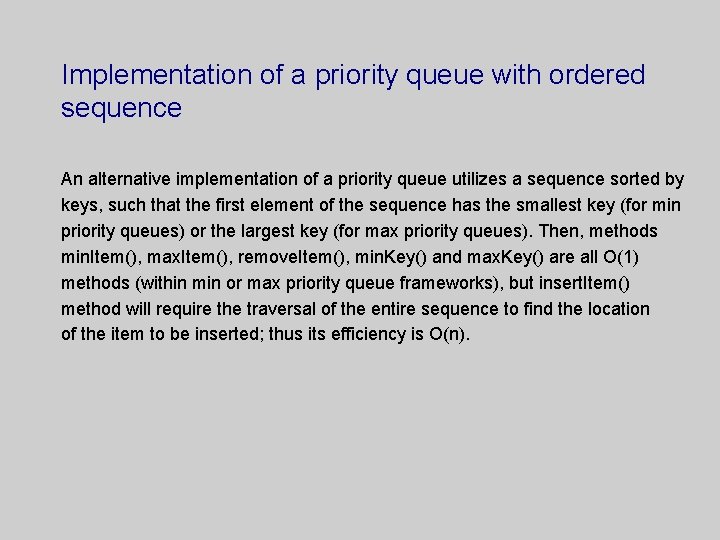
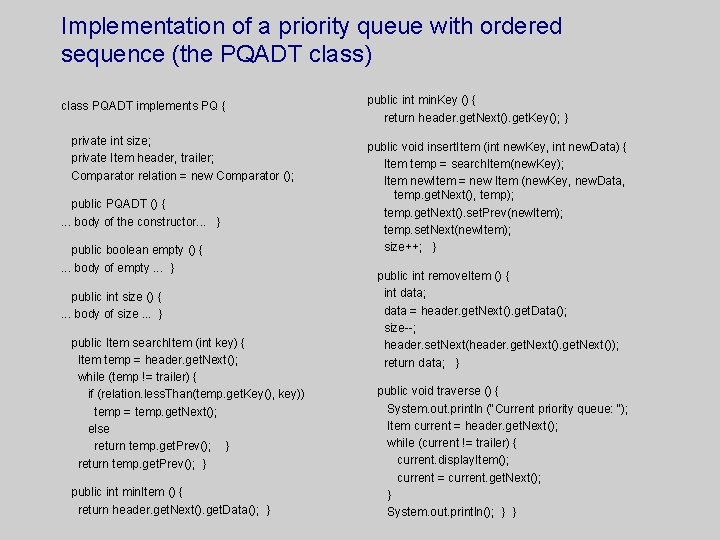
- Slides: 10
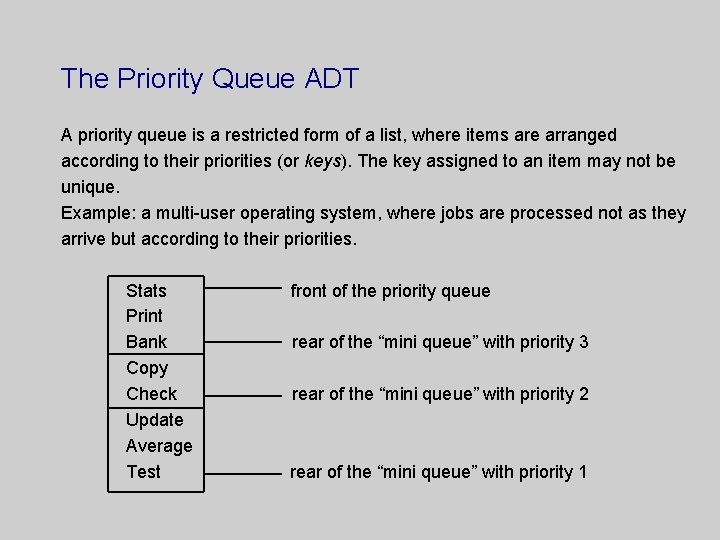
The Priority Queue ADT A priority queue is a restricted form of a list, where items are arranged according to their priorities (or keys). The key assigned to an item may not be unique. Example: a multi-user operating system, where jobs are processed not as they arrive but according to their priorities. Stats Print Bank Copy Check Update Average Test front of the priority queue rear of the “mini queue” with priority 3 rear of the “mini queue” with priority 2 rear of the “mini queue” with priority 1
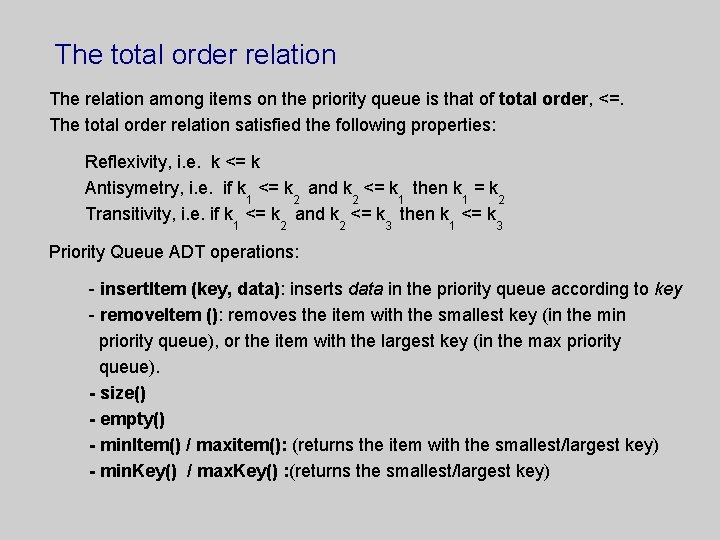
The total order relation The relation among items on the priority queue is that of total order, <=. The total order relation satisfied the following properties: Reflexivity, i. e. k <= k Antisymetry, i. e. if k <= k and k <= k then k = k 1 2 2 1 1 2 Transitivity, i. e. if k <= k and k <= k then k <= k 1 2 2 3 1 3 Priority Queue ADT operations: - insert. Item (key, data): inserts data in the priority queue according to key - remove. Item (): removes the item with the smallest key (in the min priority queue), or the item with the largest key (in the max priority queue). - size() - empty() - min. Item() / maxitem(): (returns the item with the smallest/largest key) - min. Key() / max. Key() : (returns the smallest/largest key)
