Conditional Jump Conditional Loop Instructions and Conditional Structures
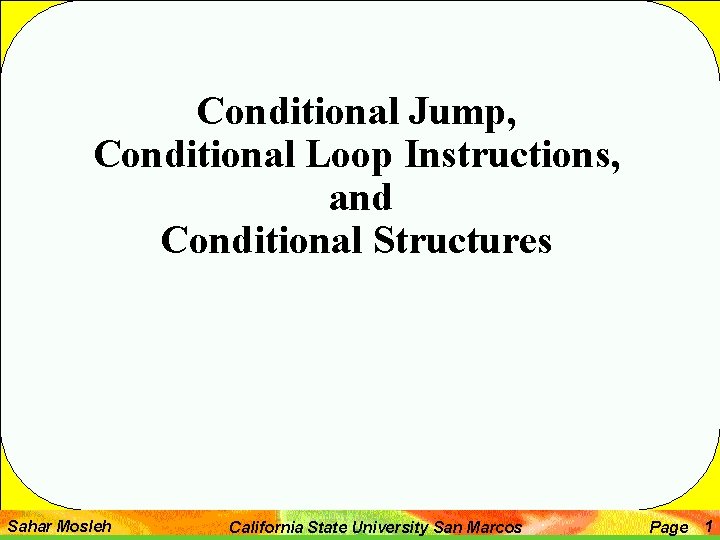
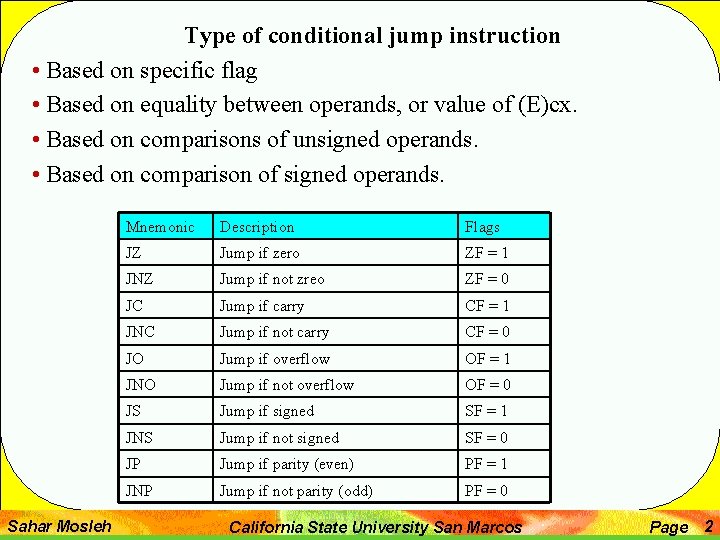
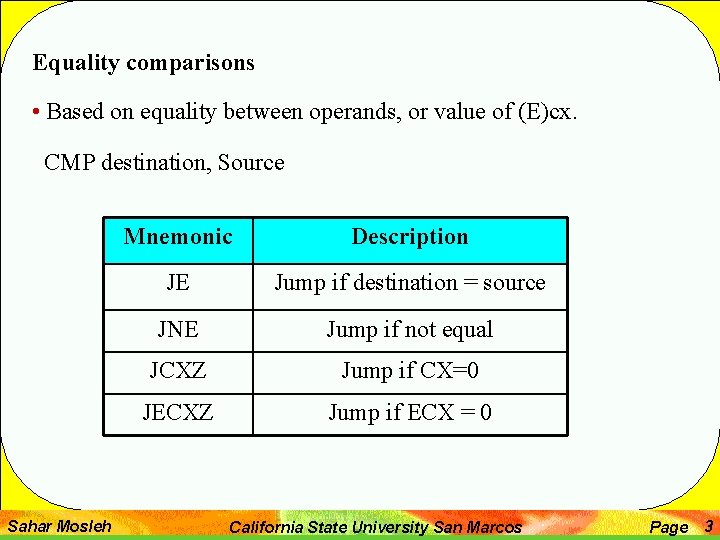
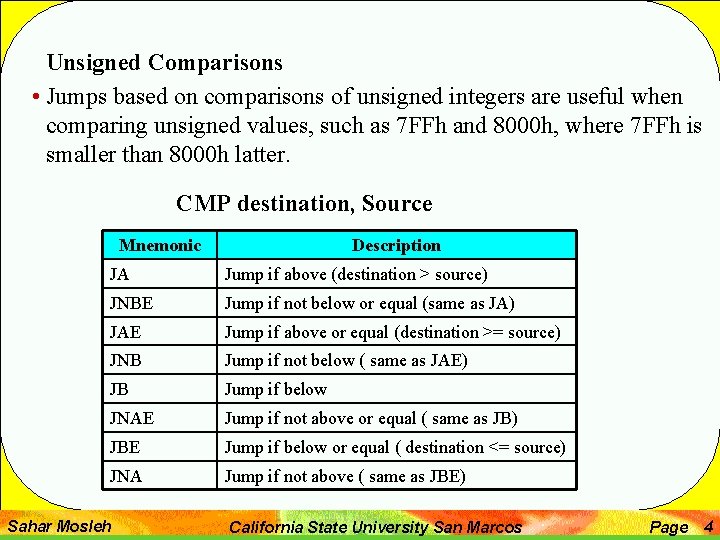
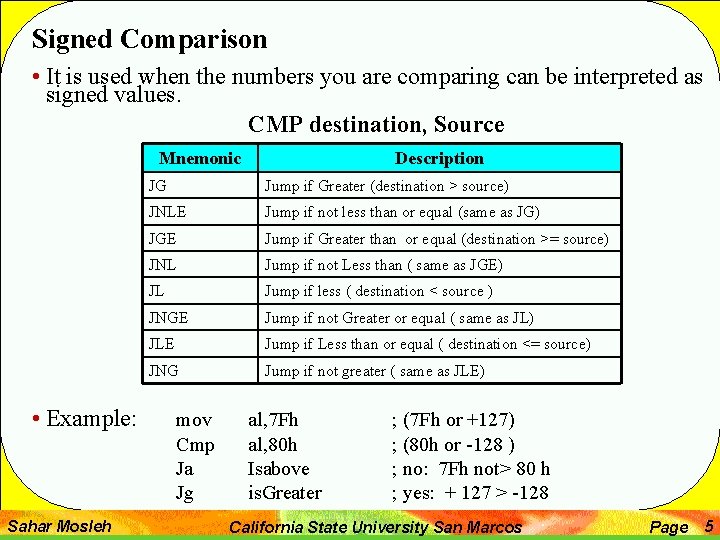
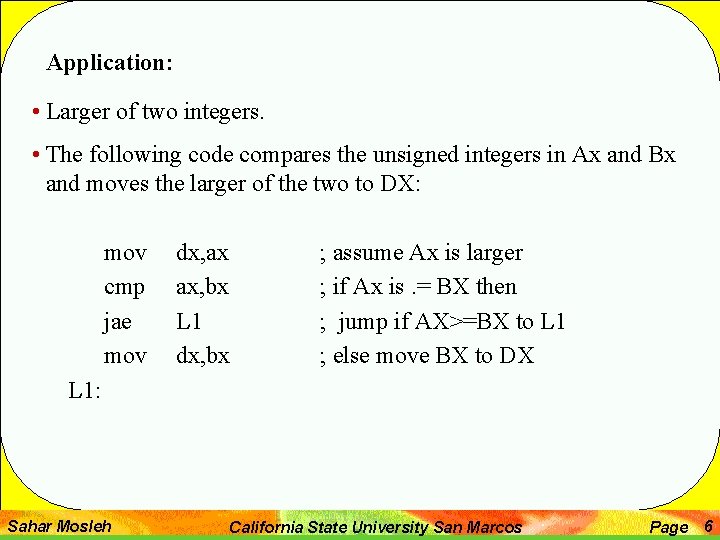
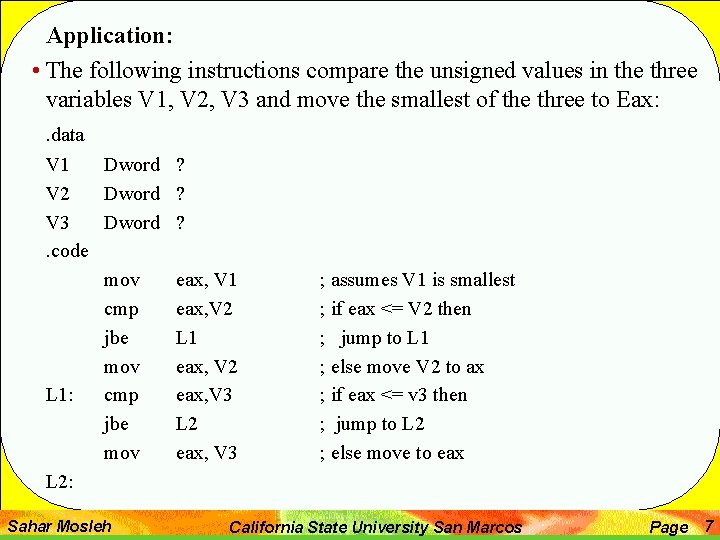
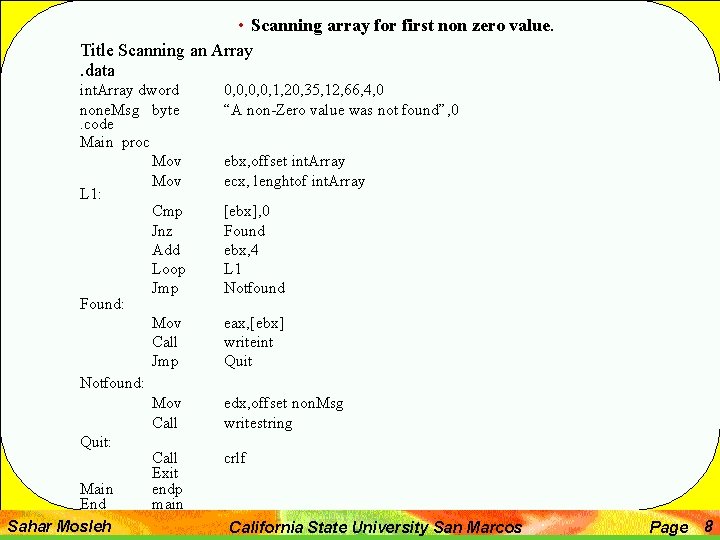
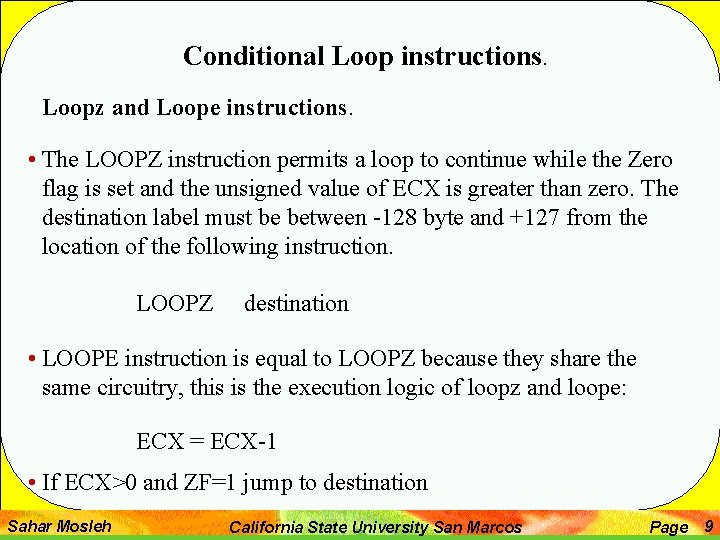
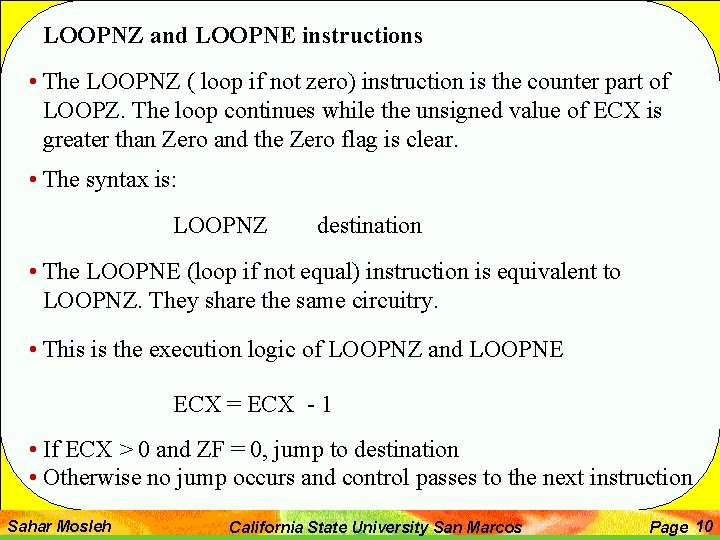
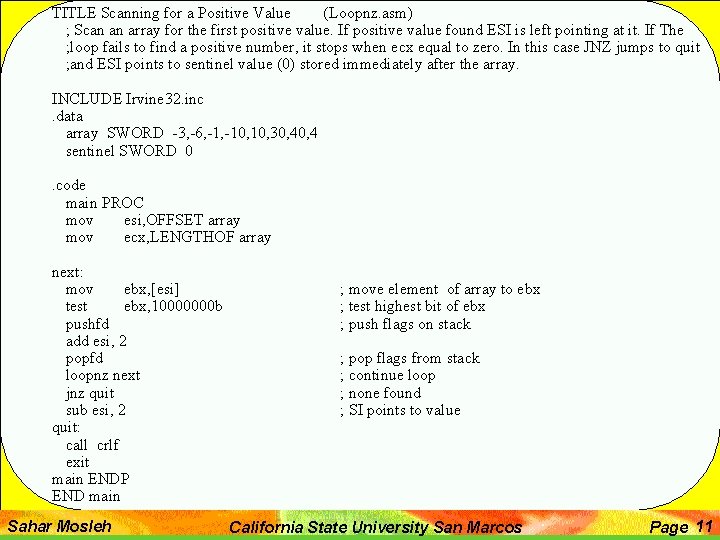
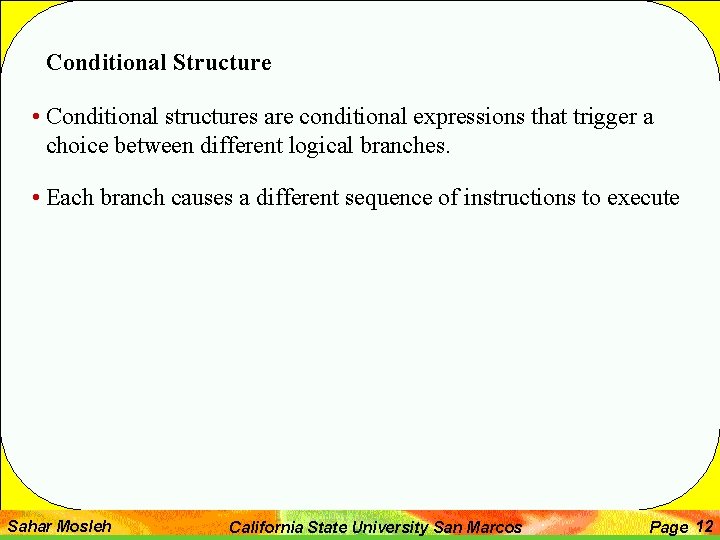
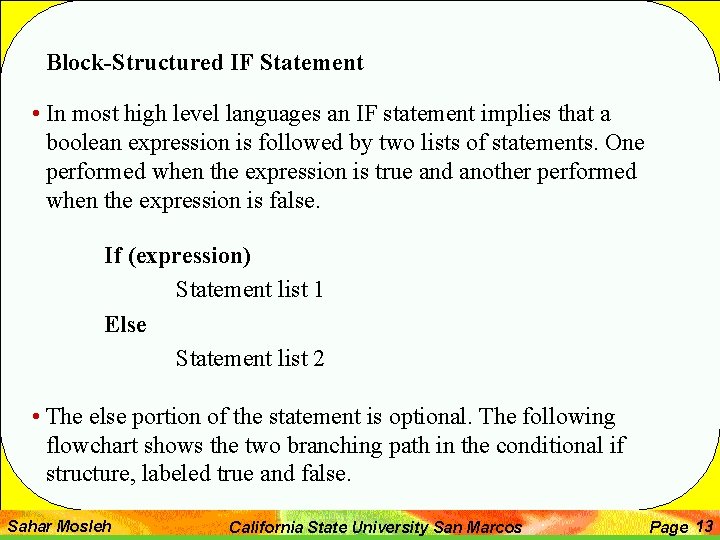
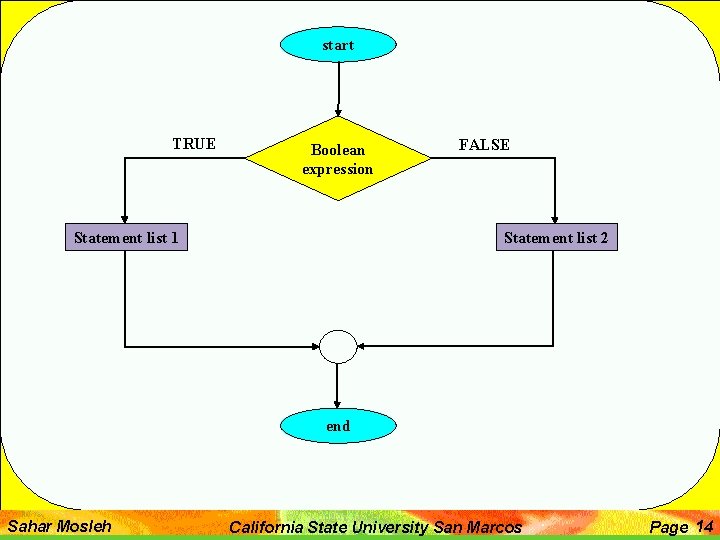
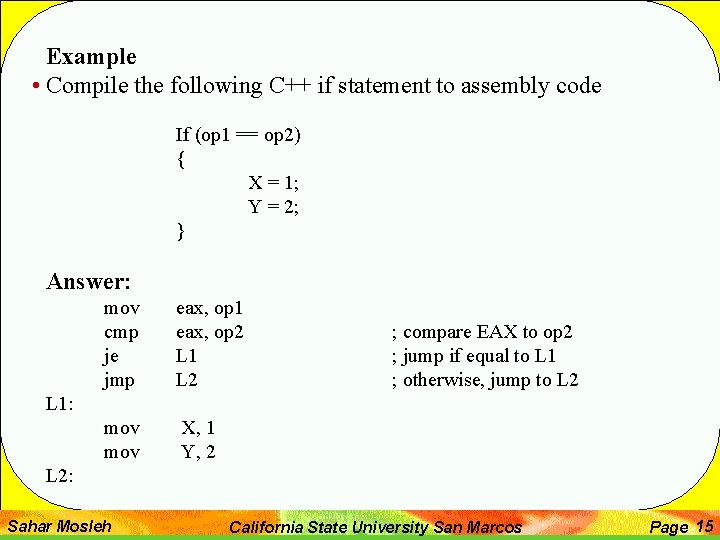
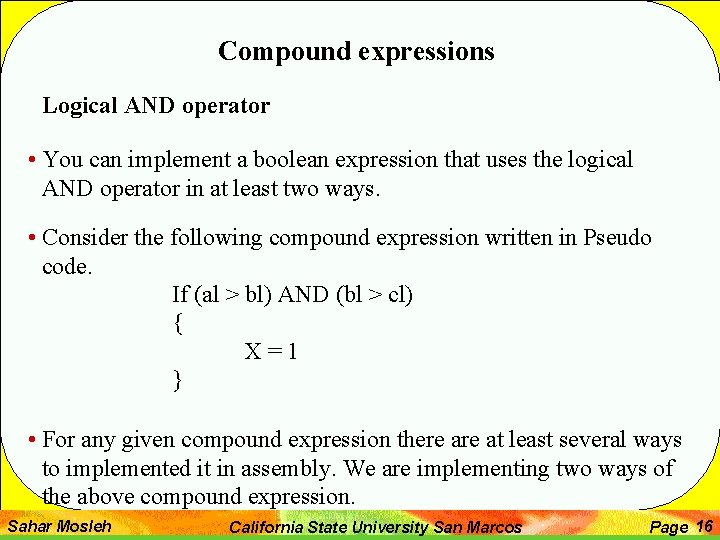
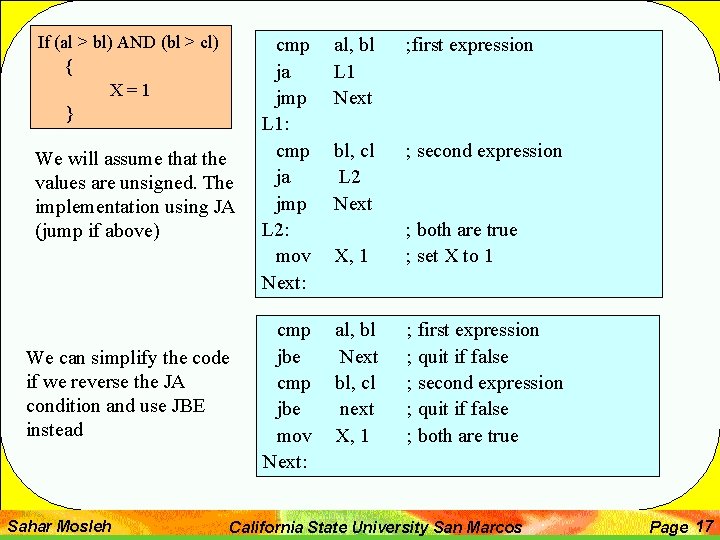
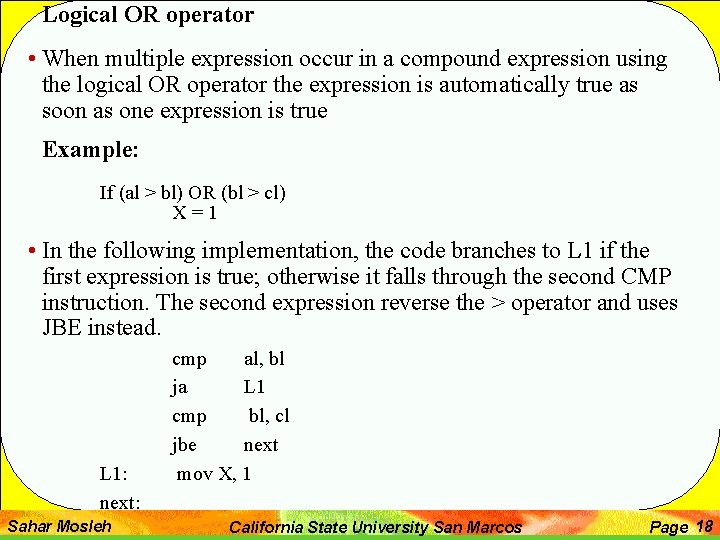
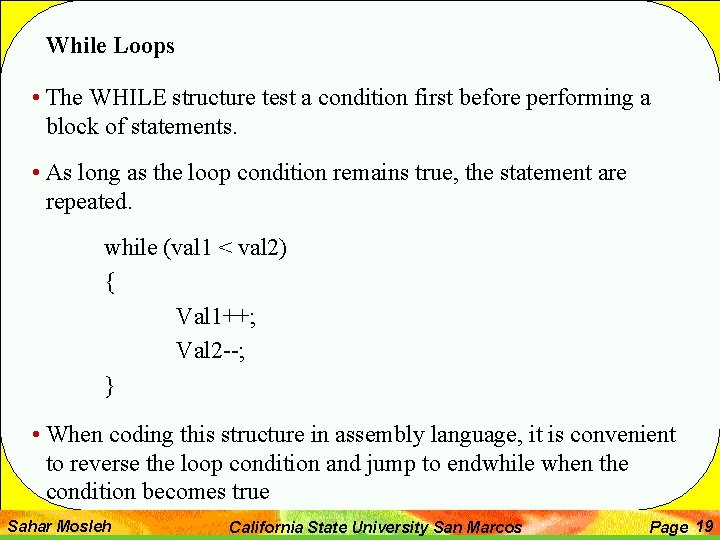
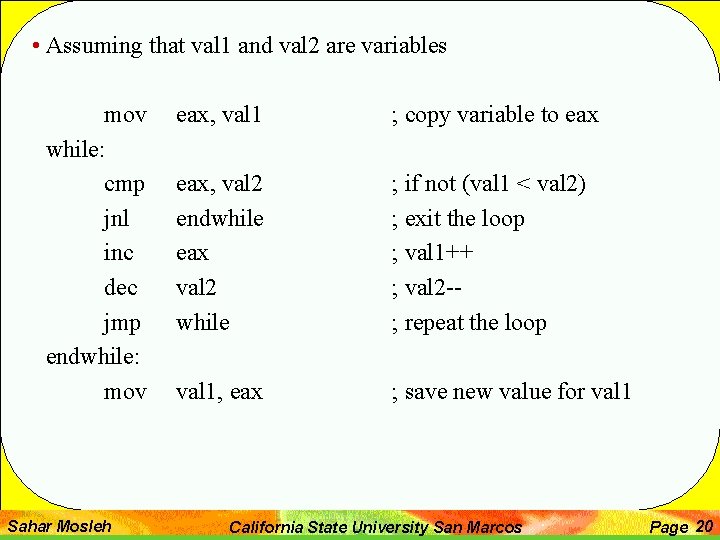
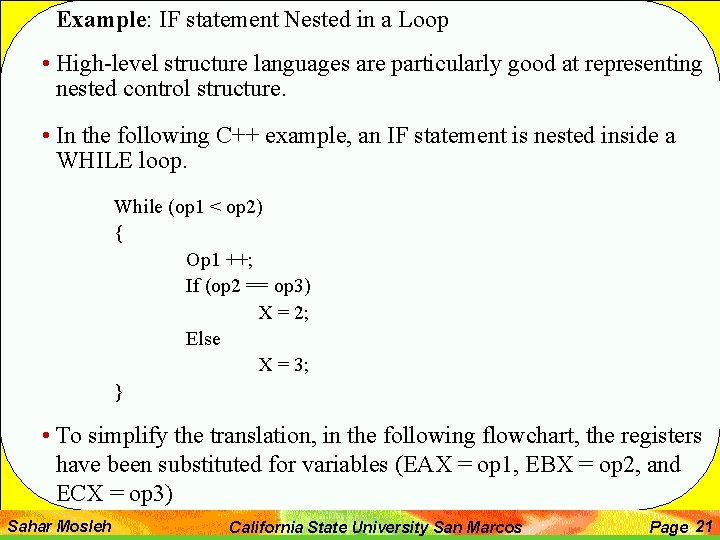
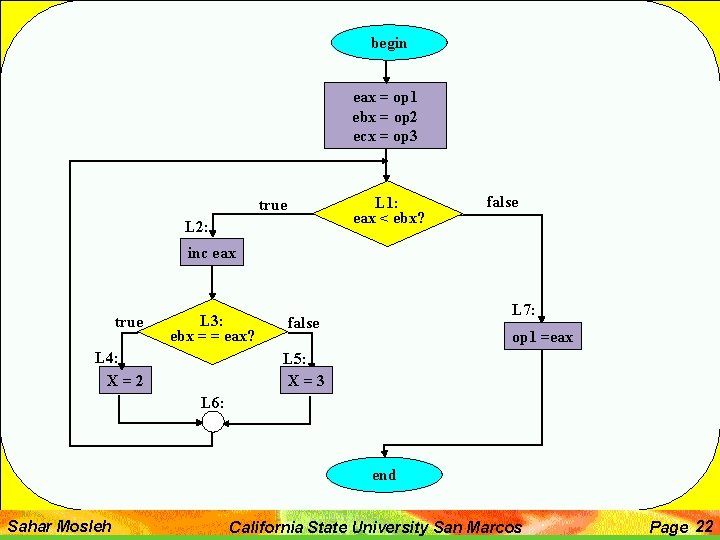
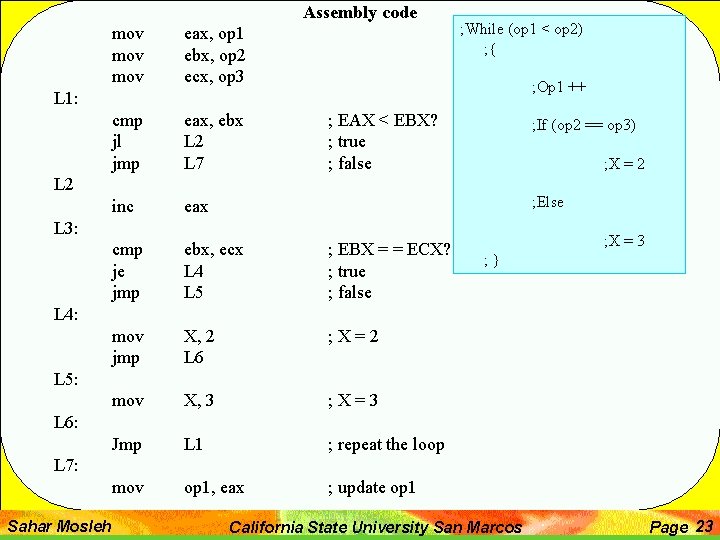
- Slides: 23
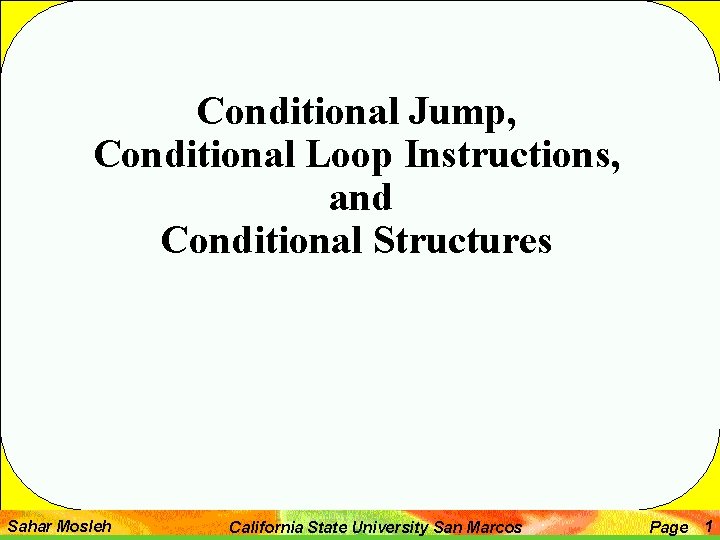
Conditional Jump, Conditional Loop Instructions, and Conditional Structures Sahar Mosleh California State University San Marcos Page 1
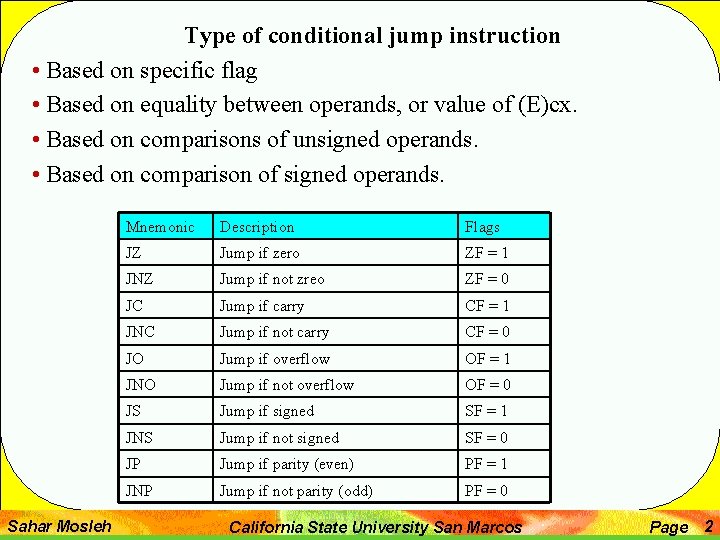
Type of conditional jump instruction • Based on specific flag • Based on equality between operands, or value of (E)cx. • Based on comparisons of unsigned operands. • Based on comparison of signed operands. Sahar Mosleh Mnemonic Description Flags JZ Jump if zero ZF = 1 JNZ Jump if not