CS 177 Week 2 Recitation Primitive data types
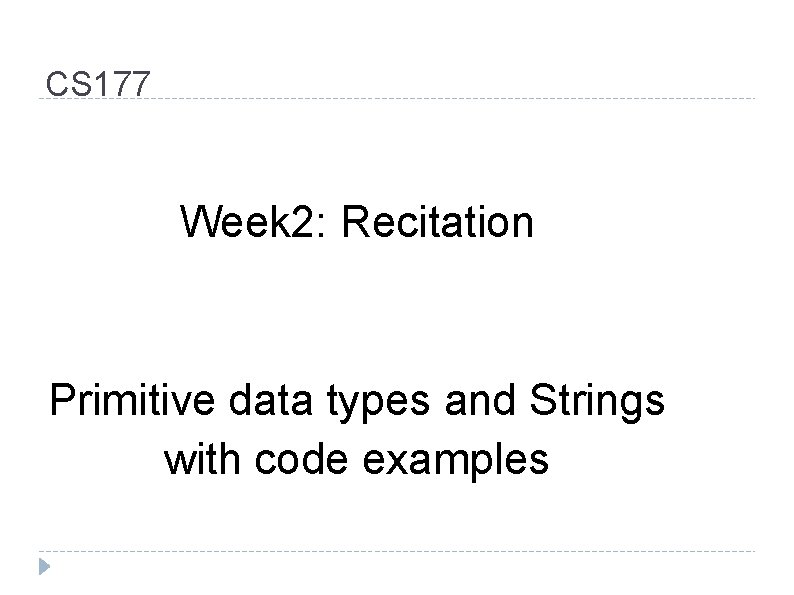
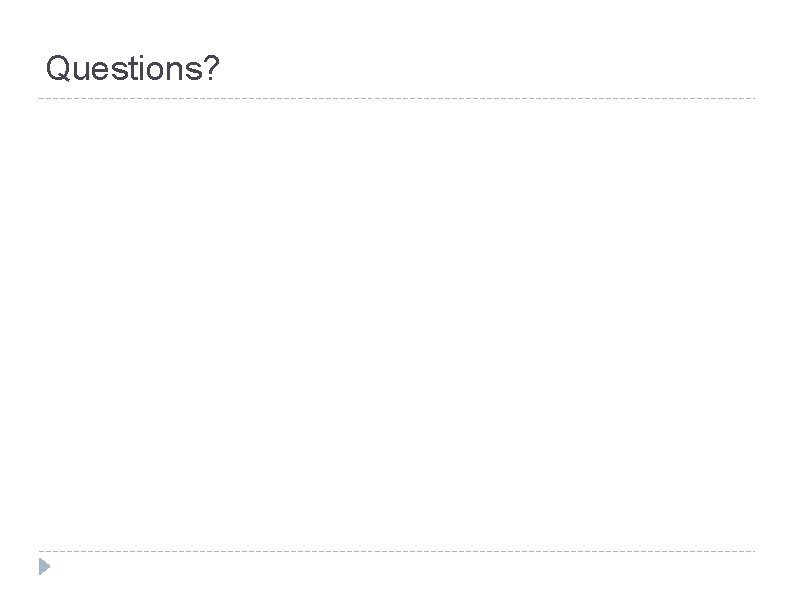
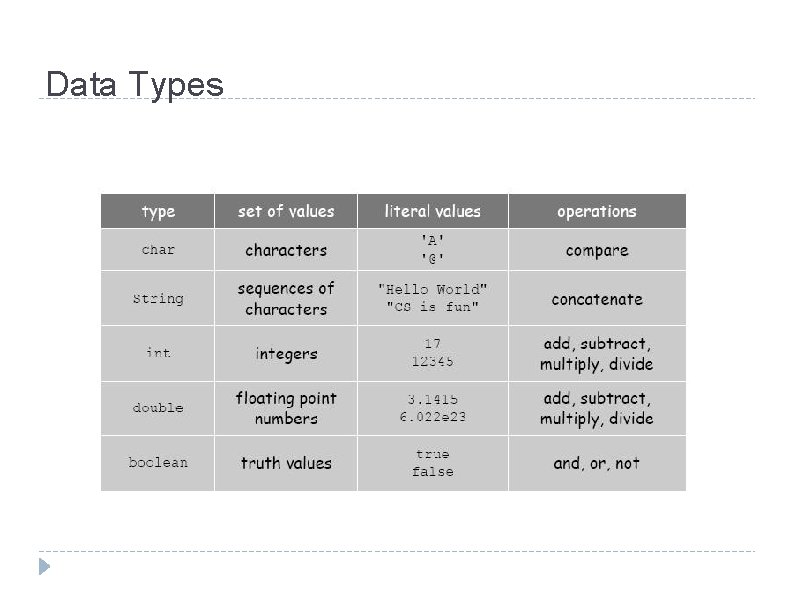
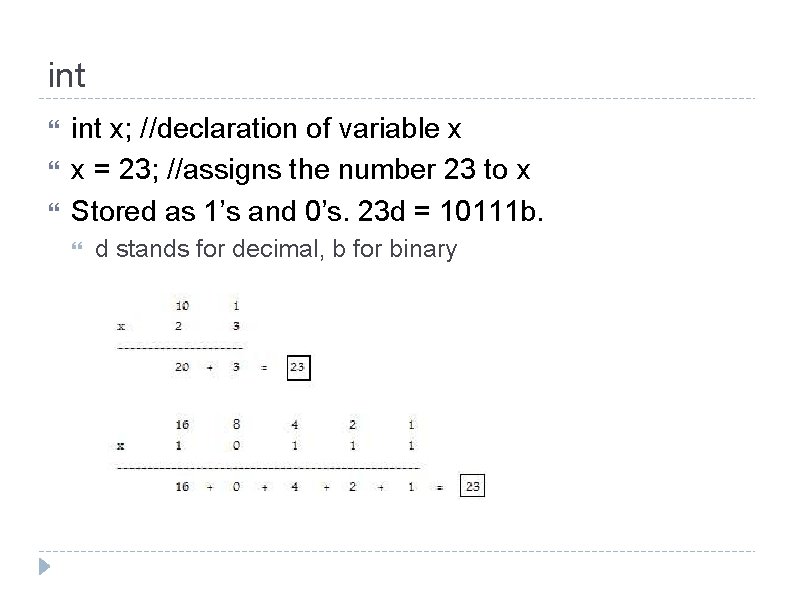
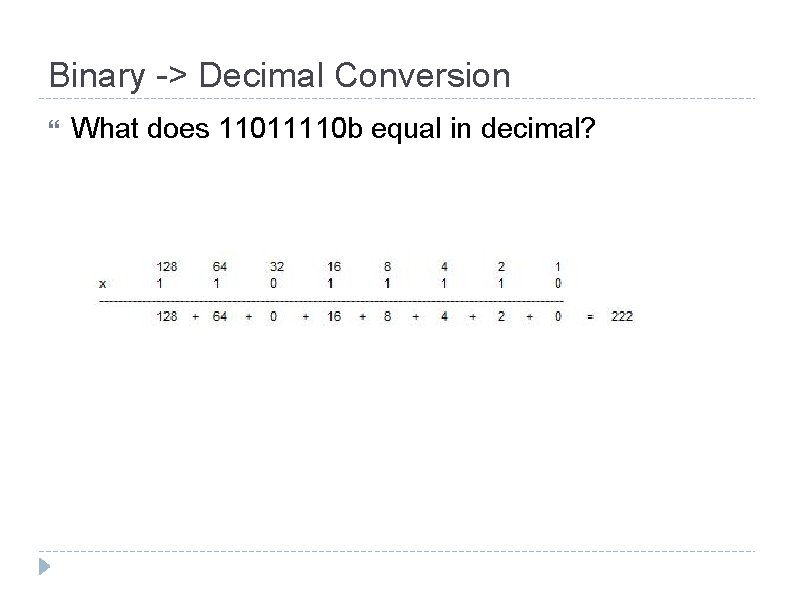
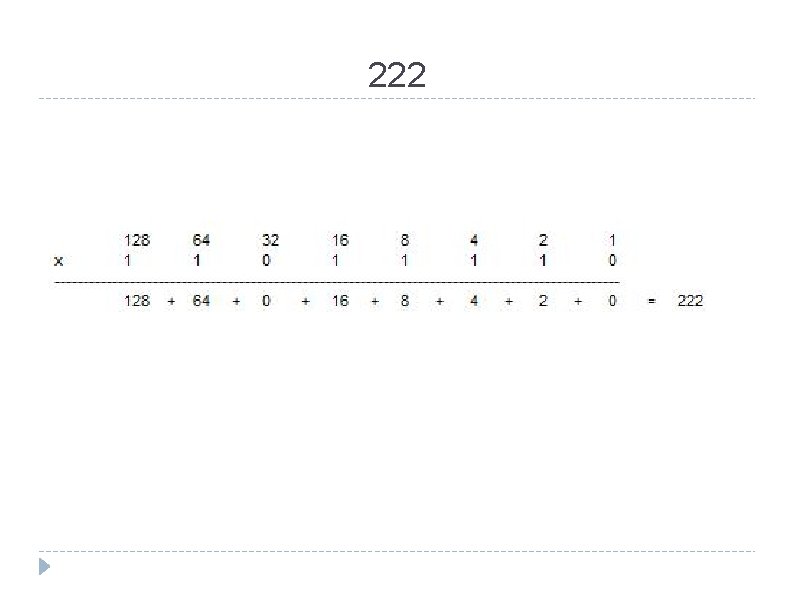
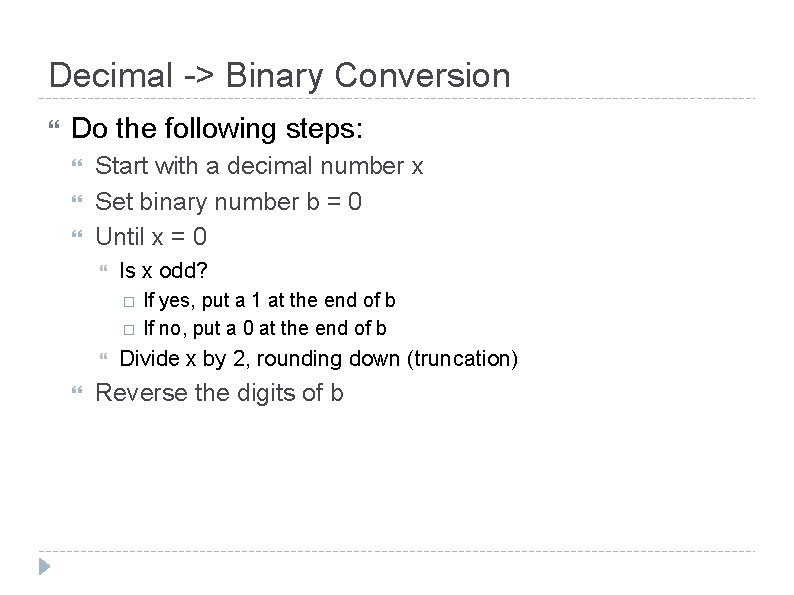
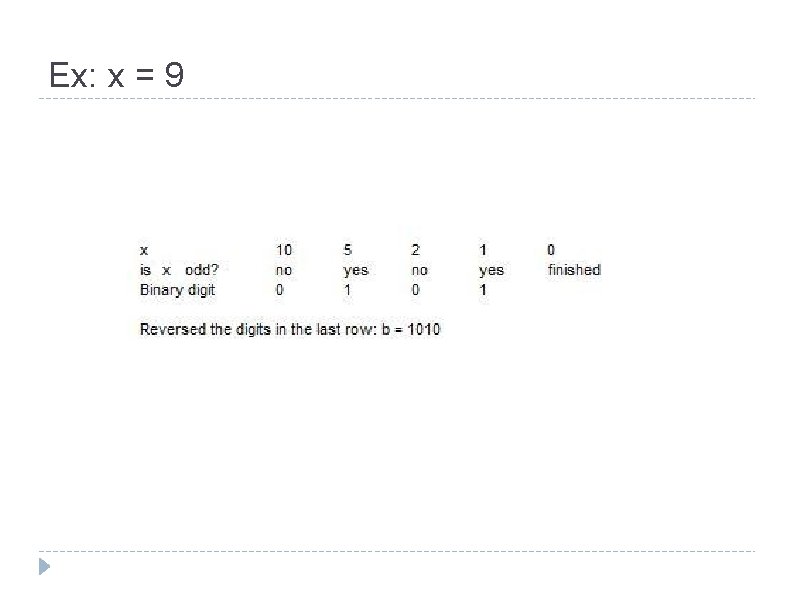
