Java for C Programmers First Night Overview First
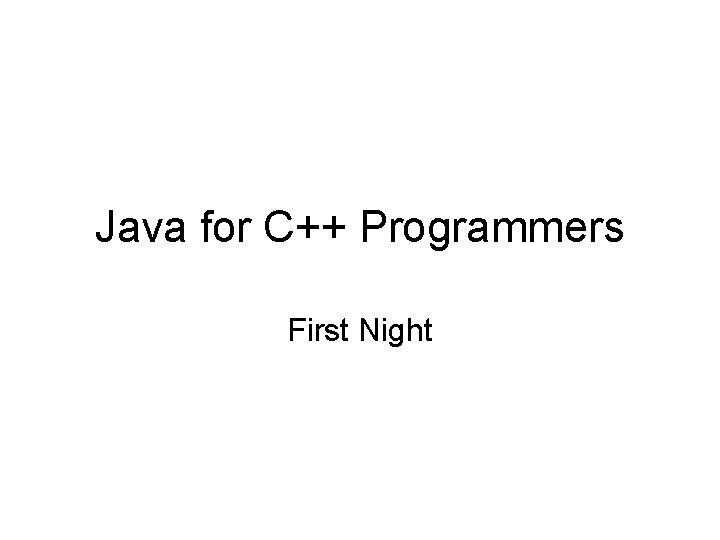
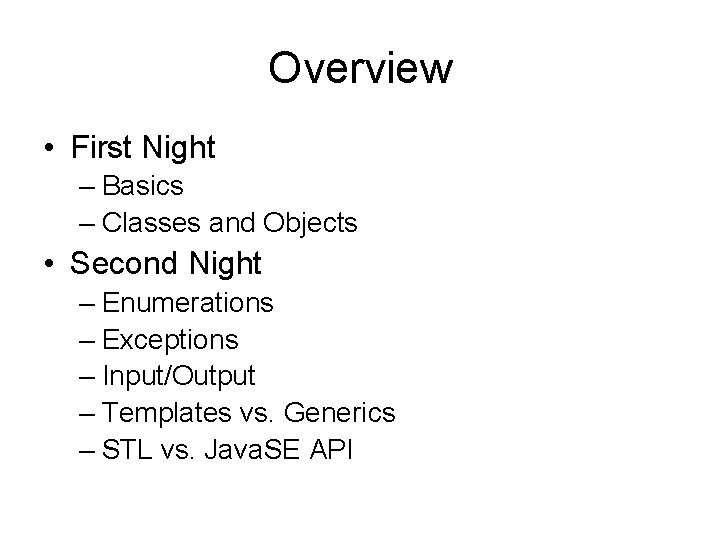
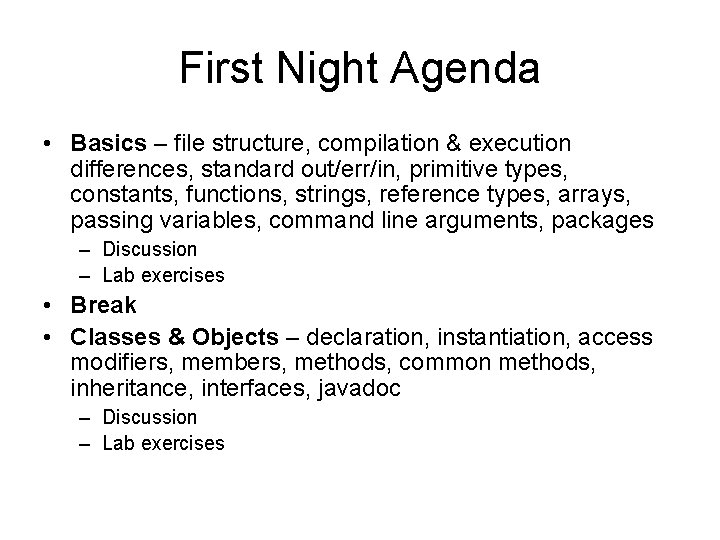
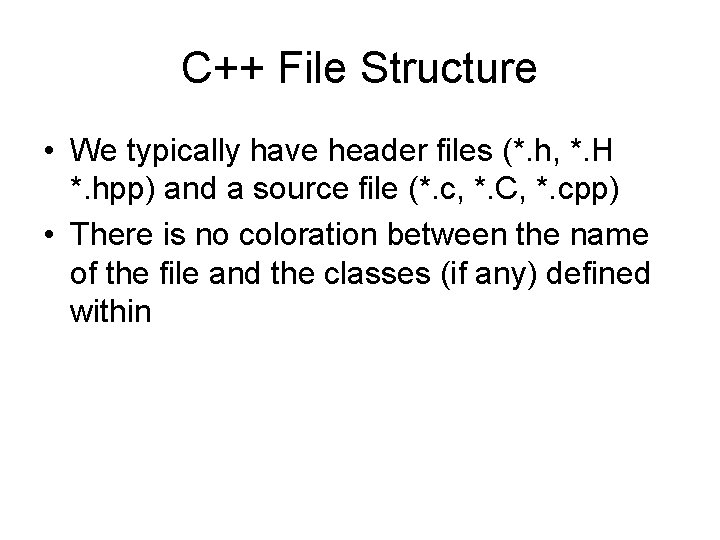
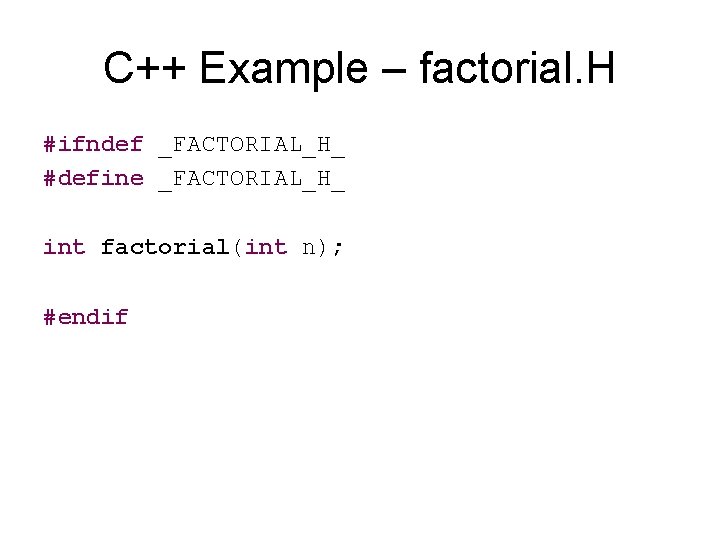
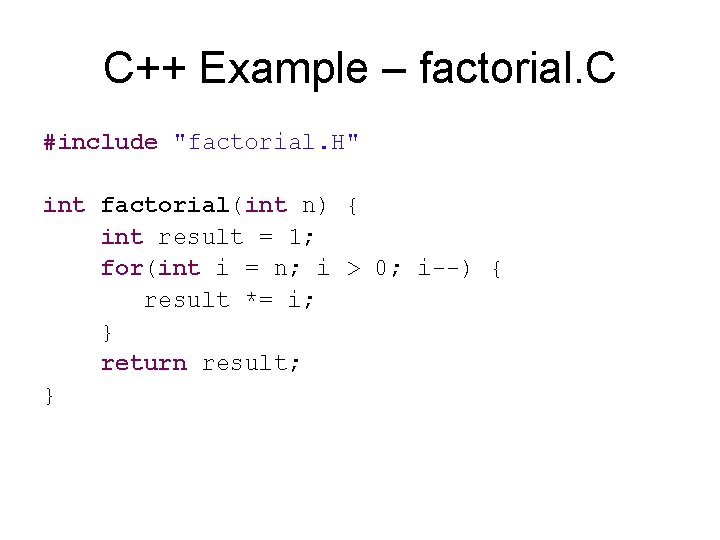
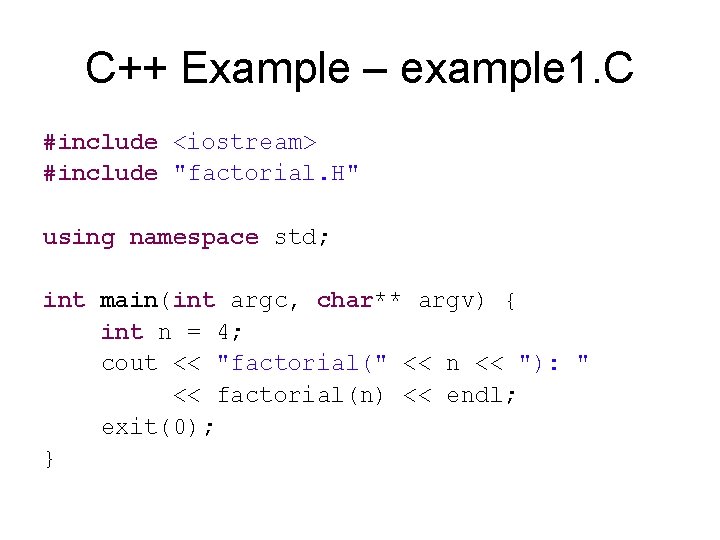
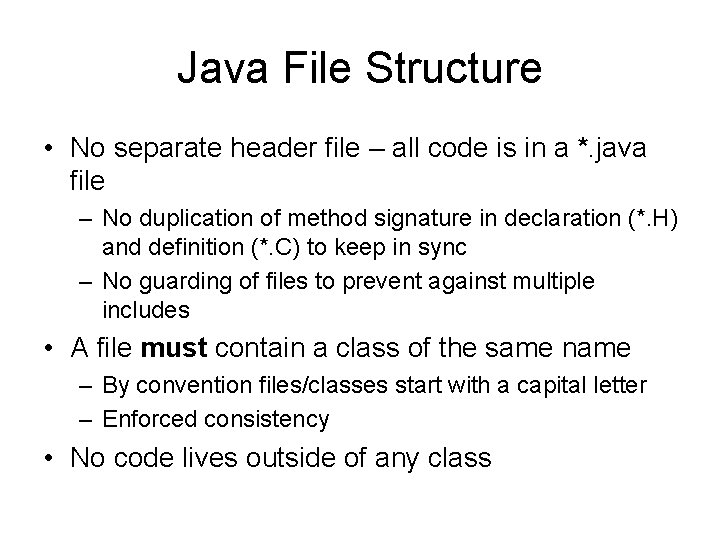
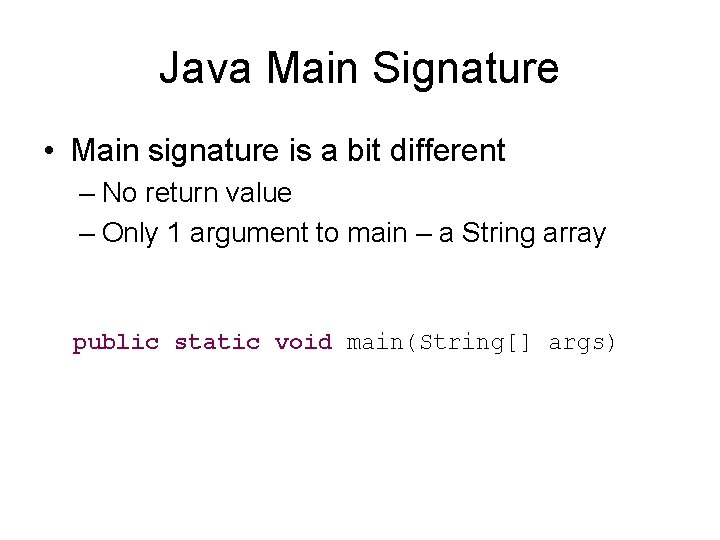
![Java Factorial Example public class Factorial { public static void main(String[] args) { int Java Factorial Example public class Factorial { public static void main(String[] args) { int](https://slidetodoc.com/presentation_image_h/349b7b536872183dfb14e0b76266de9e/image-10.jpg)
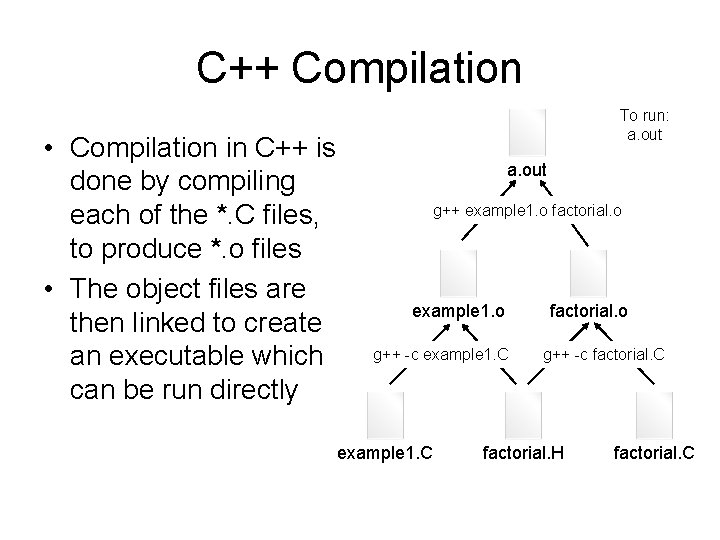
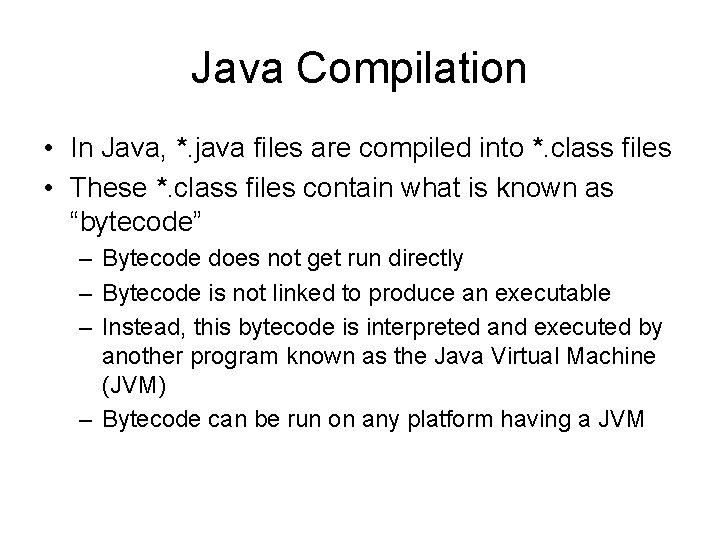
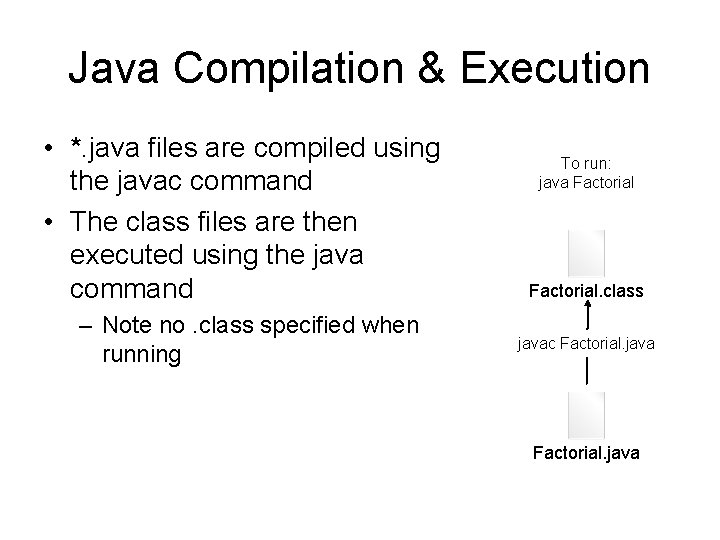
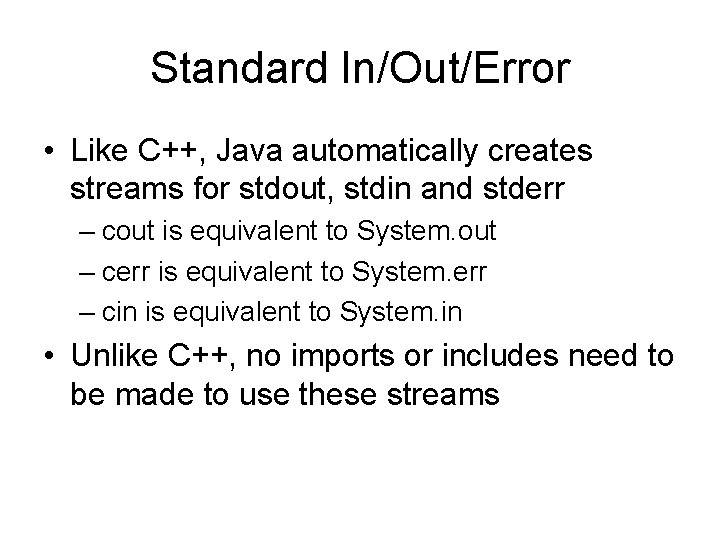
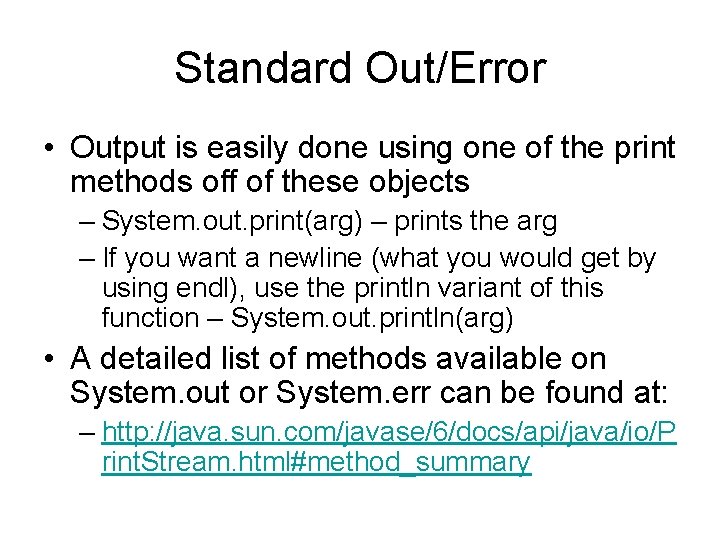
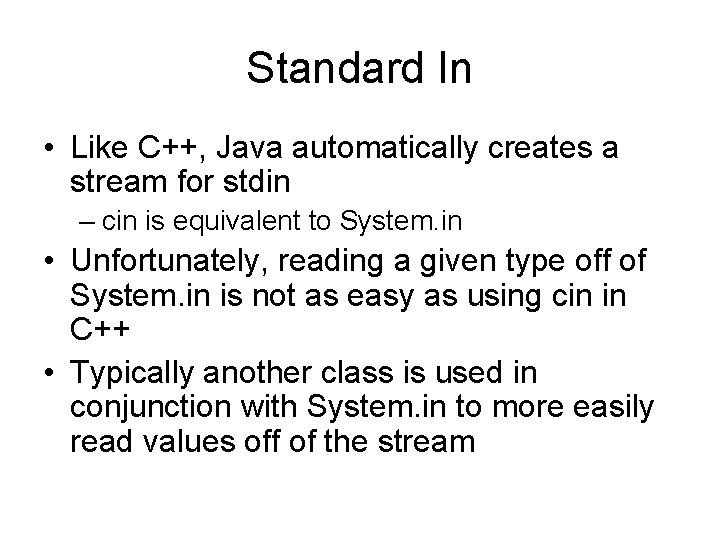
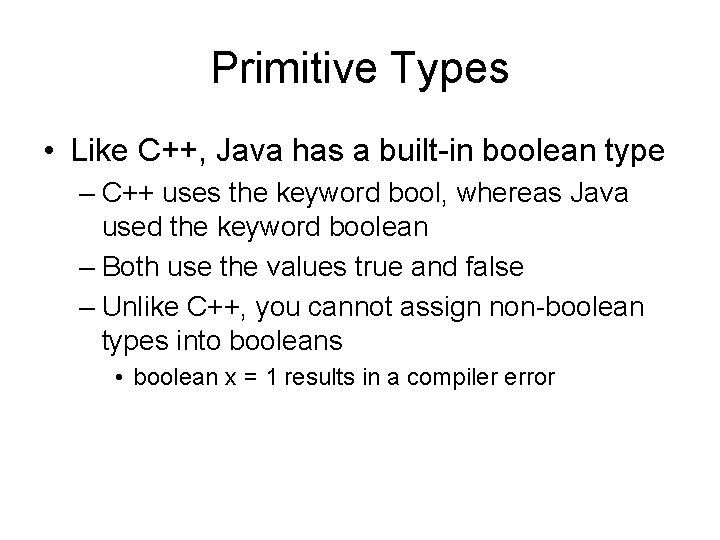
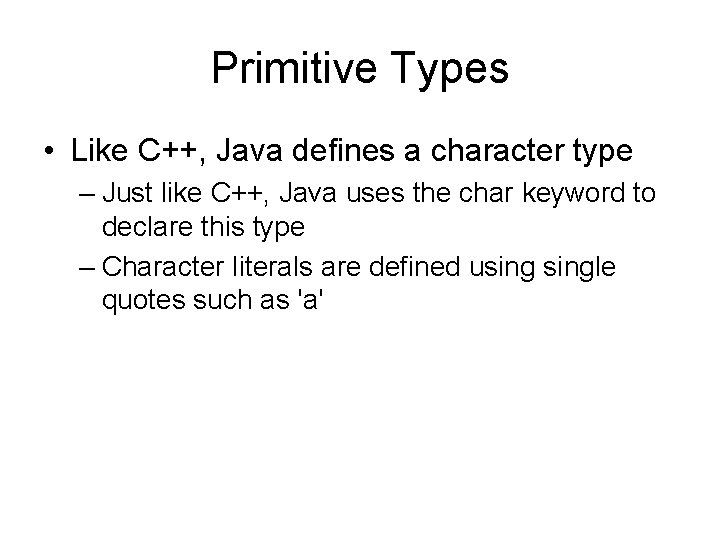
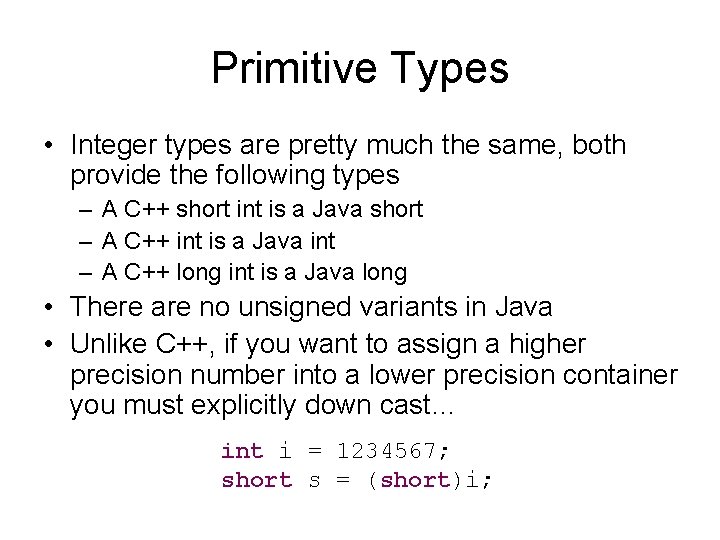
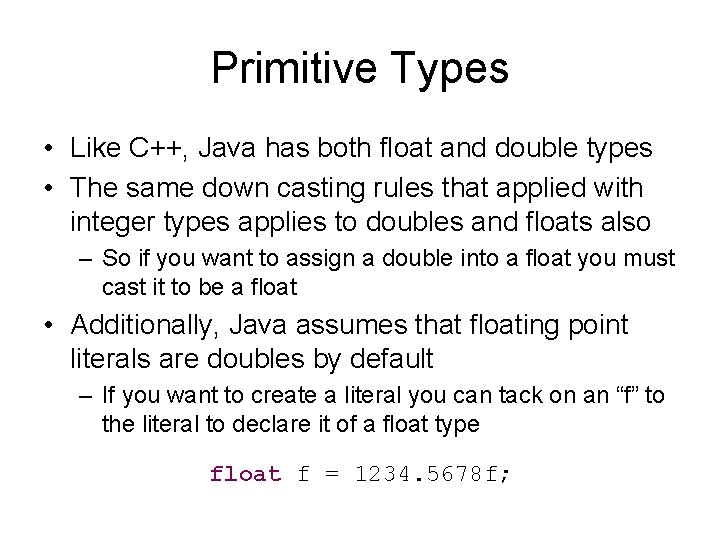
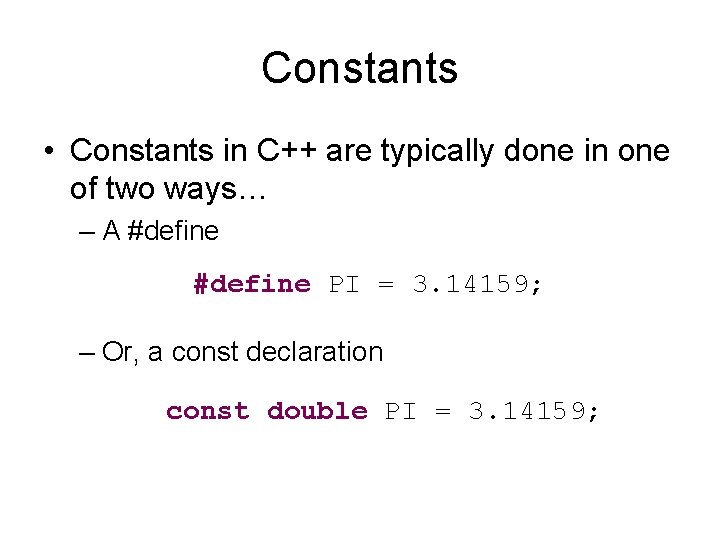
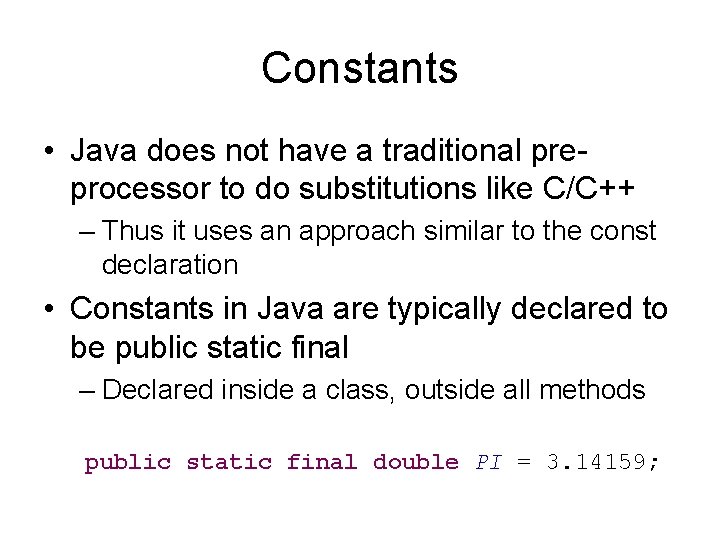
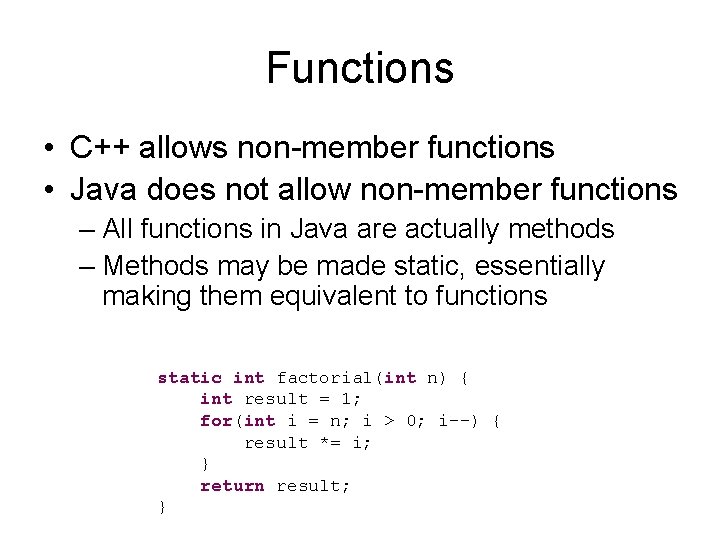
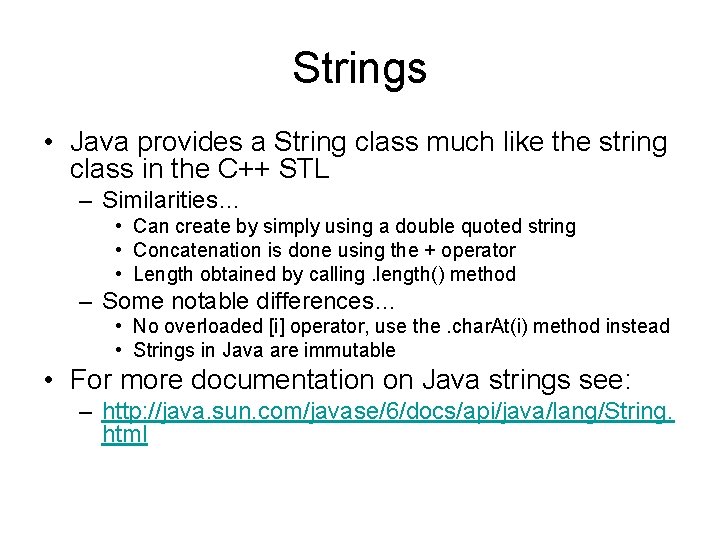
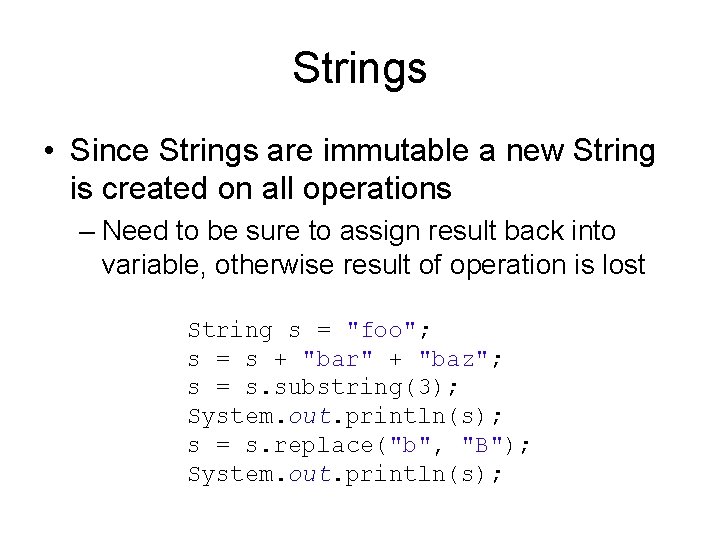
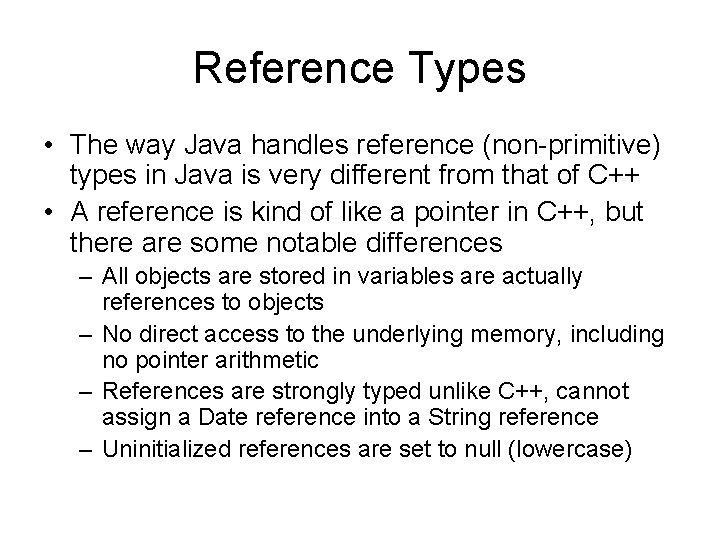
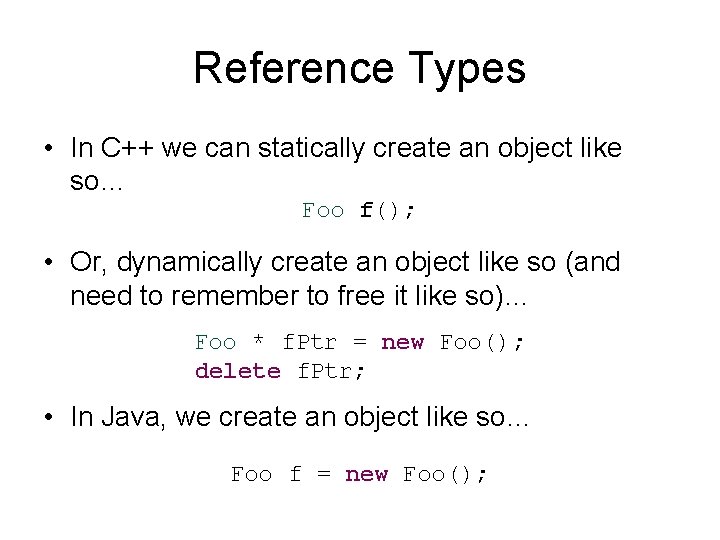
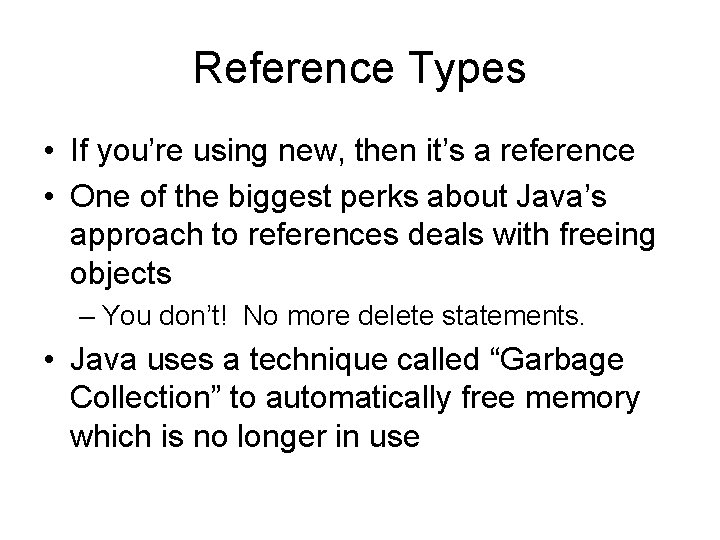
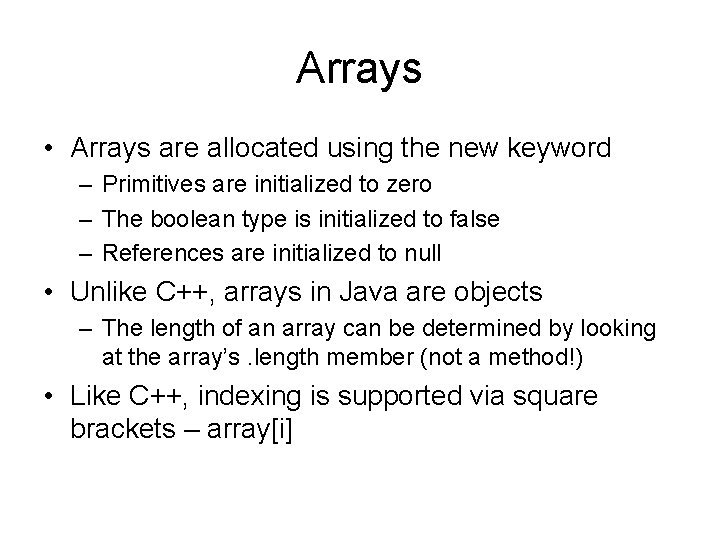
![Array Allocation // example declarations int[] int. Array = new int[10]; boolean[] bool. Array Array Allocation // example declarations int[] int. Array = new int[10]; boolean[] bool. Array](https://slidetodoc.com/presentation_image_h/349b7b536872183dfb14e0b76266de9e/image-30.jpg)
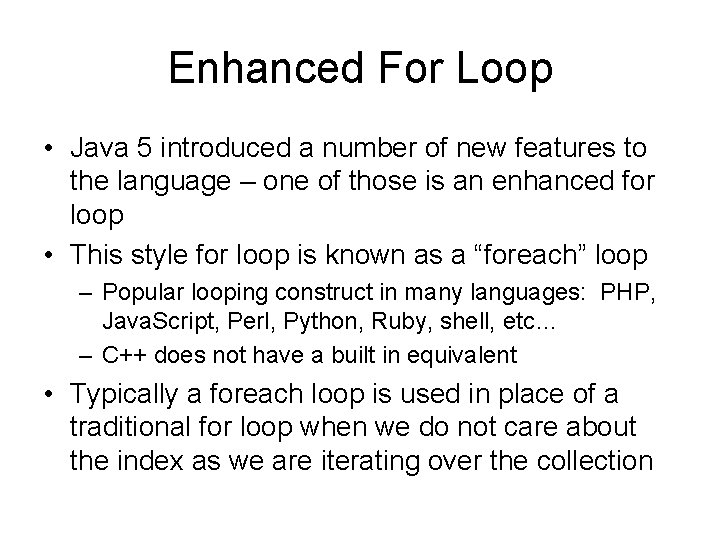
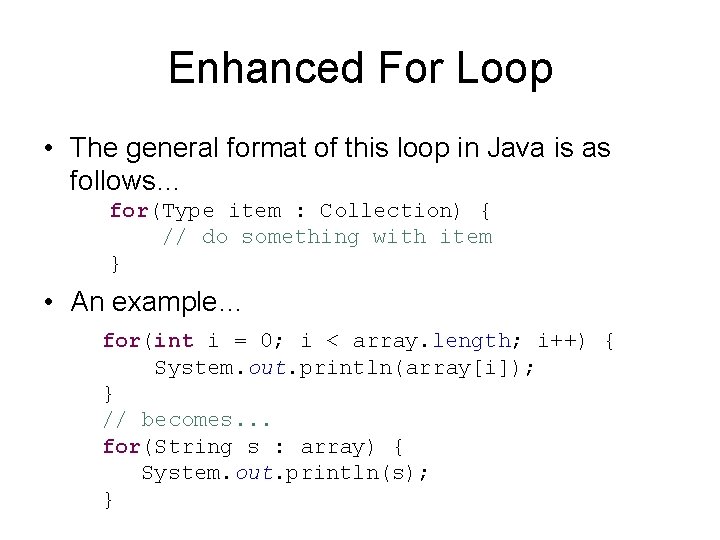
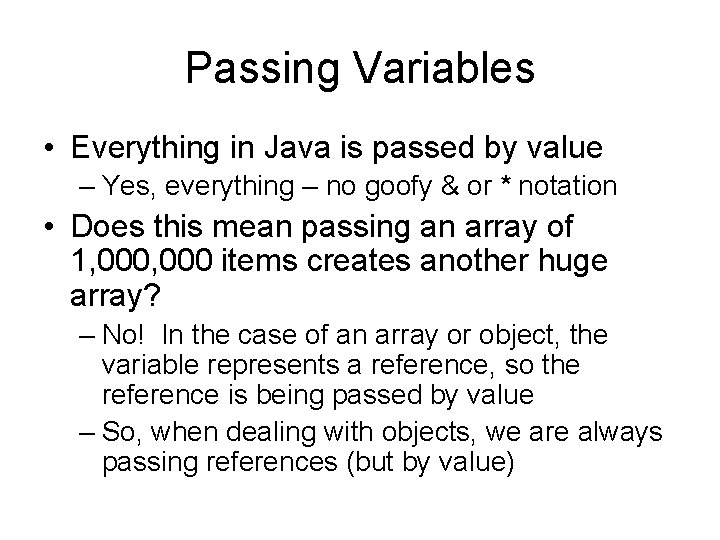
![Passing Variables public class Test { public static void main(String[] args) { int[] array Passing Variables public class Test { public static void main(String[] args) { int[] array](https://slidetodoc.com/presentation_image_h/349b7b536872183dfb14e0b76266de9e/image-34.jpg)
![Passing Variables public class Test { public static void main(String[] args) { int[] array Passing Variables public class Test { public static void main(String[] args) { int[] array](https://slidetodoc.com/presentation_image_h/349b7b536872183dfb14e0b76266de9e/image-35.jpg)
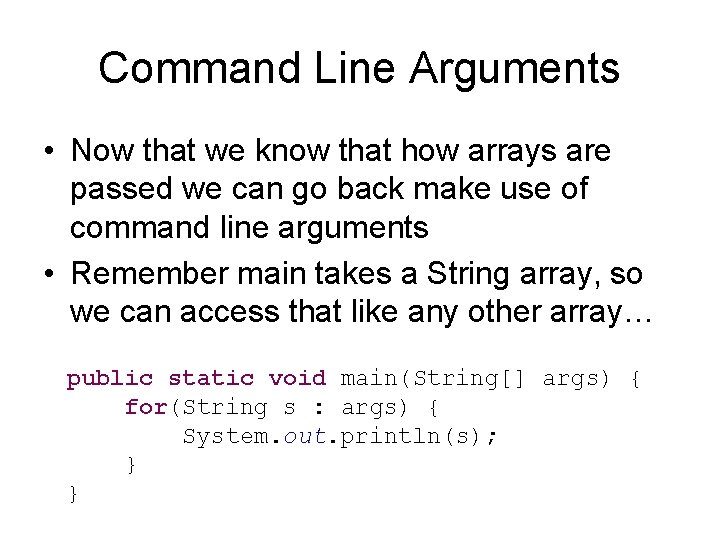
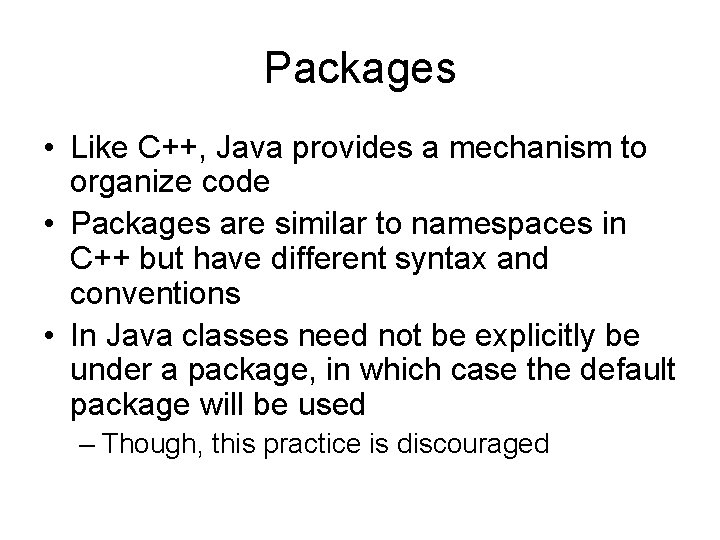
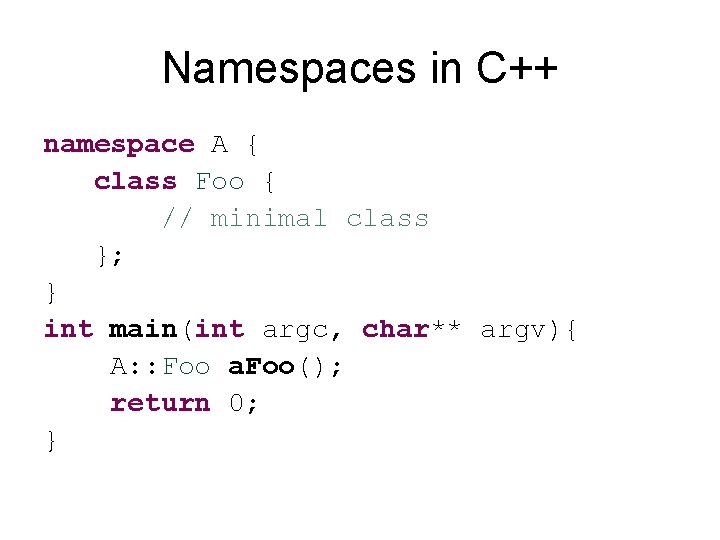
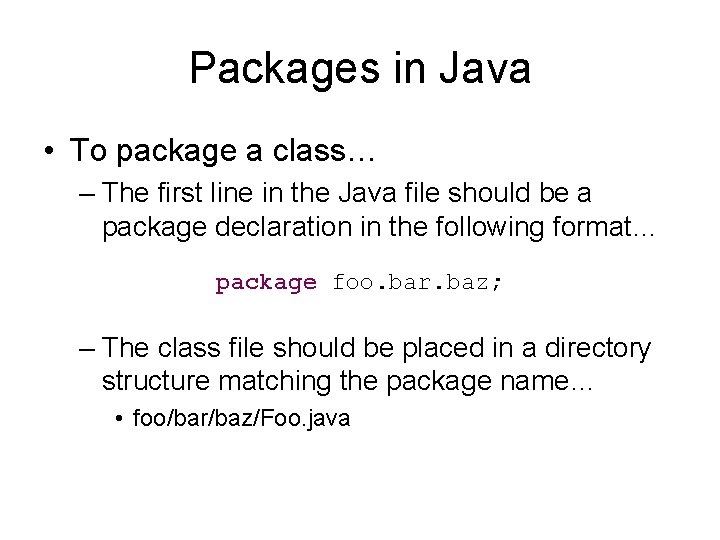
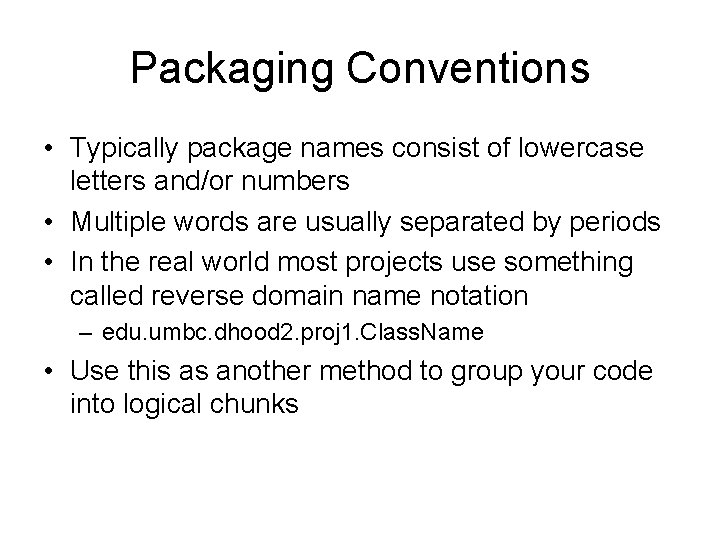
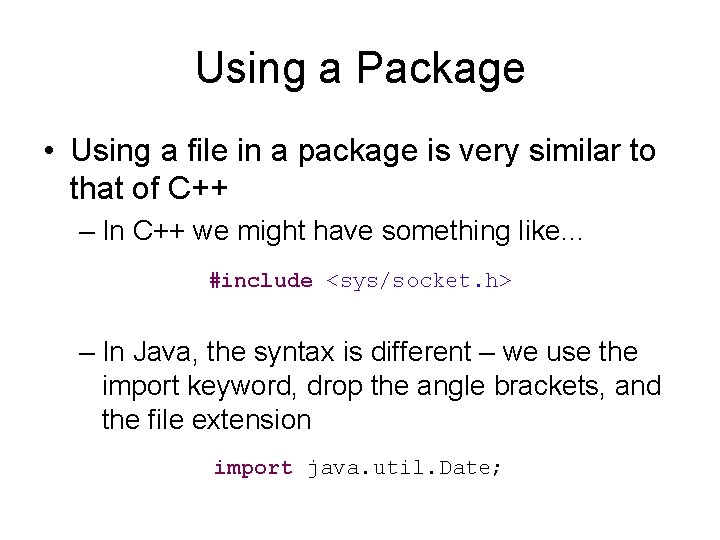
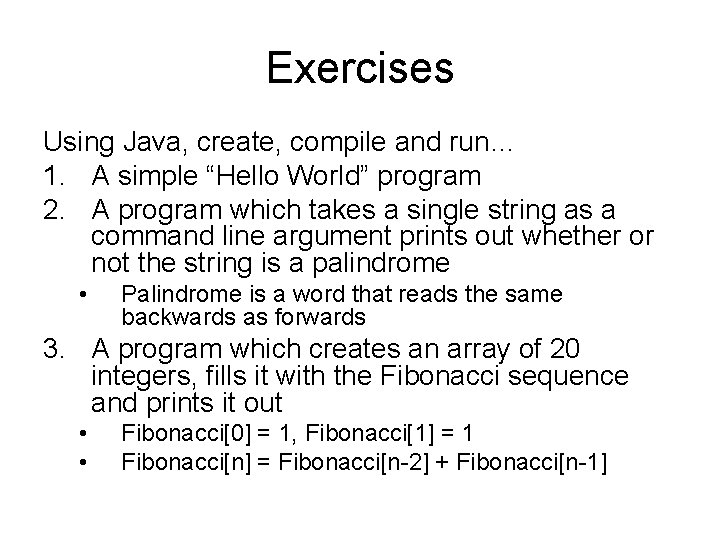
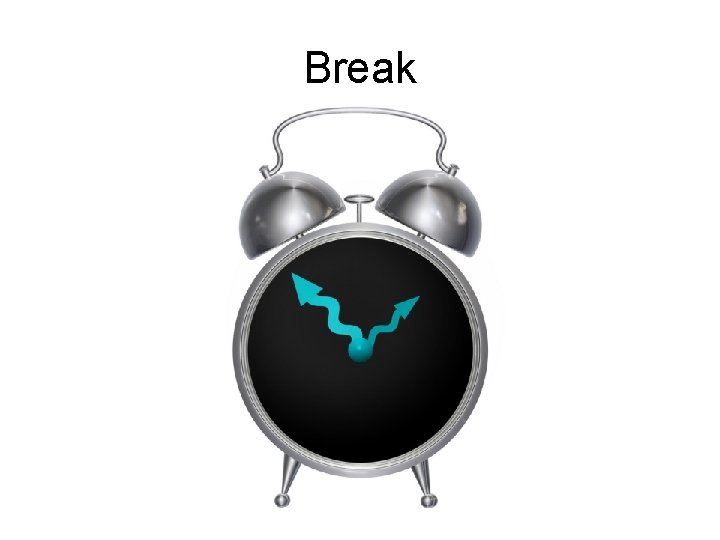
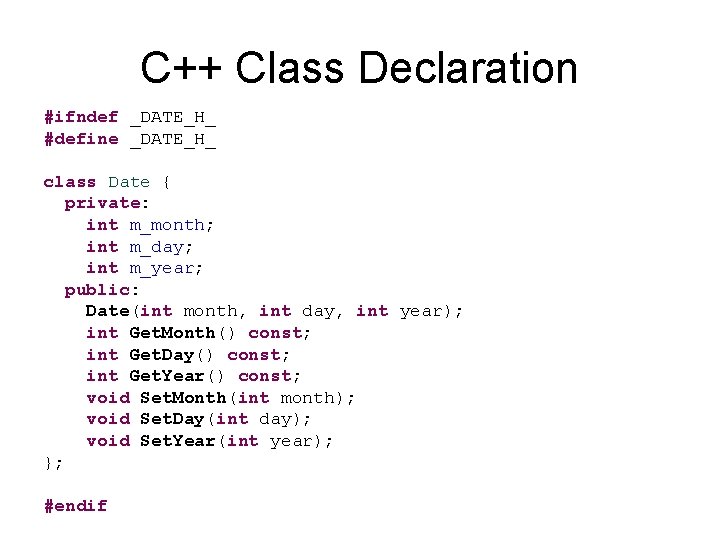
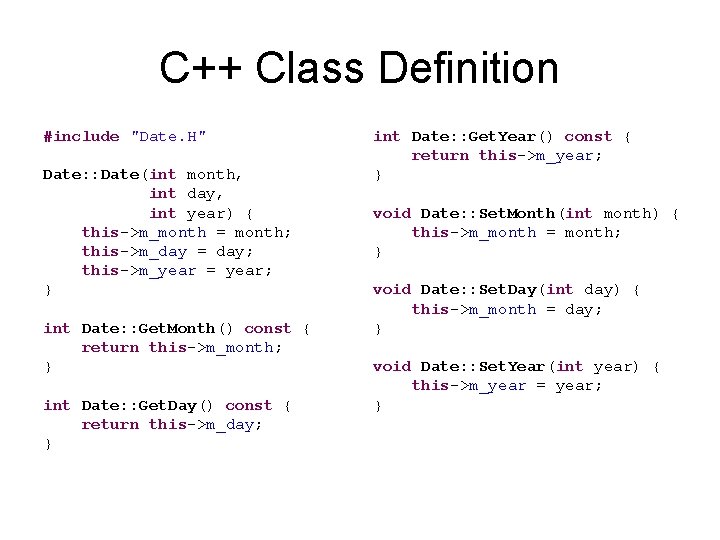
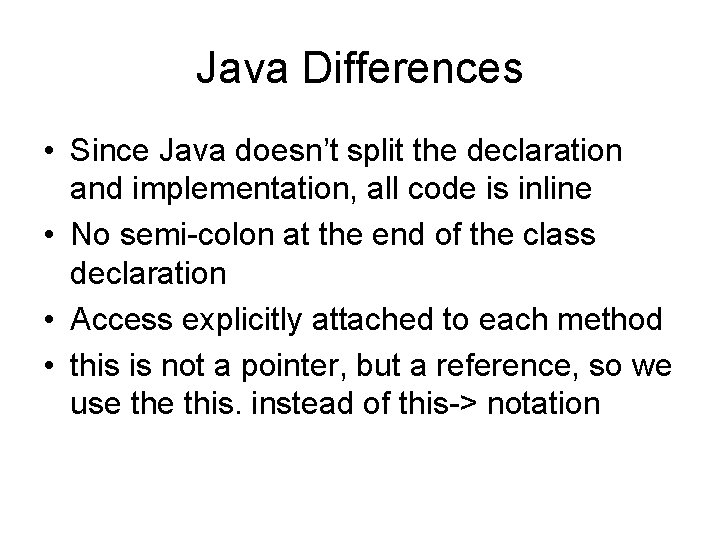
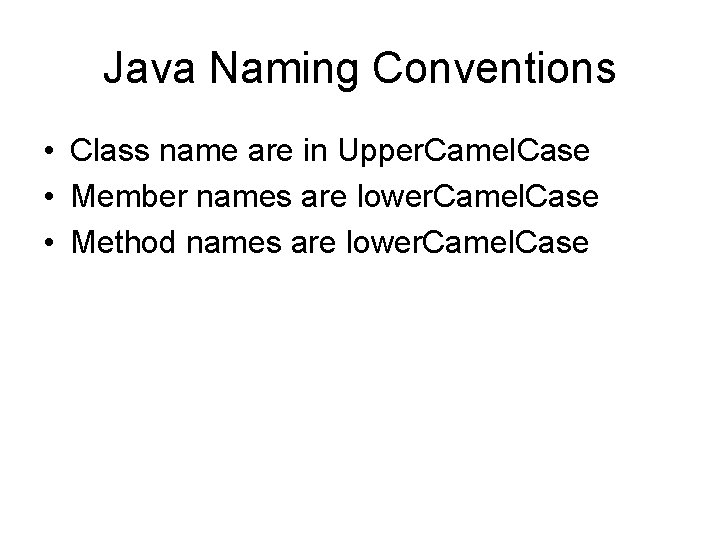
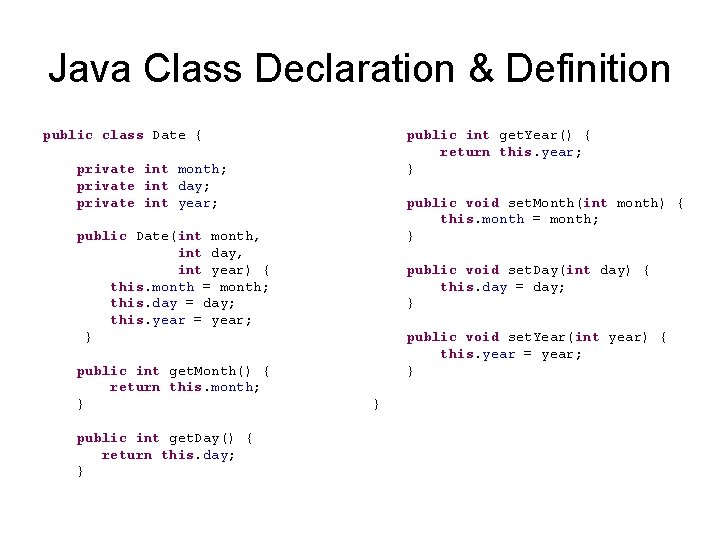
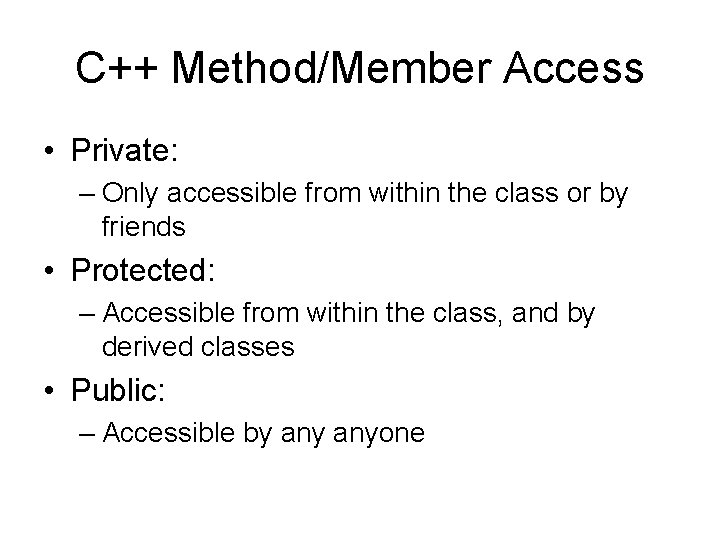
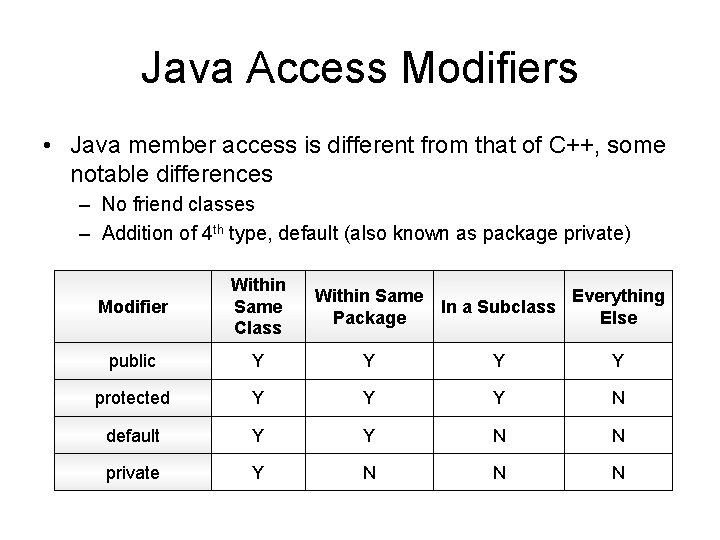
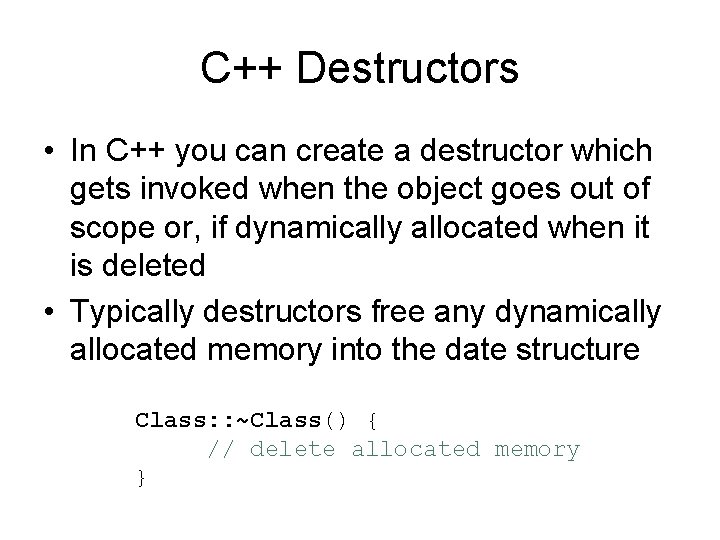
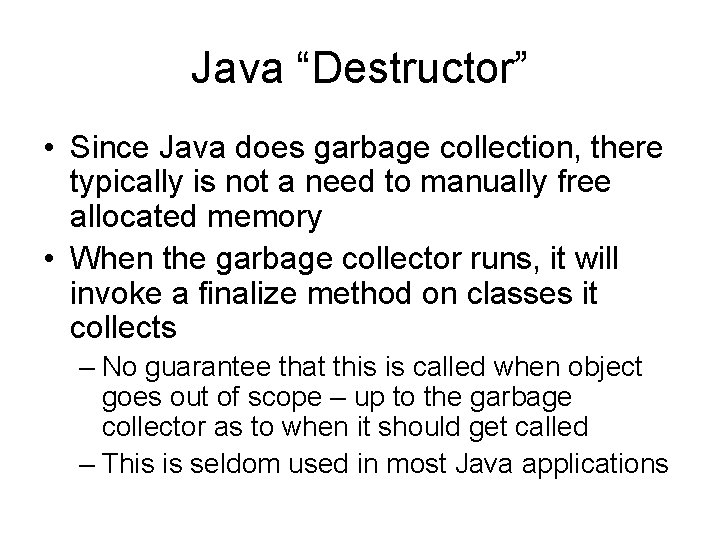
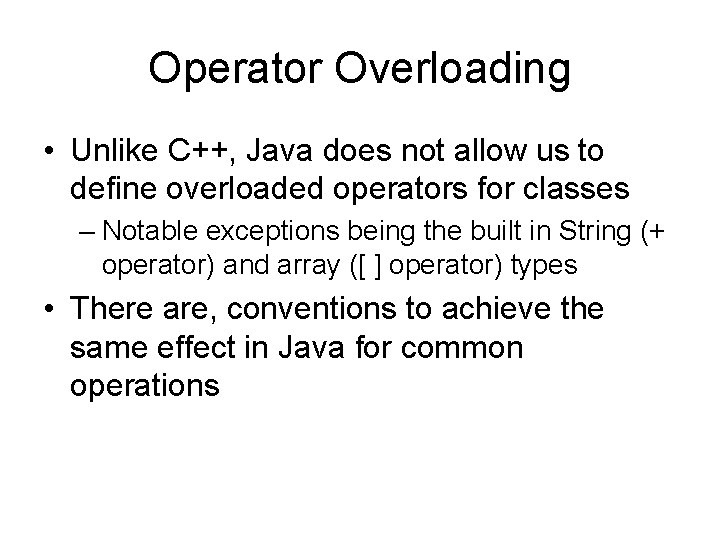
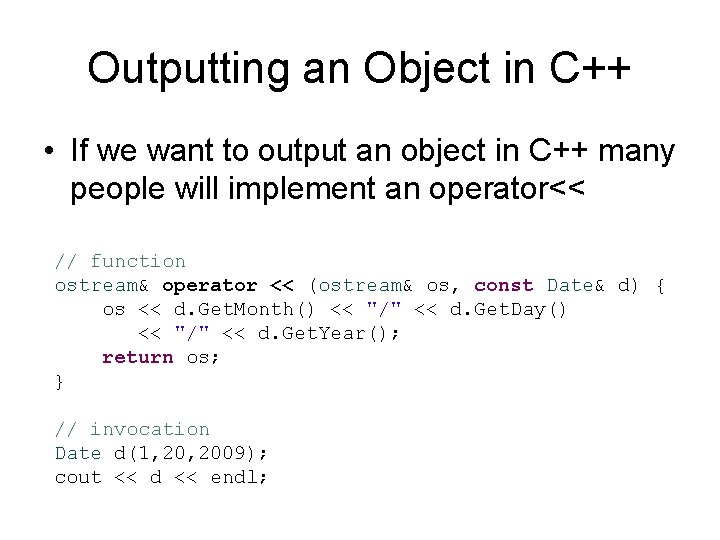
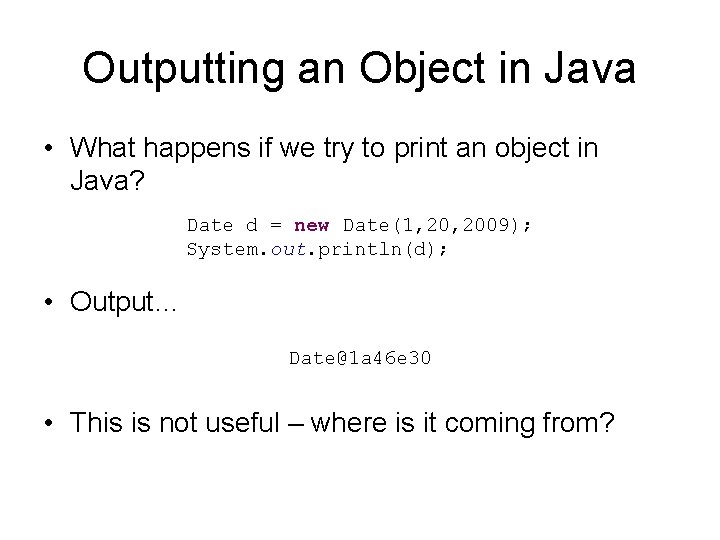
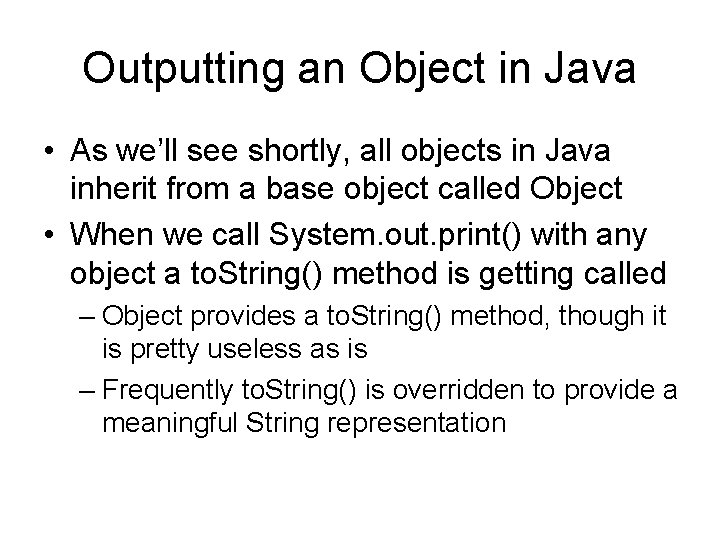
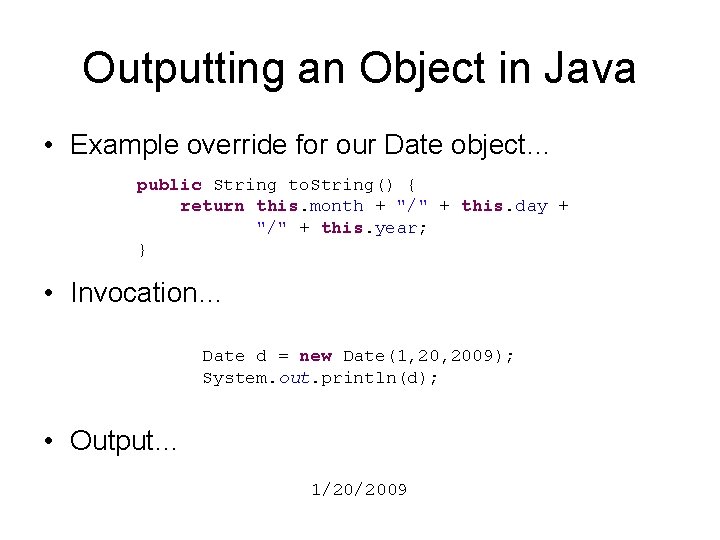
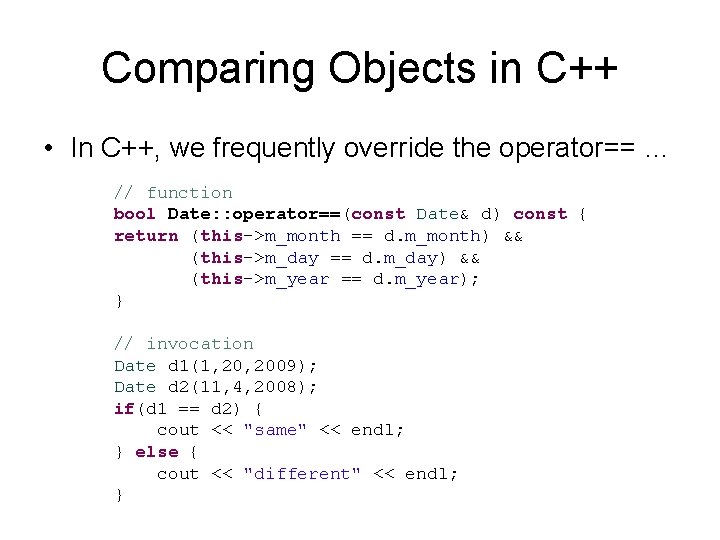
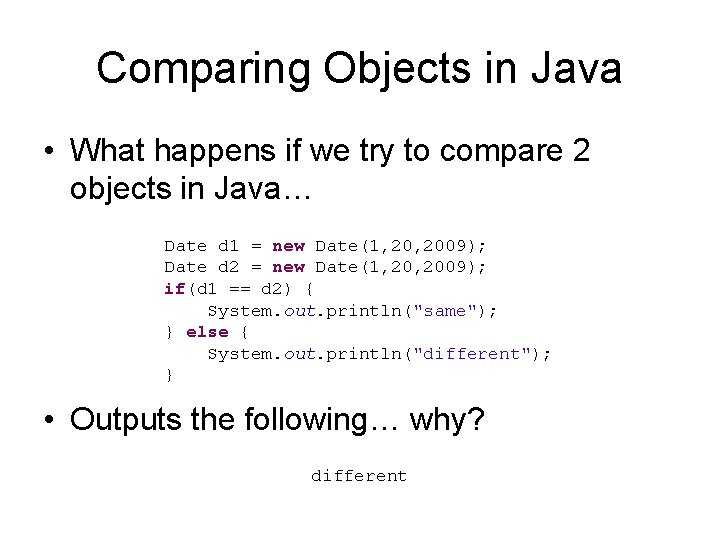
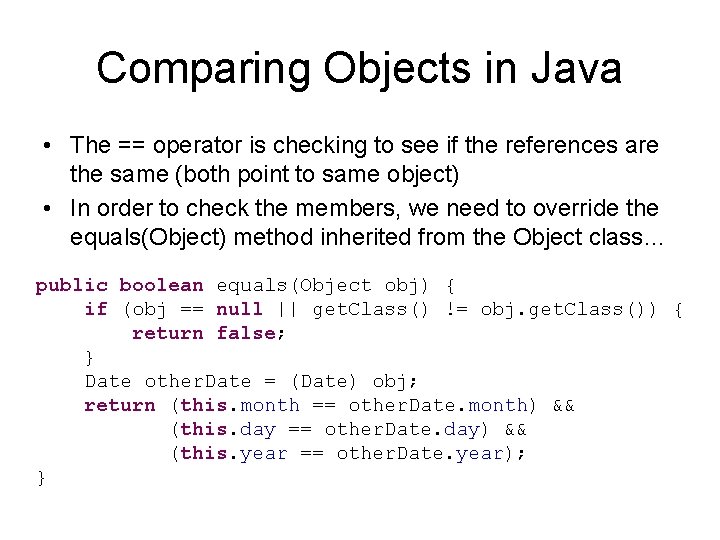
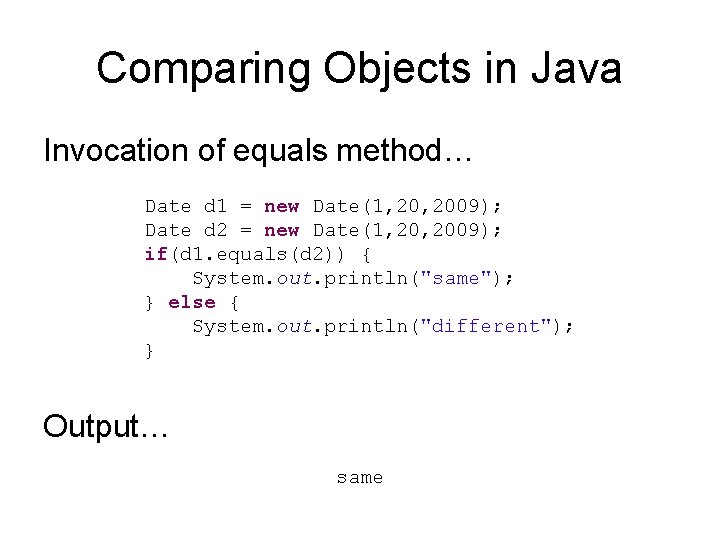
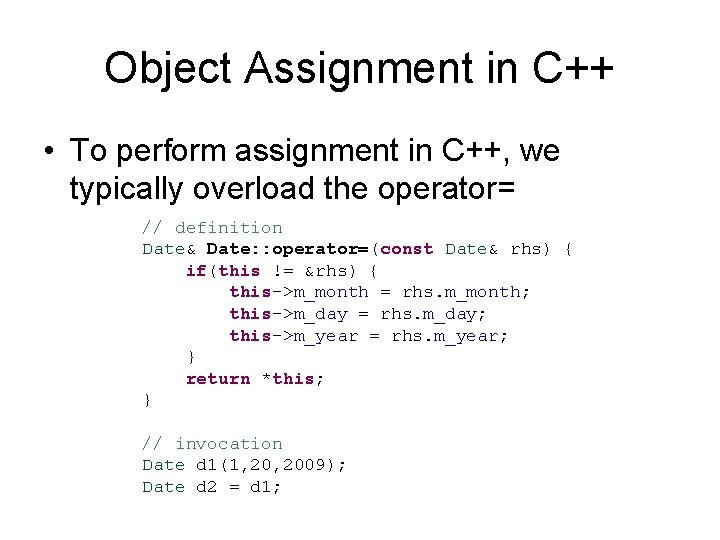
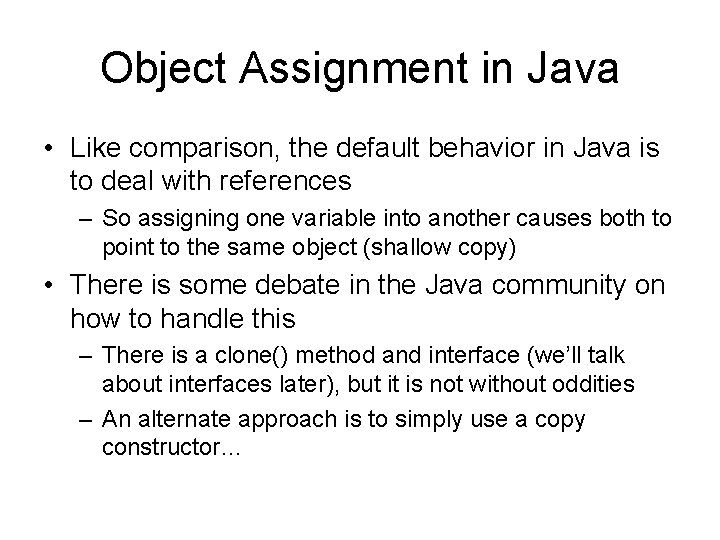
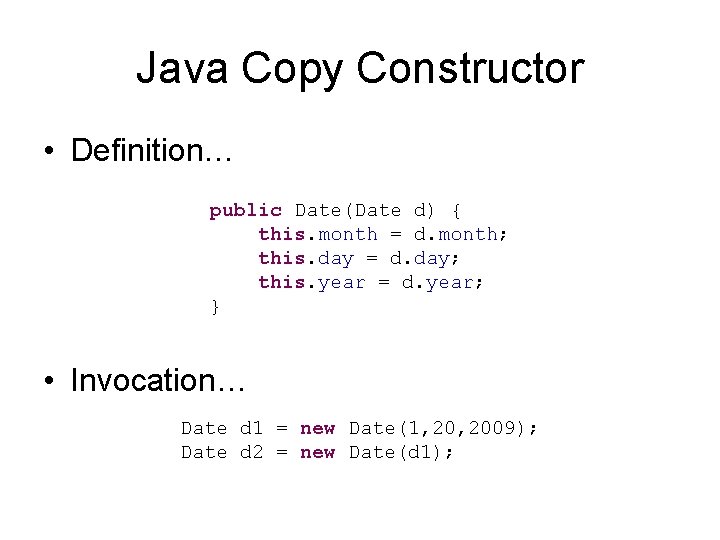
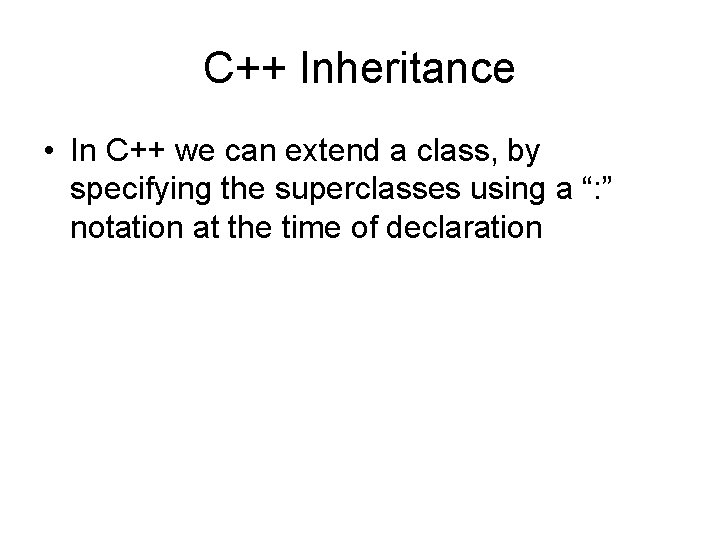
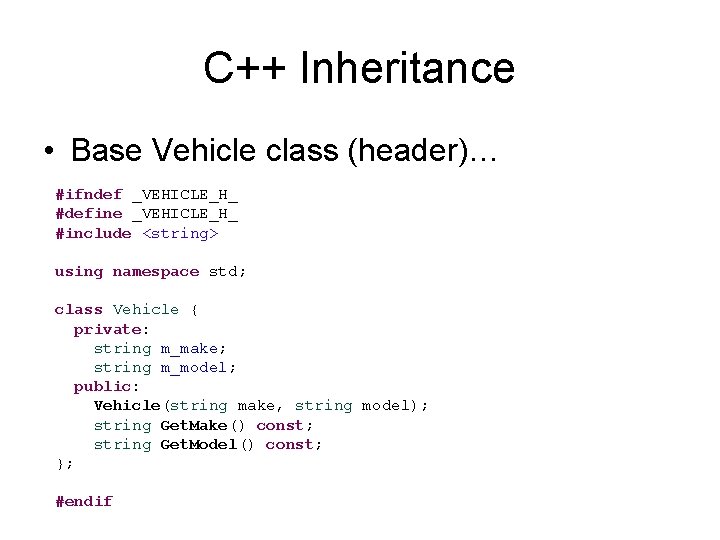
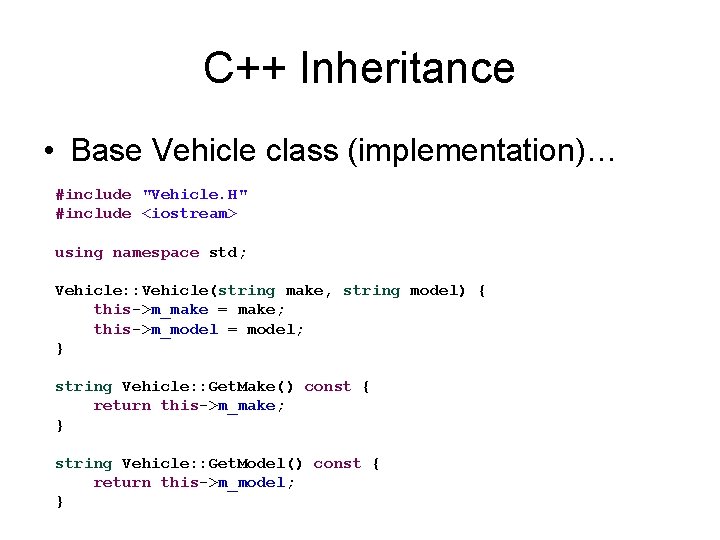
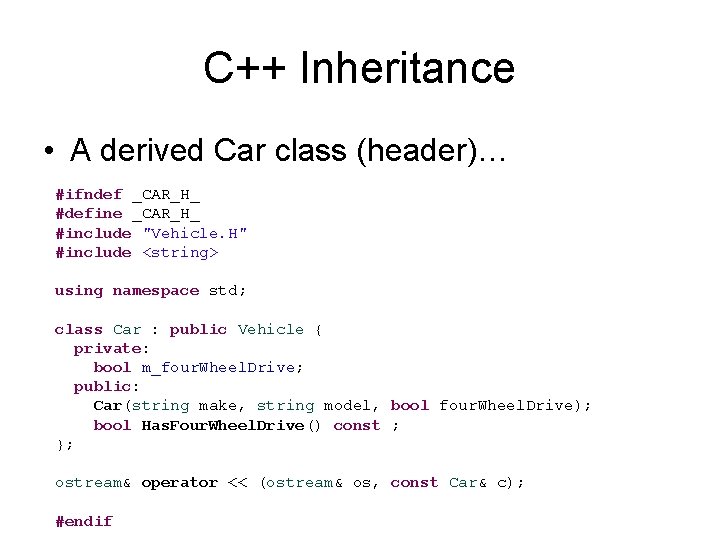
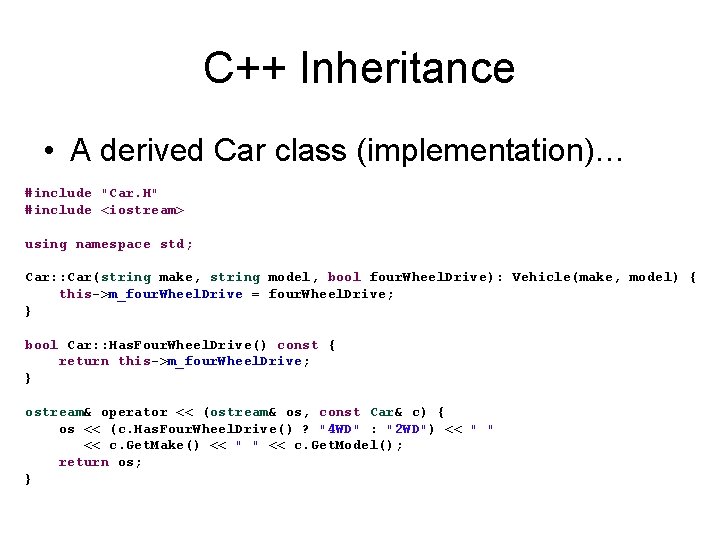
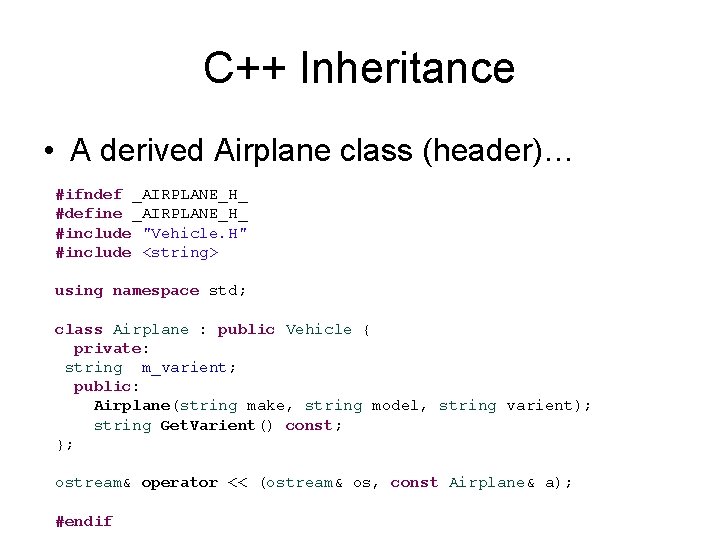
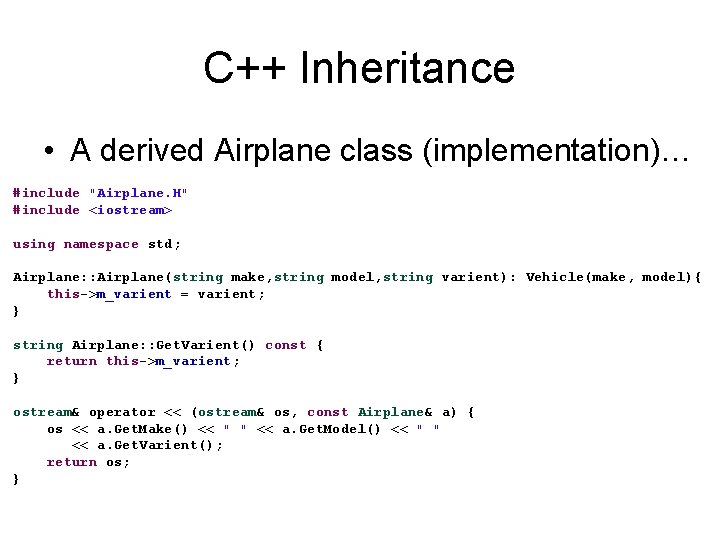
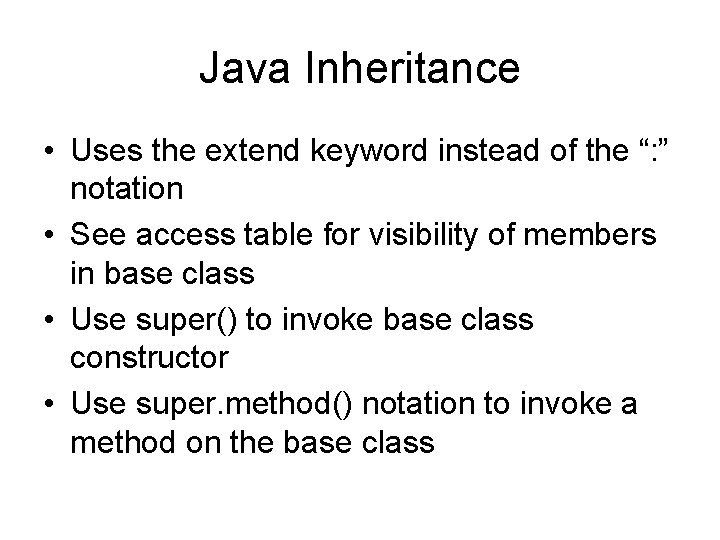
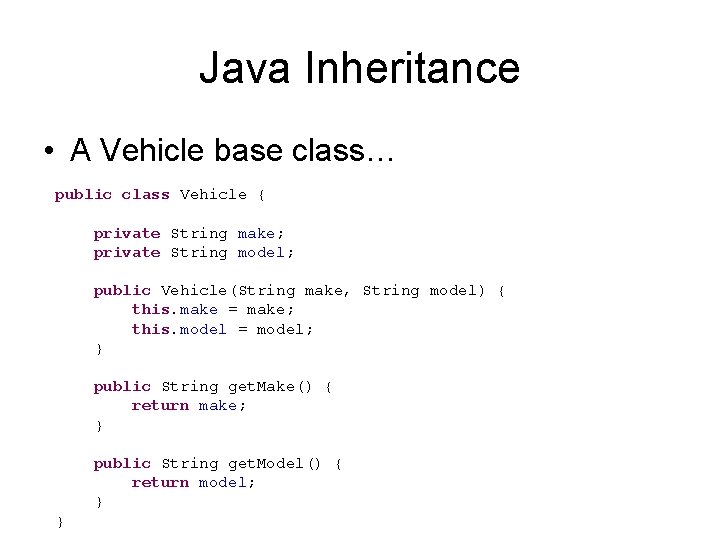
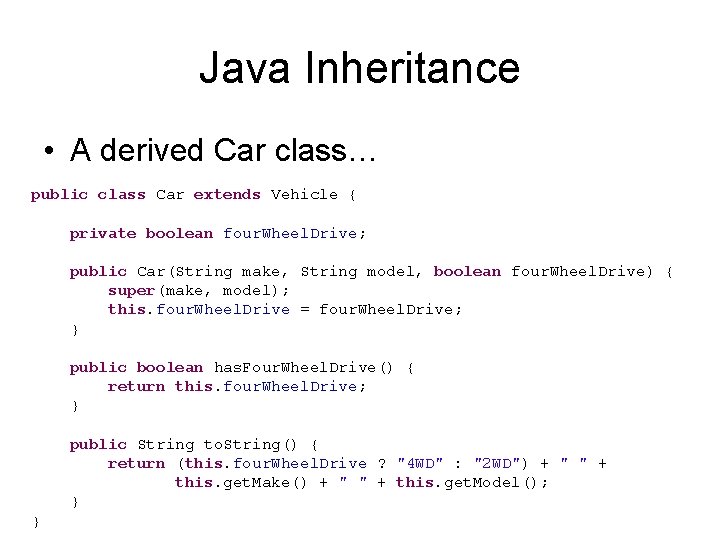
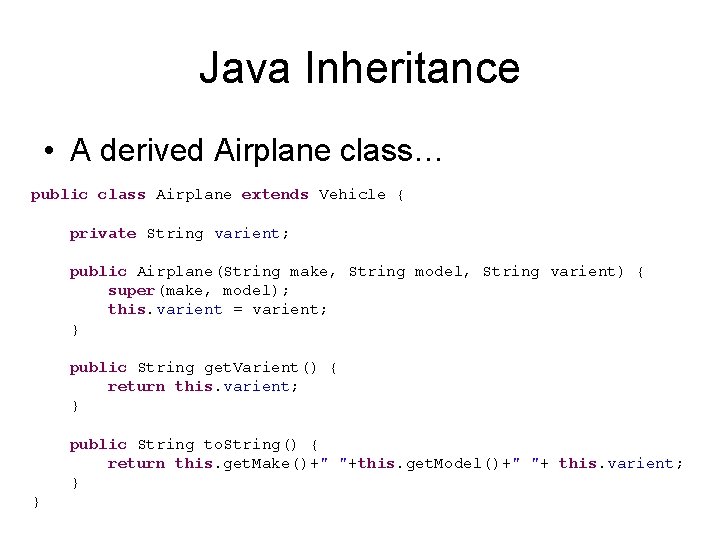