zreo ZF = 0 JC Jump if carry CF = 1 JNC Jump if not carry CF = 0 JO Jump if overflow OF = 1 JNO Jump if not overflow OF = 0 JS Jump if signed SF = 1 JNS Jump if not signed SF = 0 JP Jump if parity (even) PF = 1 JNP Jump if not parity (odd) PF = 0 California State University San Marcos Page 2
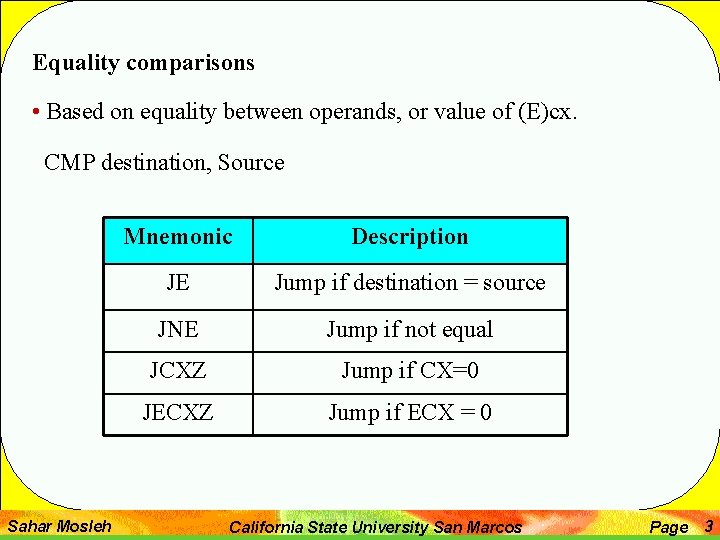
Equality comparisons • Based on equality between operands, or value of (E)cx. CMP destination, Source Sahar Mosleh Mnemonic Description JE Jump if destination = source JNE Jump if not equal JCXZ Jump if CX=0 JECXZ Jump if ECX = 0 California State University San Marcos Page 3
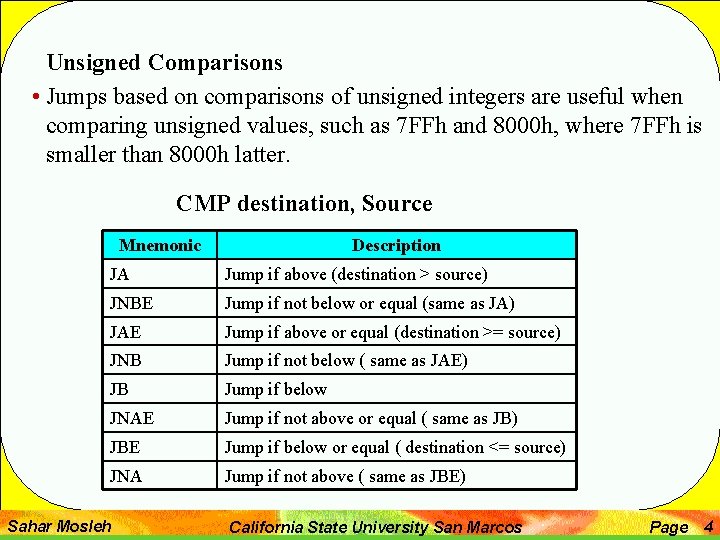
Unsigned Comparisons • Jumps based on comparisons of unsigned integers are useful when comparing unsigned values, such as 7 FFh and 8000 h, where 7 FFh is smaller than 8000 h latter. CMP destination, Source Mnemonic Description JA Jump if above (destination > source) JNBE Jump if not below or equal (same as JA) JAE Jump if above or equal (destination >= source) JNB Jump if not below ( same as JAE) JB Jump if below JNAE Jump if not above or equal ( same as JB) JBE Jump if below or equal ( destination <= source) JNA Jump if not above ( same as JBE) Sahar Mosleh California State University San Marcos Page 4
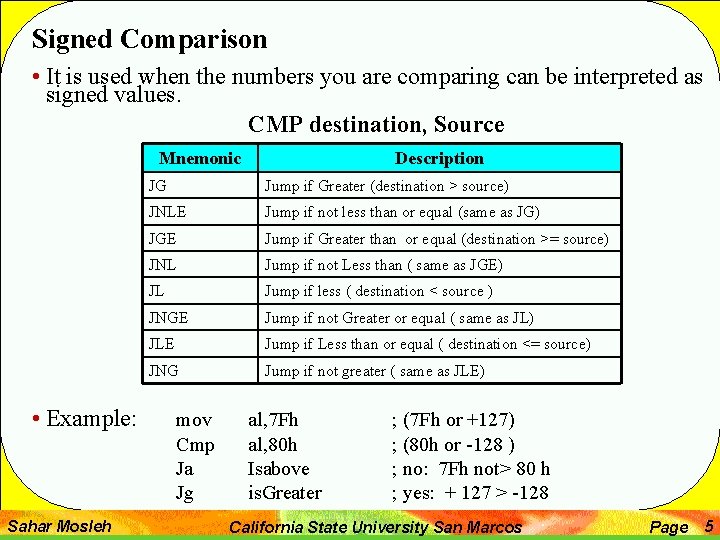
