1 Chapter 23 Data Structures Outline 23 1
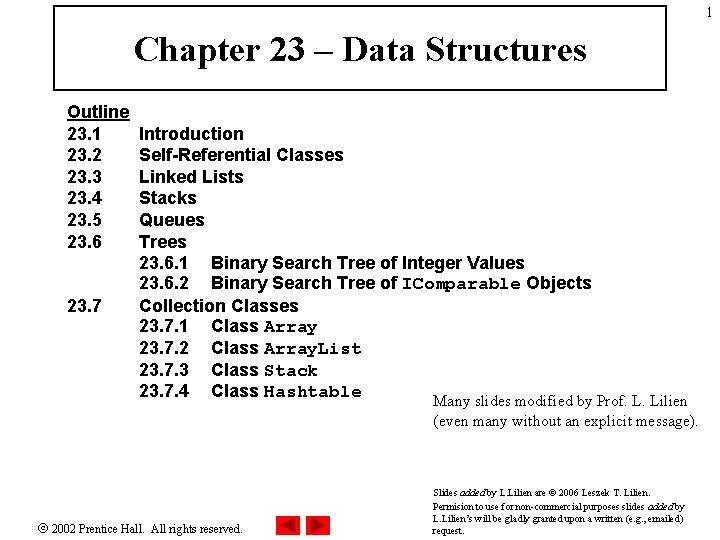
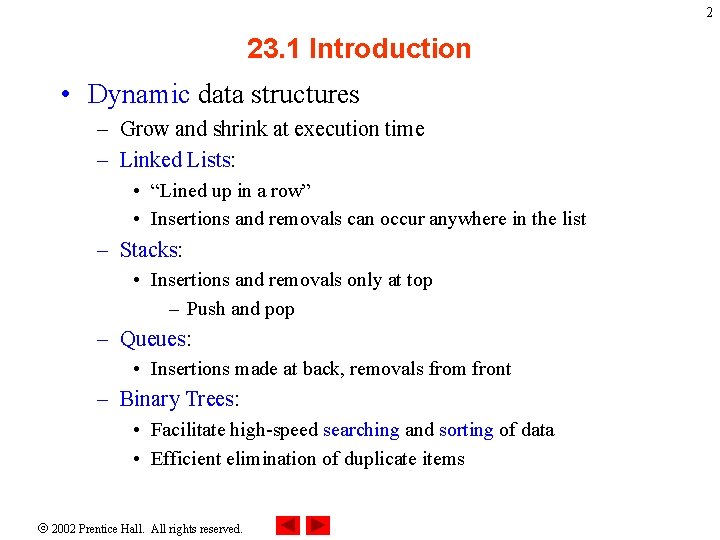
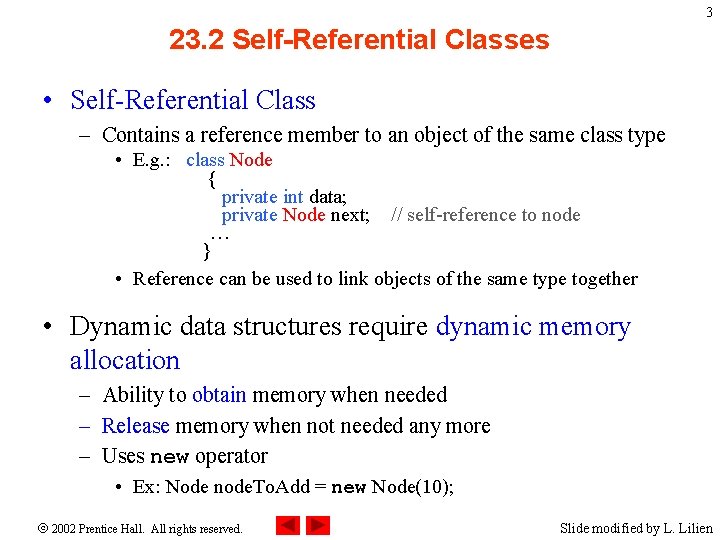
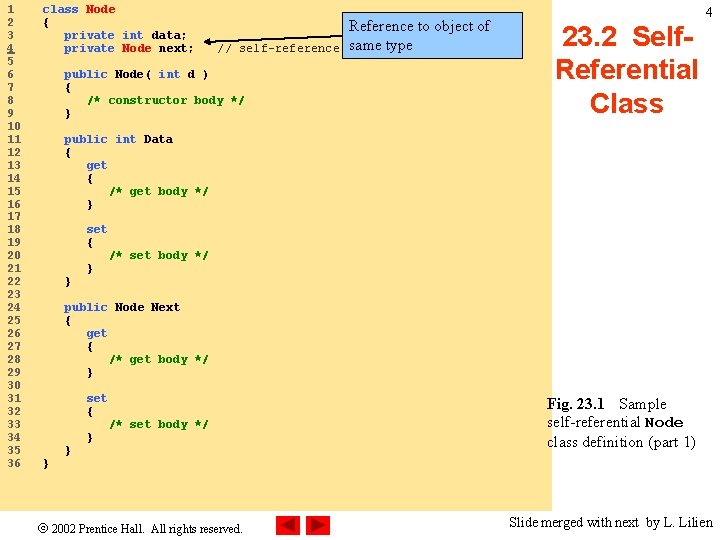
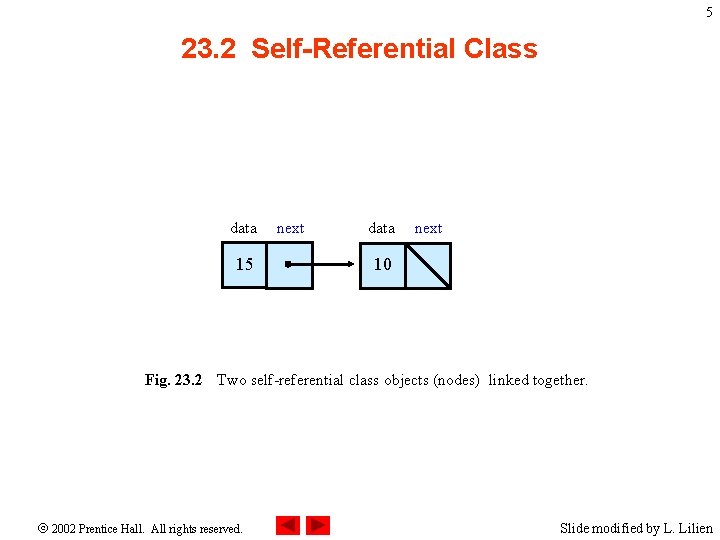
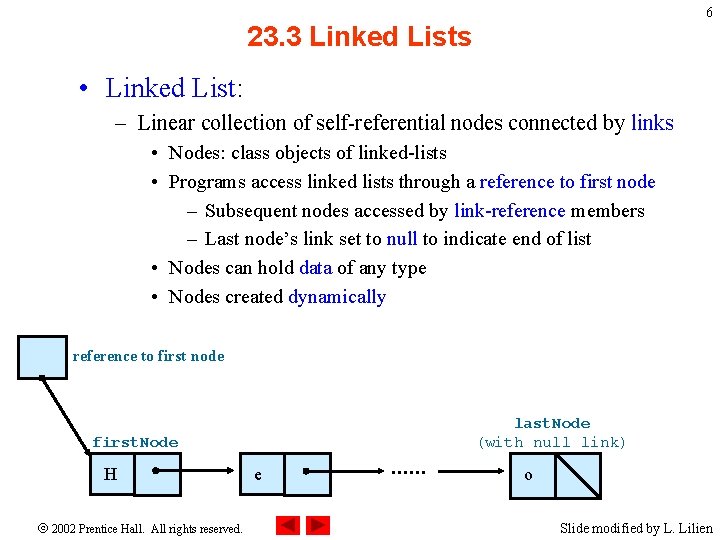
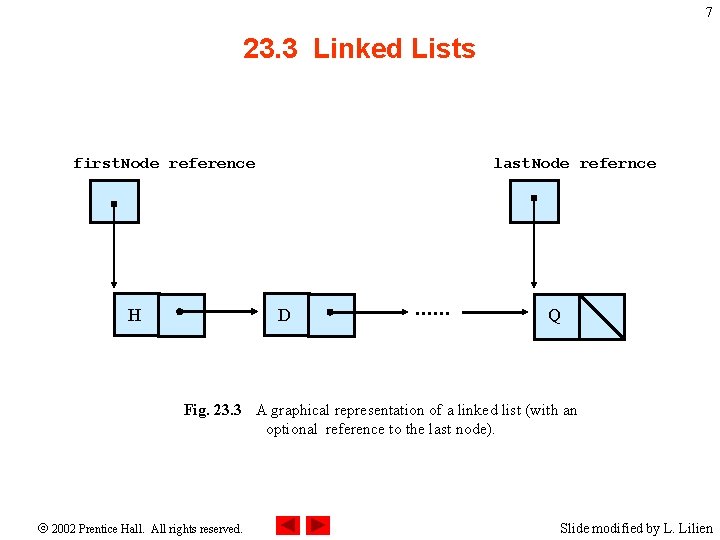
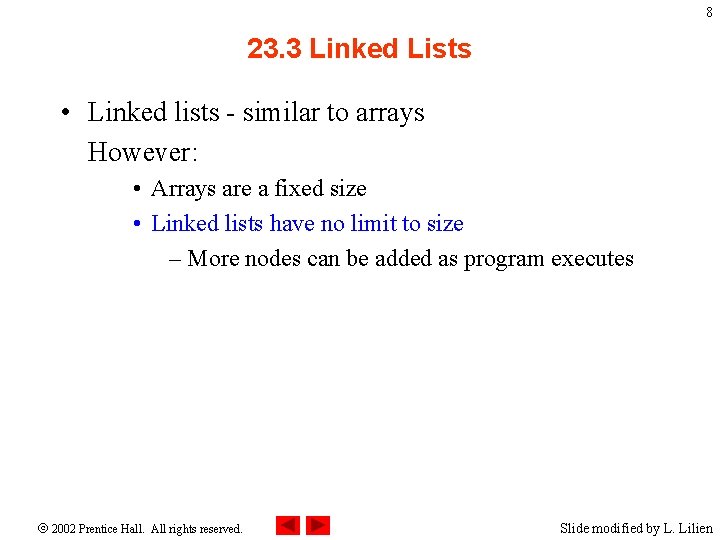
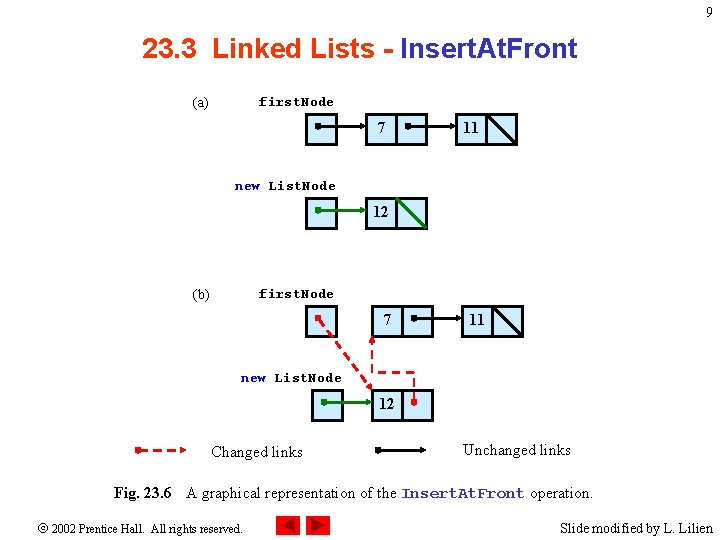
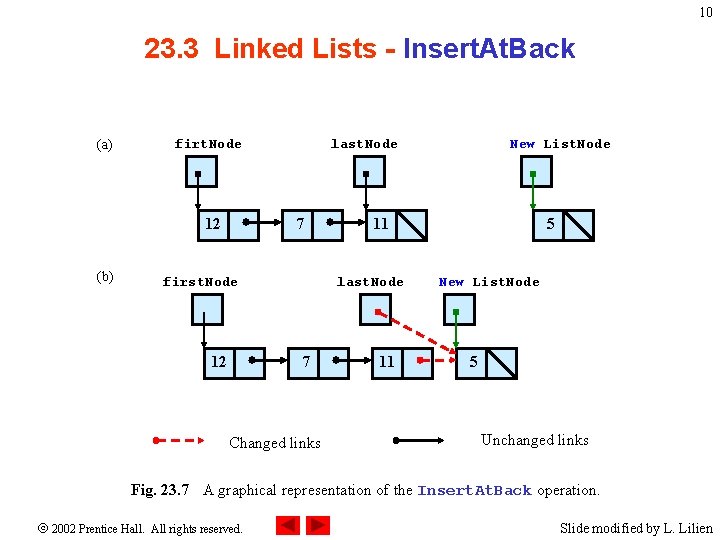
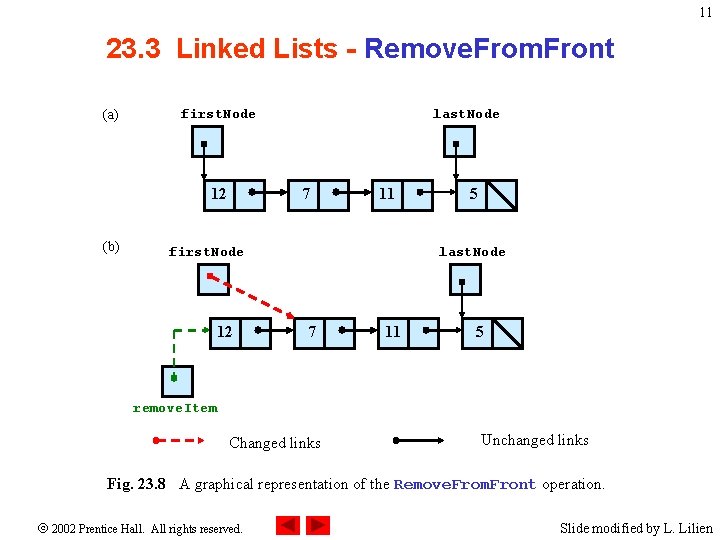
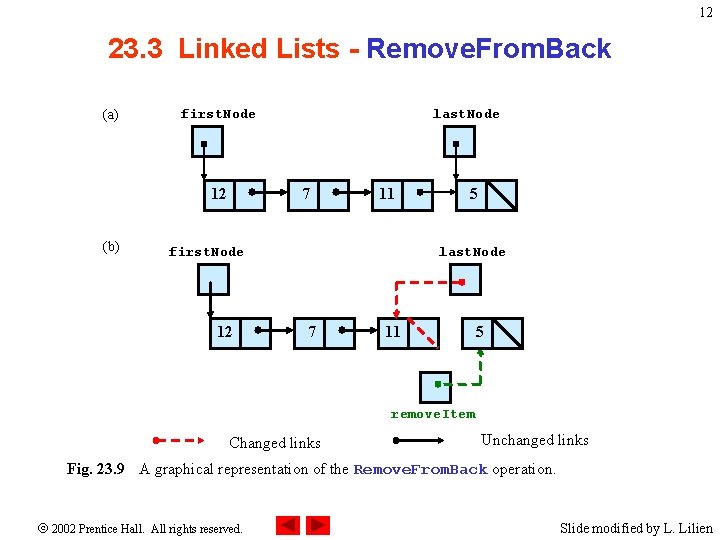
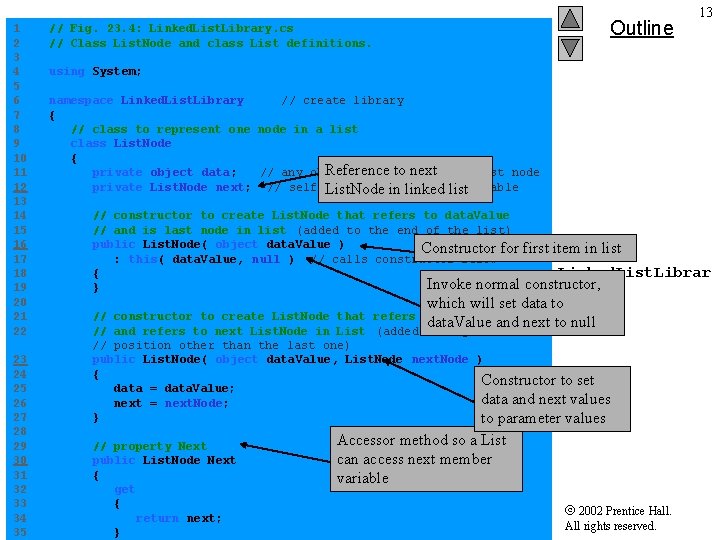
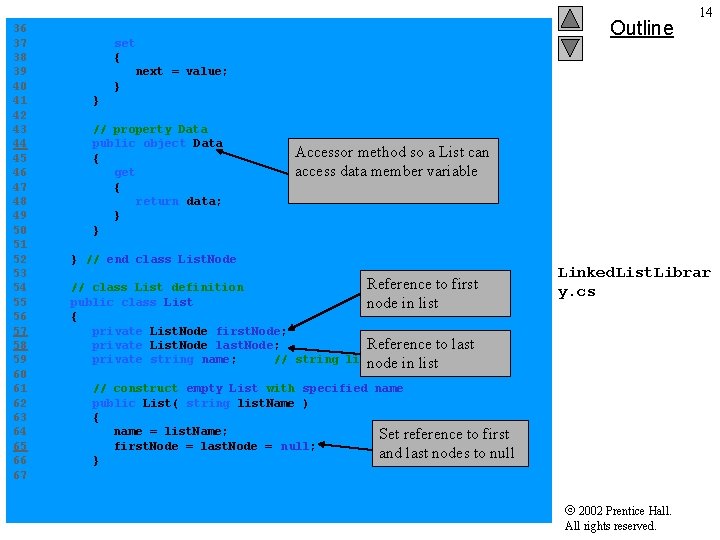
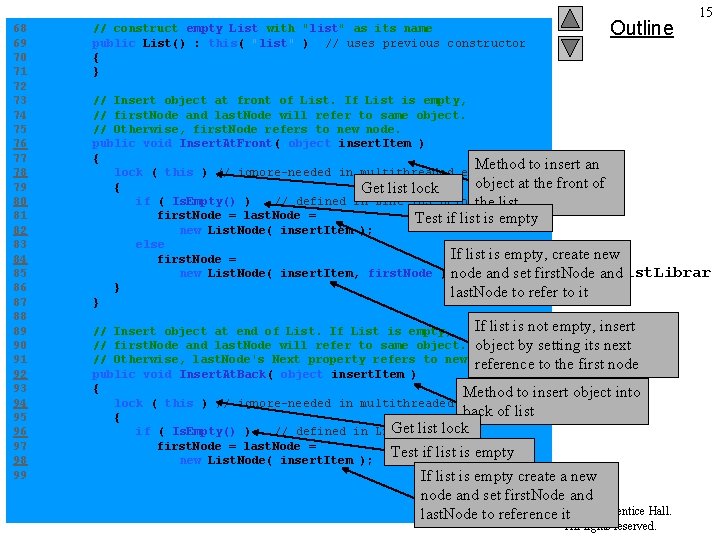
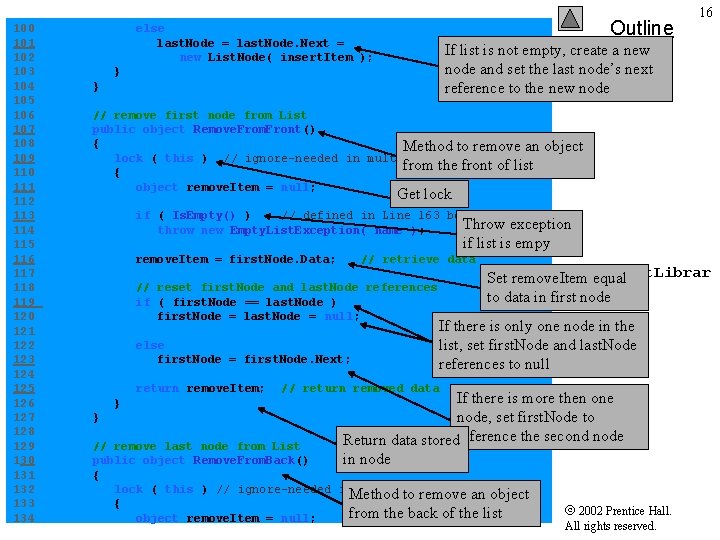
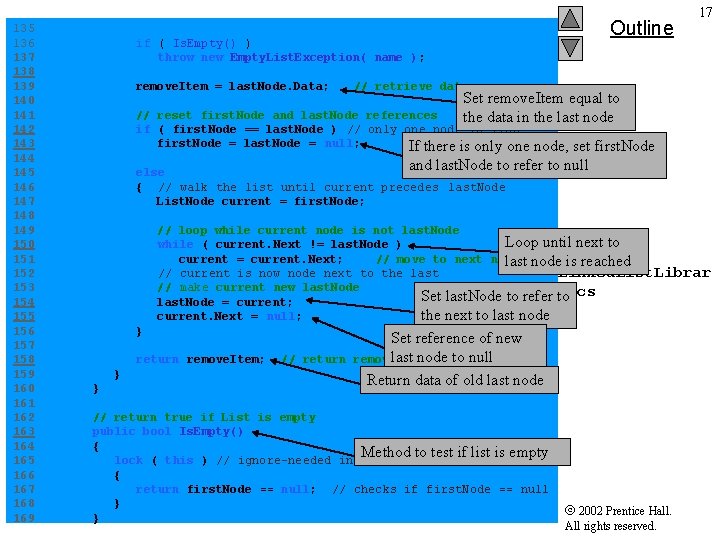
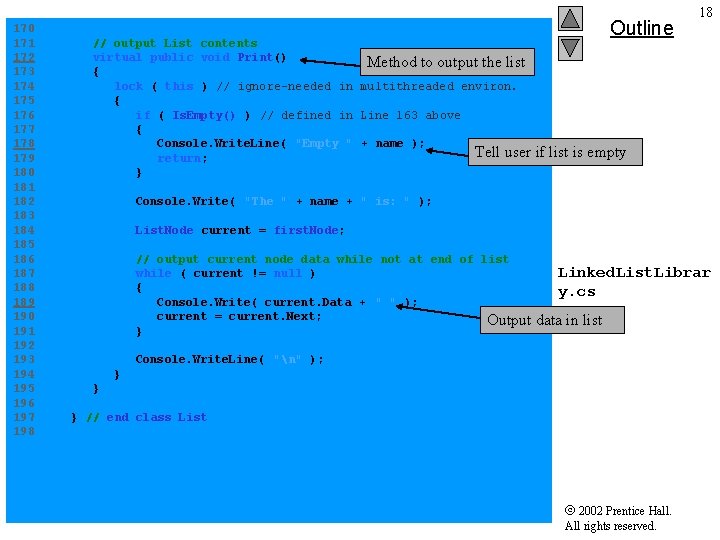
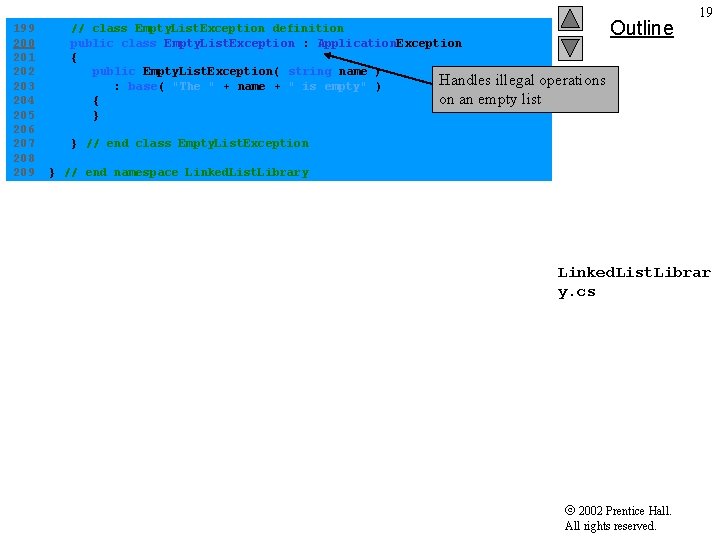
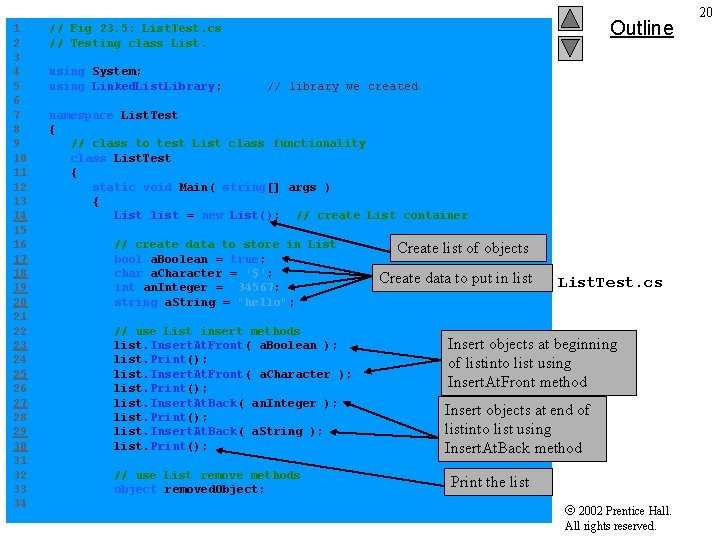
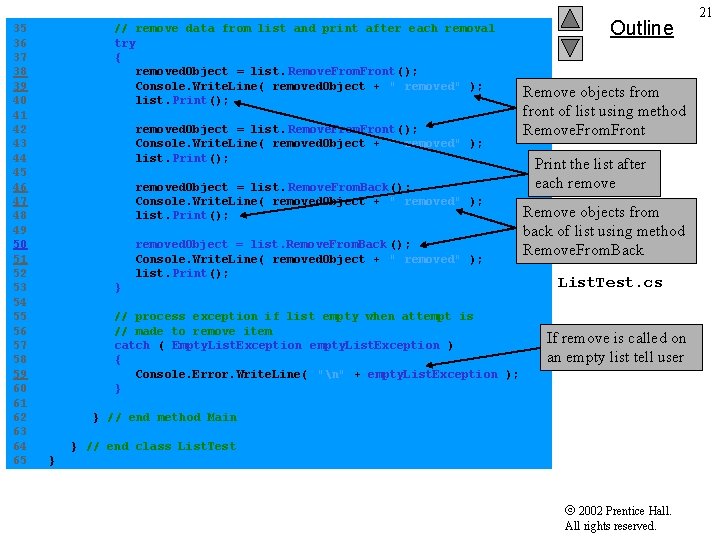
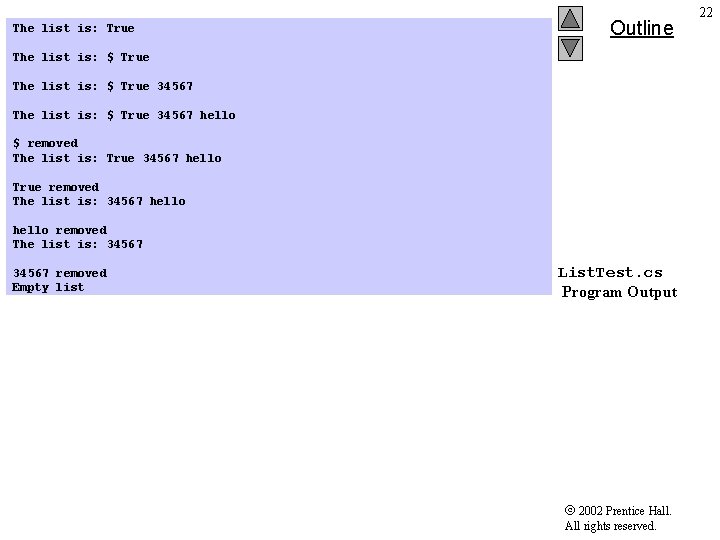
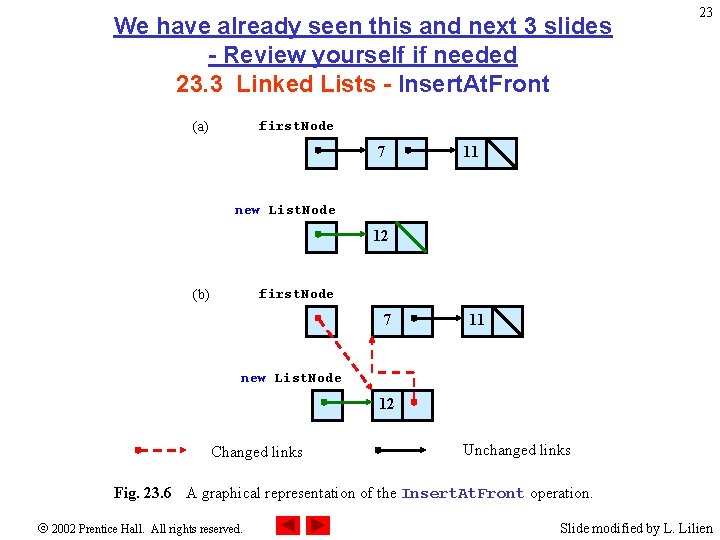
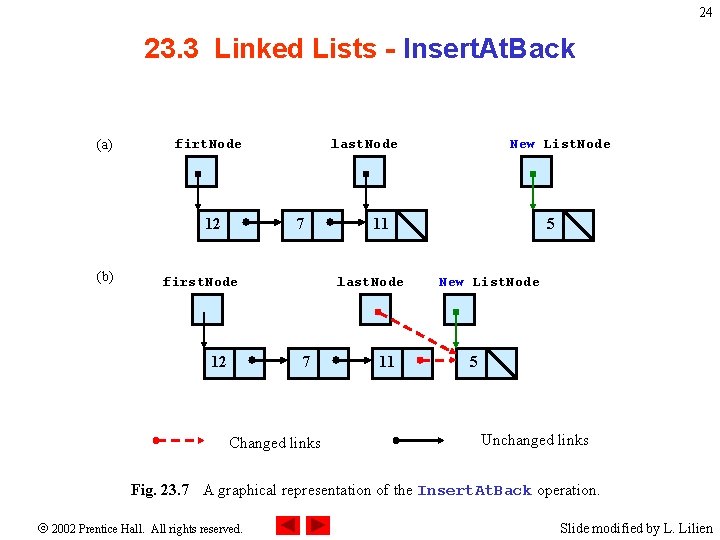
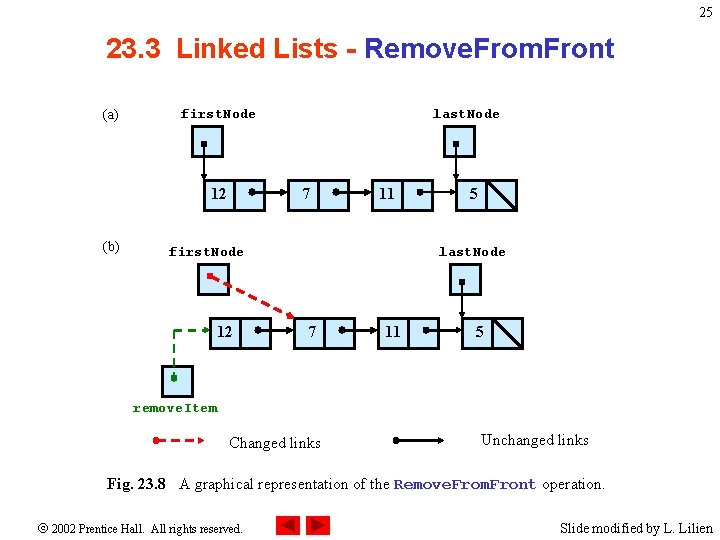
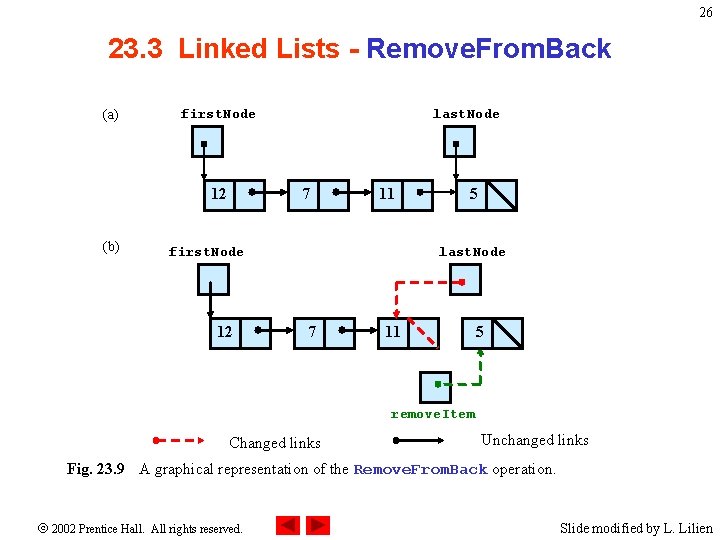
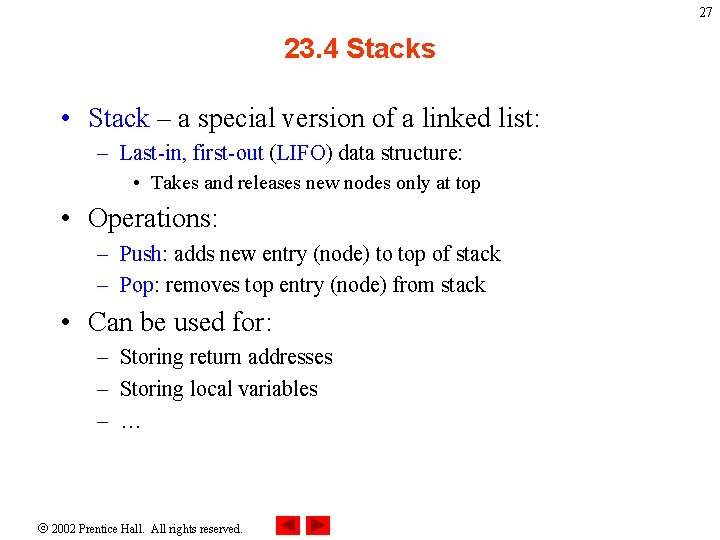
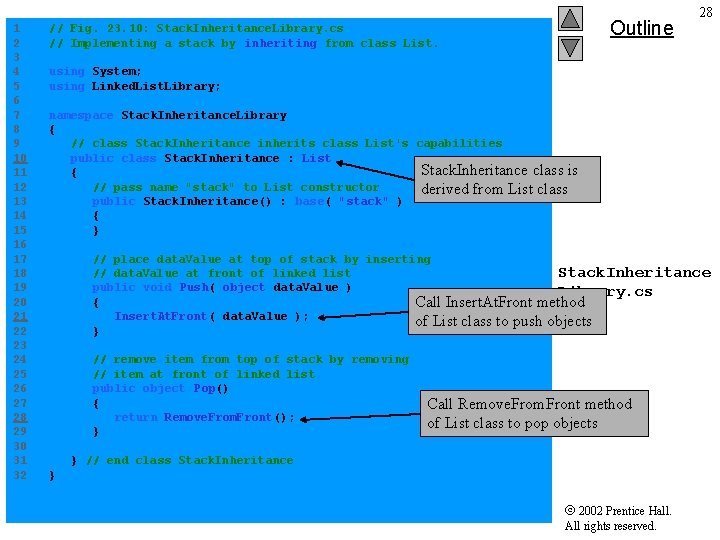
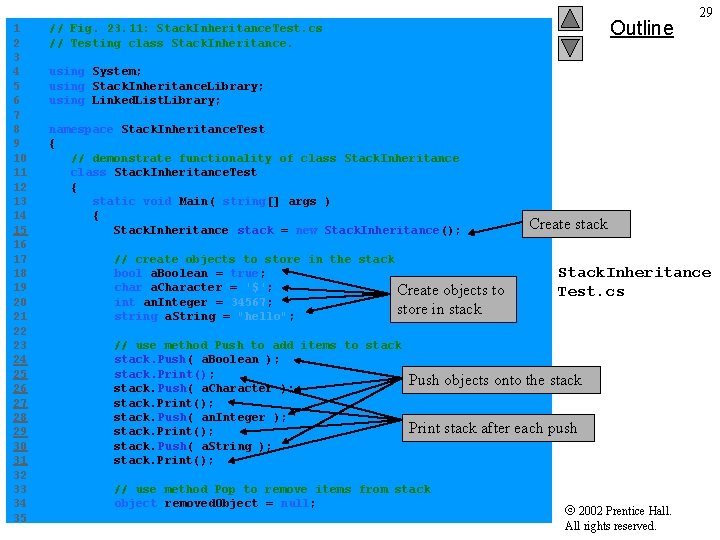
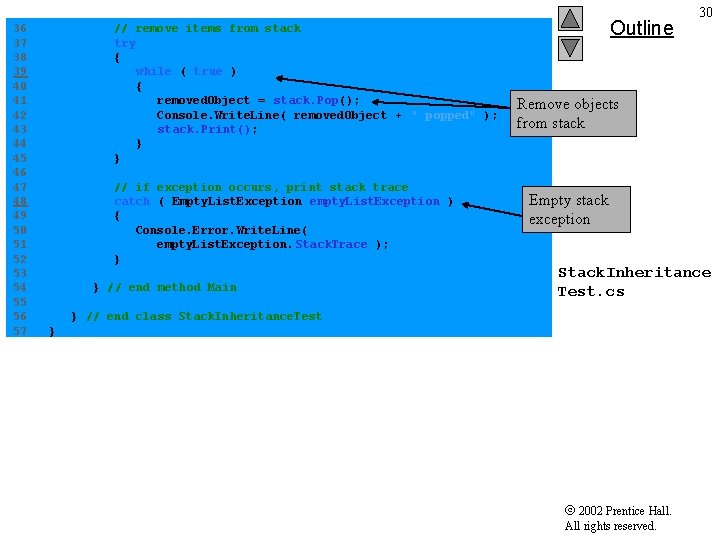
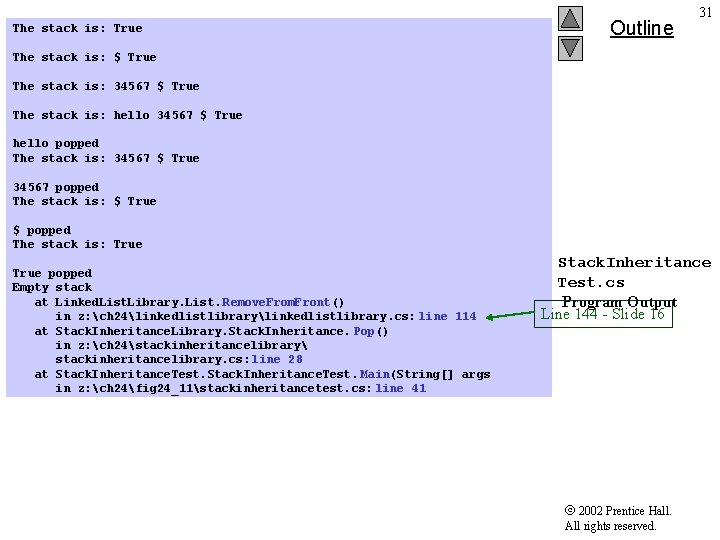
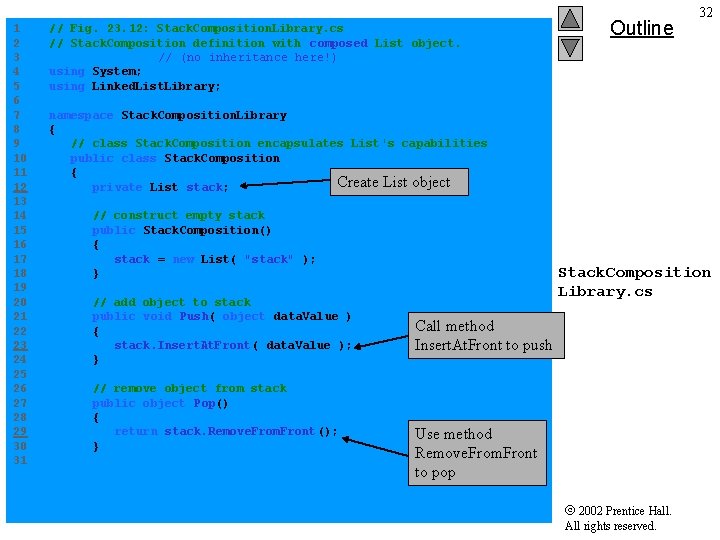
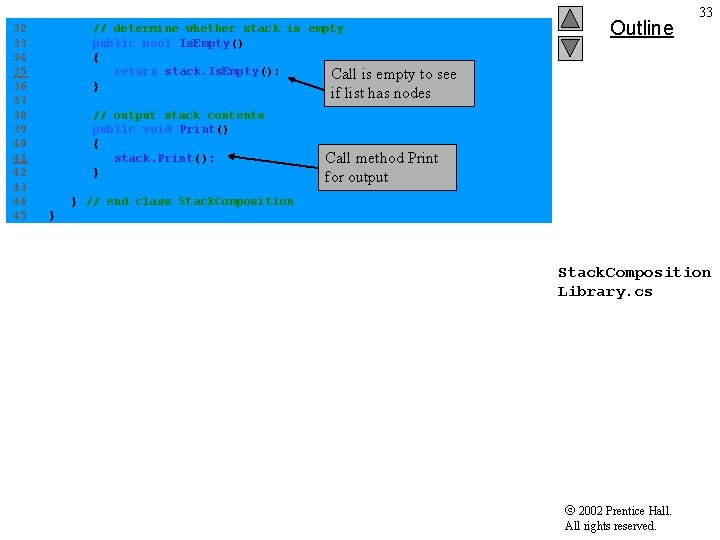
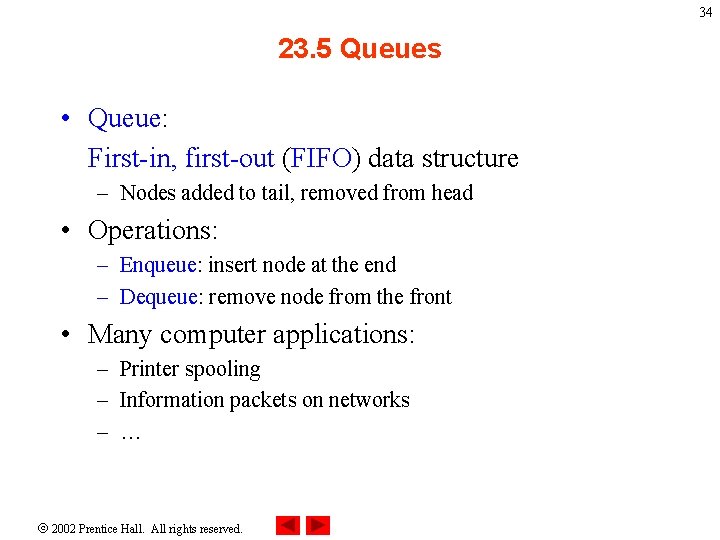
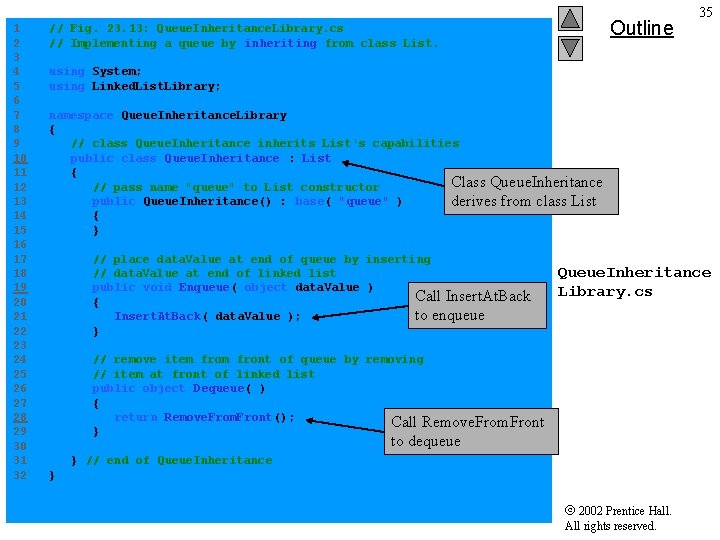
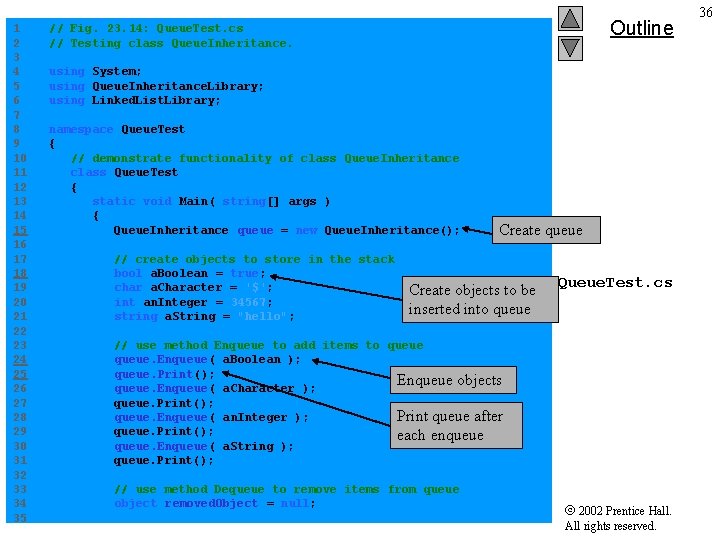
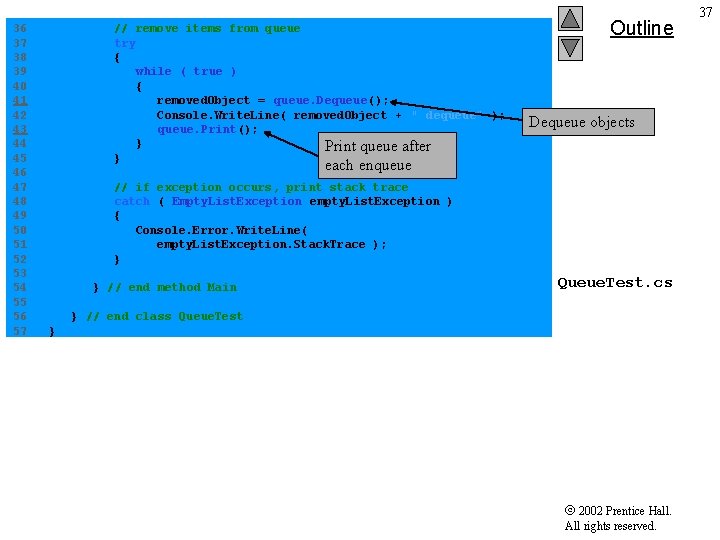
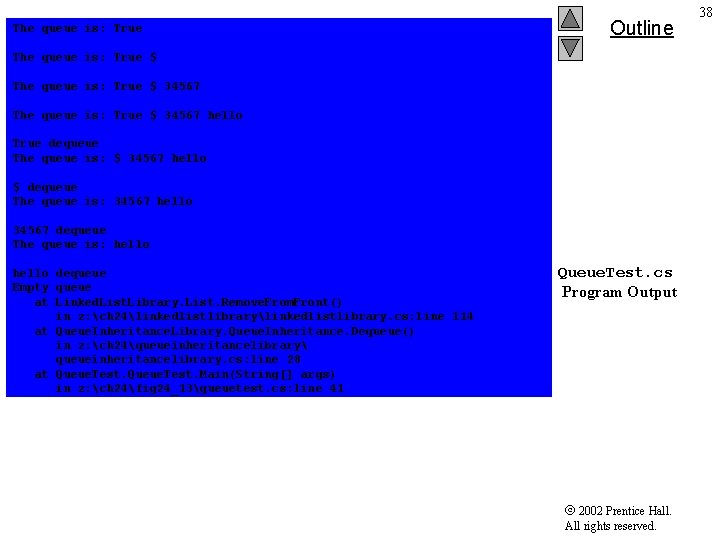
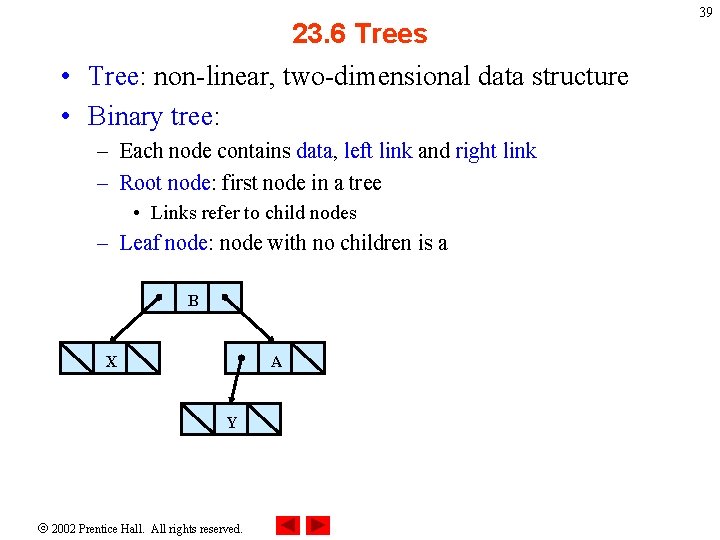
