Primitive Data Types There are exactly eight primitive
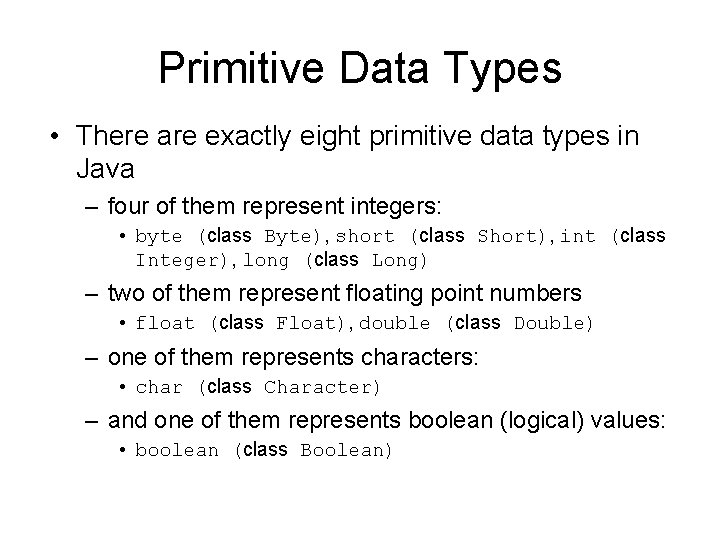
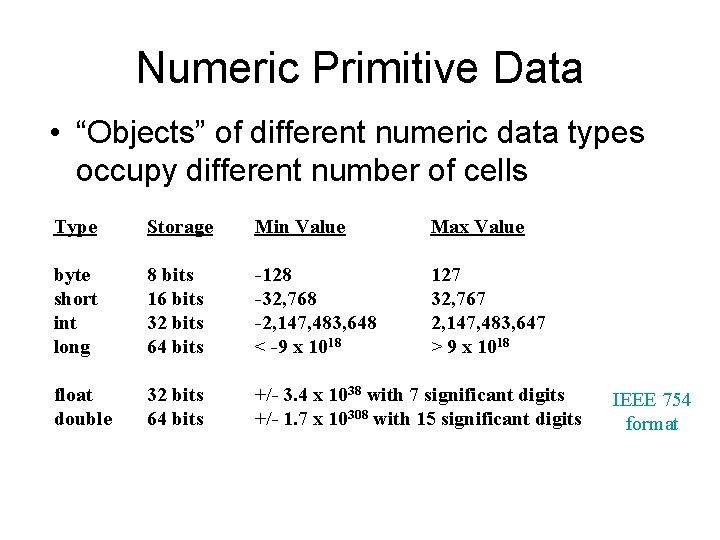
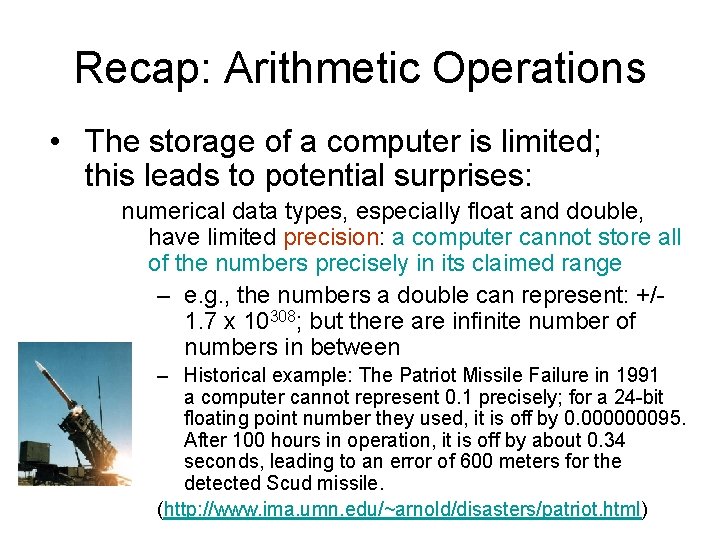
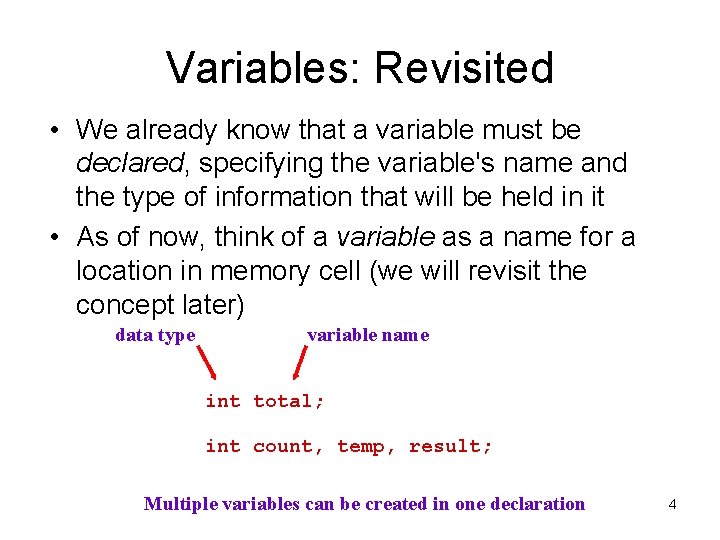
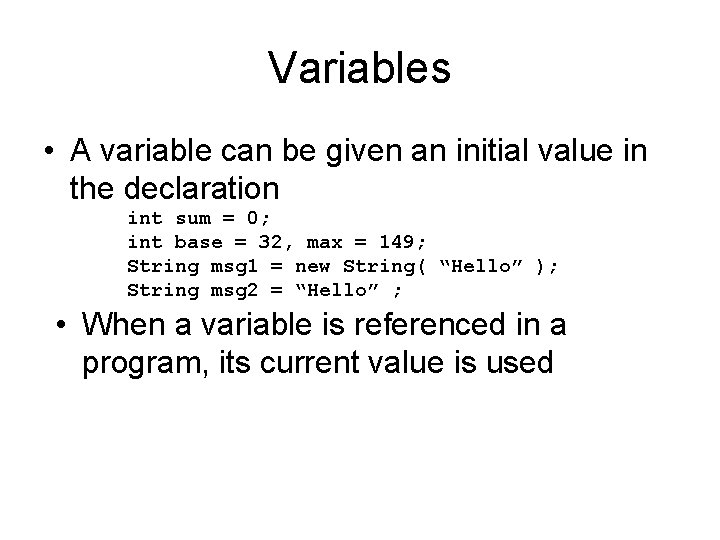
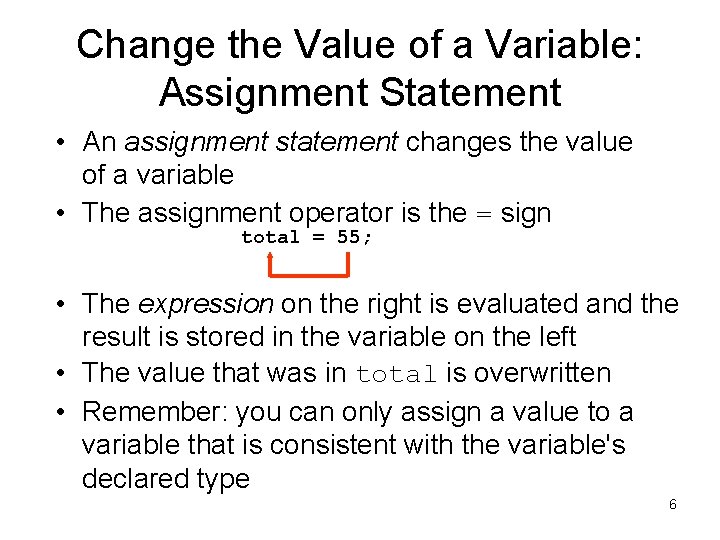
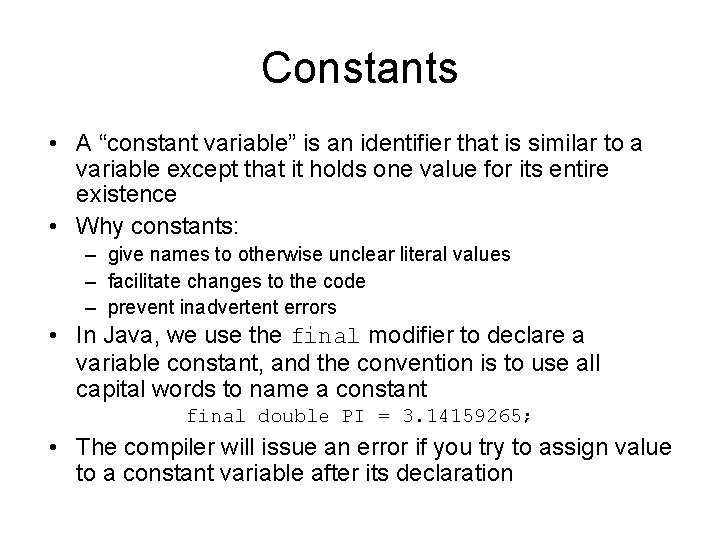
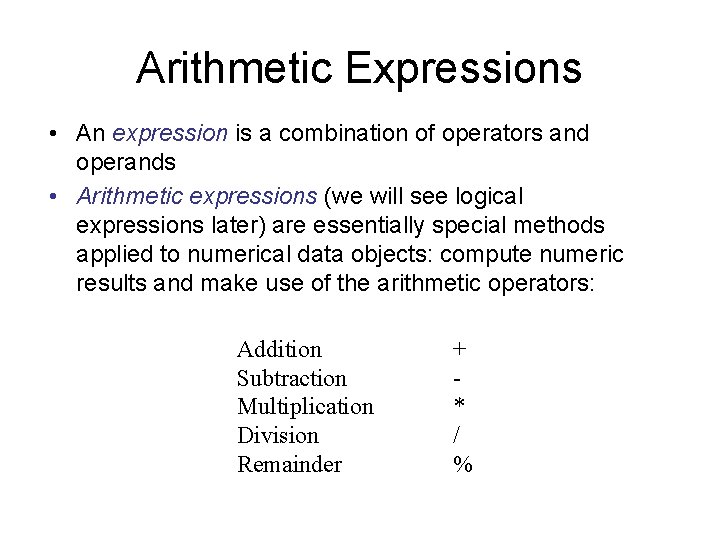
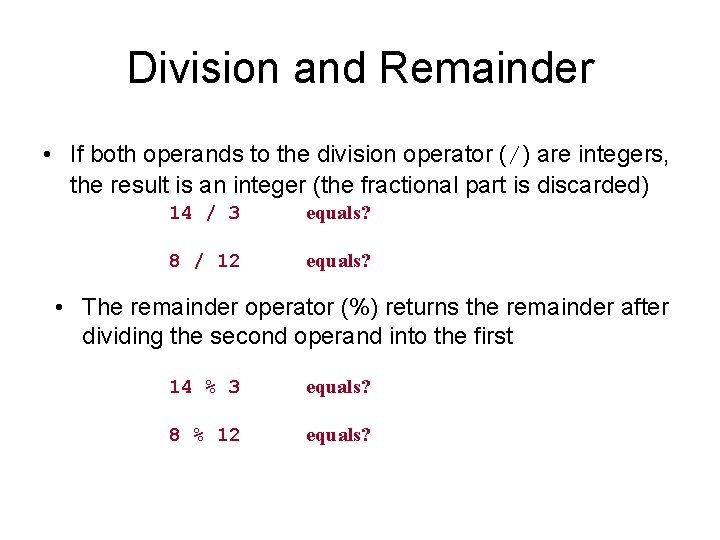
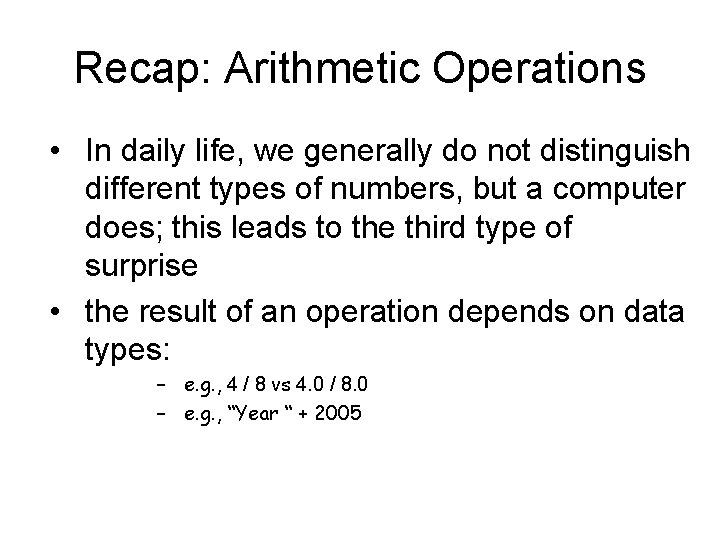
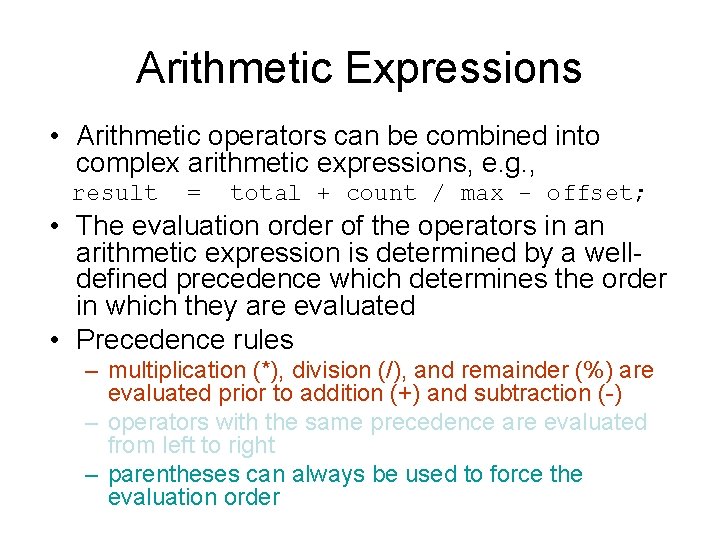
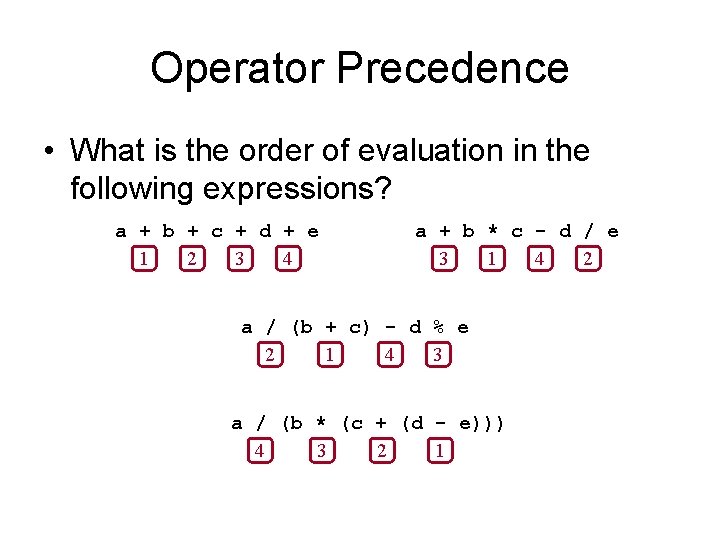
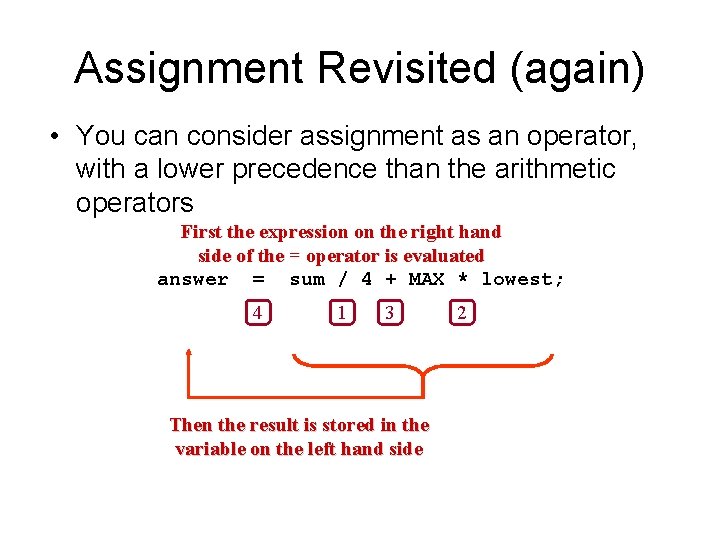
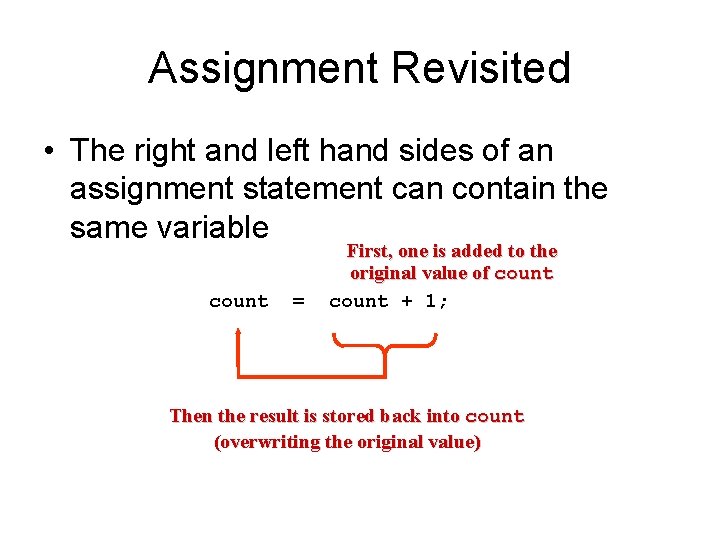
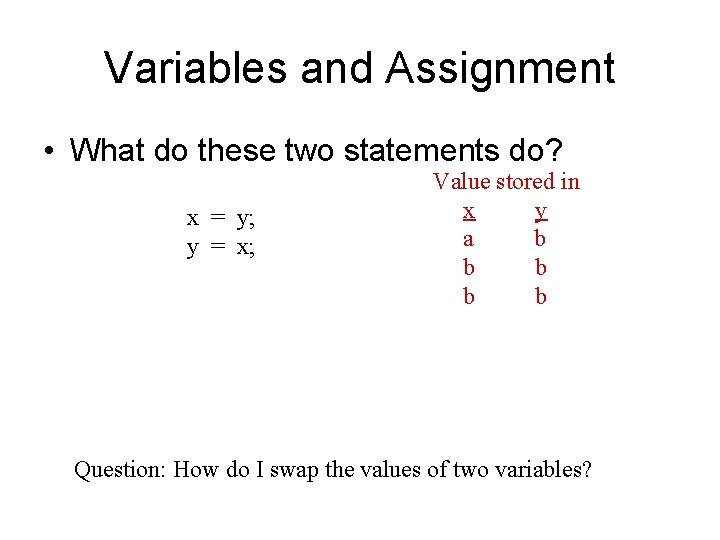
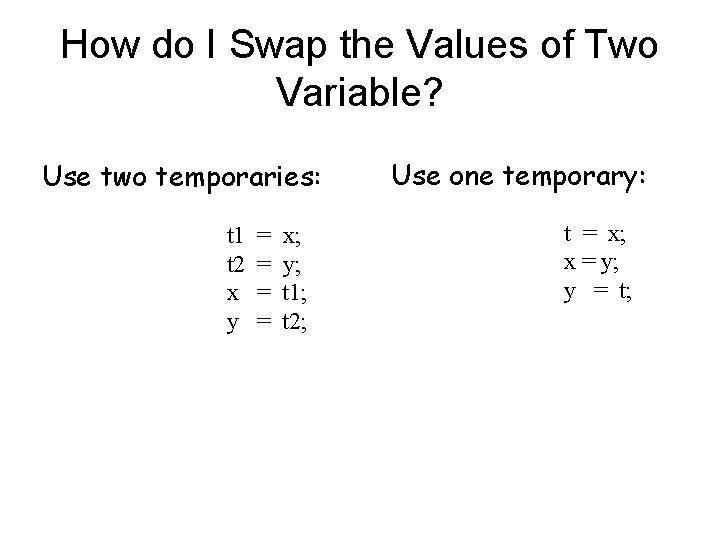
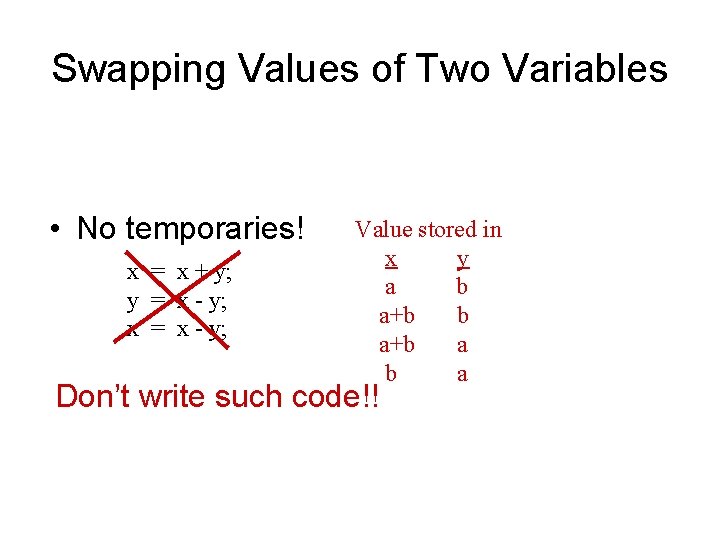
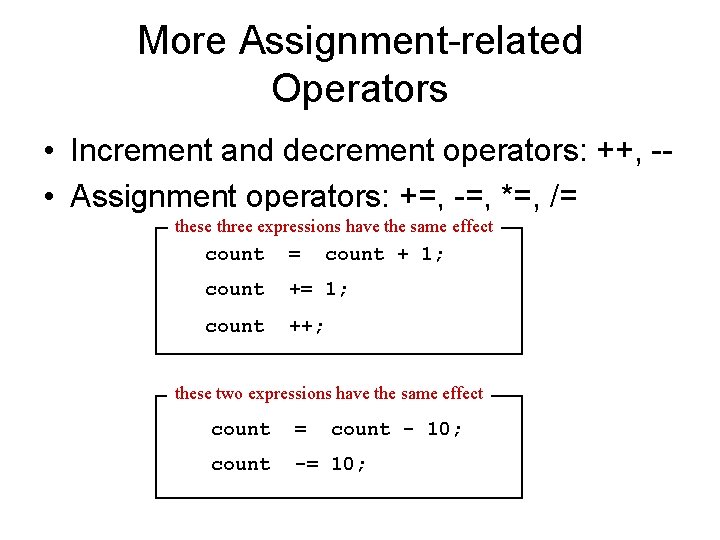
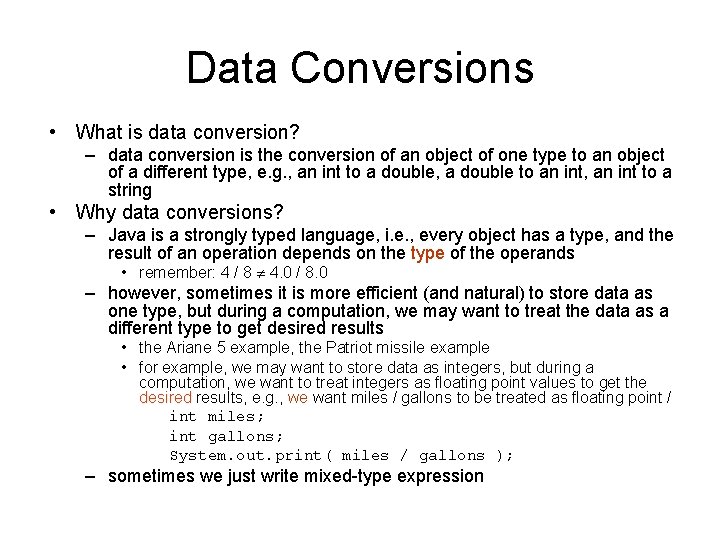
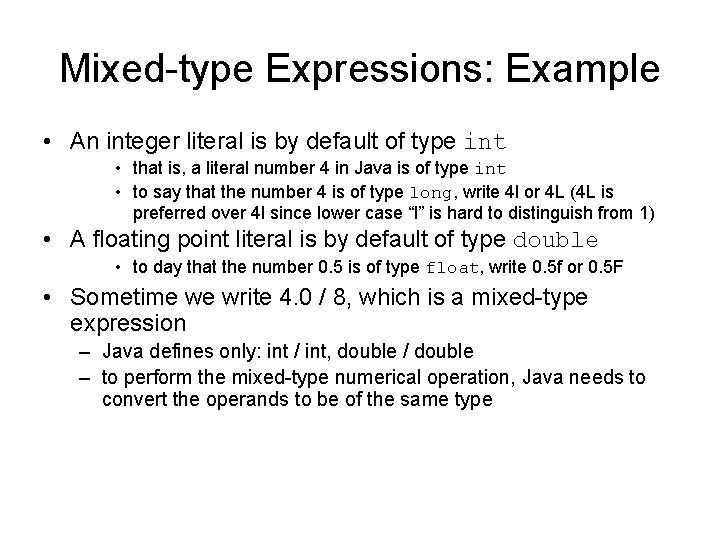
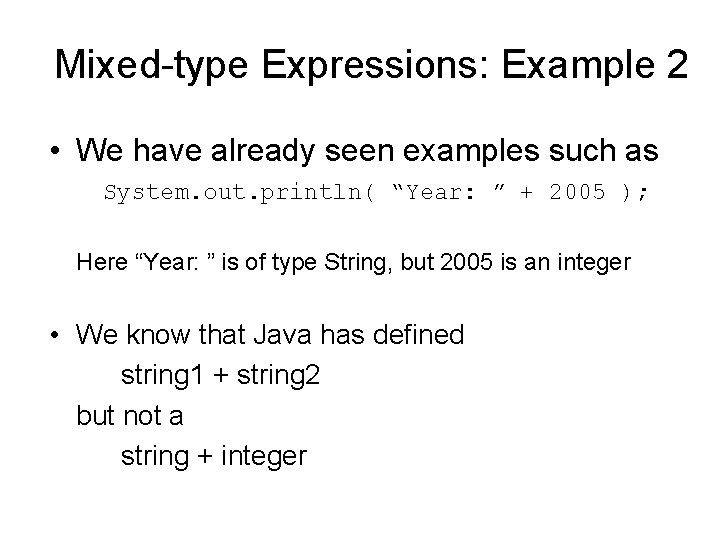
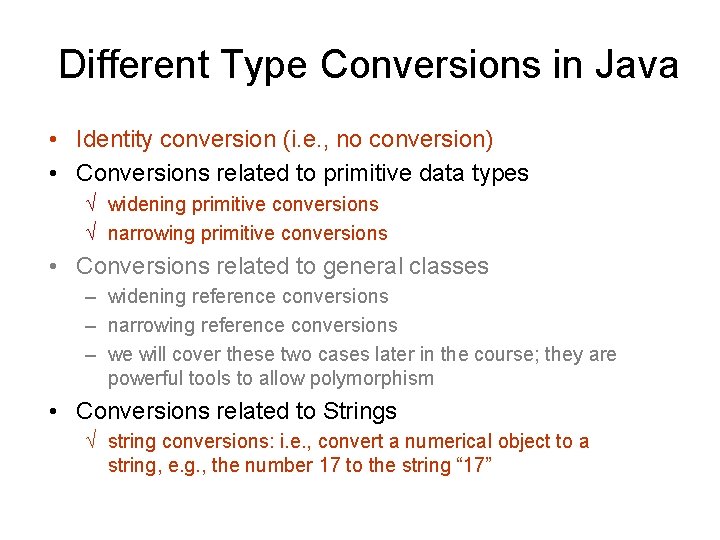
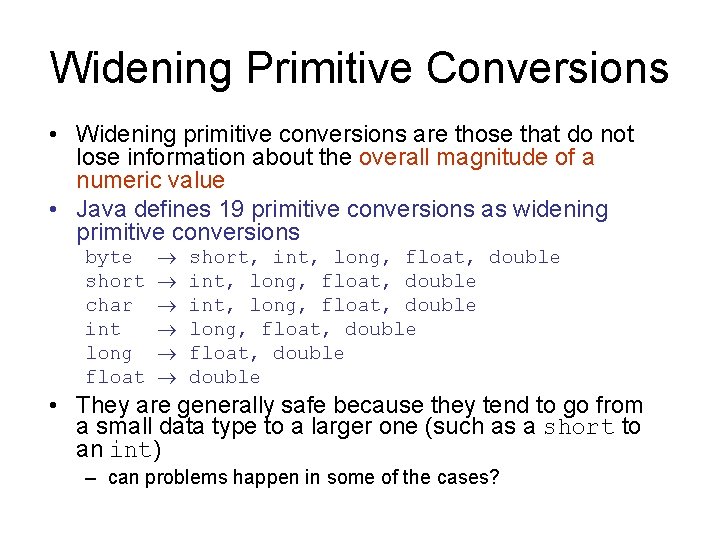
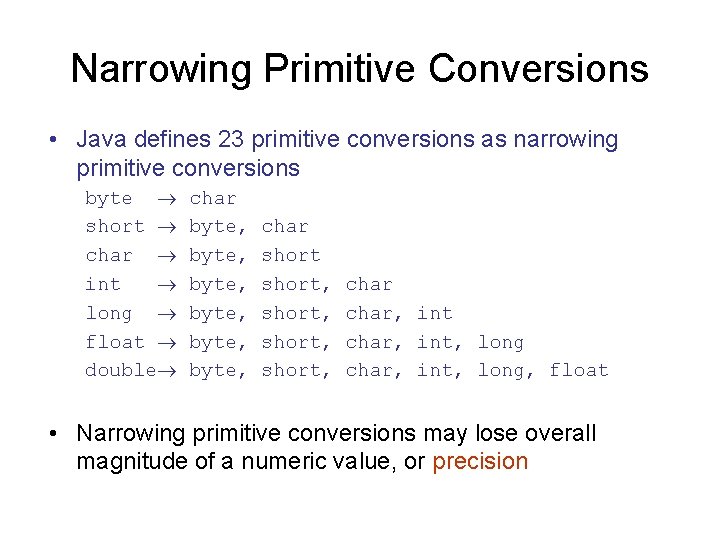
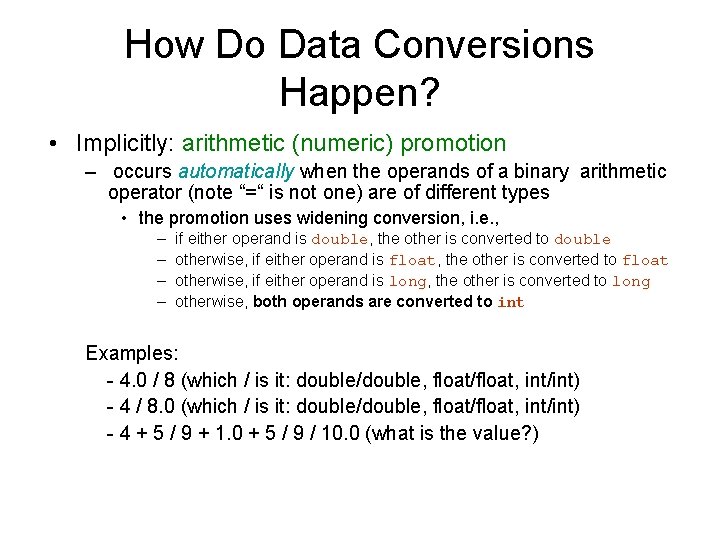
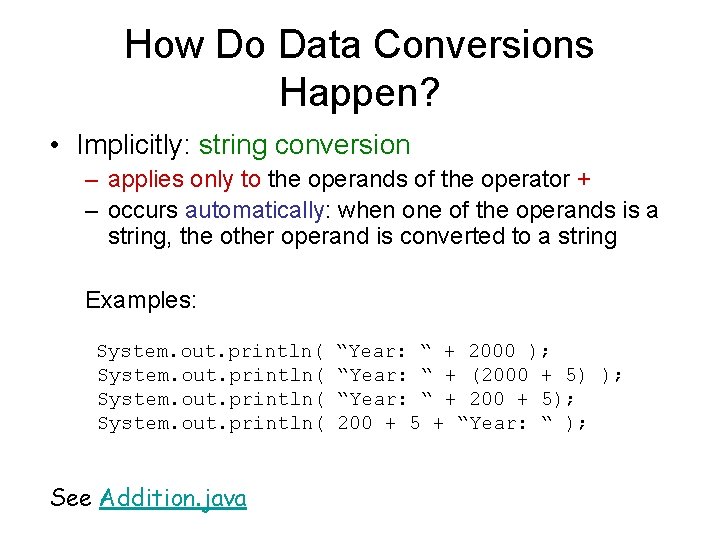
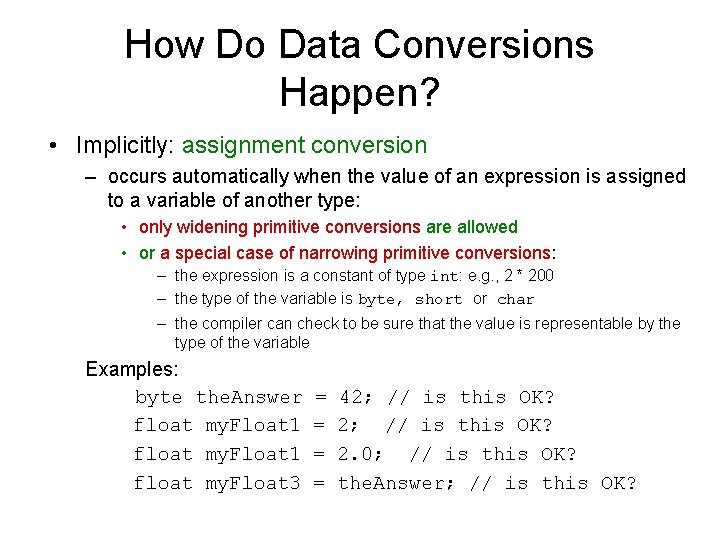
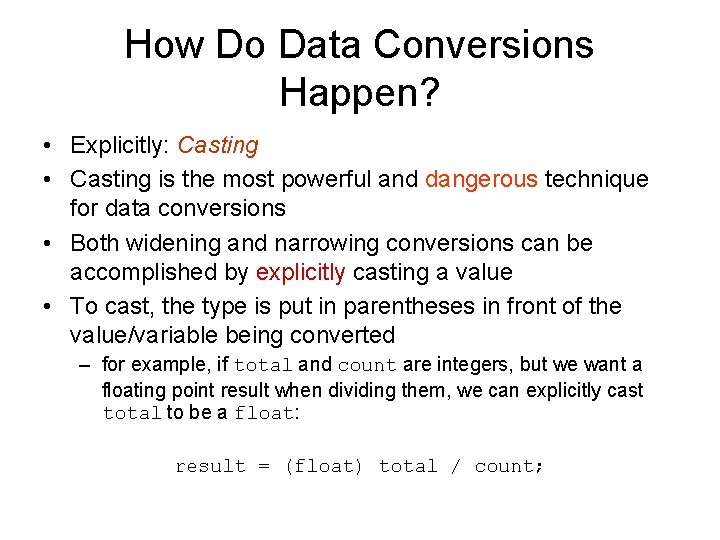
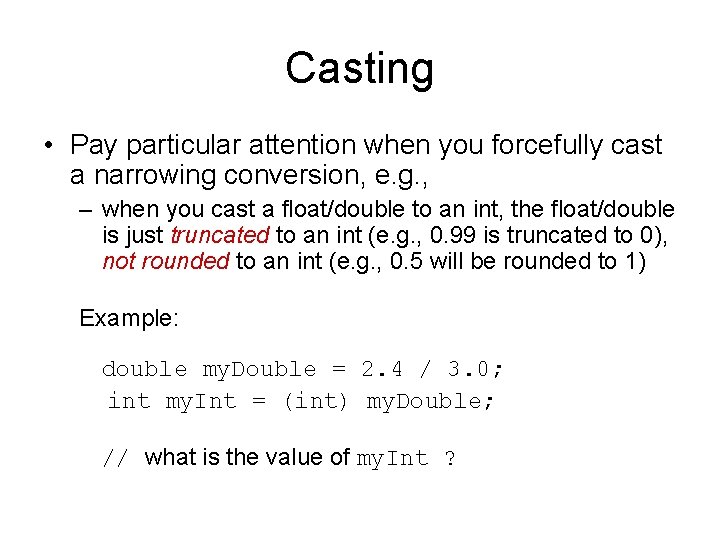
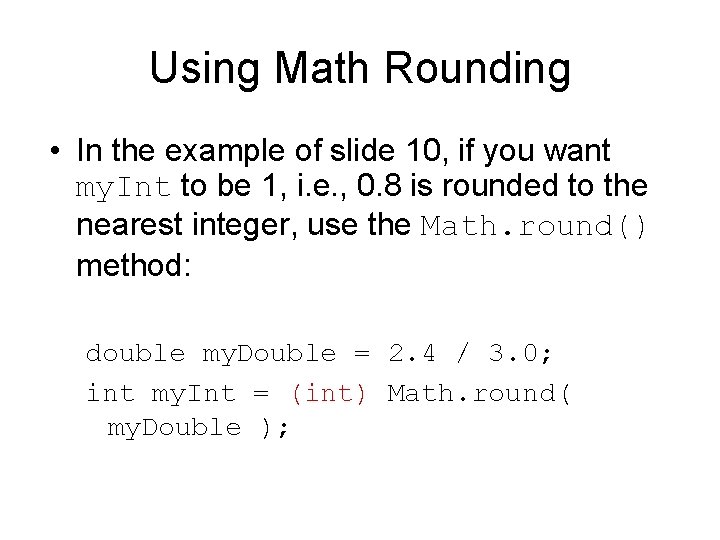
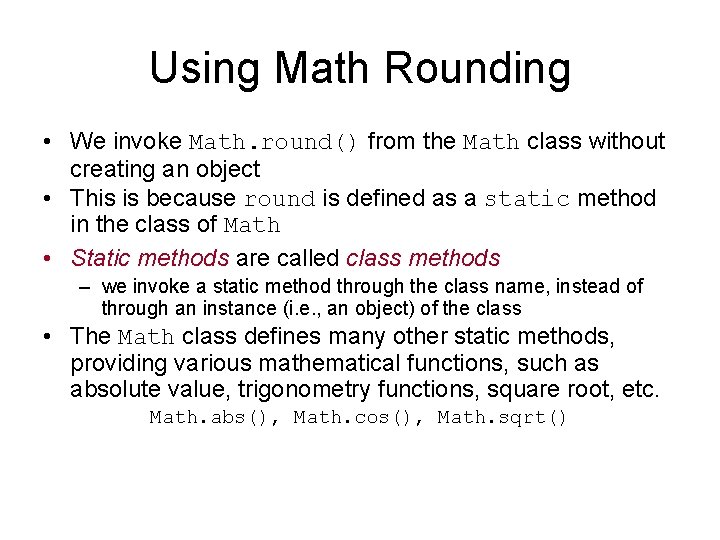
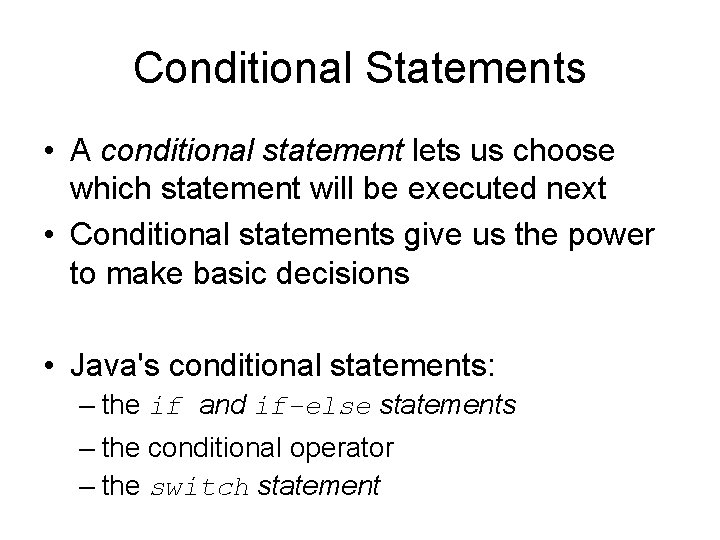
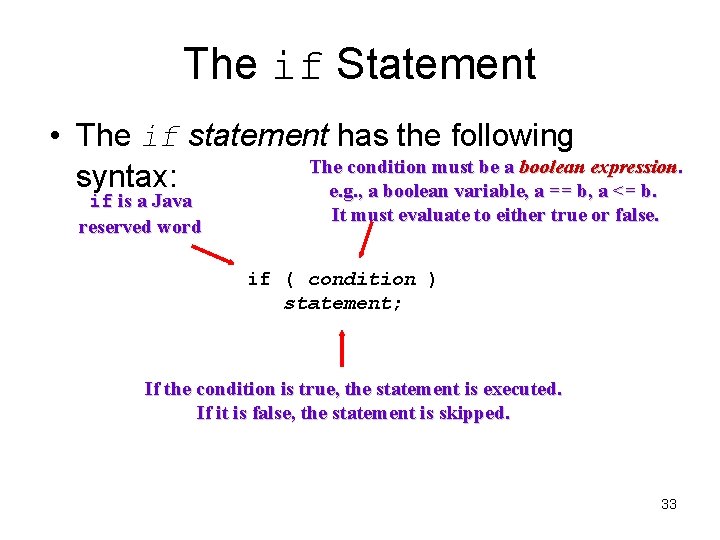
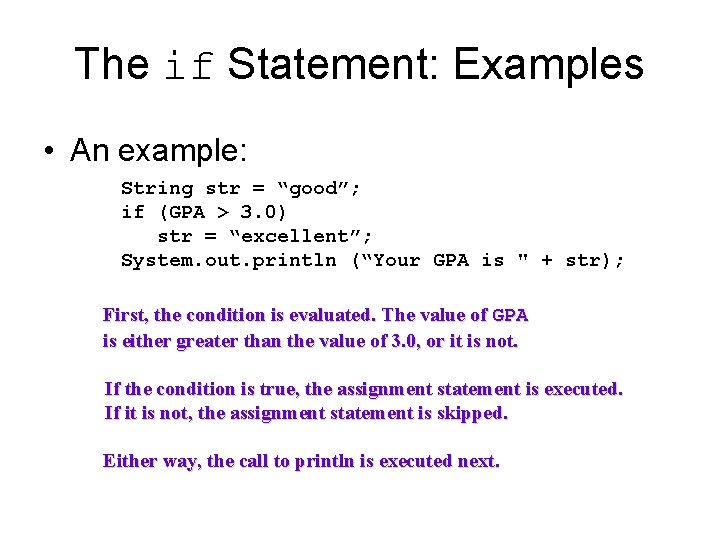
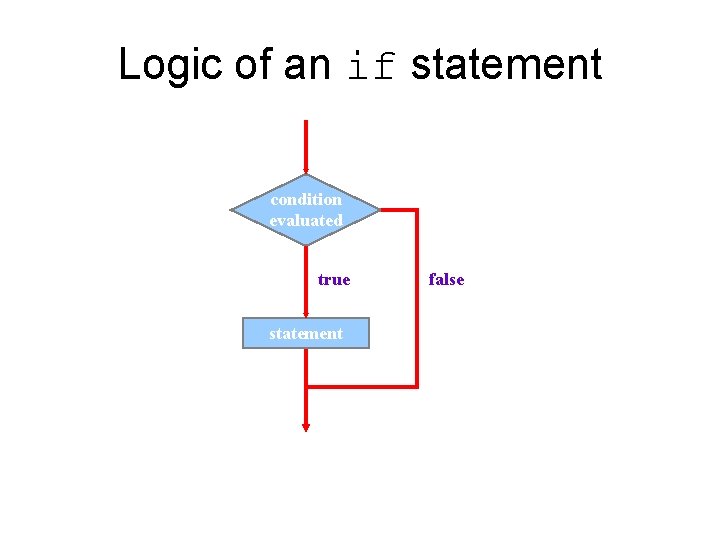
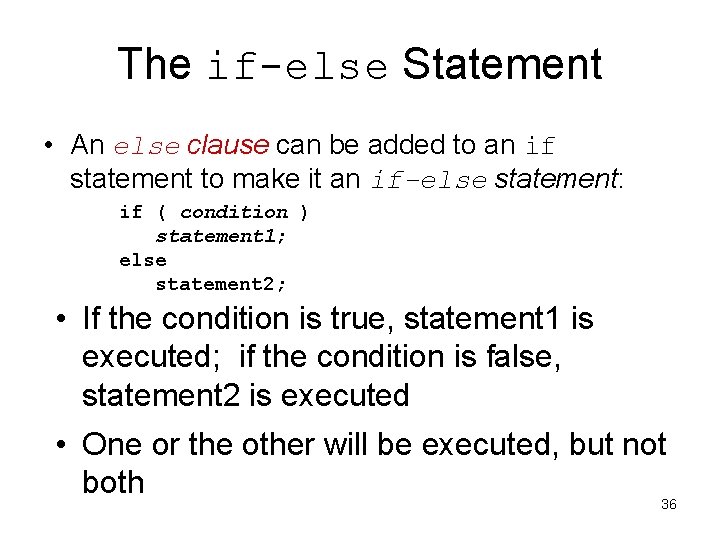
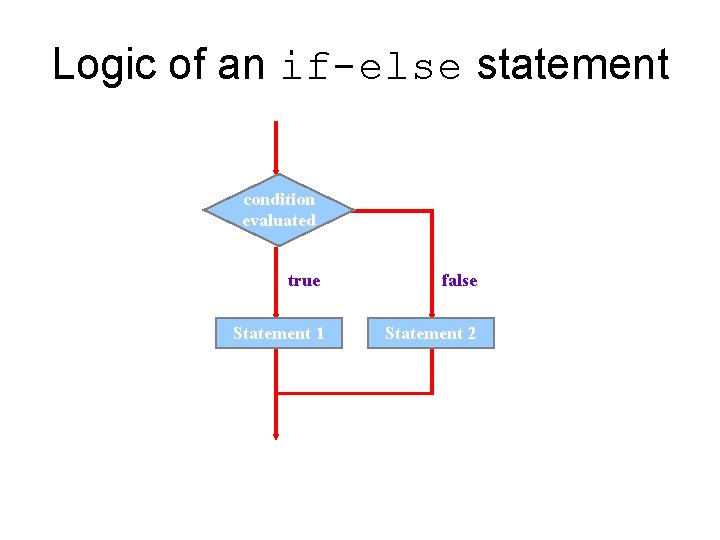
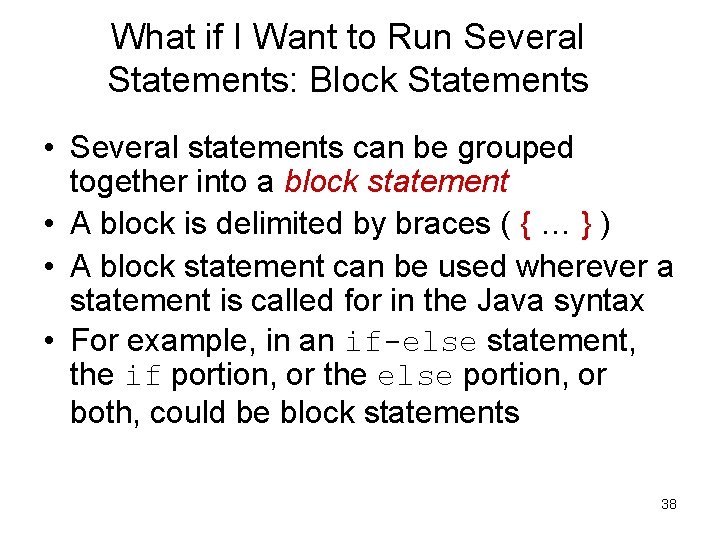
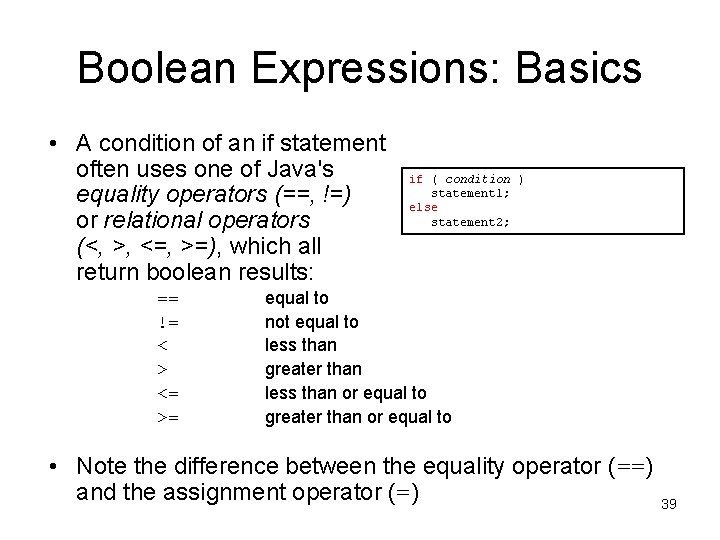
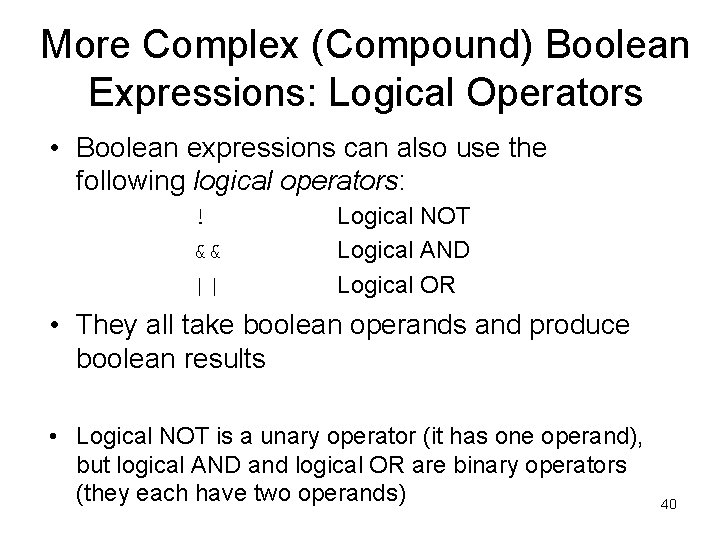
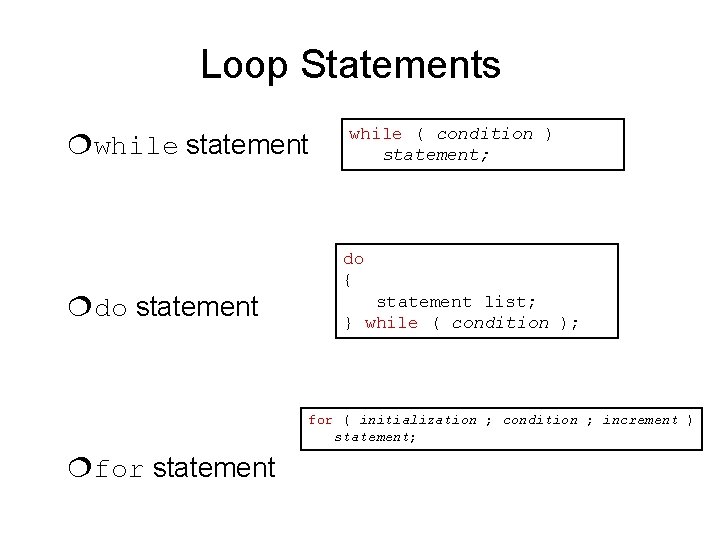
