C for Java Programmers Chapter 2 Fundamental Data
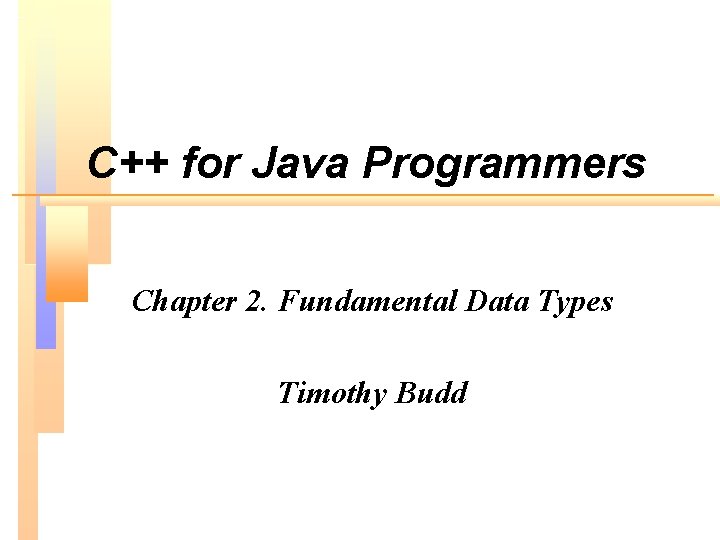
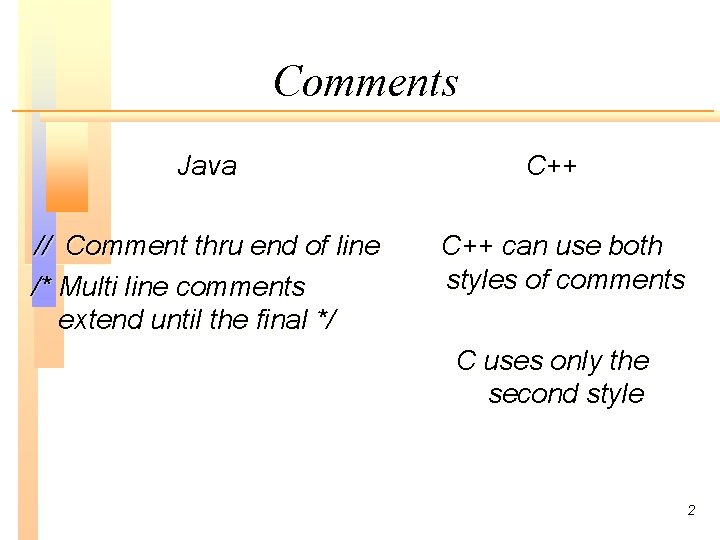
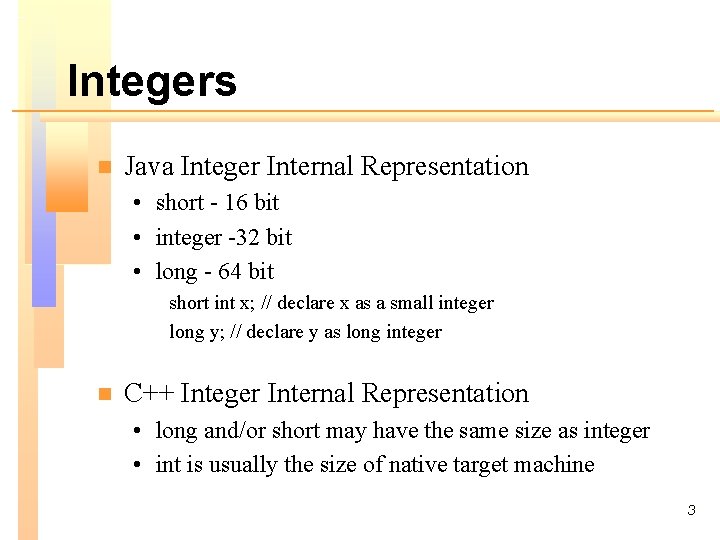
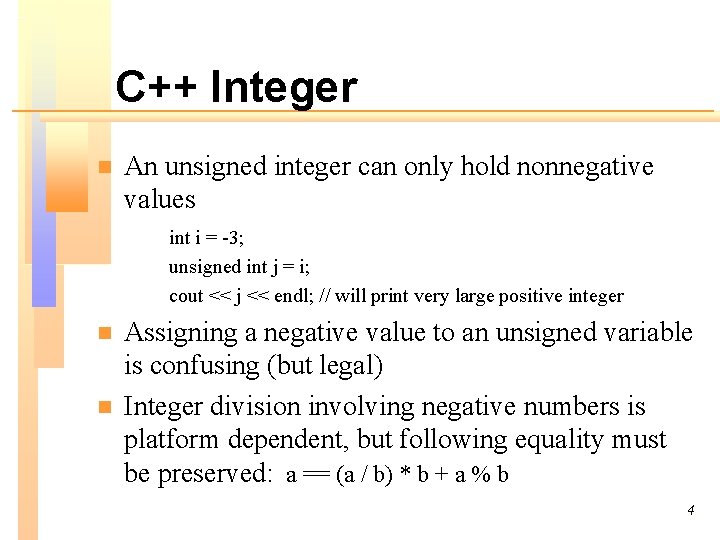
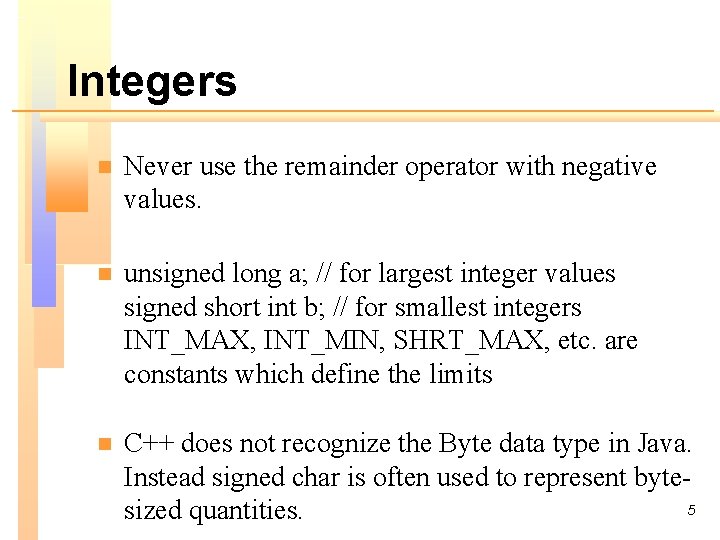
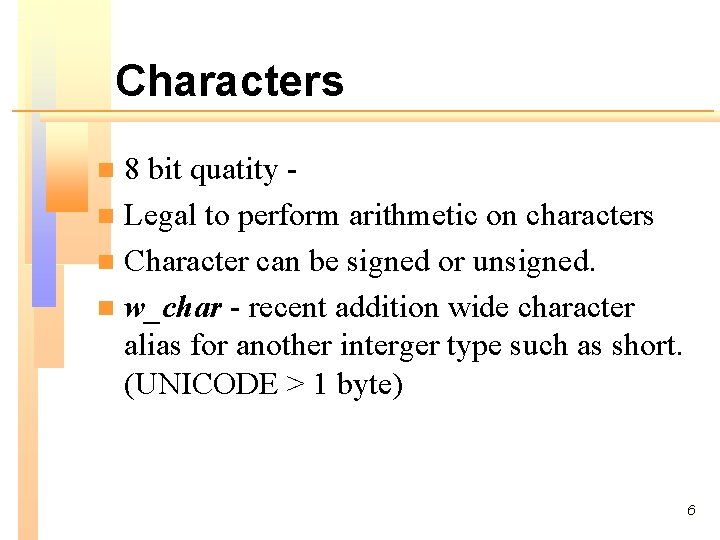
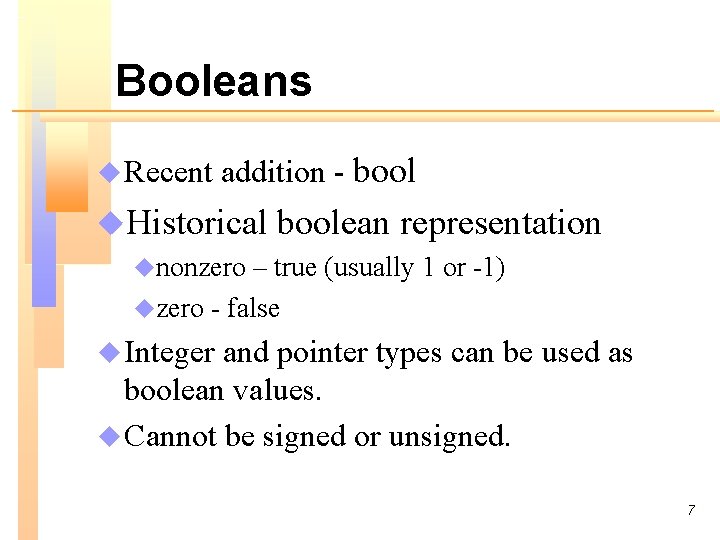
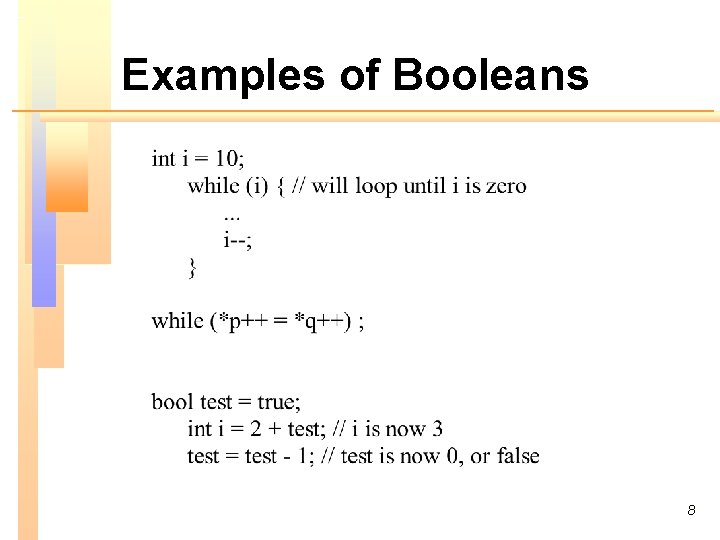
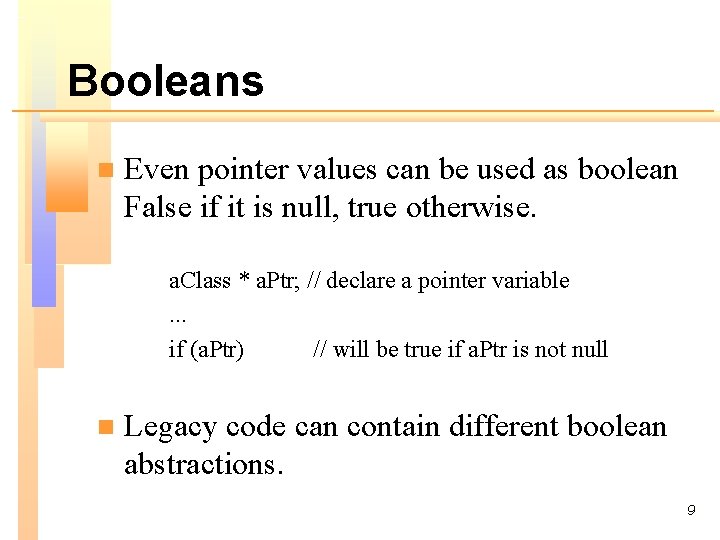
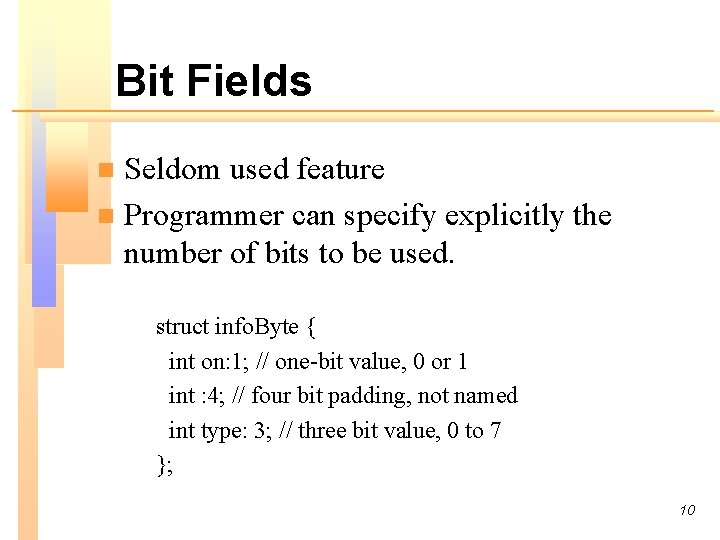
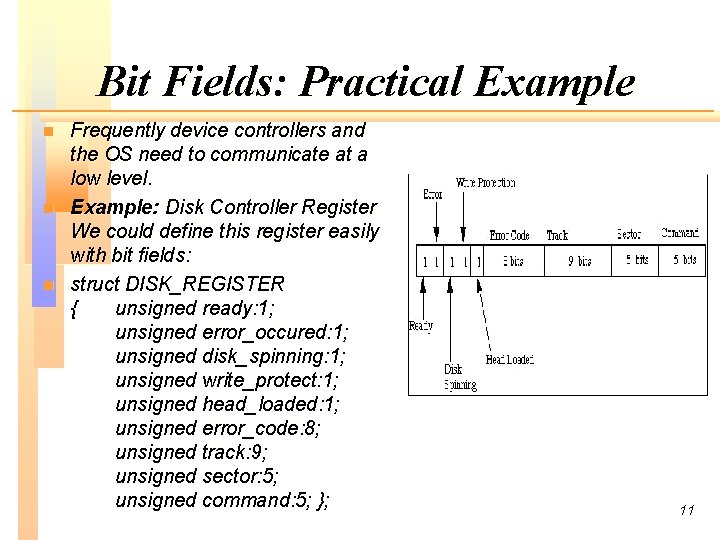
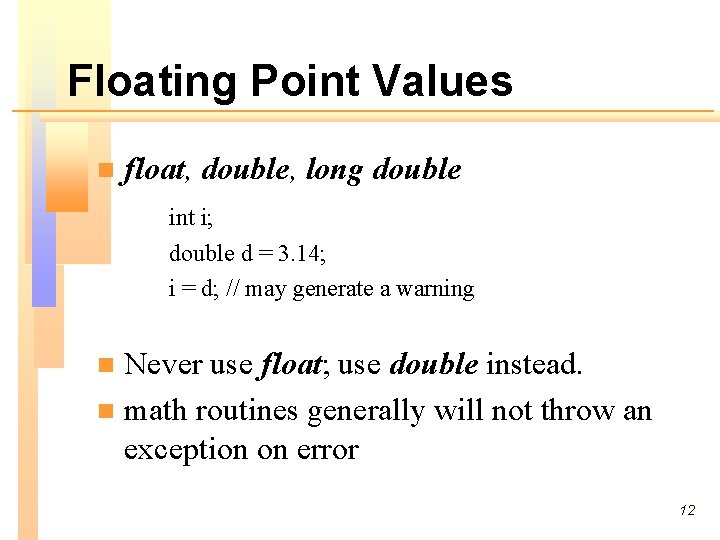
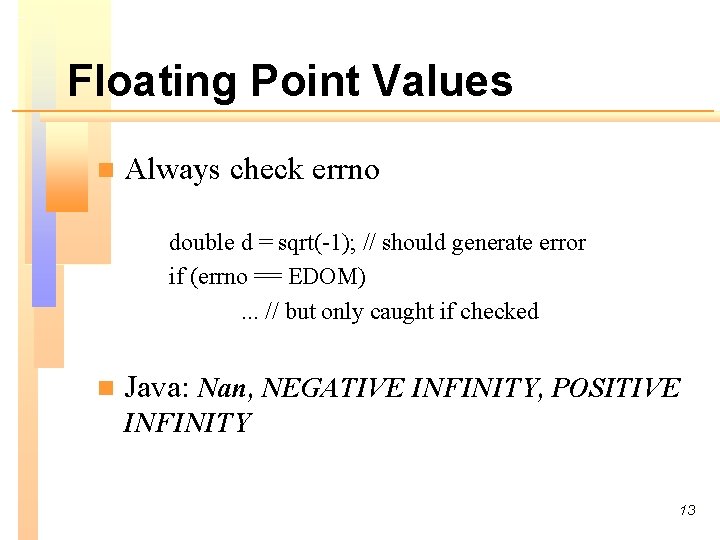
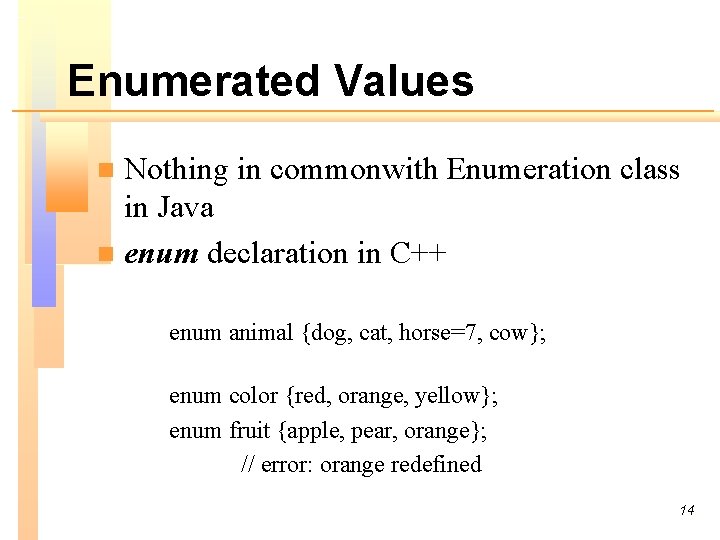
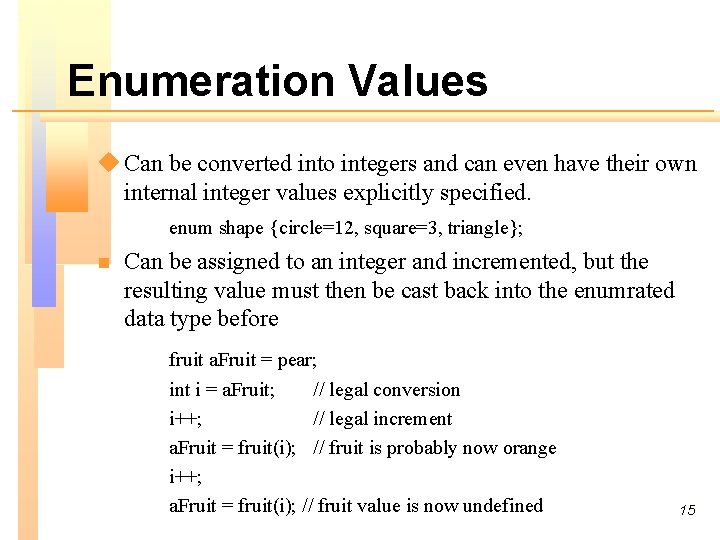
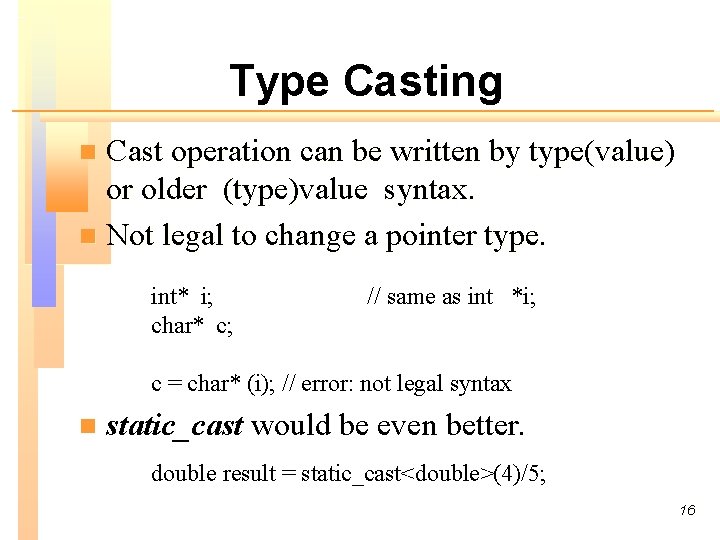
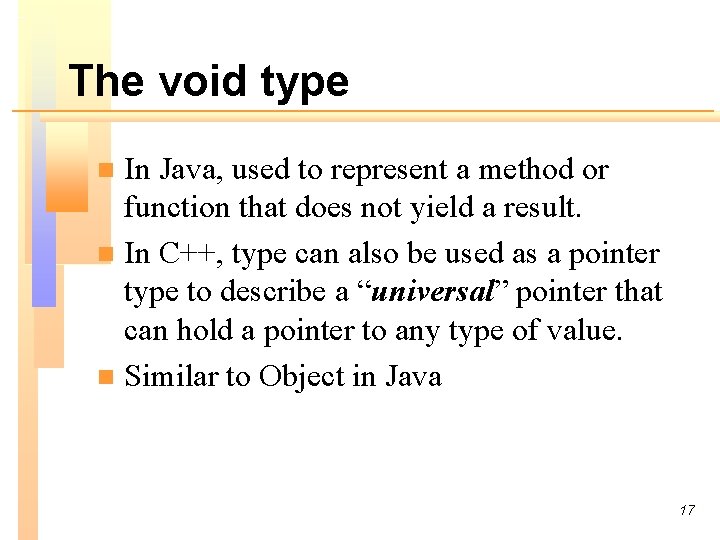
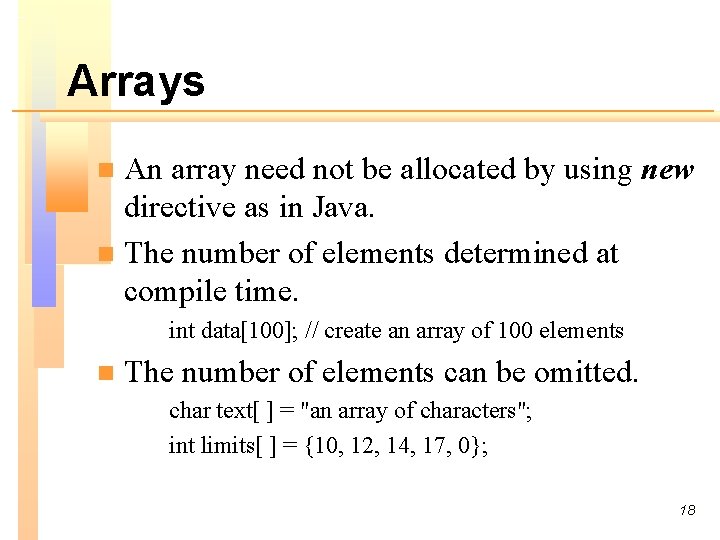
