Queue Deque and Priority Queue Implementations Chapter 11
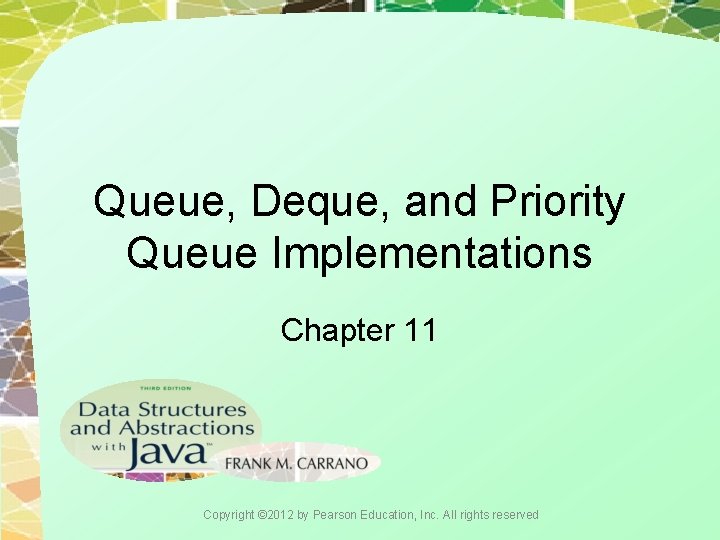
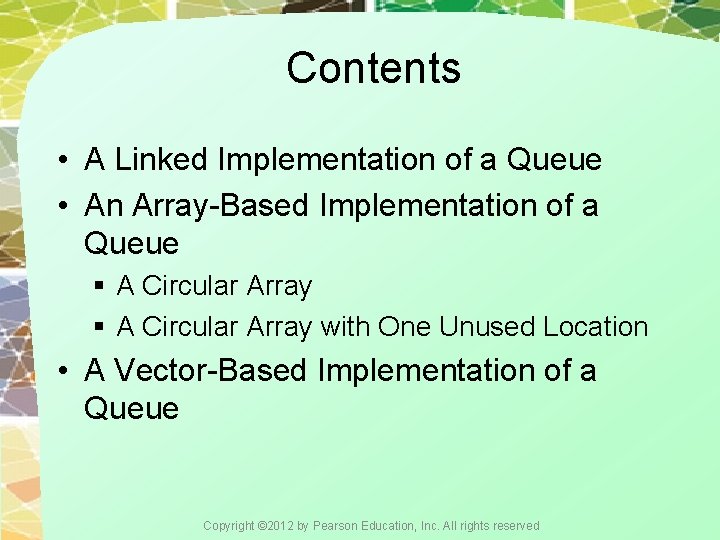
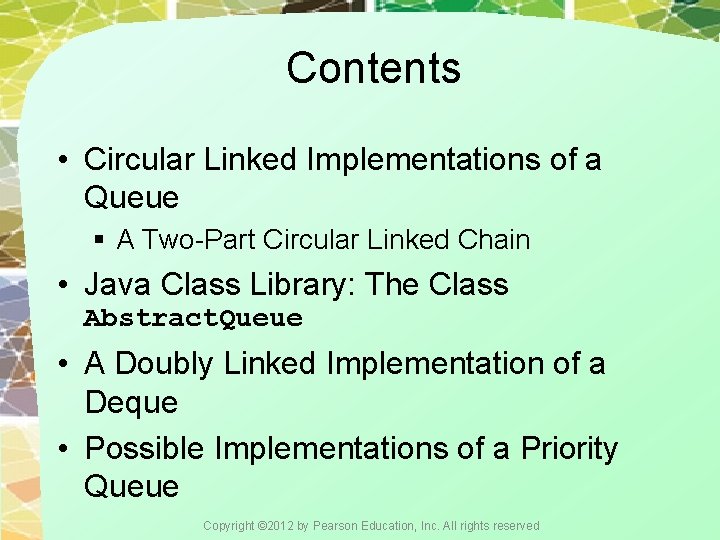
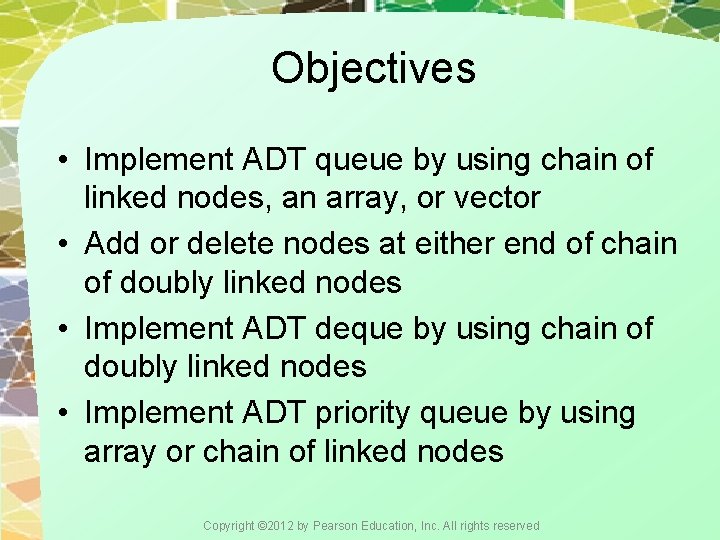
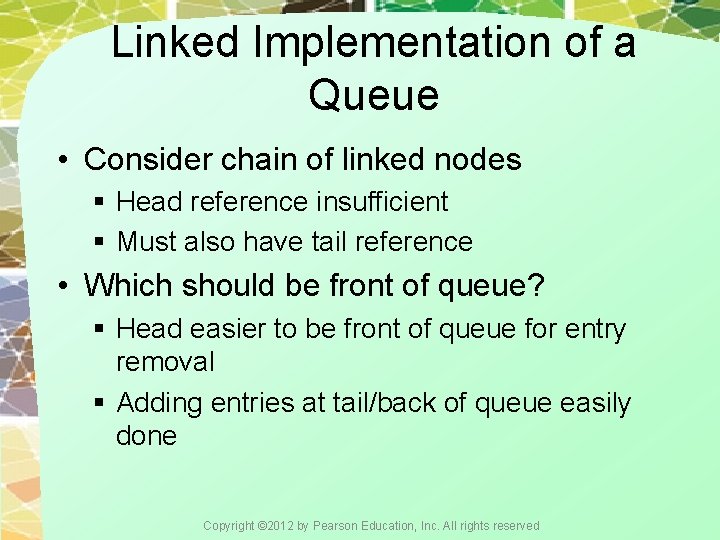
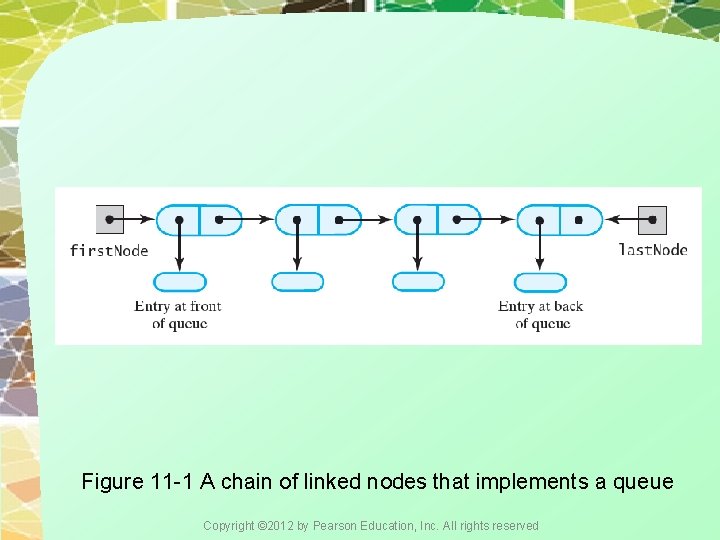
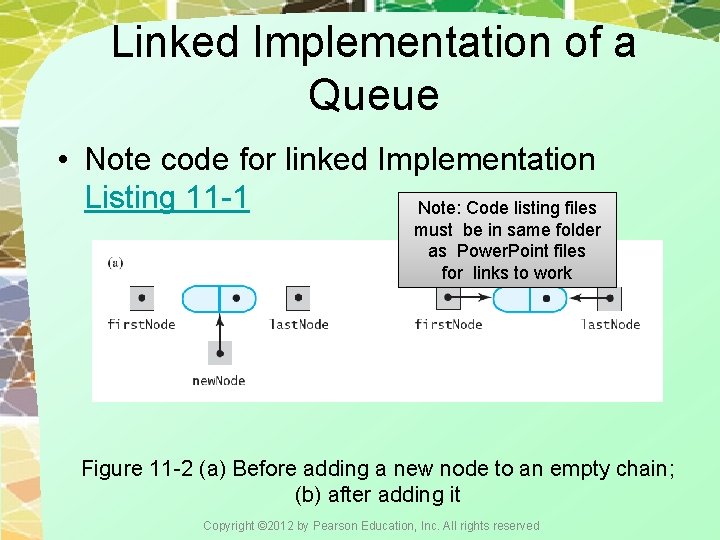
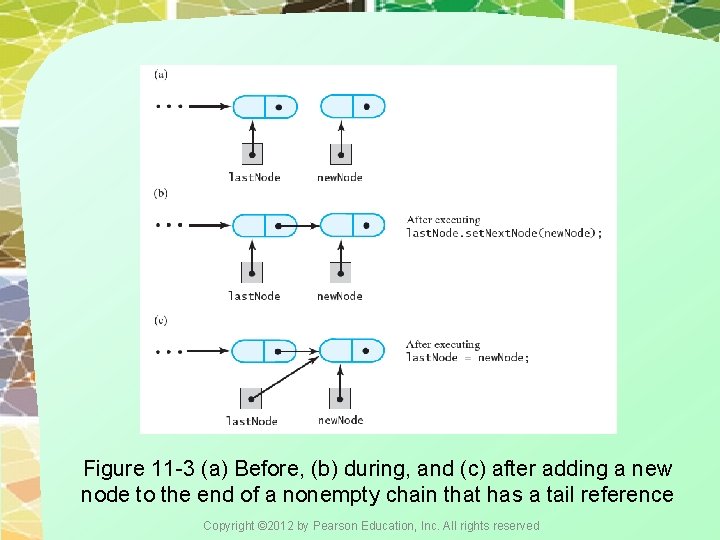
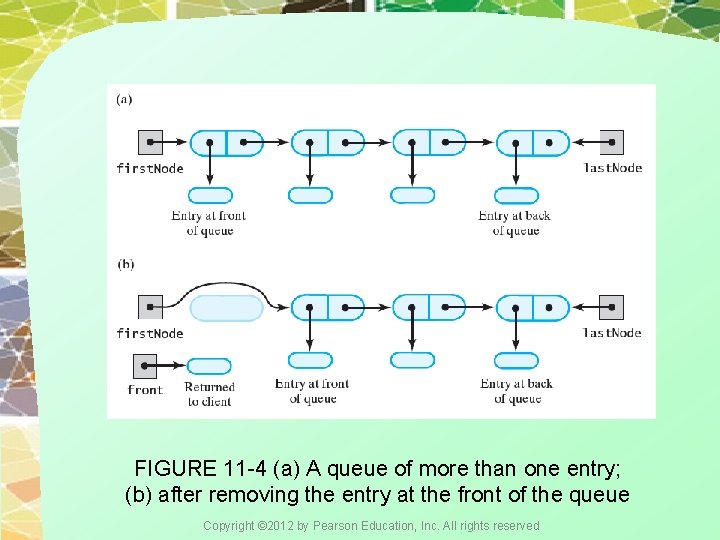
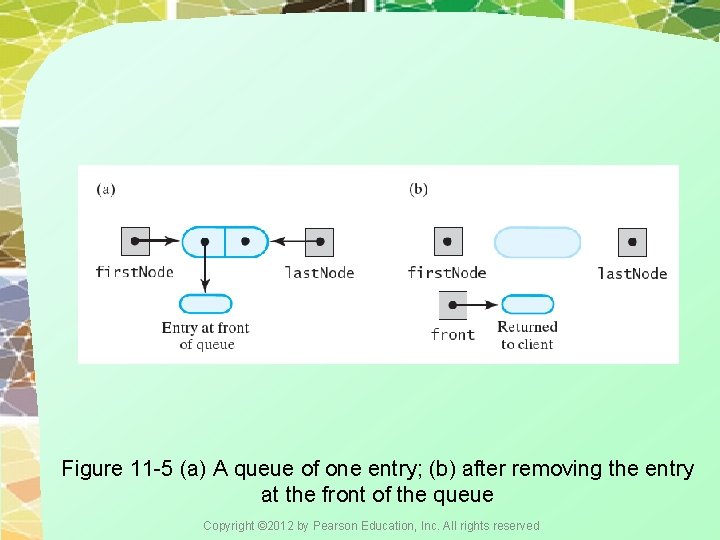
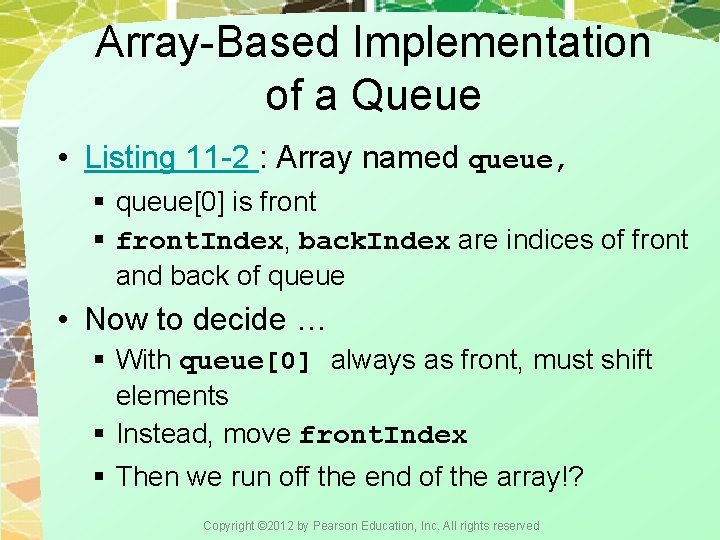
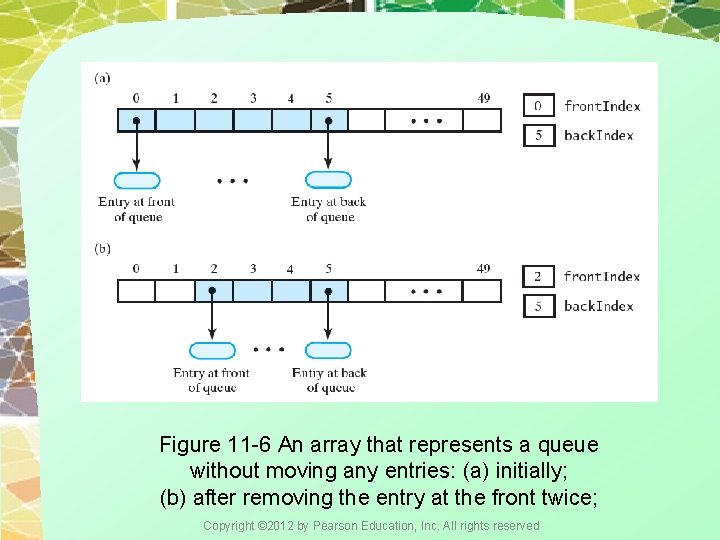
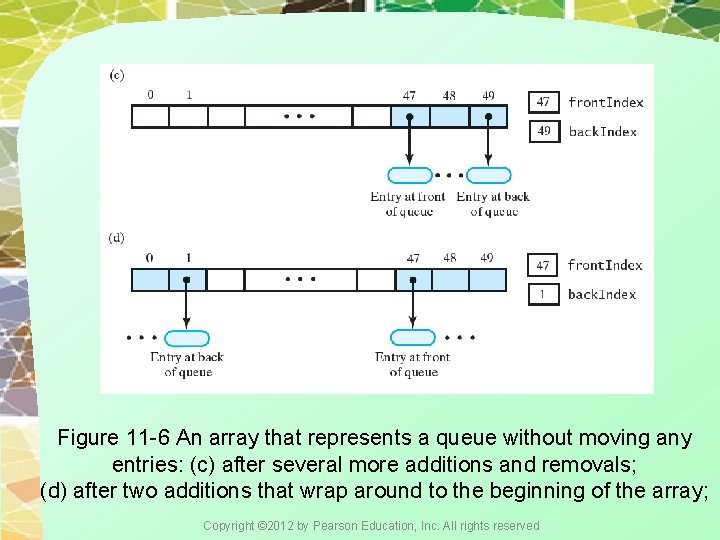
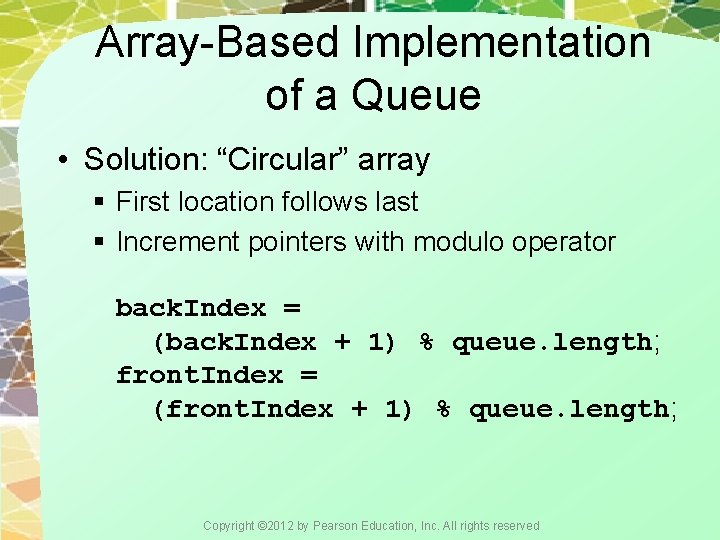
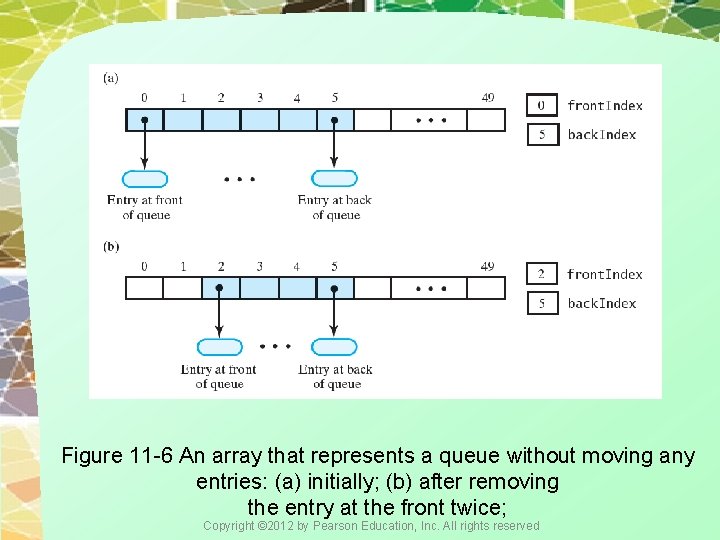
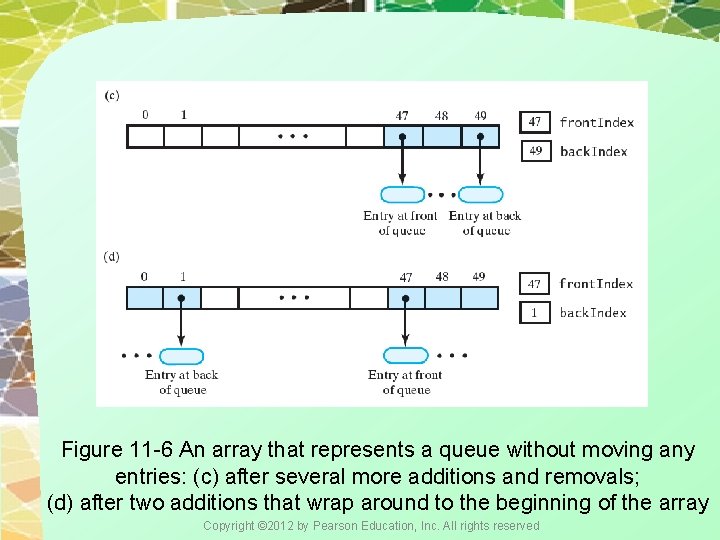
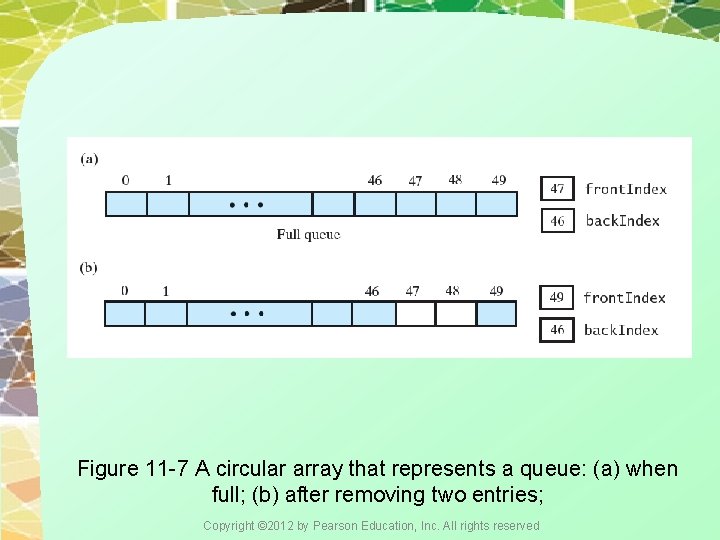
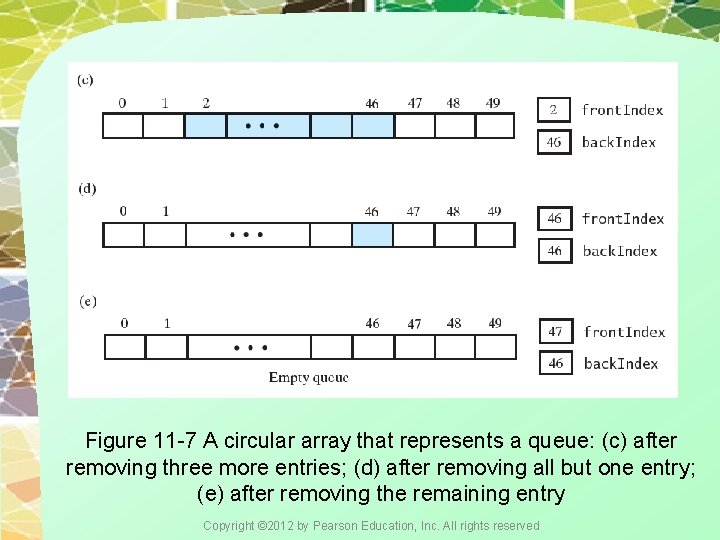
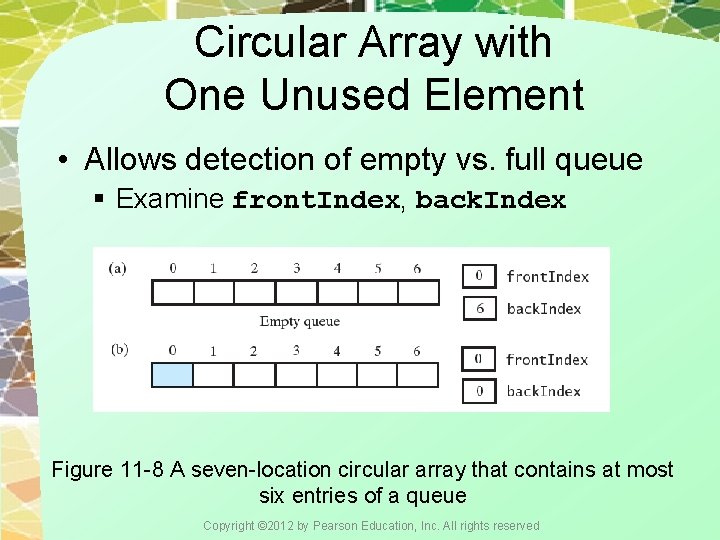
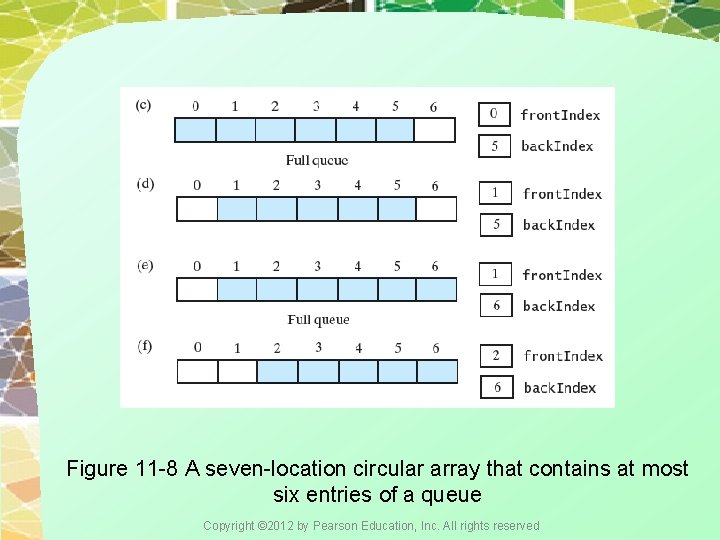
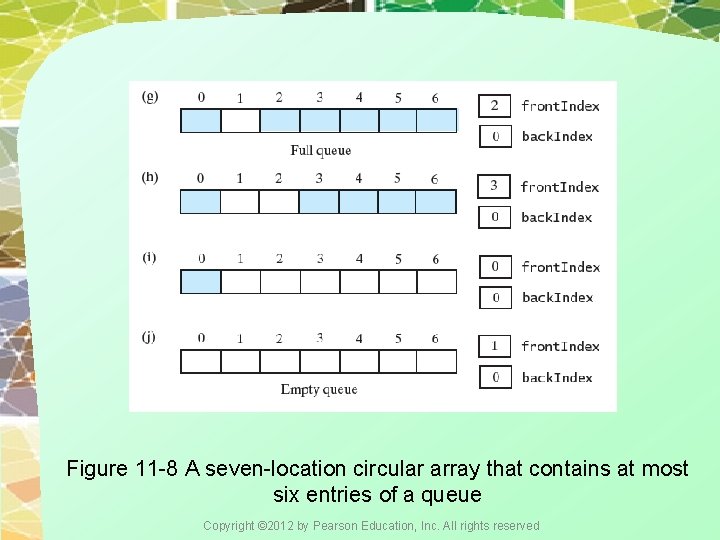
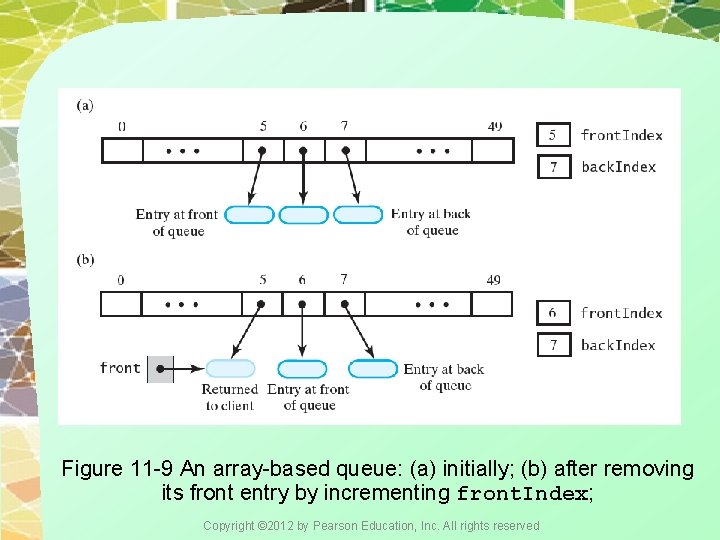
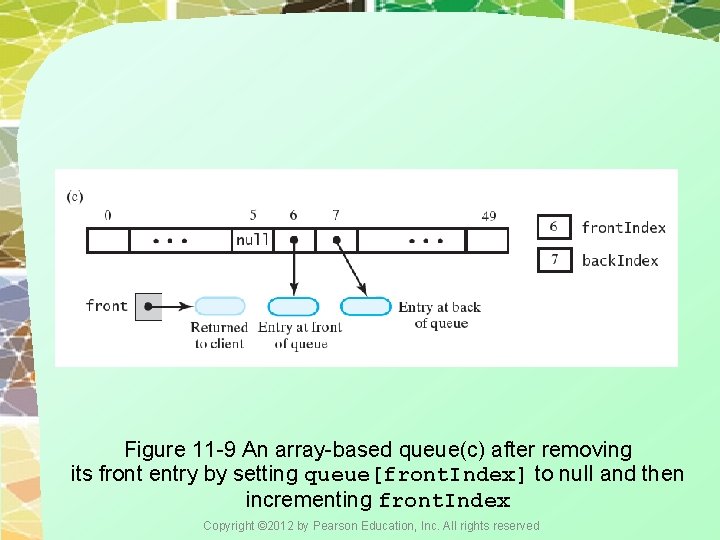
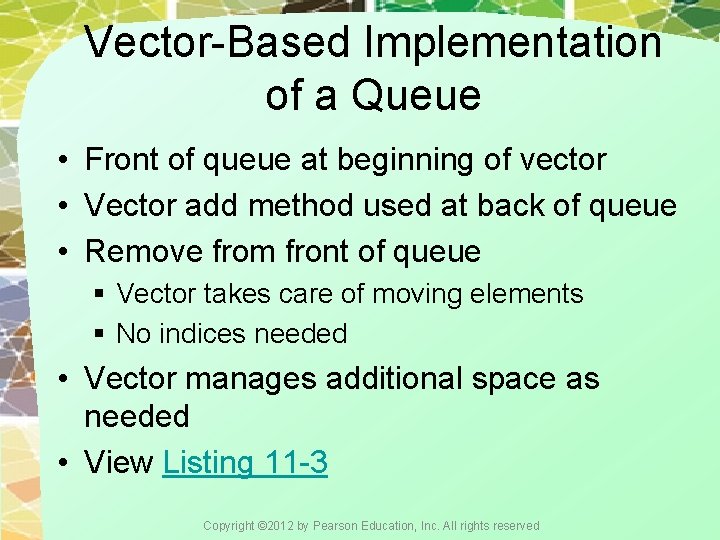
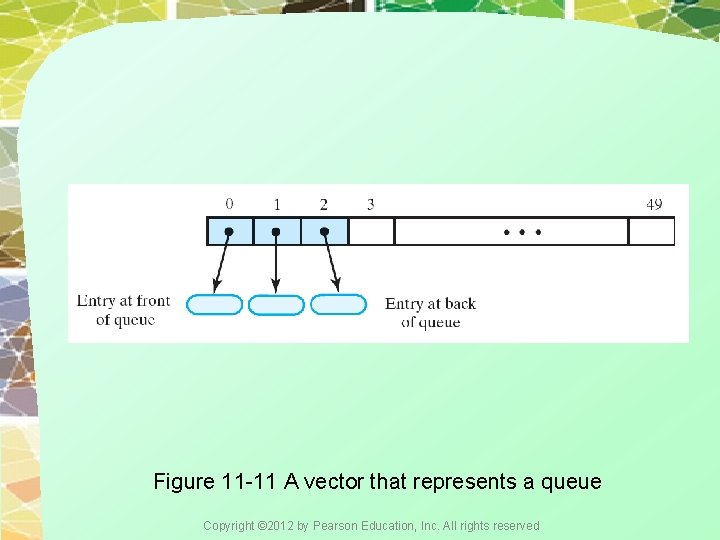
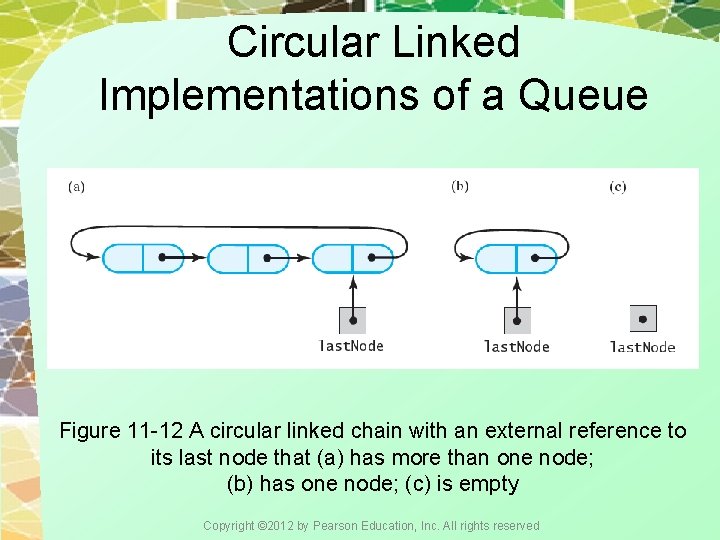
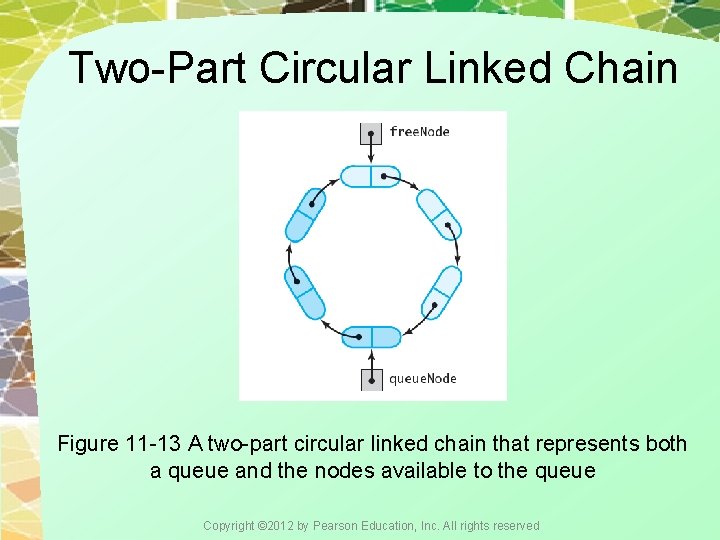
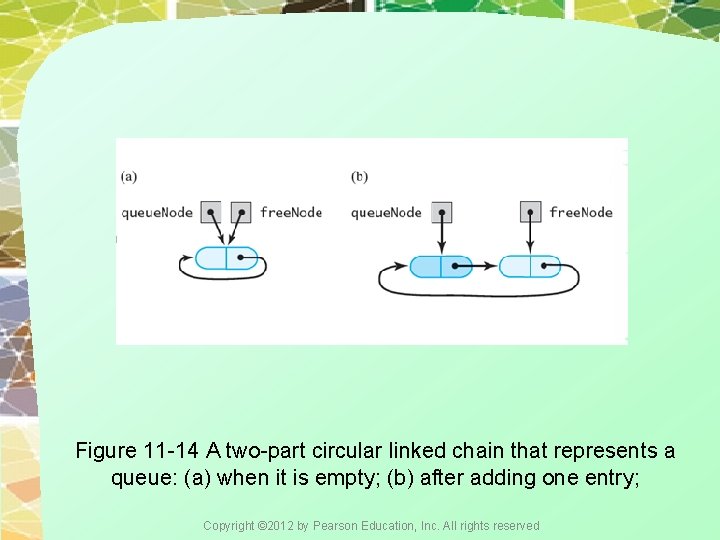
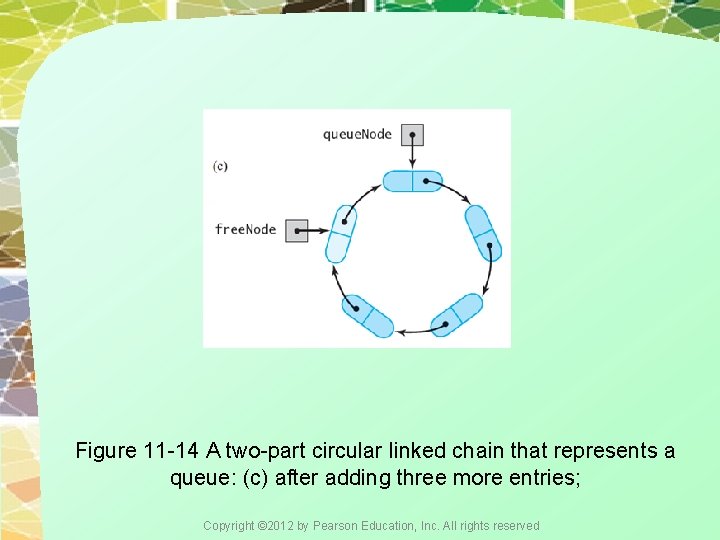
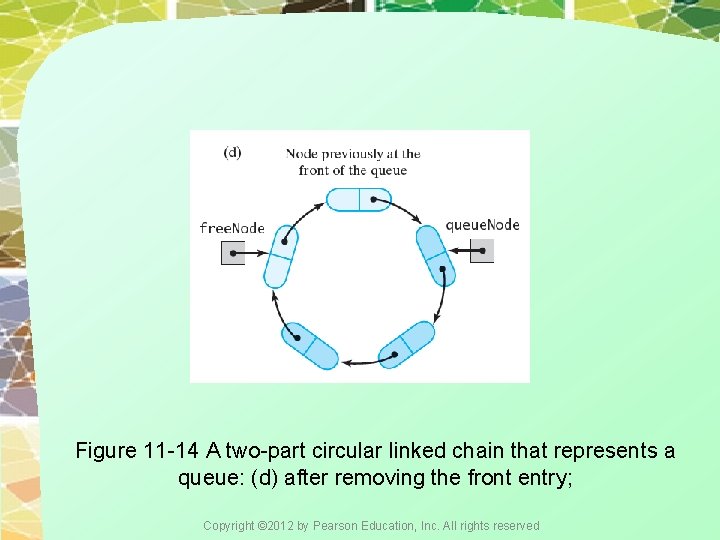
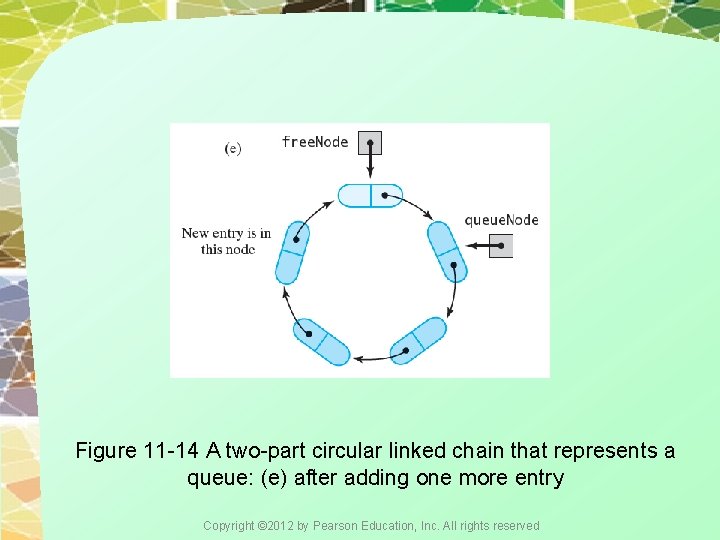
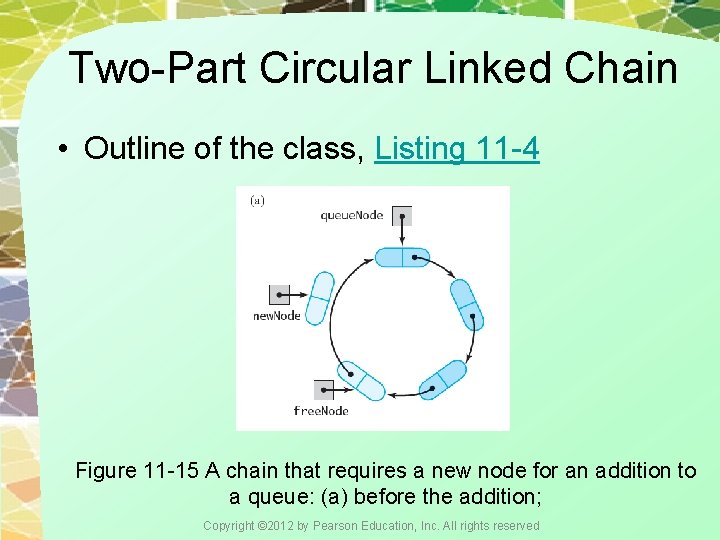
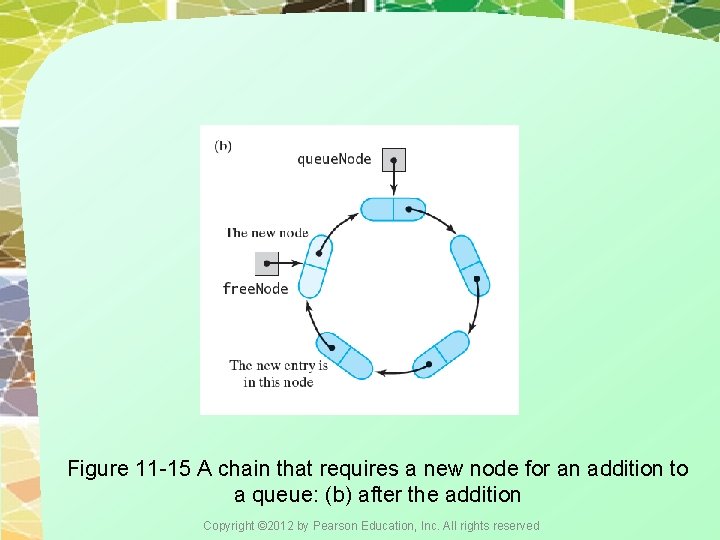
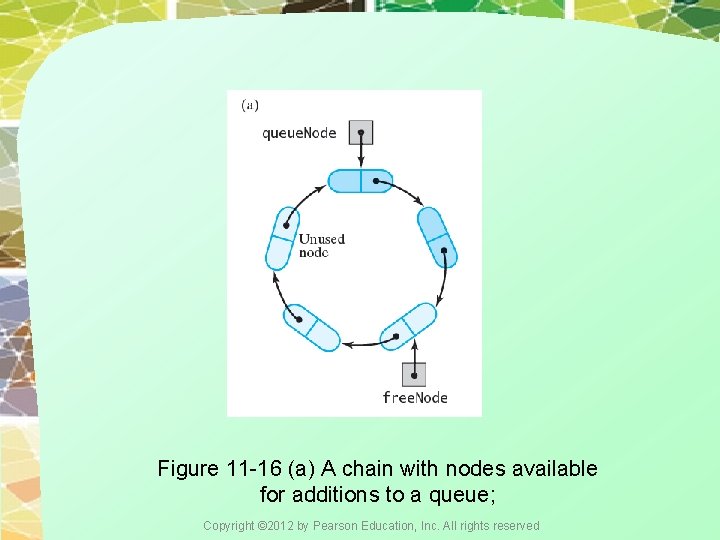
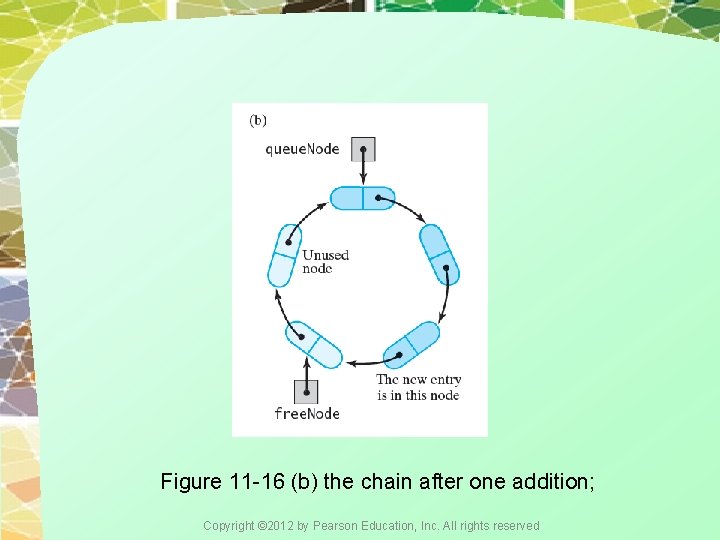
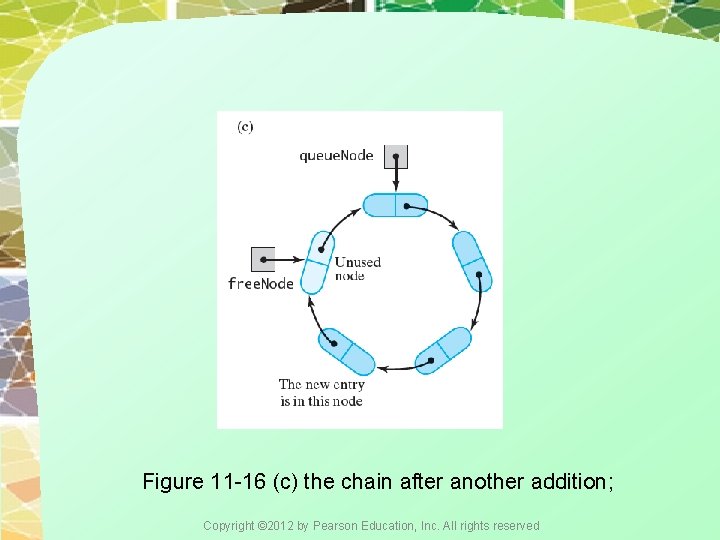
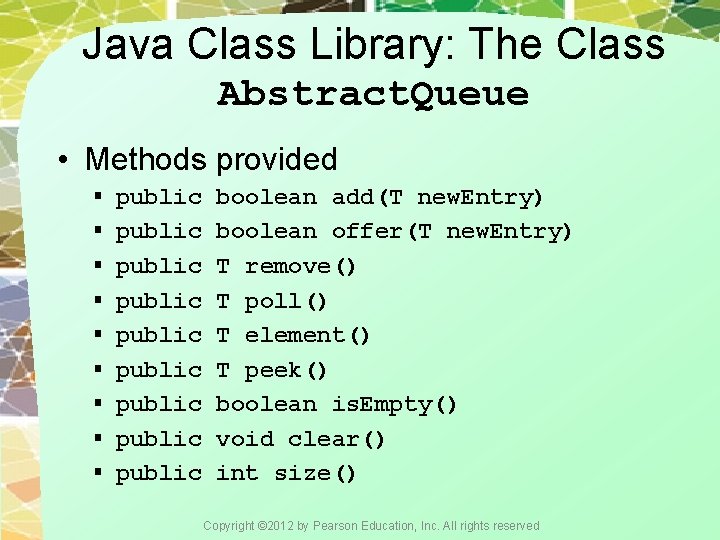
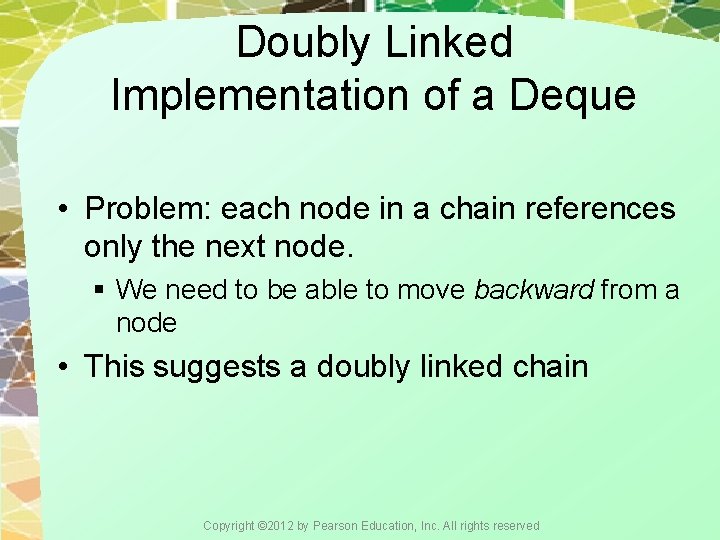
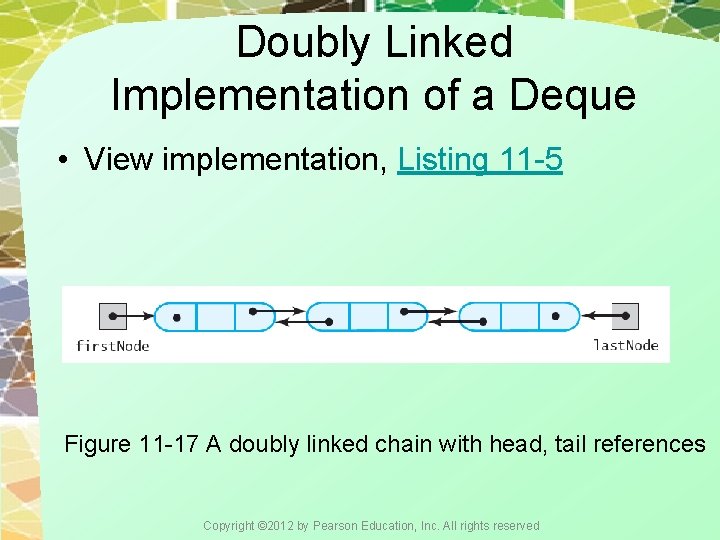
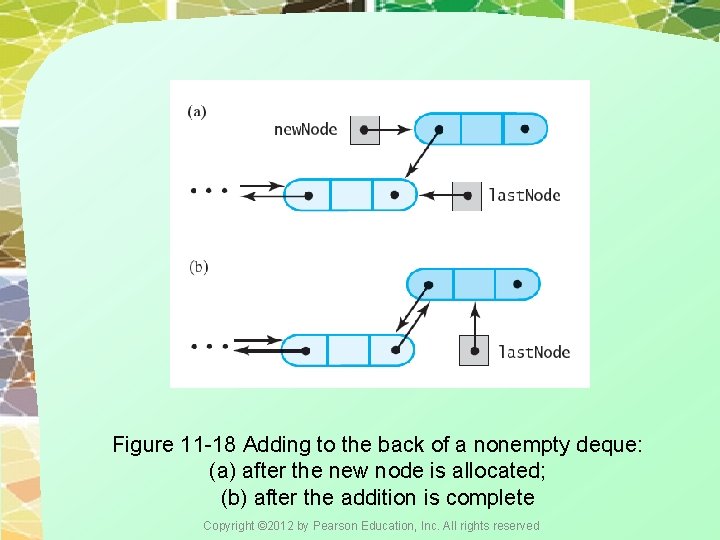
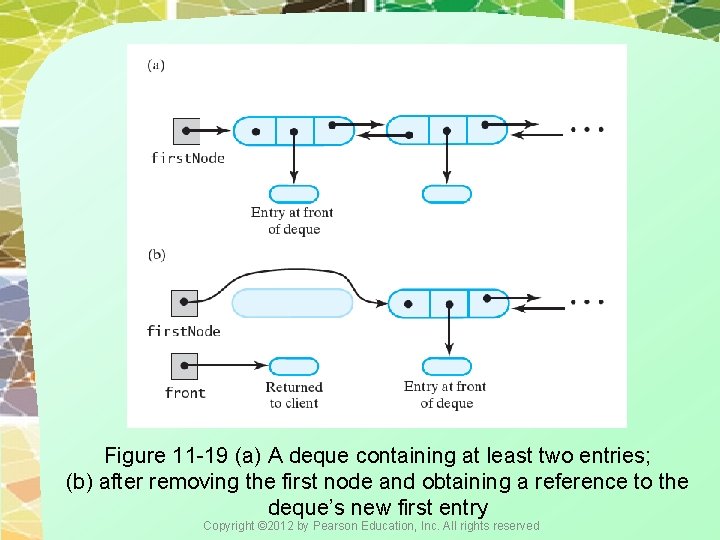