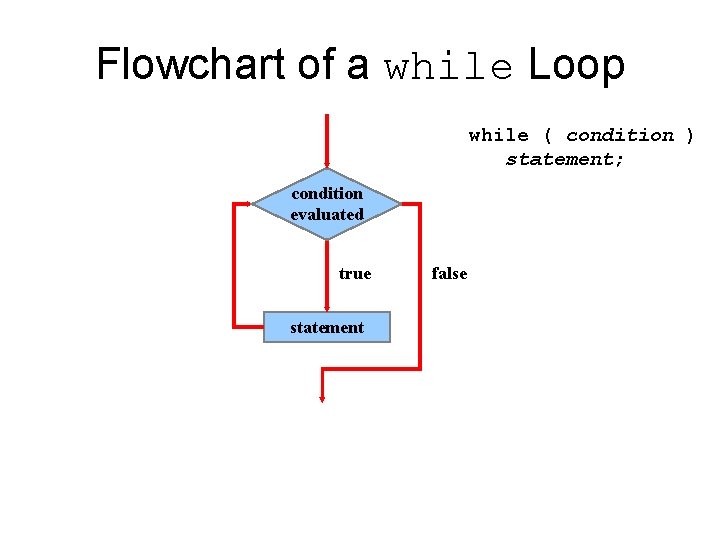
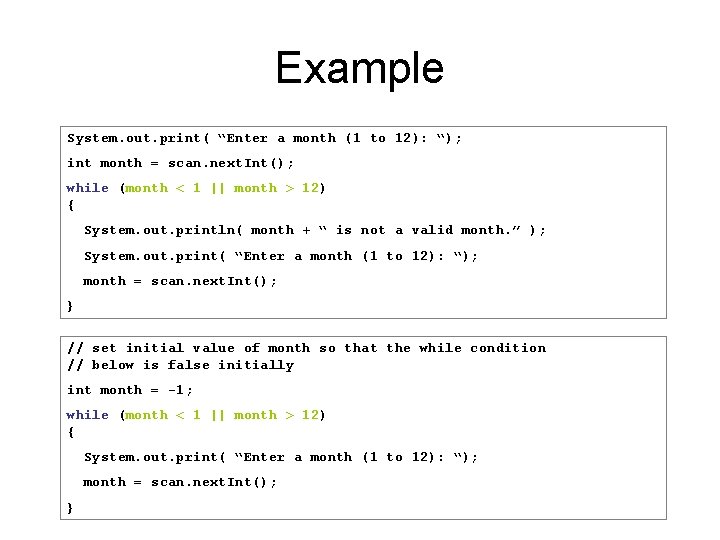
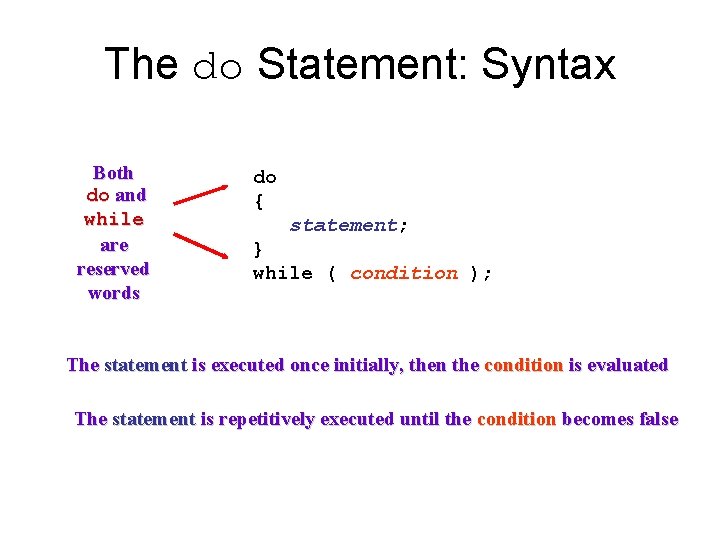
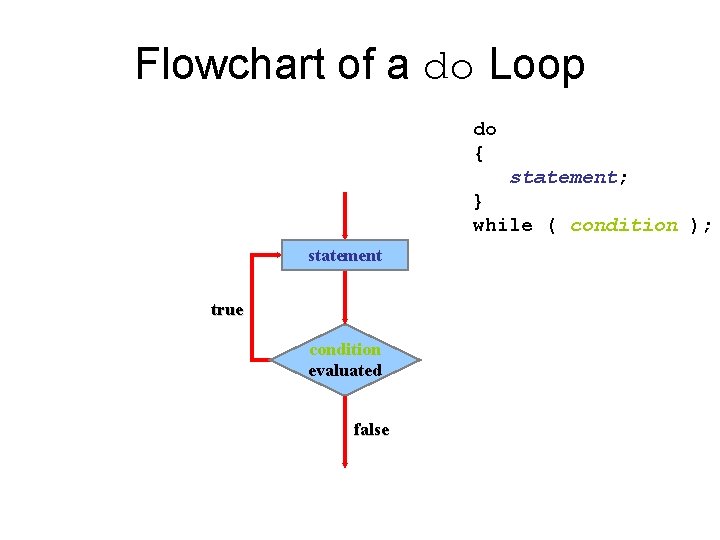
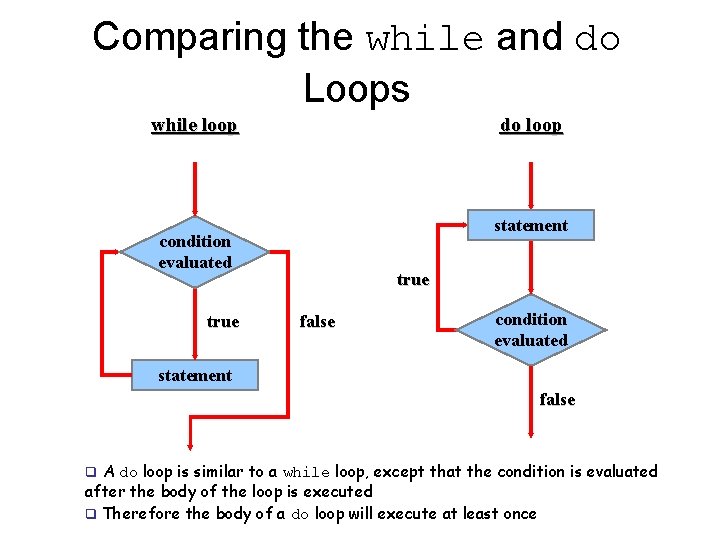
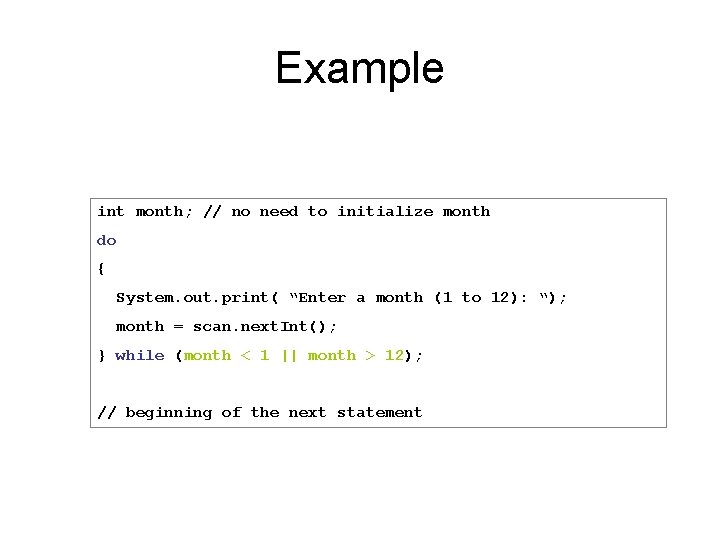
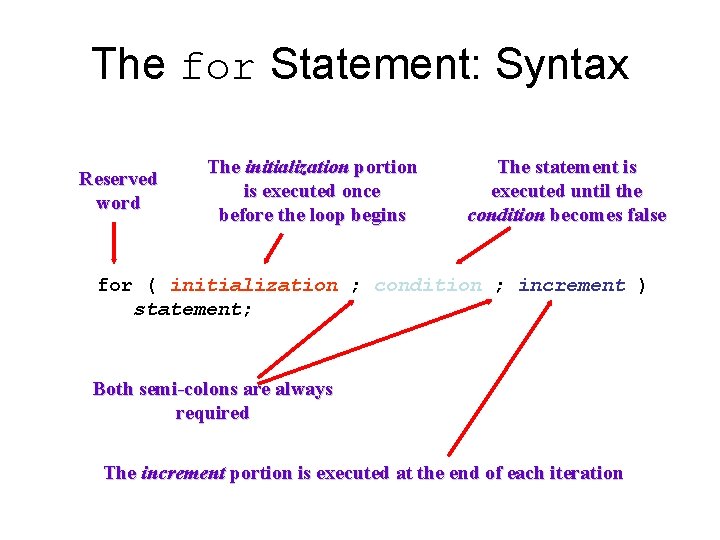
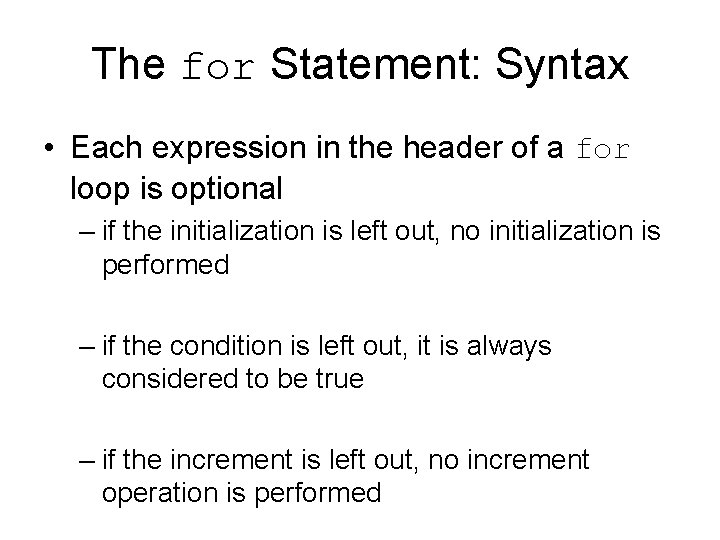
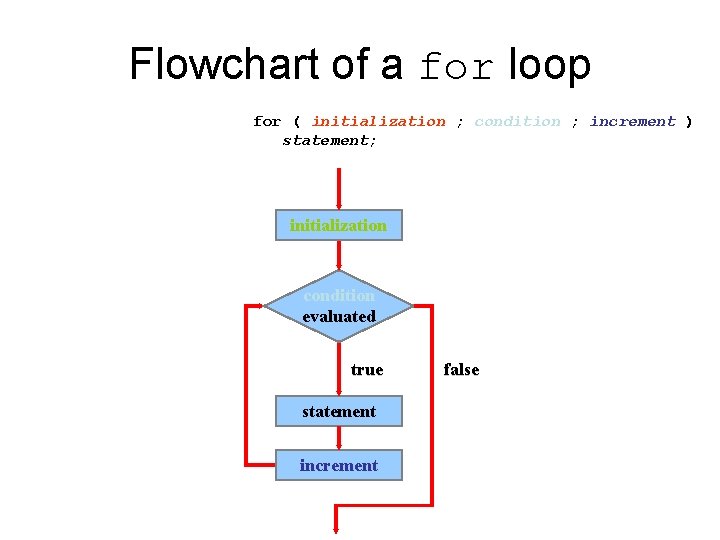
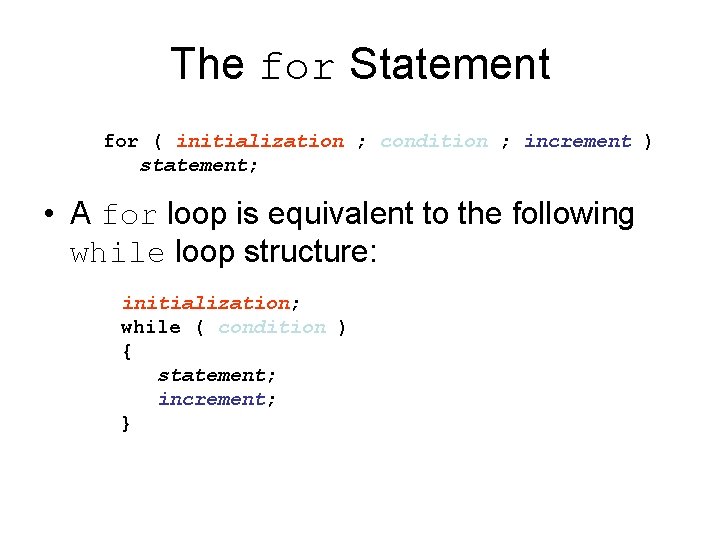
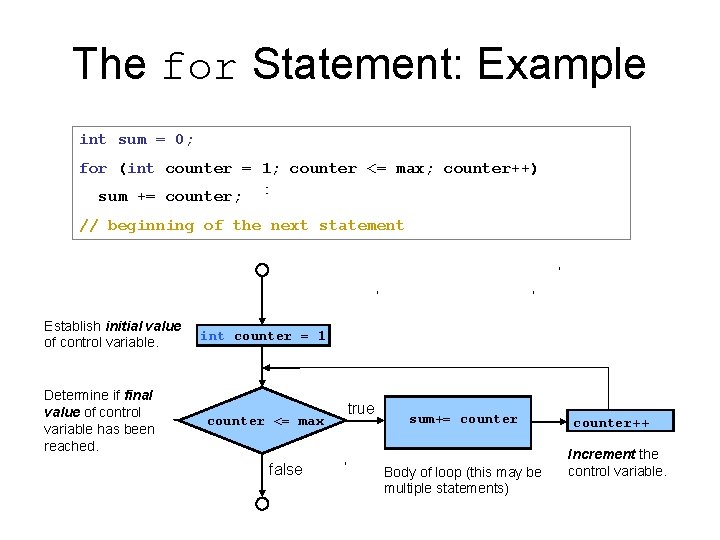
- Slides: 52
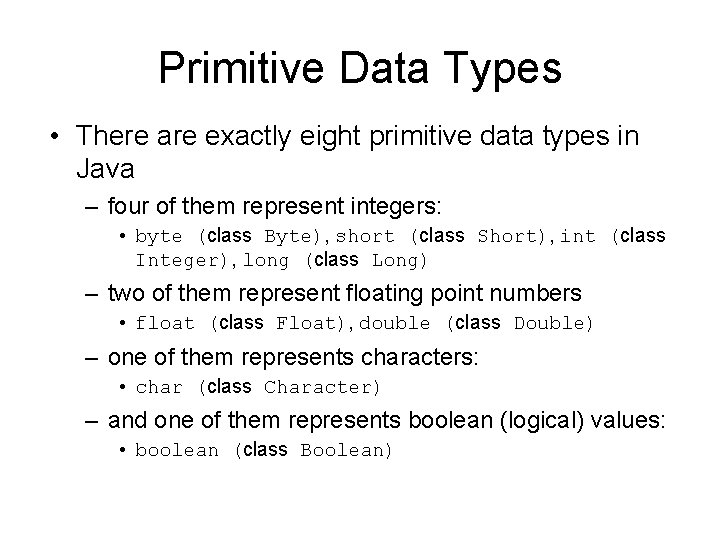
Primitive Data Types • There are exactly eight primitive data types in Java – four of them represent integers: • byte (class Byte), short (class Short), int (class Integer), long (class Long) – two of them represent floating point numbers • float (class Float), double (class Double) – one of them represents characters: • char (class Character) – and one of them represents boolean (logical) values: • boolean (class Boolean)
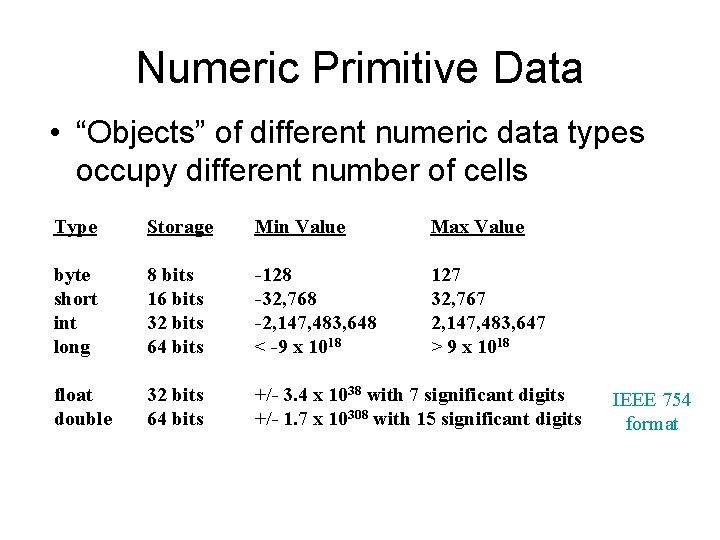
Numeric Primitive Data • “Objects” of different numeric data types occupy different number of cells Type Storage Min Value Max Value byte short int long 8 bits 16 bits 32 bits 64 bits -128 -32, 768 -2, 147, 483, 648 < -9 x 1018 127 32, 767 2, 147, 483, 647 > 9 x 1018 float double 32 bits 64 bits +/- 3. 4 x 1038 with 7 significant digits +/- 1. 7 x 10308 with 15 significant digits IEEE 754 format
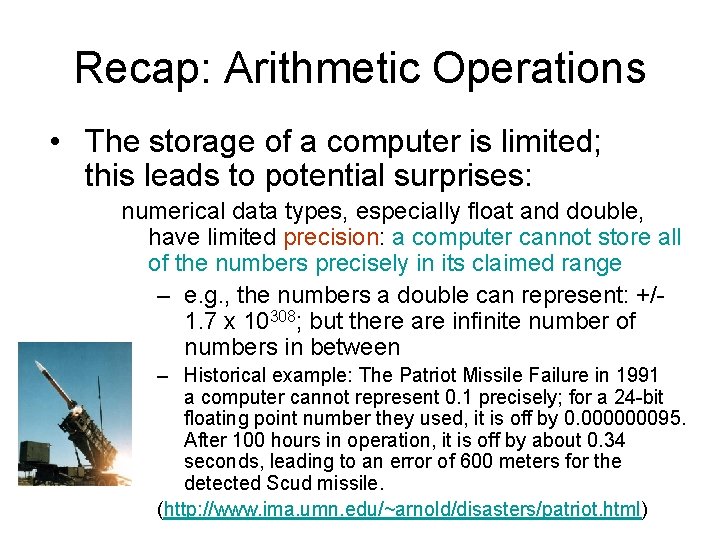
Recap: Arithmetic Operations • The storage of a computer is limited; this leads to potential surprises: numerical data types, especially float and double, have limited precision: a computer cannot store all of the numbers precisely in its claimed range – e. g. , the numbers a double can represent: +/1. 