Chapter 6 Arrays Liang Introduction to Java Programming
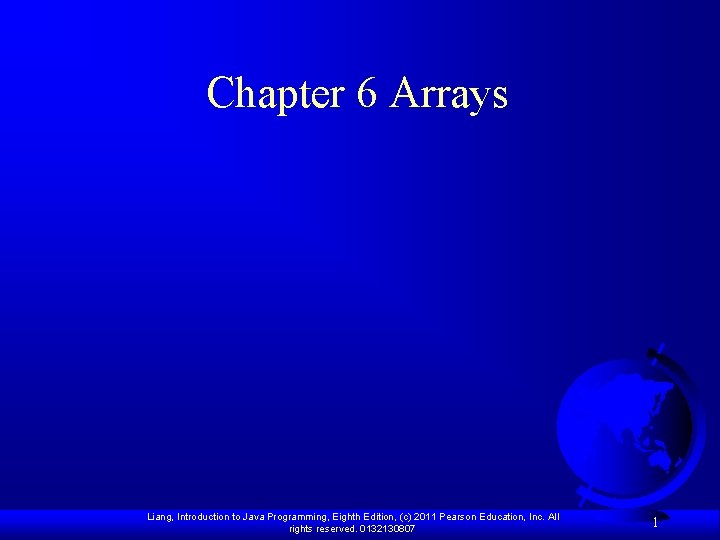
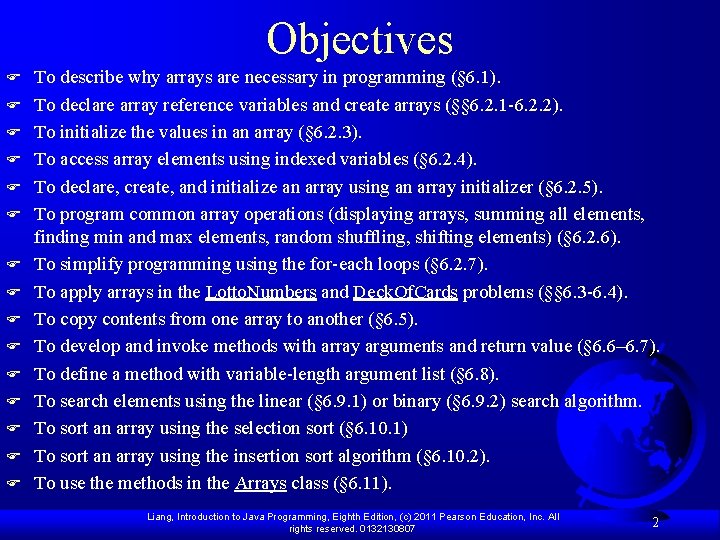
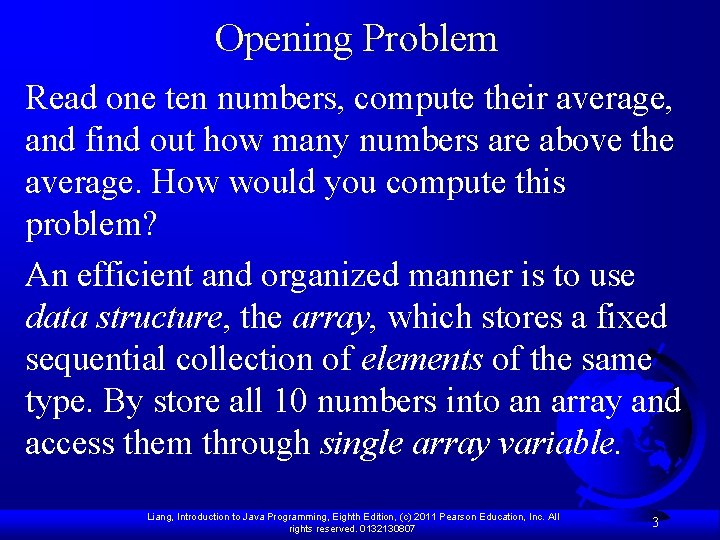
![Solution public class Analyze. Numbers { public static void main(String[] args) { final int Solution public class Analyze. Numbers { public static void main(String[] args) { final int](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-4.jpg)
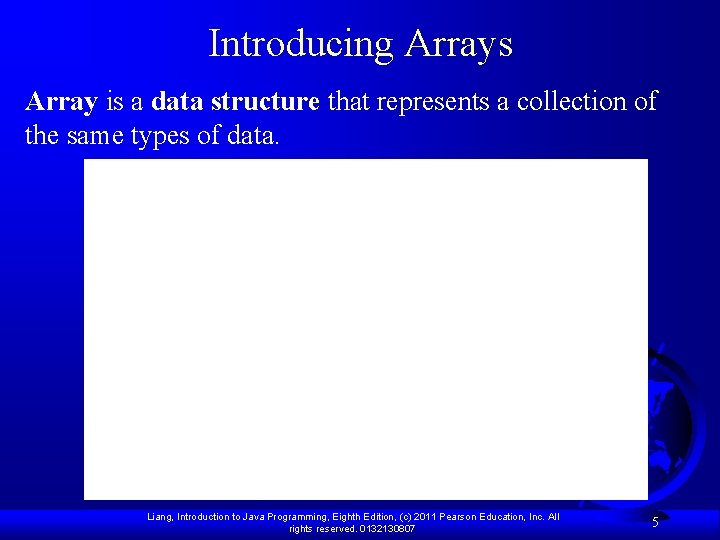
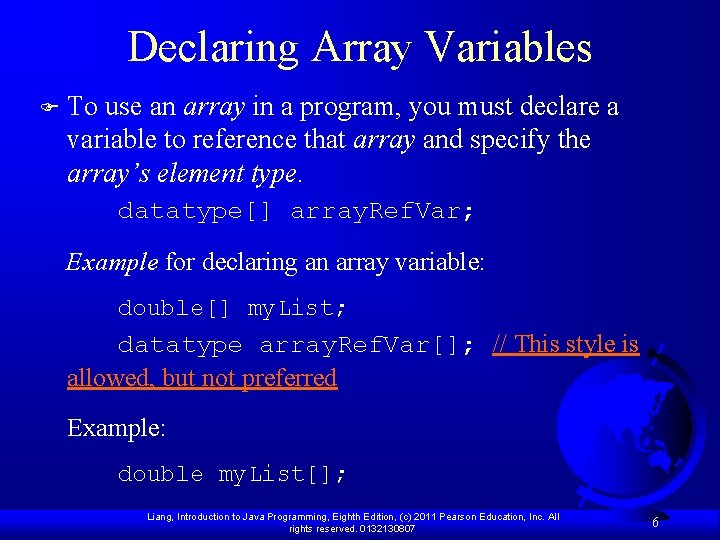
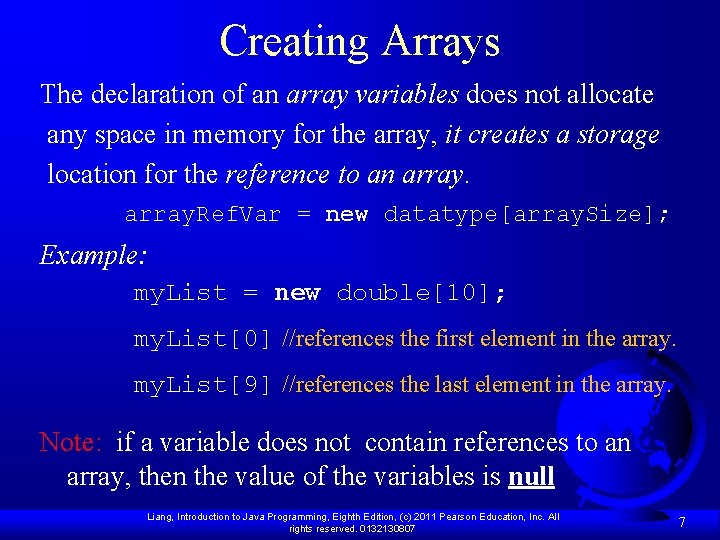
![Declaring and Creating in One Step datatype[]array. Ref. Var = new datatype[array. Size]; F Declaring and Creating in One Step datatype[]array. Ref. Var = new datatype[array. Size]; F](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-8.jpg)
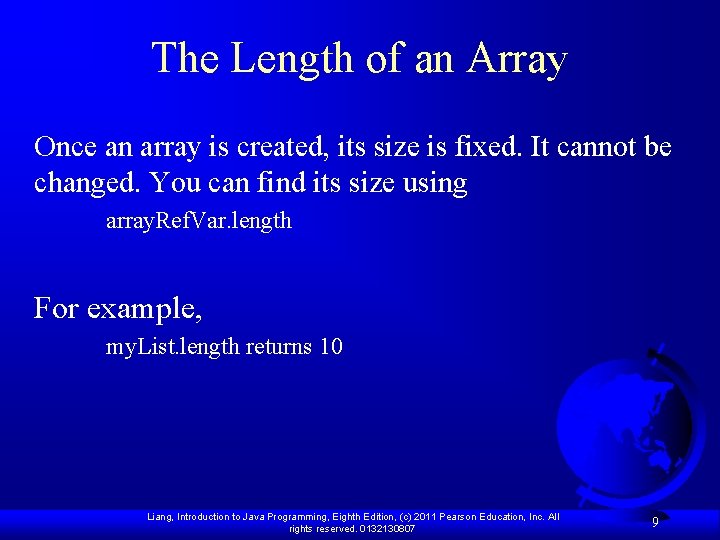
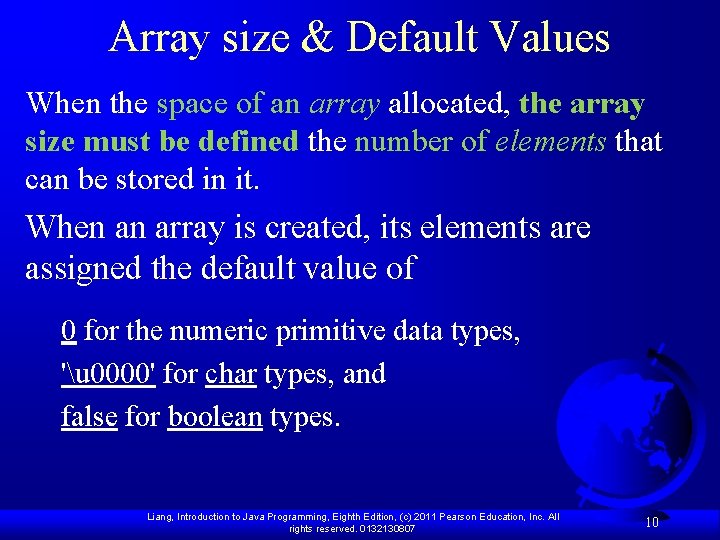
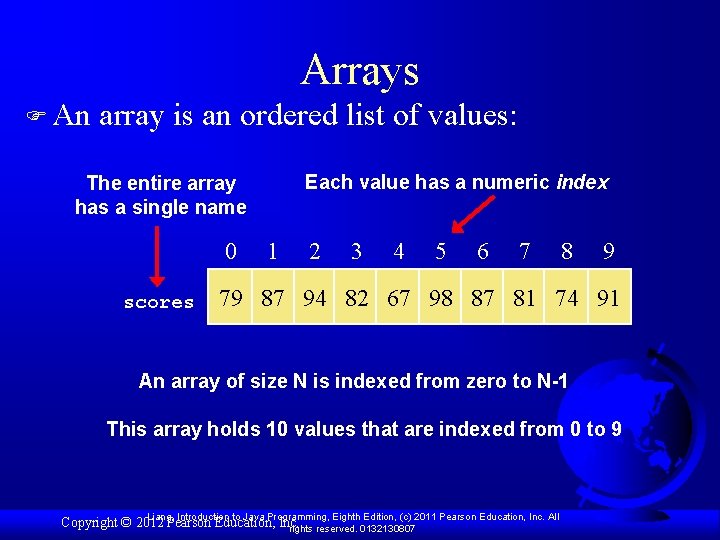
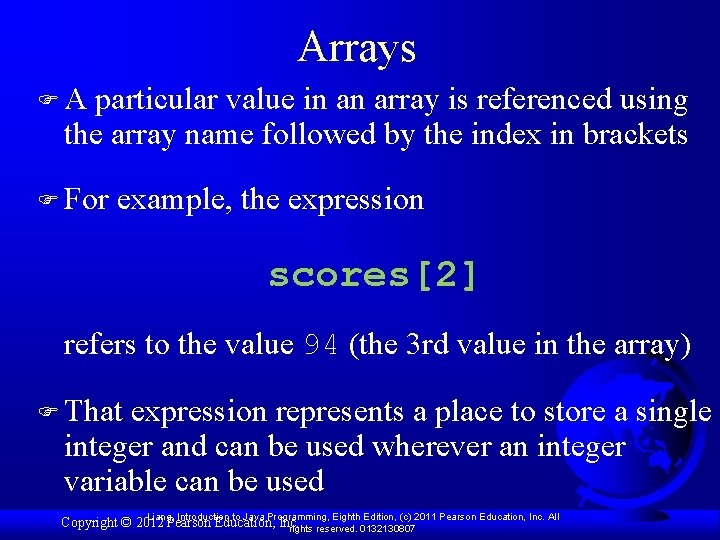
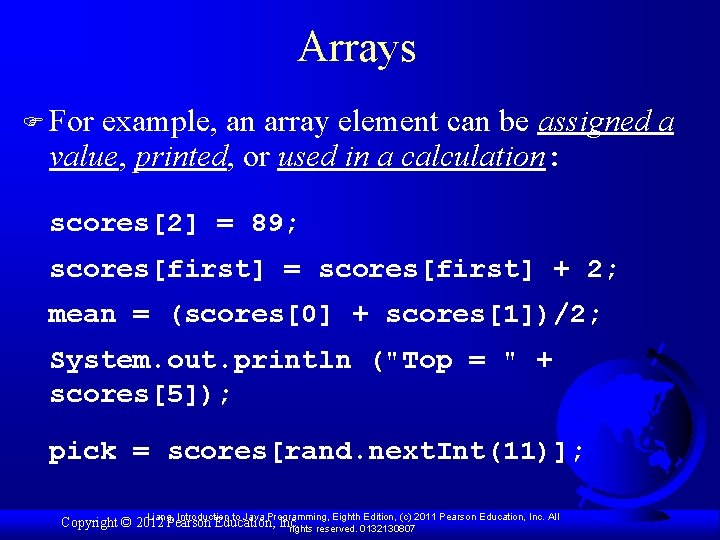
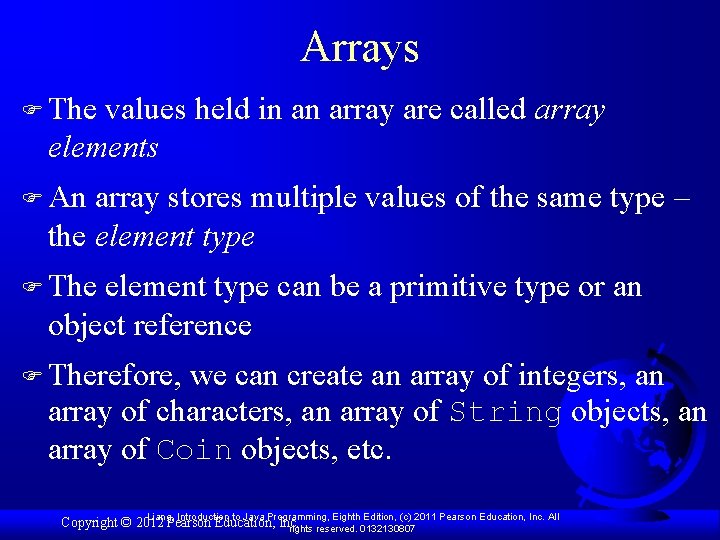
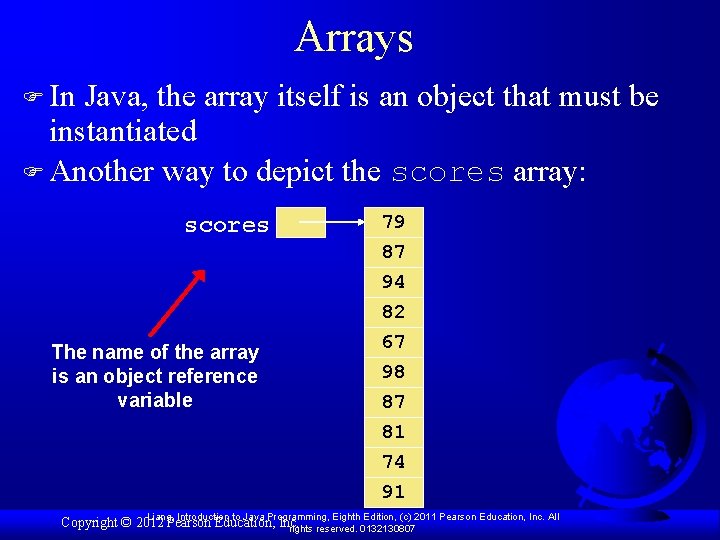
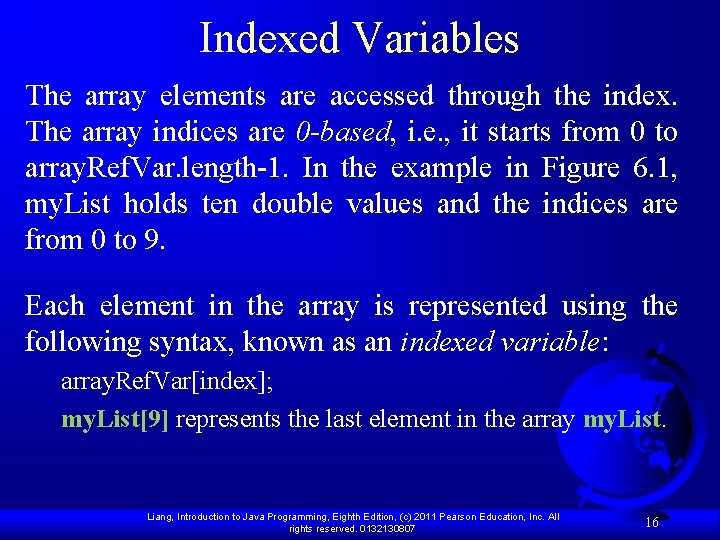
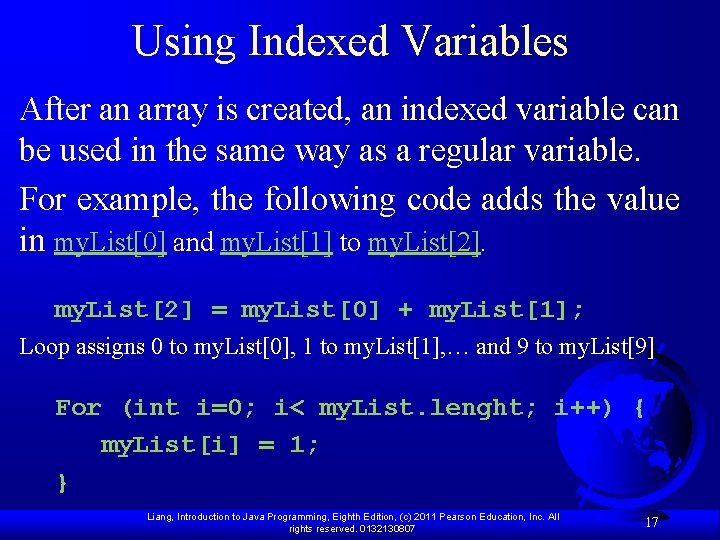
![Array Initializers double[] my. List; //declare my. List = new double[4]; //create Declaring, creating, Array Initializers double[] my. List; //declare my. List = new double[4]; //create Declaring, creating,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-18.jpg)
![Declaring, creating, initializing Using the Shorthand Notation double[] my. List = {1. 9, 2. Declaring, creating, initializing Using the Shorthand Notation double[] my. List = {1. 9, 2.](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-19.jpg)
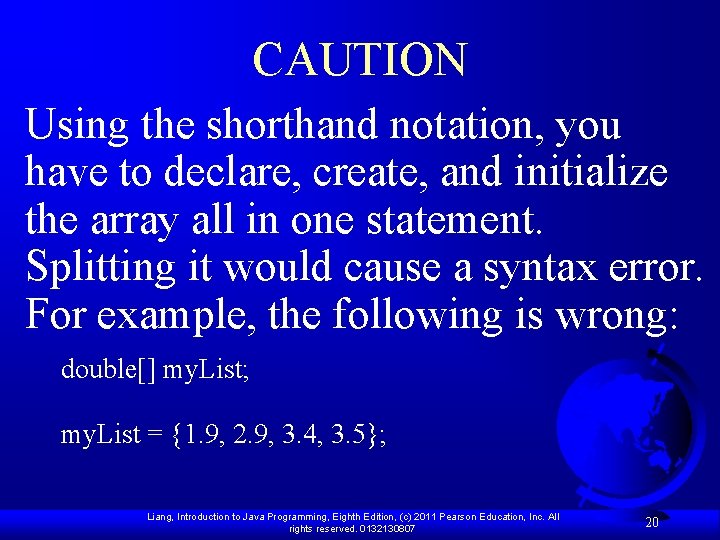
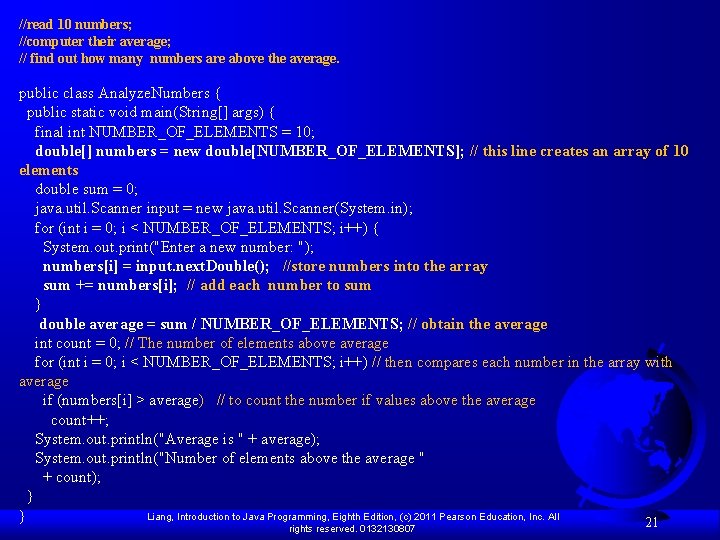
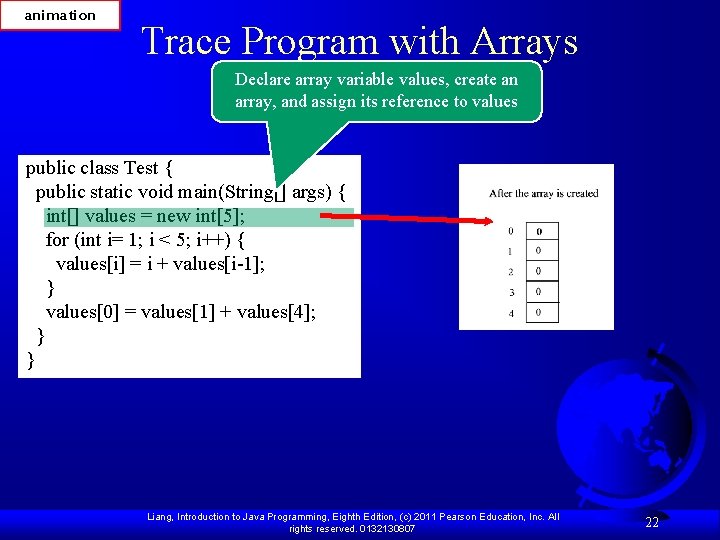
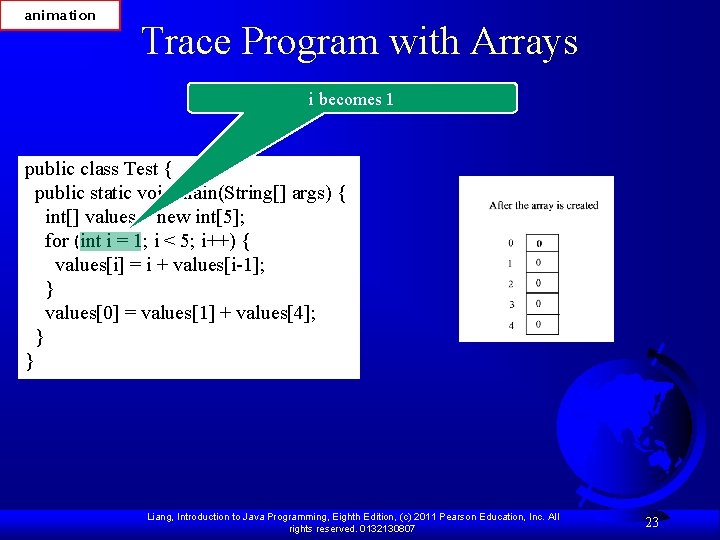
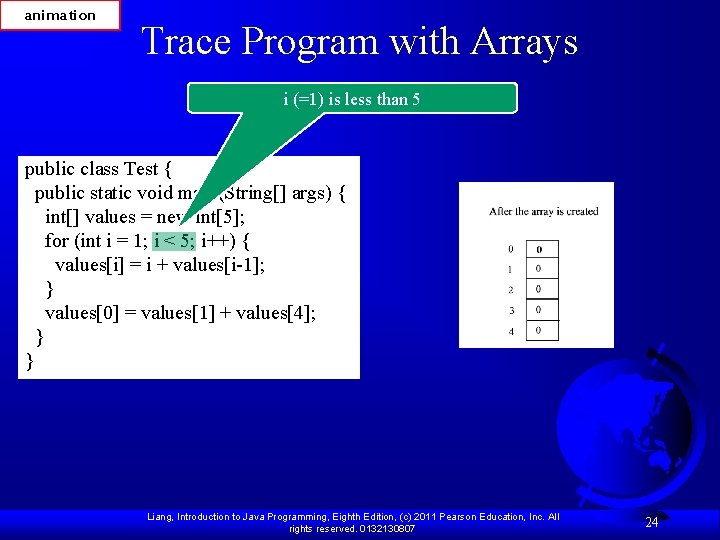
![animation Trace Program with Arrays After this line is executed, value[1] is 1 public animation Trace Program with Arrays After this line is executed, value[1] is 1 public](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-25.jpg)
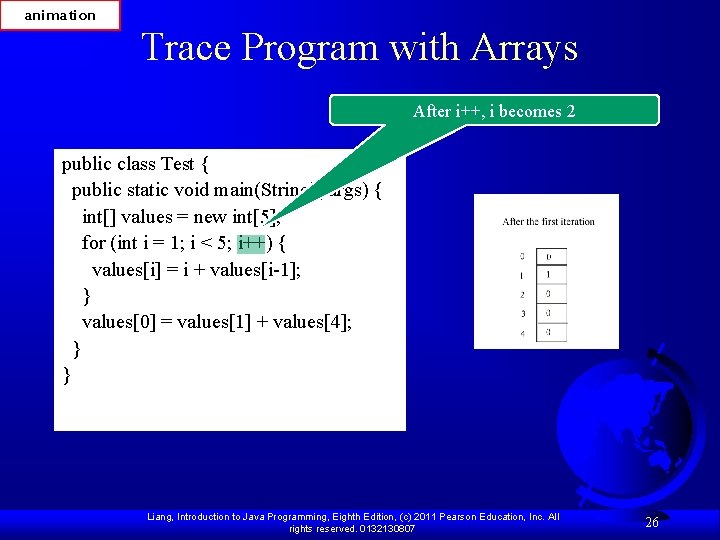
![animation Trace Program with Arrays public class Test { public static void main(String[] args) animation Trace Program with Arrays public class Test { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-27.jpg)
![animation Trace Program with Arrays After this line is executed, values[2] is 3 (2 animation Trace Program with Arrays After this line is executed, values[2] is 3 (2](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-28.jpg)
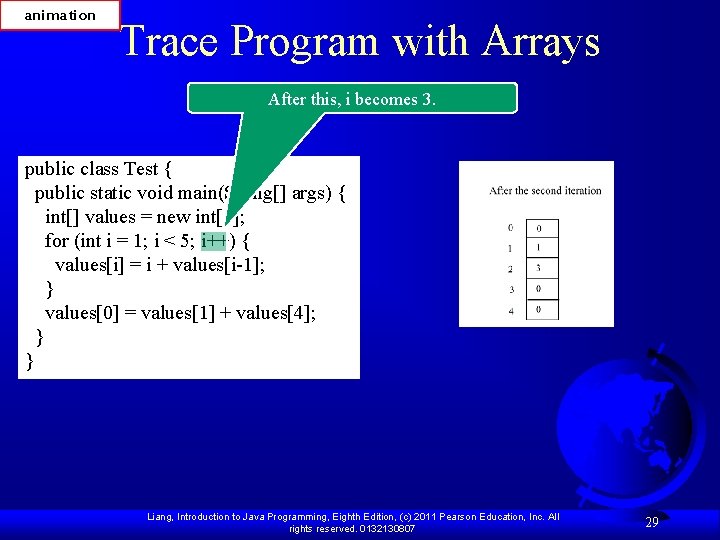
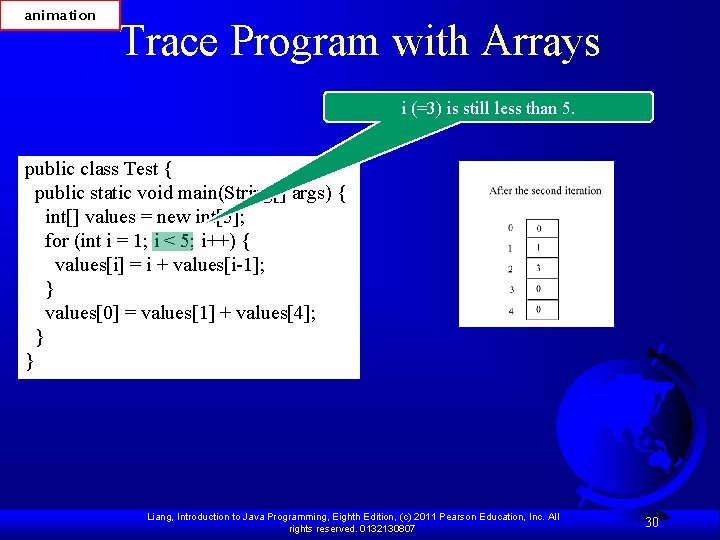
![animation Trace Program with Arrays After this line, values[3] becomes 6 (3 + 3) animation Trace Program with Arrays After this line, values[3] becomes 6 (3 + 3)](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-31.jpg)
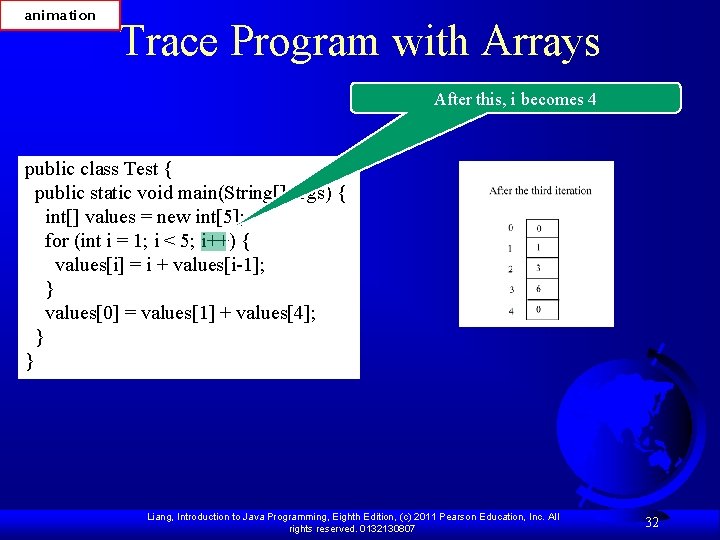
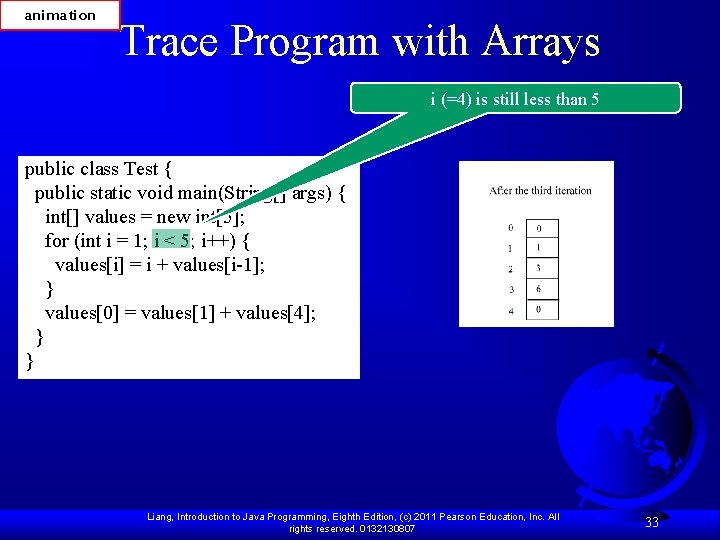
![animation Trace Program with Arrays After this, values[4] becomes 10 (4 + 6) public animation Trace Program with Arrays After this, values[4] becomes 10 (4 + 6) public](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-34.jpg)
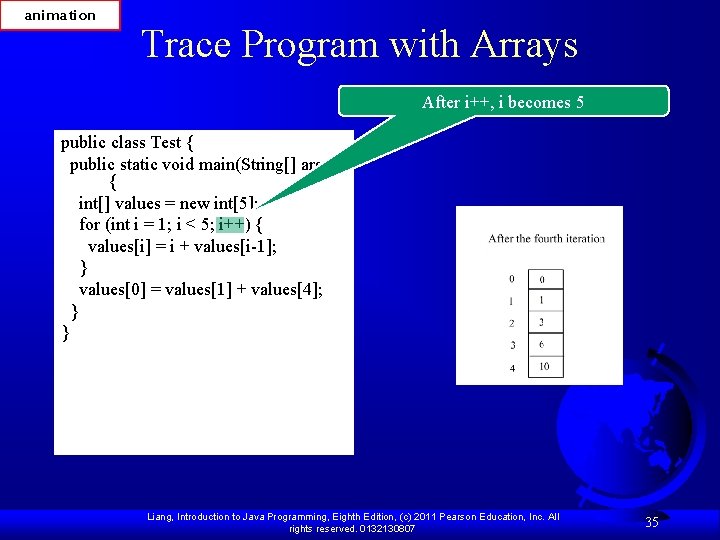
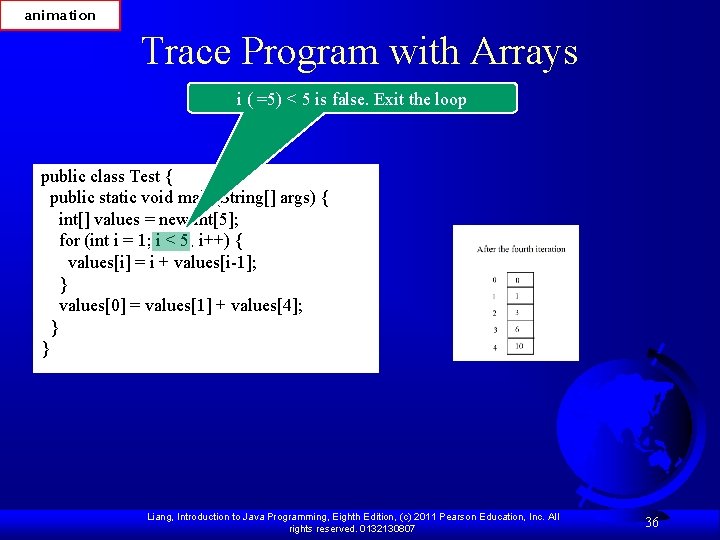
![animation Trace Program with Arrays After this line, values[0] is 11 (1 + 10) animation Trace Program with Arrays After this line, values[0] is 11 (1 + 10)](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-37.jpg)
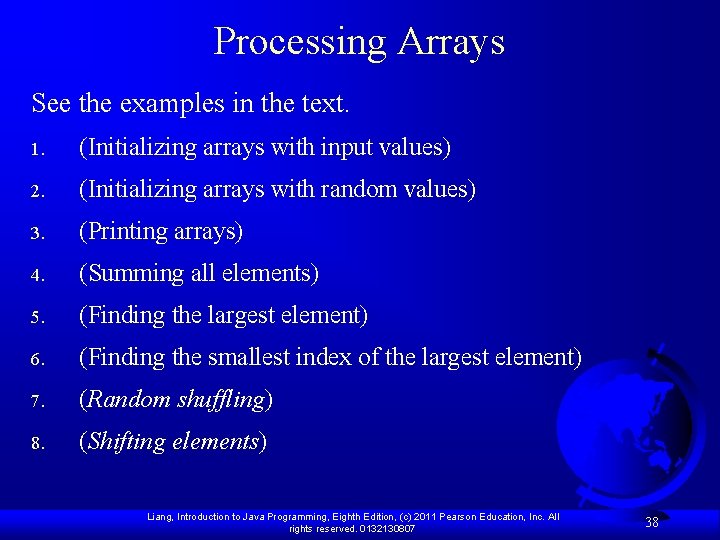
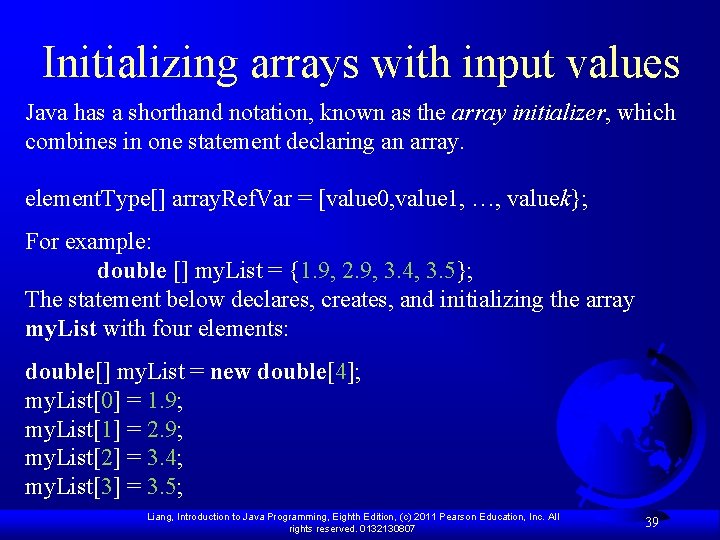
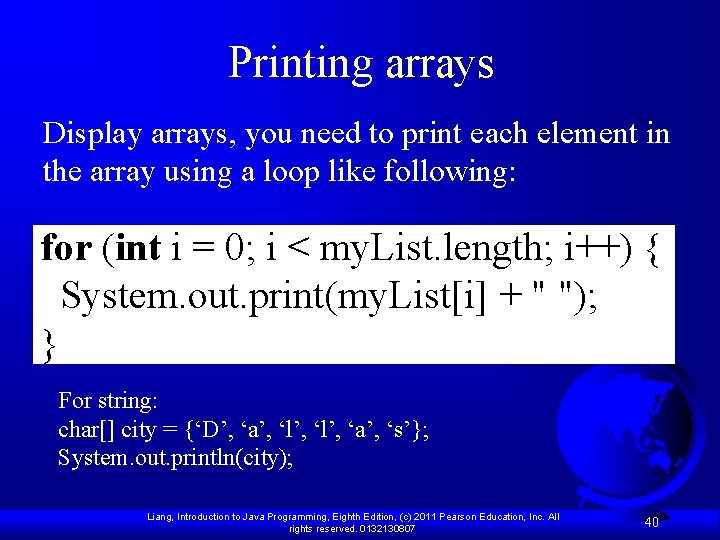
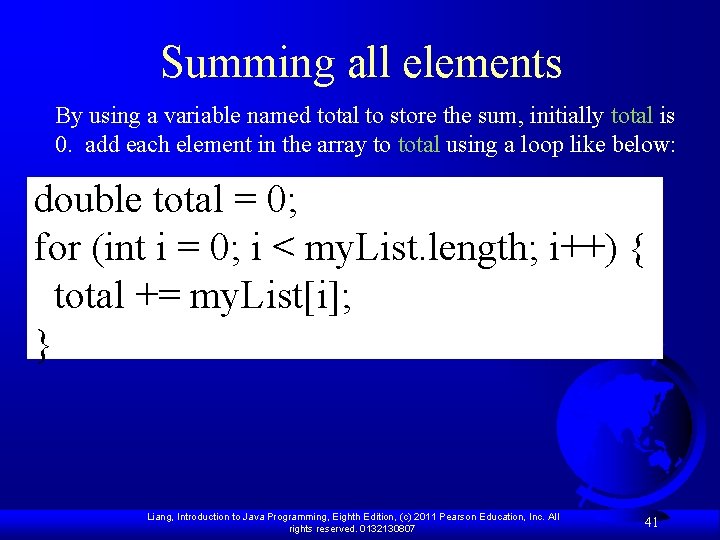
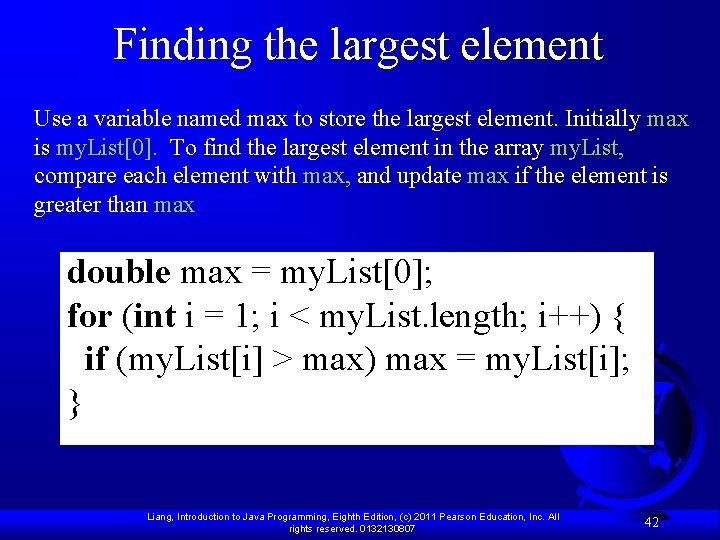
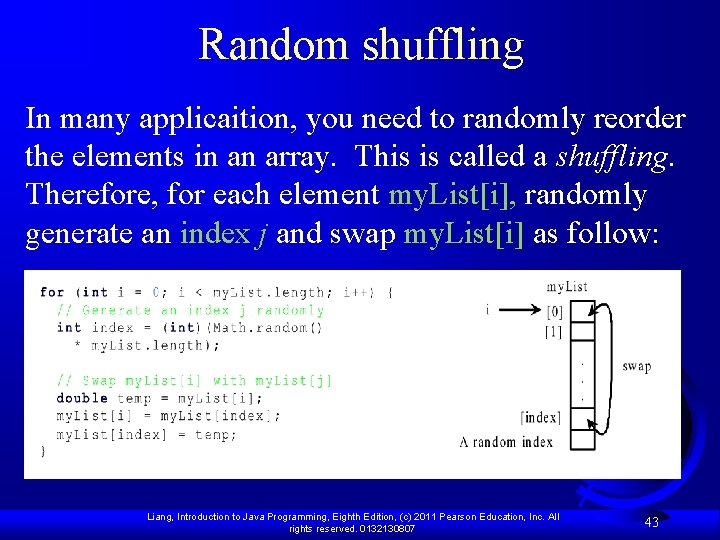
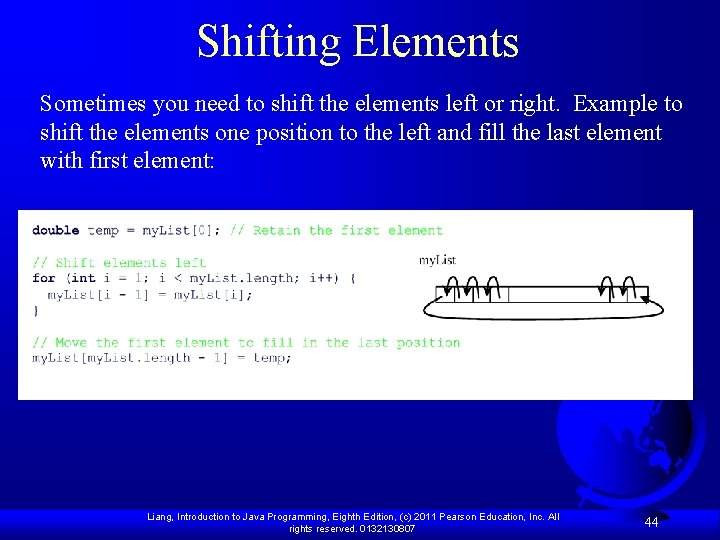
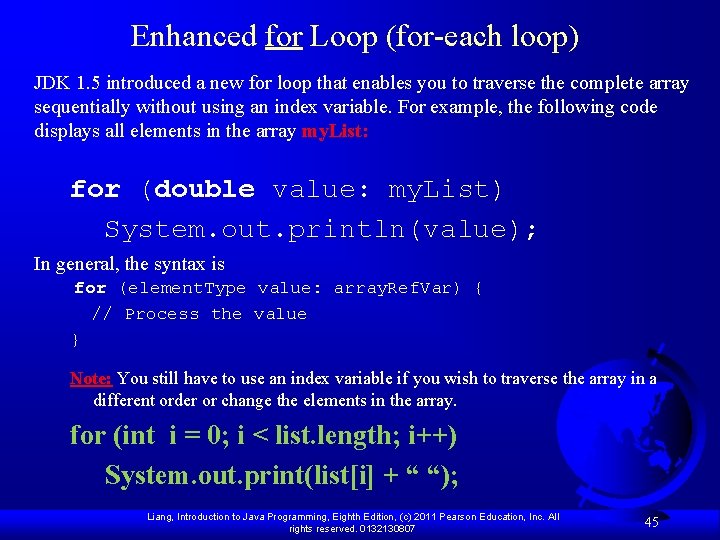
![Enhanced For Loop F class Enhanced. For. Test{ 4 public static void main(String[] args) Enhanced For Loop F class Enhanced. For. Test{ 4 public static void main(String[] args)](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-46.jpg)
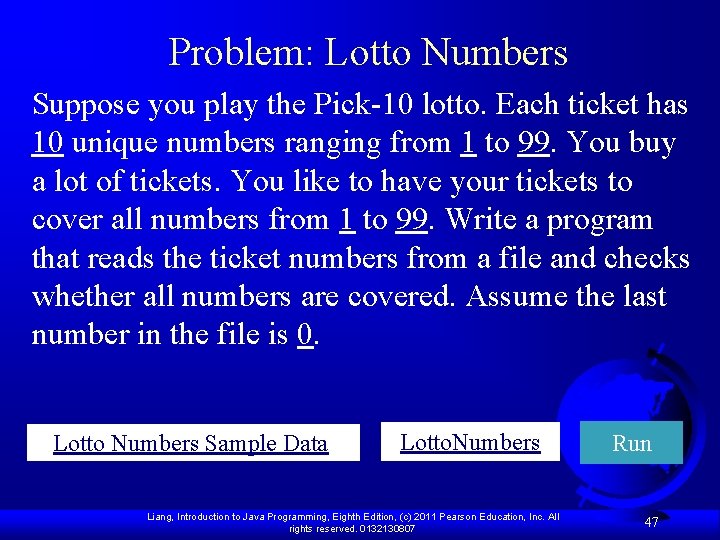
![import java. util. Scanner; public class Lotto. Numbers { public static void main(String args[]) import java. util. Scanner; public class Lotto. Numbers { public static void main(String args[])](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-48.jpg)
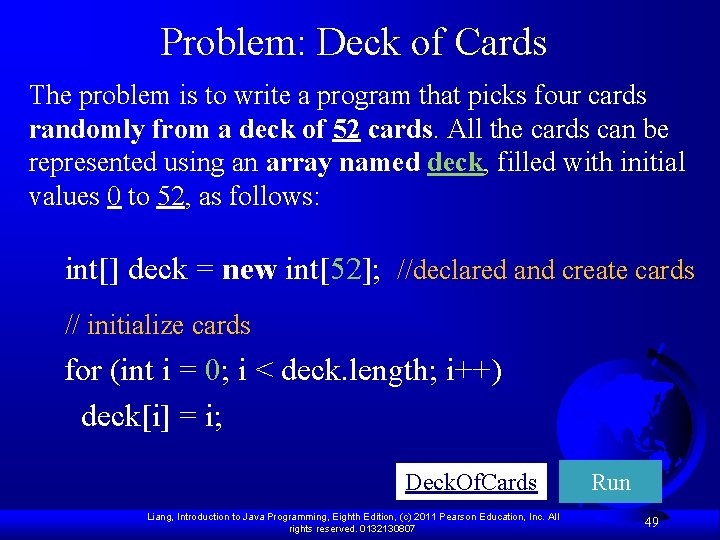
![public class Deck. Of. Cards { public static void main(String[] args) { int[] deck public class Deck. Of. Cards { public static void main(String[] args) { int[] deck](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-50.jpg)
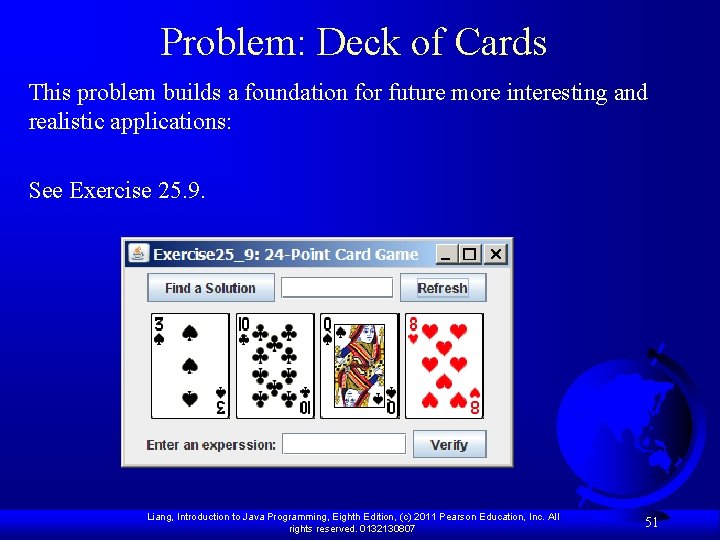
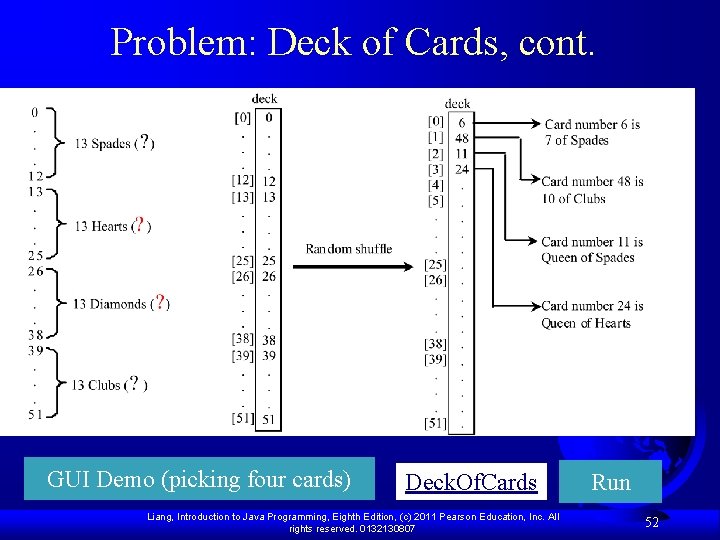
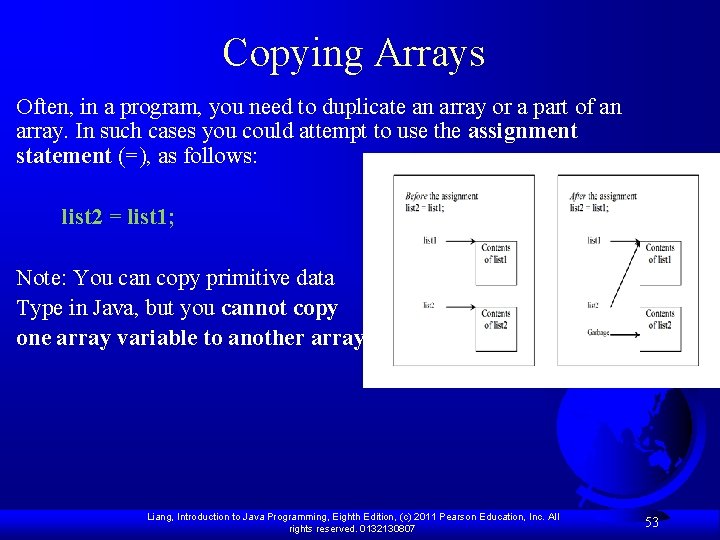
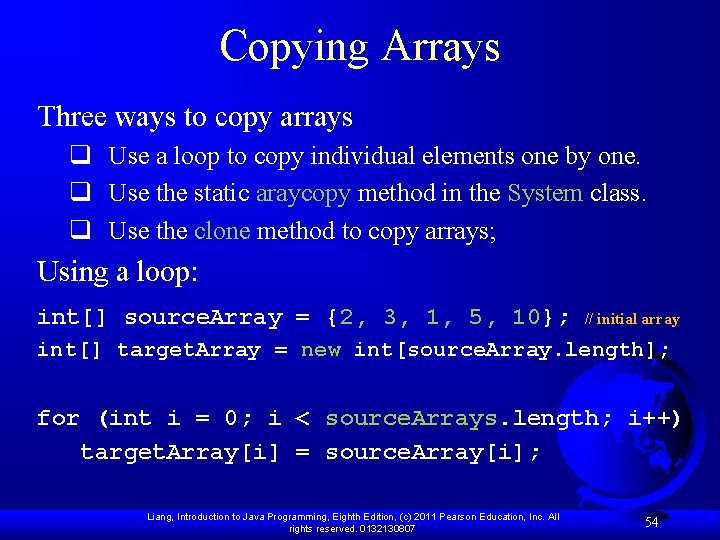
![Passing Arrays to Methods public static void print. Array(int[] array) { for (int i Passing Arrays to Methods public static void print. Array(int[] array) { for (int i](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-55.jpg)
![Anonymous Array The statement print. Array(new int[]{3, 1, 2, 6, 4, 2}); creates an Anonymous Array The statement print. Array(new int[]{3, 1, 2, 6, 4, 2}); creates an](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-56.jpg)
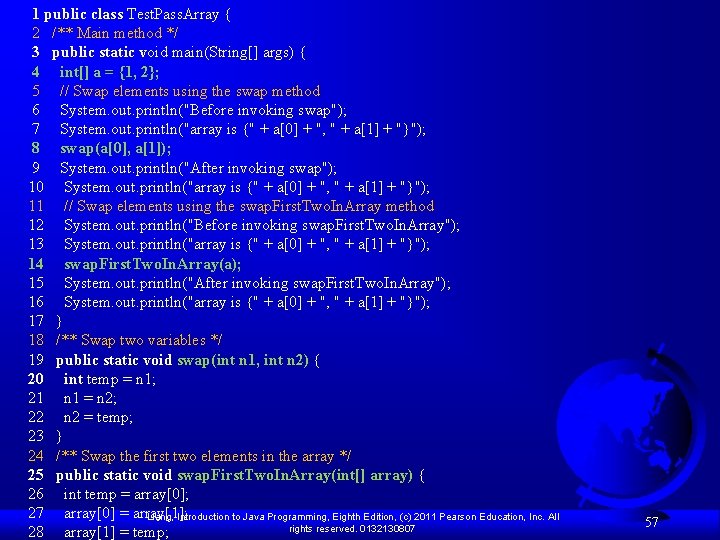
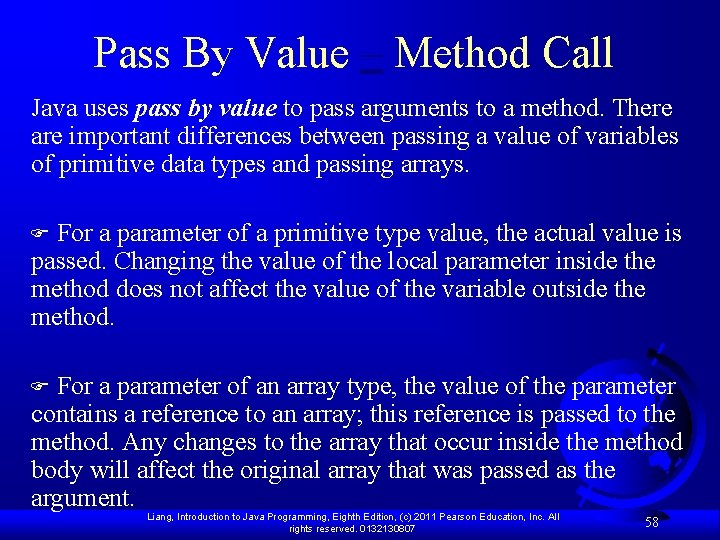
![Simple Example public class Test { public static void main(String[] args) { int x Simple Example public class Test { public static void main(String[] args) { int x](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-59.jpg)
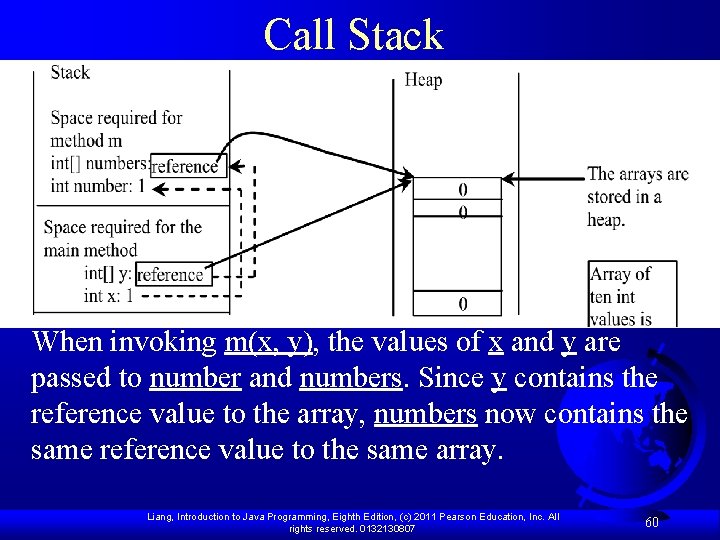
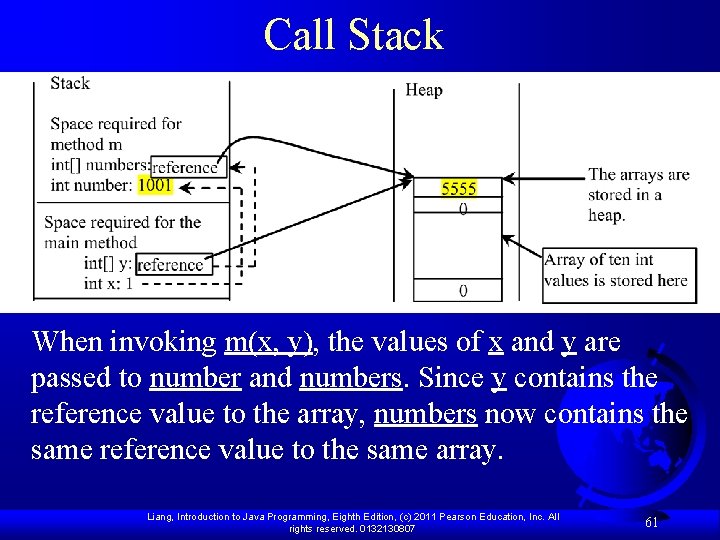
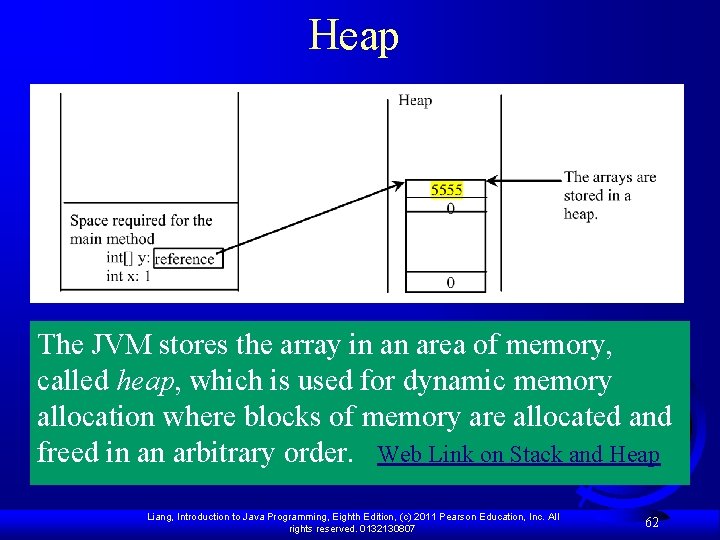
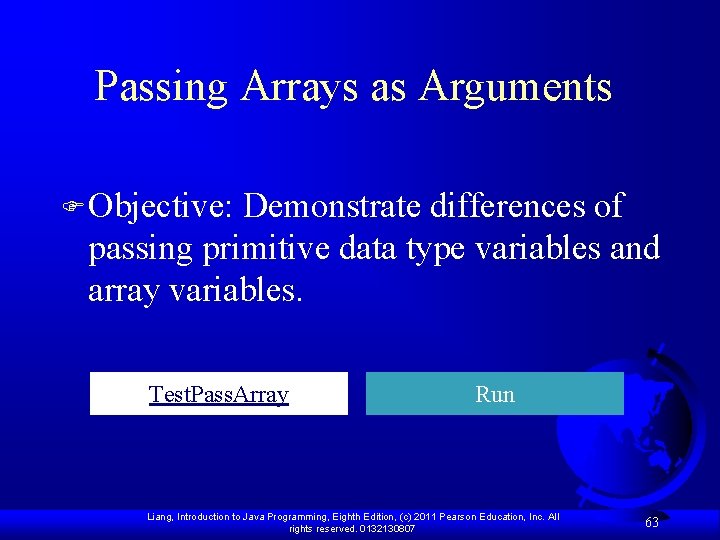
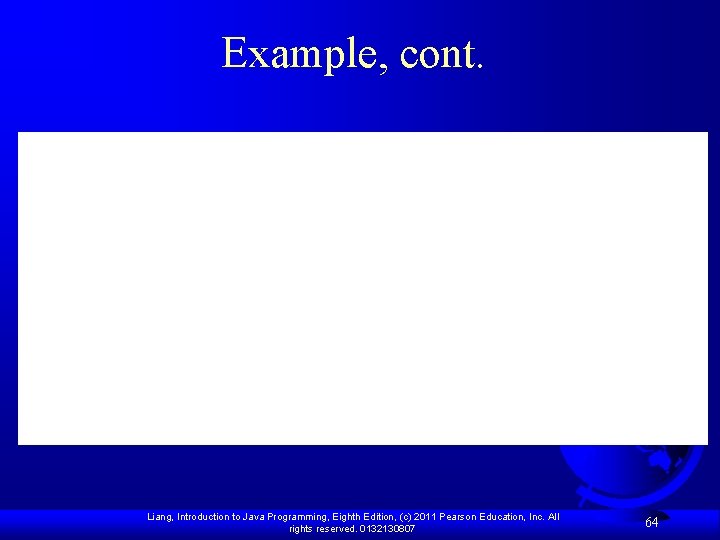
![Returning an Array from a Method public static int[] reverse(int[] list) { int[] result Returning an Array from a Method public static int[] reverse(int[] list) { int[] result](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-65.jpg)
![animation Trace the reverse Method int[] list 1 = {1, 2, 3, 4, 5, animation Trace the reverse Method int[] list 1 = {1, 2, 3, 4, 5,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-66.jpg)
![animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-67.jpg)
![animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-68.jpg)
![animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-69.jpg)
![animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-70.jpg)
![animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-71.jpg)
![animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-72.jpg)
![animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-73.jpg)
![animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-74.jpg)
![animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-75.jpg)
![animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-76.jpg)
![animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-77.jpg)
![animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-78.jpg)
![animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-79.jpg)
![animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-80.jpg)
![animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-81.jpg)
![animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-82.jpg)
![animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-83.jpg)
![animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-84.jpg)
![animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-85.jpg)
![animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-86.jpg)
![animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-87.jpg)
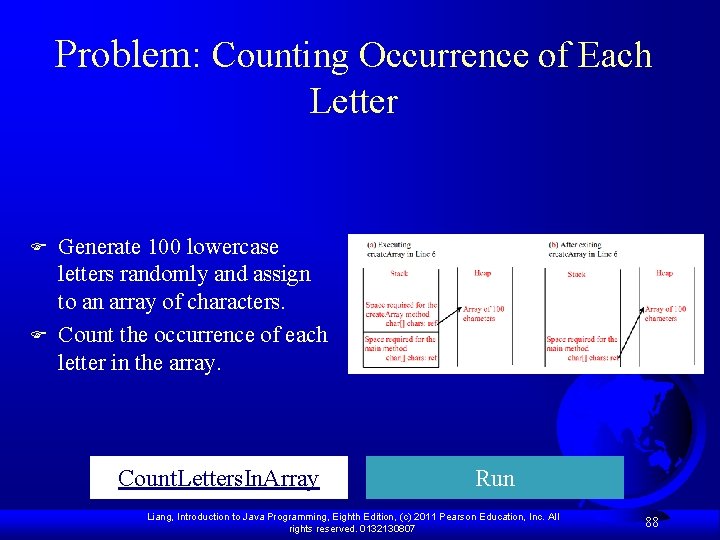
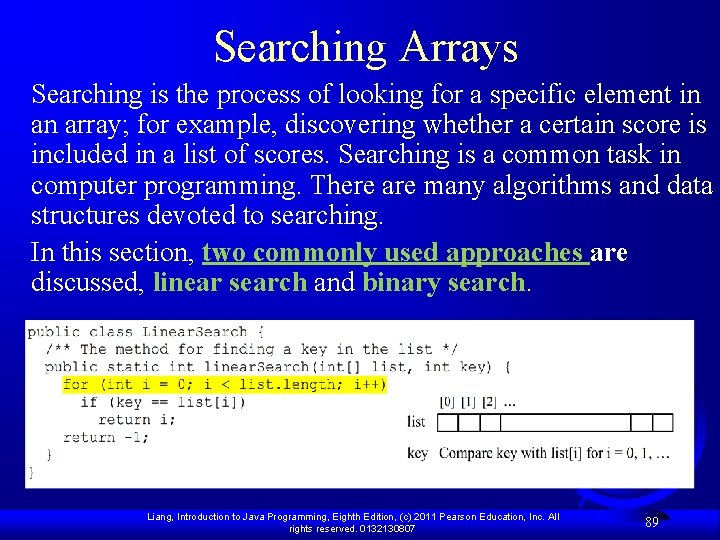
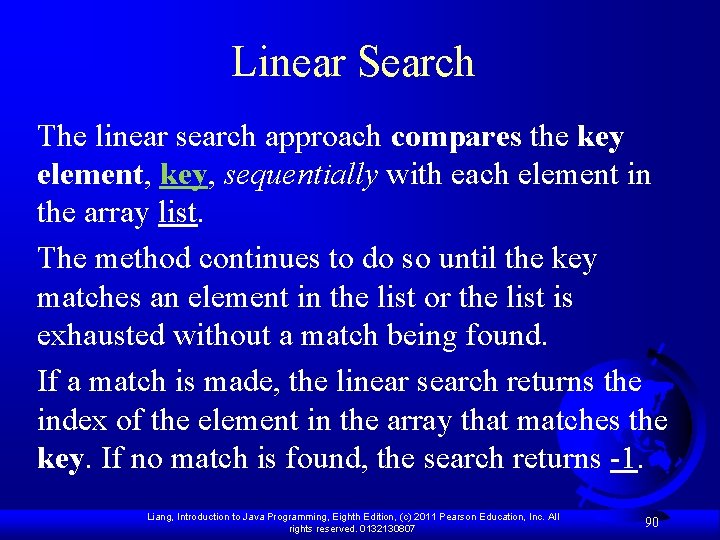
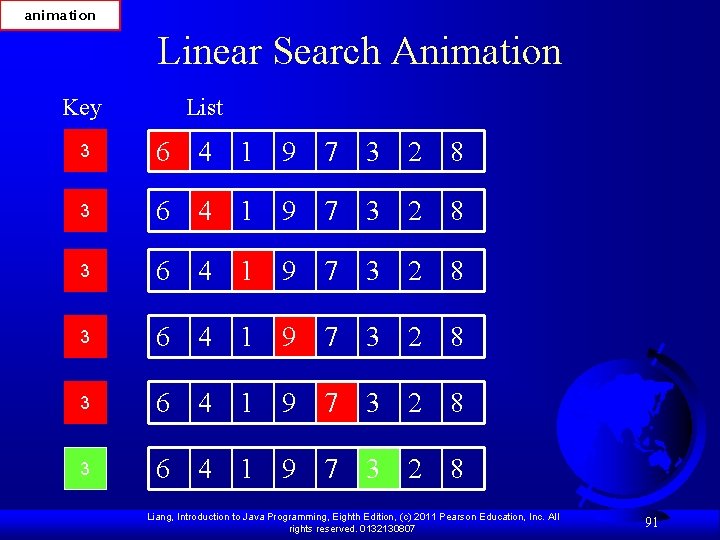
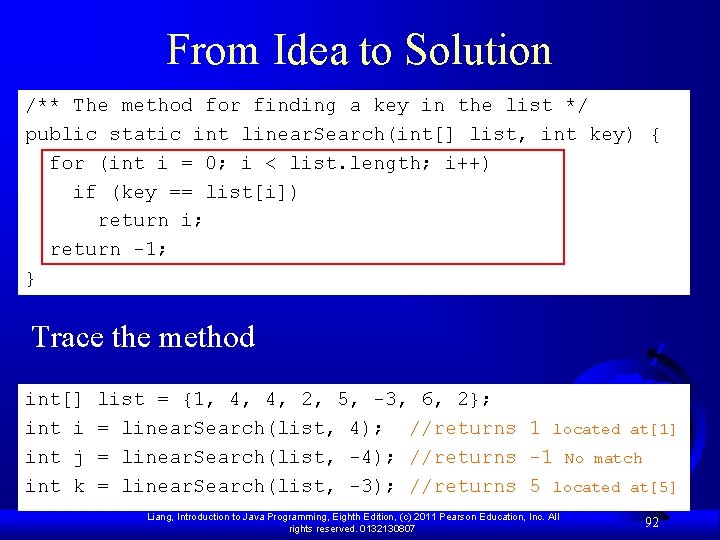
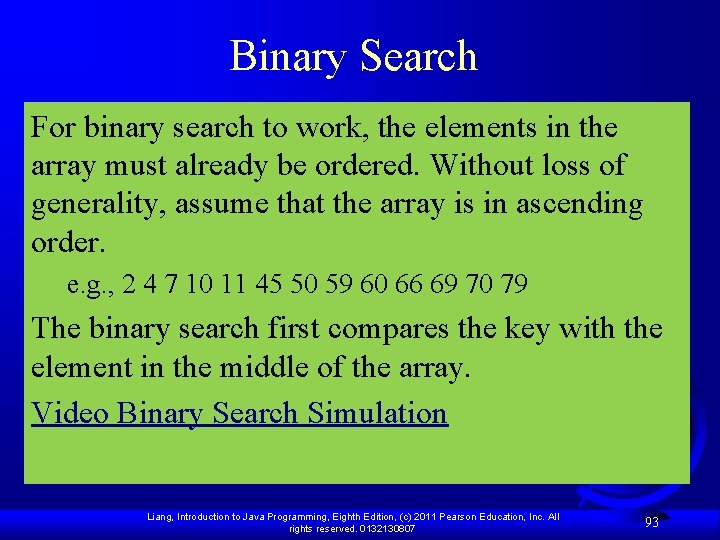
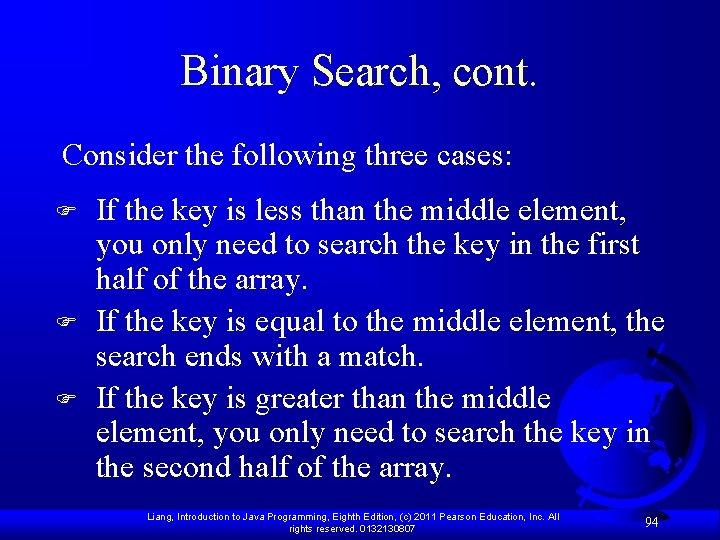
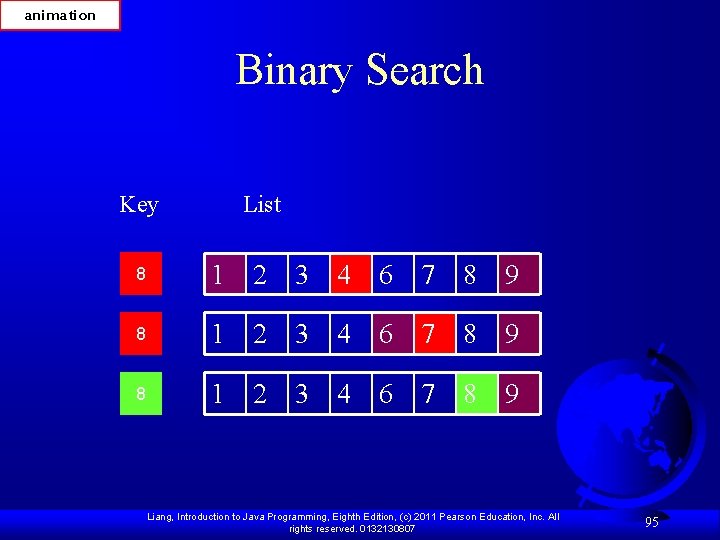
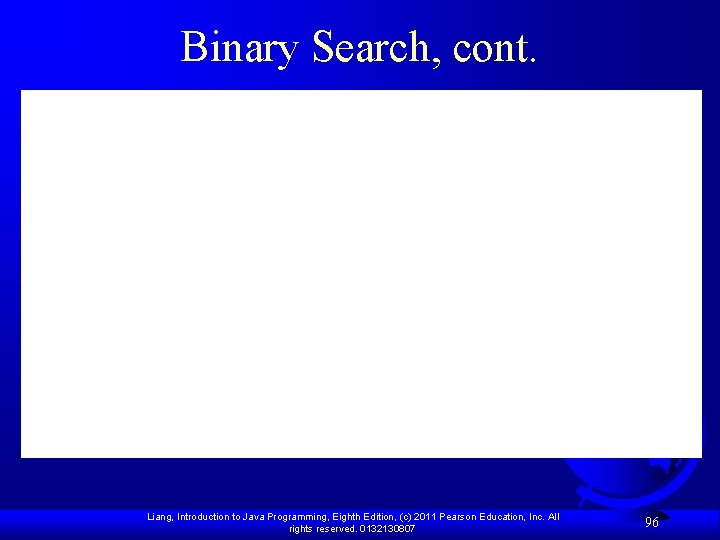
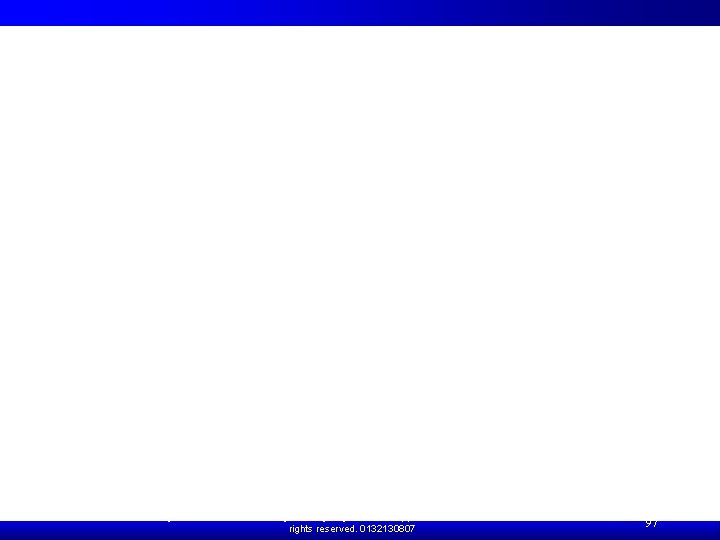
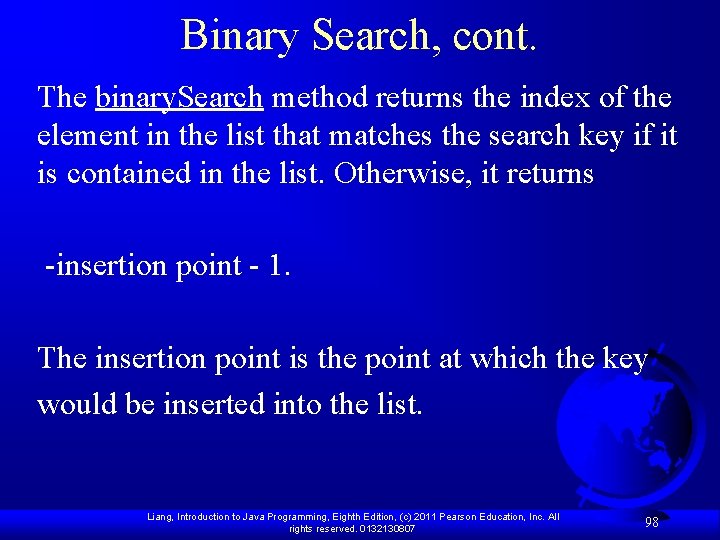
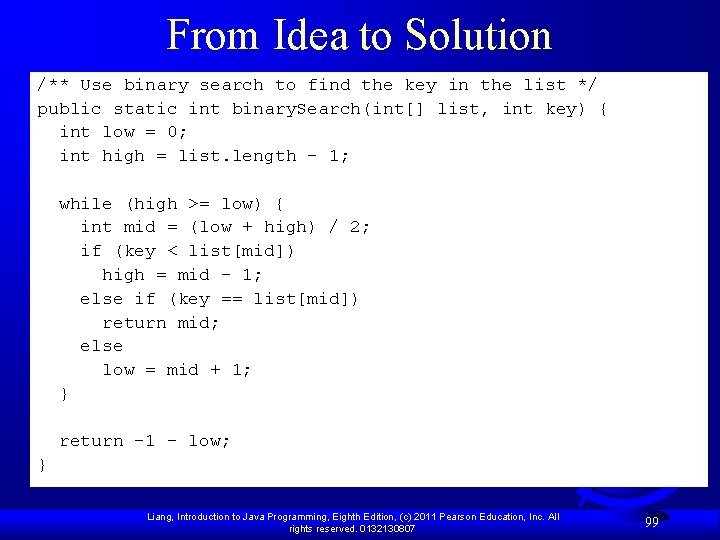
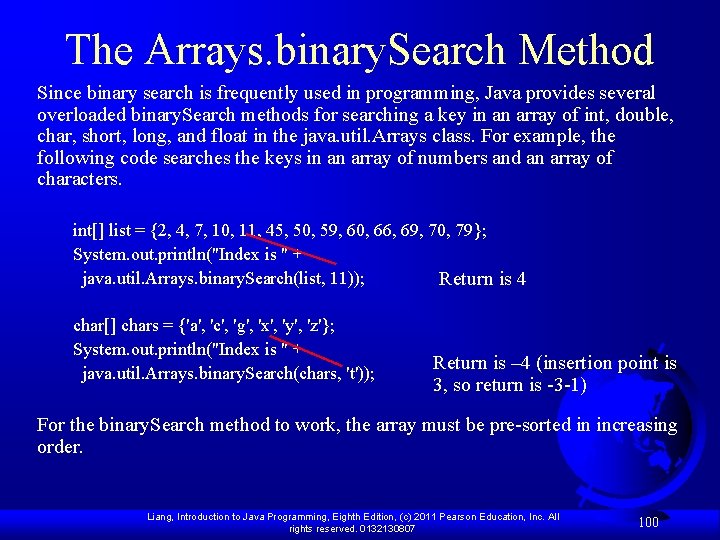
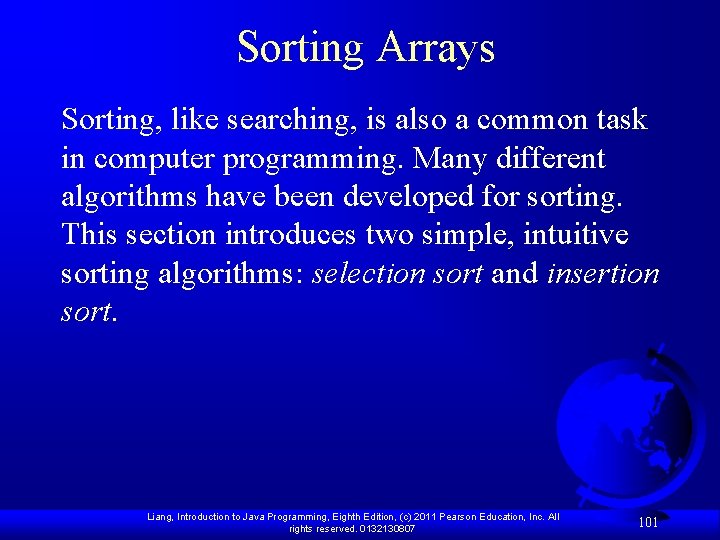
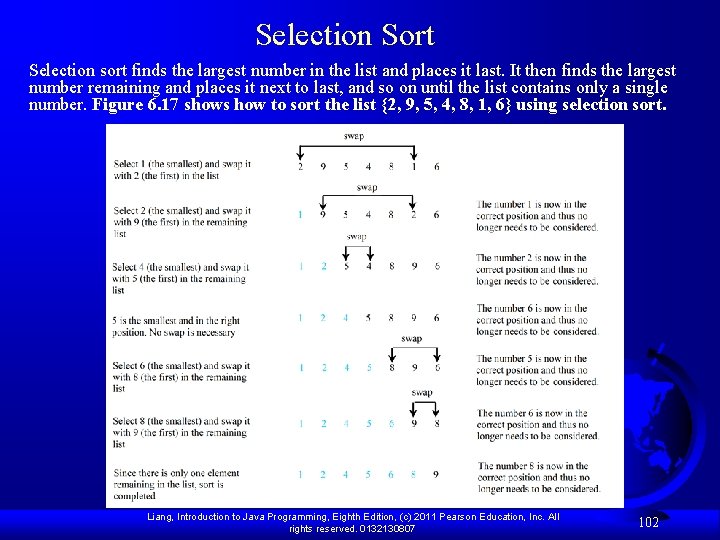
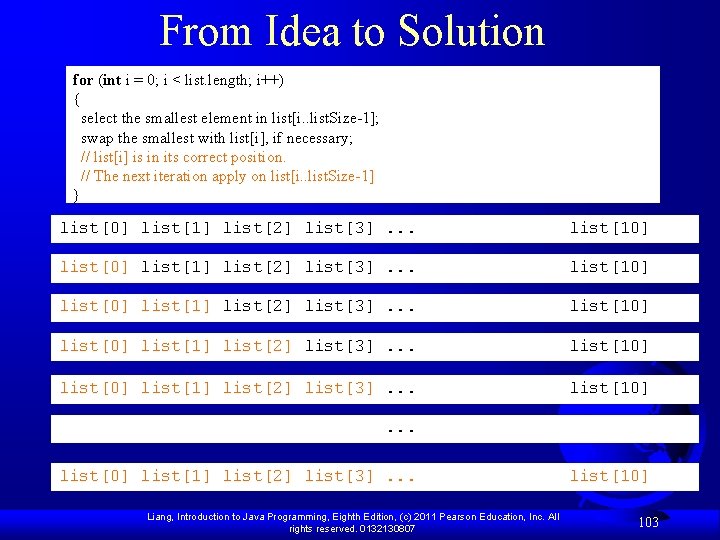
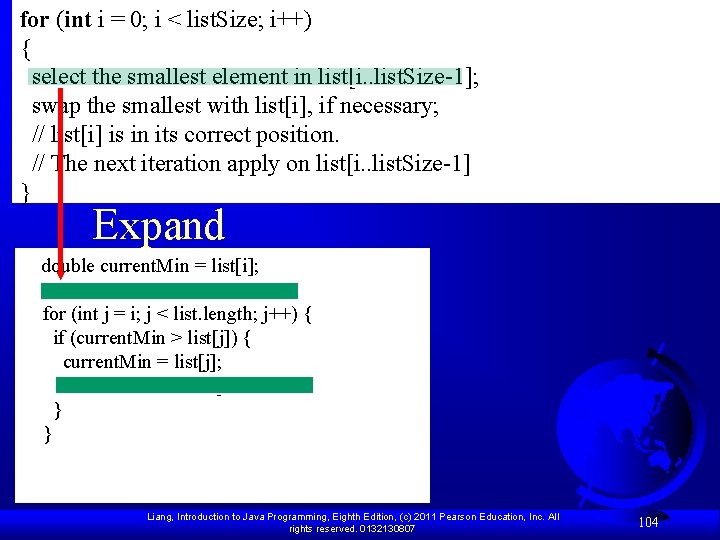
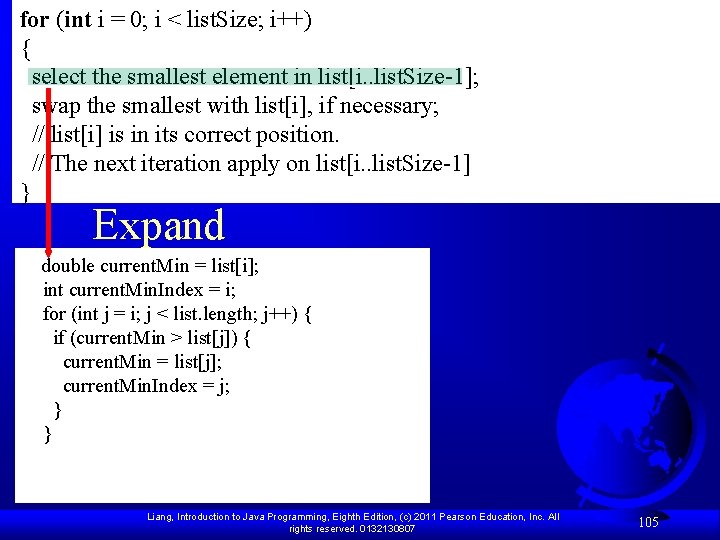
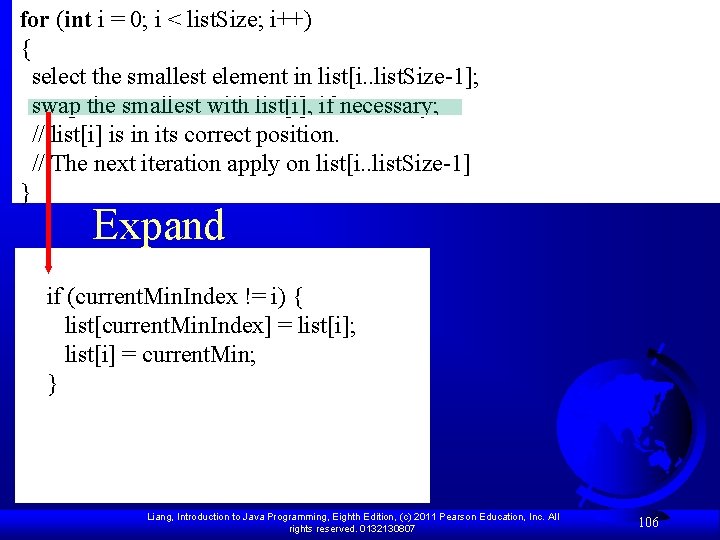
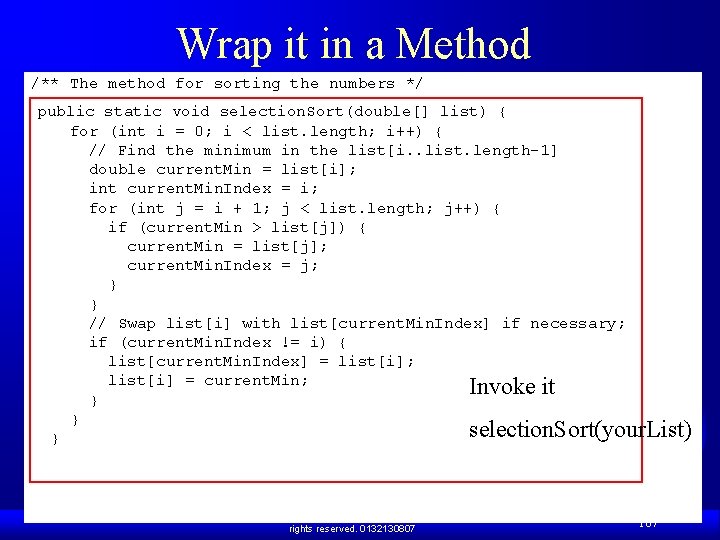
![Insertion Sort int[] my. List = {2, 9, 5, 4, 8, 1, 6}; // Insertion Sort int[] my. List = {2, 9, 5, 4, 8, 1, 6}; //](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-108.jpg)
![animation Insertion Sort int[] my. List = {2, 9, 5, 4, 8, 1, 6}; animation Insertion Sort int[] my. List = {2, 9, 5, 4, 8, 1, 6};](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-109.jpg)
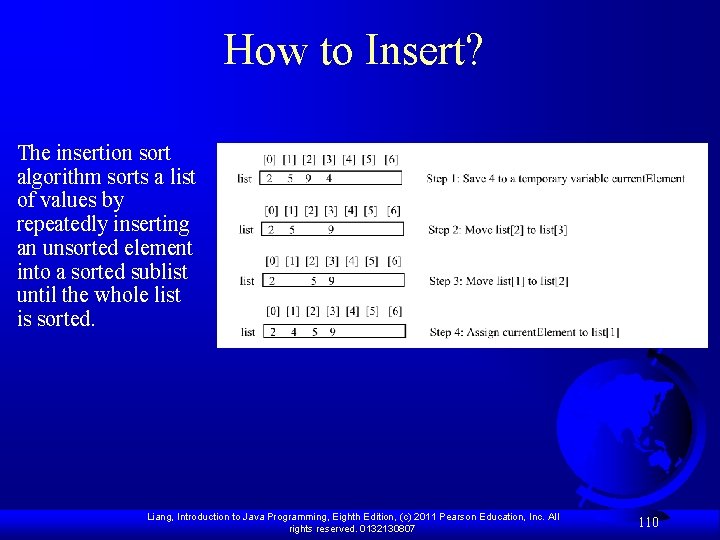
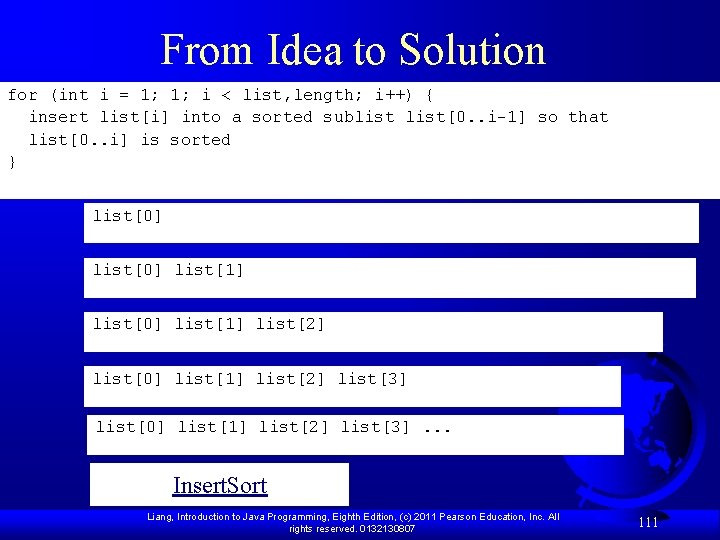
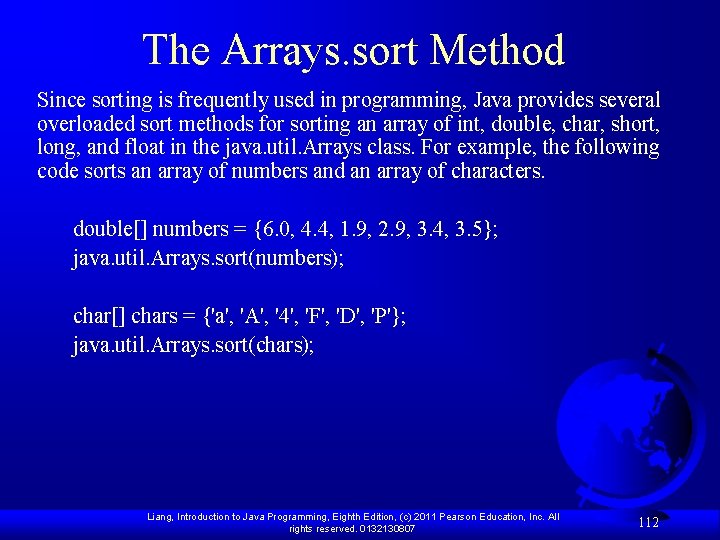
- Slides: 112
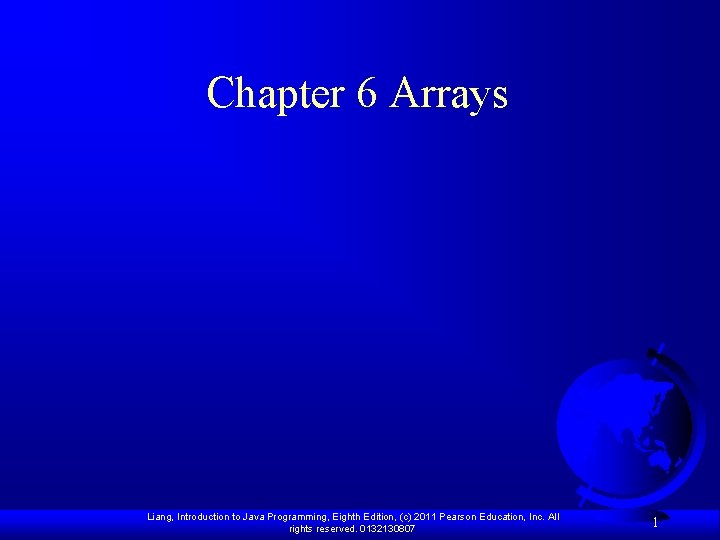
Chapter 6 Arrays Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 1
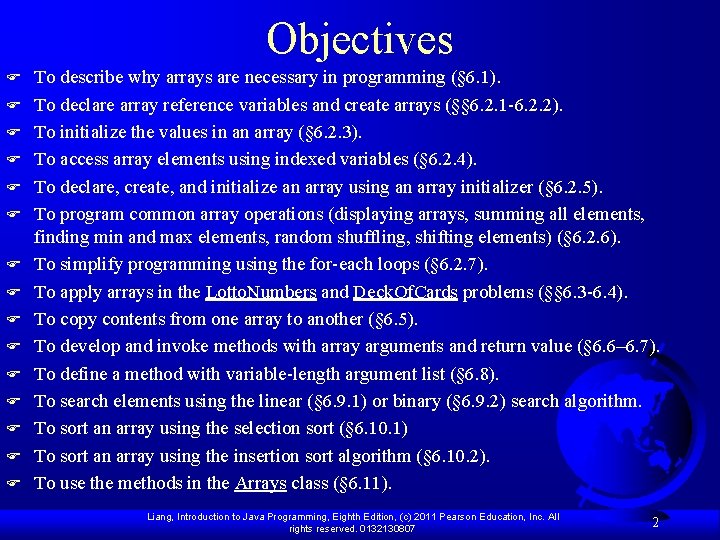
Objectives F F F F To describe why arrays are necessary in programming (§ 6. 1). To declare array reference variables and create arrays (§§ 6. 2. 1 -6. 2. 2). To initialize the values in an array (§ 6. 2. 3). To access array elements using indexed variables (§ 6. 2. 4). To declare, create, and initialize an array using an array initializer (§ 6. 2. 5). To program common array operations (displaying arrays, summing all elements, finding min and max elements, random shuffling, shifting elements) (§ 6. 2. 6). To simplify programming using the for-each loops (§ 6. 2. 7). To apply arrays in the Lotto. Numbers and Deck. Of. Cards problems (§§ 6. 3 -6. 4). To copy contents from one array to another (§ 6. 5). To develop and invoke methods with array arguments and return value (§ 6. 6– 6. 7). To define a method with variable-length argument list (§ 6. 8). To search elements using the linear (§ 6. 9. 1) or binary (§ 6. 9. 2) search algorithm. To sort an array using the selection sort (§ 6. 10. 1) To sort an array using the insertion sort algorithm (§ 6. 10. 2). To use the methods in the Arrays class (§ 6. 11). Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 2
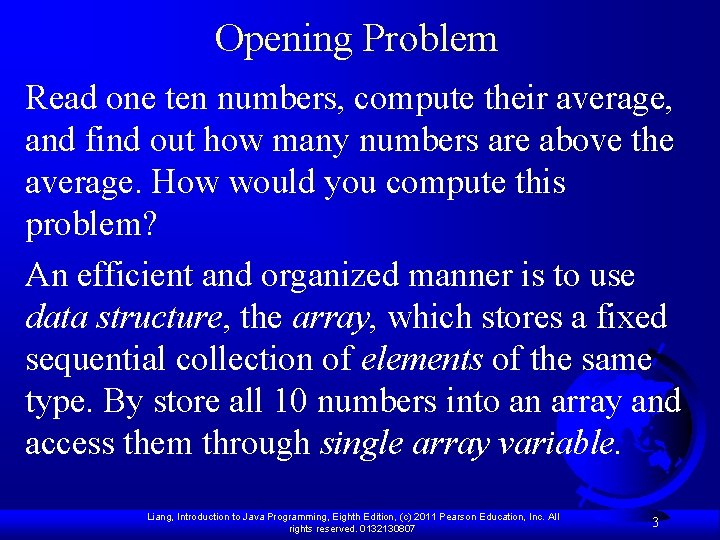
Opening Problem Read one ten numbers, compute their average, and find out how many numbers are above the average. How would you compute this problem? An efficient and organized manner is to use data structure, the array, which stores a fixed sequential collection of elements of the same type. By store all 10 numbers into an array and access them through single array variable. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 3
![Solution public class Analyze Numbers public static void mainString args final int Solution public class Analyze. Numbers { public static void main(String[] args) { final int](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-4.jpg)
Solution public class Analyze. Numbers { public static void main(String[] args) { final int NUMBER_OF_ELEMENTS = 10; double[] numbers = new double[NUMBER_OF_ELEMENTS]; // Creates & Declare a array of 10 elements double sum = 0; java. util. Scanner input = new java. util. Scanner(System. in); for (int i = 0; i < NUMBER_OF_ELEMENTS; i++) { System. out. print("Enter a new number: "); numbers[i] = input. next. Double(); // store numbers into the array sum += numbers[i]; //add each number to sum } double average = sum / NUMBER_OF_ELEMENTS; //obtain the average int count = 0; // The number of elements above average for (int i = 0; i < NUMBER_OF_ELEMENTS; i++) // compares each number in the array if (numbers[i] > average) // compares each number in the array count++; System. out. println("Average is " + average); System. out. println("Number of elements above the average " + count); } } Run with prepared input Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 4
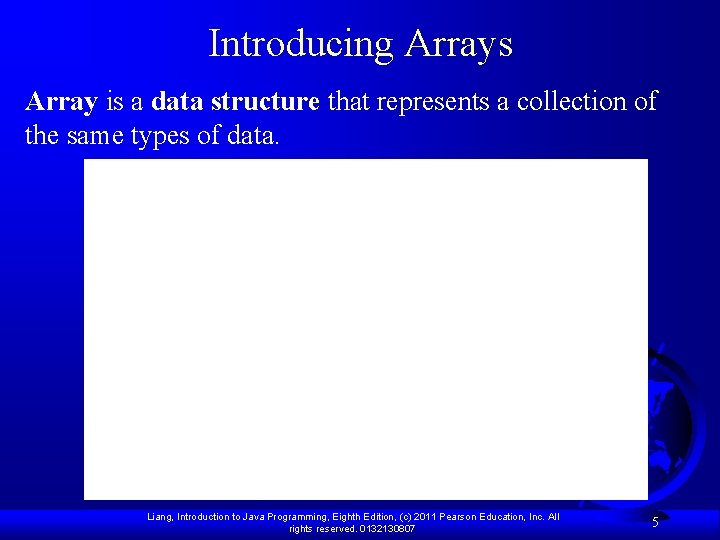
Introducing Arrays Array is a data structure that represents a collection of the same types of data. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 5
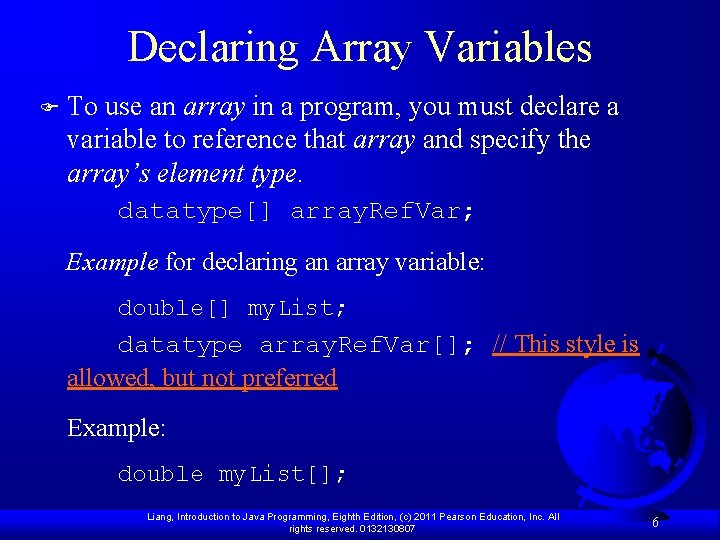
Declaring Array Variables F To use an array in a program, you must declare a variable to reference that array and specify the array’s element type. datatype[] array. Ref. Var; Example for declaring an array variable: double[] my. List; datatype array. Ref. Var[]; // This style is allowed, but not preferred Example: double my. List[]; Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 6
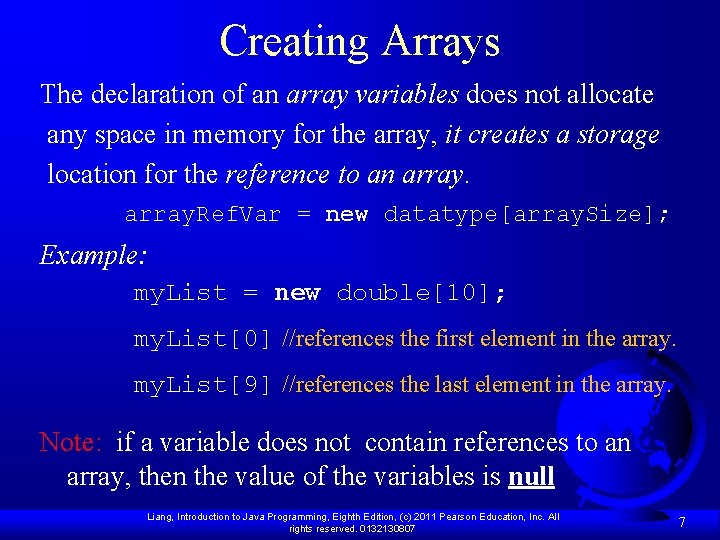
Creating Arrays The declaration of an array variables does not allocate any space in memory for the array, it creates a storage location for the reference to an array. Ref. Var = new datatype[array. Size]; Example: my. List = new double[10]; my. List[0] //references the first element in the array. my. List[9] //references the last element in the array. Note: if a variable does not contain references to an array, then the value of the variables is null Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 7
![Declaring and Creating in One Step datatypearray Ref Var new datatypearray Size F Declaring and Creating in One Step datatype[]array. Ref. Var = new datatype[array. Size]; F](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-8.jpg)
Declaring and Creating in One Step datatype[]array. Ref. Var = new datatype[array. Size]; F double[] my. List = new double[10]; //declare array name my. List as double F datatype array. Ref. Var[] = new datatype[array. Size]; //create and create Arrays double my. List[] = new double[10]; //declare and create array called my. List[] Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 8
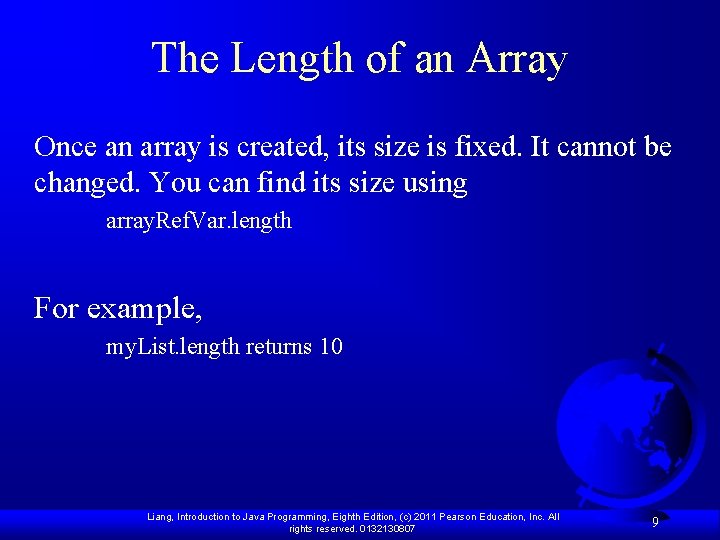
The Length of an Array Once an array is created, its size is fixed. It cannot be changed. You can find its size using array. Ref. Var. length For example, my. List. length returns 10 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 9
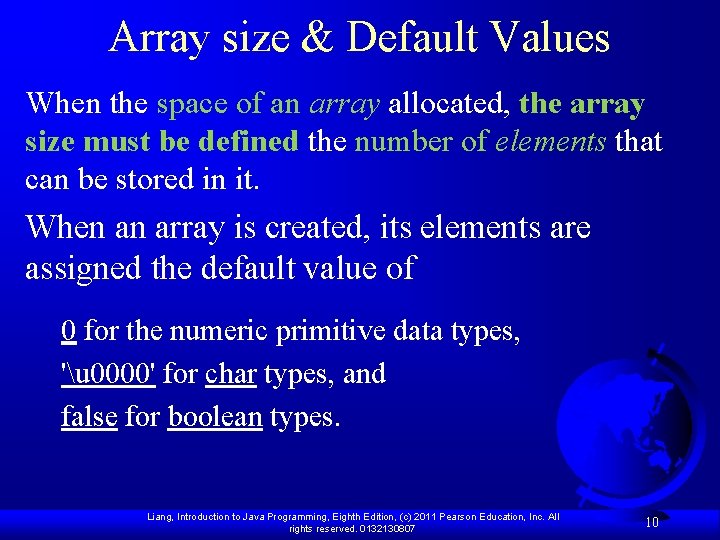
Array size & Default Values When the space of an array allocated, the array size must be defined the number of elements that can be stored in it. When an array is created, its elements are assigned the default value of 0 for the numeric primitive data types, 'u 0000' for char types, and false for boolean types. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 10
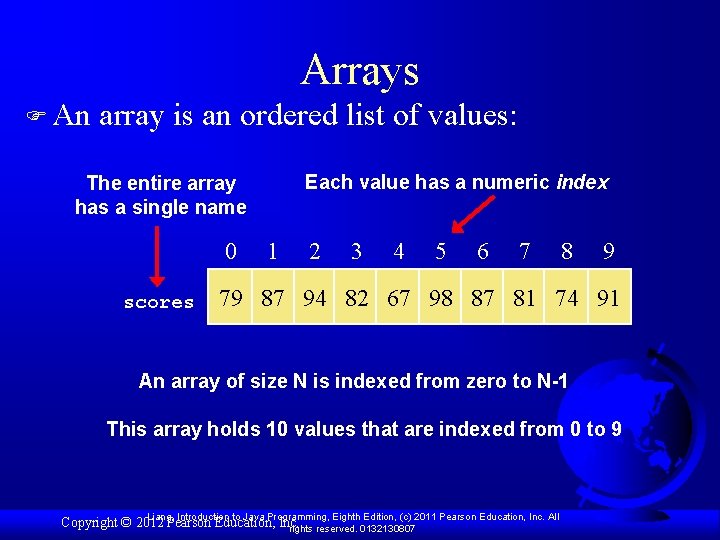
Arrays F An array is an ordered list of values: The entire array has a single name Each value has a numeric index 0 1 2 3 4 5 6 7 8 9 scores 79 87 94 82 67 98 87 81 74 91 An array of size N is indexed from zero to N-1 This array holds 10 values that are indexed from 0 to 9 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All Copyright © 2012 Pearson Education, Inc. rights reserved. 0132130807
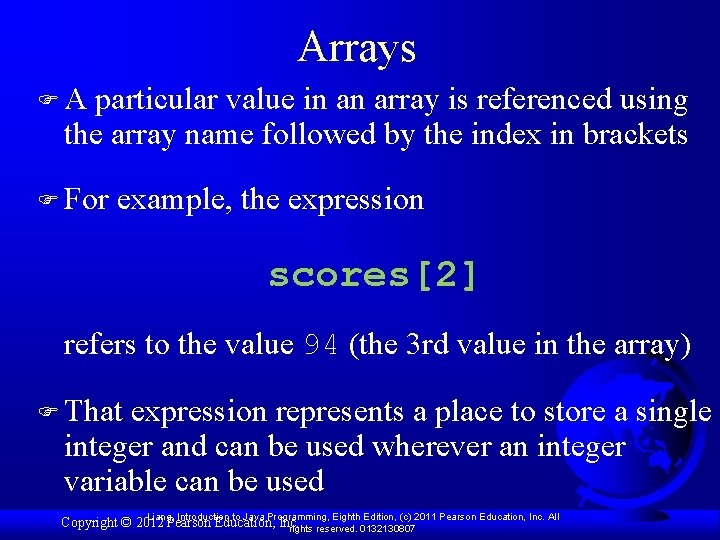