Signed Comparison • It is used when the numbers you are comparing can be interpreted as signed values. CMP destination, Source Mnemonic • Example: Sahar Mosleh Description JG Jump if Greater (destination > source) JNLE Jump if not less than or equal (same as JG) JGE Jump if Greater than or equal (destination >= source) JNL Jump if not Less than ( same as JGE) JL Jump if less ( destination < source ) JNGE Jump if not Greater or equal ( same as JL) JLE Jump if Less than or equal ( destination <= source) JNG Jump if not greater ( same as JLE) mov Cmp Ja Jg al, 7 Fh al, 80 h Isabove is. Greater ; (7 Fh or +127) ; (80 h or -128 ) ; no: 7 Fh not> 80 h ; yes: + 127 > -128 California State University San Marcos Page 5
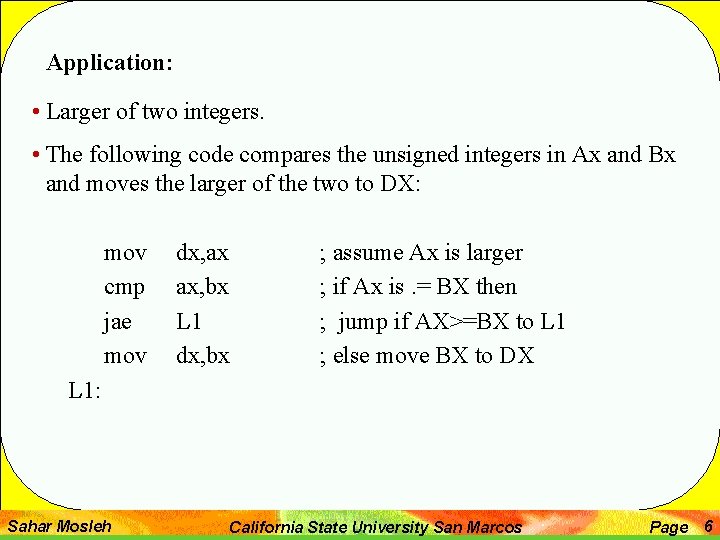
Application: • Larger of two integers. • The following code compares the unsigned integers in Ax and Bx and moves the larger of the two to DX: mov cmp jae mov dx, ax ax, bx L 1 dx, bx ; assume Ax is larger ; if Ax is. = BX then ; jump if AX>=BX to L 1 ; else move BX to DX L 1: Sahar Mosleh California State University San Marcos Page 6
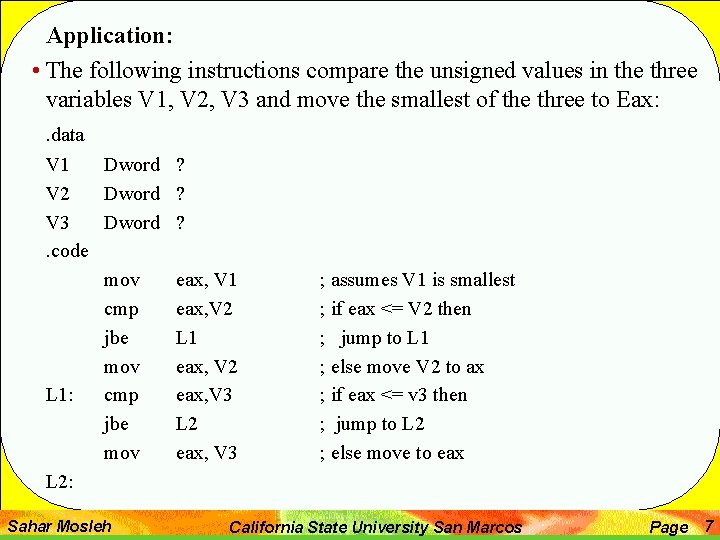
Application: • The following instructions compare the unsigned values in the three variables V 1, V 2, V 3 and move the smallest of the three to Eax: . data V 1 Dword ? V 2 Dword ? V 3 Dword ? . code mov eax, V 1 cmp eax, V 2 jbe L 1 mov eax, V 2 L 1: cmp eax, V 3 jbe L 2 mov eax, V 3 L 2: Sahar Mosleh ; assumes V 1 is smallest ; if eax <= V 2 then ; jump to L 1 ; else move V 2 to ax ; if eax <= v 3 then ; jump to L 2 ; else move to eax California State University San Marcos Page 7
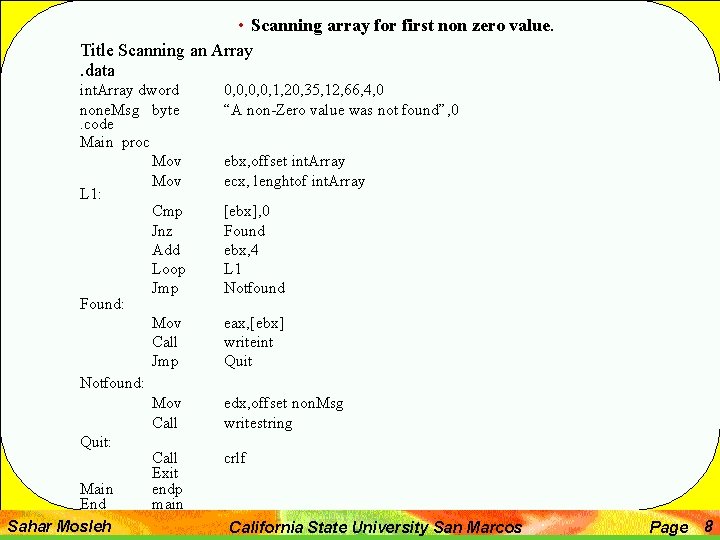