7 x 10308; but there are infinite number of numbers in between – Historical example: The Patriot Missile Failure in 1991 a computer cannot represent 0. 1 precisely; for a 24 -bit floating point number they used, it is off by 0. 000000095. After 100 hours in operation, it is off by about 0. 34 seconds, leading to an error of 600 meters for the detected Scud missile. (http: //www. ima. umn. edu/~arnold/disasters/patriot. html)
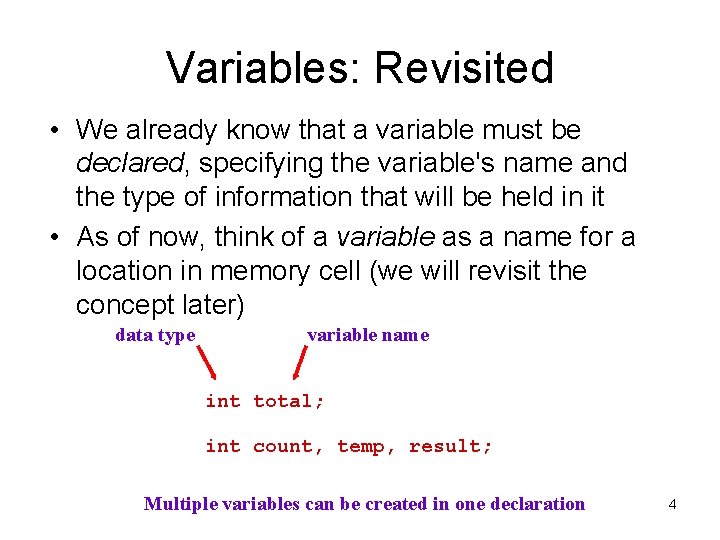
Variables: Revisited • We already know that a variable must be declared, specifying the variable's name and the type of information that will be held in it • As of now, think of a variable as a name for a location in memory cell (we will revisit the concept later) data type variable name int total; int count, temp, result; Multiple variables can be created in one declaration 4
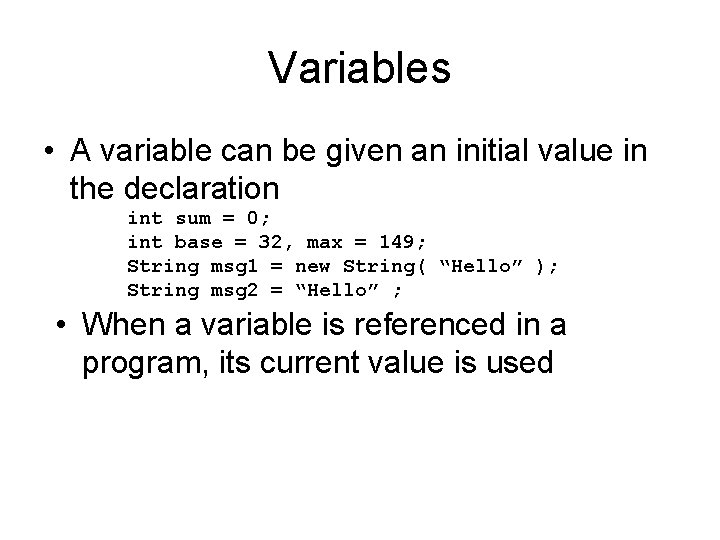
Variables • A variable can be given an initial value in the declaration int sum = 0; int base = 32, max = 149; String msg 1 = new String( “Hello” ); String msg 2 = “Hello” ; • When a variable is referenced in a program, its current value is used
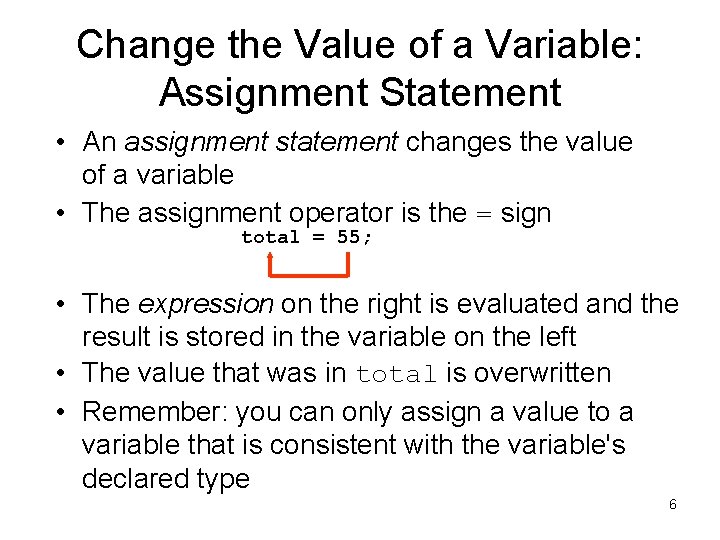
Change the Value of a Variable: Assignment Statement • An assignment statement changes the value of a variable • The assignment operator is the = sign total = 55; • The expression on the right is evaluated and the result is stored in the variable on the left • The value that was in total is overwritten • Remember: you can only assign a value to a variable that is consistent with the variable's declared type 6
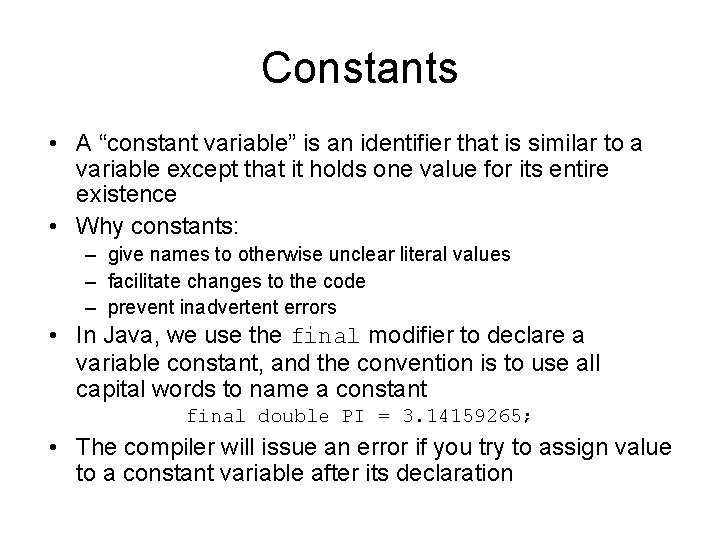