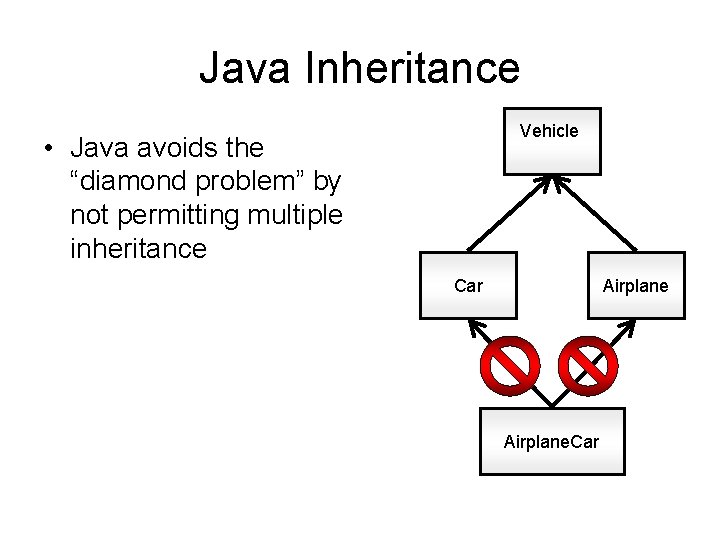
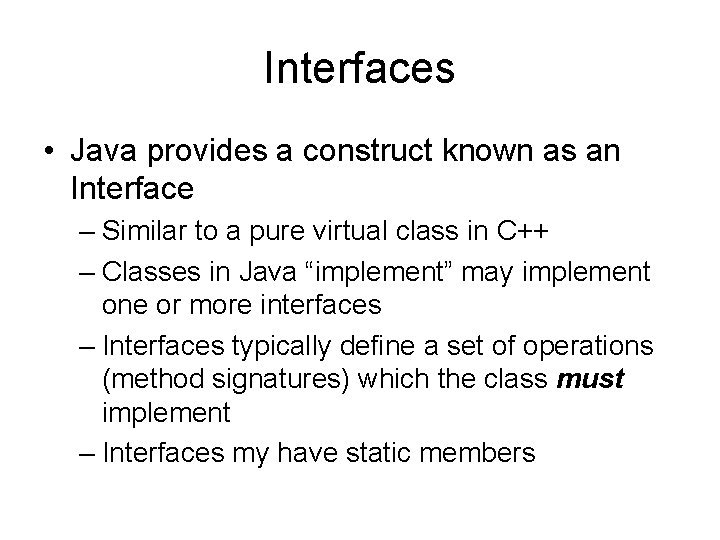
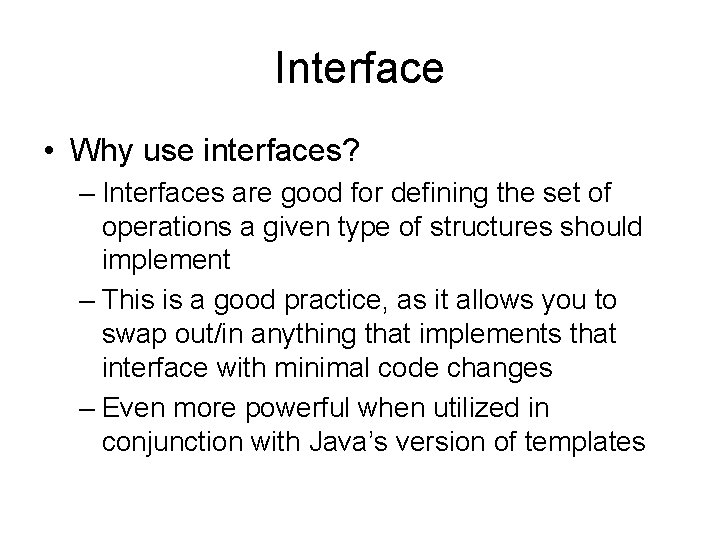
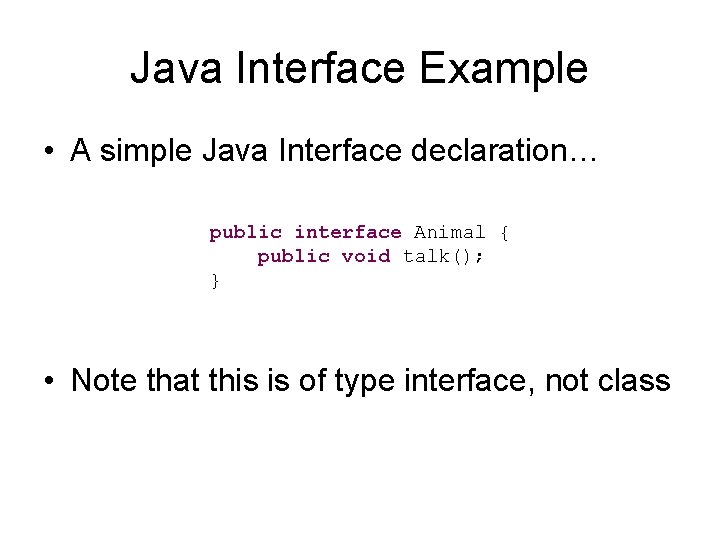
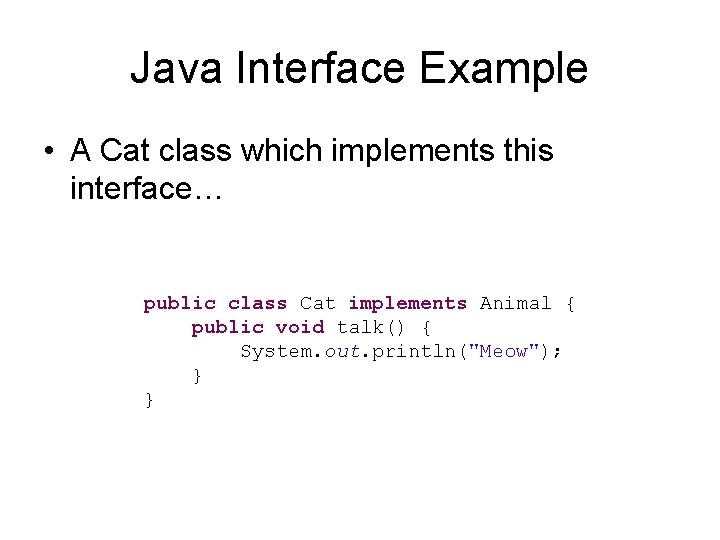
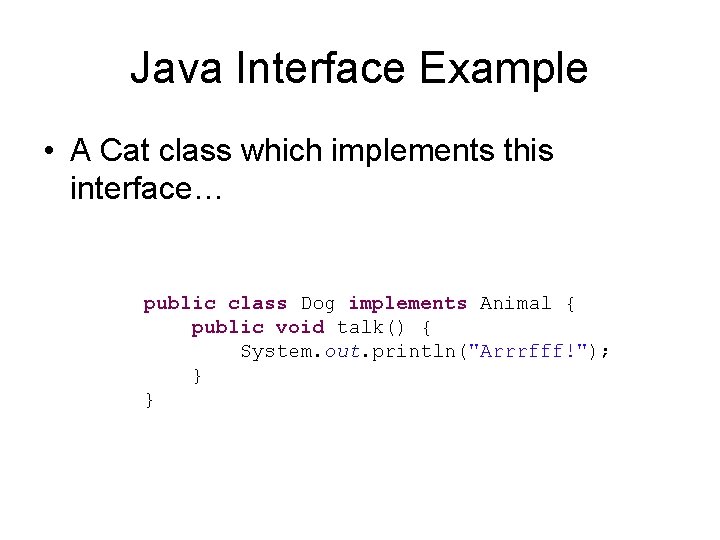
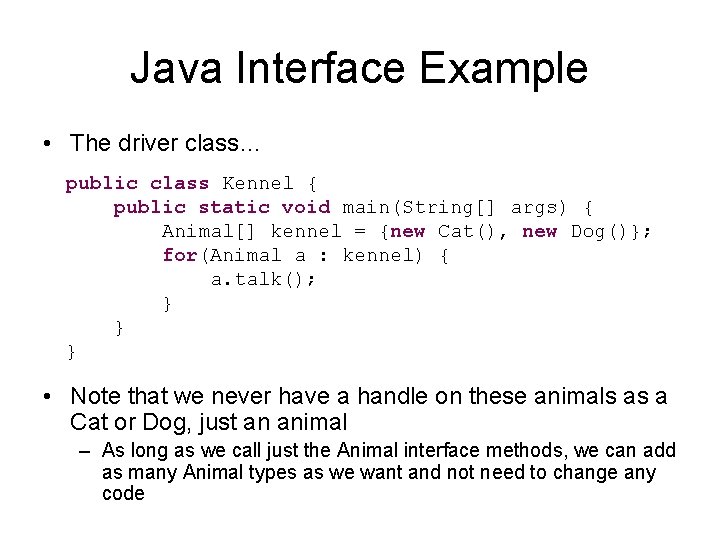
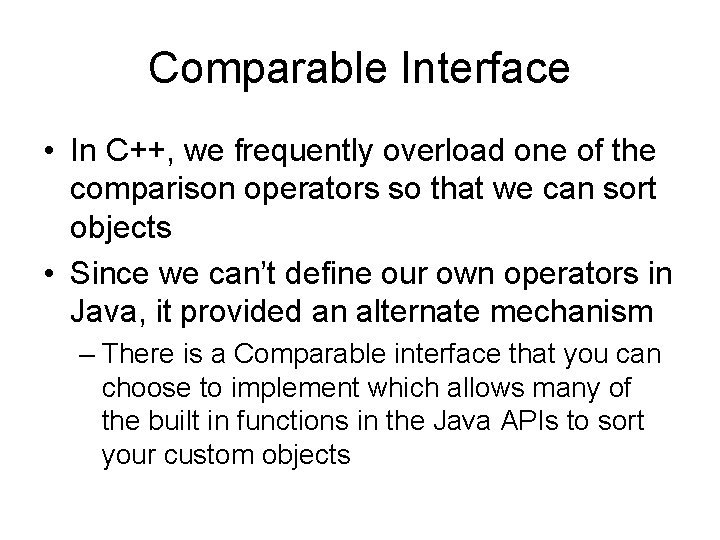
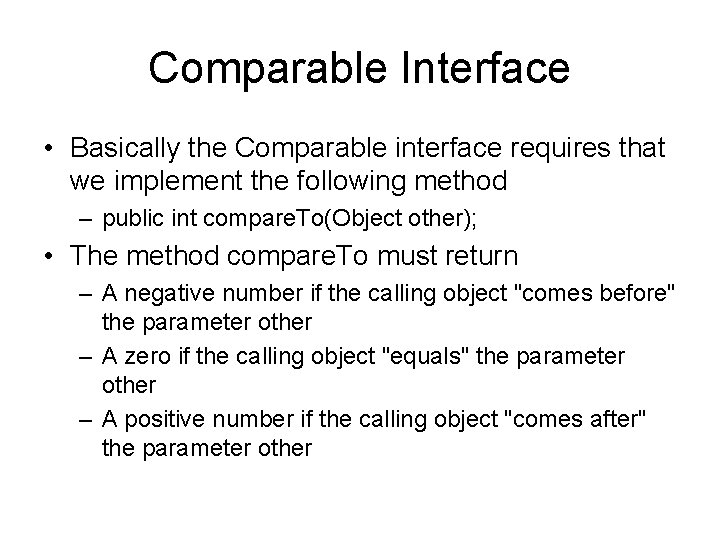
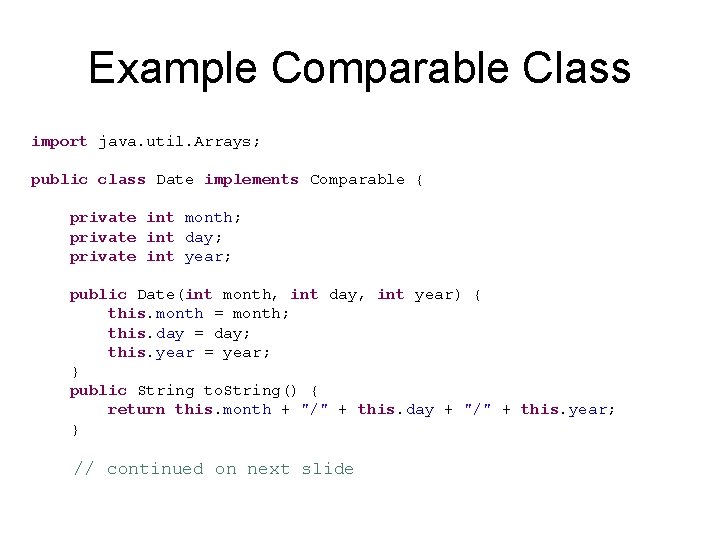
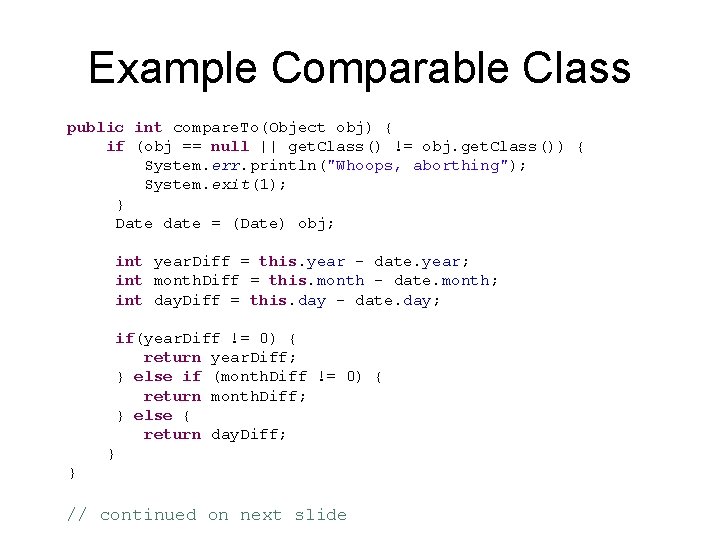
![Example Comparable Class public static void main(String[] args) { Date[] dates = { new Example Comparable Class public static void main(String[] args) { Date[] dates = { new](https://slidetodoc.com/presentation_image_h/349b7b536872183dfb14e0b76266de9e/image-87.jpg)
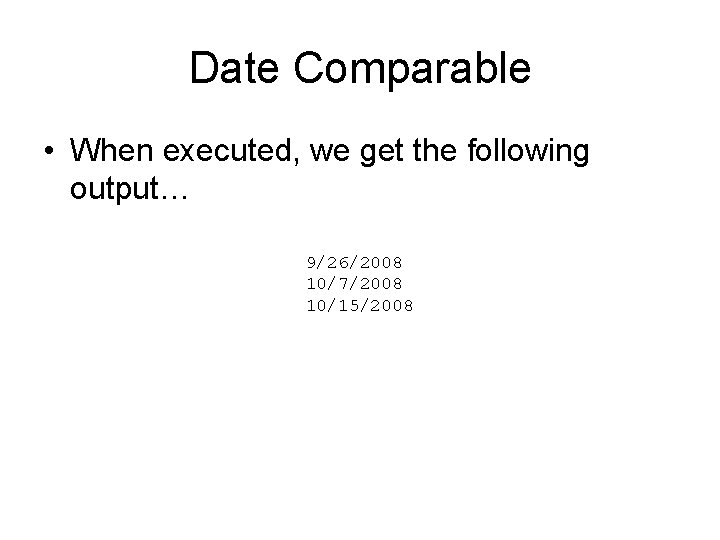
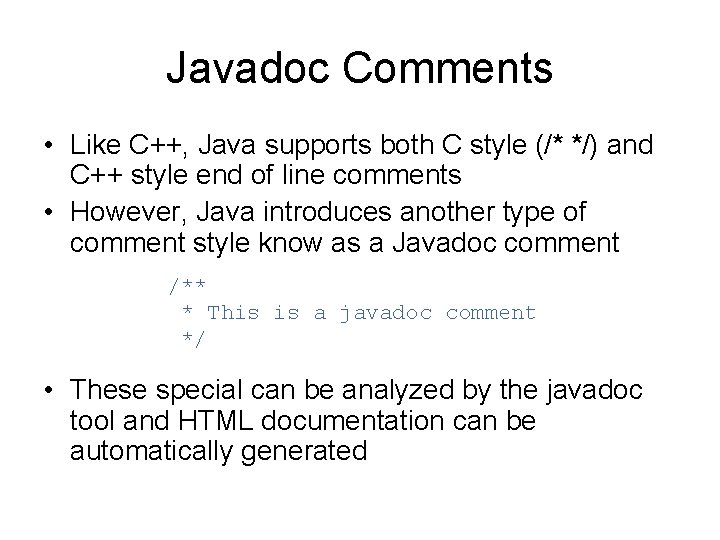
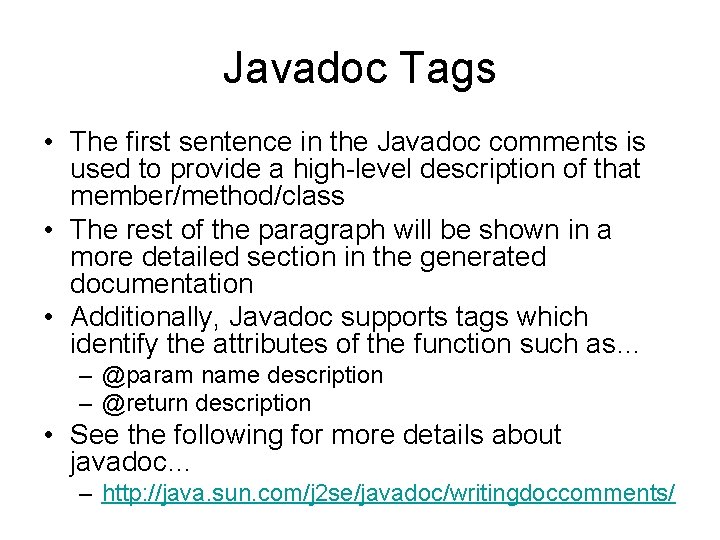
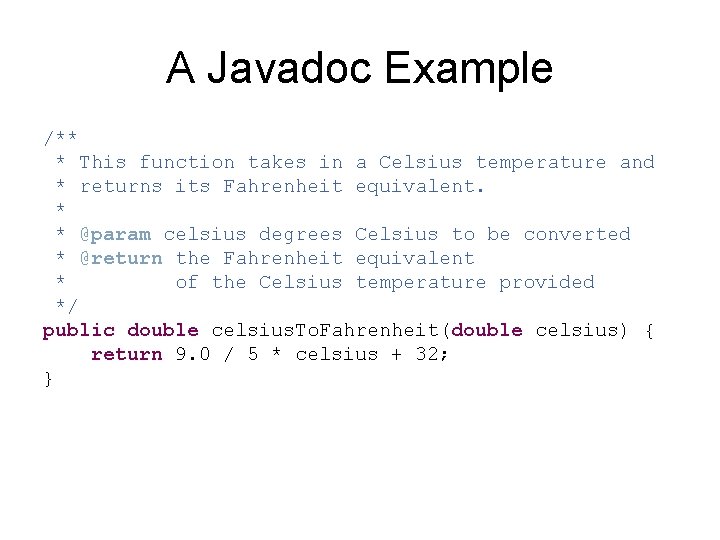
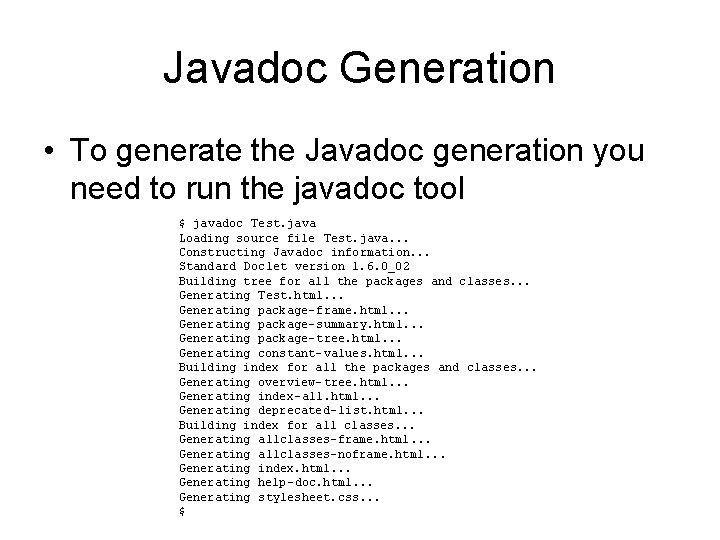
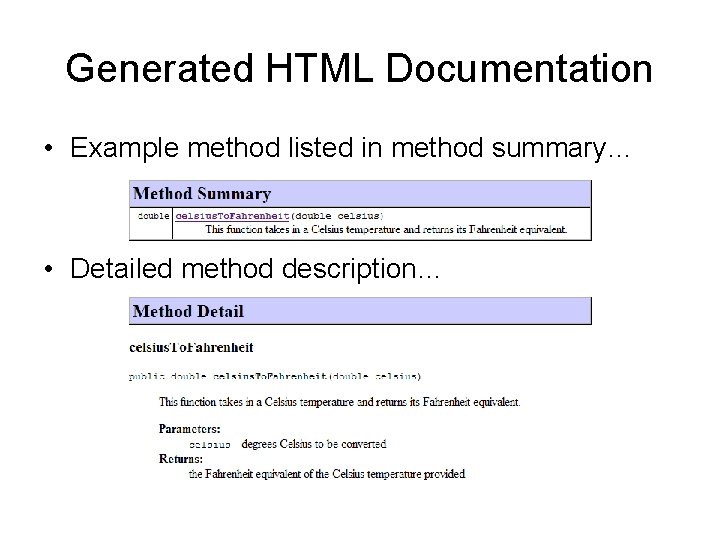
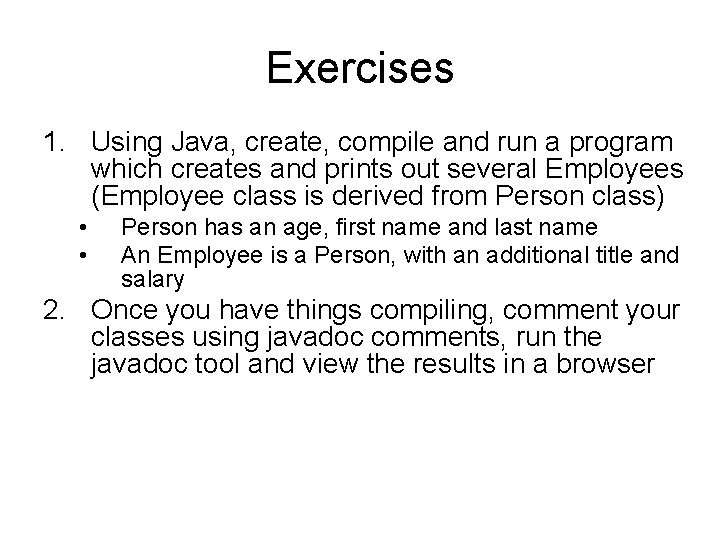
- Slides: 94
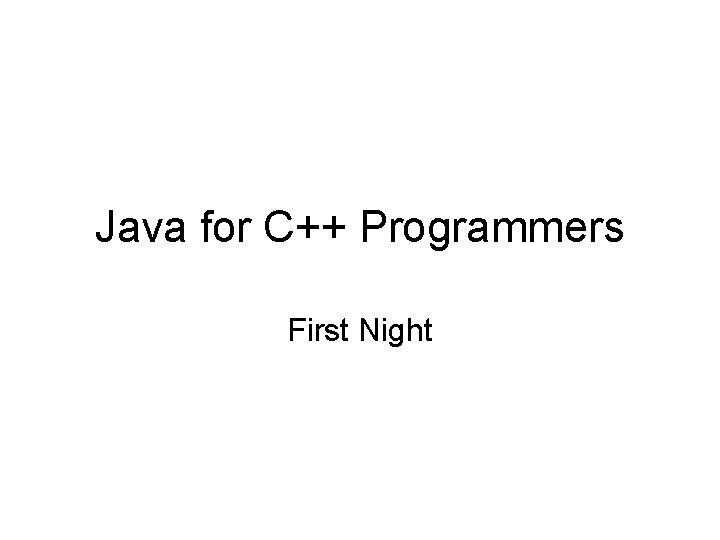
Java for C++ Programmers First Night
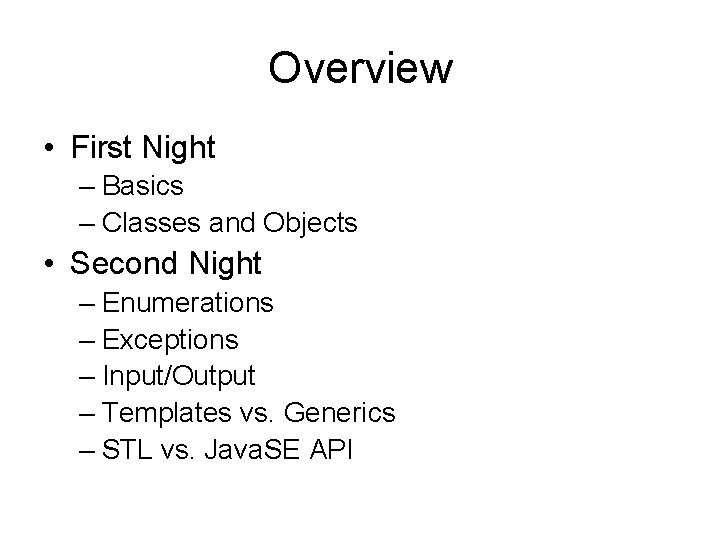
Overview • First Night – Basics – Classes and Objects • Second Night – Enumerations – Exceptions – Input/Output – Templates vs. Generics – STL vs. Java. SE API
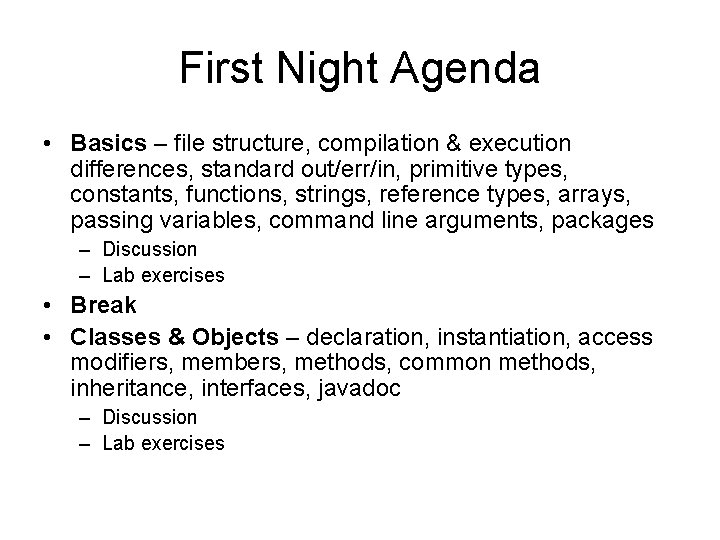
First Night Agenda • Basics – file structure, compilation & execution differences, standard out/err/in, primitive types, constants, functions, strings, reference types, arrays, passing variables, command line arguments, packages – Discussion – Lab exercises • Break • Classes & Objects – declaration, instantiation, access modifiers, members, methods, common methods, inheritance, interfaces, javadoc – Discussion – Lab exercises
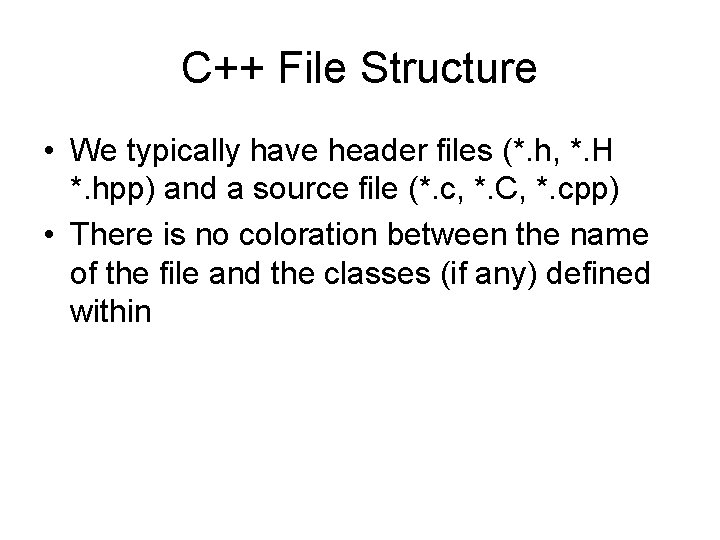
C++ File Structure • We typically have header files (*. h, *. H *. hpp) and a source file (*. c, *. C, *. cpp) • There is no coloration between the name of the file and the classes (if any) defined within
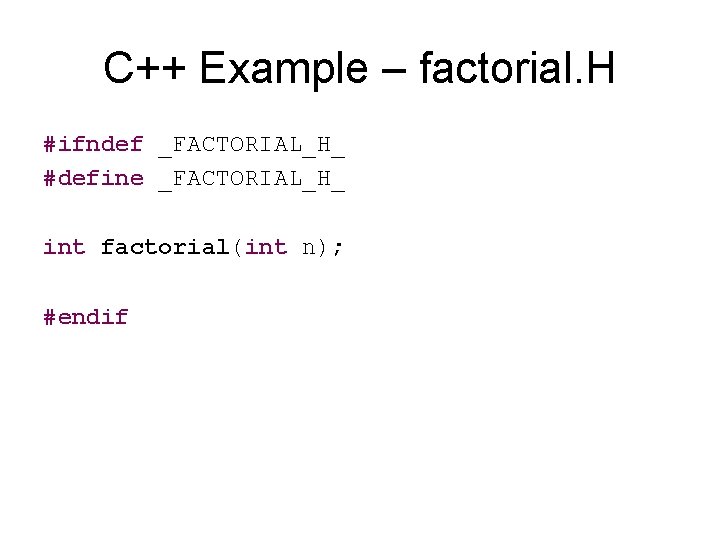
C++ Example – factorial. H #ifndef _FACTORIAL_H_ #define _FACTORIAL_H_ int factorial(int n); #endif
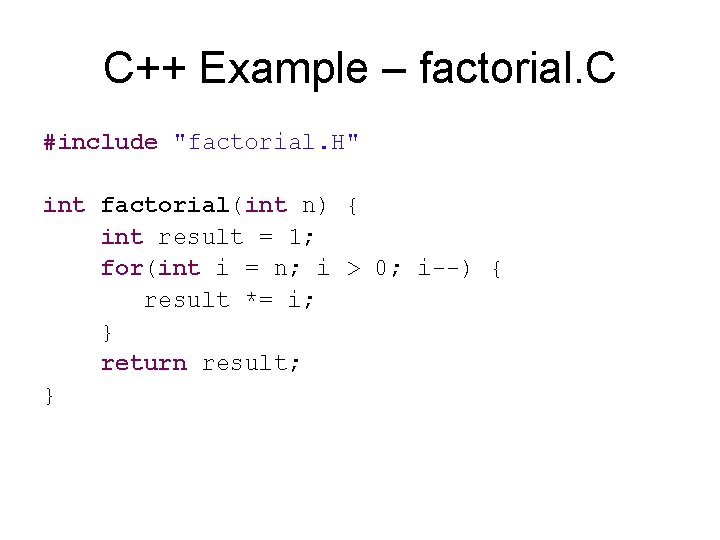
C++ Example – factorial. C #include "factorial. H" int factorial(int n) { int result = 1; for(int i = n; i > 0; i--) { result *= i; } return result; }
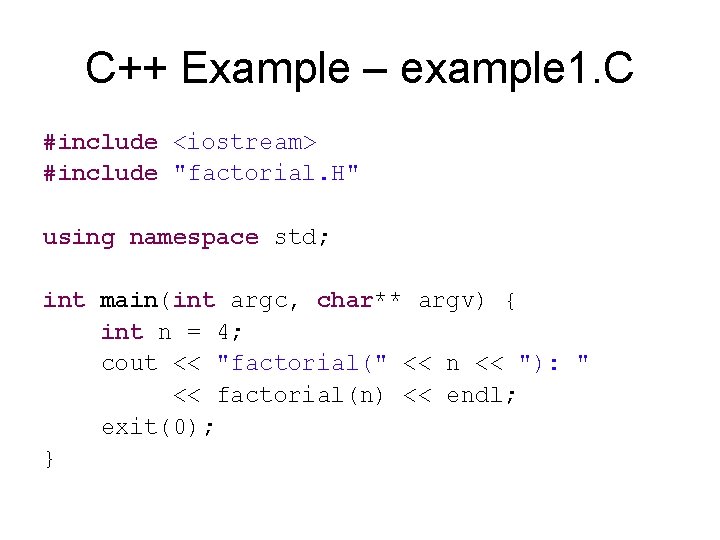
C++ Example – example 1. C #include <iostream> #include "factorial. H" using namespace std; int main(int argc, char** argv) { int n = 4; cout << "factorial(" << n << "): " << factorial(n) << endl; exit(0); }
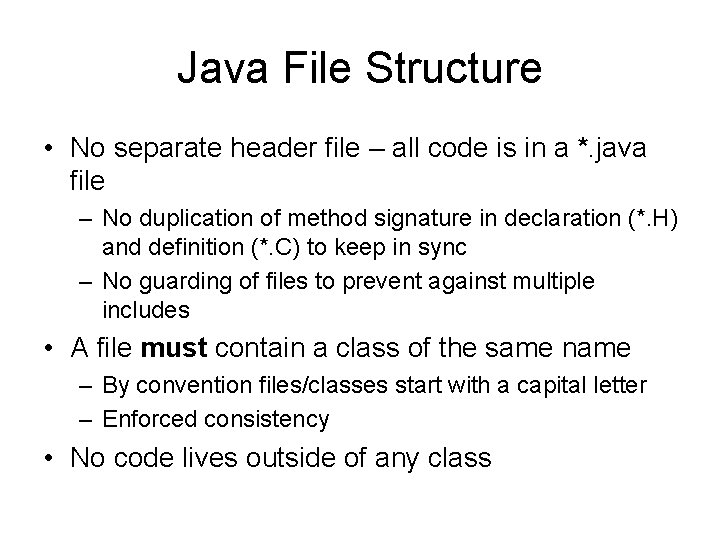
Java File Structure • No separate header file – all code is in a *. java file – No duplication of method signature in declaration (*. H) and definition (*. C) to keep in sync – No guarding of files to prevent against multiple includes • A file must contain a class of the same name – By convention files/classes start with a capital letter – Enforced consistency • No code lives outside of any class
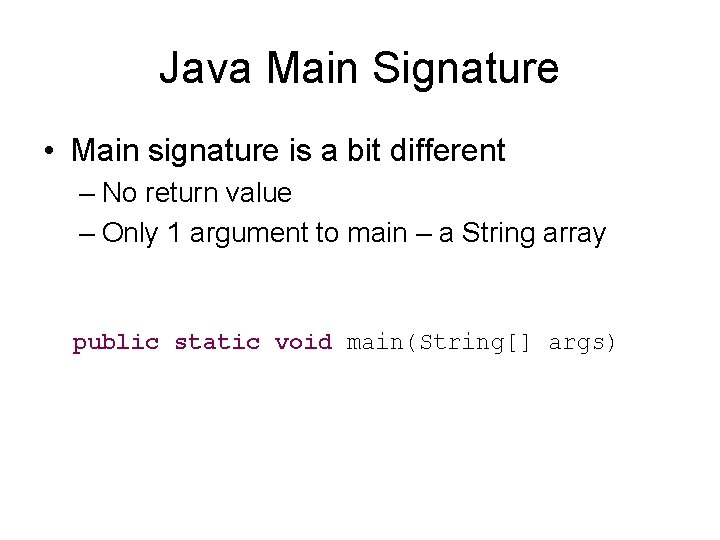
Java Main Signature • Main signature is a bit different – No return value – Only 1 argument to main – a String array public static void main(String[] args)
![Java Factorial Example public class Factorial public static void mainString args int Java Factorial Example public class Factorial { public static void main(String[] args) { int](https://slidetodoc.com/presentation_image_h/349b7b536872183dfb14e0b76266de9e/image-10.jpg)
Java Factorial Example public class Factorial { public static void main(String[] args) { int n = 4; System. out. print("factorial("); System. out. print(n); System. out. print("): "); System. out. println(factorial(n)); } static int factorial(int n) { int result = 1; for(int i = n; i > 0; i--) { result *= i; } return result; } }
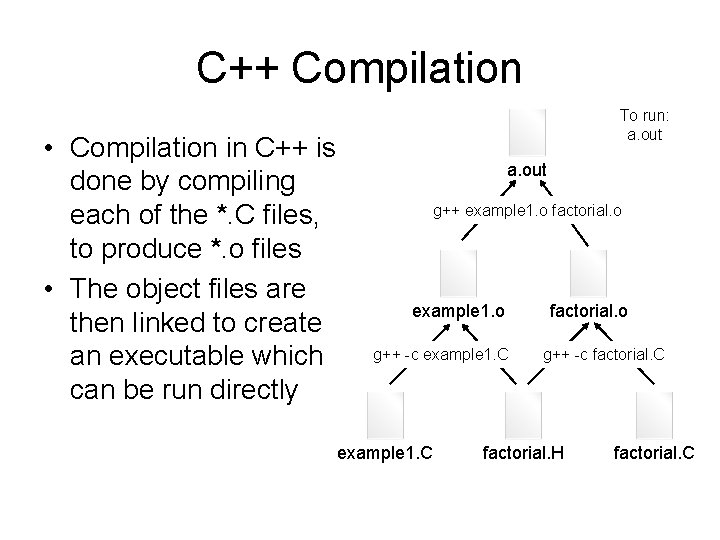
C++ Compilation • Compilation in C++ is done by compiling each of the *. C files, to produce *. o files • The object files are then linked to create an executable which can be run directly To run: a. out g++ example 1. o factorial. o example 1. o g++ -c example 1. C factorial. o g++ -c factorial. C factorial. H factorial. C