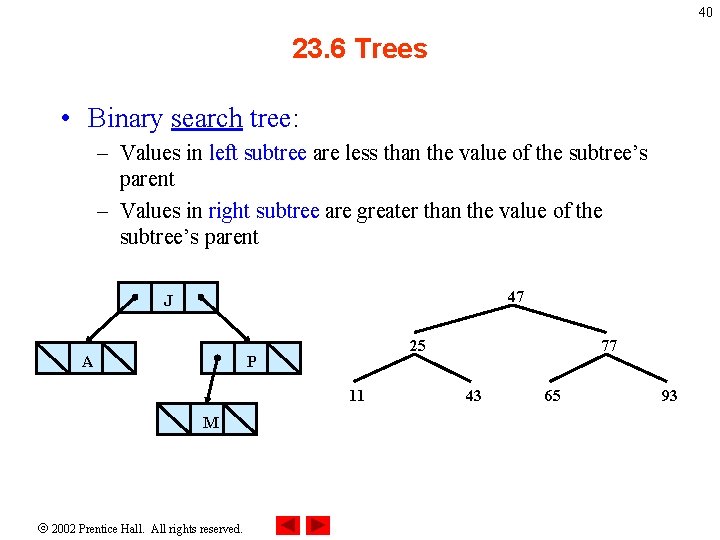
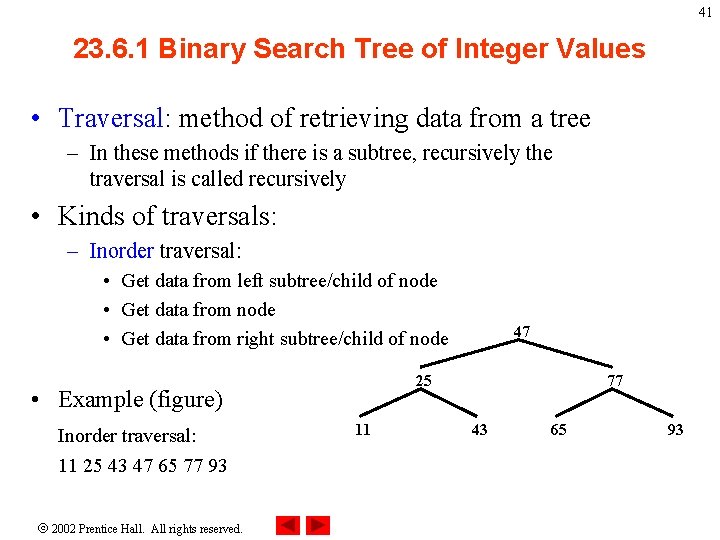
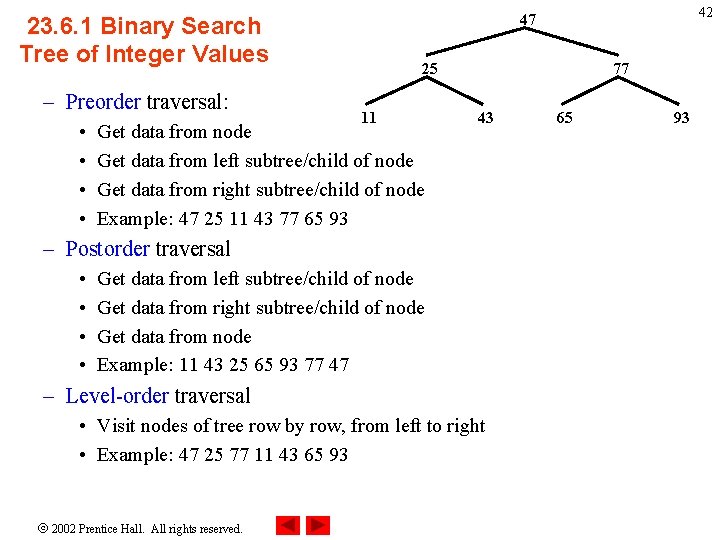
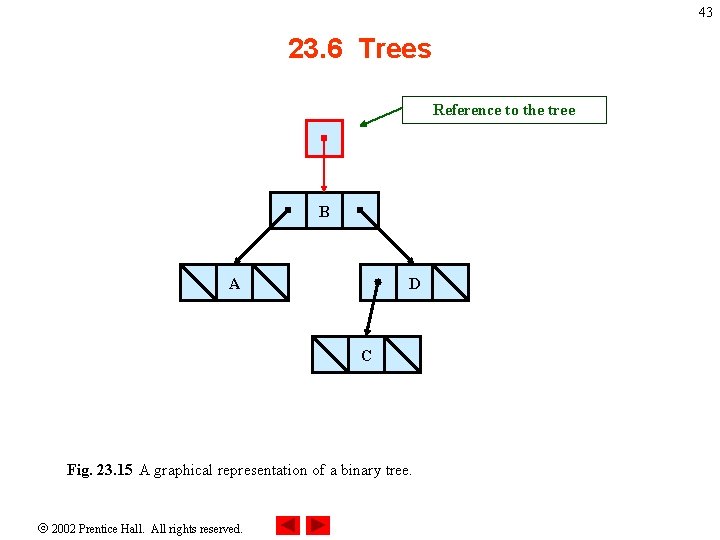
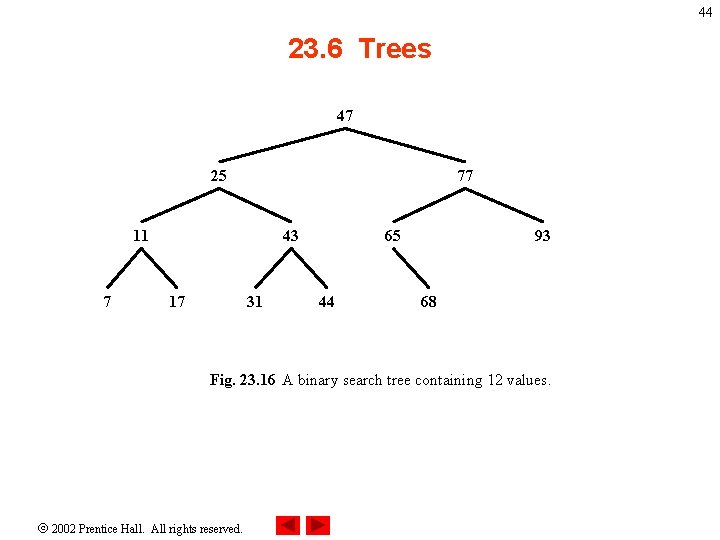
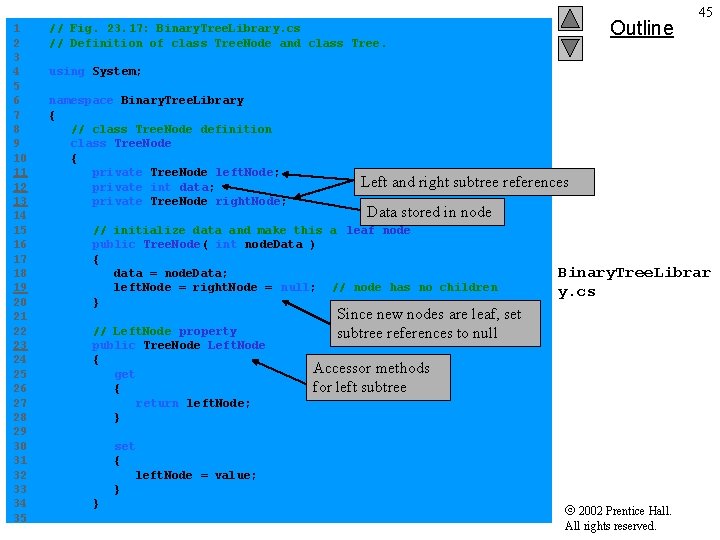
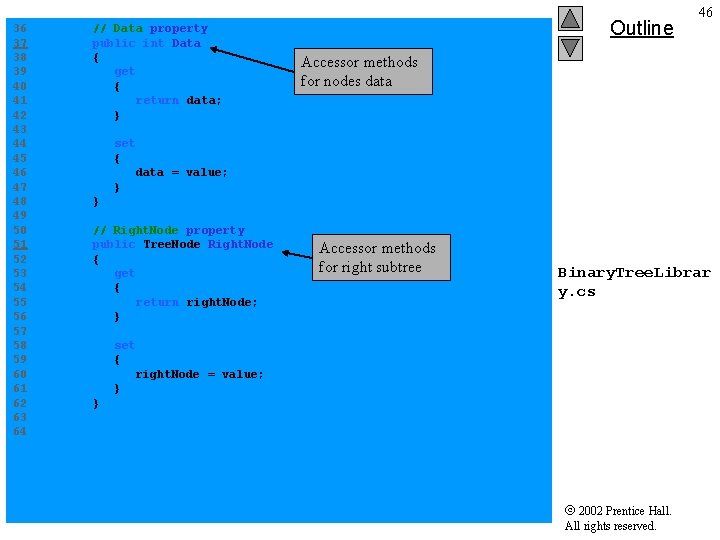
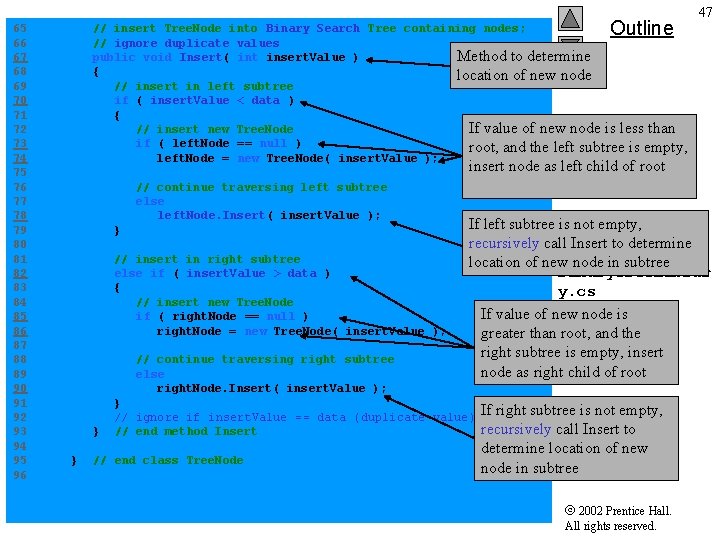
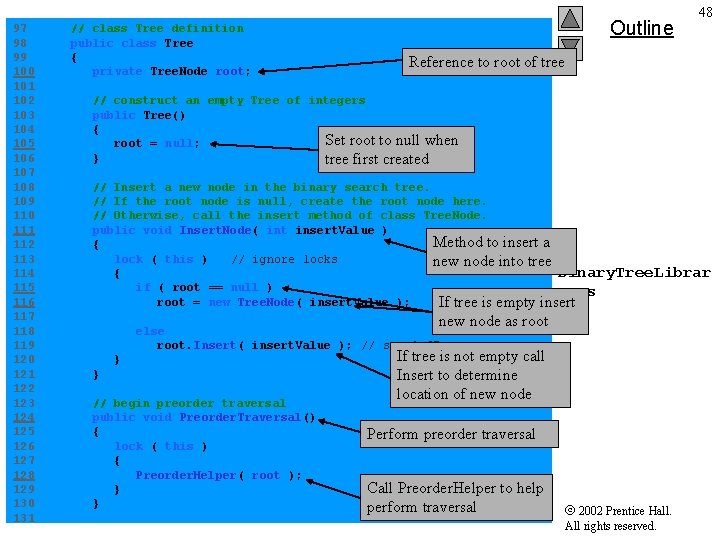
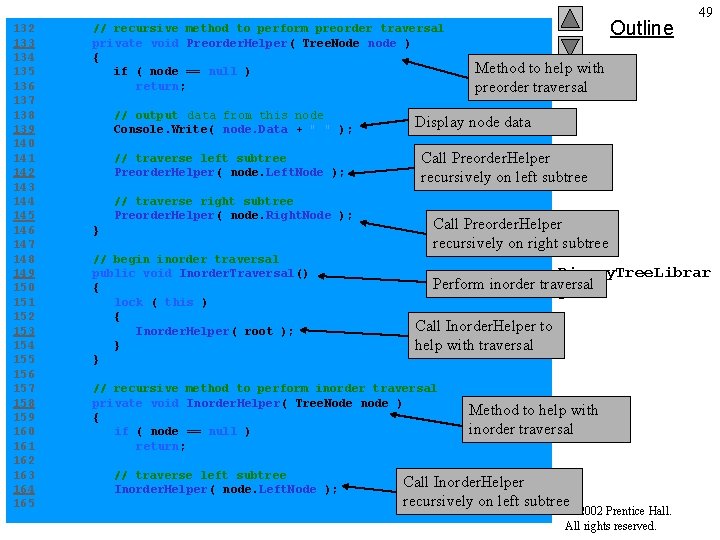
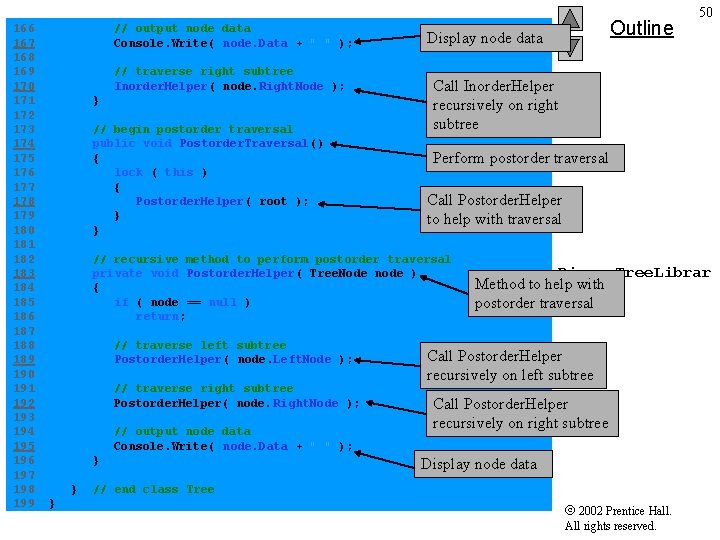
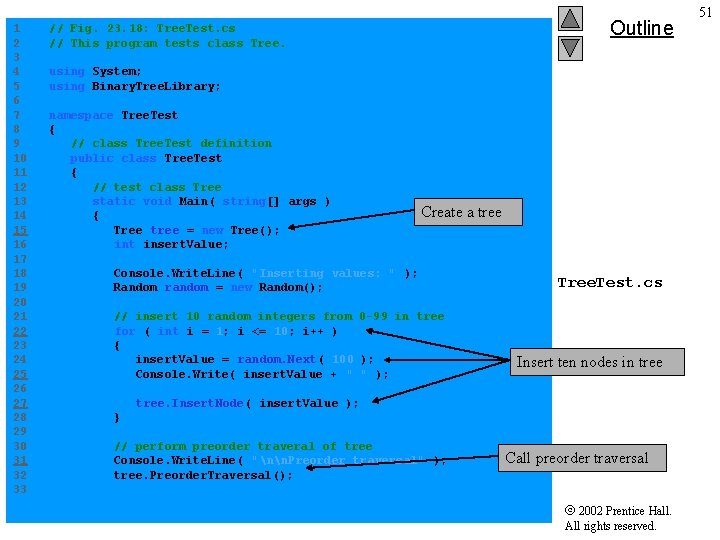
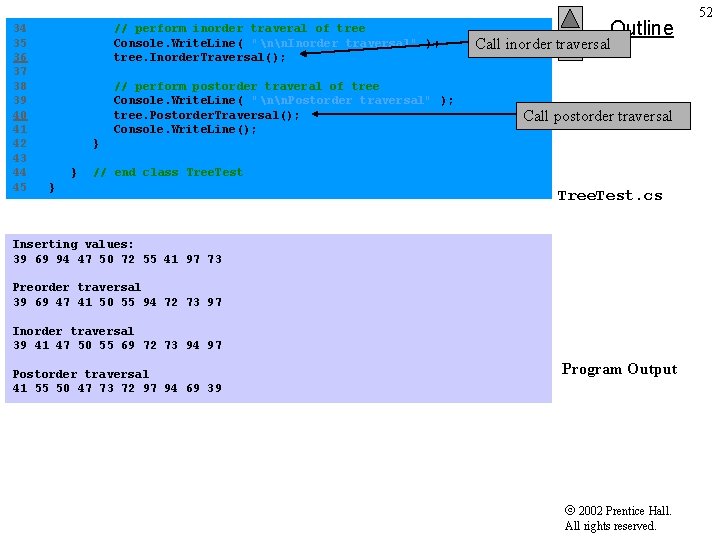
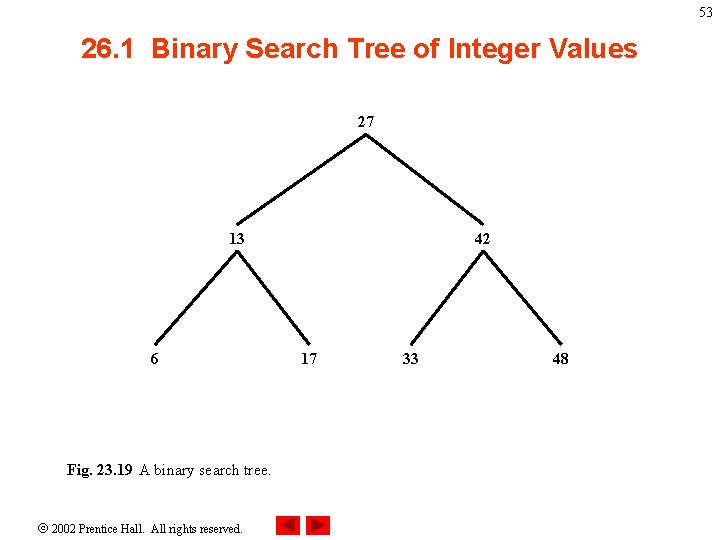
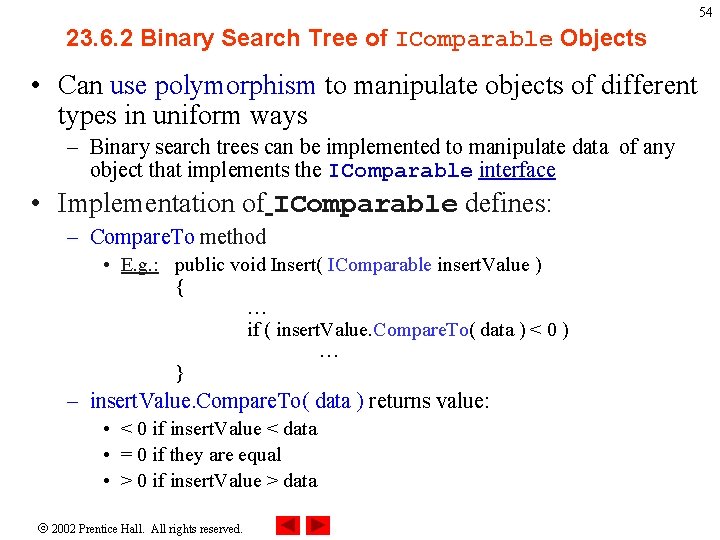
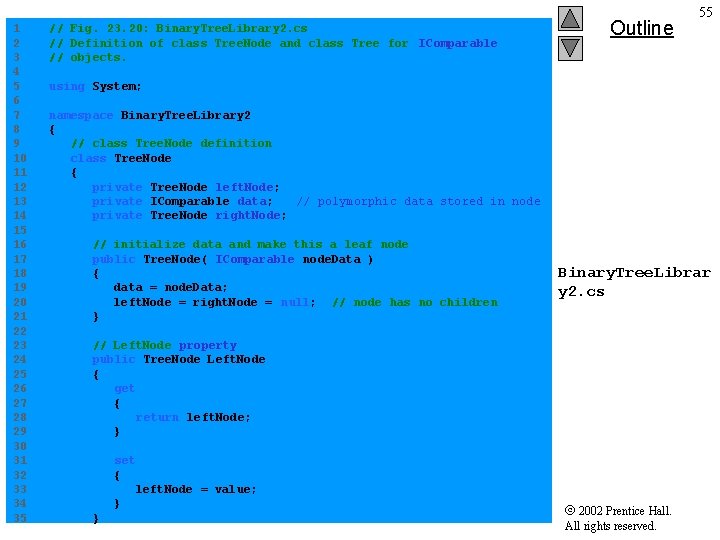
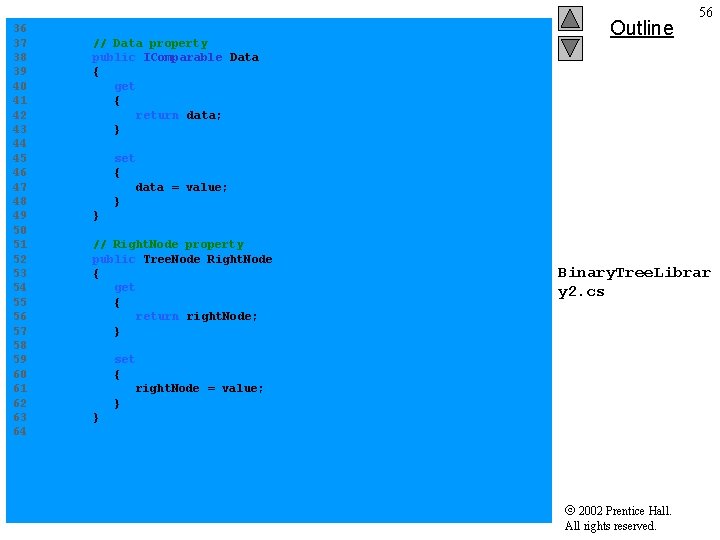
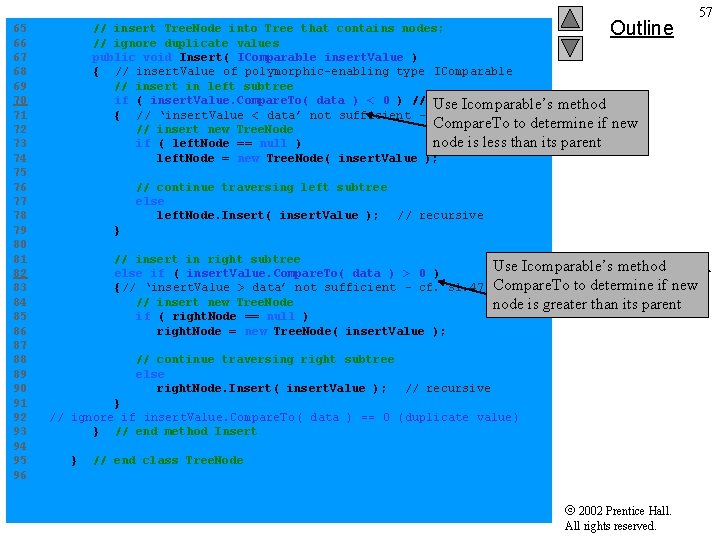
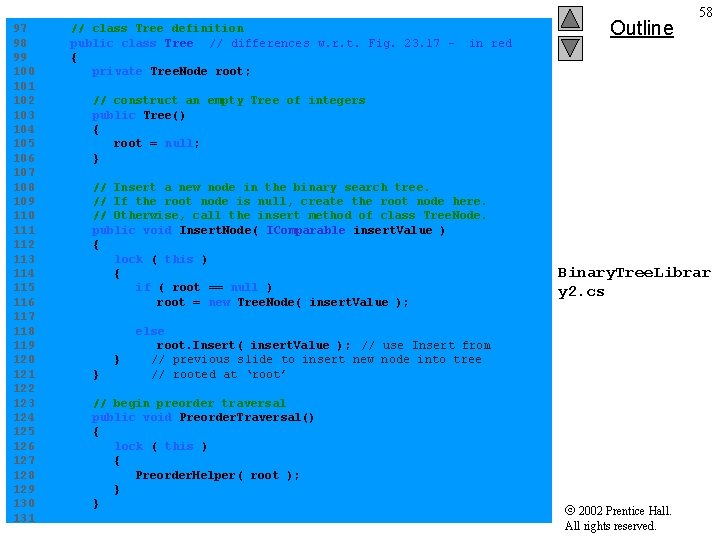
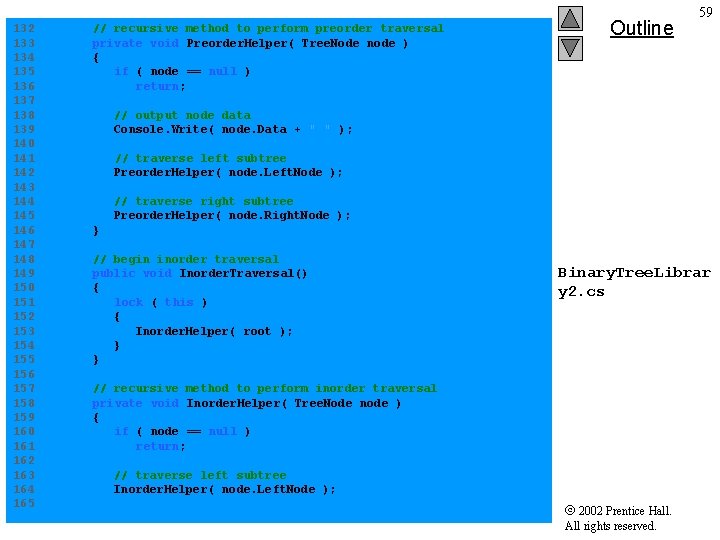
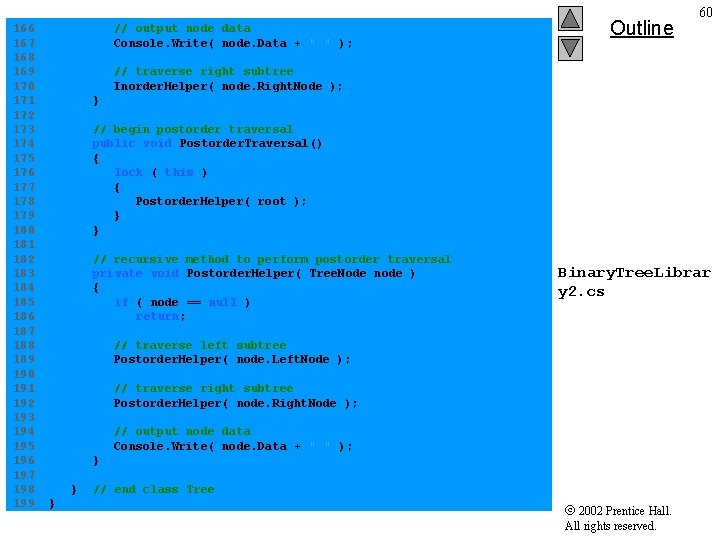
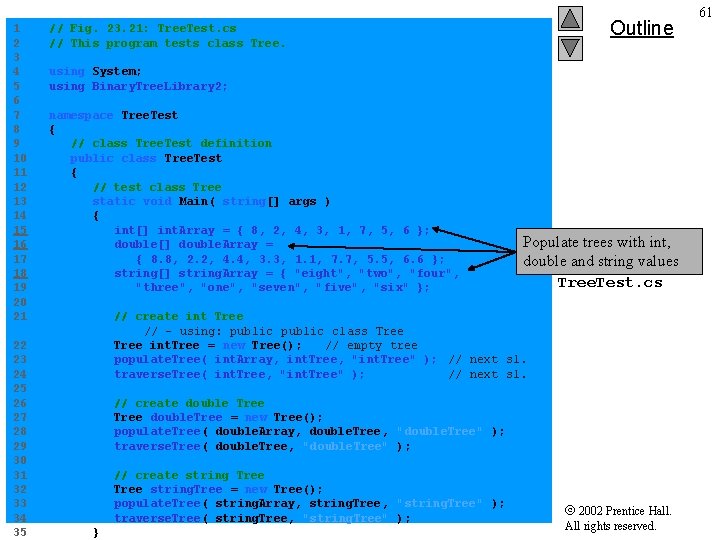
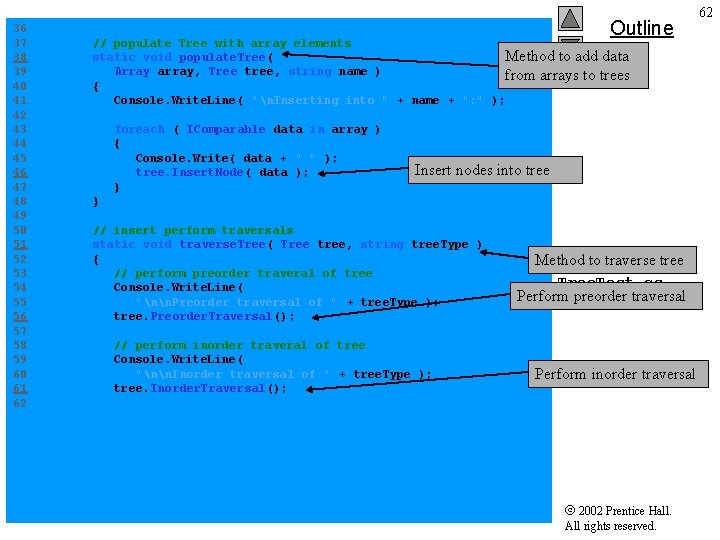
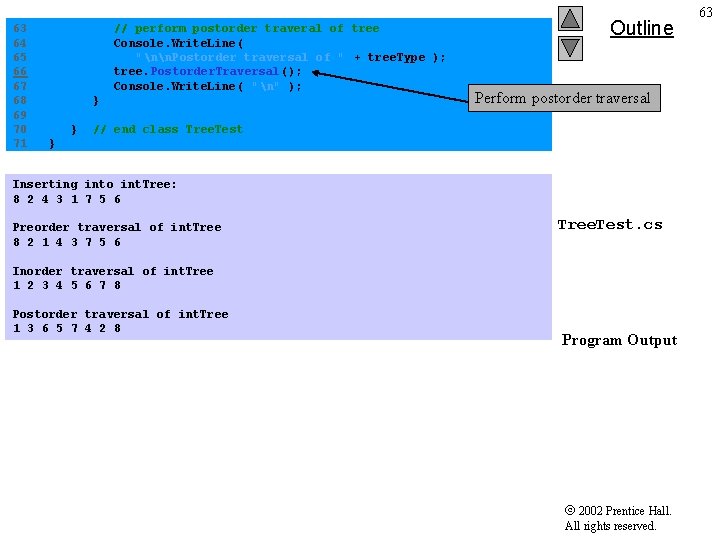
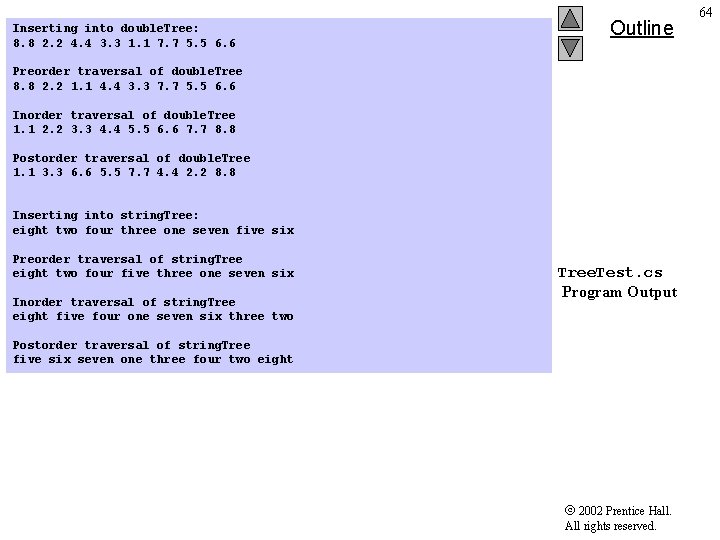
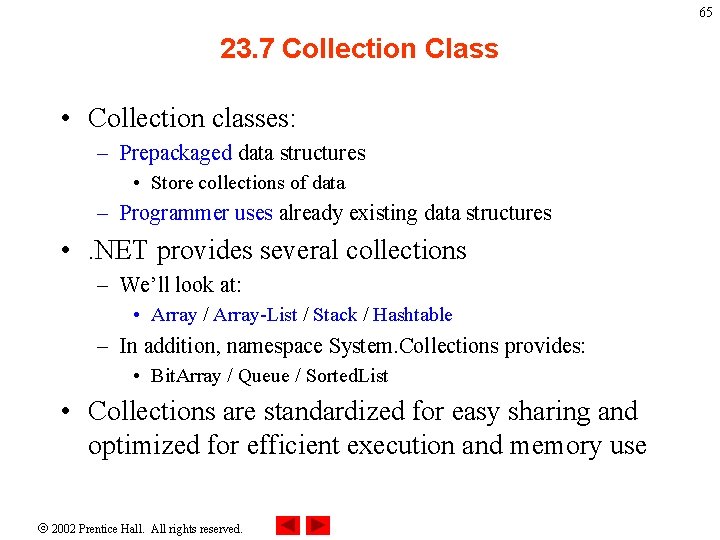
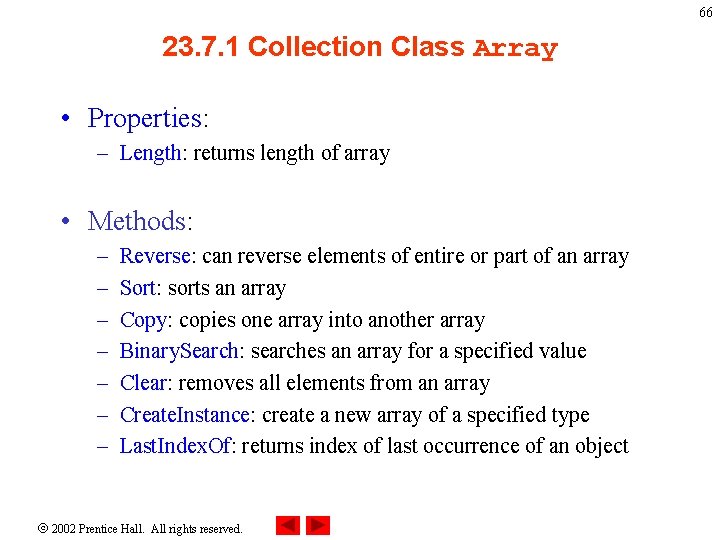
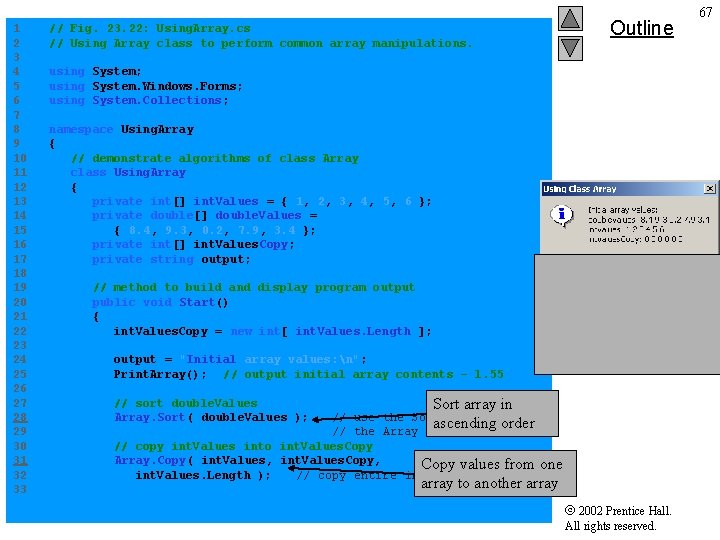
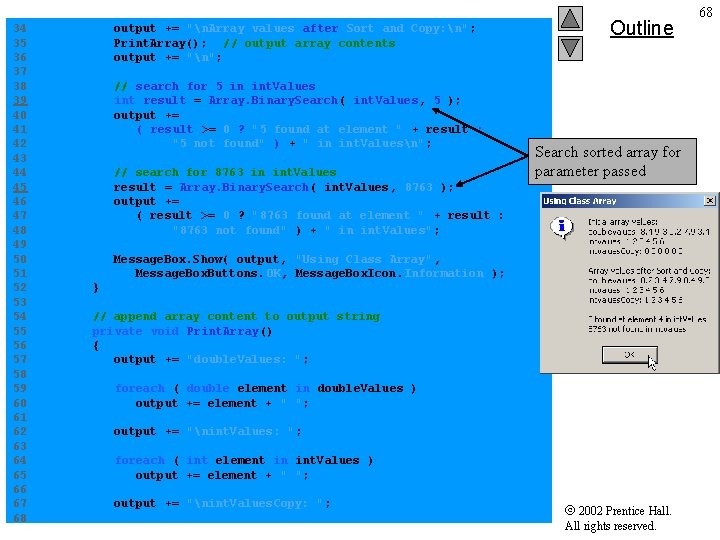
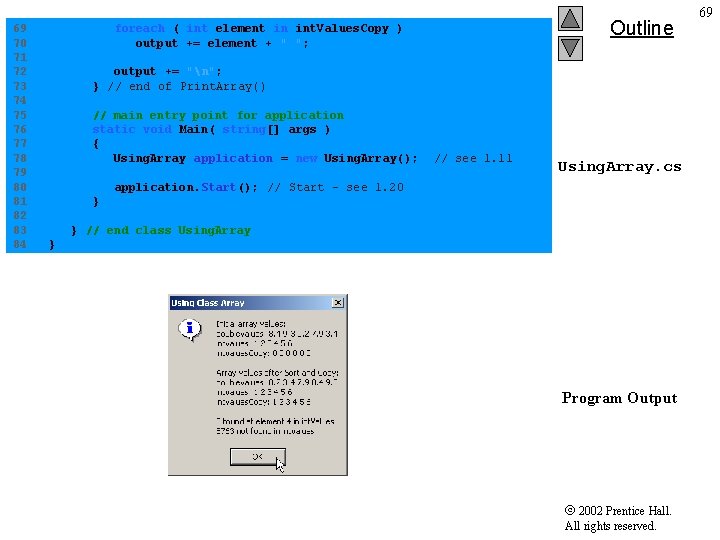
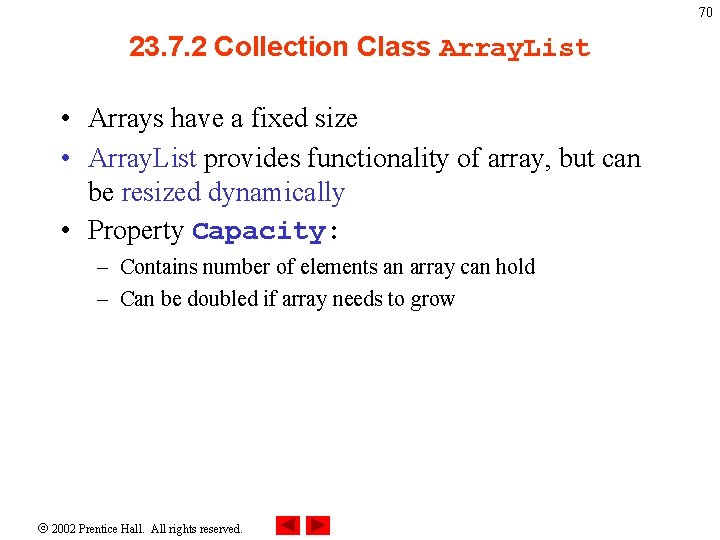
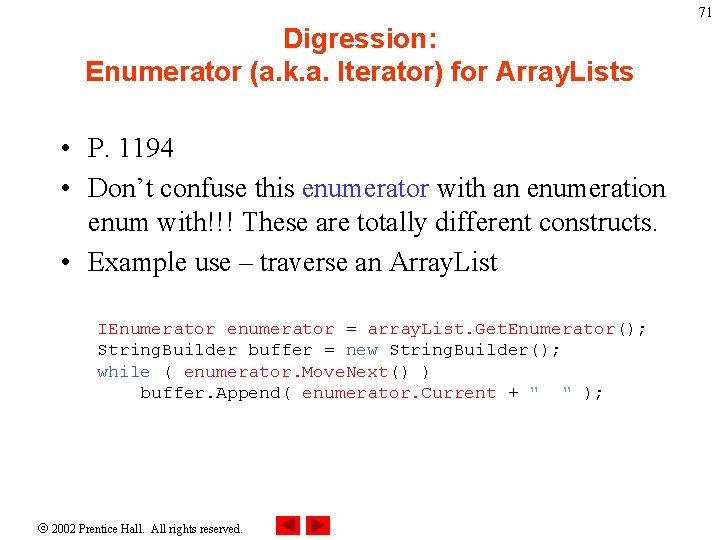
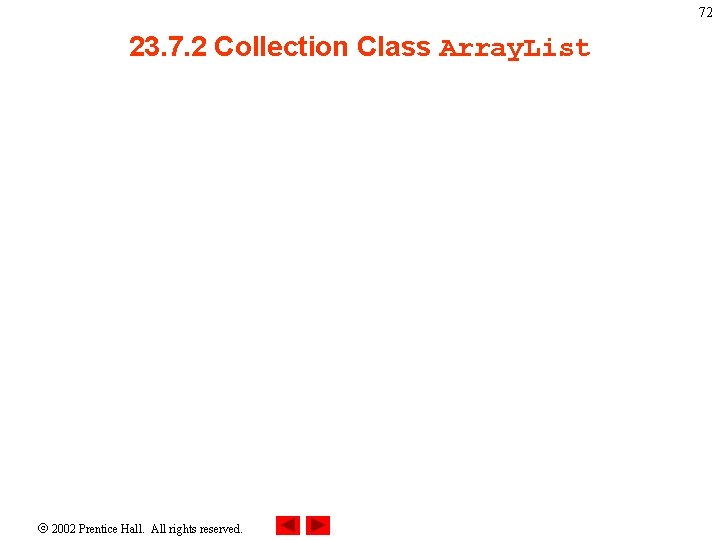
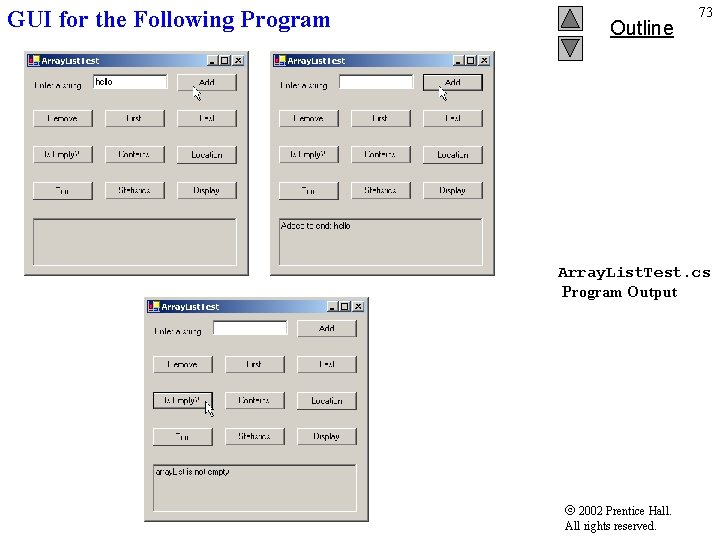
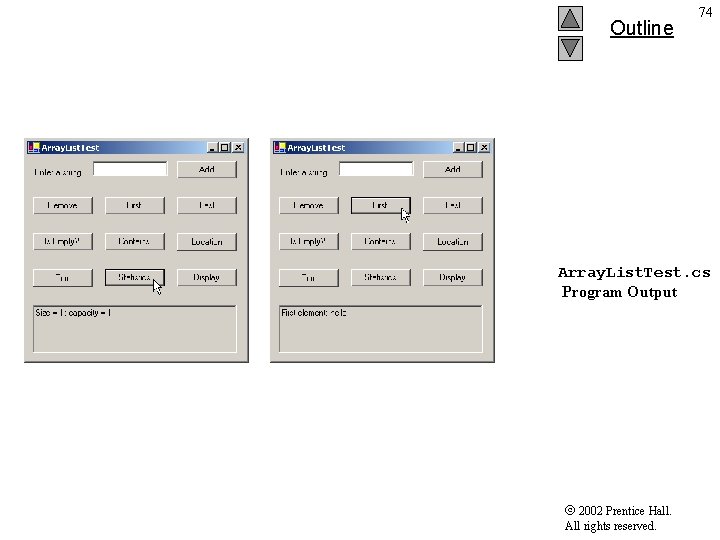
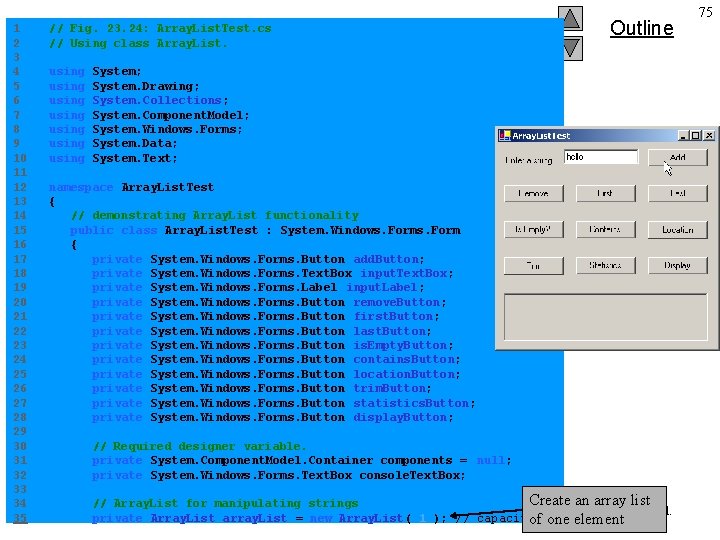
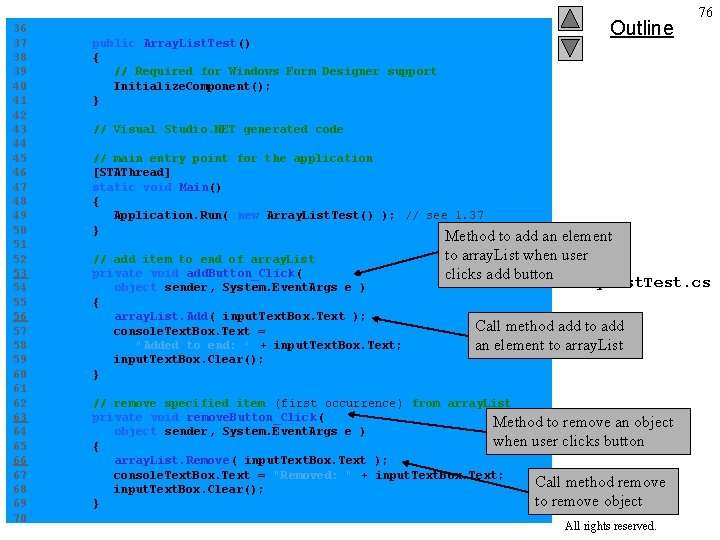
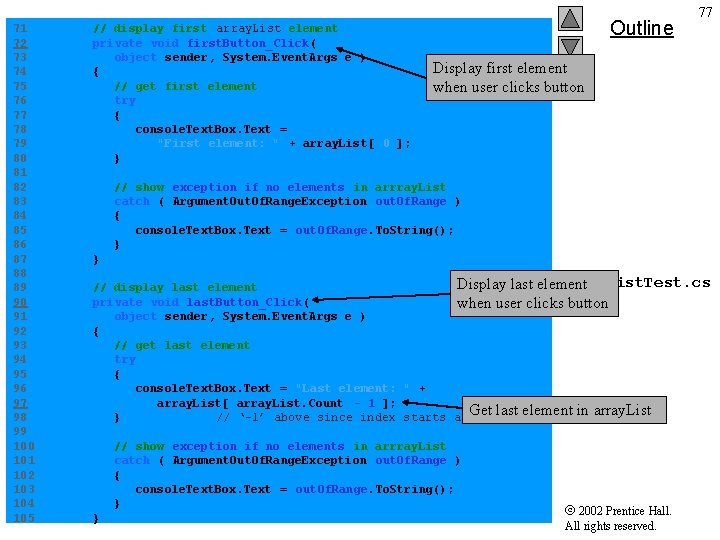
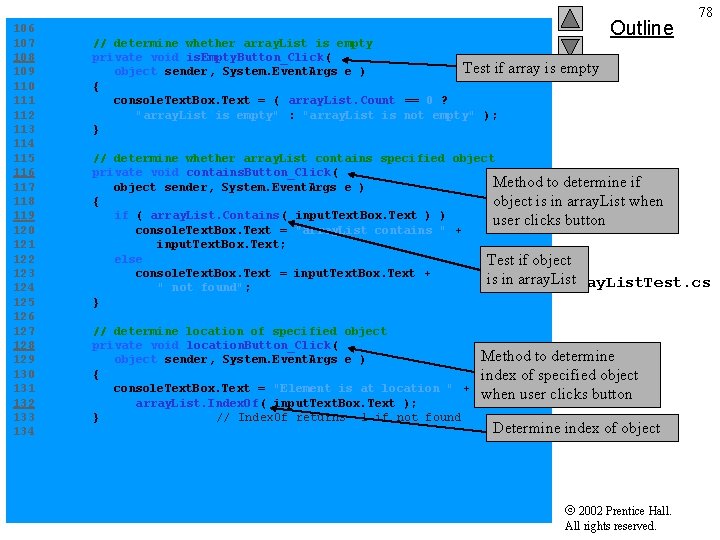