Constants • A “constant variable” is an identifier that is similar to a variable except that it holds one value for its entire existence • Why constants: – give names to otherwise unclear literal values – facilitate changes to the code – prevent inadvertent errors • In Java, we use the final modifier to declare a variable constant, and the convention is to use all capital words to name a constant final double PI = 3. 14159265; • The compiler will issue an error if you try to assign value to a constant variable after its declaration
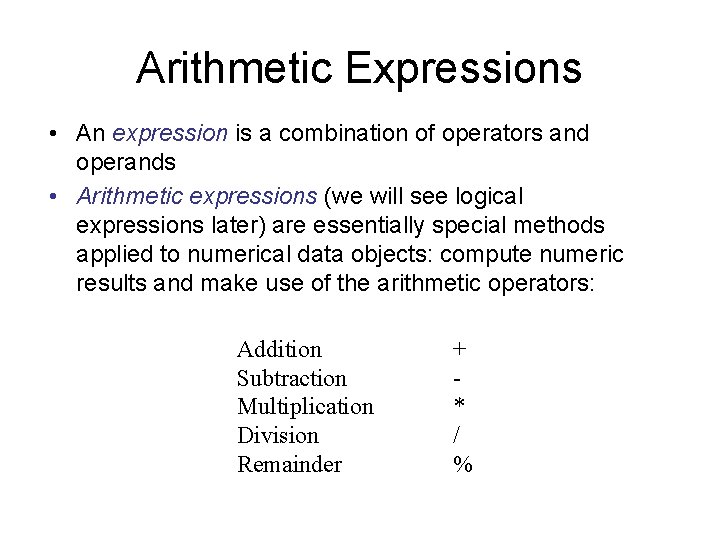
Arithmetic Expressions • An expression is a combination of operators and operands • Arithmetic expressions (we will see logical expressions later) are essentially special methods applied to numerical data objects: compute numeric results and make use of the arithmetic operators: Addition Subtraction Multiplication Division Remainder + * / %
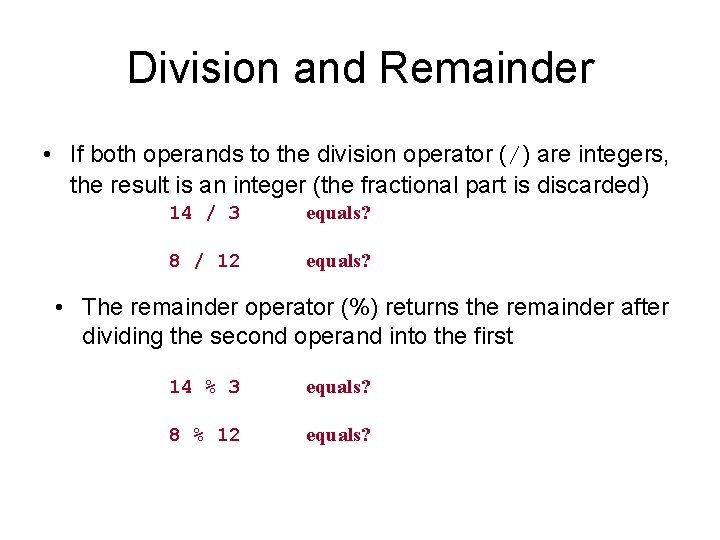
Division and Remainder • If both operands to the division operator (/) are integers, the result is an integer (the fractional part is discarded) 14 / 3 equals? 8 / 12 equals? • The remainder operator (%) returns the remainder after dividing the second operand into the first 14 % 3 equals? 8 % 12 equals?
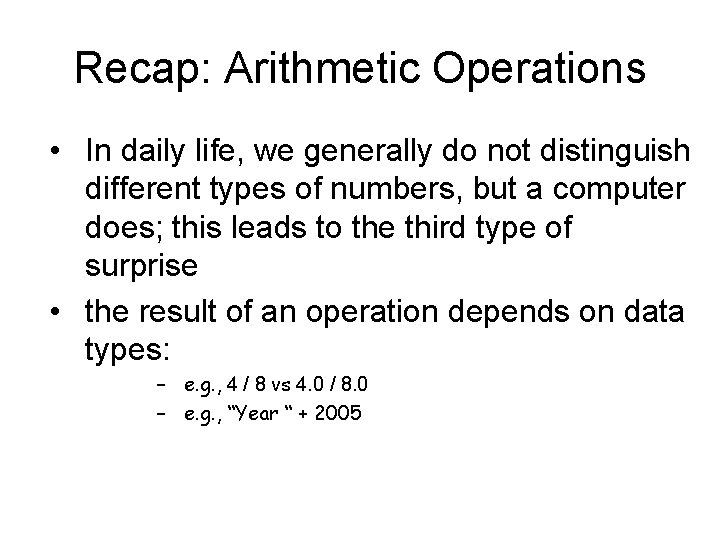
Recap: Arithmetic Operations • In daily life, we generally do not distinguish different types of numbers, but a computer does; this leads to the third type of surprise • the result of an operation depends on data types: – e. g. , 4 / 8 vs 4. 0 / 8. 0 – e. g. , “Year “ + 2005
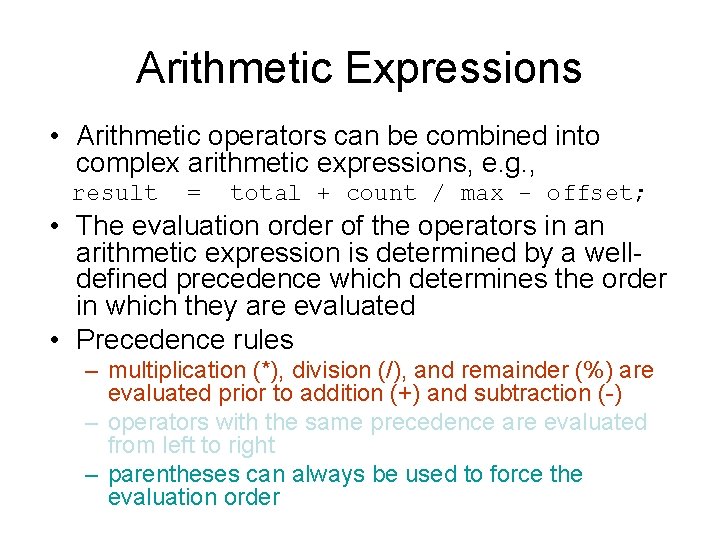
Arithmetic Expressions • Arithmetic operators can be combined into complex arithmetic expressions, e. g. , result = total + count / max - offset; • The evaluation order of the operators in an arithmetic expression is determined by a welldefined precedence which