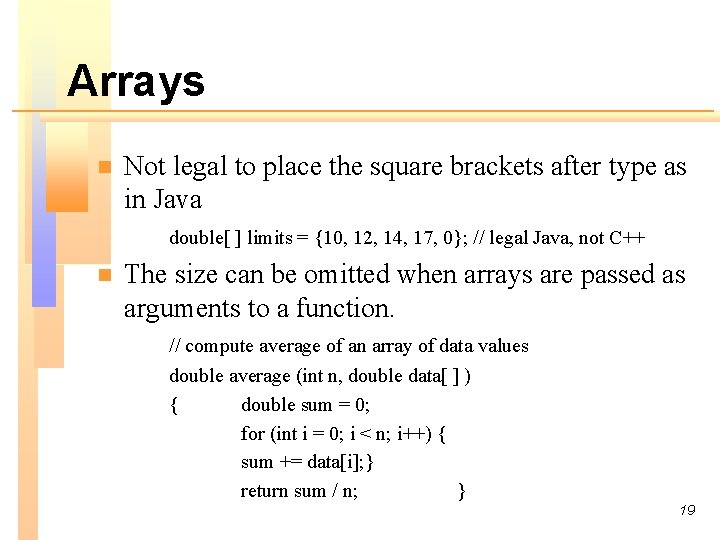
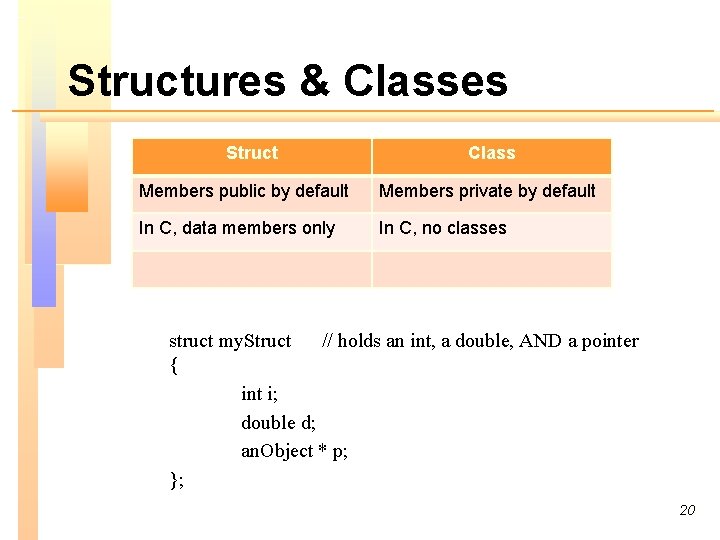
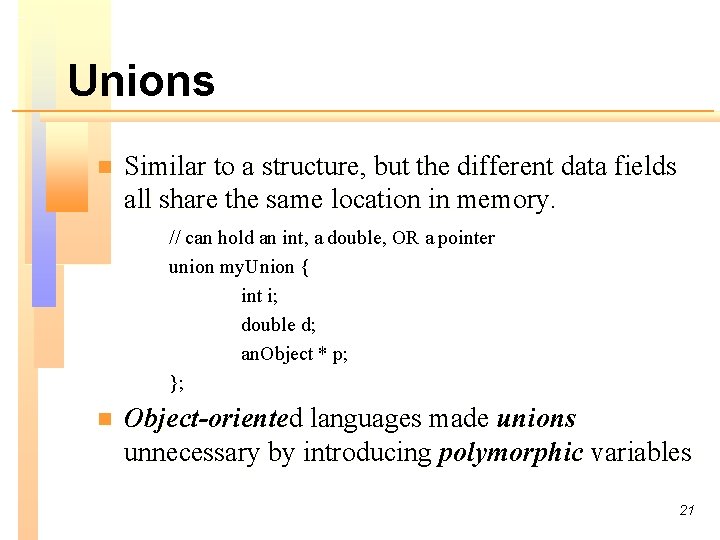
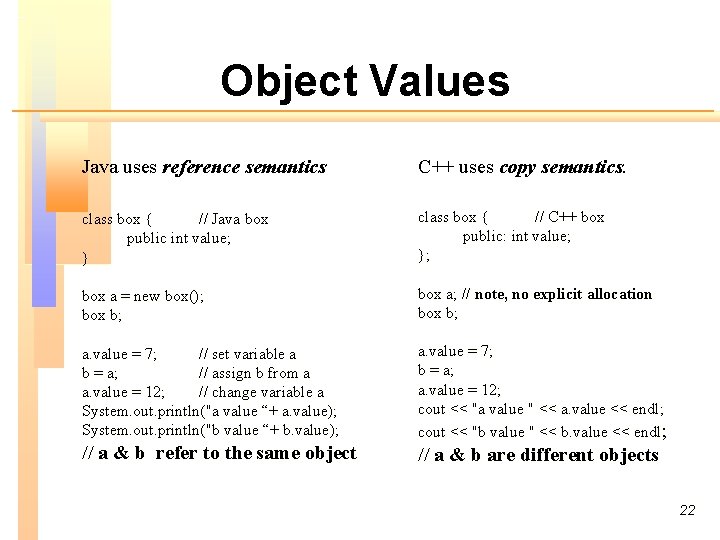
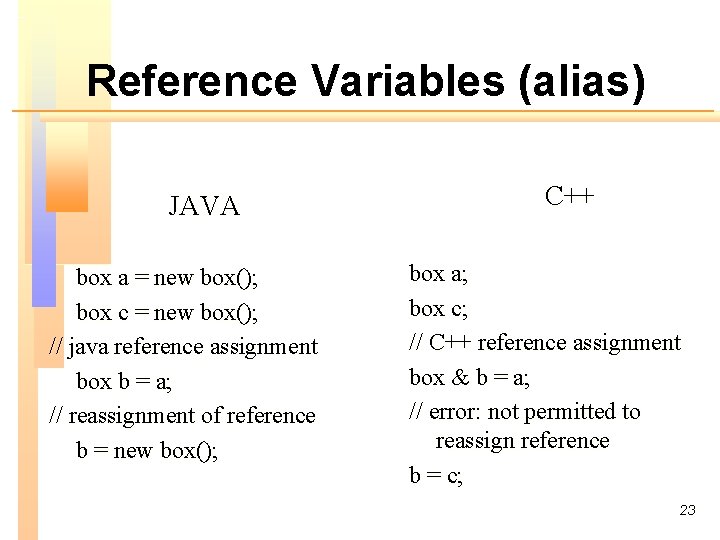
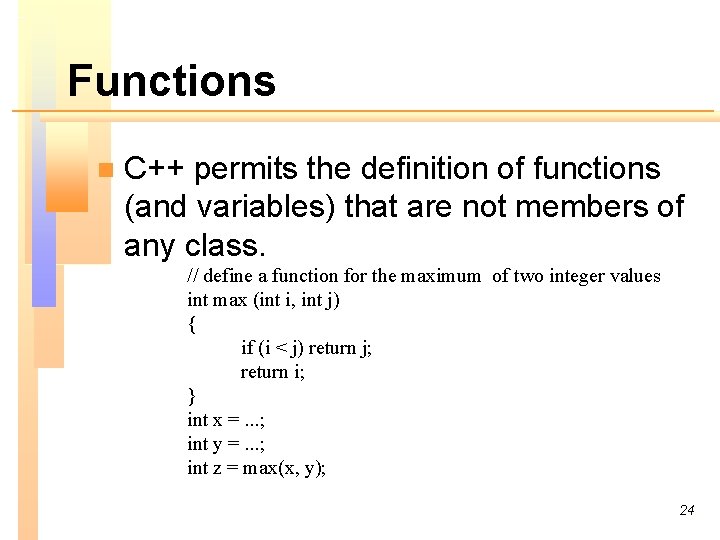
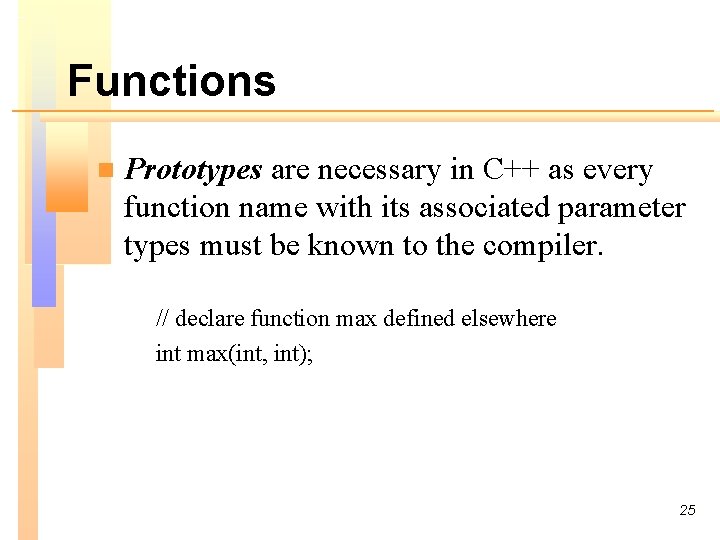
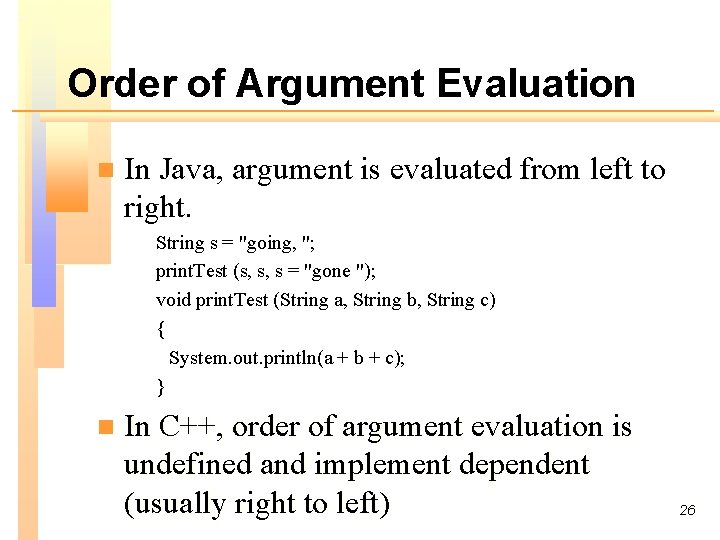
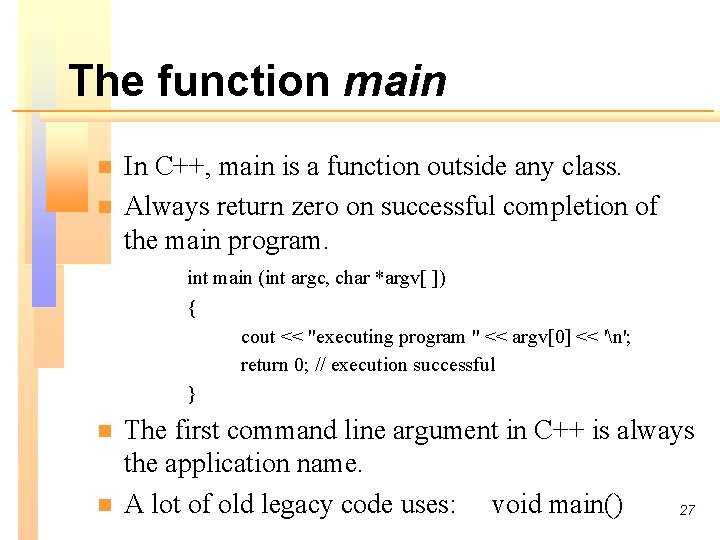
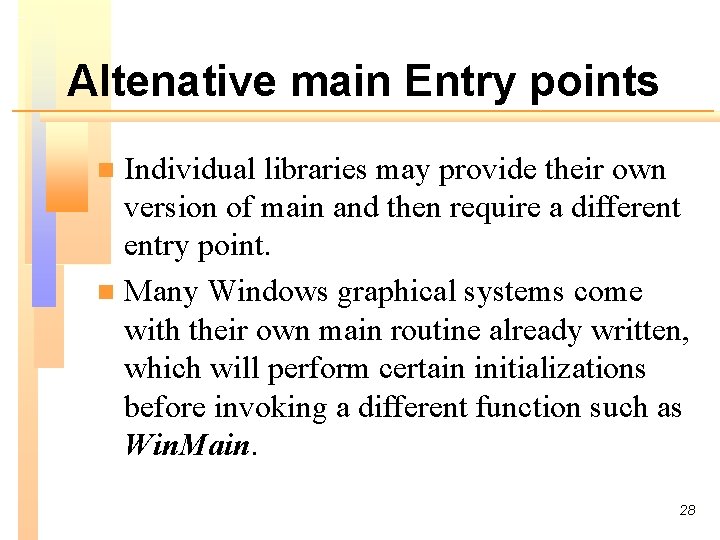
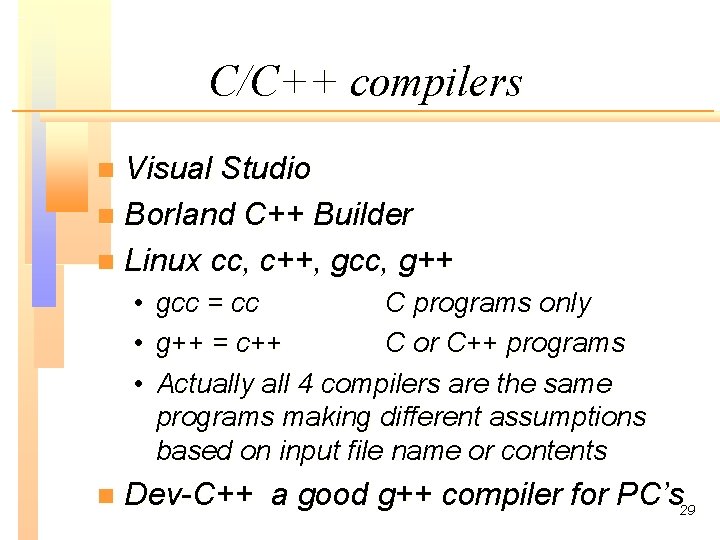
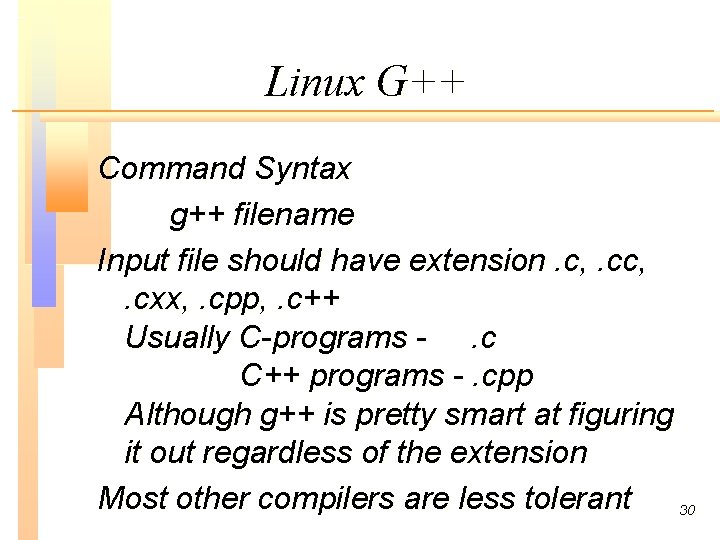
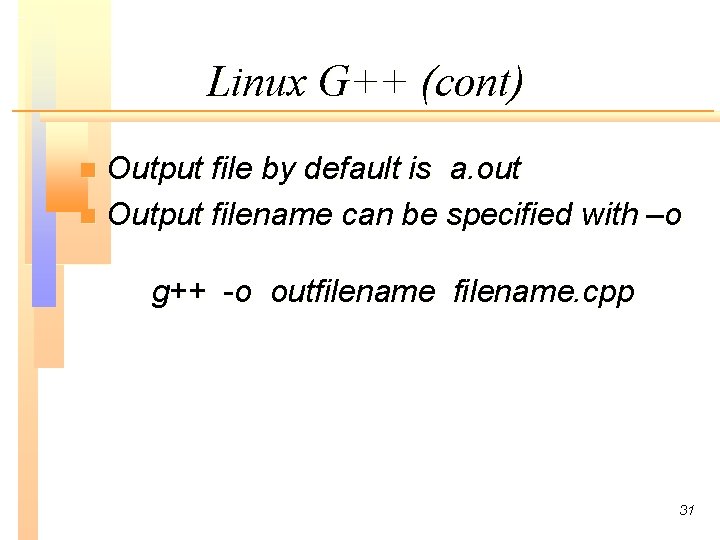
![Simple Programs JAVA public class Hello. World { public static void main(String[] args) { Simple Programs JAVA public class Hello. World { public static void main(String[] args) {](https://slidetodoc.com/presentation_image/4ab0bac430ad4fbae65cfa0225809e91/image-32.jpg)
