The Class Chain first Node The Class Chain
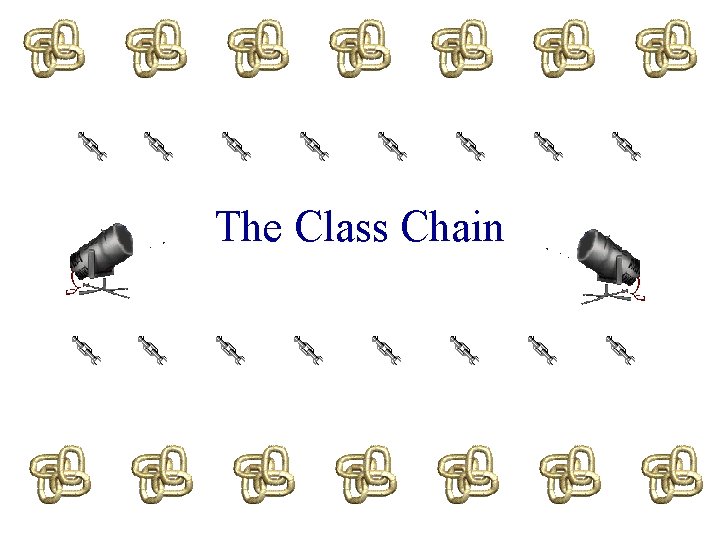
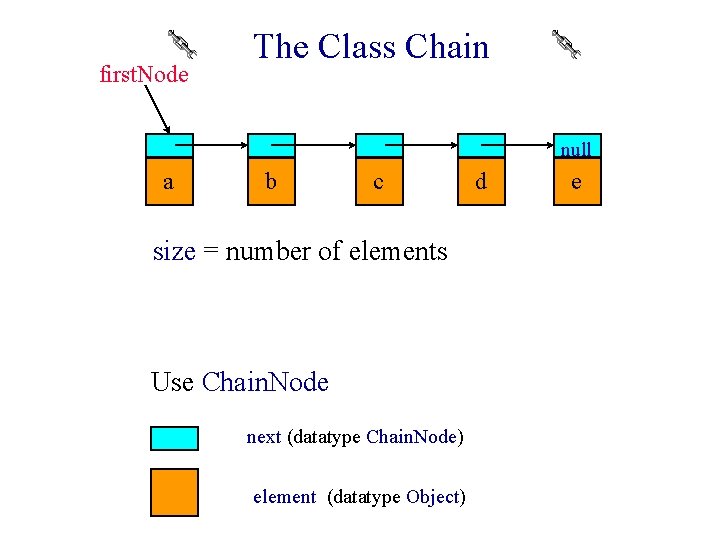
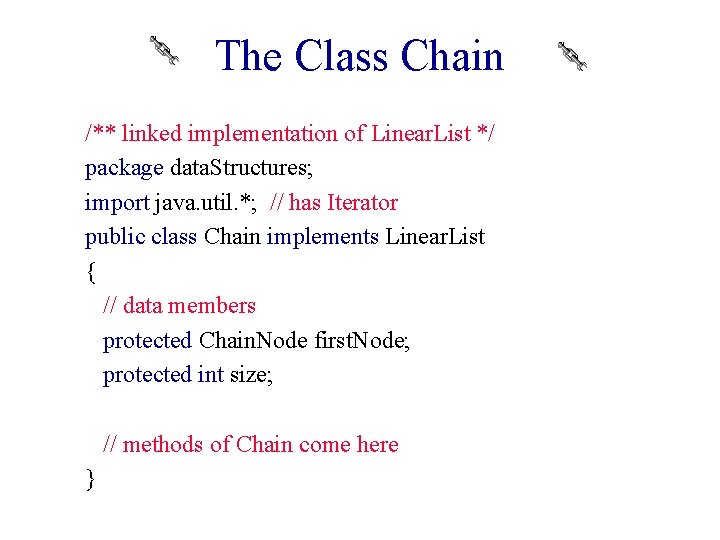
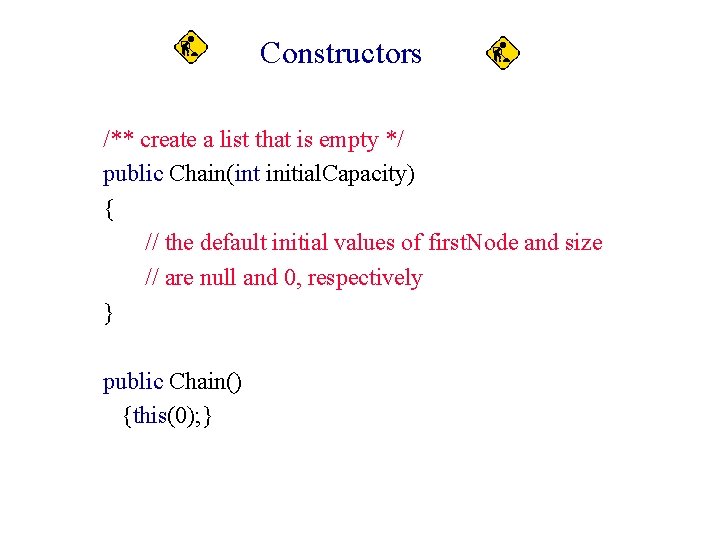
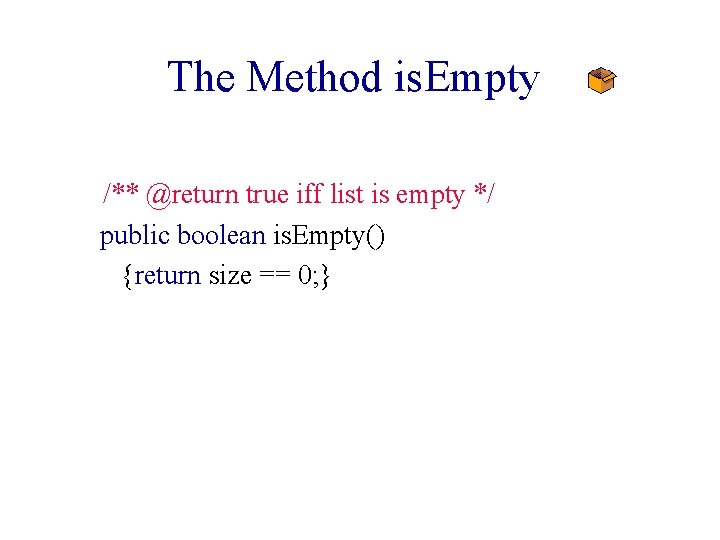
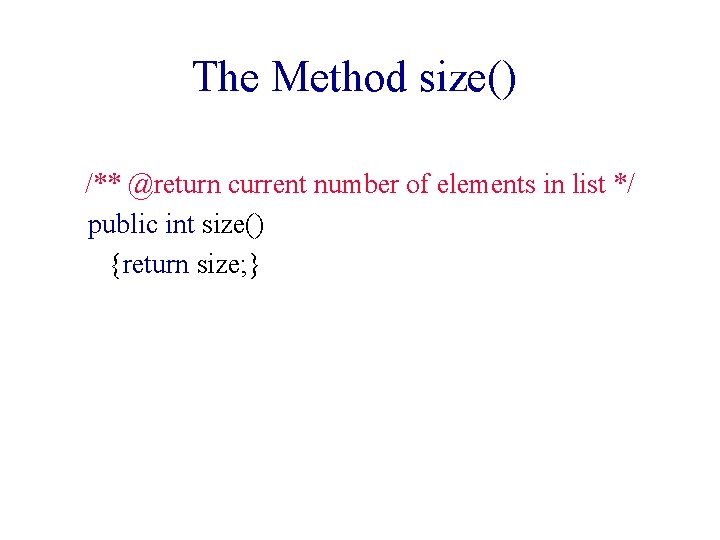
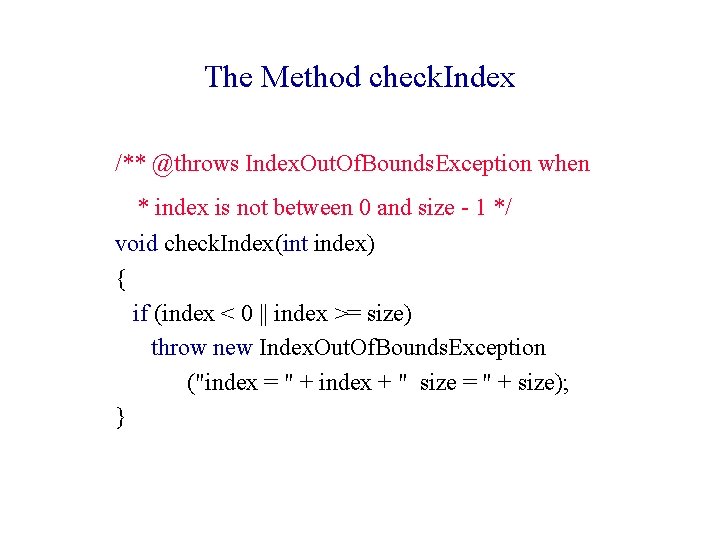
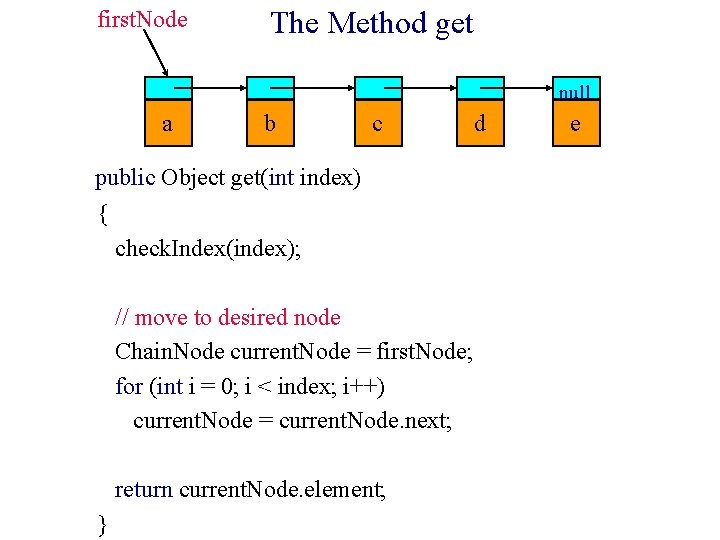
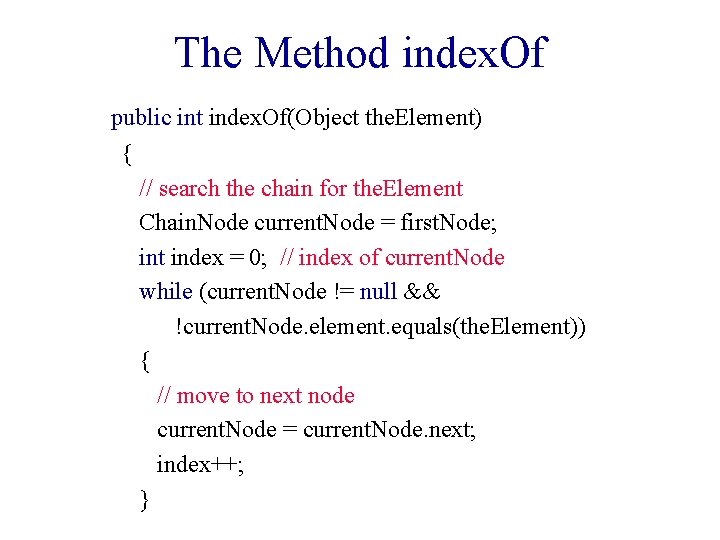
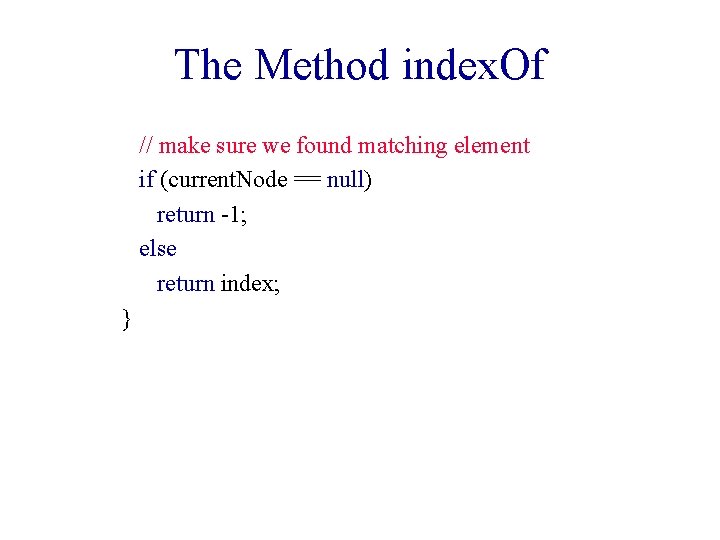
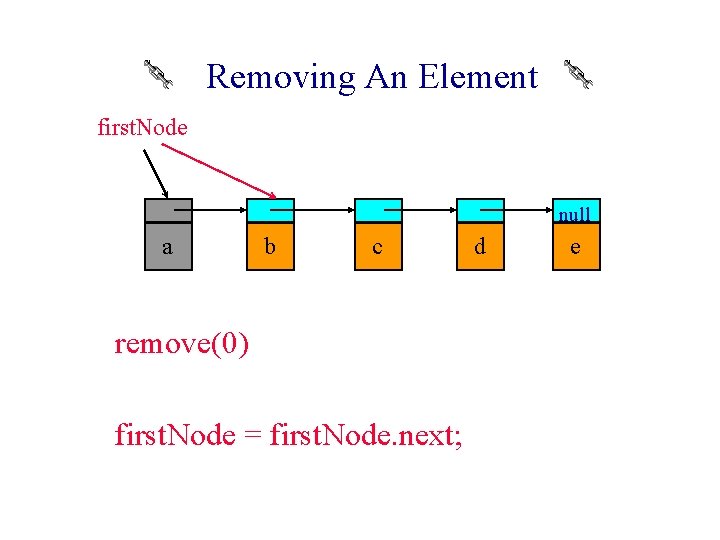
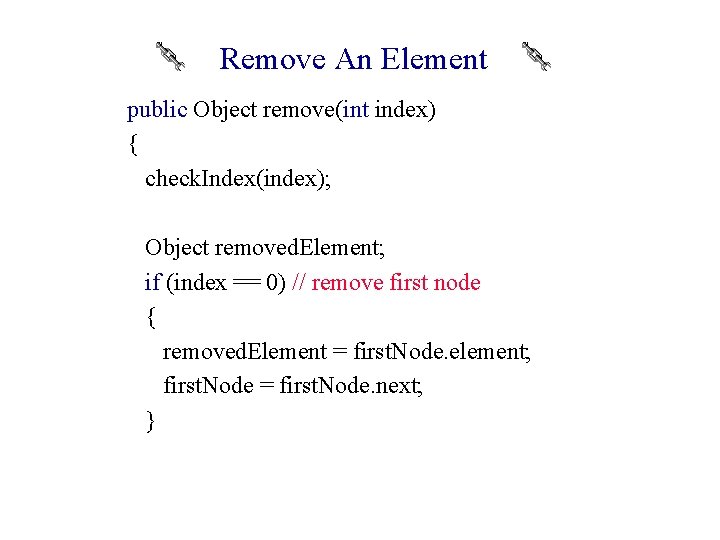
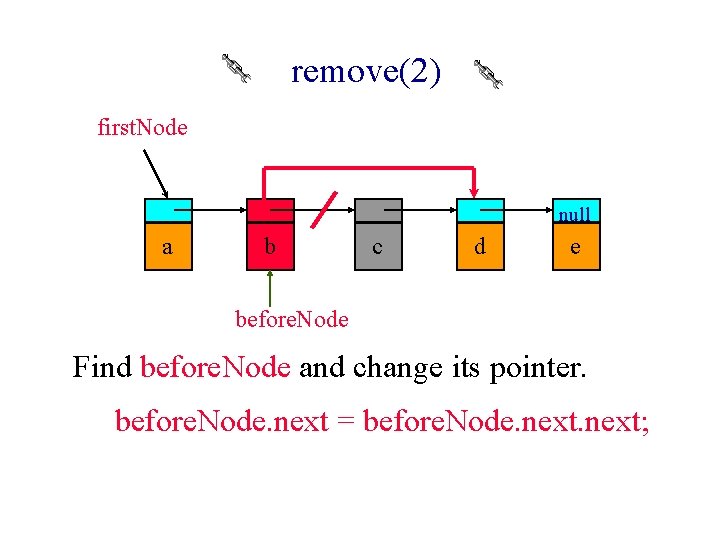
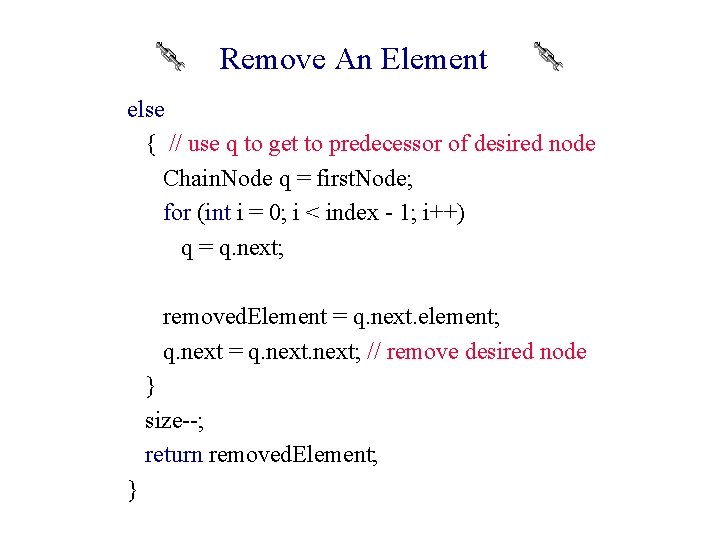
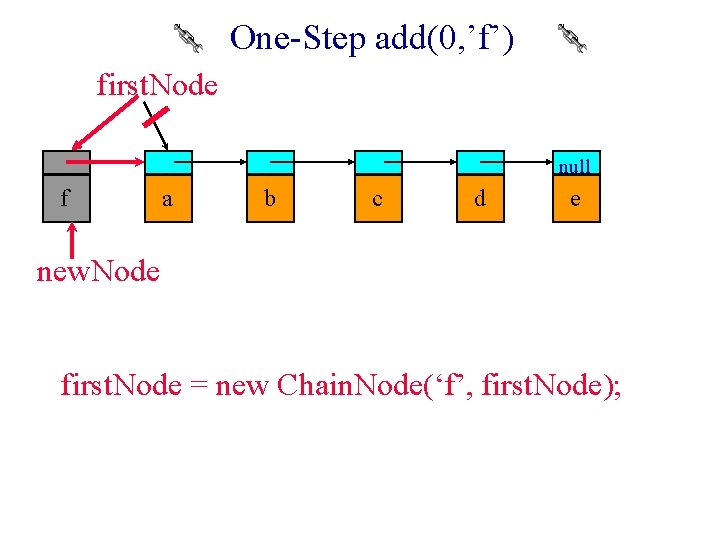
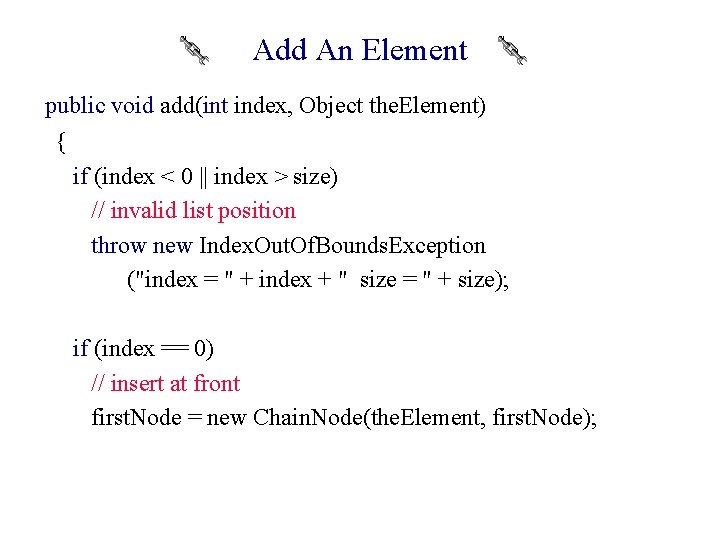
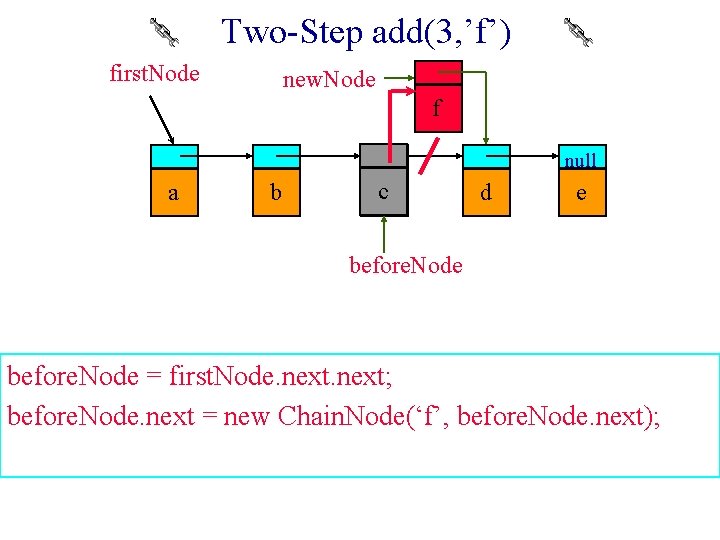
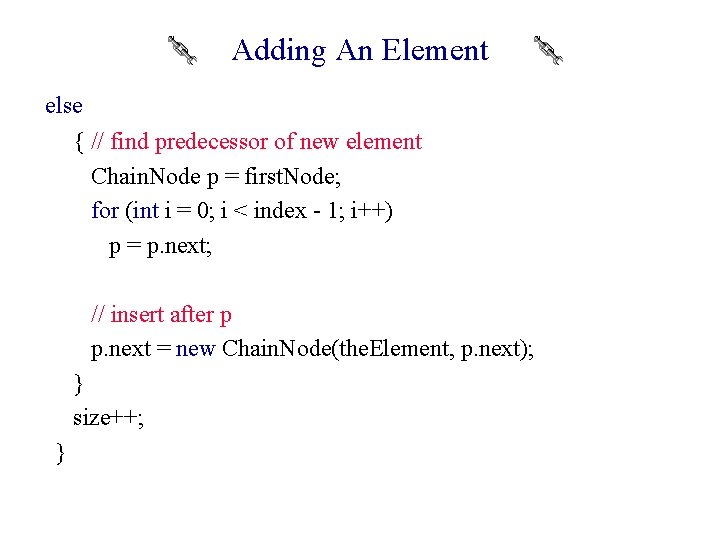
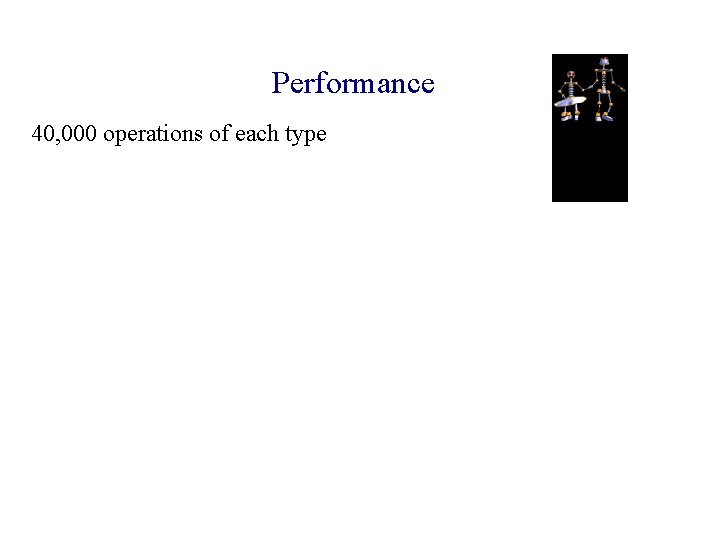
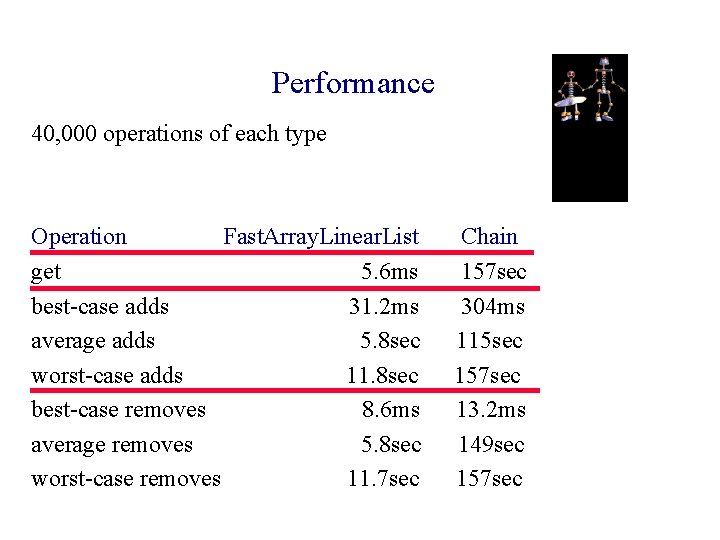
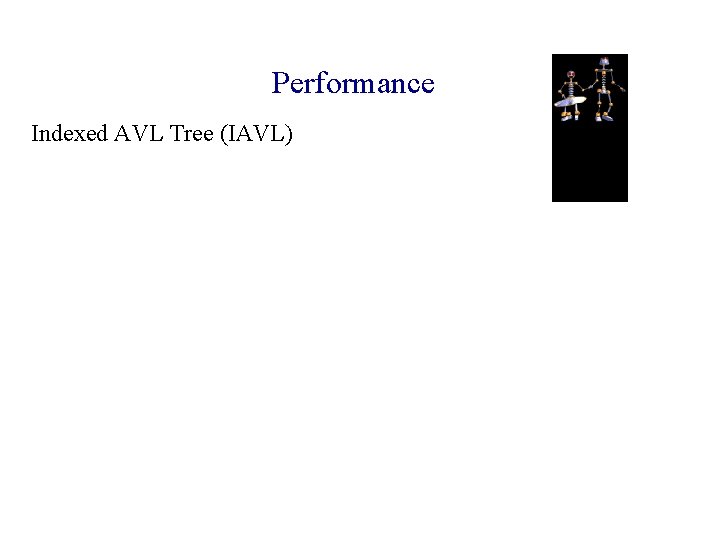
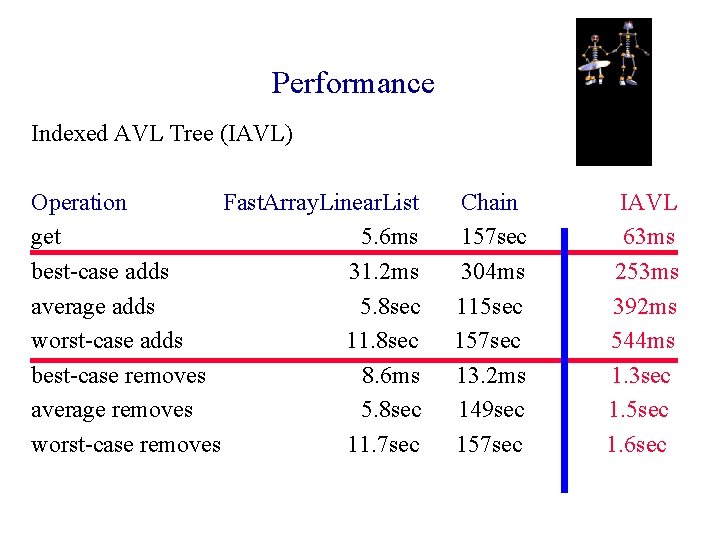
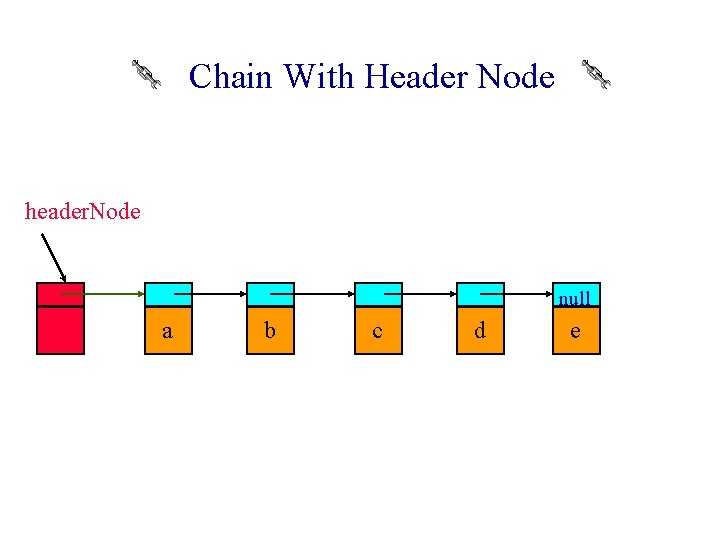