determines the order in which they are evaluated • Precedence rules – multiplication (*), division (/), and remainder (%) are evaluated prior to addition (+) and subtraction (-) – operators with the same precedence are evaluated from left to right – parentheses can always be used to force the evaluation order
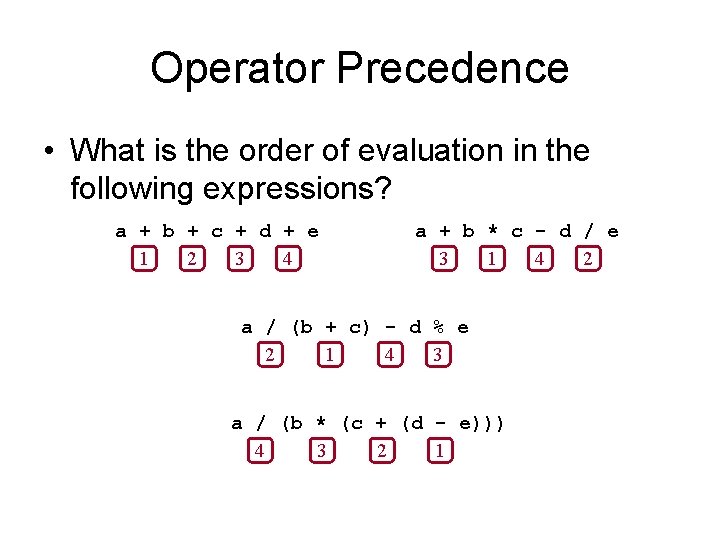
Operator Precedence • What is the order of evaluation in the following expressions? a + b + c + d + e 1 2 3 4 a + b * c - d / e 3 1 4 2 a / (b + c) - d % e 2 1 4 3 a / (b * (c + (d - e))) 4 3 2 1
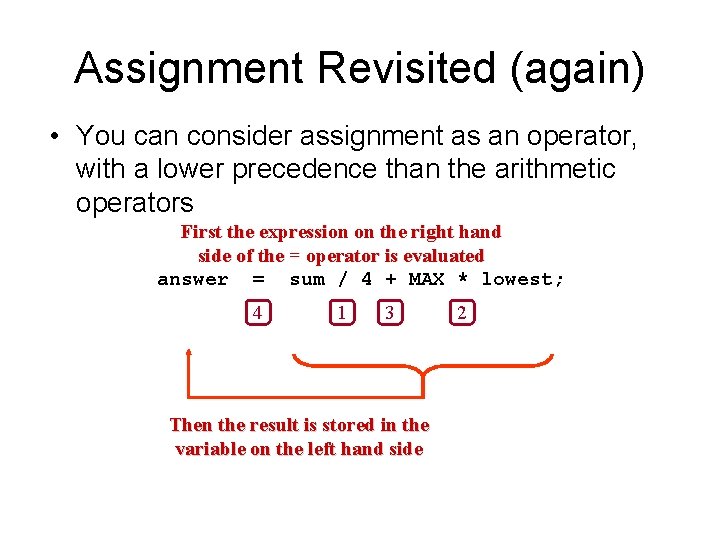
Assignment Revisited (again) • You can consider assignment as an operator, with a lower precedence than the arithmetic operators First the expression on the right hand side of the = operator is evaluated answer = sum / 4 + MAX * lowest; 4 1 3 Then the result is stored in the variable on the left hand side 2
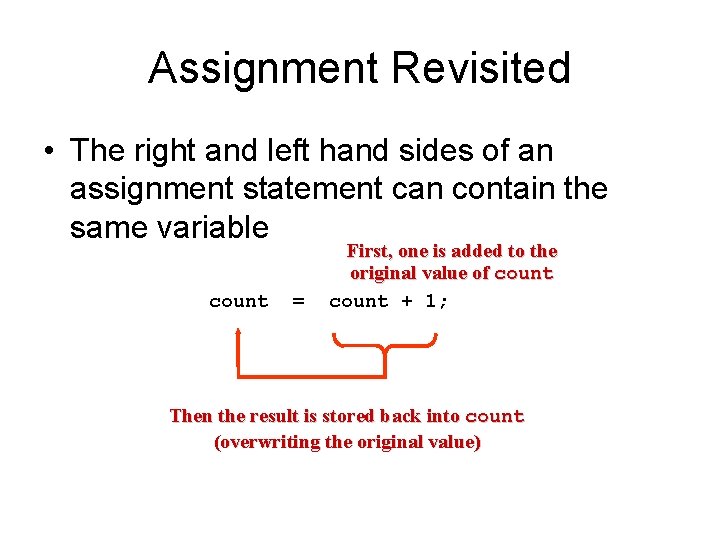
Assignment Revisited • The right and left hand sides of an assignment statement can contain the same variable count = First, one is added to the original value of count + 1; Then the result is stored back into count (overwriting the original value)
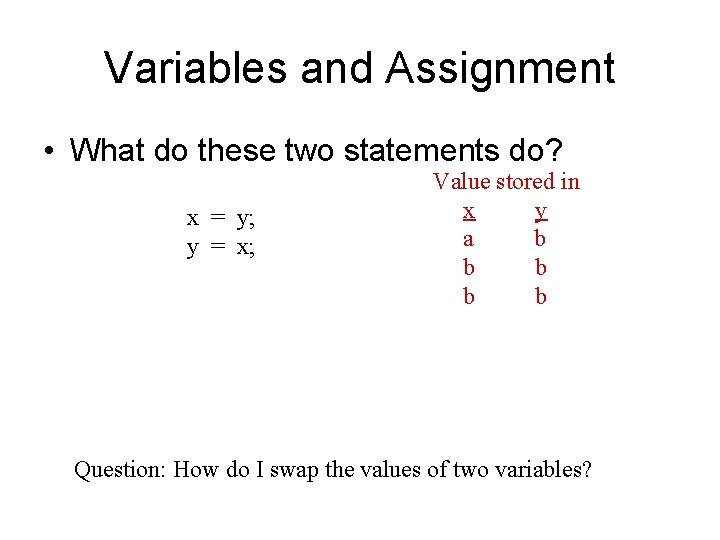
Variables and Assignment • What do these two statements do? x = y; y = x; Value stored in x y a b b b Question: How do I swap the values of two variables?
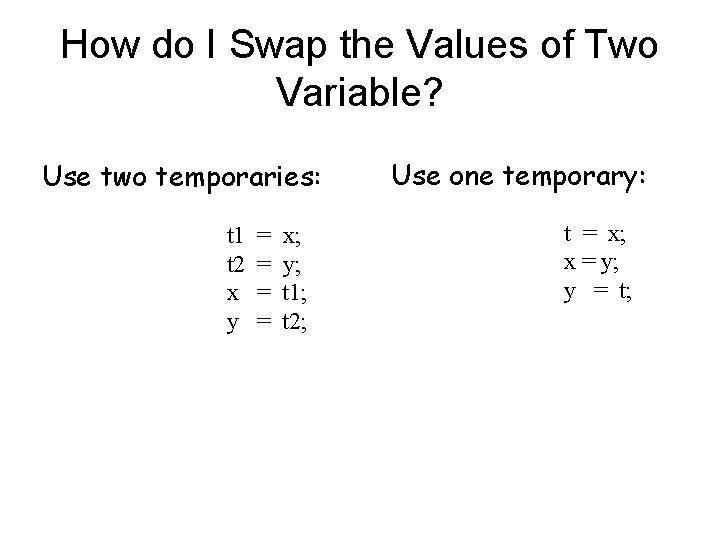
How do I Swap the Values of Two Variable? Use two temporaries: t 1 t 2 x y = = x; y; t 1; t 2; Use one temporary: t = x; x = y; y = t;
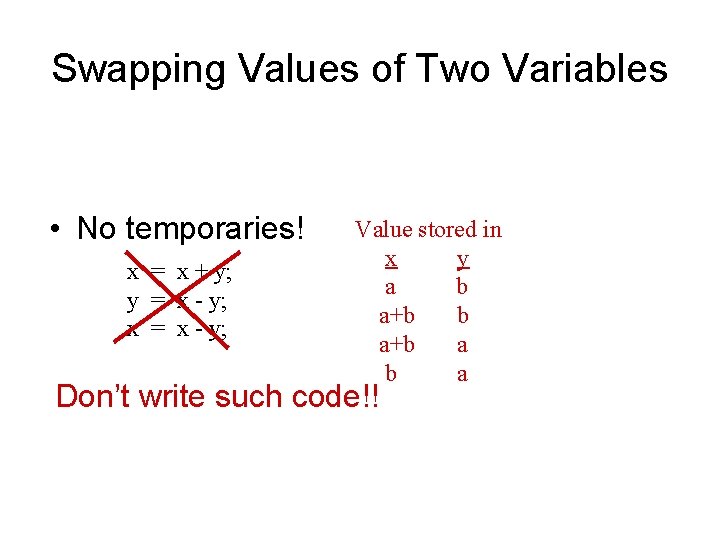
Swapping Values of Two Variables • No temporaries! x = x + y; y = x - y; x = x - y; Value stored in x y a b a+b a b a Don’t write such code!!
