Data Structures and Algorithms Data Structure Lecture Priority
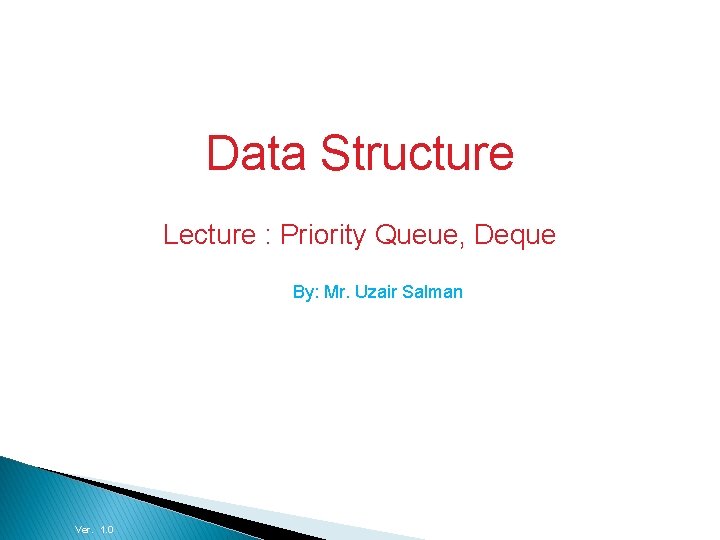
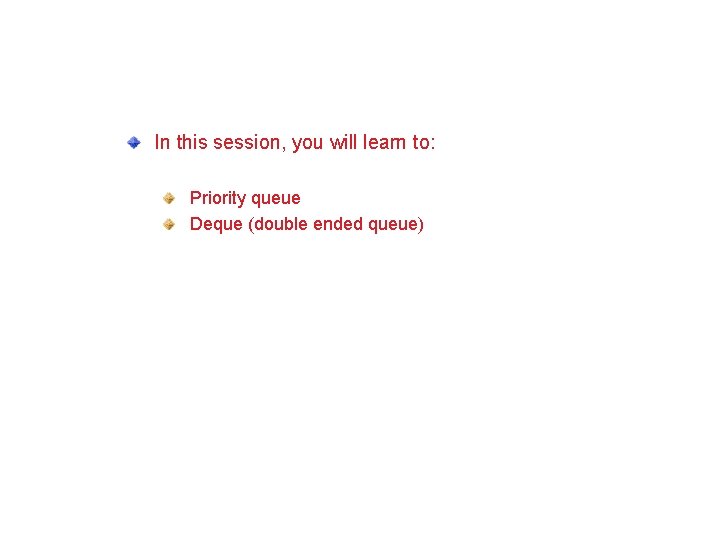
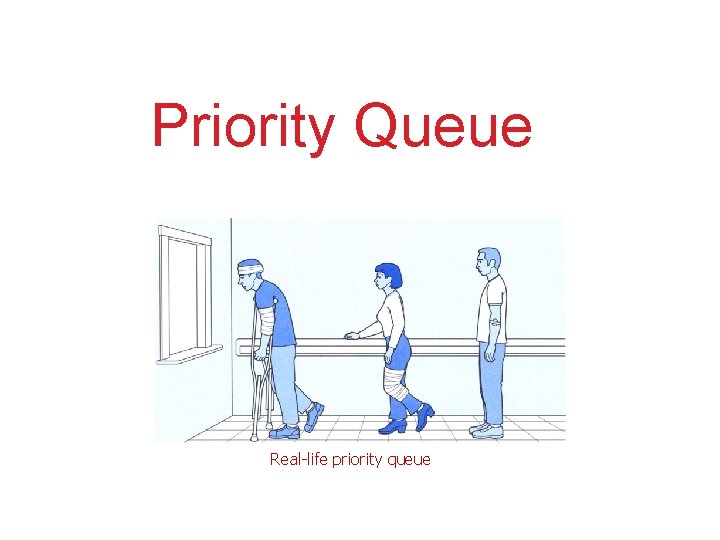
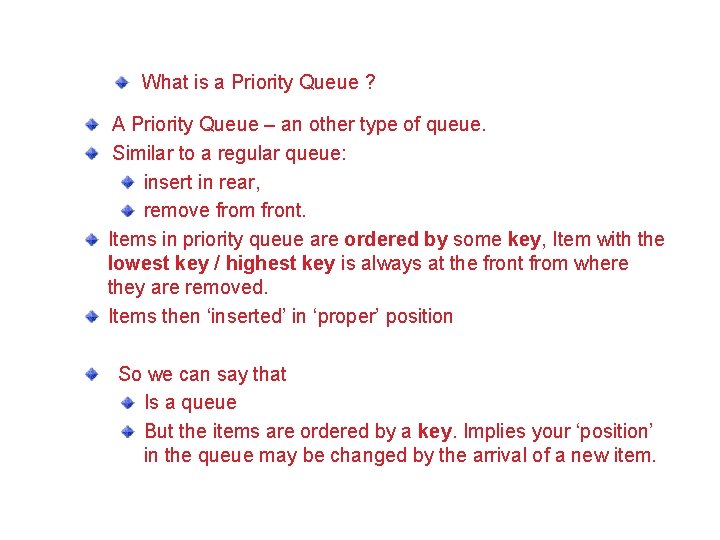
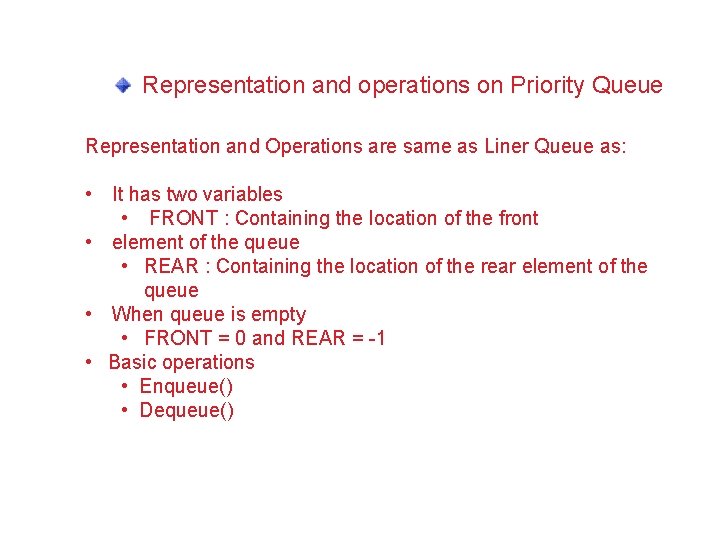
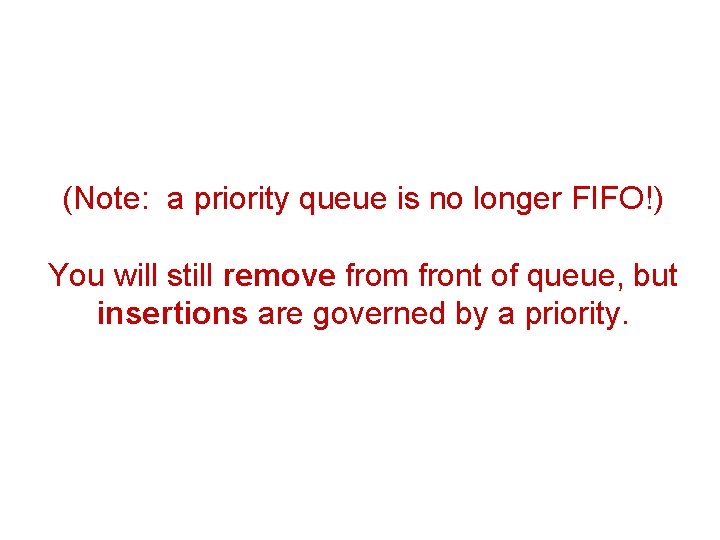
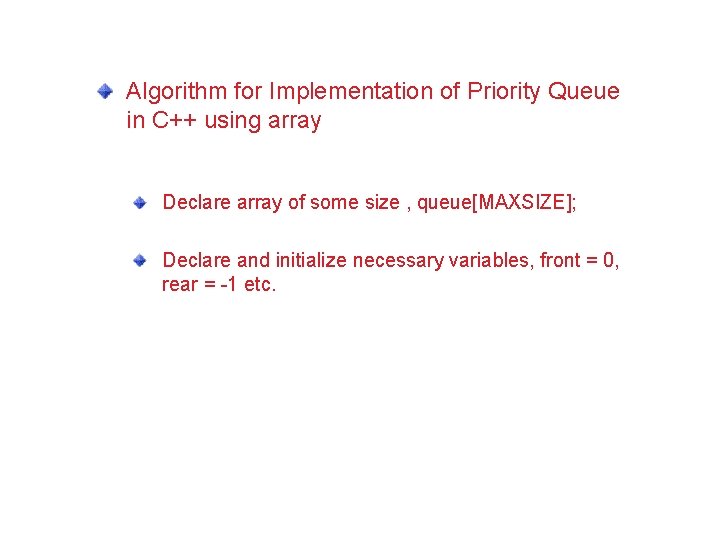
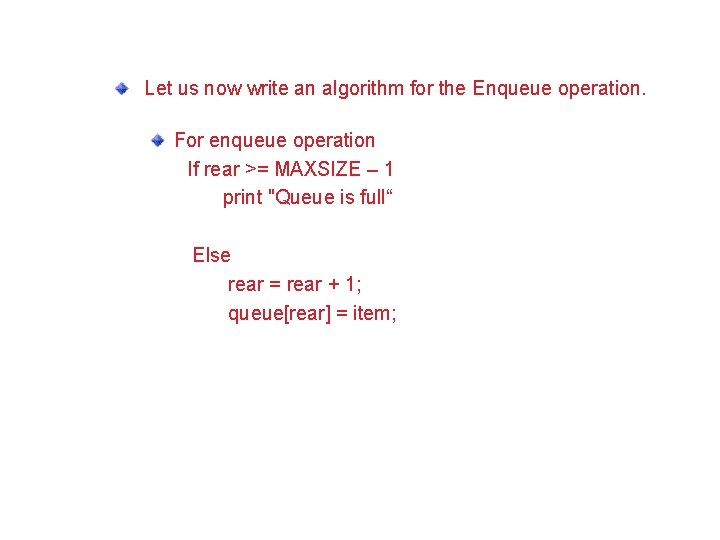
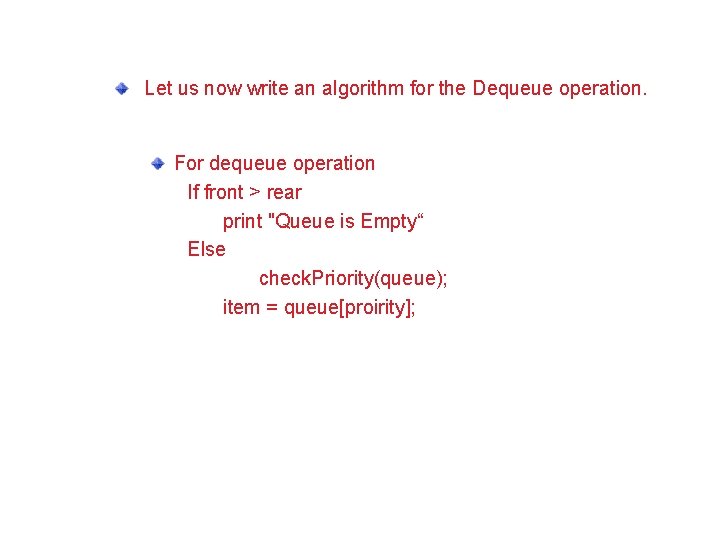
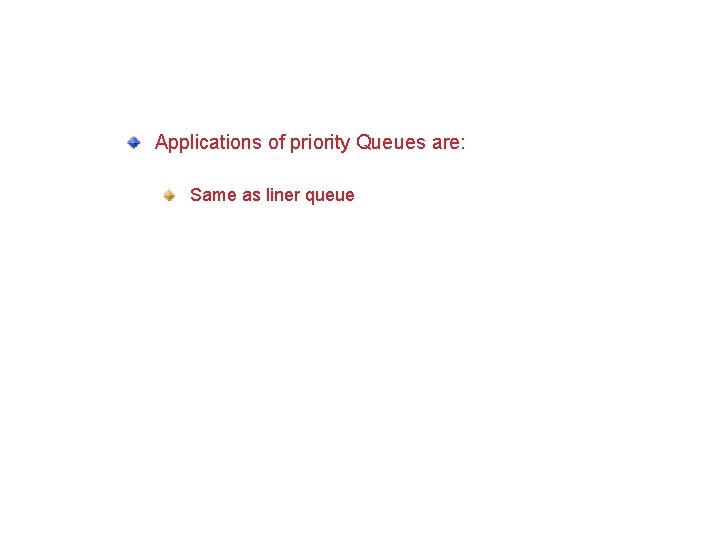
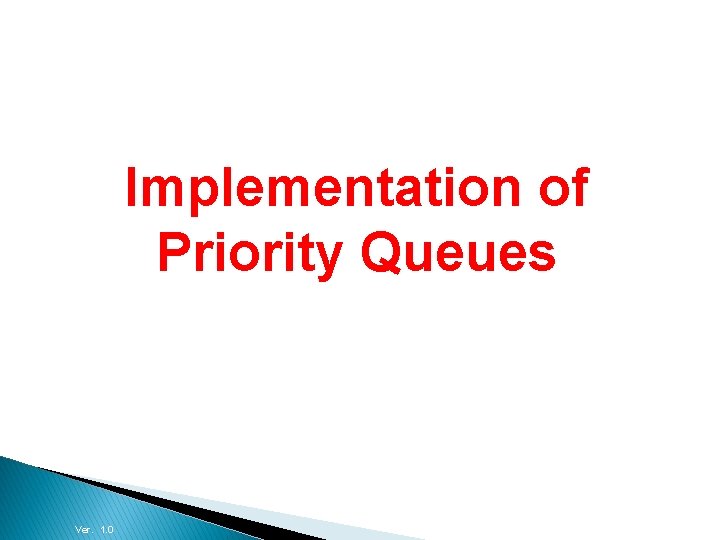
![Class queue { private: int size; int queue[size]; int front; int rear; public: queue() Class queue { private: int size; int queue[size]; int front; int