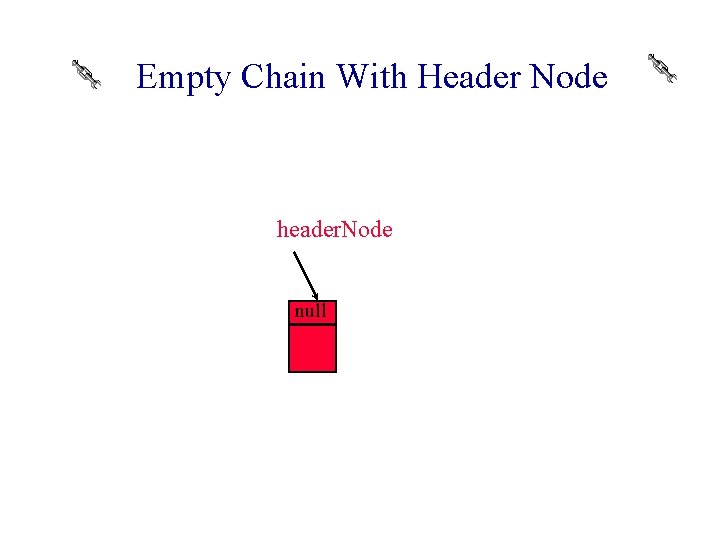
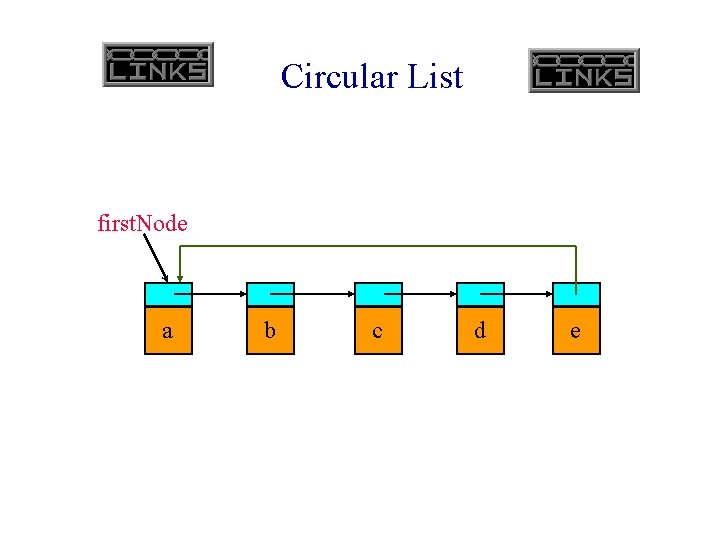
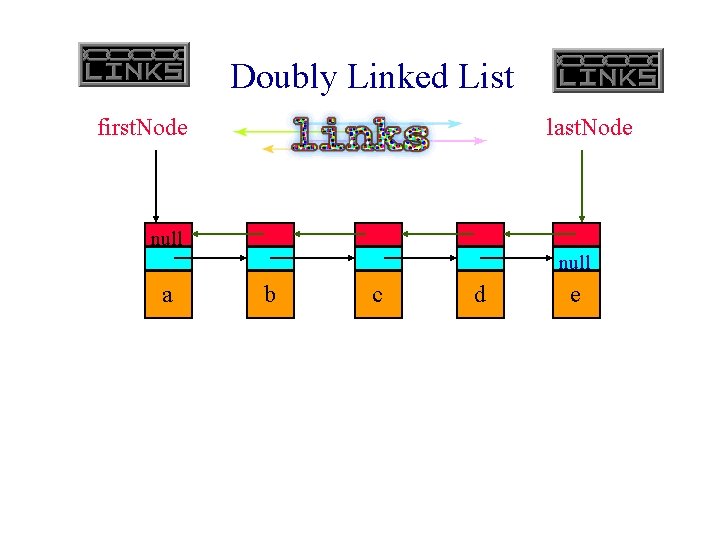
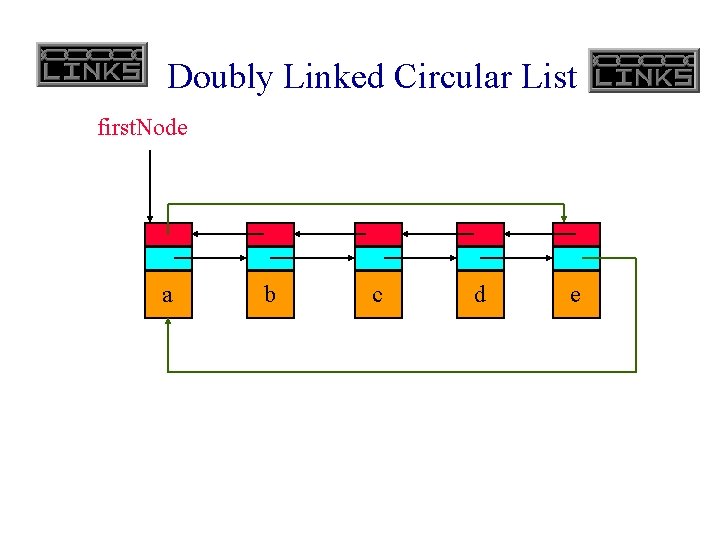
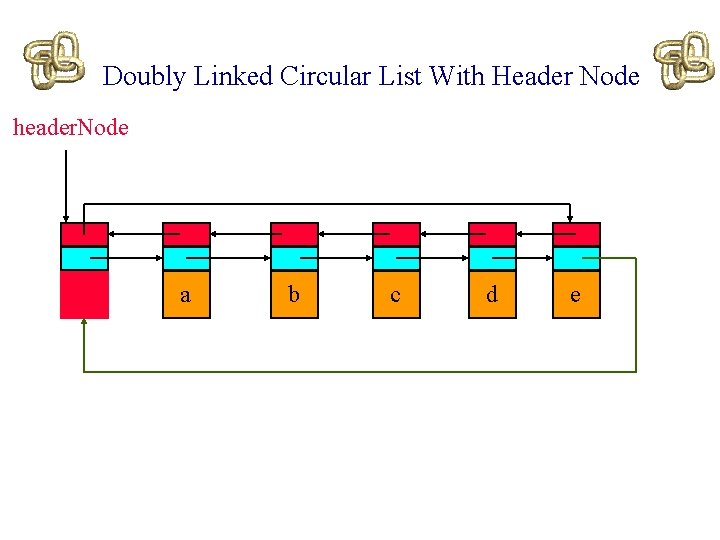
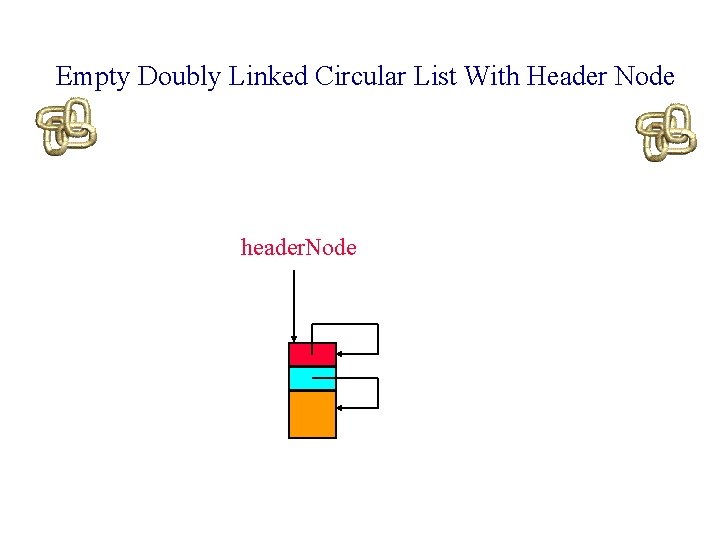
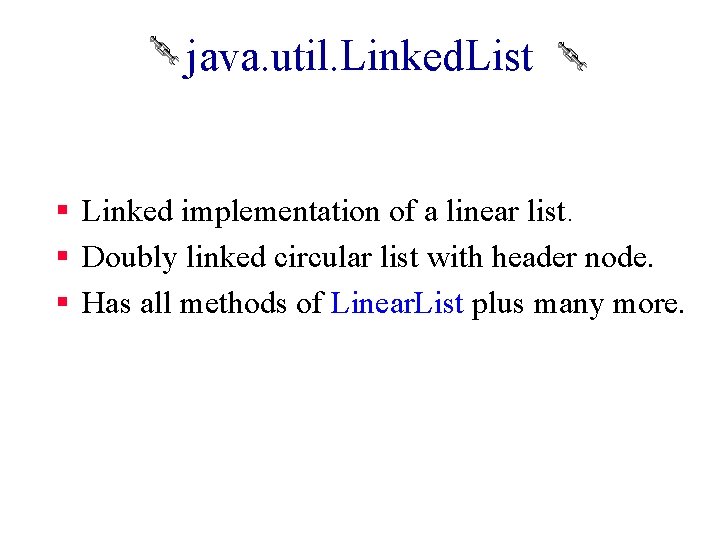
- Slides: 30
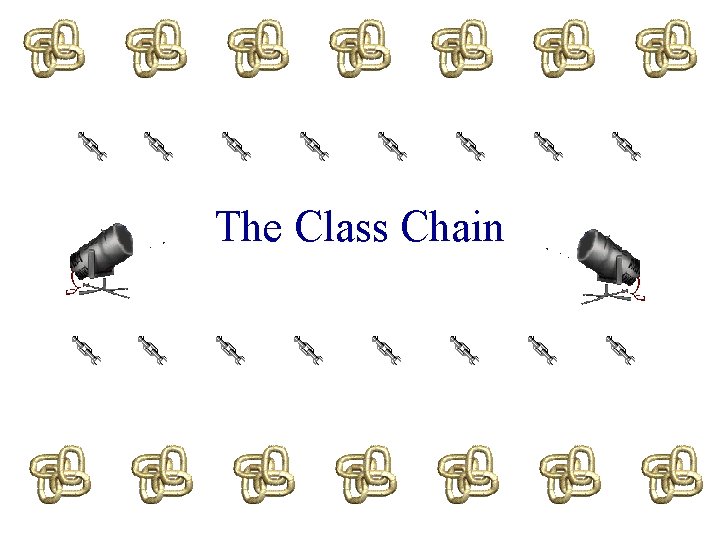
The Class Chain
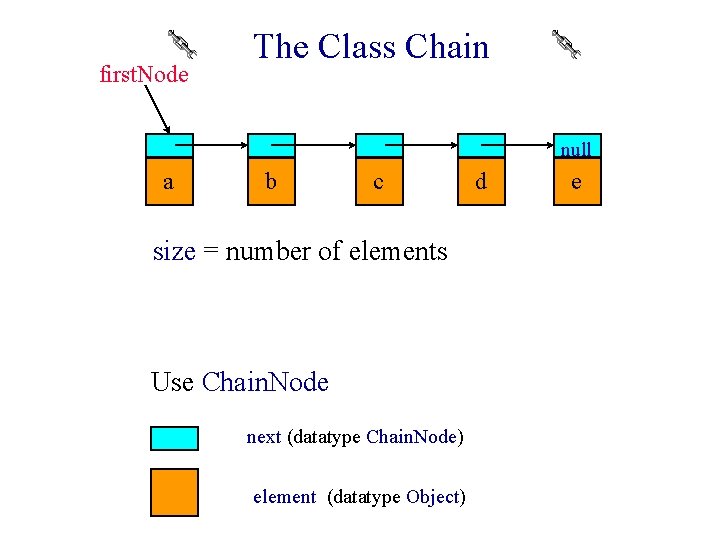
first. Node The Class Chain null a b c size = number of elements Use Chain. Node next (datatype Chain. Node) element (datatype Object) d e
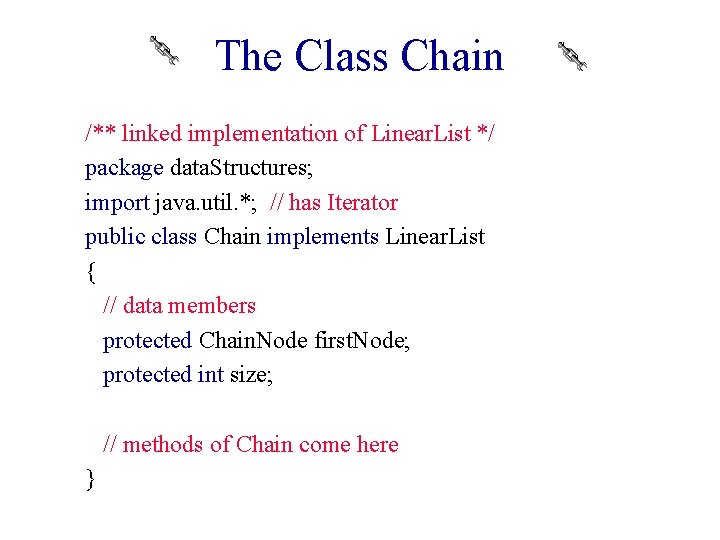
The Class Chain /** linked implementation of Linear. List */ package data. Structures; import java. util. *; // has Iterator public class Chain implements Linear. List { // data members protected Chain. Node first. Node; protected int size; // methods of Chain come here }
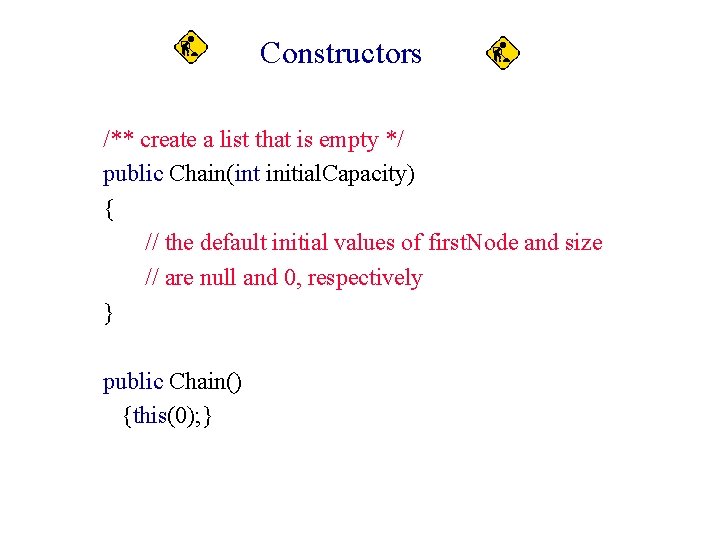
Constructors /** create a list that is empty */ public Chain(int initial. Capacity) { // the default initial values of first. Node and size // are null and 0, respectively } public Chain() {this(0); }
