Python Tutorial Why Python Python is a generalpurpose
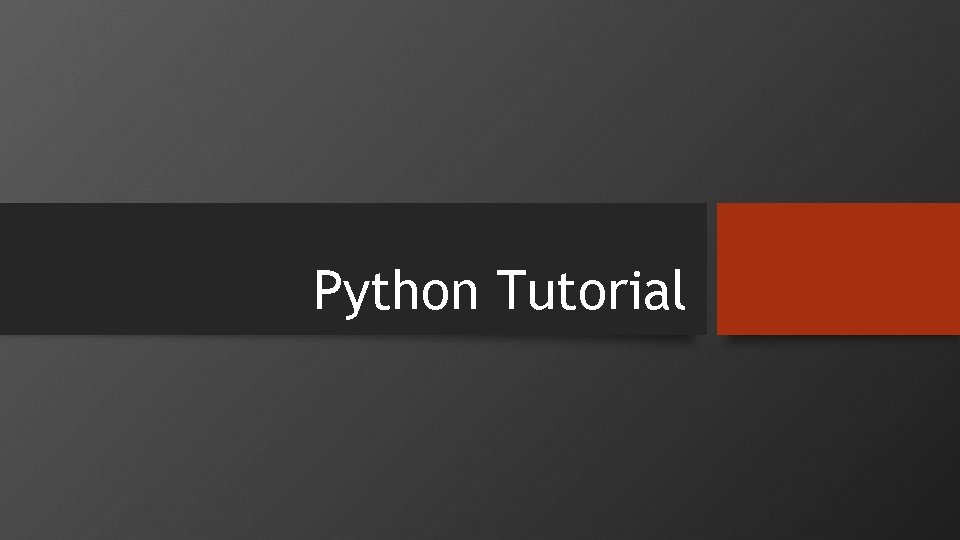
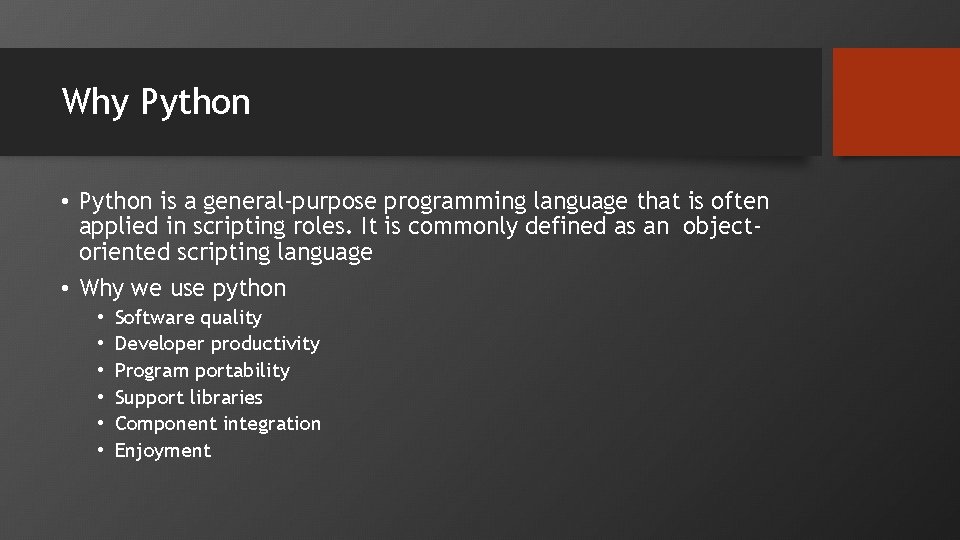
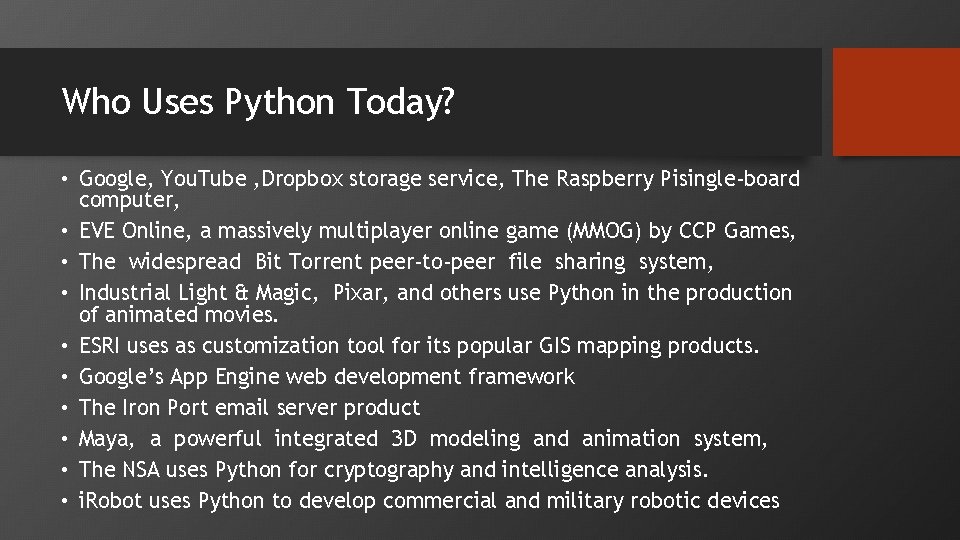
