Week 4 Conditional Execution CS 177 1 Conditional
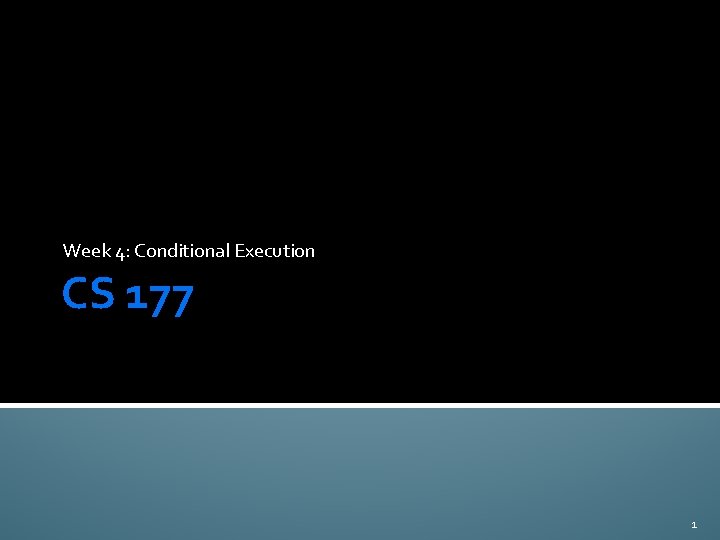
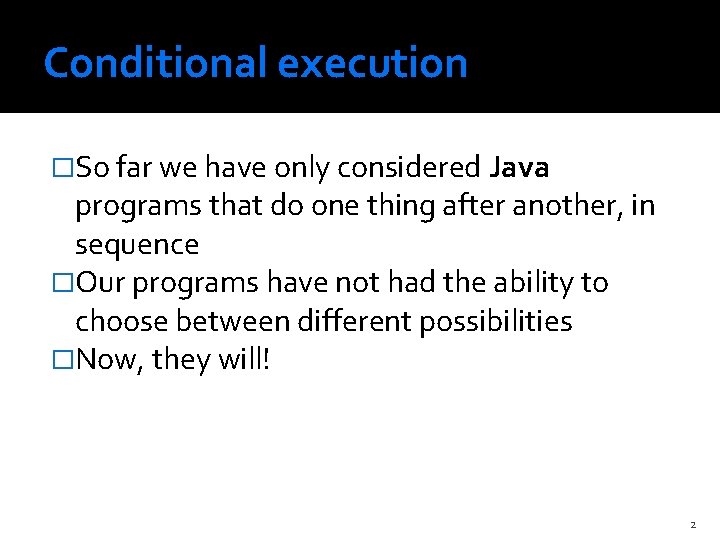
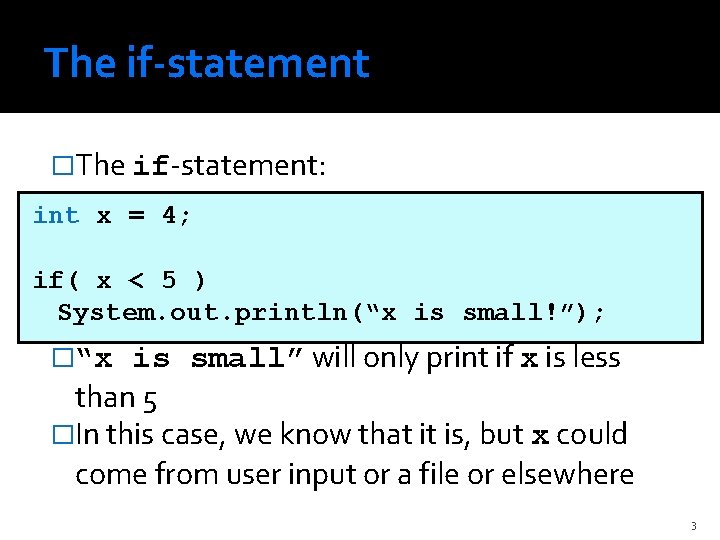
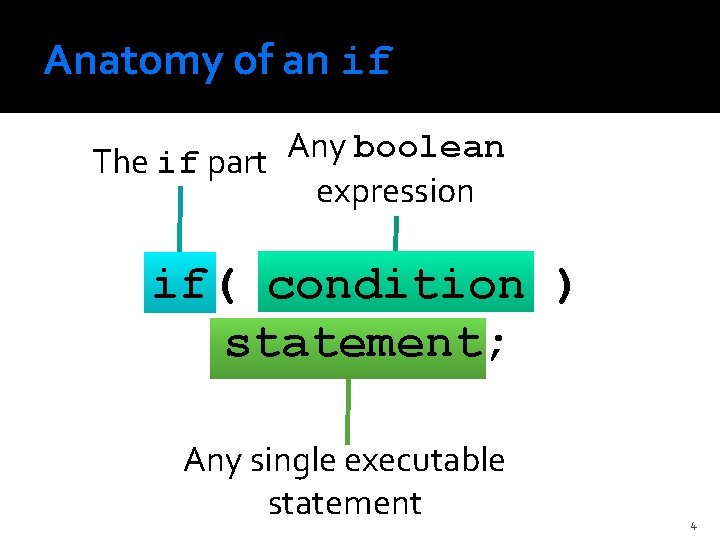
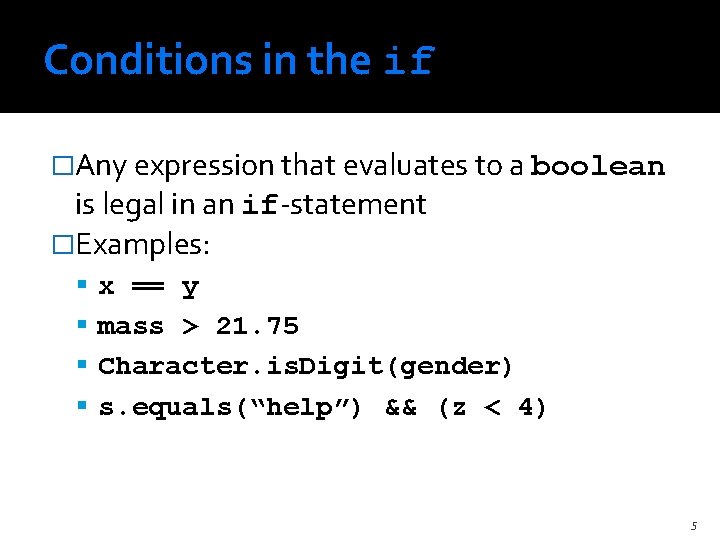
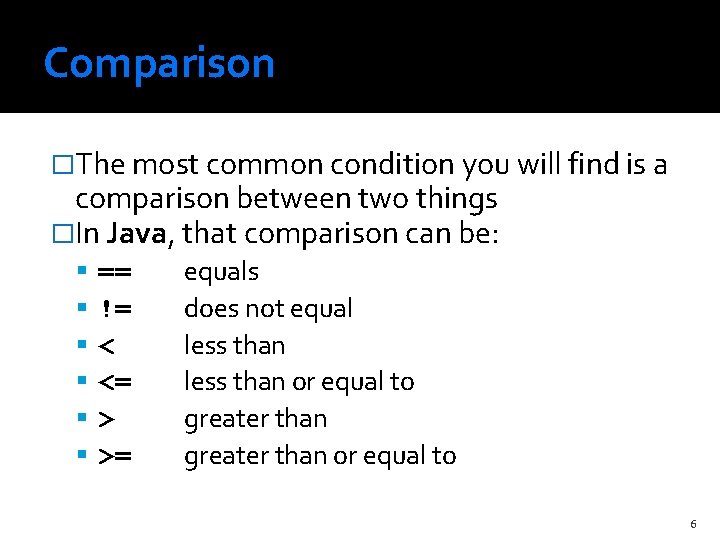
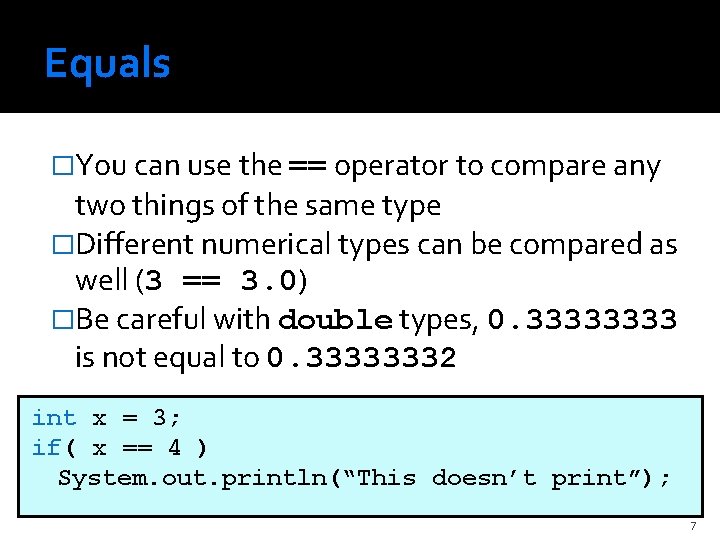
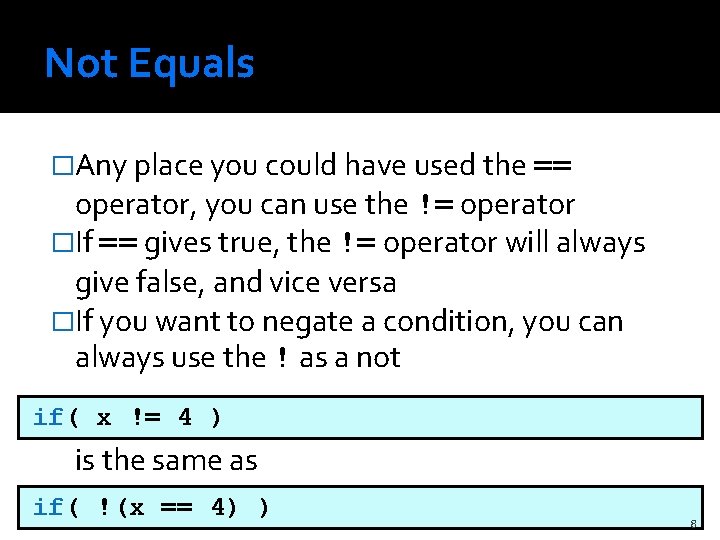
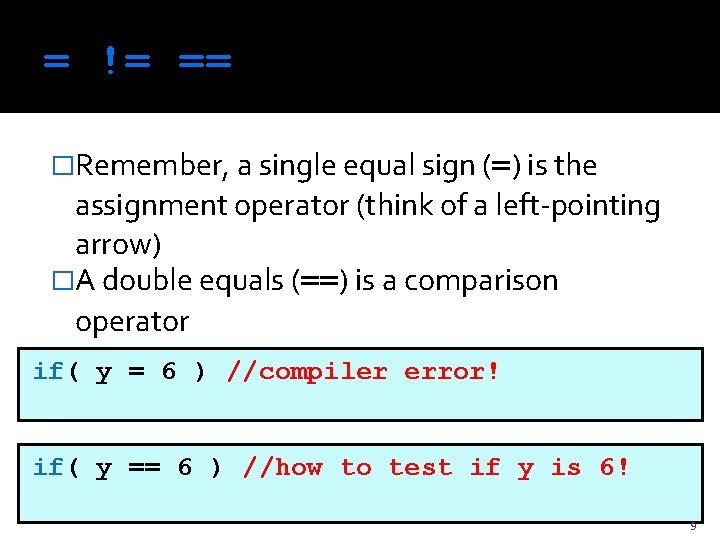
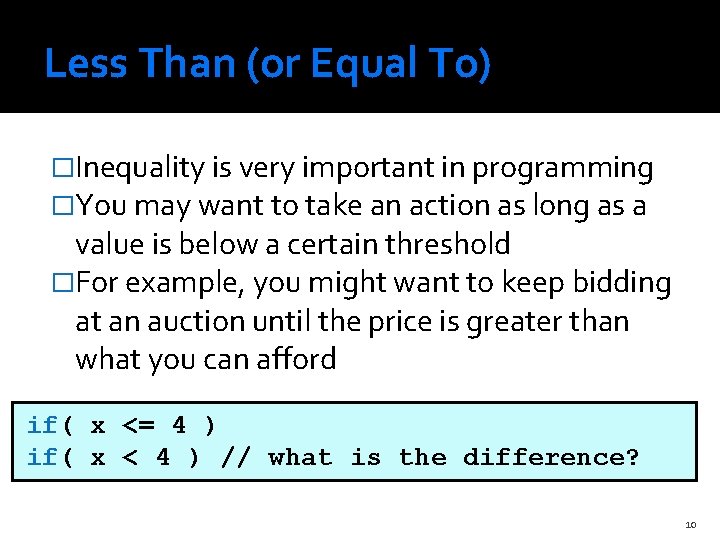
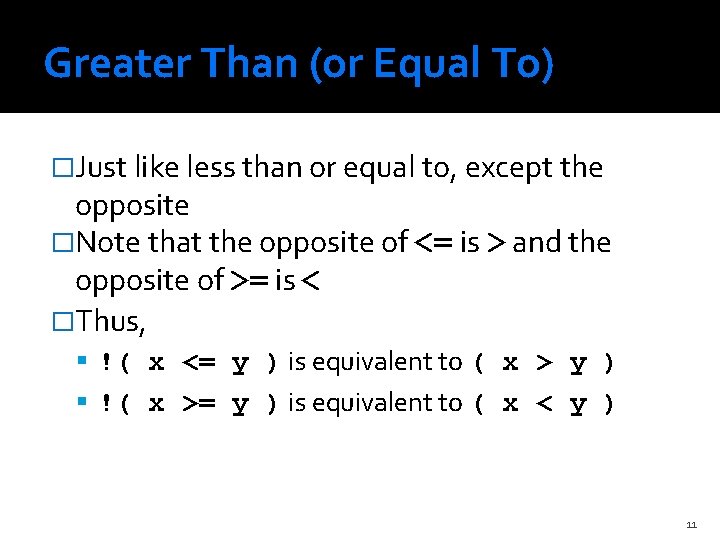
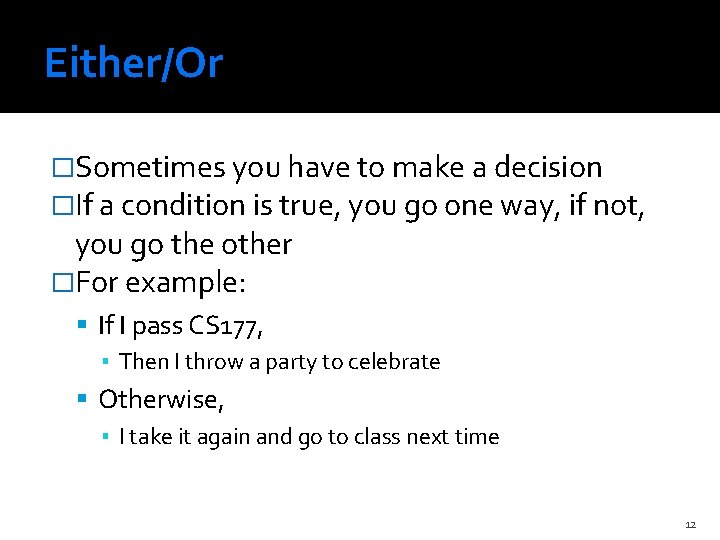
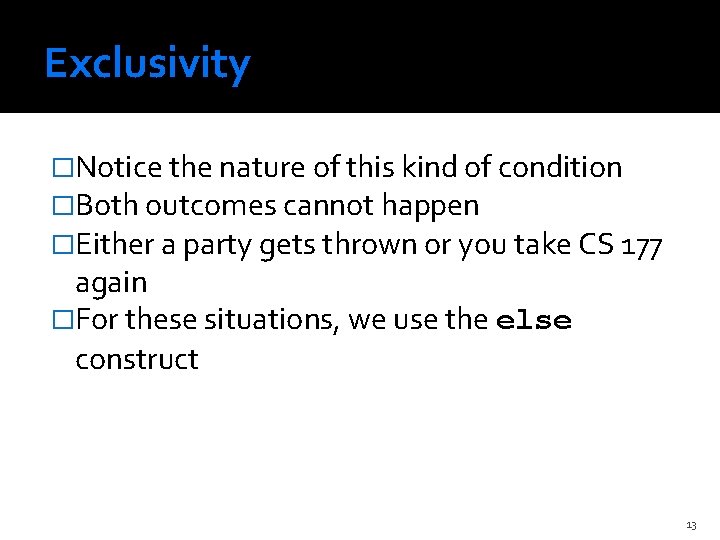
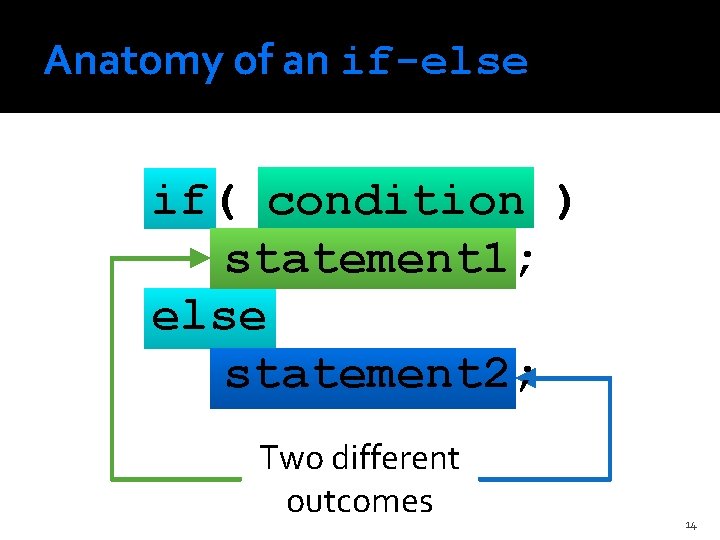
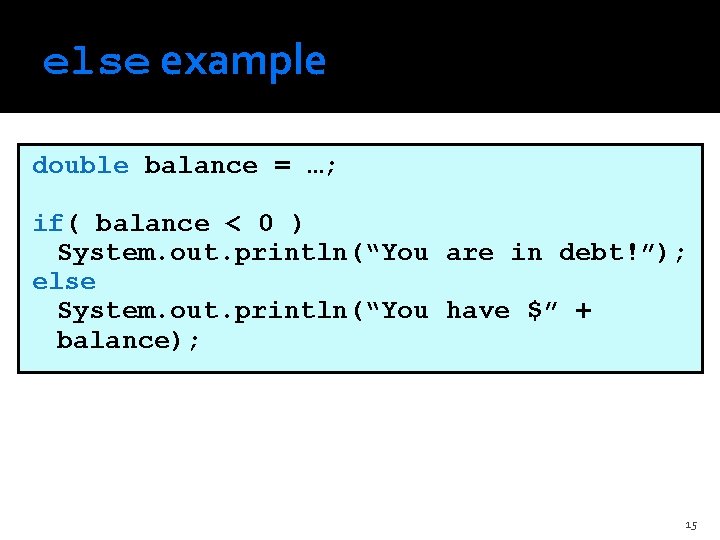
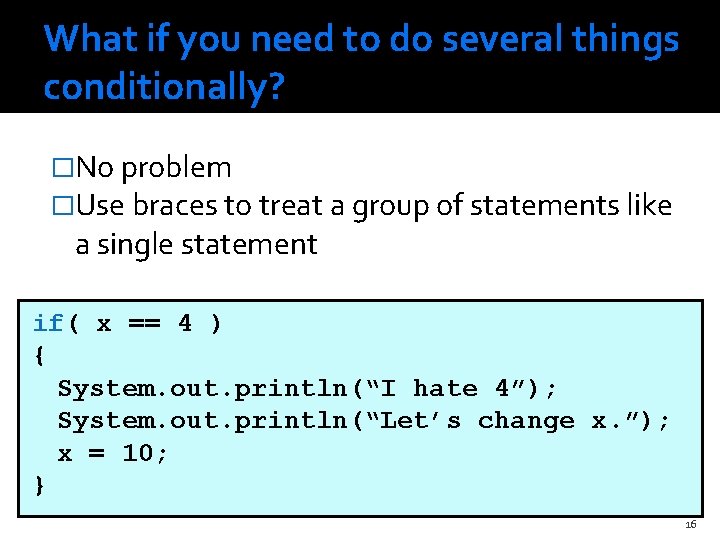
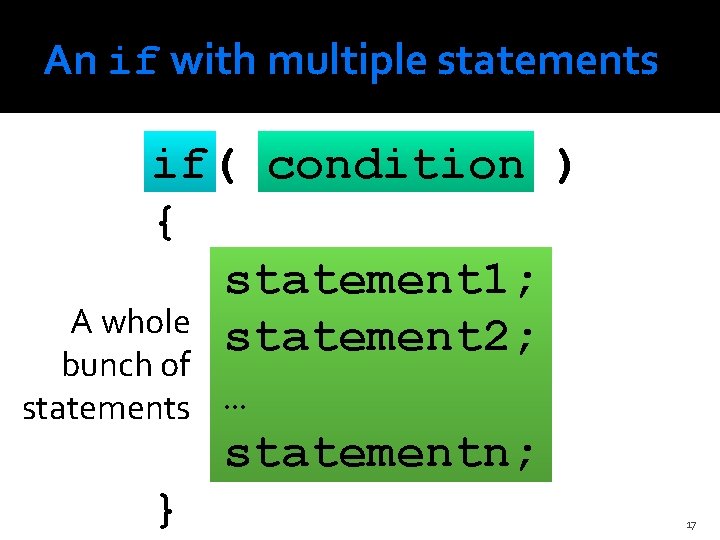
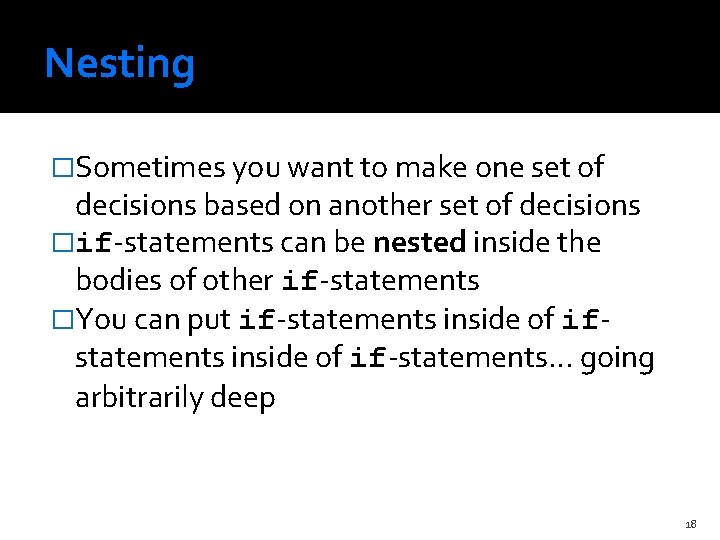
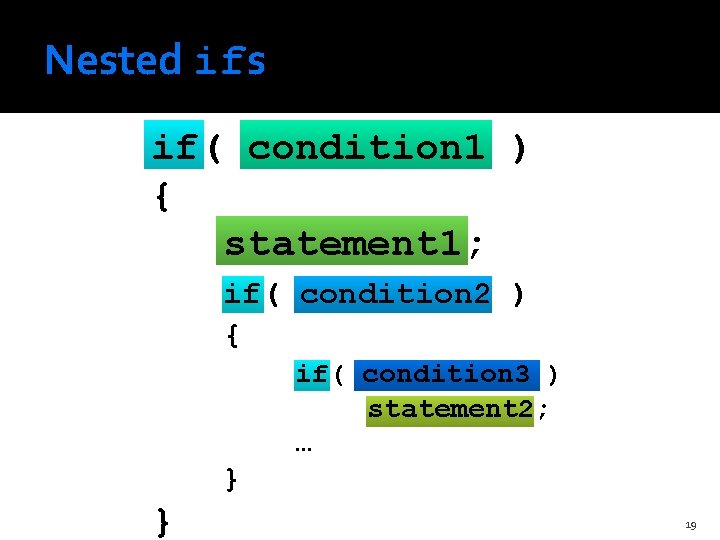
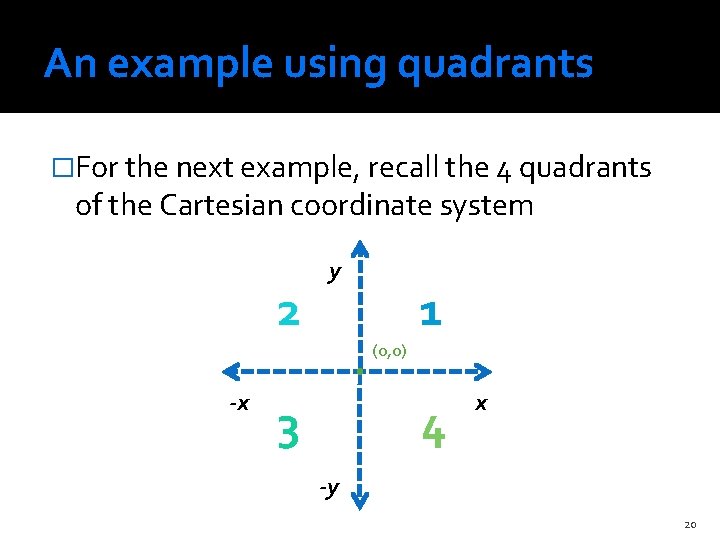
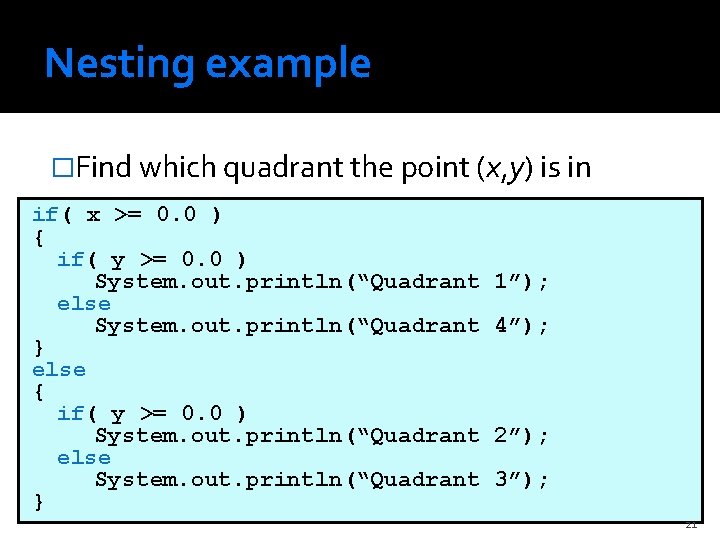
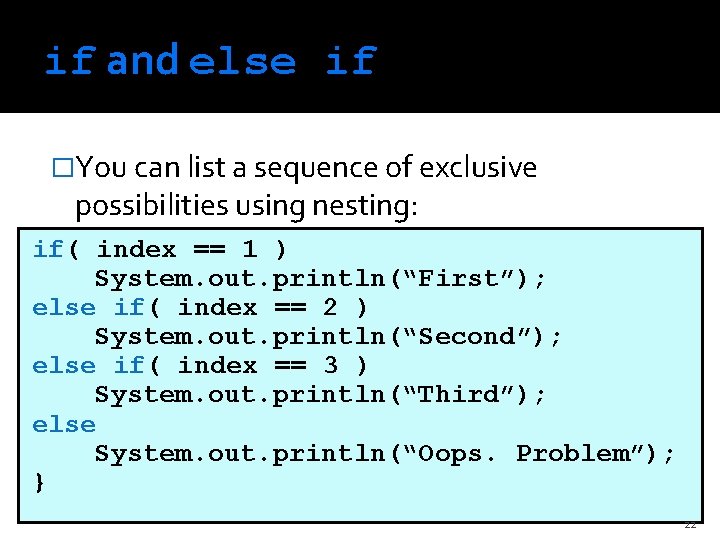
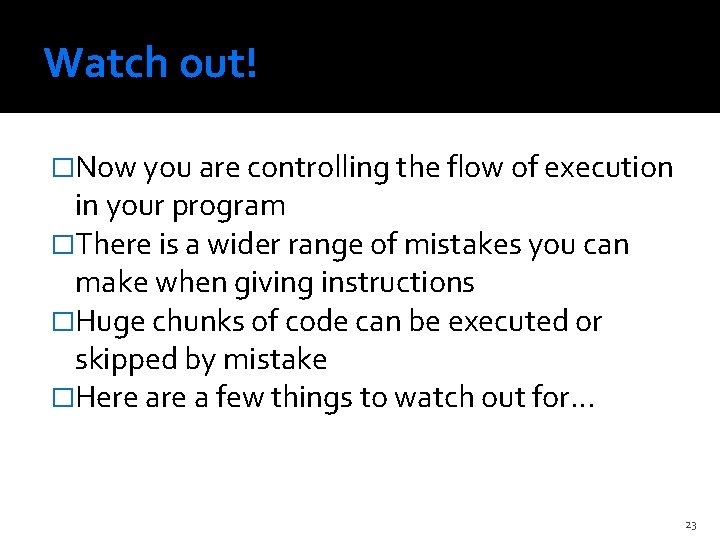
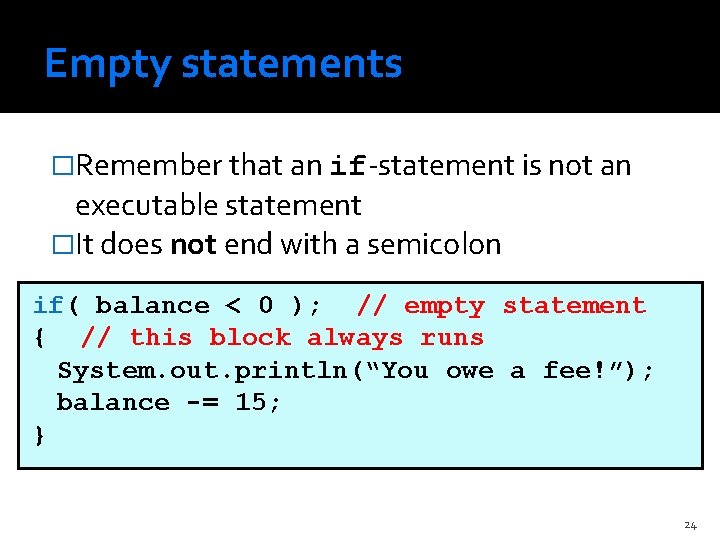
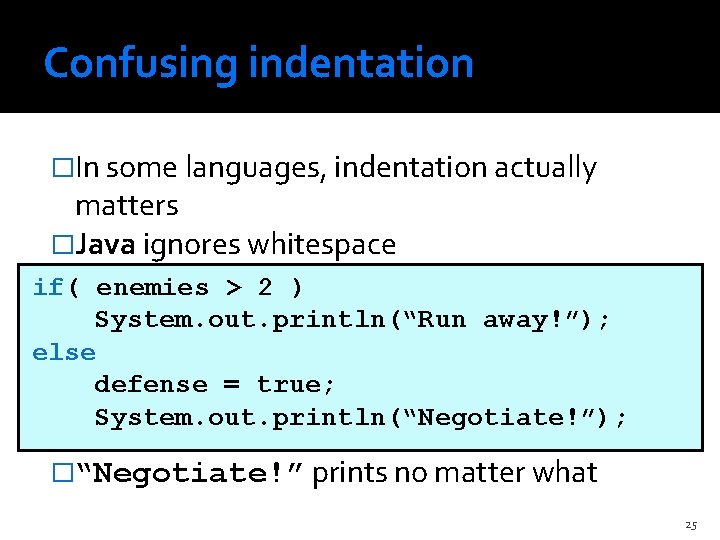
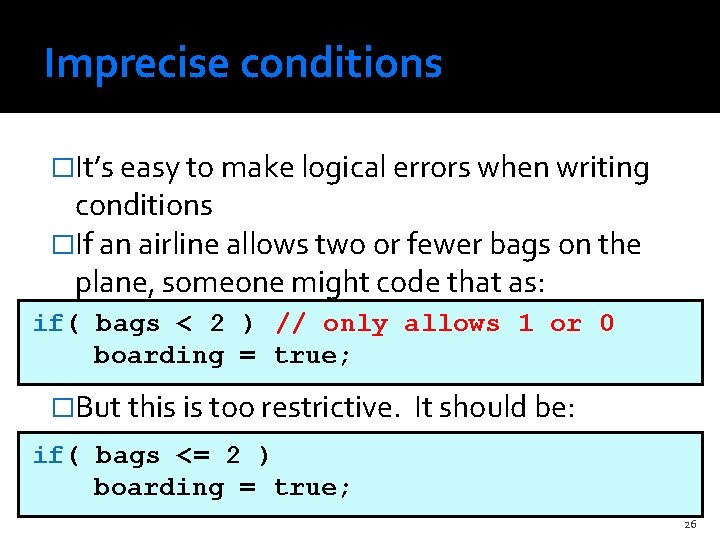
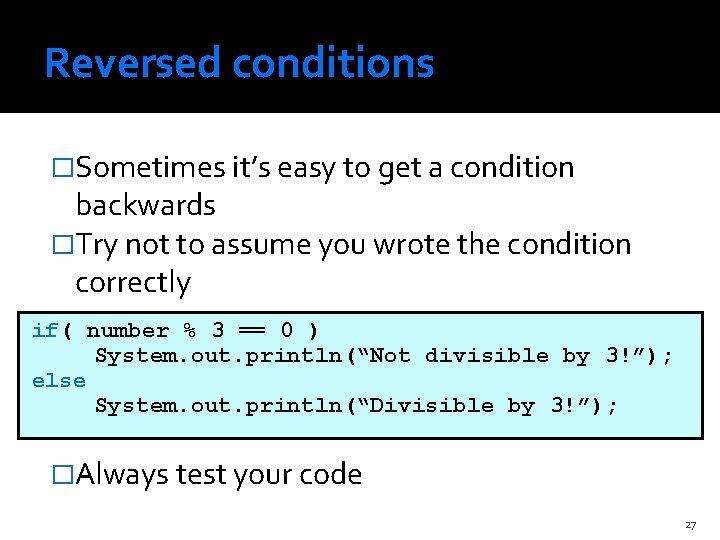
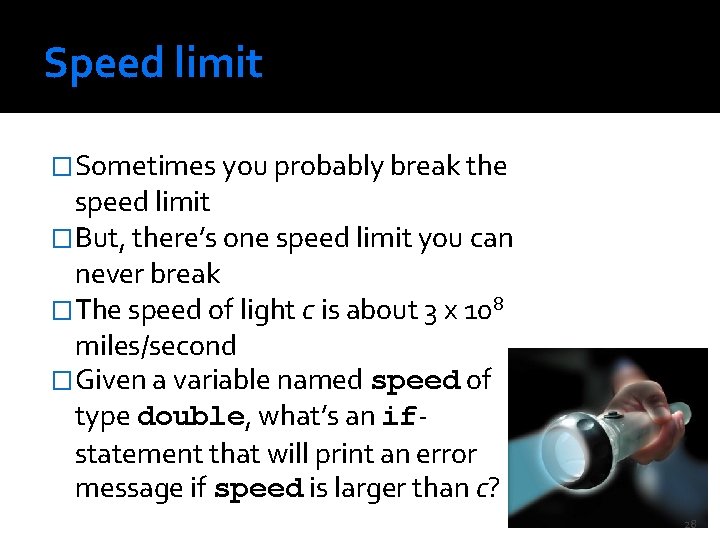
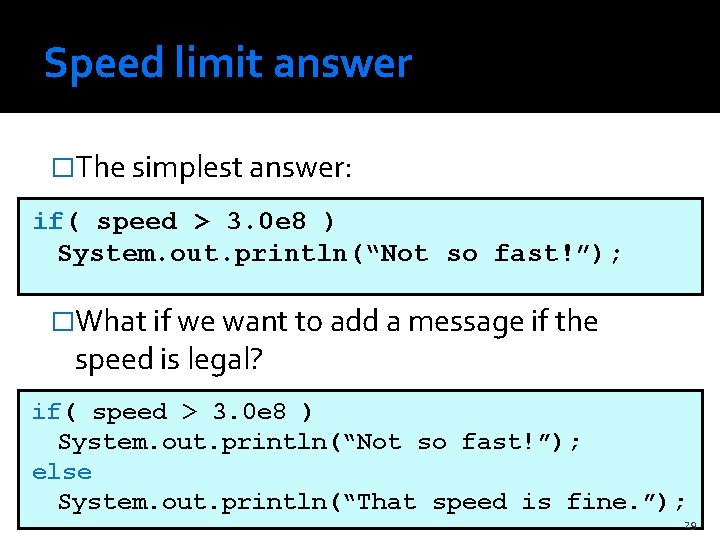
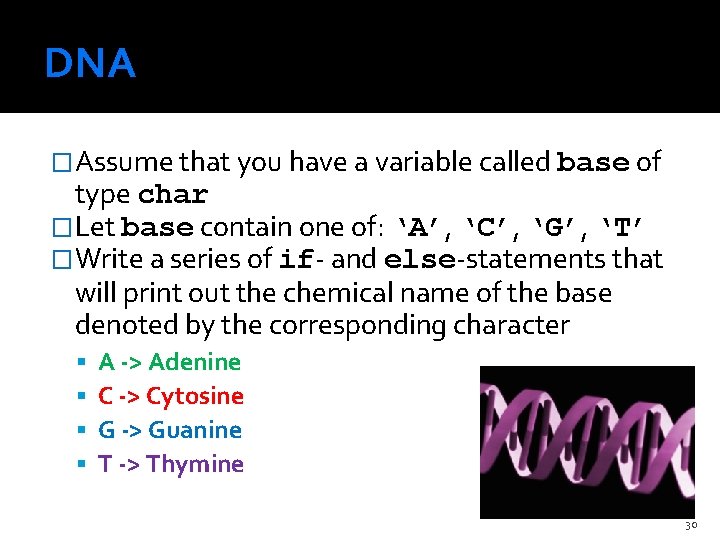
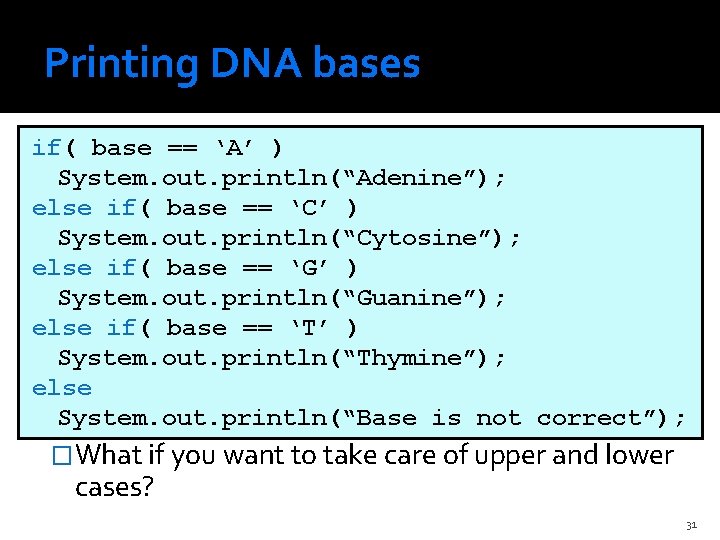
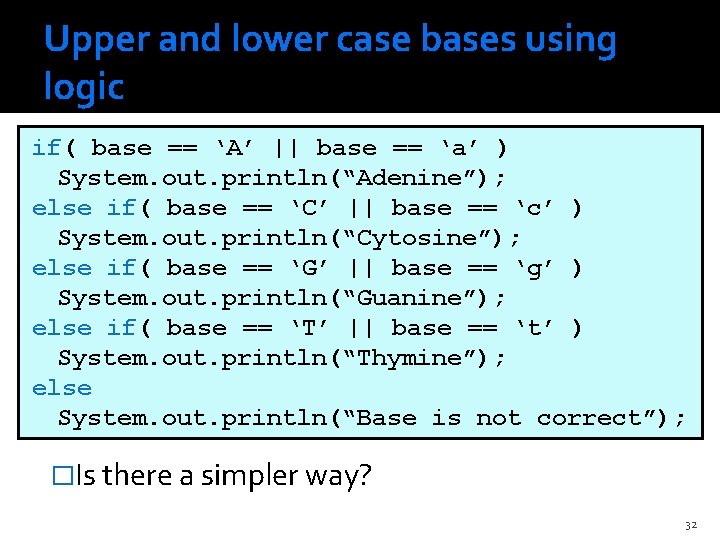
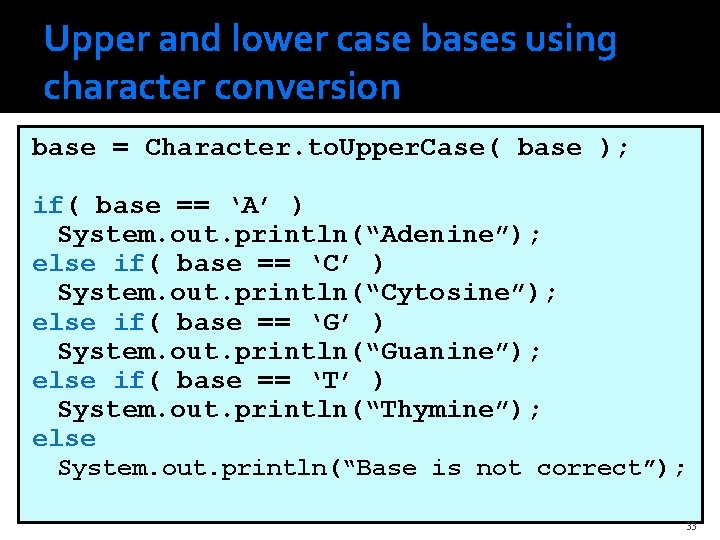
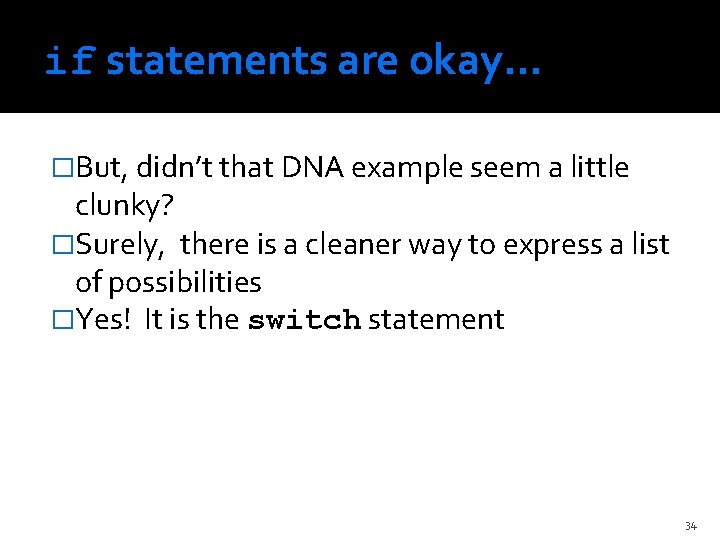
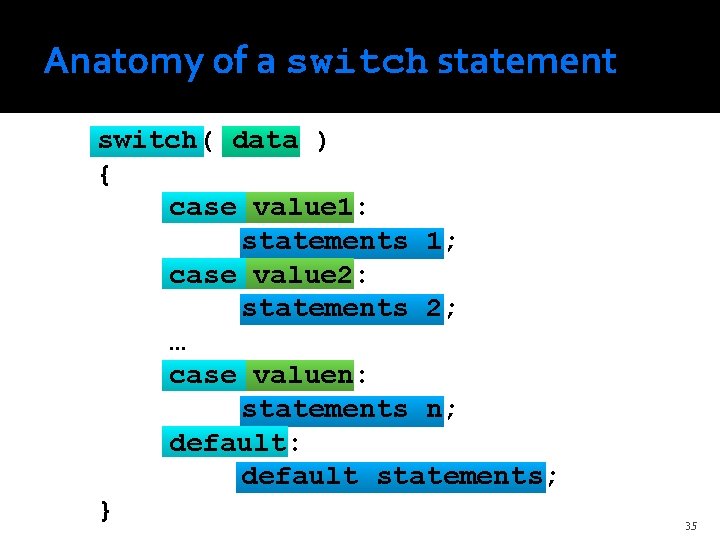
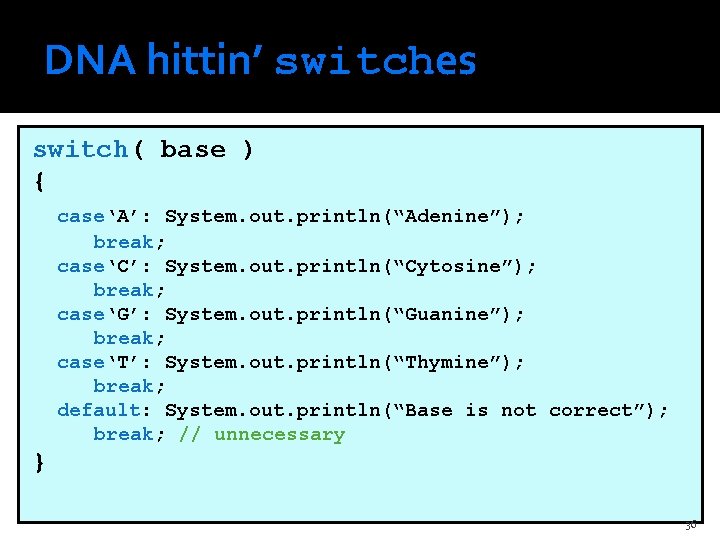
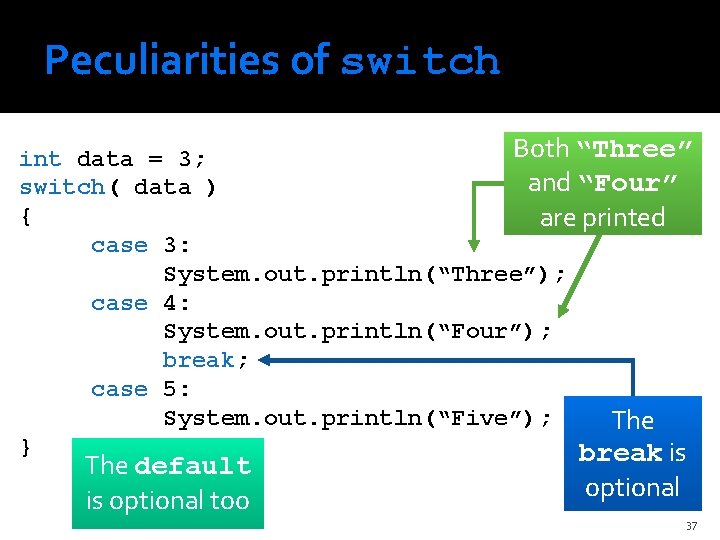
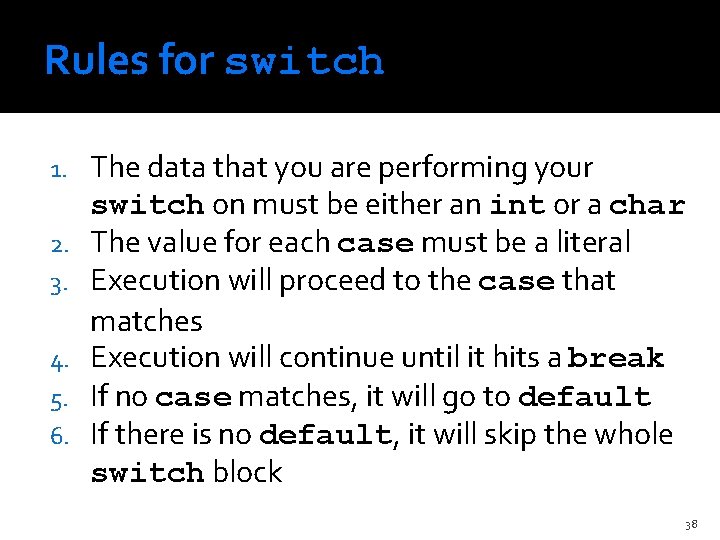
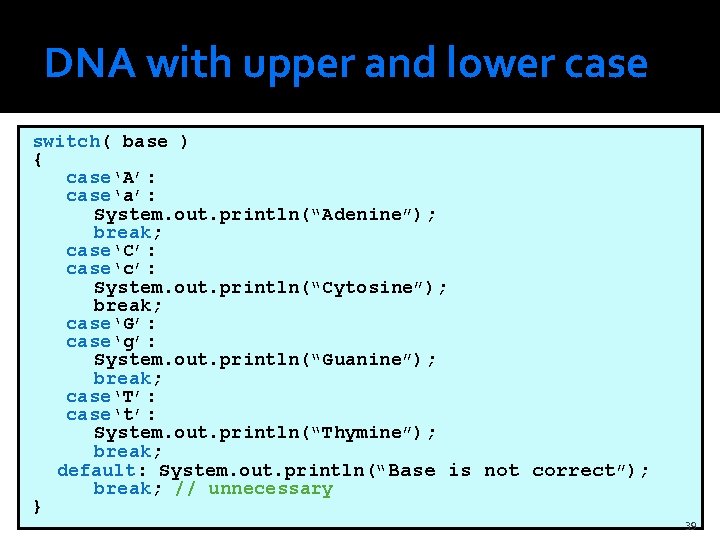
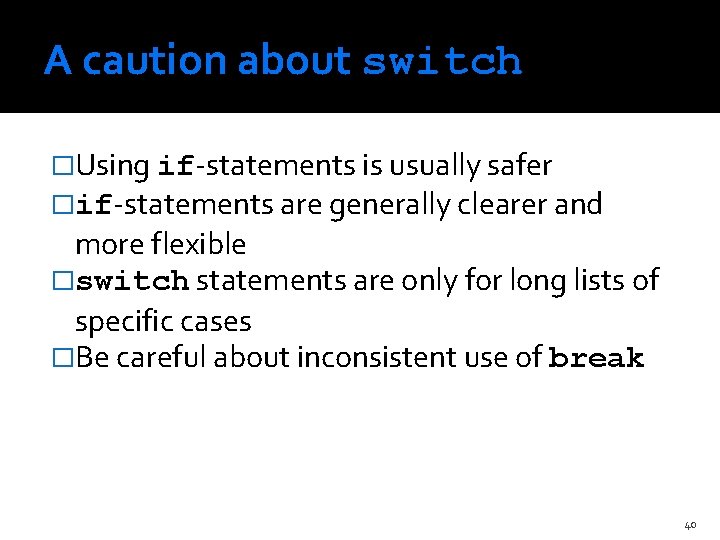
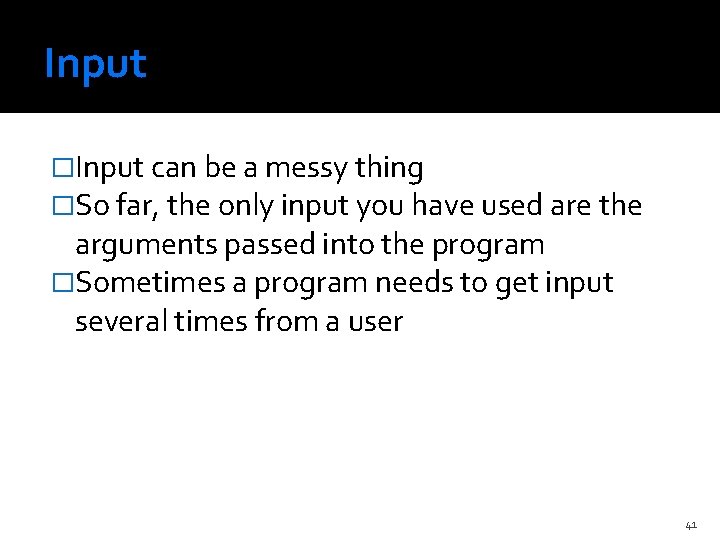
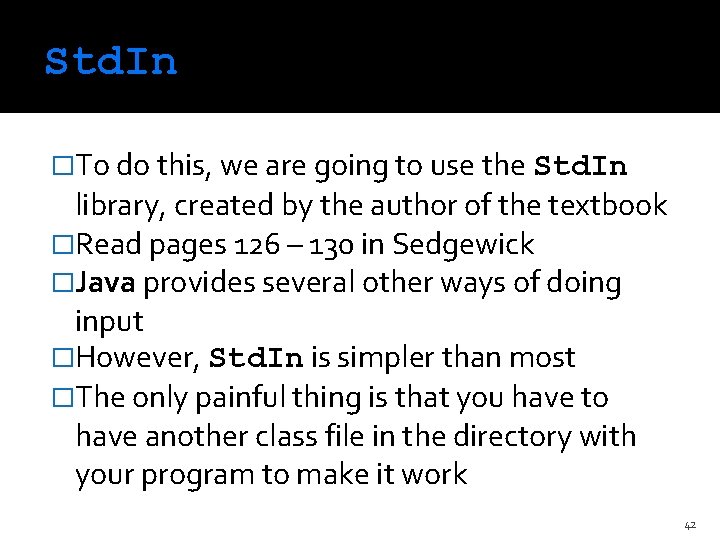
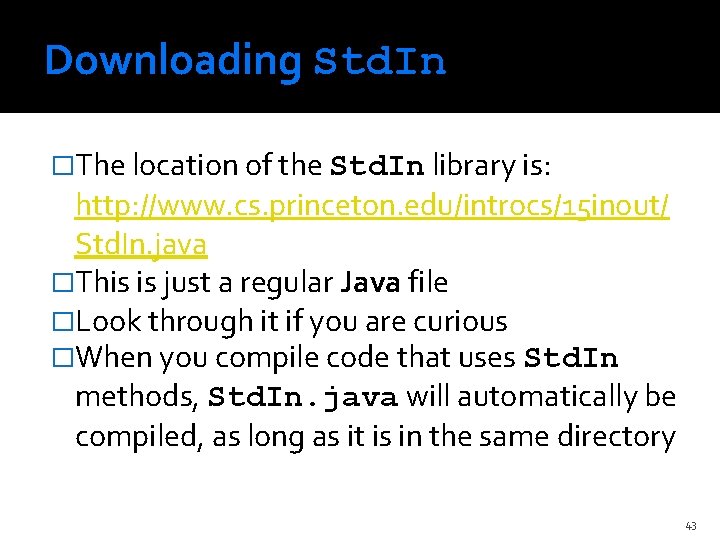
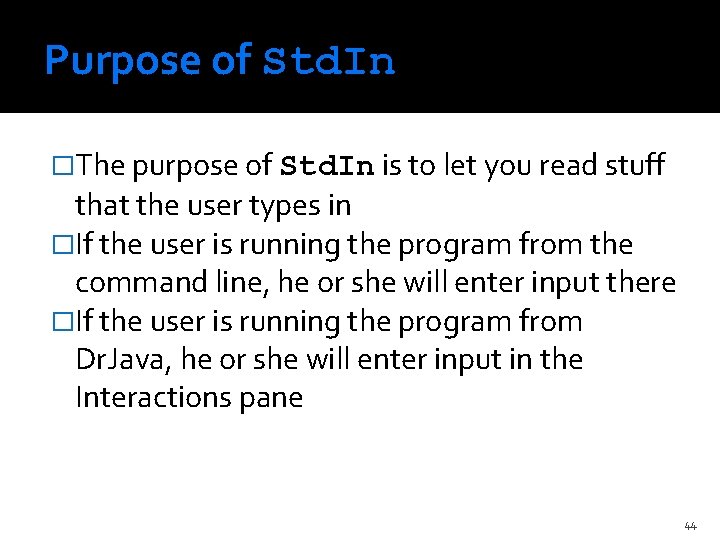
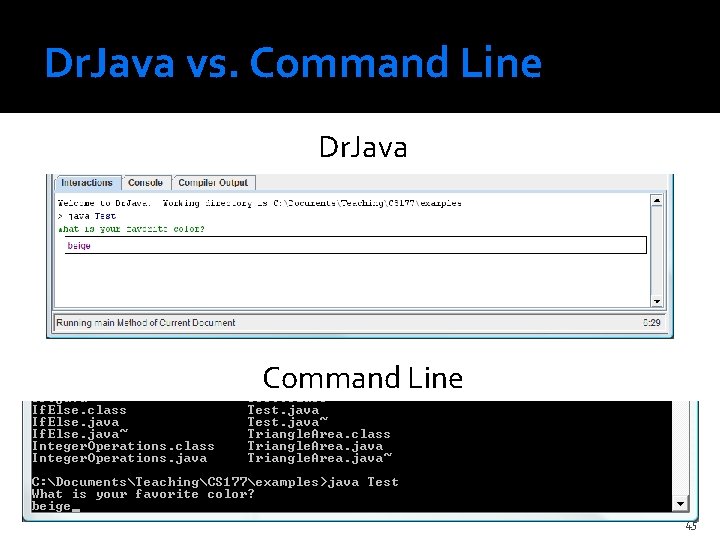
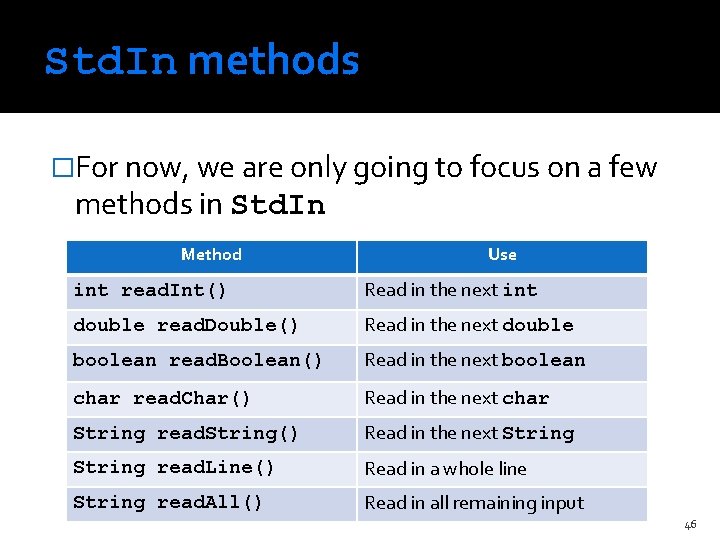
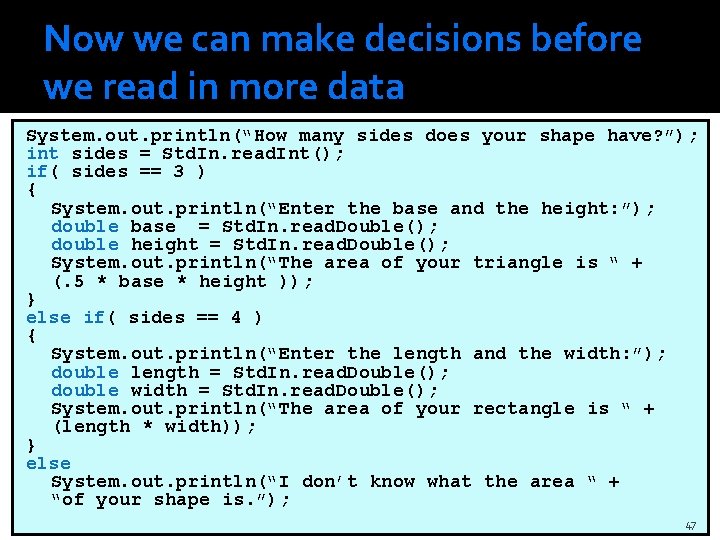
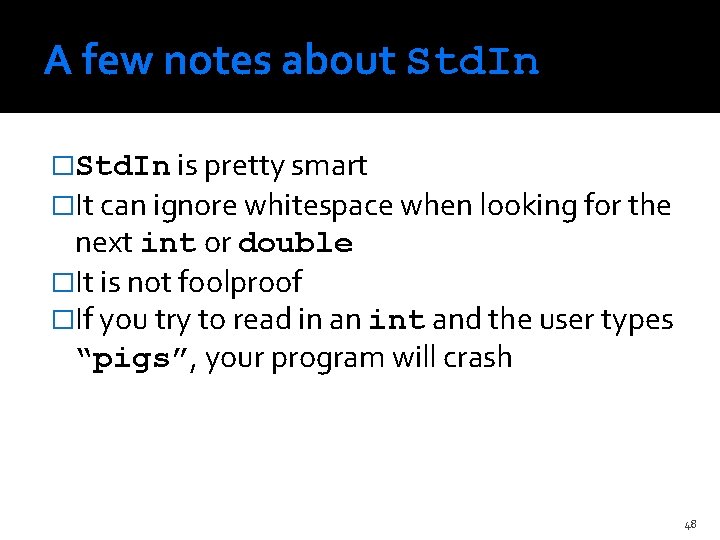
- Slides: 48
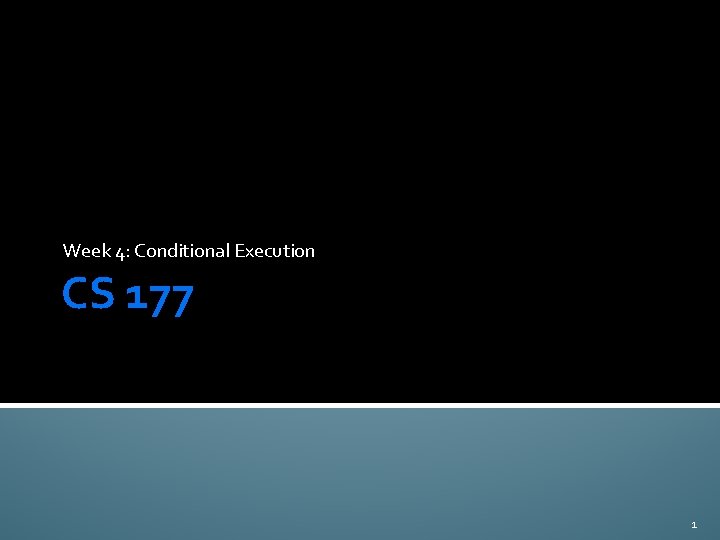
Week 4: Conditional Execution CS 177 1
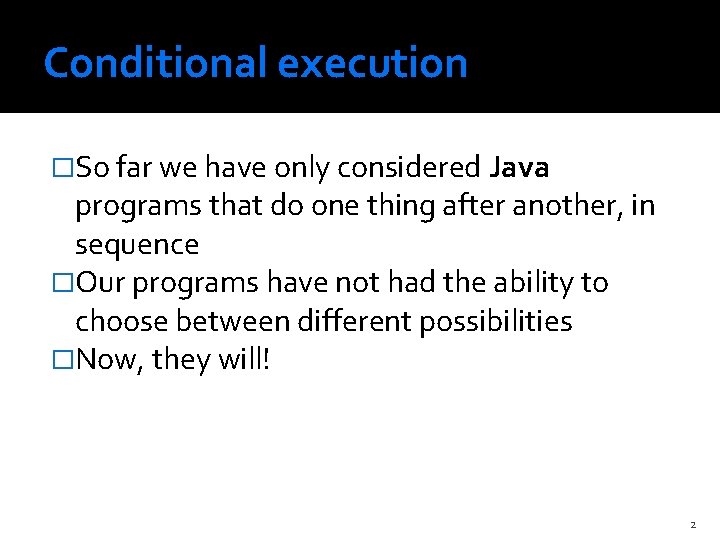