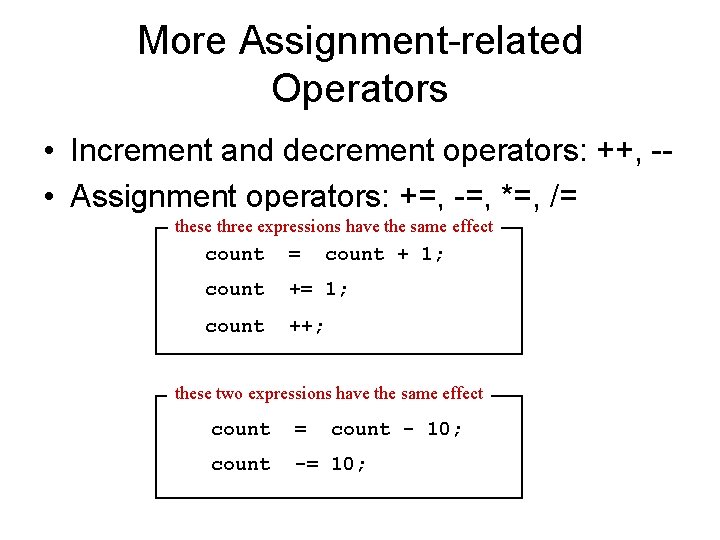
More Assignment-related Operators • Increment and decrement operators: ++, - • Assignment operators: +=, -=, *=, /= these three expressions have the same effect count = count + 1; count += 1; count ++; these two expressions have the same effect count = count - 10; count -= 10;
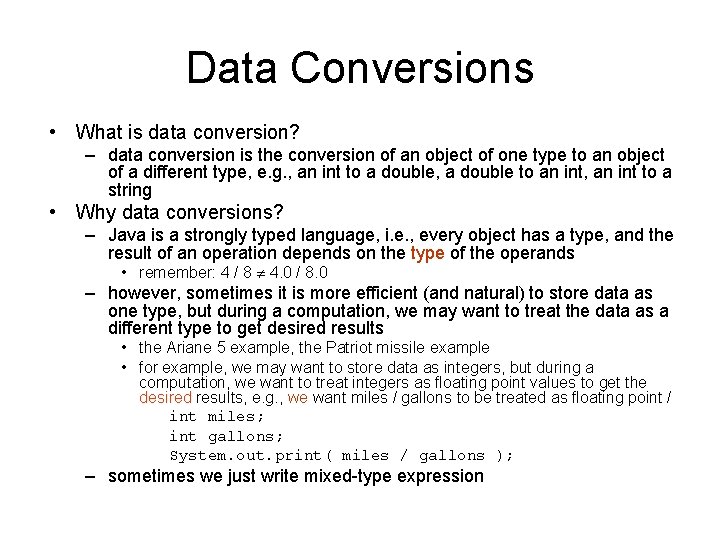
Data Conversions • What is data conversion? – data conversion is the conversion of an object of one type to an object of a different type, e. g. , an int to a double, a double to an int, an int to a string • Why data conversions? – Java is a strongly typed language, i. e. , every object has a type, and the result of an operation depends on the type of the operands • remember: 4 / 8 4. 0 / 8. 0 – however, sometimes it is more efficient (and natural) to store data as one type, but during a computation, we may want to treat the data as a different type to get desired results • the Ariane 5 example, the Patriot missile example • for example, we may want to store data as integers, but during a computation, we want to treat integers as floating point values to get the desired results, e. g. , we want miles / gallons to be treated as floating point / int miles; int gallons; System. out. print( miles / gallons ); – sometimes we just write mixed-type expression
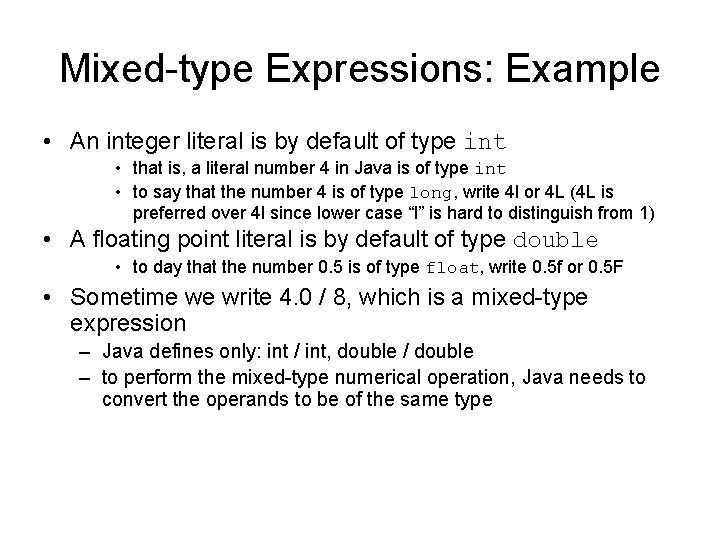
Mixed-type Expressions: Example • An integer literal is by default of type int • that is, a literal number 4 in Java is of type int • to say that the number 4 is of type long, write 4 l or 4 L (4 L is preferred over 4 l since lower case “l” is hard to distinguish from 1) • A floating point literal is by default of type double • to day that the number 0. 5 is of type float, write 0. 5 f or 0. 5 F • Sometime we write 4. 0 / 8, which is a mixed-type expression – Java defines only: int / int, double / double – to perform the mixed-type numerical operation, Java needs to convert the operands to be of the same type
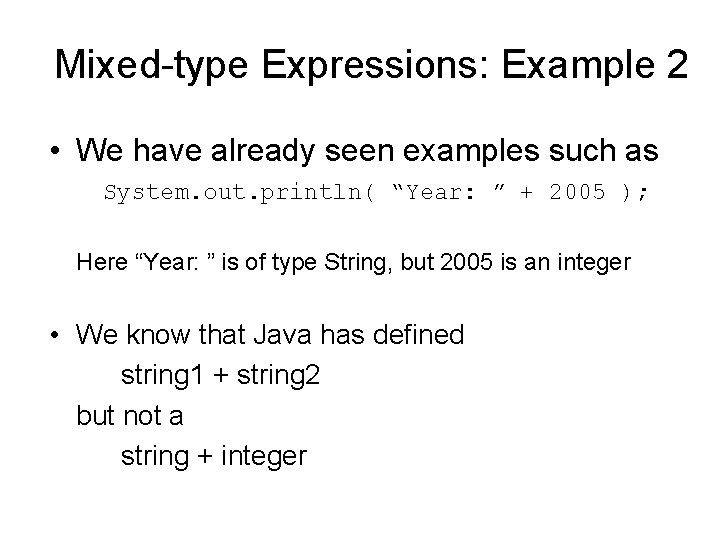
Mixed-type Expressions: Example 2 • We have already seen examples such as System. out. println( “Year: ” + 2005 ); Here “Year: ” is of type String, but 2005 is an integer • We know that Java has defined string 1 + string 2 but not a string + integer
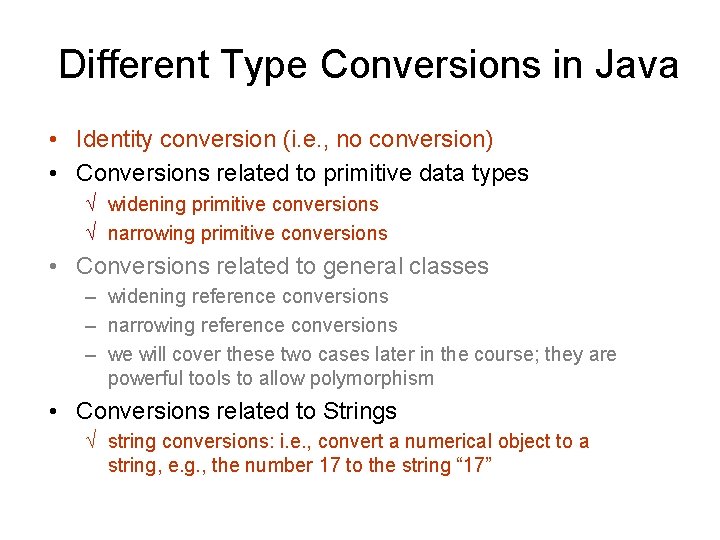
Different Type Conversions in Java • Identity conversion (i. e. , no conversion) • Conversions related to primitive data types Ö widening primitive conversions Ö narrowing primitive conversions • Conversions related to general classes – widening reference conversions – narrowing reference conversions – we will cover these two cases later in the course; they are powerful tools to allow polymorphism • Conversions related to Strings Ö string conversions: i. e. , convert a numerical object to a string, e. g. , the number 17 to the string “ 17”
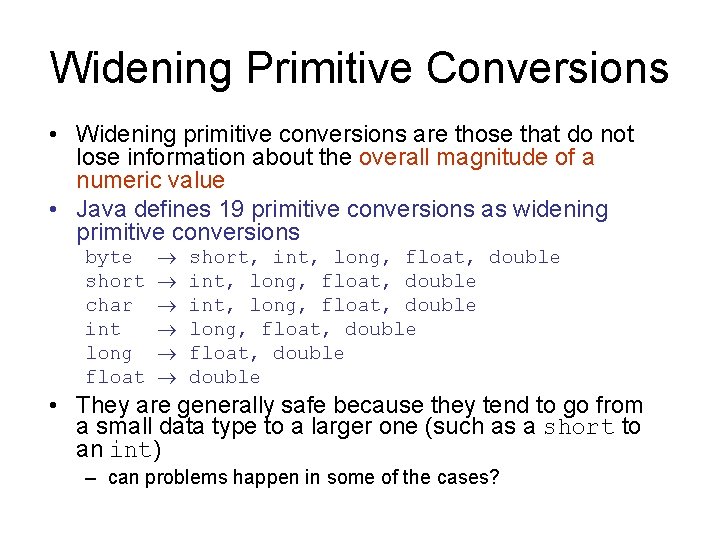
Widening Primitive Conversions • Widening primitive conversions are those that do not lose information about the overall magnitude of a numeric value • Java defines 19 primitive conversions as widening primitive conversions byte short char int long float short, int, long, float, double float, double • They are generally safe because they tend to go from a small data type to a larger one (such as a short to an int) – can problems happen in some of the cases?
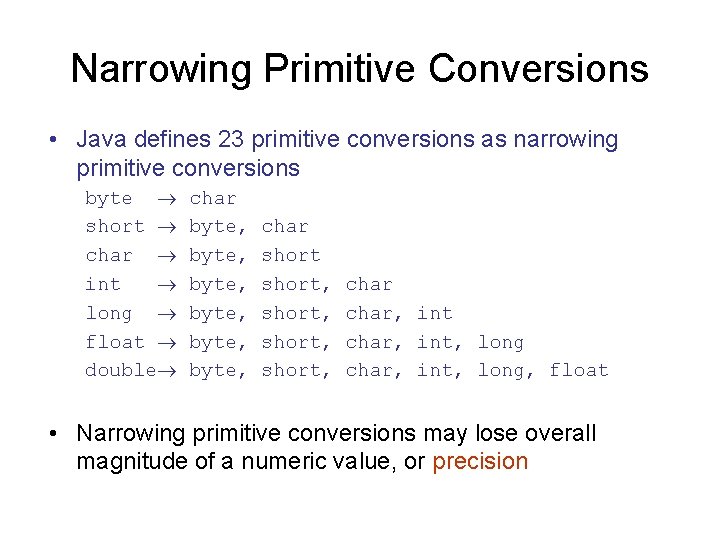
Narrowing Primitive Conversions • Java defines 23 primitive conversions as narrowing primitive conversions byte short char int long float double char byte, byte, char short, short, char, int, long, float • Narrowing primitive conversions may lose overall magnitude of a numeric value, or precision
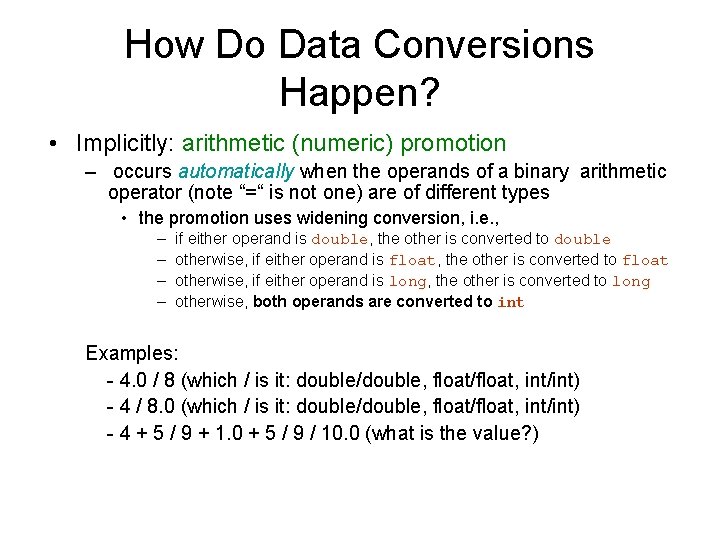
How Do Data Conversions Happen? • Implicitly: arithmetic (numeric) promotion – occurs automatically when the operands of a binary arithmetic operator (note “=“ is not one) are of different types • the promotion uses widening conversion, i. e. , – – if either operand is double, the other is converted to double otherwise, if either operand is float, the other is converted to float otherwise, if either operand is long, the other is converted to long otherwise, both operands are converted to int Examples: - 4. 0 / 8 (which / is it: double/double, float/float, int/int) - 4 / 8. 0 (which / is it: double/double, float/float, int/int) - 4 + 5 / 9 + 1. 0 + 5 / 9 / 10. 0 (what is the value? )
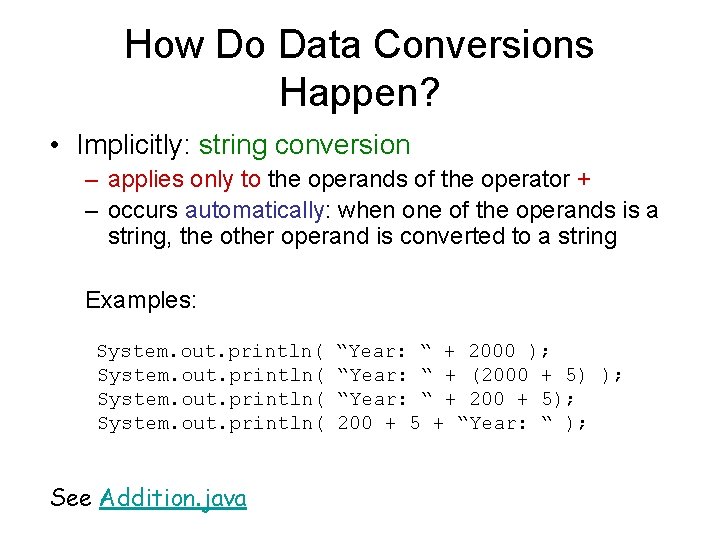
How Do Data Conversions Happen? • Implicitly: string conversion – applies only to the operands of the operator + – occurs automatically: when one of the operands is a string, the other operand is converted to a string Examples: System. out. println( See Addition. java “Year: “ + 2000 ); “Year: “ + (2000 + 5) ); “Year: “ + 200 + 5); 200 + 5 + “Year: “ );
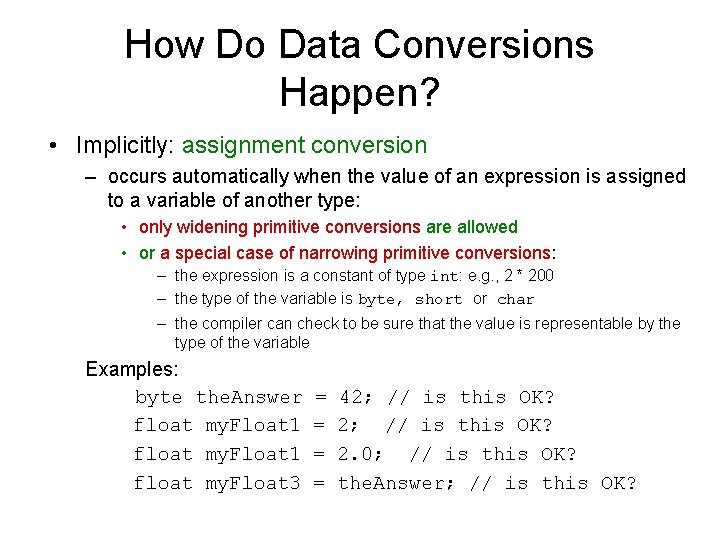
How Do Data Conversions Happen? • Implicitly: assignment conversion – occurs automatically when the value of an expression is assigned to a variable of another type: • only widening primitive conversions are allowed • or a special case of narrowing primitive conversions: – the expression is a constant of type int: e. g. , 2 * 200 – the type of the variable is byte, short or char – the compiler can check to be sure that the value is representable by the type of the variable Examples: byte the. Answer float my. Float 1 float my. Float 3 = = 42; // is this OK? 2. 0; // is this OK? the. Answer; // is this OK?