• Scanning array for first non zero value. Title Scanning an Array. data int. Array dword none. Msg byte. code Main proc Mov L 1: Cmp Jnz Add Loop Jmp Found: Mov Call Jmp Notfound: Mov Call Quit: Call Exit Main endp End main Sahar Mosleh 0, 0, 1, 20, 35, 12, 66, 4, 0 “A non-Zero value was not found”, 0 ebx, offset int. Array ecx, lenghtof int. Array [ebx], 0 Found ebx, 4 L 1 Notfound eax, [ebx] writeint Quit edx, offset non. Msg writestring crlf California State University San Marcos Page 8
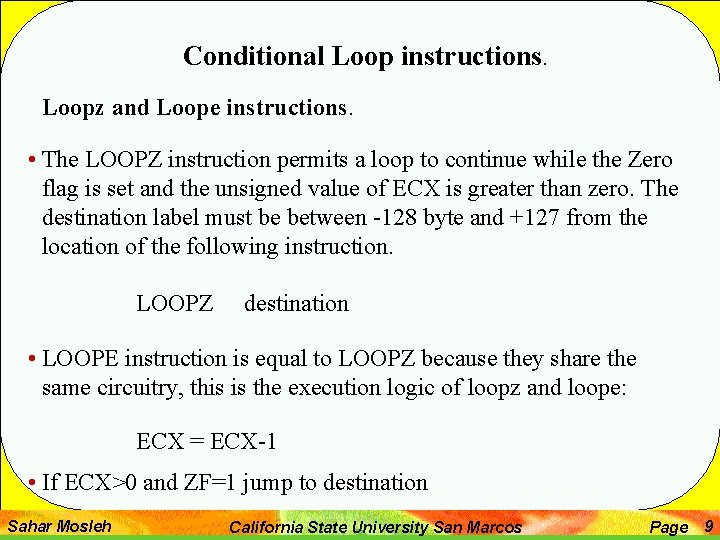
Conditional Loop instructions. Loopz and Loope instructions. • The LOOPZ instruction permits a loop to continue while the Zero flag is set and the unsigned value of ECX is greater than zero. The destination label must be between -128 byte and +127 from the location of the following instruction. LOOPZ destination • LOOPE instruction is equal to LOOPZ because they share the same circuitry, this is the execution logic of loopz and loope: ECX = ECX-1 • If ECX>0 and ZF=1 jump to destination Sahar Mosleh California State University San Marcos Page 9
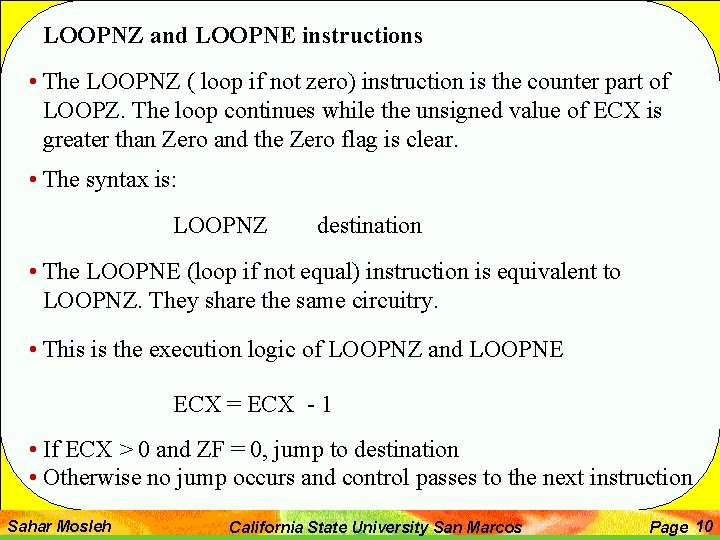
LOOPNZ and LOOPNE instructions • The LOOPNZ ( loop if not zero) instruction is the counter part of LOOPZ. The loop continues while the unsigned value of ECX is greater than Zero and the Zero flag is clear. • The syntax is: LOOPNZ destination • The LOOPNE (loop if not equal) instruction is equivalent to LOOPNZ. They share the same circuitry. • This is the execution logic of LOOPNZ and LOOPNE ECX = ECX - 1 • If ECX > 0 and ZF = 0, jump to destination • Otherwise no jump occurs and control passes to the next instruction Sahar Mosleh California State University San Marcos Page 10
