Conditionals Conditional Execution A conditional statement allows the
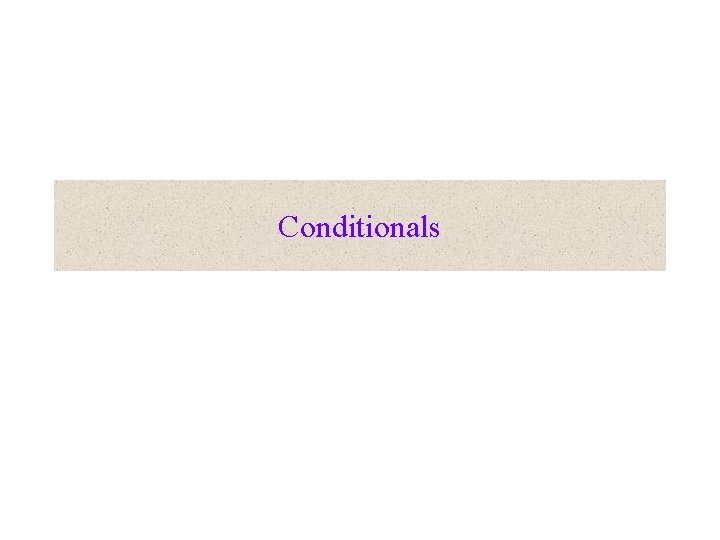
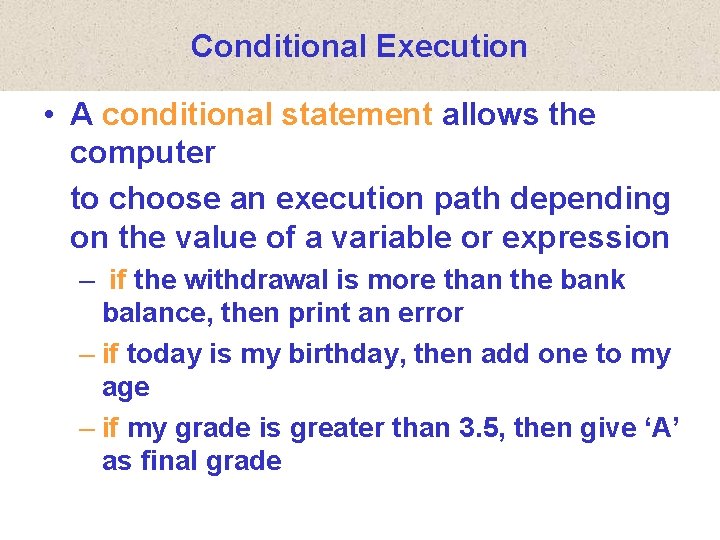
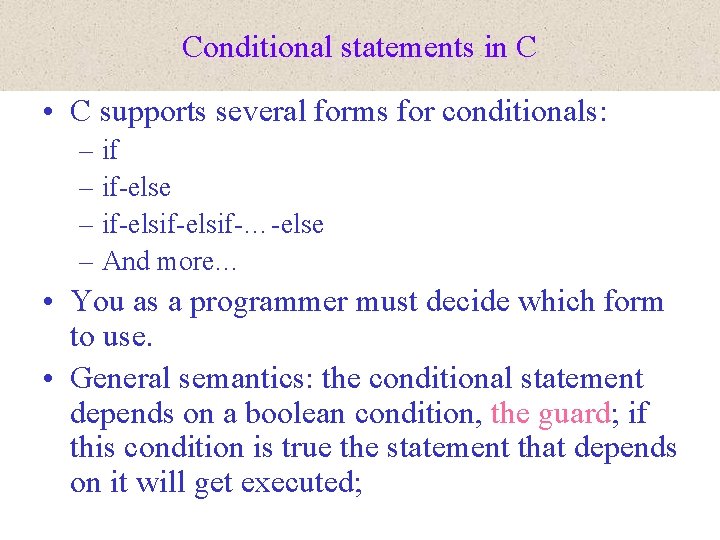
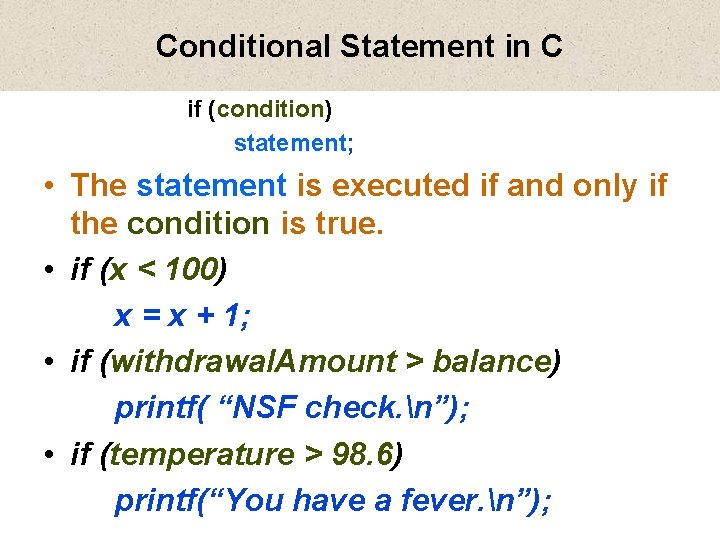
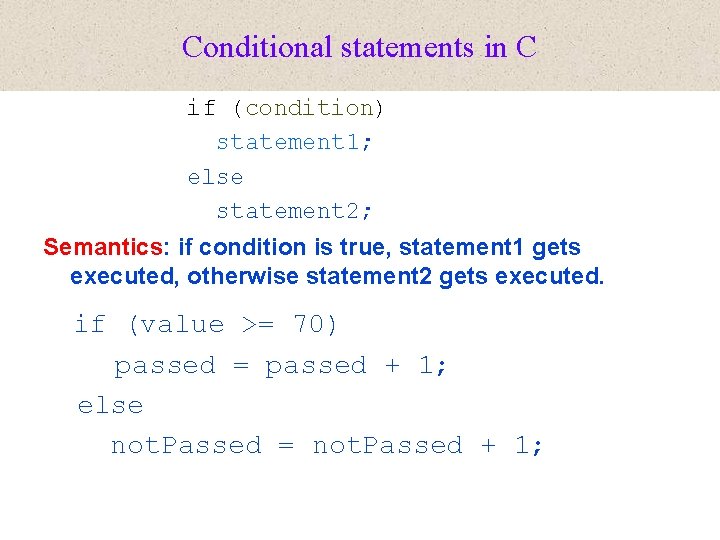
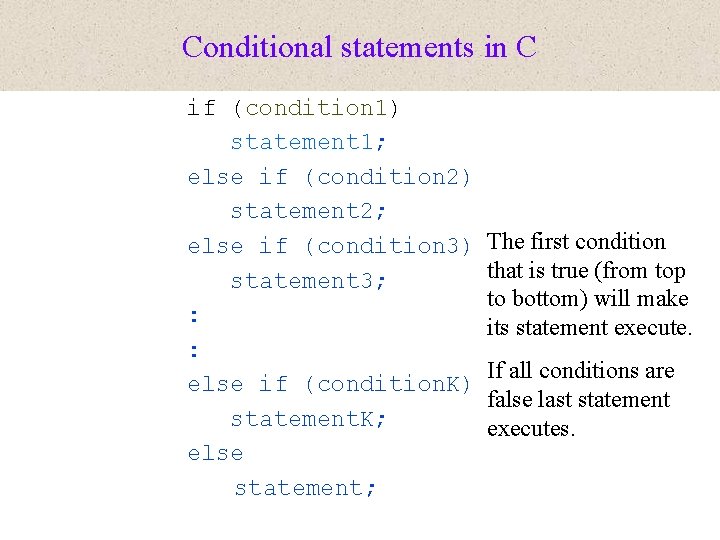
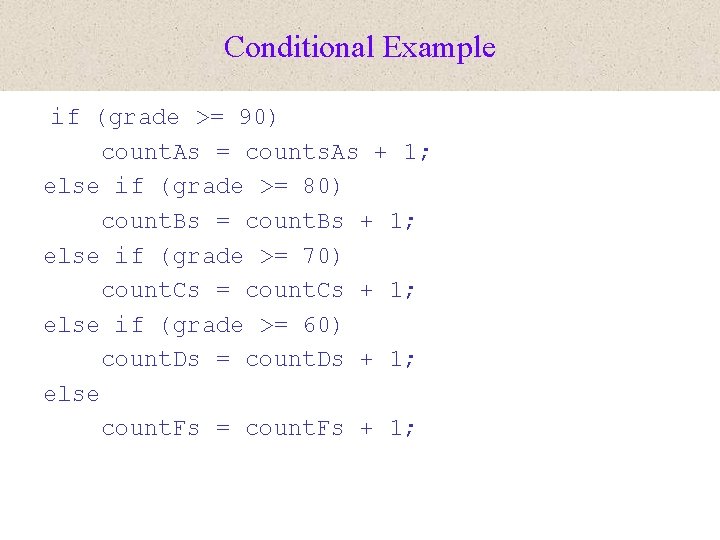
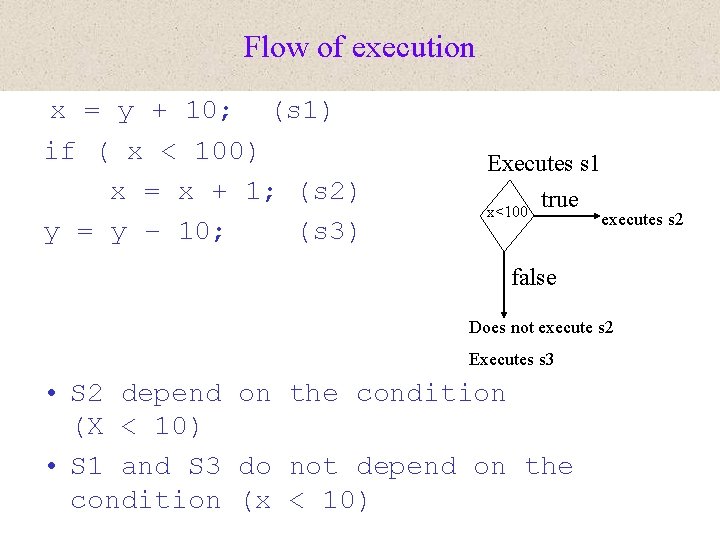
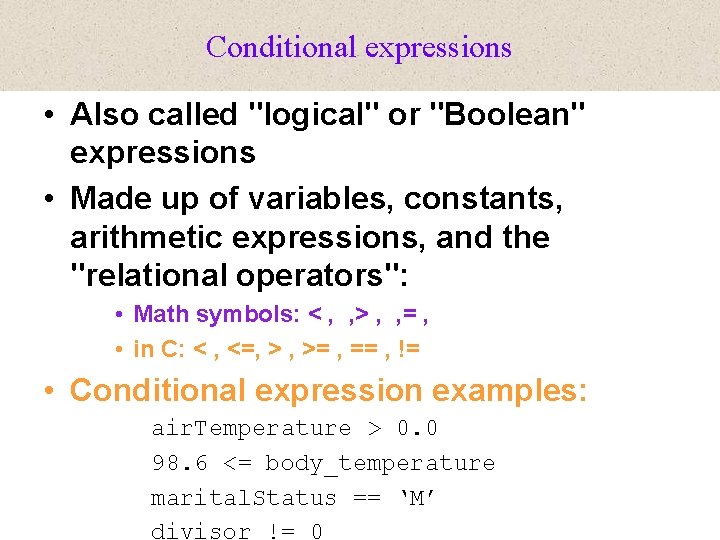
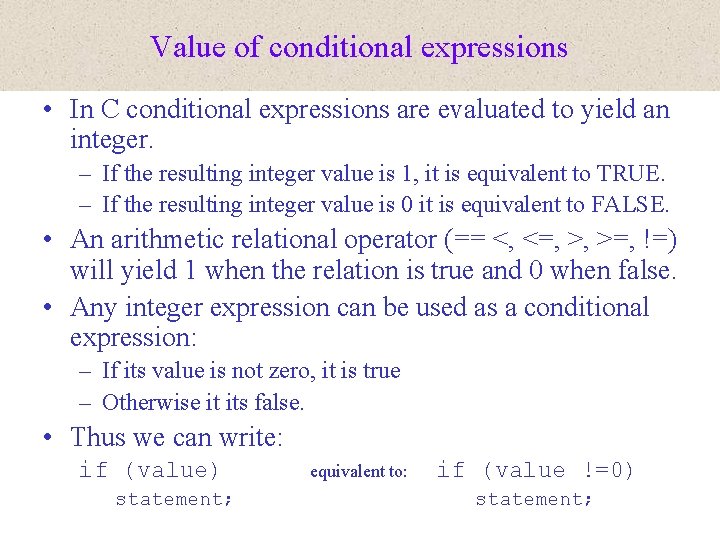
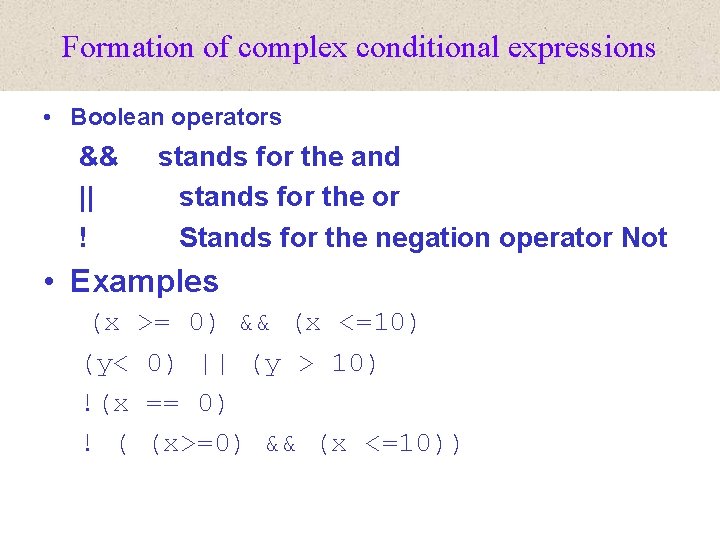