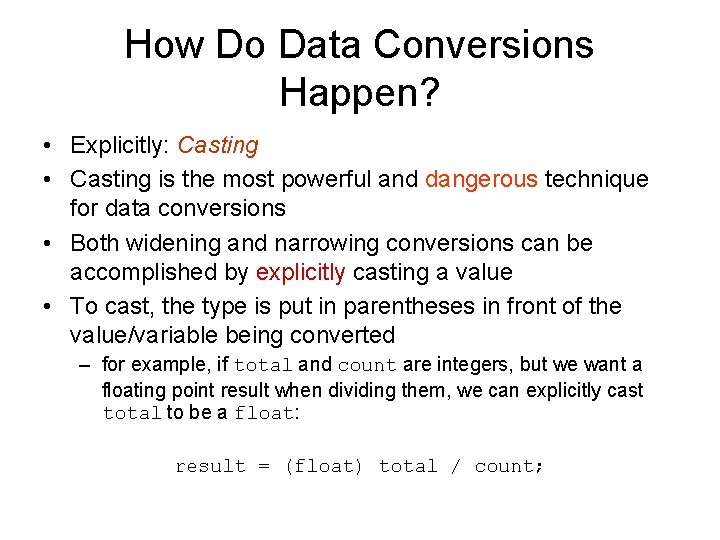
How Do Data Conversions Happen? • Explicitly: Casting • Casting is the most powerful and dangerous technique for data conversions • Both widening and narrowing conversions can be accomplished by explicitly casting a value • To cast, the type is put in parentheses in front of the value/variable being converted – for example, if total and count are integers, but we want a floating point result when dividing them, we can explicitly cast total to be a float: result = (float) total / count;
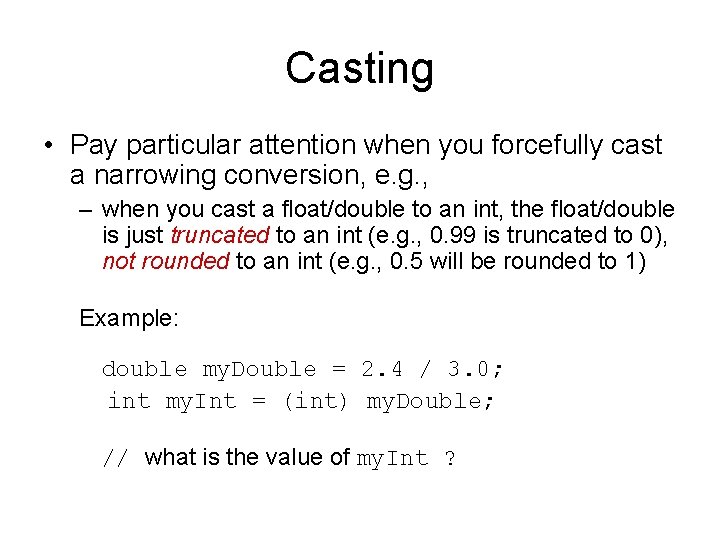
Casting • Pay particular attention when you forcefully cast a narrowing conversion, e. g. , – when you cast a float/double to an int, the float/double is just truncated to an int (e. g. , 0. 99 is truncated to 0), not rounded to an int (e. g. , 0. 5 will be rounded to 1) Example: double my. Double = 2. 4 / 3. 0; int my. Int = (int) my. Double; // what is the value of my. Int ?
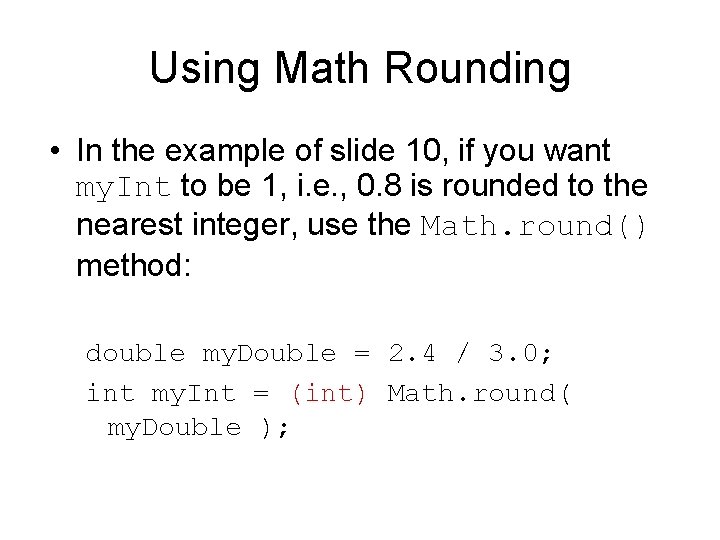
Using Math Rounding • In the example of slide 10, if you want my. Int to be 1, i. e. , 0. 8 is rounded to the nearest integer, use the Math. round() method: double my. Double = 2. 4 / 3. 0; int my. Int = (int) Math. round( my. Double );
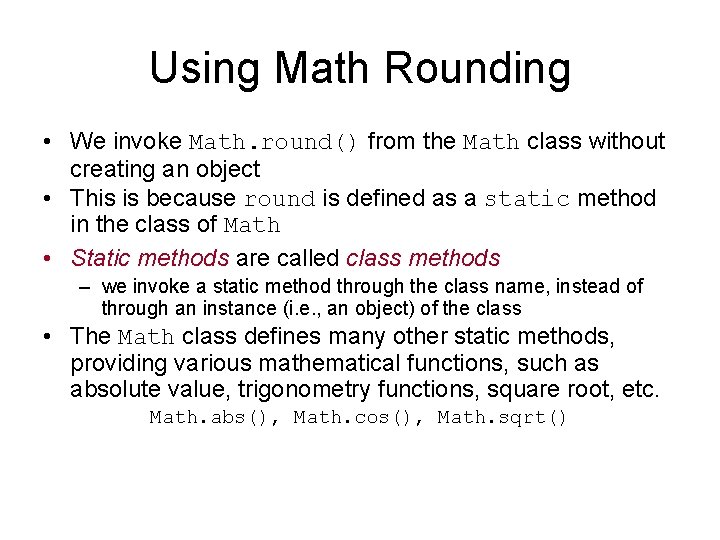
Using Math Rounding • We invoke Math. round() from the Math class without creating an object • This is because round is defined as a static method in the class of Math • Static methods are called class methods – we invoke a static method through the class name, instead of through an instance (i. e. , an object) of the class • The Math class defines many other static methods, providing various mathematical functions, such as absolute value, trigonometry functions, square root, etc. Math. abs(), Math. cos(), Math. sqrt()
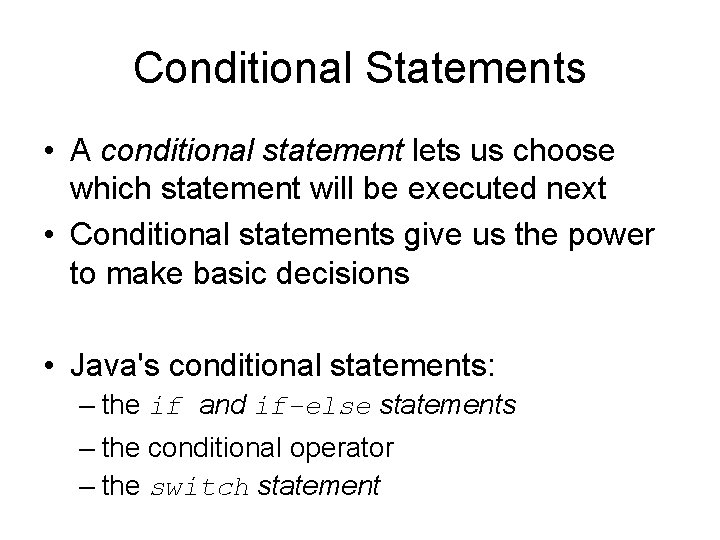
Conditional Statements • A conditional statement lets us choose which statement will be executed next • Conditional statements give us the power to make basic decisions • Java's conditional statements: – the if and if-else statements – the conditional operator – the switch statement
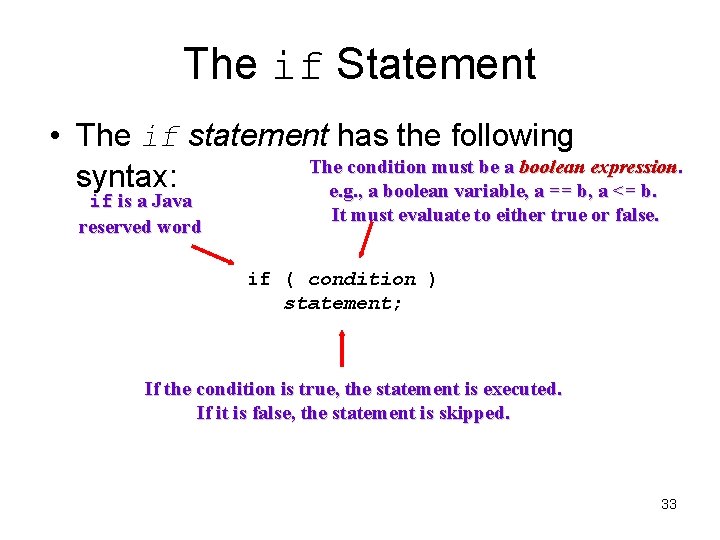
The if Statement • The if statement has the following The condition must be a boolean expression. syntax: e. g. , a boolean variable, a == b, a <= b. if is a Java reserved word It must evaluate to either true or false. if ( condition ) statement; If the condition is true, the statement is executed. If it is false, the statement is skipped. 33
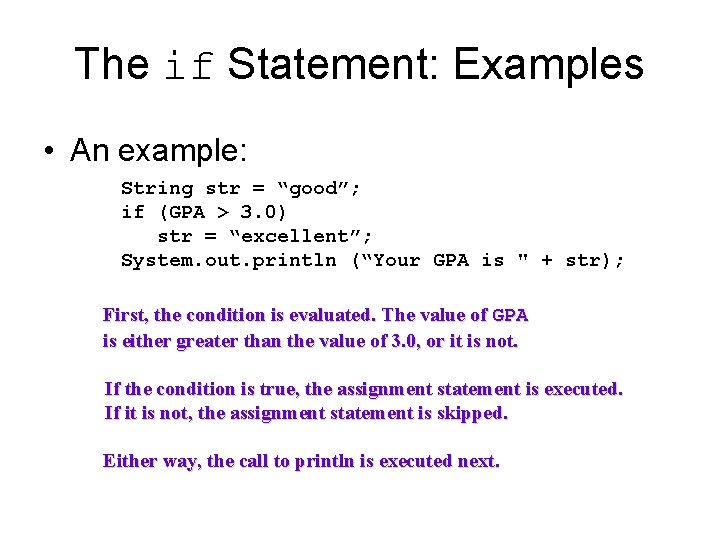
The if Statement: Examples • An example: String str = “good”; if (GPA > 3. 0) str = “excellent”; System. out. println (“Your GPA is " + str); First, the condition is evaluated. The value of GPA is either greater than the value of 3. 0, or it is not. If the condition is true, the assignment statement is executed. If it is not, the assignment statement is skipped. Either way, the call to println is executed next.
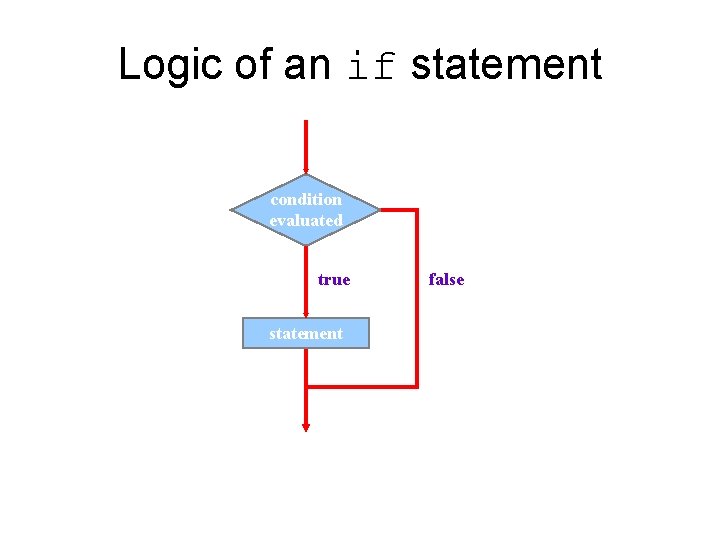
Logic of an if statement condition evaluated true statement false
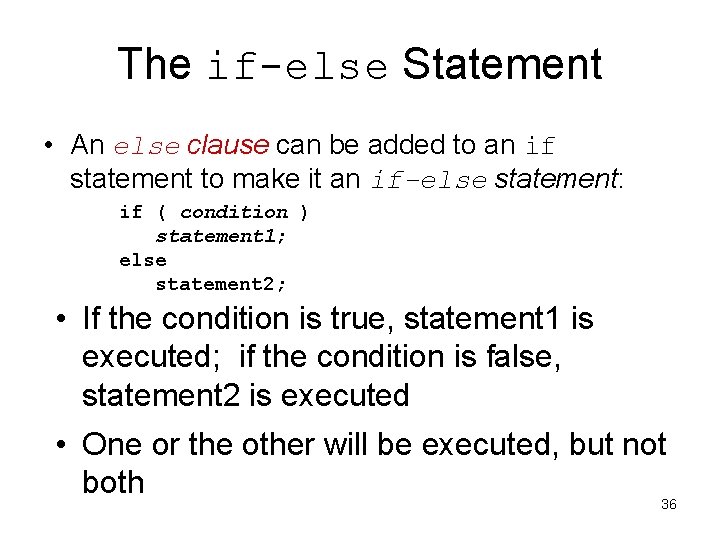
The if-else Statement • An else clause can be added to an if statement to make it an if-else statement: if ( condition ) statement 1; else statement 2; • If the condition is true, statement 1 is executed; if the condition is false, statement 2 is executed • One or the other will be executed, but not both 36
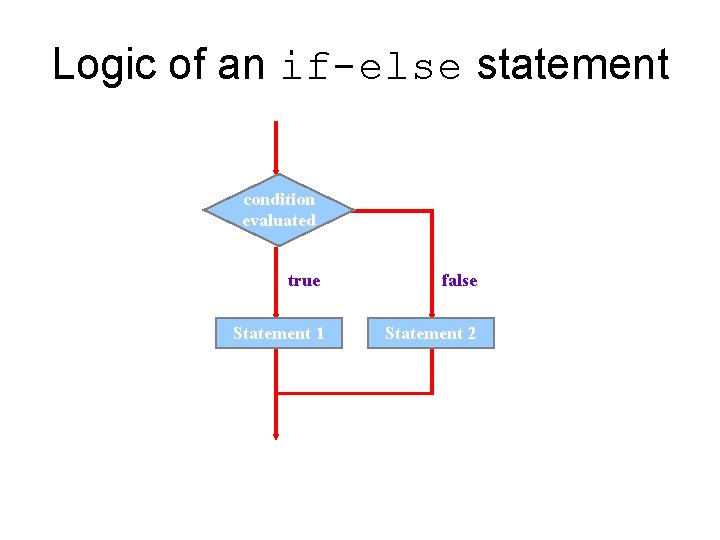
Logic of an if-else statement condition evaluated true false Statement 1 Statement 2
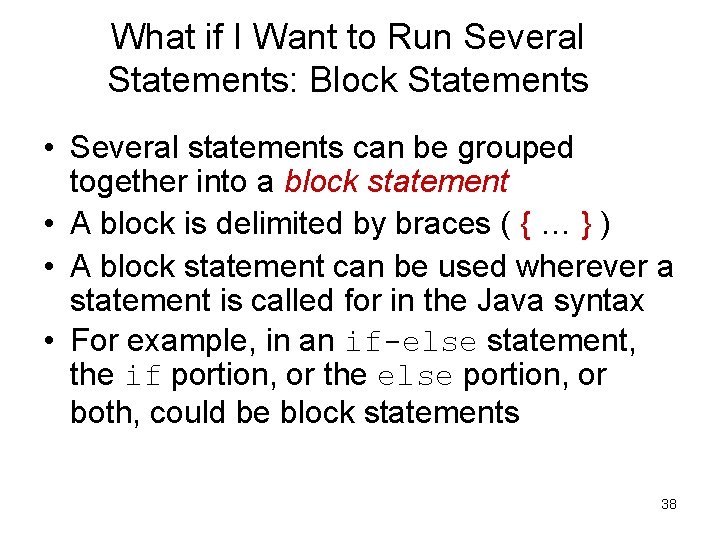
What if I Want to Run Several Statements: Block Statements • Several statements can be grouped together into a block statement • A block is delimited by braces ( { … } ) • A block statement can be used wherever a statement is called for in the Java syntax • For example, in an if-else statement, the if portion, or the else portion, or both, could be block statements 38
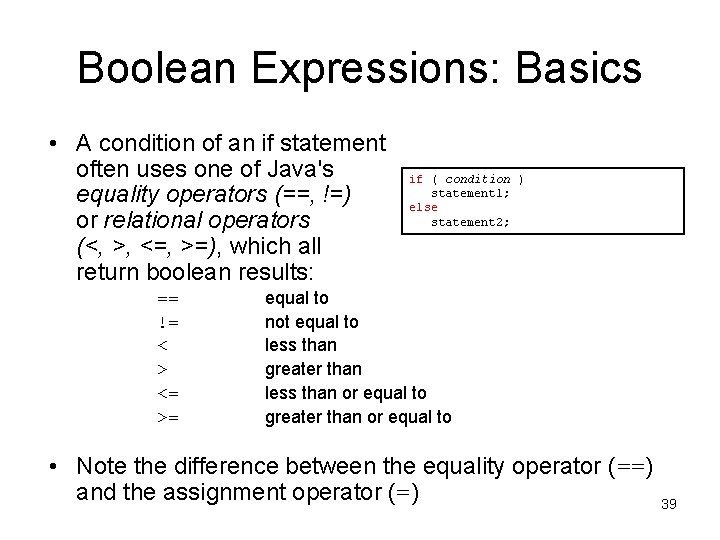