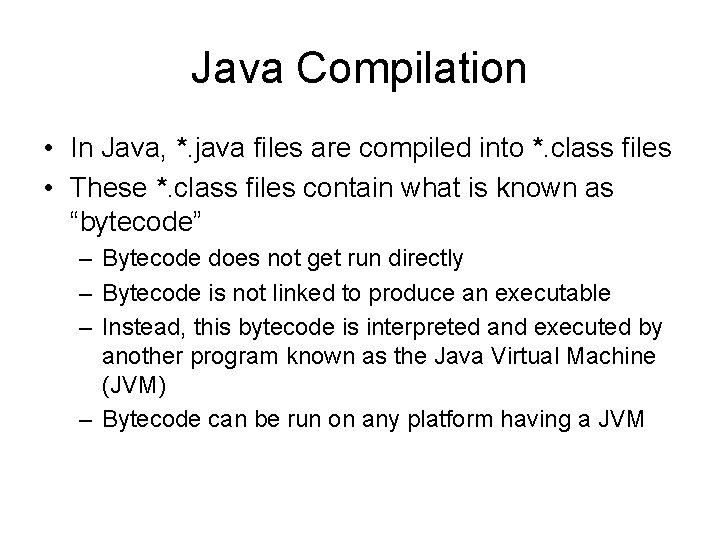
Java Compilation • In Java, *. java files are compiled into *. class files • These *. class files contain what is known as “bytecode” – Bytecode does not get run directly – Bytecode is not linked to produce an executable – Instead, this bytecode is interpreted and executed by another program known as the Java Virtual Machine (JVM) – Bytecode can be run on any platform having a JVM
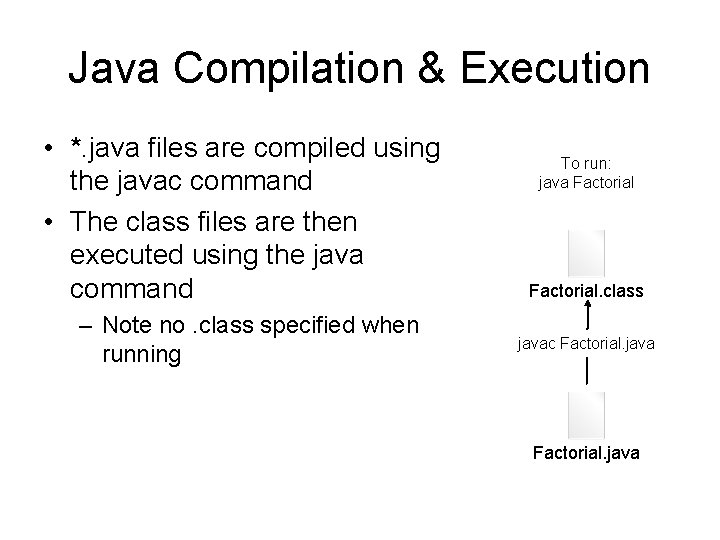
Java Compilation & Execution • *. java files are compiled using the javac command • The class files are then executed using the java command – Note no. class specified when running To run: java Factorial. class javac Factorial. java
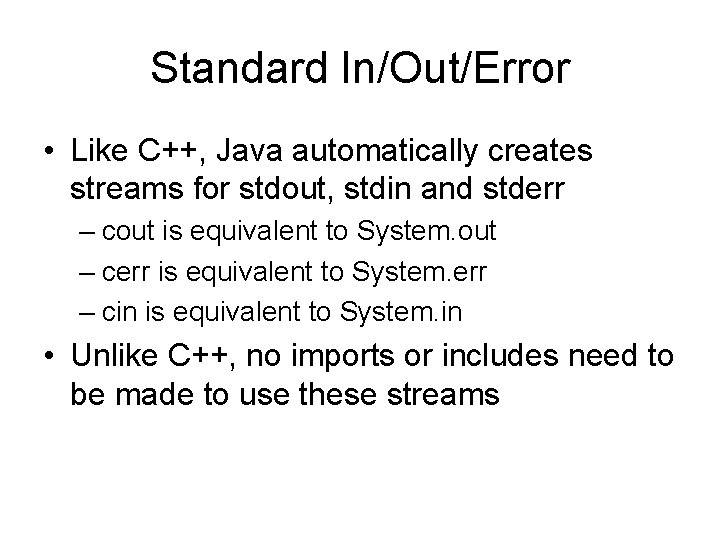
Standard In/Out/Error • Like C++, Java automatically creates streams for stdout, stdin and stderr – cout is equivalent to System. out – cerr is equivalent to System. err – cin is equivalent to System. in • Unlike C++, no imports or includes need to be made to use these streams
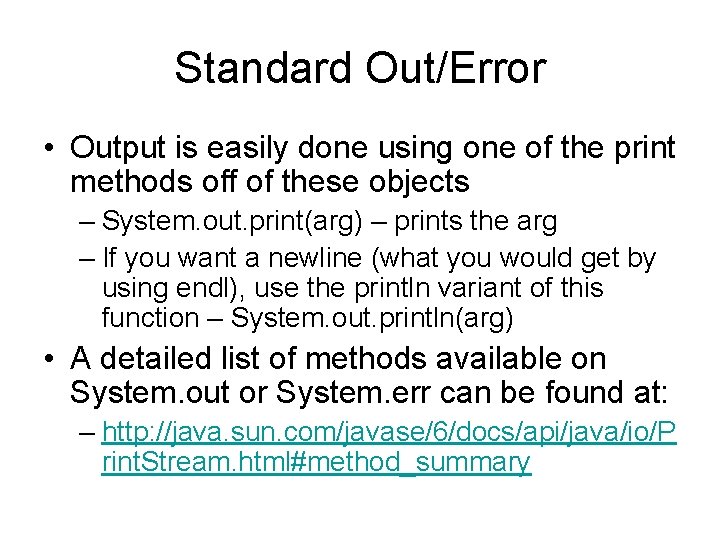
Standard Out/Error • Output is easily done using one of the print methods off of these objects – System. out. print(arg) – prints the arg – If you want a newline (what you would get by using endl), use the println variant of this function – System. out. println(arg) • A detailed list of methods available on System. out or System. err can be found at: – http: //java. sun. com/javase/6/docs/api/java/io/P rint. Stream. html#method_summary
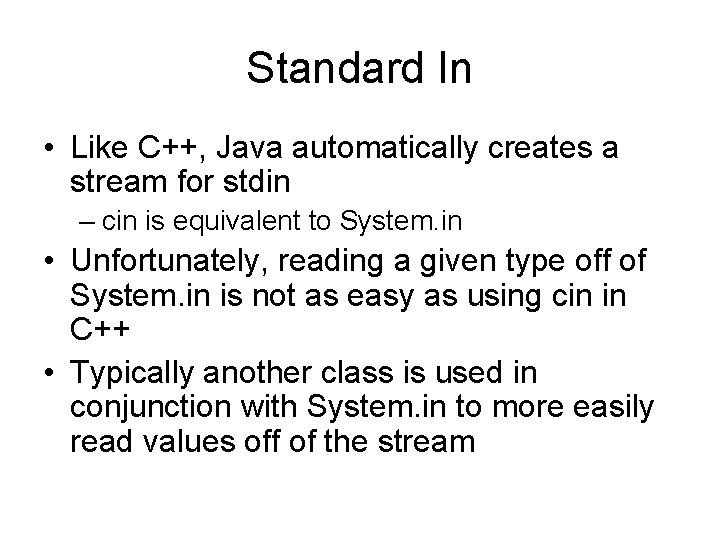
Standard In • Like C++, Java automatically creates a stream for stdin – cin is equivalent to System. in • Unfortunately, reading a given type off of System. in is not as easy as using cin in C++ • Typically another class is used in conjunction with System. in to more easily read values off of the stream
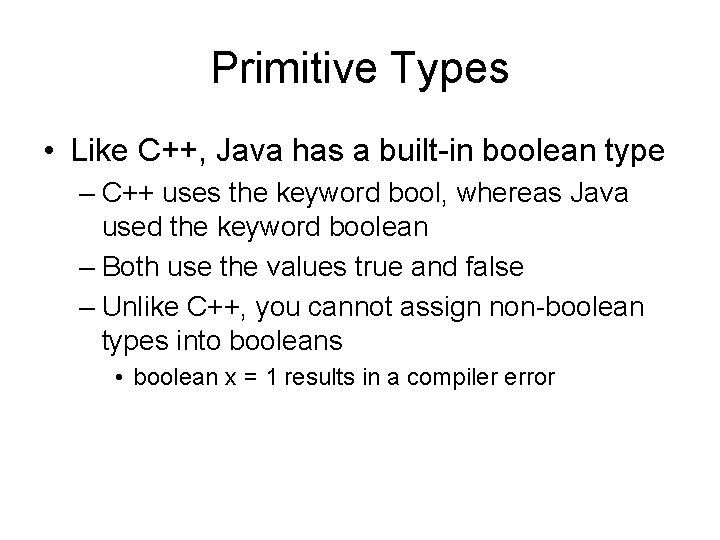
Primitive Types • Like C++, Java has a built-in boolean type – C++ uses the keyword bool, whereas Java used the keyword boolean – Both use the values true and false – Unlike C++, you cannot assign non-boolean types into booleans • boolean x = 1 results in a compiler error
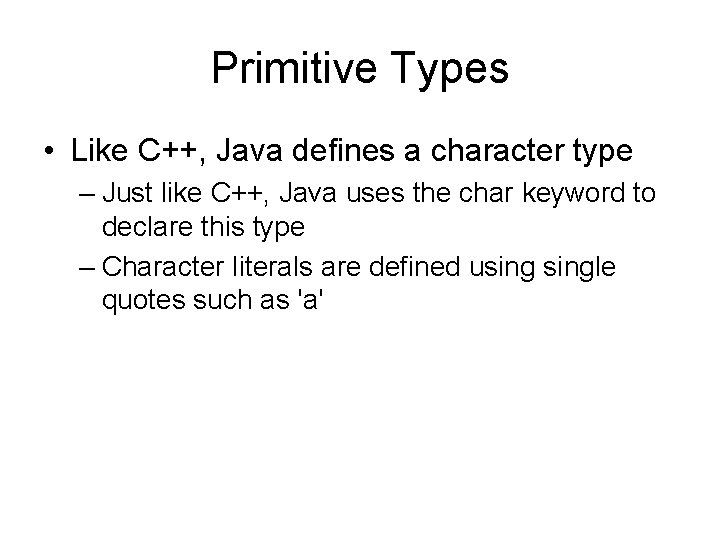
Primitive Types • Like C++, Java defines a character type – Just like C++, Java uses the char keyword to declare this type – Character literals are defined usingle quotes such as 'a'
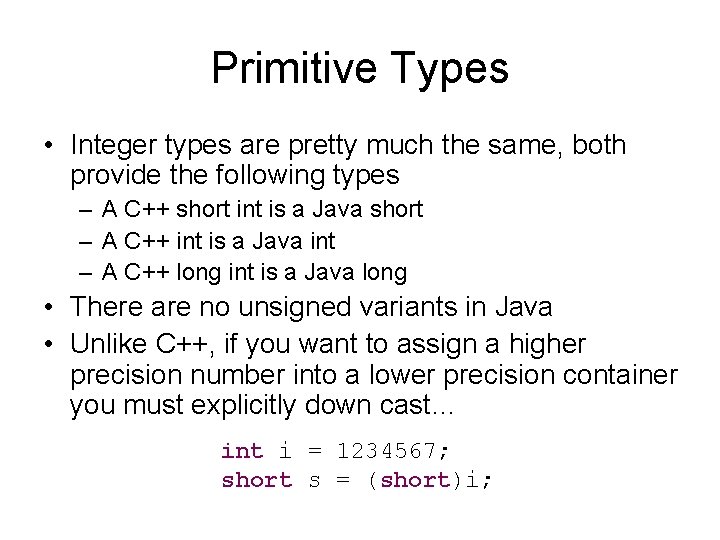
Primitive Types • Integer types are pretty much the same, both provide the following types – A C++ short int is a Java short – A C++ int is a Java int – A C++ long int is a Java long • There are no unsigned variants in Java • Unlike C++, if you want to assign a higher precision number into a lower precision container you must explicitly down cast… int i = 1234567; short s = (short)i;
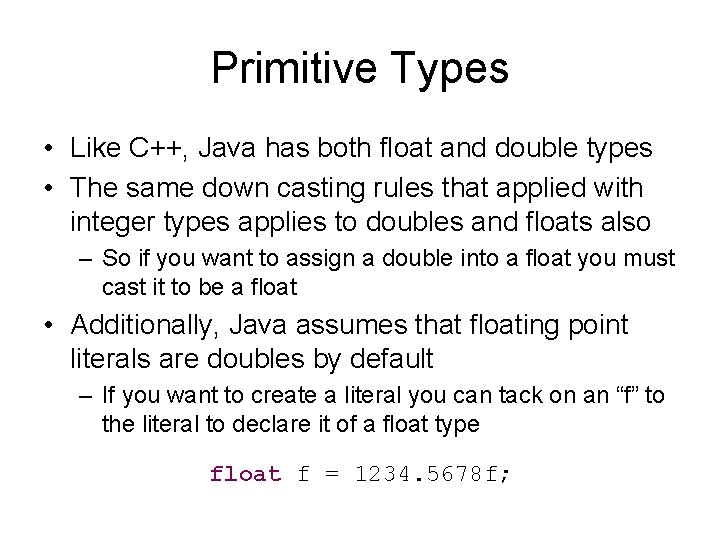
Primitive Types • Like C++, Java has both float and double types • The same down casting rules that applied with integer types applies to doubles and floats also – So if you want to assign a double into a float you must cast it to be a float • Additionally, Java assumes that floating point literals are doubles by default – If you want to create a literal you can tack on an “f” to the literal to declare it of a float type float f = 1234. 5678 f;
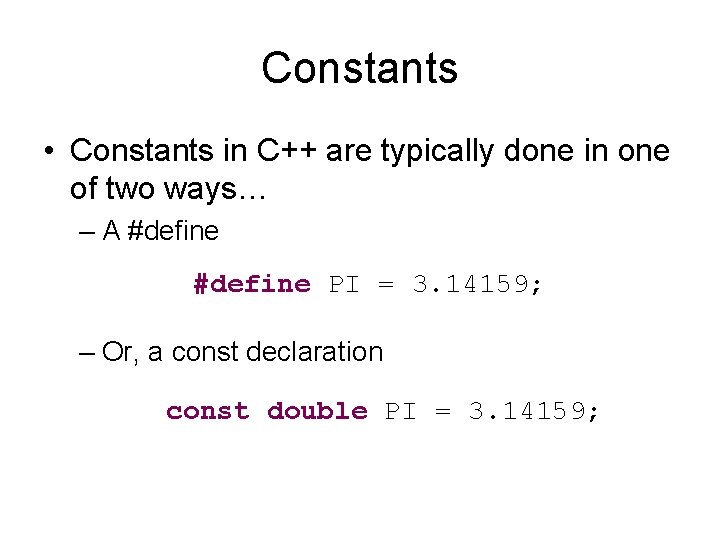
Constants • Constants in C++ are typically done in one of two ways… – A #define PI = 3. 14159; – Or, a const declaration const double PI = 3. 14159;
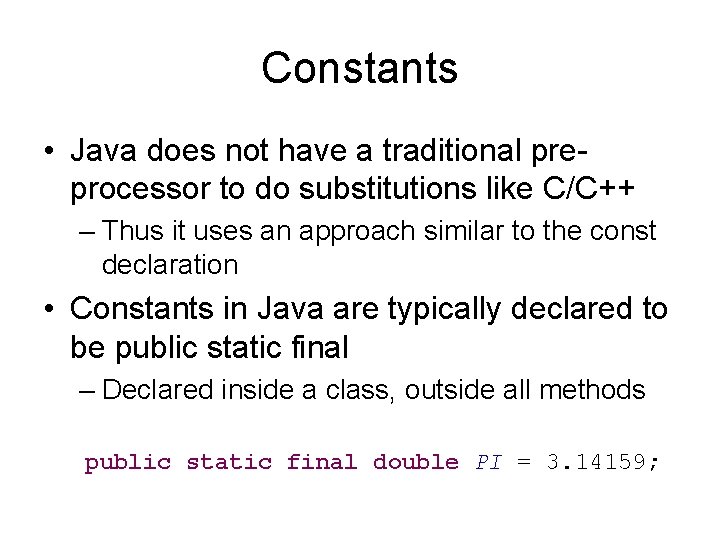
Constants • Java does not have a traditional preprocessor to do substitutions like C/C++ – Thus it uses an approach similar to the const declaration • Constants in Java are typically declared to be public static final – Declared inside a class, outside all methods public static final double PI = 3. 14159;
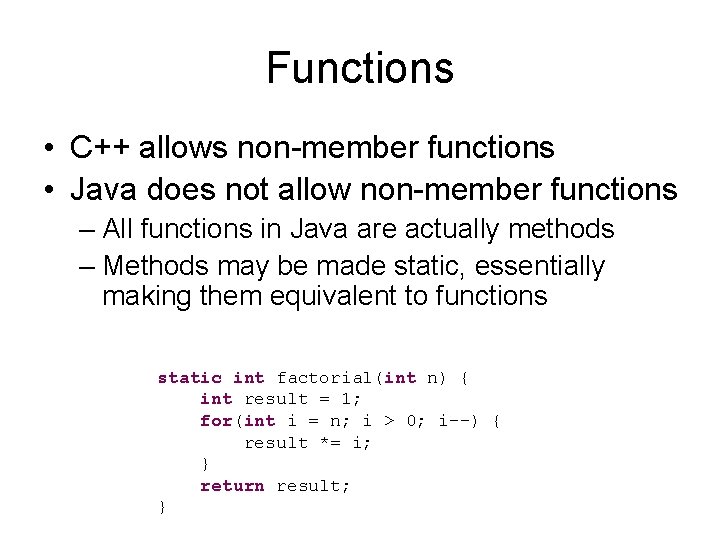