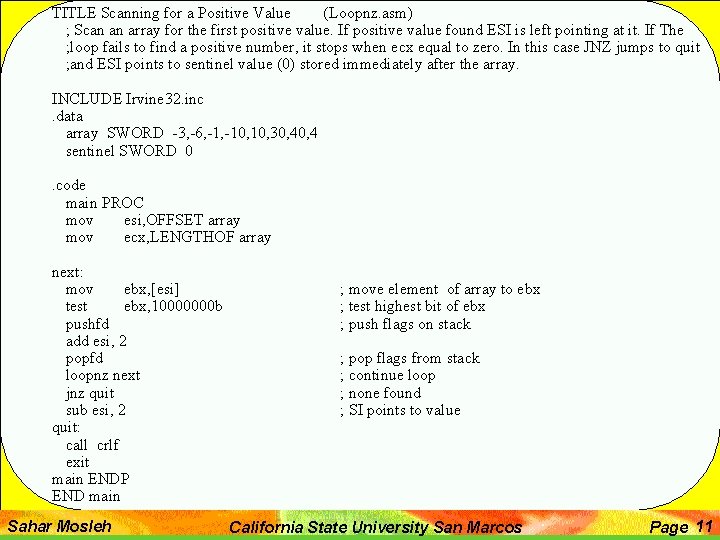
TITLE Scanning for a Positive Value (Loopnz. asm) ; Scan an array for the first positive value. If positive value found ESI is left pointing at it. If The ; loop fails to find a positive number, it stops when ecx equal to zero. In this case JNZ jumps to quit ; and ESI points to sentinel value (0) stored immediately after the array. INCLUDE Irvine 32. inc. data array SWORD -3, -6, -10, 10, 30, 4 sentinel SWORD 0. code main PROC mov esi, OFFSET array mov ecx, LENGTHOF array next: mov ebx, [esi] test ebx, 10000000 b pushfd add esi, 2 popfd loopnz next jnz quit sub esi, 2 quit: call crlf exit main ENDP END main Sahar Mosleh ; move element of array to ebx ; test highest bit of ebx ; push flags on stack ; pop flags from stack ; continue loop ; none found ; SI points to value California State University San Marcos Page 11
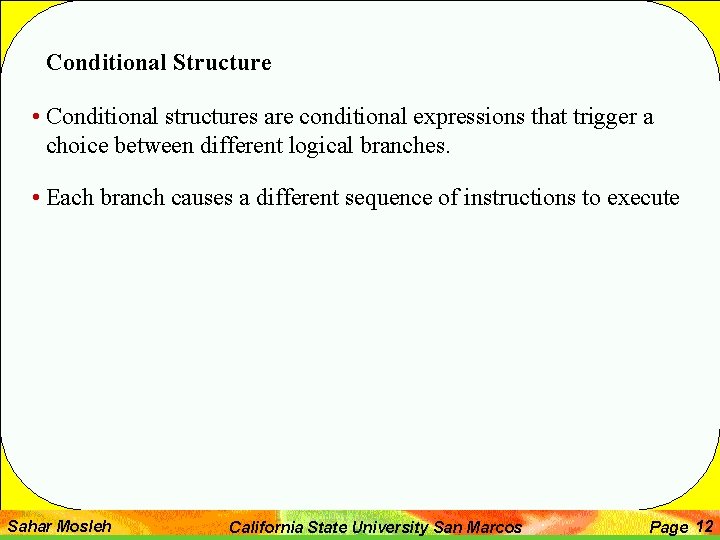
Conditional Structure • Conditional structures are conditional expressions that trigger a choice between different logical branches. • Each branch causes a different sequence of instructions to execute Sahar Mosleh California State University San Marcos Page 12
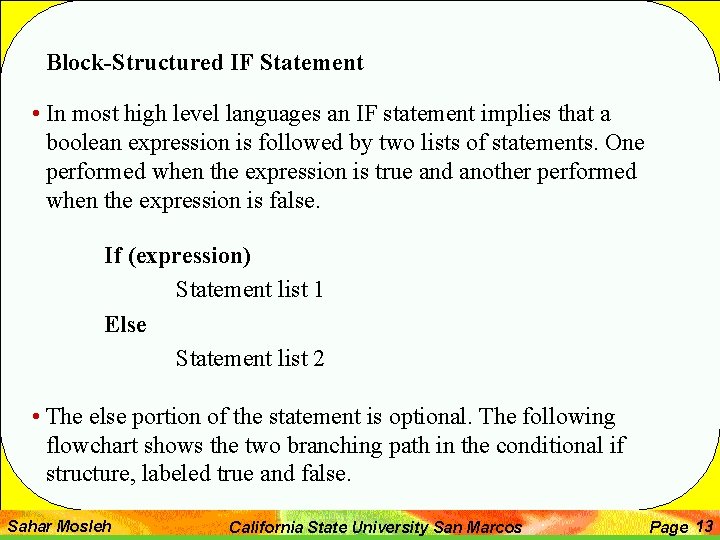
Block-Structured IF Statement • In most high level languages an IF statement implies that a boolean expression is followed by two lists of statements. One performed when the expression is true and another performed when the expression