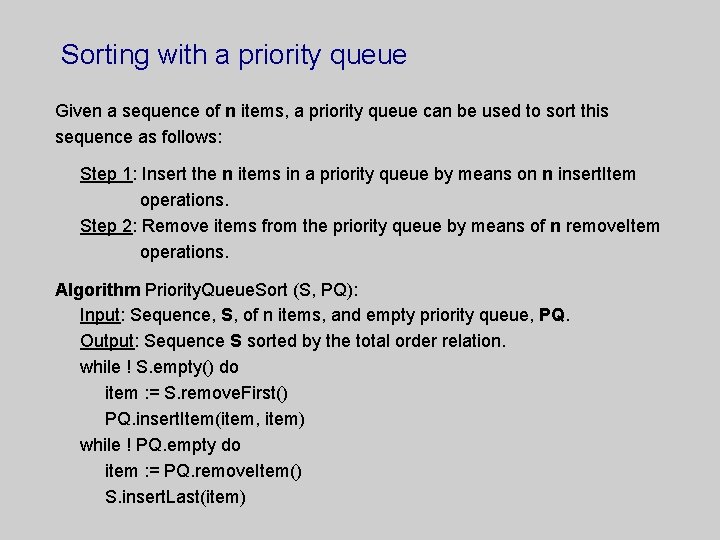
Sorting with a priority queue Given a sequence of n items, a priority queue can be used to sort this sequence as follows: Step 1: Insert the n items in a priority queue by means on n insert. Item operations. Step 2: Remove items from the priority queue by means of n remove. Item operations. Algorithm Priority. Queue. Sort (S, PQ): Input: Sequence, S, of n items, and empty priority queue, PQ. Output: Sequence S sorted by the total order relation. while ! S. empty() do item : = S. remove. First() PQ. insert. Item(item, item) while ! PQ. empty do item : = PQ. remove. Item() S. insert. Last(item)
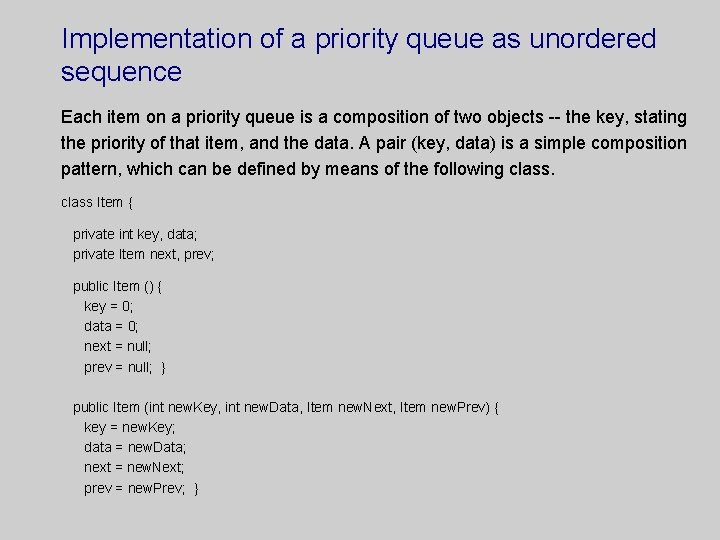
Implementation of a priority queue as unordered sequence Each item on a priority queue is a composition of two objects -- the key, stating the priority of that item, and the data. A pair (key, data) is a simple composition pattern, which can be defined by means of the following class Item { private int key, data; private Item next, prev; public Item () { key = 0; data = 0; next = null; prev = null; } public Item (int new. Key, int new. Data, Item new. Next, Item new. Prev) { key = new. Key; data = new. Data; next = new. Next; prev = new. Prev; }
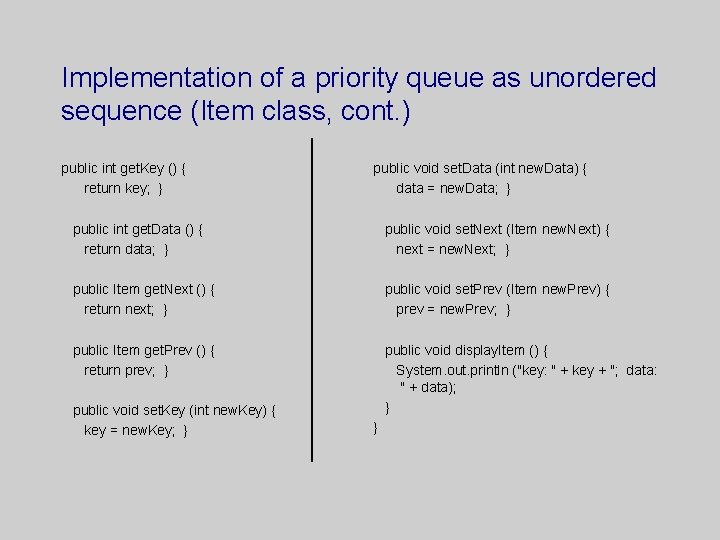