Functions • C++ allows non-member functions • Java does not allow non-member functions – All functions in Java are actually methods – Methods may be made static, essentially making them equivalent to functions static int factorial(int n) { int result = 1; for(int i = n; i > 0; i--) { result *= i; } return result; }
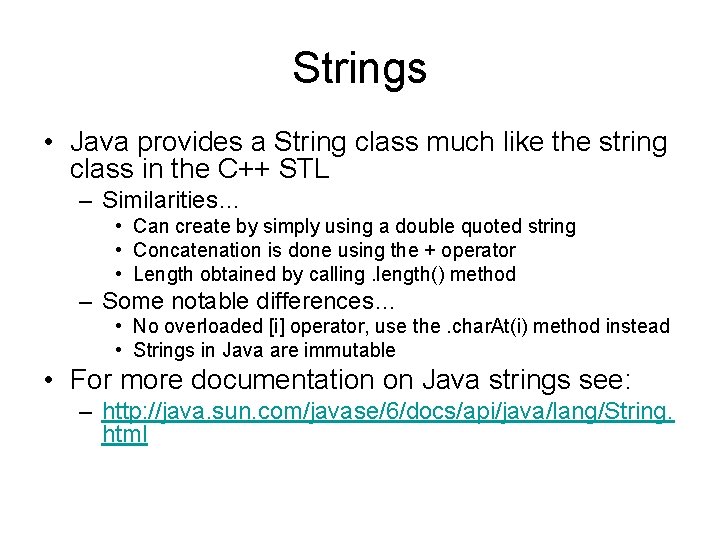
Strings • Java provides a String class much like the string class in the C++ STL – Similarities… • Can create by simply using a double quoted string • Concatenation is done using the + operator • Length obtained by calling. length() method – Some notable differences… • No overloaded [i] operator, use the. char. At(i) method instead • Strings in Java are immutable • For more documentation on Java strings see: – http: //java. sun. com/javase/6/docs/api/java/lang/String. html
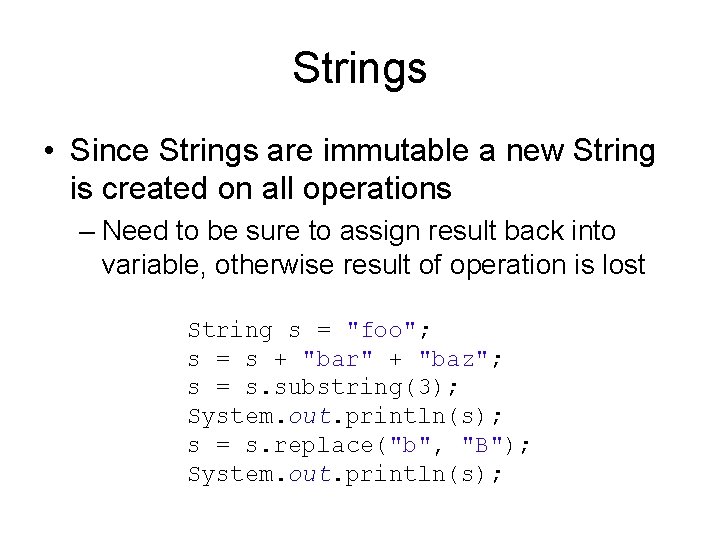
Strings • Since Strings are immutable a new String is created on all operations – Need to be sure to assign result back into variable, otherwise result of operation is lost String s = "foo"; s = s + "bar" + "baz"; s = s. substring(3); System. out. println(s); s = s. replace("b", "B"); System. out. println(s);
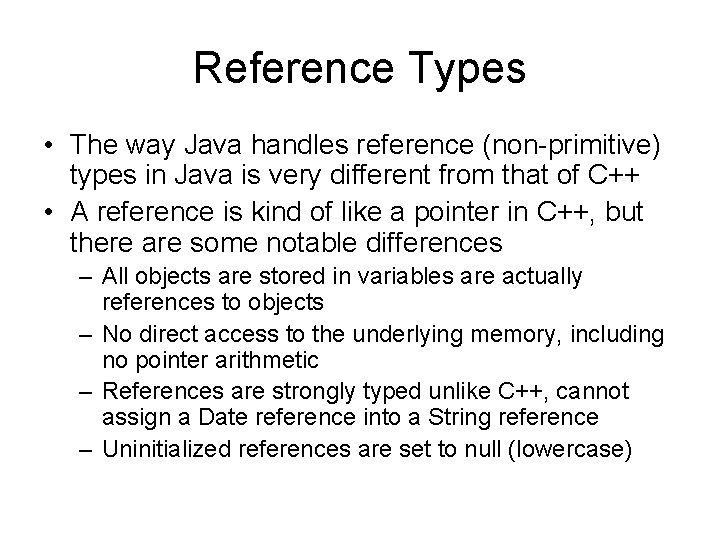
Reference Types • The way Java handles reference (non-primitive) types in Java is very different from that of C++ • A reference is kind of like a pointer in C++, but there are some notable differences – All objects are stored in variables are actually references to objects – No direct access to the underlying memory, including no pointer arithmetic – References are strongly typed unlike C++, cannot assign a Date reference into a String reference – Uninitialized references are set to null (lowercase)
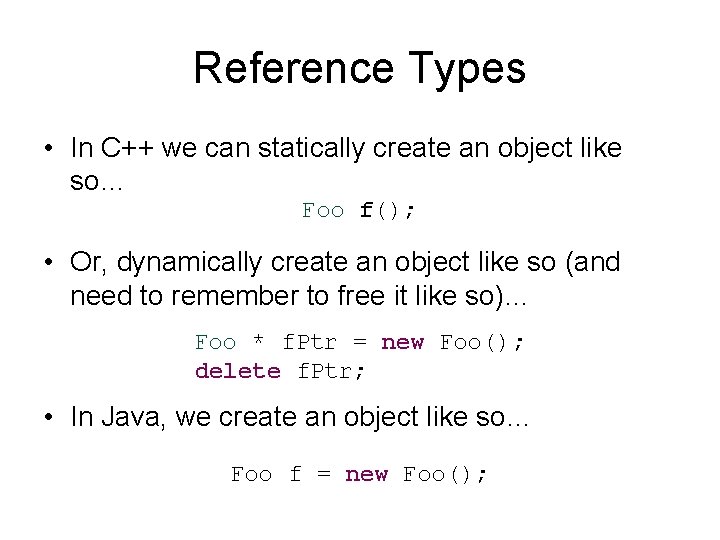
Reference Types • In C++ we can statically create an object like so… Foo f(); • Or, dynamically create an object like so (and need to remember to free it like so)… Foo * f. Ptr = new Foo(); delete f. Ptr; • In Java, we create an object like so… Foo f = new Foo();
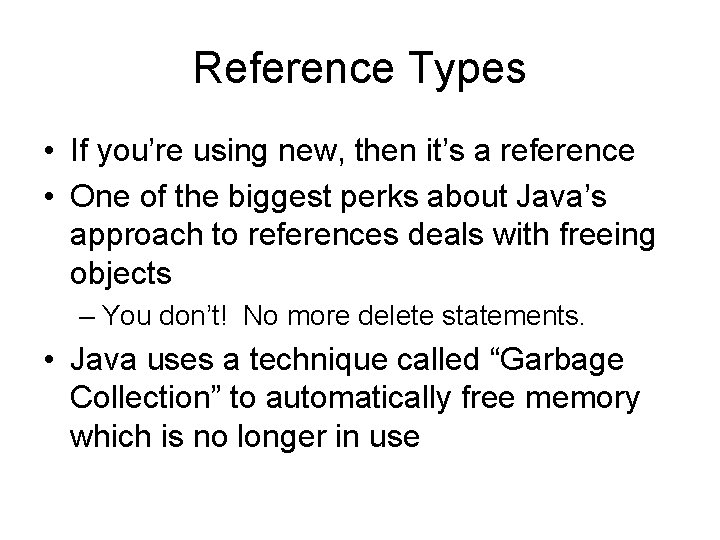
Reference Types • If you’re using new, then it’s a reference • One of the biggest perks about Java’s approach to references deals with freeing objects – You don’t! No more delete statements. • Java uses a technique called “Garbage Collection” to automatically free memory which is no longer in use
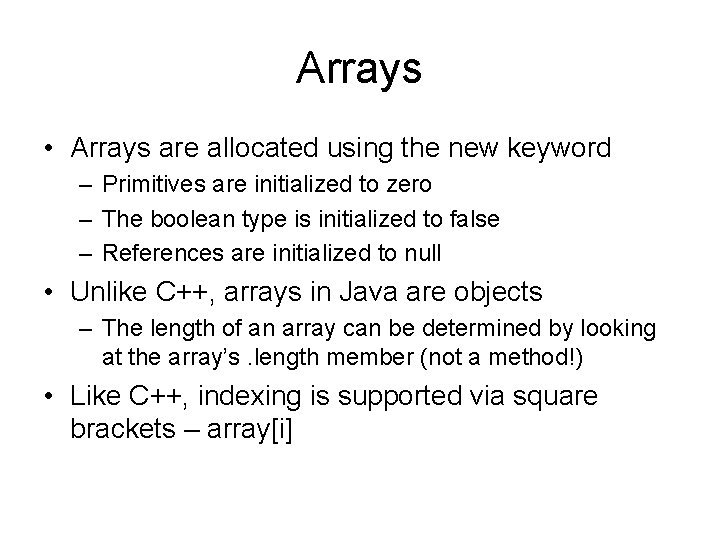
Arrays • Arrays are allocated using the new keyword – Primitives are initialized to zero – The boolean type is initialized to false – References are initialized to null • Unlike C++, arrays in Java are objects – The length of an array can be determined by looking at the array’s. length member (not a method!) • Like C++, indexing is supported via square brackets – array[i]
![Array Allocation example declarations int int Array new int10 boolean bool Array Array Allocation // example declarations int[] int. Array = new int[10]; boolean[] bool. Array](https://slidetodoc.com/presentation_image_h/349b7b536872183dfb14e0b76266de9e/image-30.jpg)
Array Allocation // example declarations int[] int. Array = new int[10]; boolean[] bool. Array = new boolean[10]; // note [] may appear before of after, // before is the more popular Java convention String[] str. Array 1 = new String[10]; String str. Array 2[] = new String[10]; // may alternately use curly brace syntax String[] str. Array 3 = {"foo", "bar", "baz"};
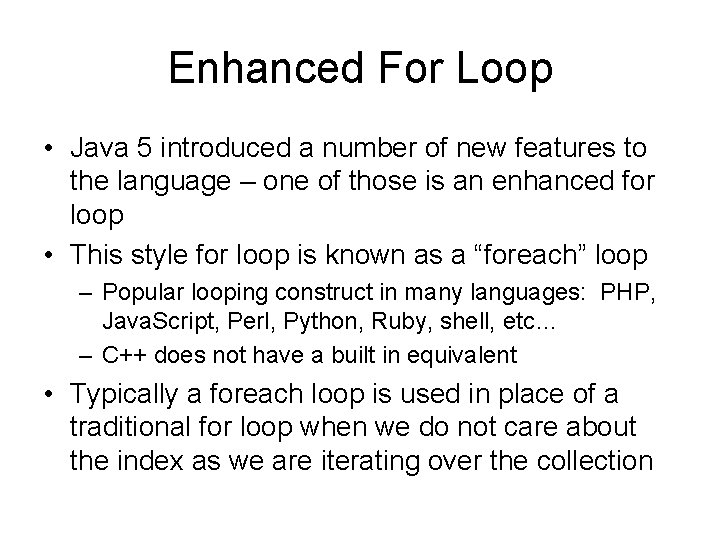
Enhanced For Loop • Java 5 introduced a number of new features to the language – one of those is an enhanced for loop • This style for loop is known as a “foreach” loop – Popular looping construct in many languages: PHP, Java. Script, Perl, Python, Ruby, shell, etc… – C++ does not have a built in equivalent • Typically a foreach loop is used in place of a traditional for loop when we do not care about the index as we are iterating over the collection
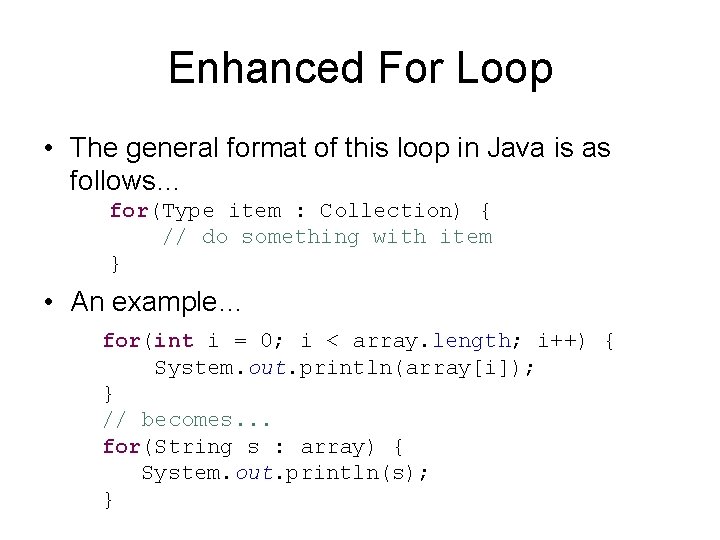
Enhanced For Loop • The general format of this loop in Java is as follows… for(Type item : Collection) { // do something with item } • An example… for(int i = 0; i < array. length; i++) { System. out. println(array[i]); } // becomes. . . for(String s : array) { System. out. println(s); }