Boolean Expressions: Basics • A condition of an if statement often uses one of Java's equality operators (==, !=) or relational operators (<, >, <=, >=), which all return boolean results: == != < > <= >= if ( condition ) statement 1; else statement 2; equal to not equal to less than greater than less than or equal to greater than or equal to • Note the difference between the equality operator (==) and the assignment operator (=) 39
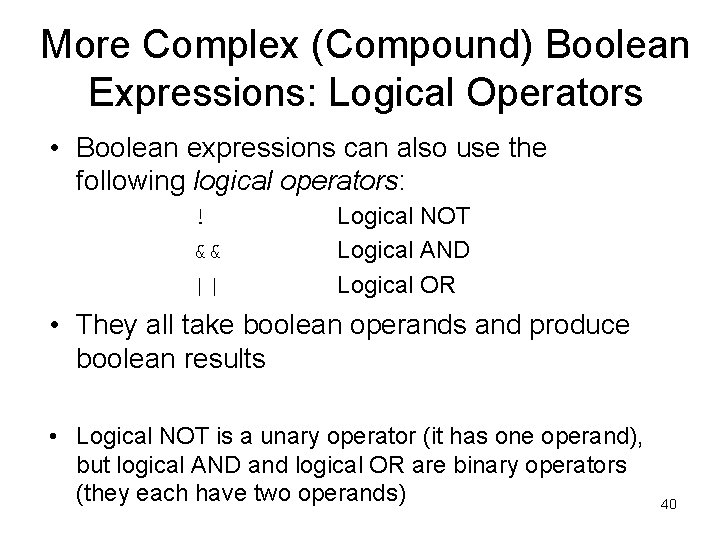
More Complex (Compound) Boolean Expressions: Logical Operators • Boolean expressions can also use the following logical operators: ! && || Logical NOT Logical AND Logical OR • They all take boolean operands and produce boolean results • Logical NOT is a unary operator (it has one operand), but logical AND and logical OR are binary operators (they each have two operands) 40
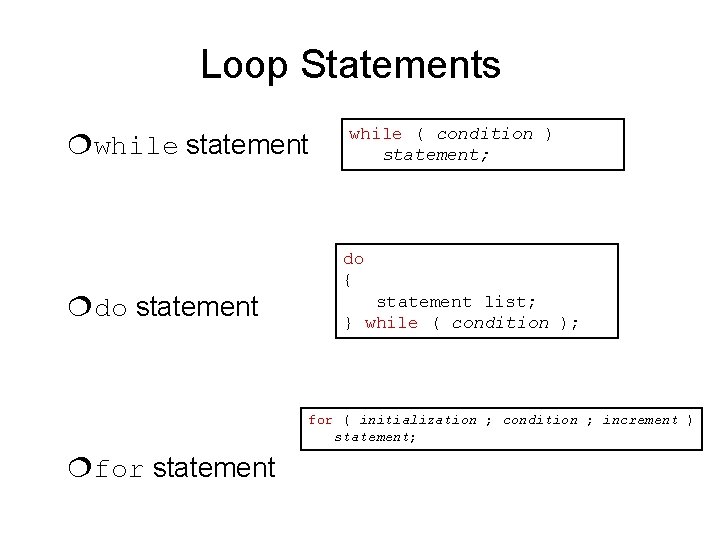
Loop Statements ¦while statement ¦do statement while ( condition ) statement; do { statement list; } while ( condition ); for ( initialization ; condition ; increment ) statement; ¦for statement
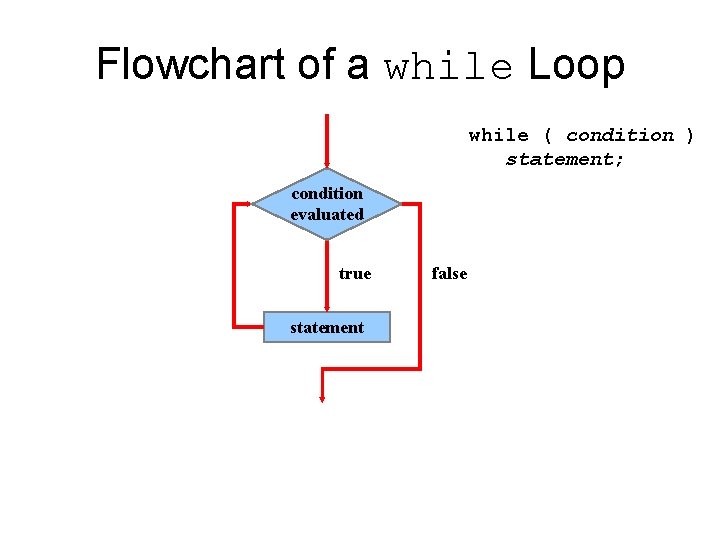
Flowchart of a while Loop while ( condition ) statement; condition evaluated true statement false
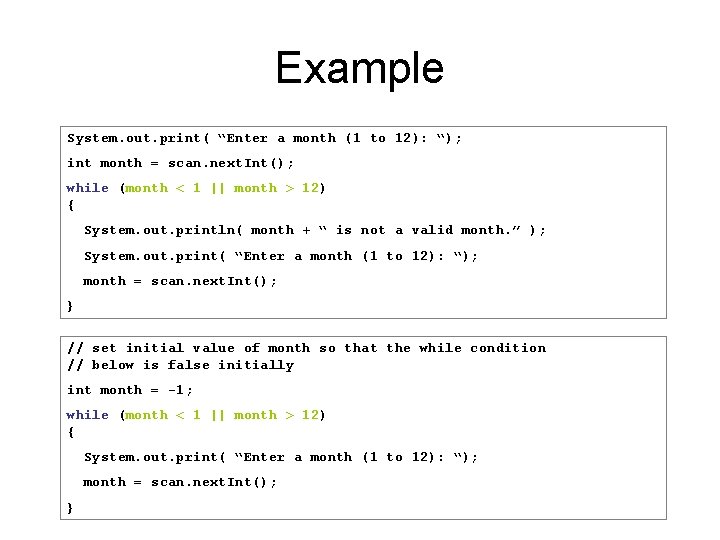
Example System. out. print( “Enter a month (1 to 12): “); int month = scan. next. Int(); while (month < 1 || month > 12) { System. out. println( month + “ is not a valid month. ” ); System. out. print( “Enter a month (1 to 12): “); month = scan. next. Int(); } // set initial value of month so that the while condition // below is false initially int month = -1; while (month < 1 || month > 12) { System. out. print( “Enter a month (1 to 12): “); month = scan. next. Int(); }
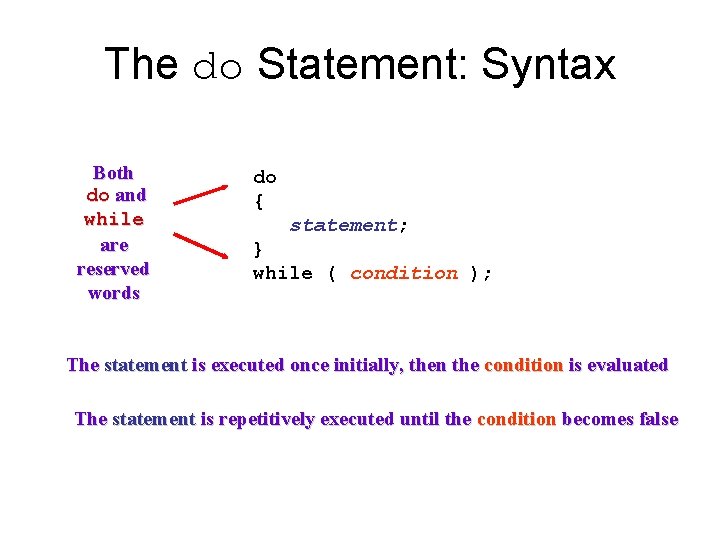
The do Statement: Syntax Both do and while are reserved words do { statement; } while ( condition ); The statement is executed once initially, then the condition is evaluated The statement is repetitively executed until the condition becomes false
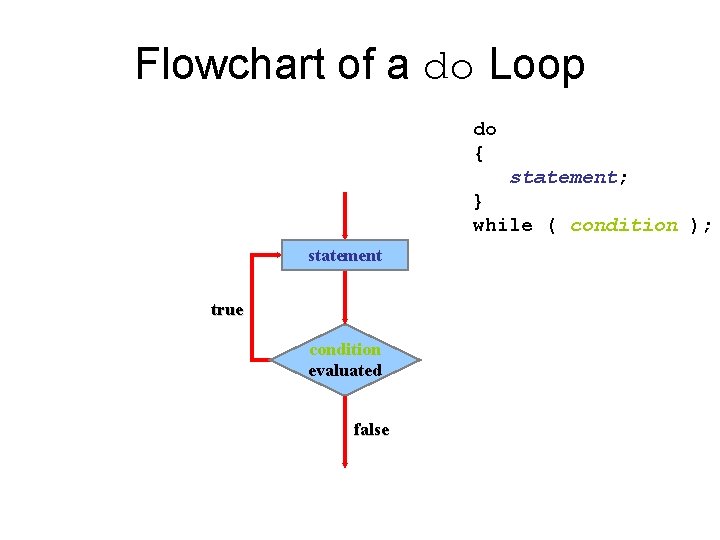
Flowchart of a do Loop do { statement; } while ( condition ); statement true condition evaluated false
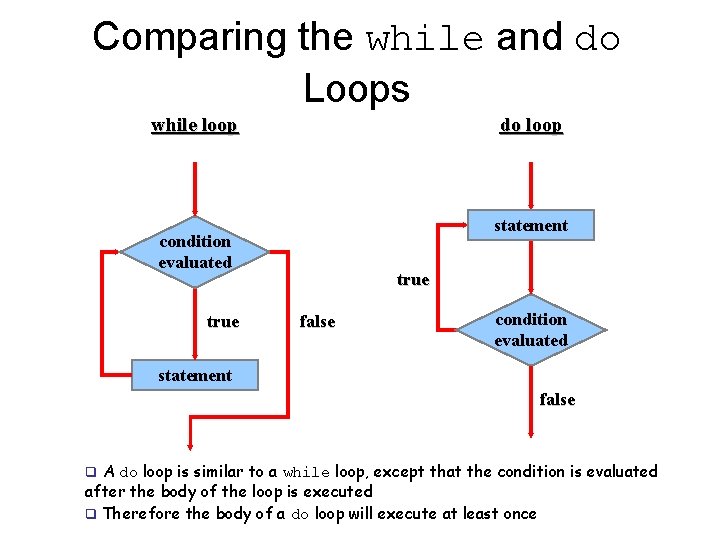
Comparing the while and do Loops while loop do loop statement condition evaluated true false condition evaluated statement false q A do loop is similar to a while loop, except that the condition is evaluated after the body of the loop is executed q Therefore the body of a do loop will execute at least once
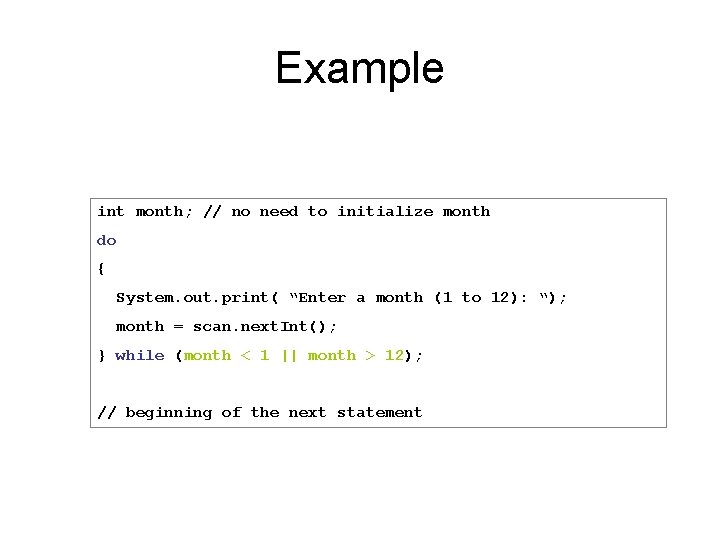
Example int month; // no need to initialize month do { System. out. print( “Enter a month (1 to 12): “); month = scan. next. Int(); } while (month < 1 || month > 12); // beginning of the next statement
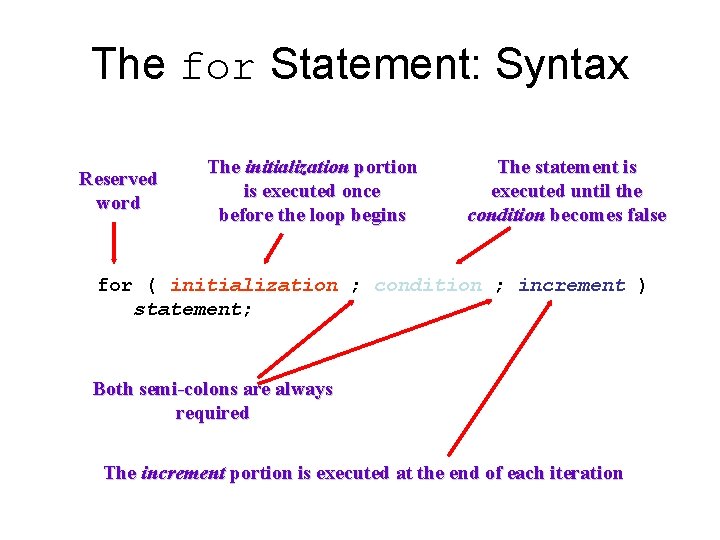
The for Statement: Syntax Reserved word The initialization portion is executed once before the loop begins The statement is executed until the condition becomes false for ( initialization ; condition ; increment ) statement; Both semi-colons are always required The increment portion is executed at the end of each iteration
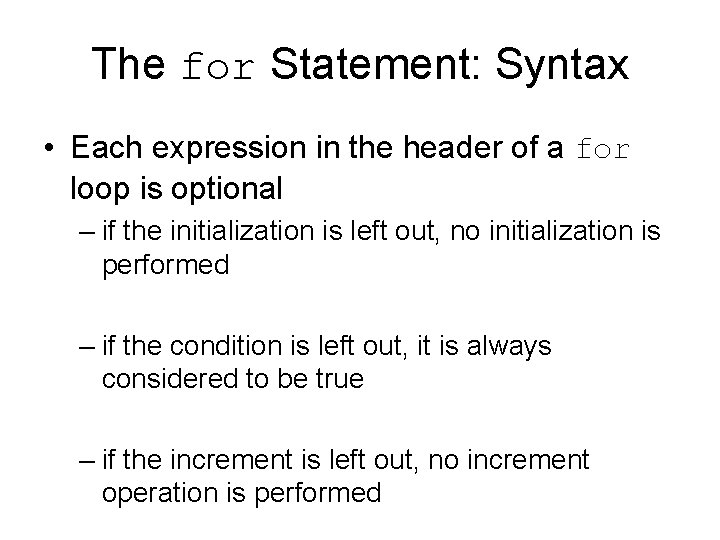
The for Statement: Syntax • Each expression in the header of a for loop is optional – if the initialization is left out, no initialization is performed – if the condition is left out, it is always considered to be true – if the increment is left out, no increment operation is performed
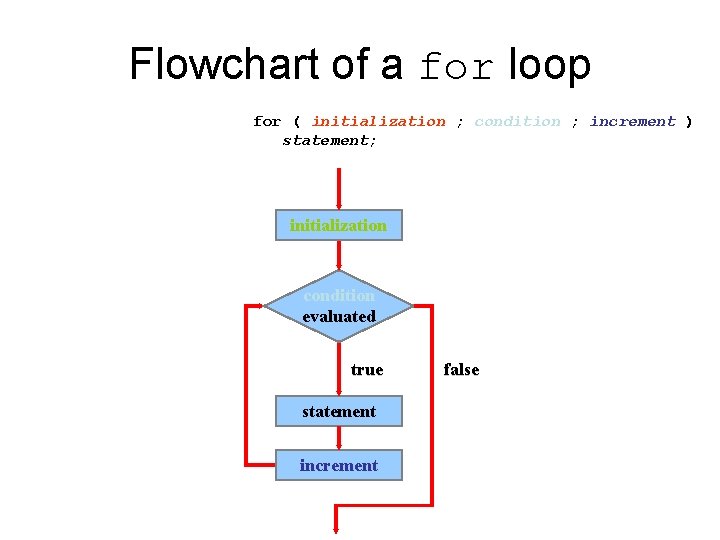
Flowchart of a for loop for ( initialization ; condition ; increment ) statement; initialization condition evaluated true statement increment false
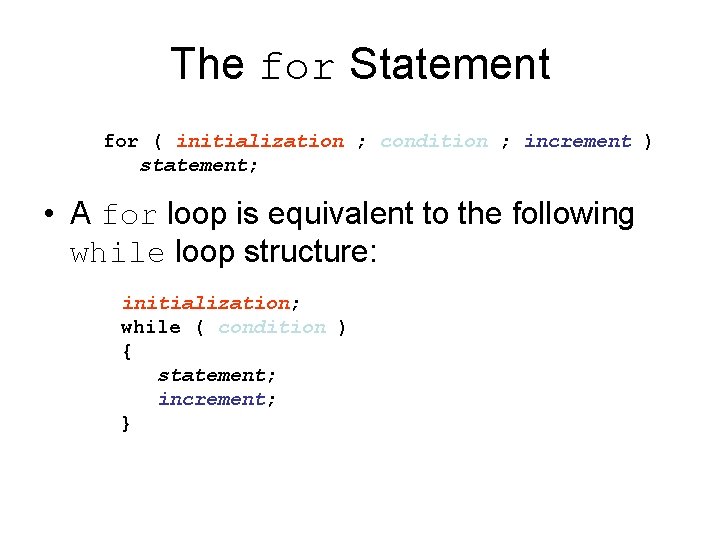
The for Statement for ( initialization ; condition ; increment ) statement; • A for loop is equivalent to the following while loop structure: initialization; while ( condition ) { statement; increment; }
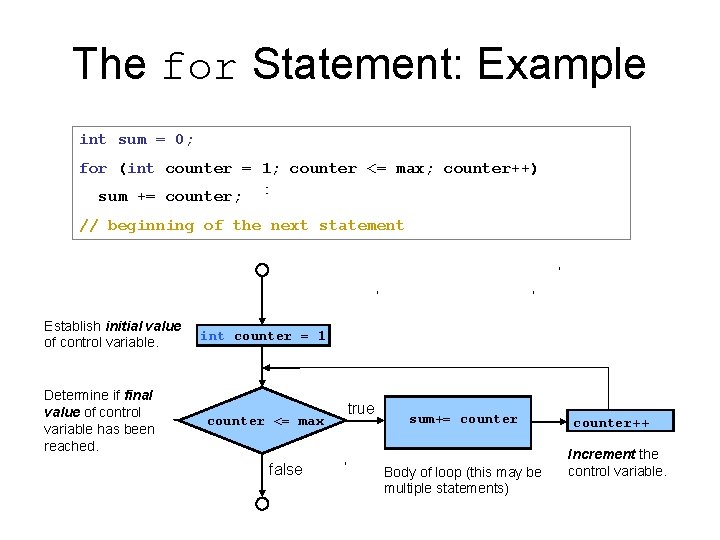
The for Statement: Example int sum = 0; for (int counter = 1; counter <= max; counter++) sum += counter; // beginning of the next statement Establish initial value of control variable. Determine if final value of control variable has been reached. int counter = 1 counter <= max false true sum+= counter Body of loop (this may be multiple statements) counter++ Increment the control variable.