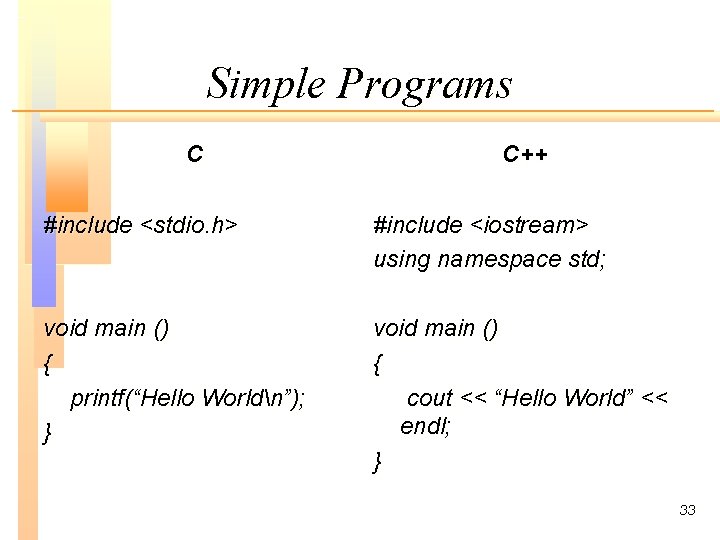
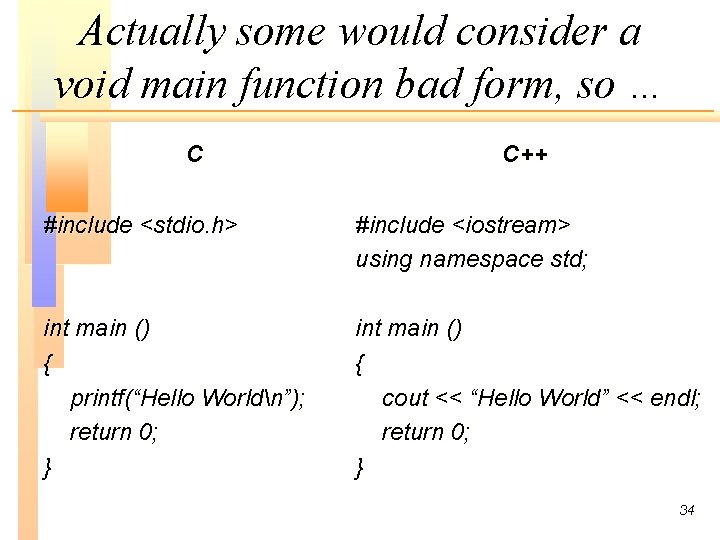
- Slides: 34
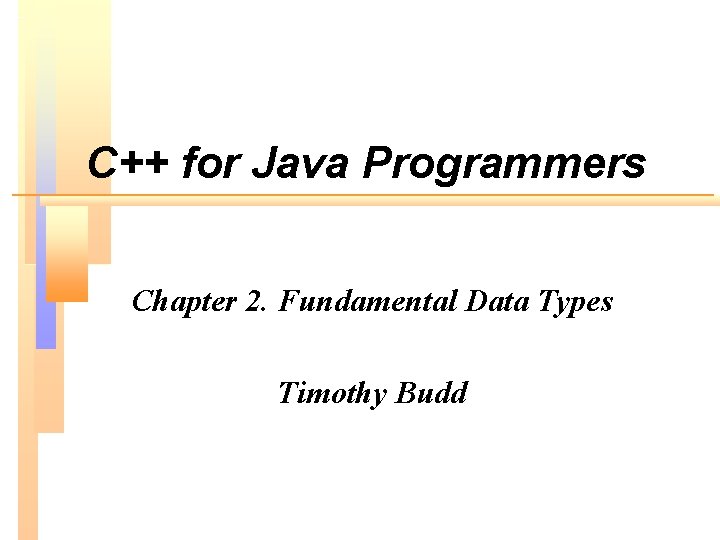
C++ for Java Programmers Chapter 2. Fundamental Data Types Timothy Budd
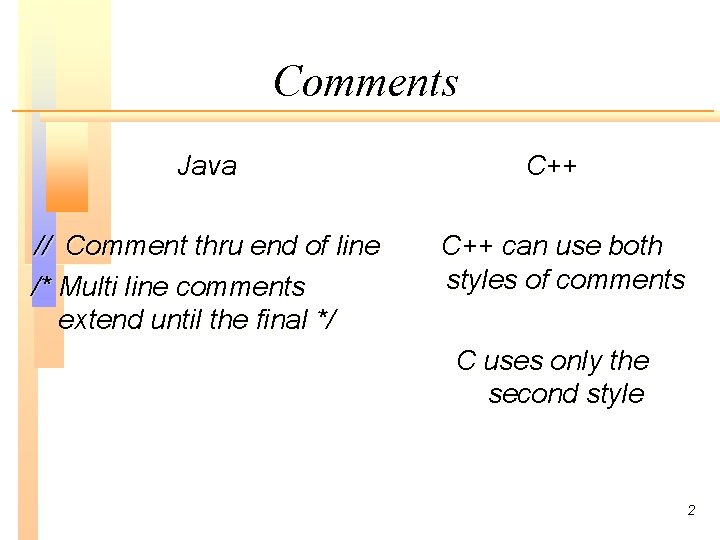
Comments Java // Comment thru end of line /* Multi line comments extend until the final */ C++ can use both styles of comments C uses only the second style 2
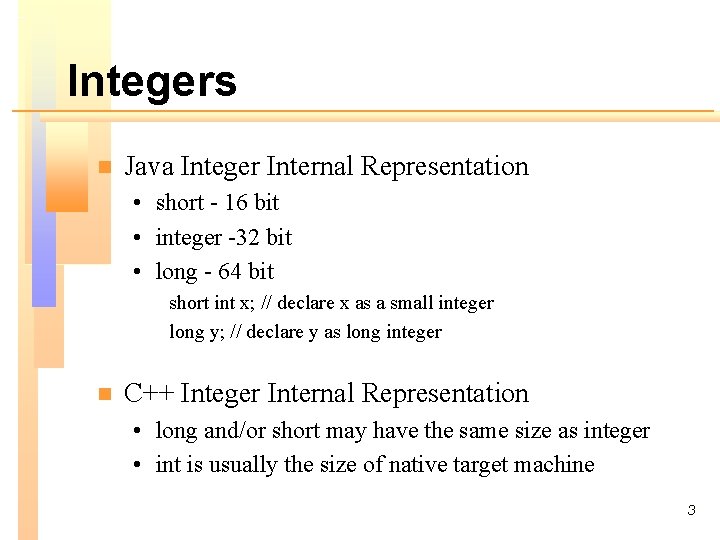
Integers n Java Integer Internal Representation • short - 16 bit • integer -32 bit • long - 64 bit short int x; // declare x as a small integer long y; // declare y as long integer n C++ Integer Internal Representation • long and/or short may have the same size as integer • int is usually the size of native target machine 3