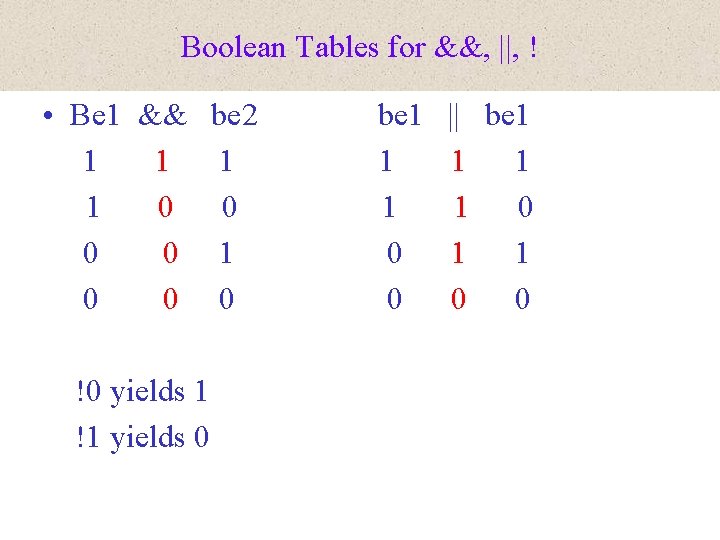
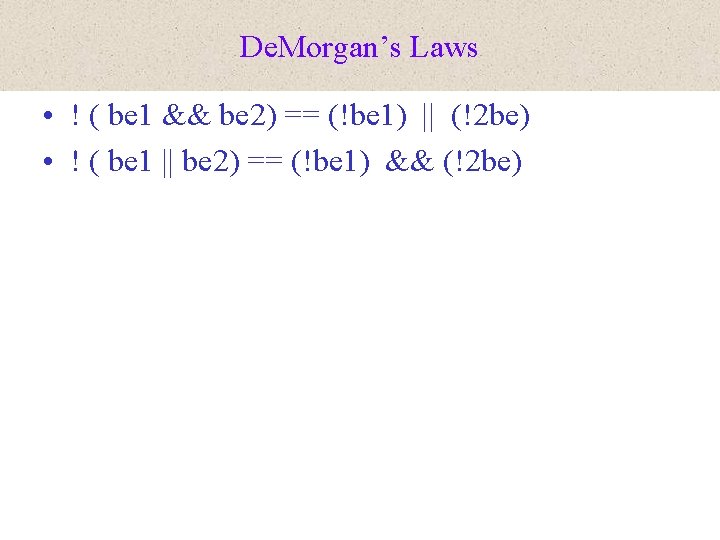
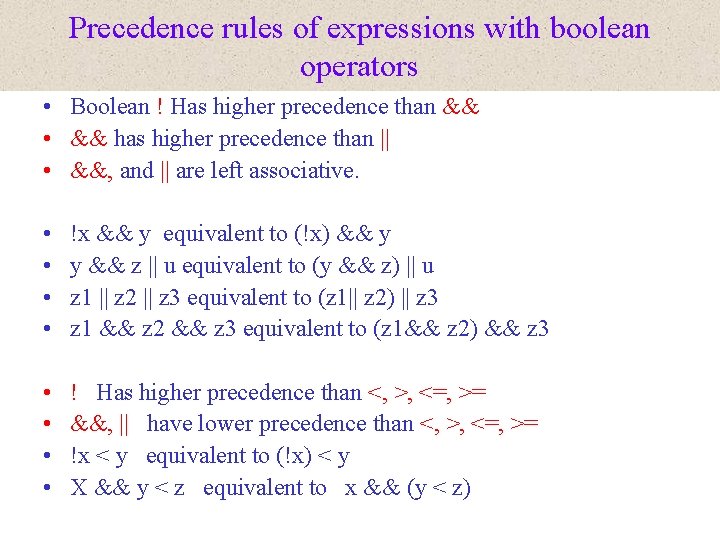
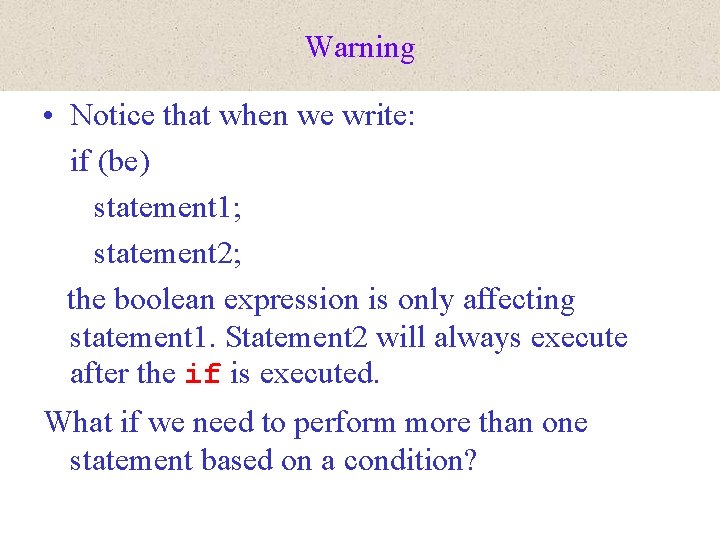
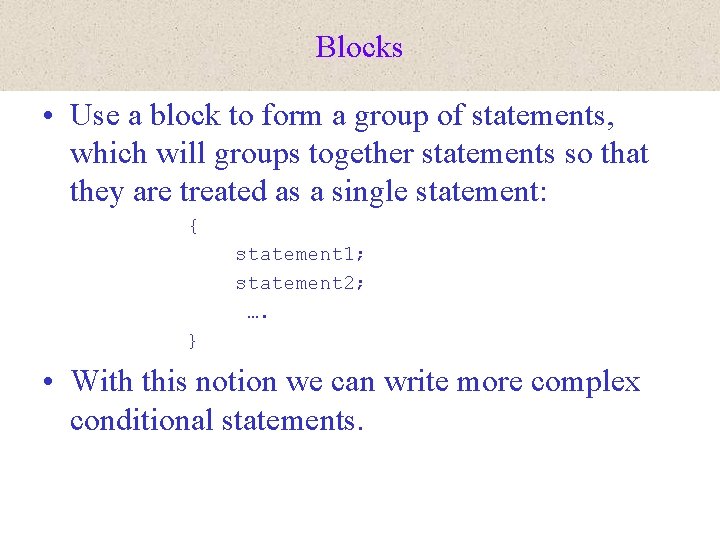
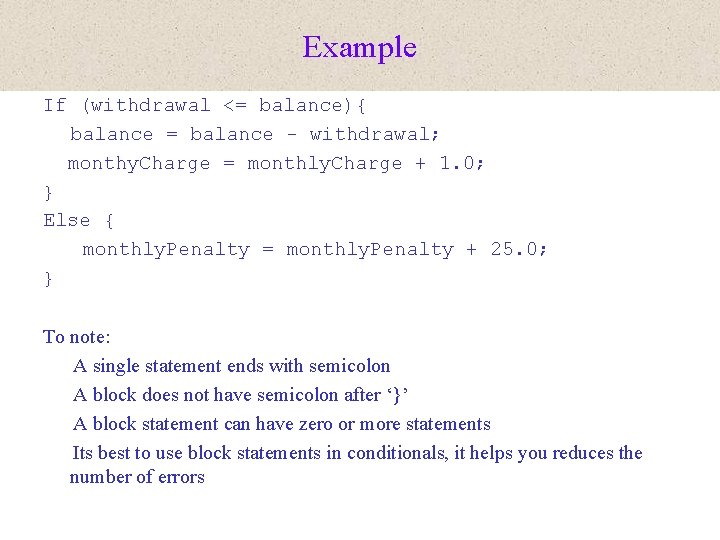
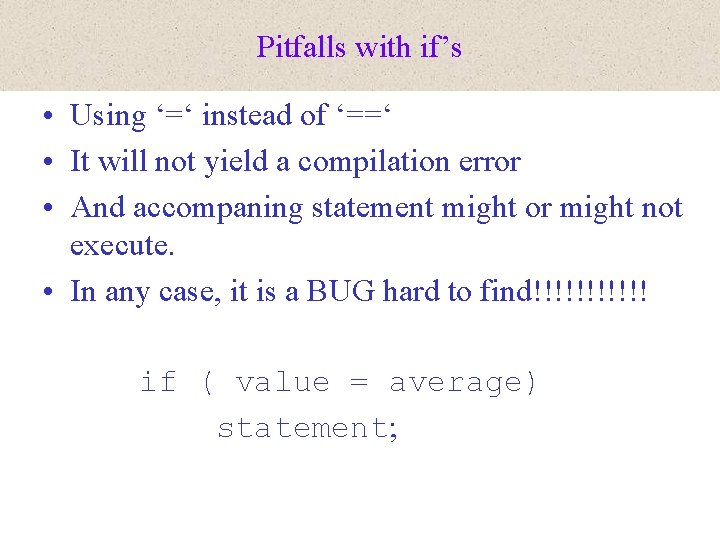
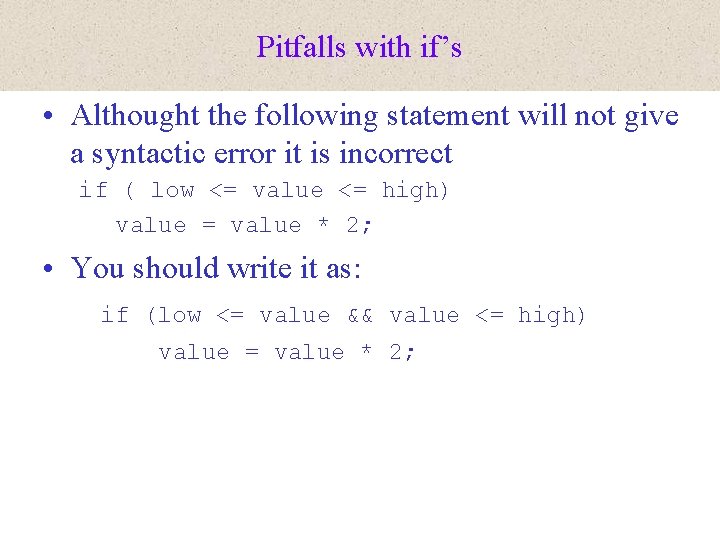
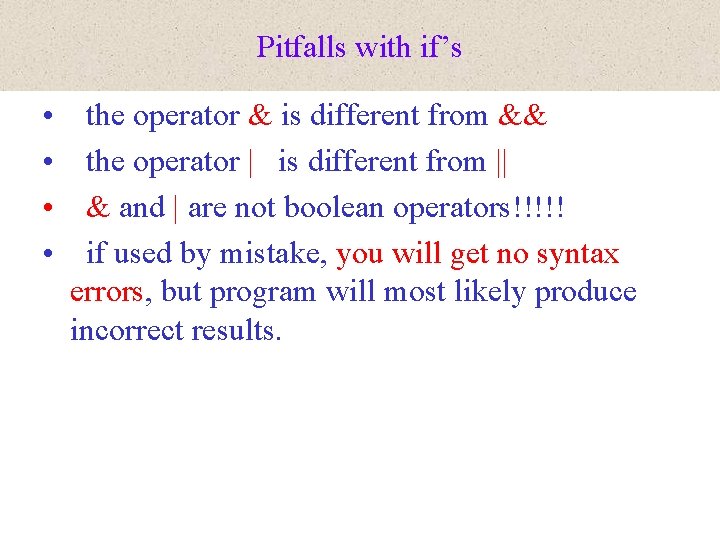
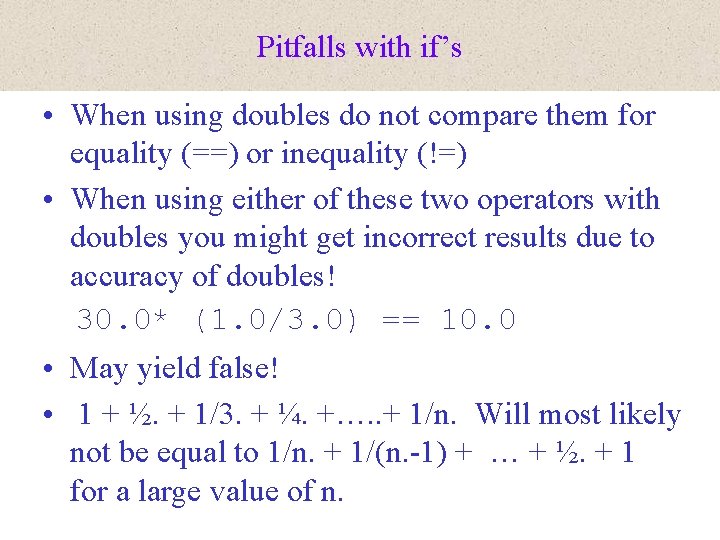
- Slides: 21
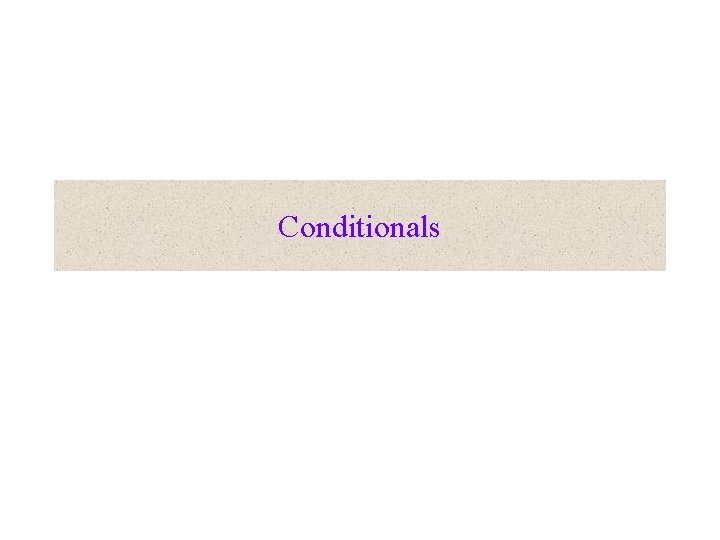
Conditionals
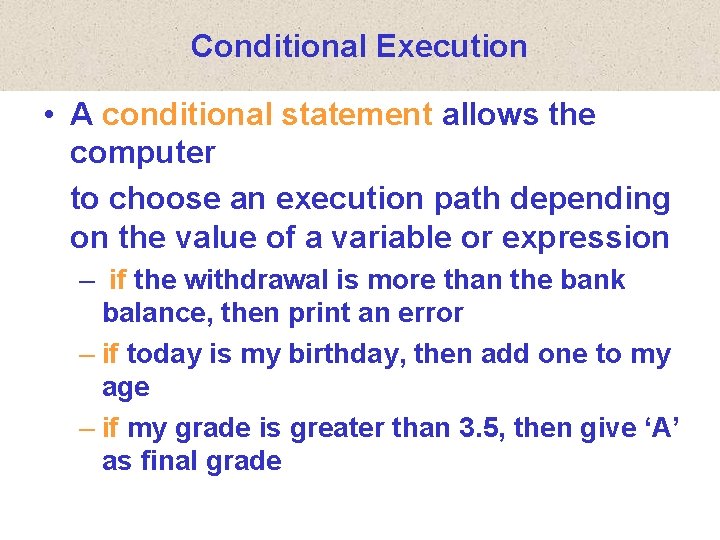