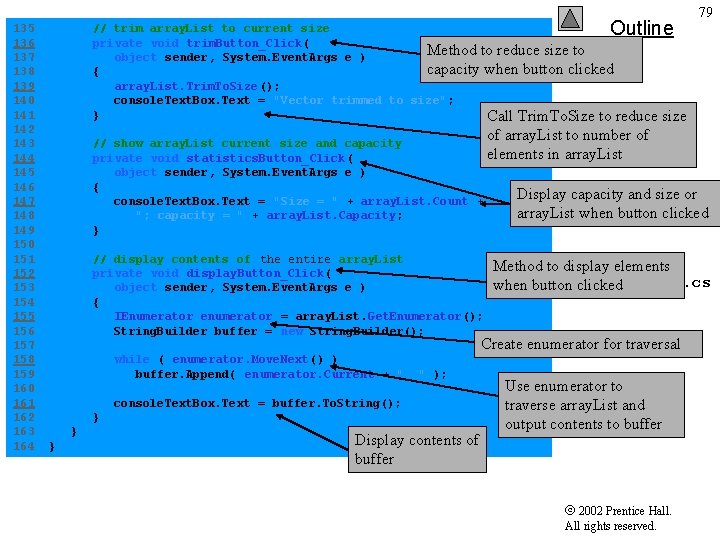
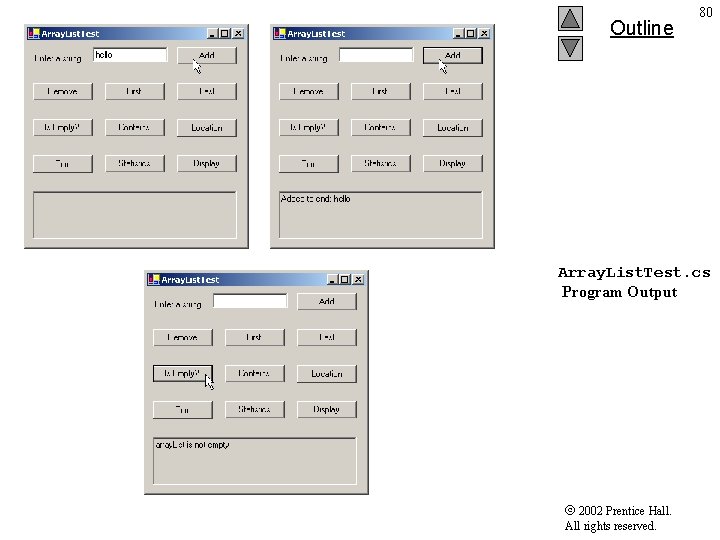
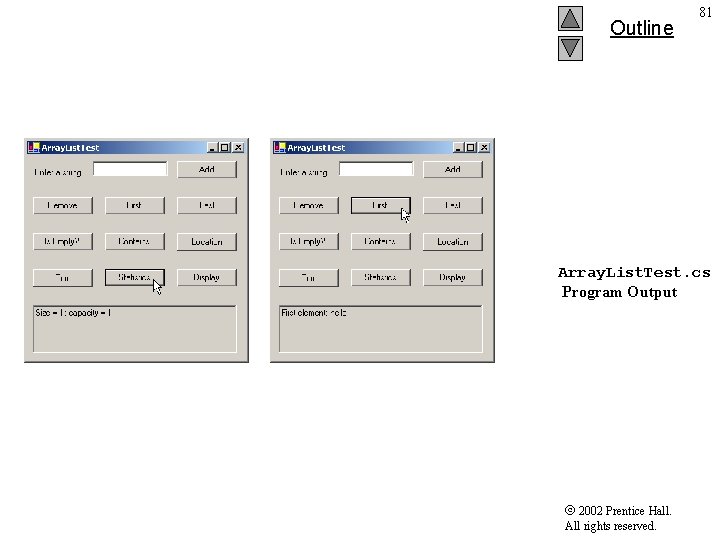
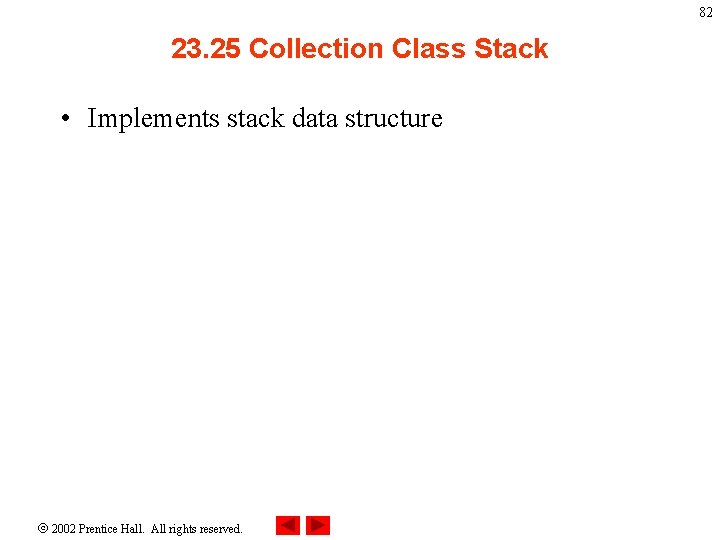
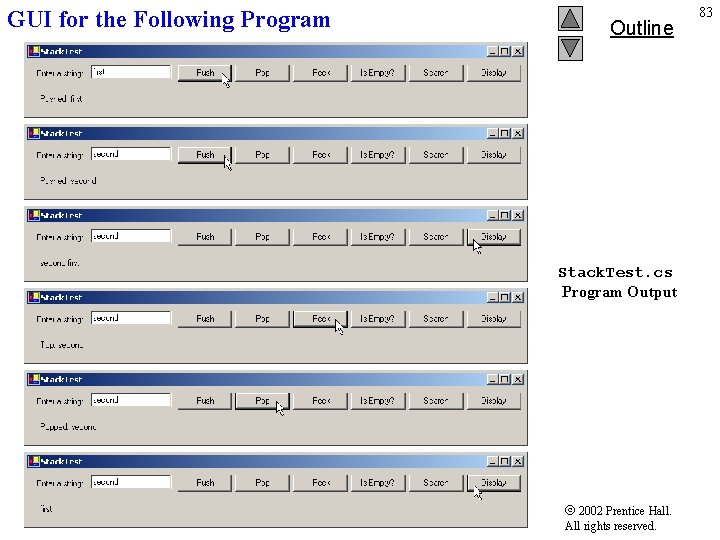
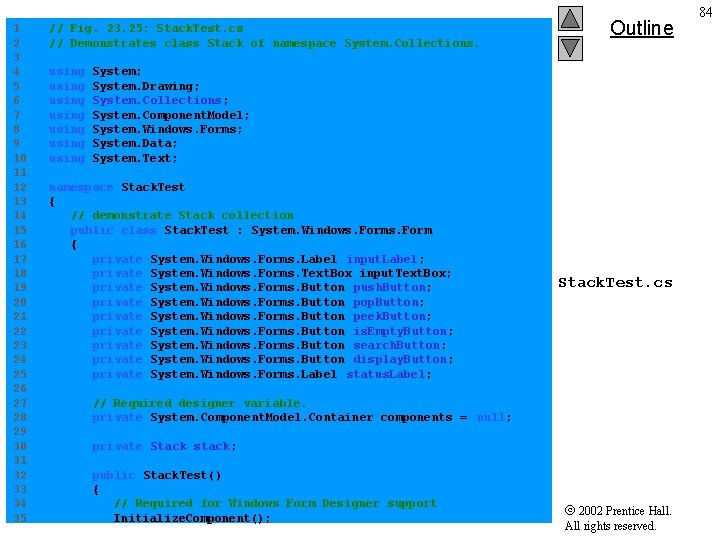
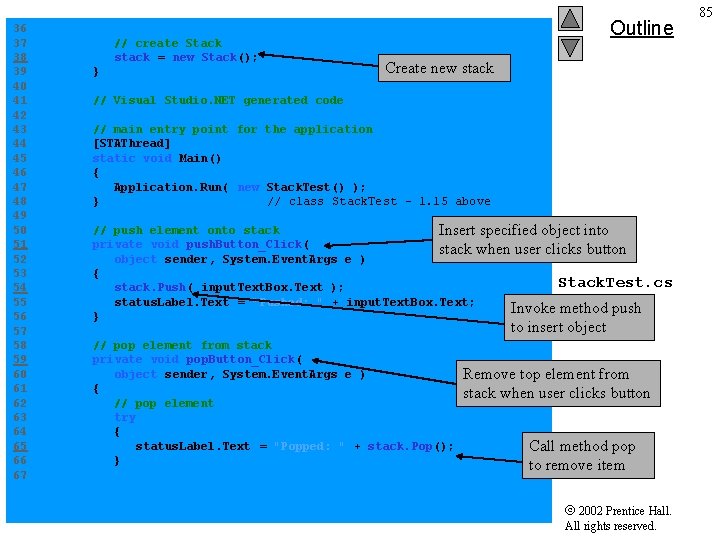
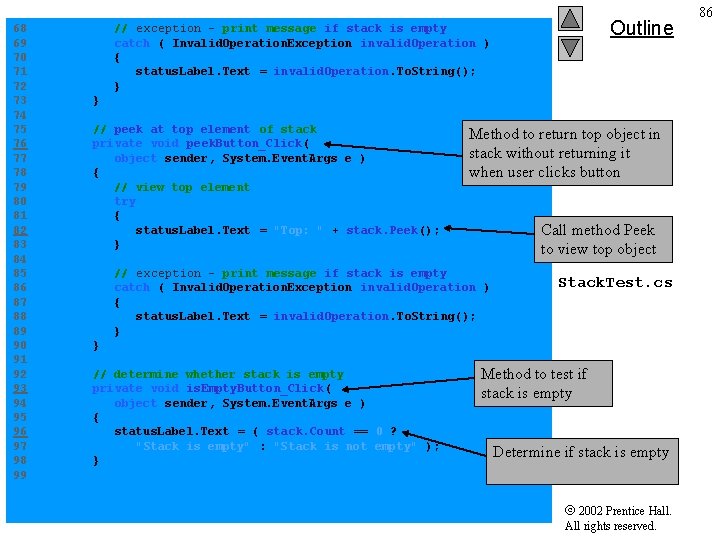
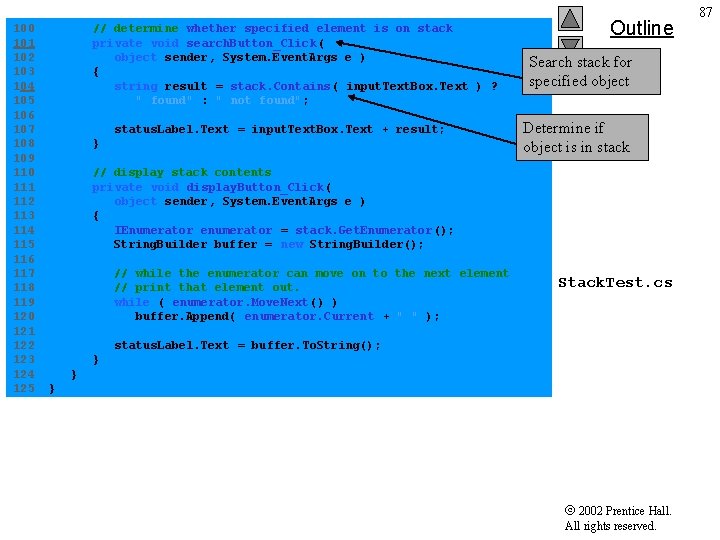
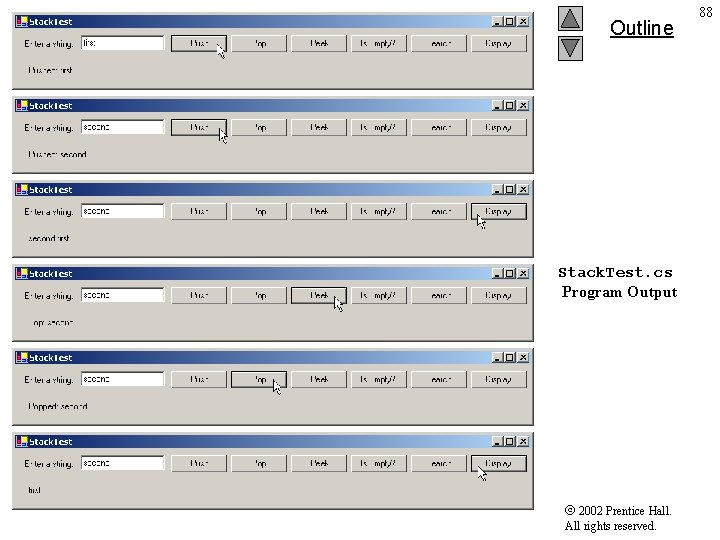
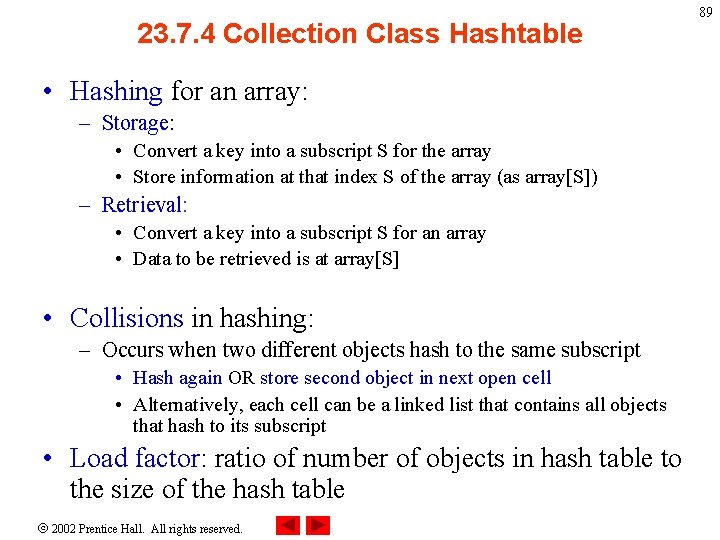
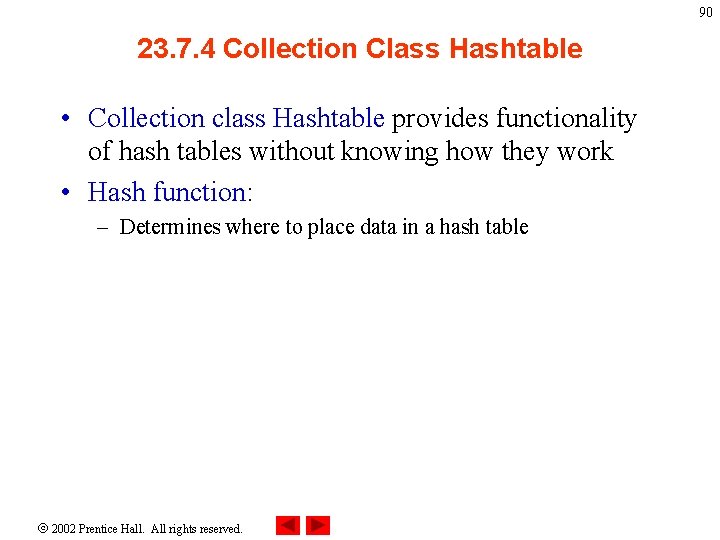
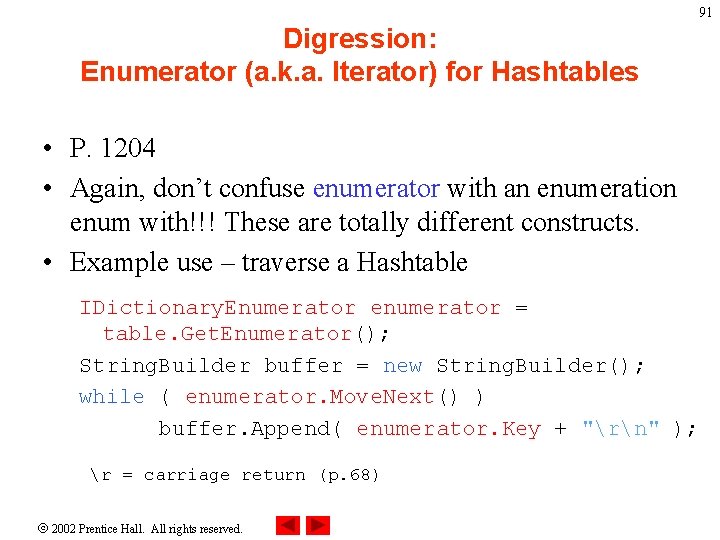
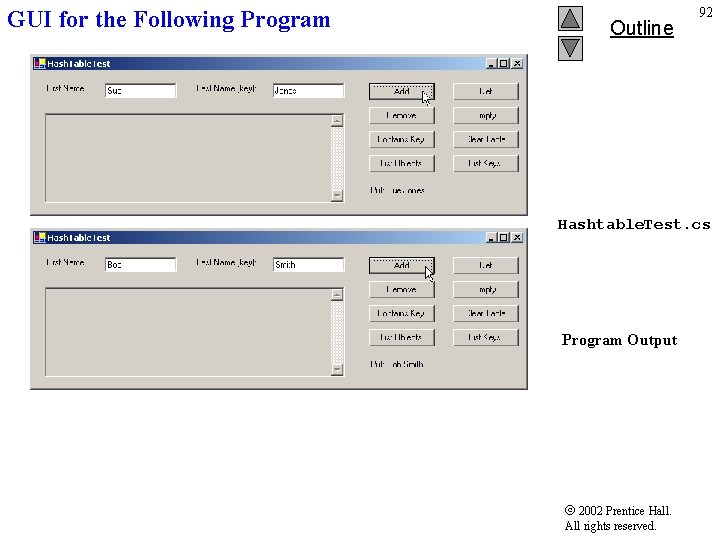
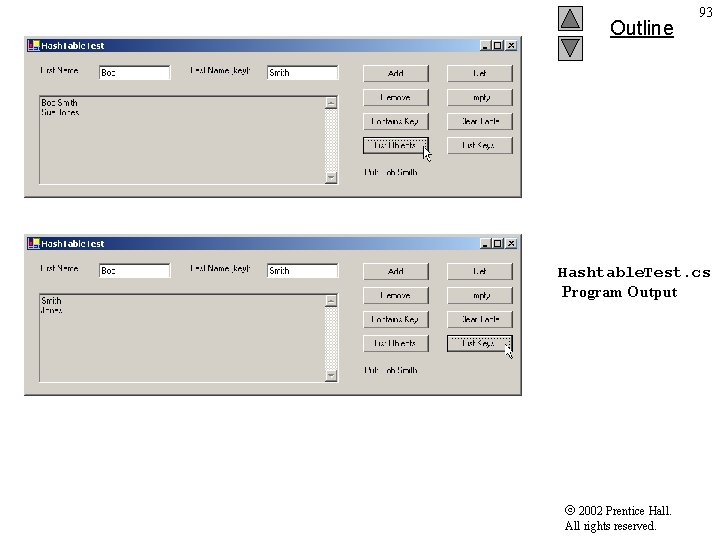
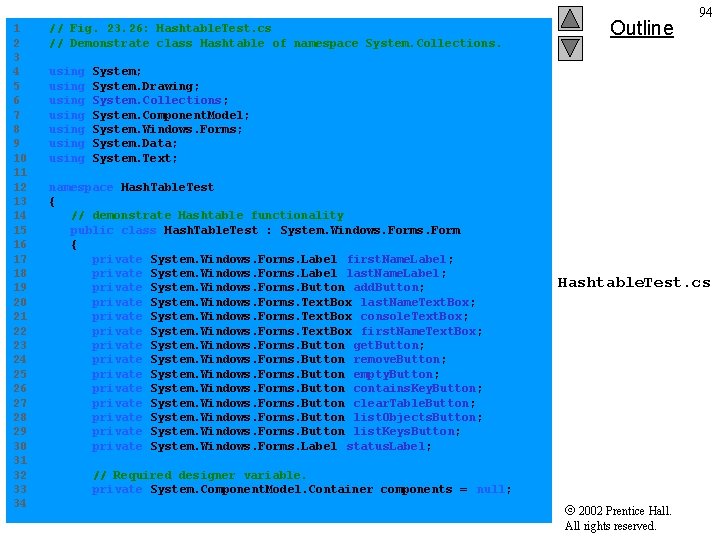
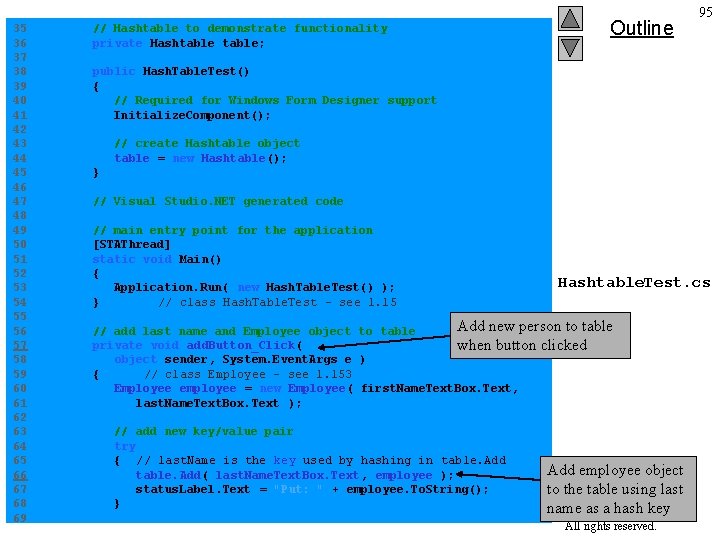
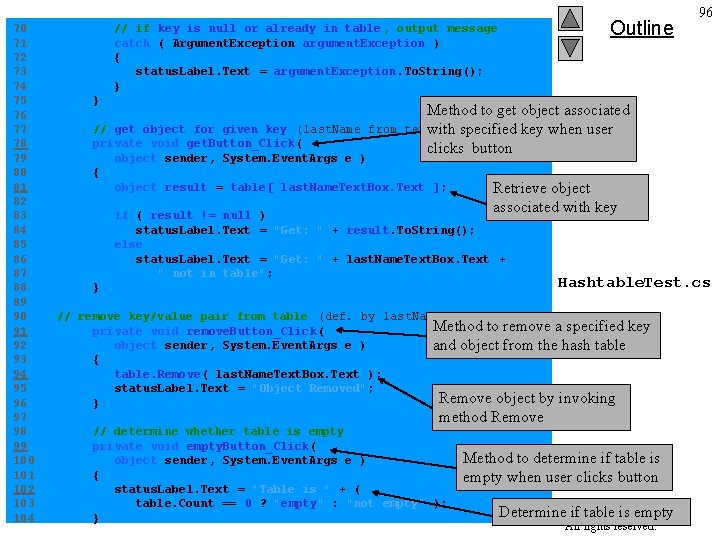
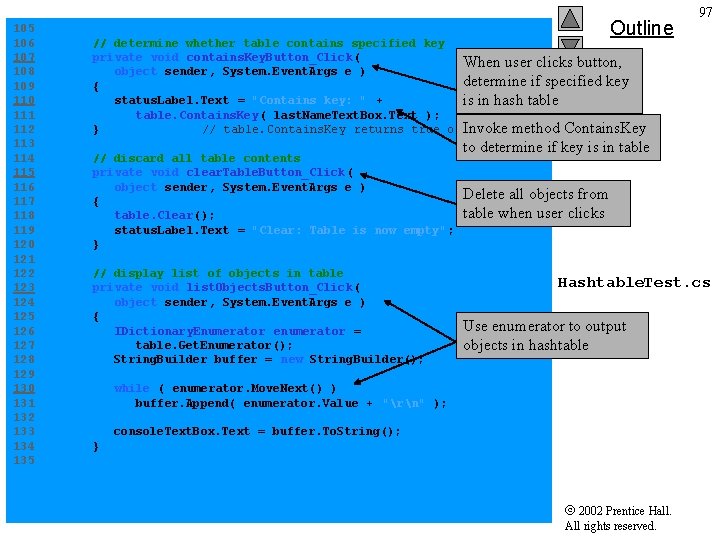
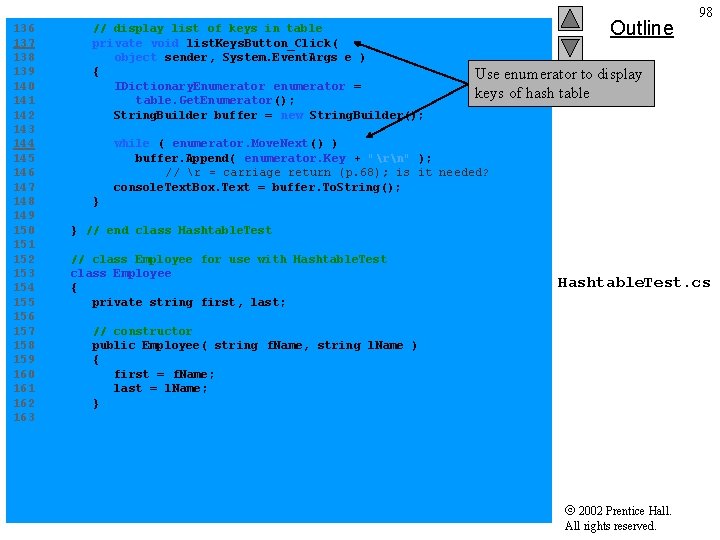
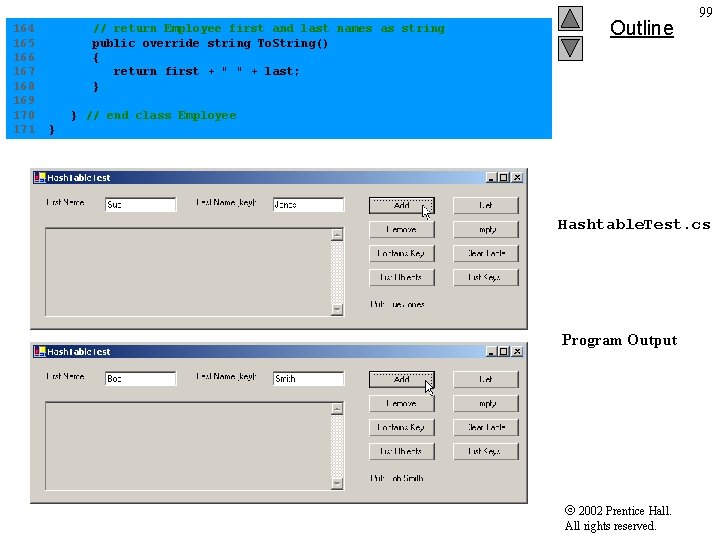
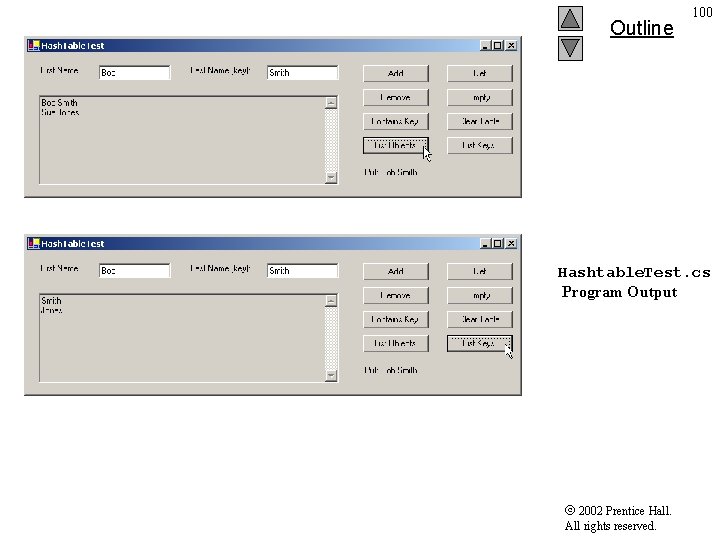
- Slides: 100
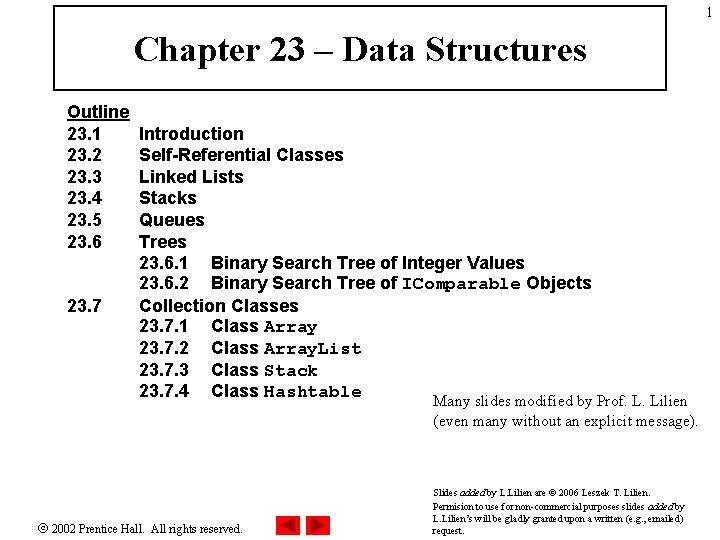
1 Chapter 23 – Data Structures Outline 23. 1 23. 2 23. 3 23. 4 23. 5 23. 6 23. 7 Introduction Self-Referential Classes Linked Lists Stacks Queues Trees 23. 6. 1 Binary Search Tree of Integer Values 23. 6. 2 Binary Search Tree of IComparable Objects Collection Classes 23. 7. 1 Class Array 23. 7. 2 Class Array. List 23. 7. 3 Class Stack 23. 7. 4 Class Hashtable Many slides modified by Prof. L. Lilien (even many without an explicit message). 2002 Prentice Hall. All rights reserved. Slides added by L. Lilien are © 2006 Leszek T. Lilien. Permision to use for non-commercial purposes slides added by L. Lilien’s will be gladly granted upon a written (e. g. , emailed) request.