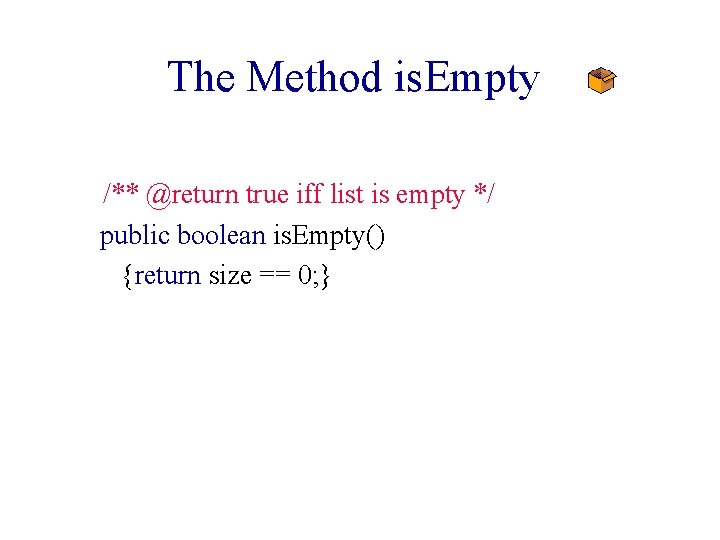
The Method is. Empty /** @return true iff list is empty */ public boolean is. Empty() {return size == 0; }
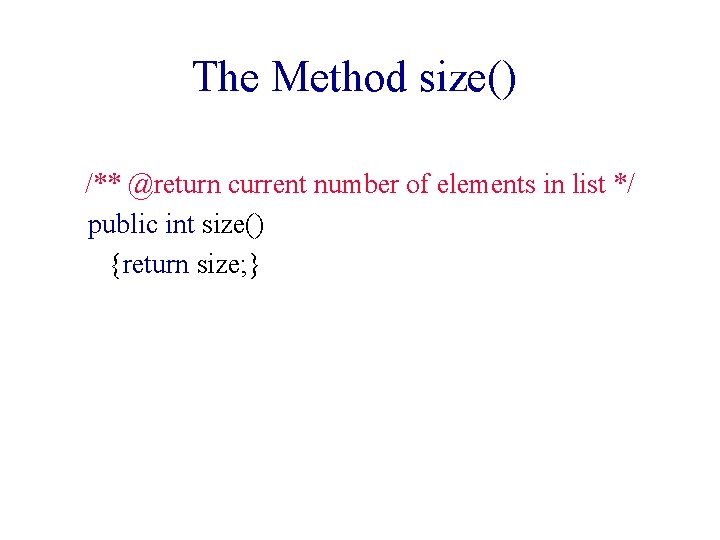
The Method size() /** @return current number of elements in list */ public int size() {return size; }
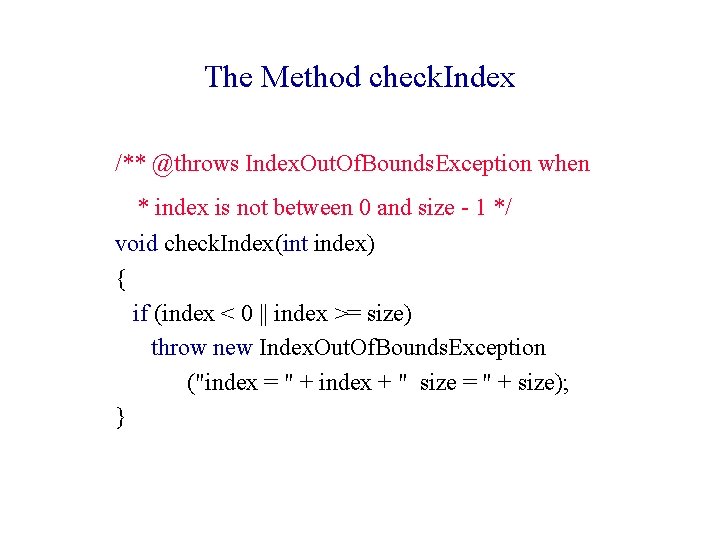
The Method check. Index /** @throws Index. Out. Of. Bounds. Exception when * index is not between 0 and size - 1 */ void check. Index(int index) { if (index < 0 || index >= size) throw new Index. Out. Of. Bounds. Exception ("index = " + index + " size = " + size); }
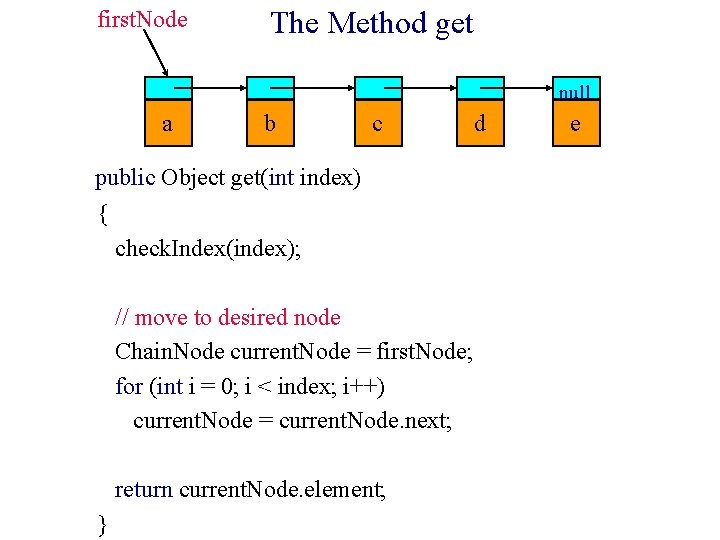
first. Node The Method get null a b c public Object get(int index) { check. Index(index); // move to desired node Chain. Node current. Node = first. Node; for (int i = 0; i < index; i++) current. Node = current. Node. next; return current. Node. element; } d e