Conditional execution �So far we have only considered Java programs that do one thing after another, in sequence �Our programs have not had the ability to choose between different possibilities �Now, they will! 2
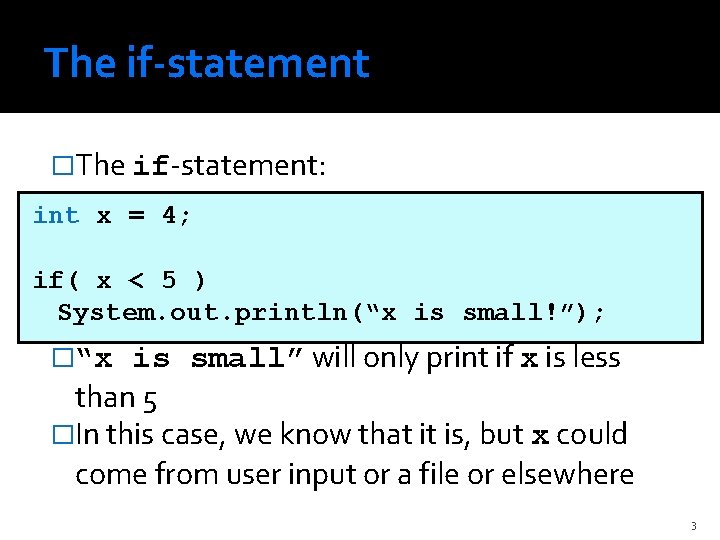
The if-statement �The if-statement: int x = 4; if( x < 5 ) System. out. println(“x is small!”); is small” will only print if x is less than 5 �In this case, we know that it is, but x could come from user input or a file or elsewhere �“x 3
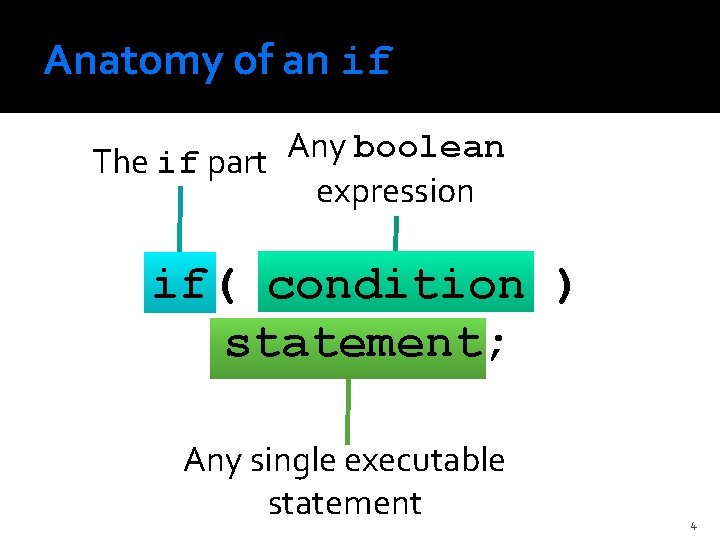
Anatomy of an if Any boolean The if part expression if( condition ) statement; Any single executable statement 4
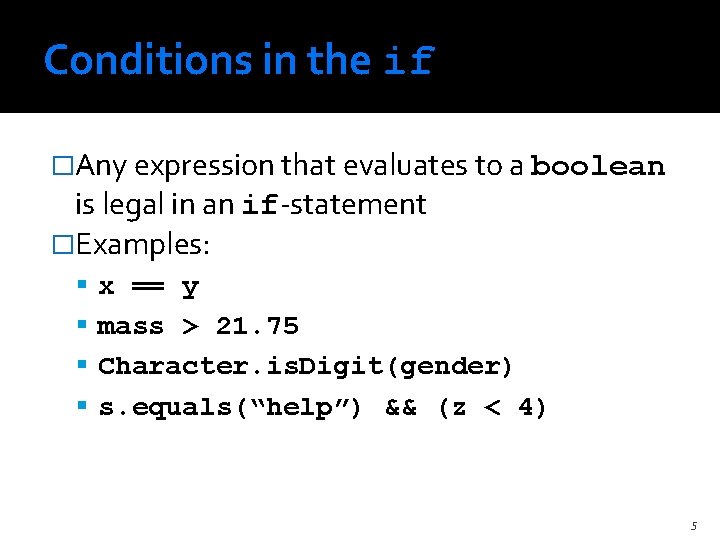
Conditions in the if �Any expression that evaluates to a boolean is legal in an if-statement �Examples: x == y mass > 21. 75 Character. is. Digit(gender) s. equals(“help”) && (z < 4) 5
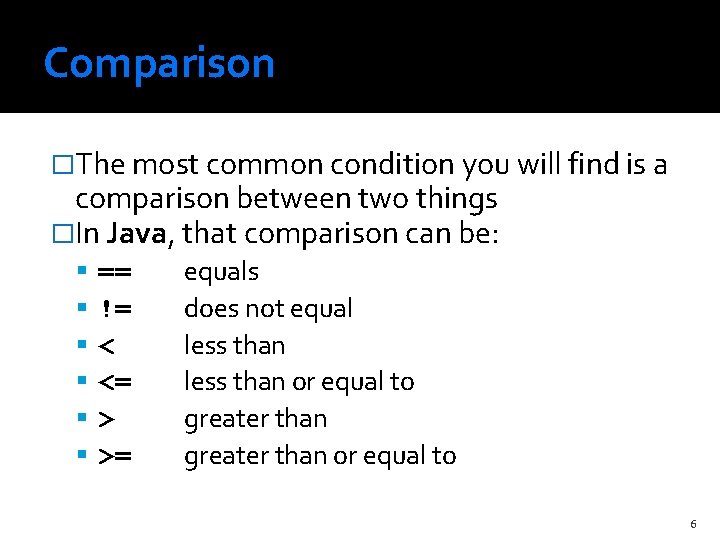
Comparison �The most common condition you will find is a comparison between two things �In Java, that comparison can be: == != < <= > >= equals does not equal less than or equal to greater than or equal to 6
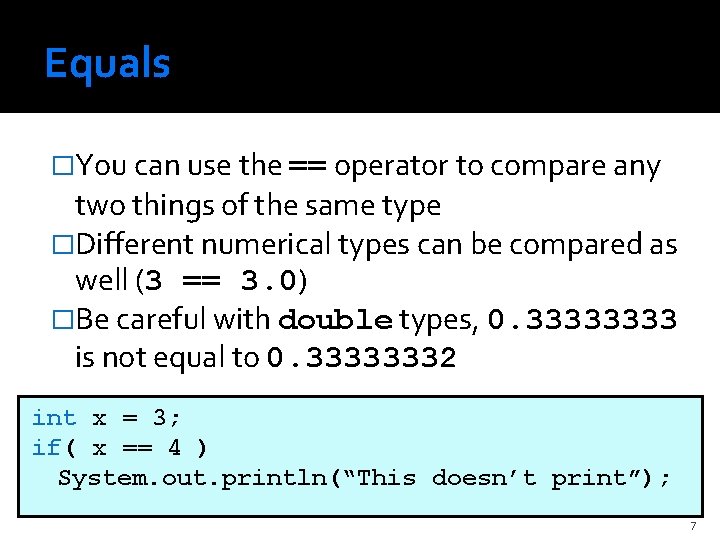
Equals �You can use the == operator to compare any two things of the same type �Different numerical types can be compared as well (3 == 3. 0) �Be careful with double types, 0. 3333 is not equal to 0. 33333332 int x = 3; if( x == 4 ) System. out. println(“This doesn’t print”); 7
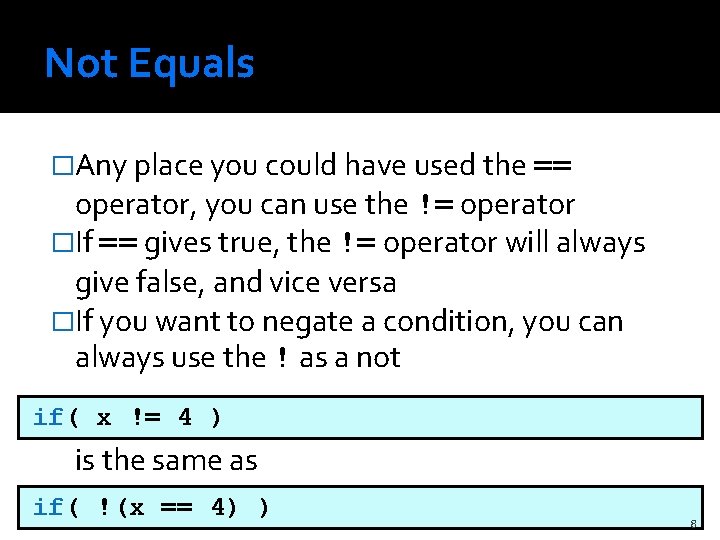
Not Equals �Any place you could have used the == operator, you can use the != operator �If == gives true, the != operator will always give false, and vice versa �If you want to negate a condition, you can always use the ! as a not if( x != 4 ) is the same as if( !(x == 4) ) 8
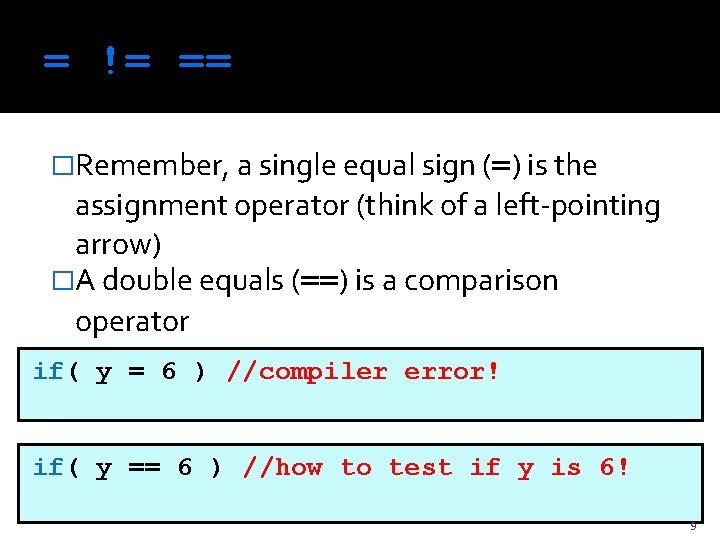
= != == �Remember, a single equal sign (=) is the assignment operator (think of a left-pointing arrow) �A double equals (==) is a comparison operator if( y = 6 ) //compiler error! if( y == 6 ) //how to test if y is 6! 9
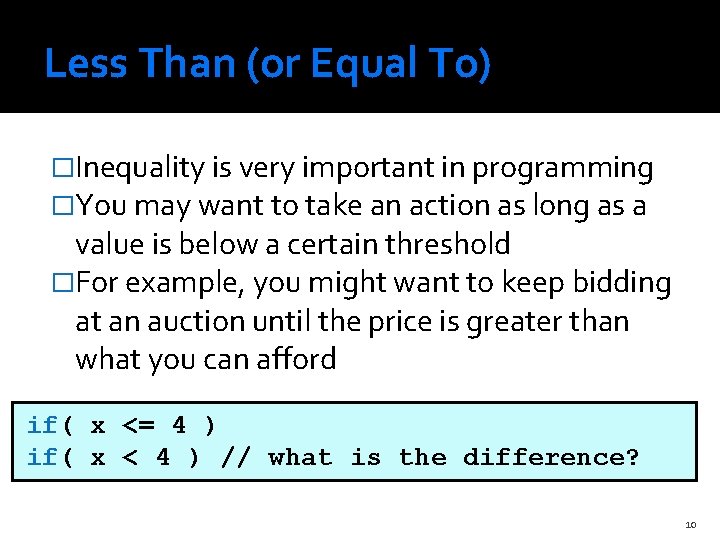