is false. If (expression) Statement list 1 Else Statement list 2 • The else portion of the statement is optional. The following flowchart shows the two branching path in the conditional if structure, labeled true and false. Sahar Mosleh California State University San Marcos Page 13
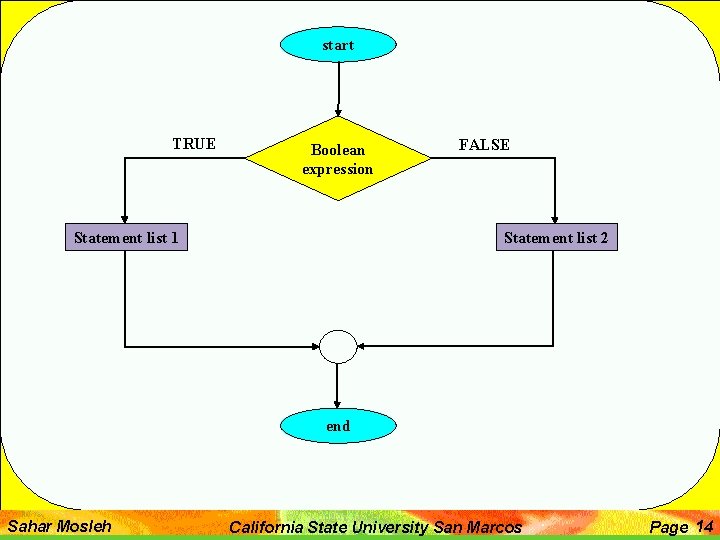
start TRUE Boolean expression Statement list 1 FALSE Statement list 2 end Sahar Mosleh California State University San Marcos Page 14
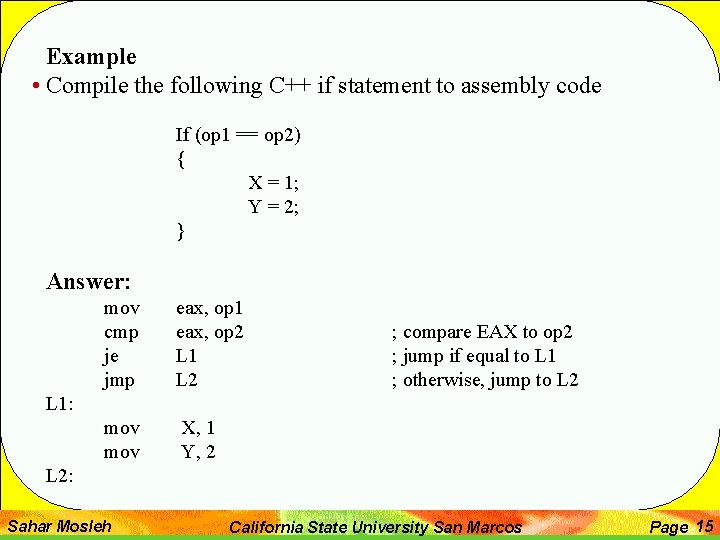
Example • Compile the following C++ if statement to assembly code If (op 1 == op 2) { X = 1; Y = 2; } Answer: mov cmp je jmp eax, op 1 eax, op 2 L 1 L 2 mov X, 1 Y, 2 ; compare EAX to op 2 ; jump if equal to L 1 ; otherwise, jump to L 2 L 1: L 2: Sahar Mosleh California State University San Marcos Page 15
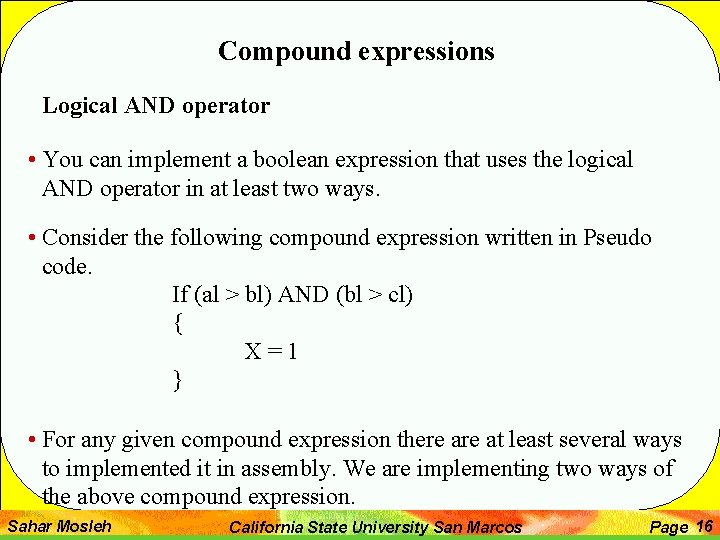
Compound expressions Logical AND operator • You can implement a boolean expression that uses the logical AND operator in at least two ways. • Consider the following compound expression written in Pseudo code. If (al > bl) AND (bl > cl) { X=1 } • For any given compound expression there at least several ways to implemented it in assembly. We are implementing two ways of the above compound expression. Sahar Mosleh California State University San Marcos Page 16
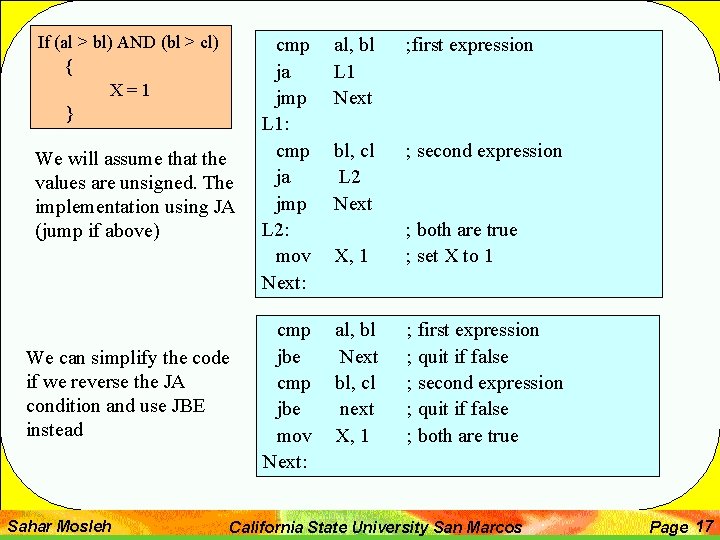