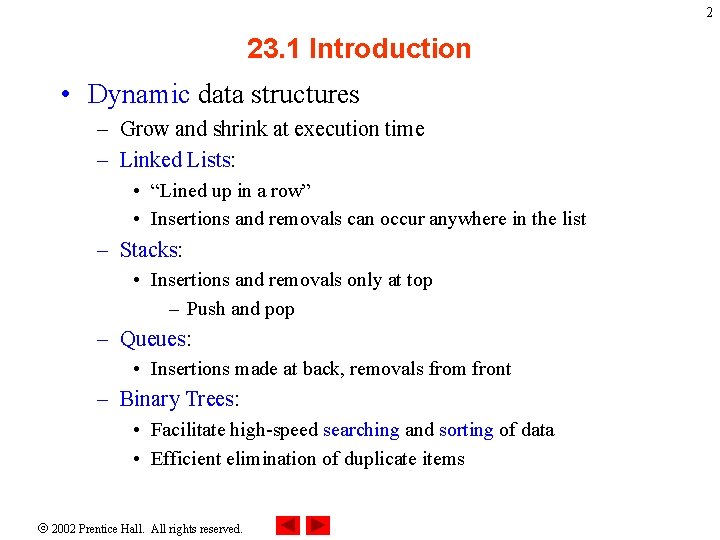
2 23. 1 Introduction • Dynamic data structures – Grow and shrink at execution time – Linked Lists: • “Lined up in a row” • Insertions and removals can occur anywhere in the list – Stacks: • Insertions and removals only at top – Push and pop – Queues: • Insertions made at back, removals from front – Binary Trees: • Facilitate high-speed searching and sorting of data • Efficient elimination of duplicate items 2002 Prentice Hall. All rights reserved.
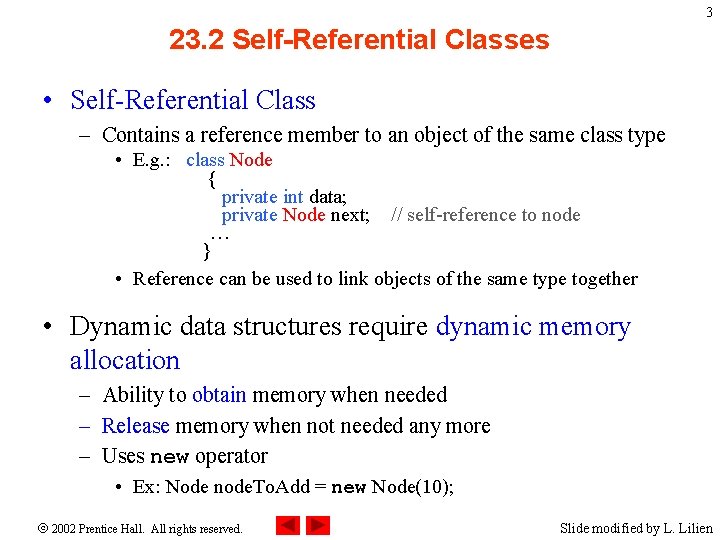
3 23. 2 Self-Referential Classes • Self-Referential Class – Contains a reference member to an object of the same class type • E. g. : class Node { private int data; private Node next; // self-reference to node … } • Reference can be used to link objects of the same type together • Dynamic data structures require dynamic memory allocation – Ability to obtain memory when needed – Release memory when not needed any more – Uses new operator • Ex: Node node. To. Add = new Node(10); 2002 Prentice Hall. All rights reserved. Slide modified by L. Lilien
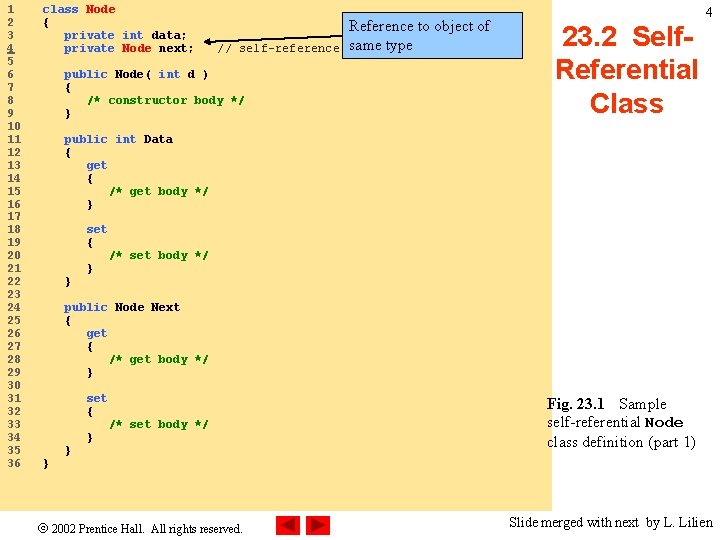
1 class Node 2 { 3 private int data; 4 private Node next; // self-reference 5 6 public Node( int d ) 7 { 8 /* constructor body */ 9 } 10 11 public int Data 12 { 13 get 14 { 15 /* get body */ 16 } 17 18 set 19 { 20 /* set body */ 21 } 22 } 23 24 public Node Next 25 { 26 get 27 { 28 /* get body */ 29 } 30 31 set 32 { 33 /* set body */ 34 } 35 } 36 } 2002 Prentice Hall. All rights reserved. Reference to object of same type 4 23. 2 Self. Referential Class Fig. 23. 1 Sample self-referential Node class definition (part 1) Slide merged with next by L. Lilien
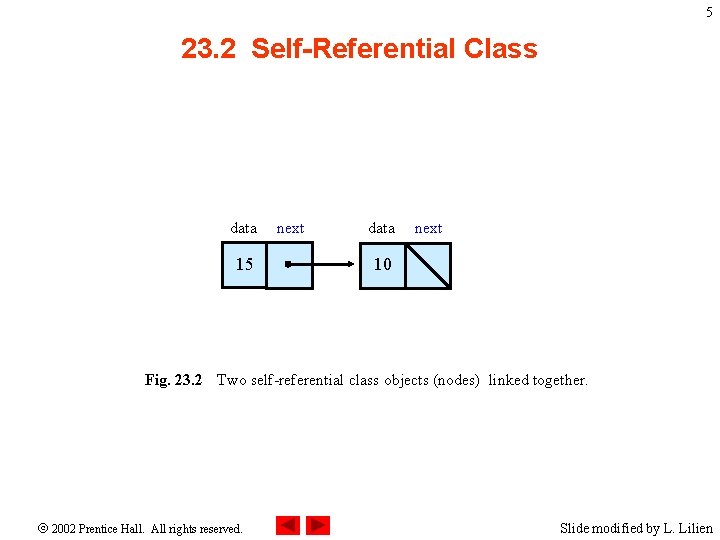
5 23. 2 Self-Referential Class data 15 next data next 10 Fig. 23. 2 Two self-referential class objects (nodes) linked together. 2002 Prentice Hall. All rights reserved. Slide modified by L. Lilien
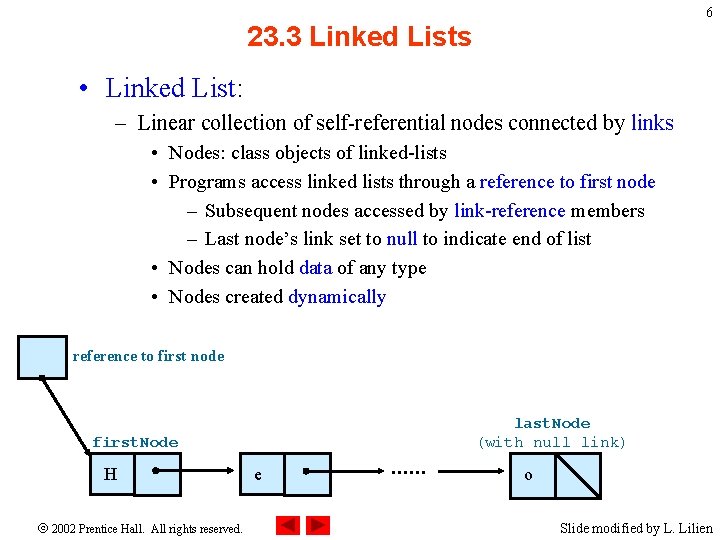
6 23. 3 Linked Lists • Linked List: – Linear collection of self-referential nodes connected by links • Nodes: class objects of linked-lists • Programs access linked lists through a reference to first node – Subsequent nodes accessed by link-reference members – Last node’s link set to null to indicate end of list • Nodes can hold data of any type • Nodes created dynamically reference to first node last. Node (with null link) first. Node H 2002 Prentice Hall. All rights reserved. e …… o Slide modified by L. Lilien
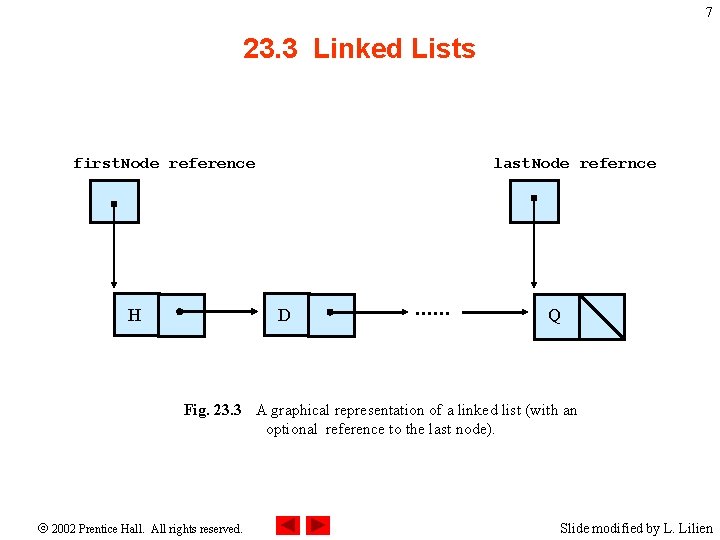
7 23. 3 Linked Lists first. Node reference H last. Node refernce D …… Q Fig. 23. 3 A graphical representation of a linked list (with an optional reference to the last node). 2002 Prentice Hall. All rights reserved. Slide modified by L. Lilien
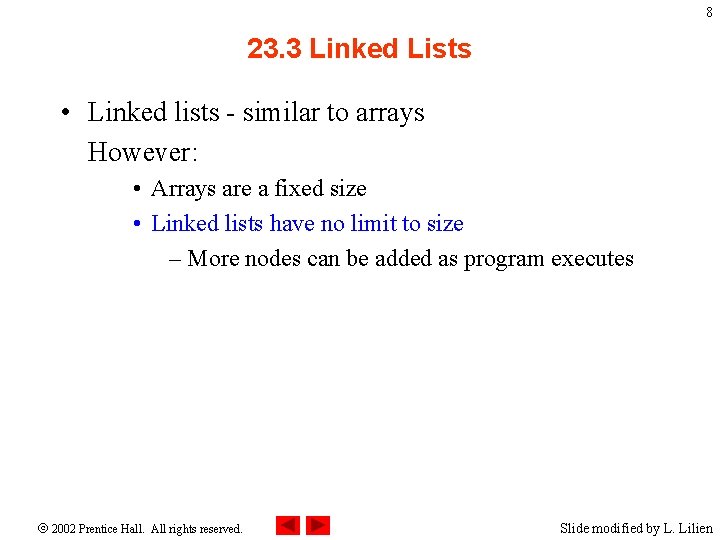
8 23. 3 Linked Lists • Linked lists - similar to arrays However: • Arrays are a fixed size • Linked lists have no limit to size – More nodes can be added as program executes 2002 Prentice Hall. All rights reserved. Slide modified by L. Lilien
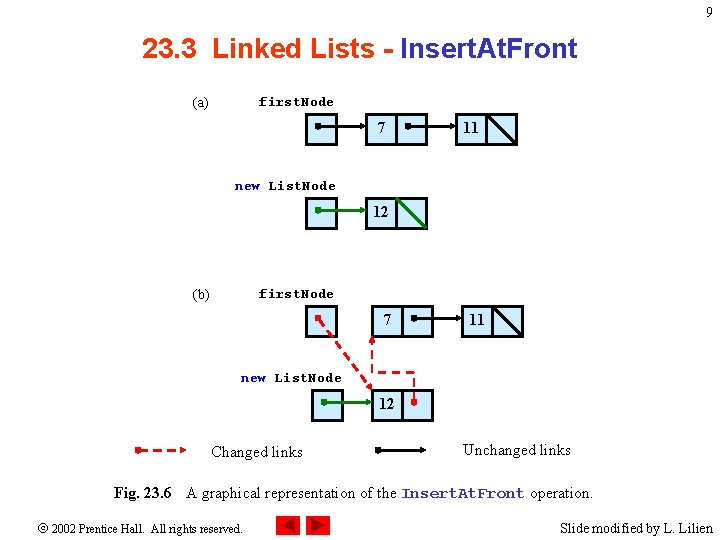
9 23. 3 Linked Lists - Insert. At. Front first. Node (a) 7 11 new List. Node 12 first. Node (b) 7 11 new List. Node 12 Changed links Unchanged links Fig. 23. 6 A graphical representation of the Insert. At. Front operation. 2002 Prentice Hall. All rights reserved. Slide modified by L. Lilien
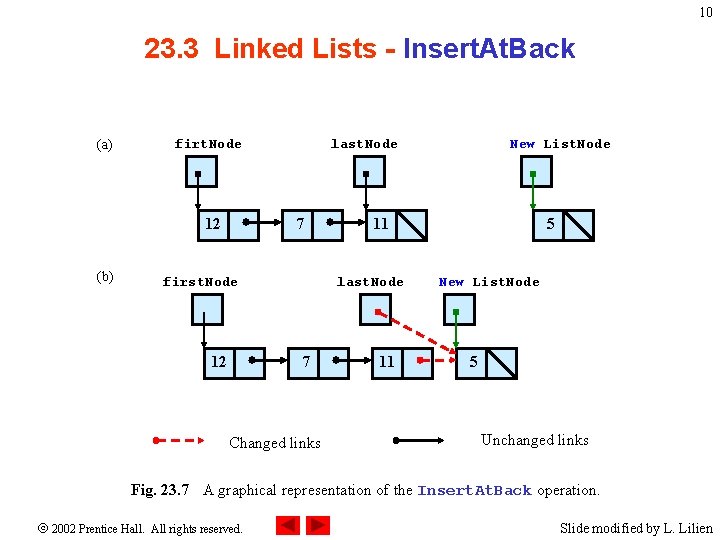
10 23. 3 Linked Lists - Insert. At. Back (a) firt. Node 12 (b) last. Node 7 first. Node 12 11 last. Node 7 Changed links New List. Node 11 5 New List. Node 5 Unchanged links Fig. 23. 7 A graphical representation of the Insert. At. Back operation. 2002 Prentice Hall. All rights reserved. Slide modified by L. Lilien
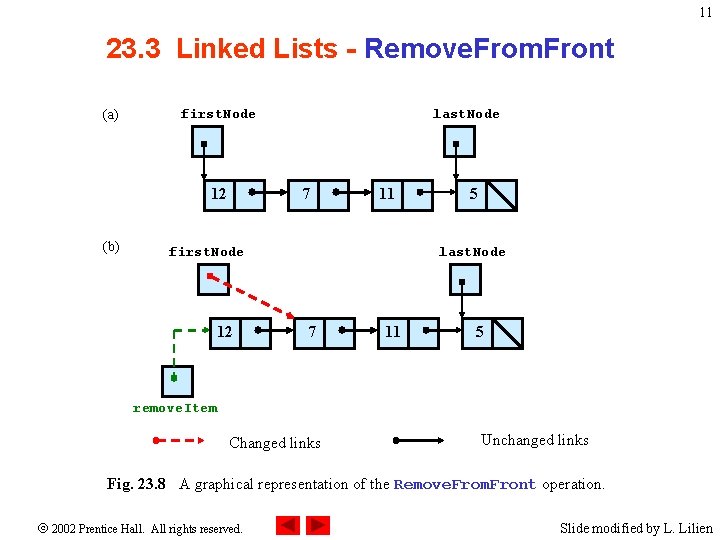
11 23. 3 Linked Lists - Remove. From. Front (a) first. Node 12 (b) last. Node 7 11 first. Node 12 5 last. Node 7 11 5 remove. Item Changed links Unchanged links Fig. 23. 8 A graphical representation of the Remove. From. Front operation. 2002 Prentice Hall. All rights reserved. Slide modified by L. Lilien
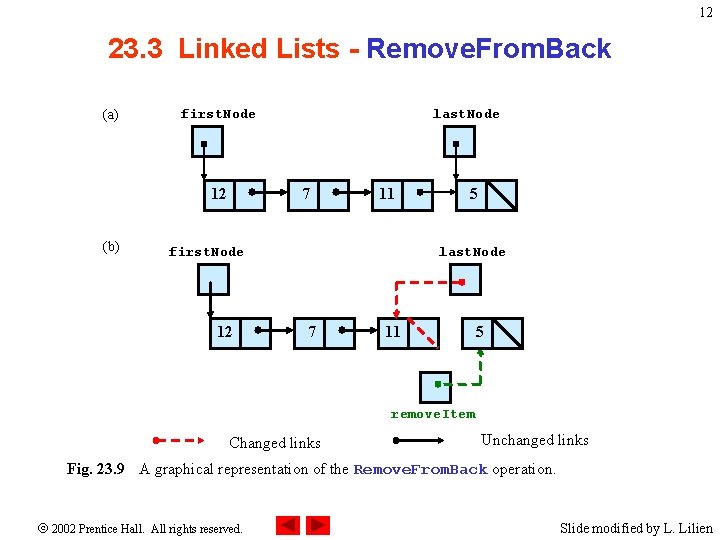
12 23. 3 Linked Lists - Remove. From. Back (a) first. Node 12 (b) last. Node 7 11 first. Node 12 5 last. Node 7 11 5 remove. Item Changed links Unchanged links Fig. 23. 9 A graphical representation of the Remove. From. Back operation. 2002 Prentice Hall. All rights reserved. Slide modified by L. Lilien
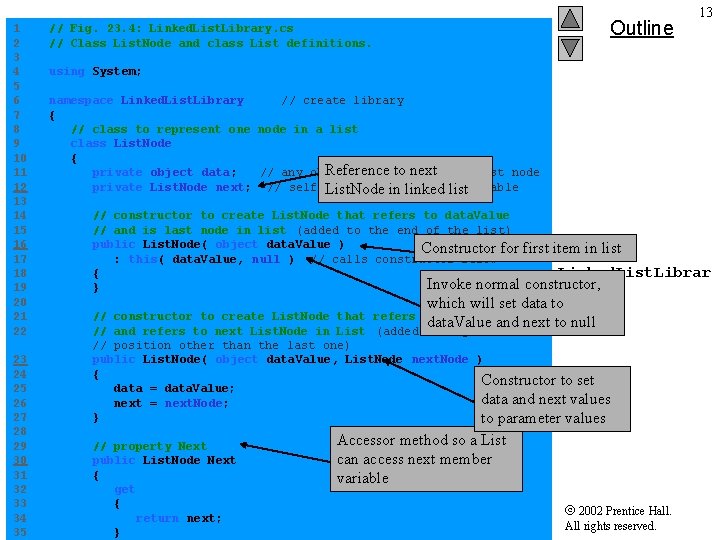
Outline 13 1 // Fig. 23. 4: Linked. List. Library. cs 2 // Class List. Node and class List definitions. 3 4 using System; 5 6 namespace Linked. List. Library // create library 7 { 8 // class to represent one node in a list 9 class List. Node 10 { Reference to next 11 private object data; // any object can be within a list node 12 private List. Node next; // self-referential member variable List. Node in linked list 13 14 // constructor to create List. Node that refers to data. Value 15 // and is last node in list (added to the end of the list) 16 public List. Node( object data. Value ) Constructor first item in list 17 : this( data. Value, null ) // calls constructor below Linked. List. Librar 18 { Invoke normal constructor, 19 } y. cs 20 which will set data to 21 // constructor to create List. Node that refers to data. Value and next to null 22 // and refers to next List. Node in List (added to any list // position other than the last one) 23 public List. Node( object data. Value, List. Node next. Node ) 24 { Constructor to set 25 data = data. Value; data and next values 26 next = next. Node; 27 } to parameter values 28 Accessor method so a List 29 // property Next 30 public List. Node Next can access next member 31 { variable 32 get 33 { 2002 Prentice Hall. 34 return next; All rights reserved. 35 }
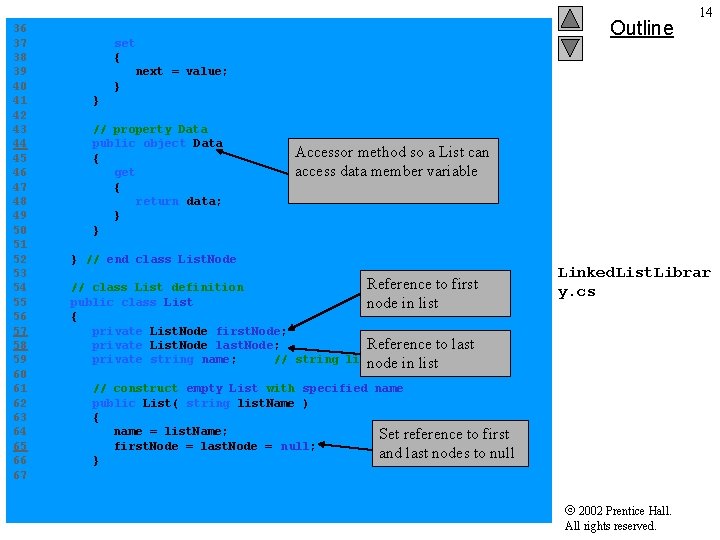
36 37 set 38 { 39 next = value; 40 } 41 } 42 43 // property Data 44 public object Data Accessor method so a List can 45 { 46 get access data member variable 47 { 48 return data; 49 } 50 } 51 52 } // end class List. Node 53 Reference to first 54 // class List definition 55 public class List node in list 56 { 57 private List. Node first. Node; 58 private List. Node last. Node; Reference to last 59 private string name; // string like "list" to display node in list 60 61 // construct empty List with specified name 62 public List( string list. Name ) 63 { 64 name = list. Name; Set reference to first 65 first. Node = last. Node = null; and last nodes to null 66 } 67 Outline 14 Linked. List. Librar y. cs 2002 Prentice Hall. All rights reserved.
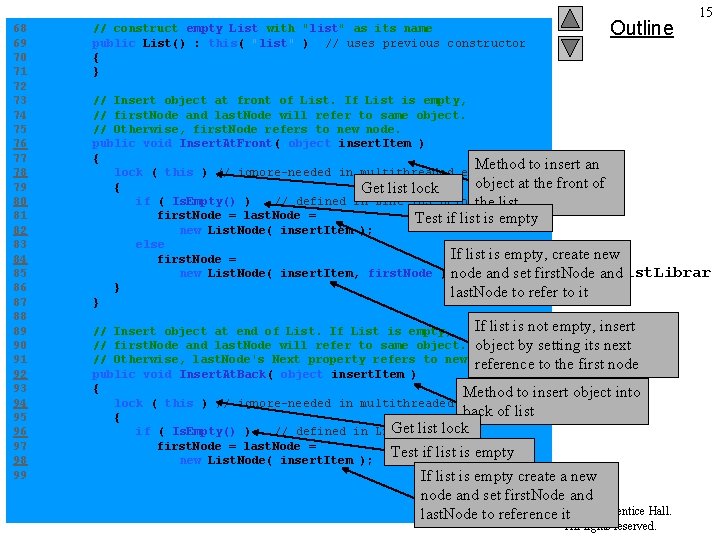
Outline 15 68 // construct empty List with "list" as its name 69 public List() : this( "list" ) // uses previous constructor 70 { 71 } 72 73 // Insert object at front of List. If List is empty, 74 // first. Node and last. Node will refer to same object. 75 // Otherwise, first. Node refers to new node. 76 public void Insert. At. Front( object insert. Item ) 77 { Method to insert an 78 lock ( this ) // ignore-needed in multithreaded environment object at the front of 79 { Get list lock 80 if ( Is. Empty() ) // defined in Line 163 belowthe list 81 first. Node = last. Node = Test if list is empty 82 new List. Node( insert. Item ); 83 else If list is empty, create new 84 first. Node = Linked. List. Librar 85 new List. Node( insert. Item, first. Node ); node and set first. Node and 86 } y. cs last. Node to refer to it 87 } 88 89 // Insert object at end of List. If List is empty, If list is not empty, insert 90 // first. Node and last. Node will refer to same object by setting its next 91 // Otherwise, last. Node's Next property refers to new node. reference to the first node 92 public void Insert. At. Back( object insert. Item ) 93 { Method to insert object into 94 lock ( this ) // ignore-needed in multithreaded environment back of list 95 { Get list lock 96 if ( Is. Empty() ) // defined in Line 163 below 97 first. Node = last. Node = Test if list is empty 98 new List. Node( insert. Item ); 99 If list is empty create a new node and set first. Node and last. Node to reference it 2002 Prentice Hall. All rights reserved.
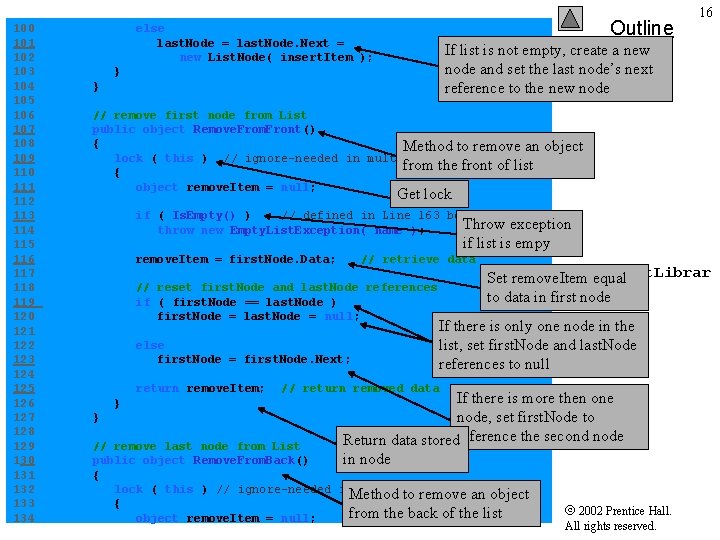
Outline 16 100 else 101 last. Node = last. Node. Next = If list is not empty, create a new 102 new List. Node( insert. Item ); node and set the last node’s next 103 } 104 } reference to the new node 105 106 // remove first node from List 107 public object Remove. From. Front() 108 { Method to remove an object 109 lock ( this ) // ignore-needed in multithreaded environ. from the front of list 110 { 111 object remove. Item = null; Get lock 112 113 if ( Is. Empty() ) // defined in Line 163 below Throw exception 114 throw new Empty. List. Exception( name ) ; 115 if list is empy 116 remove. Item = first. Node. Data; // retrieve data Linked. List. Librar 117 Set remove. Item equal 118 // reset first. Node and last. Node references y. cs to data in first node 119 if ( first. Node == last. Node ) 120 first. Node = last. Node = null; If there is only one node in the 121 122 else list, set first. Node and last. Node 123 first. Node = first. Node. Next; references to null 124 125 return remove. Item; // return removed data If there is more then one 126 } 127 } node, set first. Node to 128 Return data stored reference the second node 129 // remove last node from List 130 public object Remove. From. Back() in node 131 { 132 lock ( this ) // ignore-needed in multithreaded environ. Method to remove an object 133 { 2002 Prentice Hall. from the back of the list 134 object remove. Item = null; All rights reserved.
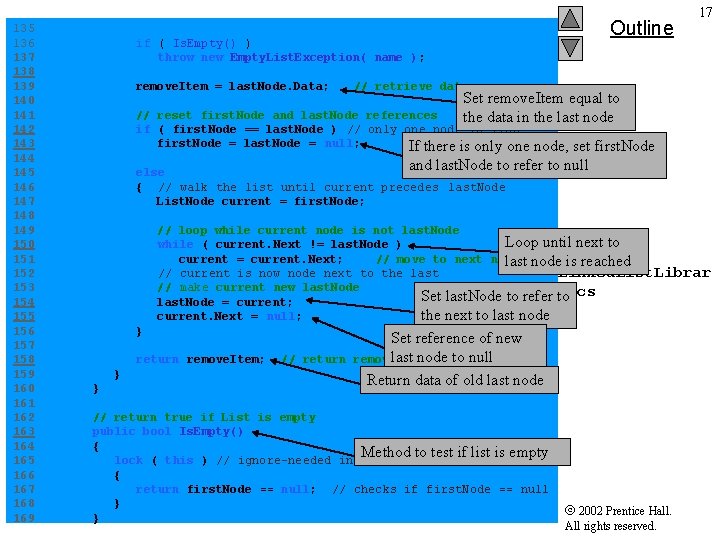
Outline 17 135 136 if ( Is. Empty() ) 137 throw new Empty. List. Exception( name ) ; 138 139 remove. Item = last. Node. Data; // retrieve data Set remove. Item equal to 140 141 // reset first. Node and last. Node references the data in the last node 142 if ( first. Node == last. Node ) // only one node in list 143 first. Node = last. Node = null; If there is only one node, set first. Node 144 and last. Node to refer to null 145 else 146 { // walk the list until current precedes last. Node 147 List. Node current = first. Node; 148 149 // loop while current node is not last. Node Loop until next to 150 while ( current. Next != last. Node ) 151 current = current. Next; // move to next node last node is reached Linked. List. Librar 152 // current is now node next to the last 153 // make current new last. Node y. cs Set last. Node to refer to 154 last. Node = current; 155 current. Next = null; the next to last node 156 } Set reference of new 157 last node to null 158 return remove. Item; // return removed data 159 } Return data of old last node 160 } 161 162 // return true if List is empty 163 public bool Is. Empty() 164 { Method to test if list is empty 165 lock ( this ) // ignore-needed in multithreaded environment 166 { 167 return first. Node == null; // checks if first. Node == null 168 } 2002 Prentice Hall. 169 } All rights reserved.
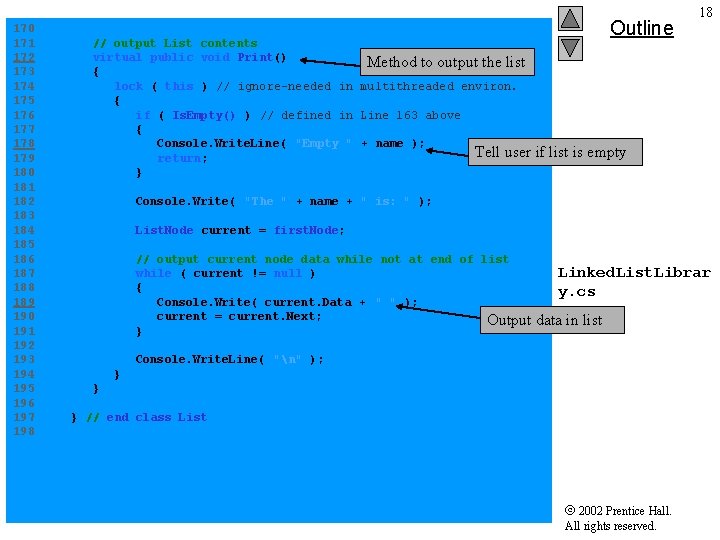
Outline 18 170 171 // output List contents 172 virtual public void Print() Method to output the list 173 { 174 lock ( this ) // ignore-needed in multithreaded environ. 175 { 176 if ( Is. Empty() ) // defined in Line 163 above 177 { 178 Console. Write. Line( "Empty " + name ); Tell user if list is empty 179 return; 180 } 181 182 Console. Write( "The " + name + " is: " ); 183 184 List. Node current = first. Node; 185 186 // output current node data while not at end of list Linked. List. Librar 187 while ( current != null ) 188 { y. cs 189 Console. Write( current. Data + " " ); 190 current = current. Next; Output data in list 191 } 192 193 Console. Write. Line( "n" ); 194 } 195 } 196 197 } // end class List 198 2002 Prentice Hall. All rights reserved.