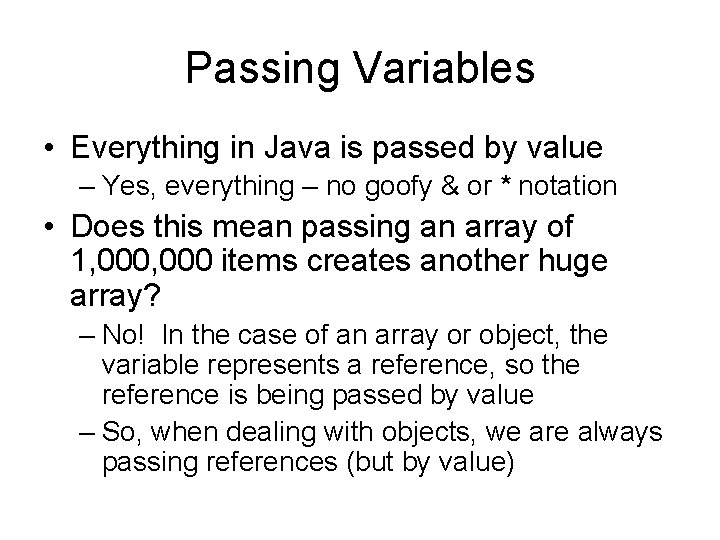
Passing Variables • Everything in Java is passed by value – Yes, everything – no goofy & or * notation • Does this mean passing an array of 1, 000 items creates another huge array? – No! In the case of an array or object, the variable represents a reference, so the reference is being passed by value – So, when dealing with objects, we are always passing references (but by value)
![Passing Variables public class Test public static void mainString args int array Passing Variables public class Test { public static void main(String[] args) { int[] array](https://slidetodoc.com/presentation_image_h/349b7b536872183dfb14e0b76266de9e/image-34.jpg)
Passing Variables public class Test { public static void main(String[] args) { int[] array = new int[10]; for(int i = 0; i < array. length; i++) { array[i] = i; } zero. Array(array); // what is in array? } static void zero. Array(int[] array) { for(int i = 0; i < array. length; i++) { array[i] = 0; } } }
![Passing Variables public class Test public static void mainString args int array Passing Variables public class Test { public static void main(String[] args) { int[] array](https://slidetodoc.com/presentation_image_h/349b7b536872183dfb14e0b76266de9e/image-35.jpg)
Passing Variables public class Test { public static void main(String[] args) { int[] array = new int[10]; for(int i = 0; i < array. length; i++) { array[i] = i; } zero. Array(array); // what is in array? } static void zero. Array(int[] array) { array = new int[array. length]; } }
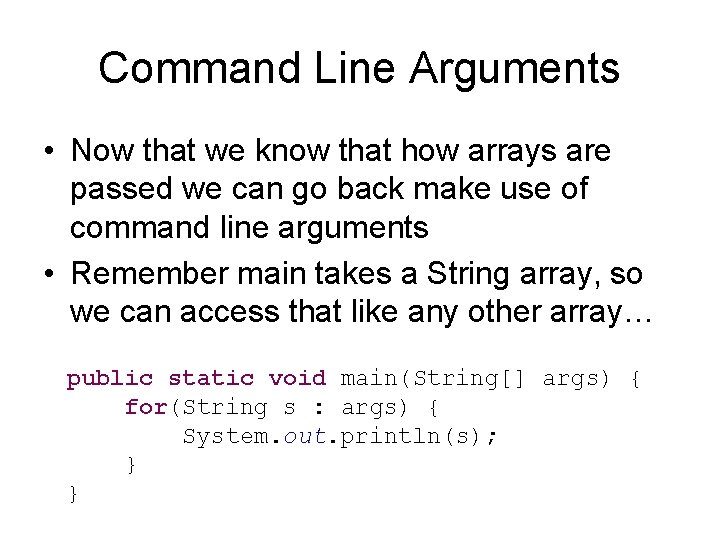
Command Line Arguments • Now that we know that how arrays are passed we can go back make use of command line arguments • Remember main takes a String array, so we can access that like any other array… public static void main(String[] args) { for(String s : args) { System. out. println(s); } }
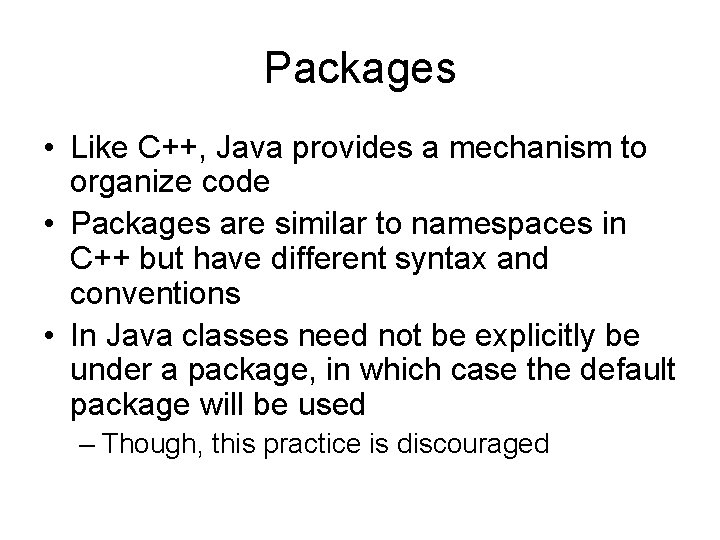
Packages • Like C++, Java provides a mechanism to organize code • Packages are similar to namespaces in C++ but have different syntax and conventions • In Java classes need not be explicitly be under a package, in which case the default package will be used – Though, this practice is discouraged
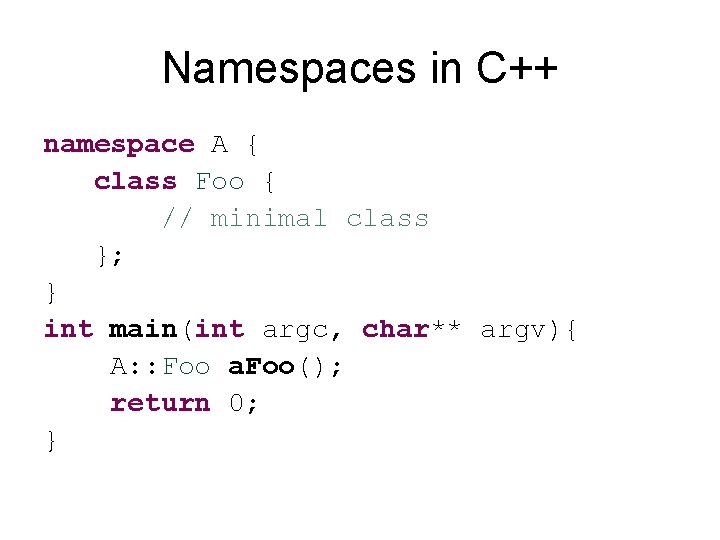
Namespaces in C++ namespace A { class Foo { // minimal class }; } int main(int argc, char** argv){ A: : Foo a. Foo(); return 0; }
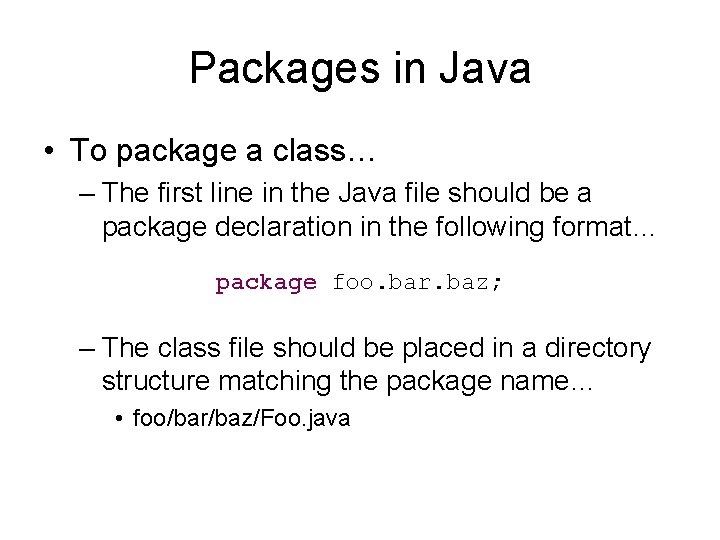
Packages in Java • To package a class… – The first line in the Java file should be a package declaration in the following format… package foo. bar. baz; – The class file should be placed in a directory structure matching the package name… • foo/bar/baz/Foo. java
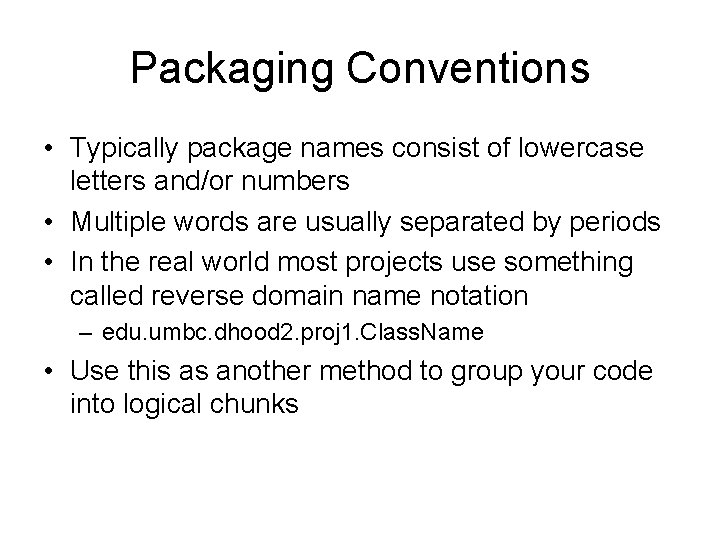
Packaging Conventions • Typically package names consist of lowercase letters and/or numbers • Multiple words are usually separated by periods • In the real world most projects use something called reverse domain name notation – edu. umbc. dhood 2. proj 1. Class. Name • Use this as another method to group your code into logical chunks
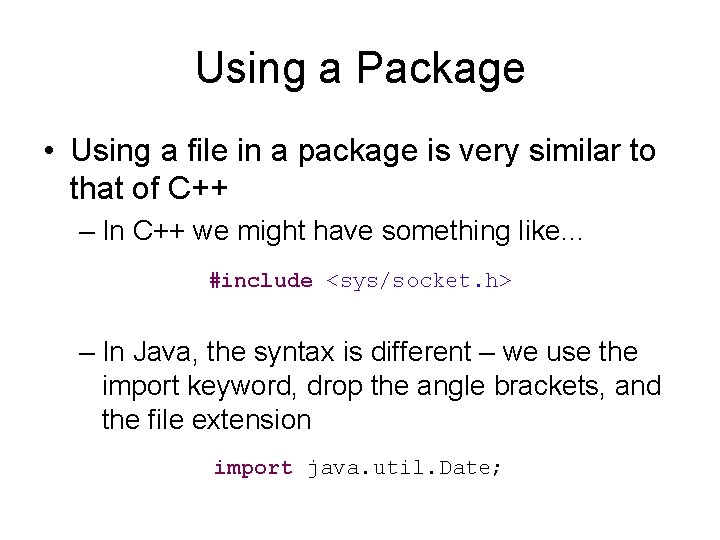
Using a Package • Using a file in a package is very similar to that of C++ – In C++ we might have something like… #include <sys/socket. h> – In Java, the syntax is different – we use the import keyword, drop the angle brackets, and the file extension import java. util. Date;
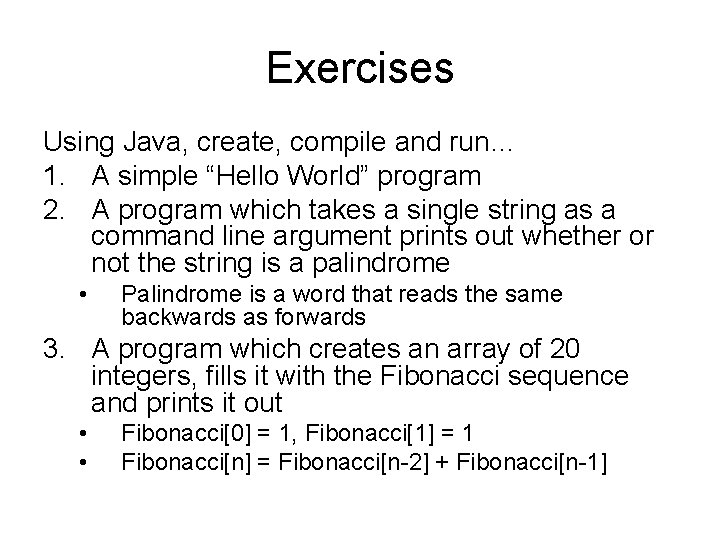
Exercises Using Java, create, compile and run… 1. A simple “Hello World” program 2. A program which takes a single string as a command line argument prints out whether or not the string is a palindrome • Palindrome is a word that reads the same backwards as forwards 3. A program which creates an array of 20 integers, fills it with the Fibonacci sequence and prints it out • • Fibonacci[0] = 1, Fibonacci[1] = 1 Fibonacci[n] = Fibonacci[n-2] + Fibonacci[n-1]
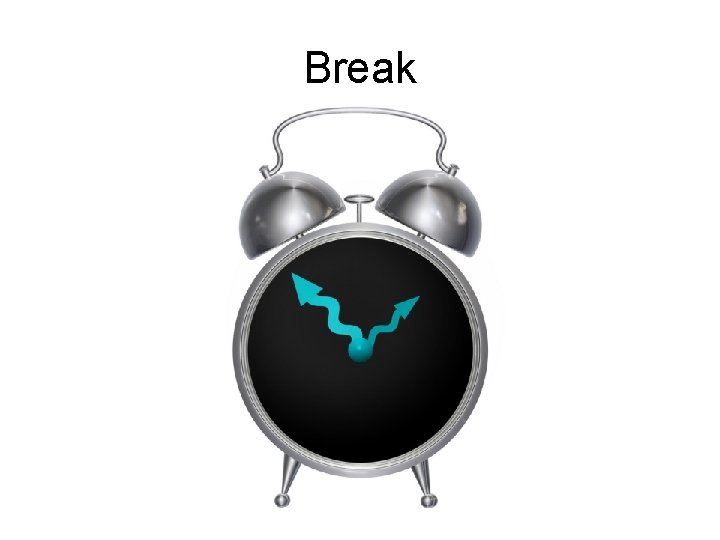
Break
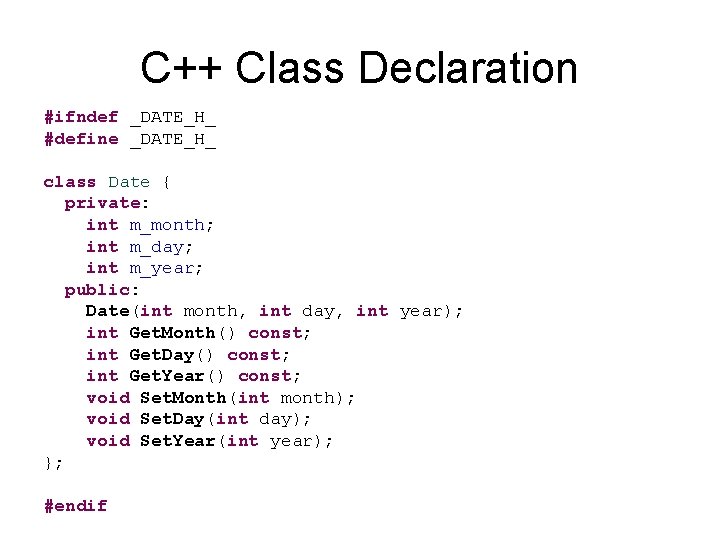