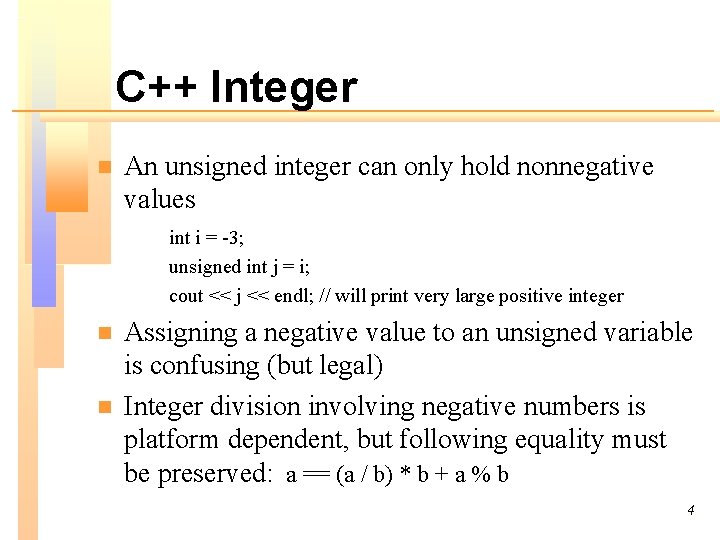
C++ Integer n An unsigned integer can only hold nonnegative values int i = -3; unsigned int j = i; cout << j << endl; // will print very large positive integer n n Assigning a negative value to an unsigned variable is confusing (but legal) Integer division involving negative numbers is platform dependent, but following equality must be preserved: a == (a / b) * b + a % b 4
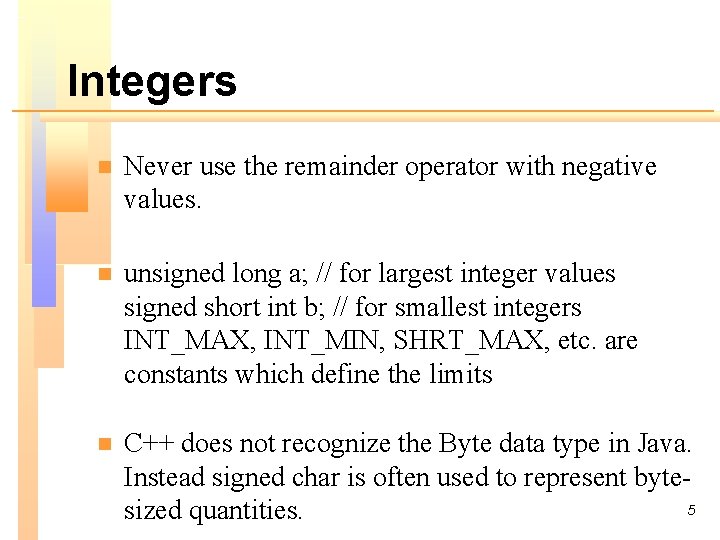
Integers n Never use the remainder operator with negative values. n unsigned long a; // for largest integer values signed short int b; // for smallest integers INT_MAX, INT_MIN, SHRT_MAX, etc. are constants which define the limits n C++ does not recognize the Byte data type in Java. Instead signed char is often used to represent byte 5 sized quantities.