Conditional Execution • A conditional statement allows the computer to choose an execution path depending on the value of a variable or expression – if the withdrawal is more than the bank balance, then print an error – if today is my birthday, then add one to my age – if my grade is greater than 3. 5, then give ‘A’ as final grade
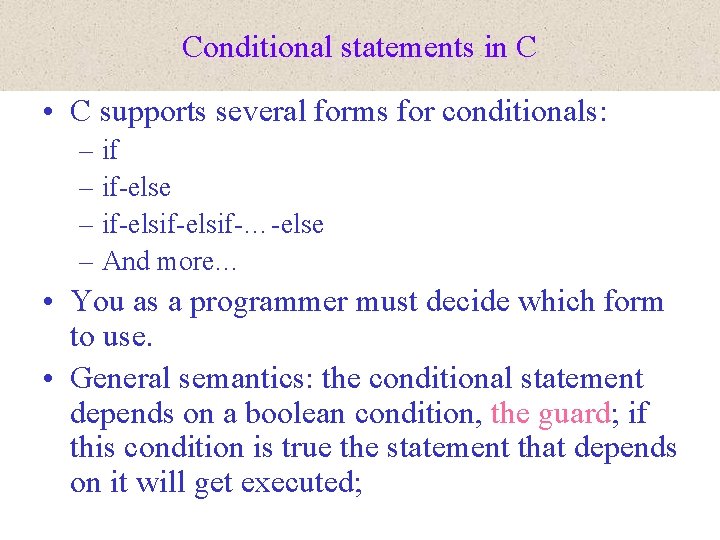
Conditional statements in C • C supports several forms for conditionals: – if-else – if-elsif-…-else – And more… • You as a programmer must decide which form to use. • General semantics: the conditional statement