Arrays F A particular value in an array is referenced using the array name followed by the index in brackets F For example, the expression scores[2] refers to the value 94 (the 3 rd value in the array) F That expression represents a place to store a single integer and can be used wherever an integer variable can be used Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All Copyright © 2012 Pearson Education, Inc. rights reserved. 0132130807
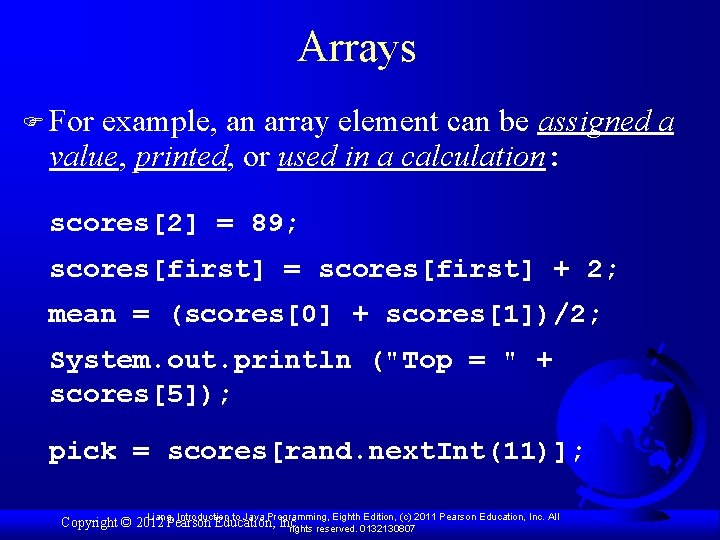
Arrays F For example, an array element can be assigned value, printed, or used in a calculation: scores[2] = 89; scores[first] = scores[first] + 2; mean = (scores[0] + scores[1])/2; System. out. println ("Top = " + scores[5]); pick = scores[rand. next. Int(11)]; Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All Copyright © 2012 Pearson Education, Inc. rights reserved. 0132130807 a
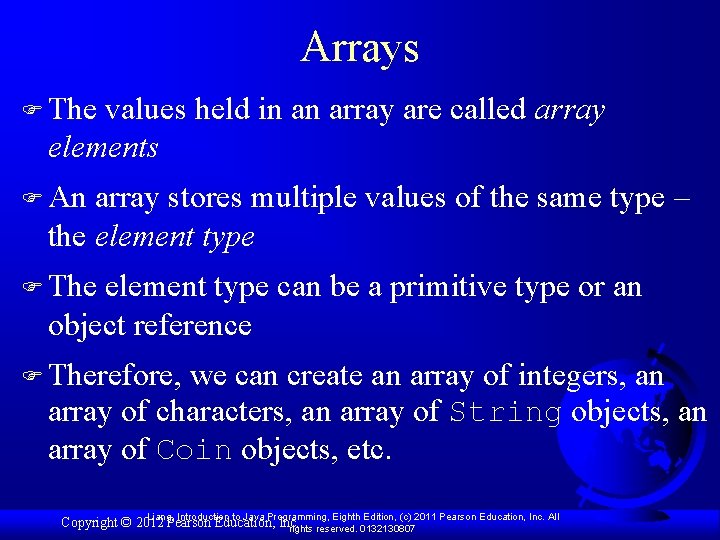
Arrays F The values held in an array are called array elements F An array stores multiple values of the same type – the element type F The element type can be a primitive type or an object reference F Therefore, we can create an array of integers, an array of characters, an array of String objects, an array of Coin objects, etc. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All Copyright © 2012 Pearson Education, Inc. rights reserved. 0132130807
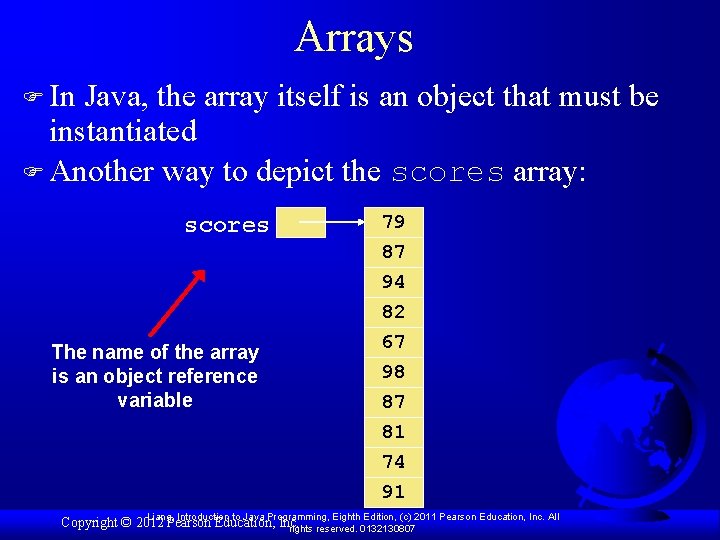
Arrays F In Java, the array itself is an object that must be instantiated F Another way to depict the scores array: scores 79 87 94 The name of the array is an object reference variable 82 67 98 87 81 74 91 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All Copyright © 2012 Pearson Education, Inc. rights reserved. 0132130807
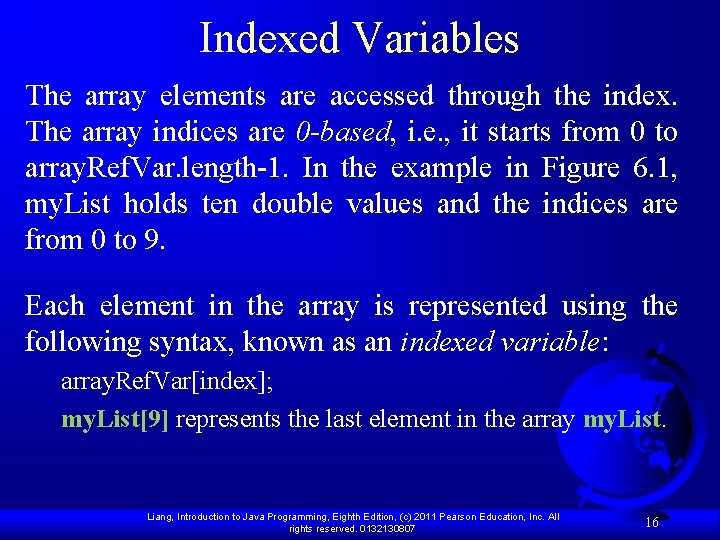
Indexed Variables The array elements are accessed through the index. The array indices are 0 -based, i. e. , it starts from 0 to array. Ref. Var. length-1. In the example in Figure 6. 1, my. List holds ten double values and the indices are from 0 to 9. Each element in the array is represented using the following syntax, known as an indexed variable: array. Ref. Var[index]; my. List[9] represents the last element in the array my. List. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 16
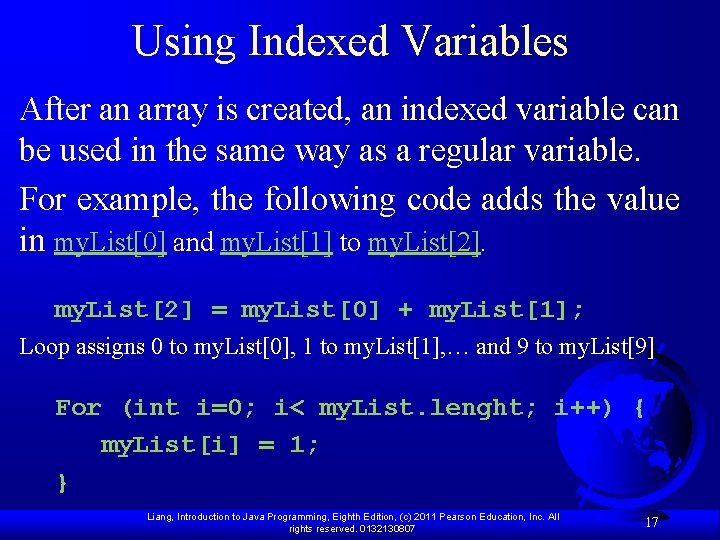
Using Indexed Variables After an array is created, an indexed variable can be used in the same way as a regular variable. For example, the following code adds the value in my. List[0] and my. List[1] to my. List[2] = my. List[0] + my. List[1]; Loop assigns 0 to my. List[0], 1 to my. List[1], … and 9 to my. List[9] For (int i=0; i< my. List. lenght; i++) { my. List[i] = 1; } Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 17
![Array Initializers double my List declare my List new double4 create Declaring creating Array Initializers double[] my. List; //declare my. List = new double[4]; //create Declaring, creating,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-18.jpg)
Array Initializers double[] my. List; //declare my. List = new double[4]; //create Declaring, creating, initializing in one step: double[] my. List = {1. 9, 2. 9, 3. 4, 3. 5}; This shorthand syntax must be in one statement. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 18
![Declaring creating initializing Using the Shorthand Notation double my List 1 9 2 Declaring, creating, initializing Using the Shorthand Notation double[] my. List = {1. 9, 2.](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-19.jpg)
Declaring, creating, initializing Using the Shorthand Notation double[] my. List = {1. 9, 2. 9, 3. 4, 3. 5}; This shorthand notation is equivalent to the following statements: double[] my. List = new double[4]; my. List[0] = 1. 9; my. List[1] = 2. 9; my. List[2] = 3. 4; my. List[3] = 3. 5; Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 19
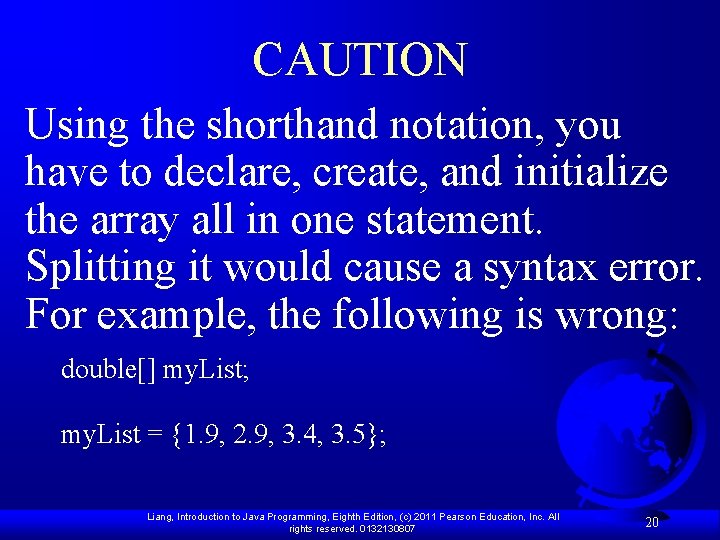
CAUTION Using the shorthand notation, you have to declare, create, and initialize the array all in one statement. Splitting it would cause a syntax error. For example, the following is wrong: double[] my. List; my. List = {1. 9, 2. 9, 3. 4, 3. 5}; Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 20
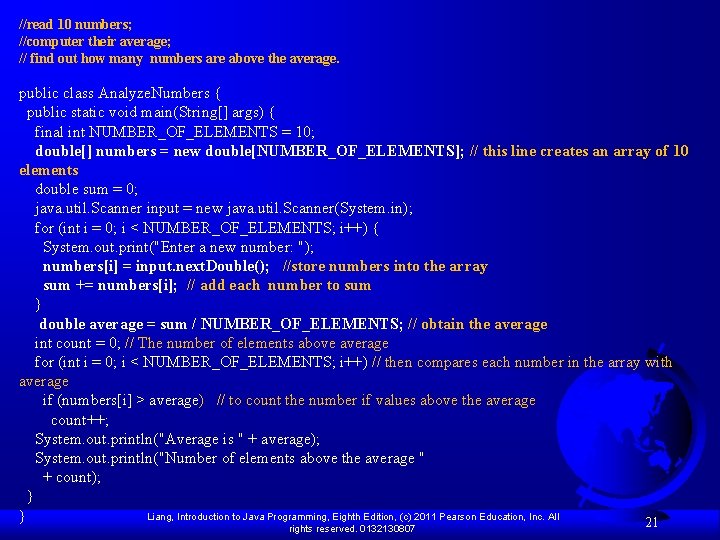
//read 10 numbers; //computer their average; // find out how many numbers are above the average. public class Analyze. Numbers { public static void main(String[] args) { final int NUMBER_OF_ELEMENTS = 10; double[] numbers = new double[NUMBER_OF_ELEMENTS]; // this line creates an array of 10 elements double sum = 0; java. util. Scanner input = new java. util. Scanner(System. in); for (int i = 0; i < NUMBER_OF_ELEMENTS; i++) { System. out. print("Enter a new number: "); numbers[i] = input. next. Double(); //store numbers into the array sum += numbers[i]; // add each number to sum } double average = sum / NUMBER_OF_ELEMENTS; // obtain the average int count = 0; // The number of elements above average for (int i = 0; i < NUMBER_OF_ELEMENTS; i++) // then compares each number in the array with average if (numbers[i] > average) // to count the number if values above the average count++; System. out. println("Average is " + average); System. out. println("Number of elements above the average " + count); } Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All } 21 rights reserved. 0132130807
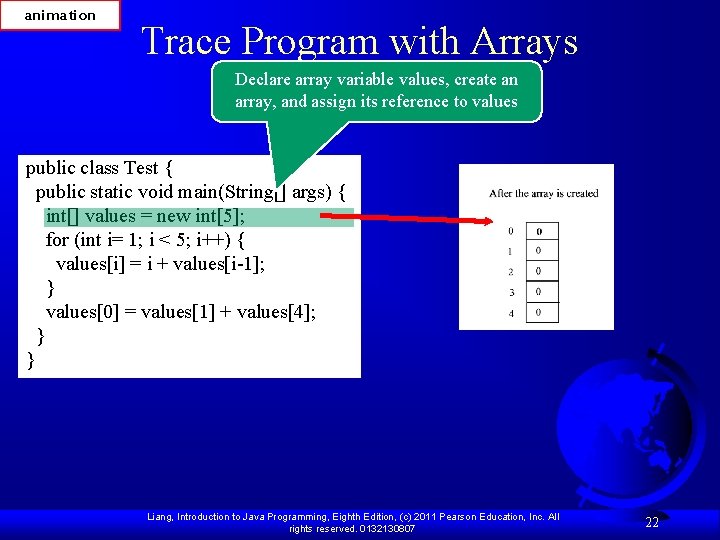
animation Trace Program with Arrays Declare array variable values, create an array, and assign its reference to values public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i= 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 22
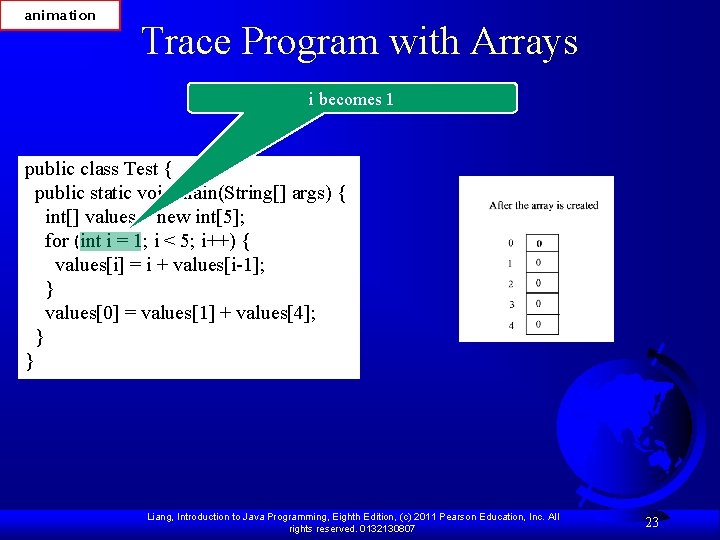
animation Trace Program with Arrays i becomes 1 public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 23
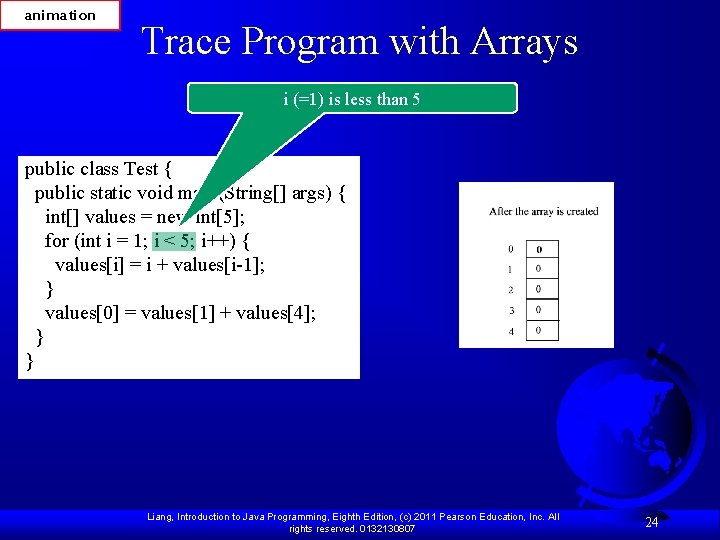
animation Trace Program with Arrays i (=1) is less than 5 public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 24
![animation Trace Program with Arrays After this line is executed value1 is 1 public animation Trace Program with Arrays After this line is executed, value[1] is 1 public](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-25.jpg)
animation Trace Program with Arrays After this line is executed, value[1] is 1 public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 25
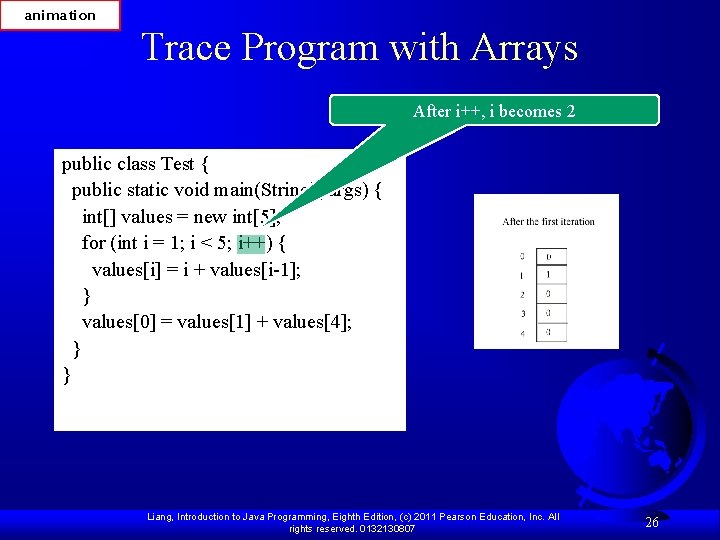
animation Trace Program with Arrays After i++, i becomes 2 public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 26
![animation Trace Program with Arrays public class Test public static void mainString args animation Trace Program with Arrays public class Test { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-27.jpg)
animation Trace Program with Arrays public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } i (= 2) is less than 5 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 27
![animation Trace Program with Arrays After this line is executed values2 is 3 2 animation Trace Program with Arrays After this line is executed, values[2] is 3 (2](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-28.jpg)
animation Trace Program with Arrays After this line is executed, values[2] is 3 (2 + 1) public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 28
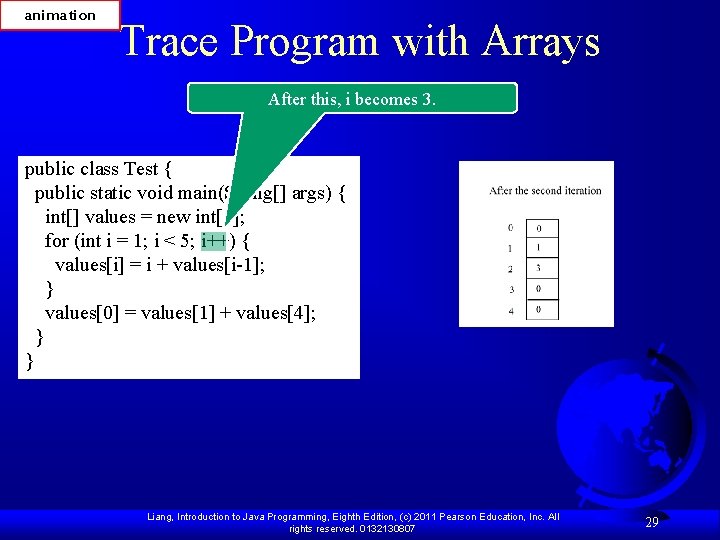
animation Trace Program with Arrays After this, i becomes 3. public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 29
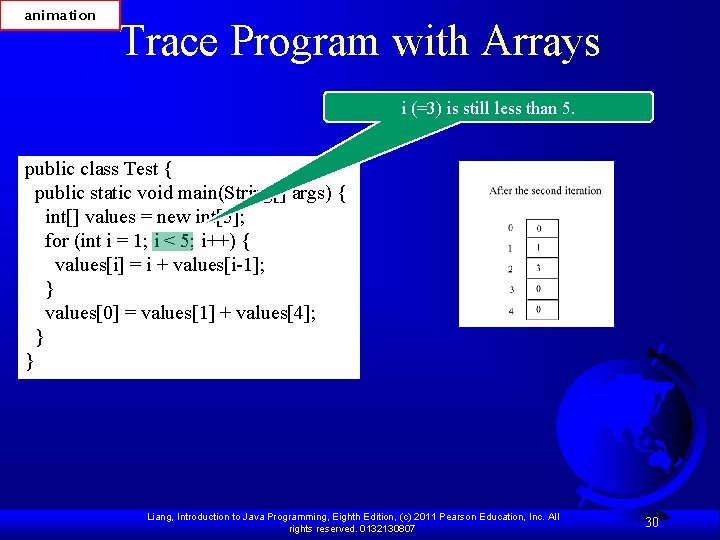
animation Trace Program with Arrays i (=3) is still less than 5. public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 30
![animation Trace Program with Arrays After this line values3 becomes 6 3 3 animation Trace Program with Arrays After this line, values[3] becomes 6 (3 + 3)](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-31.jpg)