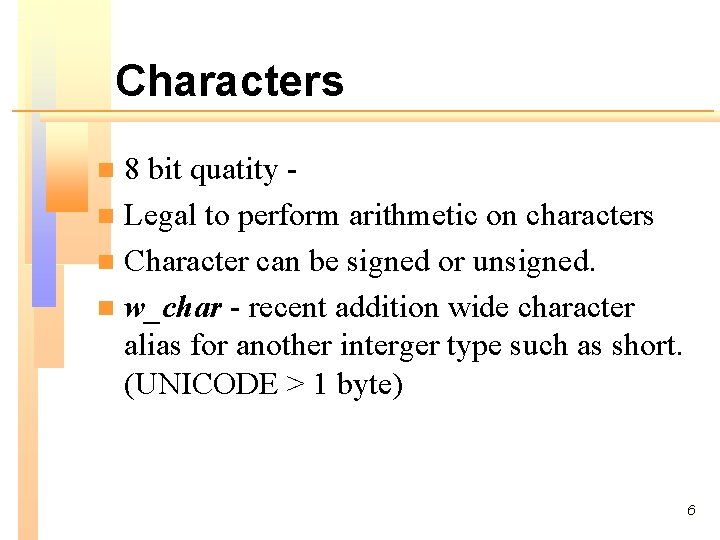
Characters 8 bit quatity n Legal to perform arithmetic on characters n Character can be signed or unsigned. n w_char - recent addition wide character alias for another interger type such as short. (UNICODE > 1 byte) n 6
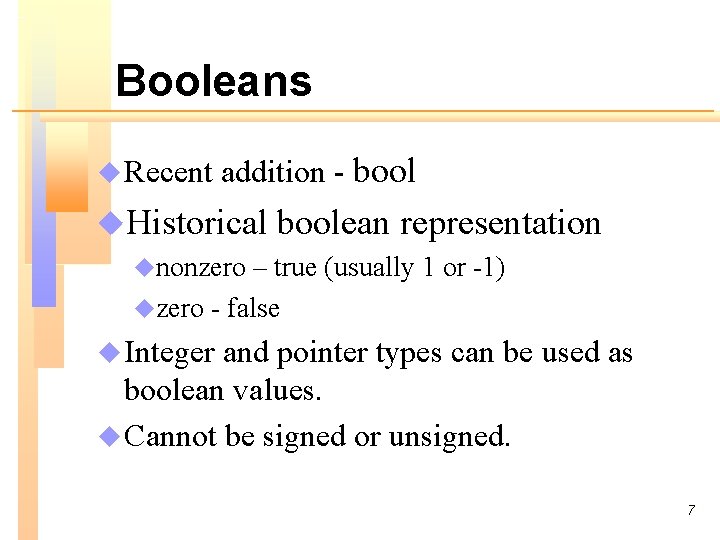
Booleans u Recent addition - bool u. Historical boolean representation unonzero – true (usually 1 or -1) uzero - false u Integer and pointer types can be used as boolean values. u Cannot be signed or unsigned. 7
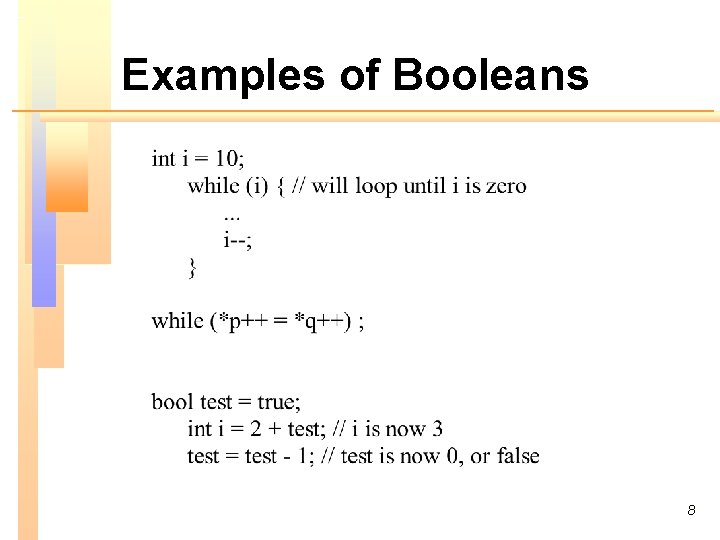
Examples of Booleans 8
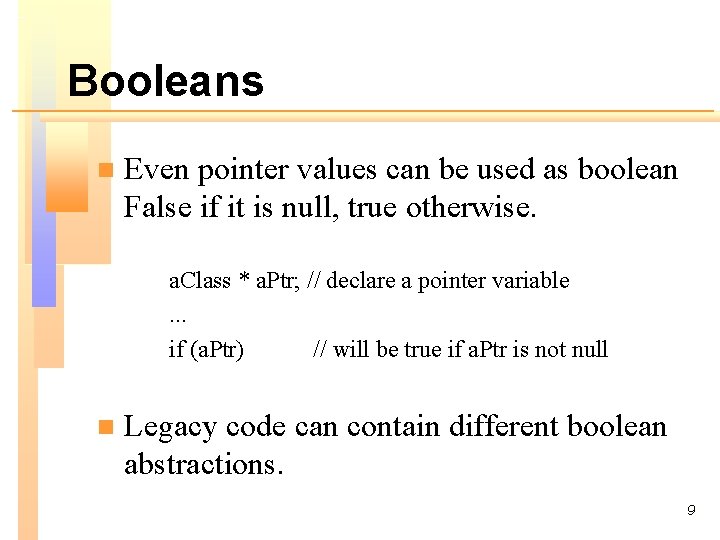
Booleans n Even pointer values can be used as boolean False if it is null, true otherwise. a. Class * a. Ptr; // declare a pointer variable. . . if (a. Ptr) // will be true if a. Ptr is not null n Legacy code can contain different boolean abstractions. 9
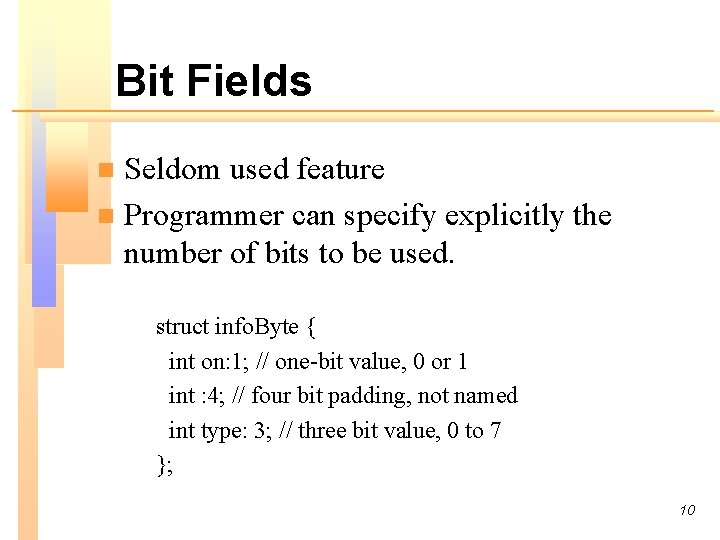
Bit Fields Seldom used feature n Programmer can specify explicitly the number of bits to be used. n struct info. Byte { int on: 1; // one-bit value, 0 or 1 int : 4; // four bit padding, not named int type: 3; // three bit value, 0 to 7 }; 10
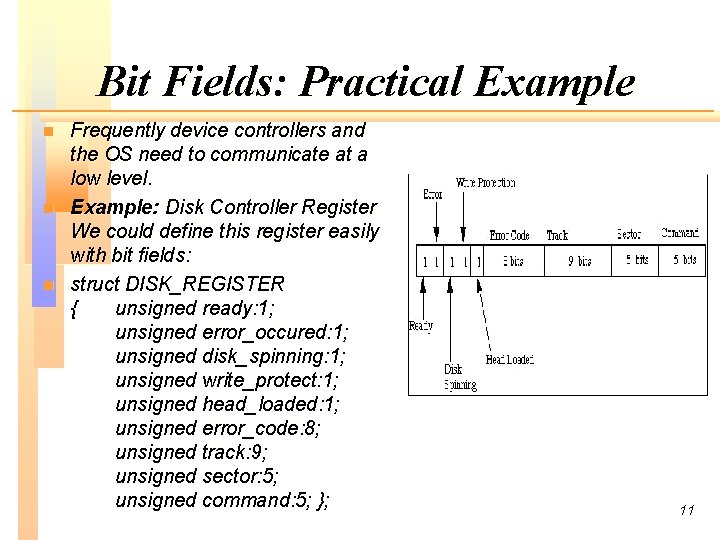
Bit Fields: Practical Example n n n Frequently device controllers and the OS need to communicate at a low level. Example: Disk Controller Register We could define this register easily with bit fields: struct DISK_REGISTER { unsigned ready: 1; unsigned error_occured: 1; unsigned disk_spinning: 1; unsigned write_protect: 1; unsigned head_loaded: 1; unsigned error_code: 8; unsigned track: 9; unsigned sector: 5; unsigned command: 5; }; 11
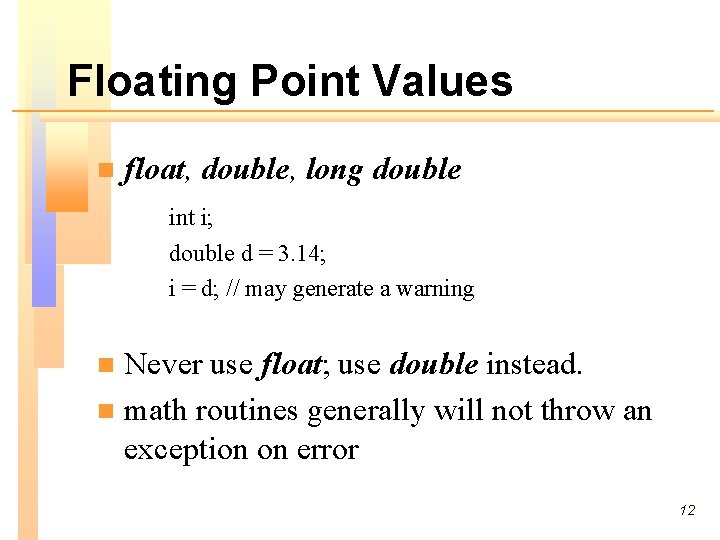
Floating Point Values n float, double, long double int i; double d = 3. 14; i = d; // may generate a warning Never use float; use double instead. n math routines generally will not throw an exception on error n 12
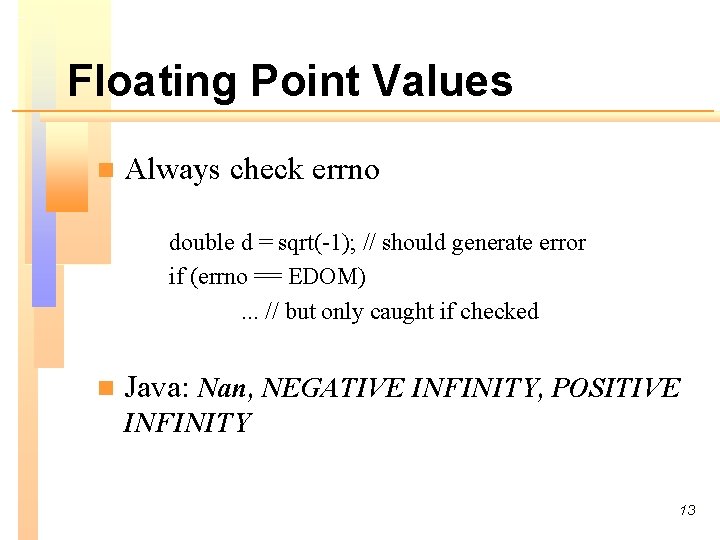
Floating Point Values n Always check errno double d = sqrt(-1); // should generate error if (errno == EDOM). . . // but only caught if checked n Java: Nan, NEGATIVE INFINITY, POSITIVE INFINITY 13
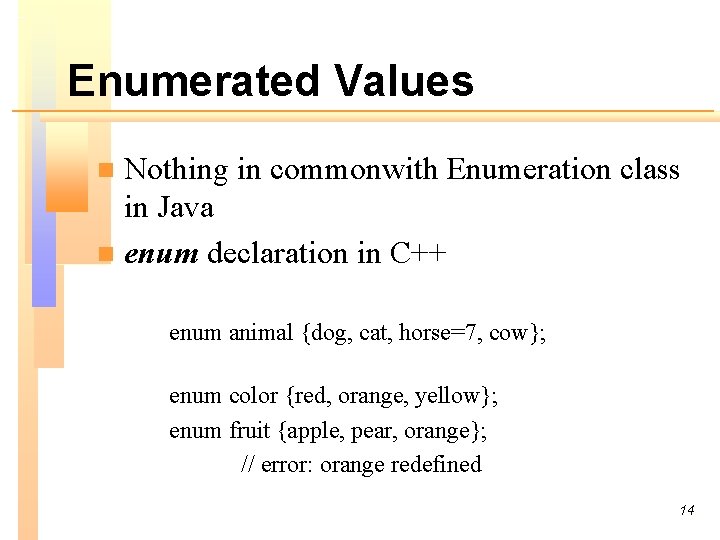
Enumerated Values Nothing in commonwith Enumeration class in Java n enum declaration in C++ n enum animal {dog, cat, horse=7, cow}; enum color {red, orange, yellow}; enum fruit {apple, pear, orange}; // error: orange redefined 14
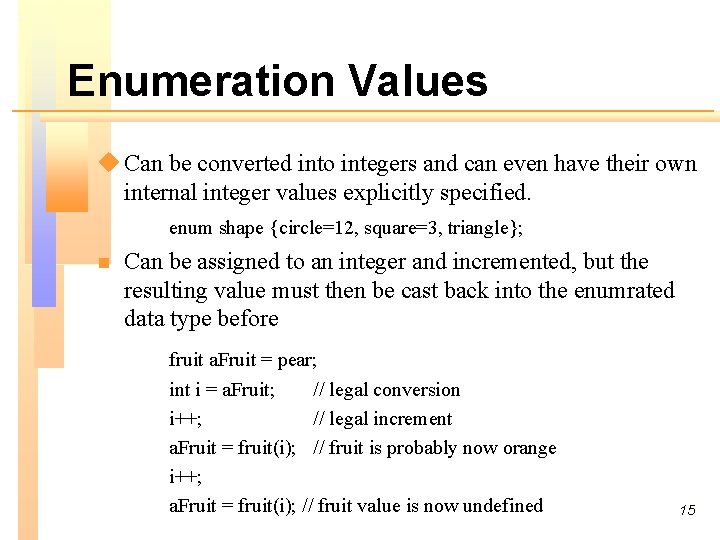