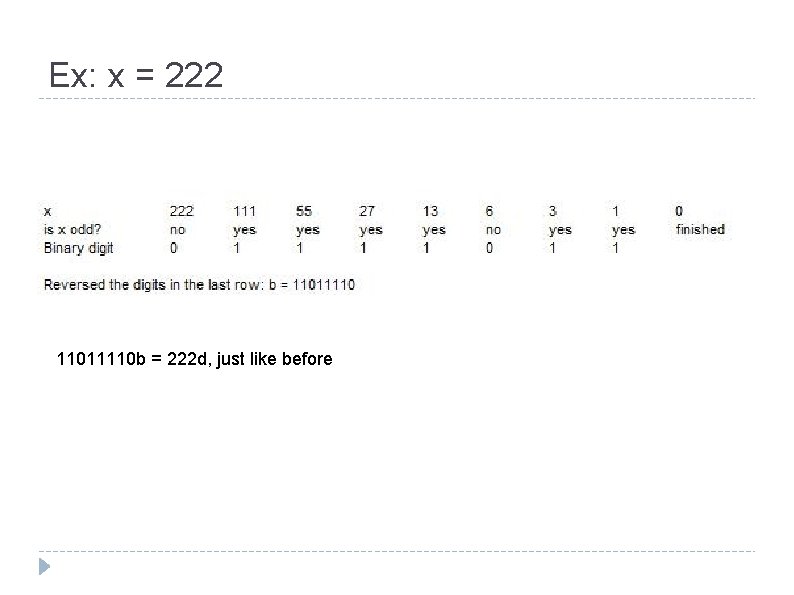
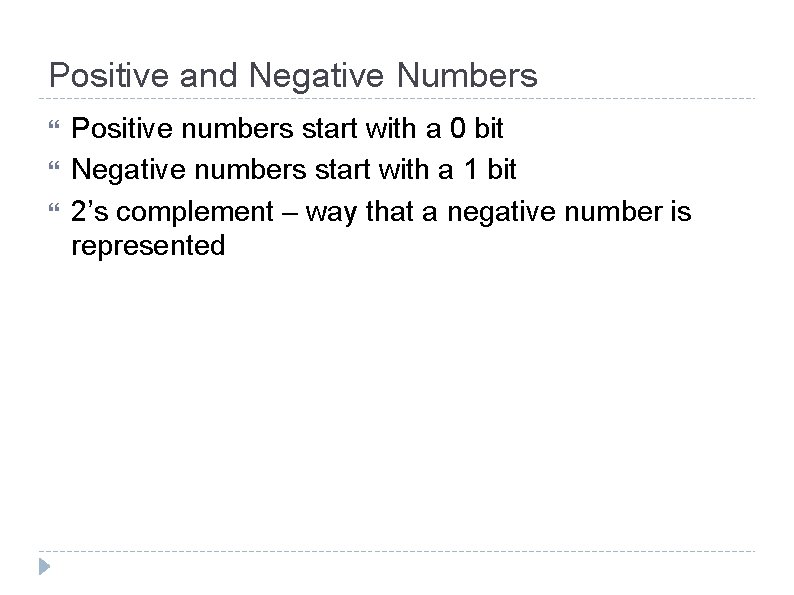
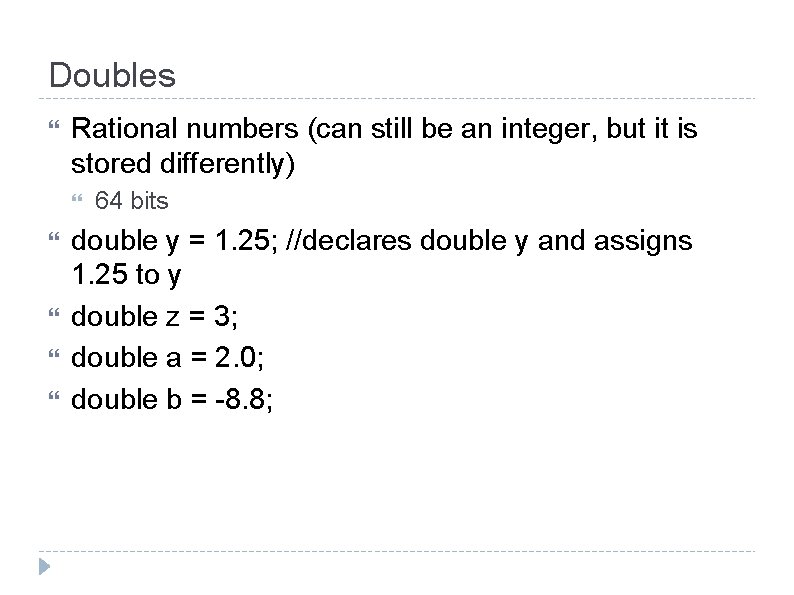
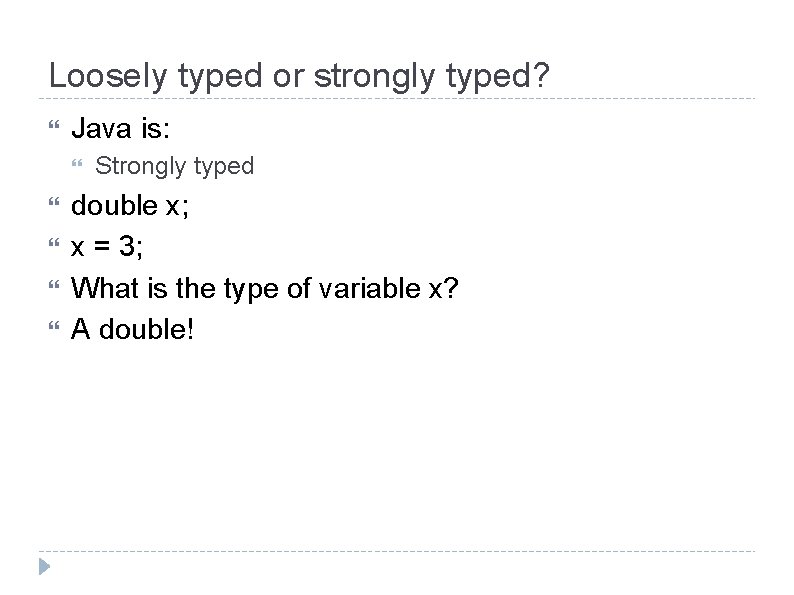
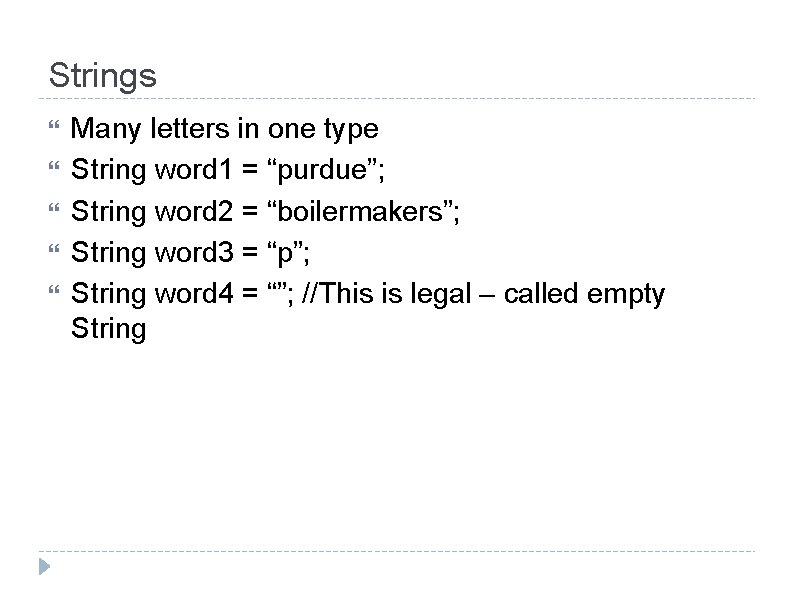
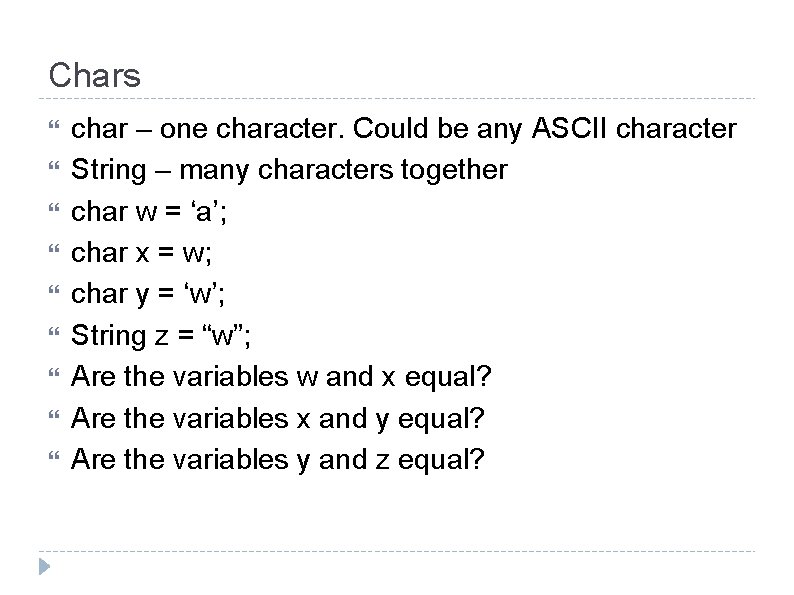
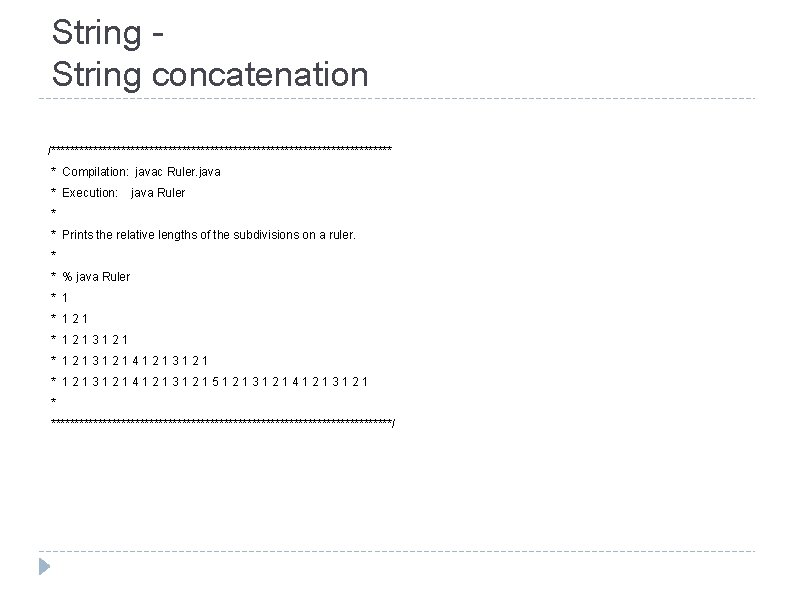
![public class Ruler { public static void main(String[] args) { String ruler 1 = public class Ruler { public static void main(String[] args) { String ruler 1 =](https://slidetodoc.com/presentation_image/21c8093f457b5e833fcdf5ae032283bc/image-16.jpg)
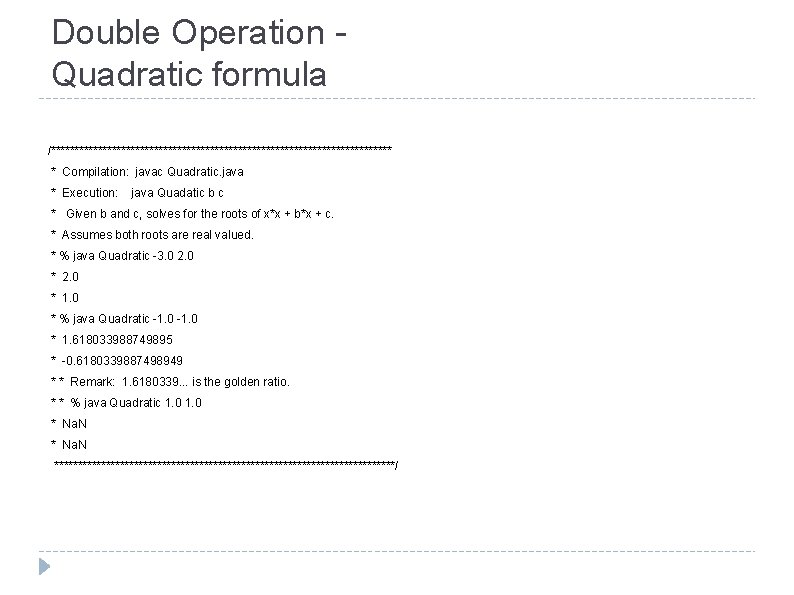
![public class Quadratic { public static void main(String[] args) { double b = Double. public class Quadratic { public static void main(String[] args) { double