animation Trace Program with Arrays After this line, values[3] becomes 6 (3 + 3) public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 31
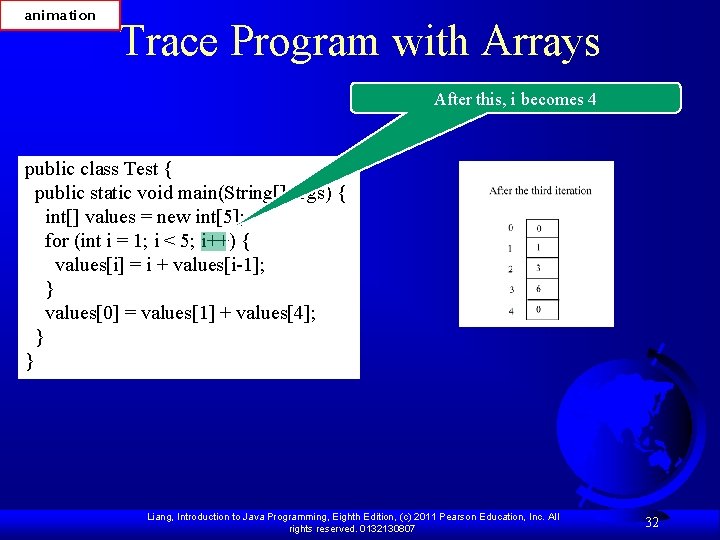
animation Trace Program with Arrays After this, i becomes 4 public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 32
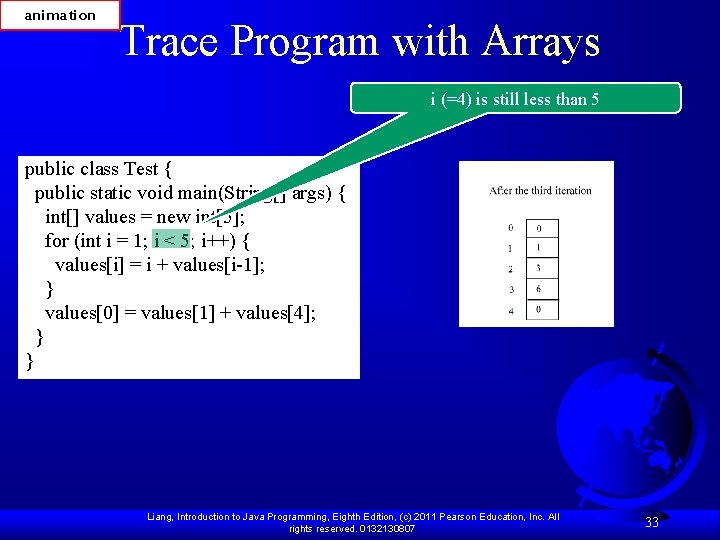
animation Trace Program with Arrays i (=4) is still less than 5 public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 33
![animation Trace Program with Arrays After this values4 becomes 10 4 6 public animation Trace Program with Arrays After this, values[4] becomes 10 (4 + 6) public](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-34.jpg)
animation Trace Program with Arrays After this, values[4] becomes 10 (4 + 6) public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 34
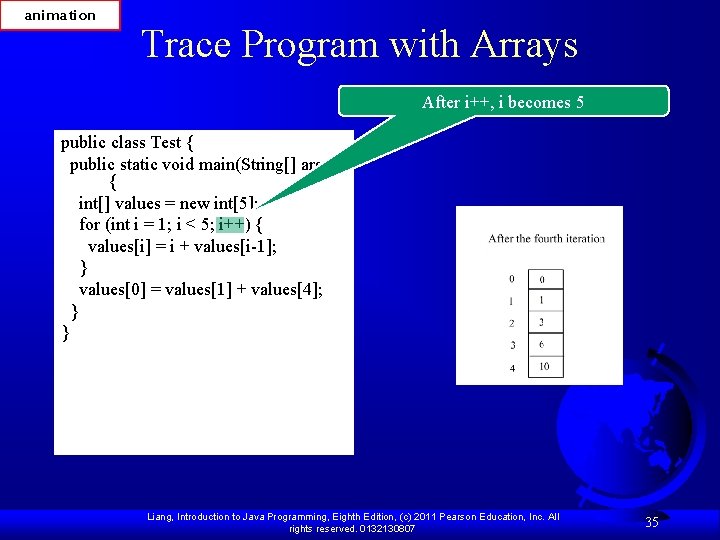
animation Trace Program with Arrays After i++, i becomes 5 public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 35
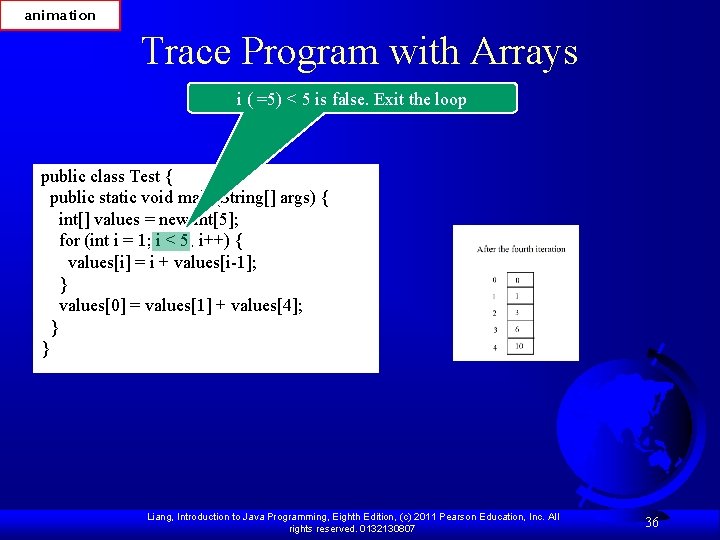
animation Trace Program with Arrays i ( =5) < 5 is false. Exit the loop public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 36
![animation Trace Program with Arrays After this line values0 is 11 1 10 animation Trace Program with Arrays After this line, values[0] is 11 (1 + 10)](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-37.jpg)
animation Trace Program with Arrays After this line, values[0] is 11 (1 + 10) public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 37
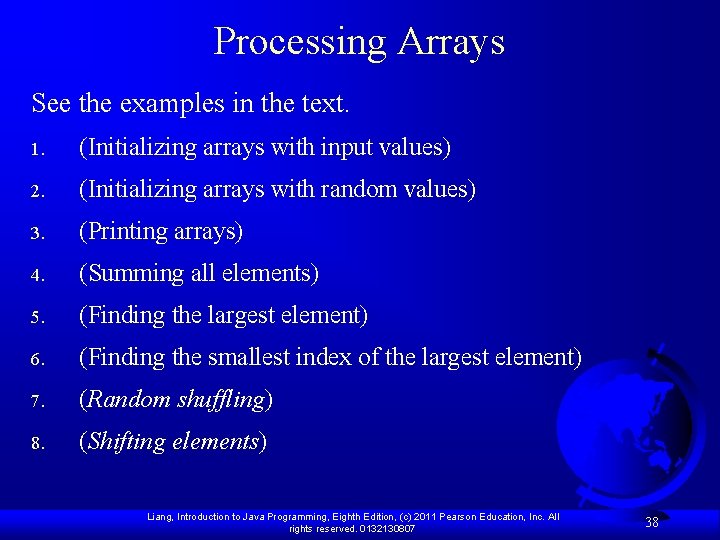
Processing Arrays See the examples in the text. 1. (Initializing arrays with input values) 2. (Initializing arrays with random values) 3. (Printing arrays) 4. (Summing all elements) 5. (Finding the largest element) 6. (Finding the smallest index of the largest element) 7. (Random shuffling) 8. (Shifting elements) Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 38
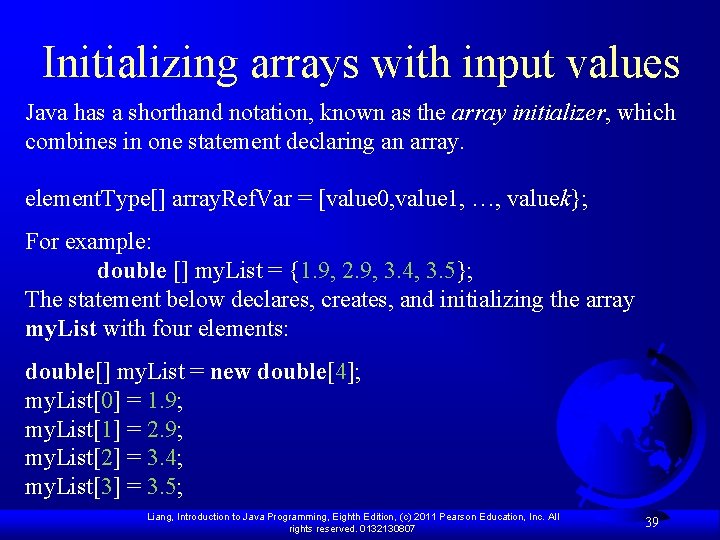
Initializing arrays with input values Java has a shorthand notation, known as the array initializer, which combines in one statement declaring an array. element. Type[] array. Ref. Var = [value 0, value 1, …, valuek}; For example: double [] my. List = {1. 9, 2. 9, 3. 4, 3. 5}; The statement below declares, creates, and initializing the array my. List with four elements: double[] my. List = new double[4]; my. List[0] = 1. 9; my. List[1] = 2. 9; my. List[2] = 3. 4; my. List[3] = 3. 5; Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 39
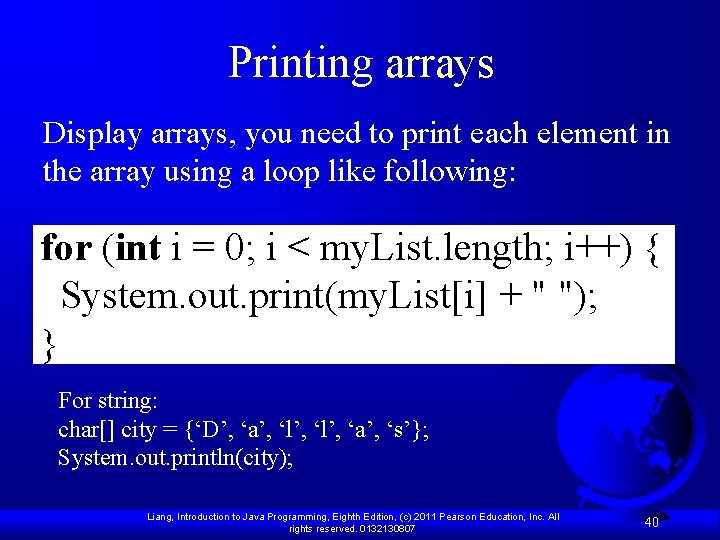
Printing arrays Display arrays, you need to print each element in the array using a loop like following: for (int i = 0; i < my. List. length; i++) { System. out. print(my. List[i] + " "); } For string: char[] city = {‘D’, ‘a’, ‘l’, ‘a’, ‘s’}; System. out. println(city); Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 40
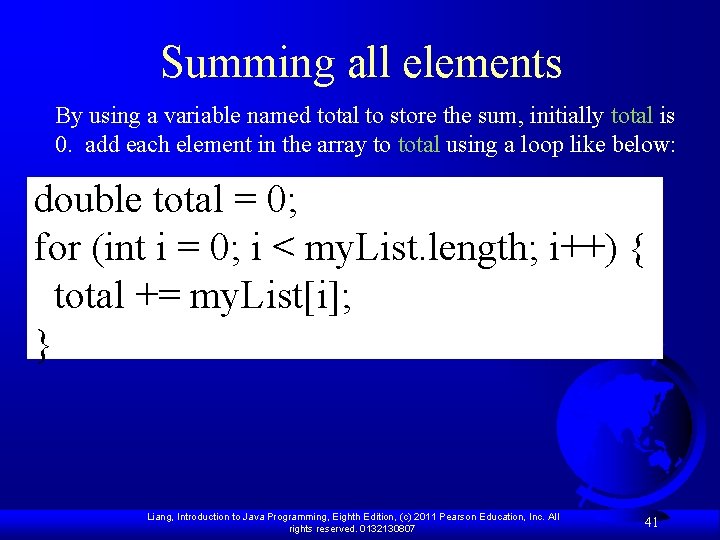
Summing all elements By using a variable named total to store the sum, initially total is 0. add each element in the array to total using a loop like below: double total = 0; for (int i = 0; i < my. List. length; i++) { total += my. List[i]; } Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 41
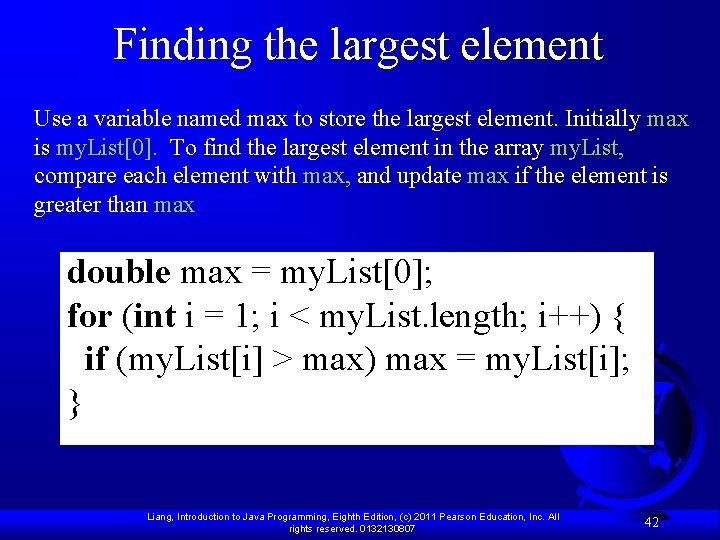
Finding the largest element Use a variable named max to store the largest element. Initially max is my. List[0]. To find the largest element in the array my. List, compare each element with max, and update max if the element is greater than max double max = my. List[0]; for (int i = 1; i < my. List. length; i++) { if (my. List[i] > max) max = my. List[i]; } Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 42
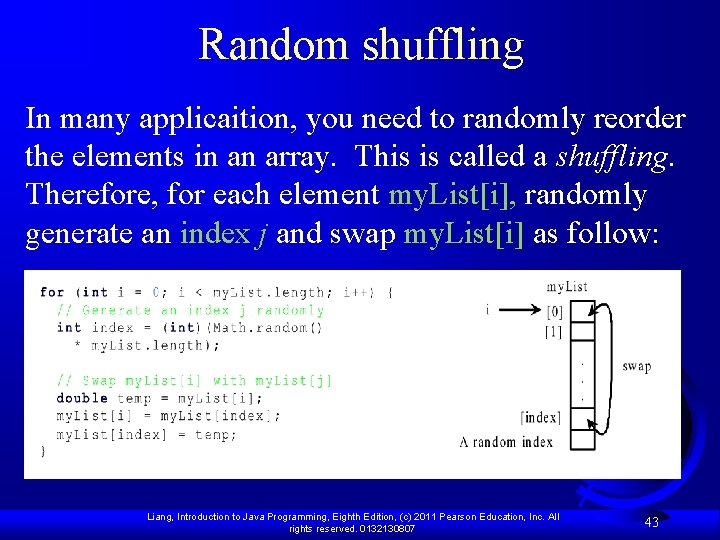
Random shuffling In many applicaition, you need to randomly reorder the elements in an array. This is called a shuffling. Therefore, for each element my. List[i], randomly generate an index j and swap my. List[i] as follow: Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 43
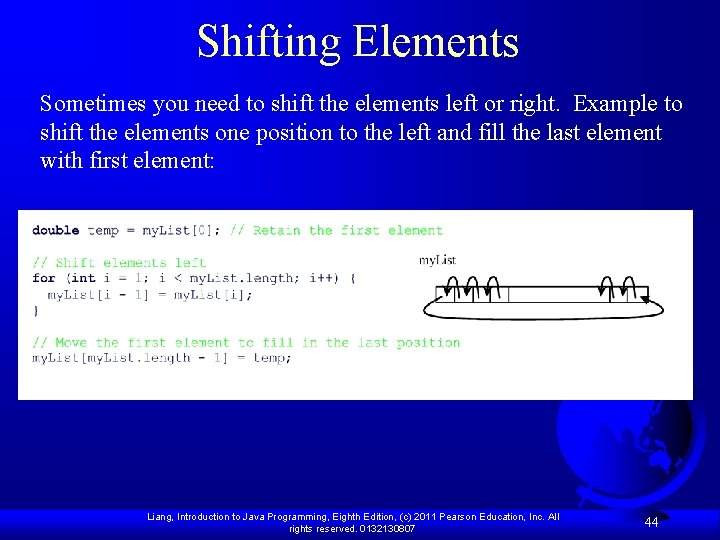
Shifting Elements Sometimes you need to shift the elements left or right. Example to shift the elements one position to the left and fill the last element with first element: Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 44
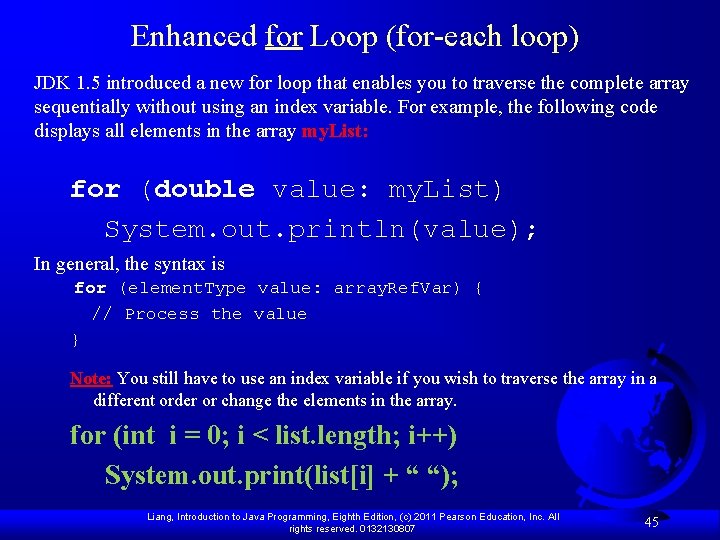
Enhanced for Loop (for-each loop) JDK 1. 5 introduced a new for loop that enables you to traverse the complete array sequentially without using an index variable. For example, the following code displays all elements in the array my. List: for (double value: my. List) System. out. println(value); In general, the syntax is for (element. Type value: array. Ref. Var) { // Process the value } Note: You still have to use an index variable if you wish to traverse the array in a different order or change the elements in the array. for (int i = 0; i < list. length; i++) System. out. print(list[i] + “ “); Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 45
![Enhanced For Loop F class Enhanced For Test 4 public static void mainString args Enhanced For Loop F class Enhanced. For. Test{ 4 public static void main(String[] args)](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-46.jpg)
Enhanced For Loop F class Enhanced. For. Test{ 4 public static void main(String[] args) 5 { 6 int[] array. List = {1, 12, 3, 4, 15, 6, 67}; 8 int total = 0; 9 10 // add each element's value to total F 11 for (int number : array. List ) 12 total += number; F F 13 //line List 11 & 12 equivalent to the following counter controlled repetition used in // line 14 & 15 14 //for (int counter =0; counter <array. List. length; counter++) 15 // total += array[counter]; 16 17 System. out. printf("Total of array elements: %dn" , total); 18 } 19 } Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 46
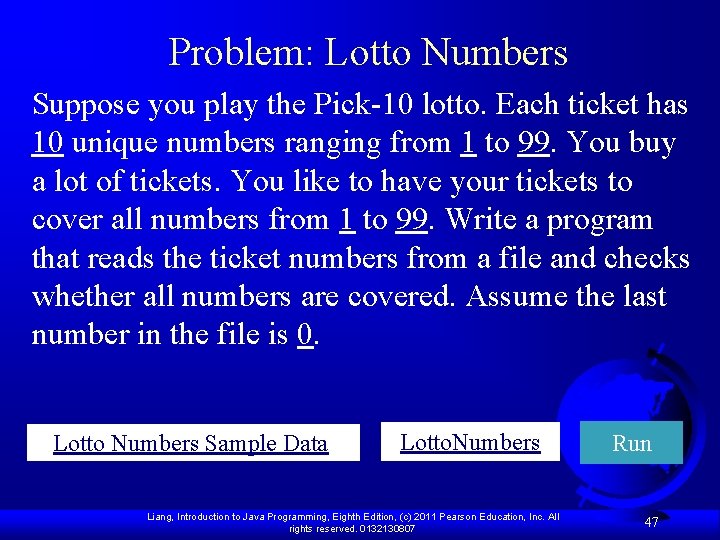
Problem: Lotto Numbers Suppose you play the Pick-10 lotto. Each ticket has 10 unique numbers ranging from 1 to 99. You buy a lot of tickets. You like to have your tickets to cover all numbers from 1 to 99. Write a program that reads the ticket numbers from a file and checks whether all numbers are covered. Assume the last number in the file is 0. Lotto Numbers Sample Data Lotto. Numbers Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 Run 47
![import java util Scanner public class Lotto Numbers public static void mainString args import java. util. Scanner; public class Lotto. Numbers { public static void main(String args[])](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-48.jpg)
import java. util. Scanner; public class Lotto. Numbers { public static void main(String args[]) { Scanner input = new Scanner(System. in); boolean[] is. Covered = new boolean[99]; // default false -- create and initialize array // Read all numbers and mark corresponding element covered -- read number int number = input. next. Int(); // read the number while (number != 0) { is. Covered[number - 1] = true; // mark number covered – “true” number = input. next. Int(); // read the number } // Check if all covered boolean all. Covered = true; // Assume all covered for (int i = 0; i < 99; i++) if (!is. Covered[i]) { all. Covered = false; // Find one number is not covered “false” break; } // Display result if (all. Covered) // check all. Covered? System. out. println("The tickets cover all numbers"); else System. out. println("The tickets don’t cover all numbers"); } } Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 48
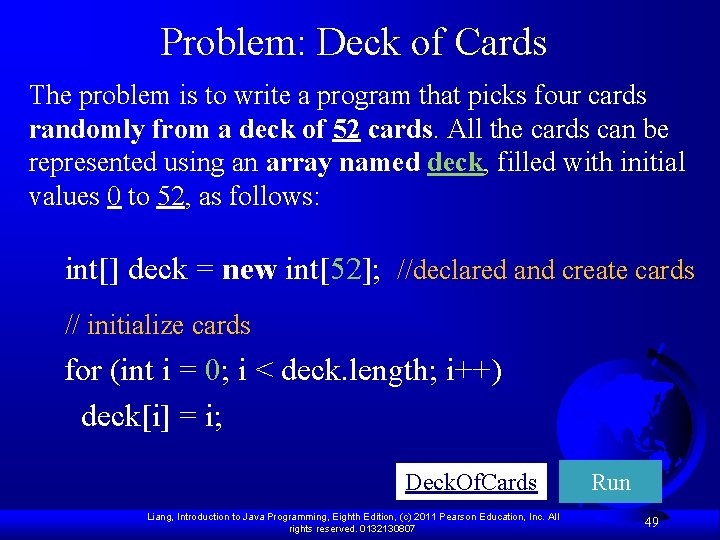