depends on a boolean condition, the guard; if this condition is true the statement that depends on it will get executed;
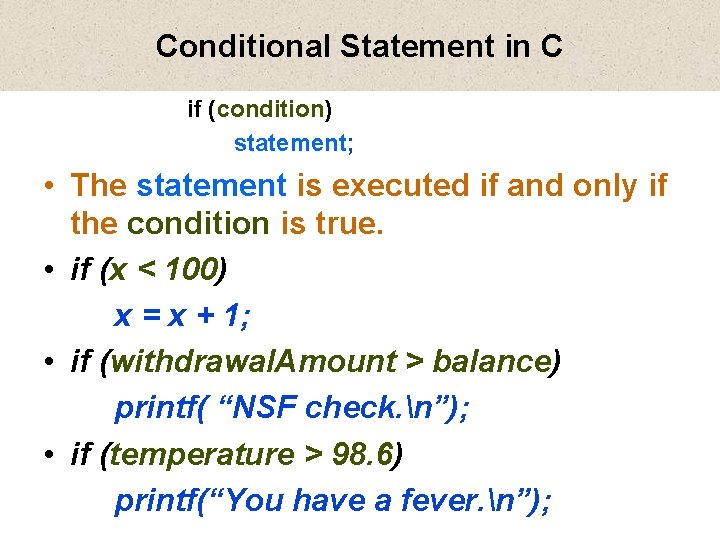
Conditional Statement in C if (condition) statement; • The statement is executed if and only if the condition is true. • if (x < 100) x = x + 1; • if (withdrawal. Amount > balance) printf( “NSF check. n”); • if (temperature > 98. 6) printf(“You have a fever. n”);
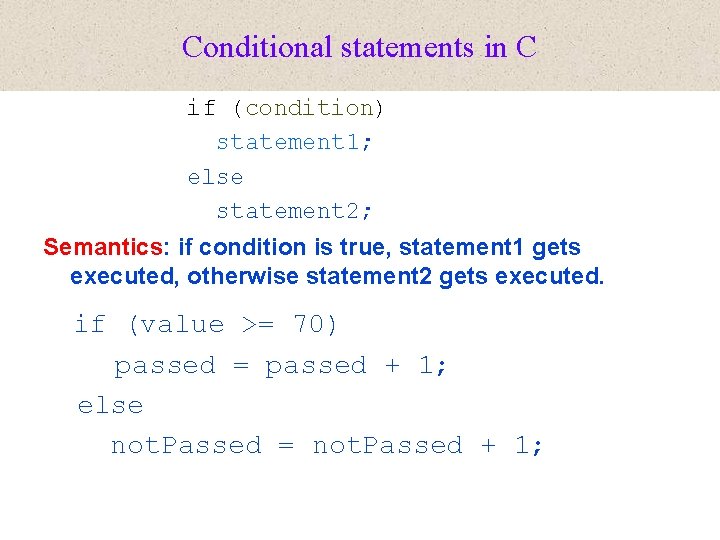