b = Double.](https://slidetodoc.com/presentation_image/21c8093f457b5e833fcdf5ae032283bc/image-18.jpg)
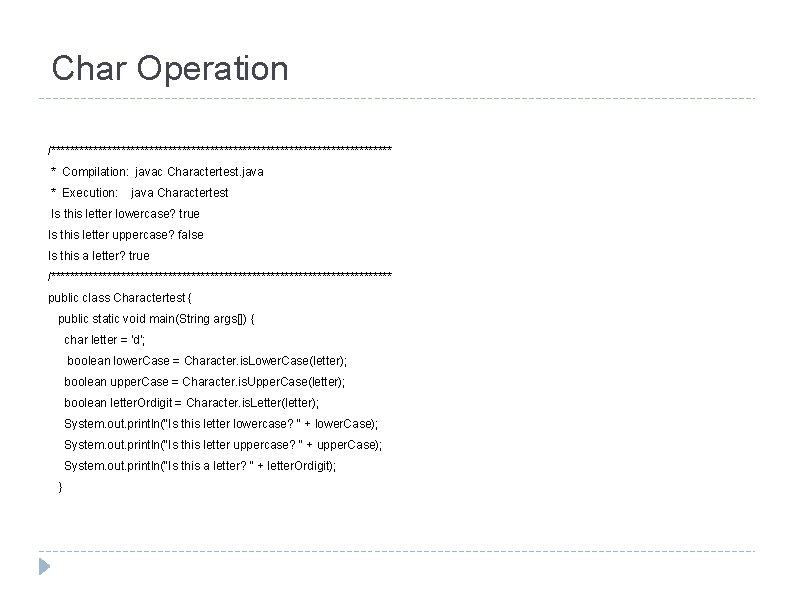
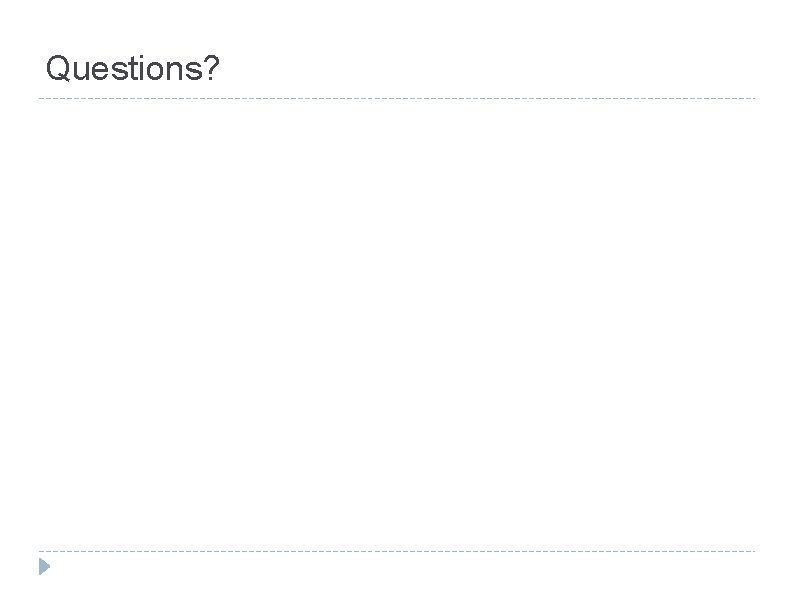
- Slides: 20
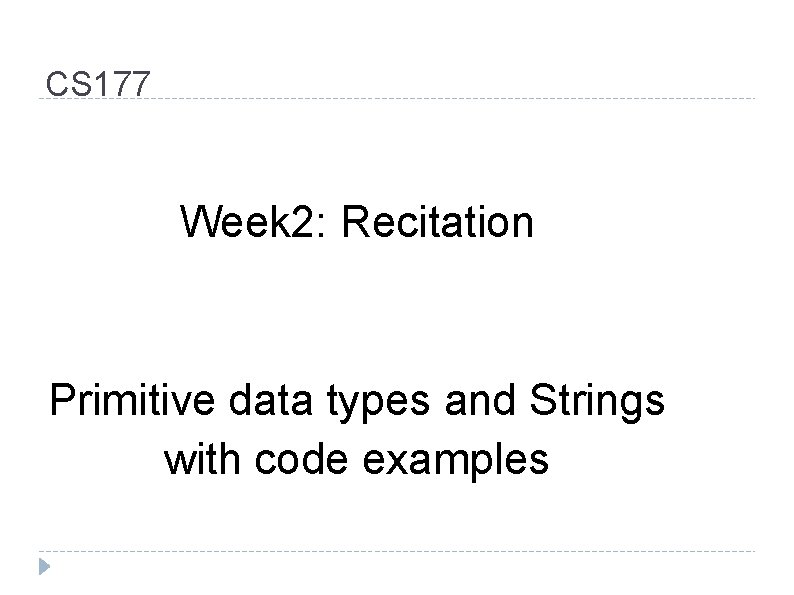
CS 177 Week 2: Recitation Primitive data types and Strings with code examples
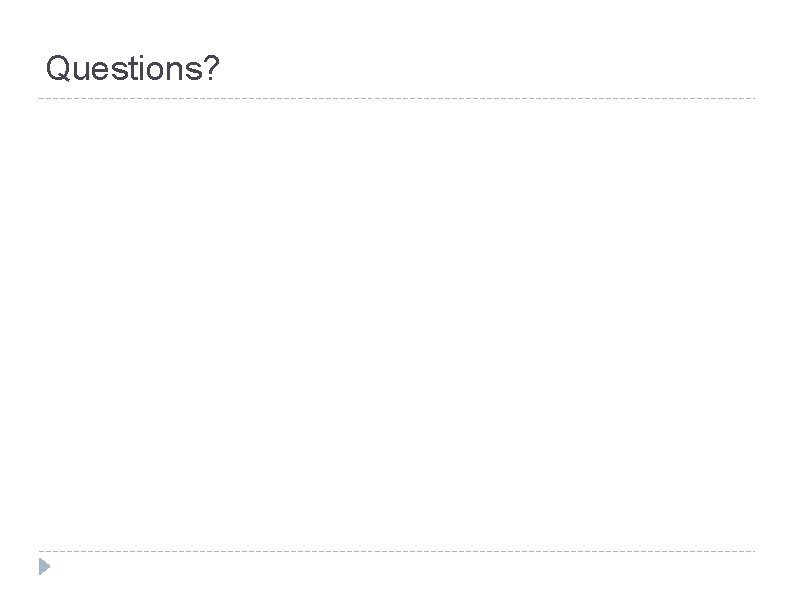
Questions?