rear; public: queue()](https://slidetodoc.com/presentation_image_h/02fe0b78017179d82cf1a2c51f1f726b/image-12.jpg)
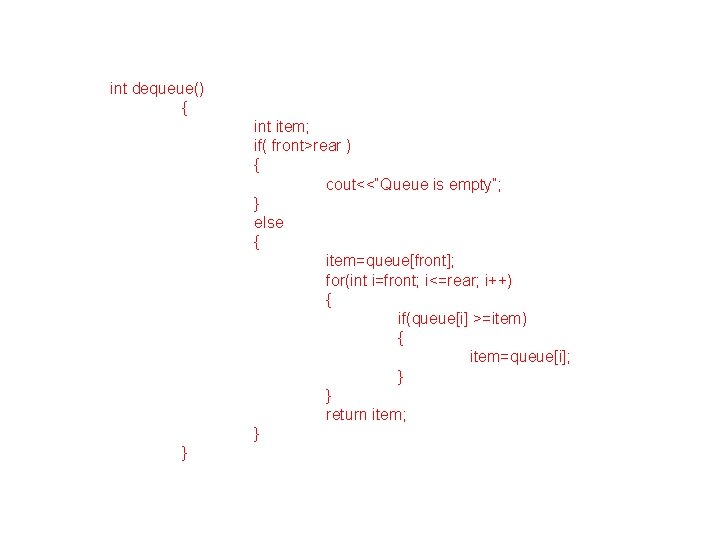
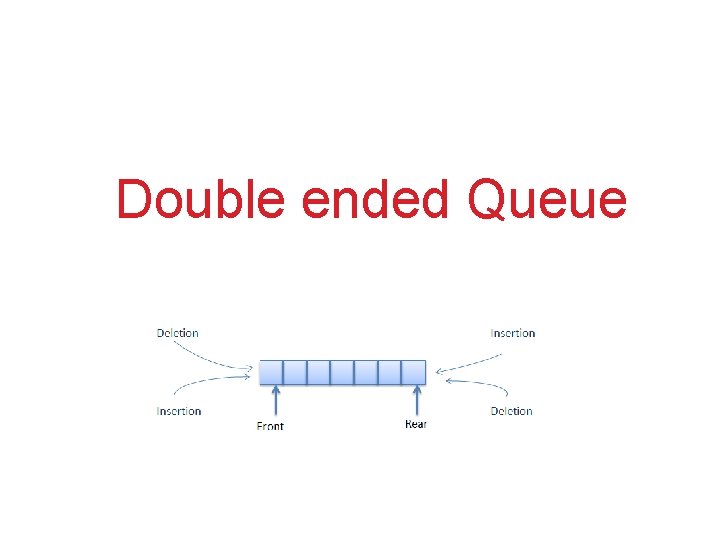
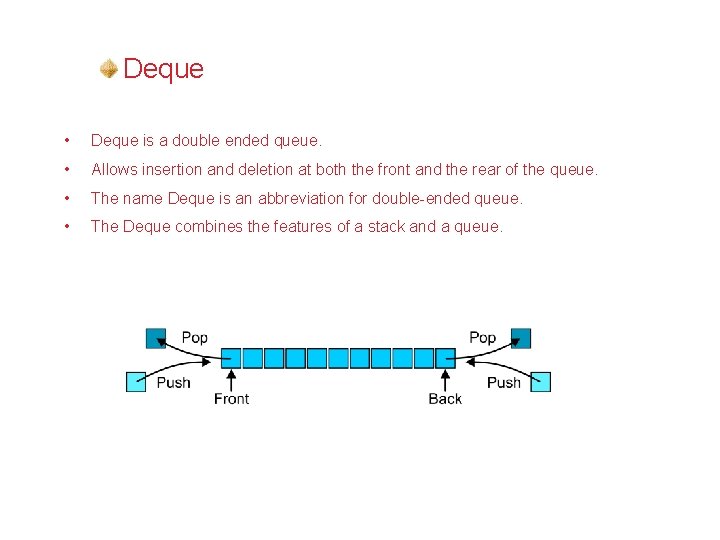
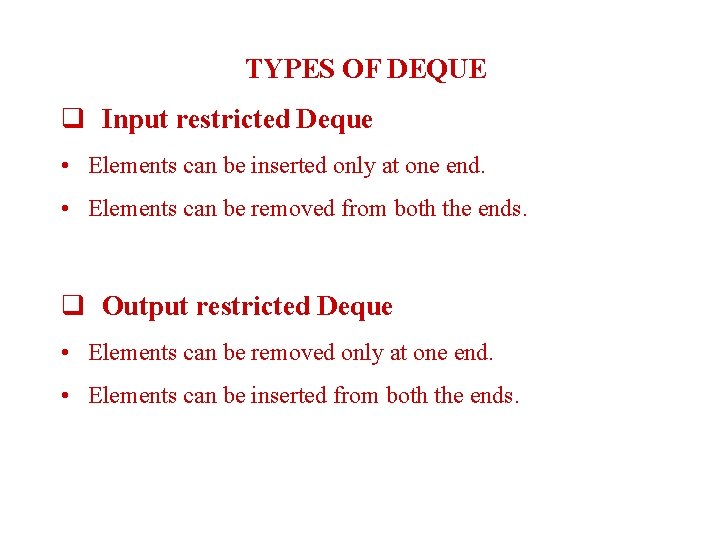
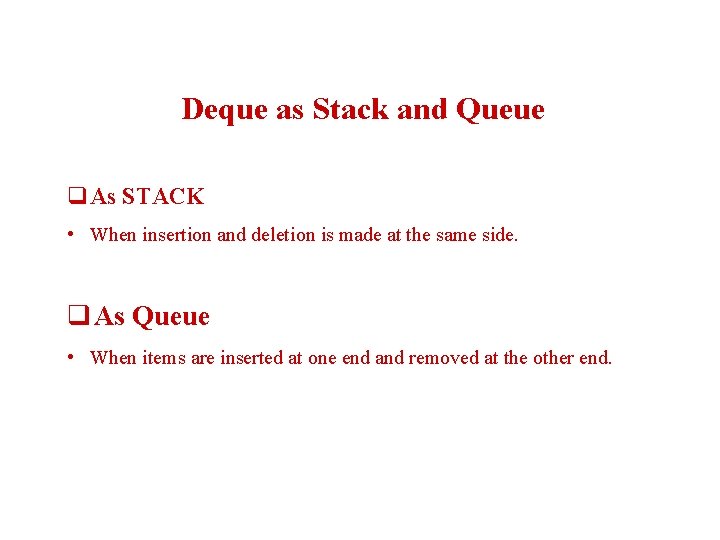
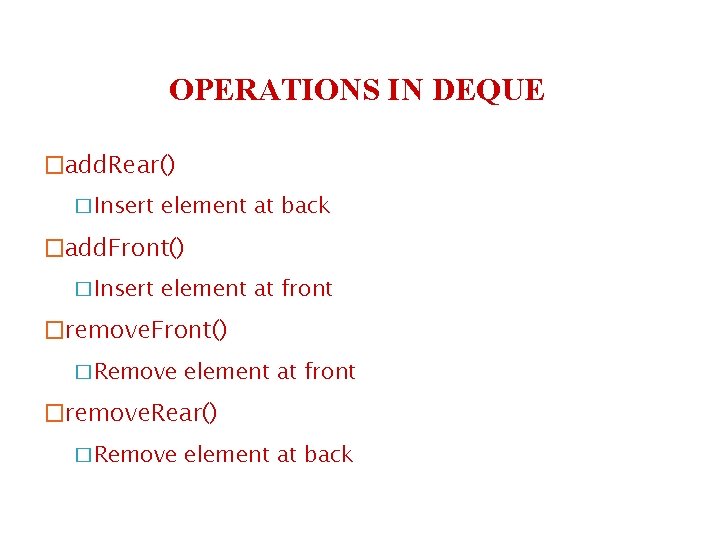
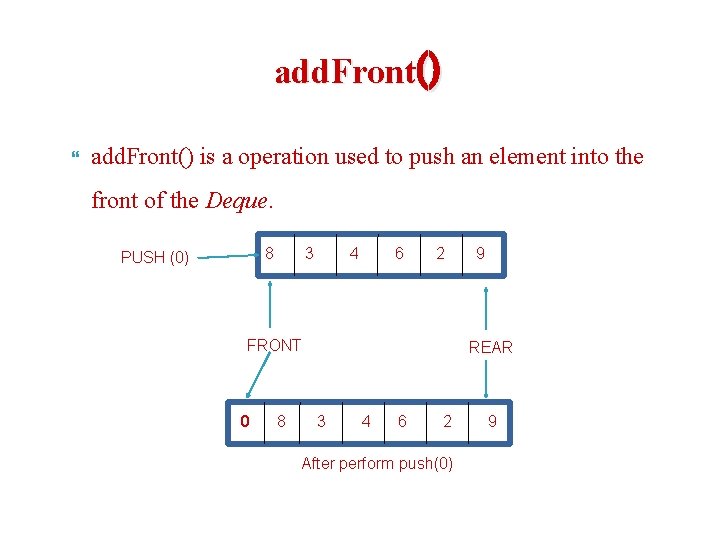
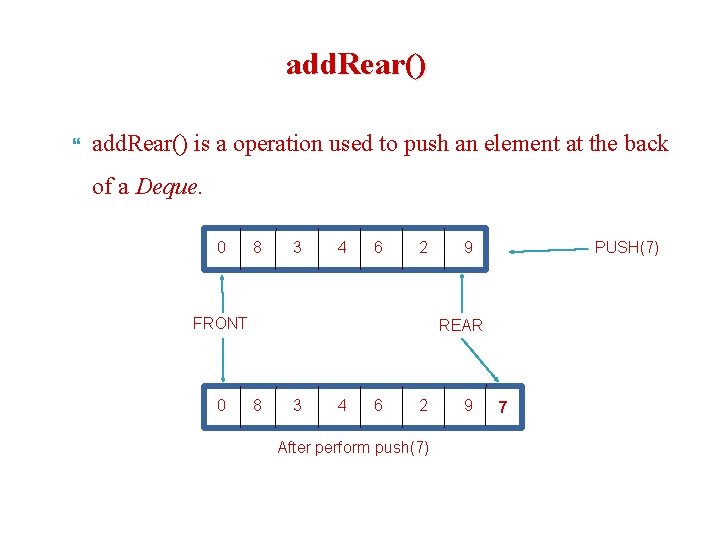
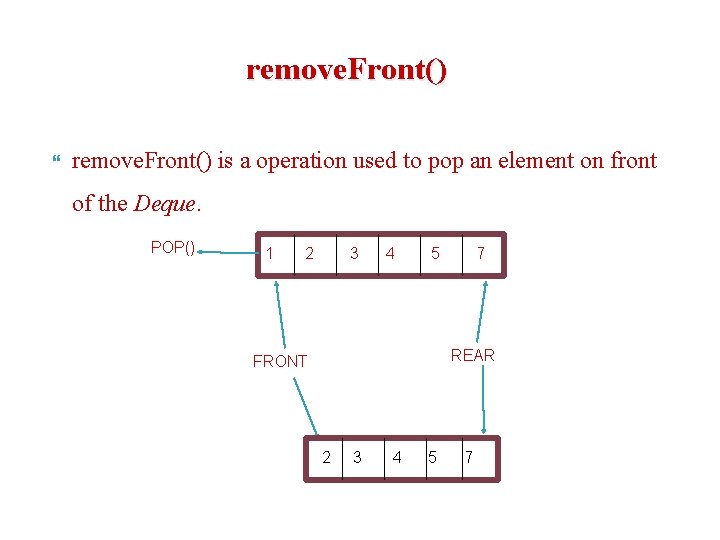
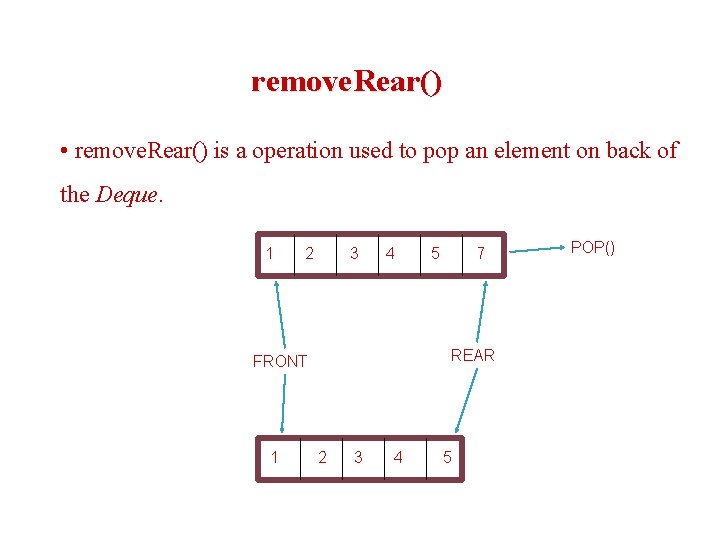
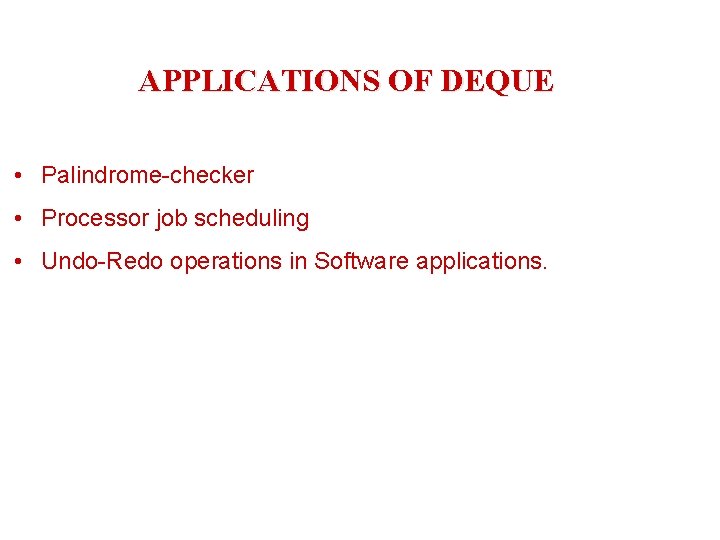
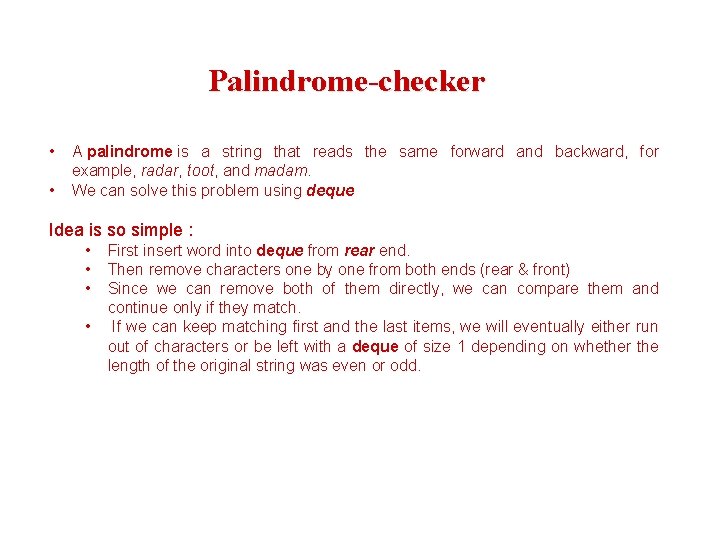
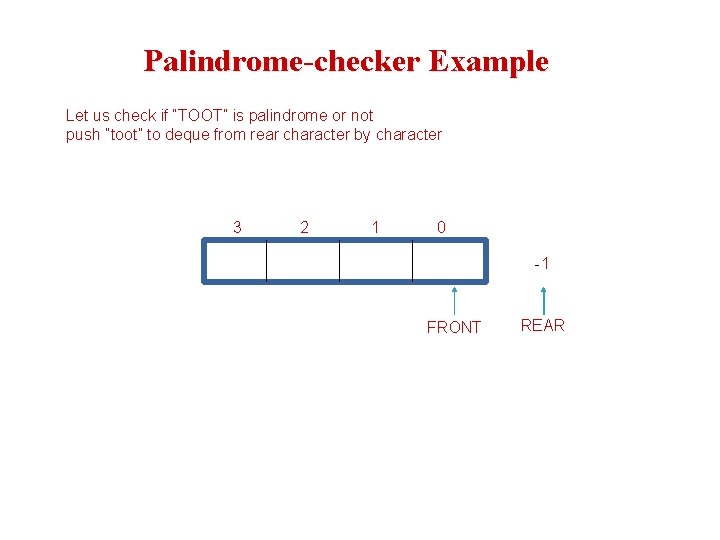
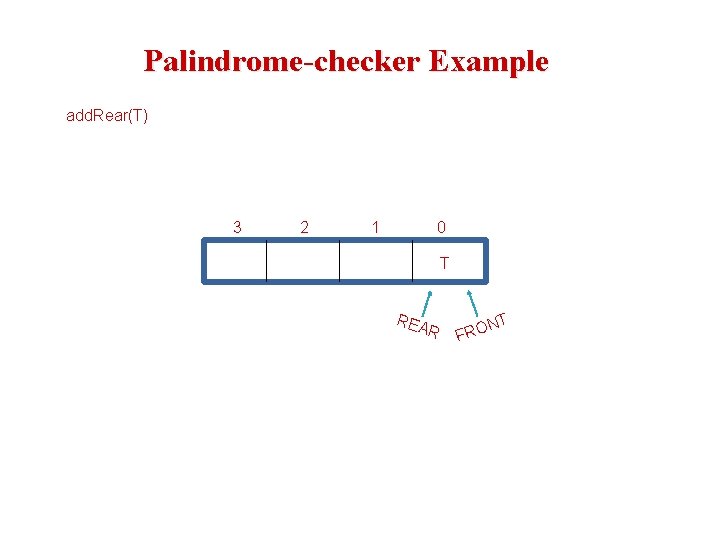