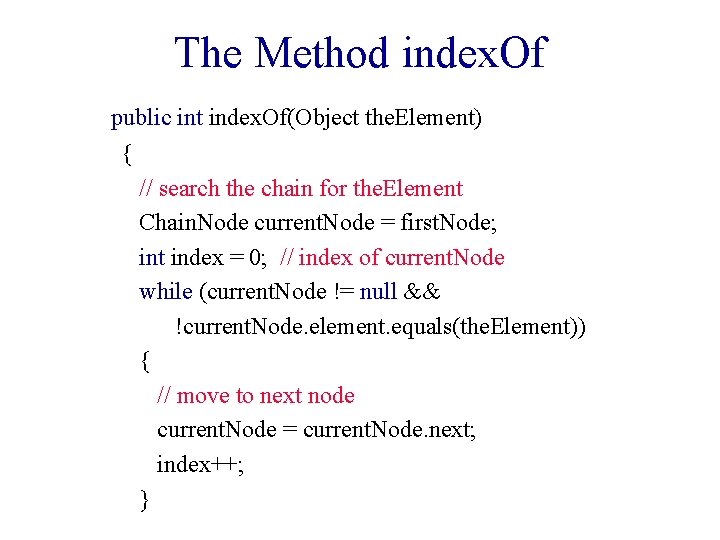
The Method index. Of public int index. Of(Object the. Element) { // search the chain for the. Element Chain. Node current. Node = first. Node; int index = 0; // index of current. Node while (current. Node != null && !current. Node. element. equals(the. Element)) { // move to next node current. Node = current. Node. next; index++; }
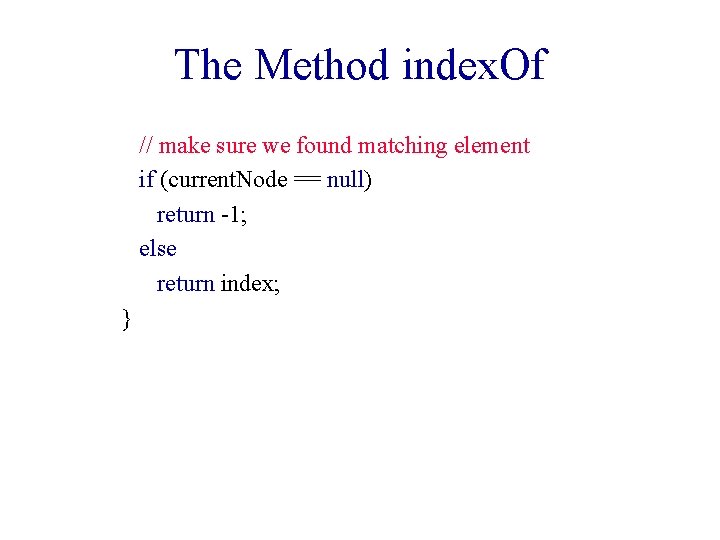
The Method index. Of // make sure we found matching element if (current. Node == null) return -1; else return index; }
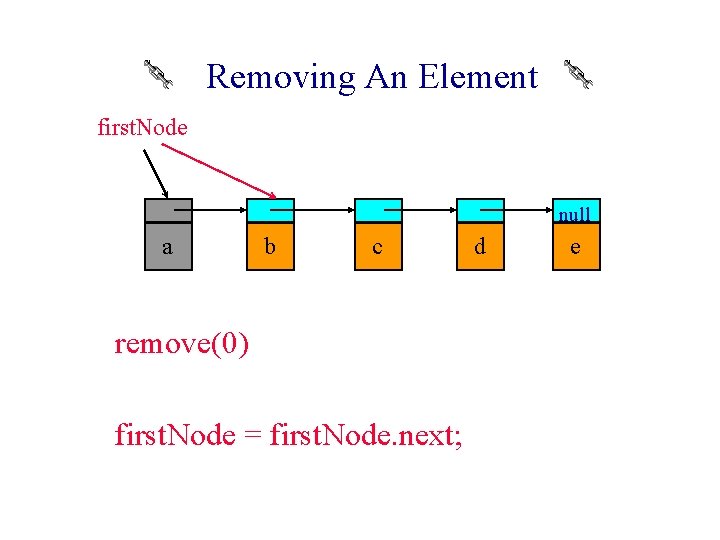
Removing An Element first. Node null a b c remove(0) first. Node = first. Node. next; d e
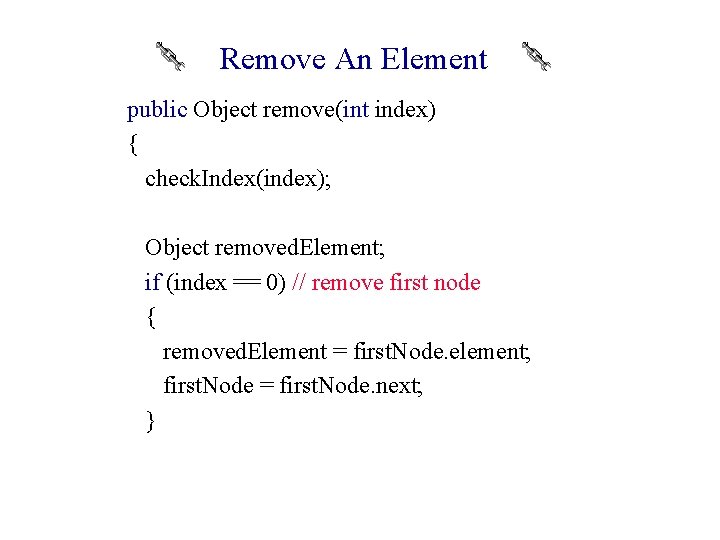
Remove An Element public Object remove(int index) { check. Index(index); Object removed. Element; if (index == 0) // remove first node { removed. Element = first. Node. element; first. Node = first. Node. next; }
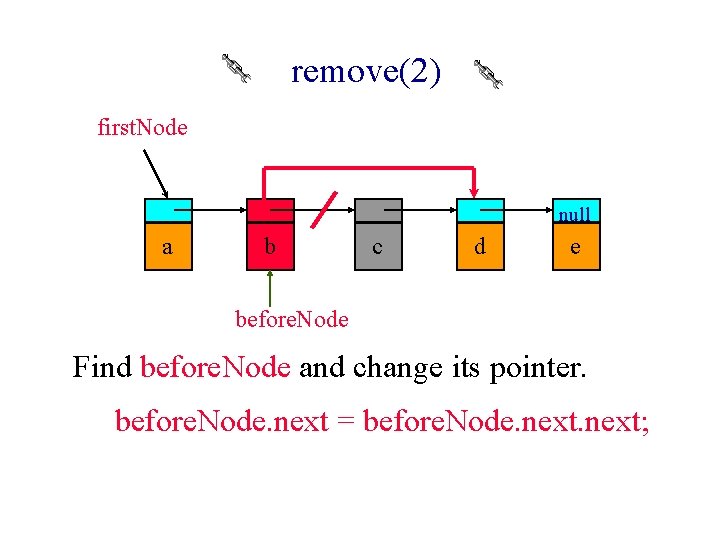