Problem: Deck of Cards The problem is to write a program that picks four cards randomly from a deck of 52 cards. All the cards can be represented using an array named deck, filled with initial values 0 to 52, as follows: int[] deck = new int[52]; //declared and create cards // initialize cards for (int i = 0; i < deck. length; i++) deck[i] = i; Deck. Of. Cards Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 Run 49
![public class Deck Of Cards public static void mainString args int deck public class Deck. Of. Cards { public static void main(String[] args) { int[] deck](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-50.jpg)
public class Deck. Of. Cards { public static void main(String[] args) { int[] deck = new int[52]; String[] suits = {"Spades", "Hearts", "Clubs", "Diamonds"}; // create array deck – array of string String[] ranks = {"Ace", "2", "3", "4", "5", "6", "7", "8", "9", // array of strings "10", "Jack", "Queen", "King"}; // Initialize deck for (int i = 0; i < deck. length; i++) deck[i] = i; // Shuffle the deck for (int i = 0; i < deck. length; i++) { // Generate an index randomly int index = (int)(Math. random() * deck. length); int temp = deck[i]; deck[i] = deck[index]; deck[index] = temp; } // Display the first four cards for (int i = 0; i < 4; i++) { String suit = suits[deck[i] / 13]; // suite of card String rank = ranks[deck[i] % 13]; // rank of a card System. out. println("Card number " + deck[i] + ": " + rank + " of " + suit); } Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All } 50 rights reserved. 0132130807 }
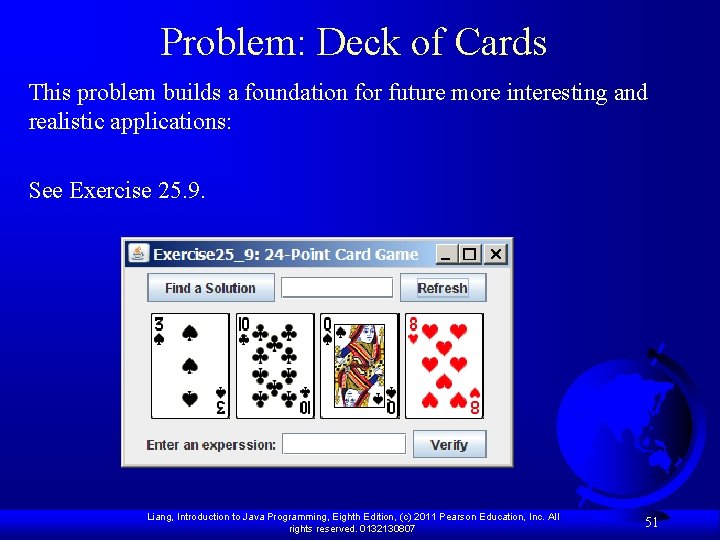
Problem: Deck of Cards This problem builds a foundation for future more interesting and realistic applications: See Exercise 25. 9. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 51
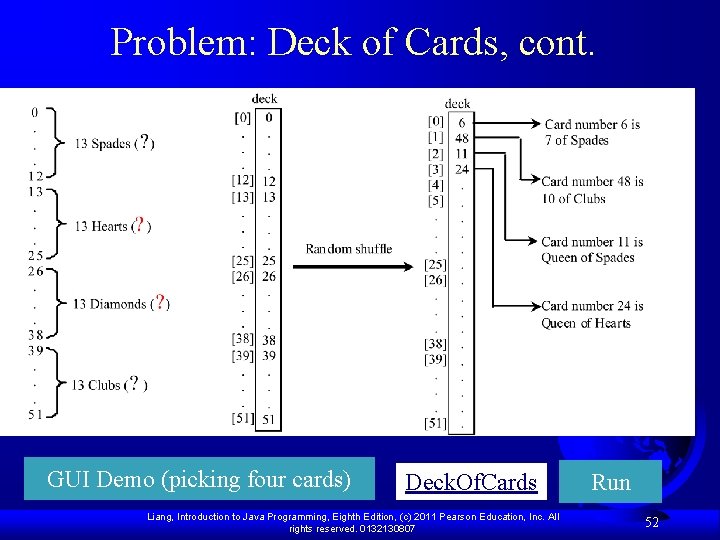
Problem: Deck of Cards, cont. GUI Demo (picking four cards) Deck. Of. Cards Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 Run 52
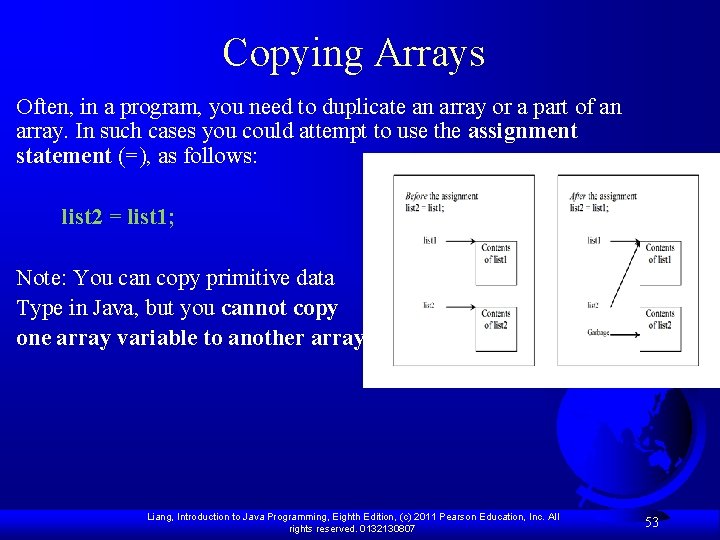
Copying Arrays Often, in a program, you need to duplicate an array or a part of an array. In such cases you could attempt to use the assignment statement (=), as follows: list 2 = list 1; Note: You can copy primitive data Type in Java, but you cannot copy one array variable to another array Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 53
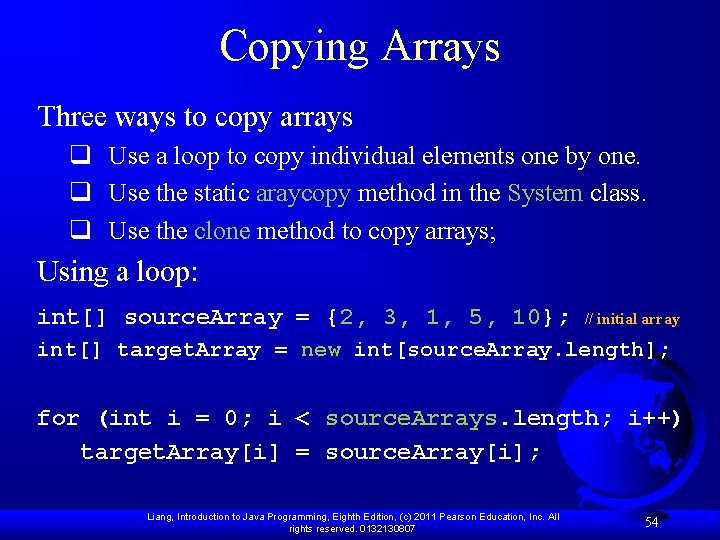
Copying Arrays Three ways to copy arrays q Use a loop to copy individual elements one by one. q Use the static araycopy method in the System class. q Use the clone method to copy arrays; Using a loop: int[] source. Array = {2, 3, 1, 5, 10}; // initial array int[] target. Array = new int[source. Array. length]; for (int i = 0; i < source. Arrays. length; i++) target. Array[i] = source. Array[i]; Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 54
![Passing Arrays to Methods public static void print Arrayint array for int i Passing Arrays to Methods public static void print. Array(int[] array) { for (int i](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-55.jpg)
Passing Arrays to Methods public static void print. Array(int[] array) { for (int i = 0; i < array. length; i++) { System. out. print(array[i] + " "); } } //Invoke the method int[] list = {3, 1, 2, 6, 4, 2}; print. Array(list); //initialize array //Invoke the method print. Array(new int[]{3, 1, 2, 6, 4, 2}); Anonymous array Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 55
![Anonymous Array The statement print Arraynew int3 1 2 6 4 2 creates an Anonymous Array The statement print. Array(new int[]{3, 1, 2, 6, 4, 2}); creates an](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-56.jpg)
Anonymous Array The statement print. Array(new int[]{3, 1, 2, 6, 4, 2}); creates an array using the following syntax: new data. Type[]{literal 0, literal 1, . . . , literalk}; There is no explicit reference variable for the array. Such array is called an anonymous array. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 56
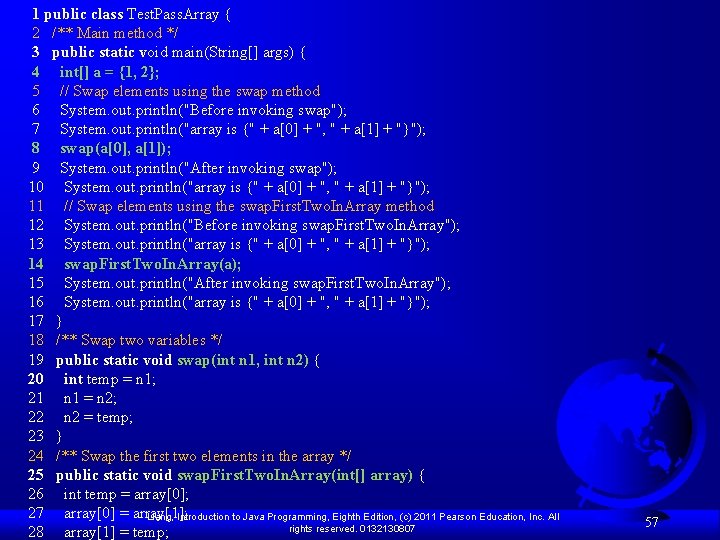
1 public class Test. Pass. Array { 2 /** Main method */ 3 public static void main(String[] args) { 4 int[] a = {1, 2}; 5 // Swap elements using the swap method 6 System. out. println("Before invoking swap"); 7 System. out. println("array is {" + a[0] + ", " + a[1] + "}"); 8 swap(a[0], a[1]); 9 System. out. println("After invoking swap"); 10 System. out. println("array is {" + a[0] + ", " + a[1] + "}"); 11 // Swap elements using the swap. First. Two. In. Array method 12 System. out. println("Before invoking swap. First. Two. In. Array"); 13 System. out. println("array is {" + a[0] + ", " + a[1] + "}"); 14 swap. First. Two. In. Array(a); 15 System. out. println("After invoking swap. First. Two. In. Array"); 16 System. out. println("array is {" + a[0] + ", " + a[1] + "}"); 17 } 18 /** Swap two variables */ 19 public static void swap(int n 1, int n 2) { 20 int temp = n 1; 21 n 1 = n 2; 22 n 2 = temp; 23 } 24 /** Swap the first two elements in the array */ 25 public static void swap. First. Two. In. Array(int[] array) { 26 int temp = array[0]; 27 array[0] = array[1]; Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 28 array[1] = temp; 57
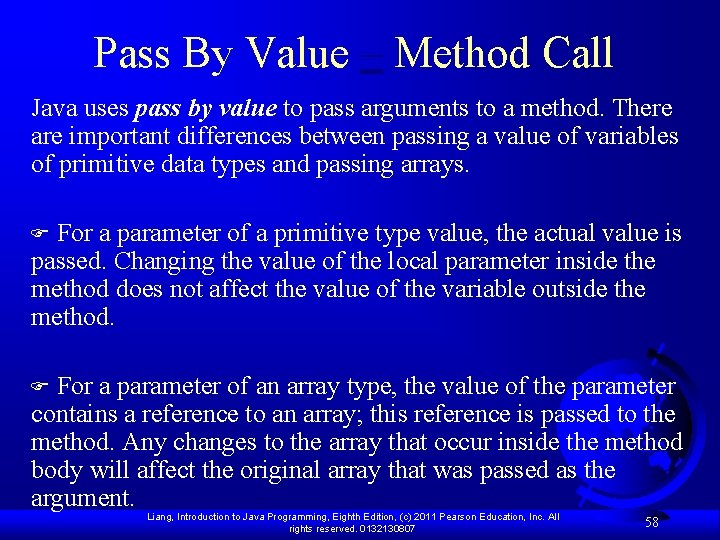
Pass By Value – Method Call Java uses pass by value to pass arguments to a method. There are important differences between passing a value of variables of primitive data types and passing arrays. F For a parameter of a primitive type value, the actual value is passed. Changing the value of the local parameter inside the method does not affect the value of the variable outside the method. F For a parameter of an array type, the value of the parameter contains a reference to an array; this reference is passed to the method. Any changes to the array that occur inside the method body will affect the original array that was passed as the argument. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 58
![Simple Example public class Test public static void mainString args int x Simple Example public class Test { public static void main(String[] args) { int x](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-59.jpg)
Simple Example public class Test { public static void main(String[] args) { int x = 1; // x represents an int value int[] y = new int[10]; // y represents an array of int values m(x, y); // Invoke m with arguments x and y System. out. println("x is " + x); System. out. println("y[0] is " + y[0]); } public static void m(int number, int[] numbers) { number = 1001; // Assign a new value to numbers[0] = 5555; // Assign a new value to numbers[0] } } Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 59
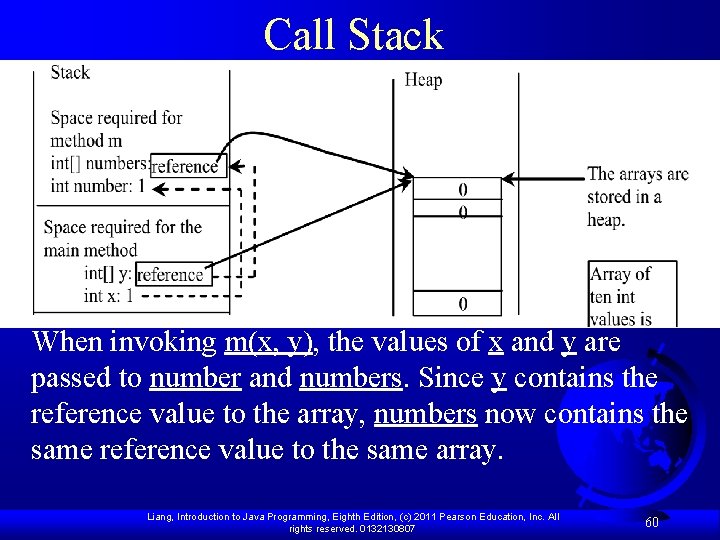
Call Stack When invoking m(x, y), the values of x and y are passed to number and numbers. Since y contains the reference value to the array, numbers now contains the same reference value to the same array. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 60
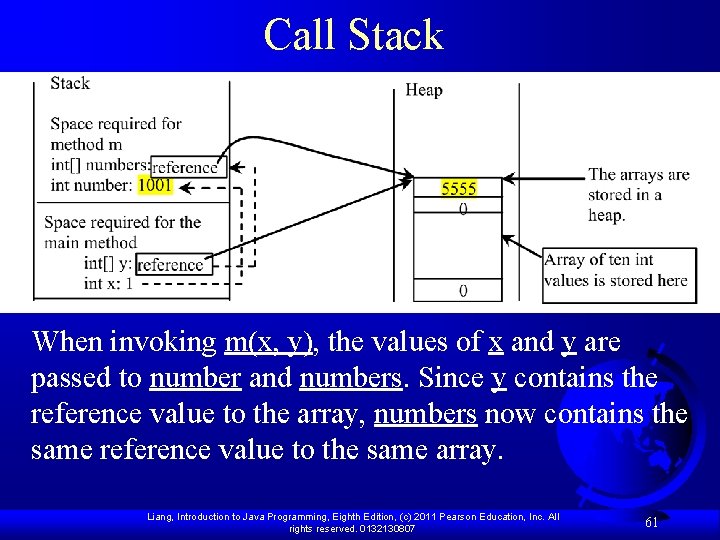
Call Stack When invoking m(x, y), the values of x and y are passed to number and numbers. Since y contains the reference value to the array, numbers now contains the same reference value to the same array. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 61
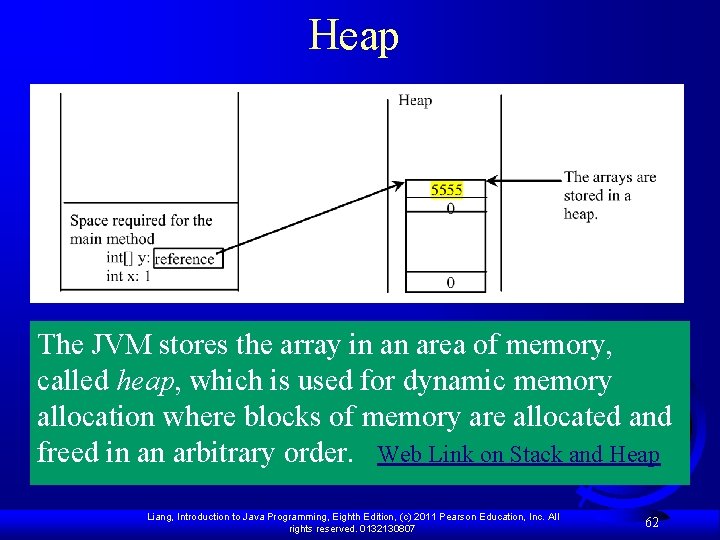
Heap The JVM stores the array in an area of memory, called heap, which is used for dynamic memory allocation where blocks of memory are allocated and freed in an arbitrary order. Web Link on Stack and Heap Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 62
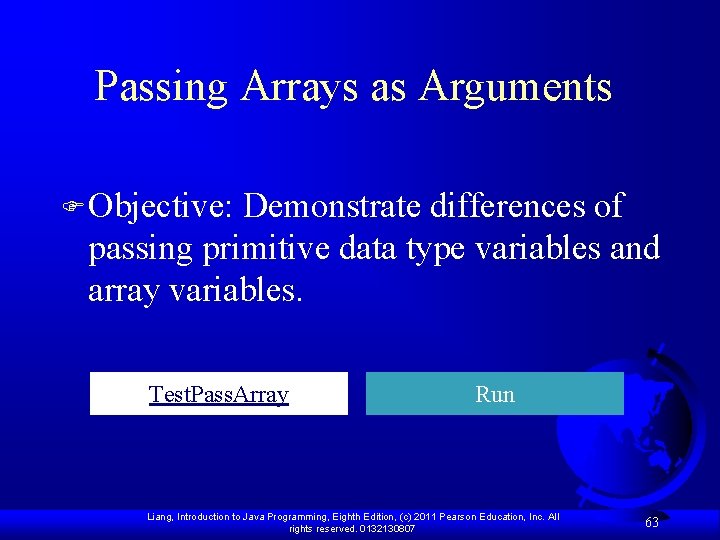