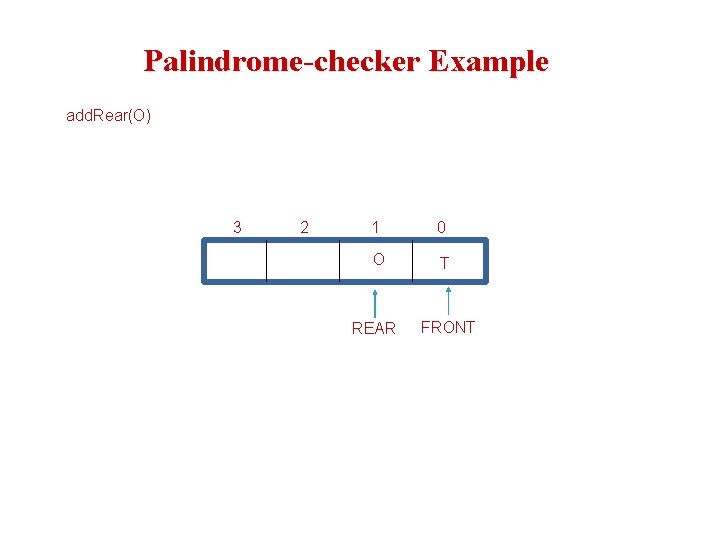
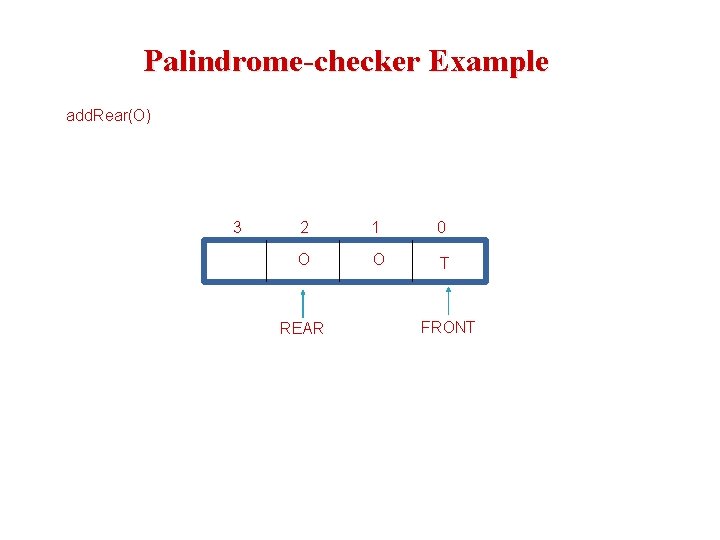
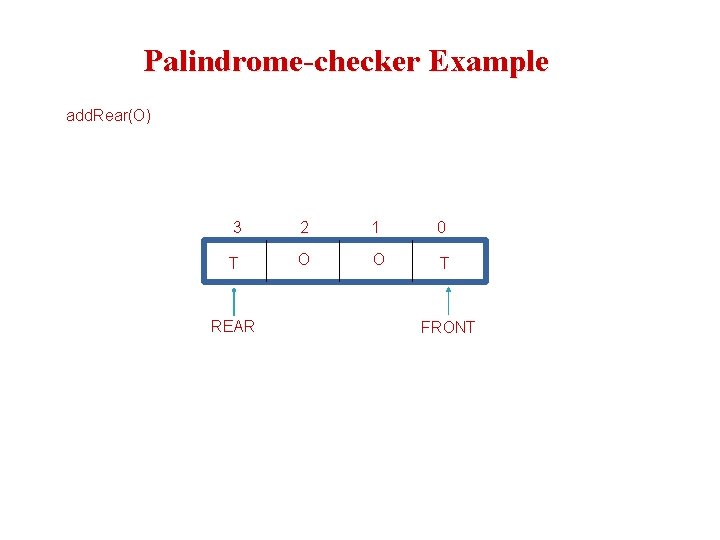
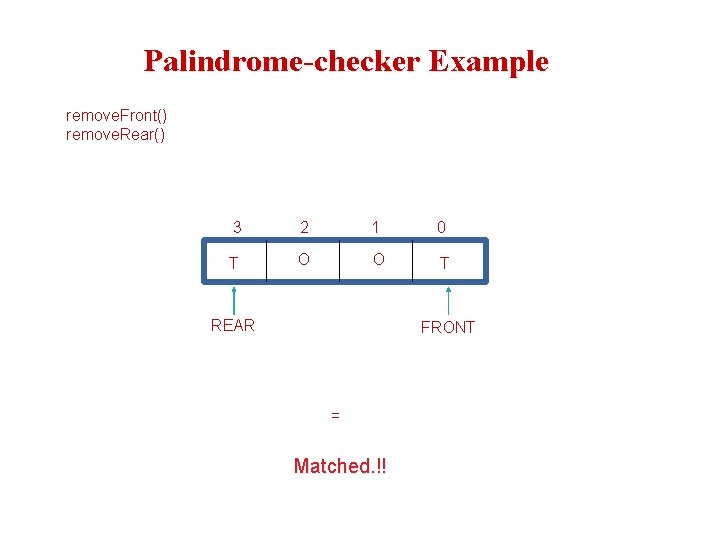
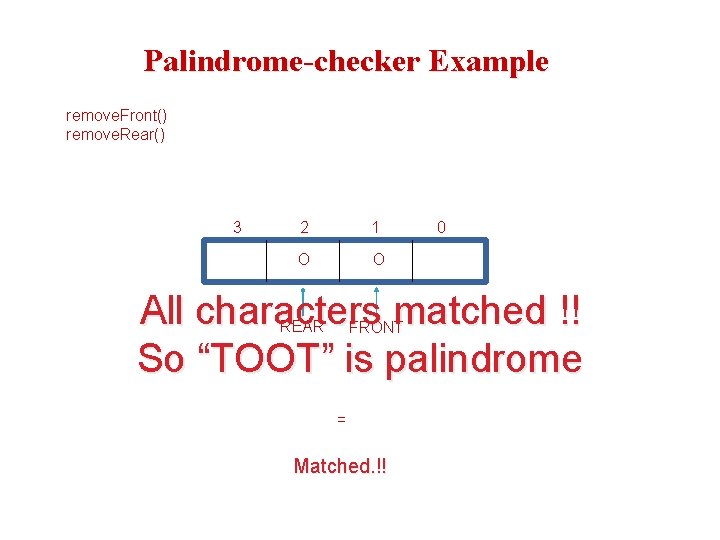
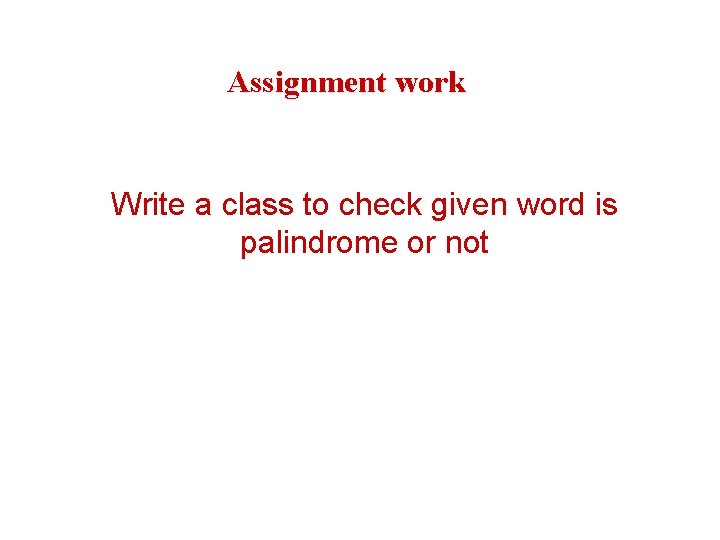
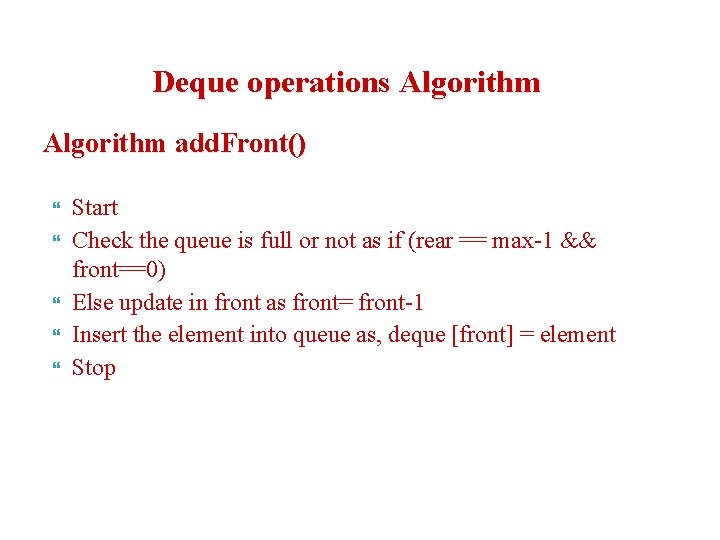
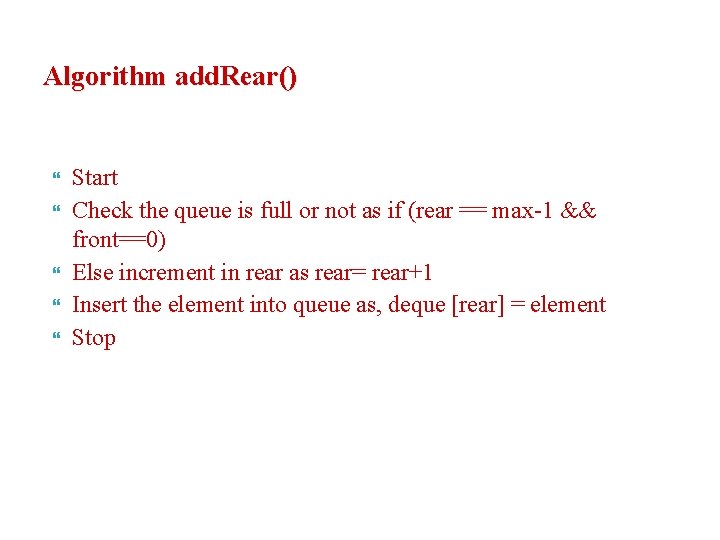
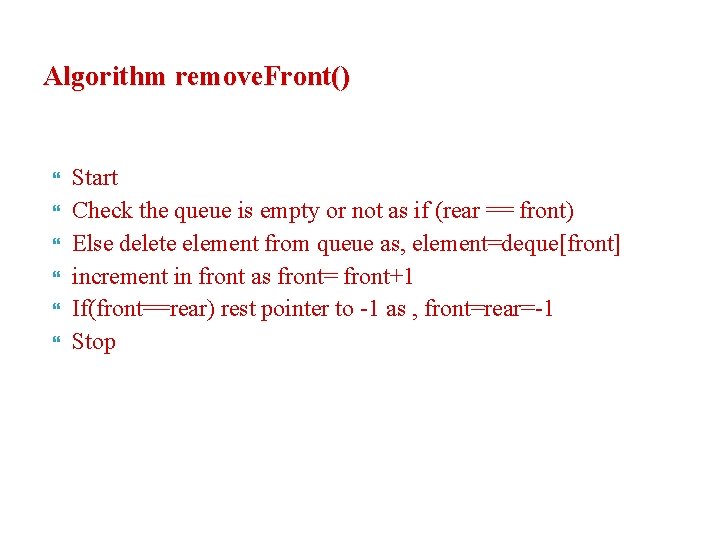
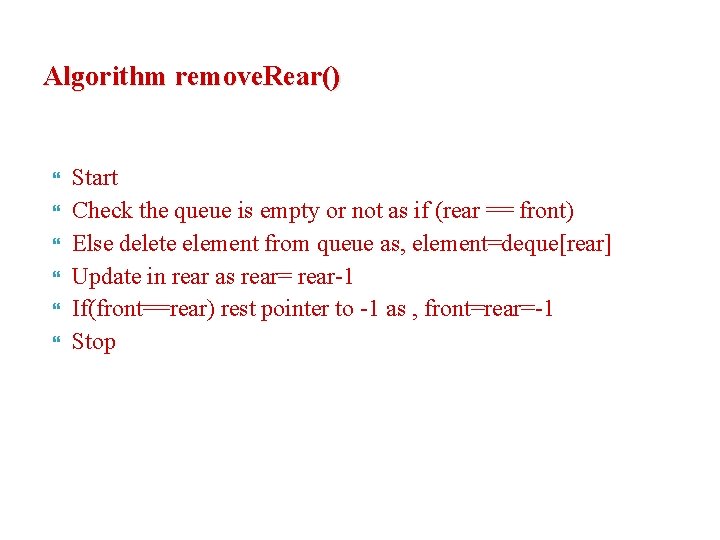
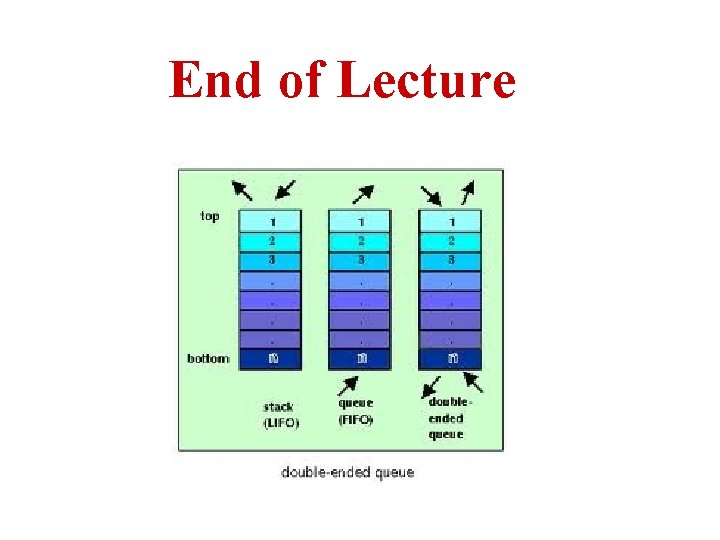
- Slides: 37
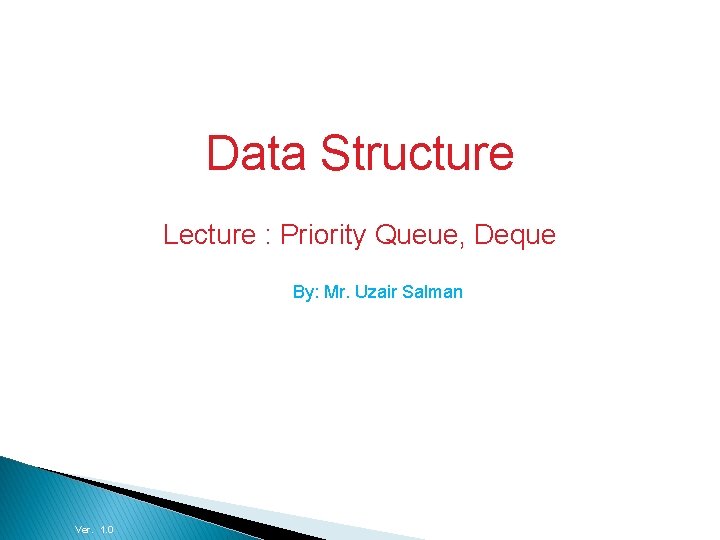
Data Structures and Algorithms Data Structure Lecture : Priority Queue, Deque By: Mr. Uzair Salman Ver. 1. 0 Session 10
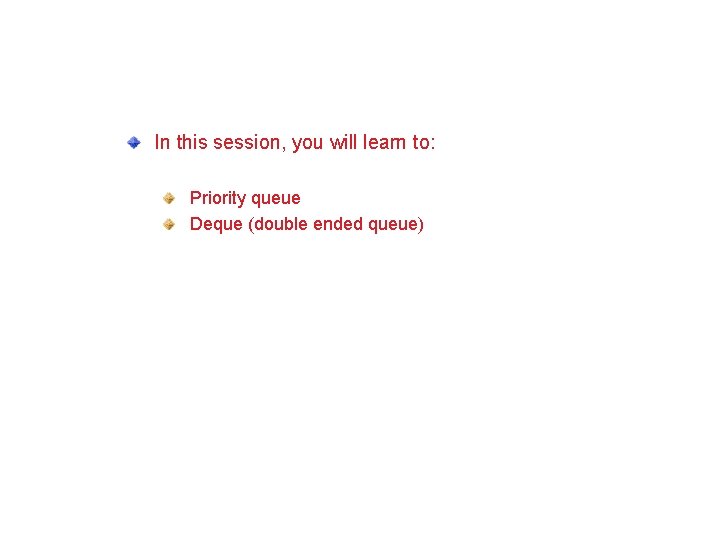