C++ Class Declaration #ifndef _DATE_H_ #define _DATE_H_ class Date { private: int m_month; int m_day; int m_year; public: Date(int month, int day, int year); int Get. Month() const; int Get. Day() const; int Get. Year() const; void Set. Month(int month); void Set. Day(int day); void Set. Year(int year); }; #endif
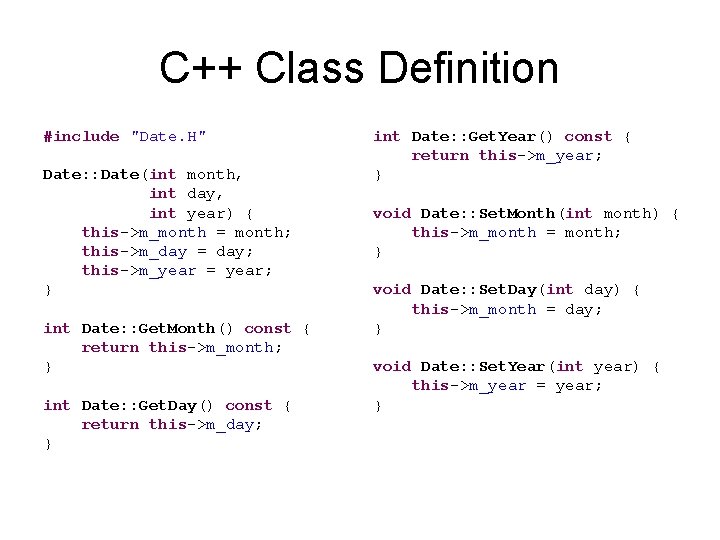
C++ Class Definition #include "Date. H" Date: : Date(int month, int day, int year) { this->m_month = month; this->m_day = day; this->m_year = year; } int Date: : Get. Month() const { return this->m_month; } int Date: : Get. Day() const { return this->m_day; } int Date: : Get. Year() const { return this->m_year; } void Date: : Set. Month(int month) { this->m_month = month; } void Date: : Set. Day(int day) { this->m_month = day; } void Date: : Set. Year(int year) { this->m_year = year; }
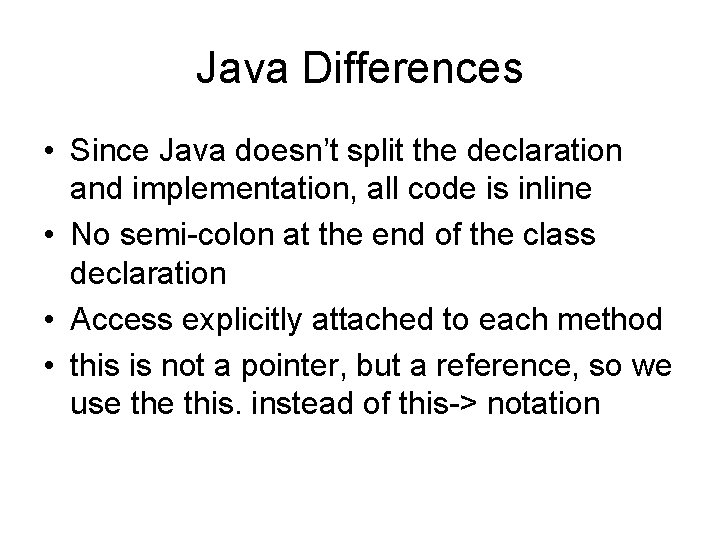
Java Differences • Since Java doesn’t split the declaration and implementation, all code is inline • No semi-colon at the end of the class declaration • Access explicitly attached to each method • this is not a pointer, but a reference, so we use this. instead of this-> notation
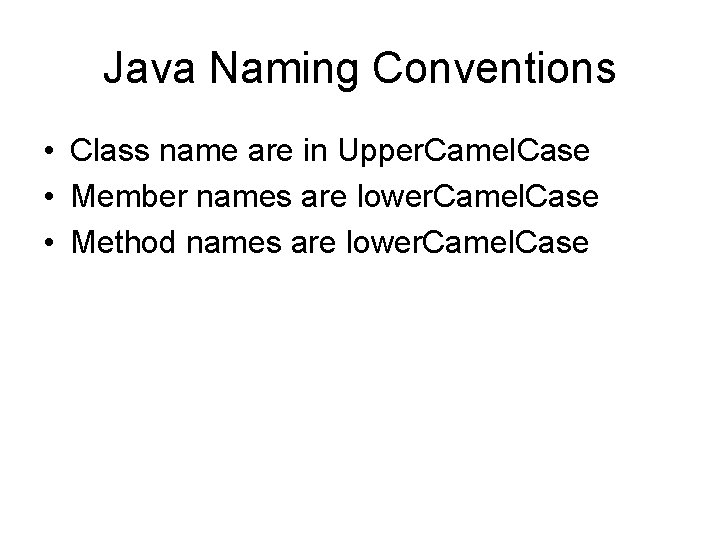
Java Naming Conventions • Class name are in Upper. Camel. Case • Member names are lower. Camel. Case • Method names are lower. Camel. Case
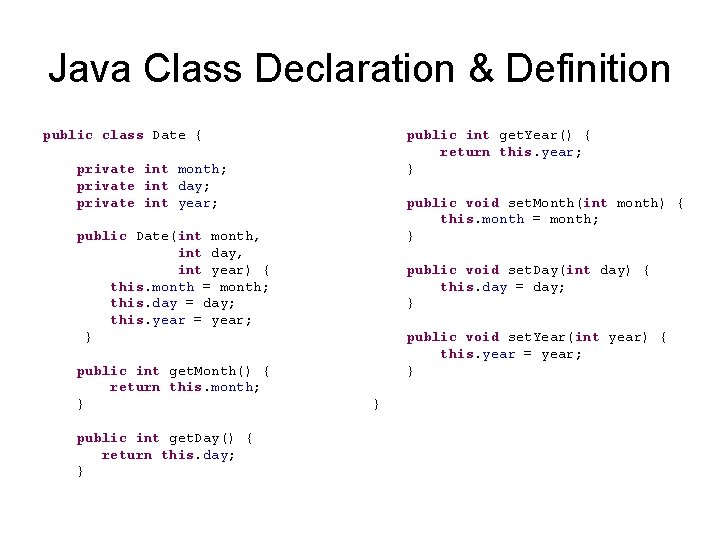
Java Class Declaration & Definition public class Date { public int get. Year() { return this. year; } private int month; private int day; private int year; public void set. Month(int month) { this. month = month; } public Date(int month, int day, int year) { this. month = month; this. day = day; this. year = year; } public int get. Month() { return this. month; } public int get. Day() { return this. day; } public void set. Day(int day) { this. day = day; } public void set. Year(int year) { this. year = year; } }
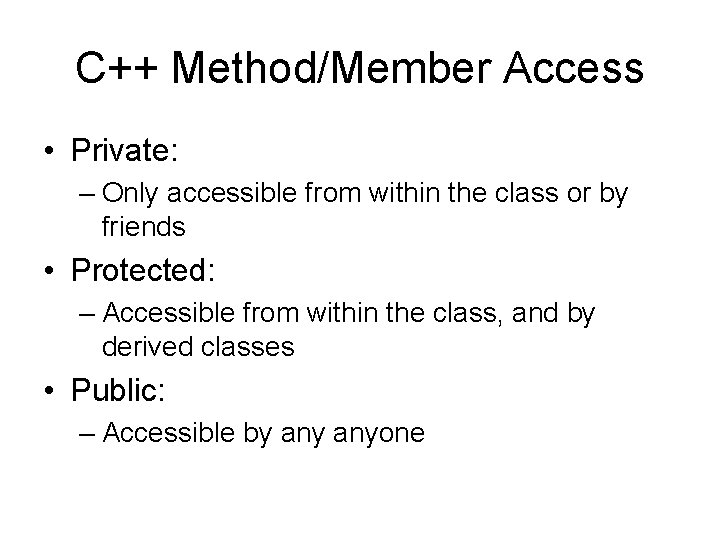
C++ Method/Member Access • Private: – Only accessible from within the class or by friends • Protected: – Accessible from within the class, and by derived classes • Public: – Accessible by anyone
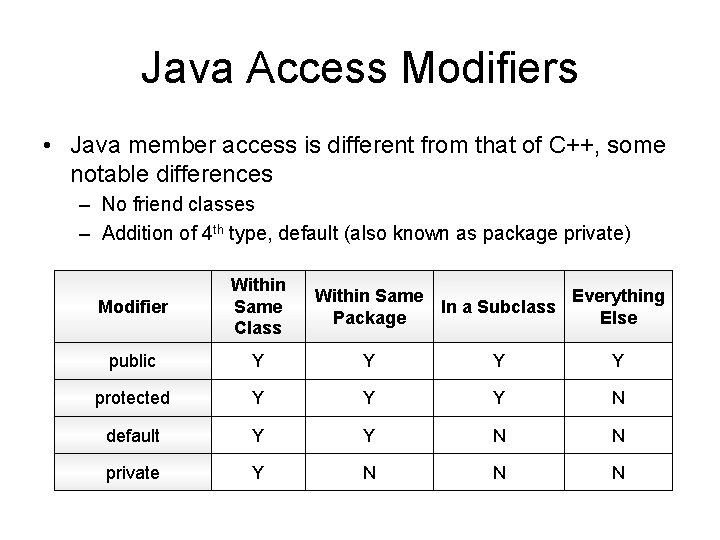
Java Access Modifiers • Java member access is different from that of C++, some notable differences – No friend classes – Addition of 4 th type, default (also known as package private) Modifier Within Same Class Within Same Package In a Subclass Everything Else public Y Y protected Y Y Y N default Y Y N N private Y N N N
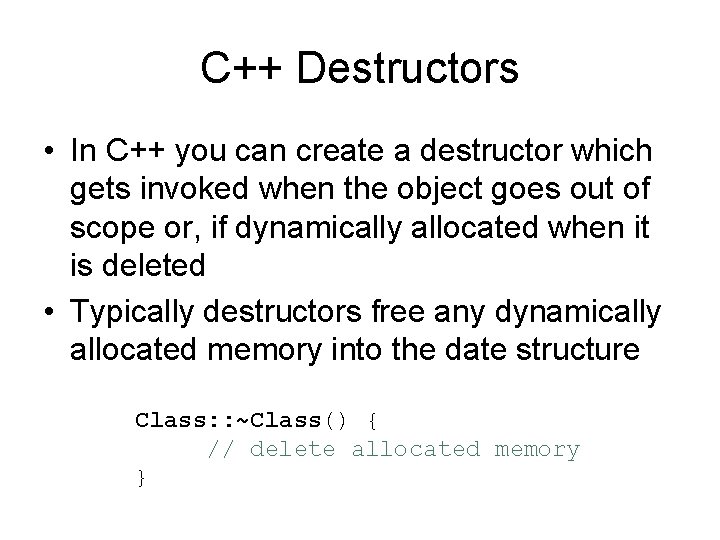
C++ Destructors • In C++ you can create a destructor which gets invoked when the object goes out of scope or, if dynamically allocated when it is deleted • Typically destructors free any dynamically allocated memory into the date structure Class: : ~Class() { // delete allocated memory }
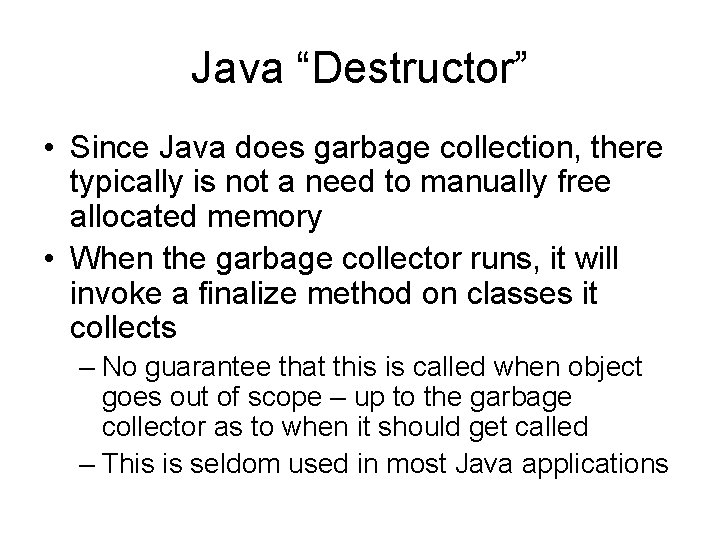
Java “Destructor” • Since Java does garbage collection, there typically is not a need to manually free allocated memory • When the garbage collector runs, it will invoke a finalize method on classes it collects – No guarantee that this is called when object goes out of scope – up to the garbage collector as to when it should get called – This is seldom used in most Java applications
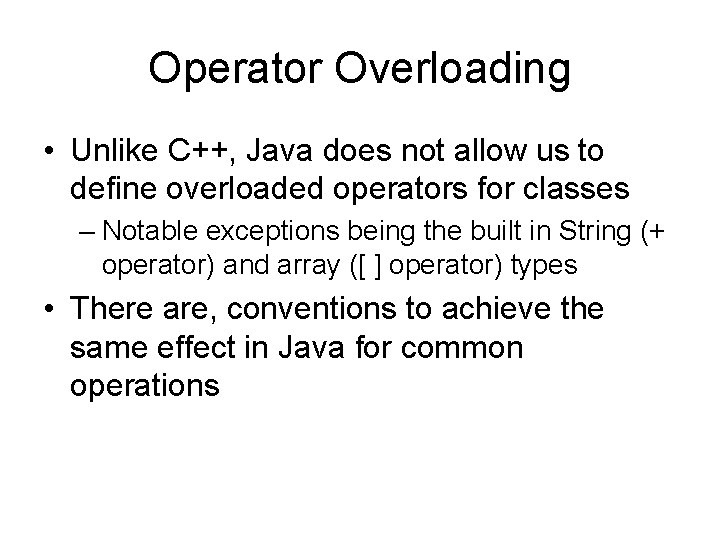
Operator Overloading • Unlike C++, Java does not allow us to define overloaded operators for classes – Notable exceptions being the built in String (+ operator) and array ([ ] operator) types • There are, conventions to achieve the same effect in Java for common operations
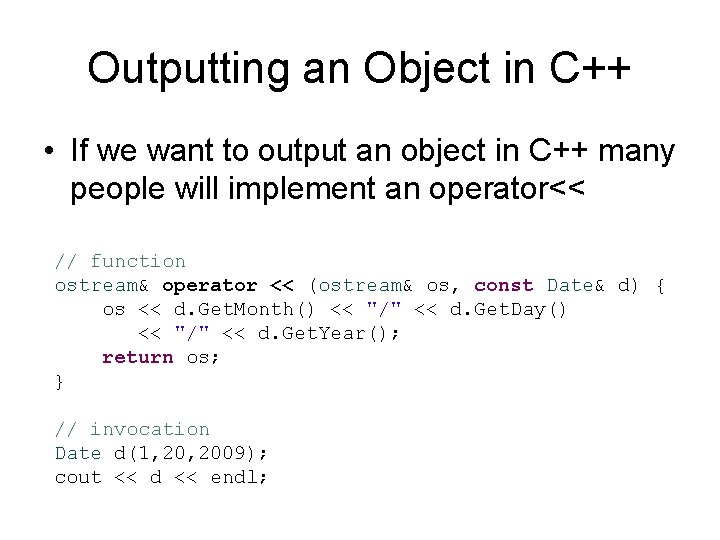
Outputting an Object in C++ • If we want to output an object in C++ many people will implement an operator<< // function ostream& operator << (ostream& os, const Date& d) { os << d. Get. Month() << "/" << d. Get. Day() << "/" << d. Get. Year(); return os; } // invocation Date d(1, 2009); cout << d << endl;
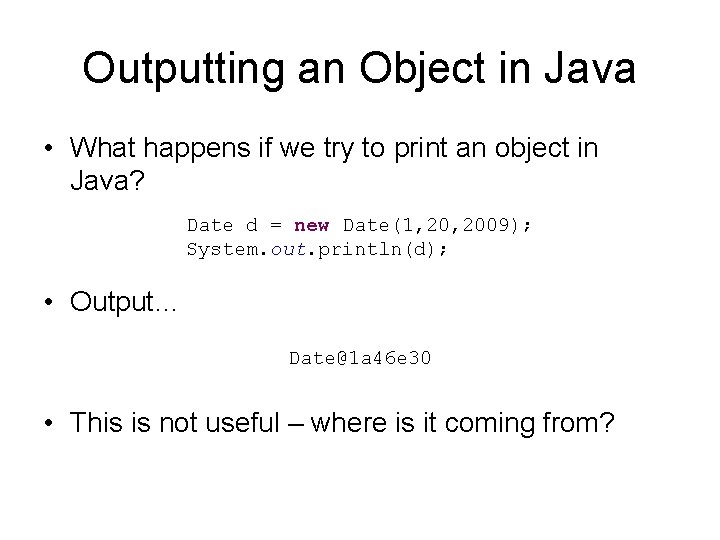
Outputting an Object in Java • What happens if we try to print an object in Java? Date d = new Date(1, 2009); System. out. println(d); • Output… Date@1 a 46 e 30 • This is not useful – where is it coming from?