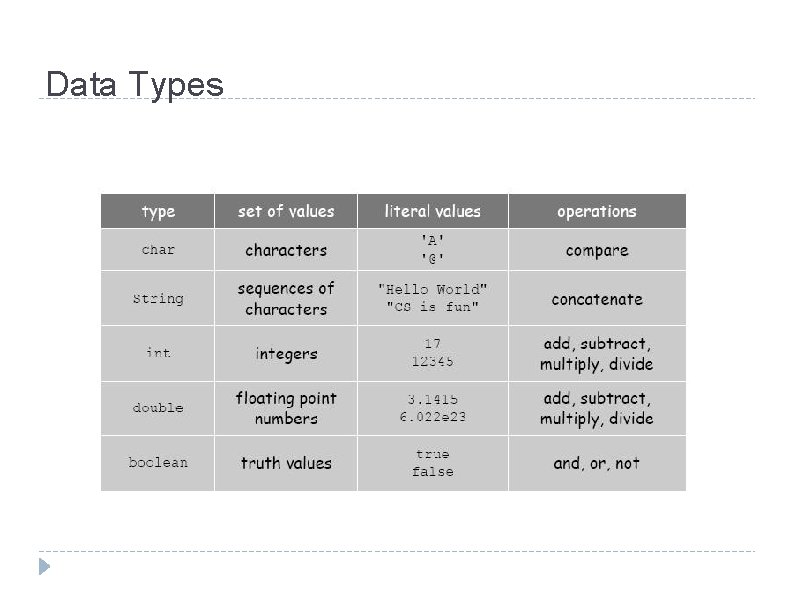
Data Types
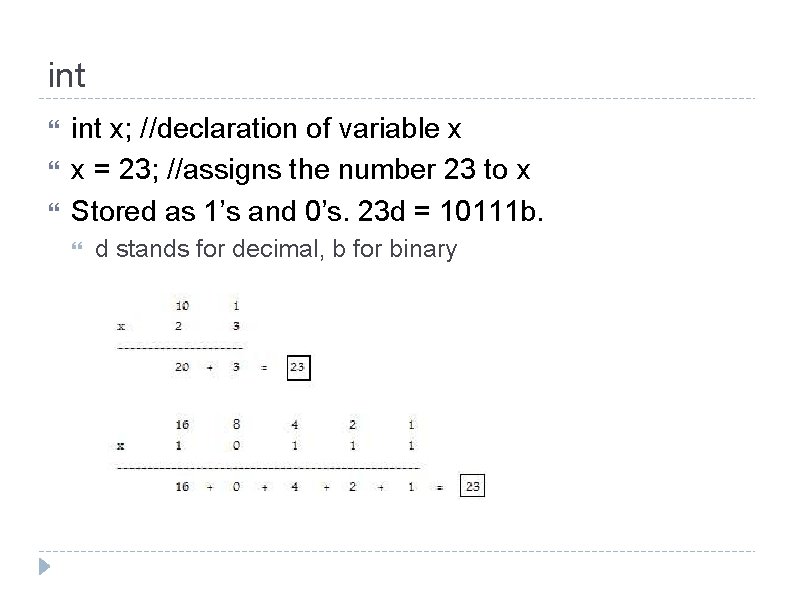
int x; //declaration of variable x x = 23; //assigns the number 23 to x Stored as 1’s and 0’s. 23 d = 10111 b. d stands for decimal, b for binary
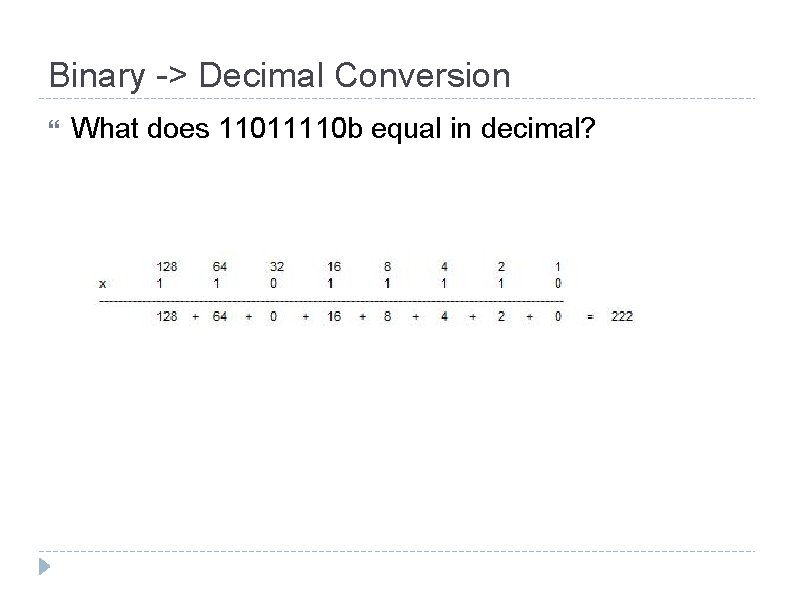
Binary -> Decimal Conversion What does 11011110 b equal in decimal?