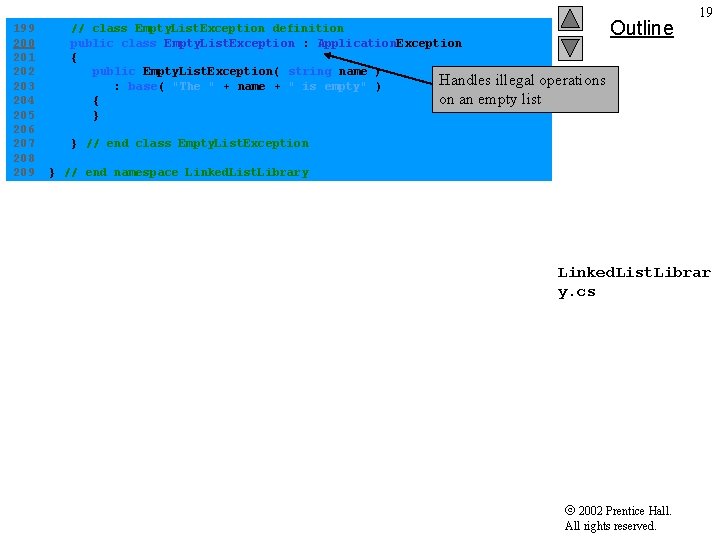
199 // class Empty. List. Exception definition 200 public class Empty. List. Exception : Application. Exception 201 { 202 public Empty. List. Exception( string name ) Handles illegal operations 203 : base( "The " + name + " is empty" ) 204 { on an empty list 205 } 206 207 } // end class Empty. List. Exception 208 209 } // end namespace Linked. List. Library Outline 19 Linked. List. Librar y. cs 2002 Prentice Hall. All rights reserved.
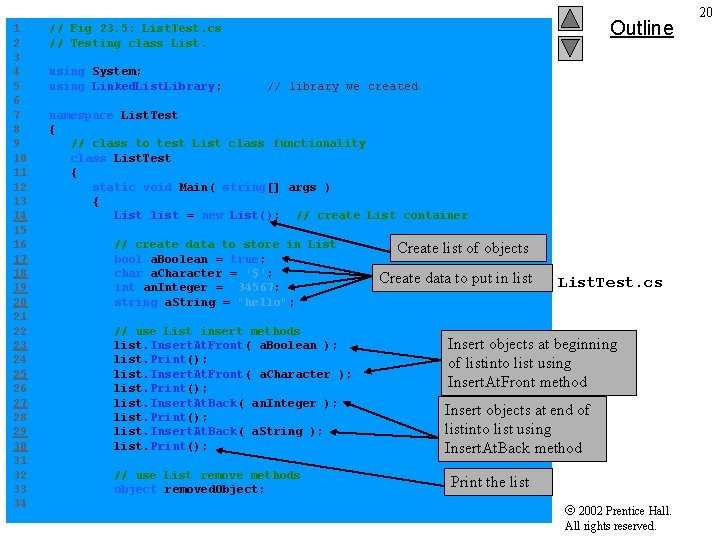
Outline 1 // Fig 23. 5: List. Test. cs 2 // Testing class List. 3 4 using System; 5 using Linked. List. Library; // library we created 6 7 namespace List. Test 8 { 9 // class to test List class functionality 10 class List. Test 11 { 12 static void Main( string[] args ) 13 { 14 List list = new List(); // create List container 15 16 // create data to store in List Create list of objects 17 bool a. Boolean = true; 18 char a. Character = '$'; Create data to put in list List. Test. cs 19 int an. Integer = 34567; 20 string a. String = "hello"; 21 22 // use List insert methods 23 list. Insert. At. Front( a. Boolean ); Insert objects at beginning 24 list. Print(); of listinto list using 25 list. Insert. At. Front( a. Character ); Insert. At. Front method 26 list. Print(); 27 list. Insert. At. Back( an. Integer ); Insert objects at end of 28 list. Print(); listinto list using 29 list. Insert. At. Back( a. String ); 30 list. Print(); Insert. At. Back method 31 32 // use List remove methods Print the list 33 object removed. Object; 34 2002 Prentice Hall. All rights reserved. 20
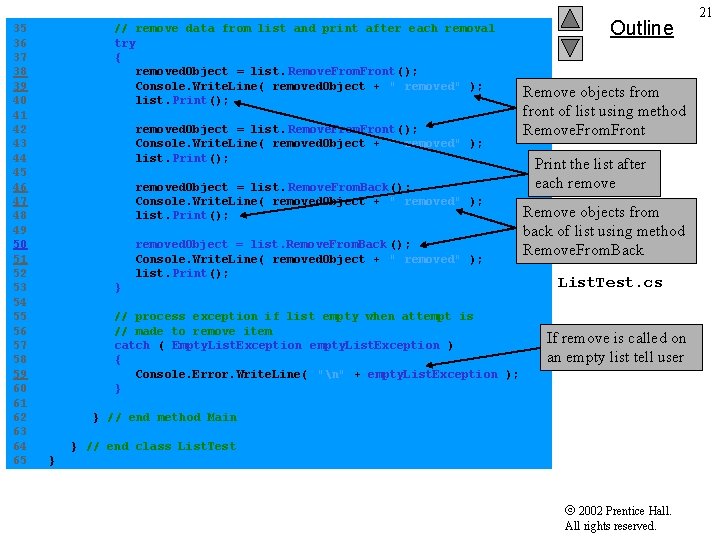
Outline 35 // remove data from list and print after each removal 36 try 37 { 38 removed. Object = list. Remove. From. Front(); 39 Console. Write. Line( removed. Object + " removed" ); Remove objects from 40 list. Print(); front of list using method 41 42 removed. Object = list. Remove. From. Front(); Remove. From. Front 43 Console. Write. Line( removed. Object + " removed" ); 44 list. Print(); Print the list after 45 each remove 46 removed. Object = list. Remove. From. Back(); 47 Console. Write. Line( removed. Object + " removed" ); Remove objects from 48 list. Print(); 49 back of list using method 50 removed. Object = list. Remove. From. Back (); Remove. From. Back 51 Console. Write. Line( removed. Object + " removed" ); 52 list. Print(); List. Test. cs 53 } 54 55 // process exception if list empty when attempt is 56 // made to remove item If remove is called on 57 catch ( Empty. List. Exception empty. List. Exception ) an empty list tell user 58 { 59 Console. Error. Write. Line( "n" + empty. List. Exception ); 60 } 61 62 } // end method Main 63 64 } // end class List. Test 65 } 2002 Prentice Hall. All rights reserved. 21
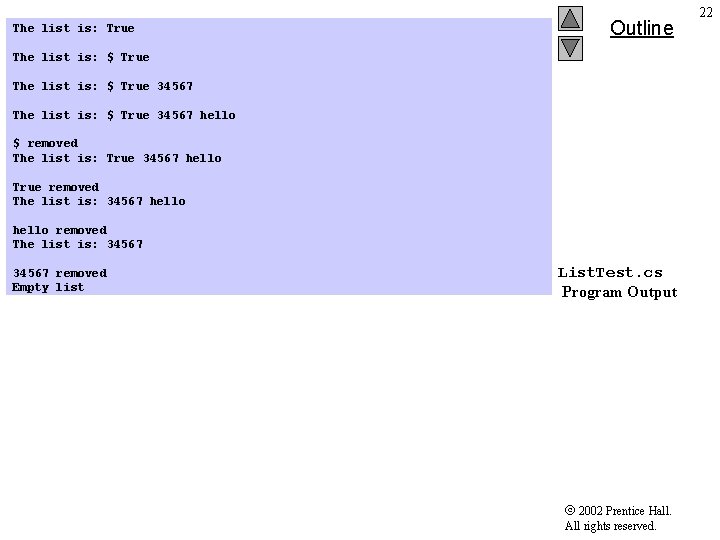
The list is: True The list is: $ True 34567 hello $ removed The list is: True 34567 hello True removed The list is: 34567 hello removed The list is: 34567 removed Empty list Outline List. Test. cs Program Output 2002 Prentice Hall. All rights reserved. 22
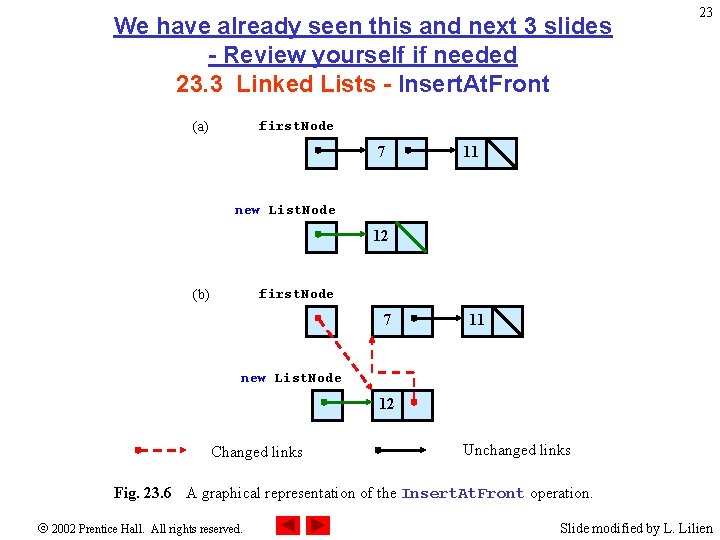
We have already seen this and next 3 slides - Review yourself if needed 23. 3 Linked Lists - Insert. At. Front 23 first. Node (a) 7 11 new List. Node 12 first. Node (b) 7 11 new List. Node 12 Changed links Unchanged links Fig. 23. 6 A graphical representation of the Insert. At. Front operation. 2002 Prentice Hall. All rights reserved. Slide modified by L. Lilien
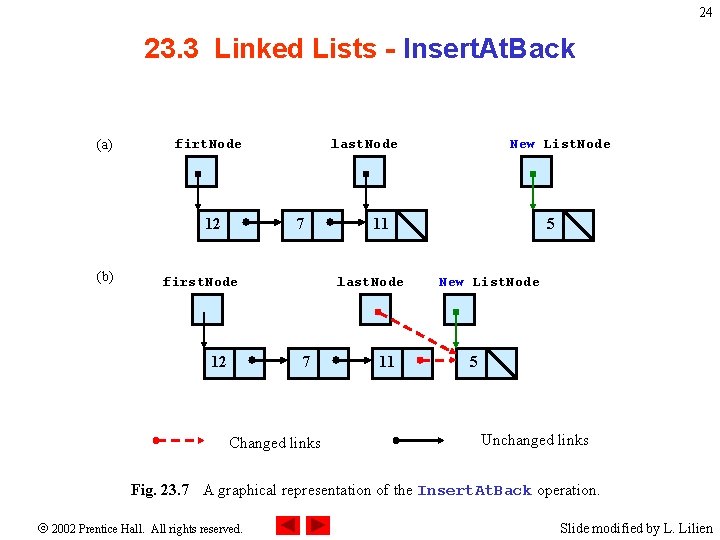
24 23. 3 Linked Lists - Insert. At. Back (a) firt. Node 12 (b) last. Node 7 first. Node 12 11 last. Node 7 Changed links New List. Node 11 5 New List. Node 5 Unchanged links Fig. 23. 7 A graphical representation of the Insert. At. Back operation. 2002 Prentice Hall. All rights reserved. Slide modified by L. Lilien
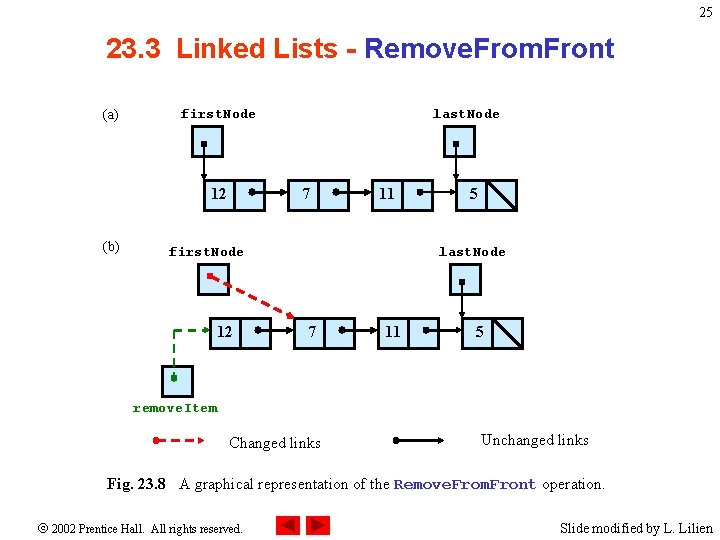
25 23. 3 Linked Lists - Remove. From. Front (a) first. Node 12 (b) last. Node 7 11 first. Node 12 5 last. Node 7 11 5 remove. Item Changed links Unchanged links Fig. 23. 8 A graphical representation of the Remove. From. Front operation. 2002 Prentice Hall. All rights reserved. Slide modified by L. Lilien
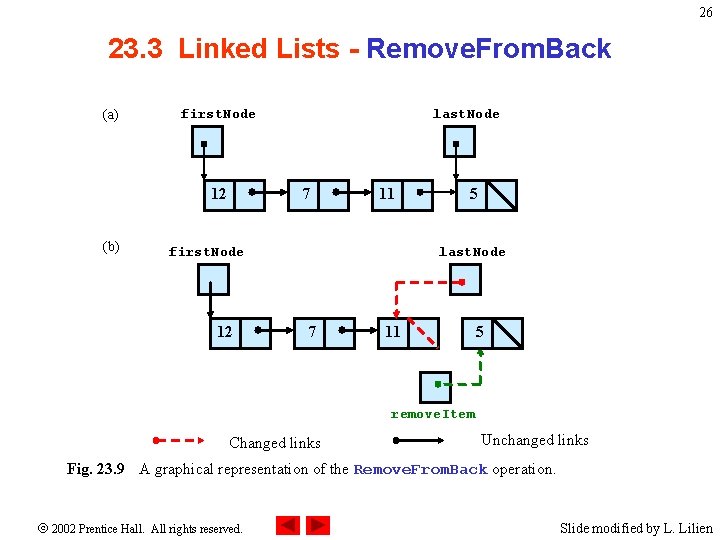
26 23. 3 Linked Lists - Remove. From. Back (a) first. Node 12 (b) last. Node 7 11 first. Node 12 5 last. Node 7 11 5 remove. Item Changed links Unchanged links Fig. 23. 9 A graphical representation of the Remove. From. Back operation. 2002 Prentice Hall. All rights reserved. Slide modified by L. Lilien
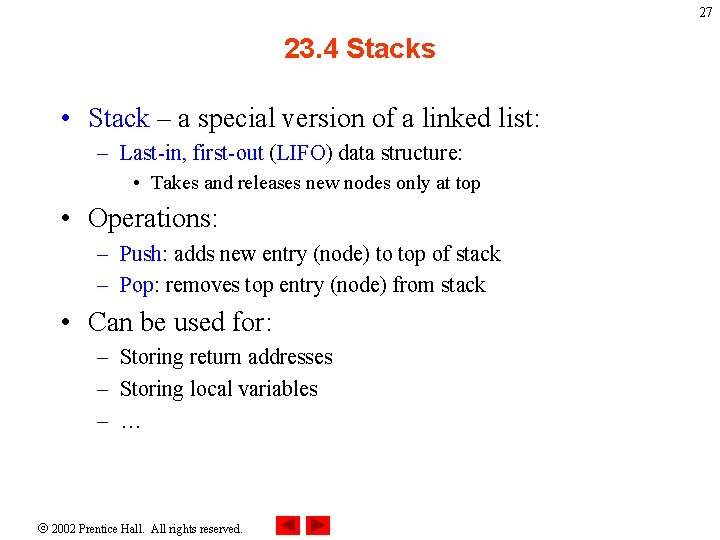
27 23. 4 Stacks • Stack – a special version of a linked list: – Last-in, first-out (LIFO) data structure: • Takes and releases new nodes only at top • Operations: – Push: adds new entry (node) to top of stack – Pop: removes top entry (node) from stack • Can be used for: – Storing return addresses – Storing local variables – … 2002 Prentice Hall. All rights reserved.
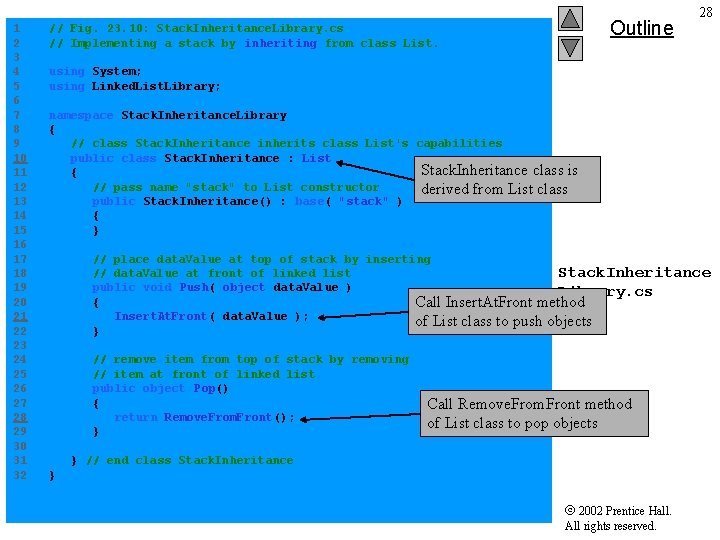
Outline 28 1 // Fig. 23. 10: Stack. Inheritance. Library. cs 2 // Implementing a stack by inheriting from class List. 3 4 using System; 5 using Linked. List. Library; 6 7 namespace Stack. Inheritance. Library 8 { 9 // class Stack. Inheritance inherits class List's capabilities 10 public class Stack. Inheritance : List Stack. Inheritance class is 11 { 12 // pass name "stack" to List constructor derived from List class 13 public Stack. Inheritance() : base( "stack" ) 14 { 15 } 16 17 // place data. Value at top of stack by inserting Stack. Inheritance 18 // data. Value at front of linked list 19 public void Push( object data. Value ) Library. cs 20 { Call Insert. At. Front method 21 Insert. At. Front( data. Value ); of List class to push objects 22 } 23 24 // remove item from top of stack by removing 25 // item at front of linked list 26 public object Pop() 27 { Call Remove. From. Front method 28 return Remove. From. Front(); of List class to pop objects 29 } 30 31 } // end class Stack. Inheritance 32 } 2002 Prentice Hall. All rights reserved.
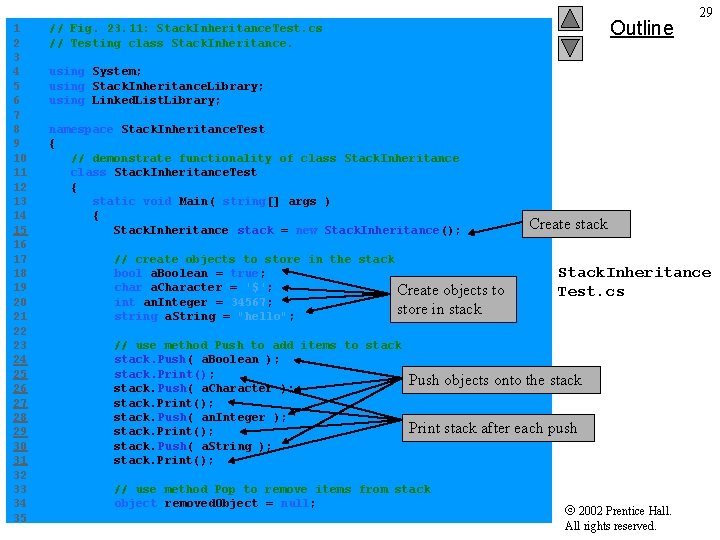
Outline 29 1 // Fig. 23. 11: Stack. Inheritance. Test. cs 2 // Testing class Stack. Inheritance. 3 4 using System; 5 using Stack. Inheritance. Library; 6 using Linked. List. Library; 7 8 namespace Stack. Inheritance. Test 9 { 10 // demonstrate functionality of class Stack. Inheritance 11 class Stack. Inheritance. Test 12 { 13 static void Main( string[] args ) 14 { Create stack 15 Stack. Inheritance stack = new Stack. Inheritance(); 16 17 // create objects to store in the stack Stack. Inheritance 18 bool a. Boolean = true; 19 char a. Character = '$'; Create objects to Test. cs 20 int an. Integer = 34567; store in stack 21 string a. String = "hello"; 22 23 // use method Push to add items to stack 24 stack. Push( a. Boolean ); 25 stack. Print(); Push objects onto the stack 26 stack. Push( a. Character ); 27 stack. Print(); 28 stack. Push( an. Integer ); Print stack after each push 29 stack. Print(); 30 stack. Push( a. String ); 31 stack. Print(); 32 33 // use method Pop to remove items from stack 34 object removed. Object = null; 2002 Prentice Hall. 35 All rights reserved.
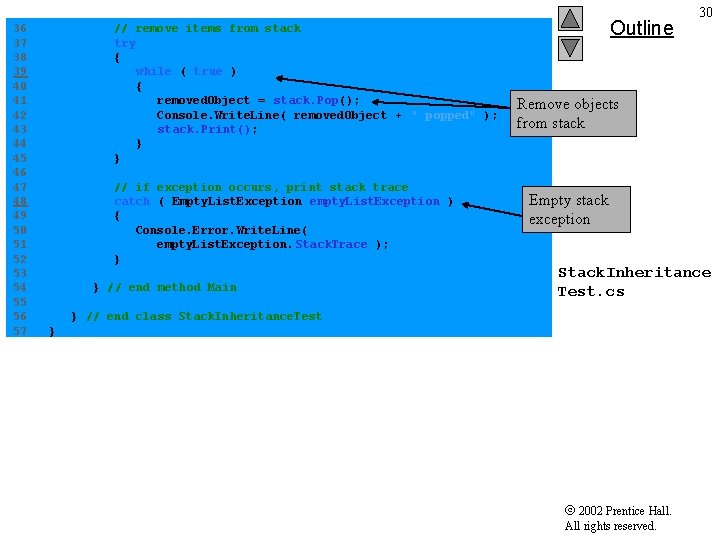
36 // remove items from stack 37 try 38 { 39 while ( true ) 40 { 41 removed. Object = stack. Pop(); 42 Console. Write. Line( removed. Object + " popped" ); 43 stack. Print(); 44 } 45 } 46 47 // if exception occurs, print stack trace 48 catch ( Empty. List. Exception empty. List. Exception ) 49 { 50 Console. Error. Write. Line( 51 empty. List. Exception. Stack. Trace ); 52 } 53 54 } // end method Main 55 56 } // end class Stack. Inheritance. Test 57 } Outline 30 Remove objects from stack Empty stack exception Stack. Inheritance Test. cs 2002 Prentice Hall. All rights reserved.
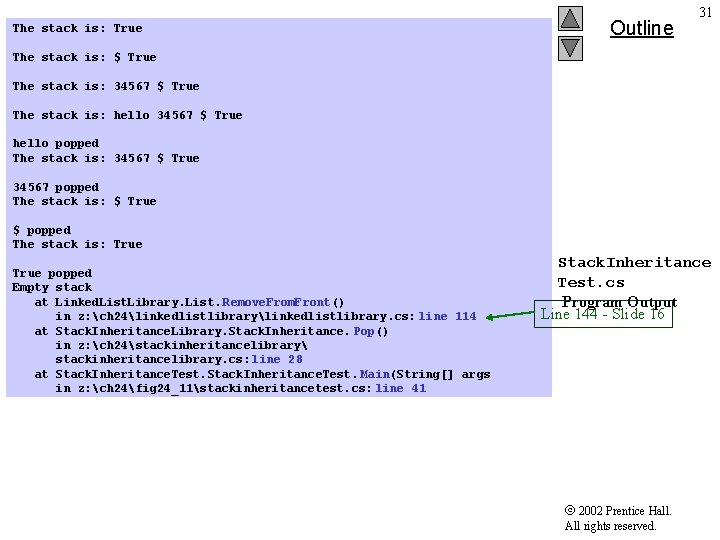
The stack is: True The stack is: $ True The stack is: 34567 $ True The stack is: hello 34567 $ True hello popped The stack is: 34567 $ True 34567 popped The stack is: $ True $ popped The stack is: True popped Empty stack at Linked. List. Library. List. Remove. From. Front() in z: ch 24linkedlistlibrary. cs: line 114 at Stack. Inheritance. Library. Stack. Inheritance. Pop() in z: ch 24stackinheritancelibrary stackinheritancelibrary. cs: line 28 at Stack. Inheritance. Test. Main(String[] args in z: ch 24fig 24_11stackinheritancetest. cs: line 41 Outline 31 Stack. Inheritance Test. cs Program Output Line 144 - Slide 16 2002 Prentice Hall. All rights reserved.
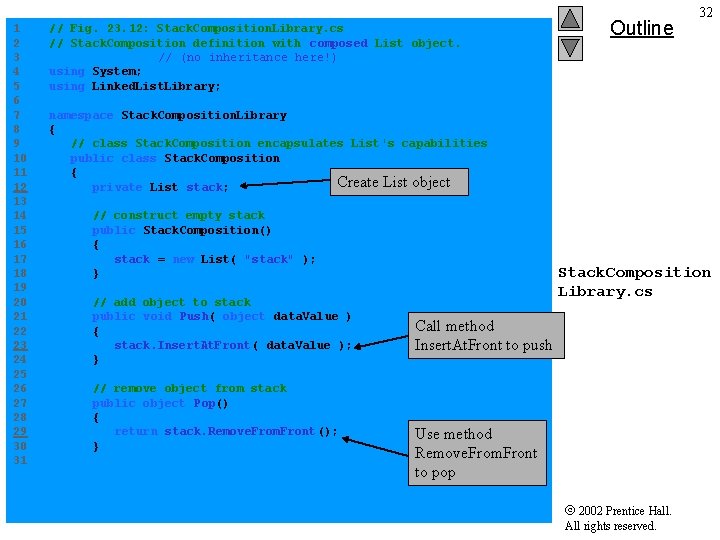
1 // Fig. 23. 12: Stack. Composition. Library. cs 2 // Stack. Composition definition with composed List object. 3 // (no inheritance here!) 4 using System; 5 using Linked. List. Library; 6 7 namespace Stack. Composition. Library 8 { 9 // class Stack. Composition encapsulates List's capabilities 10 public class Stack. Composition 11 { Create List object 12 private List stack; 13 14 // construct empty stack 15 public Stack. Composition() 16 { 17 stack = new List( "stack" ); 18 } 19 20 // add object to stack 21 public void Push( object data. Value ) Call method 22 { 23 stack. Insert. At. Front( data. Value ); Insert. At. Front to push 24 } 25 26 // remove object from stack 27 public object Pop() 28 { 29 return stack. Remove. From. Front(); Use method 30 } Remove. From. Front 31 Outline 32 Stack. Composition Library. cs to pop 2002 Prentice Hall. All rights reserved.
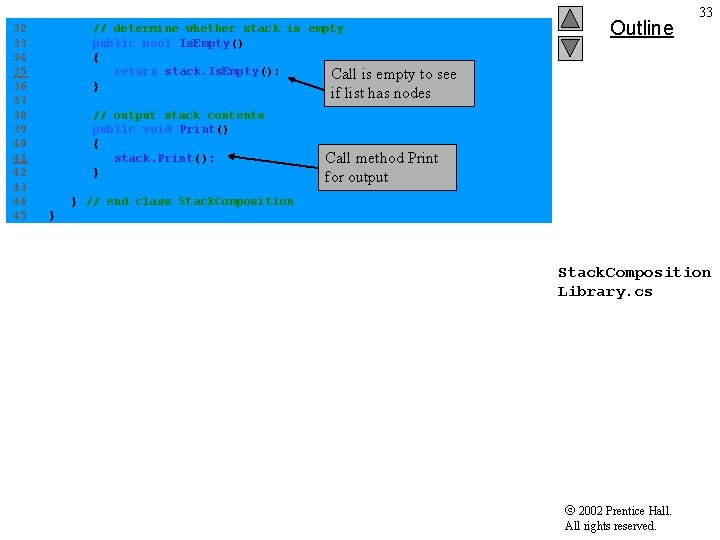
32 // determine whether stack is empty 33 public bool Is. Empty() 34 { 35 return stack. Is. Empty(); Call is empty to see 36 } if list has nodes 37 38 // output stack contents 39 public void Print() 40 { 41 stack. Print(); Call method Print 42 } for output 43 44 } // end class Stack. Composition 45 } Outline 33 Stack. Composition Library. cs 2002 Prentice Hall. All rights reserved.
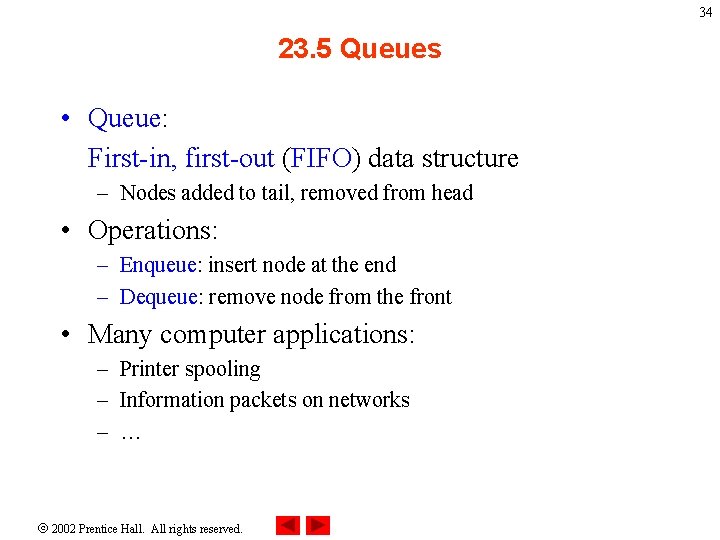
34 23. 5 Queues • Queue: First-in, first-out (FIFO) data structure – Nodes added to tail, removed from head • Operations: – Enqueue: insert node at the end – Dequeue: remove node from the front • Many computer applications: – Printer spooling – Information packets on networks – … 2002 Prentice Hall. All rights reserved.
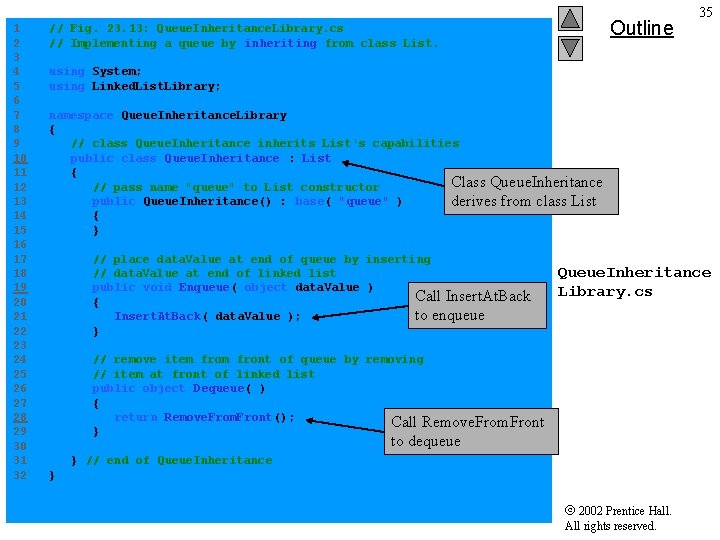
Outline 35 1 // Fig. 23. 13: Queue. Inheritance. Library. cs 2 // Implementing a queue by inheriting from class List. 3 4 using System; 5 using Linked. List. Library; 6 7 namespace Queue. Inheritance. Library 8 { 9 // class Queue. Inheritance inherits List's capabilities 10 public class Queue. Inheritance : List 11 { Class Queue. Inheritance 12 // pass name "queue" to List constructor 13 public Queue. Inheritance() : base( "queue" ) derives from class List 14 { 15 } 16 17 // place data. Value at end of queue by inserting Queue. Inheritance 18 // data. Value at end of linked list 19 public void Enqueue( object data. Value ) Call Insert. At. Back Library. cs 20 { 21 Insert. At. Back( data. Value ); to enqueue 22 } 23 24 // remove item front of queue by removing 25 // item at front of linked list 26 public object Dequeue( ) 27 { 28 return Remove. From. Front(); Call Remove. From. Front 29 } to dequeue 30 31 } // end of Queue. Inheritance 32 } 2002 Prentice Hall. All rights reserved.
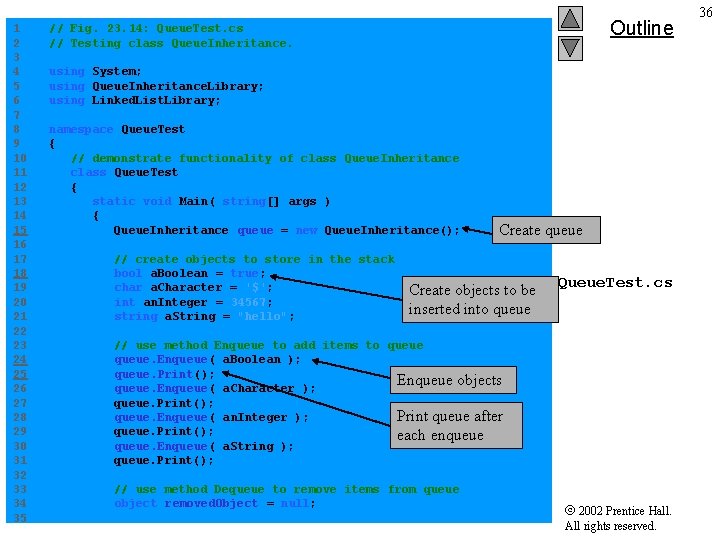
Outline 1 // Fig. 23. 14: Queue. Test. cs 2 // Testing class Queue. Inheritance. 3 4 using System; 5 using Queue. Inheritance. Library; 6 using Linked. List. Library; 7 8 namespace Queue. Test 9 { 10 // demonstrate functionality of class Queue. Inheritance 11 class Queue. Test 12 { 13 static void Main( string[] args ) 14 { 15 Queue. Inheritance queue = new Queue. Inheritance(); Create queue 16 17 // create objects to store in the stack 18 bool a. Boolean = true; Queue. Test. cs 19 char a. Character = '$'; Create objects to be 20 int an. Integer = 34567; inserted into queue 21 string a. String = "hello"; 22 23 // use method Enqueue to add items to queue 24 queue. Enqueue( a. Boolean ); 25 queue. Print(); Enqueue objects 26 queue. Enqueue( a. Character ); 27 queue. Print(); 28 queue. Enqueue( an. Integer ); Print queue after 29 queue. Print(); each enqueue 30 queue. Enqueue( a. String ); 31 queue. Print(); 32 33 // use method Dequeue to remove items from queue 34 object removed. Object = null; 2002 Prentice Hall. 35 All rights reserved. 36
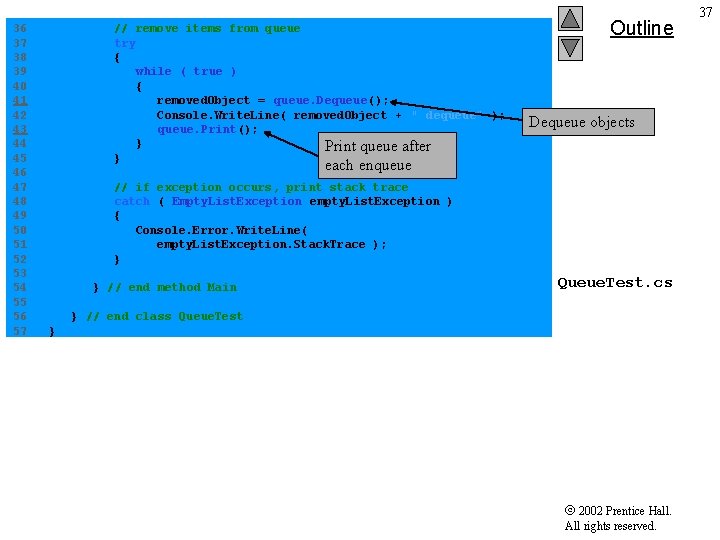
36 // remove items from queue 37 try 38 { 39 while ( true ) 40 { 41 removed. Object = queue. Dequeue(); 42 Console. Write. Line( removed. Object + " dequeue" ); 43 queue. Print(); 44 } Print queue after 45 } each enqueue 46 47 // if exception occurs, print stack trace 48 catch ( Empty. List. Exception empty. List. Exception ) 49 { 50 Console. Error. Write. Line( 51 empty. List. Exception. Stack. Trace ); 52 } 53 54 } // end method Main 55 56 } // end class Queue. Test 57 } Outline Dequeue objects Queue. Test. cs 2002 Prentice Hall. All rights reserved. 37
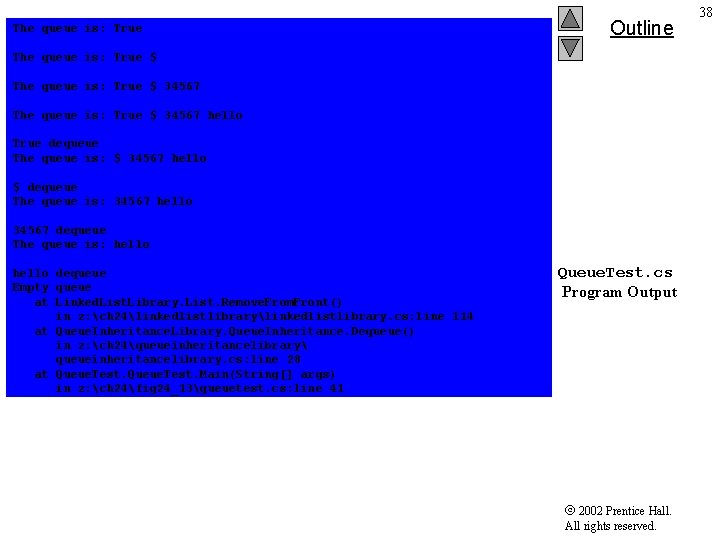
The queue is: True $ 34567 The queue is: True $ 34567 hello True dequeue The queue is: $ 34567 hello $ dequeue The queue is: 34567 hello 34567 dequeue The queue is: hello dequeue Empty queue at Linked. List. Library. List. Remove. From. Front() in z: ch 24linkedlistlibrary. cs: line 114 at Queue. Inheritance. Library. Queue. Inheritance. Dequeue() in z: ch 24queueinheritancelibrary queueinheritancelibrary. cs: line 28 at Queue. Test. Main(String[] args) in z: ch 24fig 24_13queuetest. cs: line 41 Outline Queue. Test. cs Program Output 2002 Prentice Hall. All rights reserved. 38
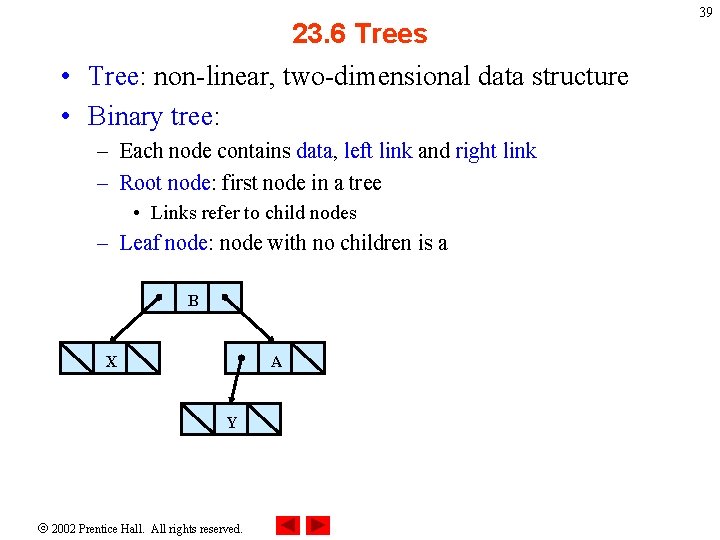
23. 6 Trees • Tree: non-linear, two-dimensional data structure • Binary tree: – Each node contains data, left link and right link – Root node: first node in a tree • Links refer to child nodes – Leaf node: node with no children is a B X A Y 2002 Prentice Hall. All rights reserved. 39
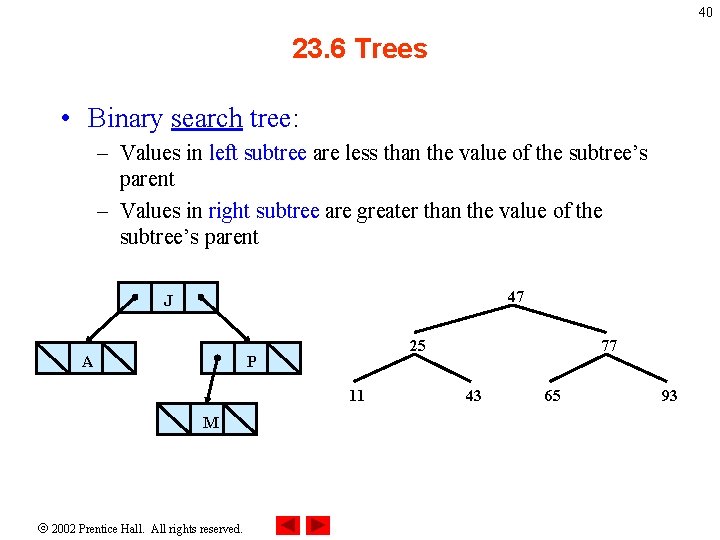
40 23. 6 Trees • Binary search tree: – Values in left subtree are less than the value of the subtree’s parent – Values in right subtree are greater than the value of the subtree’s parent 47 J A 25 P 11 M 2002 Prentice Hall. All rights reserved. 77 43 65 93
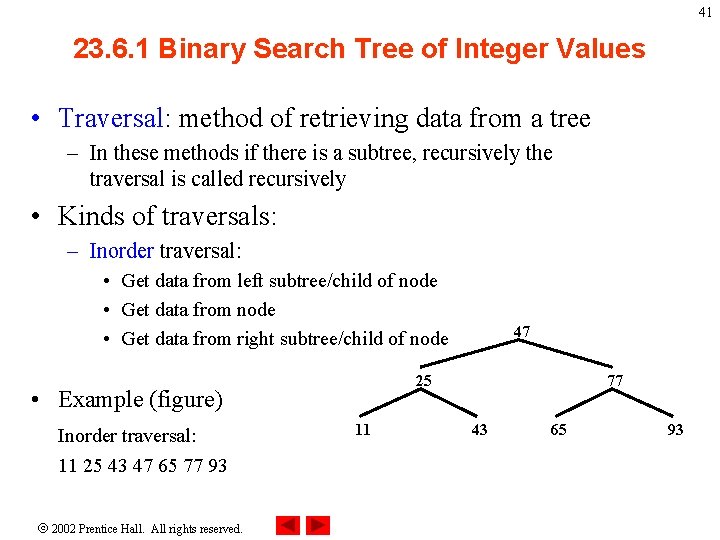
41 23. 6. 1 Binary Search Tree of Integer Values • Traversal: method of retrieving data from a tree – In these methods if there is a subtree, recursively the traversal is called recursively • Kinds of traversals: – Inorder traversal: • Get data from left subtree/child of node • Get data from right subtree/child of node 25 • Example (figure) Inorder traversal: 11 25 43 47 65 77 93 2002 Prentice Hall. All rights reserved. 47 11 77 43 65 93
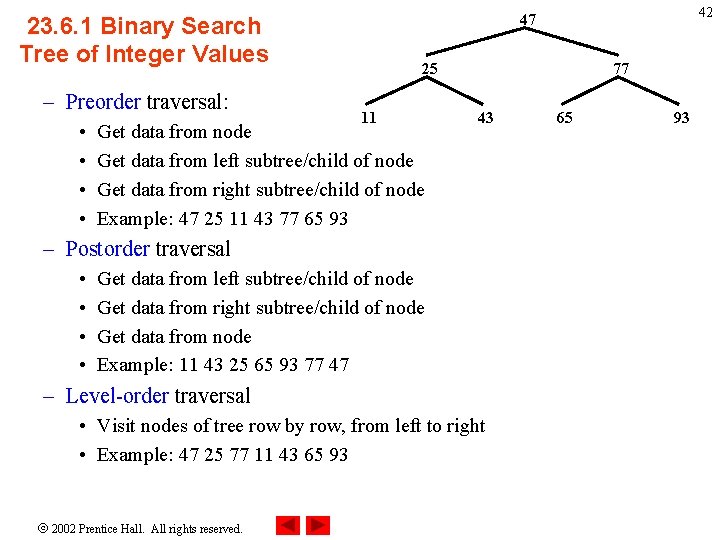
23. 6. 1 Binary Search Tree of Integer Values – Preorder traversal: • • 25 11 Get data from node Get data from left subtree/child of node Get data from right subtree/child of node Example: 47 25 11 43 77 65 93 77 43 – Postorder traversal • • Get data from left subtree/child of node Get data from right subtree/child of node Get data from node Example: 11 43 25 65 93 77 47 – Level-order traversal • Visit nodes of tree row by row, from left to right • Example: 47 25 77 11 43 65 93 2002 Prentice Hall. All rights reserved. 42 47 65 93
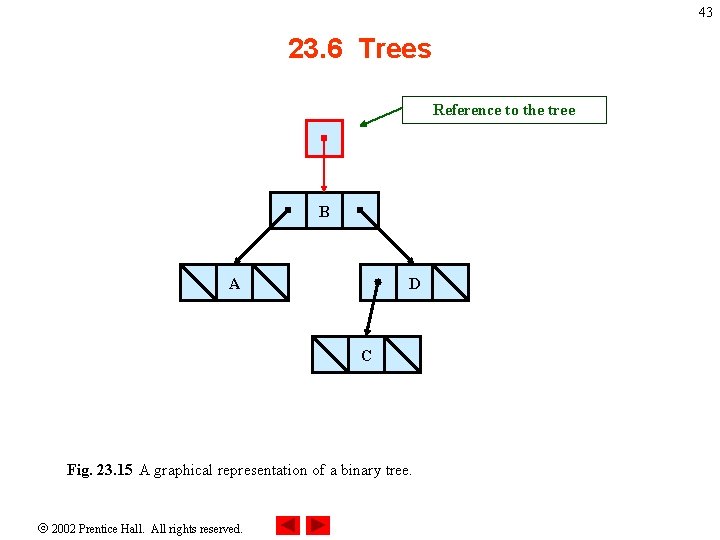
43 23. 6 Trees Reference to the tree B A D C Fig. 23. 15 A graphical representation of a binary tree. 2002 Prentice Hall. All rights reserved.