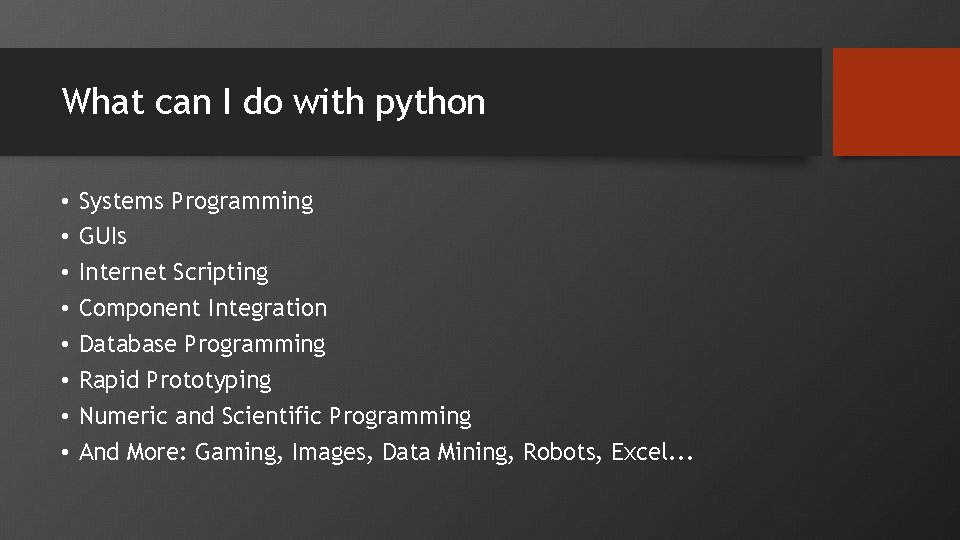
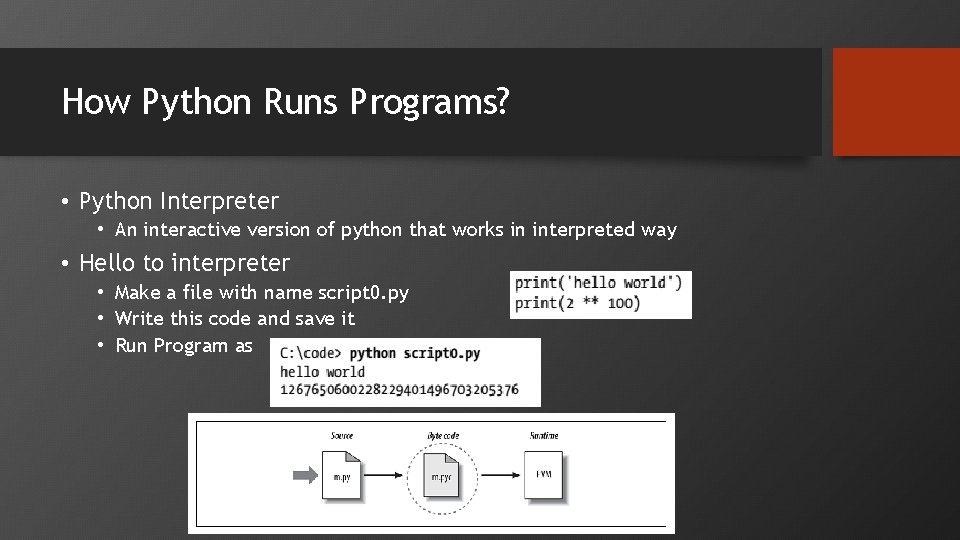
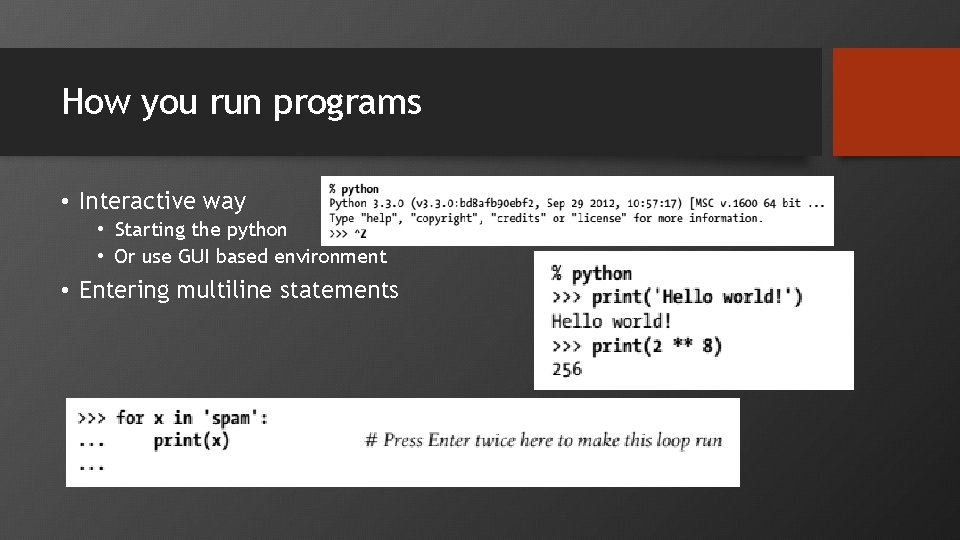
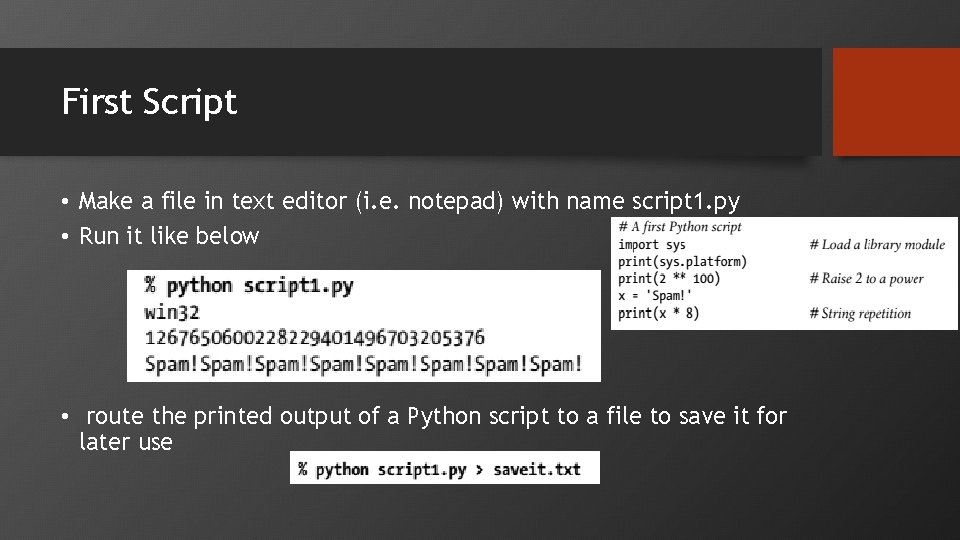