Implementation of a priority queue as unordered sequence (Item class, cont. ) public int get. Key () { return key; } public void set. Data (int new. Data) { data = new. Data; } public int get. Data () { return data; } public void set. Next (Item new. Next) { next = new. Next; } public Item get. Next () { return next; } public void set. Prev (Item new. Prev) { prev = new. Prev; } public Item get. Prev () { return prev; } public void display. Item () { System. out. println ("key: " + key + "; data: " + data); } public void set. Key (int new. Key) { key = new. Key; } }
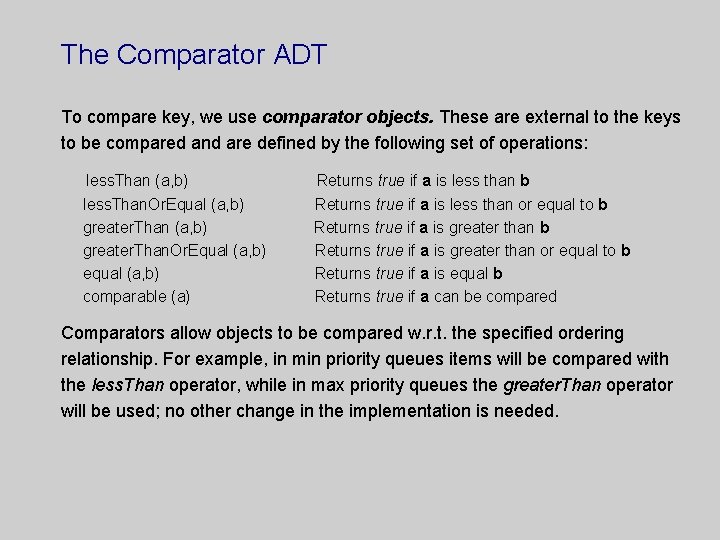
The Comparator ADT To compare key, we use comparator objects. These are external to the keys to be compared and are defined by the following set of operations: less. Than (a, b) less. Than. Or. Equal (a, b) greater. Than. Or. Equal (a, b) equal (a, b) comparable (a) Returns true if a is less than b Returns true if a is less than or equal to b Returns true if a is greater than or equal to b Returns true if a is equal b Returns true if a can be compared Comparators allow objects to be compared w. r. t. the specified ordering relationship. For example, in min priority queues items will be compared with the less. Than operator, while in max priority queues the greater. Than operator will be used; no other change in the implementation is needed.