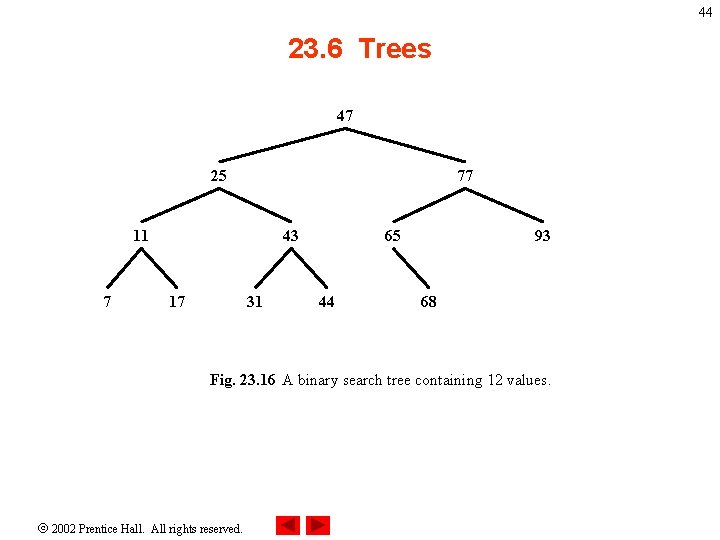
44 23. 6 Trees 47 25 77 11 7 43 17 31 65 44 93 68 Fig. 23. 16 A binary search tree containing 12 values. 2002 Prentice Hall. All rights reserved.
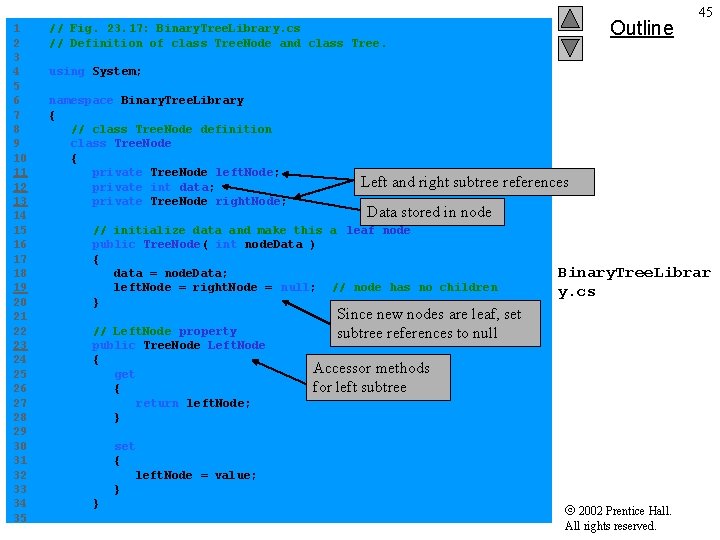
Outline 45 1 // Fig. 23. 17: Binary. Tree. Library. cs 2 // Definition of class Tree. Node and class Tree. 3 4 using System; 5 6 namespace Binary. Tree. Library 7 { 8 // class Tree. Node definition 9 class Tree. Node 10 { 11 private Tree. Node left. Node; Left and right subtree references 12 private int data; 13 private Tree. Node right. Node; Data stored in node 14 15 // initialize data and make this a leaf node 16 public Tree. Node( int node. Data ) 17 { Binary. Tree. Librar 18 data = node. Data; 19 left. Node = right. Node = null; // node has no children y. cs 20 } Since new nodes are leaf, set 21 22 // Left. Node property subtree references to null 23 public Tree. Node Left. Node 24 { Accessor methods 25 get 26 { for left subtree 27 return left. Node; 28 } 29 30 set 31 { 32 left. Node = value; 33 } 34 } 2002 Prentice Hall. 35 All rights reserved.
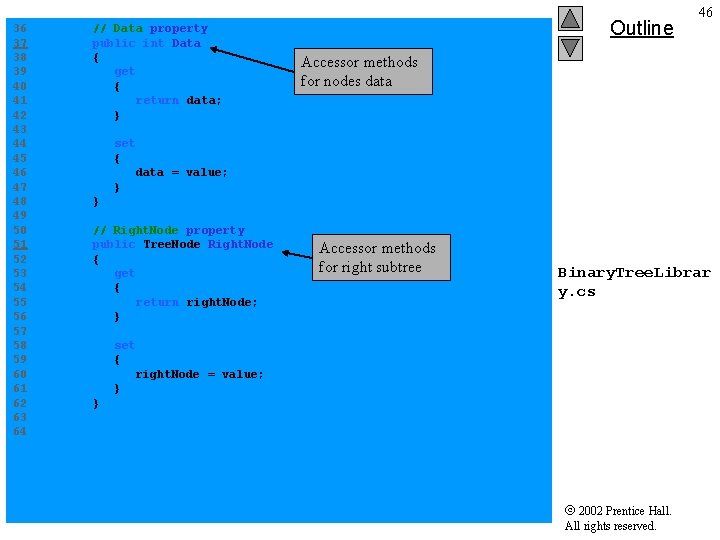
36 // Data property 37 public int Data 38 { 39 get 40 { 41 return data; 42 } 43 44 set 45 { 46 data = value; 47 } 48 } 49 50 // Right. Node property 51 public Tree. Node Right. Node 52 { 53 get 54 { 55 return right. Node; 56 } 57 58 set 59 { 60 right. Node = value; 61 } 62 } 63 64 Outline 46 Accessor methods for nodes data Accessor methods for right subtree Binary. Tree. Librar y. cs 2002 Prentice Hall. All rights reserved.
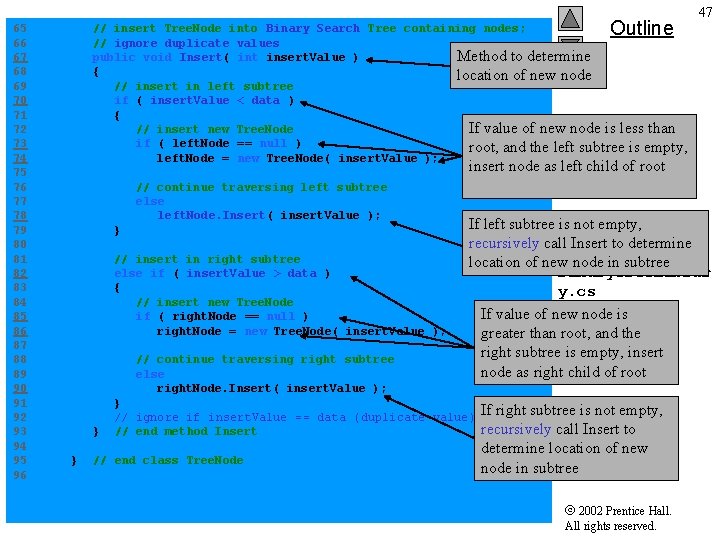
Outline 47 65 // insert Tree. Node into Binary Search Tree containing nodes; 66 // ignore duplicate values 67 public void Insert( int insert. Value ) Method to determine 68 { location of new node 69 // insert in left subtree 70 if ( insert. Value < data ) 71 { 72 // insert new Tree. Node If value of new node is less than 73 if ( left. Node == null ) root, and the left subtree is empty, 74 left. Node = new Tree. Node( insert. Value ); insert node as left child of root 75 76 // continue traversing left subtree 77 else 78 left. Node. Insert( insert. Value ); If left subtree is not empty, 79 } 80 recursively call Insert to determine 81 // insert in right subtree location of new node in subtree Binary. Tree. Librar 82 else if ( insert. Value > data ) 83 { y. cs 84 // insert new Tree. Node If value of new node is 85 if ( right. Node == null ) 86 right. Node = new Tree. Node( insert. Value ); greater than root, and the 87 right subtree is empty, insert 88 // continue traversing right subtree node as right child of root 89 else 90 right. Node. Insert( insert. Value ); 91 } 92 // ignore if insert. Value == data (duplicate value) If right subtree is not empty, recursively call Insert to 93 } // end method Insert 94 determine location of new 95 } // end class Tree. Node node in subtree 96 2002 Prentice Hall. All rights reserved.
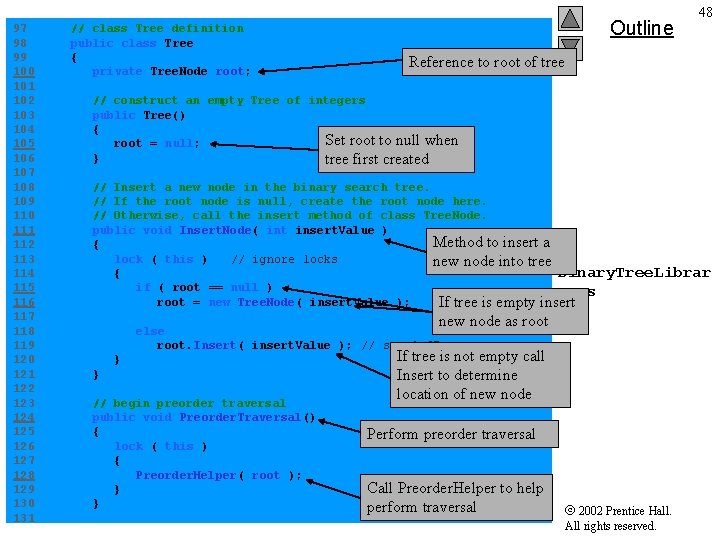
Outline 48 97 // class Tree definition 98 public class Tree 99 { Reference to root of tree 100 private Tree. Node root; 101 102 // construct an empty Tree of integers 103 public Tree() 104 { Set root to null when 105 root = null; 106 } tree first created 107 108 // Insert a new node in the binary search tree. 109 // If the root node is null, create the root node here. 110 // Otherwise, call the insert method of class Tree. Node. 111 public void Insert. Node( int insert. Value ) Method to insert a 112 { 113 lock ( this ) // ignore locks new node into tree Binary. Tree. Librar 114 { 115 if ( root == null ) y. cs 116 root = new Tree. Node( insert. Value ); If tree is empty insert 117 new node as root 118 else 119 root. Insert( insert. Value ); // see l. 67 If tree is not empty call 120 } 121 } Insert to determine 122 location of new node 123 // begin preorder traversal 124 public void Preorder. Traversal() 125 { Perform preorder traversal 126 lock ( this ) 127 { 128 Preorder. Helper( root ); 129 } Call Preorder. Helper to help 130 } perform traversal 2002 Prentice Hall. 131 All rights reserved.
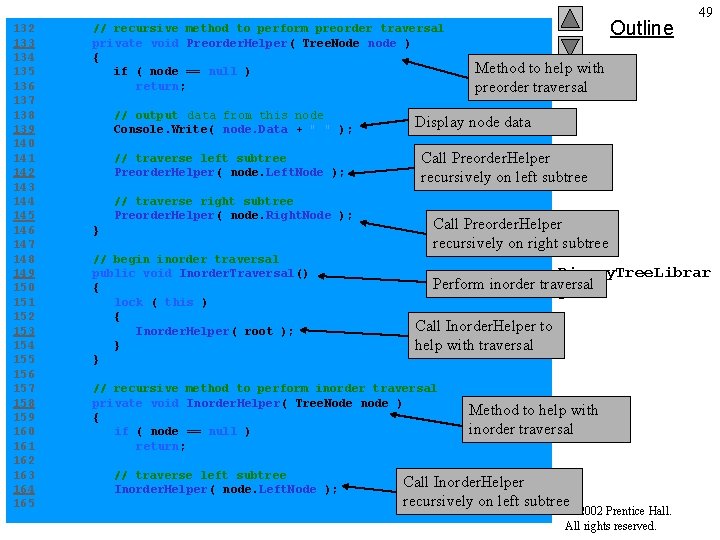
Outline 49 132 // recursive method to perform preorder traversal 133 private void Preorder. Helper( Tree. Node node ) 134 { Method to help with 135 if ( node == null ) 136 return; preorder traversal 137 138 // output data from this node Display node data 139 Console. Write( node. Data + " " ); 140 141 // traverse left subtree Call Preorder. Helper 142 Preorder. Helper( node. Left. Node ); recursively on left subtree 143 144 // traverse right subtree 145 Preorder. Helper( node. Right. Node ); Call Preorder. Helper 146 } 147 recursively on right subtree 148 // begin inorder traversal Binary. Tree. Librar 149 public void Inorder. Traversal() Perform inorder traversal 150 { y. cs 151 lock ( this ) 152 { Call Inorder. Helper to 153 Inorder. Helper( root ); 154 } help with traversal 155 } 156 157 // recursive method to perform inorder traversal 158 private void Inorder. Helper( Tree. Node node ) Method to help with 159 { inorder traversal 160 if ( node == null ) 161 return; 162 163 // traverse left subtree Call Inorder. Helper 164 Inorder. Helper( node. Left. Node ); recursively on left subtree 165 2002 Prentice Hall. All rights reserved.
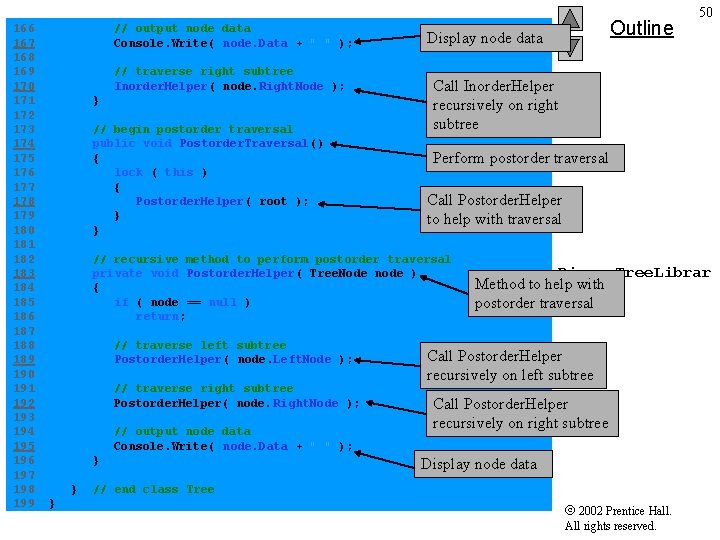
Outline 50 166 // output node data Display node data 167 Console. Write( node. Data + " " ); 168 169 // traverse right subtree 170 Inorder. Helper( node. Right. Node ); Call Inorder. Helper 171 } recursively on right 172 subtree 173 // begin postorder traversal 174 public void Postorder. Traversal() 175 { Perform postorder traversal 176 lock ( this ) 177 { 178 Postorder. Helper( root ); Call Postorder. Helper 179 } to help with traversal 180 } 181 182 // recursive method to perform postorder traversal Binary. Tree. Librar 183 private void Postorder. Helper( Tree. Node node ) Method to help with 184 { y. cs 185 if ( node == null ) postorder traversal 186 return; 187 188 // traverse left subtree Call Postorder. Helper 189 Postorder. Helper( node. Left. Node ); 190 recursively on left subtree 191 // traverse right subtree 192 Postorder. Helper( node. Right. Node ); Call Postorder. Helper 193 recursively on right subtree 194 // output node data 195 Console. Write( node. Data + " " ); 196 } Display node data 197 198 } // end class Tree 199 } 2002 Prentice Hall. All rights reserved.
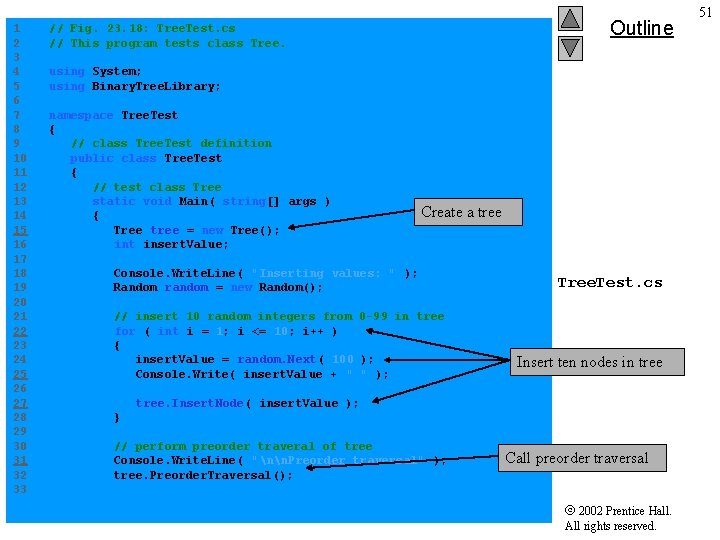
Outline 1 // Fig. 23. 18: Tree. Test. cs 2 // This program tests class Tree. 3 4 using System; 5 using Binary. Tree. Library; 6 7 namespace Tree. Test 8 { 9 // class Tree. Test definition 10 public class Tree. Test 11 { 12 // test class Tree 13 static void Main( string[] args ) Create a tree 14 { 15 Tree tree = new Tree(); 16 int insert. Value; 17 18 Console. Write. Line( "Inserting values: " ); Tree. Test. cs 19 Random random = new Random(); 20 21 // insert 10 random integers from 0 -99 in tree 22 for ( int i = 1; i <= 10; i++ ) 23 { 24 insert. Value = random. Next( 100 ); Insert ten nodes in tree 25 Console. Write( insert. Value + " " ); 26 27 tree. Insert. Node( insert. Value ); 28 } 29 30 // perform preorder traveral of tree Call preorder traversal 31 Console. Write. Line( "nn. Preorder traversal" ); 32 tree. Preorder. Traversal(); 33 2002 Prentice Hall. All rights reserved. 51
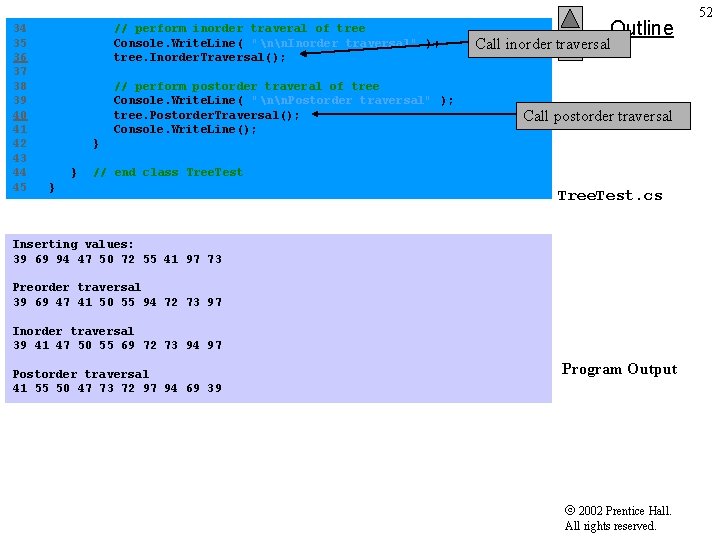
34 // perform inorder traveral of tree 35 Console. Write. Line( "nn. Inorder traversal" ); 36 tree. Inorder. Traversal(); 37 38 // perform postorder traveral of tree 39 Console. Write. Line( "nn. Postorder traversal" ); 40 tree. Postorder. Traversal(); 41 Console. Write. Line(); 42 } 43 44 } // end class Tree. Test 45 } Inserting values: 39 69 94 47 50 72 55 41 97 73 Preorder traversal 39 69 47 41 50 55 94 72 73 97 Inorder traversal 39 41 47 50 55 69 72 73 94 97 Postorder traversal 41 55 50 47 73 72 97 94 69 39 Outline Call inorder traversal Call postorder traversal Tree. Test. cs Program Output 2002 Prentice Hall. All rights reserved. 52
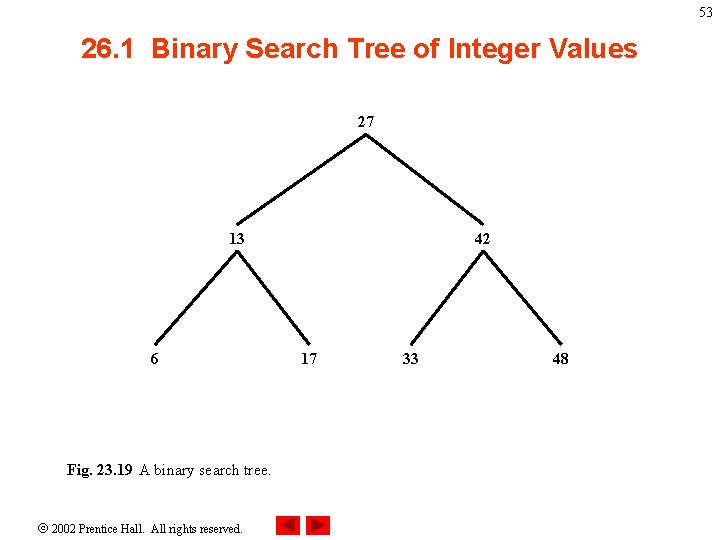
53 26. 1 Binary Search Tree of Integer Values 27 13 6 Fig. 23. 19 A binary search tree. 2002 Prentice Hall. All rights reserved. 42 17 33 48
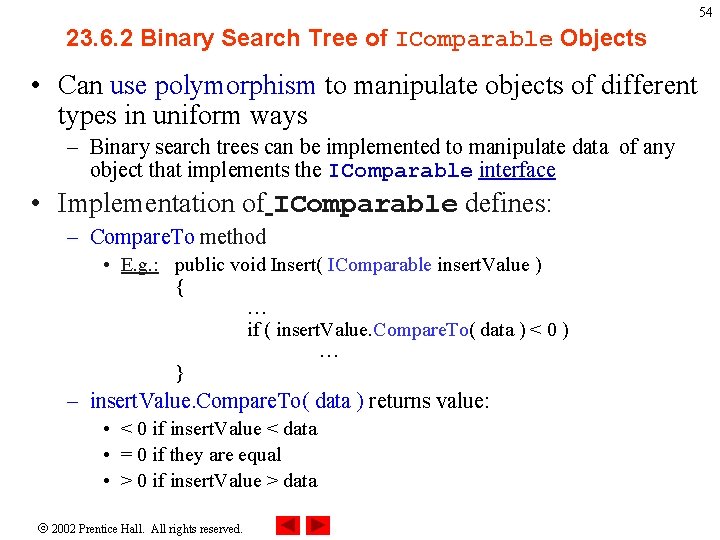
54 23. 6. 2 Binary Search Tree of IComparable Objects • Can use polymorphism to manipulate objects of different types in uniform ways – Binary search trees can be implemented to manipulate data of any object that implements the IComparable interface • Implementation of IComparable defines: – Compare. To method • E. g. : public void Insert( IComparable insert. Value ) { … if ( insert. Value. Compare. To( data ) < 0 ) … } – insert. Value. Compare. To( data ) returns value: • < 0 if insert. Value < data • = 0 if they are equal • > 0 if insert. Value > data 2002 Prentice Hall. All rights reserved.
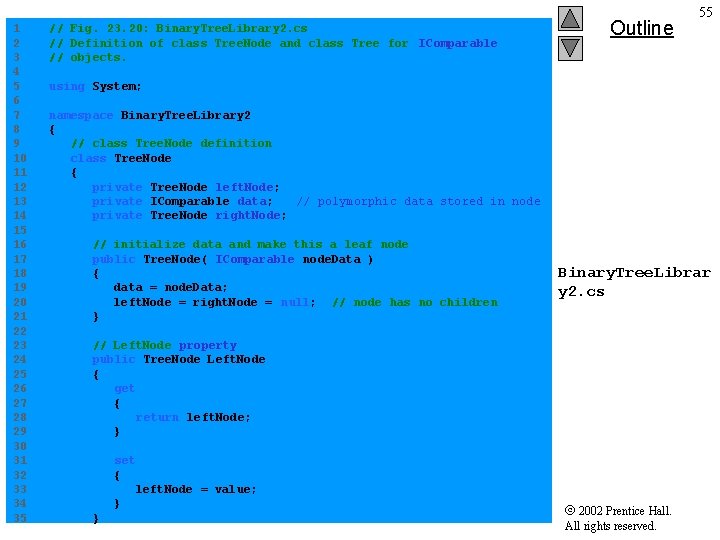
1 // Fig. 23. 20: Binary. Tree. Library 2. cs 2 // Definition of class Tree. Node and class Tree for IComparable 3 // objects. 4 5 using System; 6 7 namespace Binary. Tree. Library 2 8 { 9 // class Tree. Node definition 10 class Tree. Node 11 { 12 private Tree. Node left. Node; 13 private IComparable data; // polymorphic data stored in node 14 private Tree. Node right. Node; 15 16 // initialize data and make this a leaf node 17 public Tree. Node( IComparable node. Data ) 18 { 19 data = node. Data; 20 left. Node = right. Node = null; // node has no children 21 } 22 23 // Left. Node property 24 public Tree. Node Left. Node 25 { 26 get 27 { 28 return left. Node; 29 } 30 31 set 32 { 33 left. Node = value; 34 } 35 } Outline 55 Binary. Tree. Librar y 2. cs 2002 Prentice Hall. All rights reserved.
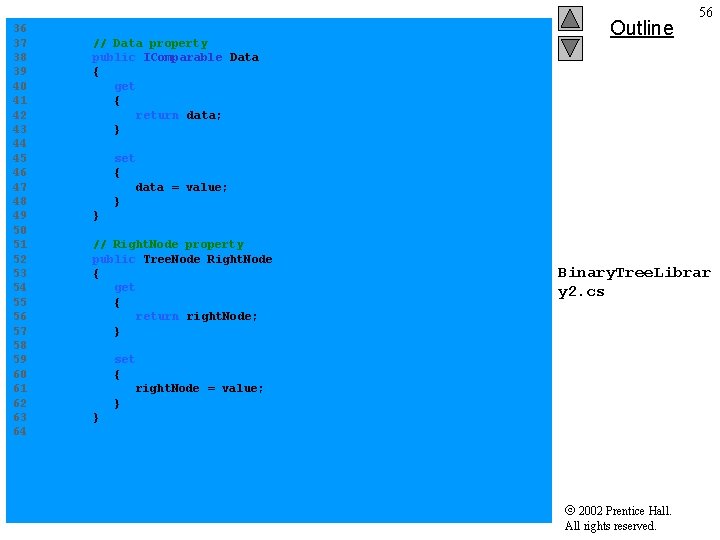
36 37 // Data property 38 public IComparable Data 39 { 40 get 41 { 42 return data; 43 } 44 45 set 46 { 47 data = value; 48 } 49 } 50 51 // Right. Node property 52 public Tree. Node Right. Node 53 { 54 get 55 { 56 return right. Node; 57 } 58 59 set 60 { 61 right. Node = value; 62 } 63 } 64 Outline 56 Binary. Tree. Librar y 2. cs 2002 Prentice Hall. All rights reserved.
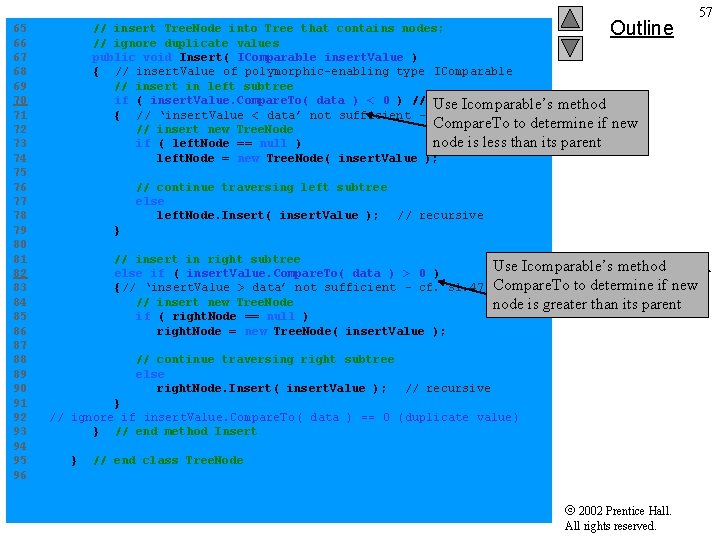
Outline 57 65 // insert Tree. Node into Tree that contains nodes; 66 // ignore duplicate values 67 public void Insert( IComparable insert. Value ) 68 { // insert. Value of polymorphic-enabling type IComparable 69 // insert in left subtree 70 if ( insert. Value. Compare. To( data ) < 0 ) // need Use Icomparable’s method 71 { // ‘insert. Value < data’ not sufficient – cf. sl. 47 l. 70) Compare. To to determine if new 72 // insert new Tree. Node 73 if ( left. Node == null ) node is less than its parent 74 left. Node = new Tree. Node( insert. Value ); 75 76 // continue traversing left subtree 77 else 78 left. Node. Insert( insert. Value ); // recursive 79 } 80 81 // insert in right subtree Use Icomparable’s method Binary. Tree. Librar 82 else if ( insert. Value. Compare. To( data ) > 0 ) Compare. To to determine if new 83 {// ‘insert. Value > data’ not sufficient – cf. sl. 47 l. 82 y 2. cs 84 // insert new Tree. Node node is greater than its parent 85 if ( right. Node == null ) 86 right. Node = new Tree. Node( insert. Value ); 87 88 // continue traversing right subtree 89 else 90 right. Node. Insert( insert. Value ); // recursive 91 } 92 // ignore if insert. Value. Compare. To( data ) == 0 (duplicate value) 93 } // end method Insert 94 95 } // end class Tree. Node 96 2002 Prentice Hall. All rights reserved.
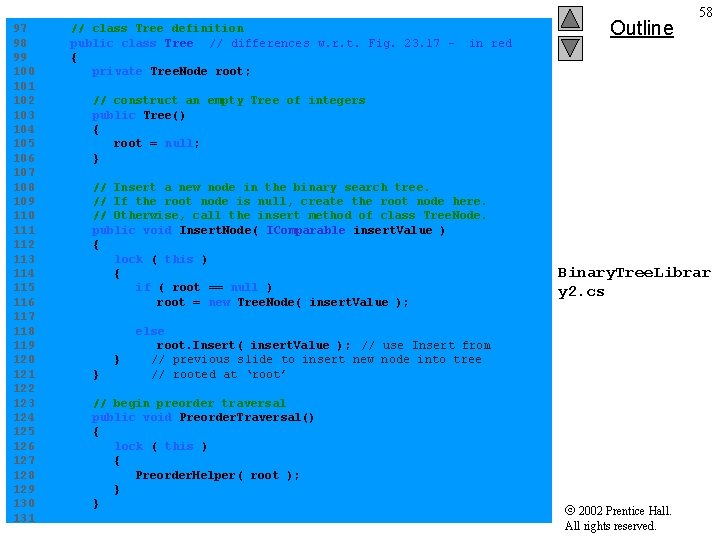
97 // class Tree definition 98 public class Tree // differences w. r. t. Fig. 23. 17 - in red 99 { 100 private Tree. Node root; 101 102 // construct an empty Tree of integers 103 public Tree() 104 { 105 root = null; 106 } 107 108 // Insert a new node in the binary search tree. 109 // If the root node is null, create the root node here. 110 // Otherwise, call the insert method of class Tree. Node. 111 public void Insert. Node( IComparable insert. Value ) 112 { 113 lock ( this ) 114 { 115 if ( root == null ) 116 root = new Tree. Node( insert. Value ); 117 118 else 119 root. Insert( insert. Value ); // use Insert from 120 } // previous slide to insert new node into tree 121 } // rooted at ‘root’ 122 123 // begin preorder traversal 124 public void Preorder. Traversal() 125 { 126 lock ( this ) 127 { 128 Preorder. Helper( root ); 129 } 130 } 131 Outline 58 Binary. Tree. Librar y 2. cs 2002 Prentice Hall. All rights reserved.
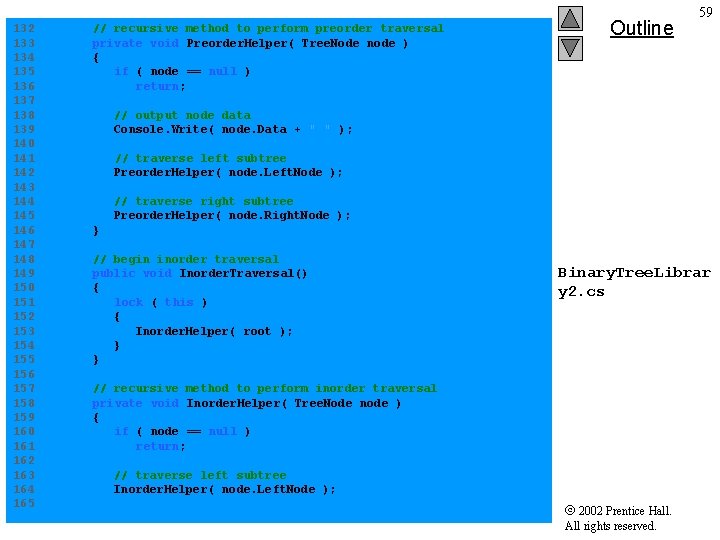
132 // recursive method to perform preorder traversal 133 private void Preorder. Helper( Tree. Node node ) 134 { 135 if ( node == null ) 136 return; 137 138 // output node data 139 Console. Write( node. Data + " " ); 140 141 // traverse left subtree 142 Preorder. Helper( node. Left. Node ); 143 144 // traverse right subtree 145 Preorder. Helper( node. Right. Node ); 146 } 147 148 // begin inorder traversal 149 public void Inorder. Traversal() 150 { 151 lock ( this ) 152 { 153 Inorder. Helper( root ); 154 } 155 } 156 157 // recursive method to perform inorder traversal 158 private void Inorder. Helper( Tree. Node node ) 159 { 160 if ( node == null ) 161 return; 162 163 // traverse left subtree 164 Inorder. Helper( node. Left. Node ); 165 Outline 59 Binary. Tree. Librar y 2. cs 2002 Prentice Hall. All rights reserved.