Passing Arrays as Arguments F Objective: Demonstrate differences of passing primitive data type variables and array variables. Test. Pass. Array Run Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 63
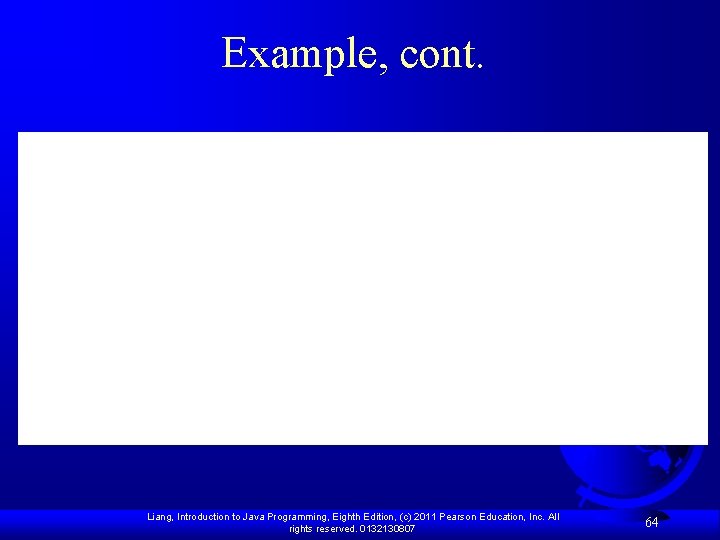
Example, cont. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 64
![Returning an Array from a Method public static int reverseint list int result Returning an Array from a Method public static int[] reverse(int[] list) { int[] result](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-65.jpg)
Returning an Array from a Method public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } list return result; } result int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; int[] list 2 = reverse(list 1); Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 65
![animation Trace the reverse Method int list 1 1 2 3 4 5 animation Trace the reverse Method int[] list 1 = {1, 2, 3, 4, 5,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-66.jpg)
animation Trace the reverse Method int[] list 1 = {1, 2, 3, 4, 5, 6}; int[] list 2 = reverse(list 1); Declare result and create array public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 1 2 3 4 5 6 0 0 0 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 66
![animation Trace the reverse Method cont int list 1 new int1 2 3 animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-67.jpg)
animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; int[] list 2 = reverse(list 1); i = 0 and j = 5 public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 1 2 3 4 5 6 0 0 0 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 67
![animation Trace the reverse Method cont int list 1 new int1 2 3 animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-68.jpg)
animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; int[] list 2 = reverse(list 1); i (= 0) is less than 6 public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 1 2 3 4 5 6 0 0 0 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 68
![animation Trace the reverse Method cont int list 1 new int1 2 3 animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-69.jpg)
animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; int[] list 2 = reverse(list 1); i = 0 and j = 5 Assign list[0] to result[5] public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 1 2 3 4 5 6 0 0 0 1 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 69
![animation Trace the reverse Method cont int list 1 new int1 2 3 animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-70.jpg)
animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; int[] list 2 = reverse(list 1); After this, i becomes 1 and j becomes 4 public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 1 2 3 4 5 6 0 0 0 1 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 70
![animation Trace the reverse Method cont int list 1 new int1 2 3 animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-71.jpg)
animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; int[] list 2 = reverse(list 1); i (=1) is less than 6 public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 1 2 3 4 5 6 0 0 0 1 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 71
![animation Trace the reverse Method cont int list 1 new int1 2 3 animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-72.jpg)
animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; int[] list 2 = reverse(list 1); i = 1 and j = 4 Assign list[1] to result[4] public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 1 2 3 4 5 6 0 0 2 1 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 72
![animation Trace the reverse Method cont int list 1 new int1 2 3 animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-73.jpg)
animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; int[] list 2 = reverse(list 1); After this, i becomes 2 and j becomes 3 public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 1 2 3 4 5 6 0 0 2 1 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 73
![animation Trace the reverse Method cont int list 1 new int1 2 3 animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-74.jpg)
animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; int[] list 2 = reverse(list 1); i (=2) is still less than 6 public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 1 2 3 4 5 6 0 0 2 1 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 74
![animation Trace the reverse Method cont int list 1 new int1 2 3 animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-75.jpg)
animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; int[] list 2 = reverse(list 1); i = 2 and j = 3 Assign list[i] to result[j] public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 1 2 3 4 5 6 0 0 0 3 2 1 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 75
![animation Trace the reverse Method cont int list 1 new int1 2 3 animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-76.jpg)
animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; int[] list 2 = reverse(list 1); After this, i becomes 3 and j becomes 2 public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 1 2 3 4 5 6 0 0 0 3 2 1 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 76
![animation Trace the reverse Method cont int list 1 new int1 2 3 animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-77.jpg)
animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; int[] list 2 = reverse(list 1); i (=3) is still less than 6 public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 1 2 3 4 5 6 0 0 0 3 2 1 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 77
![animation Trace the reverse Method cont int list 1 new int1 2 3 animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-78.jpg)
animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; int[] list 2 = reverse(list 1); i = 3 and j = 2 Assign list[i] to result[j] public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 1 2 3 4 5 6 0 0 4 3 2 1 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 78
![animation Trace the reverse Method cont int list 1 new int1 2 3 animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-79.jpg)
animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; int[] list 2 = reverse(list 1); After this, i becomes 4 and j becomes 1 public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 1 2 3 4 5 6 0 0 4 3 2 1 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 79
![animation Trace the reverse Method cont int list 1 new int1 2 3 animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-80.jpg)
animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; int[] list 2 = reverse(list 1); i (=4) is still less than 6 public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 1 2 3 4 5 6 0 0 4 3 2 1 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 80
![animation Trace the reverse Method cont int list 1 new int1 2 3 animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-81.jpg)
animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; int[] list 2 = reverse(list 1); i = 4 and j = 1 Assign list[i] to result[j] public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 1 2 3 4 5 6 0 5 4 3 2 1 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 81
![animation Trace the reverse Method cont int list 1 new int1 2 3 animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-82.jpg)
animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; int[] list 2 = reverse(list 1); After this, i becomes 5 and j becomes 0 public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 1 2 3 4 5 6 0 5 4 3 2 1 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 82
![animation Trace the reverse Method cont int list 1 new int1 2 3 animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-83.jpg)
animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; int[] list 2 = reverse(list 1); i (=5) is still less than 6 public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 1 2 3 4 5 6 0 5 4 3 2 1 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 83
![animation Trace the reverse Method cont int list 1 new int1 2 3 animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-84.jpg)
animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; int[] list 2 = reverse(list 1); i = 5 and j = 0 Assign list[i] to result[j] public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 1 2 3 4 5 6 6 5 4 3 2 1 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 84
![animation Trace the reverse Method cont int list 1 new int1 2 3 animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-85.jpg)
animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; int[] list 2 = reverse(list 1); After this, i becomes 6 and j becomes -1 public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 1 2 3 4 5 6 6 5 4 3 2 1 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 85
![animation Trace the reverse Method cont int list 1 new int1 2 3 animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-86.jpg)
animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; int[] list 2 = reverse(list 1); i (=6) < 6 is false. So exit the loop. public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 1 2 3 4 5 6 6 5 4 3 2 1 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 86
![animation Trace the reverse Method cont int list 1 new int1 2 3 animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3,](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-87.jpg)
animation Trace the reverse Method, cont. int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; int[] list 2 = reverse(list 1); Return result public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list 1 2 3 4 5 6 6 5 4 3 2 1 list 2 result Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 87
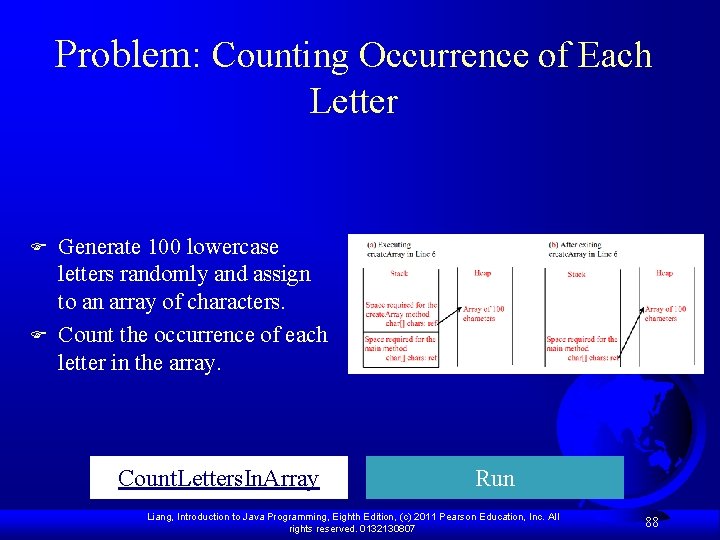
Problem: Counting Occurrence of Each Letter F F Generate 100 lowercase letters randomly and assign to an array of characters. Count the occurrence of each letter in the array. Count. Letters. In. Array Run Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 88
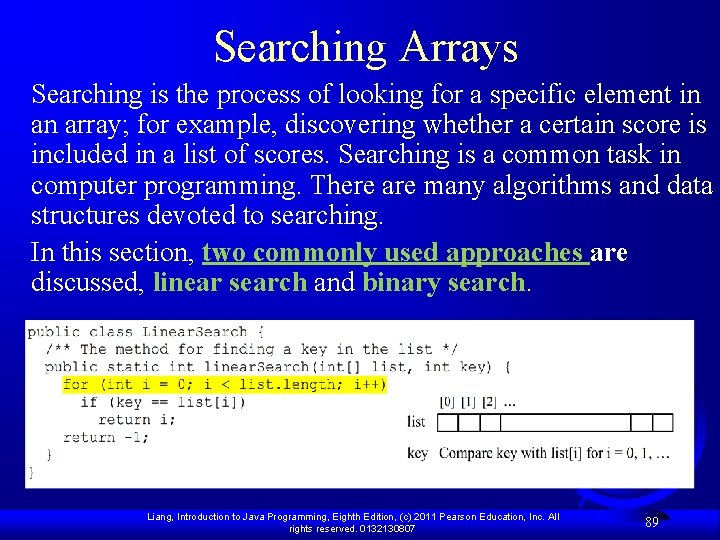
Searching Arrays Searching is the process of looking for a specific element in an array; for example, discovering whether a certain score is included in a list of scores. Searching is a common task in computer programming. There are many algorithms and data structures devoted to searching. In this section, two commonly used approaches are discussed, linear search and binary search. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 89
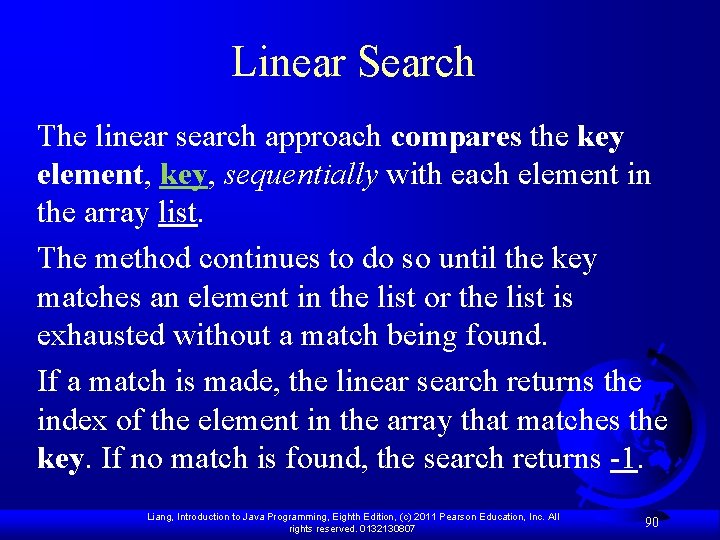
Linear Search The linear search approach compares the key element, key, sequentially with each element in the array list. The method continues to do so until the key matches an element in the list or the list is exhausted without a match being found. If a match is made, the linear search returns the index of the element in the array that matches the key. If no match is found, the search returns -1. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 90
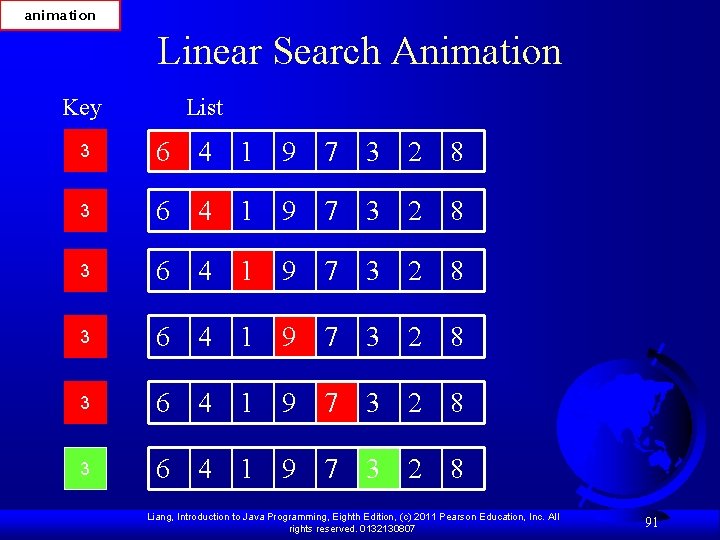
animation Linear Search Animation Key List 3 6 4 1 9 7 3 2 8 3 6 4 1 9 7 3 2 8 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 91
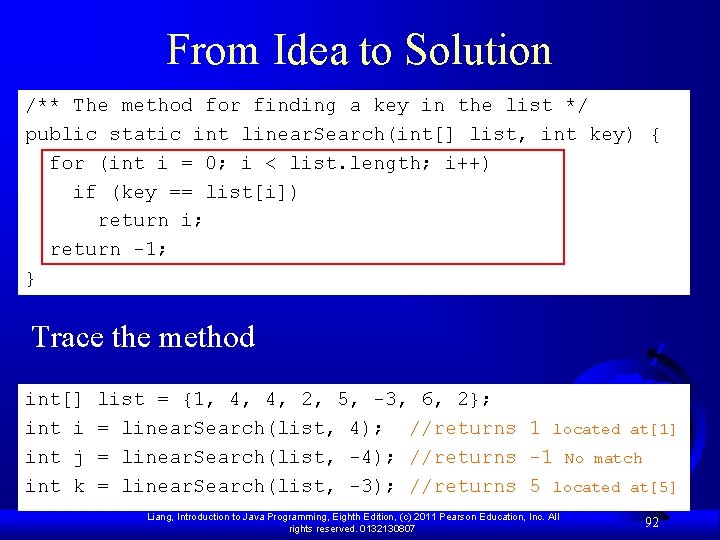
From Idea to Solution /** The method for finding a key in the list */ public static int linear. Search(int[] list, int key) { for (int i = 0; i < list. length; i++) if (key == list[i]) return i; return -1; } Trace the method int[] list = {1, 4, 4, 2, 5, -3, 6, 2}; int i = linear. Search(list, 4); //returns 1 located at[1] int j = linear. Search(list, -4); //returns -1 No match int k = linear. Search(list, -3); //returns 5 located at[5] Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 92
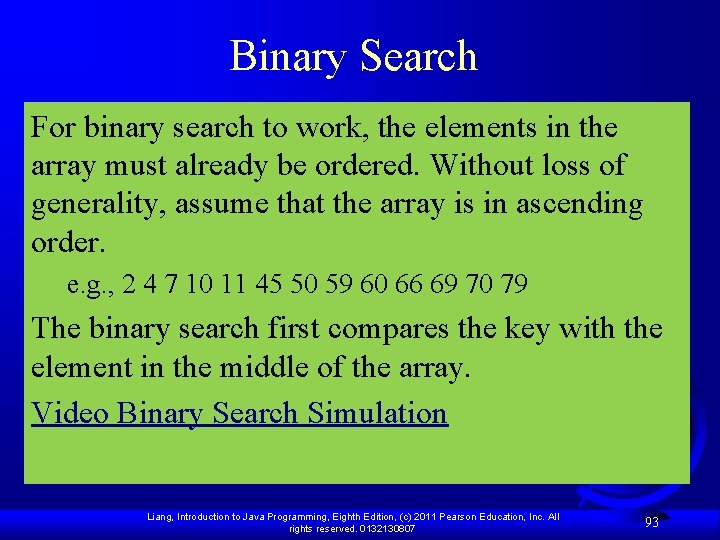
Binary Search For binary search to work, the elements in the array must already be ordered. Without loss of generality, assume that the array is in ascending order. e. g. , 2 4 7 10 11 45 50 59 60 66 69 70 79 The binary search first compares the key with the element in the middle of the array. Video Binary Search Simulation Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 93
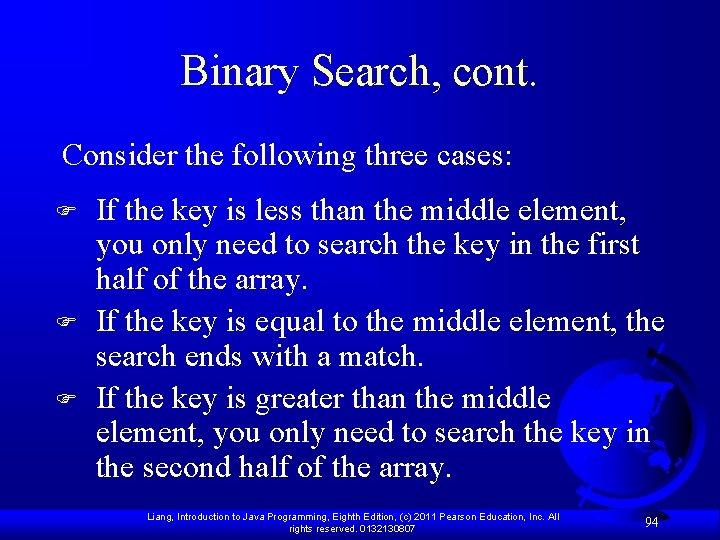
Binary Search, cont. Consider the following three cases: F F F If the key is less than the middle element, you only need to search the key in the first half of the array. If the key is equal to the middle element, the search ends with a match. If the key is greater than the middle element, you only need to search the key in the second half of the array. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 94
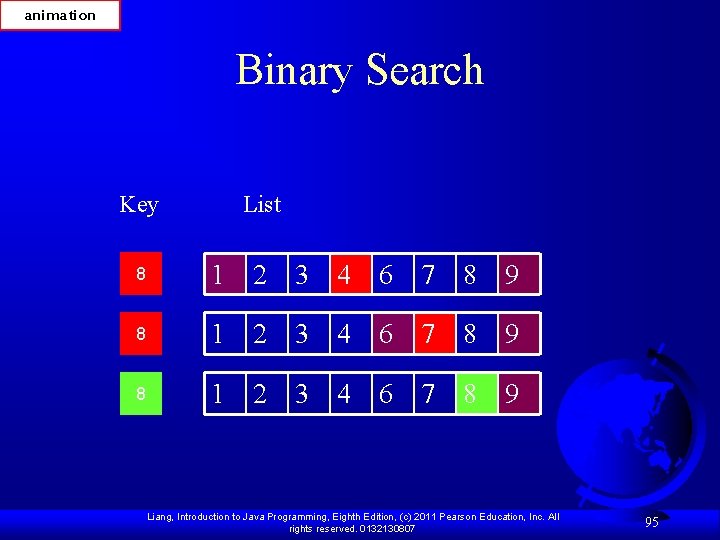
animation Binary Search Key List 8 1 2 3 4 6 7 8 9 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 95
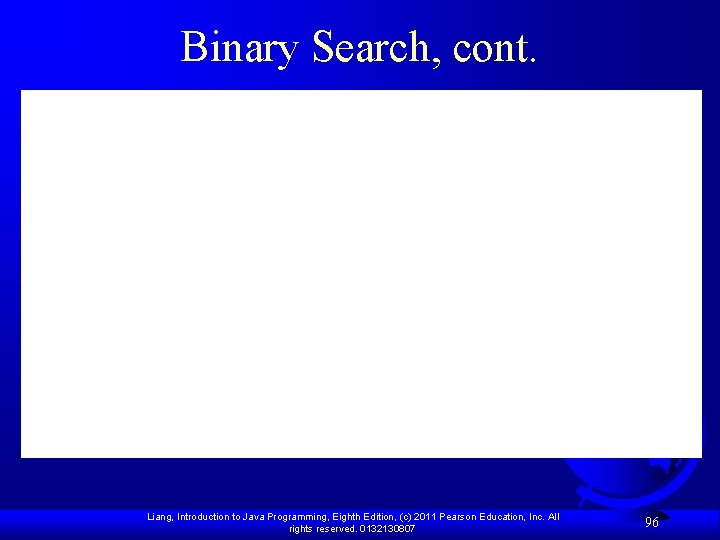
Binary Search, cont. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 96
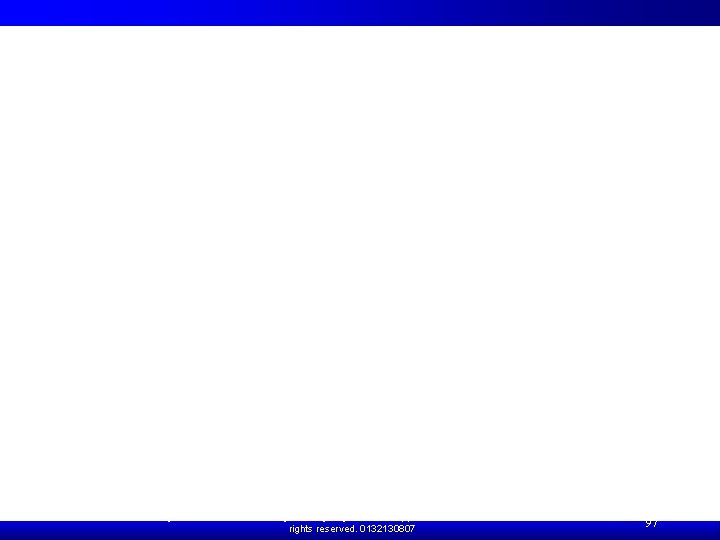
Binary Search, cont. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 97
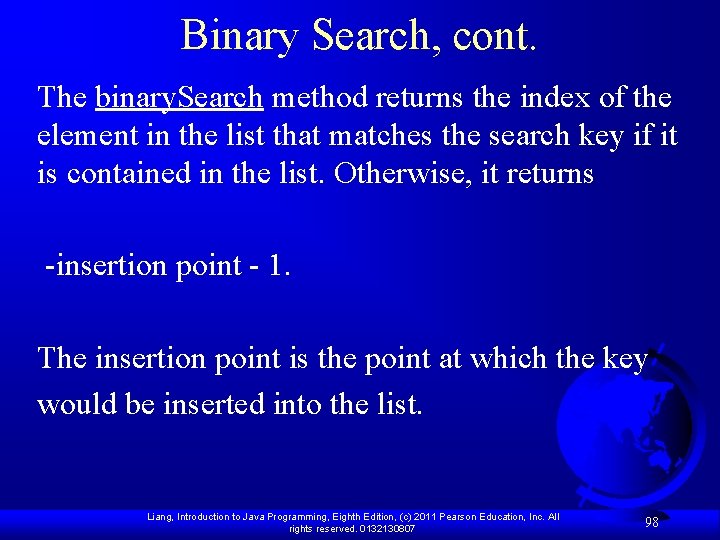
Binary Search, cont. The binary. Search method returns the index of the element in the list that matches the search key if it is contained in the list. Otherwise, it returns -insertion point - 1. The insertion point is the point at which the key would be inserted into the list. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 98
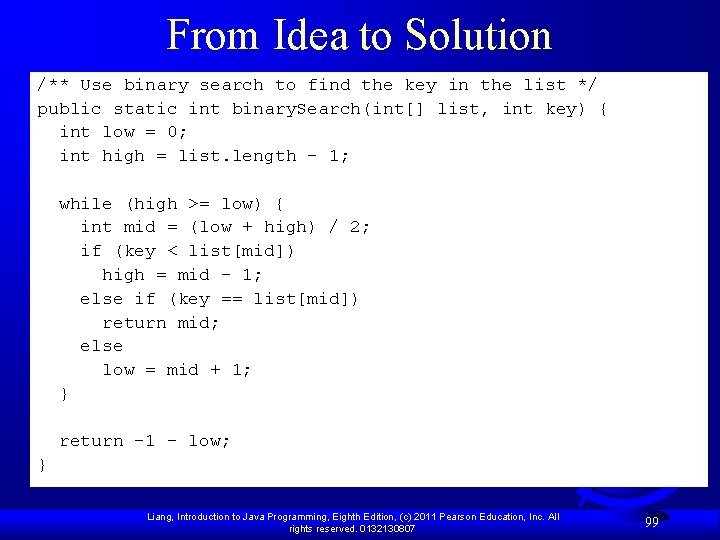
From Idea to Solution /** Use binary search to find the key in the list */ public static int binary. Search(int[] list, int key) { int low = 0; int high = list. length - 1; while (high >= low) { int mid = (low + high) / 2; if (key < list[mid]) high = mid - 1; else if (key == list[mid]) return mid; else low = mid + 1; } return -1 - low; } Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 99
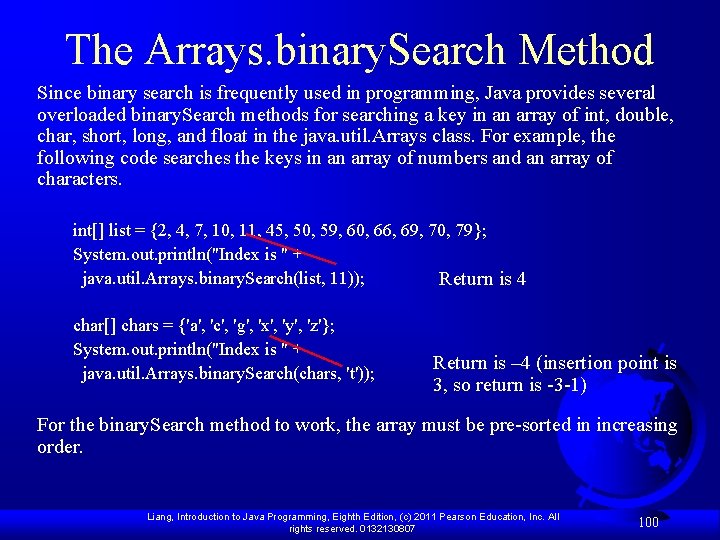
The Arrays. binary. Search Method Since binary search is frequently used in programming, Java provides several overloaded binary. Search methods for searching a key in an array of int, double, char, short, long, and float in the java. util. Arrays class. For example, the following code searches the keys in an array of numbers and an array of characters. int[] list = {2, 4, 7, 10, 11, 45, 50, 59, 60, 66, 69, 70, 79}; System. out. println("Index is " + java. util. Arrays. binary. Search(list, 11)); Return is 4 char[] chars = {'a', 'c', 'g', 'x', 'y', 'z'}; System. out. println("Index is " + Return is – 4 (insertion point is java. util. Arrays. binary. Search(chars, 't')); 3, so return is -3 -1) For the binary. Search method to work, the array must be pre-sorted in increasing order. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 100
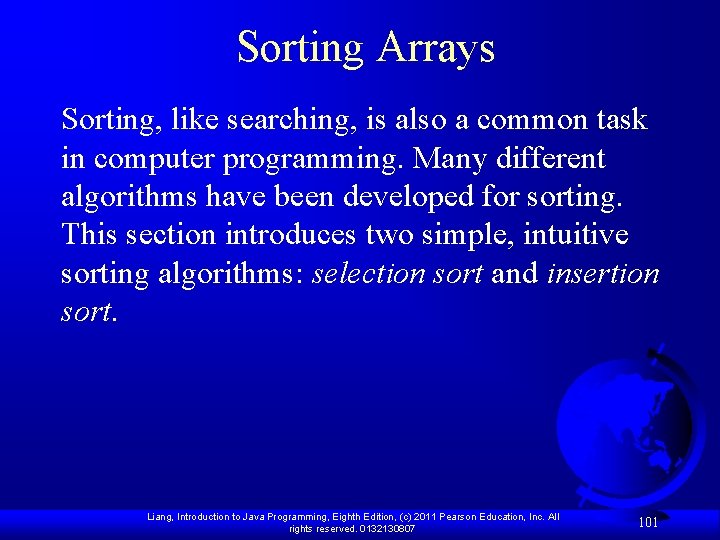
Sorting Arrays Sorting, like searching, is also a common task in computer programming. Many different algorithms have been developed for sorting. This section introduces two simple, intuitive sorting algorithms: selection sort and insertion sort. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 101
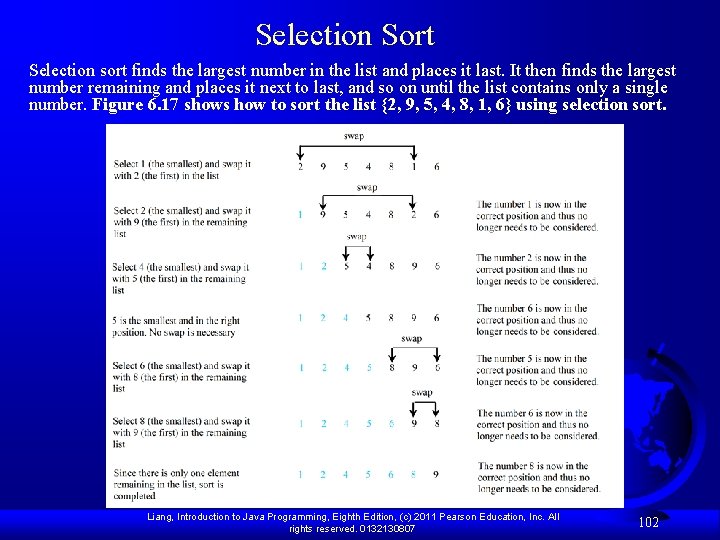
Selection Sort Selection sort finds the largest number in the list and places it last. It then finds the largest number remaining and places it next to last, and so on until the list contains only a single number. Figure 6. 17 shows how to sort the list {2, 9, 5, 4, 8, 1, 6} using selection sort. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 102