Objectives In this session, you will learn to: Priority queue Deque (double ended queue)
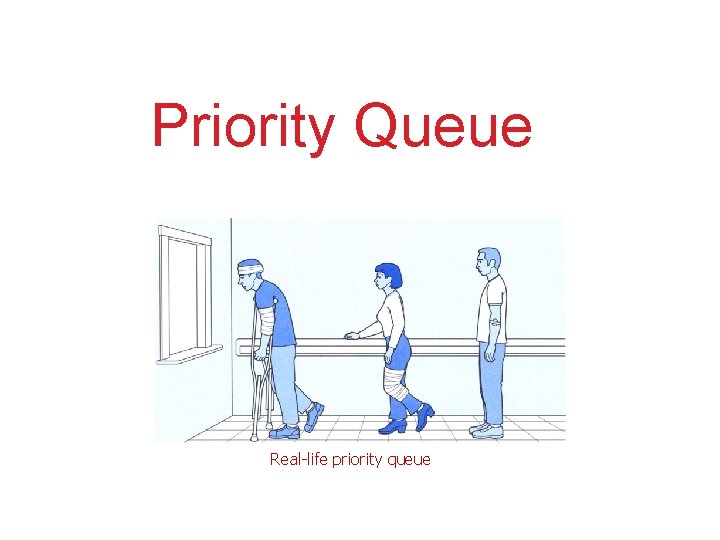
Priority Queue Real-life priority queue
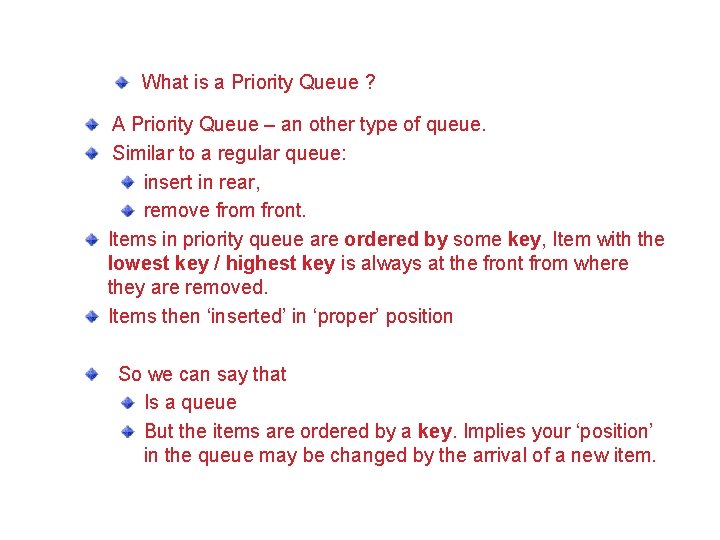
What is a Priority Queue ? A Priority Queue – an other type of queue. Similar to a regular queue: insert in rear, remove from front. Items in priority queue are ordered by some key, Item with the lowest key / highest key is always at the front from where they are removed. Items then ‘inserted’ in ‘proper’ position So we can say that Is a queue But the items are ordered by a key. Implies your ‘position’ in the queue may be changed by the arrival of a new item.