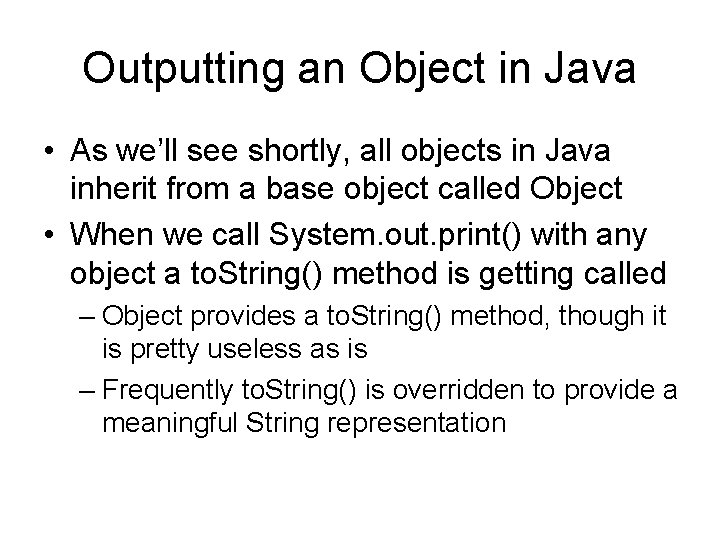
Outputting an Object in Java • As we’ll see shortly, all objects in Java inherit from a base object called Object • When we call System. out. print() with any object a to. String() method is getting called – Object provides a to. String() method, though it is pretty useless as is – Frequently to. String() is overridden to provide a meaningful String representation
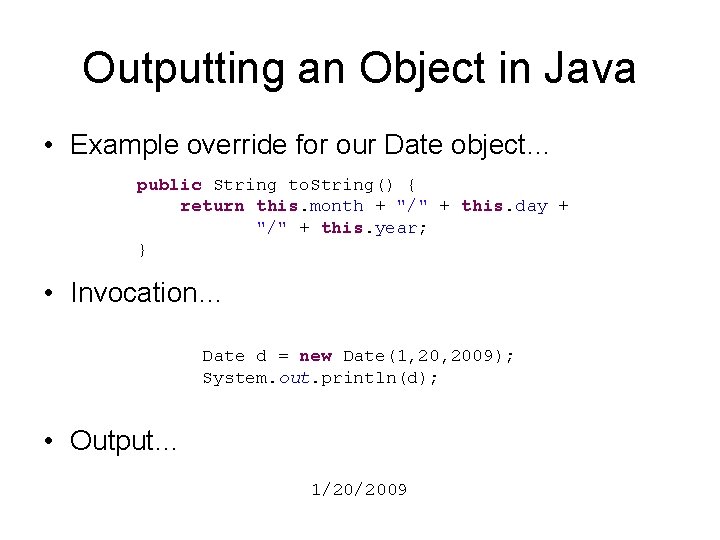
Outputting an Object in Java • Example override for our Date object… public String to. String() { return this. month + "/" + this. day + "/" + this. year; } • Invocation… Date d = new Date(1, 2009); System. out. println(d); • Output… 1/20/2009
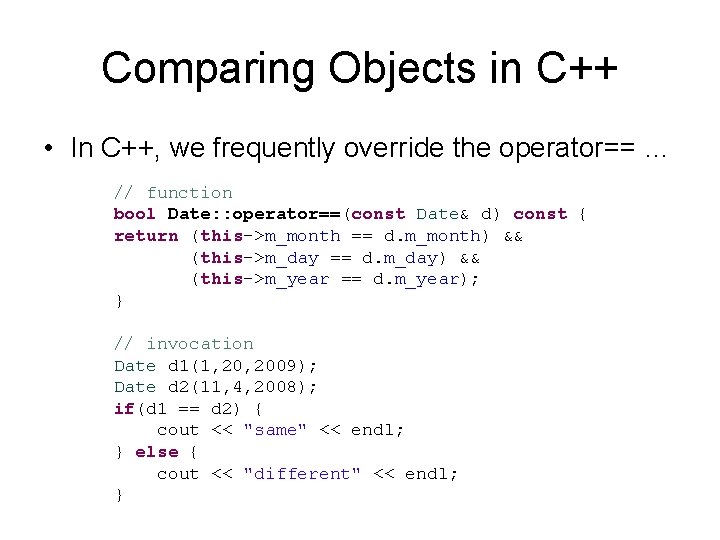
Comparing Objects in C++ • In C++, we frequently override the operator== … // function bool Date: : operator==(const Date& d) const { return (this->m_month == d. m_month) && (this->m_day == d. m_day) && (this->m_year == d. m_year); } // invocation Date d 1(1, 2009); Date d 2(11, 4, 2008); if(d 1 == d 2) { cout << "same" << endl; } else { cout << "different" << endl; }
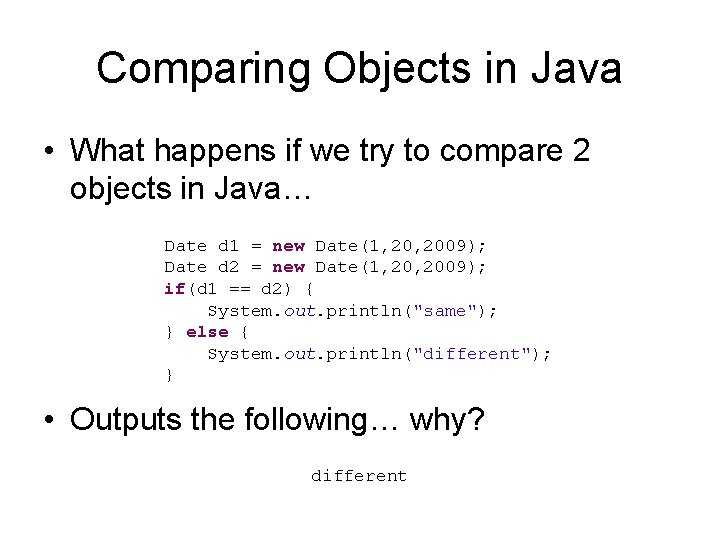
Comparing Objects in Java • What happens if we try to compare 2 objects in Java… Date d 1 = new Date(1, 2009); Date d 2 = new Date(1, 2009); if(d 1 == d 2) { System. out. println("same"); } else { System. out. println("different"); } • Outputs the following… why? different
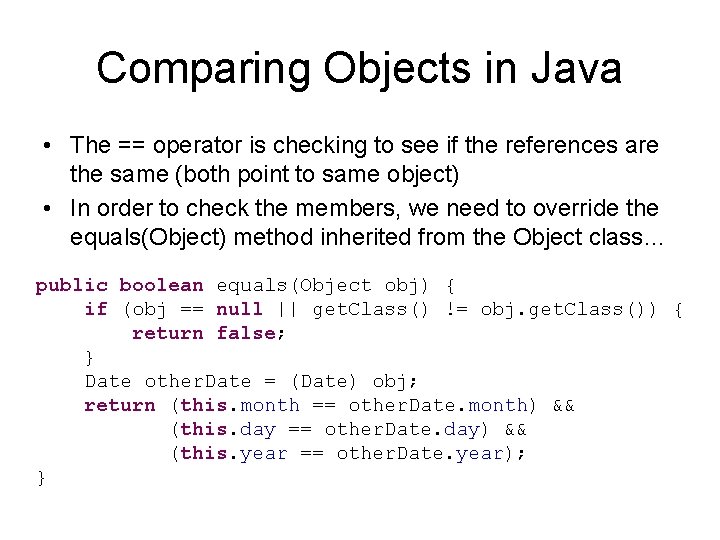
Comparing Objects in Java • The == operator is checking to see if the references are the same (both point to same object) • In order to check the members, we need to override the equals(Object) method inherited from the Object class… public boolean equals(Object obj) { if (obj == null || get. Class() != obj. get. Class()) { return false; } Date other. Date = (Date) obj; return (this. month == other. Date. month) && (this. day == other. Date. day) && (this. year == other. Date. year); }
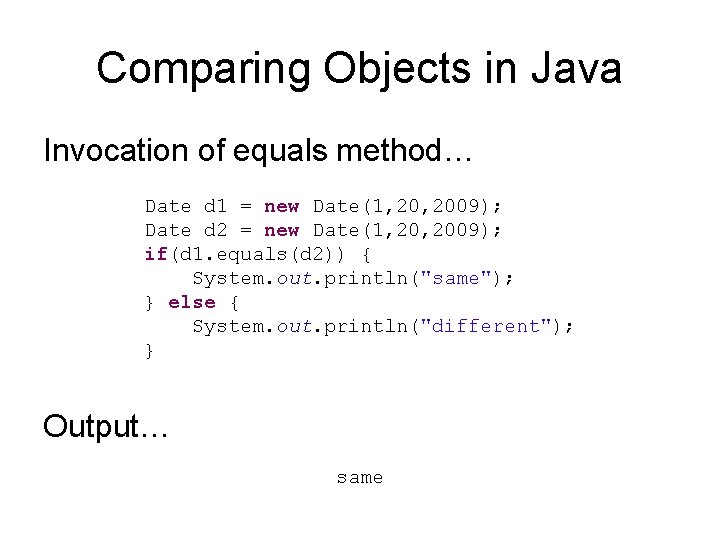
Comparing Objects in Java Invocation of equals method… Date d 1 = new Date(1, 2009); Date d 2 = new Date(1, 2009); if(d 1. equals(d 2)) { System. out. println("same"); } else { System. out. println("different"); } Output… same
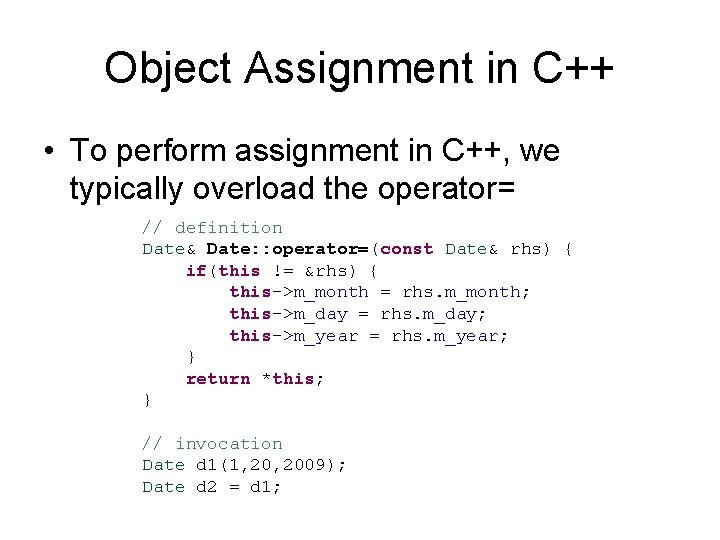
Object Assignment in C++ • To perform assignment in C++, we typically overload the operator= // definition Date& Date: : operator=(const Date& rhs) { if(this != &rhs) { this->m_month = rhs. m_month; this->m_day = rhs. m_day; this->m_year = rhs. m_year; } return *this; } // invocation Date d 1(1, 2009); Date d 2 = d 1;
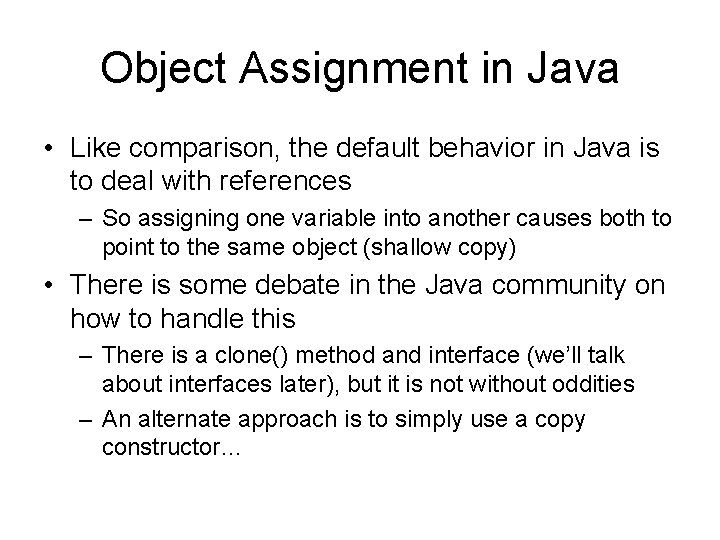
Object Assignment in Java • Like comparison, the default behavior in Java is to deal with references – So assigning one variable into another causes both to point to the same object (shallow copy) • There is some debate in the Java community on how to handle this – There is a clone() method and interface (we’ll talk about interfaces later), but it is not without oddities – An alternate approach is to simply use a copy constructor…
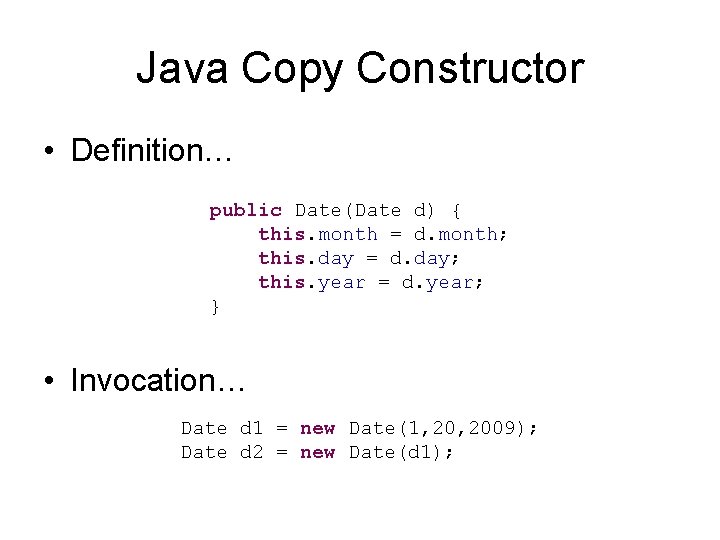
Java Copy Constructor • Definition… public Date(Date d) { this. month = d. month; this. day = d. day; this. year = d. year; } • Invocation… Date d 1 = new Date(1, 2009); Date d 2 = new Date(d 1);
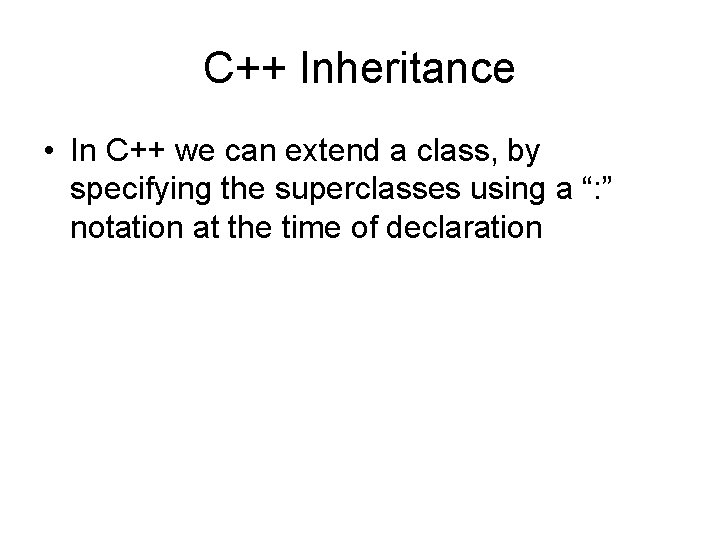
C++ Inheritance • In C++ we can extend a class, by specifying the superclasses using a “: ” notation at the time of declaration
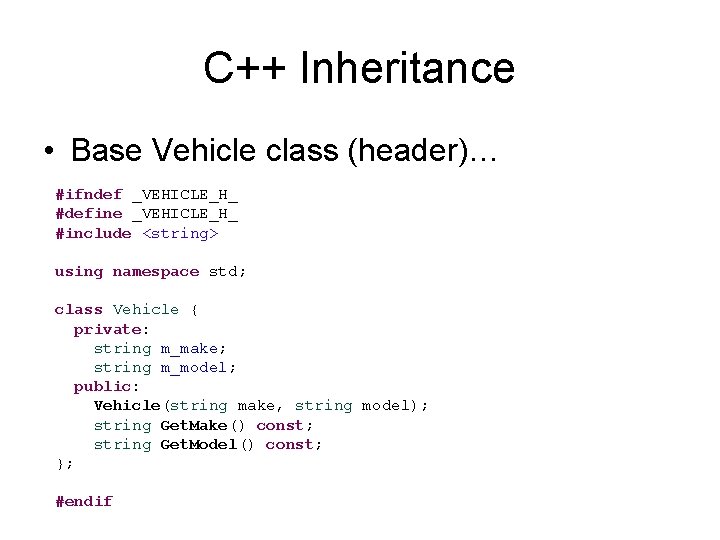
C++ Inheritance • Base Vehicle class (header)… #ifndef _VEHICLE_H_ #define _VEHICLE_H_ #include <string> using namespace std; class Vehicle { private: string m_make; string m_model; public: Vehicle(string make, string model); string Get. Make() const; string Get. Model() const; }; #endif
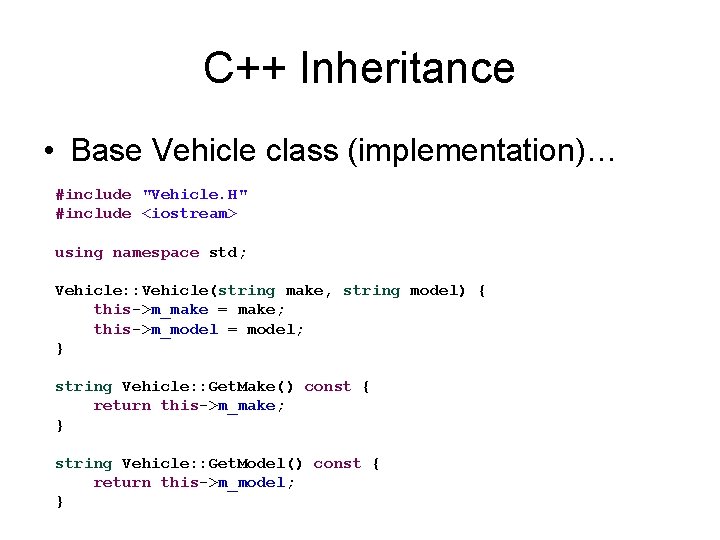
C++ Inheritance • Base Vehicle class (implementation)… #include "Vehicle. H" #include <iostream> using namespace std; Vehicle: : Vehicle(string make, string model) { this->m_make = make; this->m_model = model; } string Vehicle: : Get. Make() const { return this->m_make; } string Vehicle: : Get. Model() const { return this->m_model; }
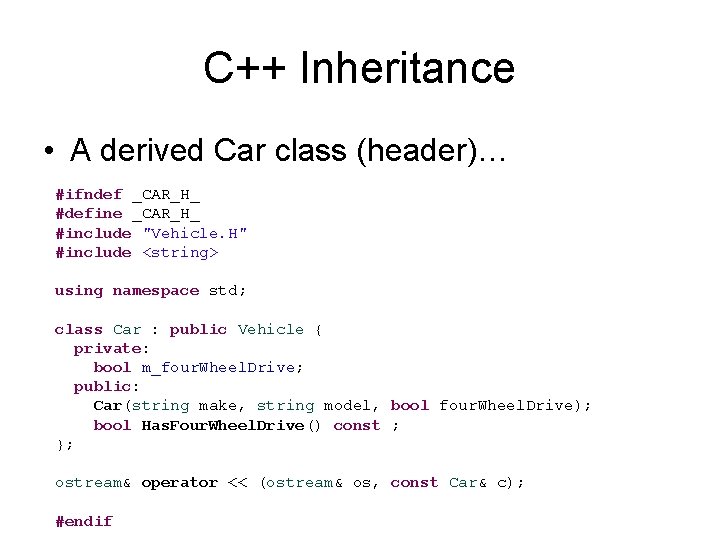
C++ Inheritance • A derived Car class (header)… #ifndef _CAR_H_ #define _CAR_H_ #include "Vehicle. H" #include <string> using namespace std; class Car : public Vehicle { private: bool m_four. Wheel. Drive; public: Car(string make, string model, bool four. Wheel. Drive); bool Has. Four. Wheel. Drive() const ; }; ostream& operator << (ostream& os, const Car& c); #endif
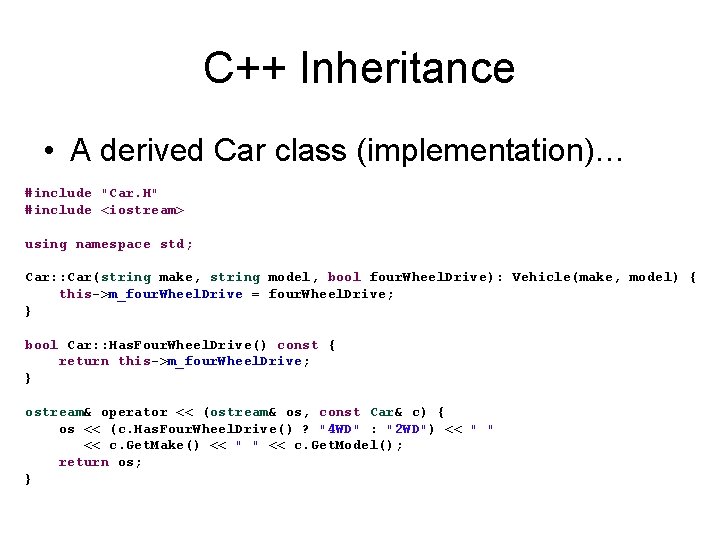
C++ Inheritance • A derived Car class (implementation)… #include "Car. H" #include <iostream> using namespace std; Car: : Car(string make, string model, bool four. Wheel. Drive): Vehicle(make, model) { this->m_four. Wheel. Drive = four. Wheel. Drive; } bool Car: : Has. Four. Wheel. Drive() const { return this->m_four. Wheel. Drive; } ostream& operator << (ostream& os, const Car& c) { os << (c. Has. Four. Wheel. Drive() ? "4 WD" : "2 WD") << " " << c. Get. Make() << " " << c. Get. Model(); return os; }
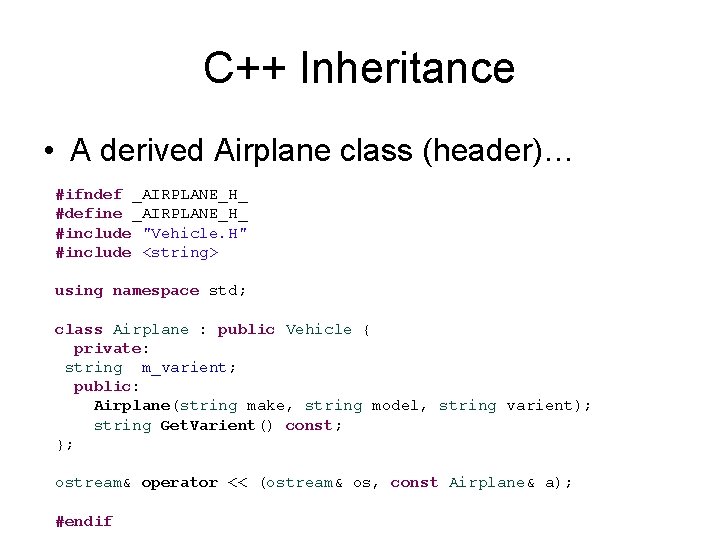
C++ Inheritance • A derived Airplane class (header)… #ifndef _AIRPLANE_H_ #define _AIRPLANE_H_ #include "Vehicle. H" #include <string> using namespace std; class Airplane : public Vehicle { private: string m_varient; public: Airplane(string make, string model, string varient); string Get. Varient() const; }; ostream& operator << (ostream& os, const Airplane& a); #endif
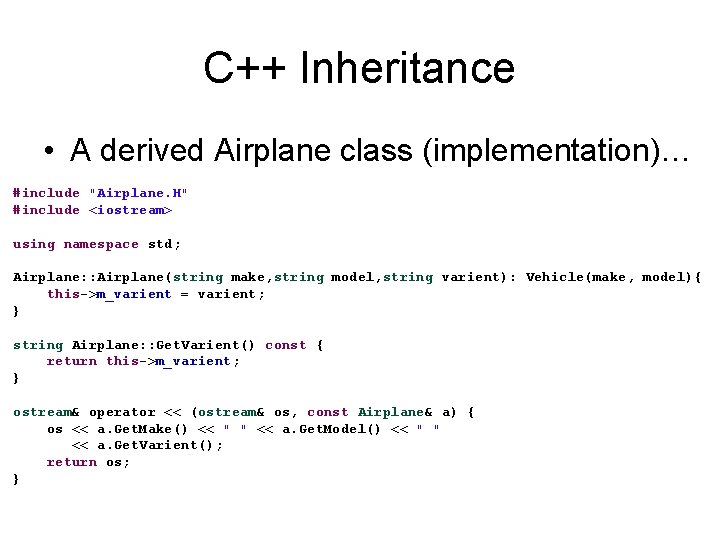
C++ Inheritance • A derived Airplane class (implementation)… #include "Airplane. H" #include <iostream> using namespace std; Airplane: : Airplane(string make, string model, string varient): Vehicle(make, model){ this->m_varient = varient; } string Airplane: : Get. Varient() const { return this->m_varient; } ostream& operator << (ostream& os, const Airplane& a) { os << a. Get. Make() << " " << a. Get. Model() << " " << a. Get. Varient(); return os; }
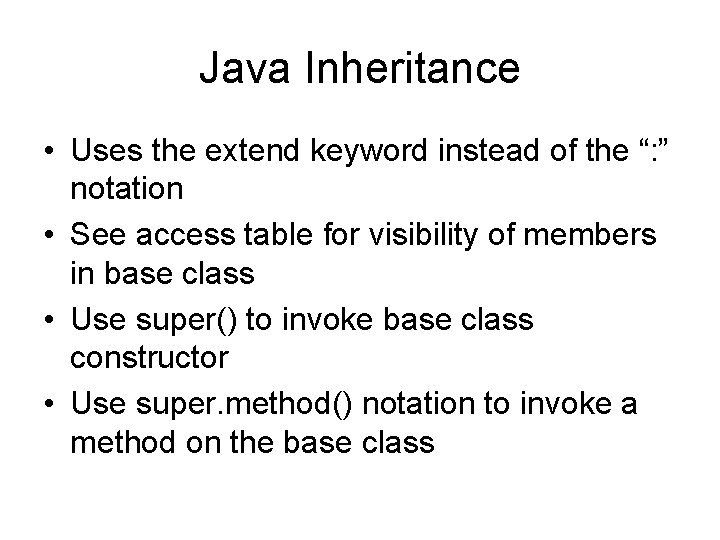
Java Inheritance • Uses the extend keyword instead of the “: ” notation • See access table for visibility of members in base class • Use super() to invoke base class constructor • Use super. method() notation to invoke a method on the base class
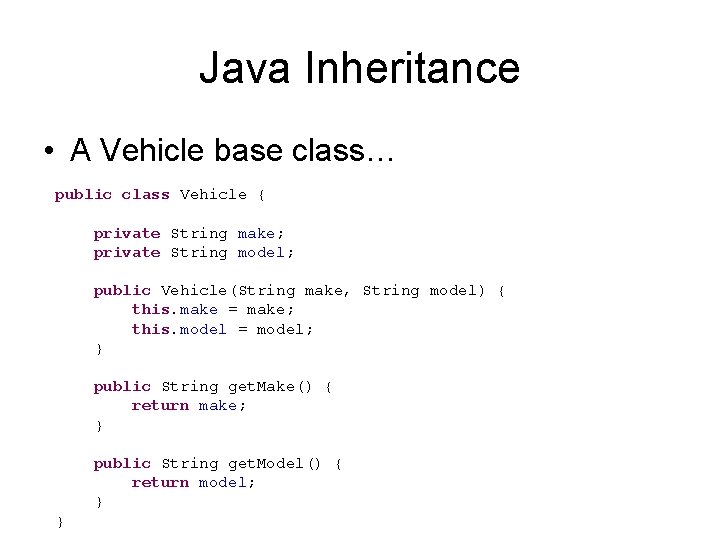
Java Inheritance • A Vehicle base class… public class Vehicle { private String make; private String model; public Vehicle(String make, String model) { this. make = make; this. model = model; } public String get. Make() { return make; } public String get. Model() { return model; } }
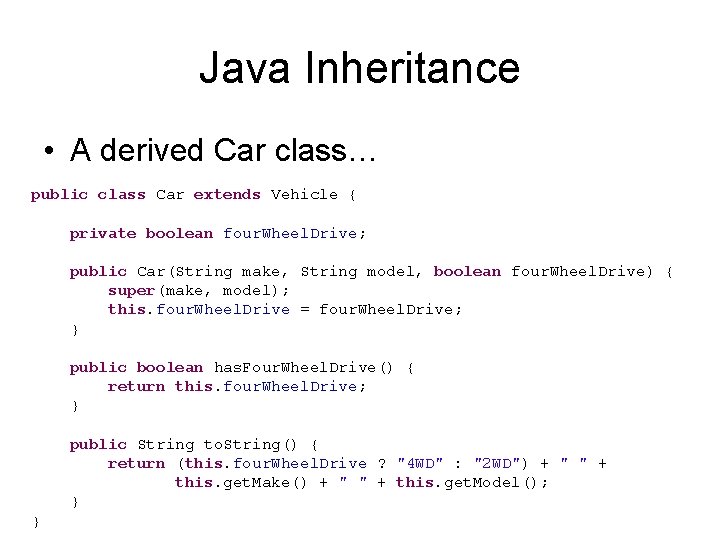
Java Inheritance • A derived Car class… public class Car extends Vehicle { private boolean four. Wheel. Drive; public Car(String make, String model, boolean four. Wheel. Drive) { super(make, model); this. four. Wheel. Drive = four. Wheel. Drive; } public boolean has. Four. Wheel. Drive() { return this. four. Wheel. Drive; } public String to. String() { return (this. four. Wheel. Drive ? "4 WD" : "2 WD") + " " + this. get. Make() + " " + this. get. Model(); } }
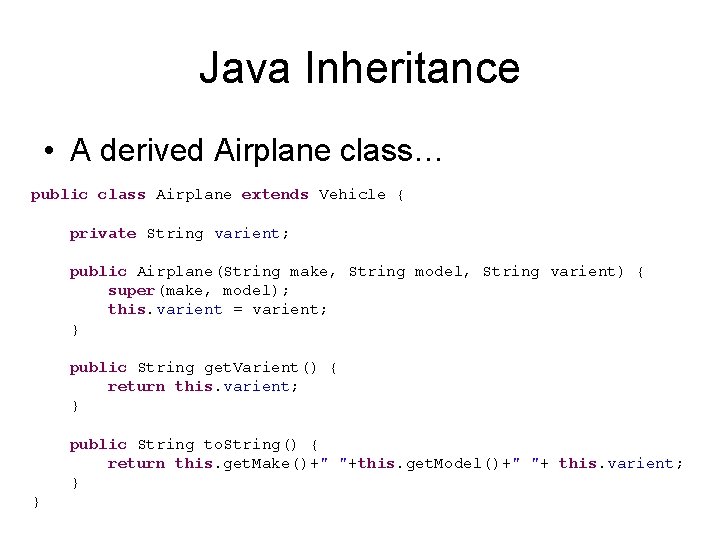