Less Than (or Equal To) �Inequality is very important in programming �You may want to take an action as long as a value is below a certain threshold �For example, you might want to keep bidding at an auction until the price is greater than what you can afford if( x <= 4 ) if( x < 4 ) // what is the difference? 10
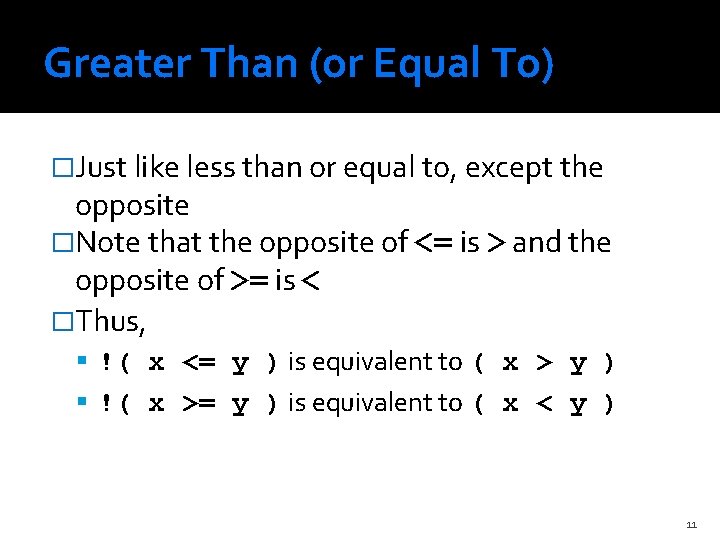
Greater Than (or Equal To) �Just like less than or equal to, except the opposite �Note that the opposite of <= is > and the opposite of >= is < �Thus, !( x <= y ) is equivalent to ( x > y ) !( x >= y ) is equivalent to ( x < y ) 11
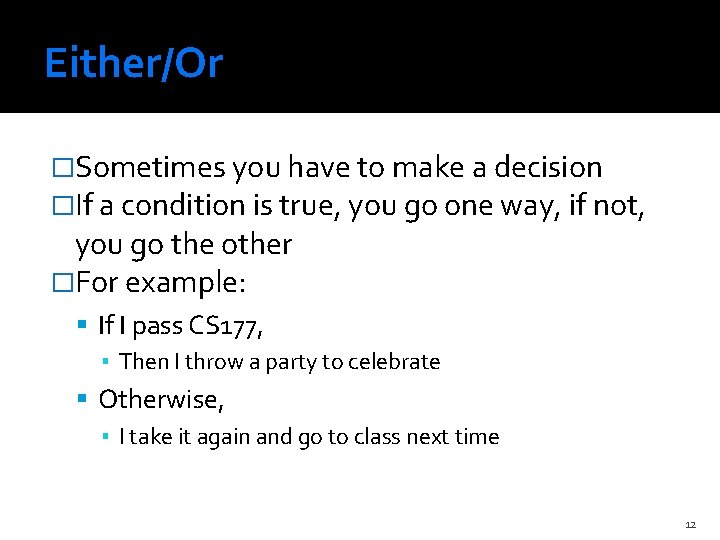
Either/Or �Sometimes you have to make a decision �If a condition is true, you go one way, if not, you go the other �For example: If I pass CS 177, ▪ Then I throw a party to celebrate Otherwise, ▪ I take it again and go to class next time 12
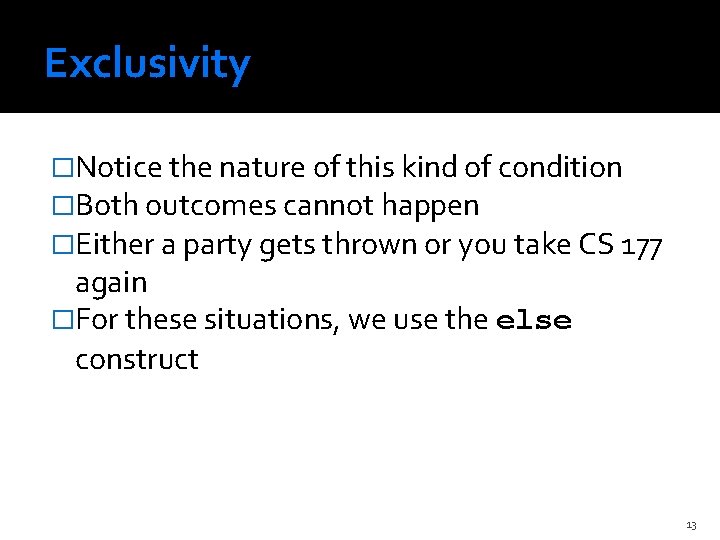
Exclusivity �Notice the nature of this kind of condition �Both outcomes cannot happen �Either a party gets thrown or you take CS 177 again �For these situations, we use the else construct 13
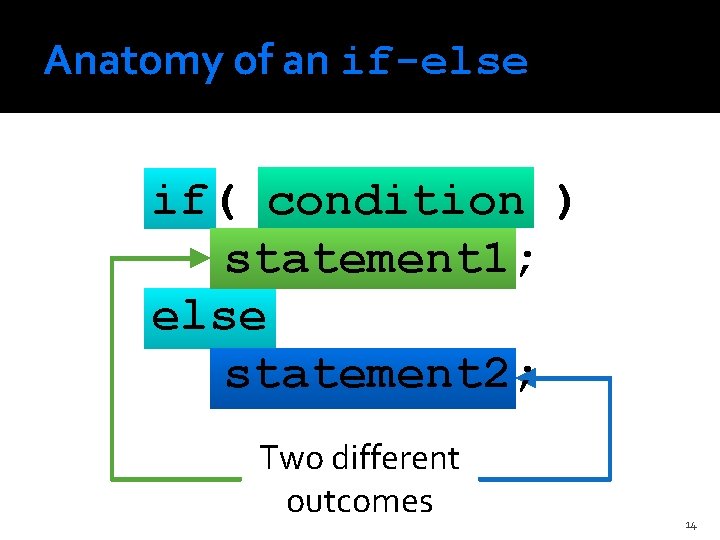
Anatomy of an if-else if( condition ) statement 1; else statement 2; Two different outcomes 14
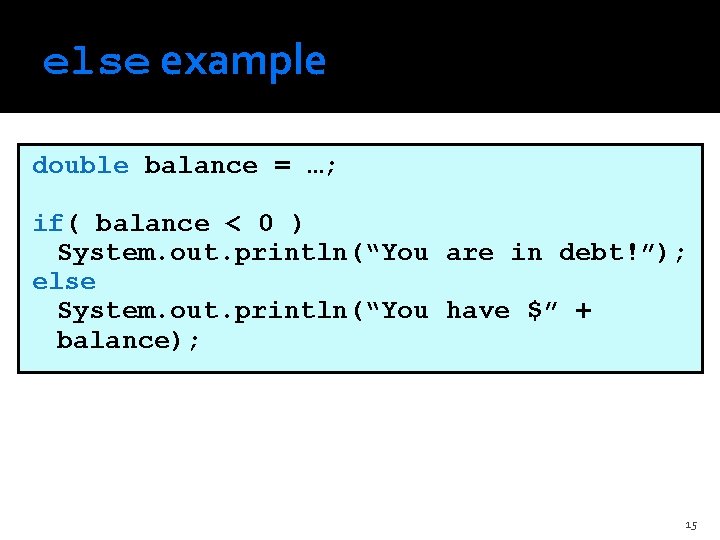
else example double balance = …; if( balance < 0 ) System. out. println(“You are in debt!”); else System. out. println(“You have $” + balance); 15
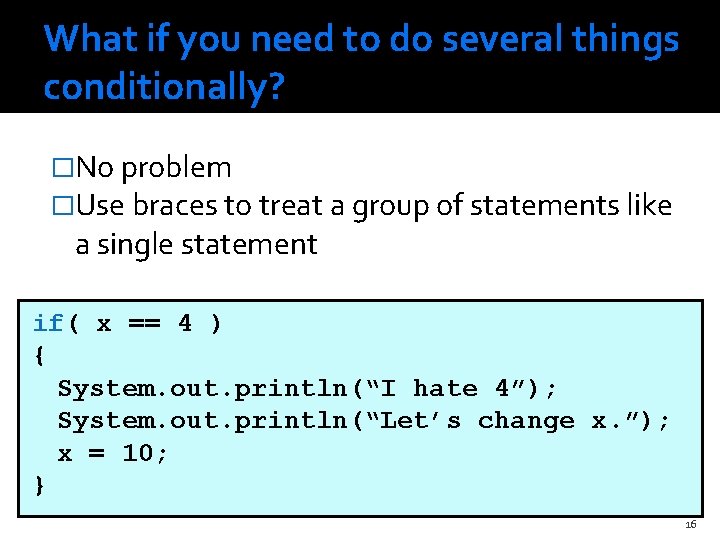
What if you need to do several things conditionally? �No problem �Use braces to treat a group of statements like a single statement if( x == 4 ) { System. out. println(“I hate 4”); System. out. println(“Let’s change x. ”); x = 10; } 16
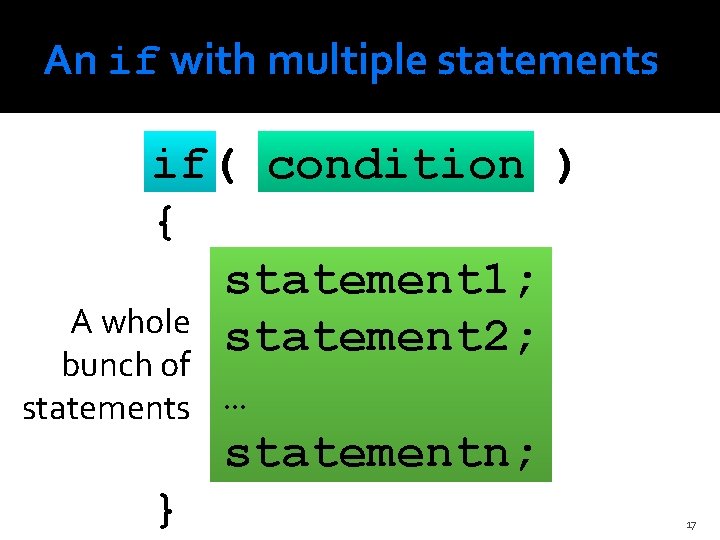
An if with multiple statements if( condition ) { statement 1; A whole statement 2; bunch of statements … statementn; } 17
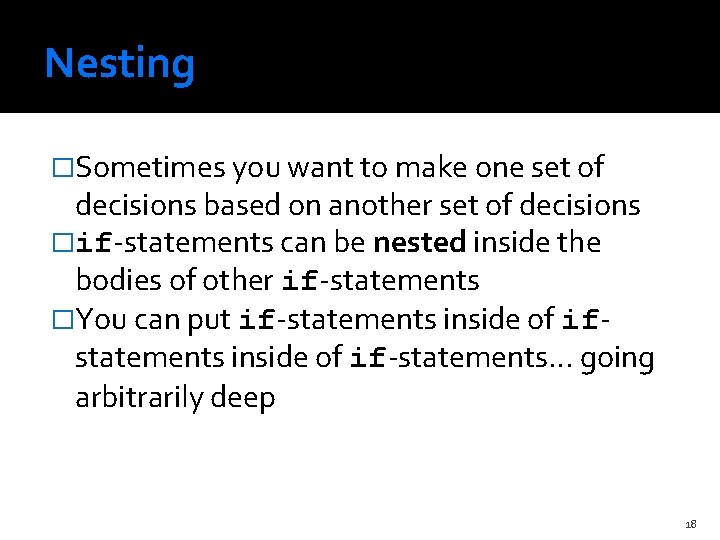
Nesting �Sometimes you want to make one set of decisions based on another set of decisions �if-statements can be nested inside the bodies of other if-statements �You can put if-statements inside of if-statements… going arbitrarily deep 18
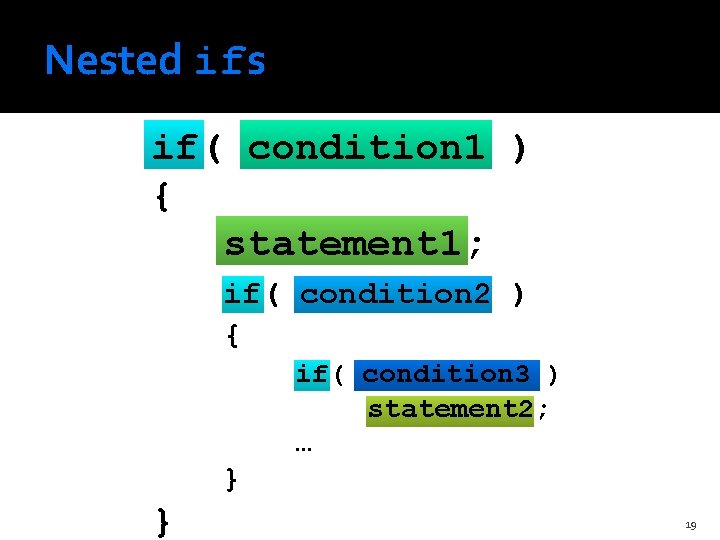
Nested ifs if( condition 1 ) { statement 1; if( condition 2 ) { if( condition 3 ) statement 2; … } } 19
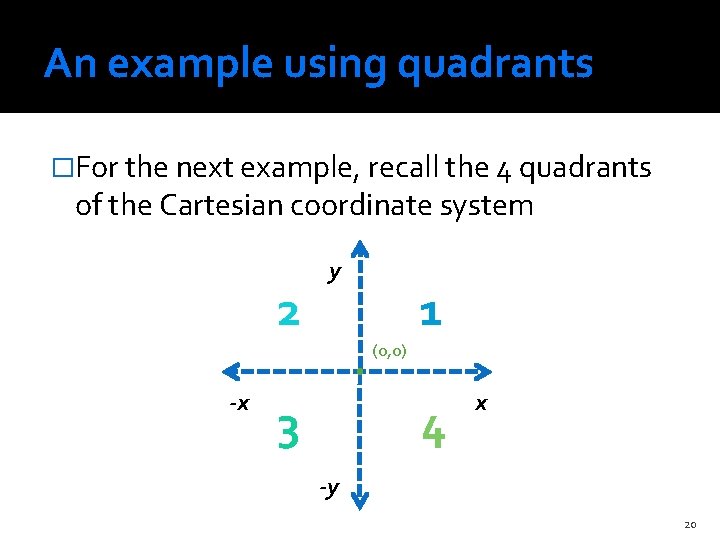