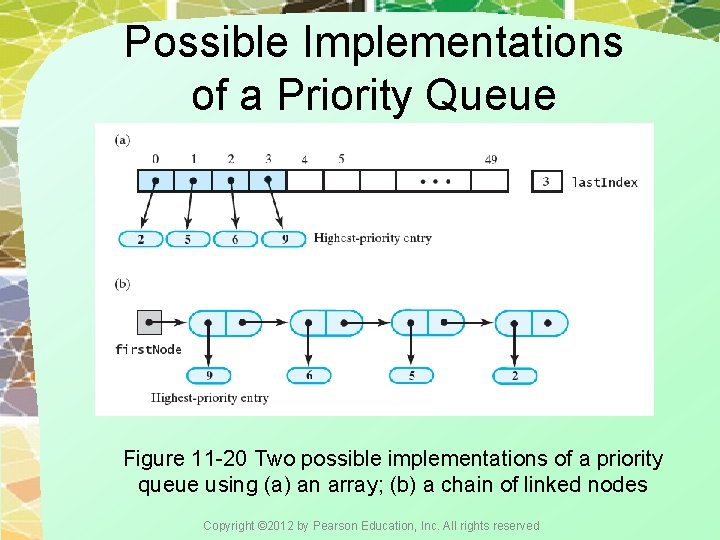
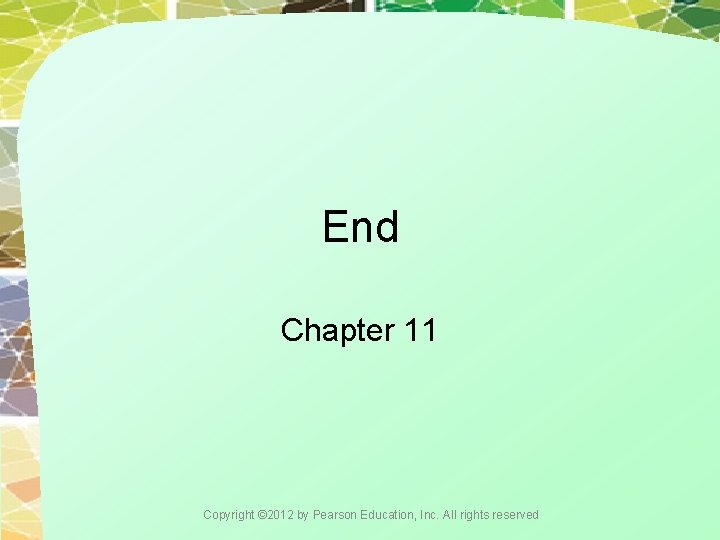
- Slides: 43
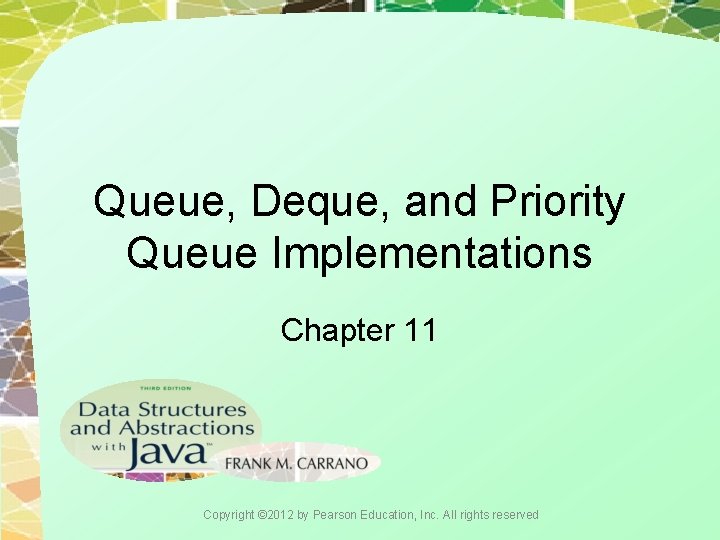
Queue, Deque, and Priority Queue Implementations Chapter 11 Copyright © 2012 by Pearson Education, Inc. All rights reserved
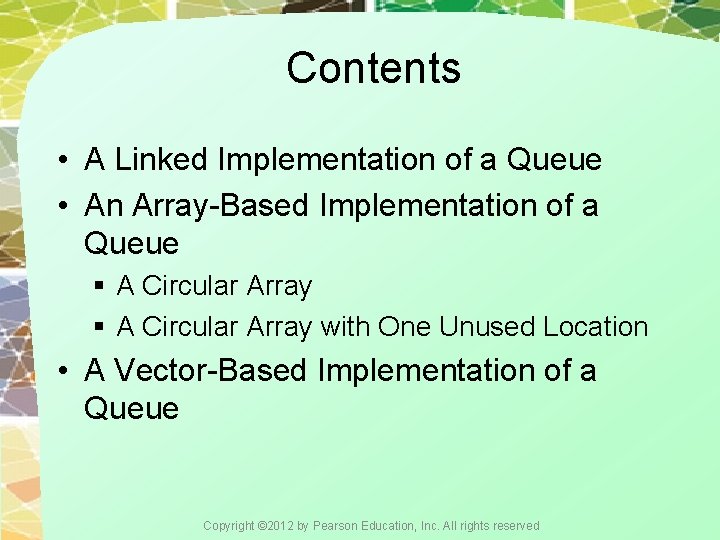
Contents • A Linked Implementation of a Queue • An Array-Based Implementation of a Queue § A Circular Array with One Unused Location • A Vector-Based Implementation of a Queue Copyright © 2012 by Pearson Education, Inc. All rights reserved
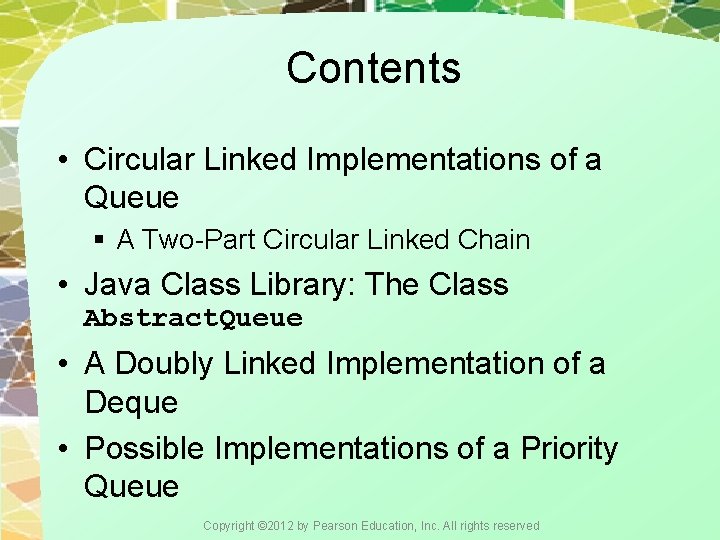
Contents • Circular Linked Implementations of a Queue § A Two-Part Circular Linked Chain • Java Class Library: The Class Abstract. Queue • A Doubly Linked Implementation of a Deque • Possible Implementations of a Priority Queue Copyright © 2012 by Pearson Education, Inc. All rights reserved
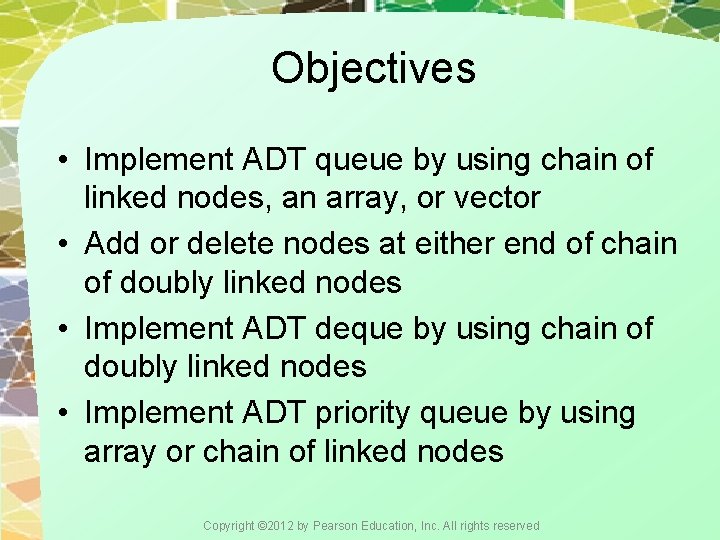
Objectives • Implement ADT queue by using chain of linked nodes, an array, or vector • Add or delete nodes at either end of chain of doubly linked nodes • Implement ADT deque by using chain of doubly linked nodes • Implement ADT priority queue by using array or chain of linked nodes Copyright © 2012 by Pearson Education, Inc. All rights reserved
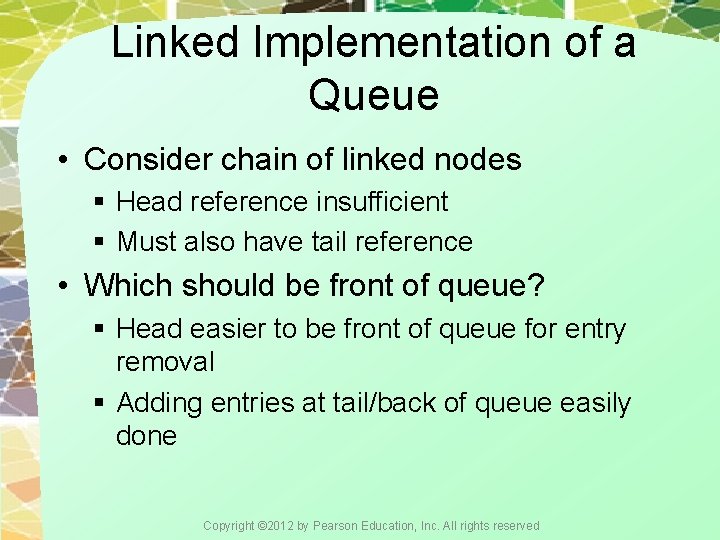
Linked Implementation of a Queue • Consider chain of linked nodes § Head reference insufficient § Must also have tail reference • Which should be front of queue? § Head easier to be front of queue for entry removal § Adding entries at tail/back of queue easily done Copyright © 2012 by Pearson Education, Inc. All rights reserved
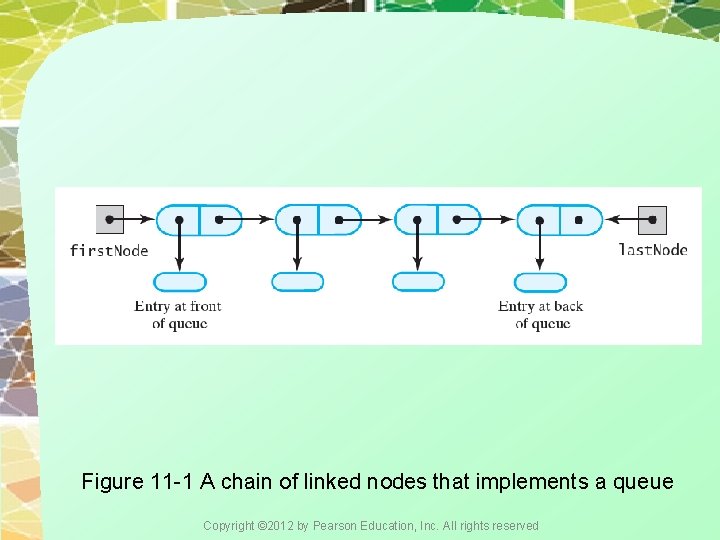
Figure 11 -1 A chain of linked nodes that implements a queue Copyright © 2012 by Pearson Education, Inc. All rights reserved