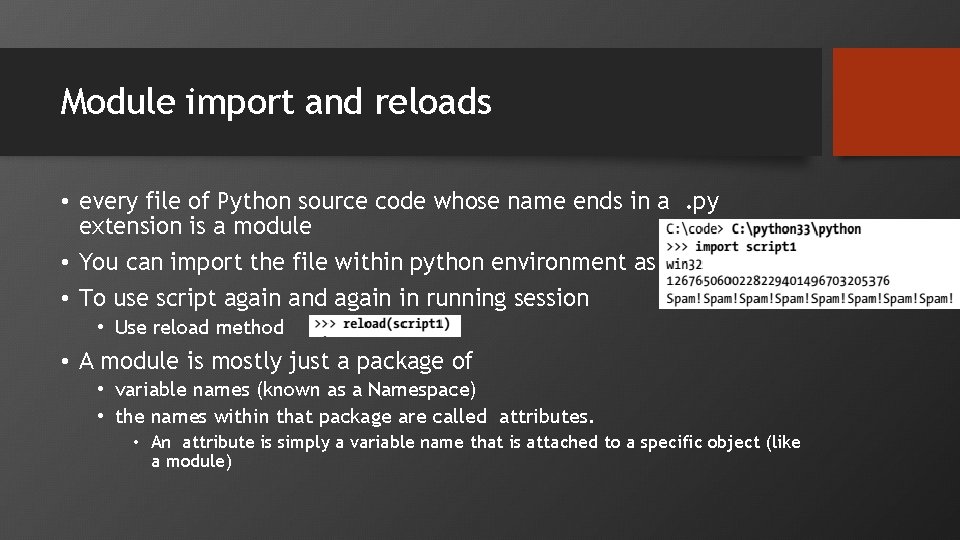
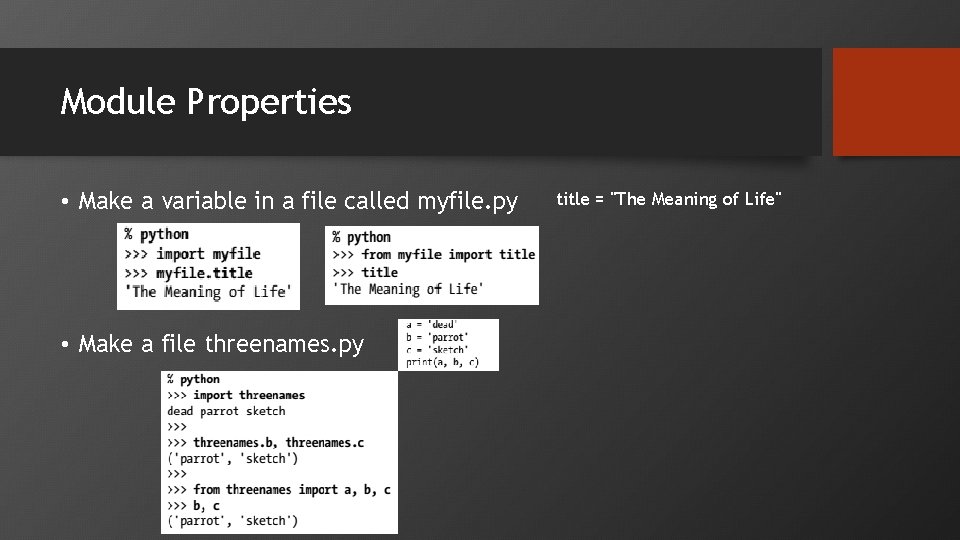
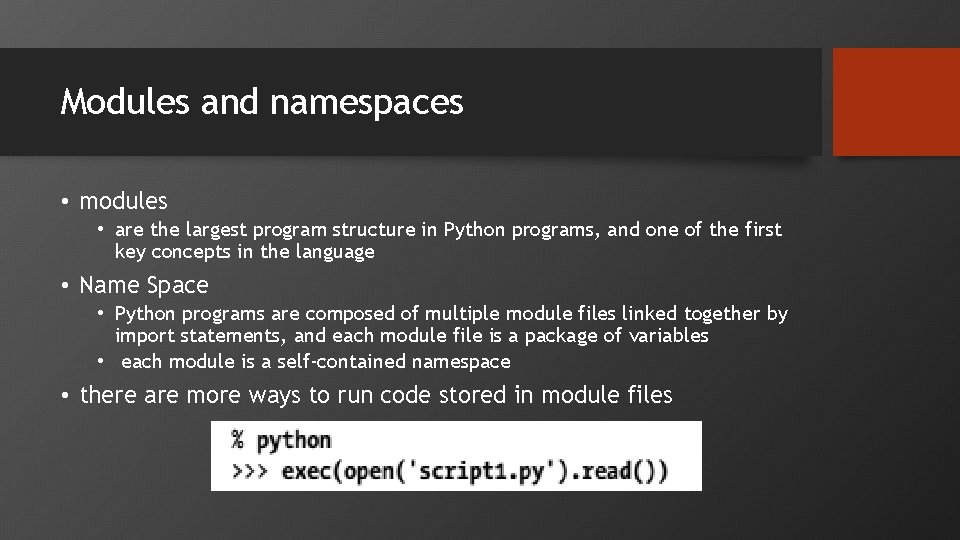
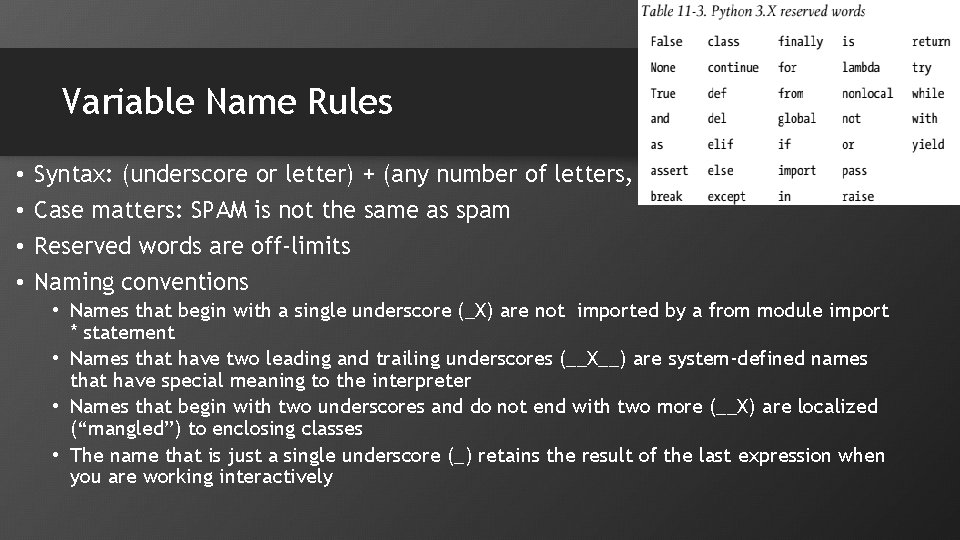