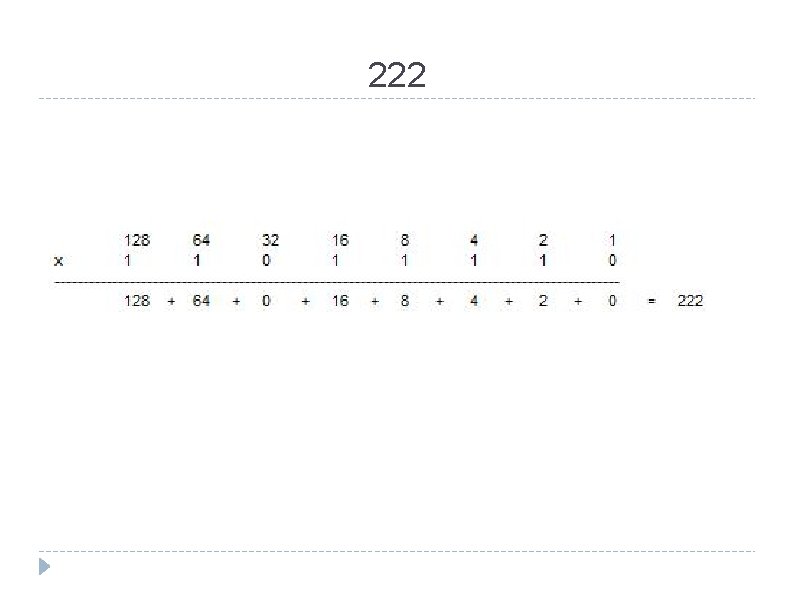
222
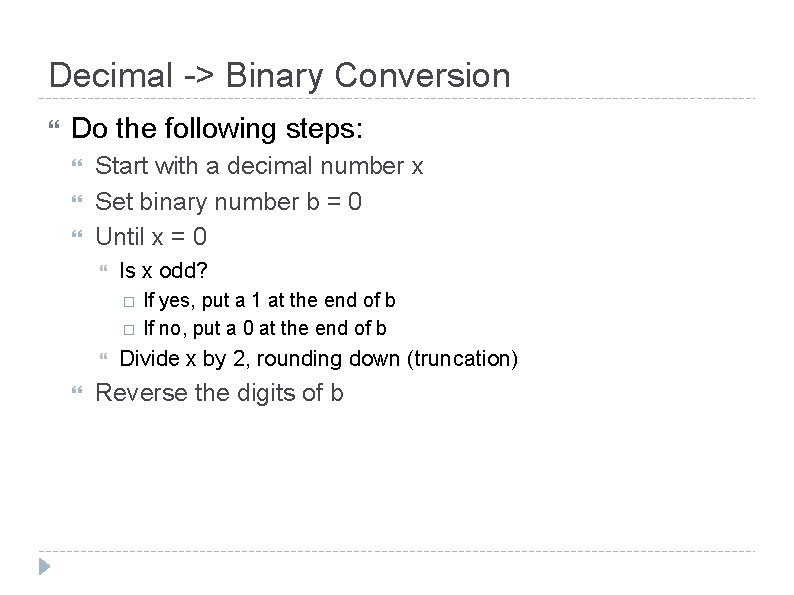
Decimal -> Binary Conversion Do the following steps: Start with a decimal number x Set binary number b = 0 Until x = 0 Is x odd? If yes, put a 1 at the end of b If no, put a 0 at the end of b Divide x by 2, rounding down (truncation) Reverse the digits of b
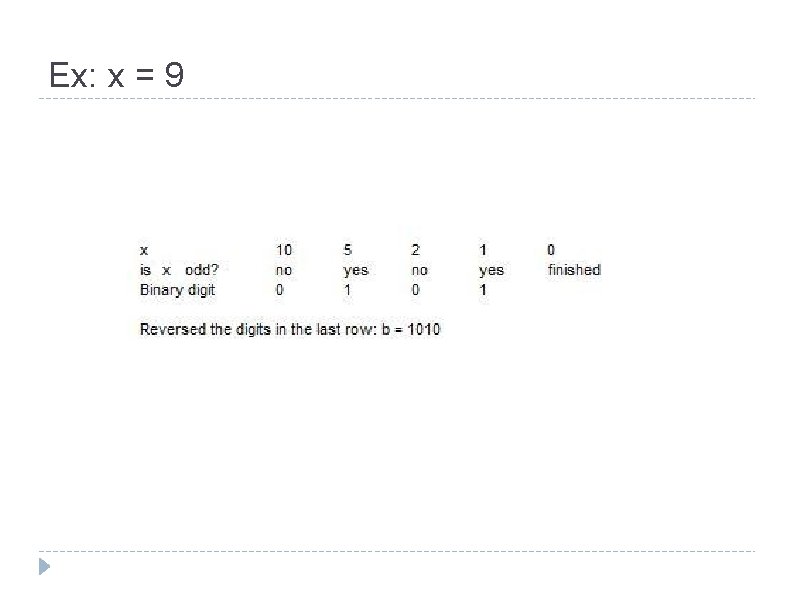
Ex: x = 9
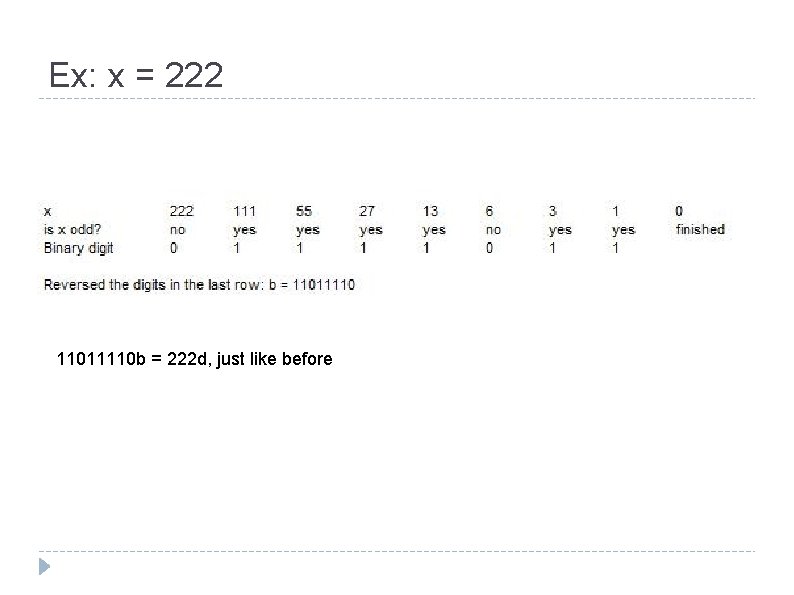
Ex: x = 222 11011110 b = 222 d, just like before
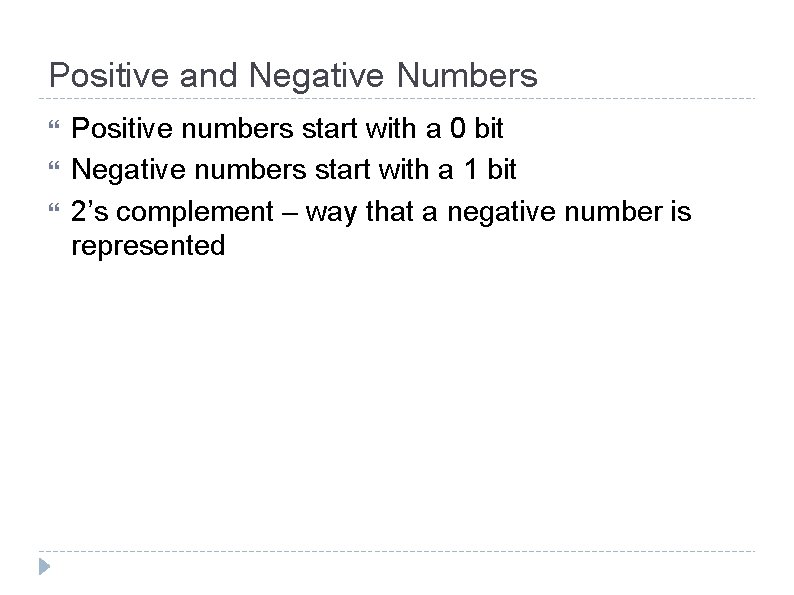
Positive and Negative Numbers Positive numbers start with a 0 bit Negative numbers start with a 1 bit 2’s complement – way that a negative number is represented
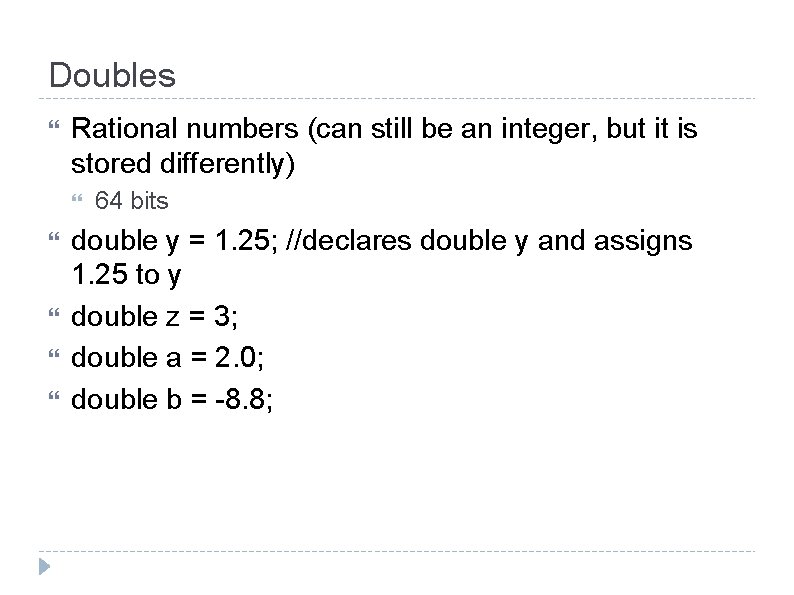