If (al > bl) AND (bl > cl) { X=1 } We will assume that the values are unsigned. The implementation using JA (jump if above) We can simplify the code if we reverse the JA condition and use JBE instead Sahar Mosleh cmp ja jmp L 1: cmp ja jmp L 2: mov Next: al, bl L 1 Next ; first expression bl, cl L 2 Next ; second expression X, 1 ; both are true ; set X to 1 cmp jbe mov Next: al, bl Next bl, cl next X, 1 ; first expression ; quit if false ; second expression ; quit if false ; both are true California State University San Marcos Page 17
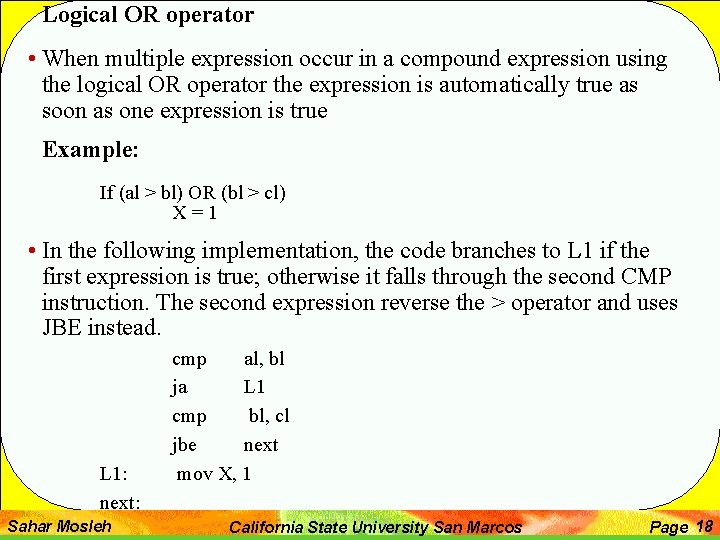
Logical OR operator • When multiple expression occur in a compound expression using the logical OR operator the expression is automatically true as soon as one expression is true Example: If (al > bl) OR (bl > cl) X=1 • In the following implementation, the code branches to L 1 if the first expression is true; otherwise it falls through the second CMP instruction. The second expression reverse the > operator and uses JBE instead. L 1: next: Sahar Mosleh cmp al, bl ja L 1 cmp bl, cl jbe next mov X, 1 California State University San Marcos Page 18
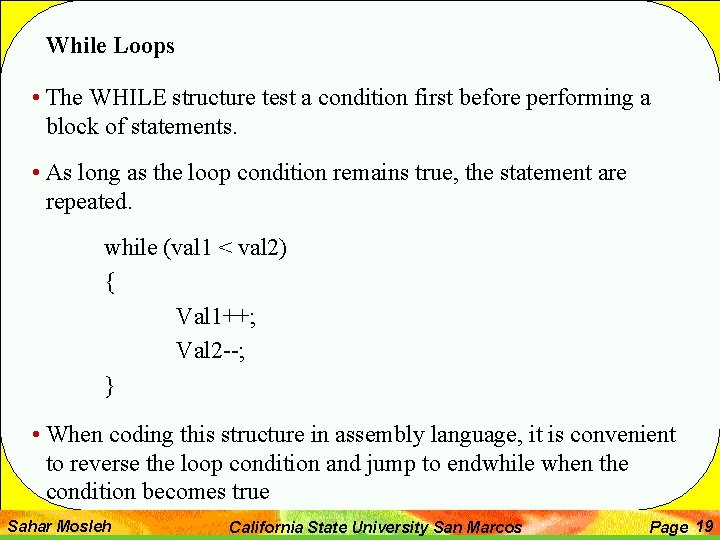
While Loops • The WHILE structure test a condition first before performing a block of statements. • As long as the loop condition remains true, the statement are repeated. while (val 1 < val 2) { Val 1++; Val 2 --; } • When coding this structure in assembly language, it is convenient to reverse the loop condition and jump to endwhile when the condition becomes true Sahar Mosleh California State University San Marcos Page 19
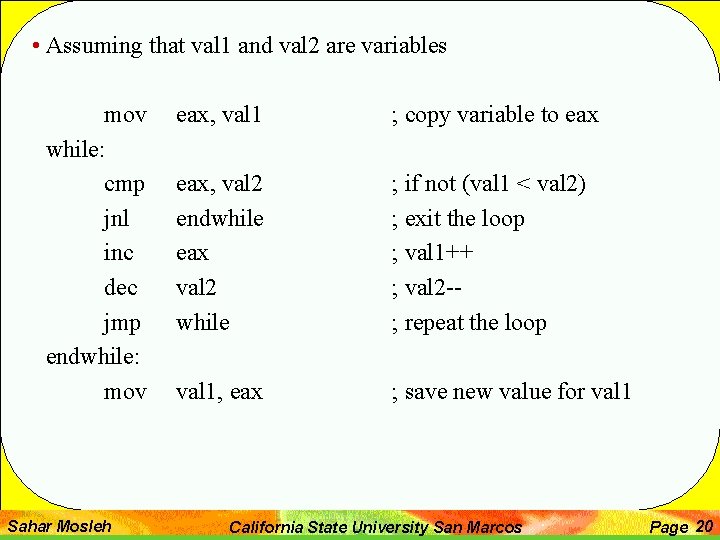