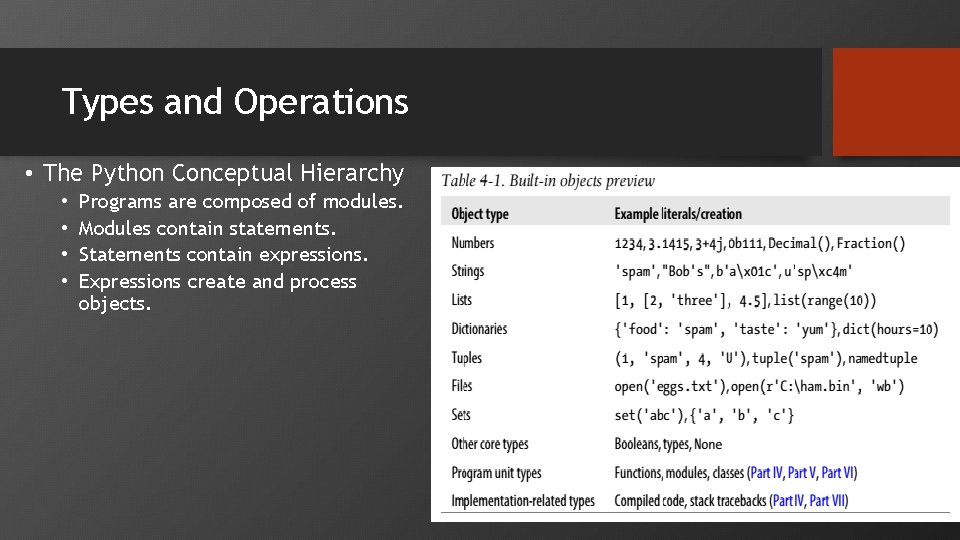
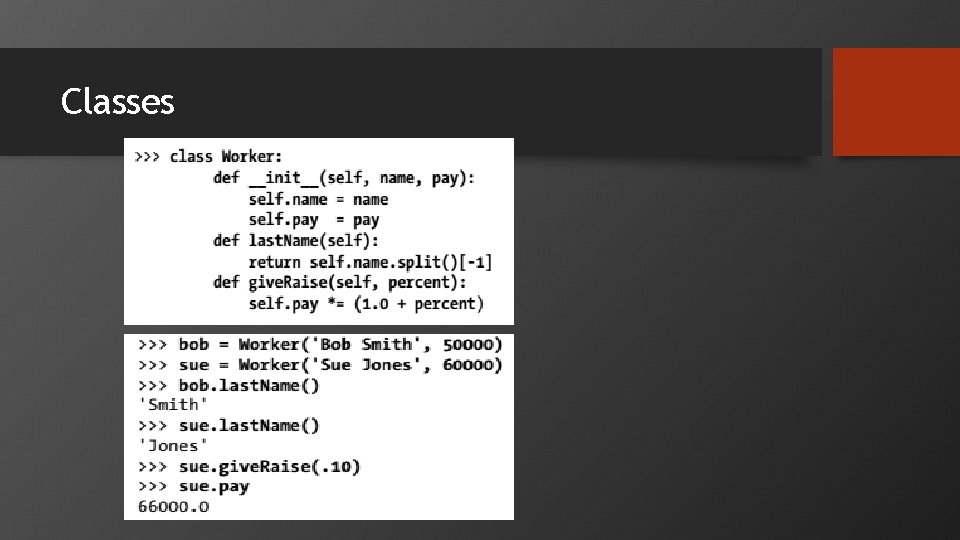
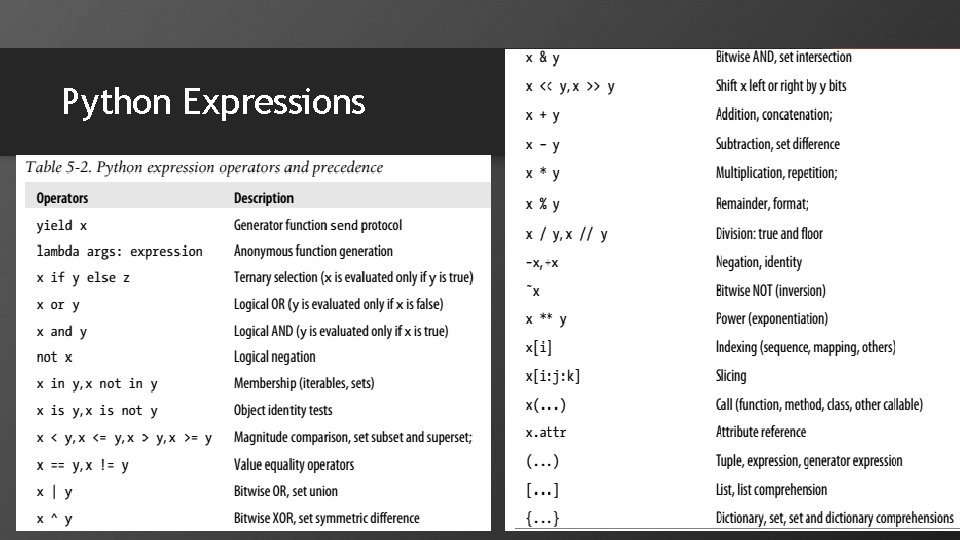
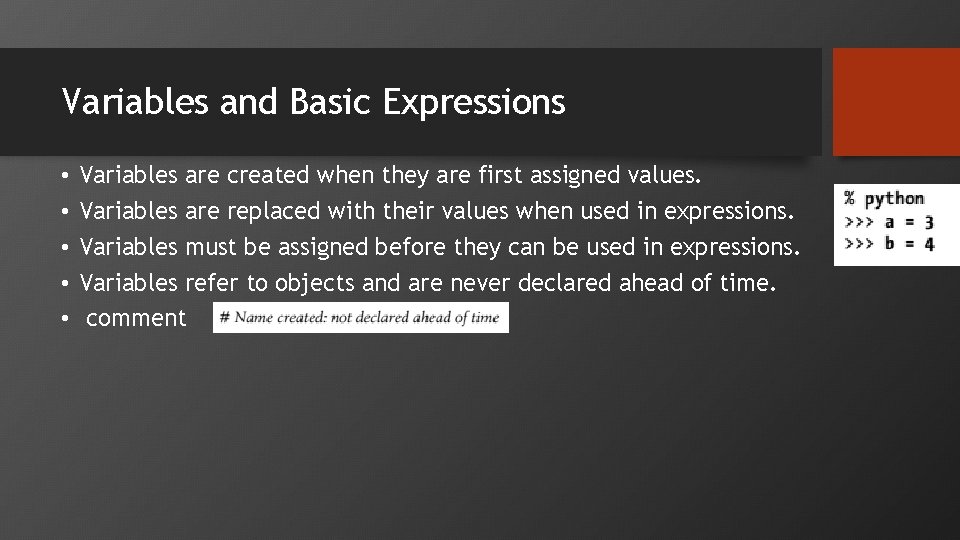