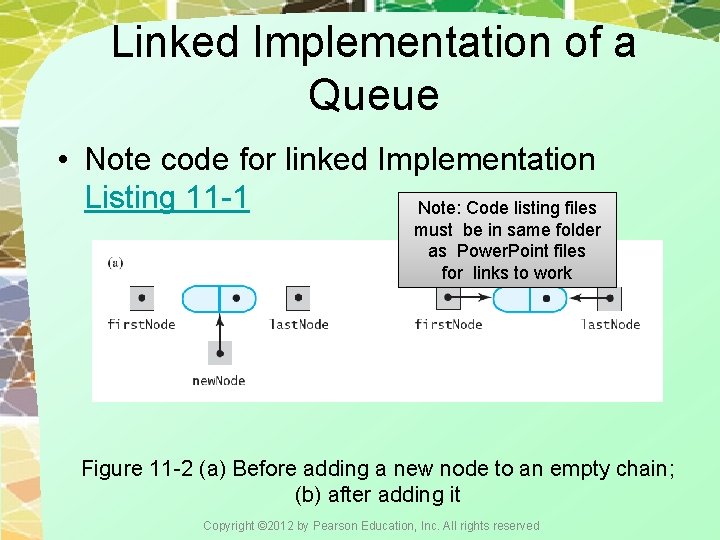
Linked Implementation of a Queue • Note code for linked Implementation Listing 11 -1 Note: Code listing files must be in same folder as Power. Point files for links to work Figure 11 -2 (a) Before adding a new node to an empty chain; (b) after adding it Copyright © 2012 by Pearson Education, Inc. All rights reserved
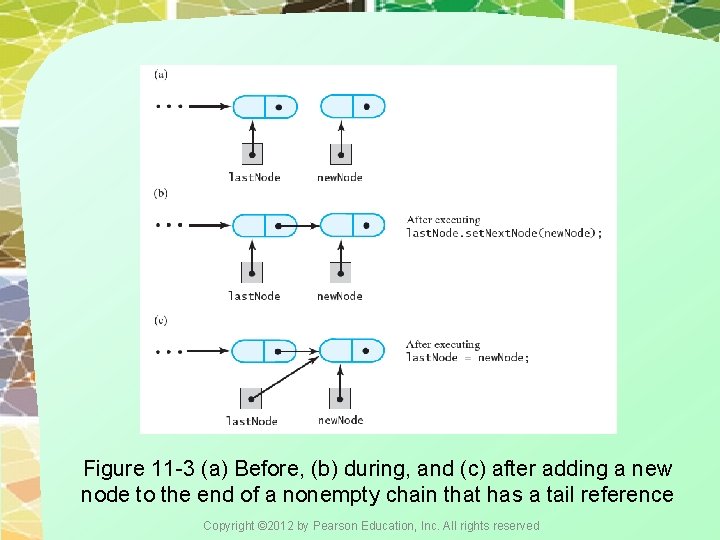
Figure 11 -3 (a) Before, (b) during, and (c) after adding a new node to the end of a nonempty chain that has a tail reference Copyright © 2012 by Pearson Education, Inc. All rights reserved
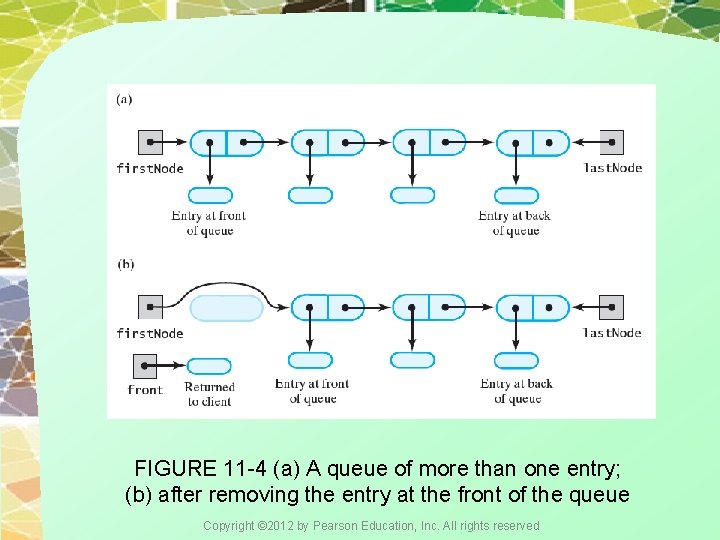
FIGURE 11 -4 (a) A queue of more than one entry; (b) after removing the entry at the front of the queue Copyright © 2012 by Pearson Education, Inc. All rights reserved
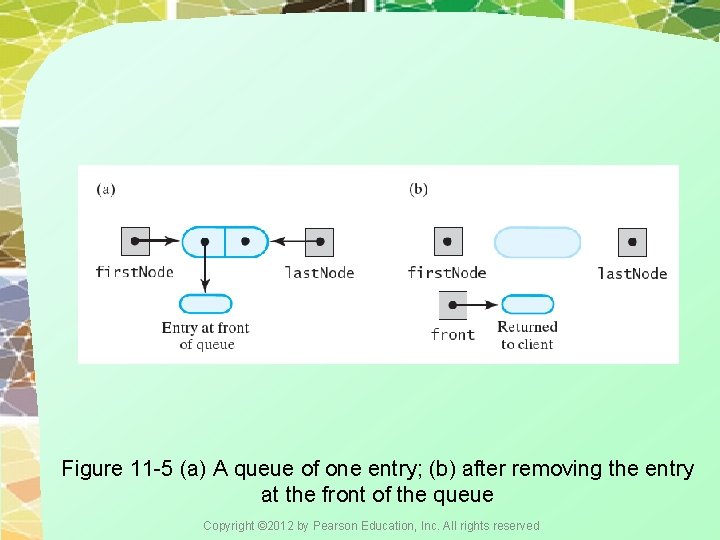
Figure 11 -5 (a) A queue of one entry; (b) after removing the entry at the front of the queue Copyright © 2012 by Pearson Education, Inc. All rights reserved
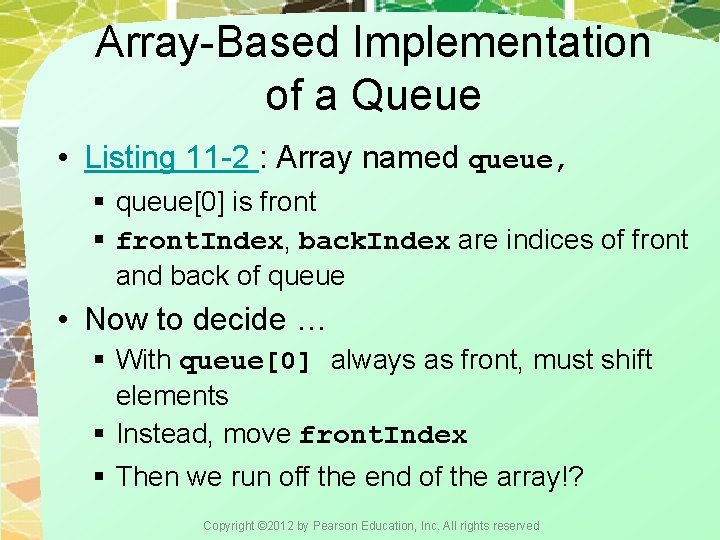
Array-Based Implementation of a Queue • Listing 11 -2 : Array named queue, § queue[0] is front § front. Index, back. Index are indices of front and back of queue • Now to decide … § With queue[0] always as front, must shift elements § Instead, move front. Index § Then we run off the end of the array!? Copyright © 2012 by Pearson Education, Inc. All rights reserved
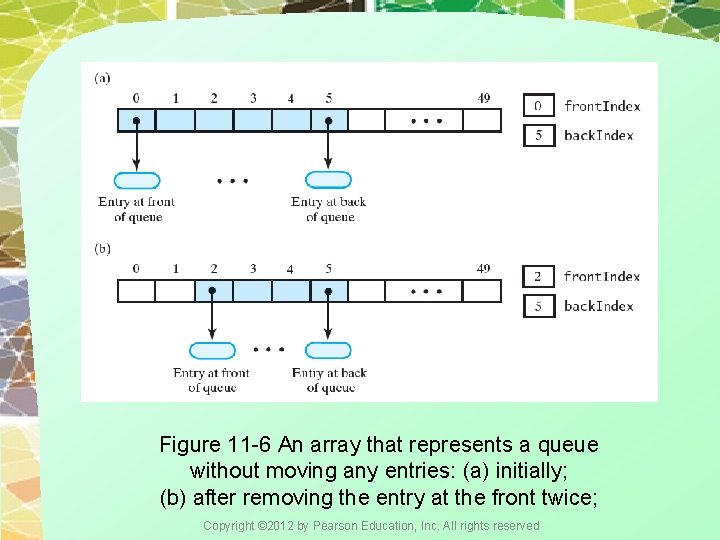
Figure 11 -6 An array that represents a queue without moving any entries: (a) initially; (b) after removing the entry at the front twice; Copyright © 2012 by Pearson Education, Inc. All rights reserved
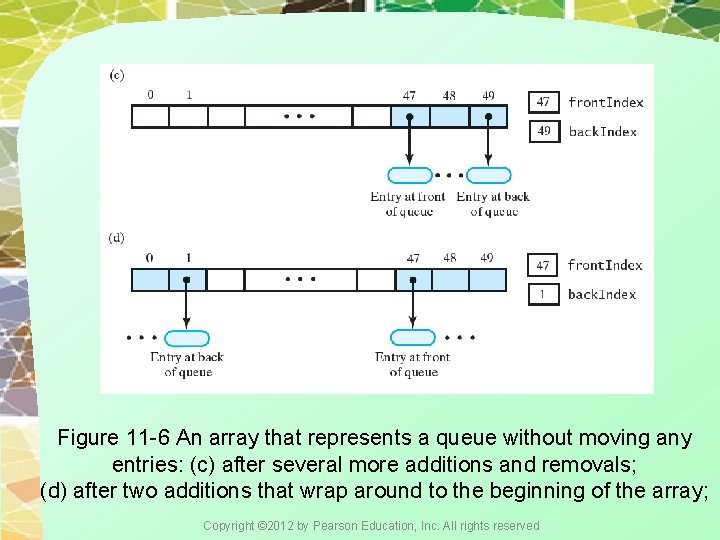