Enumeration Values u Can be converted into integers and can even have their own internal integer values explicitly specified. enum shape {circle=12, square=3, triangle}; n Can be assigned to an integer and incremented, but the resulting value must then be cast back into the enumrated data type before fruit a. Fruit = pear; int i = a. Fruit; // legal conversion i++; // legal increment a. Fruit = fruit(i); // fruit is probably now orange i++; a. Fruit = fruit(i); // fruit value is now undefined 15
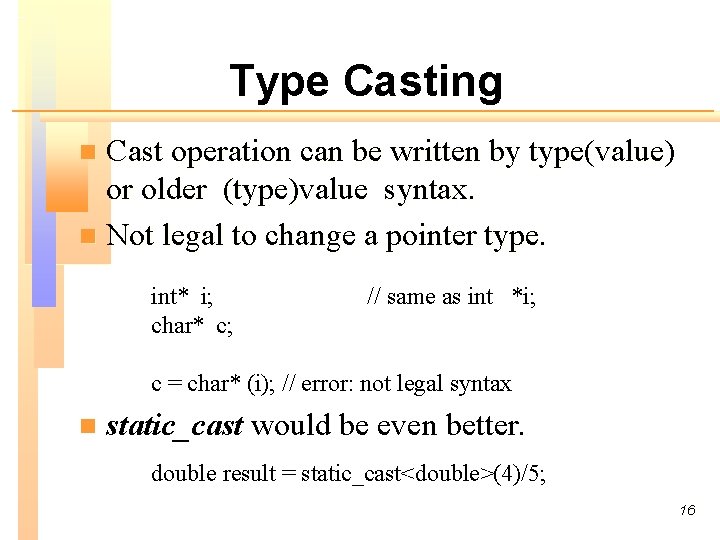
Type Casting Cast operation can be written by type(value) or older (type)value syntax. n Not legal to change a pointer type. n int* i; char* c; // same as int *i; c = char* (i); // error: not legal syntax n static_cast would be even better. double result = static_cast<double>(4)/5; 16
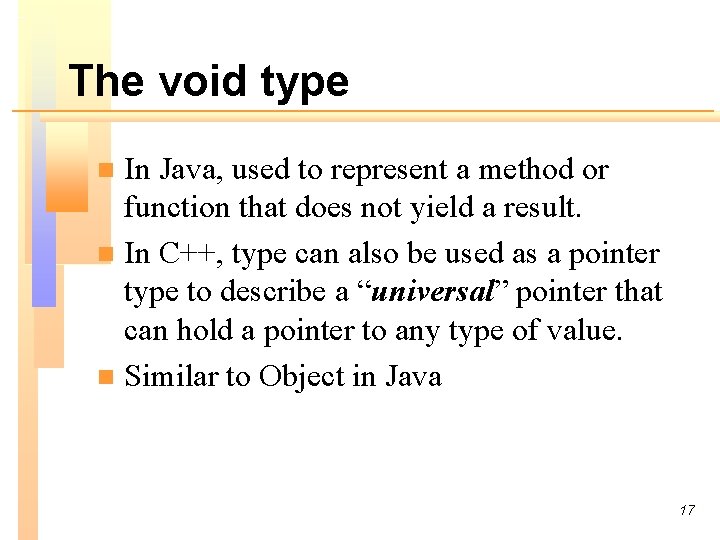
The void type In Java, used to represent a method or function that does not yield a result. n In C++, type can also be used as a pointer type to describe a “universal” pointer that can hold a pointer to any type of value. n Similar to Object in Java n 17
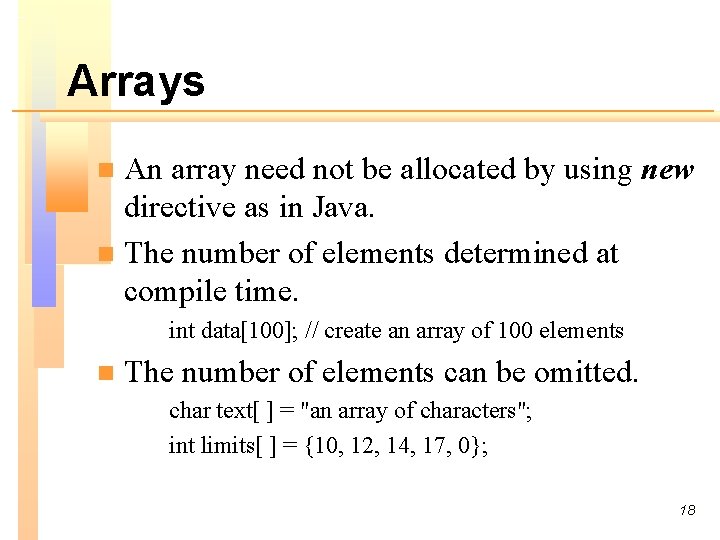
Arrays An array need not be allocated by using new directive as in Java. n The number of elements determined at compile time. n int data[100]; // create an array of 100 elements n The number of elements can be omitted. char text[ ] = "an array of characters"; int limits[ ] = {10, 12, 14, 17, 0}; 18
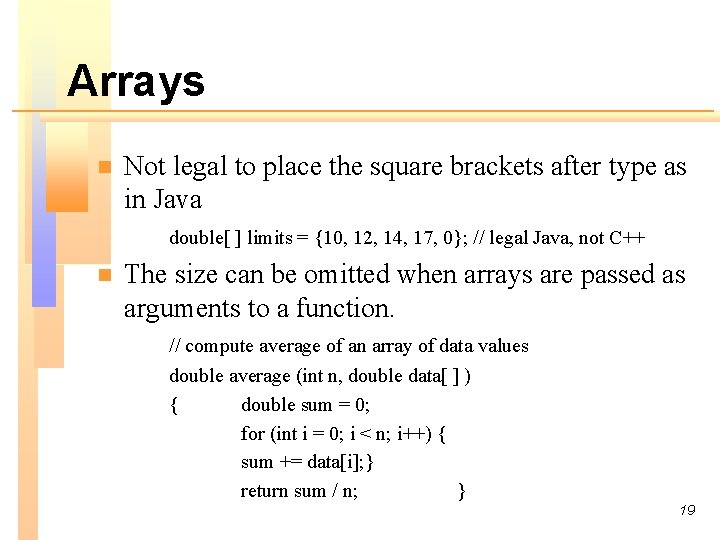
Arrays n Not legal to place the square brackets after type as in Java double[ ] limits = {10, 12, 14, 17, 0}; // legal Java, not C++ n The size can be omitted when arrays are passed as arguments to a function. // compute average of an array of data values double average (int n, double data[ ] ) { double sum = 0; for (int i = 0; i < n; i++) { sum += data[i]; } return sum / n; } 19
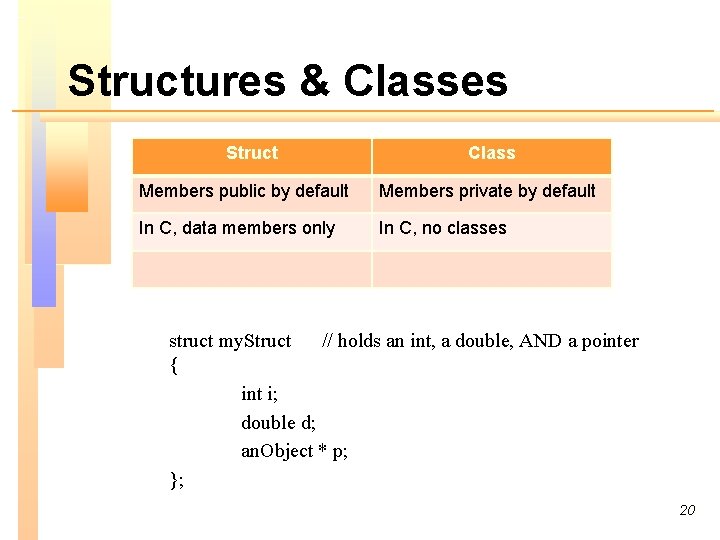
Structures & Classes Struct Class Members public by default Members private by default In C, data members only In C, no classes struct my. Struct // holds an int, a double, AND a pointer { int i; double d; an. Object * p; }; 20
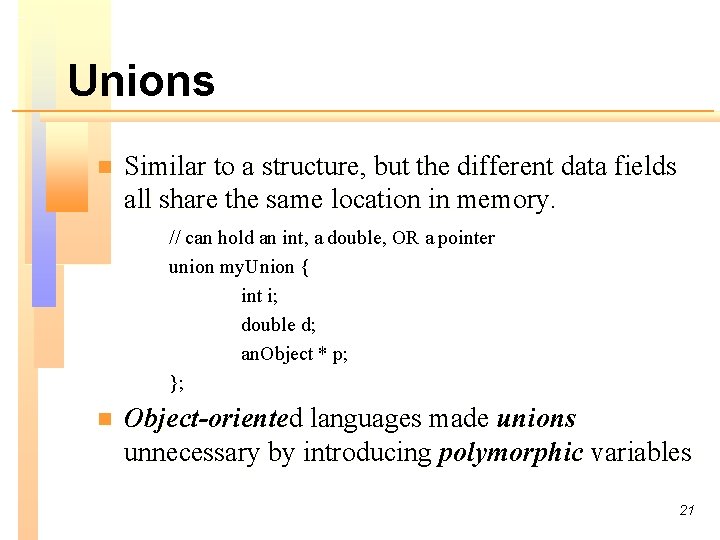
Unions n Similar to a structure, but the different data fields all share the same location in memory. // can hold an int, a double, OR a pointer union my. Union { int i; double d; an. Object * p; }; n Object-oriented languages made unions unnecessary by introducing polymorphic variables 21
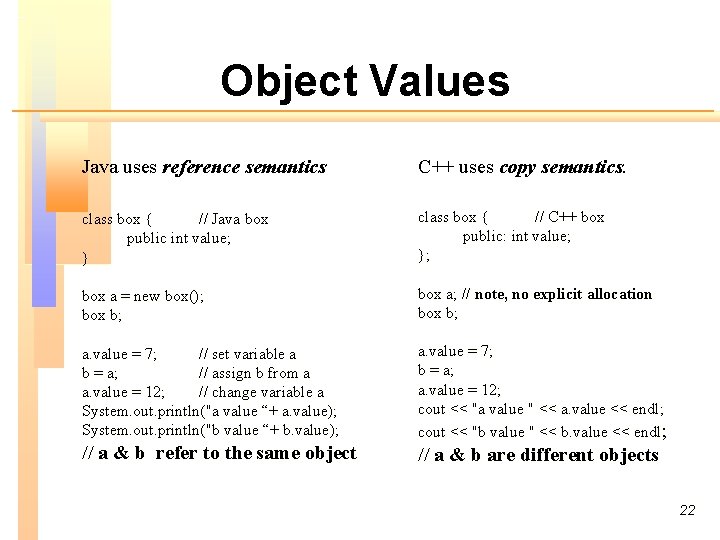