• Assuming that val 1 and val 2 are variables mov while: cmp jnl inc dec jmp endwhile: mov Sahar Mosleh eax, val 1 ; copy variable to eax, val 2 endwhile eax val 2 while ; if not (val 1 < val 2) ; exit the loop ; val 1++ ; val 2 -; repeat the loop val 1, eax ; save new value for val 1 California State University San Marcos Page 20
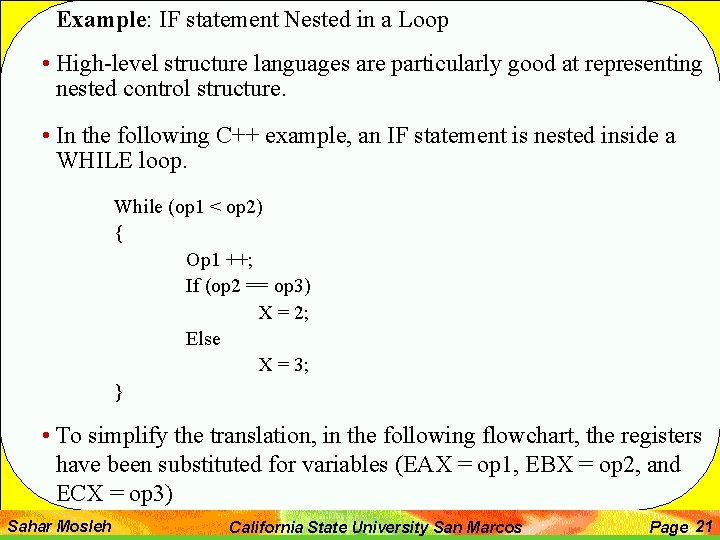
Example: IF statement Nested in a Loop • High-level structure languages are particularly good at representing nested control structure. • In the following C++ example, an IF statement is nested inside a WHILE loop. While (op 1 < op 2) { Op 1 ++; If (op 2 == op 3) X = 2; Else X = 3; } • To simplify the translation, in the following flowchart, the registers have been substituted for variables (EAX = op 1, EBX = op 2, and ECX = op 3) Sahar Mosleh California State University San Marcos Page 21
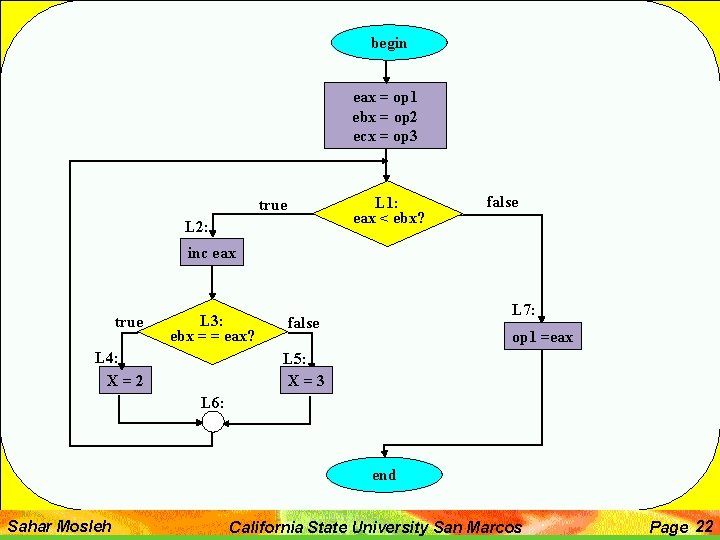
begin eax = op 1 ebx = op 2 ecx = op 3 L 1: eax < ebx? true L 2: false inc eax true L 3: ebx = = eax? L 4: X=2 L 7: false op 1 =eax L 5: X=3 L 6: end Sahar Mosleh California State University San Marcos Page 22
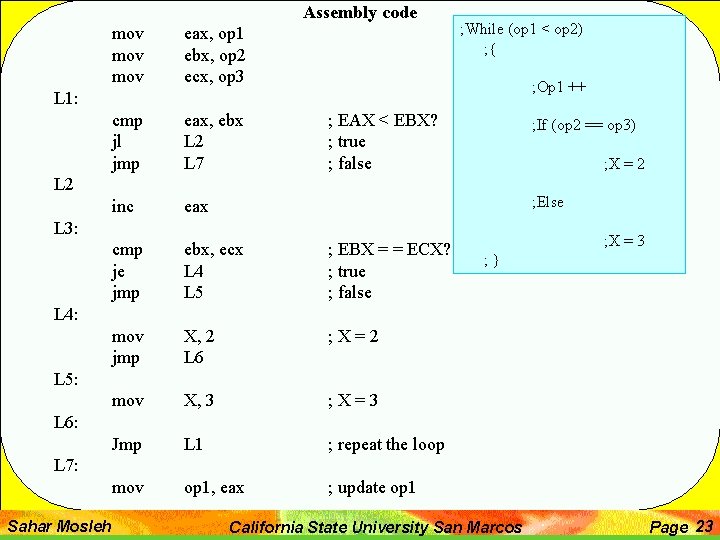
Assembly code mov mov eax, op 1 ebx, op 2 ecx, op 3 cmp jl jmp eax, ebx L 2 L 7 inc eax cmp je jmp ebx, ecx L 4 L 5 ; EBX = = ECX? ; true ; false mov jmp X, 2 L 6 ; X=2 mov X, 3 ; X=3 Jmp L 1 ; repeat the loop mov op 1, eax ; update op 1 ; While (op 1 < op 2) ; { ; Op 1 ++ L 1: ; EAX < EBX? ; true ; false ; If (op 2 == op 3) ; X = 2 L 2 ; Else L 3: ; X = 3 ; } L 4: L 5: L 6: L 7: Sahar Mosleh California State University San Marcos Page 23