An example using quadrants �For the next example, recall the 4 quadrants of the Cartesian coordinate system 2 y 1 (0, 0) -x 3 4 x -y 20
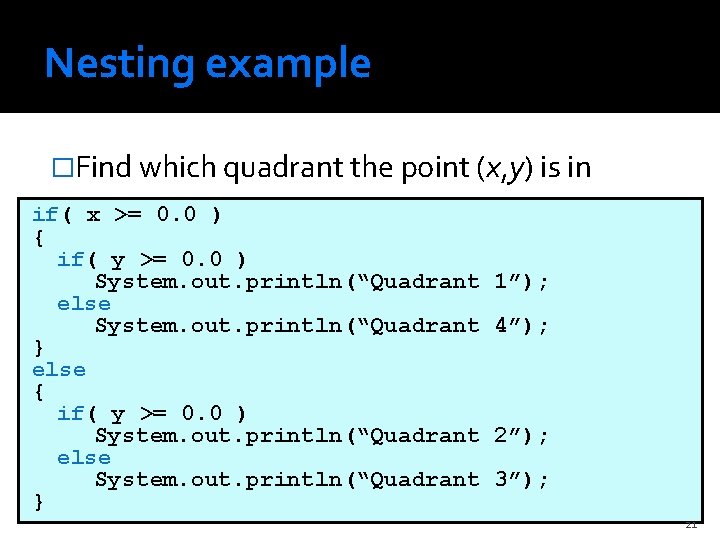
Nesting example �Find which quadrant the point (x, y) is in if( x >= 0. 0 ) { if( y >= 0. 0 ) System. out. println(“Quadrant else System. out. println(“Quadrant } else { if( y >= 0. 0 ) System. out. println(“Quadrant else System. out. println(“Quadrant } 1”); 4”); 2”); 3”); 21
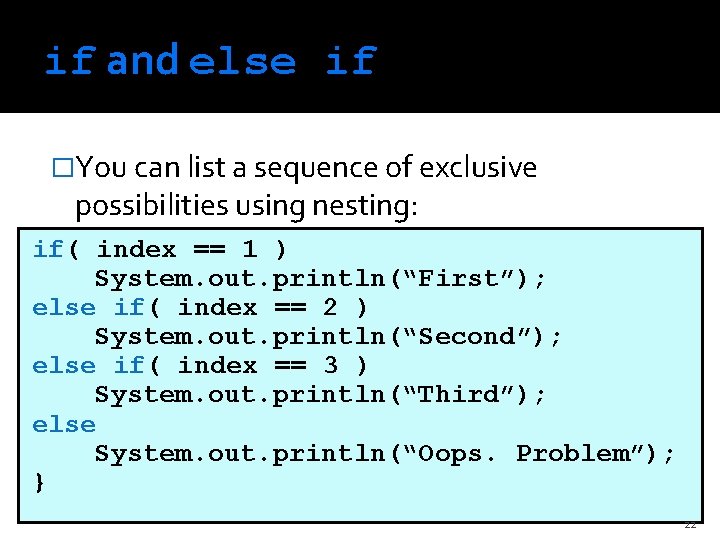
if and else if �You can list a sequence of exclusive possibilities using nesting: if( index == 1 ) System. out. println(“First”); else if( index == 2 ) System. out. println(“Second”); else if( index == 3 ) System. out. println(“Third”); else System. out. println(“Oops. Problem”); } 22
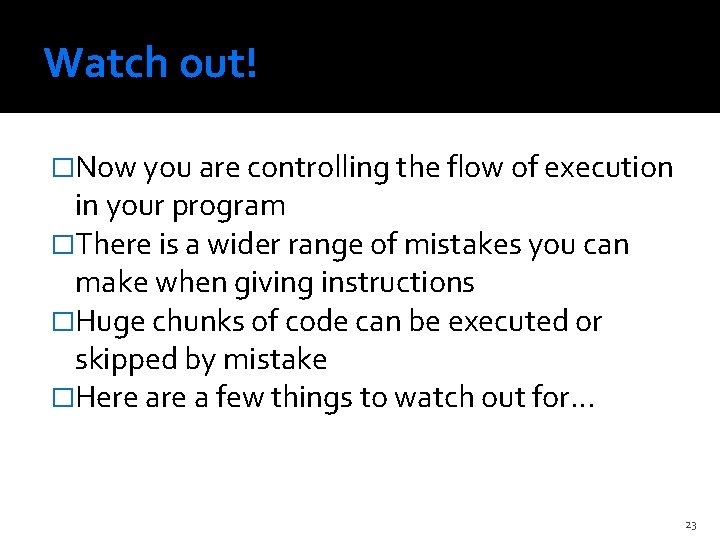
Watch out! �Now you are controlling the flow of execution in your program �There is a wider range of mistakes you can make when giving instructions �Huge chunks of code can be executed or skipped by mistake �Here a few things to watch out for… 23
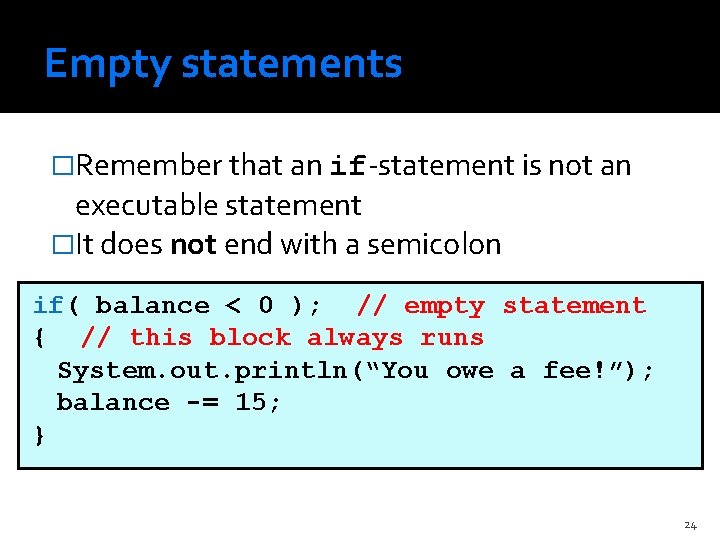
Empty statements �Remember that an if-statement is not an executable statement �It does not end with a semicolon if( balance < 0 ); // empty statement { // this block always runs System. out. println(“You owe a fee!”); balance -= 15; } 24
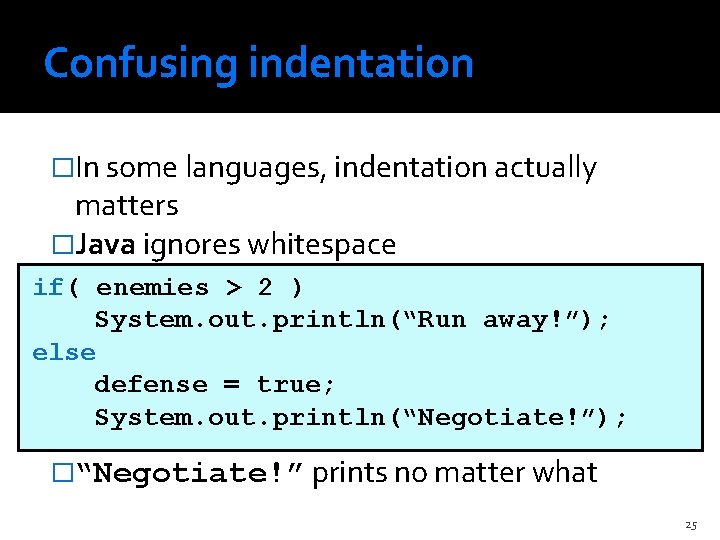
Confusing indentation �In some languages, indentation actually matters �Java ignores whitespace if( enemies > 2 ) System. out. println(“Run away!”); else defense = true; System. out. println(“Negotiate!”); �“Negotiate!” prints no matter what 25
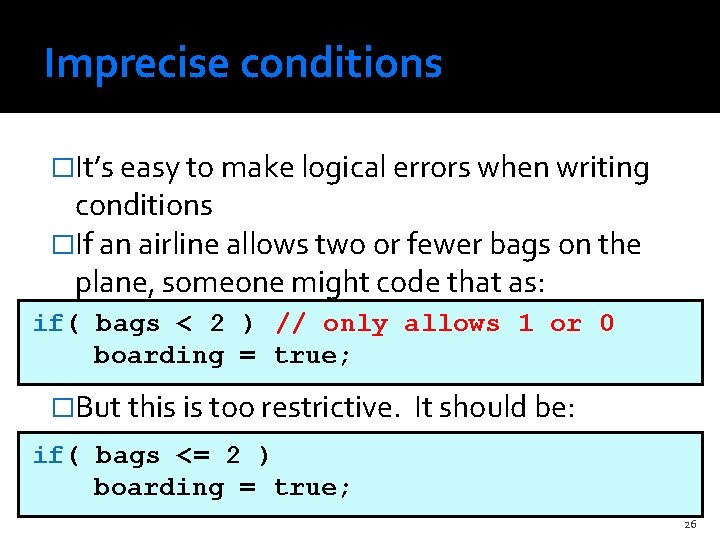
Imprecise conditions �It’s easy to make logical errors when writing conditions �If an airline allows two or fewer bags on the plane, someone might code that as: if( bags < 2 ) // only allows 1 or 0 boarding = true; �But this is too restrictive. It should be: if( bags <= 2 ) boarding = true; 26
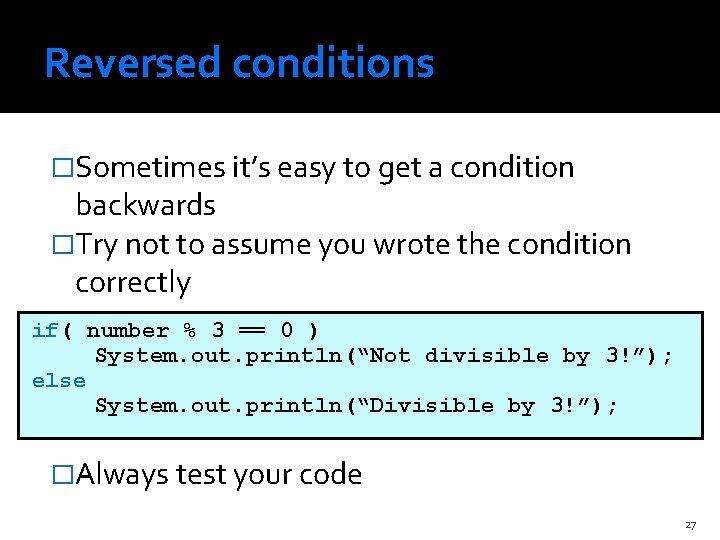
Reversed conditions �Sometimes it’s easy to get a condition backwards �Try not to assume you wrote the condition correctly if( number % 3 == 0 ) System. out. println(“Not divisible by 3!”); else System. out. println(“Divisible by 3!”); �Always test your code 27