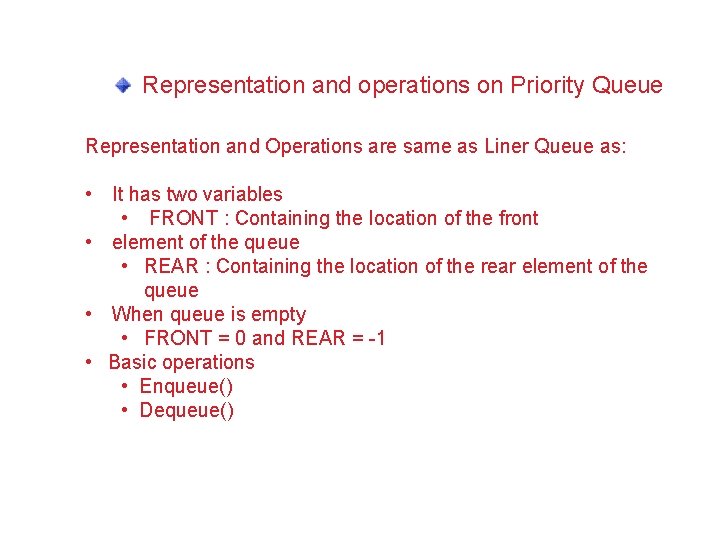
Representation and operations on Priority Queue Representation and Operations are same as Liner Queue as: • It has two variables • FRONT : Containing the location of the front • element of the queue • REAR : Containing the location of the rear element of the queue • When queue is empty • FRONT = 0 and REAR = -1 • Basic operations • Enqueue() • Dequeue()
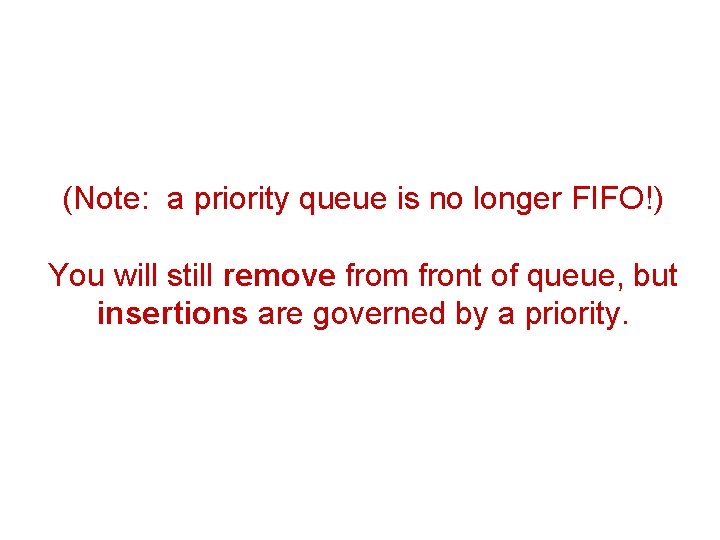
(Note: a priority queue is no longer FIFO!) You will still remove from front of queue, but insertions are governed by a priority.
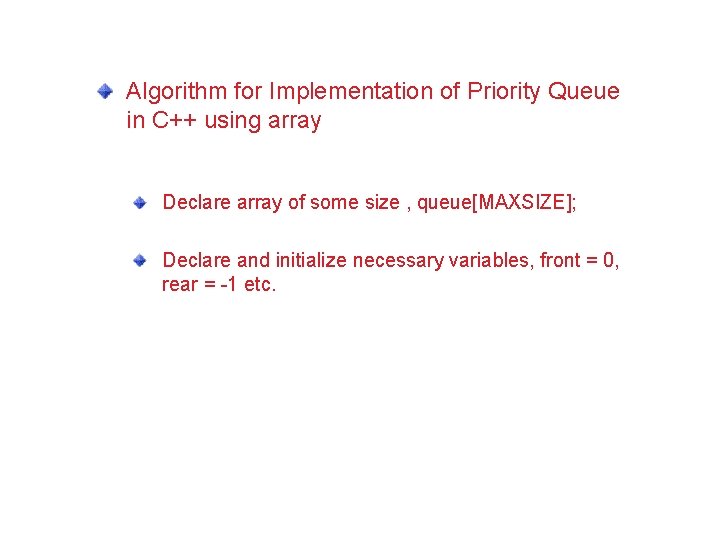
Algorithm for Implementation of Priority Queue in C++ using array Declare array of some size , queue[MAXSIZE]; Declare and initialize necessary variables, front = 0, rear = -1 etc.
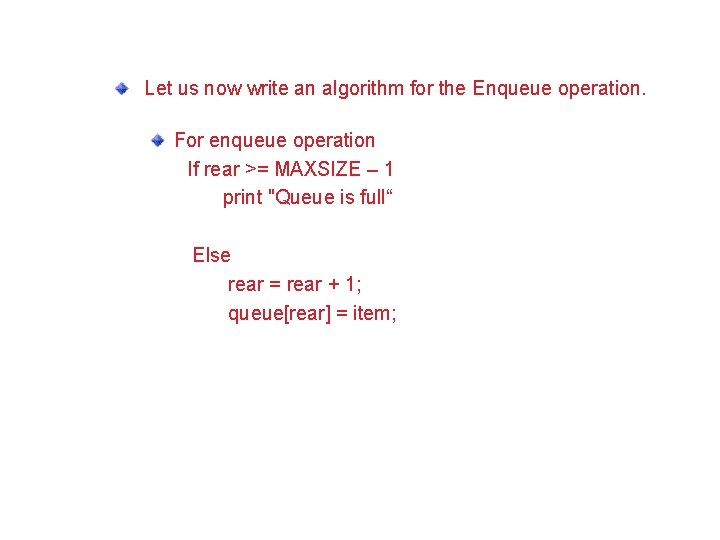
Implementing a Stack Using an Array (Contd. ) Let us now write an algorithm for the Enqueue operation. For enqueue operation If rear >= MAXSIZE – 1 print "Queue is full“ Else rear = rear + 1; queue[rear] = item;
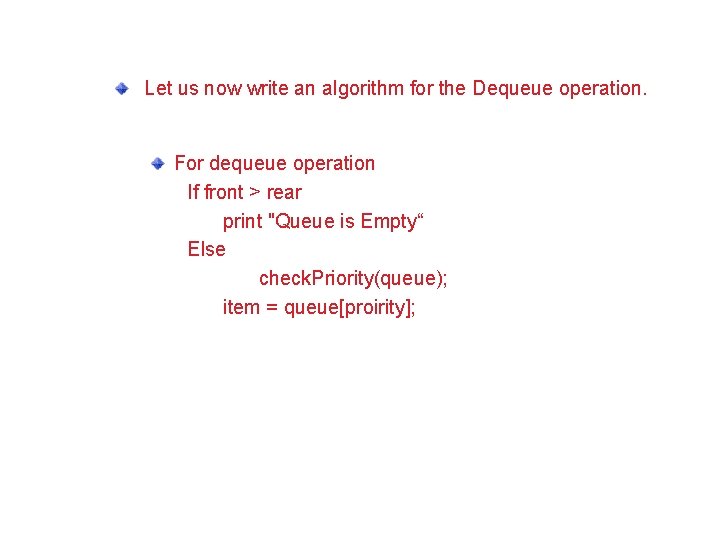
Implementing a Stack Using an Array (Contd. ) Let us now write an algorithm for the Dequeue operation. For dequeue operation If front > rear print "Queue is Empty“ Else check. Priority(queue); item = queue[proirity];
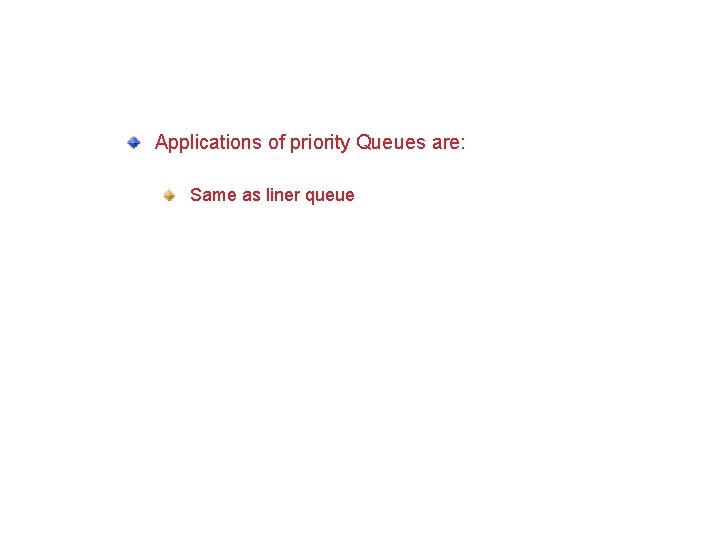
Applications of Stacks Applications of priority Queues are: Same as liner queue
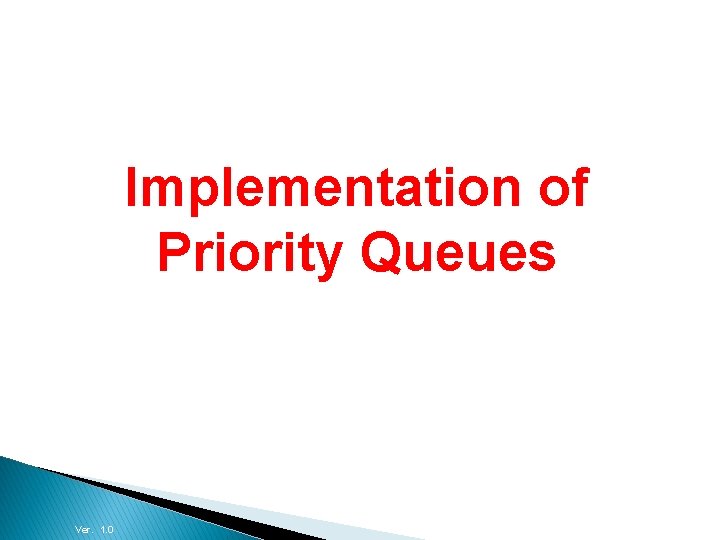
Data Structures and Algorithms Implementation of Priority Queues Ver. 1. 0 Session 10
![Class queue private int size int queuesize int front int rear public queue Class queue { private: int size; int queue[size]; int front; int rear; public: queue()](https://slidetodoc.com/presentation_image_h/02fe0b78017179d82cf1a2c51f1f726b/image-12.jpg)