Figure 11 -6 An array that represents a queue without moving any entries: (c) after several more additions and removals; (d) after two additions that wrap around to the beginning of the array; Copyright © 2012 by Pearson Education, Inc. All rights reserved
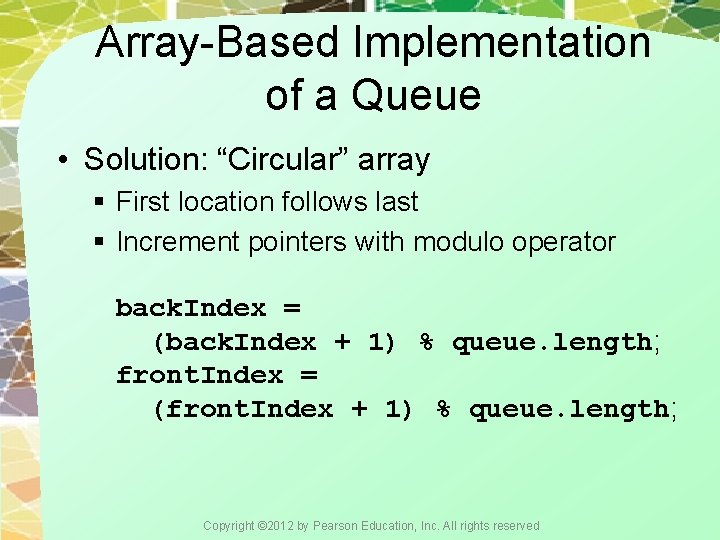
Array-Based Implementation of a Queue • Solution: “Circular” array § First location follows last § Increment pointers with modulo operator back. Index = (back. Index + 1) % queue. length; front. Index = (front. Index + 1) % queue. length; Copyright © 2012 by Pearson Education, Inc. All rights reserved
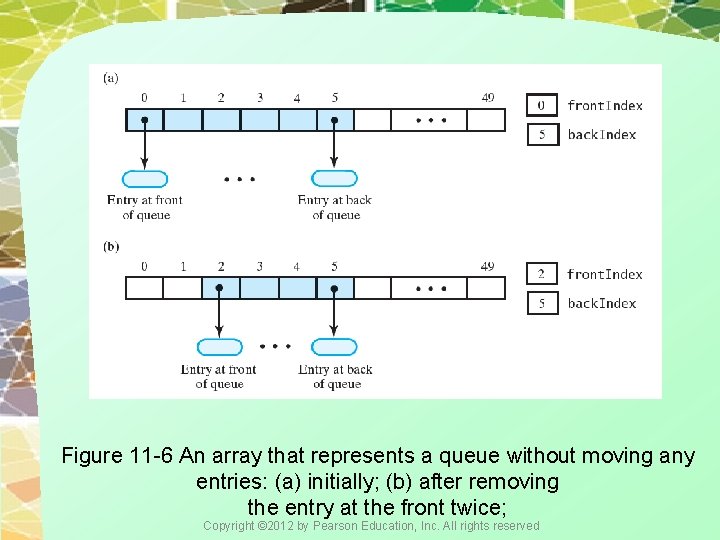
Figure 11 -6 An array that represents a queue without moving any entries: (a) initially; (b) after removing the entry at the front twice; Copyright © 2012 by Pearson Education, Inc. All rights reserved
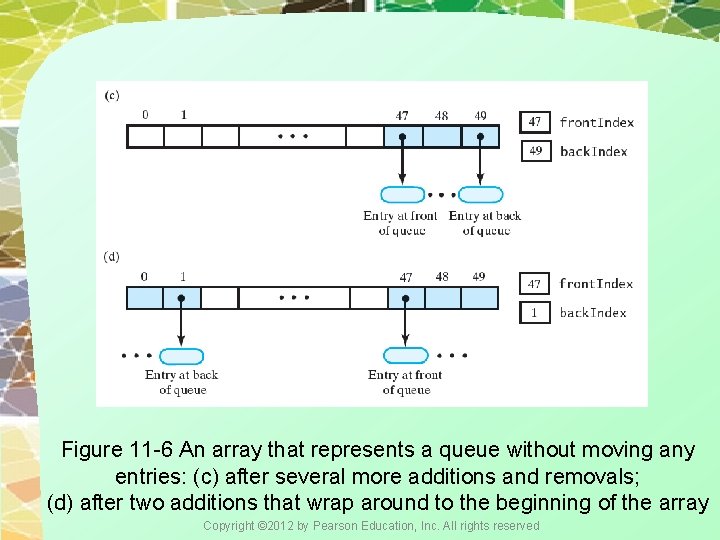
Figure 11 -6 An array that represents a queue without moving any entries: (c) after several more additions and removals; (d) after two additions that wrap around to the beginning of the array Copyright © 2012 by Pearson Education, Inc. All rights reserved
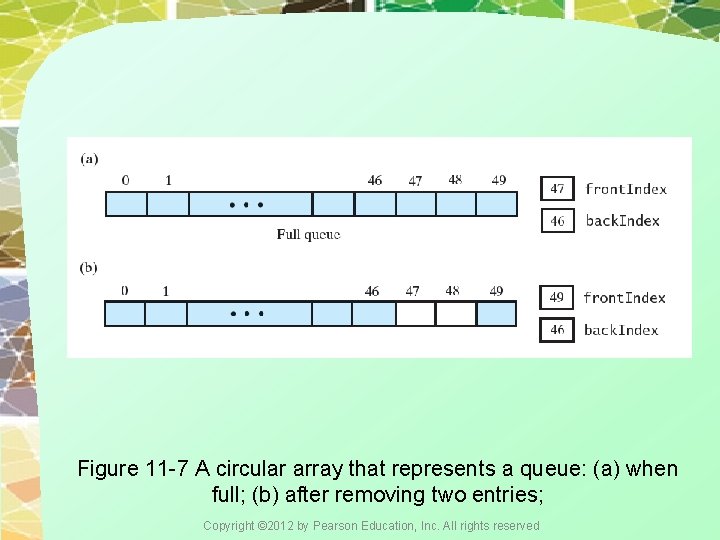
Figure 11 -7 A circular array that represents a queue: (a) when full; (b) after removing two entries; Copyright © 2012 by Pearson Education, Inc. All rights reserved
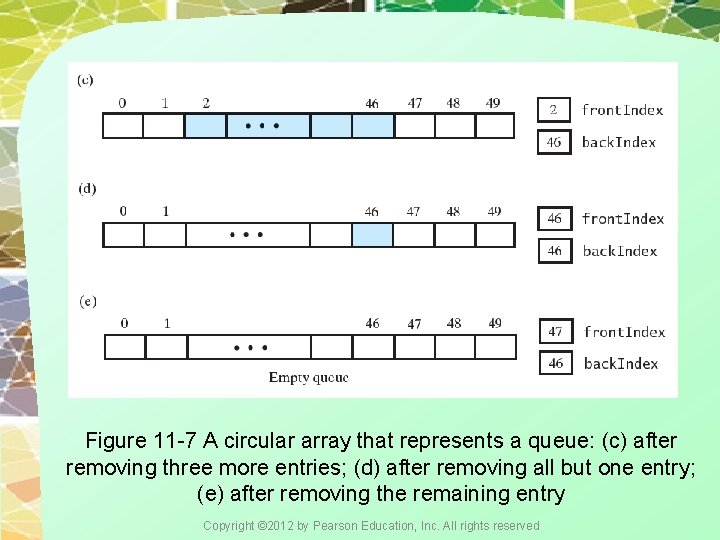
Figure 11 -7 A circular array that represents a queue: (c) after removing three more entries; (d) after removing all but one entry; (e) after removing the remaining entry Copyright © 2012 by Pearson Education, Inc. All rights reserved
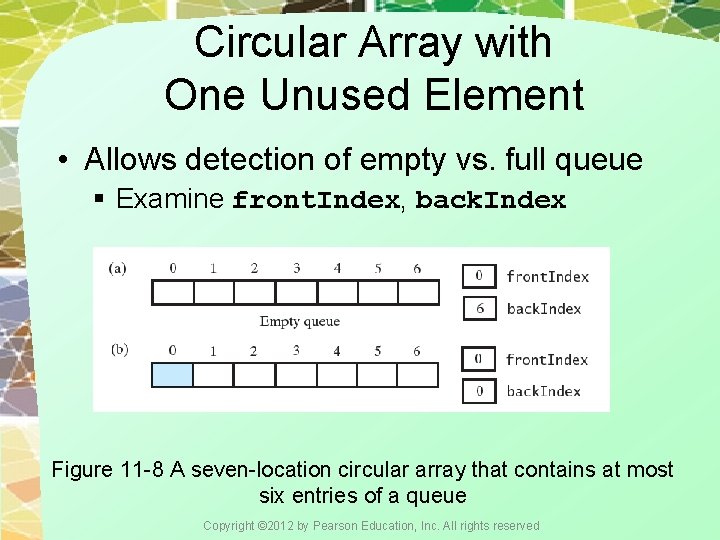