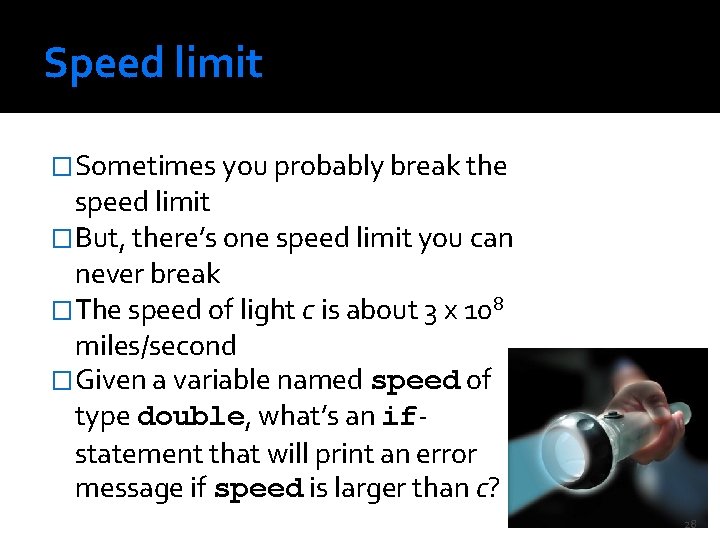
Speed limit �Sometimes you probably break the speed limit �But, there’s one speed limit you can never break �The speed of light c is about 3 x 108 miles/second �Given a variable named speed of type double, what’s an ifstatement that will print an error message if speed is larger than c? 28
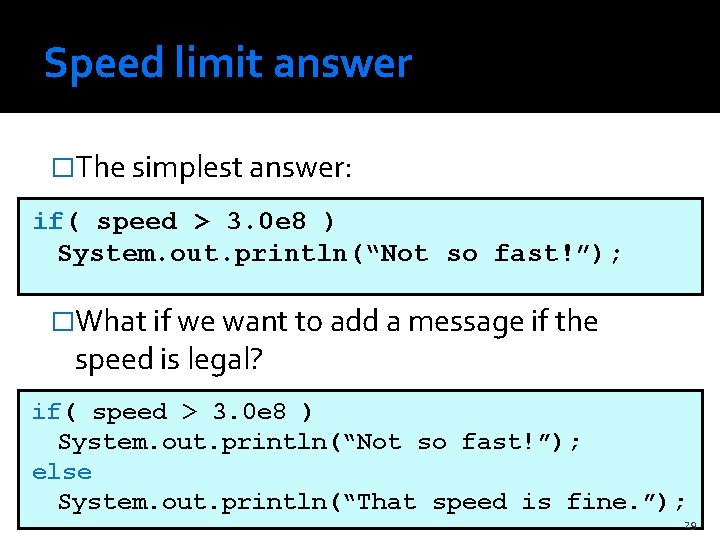
Speed limit answer �The simplest answer: if( speed > 3. 0 e 8 ) System. out. println(“Not so fast!”); �What if we want to add a message if the speed is legal? if( speed > 3. 0 e 8 ) System. out. println(“Not so fast!”); else System. out. println(“That speed is fine. ”); 29
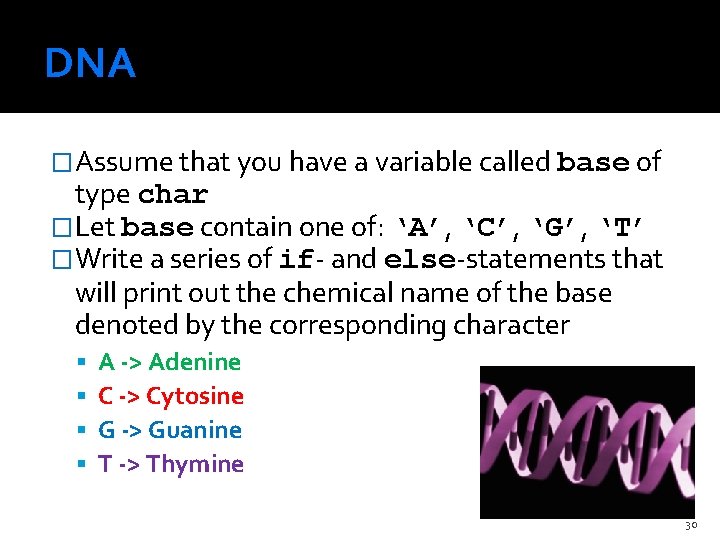
DNA �Assume that you have a variable called base of type char �Let base contain one of: ‘A’, ‘C’, ‘G’, ‘T’ �Write a series of if- and else-statements that will print out the chemical name of the base denoted by the corresponding character A -> Adenine C -> Cytosine G -> Guanine T -> Thymine 30
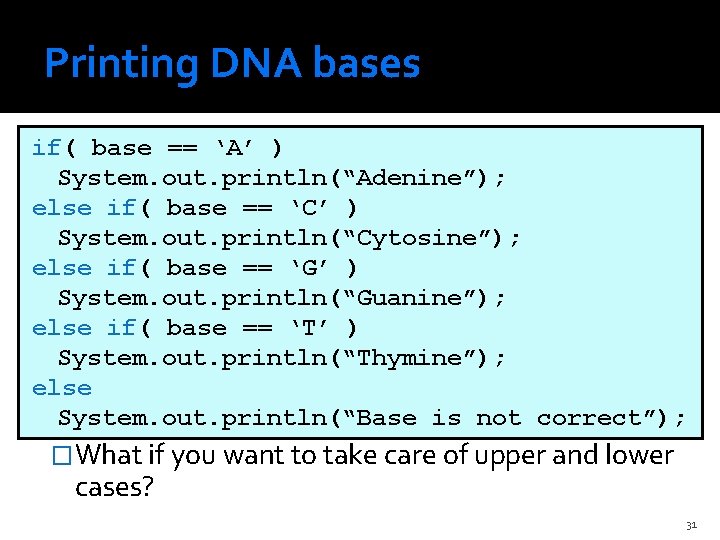
Printing DNA bases if( base == ‘A’ ) System. out. println(“Adenine”); else if( base == ‘C’ ) System. out. println(“Cytosine”); else if( base == ‘G’ ) System. out. println(“Guanine”); else if( base == ‘T’ ) System. out. println(“Thymine”); else System. out. println(“Base is not correct”); �What if you want to take care of upper and lower cases? 31
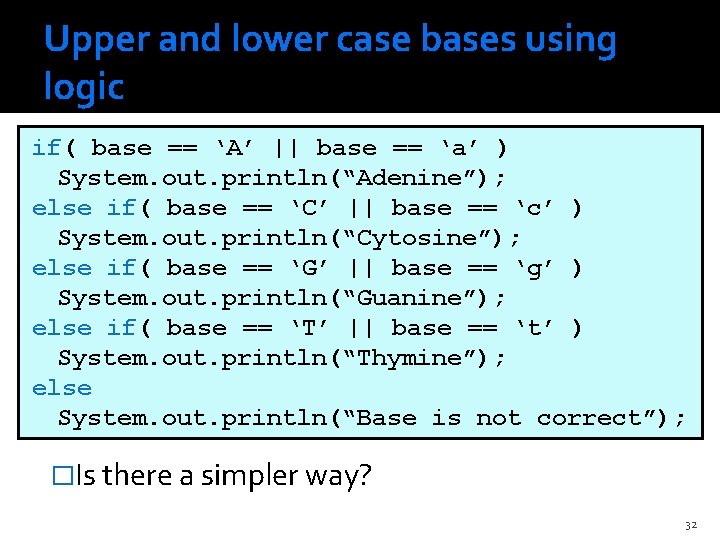
Upper and lower case bases using logic if( base == ‘A’ || base == ‘a’ ) System. out. println(“Adenine”); else if( base == ‘C’ || base == ‘c’ ) System. out. println(“Cytosine”); else if( base == ‘G’ || base == ‘g’ ) System. out. println(“Guanine”); else if( base == ‘T’ || base == ‘t’ ) System. out. println(“Thymine”); else System. out. println(“Base is not correct”); �Is there a simpler way? 32
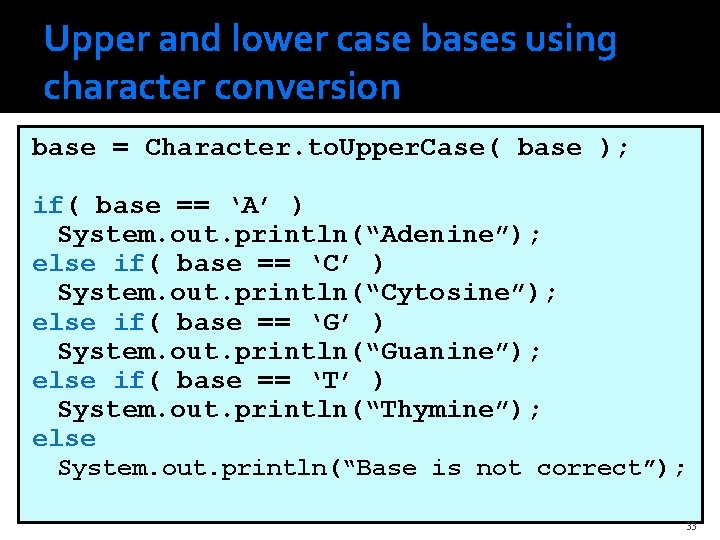
Upper and lower case bases using character conversion base = Character. to. Upper. Case( base ); if( base == ‘A’ ) System. out. println(“Adenine”); else if( base == ‘C’ ) System. out. println(“Cytosine”); else if( base == ‘G’ ) System. out. println(“Guanine”); else if( base == ‘T’ ) System. out. println(“Thymine”); else System. out. println(“Base is not correct”); 33