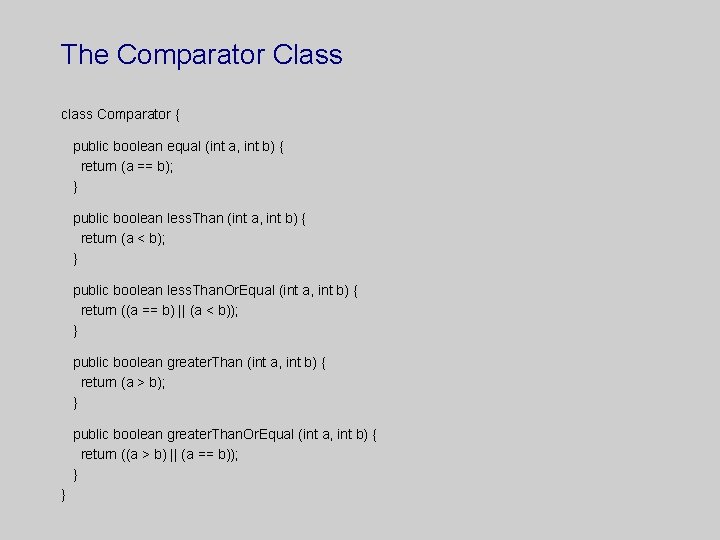
The Comparator Class class Comparator { public boolean equal (int a, int b) { return (a == b); } public boolean less. Than (int a, int b) { return (a < b); } public boolean less. Than. Or. Equal (int a, int b) { return ((a == b) || (a < b)); } public boolean greater. Than (int a, int b) { return (a > b); } public boolean greater. Than. Or. Equal (int a, int b) { return ((a > b) || (a == b)); } }
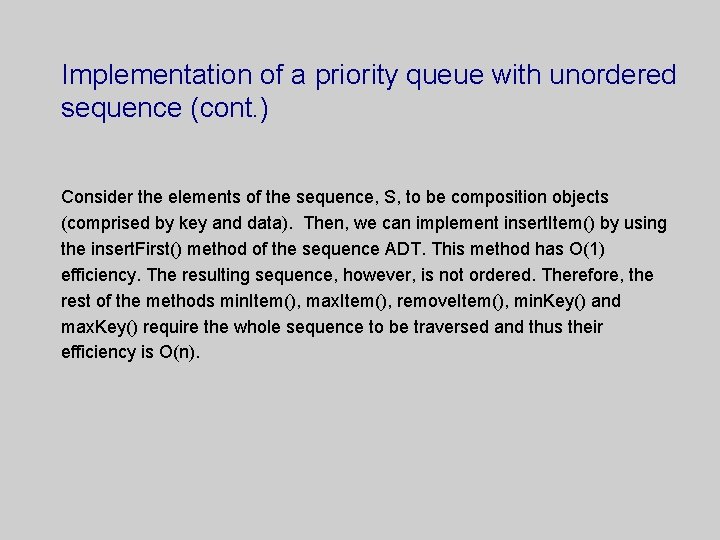
Implementation of a priority queue with unordered sequence (cont. ) Consider the elements of the sequence, S, to be composition objects (comprised by key and data). Then, we can implement insert. Item() by using the insert. First() method of the sequence ADT. This method has O(1) efficiency. The resulting sequence, however, is not ordered. Therefore, the rest of the methods min. Item(), max. Item(), remove. Item(), min. Key() and max. Key() require the whole sequence to be traversed and thus their efficiency is O(n).