Conditional statements in C if (condition) statement 1; else statement 2; Semantics: if condition is true, statement 1 gets executed, otherwise statement 2 gets executed. if (value >= 70) passed = passed + 1; else not. Passed = not. Passed + 1;
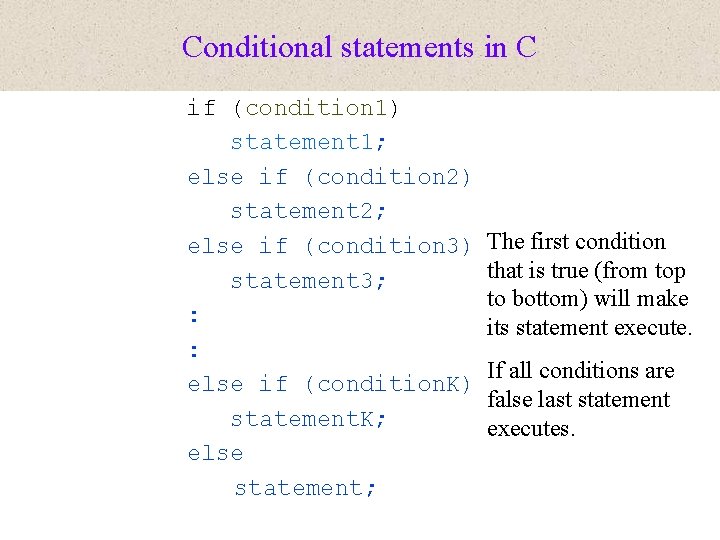
Conditional statements in C if (condition 1) statement 1; else if (condition 2) statement 2; else if (condition 3) statement 3; : : else if (condition. K) statement. K; else statement; The first condition that is true (from top to bottom) will make its statement execute. If all conditions are false last statement executes.