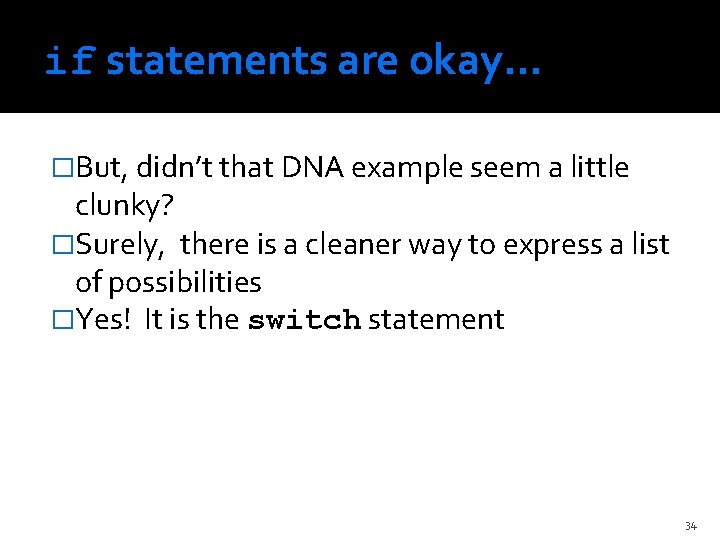
if statements are okay… �But, didn’t that DNA example seem a little clunky? �Surely, there is a cleaner way to express a list of possibilities �Yes! It is the switch statement 34
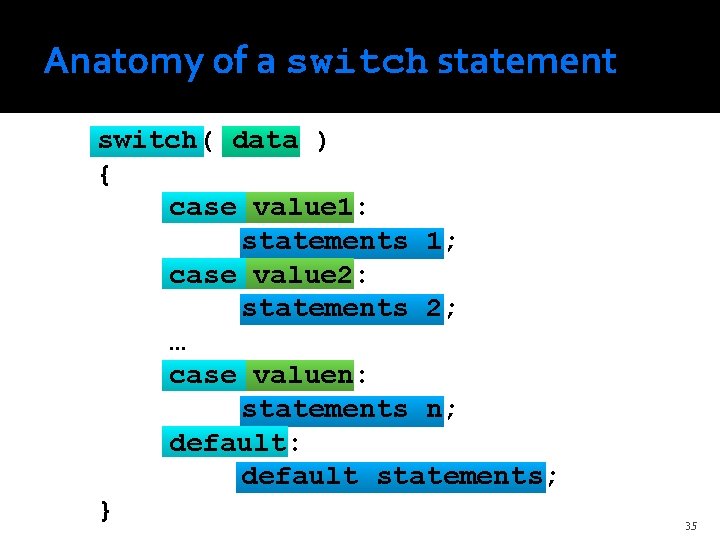
Anatomy of a switch statement switch( data ) { case value 1: statements 1; case value 2: statements 2; … case valuen: statements n; default: default statements; } 35
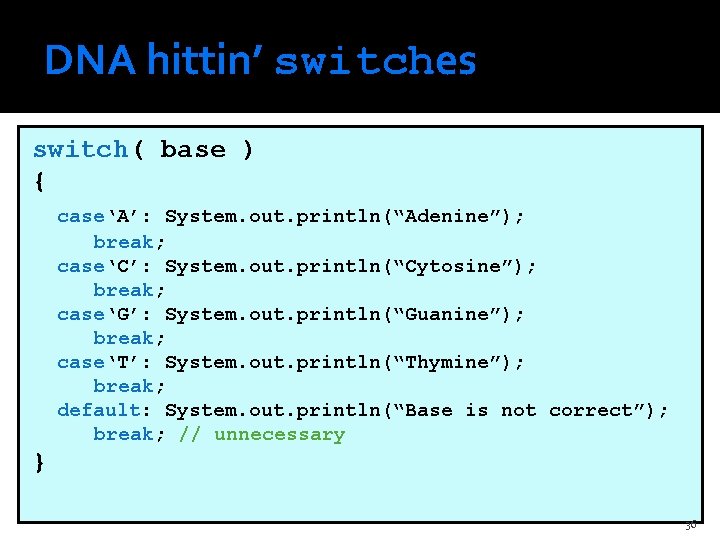
DNA hittin’ switches switch( base ) { case‘A’: System. out. println(“Adenine”); break; case‘C’: System. out. println(“Cytosine”); break; case‘G’: System. out. println(“Guanine”); break; case‘T’: System. out. println(“Thymine”); break; default: System. out. println(“Base is not correct”); break; // unnecessary } 36
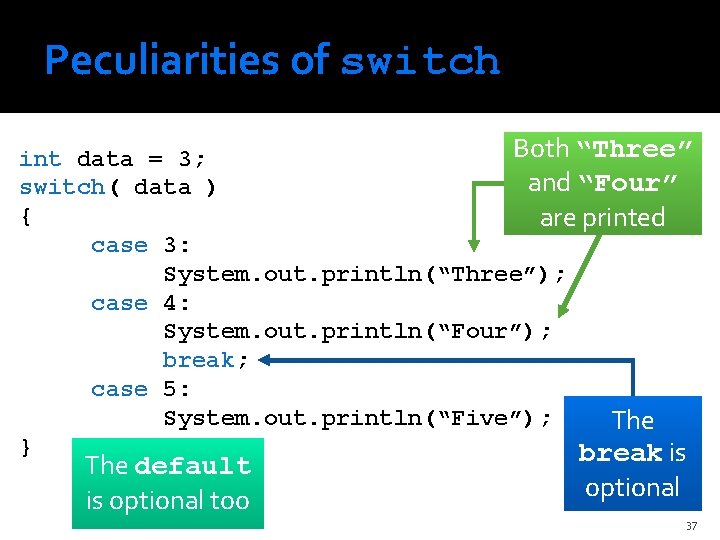
Peculiarities of switch Both “Three” int data = 3; and “Four” switch( data ) { are printed case 3: System. out. println(“Three”); case 4: System. out. println(“Four”); break; case 5: System. out. println(“Five”); The } break is The default is optional too optional 37
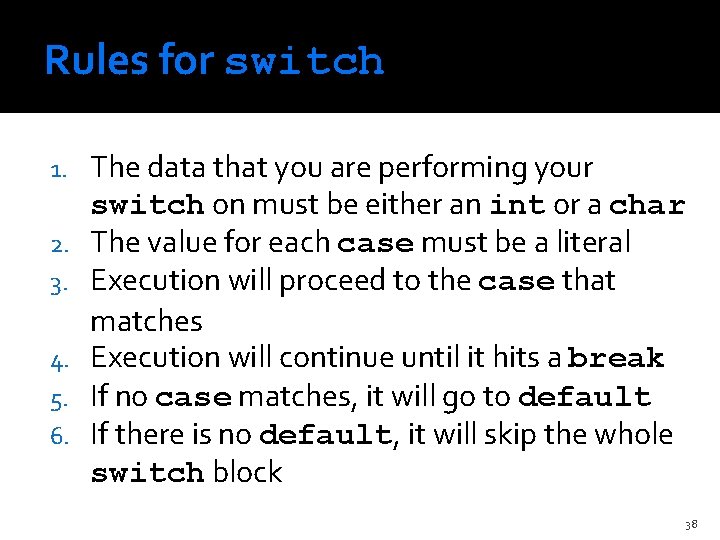
Rules for switch 1. 2. 3. 4. 5. 6. The data that you are performing your switch on must be either an int or a char The value for each case must be a literal Execution will proceed to the case that matches Execution will continue until it hits a break If no case matches, it will go to default If there is no default, it will skip the whole switch block 38
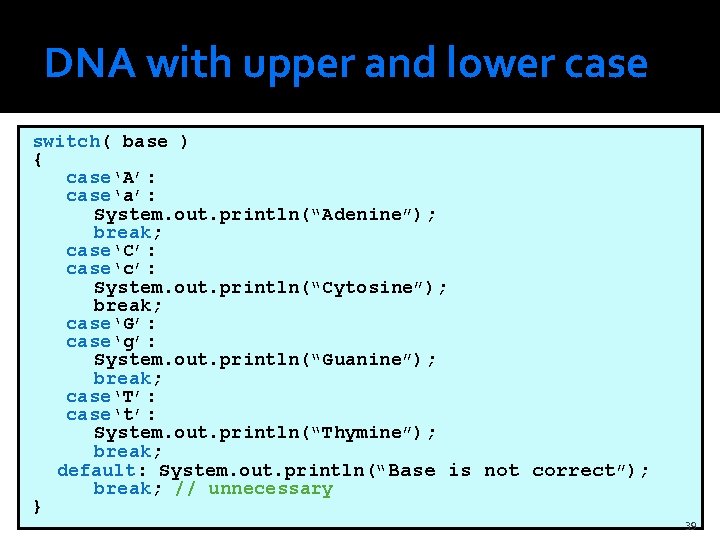
DNA with upper and lower case switch( base ) { case‘A’: case‘a’: System. out. println(“Adenine”); break; case‘C’: case‘c’: System. out. println(“Cytosine”); break; case‘G’: case‘g’: System. out. println(“Guanine”); break; case‘T’: case‘t’: System. out. println(“Thymine”); break; default: System. out. println(“Base is not correct”); break; // unnecessary } 39
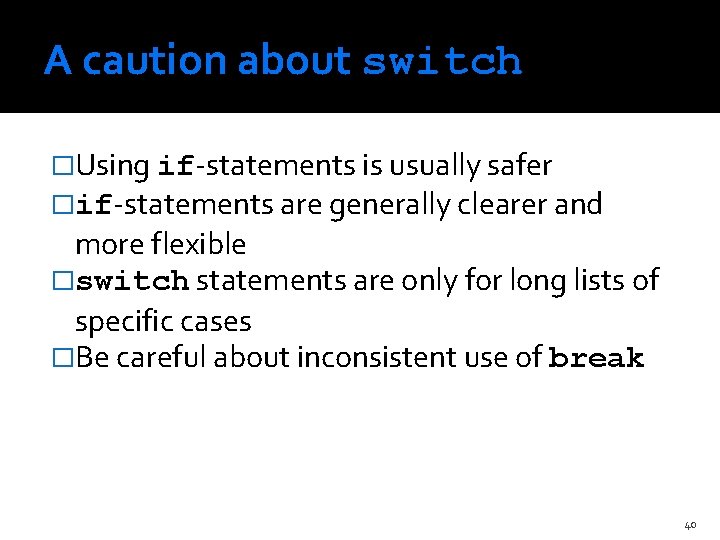