Circular Array with One Unused Element • Allows detection of empty vs. full queue § Examine front. Index, back. Index Figure 11 -8 A seven-location circular array that contains at most six entries of a queue Copyright © 2012 by Pearson Education, Inc. All rights reserved
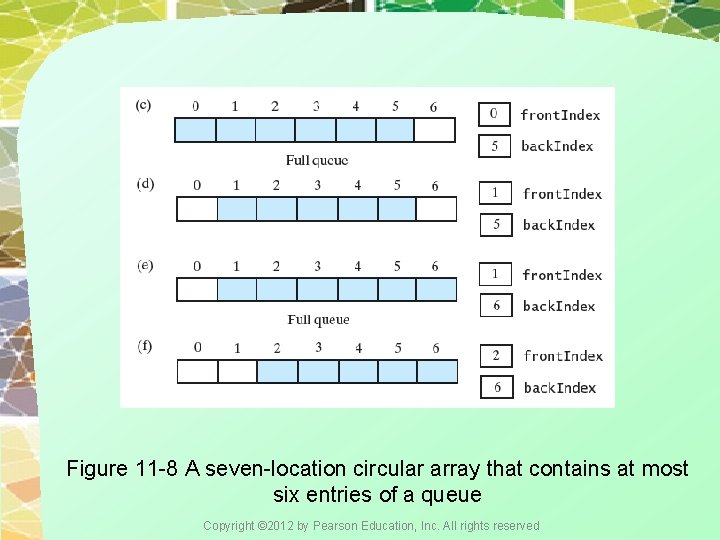
Figure 11 -8 A seven-location circular array that contains at most six entries of a queue Copyright © 2012 by Pearson Education, Inc. All rights reserved
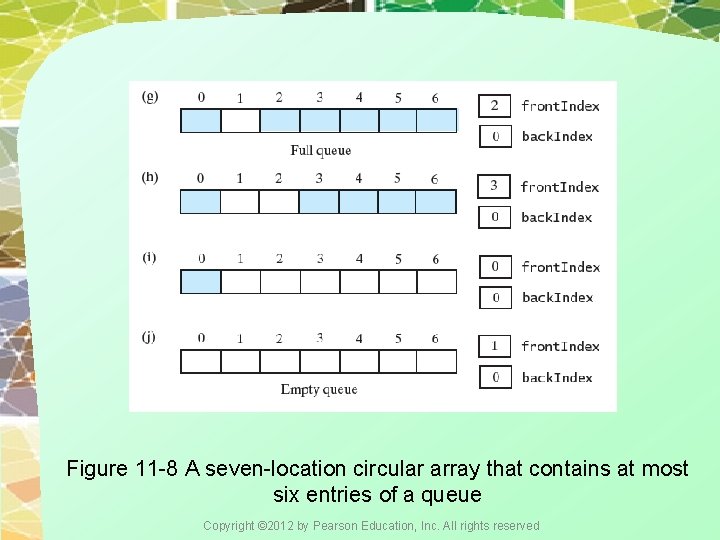
Figure 11 -8 A seven-location circular array that contains at most six entries of a queue Copyright © 2012 by Pearson Education, Inc. All rights reserved
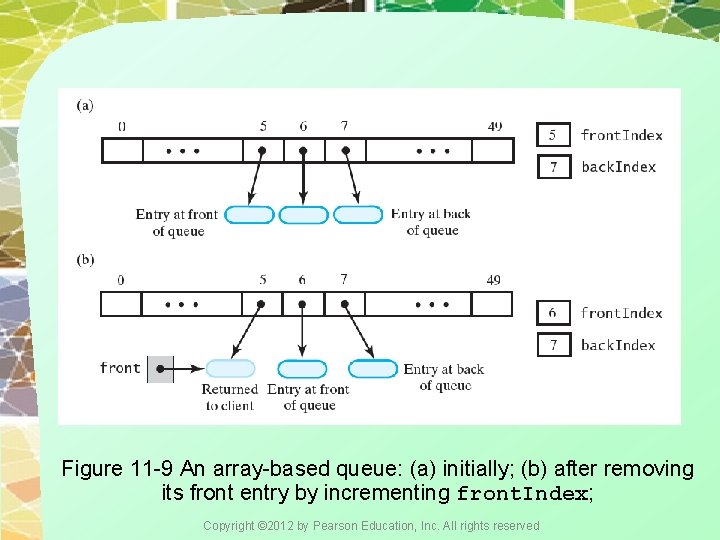
Figure 11 -9 An array-based queue: (a) initially; (b) after removing its front entry by incrementing front. Index; Copyright © 2012 by Pearson Education, Inc. All rights reserved
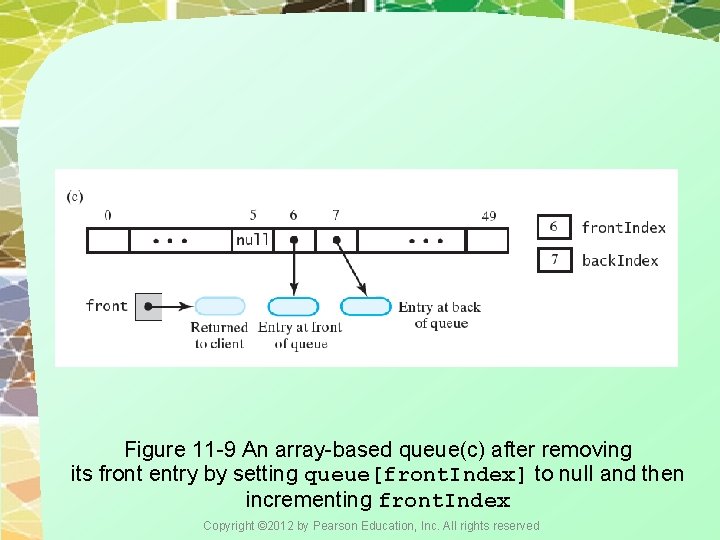
Figure 11 -9 An array-based queue(c) after removing its front entry by setting queue[front. Index] to null and then incrementing front. Index Copyright © 2012 by Pearson Education, Inc. All rights reserved
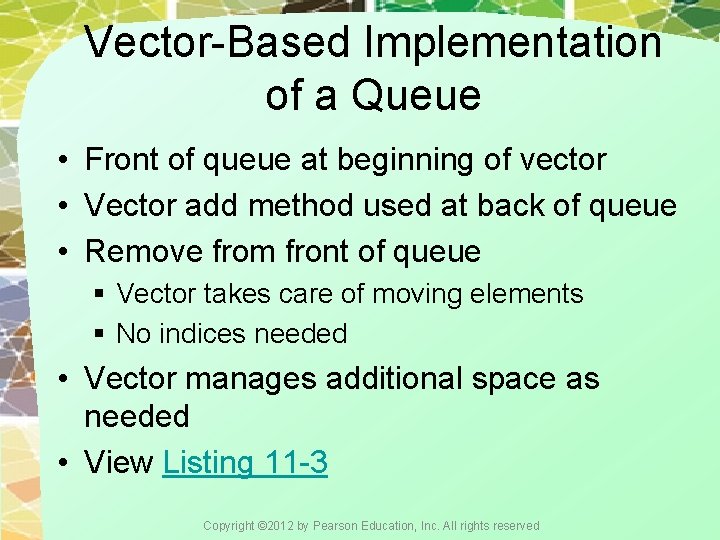
Vector-Based Implementation of a Queue • Front of queue at beginning of vector • Vector add method used at back of queue • Remove from front of queue § Vector takes care of moving elements § No indices needed • Vector manages additional space as needed • View Listing 11 -3 Copyright © 2012 by Pearson Education, Inc. All rights reserved
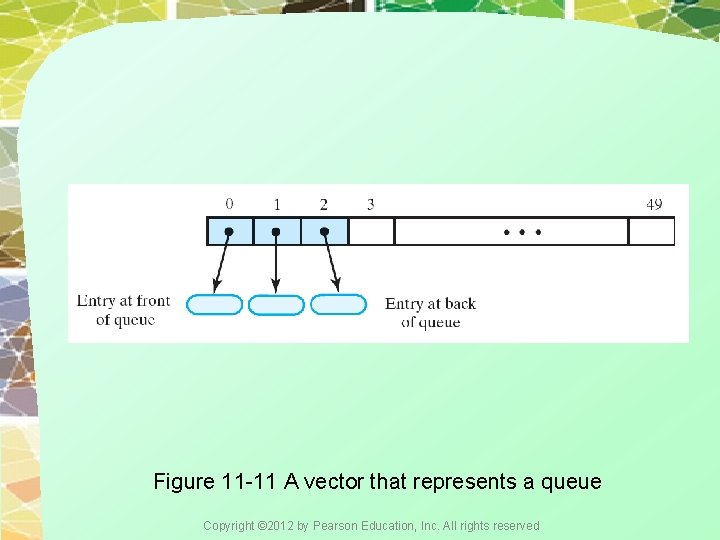
Figure 11 -11 A vector that represents a queue Copyright © 2012 by Pearson Education, Inc. All rights reserved
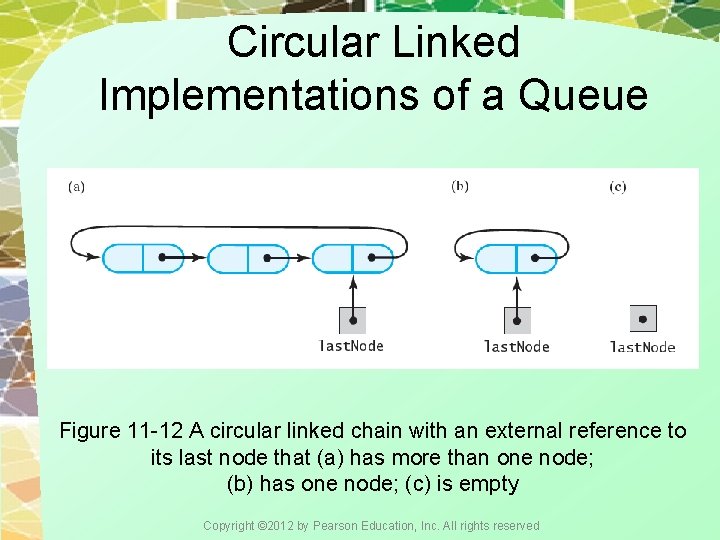
Circular Linked Implementations of a Queue Figure 11 -12 A circular linked chain with an external reference to its last node that (a) has more than one node; (b) has one node; (c) is empty Copyright © 2012 by Pearson Education, Inc. All rights reserved