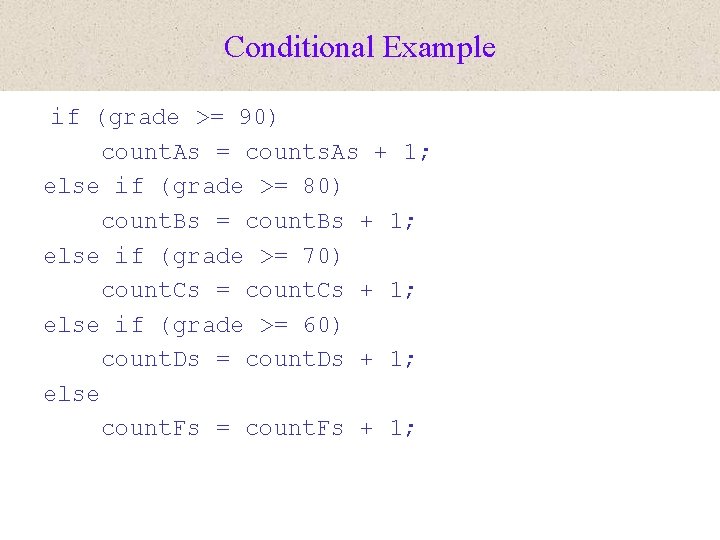
Conditional Example if (grade >= 90) count. As = counts. As + 1; else if (grade >= 80) count. Bs = count. Bs + 1; else if (grade >= 70) count. Cs = count. Cs + 1; else if (grade >= 60) count. Ds = count. Ds + 1; else count. Fs = count. Fs + 1;
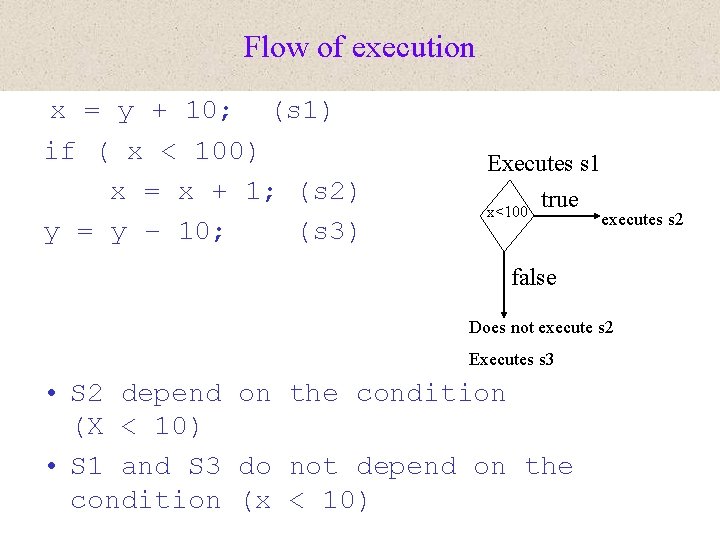
Flow of execution x = y + 10; (s 1) if ( x < 100) x = x + 1; (s 2) y = y – 10; (s 3) Executes s 1 true x<100 executes s 2 false Does not execute s 2 Executes s 3 • S 2 depend on the condition (X < 10) • S 1 and S 3 do not depend on the condition (x < 10)
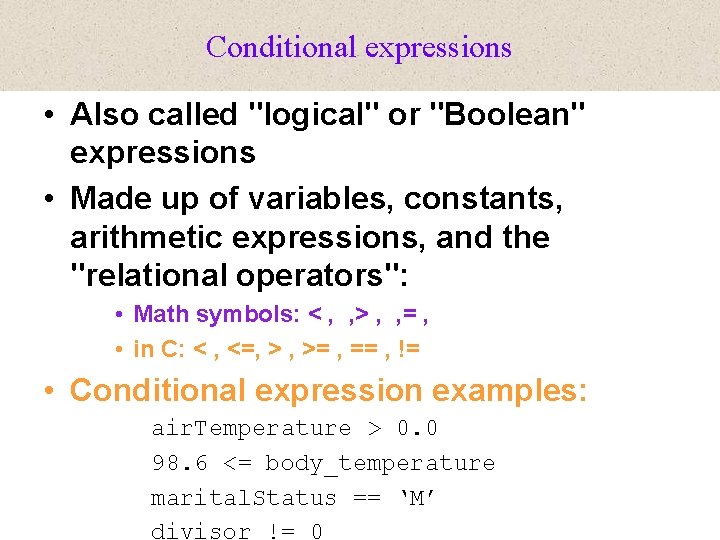
Conditional expressions • Also called "logical" or "Boolean" expressions • Made up of variables, constants, arithmetic expressions, and the "relational operators": • Math symbols: < , , > , , = , • in C: < , <=, > , >= , == , != • Conditional expression examples: air. Temperature > 0. 0 98. 6 <= body_temperature marital. Status == ‘M’ divisor != 0
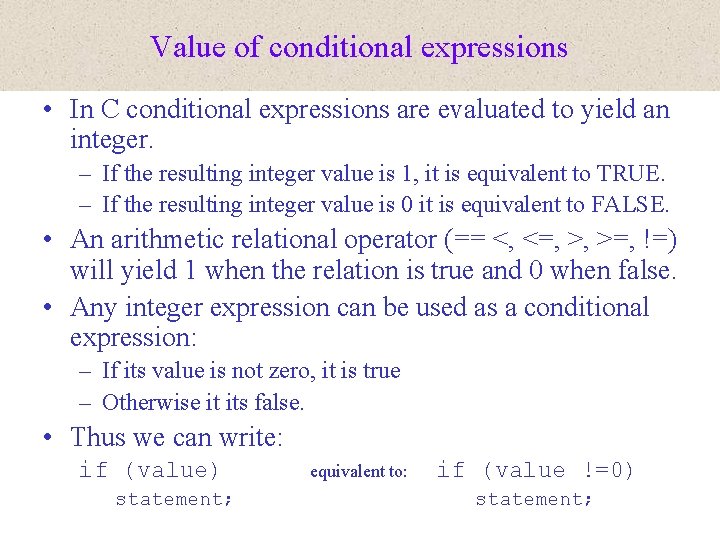
Value of conditional expressions • In C conditional expressions are evaluated to yield an integer. – If the resulting integer value is 1, it is equivalent to TRUE. – If the resulting integer value is 0 it is equivalent to FALSE. • An arithmetic relational operator (== <, <=, >, >=, !=) will yield 1 when the relation is true and 0 when false. • Any integer expression can be used as a conditional expression: – If its value is not zero, it is true – Otherwise it its false. • Thus we can write: if (value) statement; equivalent to: if (value !=0) statement;
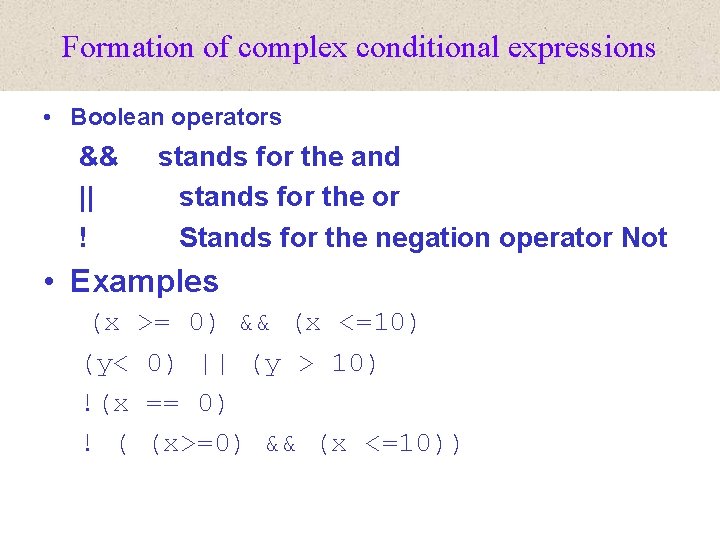