remove(2) first. Node null a b c d e before. Node Find before. Node and change its pointer. before. Node. next = before. Node. next;
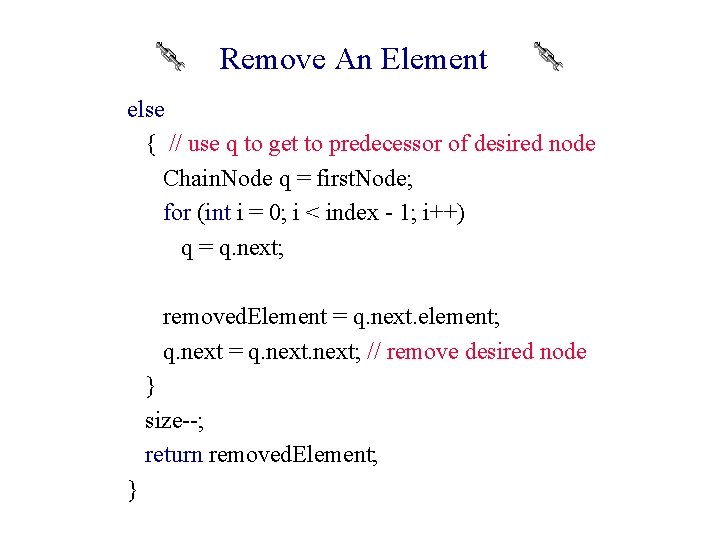
Remove An Element else { // use q to get to predecessor of desired node Chain. Node q = first. Node; for (int i = 0; i < index - 1; i++) q = q. next; removed. Element = q. next. element; q. next = q. next; // remove desired node } size--; return removed. Element; }
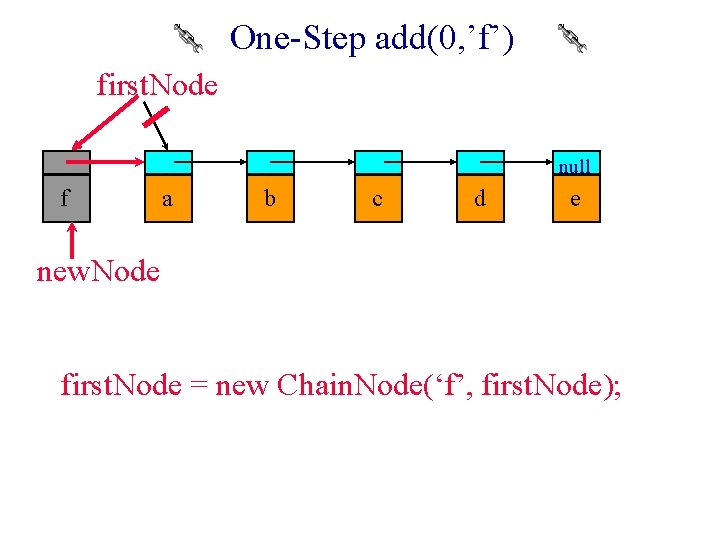
One-Step add(0, ’f’) first. Node null f a b c d e new. Node first. Node = new Chain. Node(‘f’, first. Node);
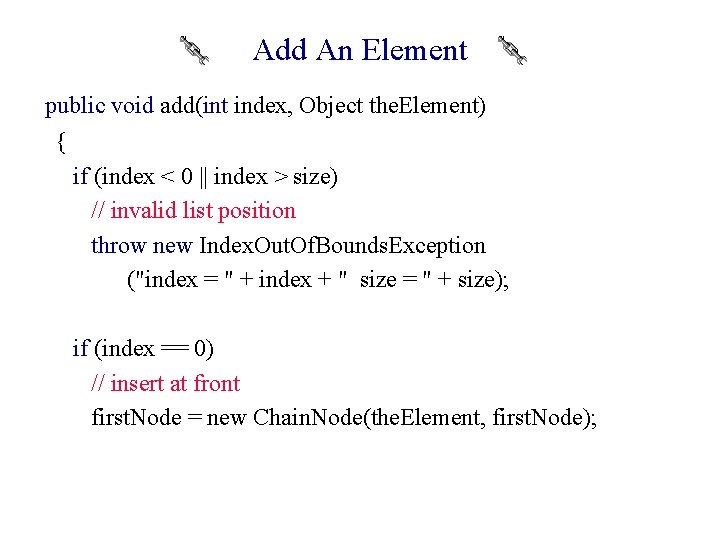
Add An Element public void add(int index, Object the. Element) { if (index < 0 || index > size) // invalid list position throw new Index. Out. Of. Bounds. Exception ("index = " + index + " size = " + size); if (index == 0) // insert at front first. Node = new Chain. Node(the. Element, first. Node);
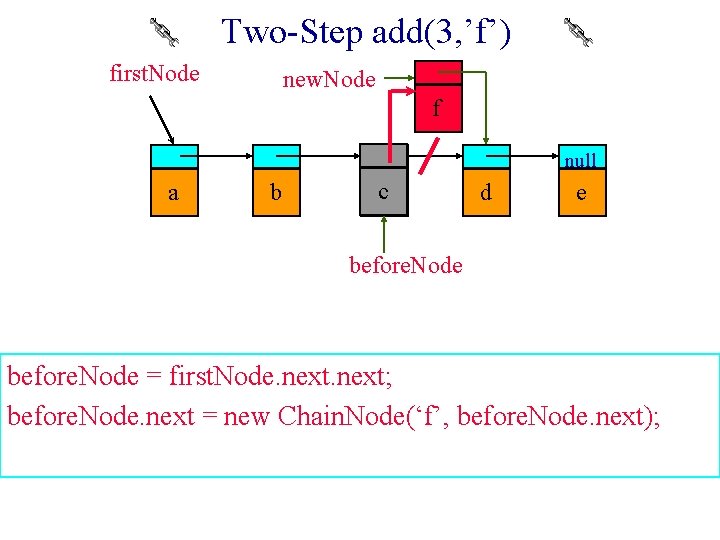