Class queue { private: int size; int queue[size]; int front; int rear; public: queue() { size=10; front=0; rear= - 1; } void enqueue( int item) { if(rear= size-1) { cout<<“Queue is Full”; } else { rear++; queue[rear]=item; } }
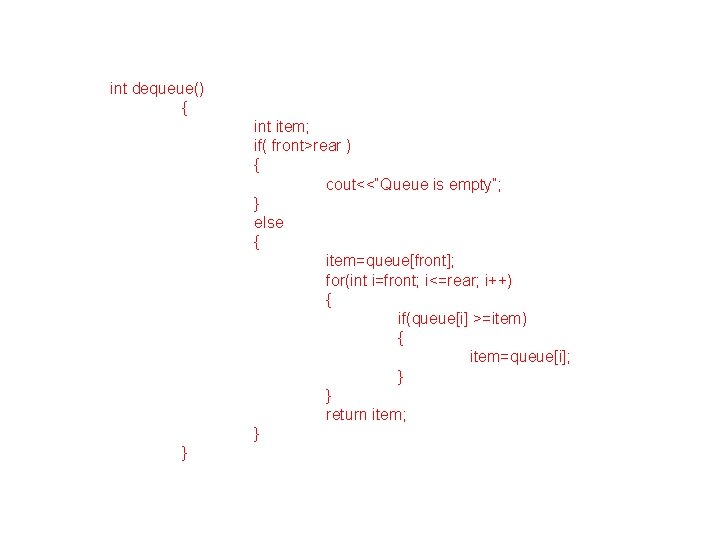
int dequeue() { int item; if( front>rear ) { cout<<“Queue is empty”; } else { item=queue[front]; for(int i=front; i<=rear; i++) { if(queue[i] >=item) { item=queue[i]; } } return item; } }
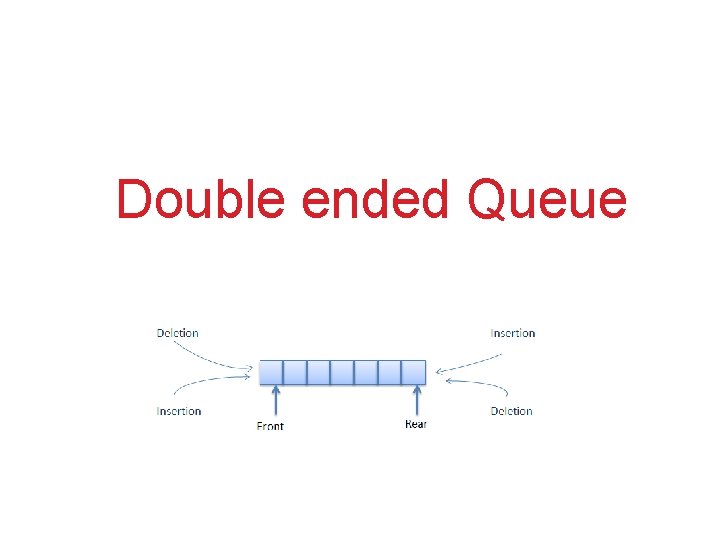
Summary (Contd. ) Double ended Queue
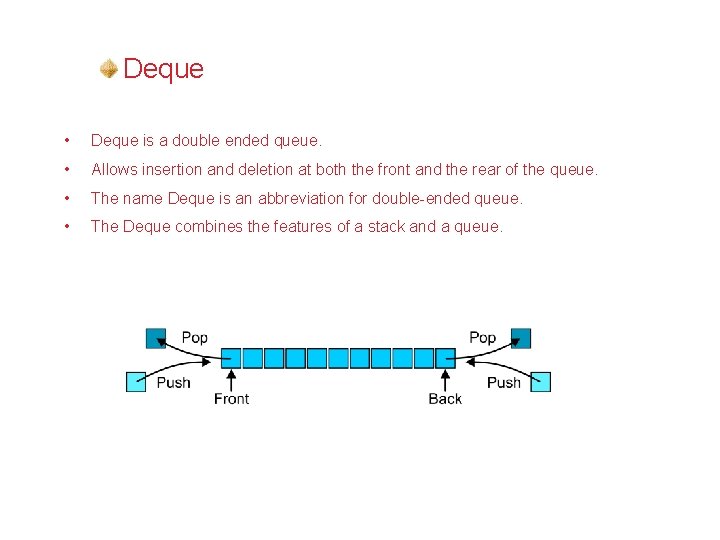
Deque Summary (Contd. ) • Deque is a double ended queue. • Allows insertion and deletion at both the front and the rear of the queue. • The name Deque is an abbreviation for double-ended queue. • The Deque combines the features of a stack and a queue.
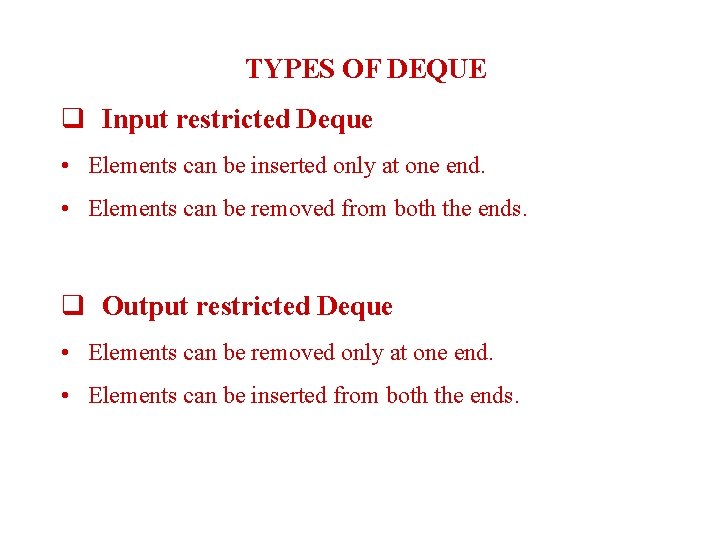
Summary (Contd. ) TYPES OF DEQUE q Input restricted Deque • Elements can be inserted only at one end. • Elements can be removed from both the ends. q Output restricted Deque • Elements can be removed only at one end. • Elements can be inserted from both the ends.
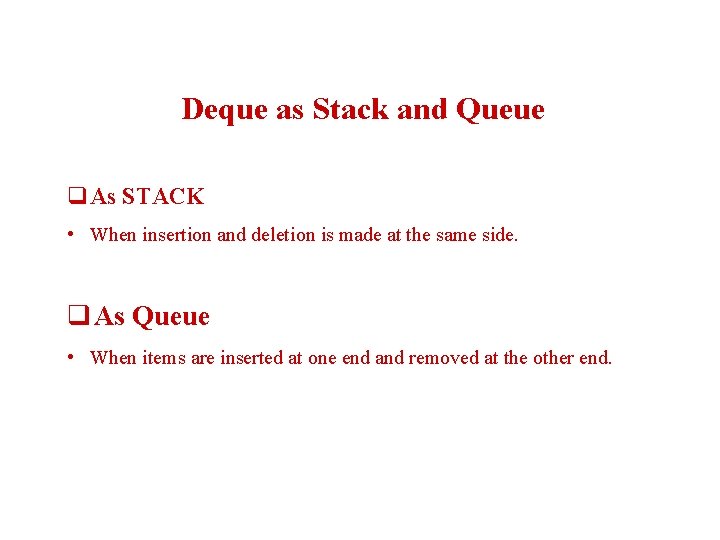
Deque as Stack and Queue q. As STACK • When insertion and deletion is made at the same side. q As Queue • When items are inserted at one end and removed at the other end.
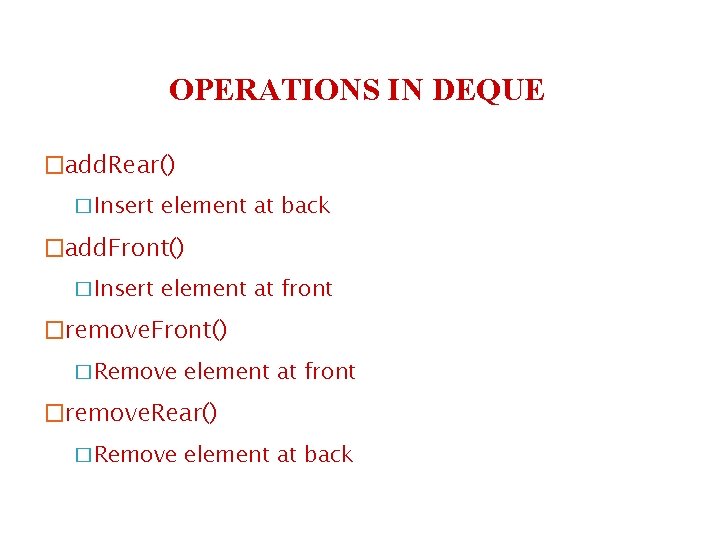
OPERATIONS IN DEQUE �add. Rear() �Insert element at back �add. Front() �Insert element at front �remove. Front() �Remove element at front �remove. Rear() �Remove element at back
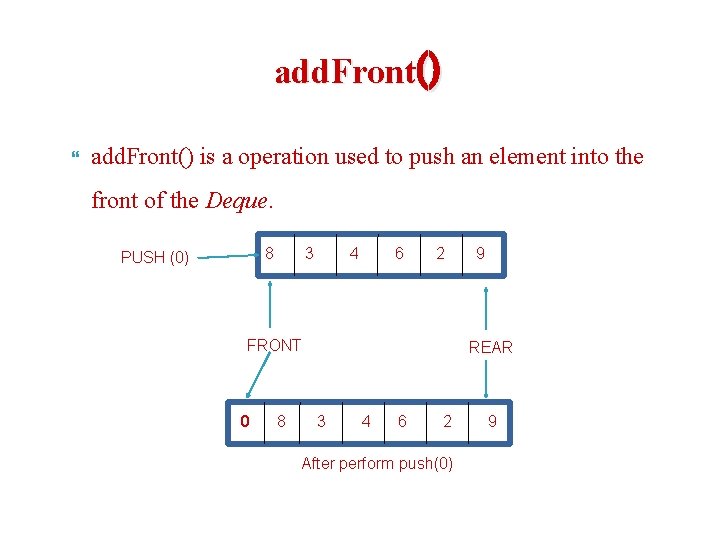
add. Front() is a operation used to push an element into the front of the Deque. 8 3 PUSH (0) 4 6 2 9 FRONT 0 8 3 REAR 4 6 2 9 After perform push(0)
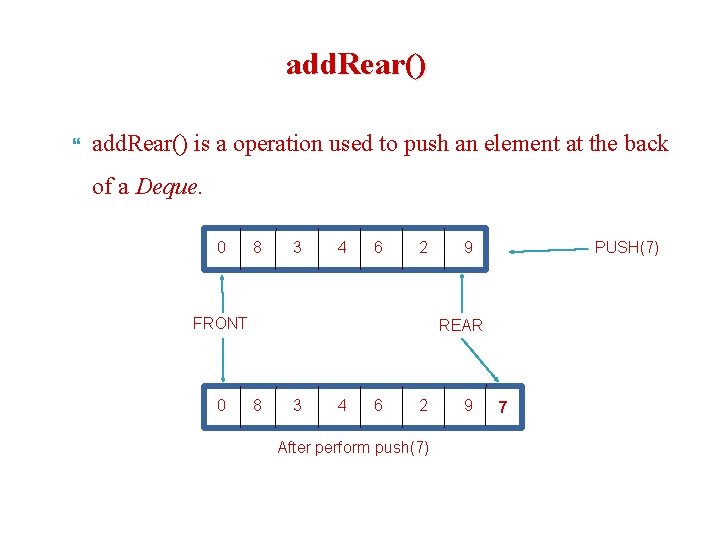
add. Rear() is a operation used to push an element at the back of a Deque. 0 8 3 4 6 2 9 FRONT 0 PUSH(7) REAR 8 3 4 6 2 9 After perform push(7) 7