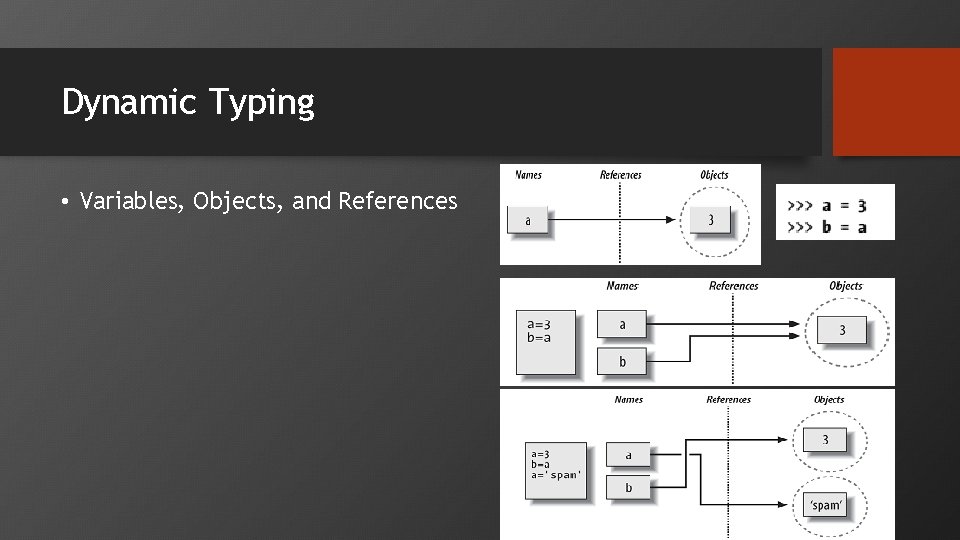
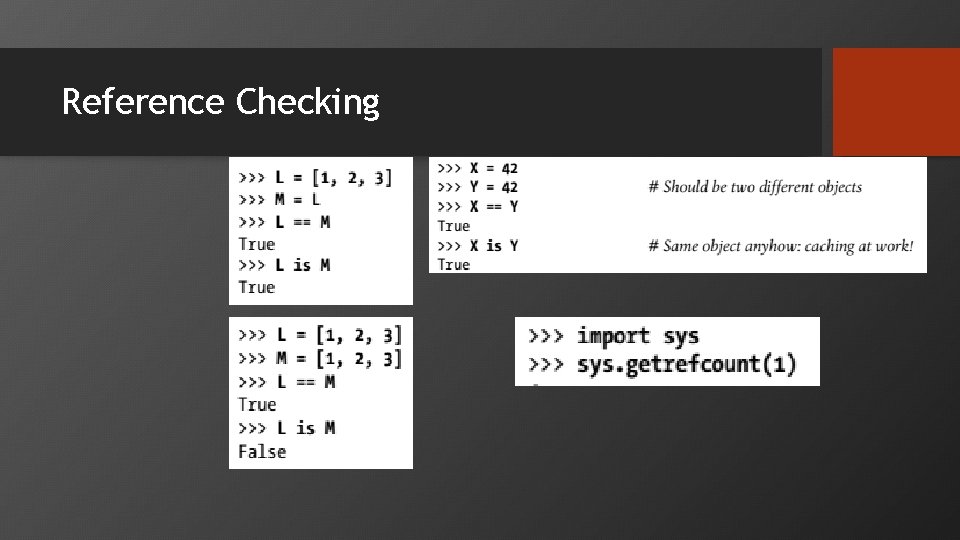
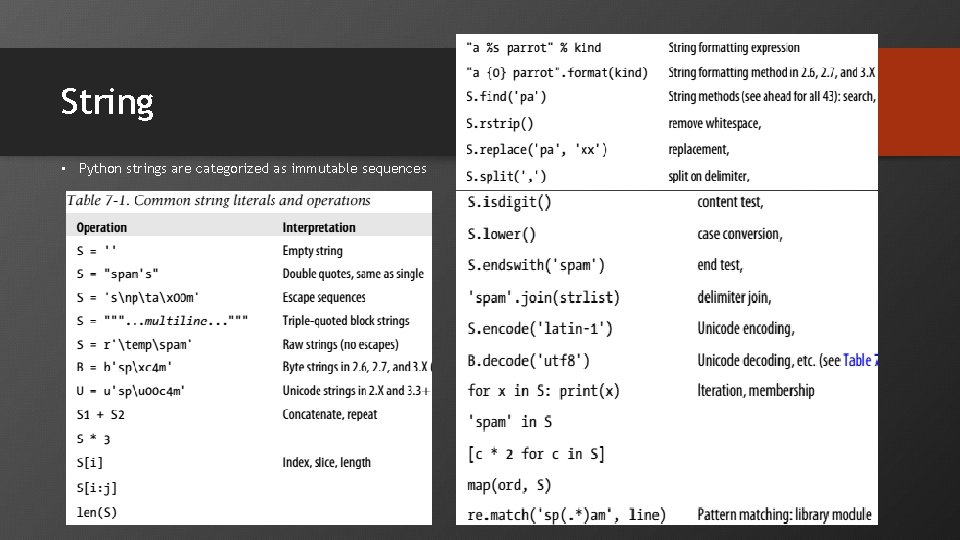
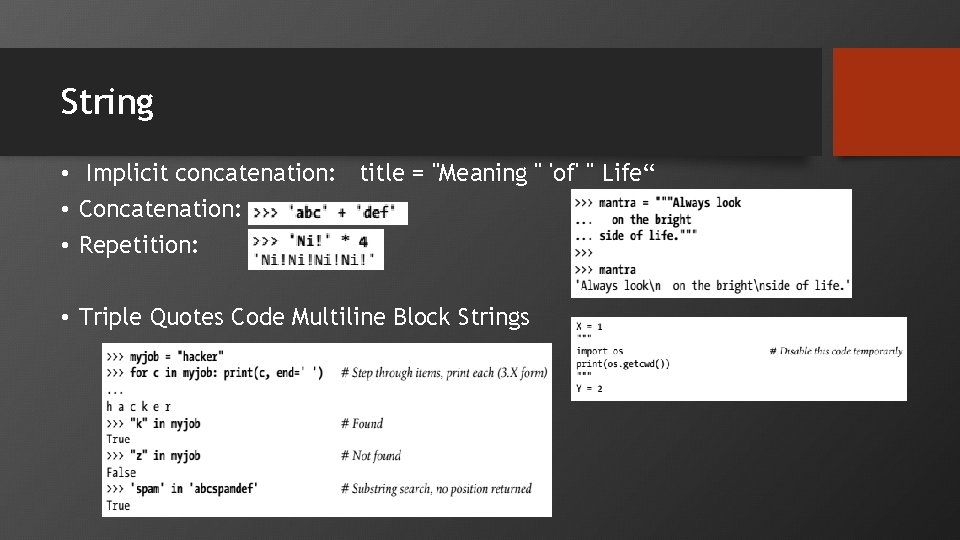