Two-Step add(3, ’f’) first. Node new. Node f null a b c d e before. Node = first. Node. next; before. Node. next = new Chain. Node(‘f’, before. Node. next);
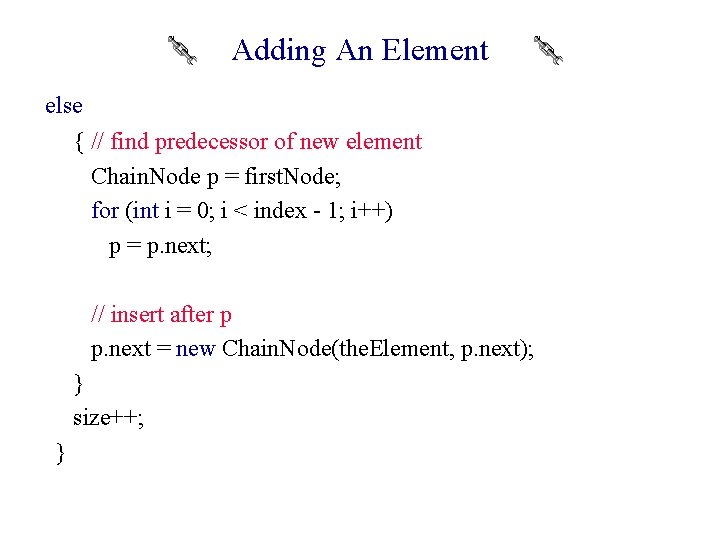
Adding An Element else { // find predecessor of new element Chain. Node p = first. Node; for (int i = 0; i < index - 1; i++) p = p. next; // insert after p p. next = new Chain. Node(the. Element, p. next); } size++; }
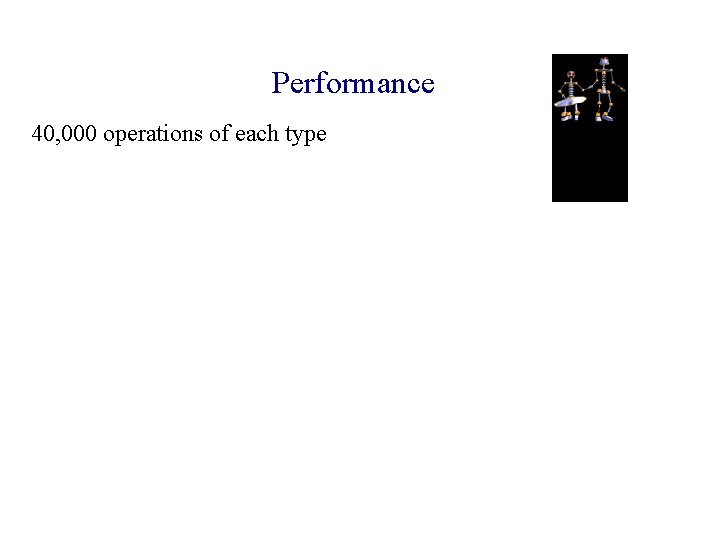
Performance 40, 000 operations of each type
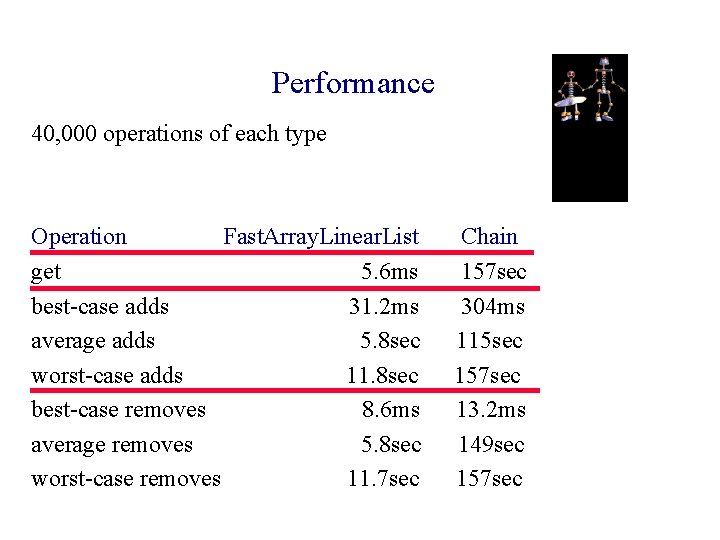
Performance 40, 000 operations of each type Operation Fast. Array. Linear. List get 5. 6 ms best-case adds 31. 2 ms average adds 5. 8 sec worst-case adds 11. 8 sec best-case removes 8. 6 ms average removes 5. 8 sec worst-case removes 11. 7 sec Chain 157 sec 304 ms 115 sec 157 sec 13. 2 ms 149 sec 157 sec
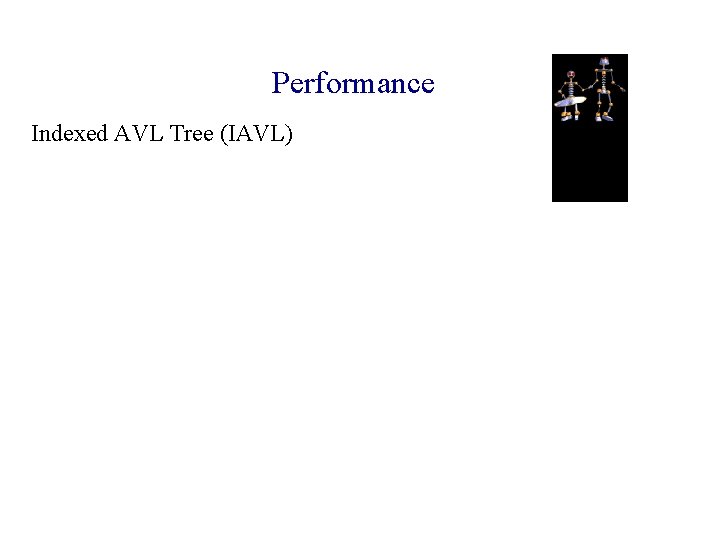
Performance Indexed AVL Tree (IAVL)
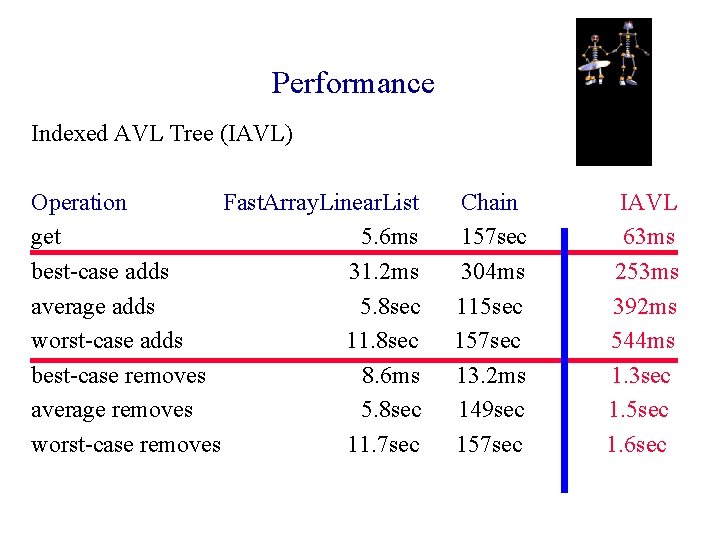