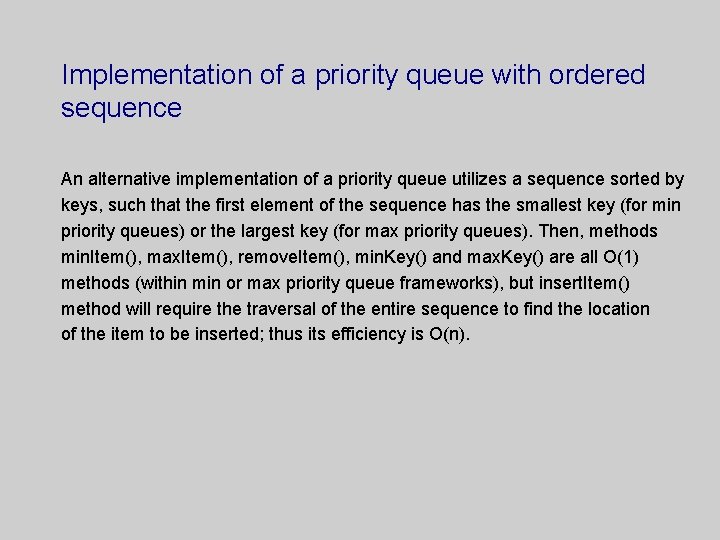
Implementation of a priority queue with ordered sequence An alternative implementation of a priority queue utilizes a sequence sorted by keys, such that the first element of the sequence has the smallest key (for min priority queues) or the largest key (for max priority queues). Then, methods min. Item(), max. Item(), remove. Item(), min. Key() and max. Key() are all O(1) methods (within min or max priority queue frameworks), but insert. Item() method will require the traversal of the entire sequence to find the location of the item to be inserted; thus its efficiency is O(n).
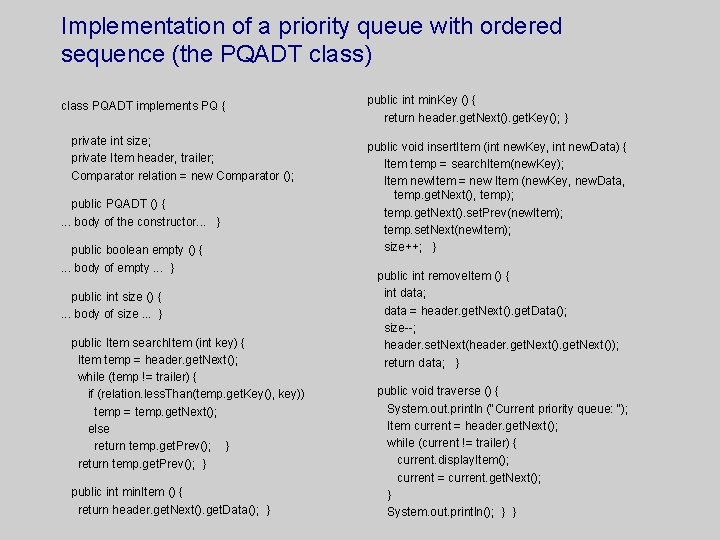
Implementation of a priority queue with ordered sequence (the PQADT class) class PQADT implements PQ { private int size; private Item header, trailer; Comparator relation = new Comparator (); public PQADT () {. . . body of the constructor. . . } public boolean empty () {. . . body of empty. . . } public int size () {. . . body of size. . . } public Item search. Item (int key) { Item temp = header. get. Next(); while (temp != trailer) { if (relation. less. Than(temp. get. Key(), key)) temp = temp. get. Next(); else return temp. get. Prev(); } public int min. Item () { return header. get. Next(). get. Data(); } public int min. Key () { return header. get. Next(). get. Key(); } public void insert. Item (int new. Key, int new. Data) { Item temp = search. Item(new. Key); Item new. Item = new Item (new. Key, new. Data, temp. get. Next(), temp); temp. get. Next(). set. Prev(new. Item); temp. set. Next(new. Item); size++; } public int remove. Item () { int data; data = header. get. Next(). get. Data(); size--; header. set. Next(header. get. Next()); return data; } public void traverse () { System. out. println ("Current priority queue: "); Item current = header. get. Next(); while (current != trailer) { current. display. Item(); current = current. get. Next(); } System. out. println(); } }