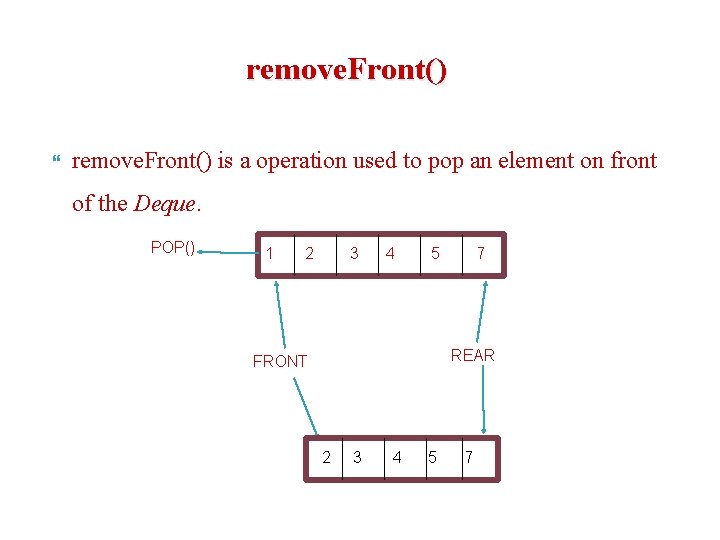
remove. Front() is a operation used to pop an element on front of the Deque. POP() 1 2 FRONT 3 4 5 7 REAR 2 3 4 5 7
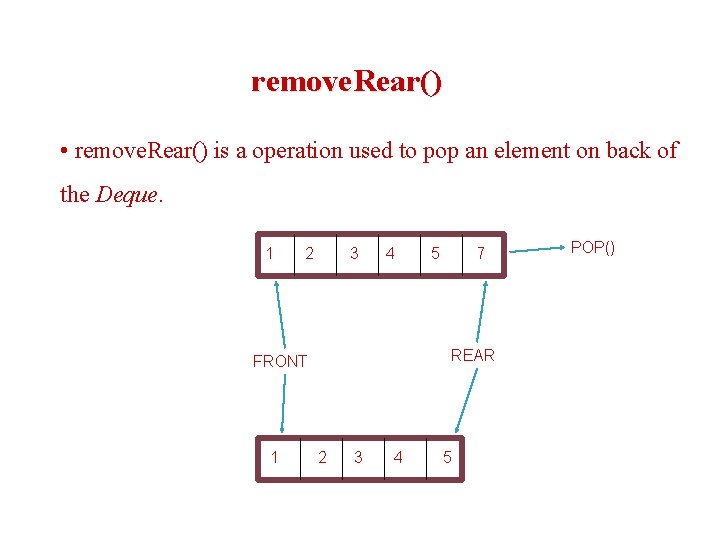
remove. Rear() • remove. Rear() is a operation used to pop an element on back of the Deque. 1 2 FRONT 3 4 5 7 REAR 1 2 3 4 5 POP()
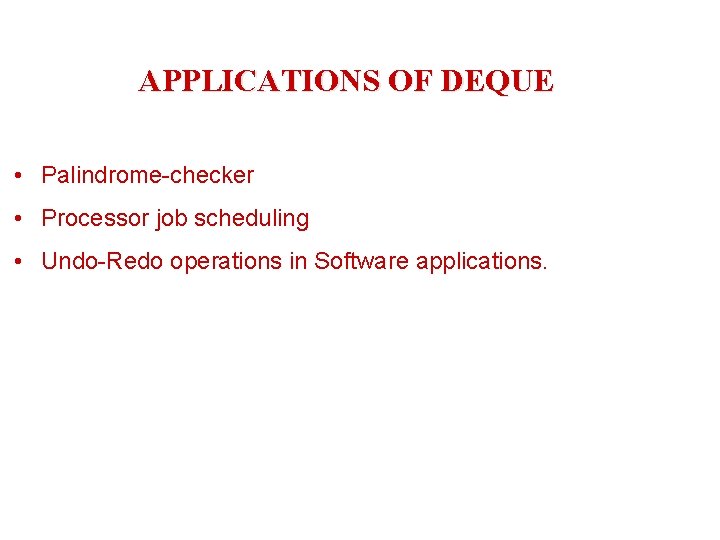
APPLICATIONS OF DEQUE • Palindrome-checker • Processor job scheduling • Undo-Redo operations in Software applications.
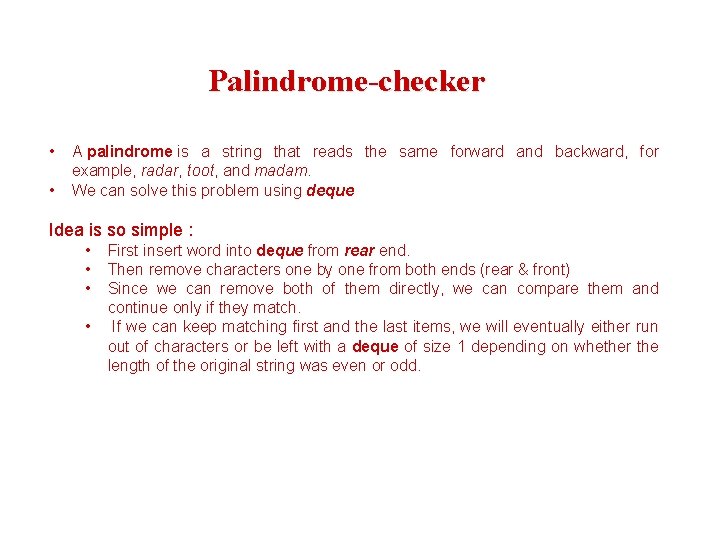
Palindrome-checker • • A palindrome is a string that reads the same forward and backward, for example, radar, toot, and madam. We can solve this problem using deque Idea is so simple : • • First insert word into deque from rear end. Then remove characters one by one from both ends (rear & front) Since we can remove both of them directly, we can compare them and continue only if they match. If we can keep matching first and the last items, we will eventually either run out of characters or be left with a deque of size 1 depending on whether the length of the original string was even or odd.
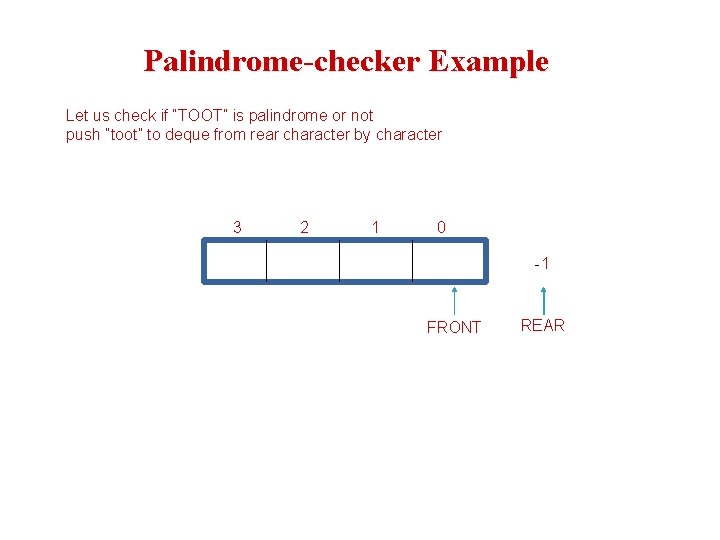
Palindrome-checker Example Let us check if “TOOT” is palindrome or not push “toot” to deque from rear character by character 3 2 1 0 -1 FRONT REAR
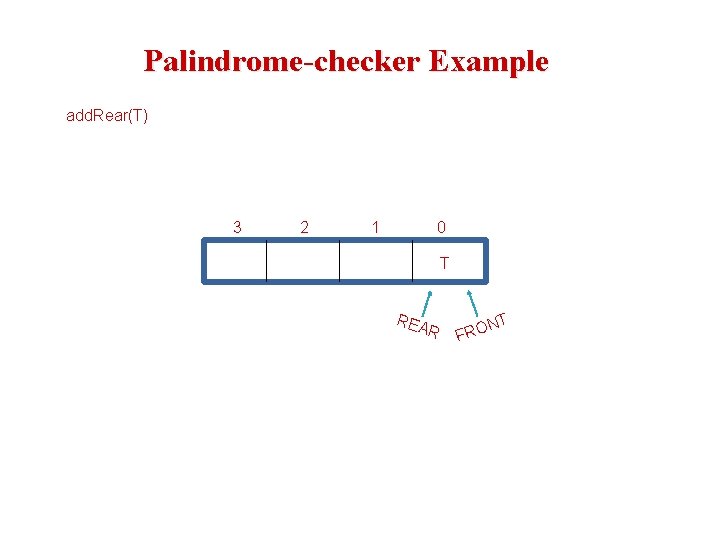
Palindrome-checker Example add. Rear(T) 3 2 1 0 T REA R NT FRO
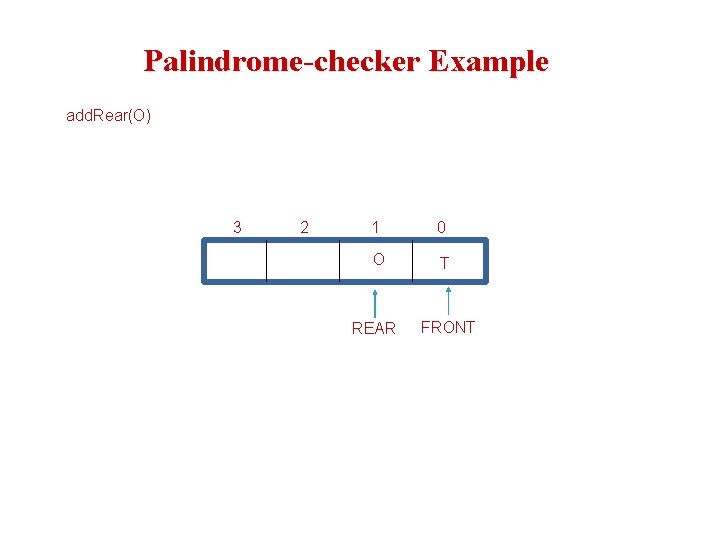
Palindrome-checker Example add. Rear(O) 3 2 1 0 O T REAR FRONT
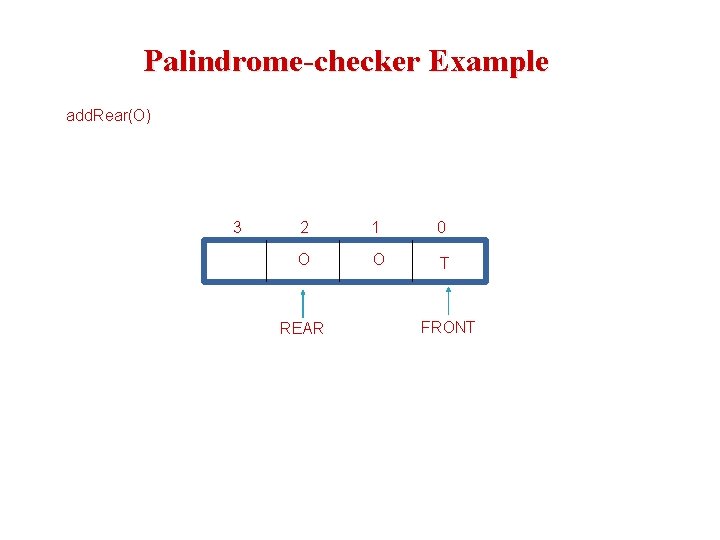
Palindrome-checker Example add. Rear(O) 3 2 1 0 O REAR O T FRONT
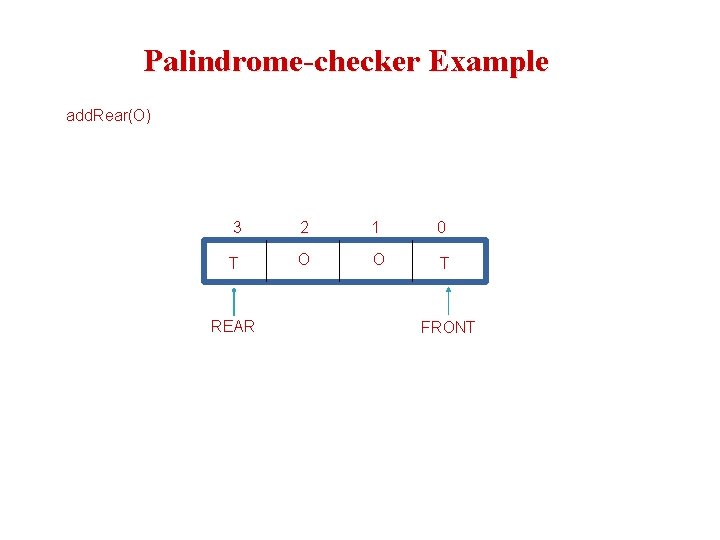
Palindrome-checker Example add. Rear(O) 3 2 1 0 T REAR O O T FRONT
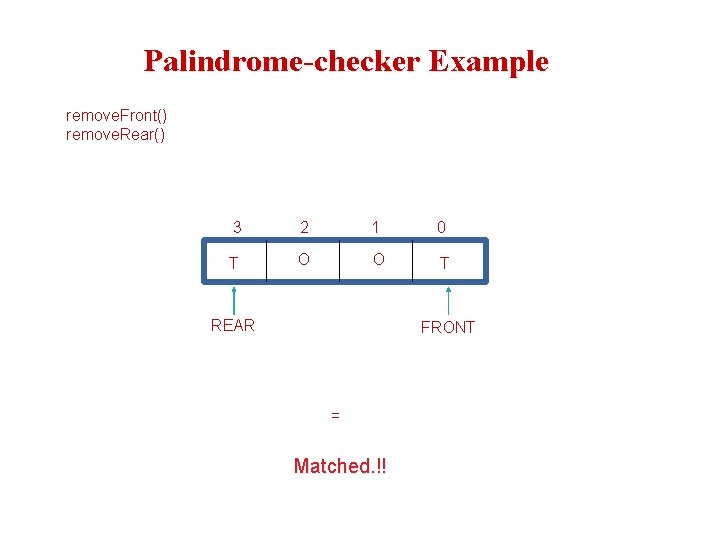
Palindrome-checker Example remove. Front() remove. Rear() 3 2 1 0 T O O REAR T FRONT = Matched. !!