Performance Indexed AVL Tree (IAVL) Operation Fast. Array. Linear. List get 5. 6 ms best-case adds 31. 2 ms average adds 5. 8 sec worst-case adds 11. 8 sec best-case removes 8. 6 ms average removes 5. 8 sec worst-case removes 11. 7 sec Chain 157 sec 304 ms 115 sec 157 sec 13. 2 ms 149 sec 157 sec IAVL 63 ms 253 ms 392 ms 544 ms 1. 3 sec 1. 5 sec 1. 6 sec
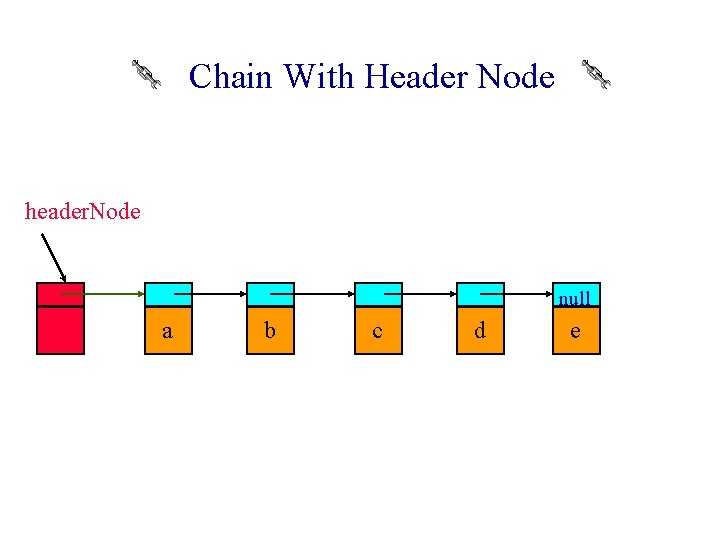
Chain With Header Node header. Node null a b c d e
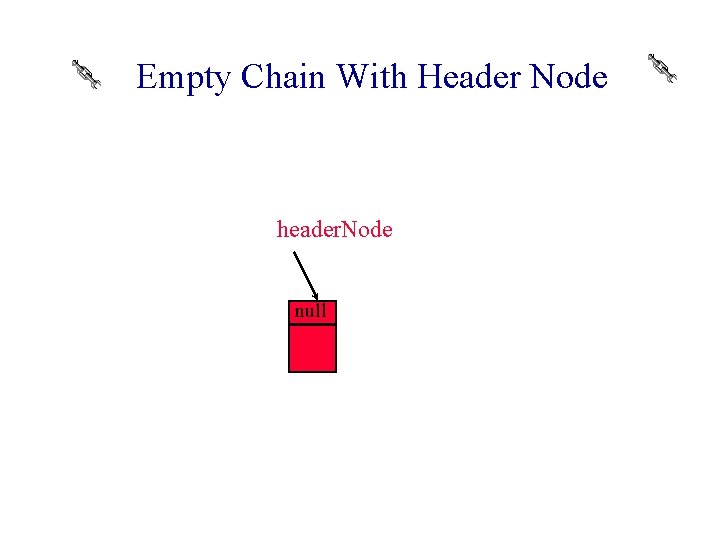
Empty Chain With Header Node header. Node null
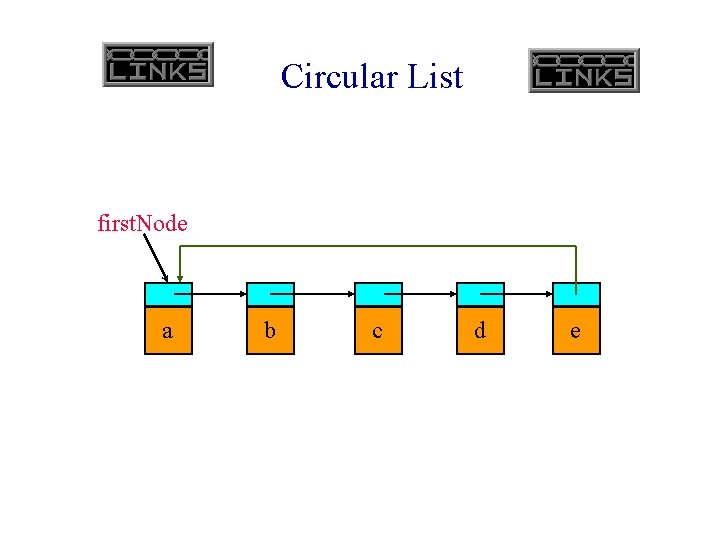
Circular List first. Node a b c d e
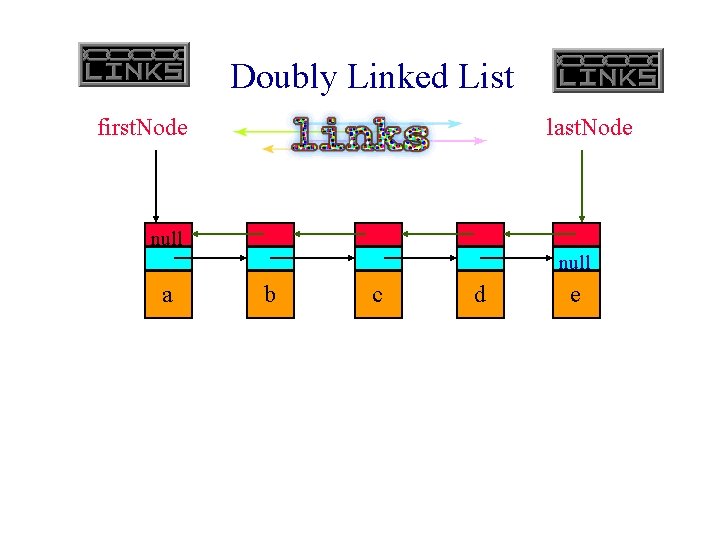
Doubly Linked List first. Node last. Node null a b c d e
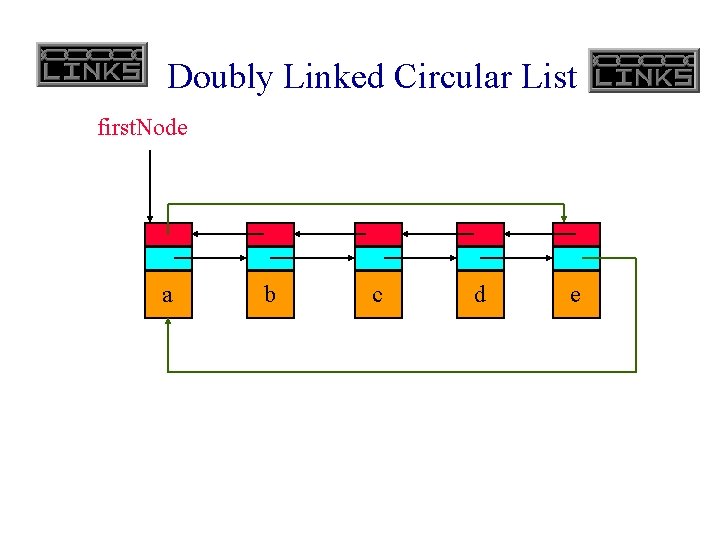
Doubly Linked Circular List first. Node a b c d e
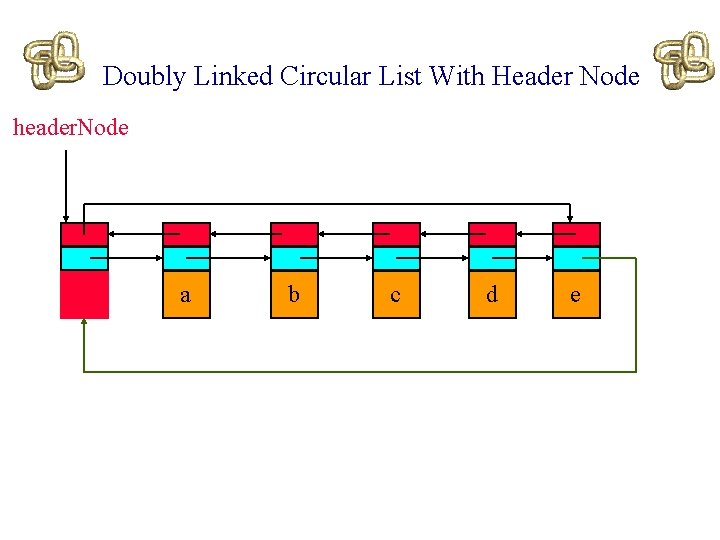
Doubly Linked Circular List With Header Node header. Node a b c d e
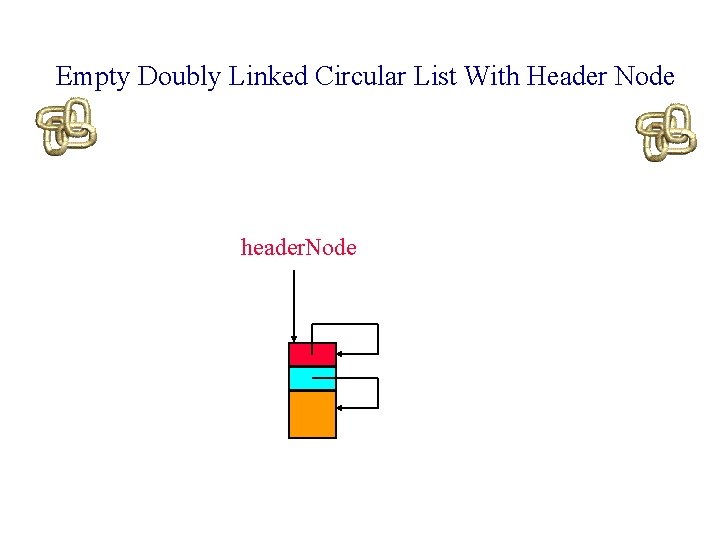
Empty Doubly Linked Circular List With Header Node header. Node
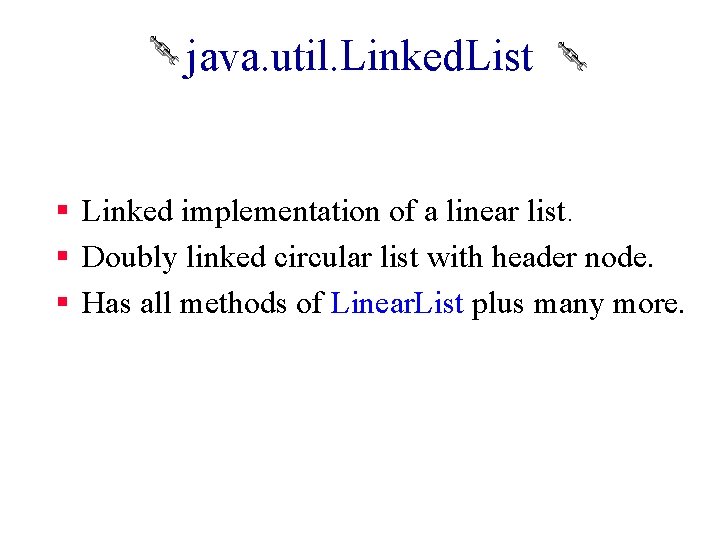
java. util. Linked. List § Linked implementation of a linear list. § Doubly linked circular list with header node. § Has all methods of Linear. List plus many more.
Reference node and non reference node
Reference node and non reference node
Constructive interference example
Nodenext
Parallel spin
Struct node int data struct node* next
Linked list remove first node
Grazing food chain diagram
Putting a package together
Package mypackage class first class body
Hát kết hợp bộ gõ cơ thể
Slidetodoc
Bổ thể
Tỉ lệ cơ thể trẻ em
Voi kéo gỗ như thế nào
Tư thế worm breton là gì
Alleluia hat len nguoi oi
Các môn thể thao bắt đầu bằng từ đua
Thế nào là hệ số cao nhất
Các châu lục và đại dương trên thế giới
Công thức tính độ biến thiên đông lượng
Trời xanh đây là của chúng ta thể thơ
Cách giải mật thư tọa độ
Phép trừ bù
độ dài liên kết
Các châu lục và đại dương trên thế giới
Thể thơ truyền thống
Quá trình desamine hóa có thể tạo ra
Một số thể thơ truyền thống
Cái miệng bé xinh thế chỉ nói điều hay thôi
Vẽ hình chiếu vuông góc của vật thể sau