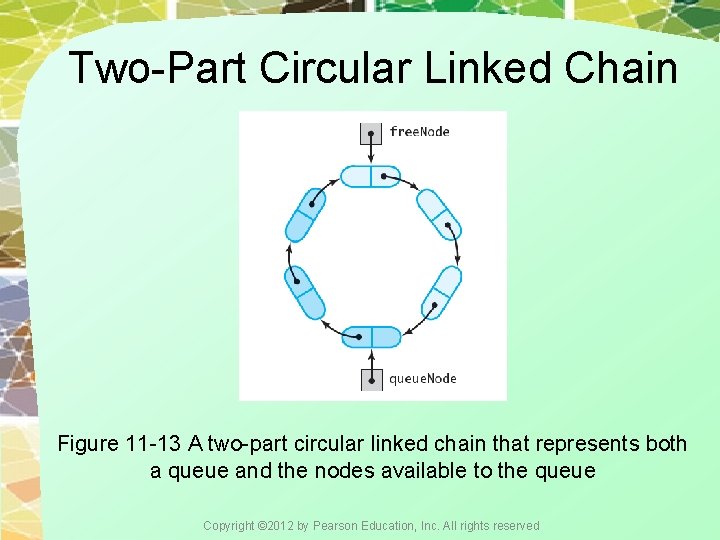
Two-Part Circular Linked Chain Figure 11 -13 A two-part circular linked chain that represents both a queue and the nodes available to the queue Copyright © 2012 by Pearson Education, Inc. All rights reserved
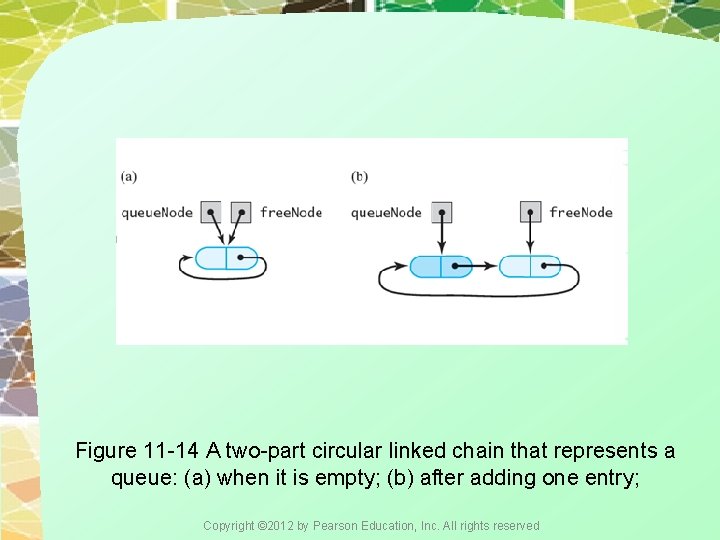
Figure 11 -14 A two-part circular linked chain that represents a queue: (a) when it is empty; (b) after adding one entry; Copyright © 2012 by Pearson Education, Inc. All rights reserved
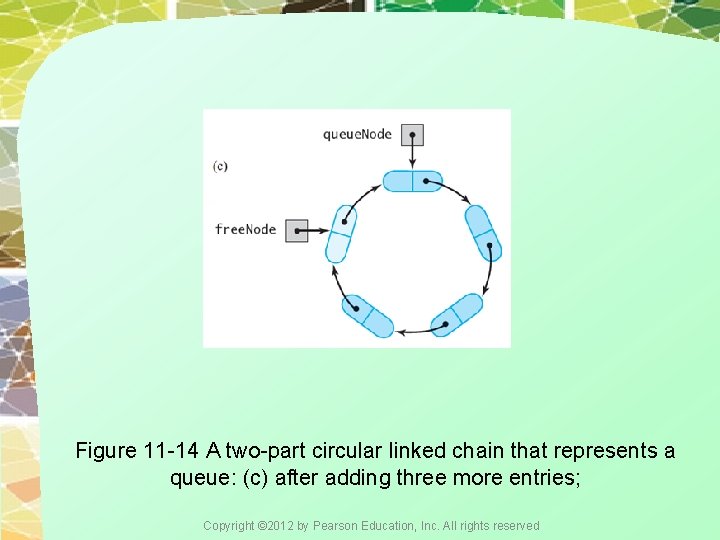
Figure 11 -14 A two-part circular linked chain that represents a queue: (c) after adding three more entries; Copyright © 2012 by Pearson Education, Inc. All rights reserved
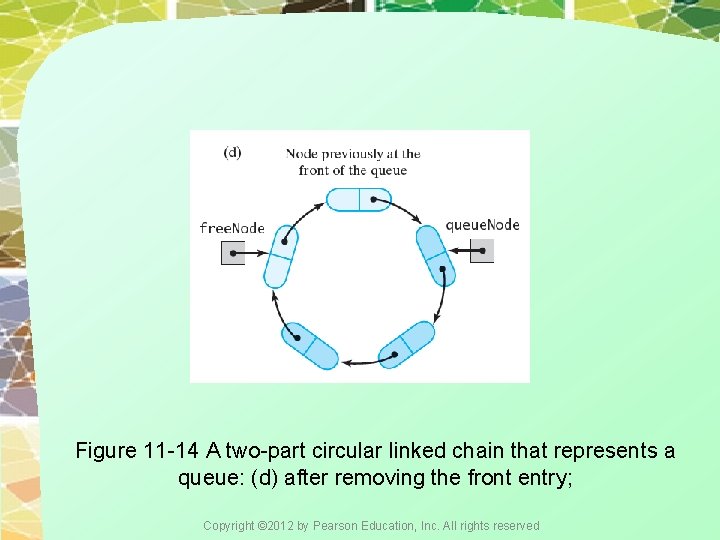
Figure 11 -14 A two-part circular linked chain that represents a queue: (d) after removing the front entry; Copyright © 2012 by Pearson Education, Inc. All rights reserved
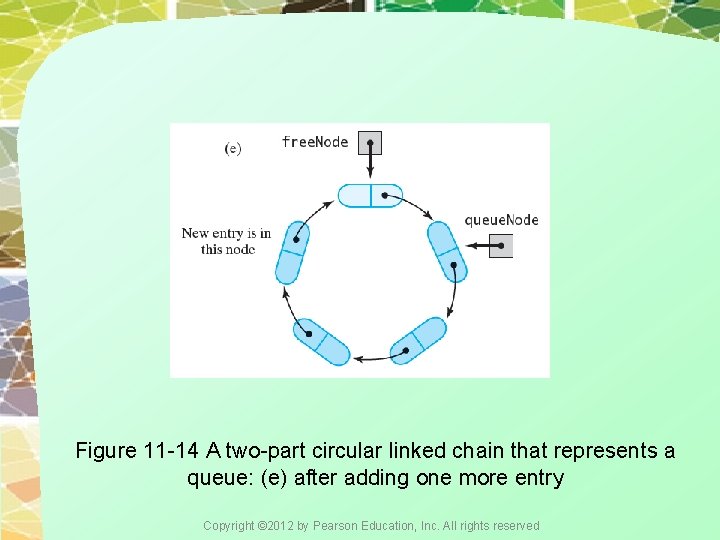
Figure 11 -14 A two-part circular linked chain that represents a queue: (e) after adding one more entry Copyright © 2012 by Pearson Education, Inc. All rights reserved
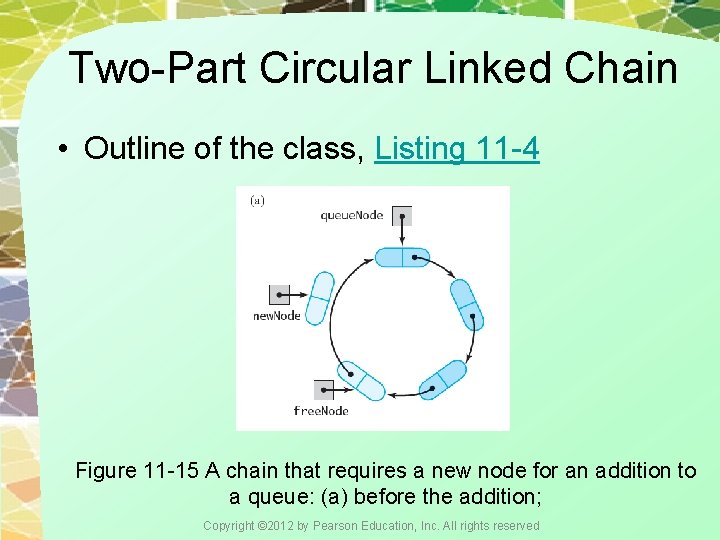
Two-Part Circular Linked Chain • Outline of the class, Listing 11 -4 Figure 11 -15 A chain that requires a new node for an addition to a queue: (a) before the addition; Copyright © 2012 by Pearson Education, Inc. All rights reserved
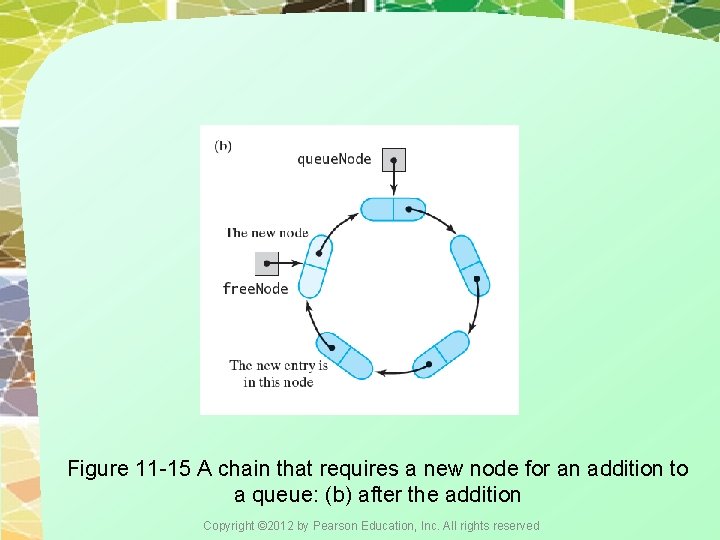
Figure 11 -15 A chain that requires a new node for an addition to a queue: (b) after the addition Copyright © 2012 by Pearson Education, Inc. All rights reserved
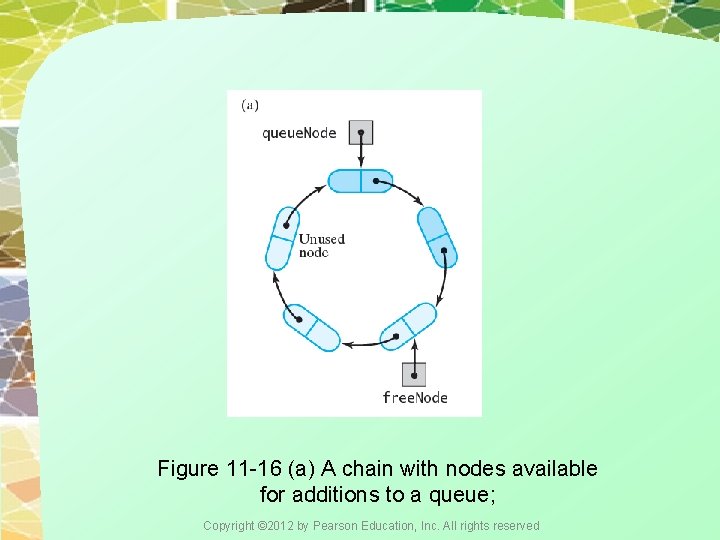