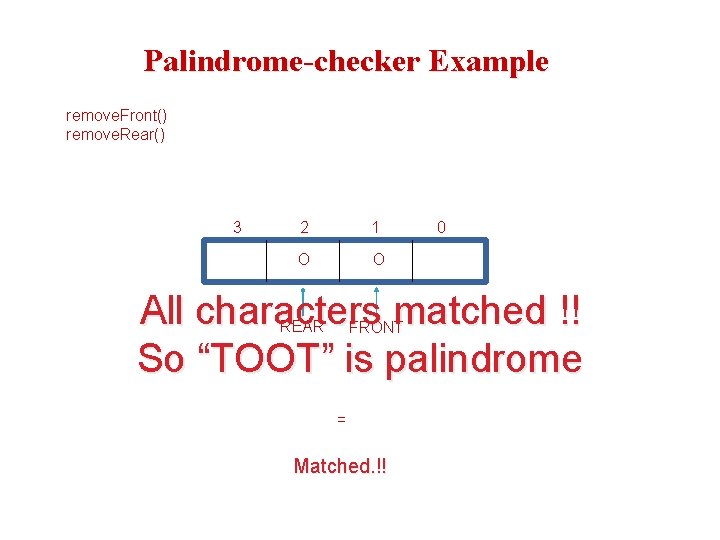
Palindrome-checker Example remove. Front() remove. Rear() 3 2 1 0 O O All characters matched !! So “TOOT” is palindrome REAR FRONT = Matched. !!
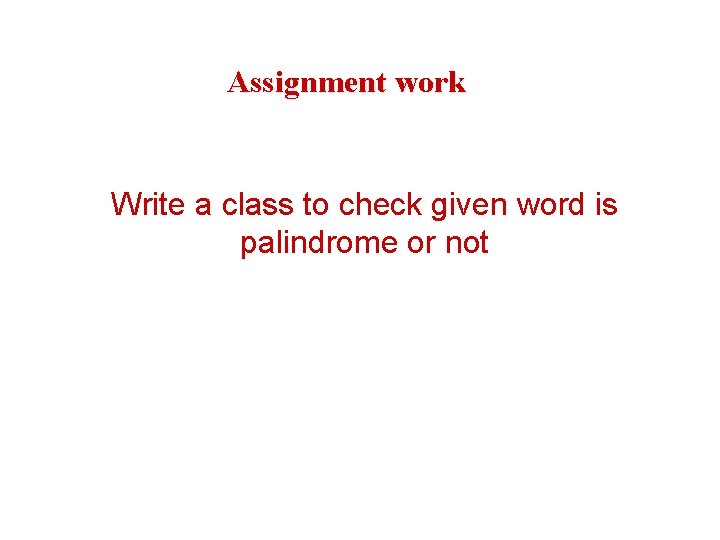
Assignment work Write a class to check given word is palindrome or not
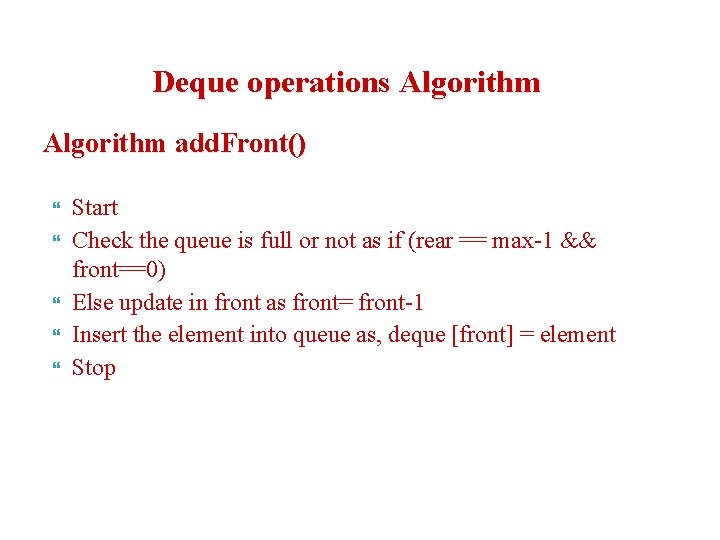
Deque operations Algorithm add. Front() Start Check the queue is full or not as if (rear == max-1 && front==0) Else update in front as front= front-1 Insert the element into queue as, deque [front] = element Stop
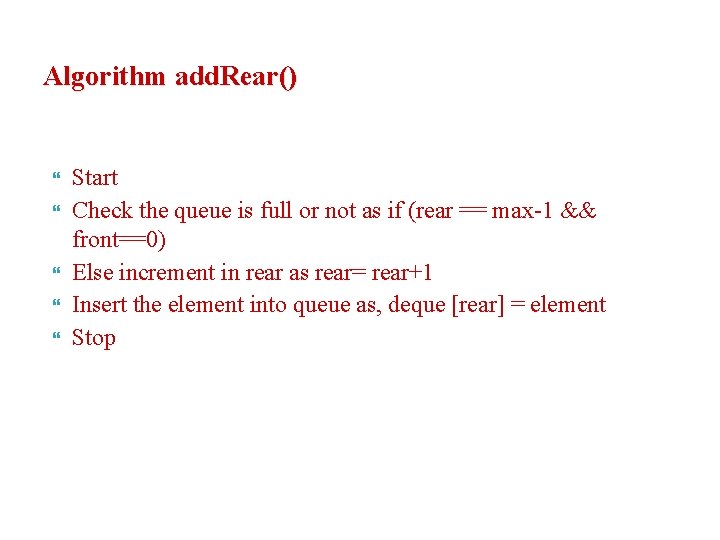
Algorithm add. Rear() Start Check the queue is full or not as if (rear == max-1 && front==0) Else increment in rear as rear= rear+1 Insert the element into queue as, deque [rear] = element Stop
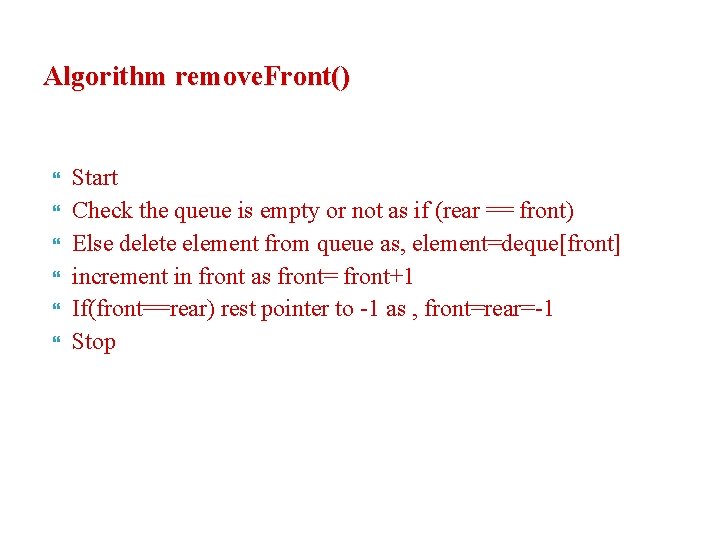
Algorithm remove. Front() Start Check the queue is empty or not as if (rear == front) Else delete element from queue as, element=deque[front] increment in front as front= front+1 If(front==rear) rest pointer to -1 as , front=rear=-1 Stop
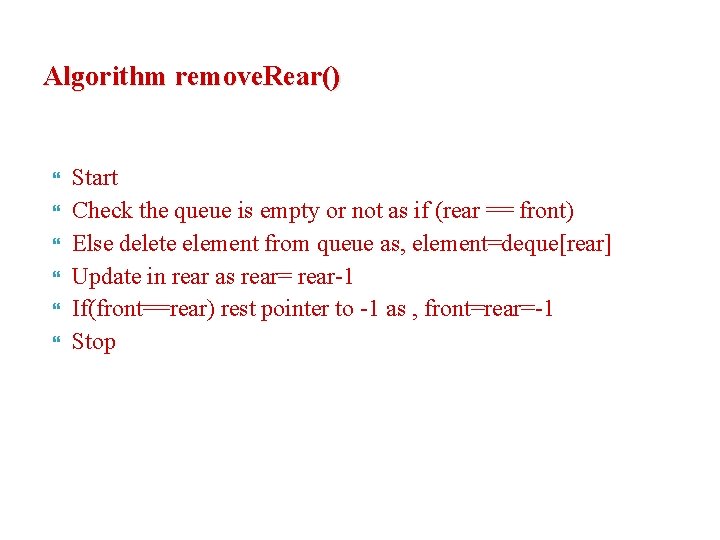
Algorithm remove. Rear() Start Check the queue is empty or not as if (rear == front) Else delete element from queue as, element=deque[rear] Update in rear as rear= rear-1 If(front==rear) rest pointer to -1 as , front=rear=-1 Stop
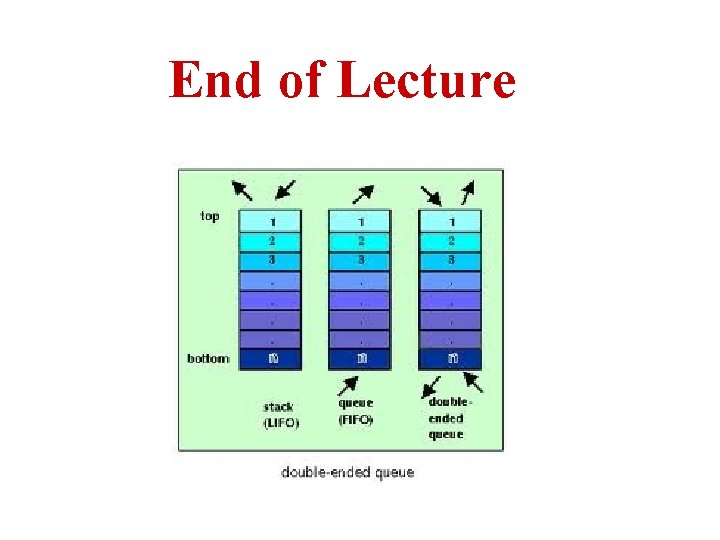
End of Lecture
Professor ajit diwan
Cos 423 princeton
Data structures and algorithms tutorial
Information retrieval data structures and algorithms
Data structures and algorithms bits pilani
Data structures and algorithms iit bombay
Data structures and algorithms
Data structures and algorithms
Ian munro waterloo
Information retrieval data structures and algorithms
Data structures and algorithms
Algorithms + data structures = programs
Burman's priority list gives priority to
Priority mail vs priority mail express
Analysis of algorithms lecture notes
Introduction to algorithms lecture notes
01:640:244 lecture notes - lecture 15: plat, idah, farad
Homologous structures example
Static data structure and dynamic data structure
Priority queue abstract data type
Data stream
What is conditional macro expansion
Assembler data structures
Data structures and abstractions with java
Adts, data structures, and problem solving with c++
Data structures and algorithm
Persistent and ephemeral data structures
Use case priority matrix for system
Use case priority matrix for system
Use case priority matrix for system
Business requirements use cases
Deque and priority queue
Use case ranking and priority matrix
Member2club
Computational thinking algorithms and programming
Design and analysis of algorithms syllabus
Association analysis: basic concepts and algorithms
Computer arithmetic: algorithms and hardware designs