Object Values Java uses reference semantics C++ uses copy semantics. class box { // Java box public int value; } class box { // C++ box public: int value; }; box a = new box(); box b; box a; // note, no explicit allocation box b; a. value = 7; // set variable a b = a; // assign b from a a. value = 12; // change variable a System. out. println("a value “+ a. value); System. out. println("b value “+ b. value); a. value = 7; b = a; a. value = 12; cout << "a value " << a. value << endl; cout << "b value " << b. value << endl; // a & b refer to the same object // a & b are different objects 22
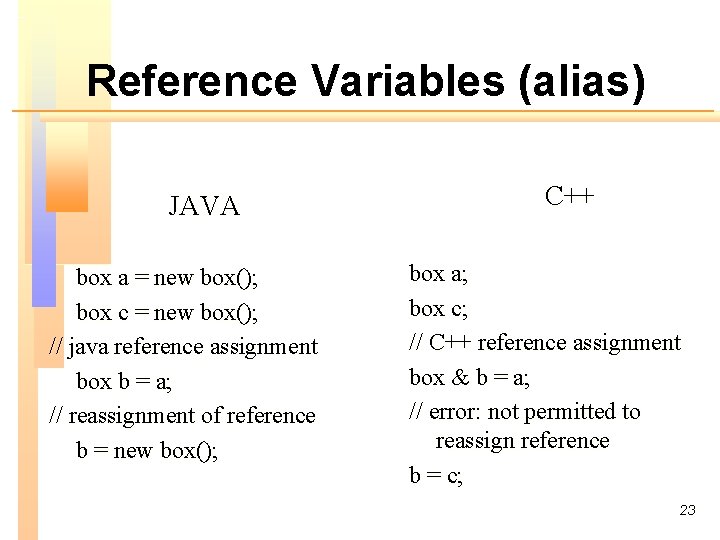
Reference Variables (alias) JAVA box a = new box(); box c = new box(); // java reference assignment box b = a; // reassignment of reference b = new box(); C++ box a; box c; // C++ reference assignment box & b = a; // error: not permitted to reassign reference b = c; 23
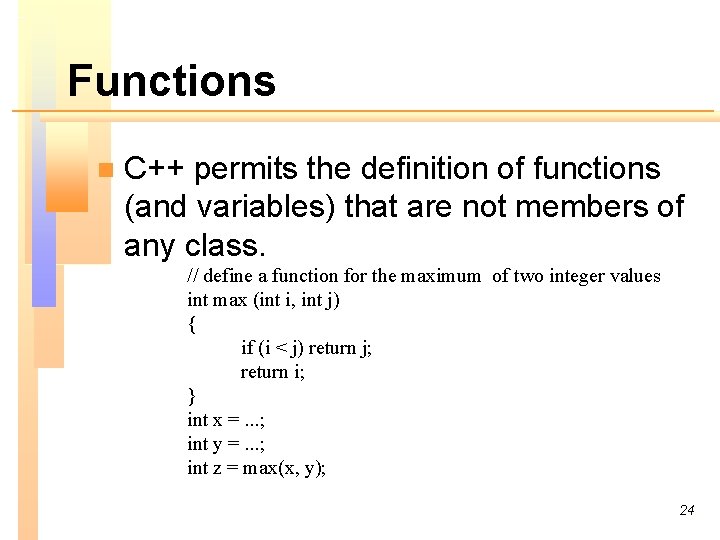
Functions n C++ permits the definition of functions (and variables) that are not members of any class. // define a function for the maximum of two integer values int max (int i, int j) { if (i < j) return j; return i; } int x =. . . ; int y =. . . ; int z = max(x, y); 24
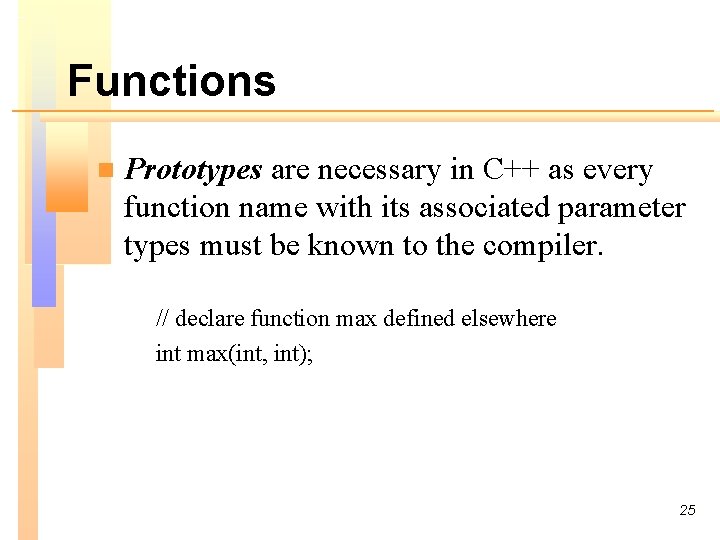
Functions n Prototypes are necessary in C++ as every function name with its associated parameter types must be known to the compiler. // declare function max defined elsewhere int max(int, int); 25
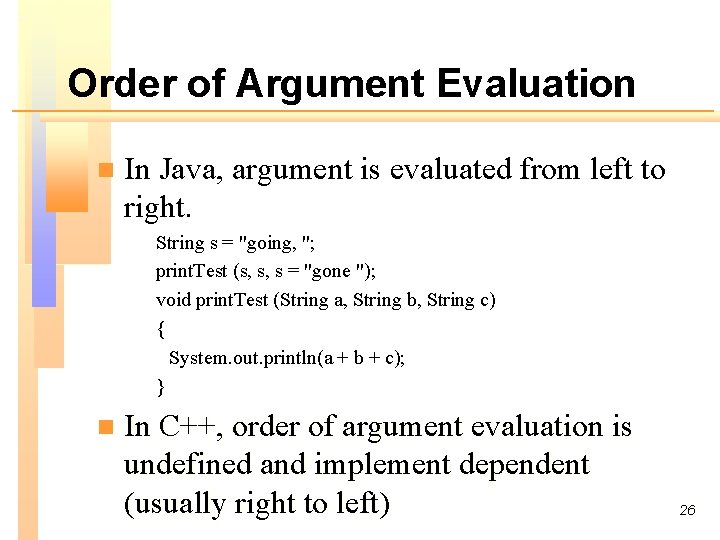
Order of Argument Evaluation n In Java, argument is evaluated from left to right. String s = "going, "; print. Test (s, s, s = "gone "); void print. Test (String a, String b, String c) { System. out. println(a + b + c); } n In C++, order of argument evaluation is undefined and implement dependent (usually right to left) 26
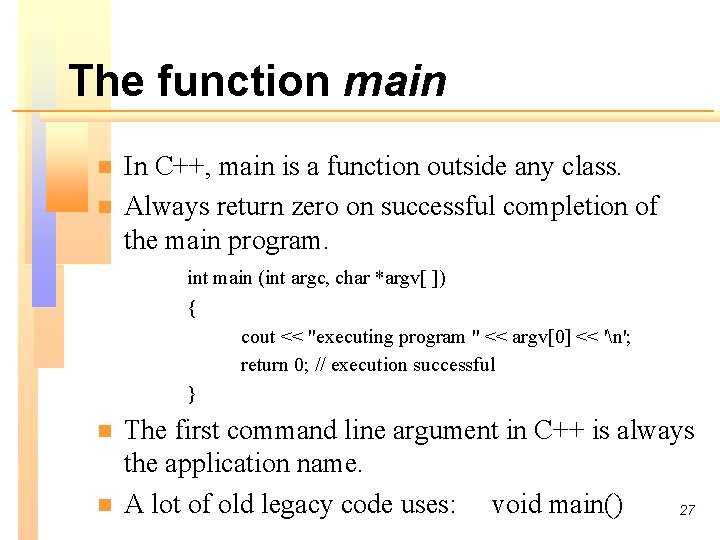
The function main n n In C++, main is a function outside any class. Always return zero on successful completion of the main program. int main (int argc, char *argv[ ]) { cout << "executing program " << argv[0] << 'n'; return 0; // execution successful } n n The first command line argument in C++ is always the application name. A lot of old legacy code uses: void main() 27
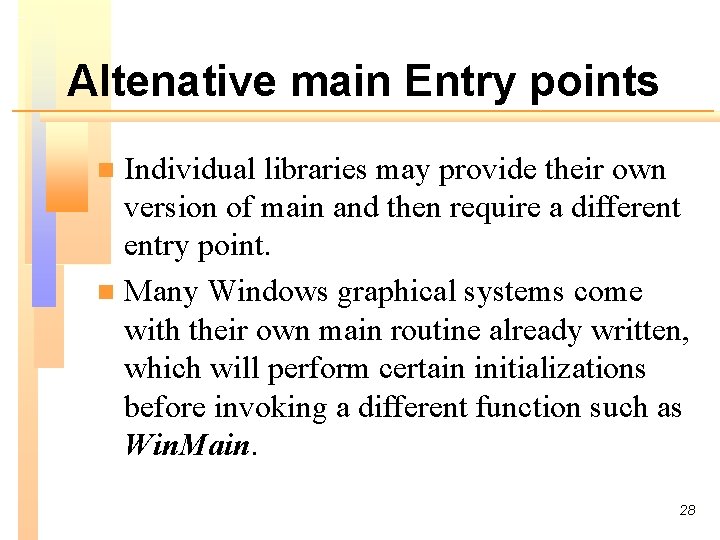
Altenative main Entry points Individual libraries may provide their own version of main and then require a different entry point. n Many Windows graphical systems come with their own main routine already written, which will perform certain initializations before invoking a different function such as Win. Main. n 28
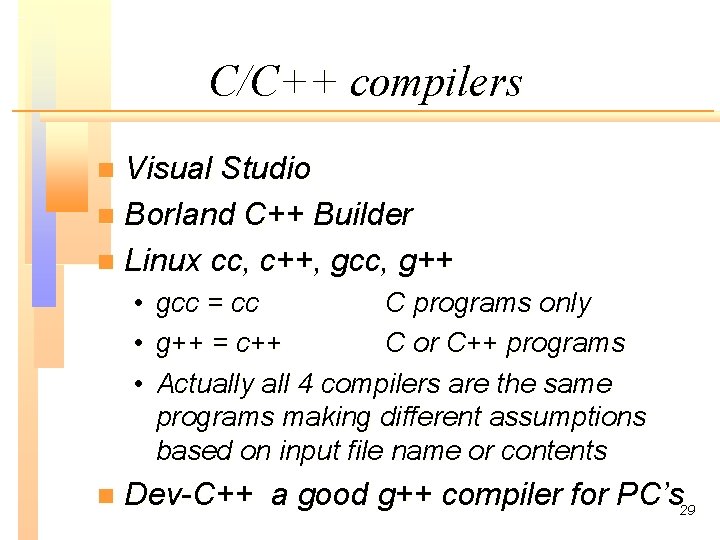