Figure 11 -16 (a) A chain with nodes available for additions to a queue; Copyright © 2012 by Pearson Education, Inc. All rights reserved
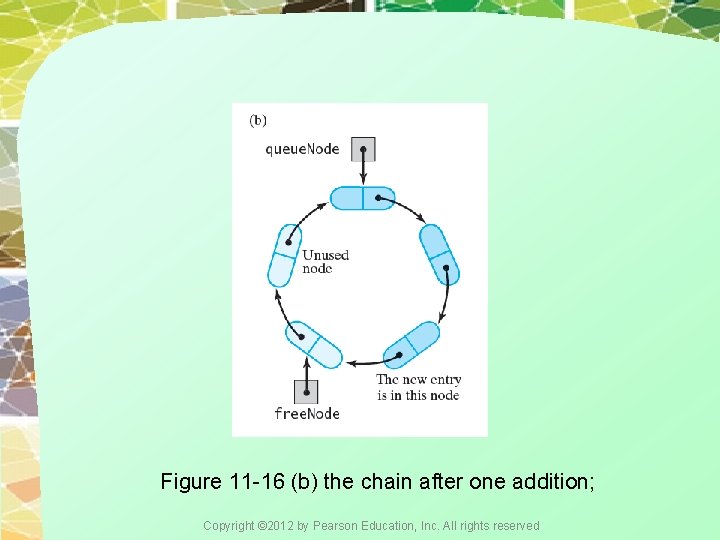
Figure 11 -16 (b) the chain after one addition; Copyright © 2012 by Pearson Education, Inc. All rights reserved
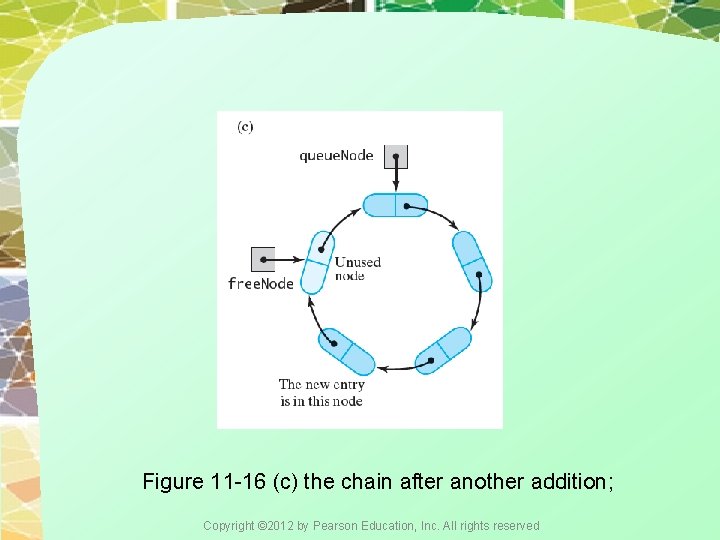
Figure 11 -16 (c) the chain after another addition; Copyright © 2012 by Pearson Education, Inc. All rights reserved
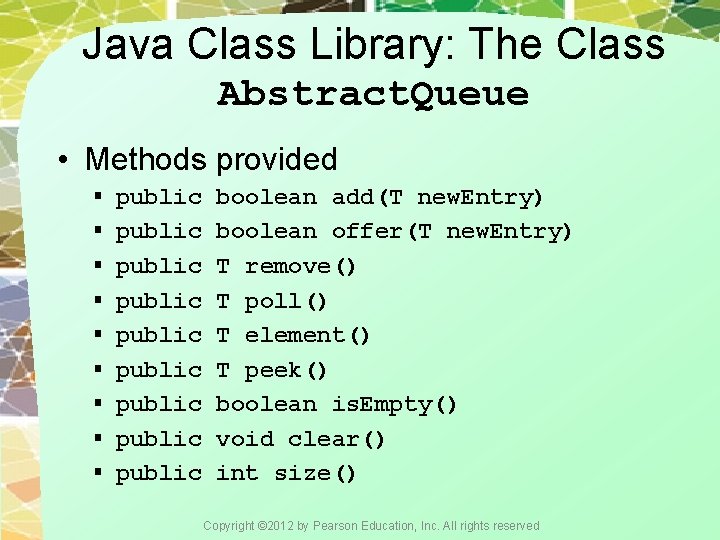
Java Class Library: The Class Abstract. Queue • Methods provided § public boolean add(T new. Entry) § public boolean offer(T new. Entry) § public T remove() § public T poll() § public T element() § public T peek() § public boolean is. Empty() § public void clear() § public int size() Copyright © 2012 by Pearson Education, Inc. All rights reserved
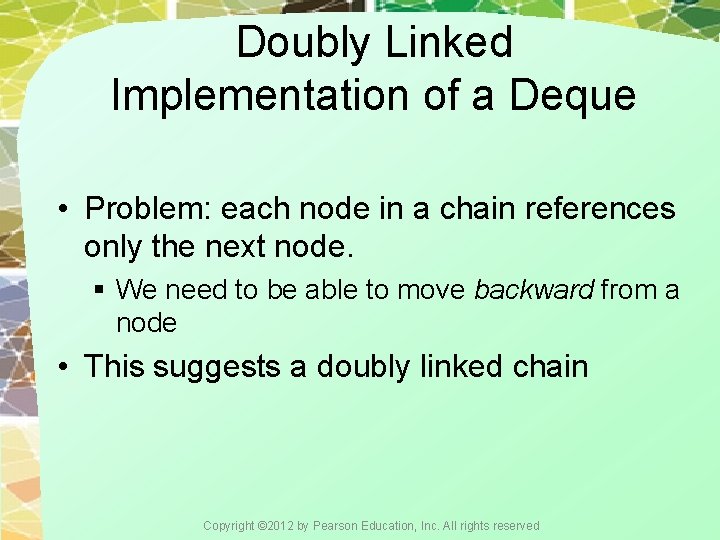
Doubly Linked Implementation of a Deque • Problem: each node in a chain references only the next node. § We need to be able to move backward from a node • This suggests a doubly linked chain Copyright © 2012 by Pearson Education, Inc. All rights reserved
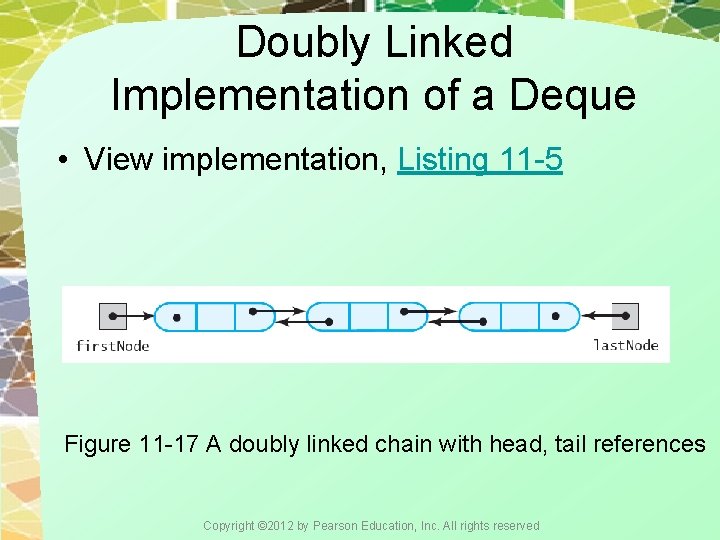
Doubly Linked Implementation of a Deque • View implementation, Listing 11 -5 Figure 11 -17 A doubly linked chain with head, tail references Copyright © 2012 by Pearson Education, Inc. All rights reserved
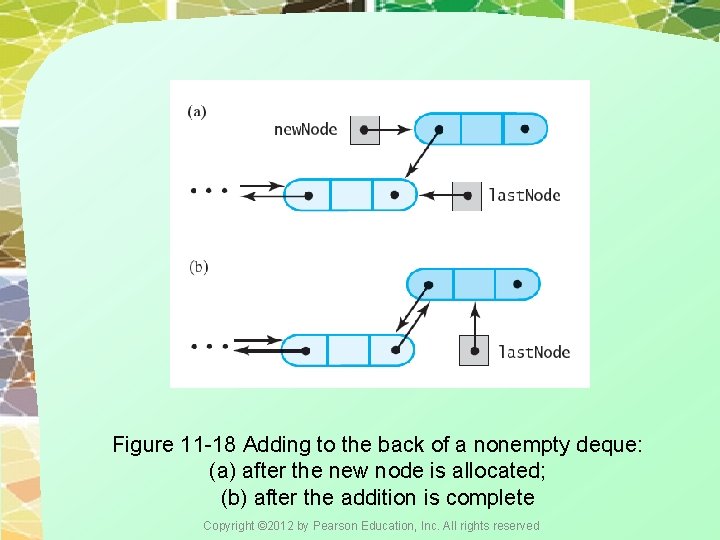
Figure 11 -18 Adding to the back of a nonempty deque: (a) after the new node is allocated; (b) after the addition is complete Copyright © 2012 by Pearson Education, Inc. All rights reserved
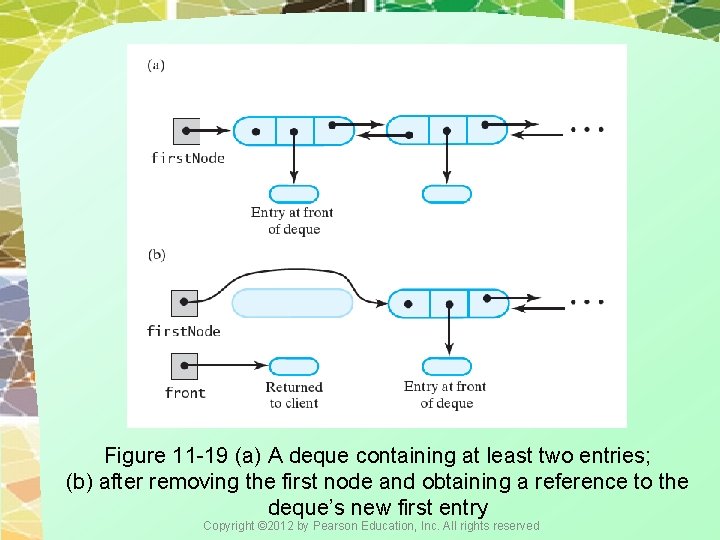