A caution about switch �Using if-statements is usually safer �if-statements are generally clearer and more flexible �switch statements are only for long lists of specific cases �Be careful about inconsistent use of break 40
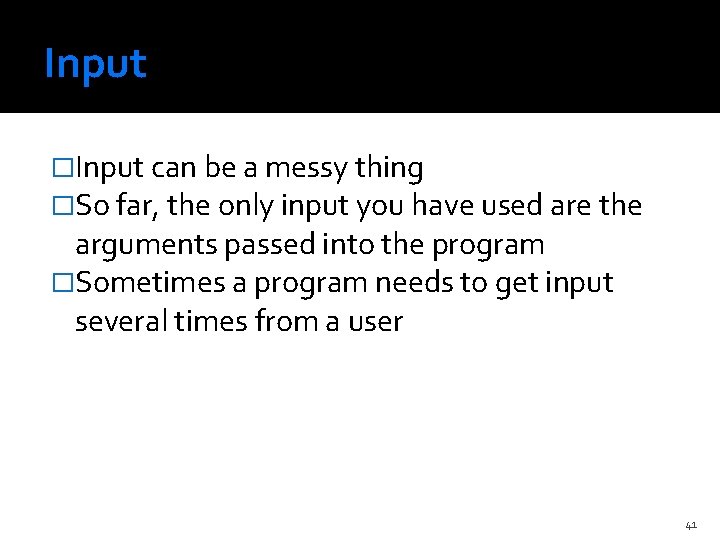
Input �Input can be a messy thing �So far, the only input you have used are the arguments passed into the program �Sometimes a program needs to get input several times from a user 41
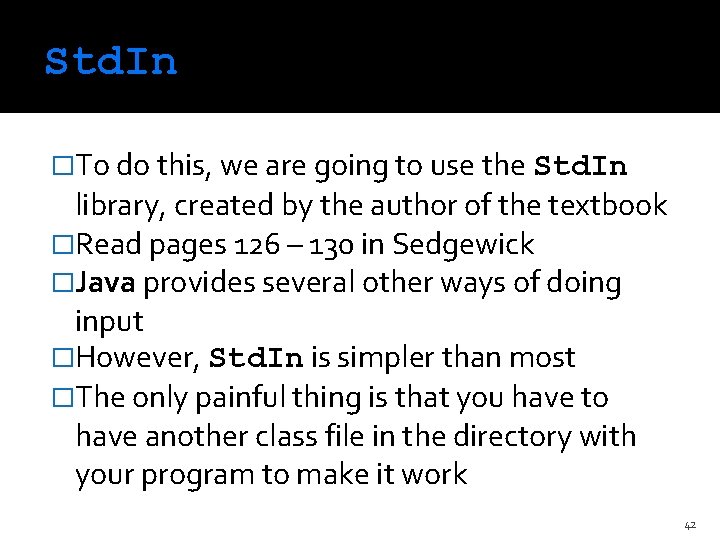
Std. In �To do this, we are going to use the Std. In library, created by the author of the textbook �Read pages 126 – 130 in Sedgewick �Java provides several other ways of doing input �However, Std. In is simpler than most �The only painful thing is that you have to have another class file in the directory with your program to make it work 42
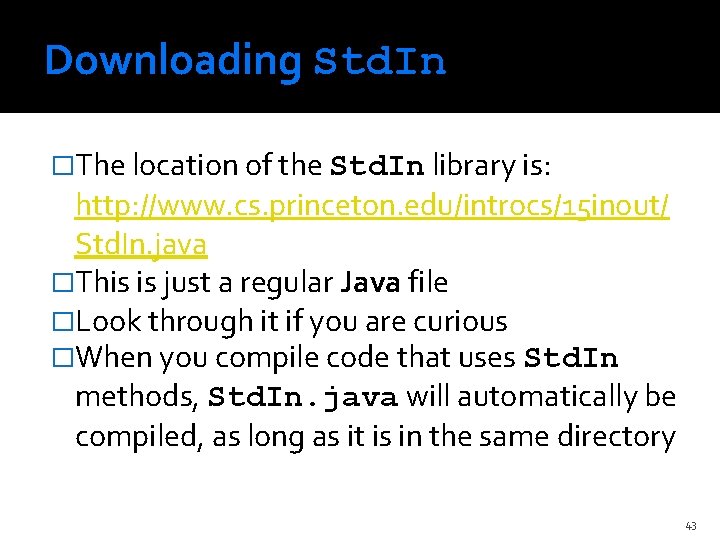
Downloading Std. In �The location of the Std. In library is: http: //www. cs. princeton. edu/introcs/15 inout/ Std. In. java �This is just a regular Java file �Look through it if you are curious �When you compile code that uses Std. In methods, Std. In. java will automatically be compiled, as long as it is in the same directory 43
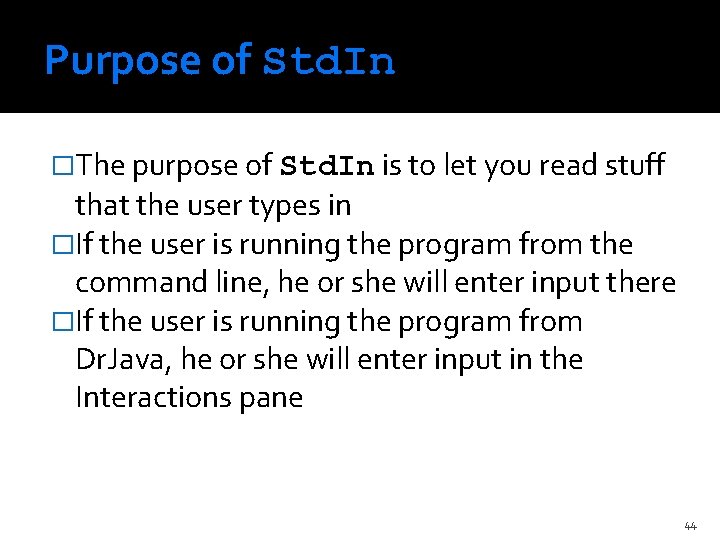
Purpose of Std. In �The purpose of Std. In is to let you read stuff that the user types in �If the user is running the program from the command line, he or she will enter input there �If the user is running the program from Dr. Java, he or she will enter input in the Interactions pane 44
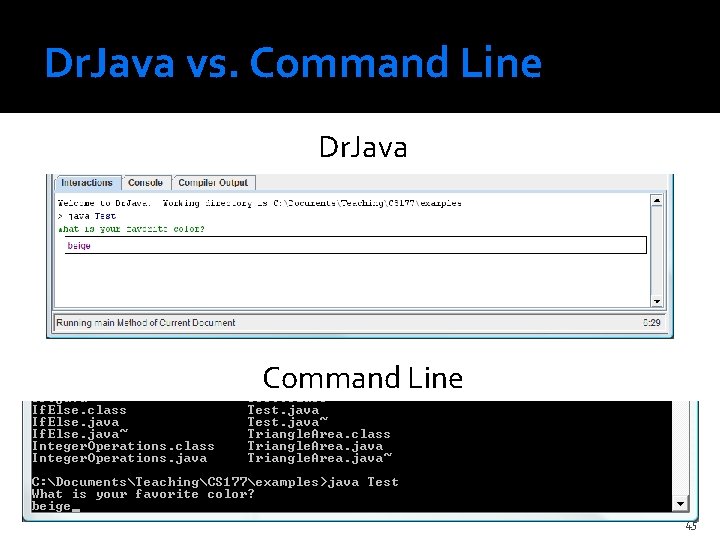
Dr. Java vs. Command Line Dr. Java Command Line 45
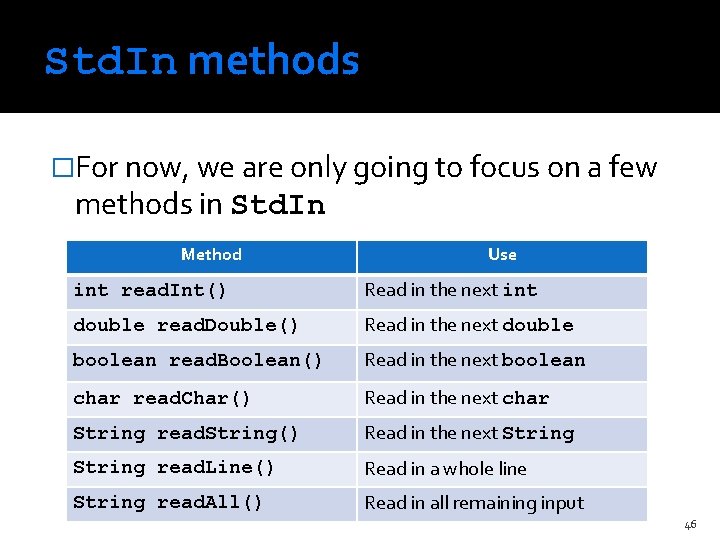
Std. In methods �For now, we are only going to focus on a few methods in Std. In Method Use int read. Int() Read in the next int double read. Double() Read in the next double boolean read. Boolean() Read in the next boolean char read. Char() Read in the next char String read. String() Read in the next String read. Line() Read in a whole line String read. All() Read in all remaining input 46
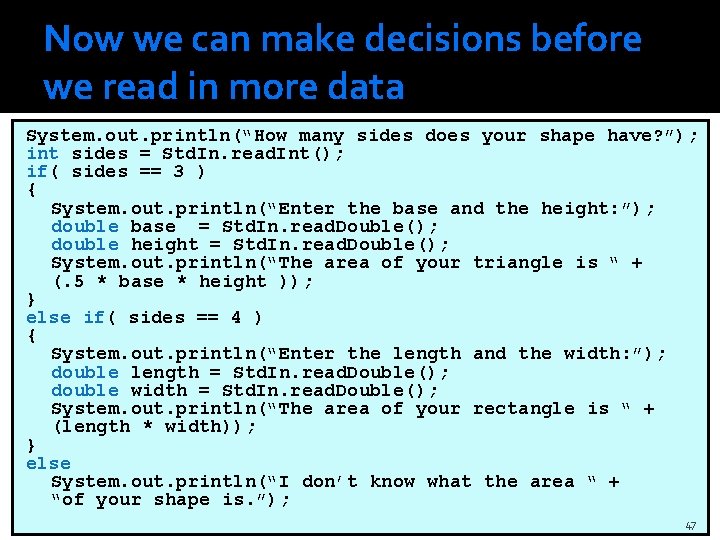