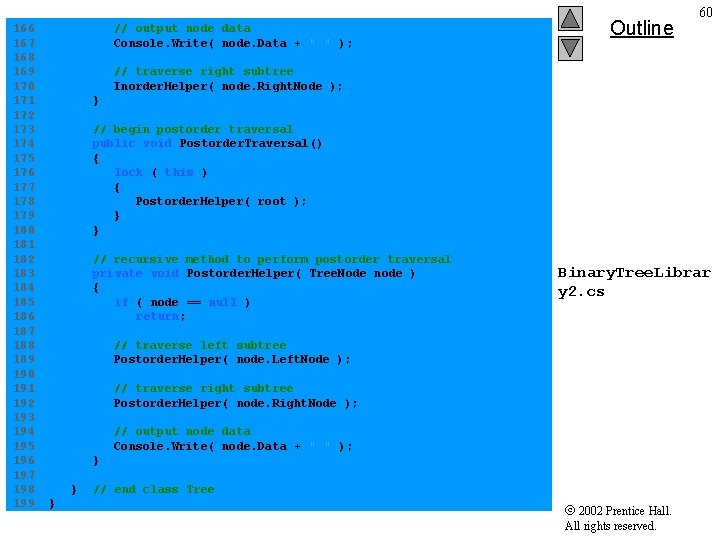
166 // output node data 167 Console. Write( node. Data + " " ); 168 169 // traverse right subtree 170 Inorder. Helper( node. Right. Node ); 171 } 172 173 // begin postorder traversal 174 public void Postorder. Traversal() 175 { 176 lock ( this ) 177 { 178 Postorder. Helper( root ); 179 } 180 } 181 182 // recursive method to perform postorder traversal 183 private void Postorder. Helper( Tree. Node node ) 184 { 185 if ( node == null ) 186 return; 187 188 // traverse left subtree 189 Postorder. Helper( node. Left. Node ); 190 191 // traverse right subtree 192 Postorder. Helper( node. Right. Node ); 193 194 // output node data 195 Console. Write( node. Data + " " ); 196 } 197 198 } // end class Tree 199 } Outline 60 Binary. Tree. Librar y 2. cs 2002 Prentice Hall. All rights reserved.
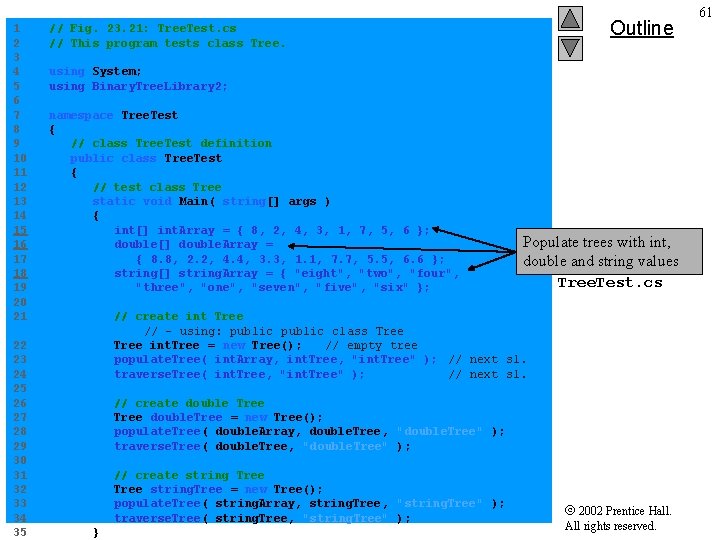
Outline 1 // Fig. 23. 21: Tree. Test. cs 2 // This program tests class Tree. 3 4 using System; 5 using Binary. Tree. Library 2; 6 7 namespace Tree. Test 8 { 9 // class Tree. Test definition 10 public class Tree. Test 11 { 12 // test class Tree 13 static void Main( string[] args ) 14 { 15 int[] int. Array = { 8, 2, 4, 3, 1, 7, 5, 6 }; Populate trees with int, 16 double[] double. Array = 17 { 8. 8, 2. 2, 4. 4, 3. 3, 1. 1, 7. 7, 5. 5, 6. 6 }; double and string values 18 string[] string. Array = { "eight", "two", "four", Tree. Test. cs 19 "three", "one", "seven", "five", "six" }; 20 21 // create int Tree // - using: public class Tree 22 Tree int. Tree = new Tree(); // empty tree 23 populate. Tree( int. Array, int. Tree, "int. Tree" ); // next sl. 24 traverse. Tree( int. Tree, "int. Tree" ); // next sl. 25 26 // create double Tree 27 Tree double. Tree = new Tree(); 28 populate. Tree( double. Array, double. Tree, "double. Tree" ); 29 traverse. Tree( double. Tree, "double. Tree" ); 30 31 // create string Tree 32 Tree string. Tree = new Tree(); 33 populate. Tree( string. Array, string. Tree, "string. Tree" ); 2002 Prentice Hall. 34 traverse. Tree( string. Tree, "string. Tree" ); All rights reserved. 35 } 61
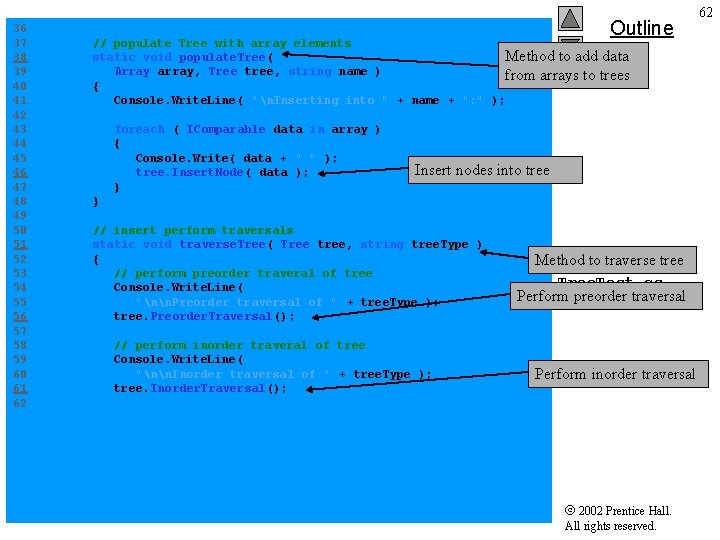
Outline 36 37 // populate Tree with array elements 38 static void populate. Tree( Method to add data 39 Array array, Tree tree, string name ) from arrays to trees 40 { 41 Console. Write. Line( "n. Inserting into " + name + ": " ); 42 43 foreach ( IComparable data in array ) 44 { 45 Console. Write( data + " " ); Insert nodes into tree 46 tree. Insert. Node( data ); 47 } 48 } 49 50 // insert perform traversals 51 static void traverse. Tree( Tree tree, string tree. Type ) 52 { Method to traverse tree 53 // perform preorder traveral of tree Tree. Test. cs 54 Console. Write. Line( Perform preorder traversal 55 "nn. Preorder traversal of " + tree. Type ); 56 tree. Preorder. Traversal(); 57 58 // perform inorder traveral of tree 59 Console. Write. Line( 60 "nn. Inorder traversal of " + tree. Type ); Perform inorder traversal 61 tree. Inorder. Traversal(); 62 2002 Prentice Hall. All rights reserved. 62
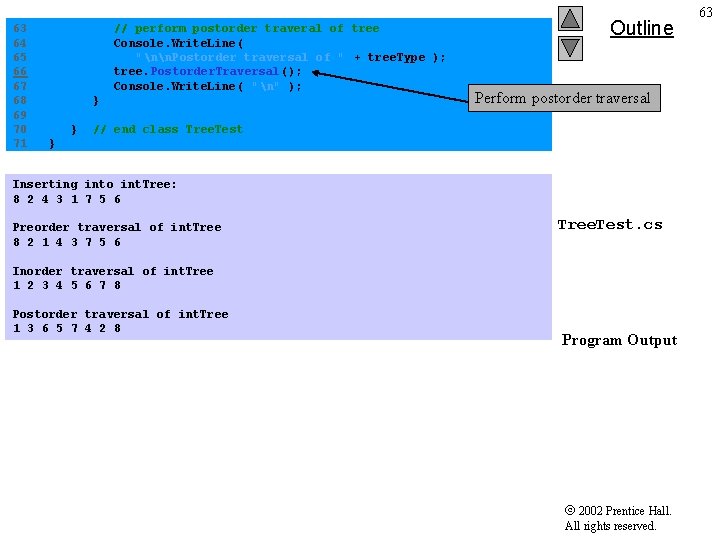
63 // perform postorder traveral of tree 64 Console. Write. Line( 65 "nn. Postorder traversal of " + tree. Type ); 66 tree. Postorder. Traversal(); 67 Console. Write. Line( "n" ); 68 } 69 70 } // end class Tree. Test 71 } Outline Perform postorder traversal Inserting into int. Tree: 8 2 4 3 1 7 5 6 Preorder traversal of int. Tree 8 2 1 4 3 7 5 6 Tree. Test. cs Inorder traversal of int. Tree 1 2 3 4 5 6 7 8 Postorder traversal of int. Tree 1 3 6 5 7 4 2 8 Program Output 2002 Prentice Hall. All rights reserved. 63
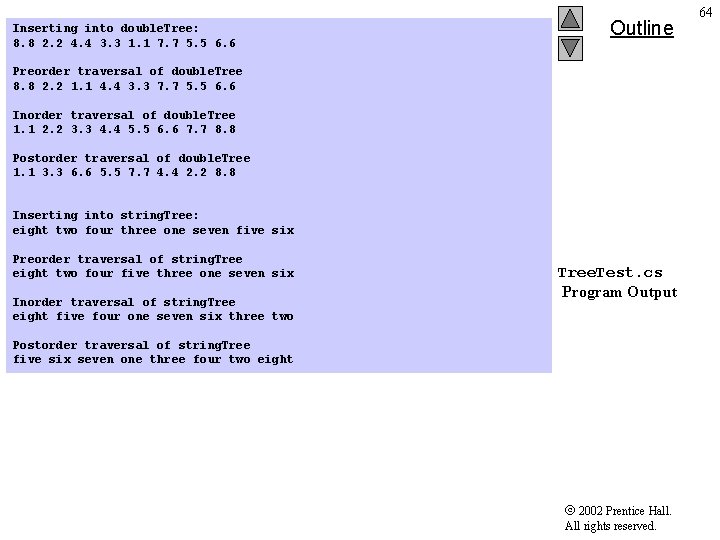
Inserting into double. Tree: 8. 8 2. 2 4. 4 3. 3 1. 1 7. 7 5. 5 6. 6 Preorder traversal of double. Tree 8. 8 2. 2 1. 1 4. 4 3. 3 7. 7 5. 5 6. 6 Inorder traversal of double. Tree 1. 1 2. 2 3. 3 4. 4 5. 5 6. 6 7. 7 8. 8 Postorder traversal of double. Tree 1. 1 3. 3 6. 6 5. 5 7. 7 4. 4 2. 2 8. 8 Inserting into string. Tree: eight two four three one seven five six Preorder traversal of string. Tree eight two four five three one seven six Inorder traversal of string. Tree eight five four one seven six three two Postorder traversal of string. Tree five six seven one three four two eight Outline Tree. Test. cs Program Output 2002 Prentice Hall. All rights reserved. 64
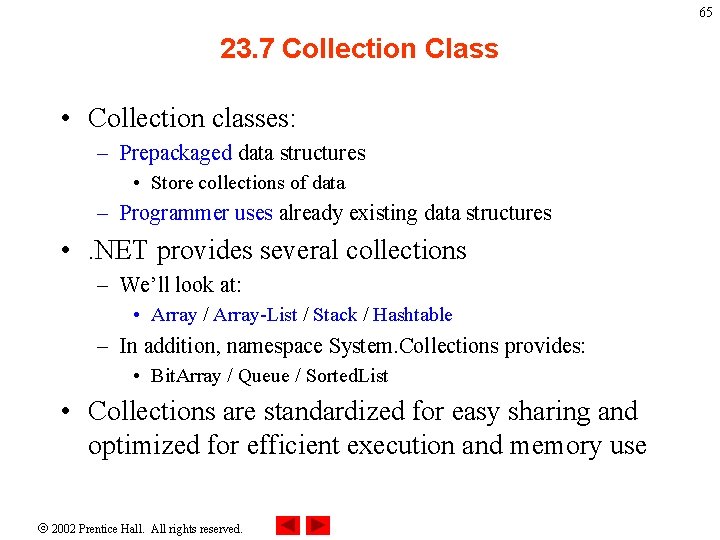
65 23. 7 Collection Class • Collection classes: – Prepackaged data structures • Store collections of data – Programmer uses already existing data structures • . NET provides several collections – We’ll look at: • Array / Array-List / Stack / Hashtable – In addition, namespace System. Collections provides: • Bit. Array / Queue / Sorted. List • Collections are standardized for easy sharing and optimized for efficient execution and memory use 2002 Prentice Hall. All rights reserved.
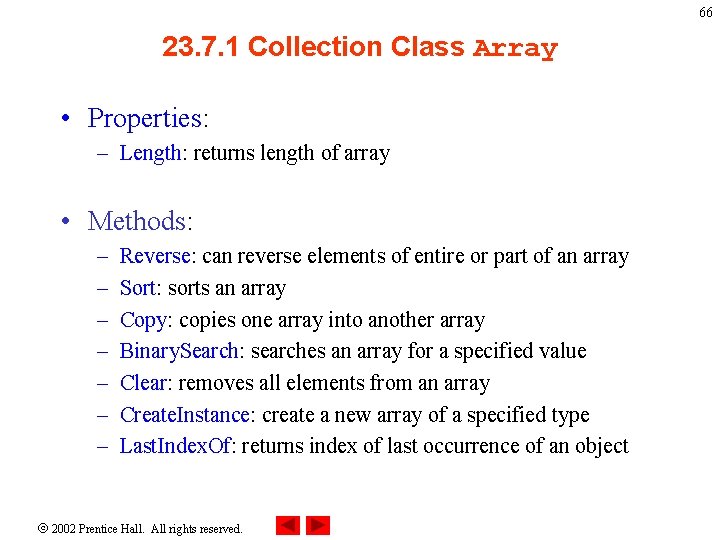
66 23. 7. 1 Collection Class Array • Properties: – Length: returns length of array • Methods: – – – – Reverse: can reverse elements of entire or part of an array Sort: sorts an array Copy: copies one array into another array Binary. Search: searches an array for a specified value Clear: removes all elements from an array Create. Instance: create a new array of a specified type Last. Index. Of: returns index of last occurrence of an object 2002 Prentice Hall. All rights reserved.
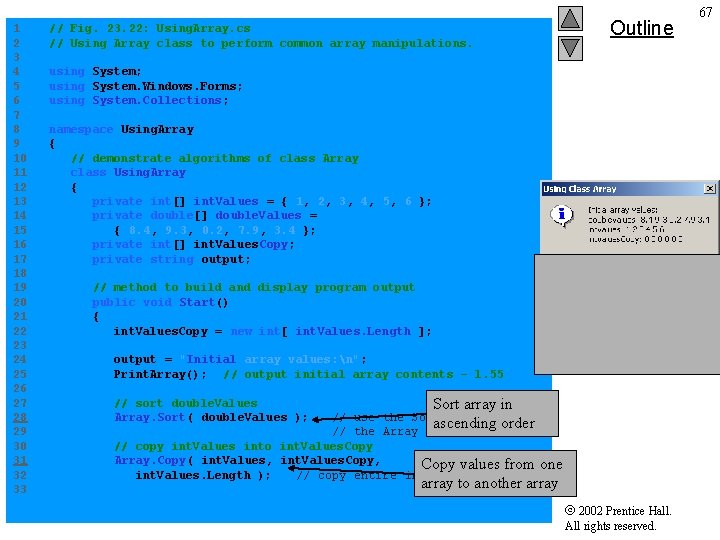
Outline 1 // Fig. 23. 22: Using. Array. cs 2 // Using Array class to perform common array manipulations. 3 4 using System; 5 using System. Windows. Forms; 6 using System. Collections; 7 8 namespace Using. Array 9 { 10 // demonstrate algorithms of class Array 11 class Using. Array 12 { 13 private int[] int. Values = { 1, 2, 3, 4, 5, 6 }; 14 private double[] double. Values = 15 { 8. 4, 9. 3, 0. 2, 7. 9, 3. 4 }; 16 private int[] int. Values. Copy; 17 private string output; 18 Using. Array. cs 19 // method to build and display program output 20 public void Start() 21 { 22 int. Values. Copy = new int[ int. Values. Length ]; 23 24 output = "Initial array values: n"; 25 Print. Array(); // output initial array contents – l. 55 26 27 // sort double. Values Sort array in 28 Array. Sort( double. Values ); // use the Sort method for ascending order 29 // the Array Collection Class 30 // copy int. Values into int. Values. Copy 31 Array. Copy( int. Values, int. Values. Copy, Copy values from one 32 int. Values. Length ); // copy entire int. Values array to another array 33 2002 Prentice Hall. All rights reserved. 67
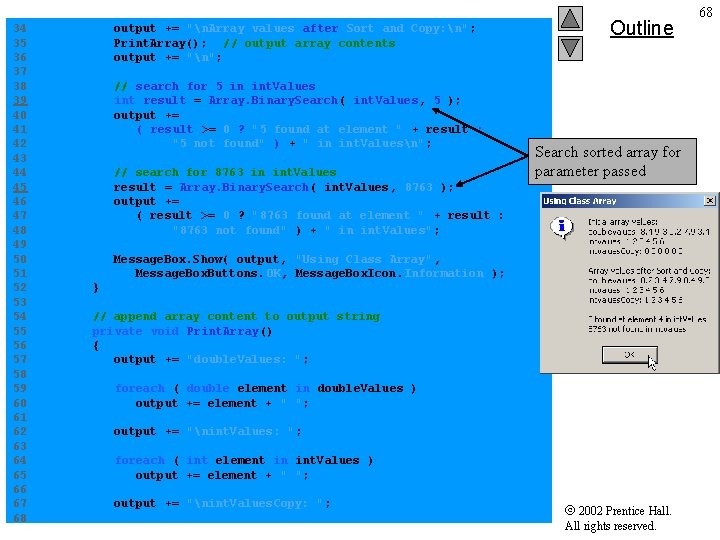
34 output += "n. Array values after Sort and Copy: n"; 35 Print. Array(); // output array contents 36 output += "n"; 37 38 // search for 5 in int. Values 39 int result = Array. Binary. Search( int. Values, 5 ); 40 output += 41 ( result >= 0 ? "5 found at element " + result : 42 "5 not found" ) + " in int. Valuesn"; 43 44 // search for 8763 in int. Values 45 result = Array. Binary. Search( int. Values, 8763 ); 46 output += 47 ( result >= 0 ? "8763 found at element " + result : 48 "8763 not found" ) + " in int. Values"; 49 50 Message. Box. Show( output, "Using Class Array", 51 Message. Box. Buttons. OK, Message. Box. Icon. Information ); 52 } 53 54 // append array content to output string 55 private void Print. Array() 56 { 57 output += "double. Values: "; 58 59 foreach ( double element in double. Values ) 60 output += element + " "; 61 62 output += "nint. Values: "; 63 64 foreach ( int element in int. Values ) 65 output += element + " "; 66 67 output += "nint. Values. Copy: "; 68 Outline Search sorted array for parameter passed Using. Array. cs 2002 Prentice Hall. All rights reserved. 68
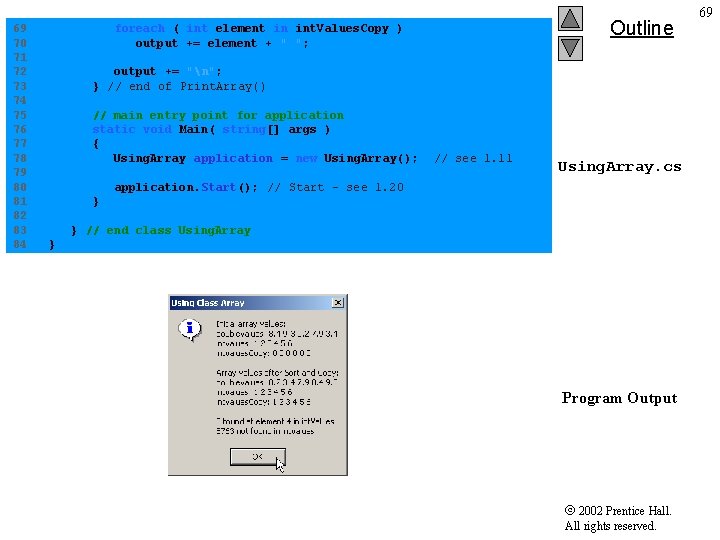
69 foreach ( int element in int. Values. Copy ) 70 output += element + " "; 71 72 output += "n"; 73 } // end of Print. Array() 74 75 // main entry point for application 76 static void Main( string[] args ) 77 { 78 Using. Array application = new Using. Array(); // see l. 11 79 80 application. Start(); // Start - see l. 20 81 } 82 83 } // end class Using. Array 84 } Outline Using. Array. cs Program Output 2002 Prentice Hall. All rights reserved. 69
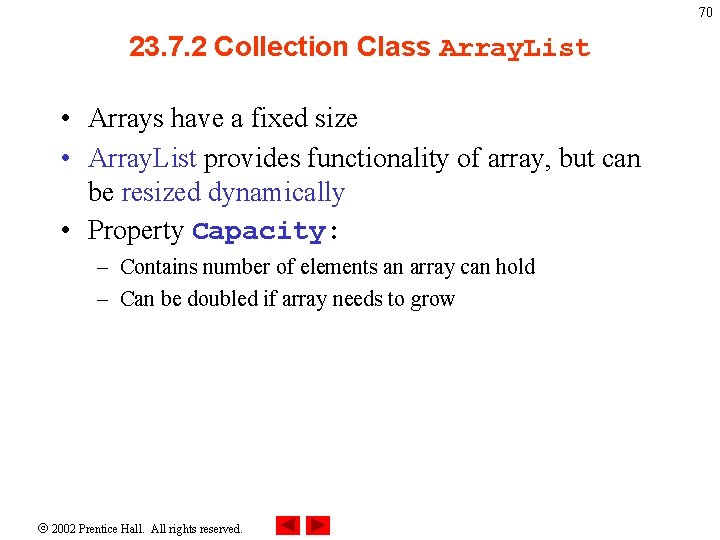
70 23. 7. 2 Collection Class Array. List • Arrays have a fixed size • Array. List provides functionality of array, but can be resized dynamically • Property Capacity: – Contains number of elements an array can hold – Can be doubled if array needs to grow 2002 Prentice Hall. All rights reserved.
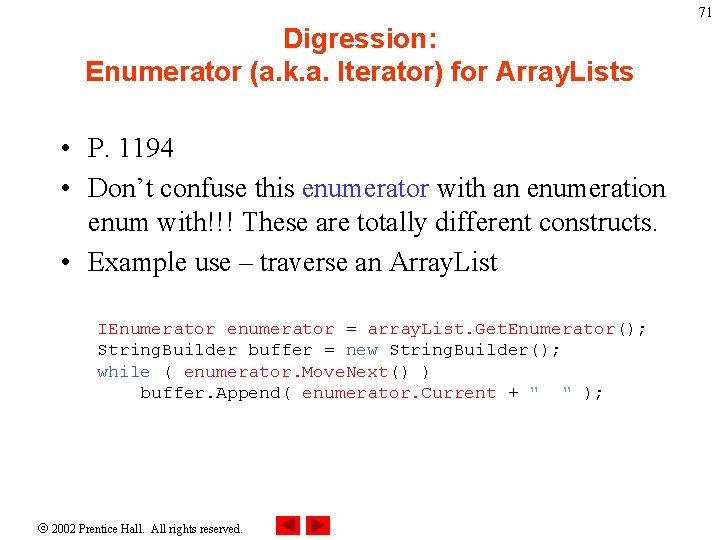
71 Digression: Enumerator (a. k. a. Iterator) for Array. Lists • P. 1194 • Don’t confuse this enumerator with an enumeration enum with!!! These are totally different constructs. • Example use – traverse an Array. List IEnumerator enumerator = array. List. Get. Enumerator(); String. Builder buffer = new String. Builder(); while ( enumerator. Move. Next() ) buffer. Append( enumerator. Current + " " ); 2002 Prentice Hall. All rights reserved.
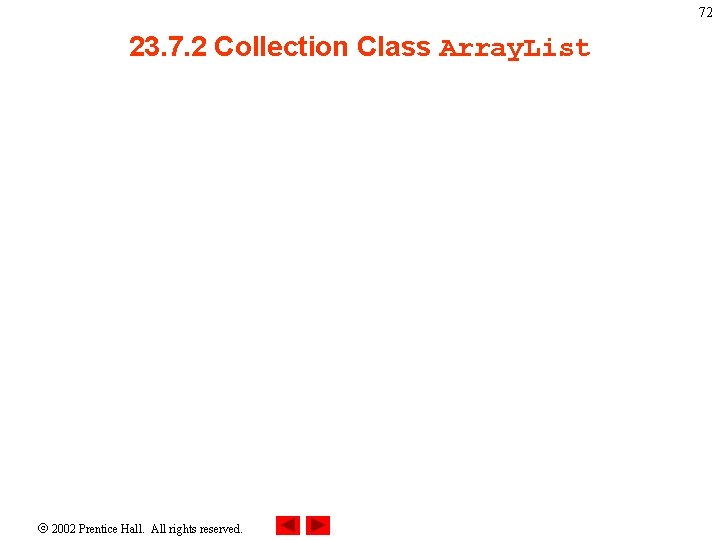
72 23. 7. 2 Collection Class Array. List 2002 Prentice Hall. All rights reserved.
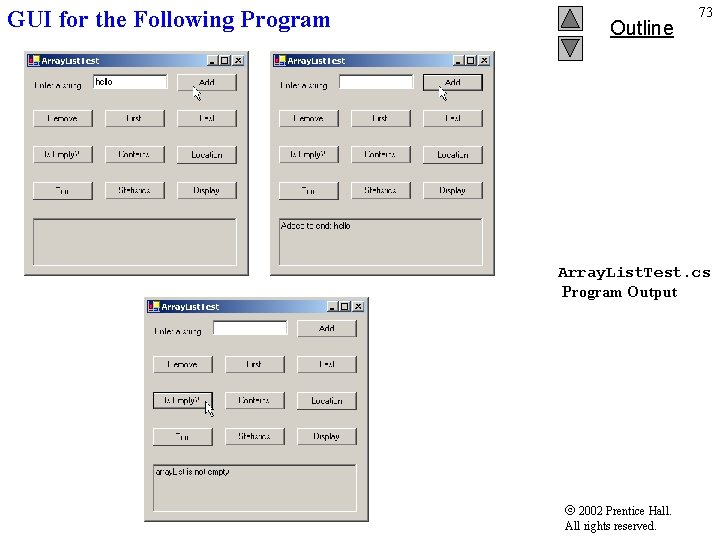
GUI for the Following Program Outline 73 Array. List. Test. cs Program Output 2002 Prentice Hall. All rights reserved.
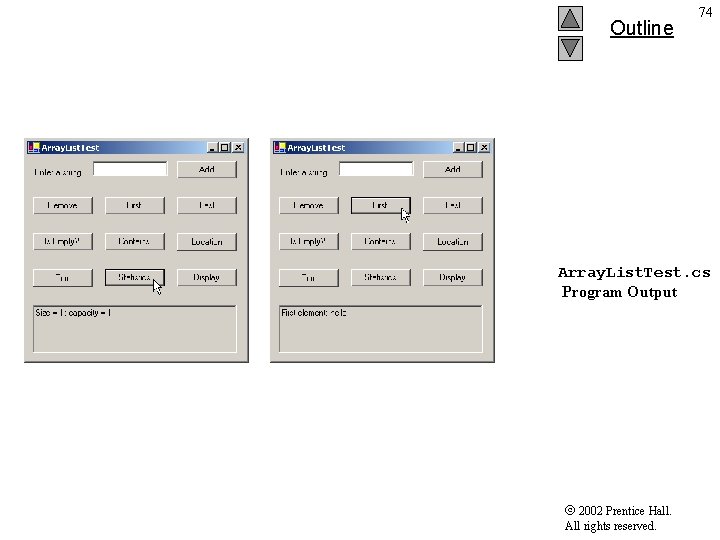
Outline 74 Array. List. Test. cs Program Output 2002 Prentice Hall. All rights reserved.
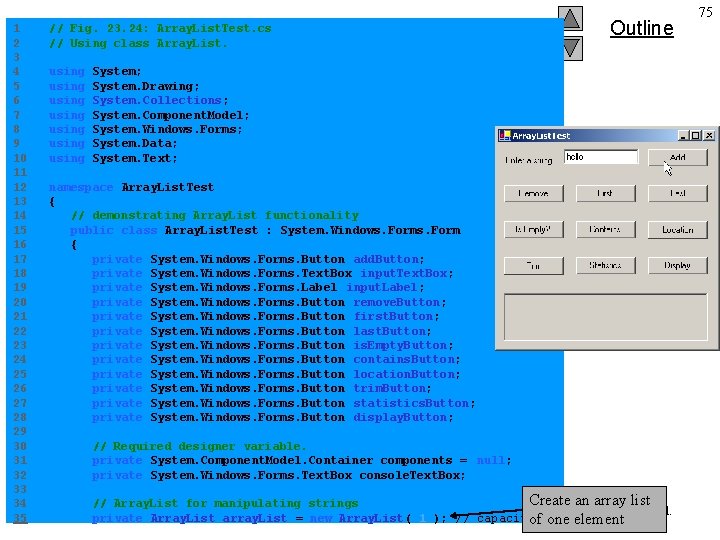
Outline 75 1 // Fig. 23. 24: Array. List. Test. cs 2 // Using class Array. List. 3 4 using System; 5 using System. Drawing; 6 using System. Collections; 7 using System. Component. Model; 8 using System. Windows. Forms; 9 using System. Data; 10 using System. Text; 11 12 namespace Array. List. Test 13 { 14 // demonstrating Array. List functionality 15 public class Array. List. Test : System. Windows. Form 16 { 17 private System. Windows. Forms. Button add. Button; 18 private System. Windows. Forms. Text. Box input. Text. Box; Array. List. Test. cs 19 private System. Windows. Forms. Label input. Label; 20 private System. Windows. Forms. Button remove. Button; 21 private System. Windows. Forms. Button first. Button; 22 private System. Windows. Forms. Button last. Button; 23 private System. Windows. Forms. Button is. Empty. Button; 24 private System. Windows. Forms. Button contains. Button; 25 private System. Windows. Forms. Button location. Button; 26 private System. Windows. Forms. Button trim. Button; 27 private System. Windows. Forms. Button statistics. Button; 28 private System. Windows. Forms. Button display. Button; 29 30 // Required designer variable. 31 private System. Component. Model. Container components = null; 32 private System. Windows. Forms. Text. Box console. Text. Box; 33 Create an array list 34 // Array. List for manipulating strings 2002 Prentice Hall. 35 private Array. List array. List = new Array. List( 1 ); // capacity=1 of one element All rights reserved.
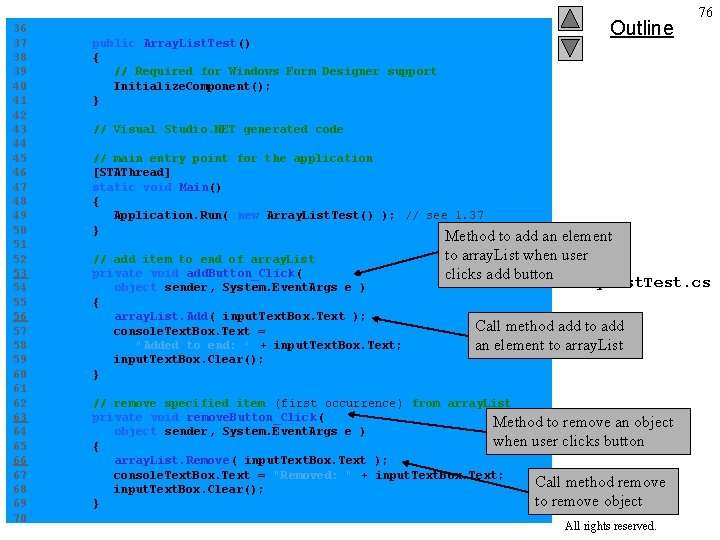
Outline 76 36 37 public Array. List. Test() 38 { 39 // Required for Windows Form Designer support 40 Initialize. Component(); 41 } 42 43 // Visual Studio. NET generated code 44 45 // main entry point for the application 46 [STAThread] 47 static void Main() 48 { 49 Application. Run( new Array. List. Test() ); // see l. 37 50 } Method to add an element 51 to array. List when user 52 // add item to end of array. List 53 private void add. Button_Click( clicks add button Array. List. Test. cs 54 object sender, System. Event. Args e ) 55 { 56 array. List. Add( input. Text. Box. Text ); Call method add to add 57 console. Text. Box. Text = 58 "Added to end: " + input. Text. Box. Text; an element to array. List 59 input. Text. Box. Clear(); 60 } 61 62 // remove specified item (first occurrence) from array. List 63 private void remove. Button_Click( Method to remove an object 64 object sender, System. Event. Args e ) when user clicks button 65 { 66 array. List. Remove( input. Text. Box. Text ); 67 console. Text. Box. Text = "Removed: " + input. Text. Box. Text; Call method remove 68 input. Text. Box. Clear(); to remove object 69 } 2002 Prentice Hall. 70 All rights reserved.
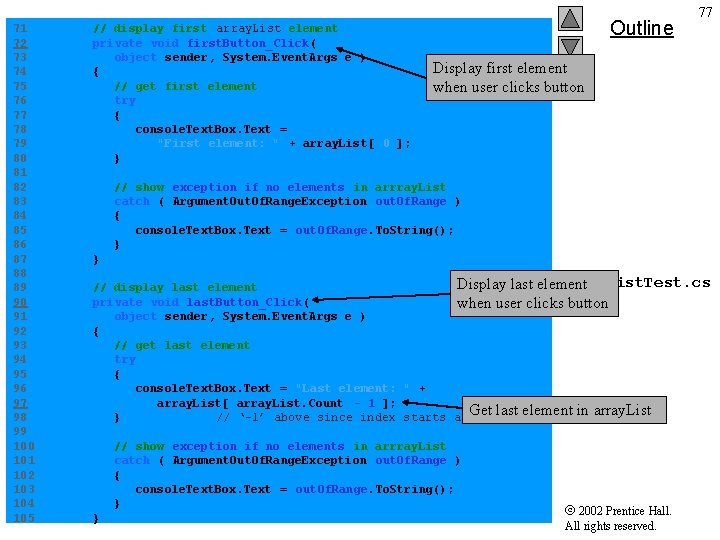
Outline 77 71 // display first array. List element 72 private void first. Button_Click( 73 object sender, System. Event. Args e ) Display first element 74 { 75 // get first element when user clicks button 76 try 77 { 78 console. Text. Box. Text = 79 "First element: " + array. List[ 0 ]; 80 } 81 82 // show exception if no elements in arrray. List 83 catch ( Argument. Out. Of. Range. Exception out. Of. Range ) 84 { 85 console. Text. Box. Text = out. Of. Range. To. String(); 86 } 87 } 88 Array. List. Test. cs Display last element 89 // display last element 90 private void last. Button_Click( when user clicks button 91 object sender, System. Event. Args e ) 92 { 93 // get last element 94 try 95 { 96 console. Text. Box. Text = "Last element: " + 97 array. List[ array. List. Count - 1 ]; 98 } // ‘-1’ above since index starts at Get last element in array. List ‘ 0’ 99 100 // show exception if no elements in arrray. List 101 catch ( Argument. Out. Of. Range. Exception out. Of. Range ) 102 { 103 console. Text. Box. Text = out. Of. Range. To. String(); 104 } 2002 Prentice Hall. 105 } All rights reserved.
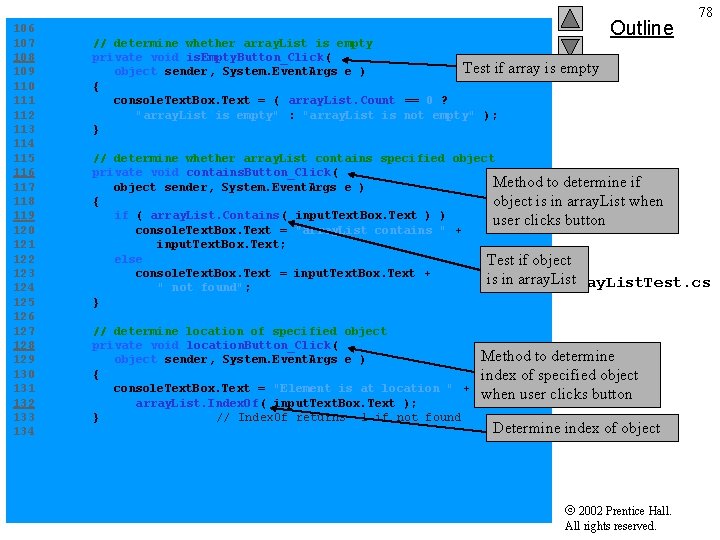
Outline 78 106 107 // determine whether array. List is empty 108 private void is. Empty. Button_Click( Test if array is empty 109 object sender, System. Event. Args e ) 110 { 111 console. Text. Box. Text = ( array. List. Count == 0 ? 112 "array. List is empty" : "array. List is not empty" ); 113 } 114 115 // determine whether array. List contains specified object 116 private void contains. Button_Click( Method to determine if 117 object sender, System. Event. Args e ) 118 { object is in array. List when 119 if ( array. List. Contains( input. Text. Box. Text ) ) user clicks button 120 console. Text. Box. Text = "array. List contains " + 121 input. Text. Box. Text; 122 else Test if object 123 console. Text. Box. Text = input. Text. Box. Text + is in array. List Array. List. Test. cs 124 " not found"; 125 } 126 127 // determine location of specified object 128 private void location. Button_Click( Method to determine 129 object sender, System. Event. Args e ) 130 { index of specified object 131 console. Text. Box. Text = "Element is at location " + when user clicks button 132 array. List. Index. Of( input. Text. Box. Text ); 133 } // Index. Of returns -1 if not found Determine index of object 134 2002 Prentice Hall. All rights reserved.
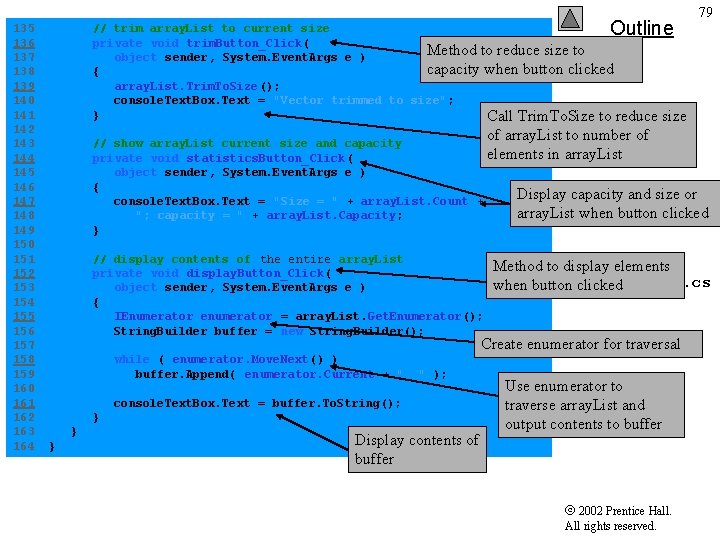
Outline 79 135 // trim array. List to current size 136 private void trim. Button_Click( Method to reduce size to 137 object sender, System. Event. Args e ) capacity when button clicked 138 { 139 array. List. Trim. To. Size(); 140 console. Text. Box. Text = "Vector trimmed to size" ; 141 } Call Trim. To. Size to reduce size 142 of array. List to number of 143 // show array. List current size and capacity elements in array. List 144 private void statistics. Button_Click( 145 object sender, System. Event. Args e ) 146 { Display capacity and size or 147 console. Text. Box. Text = "Size = " + array. List. Count + array. List when button clicked 148 "; capacity = " + array. List. Capacity; 149 } 150 151 // display contents of the entire array. List Method to display elements 152 private void display. Button_Click( Array. List. Test. cs when button clicked 153 object sender, System. Event. Args e ) 154 { 155 IEnumerator enumerator = array. List. Get. Enumerator(); 156 String. Builder buffer = new String. Builder(); 157 Create enumerator for traversal 158 while ( enumerator. Move. Next() ) 159 buffer. Append( enumerator. Current + " " ); Use enumerator to 160 161 console. Text. Box. Text = buffer. To. String(); traverse array. List and 162 } output contents to buffer 163 } Display contents of 164 } buffer 2002 Prentice Hall. All rights reserved.