C/C++ compilers Visual Studio n Borland C++ Builder n Linux cc, c++, gcc, g++ n • gcc = cc C programs only • g++ = c++ C or C++ programs • Actually all 4 compilers are the same programs making different assumptions based on input file name or contents n Dev-C++ a good g++ compiler for PC’s 29
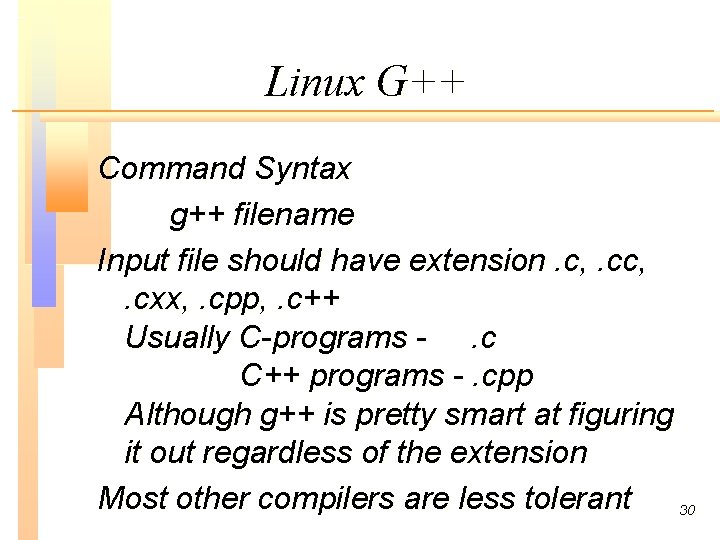
Linux G++ Command Syntax g++ filename Input file should have extension. c, . cxx, . cpp, . c++ Usually C-programs - . c C++ programs -. cpp Although g++ is pretty smart at figuring it out regardless of the extension Most other compilers are less tolerant 30
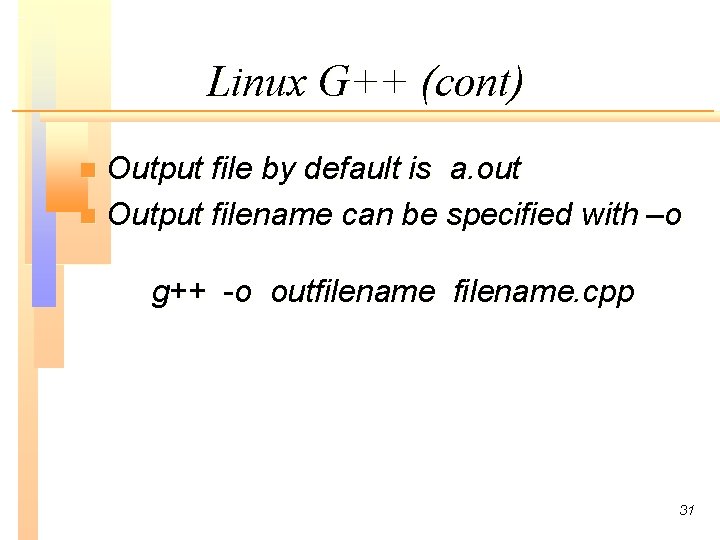
Linux G++ (cont) Output file by default is a. out n Output filename can be specified with –o g++ -o outfilename. cpp n 31
![Simple Programs JAVA public class Hello World public static void mainString args Simple Programs JAVA public class Hello. World { public static void main(String[] args) {](https://slidetodoc.com/presentation_image/4ab0bac430ad4fbae65cfa0225809e91/image-32.jpg)
Simple Programs JAVA public class Hello. World { public static void main(String[] args) { System. out. println("Hello World"); } } C #include <stdio. h> void main (int argc, char argv[]) { printf(“Hello Worldn); } 32
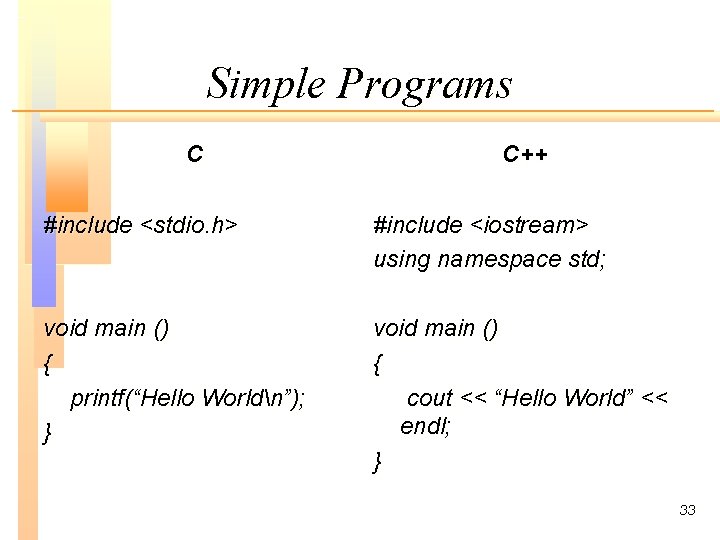
Simple Programs C C++ #include <stdio. h> #include <iostream> using namespace std; void main () { printf(“Hello Worldn”); } void main () { cout << “Hello World” << endl; } 33
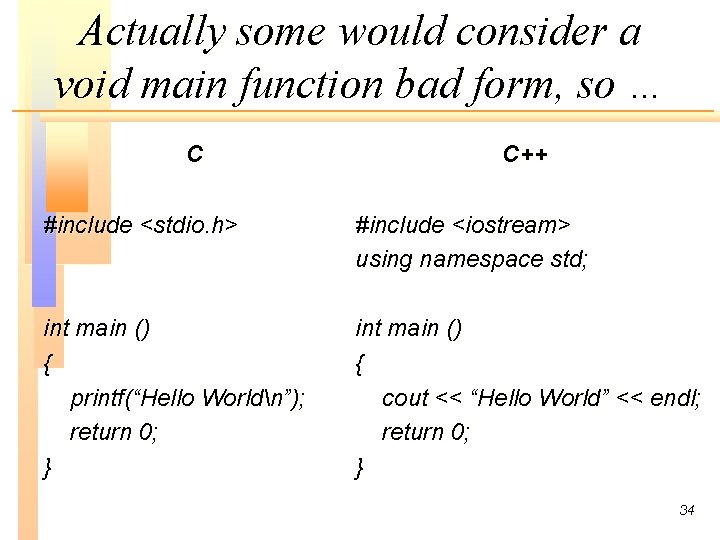
Actually some would consider a void main function bad form, so … C C++ #include <stdio. h> #include <iostream> using namespace std; int main () { printf(“Hello Worldn”); return 0; } int main () { cout << “Hello World” << endl; return 0; } 34
Uml for java programmers
Tcp/ip sockets in java: practical guide for programmers
Is bool a fundamental data type in c++
Java for c programmers
Programmers use backdoors to debug and test programs.
Implementation of uims
Linux programmers manual
Programmers guild
Kontinuitetshantering i praktiken
Typiska novell drag
Nationell inriktning för artificiell intelligens
Returpilarna
Varför kallas perioden 1918-1939 för mellankrigstiden?
En lathund för arbete med kontinuitetshantering
Kassaregister ideell förening
Tidbok
Sura för anatom
Förklara densitet för barn
Datorkunskap för nybörjare
Stig kerman
Hur skriver man en tes
Delegerande ledarskap
Nyckelkompetenser för livslångt lärande
Påbyggnader för flakfordon
Formel för lufttryck
Offentlig förvaltning
Jag har nigit för nymånens skära
Presentera för publik crossboss
Jiddisch
Kanaans land
Treserva lathund
Mjälthilus
Claes martinsson
Centrum för kunskap och säkerhet
Verifikationsplan