Formation of complex conditional expressions • Boolean operators && || ! stands for the and stands for the or Stands for the negation operator Not • Examples (x >= 0) && (x <=10) (y< 0) || (y > 10) !(x == 0) ! ( (x>=0) && (x <=10))
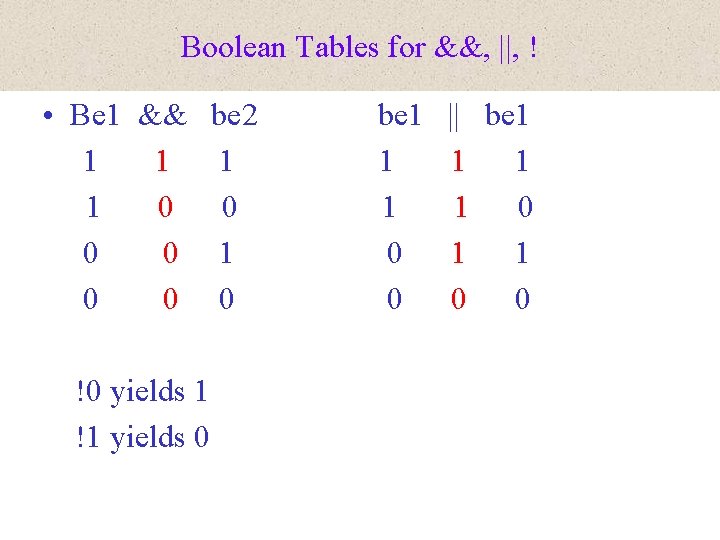
Boolean Tables for &&, ||, ! • Be 1 && be 2 1 1 0 0 0 !0 yields 1 !1 yields 0 be 1 1 1 0 0 || be 1 1 0 0
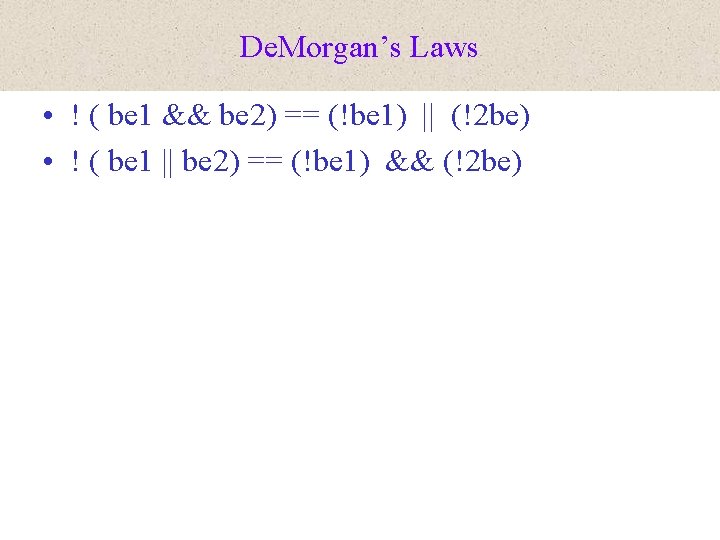
De. Morgan’s Laws • ! ( be 1 && be 2) == (!be 1) || (!2 be) • ! ( be 1 || be 2) == (!be 1) && (!2 be)
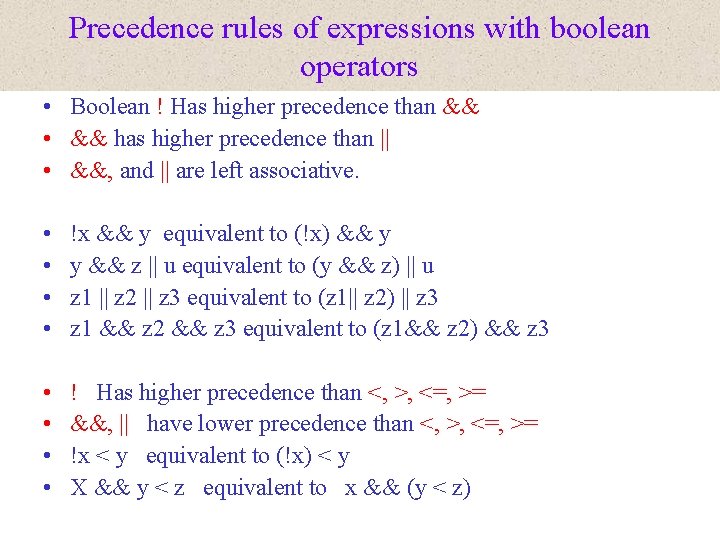
Precedence rules of expressions with boolean operators • Boolean ! Has higher precedence than && • && has higher precedence than || • &&, and || are left associative. • • !x && y equivalent to (!x) && y y && z || u equivalent to (y && z) || u z 1 || z 2 || z 3 equivalent to (z 1|| z 2) || z 3 z 1 && z 2 && z 3 equivalent to (z 1&& z 2) && z 3 • • ! Has higher precedence than <, >, <=, >= &&, || have lower precedence than <, >, <=, >= !x < y equivalent to (!x) < y X && y < z equivalent to x && (y < z)
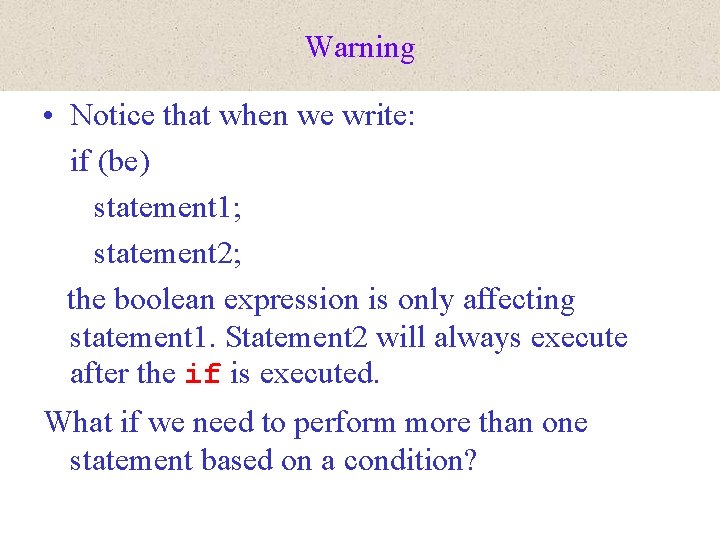
Warning • Notice that when we write: if (be) statement 1; statement 2; the boolean expression is only affecting statement 1. Statement 2 will always execute after the if is executed. What if we need to perform more than one statement based on a condition?
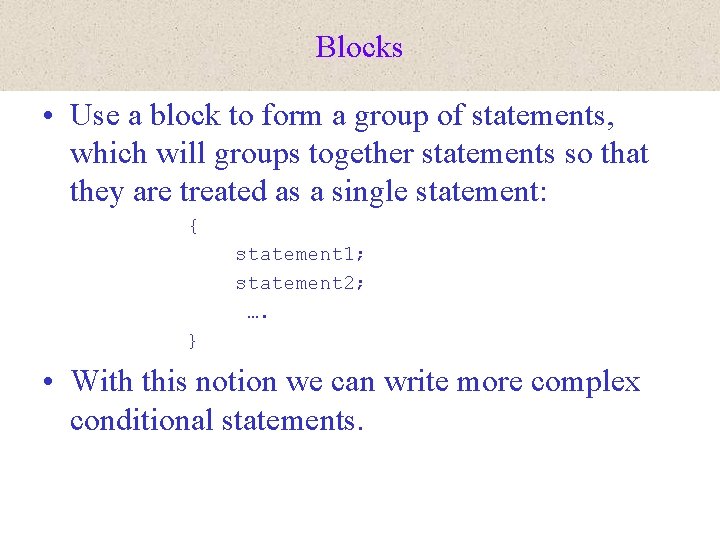
Blocks • Use a block to form a group of statements, which will groups together statements so that they are treated as a single statement: { statement 1; statement 2; …. } • With this notion we can write more complex conditional statements.