![String Indexing and Slicing • X[1: 10: 2] • will fetch every other item String Indexing and Slicing • X[1: 10: 2] • will fetch every other item](https://slidetodoc.com/presentation_image_h2/b83870a8ed120330c0c40eeb3521b05c/image-20.jpg)
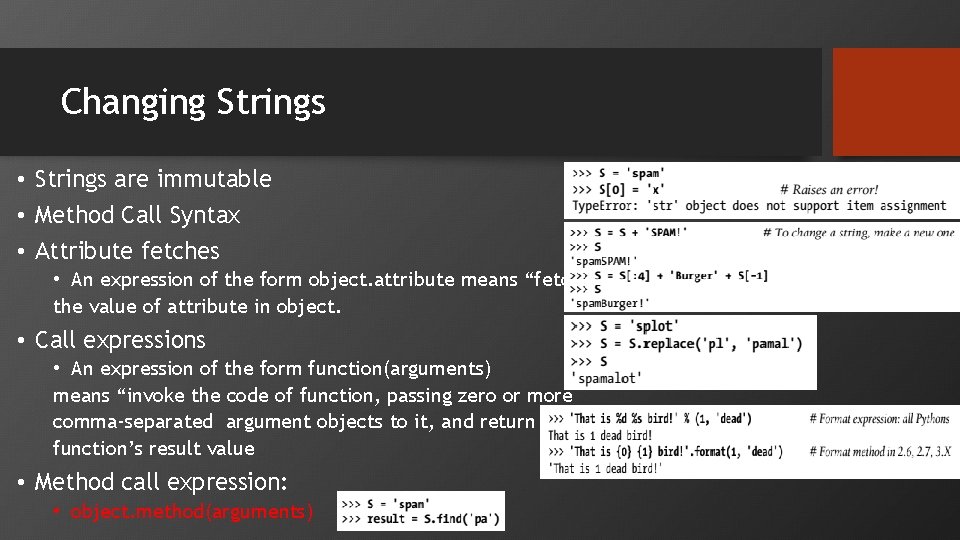
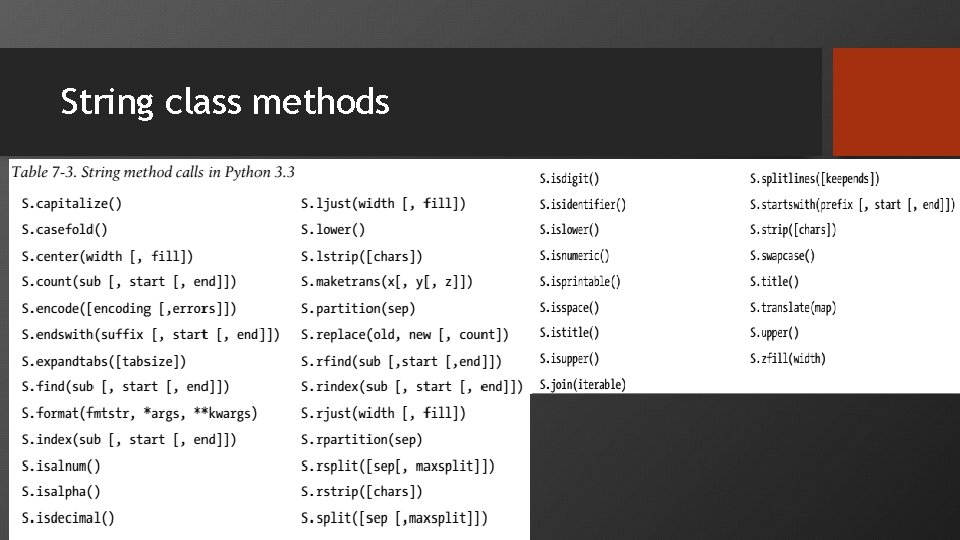
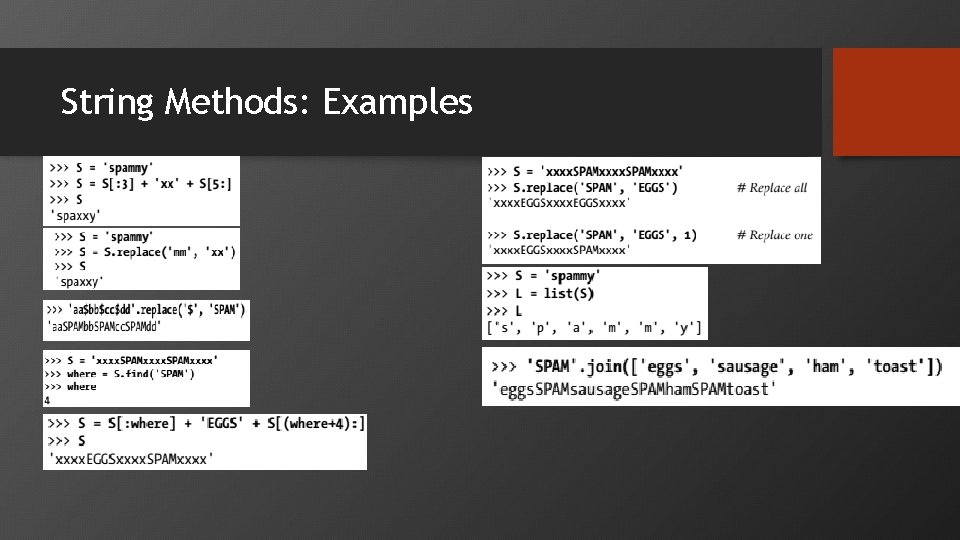