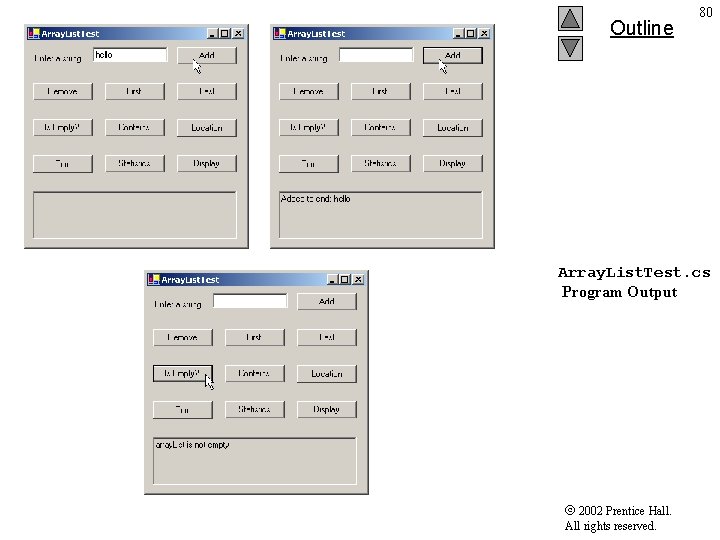
Outline 80 Array. List. Test. cs Program Output 2002 Prentice Hall. All rights reserved.
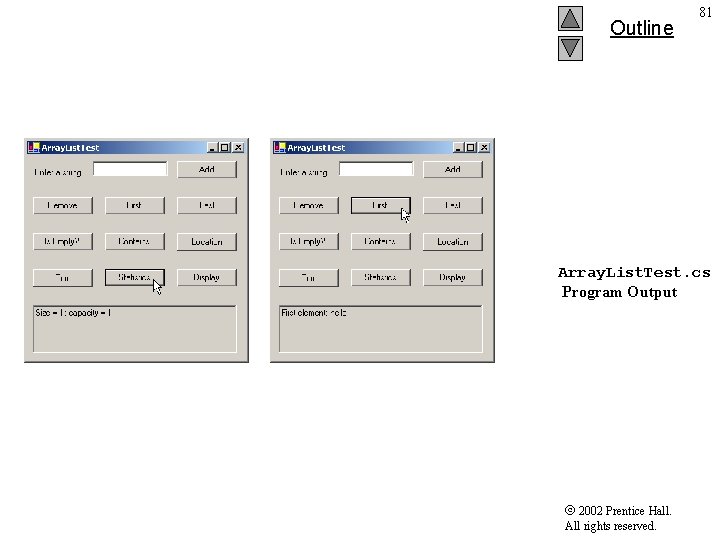
Outline 81 Array. List. Test. cs Program Output 2002 Prentice Hall. All rights reserved.
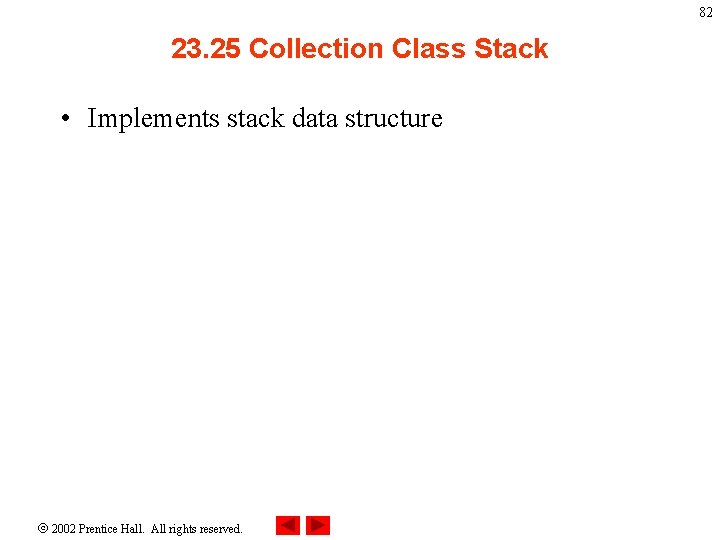
82 23. 25 Collection Class Stack • Implements stack data structure 2002 Prentice Hall. All rights reserved.
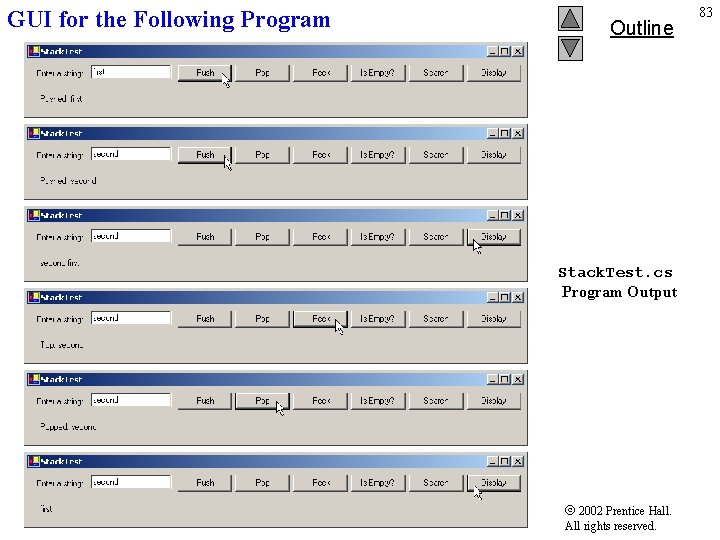
GUI for the Following Program Outline Stack. Test. cs Program Output 2002 Prentice Hall. All rights reserved. 83
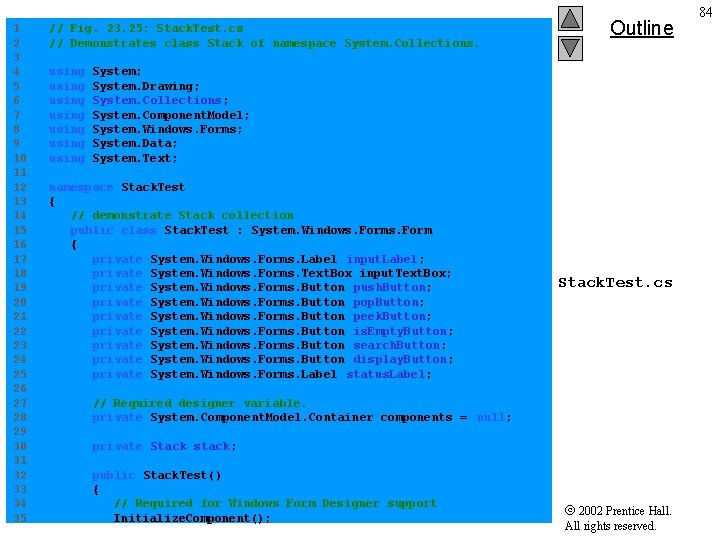
1 // Fig. 23. 25: Stack. Test. cs 2 // Demonstrates class Stack of namespace System. Collections. 3 4 using System; 5 using System. Drawing; 6 using System. Collections; 7 using System. Component. Model; 8 using System. Windows. Forms; 9 using System. Data; 10 using System. Text; 11 12 namespace Stack. Test 13 { 14 // demonstrate Stack collection 15 public class Stack. Test : System. Windows. Form 16 { 17 private System. Windows. Forms. Label input. Label; 18 private System. Windows. Forms. Text. Box input. Text. Box; 19 private System. Windows. Forms. Button push. Button; 20 private System. Windows. Forms. Button pop. Button; 21 private System. Windows. Forms. Button peek. Button; 22 private System. Windows. Forms. Button is. Empty. Button; 23 private System. Windows. Forms. Button search. Button; 24 private System. Windows. Forms. Button display. Button; 25 private System. Windows. Forms. Label status. Label; 26 27 // Required designer variable. 28 private System. Component. Model. Container components = null; 29 30 private Stack stack; 31 32 public Stack. Test() 33 { 34 // Required for Windows Form Designer support 35 Initialize. Component(); Outline Stack. Test. cs 2002 Prentice Hall. All rights reserved. 84
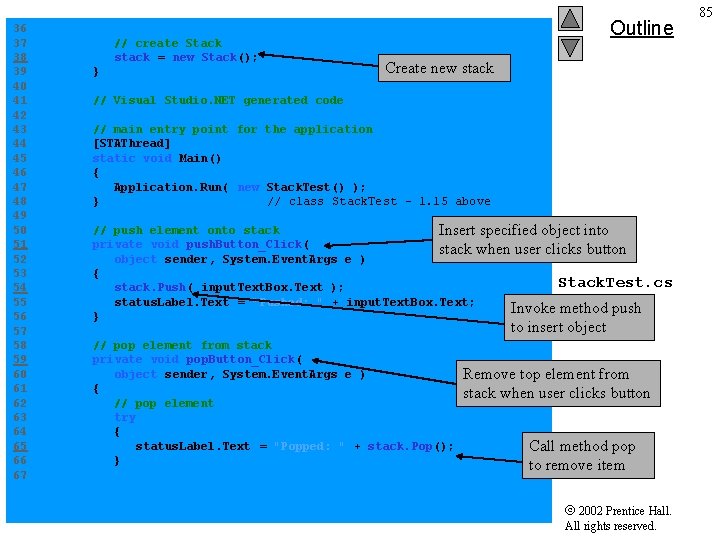
Outline 36 37 // create Stack 38 stack = new Stack(); Create new stack 39 } 40 41 // Visual Studio. NET generated code 42 43 // main entry point for the application 44 [STAThread] 45 static void Main() 46 { 47 Application. Run( new Stack. Test() ); 48 } // class Stack. Test - l. 15 above 49 50 // push element onto stack Insert specified object into 51 private void push. Button_Click( stack when user clicks button 52 object sender, System. Event. Args e ) 53 { Stack. Test. cs 54 stack. Push( input. Text. Box. Text ); 55 status. Label. Text = "Pushed: " + input. Text. Box. Text; Invoke method push 56 } to insert object 57 58 // pop element from stack 59 private void pop. Button_Click( 60 object sender, System. Event. Args e ) Remove top element from 61 { stack when user clicks button 62 // pop element 63 try 64 { 65 status. Label. Text = "Popped: " + stack. Pop(); Call method pop 66 } to remove item 67 2002 Prentice Hall. All rights reserved. 85
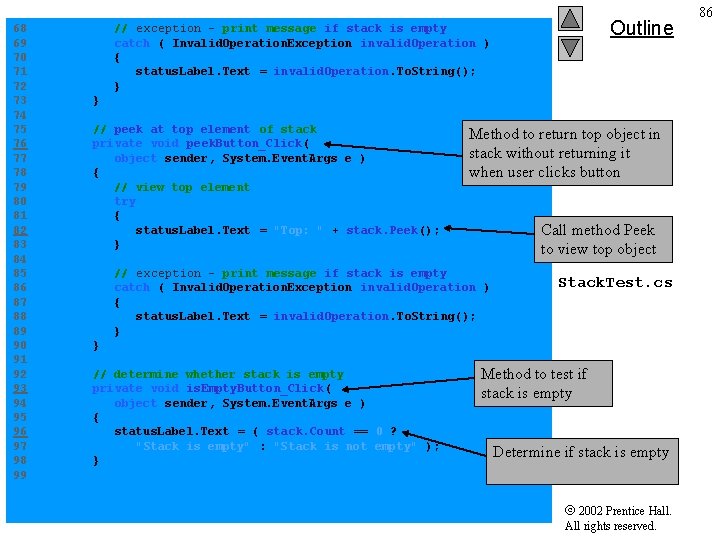
Outline 68 // exception - print message if stack is empty 69 catch ( Invalid. Operation. Exception invalid. Operation ) 70 { 71 status. Label. Text = invalid. Operation. To. String(); 72 } 73 } 74 75 // peek at top element of stack Method to return top object in 76 private void peek. Button_Click( stack without returning it 77 object sender, System. Event. Args e ) 78 { when user clicks button 79 // view top element 80 try 81 { 82 status. Label. Text = "Top: " + stack. Peek(); Call method Peek 83 } to view top object 84 85 // exception - print message if stack is empty Stack. Test. cs 86 catch ( Invalid. Operation. Exception invalid. Operation ) 87 { 88 status. Label. Text = invalid. Operation. To. String(); 89 } 90 } 91 92 // determine whether stack is empty Method to test if 93 private void is. Empty. Button_Click( stack is empty 94 object sender, System. Event. Args e ) 95 { 96 status. Label. Text = ( stack. Count == 0 ? 97 "Stack is empty" : "Stack is not empty" ); Determine if stack is empty 98 } 99 2002 Prentice Hall. All rights reserved. 86
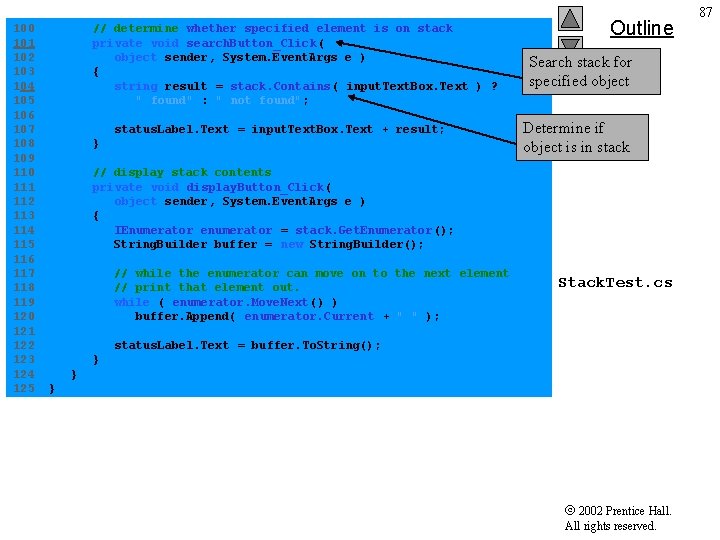
100 // determine whether specified element is on stack 101 private void search. Button_Click( 102 object sender, System. Event. Args e ) 103 { 104 string result = stack. Contains( input. Text. Box. Text ) ? 105 " found" : " not found"; 106 107 status. Label. Text = input. Text. Box. Text + result; 108 } 109 110 // display stack contents 111 private void display. Button_Click( 112 object sender, System. Event. Args e ) 113 { 114 IEnumerator enumerator = stack. Get. Enumerator(); 115 String. Builder buffer = new String. Builder(); 116 117 // while the enumerator can move on to the next element 118 // print that element out. 119 while ( enumerator. Move. Next() ) 120 buffer. Append( enumerator. Current + " " ); 121 122 status. Label. Text = buffer. To. String(); 123 } 124 } 125 } Outline Search stack for specified object Determine if object is in stack Stack. Test. cs 2002 Prentice Hall. All rights reserved. 87
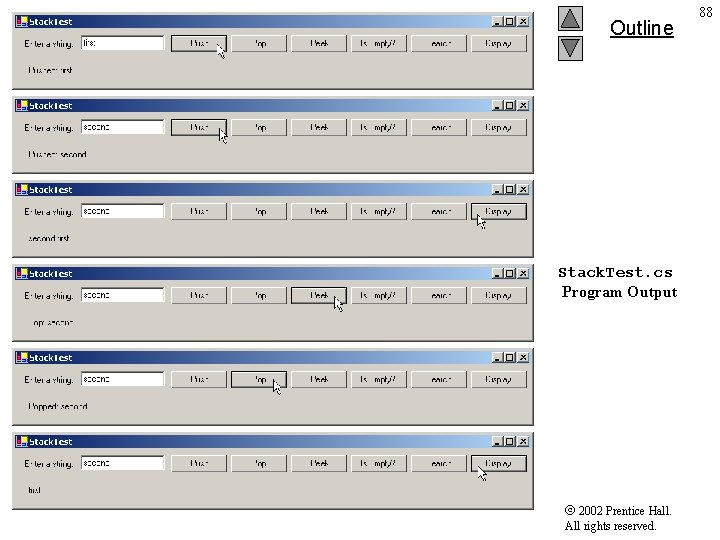
Outline Stack. Test. cs Program Output 2002 Prentice Hall. All rights reserved. 88
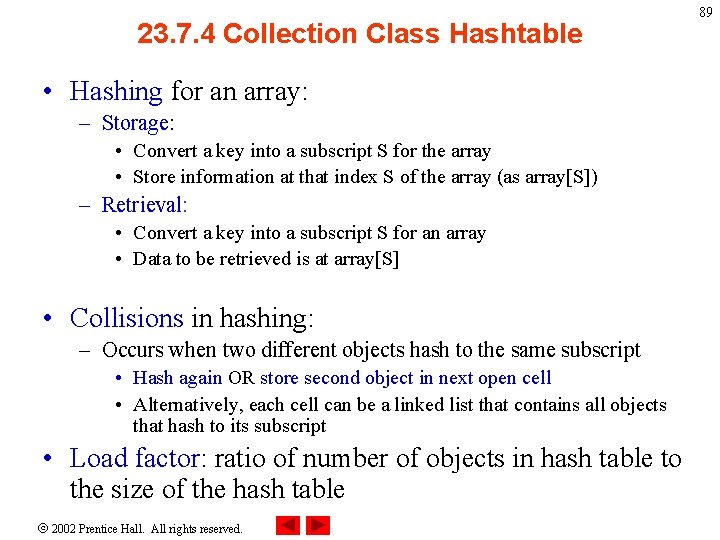
23. 7. 4 Collection Class Hashtable • Hashing for an array: – Storage: • Convert a key into a subscript S for the array • Store information at that index S of the array (as array[S]) – Retrieval: • Convert a key into a subscript S for an array • Data to be retrieved is at array[S] • Collisions in hashing: – Occurs when two different objects hash to the same subscript • Hash again OR store second object in next open cell • Alternatively, each cell can be a linked list that contains all objects that hash to its subscript • Load factor: ratio of number of objects in hash table to the size of the hash table 2002 Prentice Hall. All rights reserved. 89
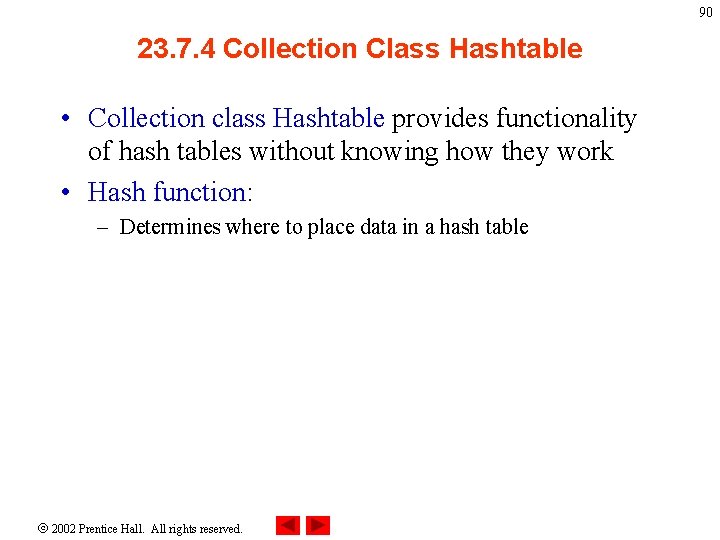
90 23. 7. 4 Collection Class Hashtable • Collection class Hashtable provides functionality of hash tables without knowing how they work • Hash function: – Determines where to place data in a hash table 2002 Prentice Hall. All rights reserved.
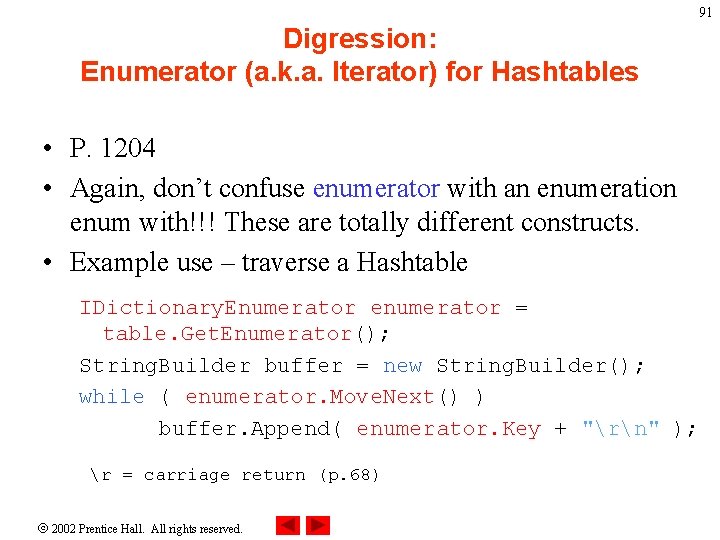
91 Digression: Enumerator (a. k. a. Iterator) for Hashtables • P. 1204 • Again, don’t confuse enumerator with an enumeration enum with!!! These are totally different constructs. • Example use – traverse a Hashtable IDictionary. Enumerator enumerator = table. Get. Enumerator(); String. Builder buffer = new String. Builder(); while ( enumerator. Move. Next() ) buffer. Append( enumerator. Key + "rn" ); r = carriage return (p. 68) 2002 Prentice Hall. All rights reserved.
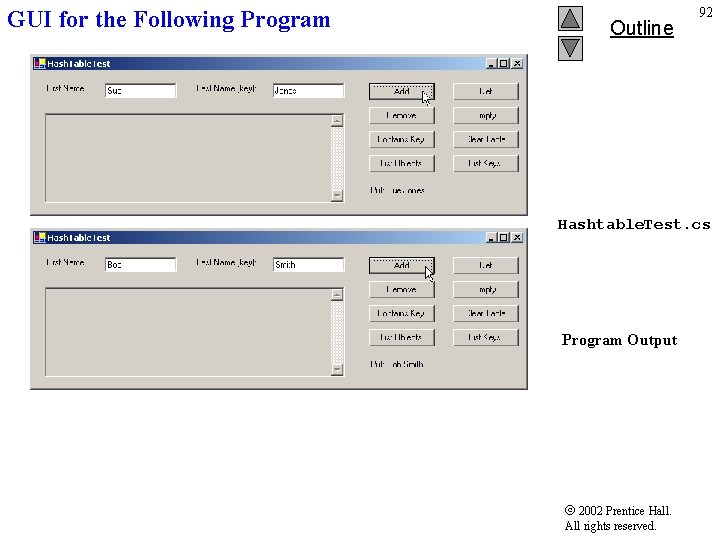
GUI for the Following Program Outline 92 Hashtable. Test. cs Program Output 2002 Prentice Hall. All rights reserved.
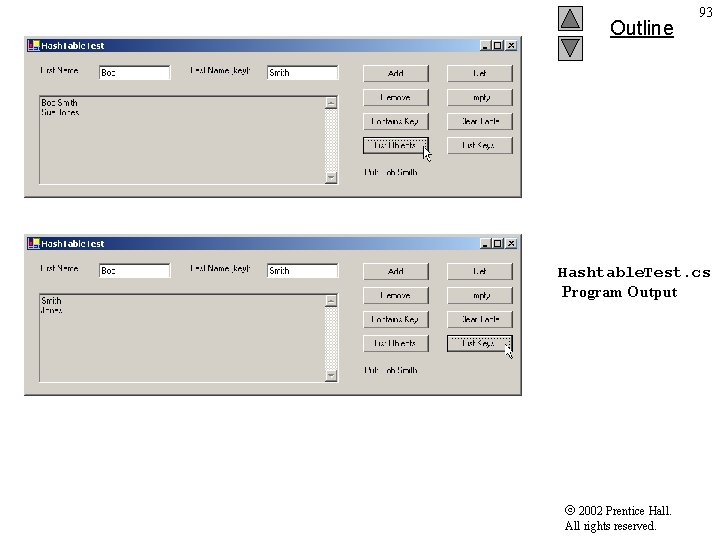
Outline 93 Hashtable. Test. cs Program Output 2002 Prentice Hall. All rights reserved.
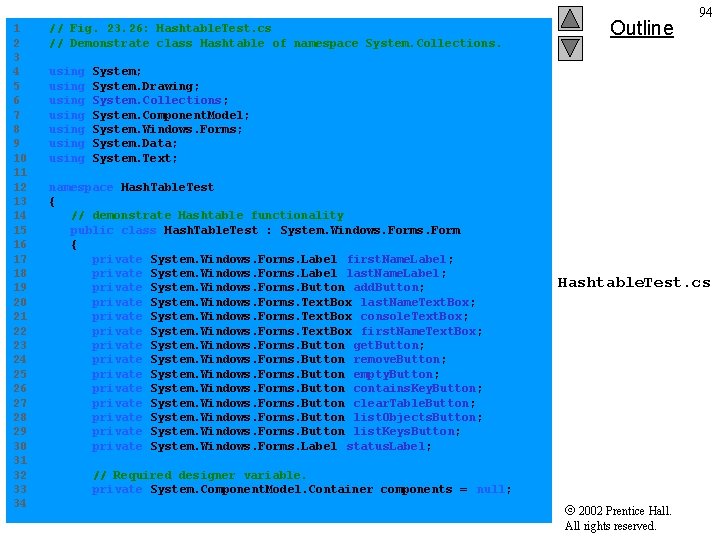
1 // Fig. 23. 26: Hashtable. Test. cs 2 // Demonstrate class Hashtable of namespace System. Collections. 3 4 using System; 5 using System. Drawing; 6 using System. Collections; 7 using System. Component. Model; 8 using System. Windows. Forms; 9 using System. Data; 10 using System. Text; 11 12 namespace Hash. Table. Test 13 { 14 // demonstrate Hashtable functionality 15 public class Hash. Table. Test : System. Windows. Form 16 { 17 private System. Windows. Forms. Label first. Name. Label; 18 private System. Windows. Forms. Label last. Name. Label; 19 private System. Windows. Forms. Button add. Button; 20 private System. Windows. Forms. Text. Box last. Name. Text. Box; 21 private System. Windows. Forms. Text. Box console. Text. Box; 22 private System. Windows. Forms. Text. Box first. Name. Text. Box; 23 private System. Windows. Forms. Button get. Button; 24 private System. Windows. Forms. Button remove. Button; 25 private System. Windows. Forms. Button empty. Button; 26 private System. Windows. Forms. Button contains. Key. Button; 27 private System. Windows. Forms. Button clear. Table. Button; 28 private System. Windows. Forms. Button list. Objects. Button; 29 private System. Windows. Forms. Button list. Keys. Button; 30 private System. Windows. Forms. Label status. Label; 31 32 // Required designer variable. 33 private System. Component. Model. Container components = null; 34 Outline 94 Hashtable. Test. cs 2002 Prentice Hall. All rights reserved.
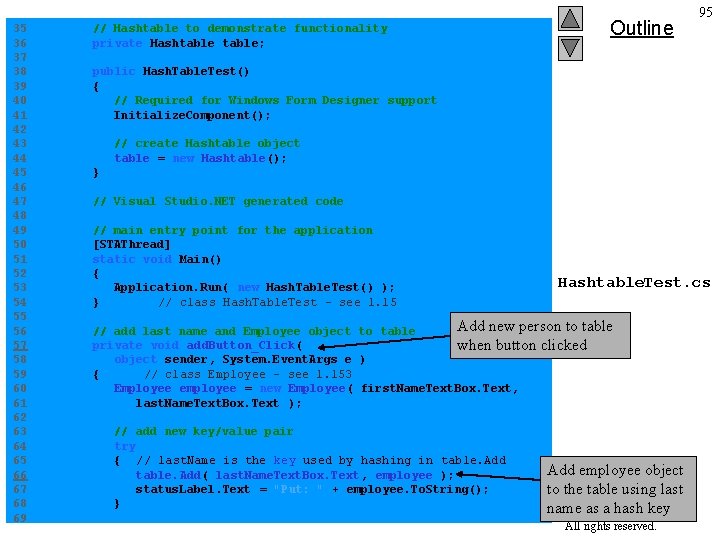
Outline 95 35 // Hashtable to demonstrate functionality 36 private Hashtable; 37 38 public Hash. Table. Test() 39 { 40 // Required for Windows Form Designer support 41 Initialize. Component(); 42 43 // create Hashtable object 44 table = new Hashtable(); 45 } 46 47 // Visual Studio. NET generated code 48 49 // main entry point for the application 50 [STAThread] 51 static void Main() 52 { Hashtable. Test. cs 53 Application. Run( new Hash. Table. Test() ); 54 } // class Hash. Table. Test – see l. 15 55 Add new person to table 56 // add last name and Employee object to table 57 private void add. Button_Click( when button clicked 58 object sender, System. Event. Args e ) 59 { // class Employee – see l. 153 60 Employee employee = new Employee( first. Name. Text. Box. Text, 61 last. Name. Text. Box. Text ); 62 63 // add new key/value pair 64 try 65 { // last. Name is the key used by hashing in table. Add employee object 66 table. Add( last. Name. Text. Box. Text, employee ); 67 status. Label. Text = "Put: " + employee. To. String(); to the table using last 68 } name as a hash key 2002 Prentice Hall. 69 All rights reserved.
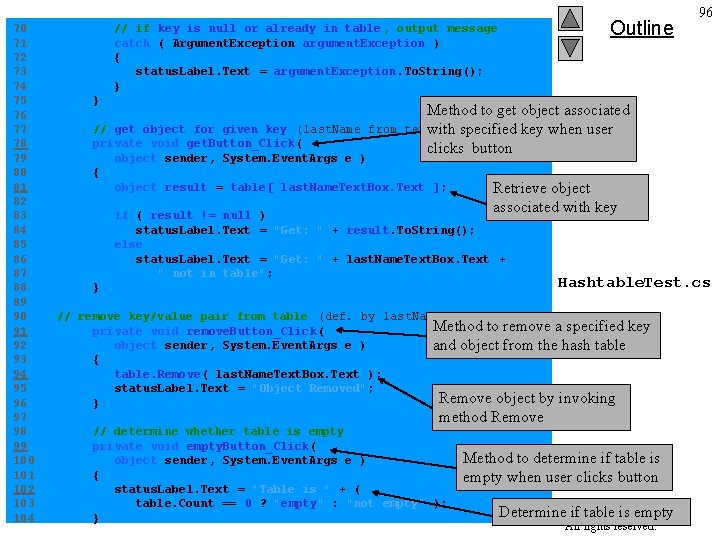
Outline 96 70 // if key is null or already in table , output message 71 catch ( Argument. Exception argument. Exception ) 72 { 73 status. Label. Text = argument. Exception. To. String(); 74 } 75 } Method to get object associated 76 77 // get object for given key (last. Name from textbox) with specified key when user 78 private void get. Button_Click( clicks button 79 object sender, System. Event. Args e ) 80 { 81 object result = table[ last. Name. Text. Box. Text ]; Retrieve object 82 associated with key 83 if ( result != null ) 84 status. Label. Text = "Get: " + result. To. String(); 85 else 86 status. Label. Text = "Get: " + last. Name. Text. Box. Text + 87 " not in table"; Hashtable. Test. cs 88 } 89 90 // remove key/value pair from table (def. by last. Name fr. textbox) Method to remove a specified key 91 private void remove. Button_Click( 92 object sender, System. Event. Args e ) and object from the hash table 93 { 94 table. Remove( last. Name. Text. Box. Text ); 95 status. Label. Text = "Object Removed"; Remove object by invoking 96 } 97 method Remove 98 // determine whether table is empty 99 private void empty. Button_Click( Method to determine if table is 100 object sender, System. Event. Args e ) 101 { empty when user clicks button 102 status. Label. Text = "Table is " + ( 103 table. Count == 0 ? "empty" : "not empty" ); 2002 Prentice Hall. Determine if table is empty 104 } All rights reserved.
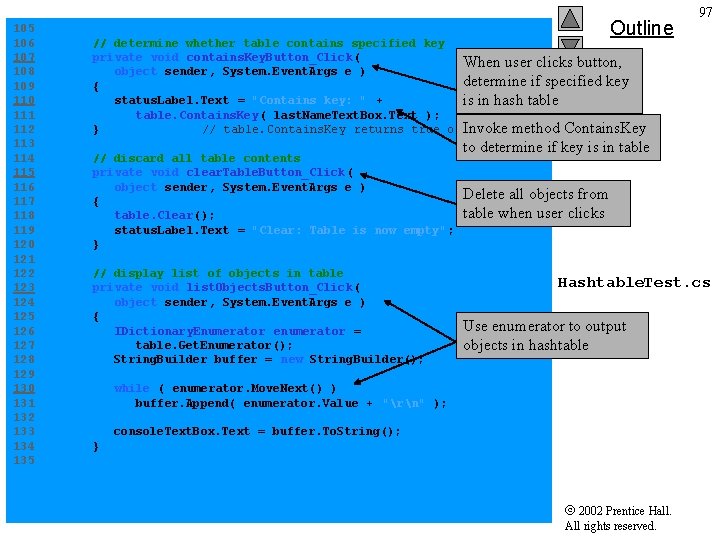
Outline 97 105 106 // determine whether table contains specified key 107 private void contains. Key. Button_Click( When user clicks button, 108 object sender, System. Event. Args e ) determine if specified key 109 { 110 status. Label. Text = "Contains key: " + is in hash table 111 table. Contains. Key( last. Name. Text. Box. Text ); 112 } // table. Contains. Key returns true or Invoke method Contains. Key false 113 to determine if key is in table 114 // discard all table contents 115 private void clear. Table. Button_Click( 116 object sender, System. Event. Args e ) Delete all objects from 117 { table when user clicks 118 table. Clear(); 119 status. Label. Text = "Clear: Table is now empty" ; 120 } 121 122 // display list of objects in table Hashtable. Test. cs 123 private void list. Objects. Button_Click( 124 object sender, System. Event. Args e ) 125 { Use enumerator to output 126 IDictionary. Enumerator enumerator = 127 table. Get. Enumerator(); objects in hashtable 128 String. Builder buffer = new String. Builder(); 129 130 while ( enumerator. Move. Next() ) 131 buffer. Append( enumerator. Value + "rn" ); 132 133 console. Text. Box. Text = buffer. To. String(); 134 } 135 2002 Prentice Hall. All rights reserved.
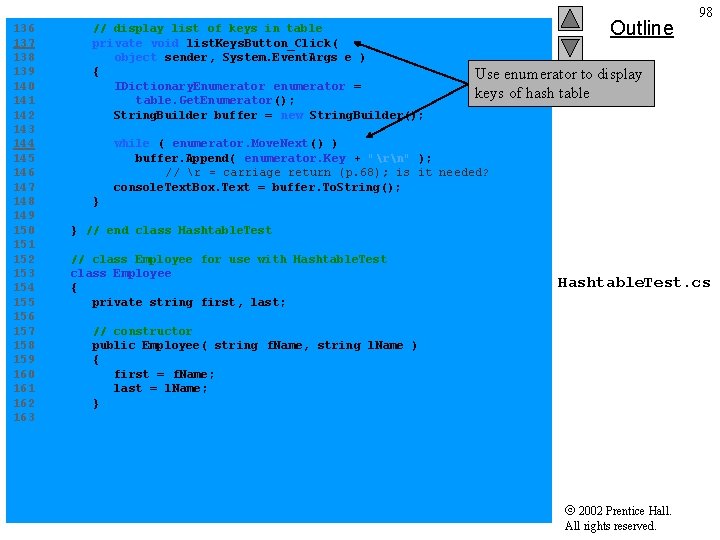
Outline 98 136 // display list of keys in table 137 private void list. Keys. Button_Click( 138 object sender, System. Event. Args e ) 139 { Use enumerator to display 140 IDictionary. Enumerator enumerator = keys of hash table 141 table. Get. Enumerator(); 142 String. Builder buffer = new String. Builder(); 143 144 while ( enumerator. Move. Next() ) 145 buffer. Append( enumerator. Key + "rn" ); 146 // r = carriage return (p. 68); is it needed? 147 console. Text. Box. Text = buffer. To. String(); 148 } 149 150 } // end class Hashtable. Test 151 152 // class Employee for use with Hashtable. Test 153 class Employee Hashtable. Test. cs 154 { 155 private string first, last; 156 157 // constructor 158 public Employee( string f. Name, string l. Name ) 159 { 160 first = f. Name; 161 last = l. Name; 162 } 163 2002 Prentice Hall. All rights reserved.
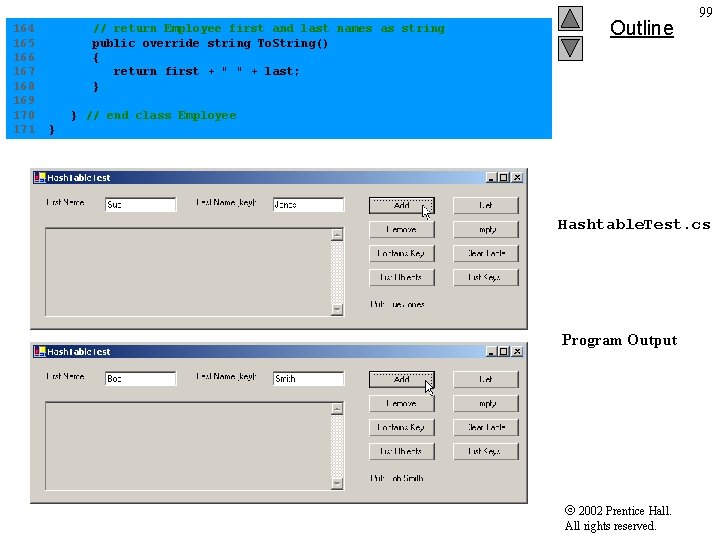
164 // return Employee first and last names as string 165 public override string To. String() 166 { 167 return first + " " + last; 168 } 169 170 } // end class Employee 171 } Outline 99 Hashtable. Test. cs Program Output 2002 Prentice Hall. All rights reserved.
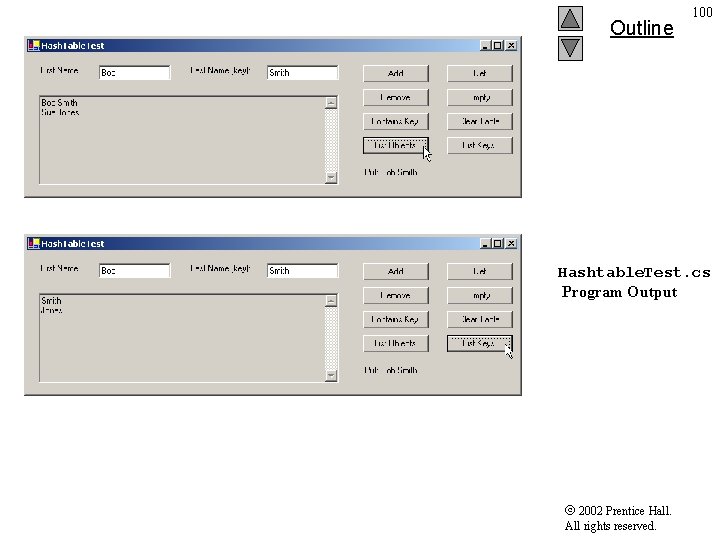
Outline 100 Hashtable. Test. cs Program Output 2002 Prentice Hall. All rights reserved.