Java Inheritance • A derived Airplane class… public class Airplane extends Vehicle { private String varient; public Airplane(String make, String model, String varient) { super(make, model); this. varient = varient; } public String get. Varient() { return this. varient; } public String to. String() { return this. get. Make()+" "+this. get. Model()+" "+ this. varient; } }
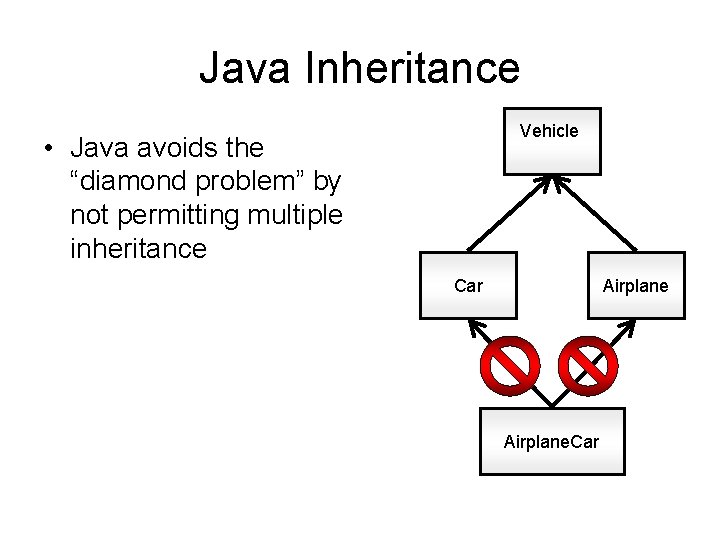
Java Inheritance Vehicle • Java avoids the “diamond problem” by not permitting multiple inheritance Car Airplane. Car
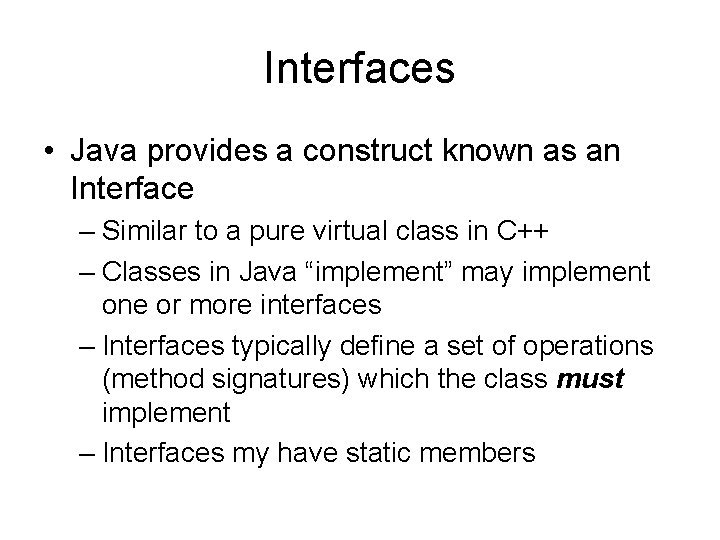
Interfaces • Java provides a construct known as an Interface – Similar to a pure virtual class in C++ – Classes in Java “implement” may implement one or more interfaces – Interfaces typically define a set of operations (method signatures) which the class must implement – Interfaces my have static members
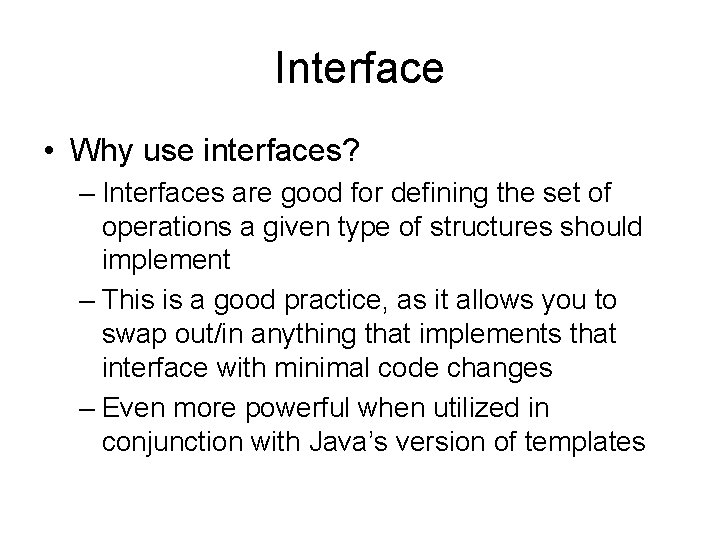
Interface • Why use interfaces? – Interfaces are good for defining the set of operations a given type of structures should implement – This is a good practice, as it allows you to swap out/in anything that implements that interface with minimal code changes – Even more powerful when utilized in conjunction with Java’s version of templates
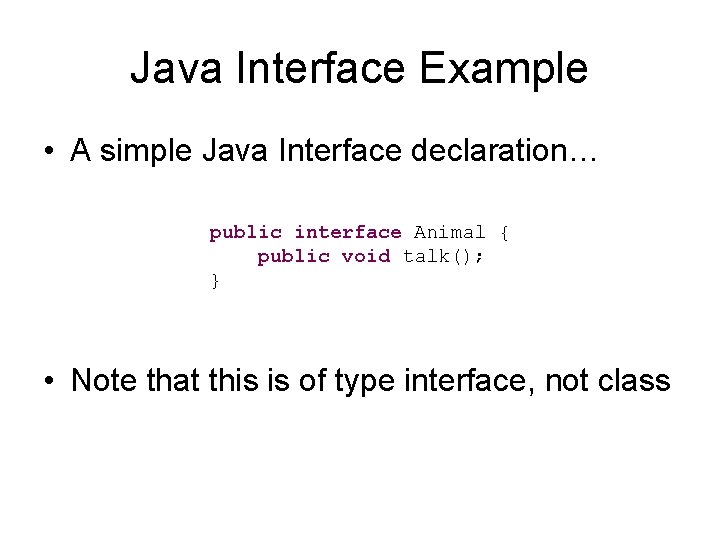
Java Interface Example • A simple Java Interface declaration… public interface Animal { public void talk(); } • Note that this is of type interface, not class
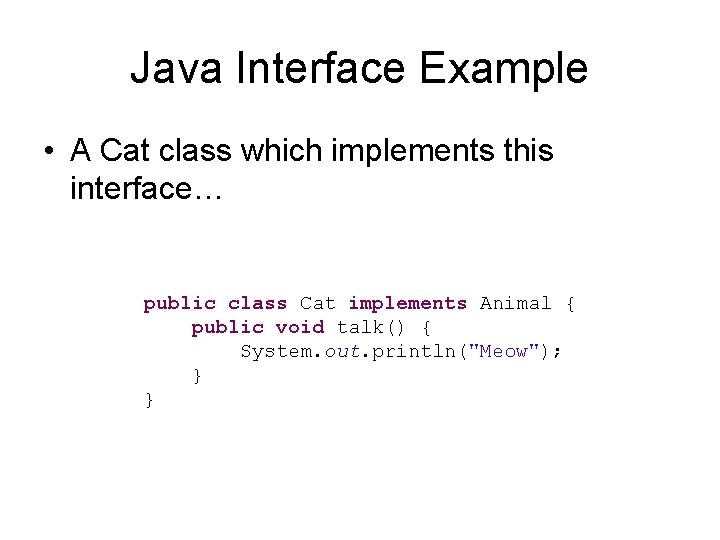
Java Interface Example • A Cat class which implements this interface… public class Cat implements Animal { public void talk() { System. out. println("Meow"); } }
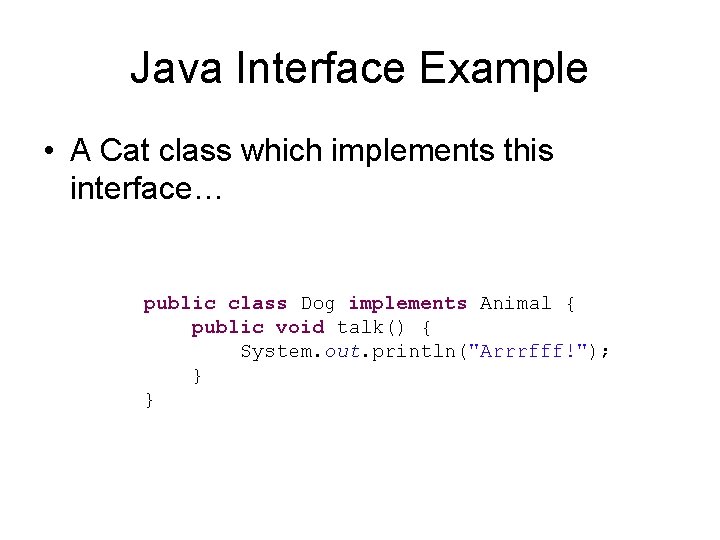
Java Interface Example • A Cat class which implements this interface… public class Dog implements Animal { public void talk() { System. out. println("Arrrfff!"); } }
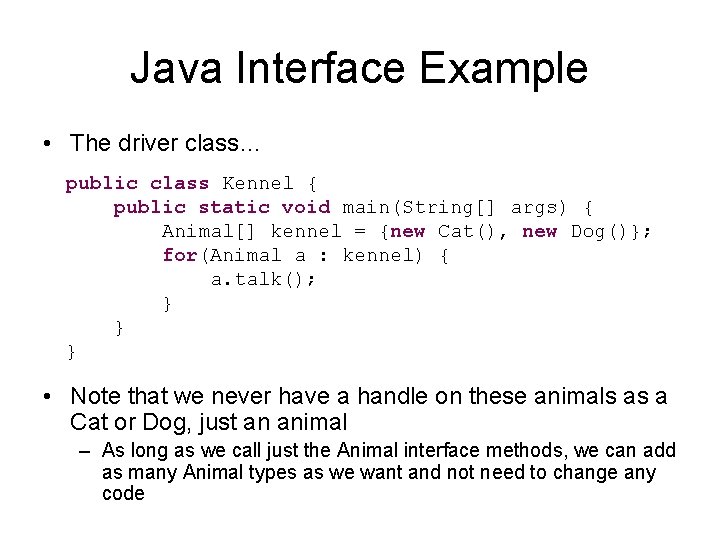
Java Interface Example • The driver class… public class Kennel { public static void main(String[] args) { Animal[] kennel = {new Cat(), new Dog()}; for(Animal a : kennel) { a. talk(); } } } • Note that we never have a handle on these animals as a Cat or Dog, just an animal – As long as we call just the Animal interface methods, we can add as many Animal types as we want and not need to change any code
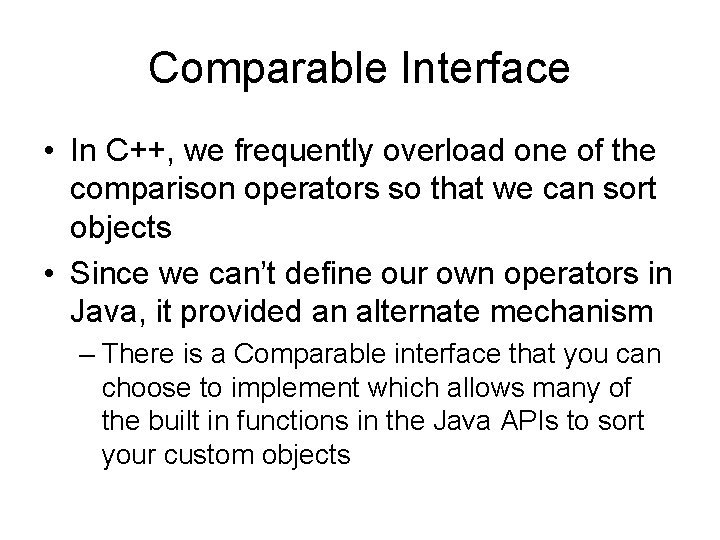
Comparable Interface • In C++, we frequently overload one of the comparison operators so that we can sort objects • Since we can’t define our own operators in Java, it provided an alternate mechanism – There is a Comparable interface that you can choose to implement which allows many of the built in functions in the Java APIs to sort your custom objects
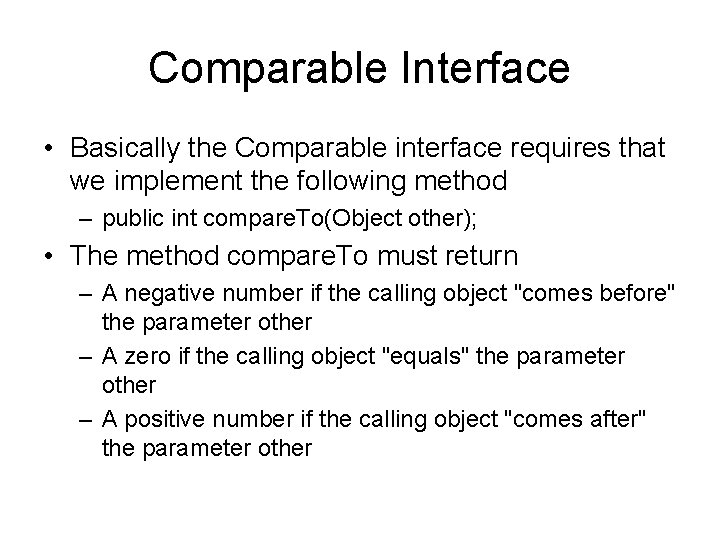
Comparable Interface • Basically the Comparable interface requires that we implement the following method – public int compare. To(Object other); • The method compare. To must return – A negative number if the calling object "comes before" the parameter other – A zero if the calling object "equals" the parameter other – A positive number if the calling object "comes after" the parameter other
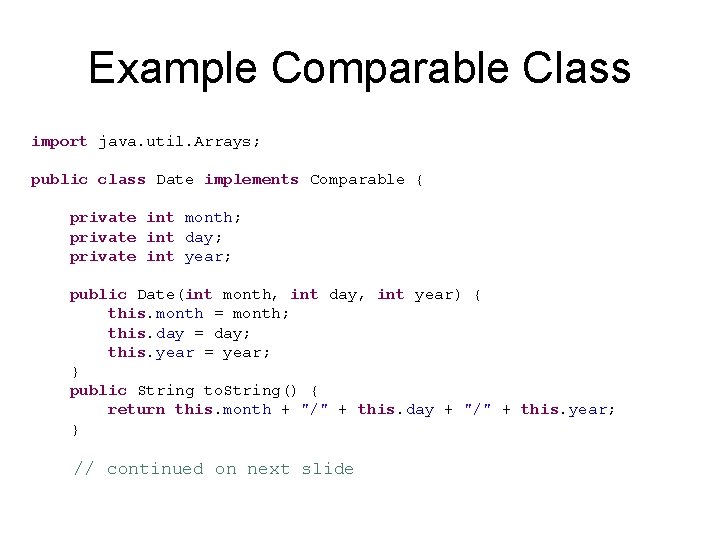
Example Comparable Class import java. util. Arrays; public class Date implements Comparable { private int month; private int day; private int year; public Date(int month, int day, int year) { this. month = month; this. day = day; this. year = year; } public String to. String() { return this. month + "/" + this. day + "/" + this. year; } // continued on next slide
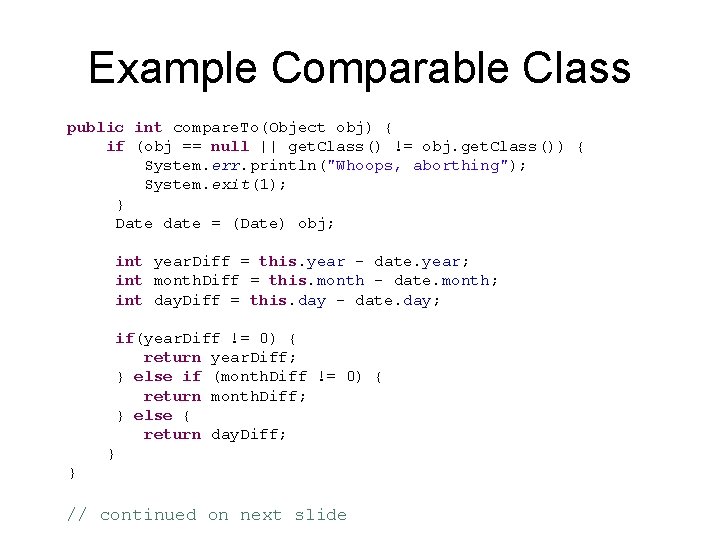
Example Comparable Class public int compare. To(Object obj) { if (obj == null || get. Class() != obj. get. Class()) { System. err. println("Whoops, aborthing"); System. exit(1); } Date date = (Date) obj; int year. Diff = this. year - date. year; int month. Diff = this. month - date. month; int day. Diff = this. day - date. day; if(year. Diff != 0) { return year. Diff; } else if (month. Diff != 0) { return month. Diff; } else { return day. Diff; } } // continued on next slide
![Example Comparable Class public static void mainString args Date dates new Example Comparable Class public static void main(String[] args) { Date[] dates = { new](https://slidetodoc.com/presentation_image_h/349b7b536872183dfb14e0b76266de9e/image-87.jpg)
Example Comparable Class public static void main(String[] args) { Date[] dates = { new Date(10, 7, 2008), new Date(10, 15, 2008), new Date(9, 26, 2008), }; Arrays. sort(dates); for(Date d : dates) a{ System. out. println(d); } } }
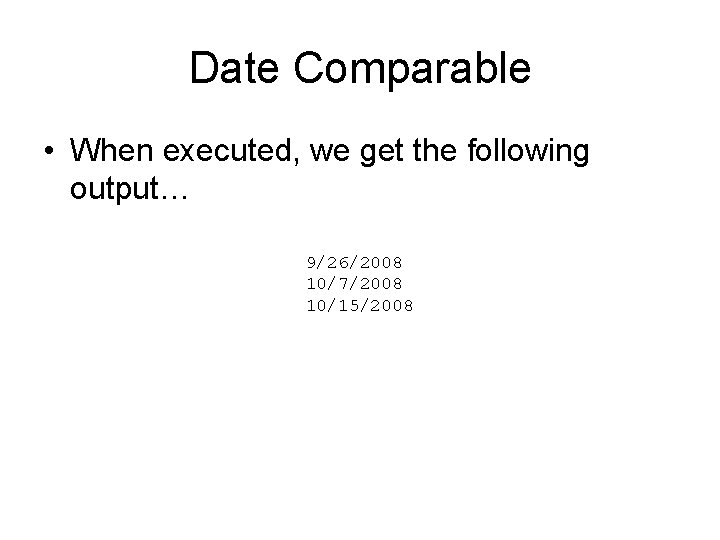
Date Comparable • When executed, we get the following output… 9/26/2008 10/7/2008 10/15/2008
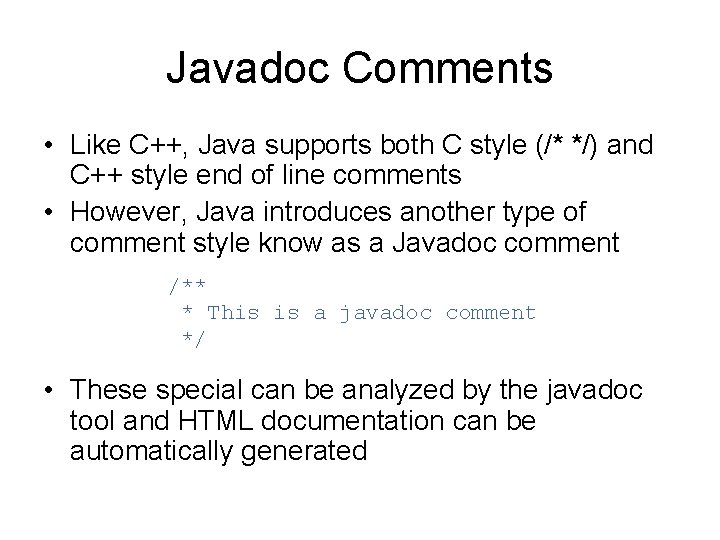
Javadoc Comments • Like C++, Java supports both C style (/* */) and C++ style end of line comments • However, Java introduces another type of comment style know as a Javadoc comment /** * This is a javadoc comment */ • These special can be analyzed by the javadoc tool and HTML documentation can be automatically generated
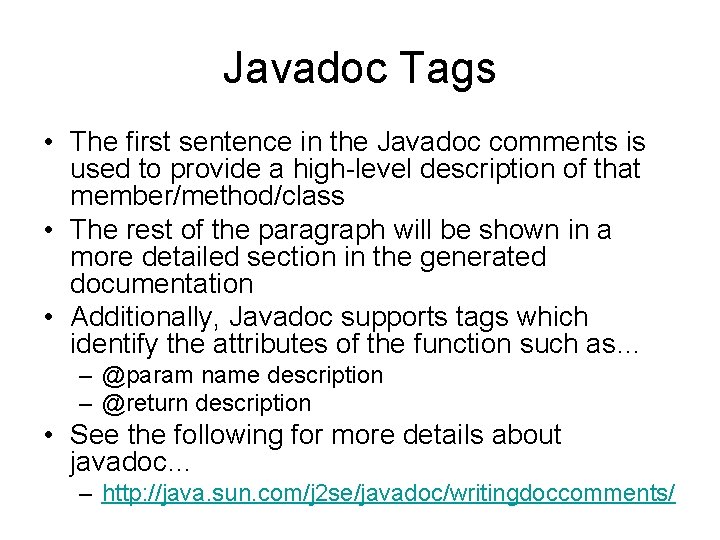
Javadoc Tags • The first sentence in the Javadoc comments is used to provide a high-level description of that member/method/class • The rest of the paragraph will be shown in a more detailed section in the generated documentation • Additionally, Javadoc supports tags which identify the attributes of the function such as… – @param name description – @return description • See the following for more details about javadoc… – http: //java. sun. com/j 2 se/javadoc/writingdoccomments/
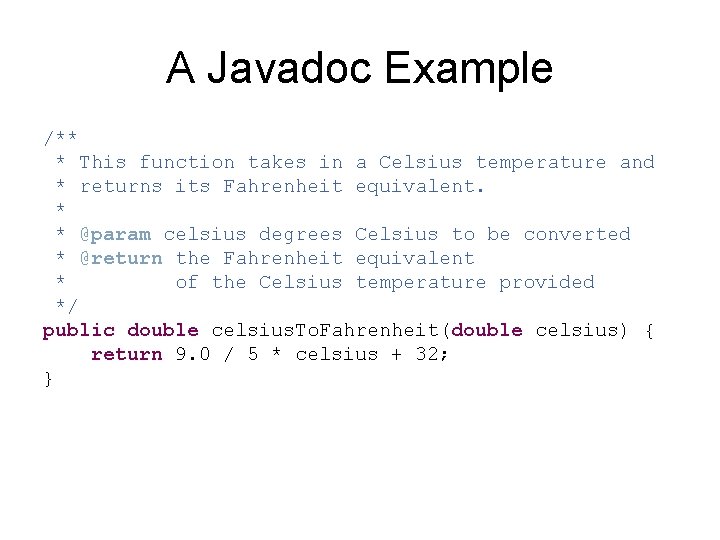
A Javadoc Example /** * This function takes in a Celsius temperature and * returns its Fahrenheit equivalent. * * @param celsius degrees Celsius to be converted * @return the Fahrenheit equivalent * of the Celsius temperature provided */ public double celsius. To. Fahrenheit(double celsius) { return 9. 0 / 5 * celsius + 32; }
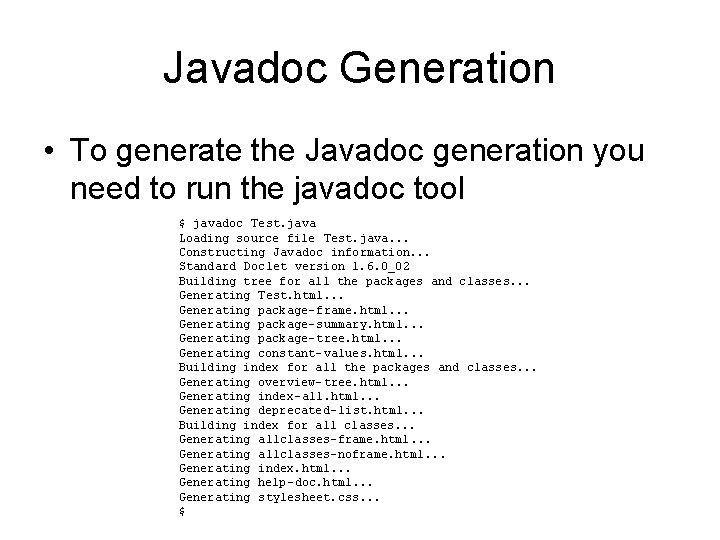
Javadoc Generation • To generate the Javadoc generation you need to run the javadoc tool $ javadoc Test. java Loading source file Test. java. . . Constructing Javadoc information. . . Standard Doclet version 1. 6. 0_02 Building tree for all the packages and classes. . . Generating Test. html. . . Generating package-frame. html. . . Generating package-summary. html. . . Generating package-tree. html. . . Generating constant-values. html. . . Building index for all the packages and classes. . . Generating overview-tree. html. . . Generating index-all. html. . . Generating deprecated-list. html. . . Building index for all classes. . . Generating allclasses-frame. html. . . Generating allclasses-noframe. html. . . Generating index. html. . . Generating help-doc. html. . . Generating stylesheet. css. . . $
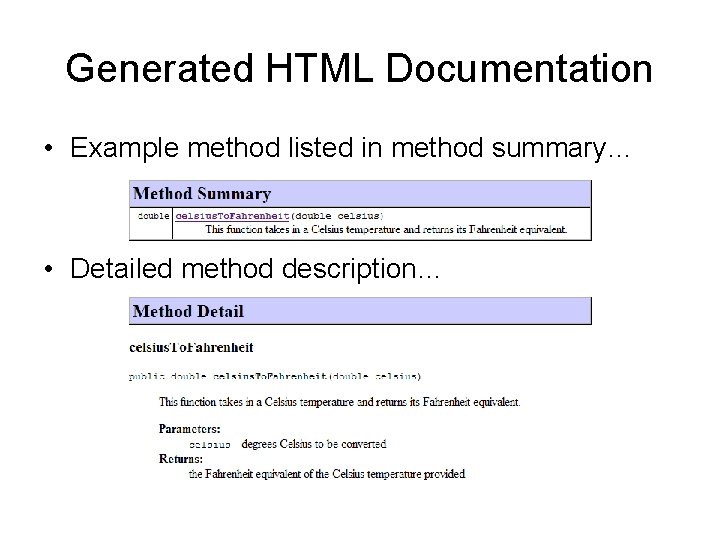
Generated HTML Documentation • Example method listed in method summary… • Detailed method description…
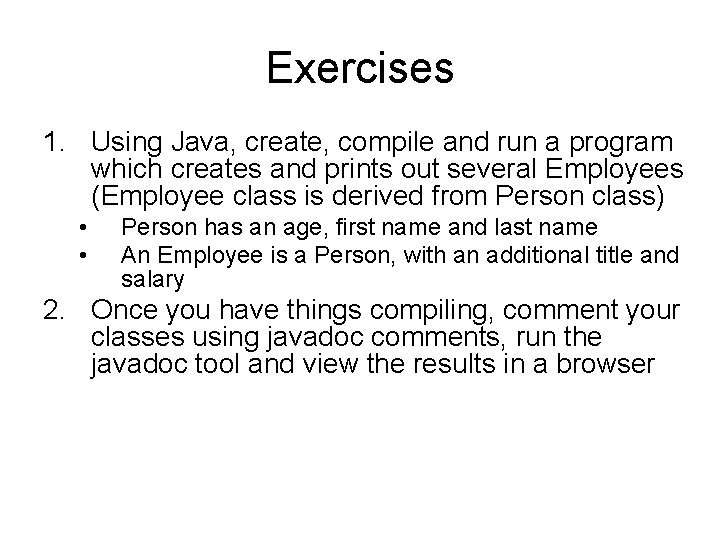
Exercises 1. Using Java, create, compile and run a program which creates and prints out several Employees (Employee class is derived from Person class) • • Person has an age, first name and last name An Employee is a Person, with an additional title and salary 2. Once you have things compiling, comment your classes using javadoc comments, run the javadoc tool and view the results in a browser