Now we can make decisions before we read in more data System. out. println(“How many sides does your shape have? ”); int sides = Std. In. read. Int(); if( sides == 3 ) { System. out. println(“Enter the base and the height: ”); double base = Std. In. read. Double(); double height = Std. In. read. Double(); System. out. println(“The area of your triangle is “ + (. 5 * base * height )); } else if( sides == 4 ) { System. out. println(“Enter the length and the width: ”); double length = Std. In. read. Double(); double width = Std. In. read. Double(); System. out. println(“The area of your rectangle is “ + (length * width)); } else System. out. println(“I don’t know what the area “ + “of your shape is. ”); 47
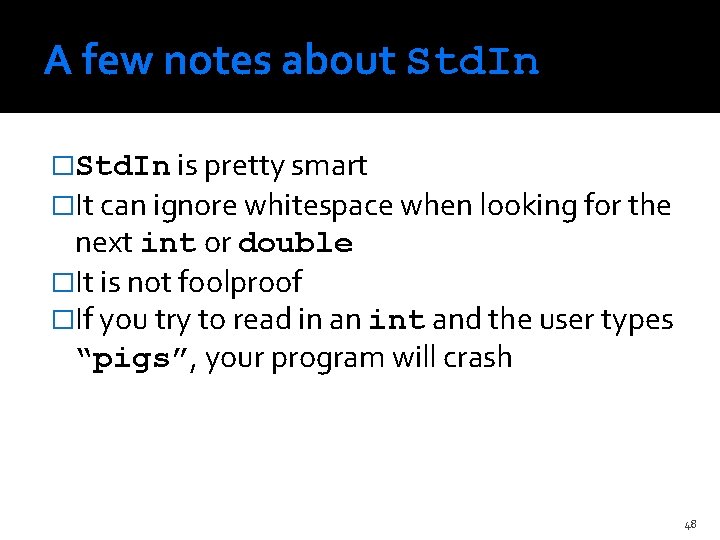
A few notes about Std. In �Std. In is pretty smart �It can ignore whitespace when looking for the next int or double �It is not foolproof �If you try to read in an int and the user types “pigs”, your program will crash 48
Week by week plans for documenting children's development
Heights of hot air balloons qualitative or quantitative
Market practice definition
Gezang 177
Cs 177
177 church street
Aku tuhan semesta
Iliad 177
177 rcacs
(r.s.n.a.o. n° 006-2016- sunat/600000
Lu-177 decay
Cs 177 purdue
Opwekking 585
177 rcacs
Magic emperor 192
Lu-177 decay
Cs 177
1st conditional structure
Past conditional
Mai lai massacre primary sources
Java path finder
Four organizational hurdles to strategy execution
Application execution environment
It portfolio management process steps
Gulfs of execution and evaluation
Machine reference model of execution virtualization
Execution power
Instruction set of 8085
Execution defects
Discipline of the ram
Cassandra stein
Visualfactory
Runahead execution
The falling man saigon execution
A mob's illegal seizure and execution of a person.
Strategy formulation process
Vsem vision strategy execution metrics
Language migrator in system programming
Build execution into strategy
In this scheme cpu execution waits while i/o proceeds.
A process is a program in execution
Build execution into strategy
Yue minjun biography
Ghidra symbolic execution
Contoh gulf of evaluation and gulf of execution
Java applications begin execution at method
In the planning programming budgeting and execution
Design fifo and non fifo execution
Recruiting and retaining capable employees