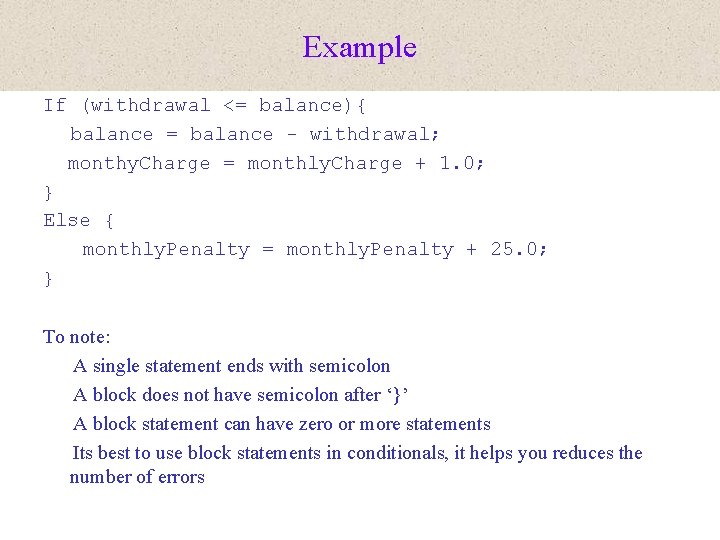
Example If (withdrawal <= balance){ balance = balance - withdrawal; monthy. Charge = monthly. Charge + 1. 0; } Else { monthly. Penalty = monthly. Penalty + 25. 0; } To note: A single statement ends with semicolon A block does not have semicolon after ‘}’ A block statement can have zero or more statements Its best to use block statements in conditionals, it helps you reduces the number of errors
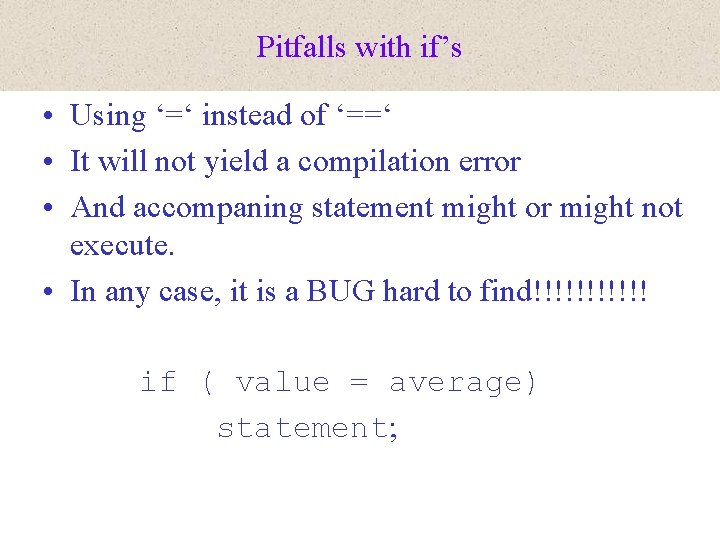
Pitfalls with if’s • Using ‘=‘ instead of ‘==‘ • It will not yield a compilation error • And accompaning statement might or might not execute. • In any case, it is a BUG hard to find!!!!!! if ( value = average) statement;
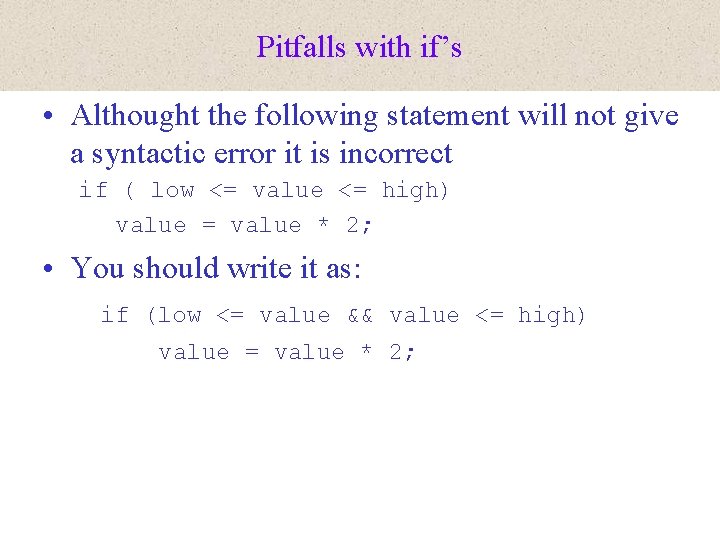
Pitfalls with if’s • Althought the following statement will not give a syntactic error it is incorrect if ( low <= value <= high) value = value * 2; • You should write it as: if (low <= value && value <= high) value = value * 2;
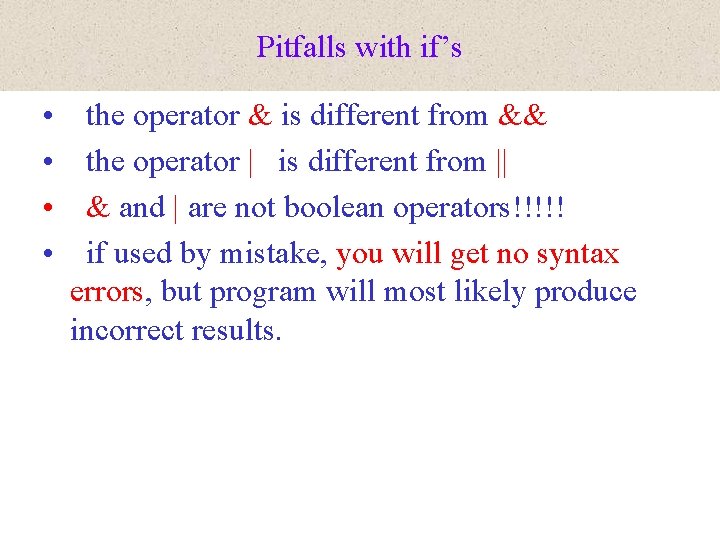
Pitfalls with if’s • • the operator & is different from && the operator | is different from || & and | are not boolean operators!!!!! if used by mistake, you will get no syntax errors, but program will most likely produce incorrect results.
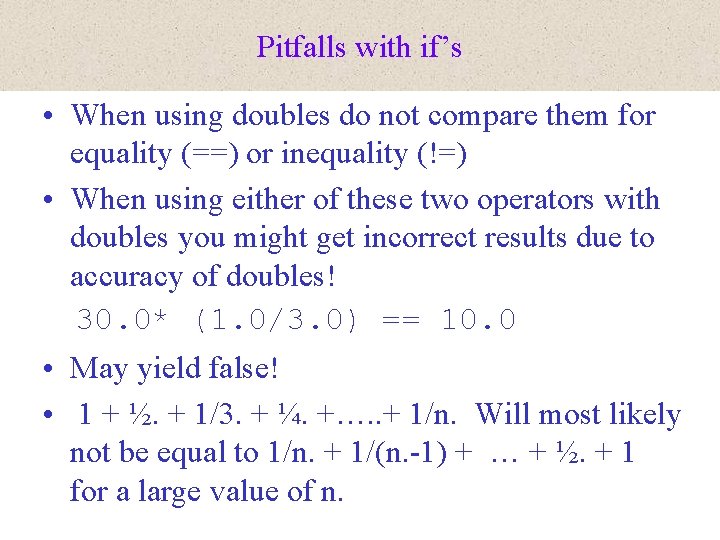
Pitfalls with if’s • When using doubles do not compare them for equality (==) or inequality (!=) • When using either of these two operators with doubles you might get incorrect results due to accuracy of doubles! 30. 0* (1. 0/3. 0) == 10. 0 • May yield false! • 1 + ½. + 1/3. + ¼. +…. . + 1/n. Will most likely not be equal to 1/n. + 1/(n. -1) + … + ½. + 1 for a large value of n.