Doubles Rational numbers (can still be an integer, but it is stored differently) 64 bits double y = 1. 25; //declares double y and assigns 1. 25 to y double z = 3; double a = 2. 0; double b = -8. 8;
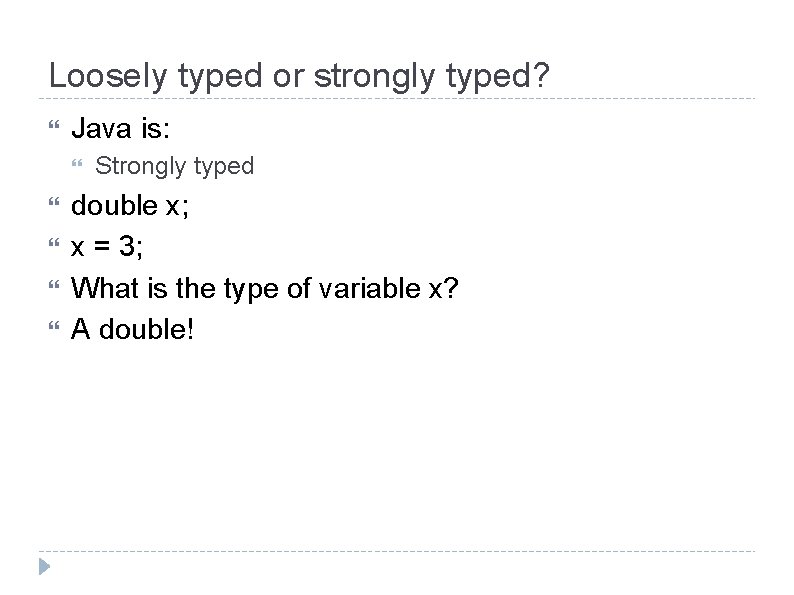
Loosely typed or strongly typed? Java is: Strongly typed double x; x = 3; What is the type of variable x? A double!
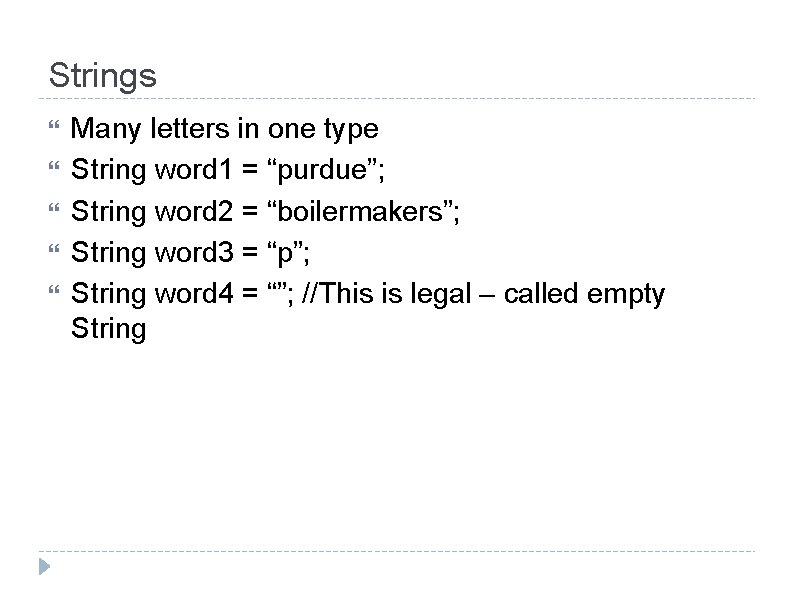
Strings Many letters in one type String word 1 = “purdue”; String word 2 = “boilermakers”; String word 3 = “p”; String word 4 = “”; //This is legal – called empty String
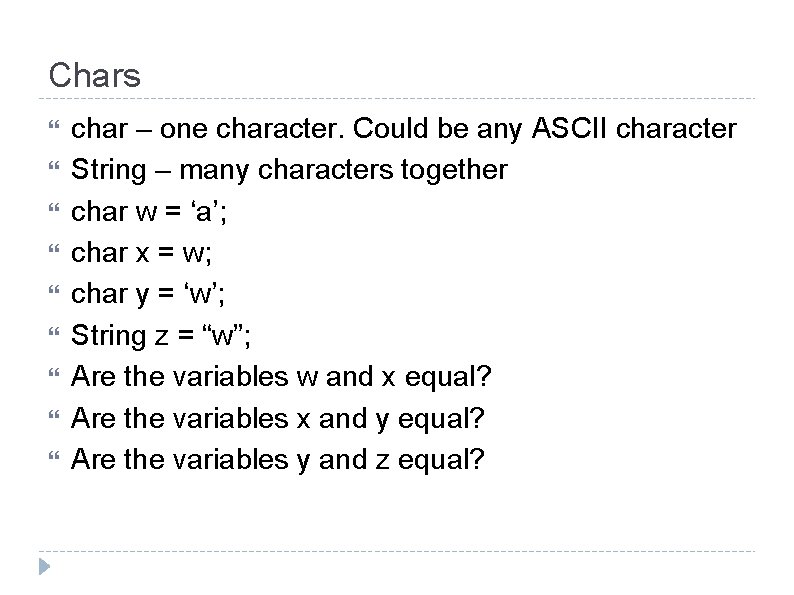
Chars char – one character. Could be any ASCII character String – many characters together char w = ‘a’; char x = w; char y = ‘w’; String z = “w”; Are the variables w and x equal? Are the variables x and y equal? Are the variables y and z equal?