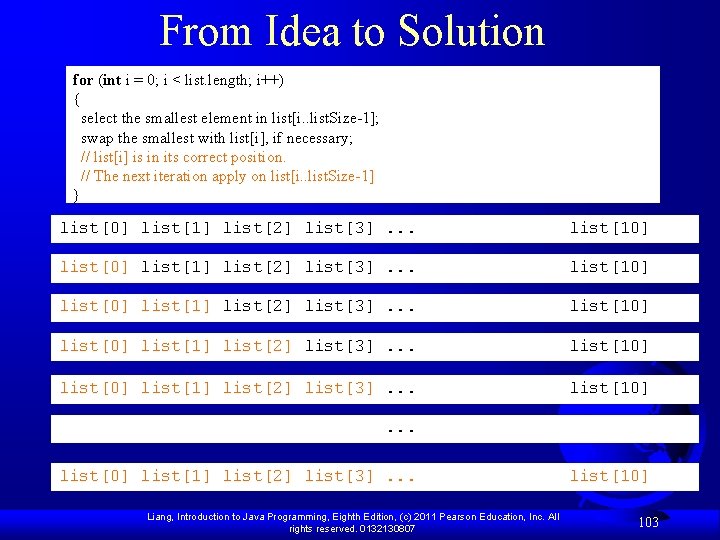
From Idea to Solution for (int i = 0; i < list. length; i++) { select the smallest element in list[i. . list. Size-1]; swap the smallest with list[i], if necessary; // list[i] is in its correct position. // The next iteration apply on list[i. . list. Size-1] } list[0] list[1] list[2] list[3]. . . list[10] . . . list[0] list[1] list[2] list[3]. . . list[10] Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 103
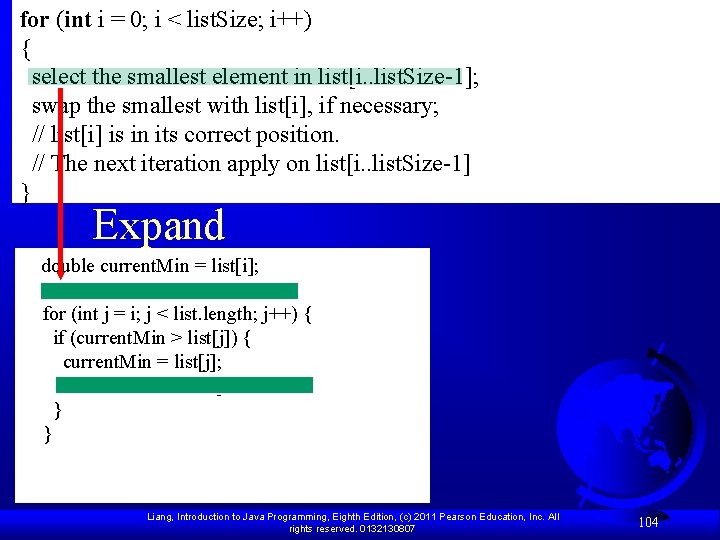
for (int i = 0; i < list. Size; i++) { select the smallest element in list[i. . list. Size-1]; swap the smallest with list[i], if necessary; // list[i] is in its correct position. // The next iteration apply on list[i. . list. Size-1] } Expand double current. Min = list[i]; int current. Min. Index = i; for (int j = i; j < list. length; j++) { if (current. Min > list[j]) { current. Min = list[j]; current. Min. Index = j; } } Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 104
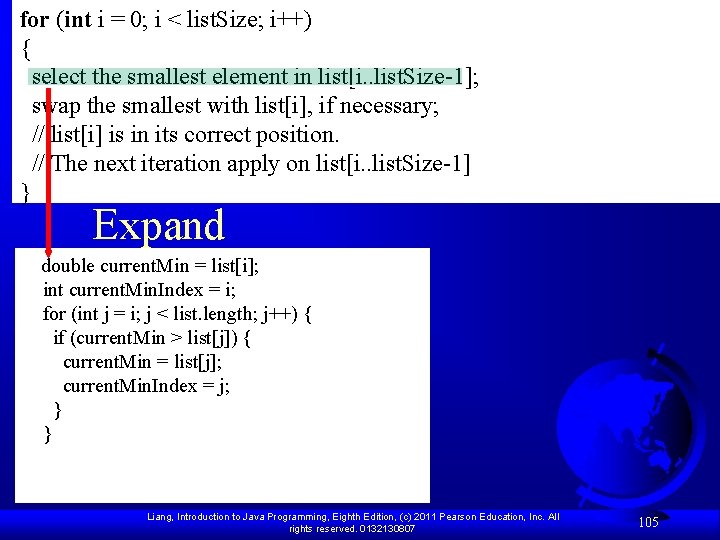
for (int i = 0; i < list. Size; i++) { select the smallest element in list[i. . list. Size-1]; swap the smallest with list[i], if necessary; // list[i] is in its correct position. // The next iteration apply on list[i. . list. Size-1] } Expand double current. Min = list[i]; int current. Min. Index = i; for (int j = i; j < list. length; j++) { if (current. Min > list[j]) { current. Min = list[j]; current. Min. Index = j; } } Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 105
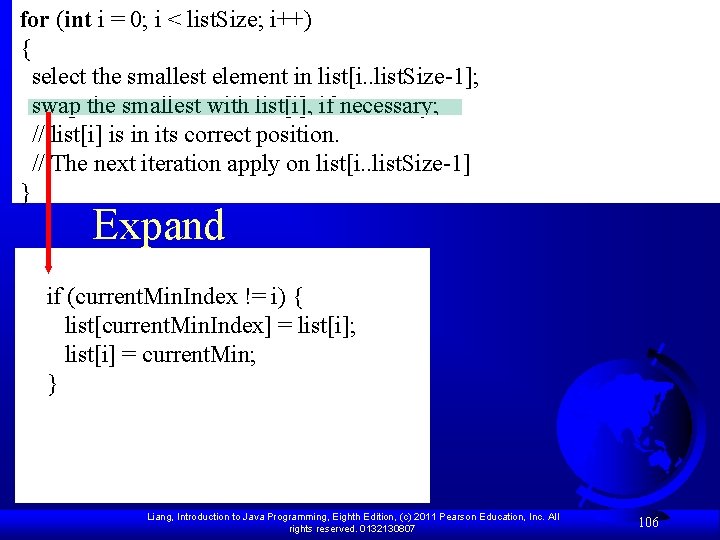
for (int i = 0; i < list. Size; i++) { select the smallest element in list[i. . list. Size-1]; swap the smallest with list[i], if necessary; // list[i] is in its correct position. // The next iteration apply on list[i. . list. Size-1] } Expand if (current. Min. Index != i) { list[current. Min. Index] = list[i]; list[i] = current. Min; } Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 106
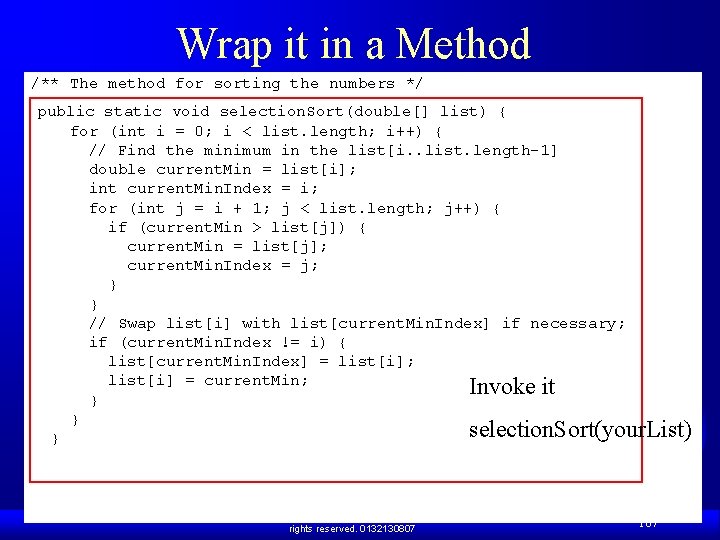
Wrap it in a Method /** The method for sorting the numbers */ public static void selection. Sort(double[] list) { for (int i = 0; i < list. length; i++) { // Find the minimum in the list[i. . list. length-1] double current. Min = list[i]; int current. Min. Index = i; for (int j = i + 1; j < list. length; j++) { if (current. Min > list[j]) { current. Min = list[j]; current. Min. Index = j; } } // Swap list[i] with list[current. Min. Index] if necessary; if (current. Min. Index != i) { list[current. Min. Index] = list[i]; list[i] = current. Min; Invoke it } } selection. Sort(your. List) } Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 107
![Insertion Sort int my List 2 9 5 4 8 1 6 Insertion Sort int[] my. List = {2, 9, 5, 4, 8, 1, 6}; //](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-108.jpg)
Insertion Sort int[] my. List = {2, 9, 5, 4, 8, 1, 6}; // Unsorted The insertion sort algorithm sorts a list of values by repeatedly inserting an unsorted element into a sorted sublist until the whole list is sorted. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 108
![animation Insertion Sort int my List 2 9 5 4 8 1 6 animation Insertion Sort int[] my. List = {2, 9, 5, 4, 8, 1, 6};](https://slidetodoc.com/presentation_image_h/00060680a3e808a0259f41bce5d5e0b7/image-109.jpg)
animation Insertion Sort int[] my. List = {2, 9, 5, 4, 8, 1, 6}; // Unsorted 2 9 5 4 8 1 6 2 5 9 4 8 1 6 2 4 1 2 5 4 8 5 9 1 6 8 2 9 5 4 8 1 6 2 4 5 9 8 1 6 1 2 4 5 8 9 6 6 9 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 109
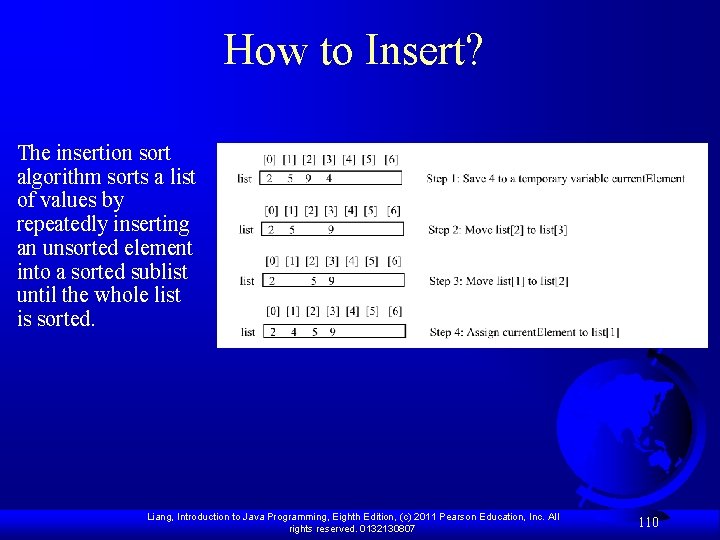
How to Insert? The insertion sort algorithm sorts a list of values by repeatedly inserting an unsorted element into a sorted sublist until the whole list is sorted. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 110
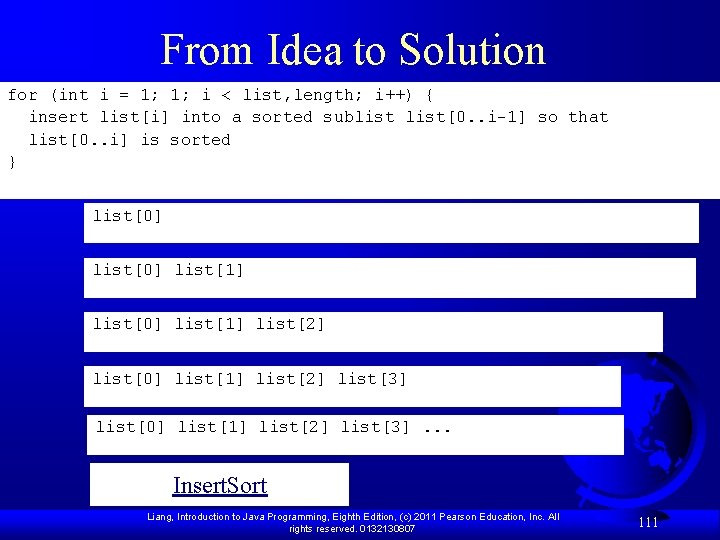
From Idea to Solution for (int i = 1; 1; i < list, length; i++) { insert list[i] into a sorted sublist[0. . i-1] so that list[0. . i] is sorted } list[0] list[1] list[2] list[3] list[0] list[1] list[2] list[3]. . . Insert. Sort Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 111
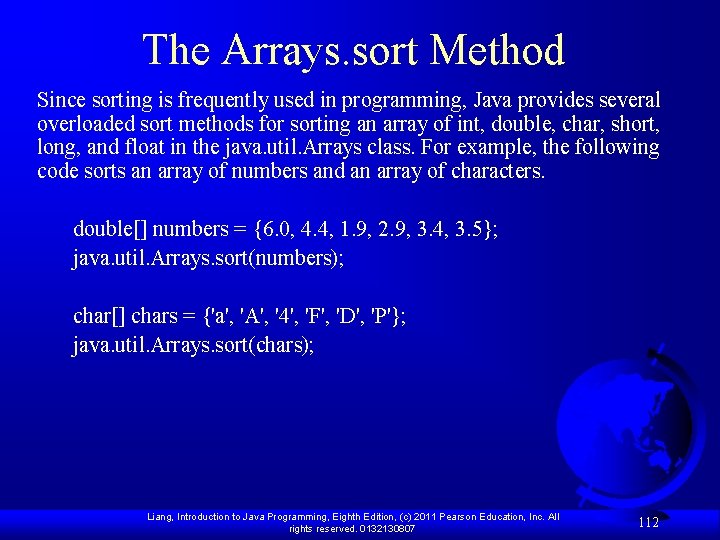
The Arrays. sort Method Since sorting is frequently used in programming, Java provides several overloaded sort methods for sorting an array of int, double, char, short, long, and float in the java. util. Arrays class. For example, the following code sorts an array of numbers and an array of characters. double[] numbers = {6. 0, 4. 4, 1. 9, 2. 9, 3. 4, 3. 5}; java. util. Arrays. sort(numbers); char[] chars = {'a', 'A', '4', 'F', 'D', 'P'}; java. util. Arrays. sort(chars); Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 112
Daniel liang introduction to java programming
Liang fontdemo.java
Liang fontdemo.java
Parallel arrays
Que son los arreglos unidimensionales en java
Arrays bidimensionales java
Java introduction to problem solving and programming
Programming and problem solving with java
Java introduction to problem solving and programming
Introduction to java programming 10th edition quizzes
Richard seow yung liang
Zhuo shi wo li liang
Zhong han liang
Yuanxing liang
Amber liang
Cara mengindeks pt pos indonesia
Randy liang
David teo choon liang
How was ma liang like
Zong-liang yang
Liang jianhui
Nba schedule maker
Chia liang cheng
Administrasi keuangan kelas 11
Mechanical engineering umbc
Zong-liang yang
Dr liang hao nan
Dr liang hao nan
Pengertian arsip menurut the liang gie
Anticorps anti-nucléaire positif moucheté
Kathleen liang
Edmund liang
Perbezaan salur darah
Sushil dubey intel
Diana liang faa
Foo joon liang
Chapter 1 introduction to computers and programming
Computer programming chapter 1
Chapter 1 introduction to computers and programming
Chapter 1 introduction to computers and programming
Array of arrays c++
Parallel arrays
潘仁義
Parallel arrays
Why do we need arrays?
Dynamic arrays and amortized analysis
Arrays mips
Polynomial representation using arrays
Strings in assembly language
Global arrays in c
Computer science arrays
Searching and sorting arrays in c++
Arrays visual basic
Python parallel arrays
Disadvantages of array
I wonder is it possible
Pascal 2d array
Mips array example
Creating arrays matlab
Arrays as adt
Partially filled array
Redundancy array of independent disk
Python list of arrays
Arrays
Day 3: arrays
A case for redundant arrays of inexpensive disks
Microsoft small basic
Disadvantages of array
Microled arrays
Are vectors dynamic arrays
Facts about arrays
Perbedaan linear programming dan integer programming
Greedy programming vs dynamic programming
What is system programming
Linear vs integer programming
Programing adalah
Application
Java parallel programming
Object oriented programming exercises java
Event-driven programming in java
Java asynchronous programming
Class dan object
Importance of java programming
Hopper khan academy
Event driven programming in java
Defensive programming java
Java programming refresher
Open source java games
Symbols used in java
Programming c
Java database programming
Java asynchronous programming
Conclusion of java programming
Java programming
Elementary programming in java
Basic elements of java
Advanced programming in java
Java language
Java enterprise architecture
Dangling else java
Import java.util.*
Import java.util.*
Java applet swing
Import java.util.* use
Java
Java gcd
Import java.util.random
Import java.io.*
Import java util
Java import java.io.*
Perbedaan java swing dan awt
Import java.awt.event.*;
Java compiler translates java source code into