Figure 11 -19 (a) A deque containing at least two entries; (b) after removing the first node and obtaining a reference to the deque’s new first entry Copyright © 2012 by Pearson Education, Inc. All rights reserved
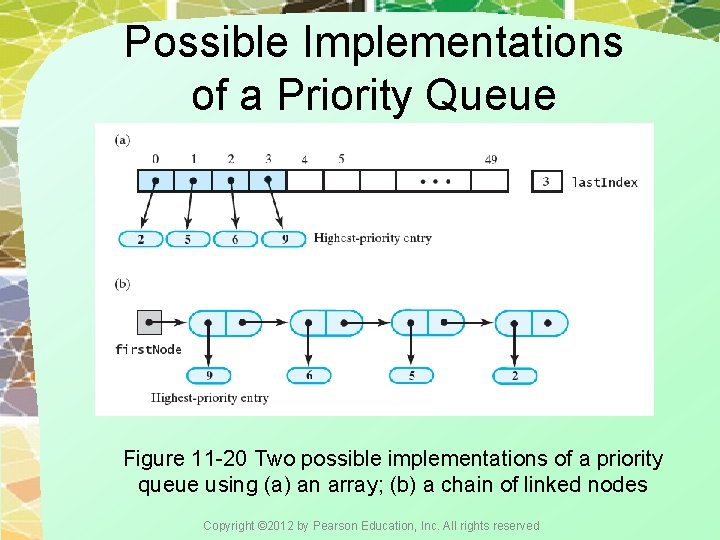
Possible Implementations of a Priority Queue Figure 11 -20 Two possible implementations of a priority queue using (a) an array; (b) a chain of linked nodes Copyright © 2012 by Pearson Education, Inc. All rights reserved
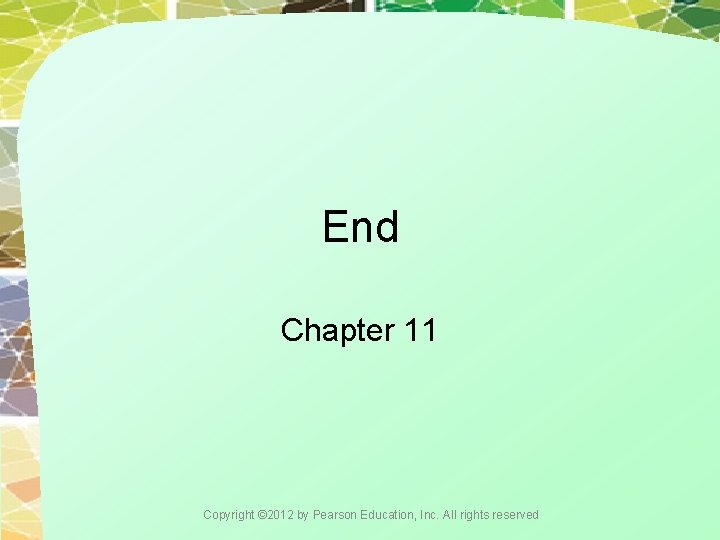
End Chapter 11 Copyright © 2012 by Pearson Education, Inc. All rights reserved
Deque and priority queue
Queue means
Burman's priority list gives priority to
Priority mail vs priority mail express
Deque salman
Deque salman
Arraydeque vs stack
Deque falls
Min-priority queue
Priority queue abstract data type
Bottom up heap construction example
Priority queue using heap
Double-ended priority queue
Priority queue doubly linked list
Praveen
Priority queue order
Adaptable priority queue java
Adaptable priority queue
Priority queue lower bound
Transform and conquer algorithm
Contoh priority queue
Java priority queue example
Priority queue animation
Small talk programming language
Common standard ethernet implementations
With erp implementations why would an auditorget involved
Chapter 3 queue
Use case priority matrix for system
Use case priority matrix for system
Use case ranking and priority matrix
Use case ranking and priority matrix
Use case ranking and priority matrix images
Use case ranking and priority matrix images
Priority of sendai framework
Priority inheritance
Stakeholder priority matrix
Sendai framework guiding principles
Verilog operator precedence
Stakeholder priority matrix
Priority improvement areas template
Prospect priority index
Priority encoder
Priority decoder
Priority care 365