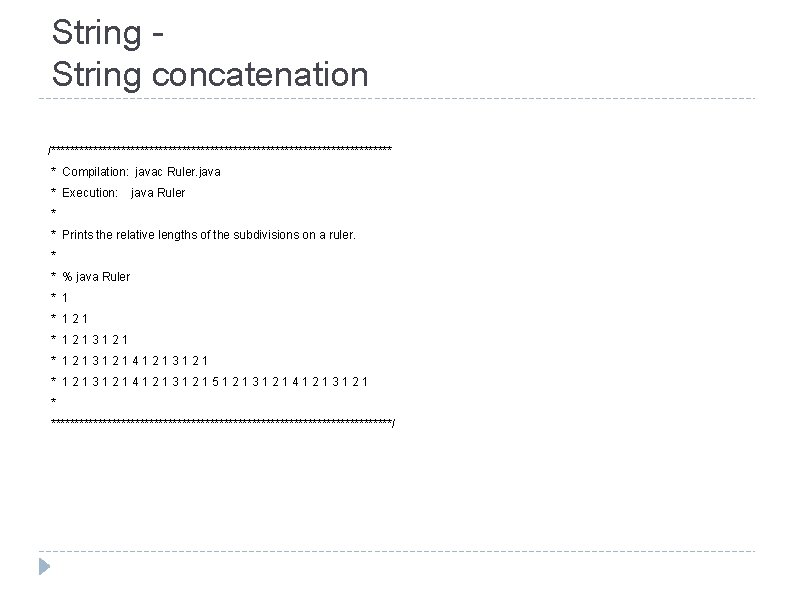
String concatenation /************************************* * Compilation: javac Ruler. java * Execution: java Ruler * * Prints the relative lengths of the subdivisions on a ruler. * * % java Ruler * 121312141213121 * 1213121412131215121312141213121 * *************************************/
![public class Ruler public static void mainString args String ruler 1 public class Ruler { public static void main(String[] args) { String ruler 1 =](https://slidetodoc.com/presentation_image/21c8093f457b5e833fcdf5ae032283bc/image-16.jpg)
public class Ruler { public static void main(String[] args) { String ruler 1 = "1 "; String ruler 2 = ruler 1 + "2 " + ruler 1; String ruler 3 = ruler 2 + "3 " + ruler 2; String ruler 4 = ruler 3 + "4 " + ruler 3; String ruler 5 = ruler 4 + "5 " + ruler 4; System. out. println(ruler 1); System. out. println(ruler 2); System. out. println(ruler 3); System. out. println(ruler 4); System. out. println(ruler 5); } }
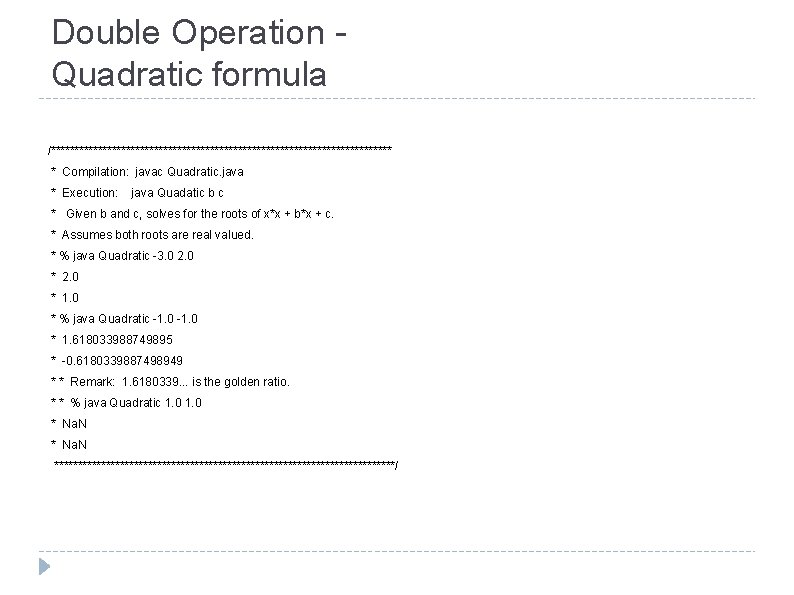
Double Operation Quadratic formula /************************************* * Compilation: javac Quadratic. java * Execution: java Quadatic b c * Given b and c, solves for the roots of x*x + b*x + c. * Assumes both roots are real valued. * % java Quadratic -3. 0 2. 0 * 1. 0 * % java Quadratic -1. 0 * 1. 618033988749895 * -0. 6180339887498949 * * Remark: 1. 6180339. . . is the golden ratio. * * % java Quadratic 1. 0 * Na. N *************************************/
![public class Quadratic public static void mainString args double b Double public class Quadratic { public static void main(String[] args) { double b = Double.](https://slidetodoc.com/presentation_image/21c8093f457b5e833fcdf5ae032283bc/image-18.jpg)
public class Quadratic { public static void main(String[] args) { double b = Double. parse. Double(args[0]); double c = Double. parse. Double(args[1]); double discriminant = b*b - 4. 0*c; double sqroot = Math. sqrt(discriminant); double root 1 = (-b + sqroot) / 2. 0; double root 2 = (-b - sqroot) / 2. 0; System. out. println(root 1); System. out. println(root 2); } }
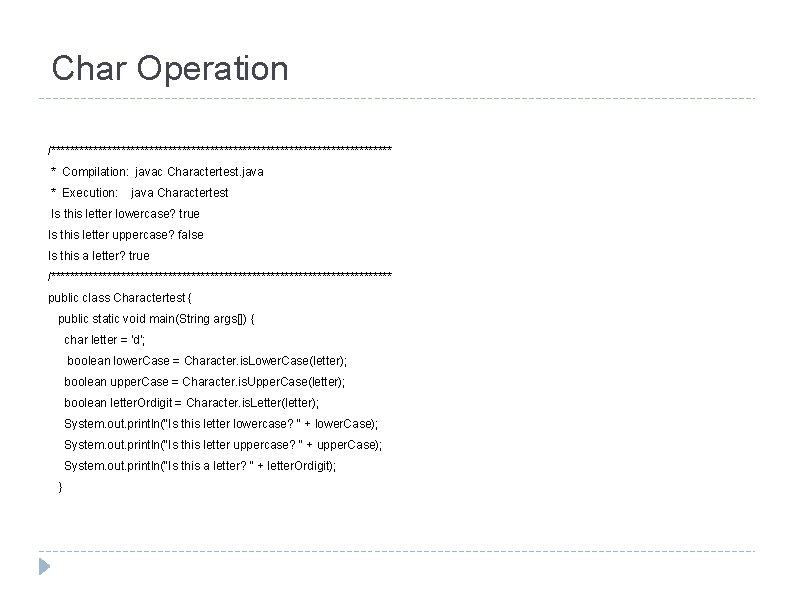
Char Operation /************************************* * Compilation: javac Charactertest. java * Execution: java Charactertest Is this letter lowercase? true Is this letter uppercase? false Is this a letter? true /************************************* public class Charactertest { public static void main(String args[]) { char letter = 'd'; boolean lower. Case = Character. is. Lower. Case(letter); boolean upper. Case = Character. is. Upper. Case(letter); boolean letter. Ordigit = Character. is. Letter(letter); System. out. println("Is this letter lowercase? " + lower. Case); System. out. println("Is this letter uppercase? " + upper. Case); System. out. println("Is this a letter? " + letter. Ordigit); }
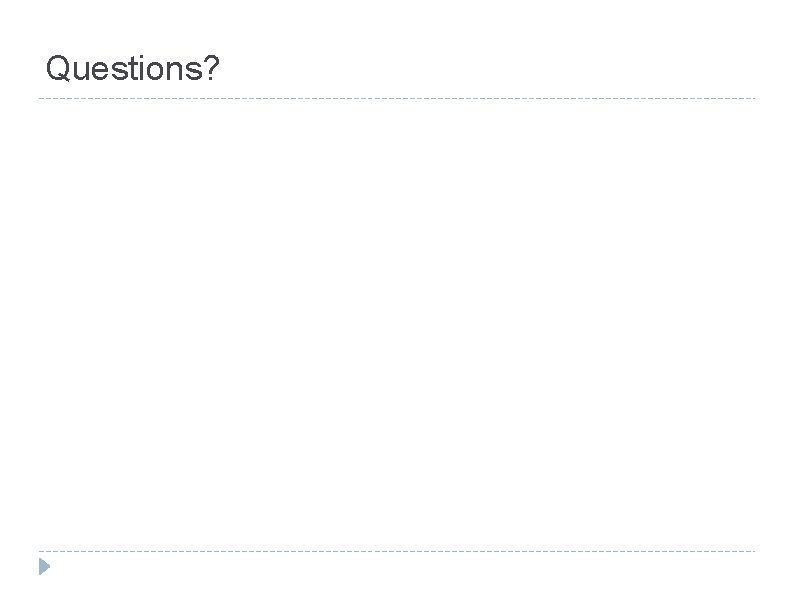
Questions?
Difference between primitive and non primitive data types
Data structures
Passive recitation
Quood posture 8 in english
Rote recitation of a written message
Learning objectives of poem recitation
Know your material
What is meant by etiquette of recitation of the holy quran
Xvvvxv
Product-oriented rubric sample
Récitation les hiboux
Week by week plans for documenting children's development
Primitive vs reference types java
Unit 1 primitive types
Non primitive data structure
Liz owns stock in nar heating/cooling
Pkj 177 aku tuhan semesta
Lu-177 decay
Lu-177 decay
Cs 177
177 rcacs