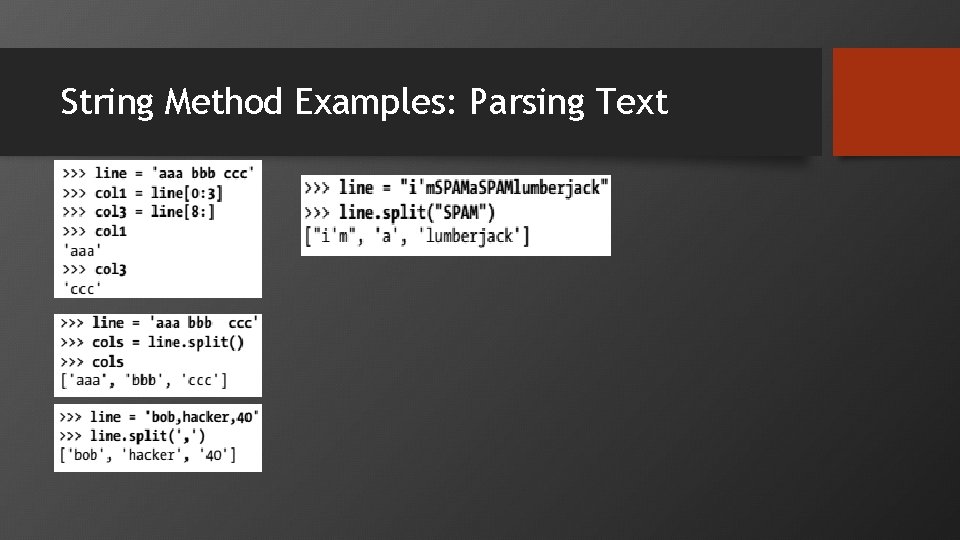
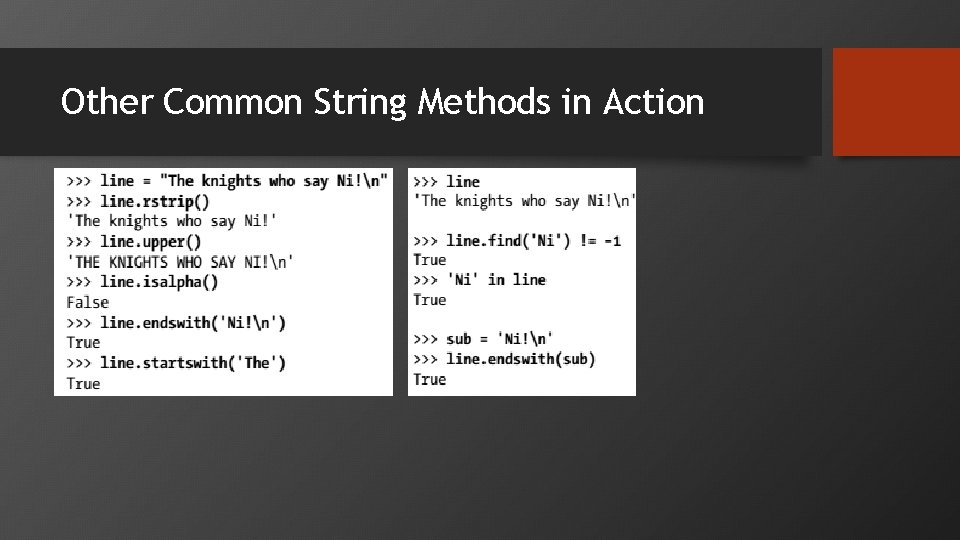
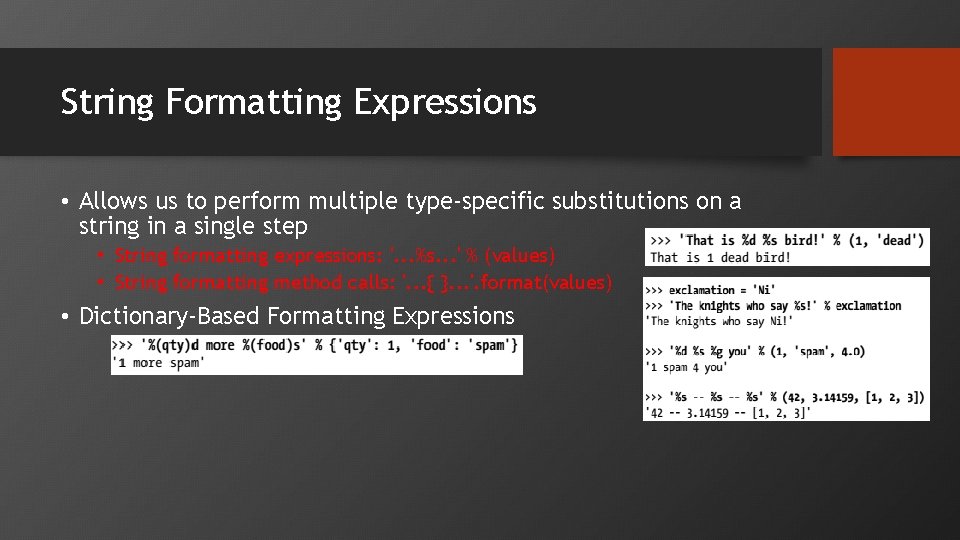
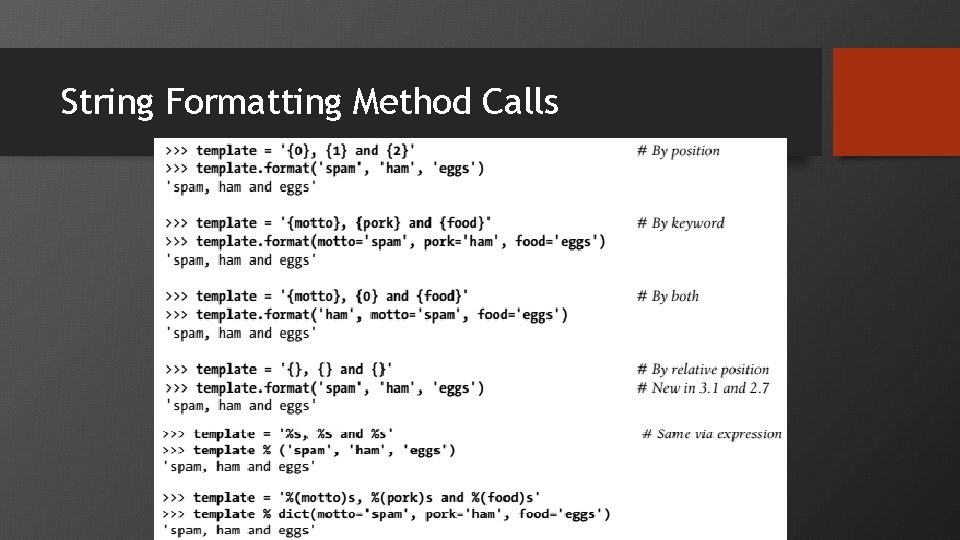
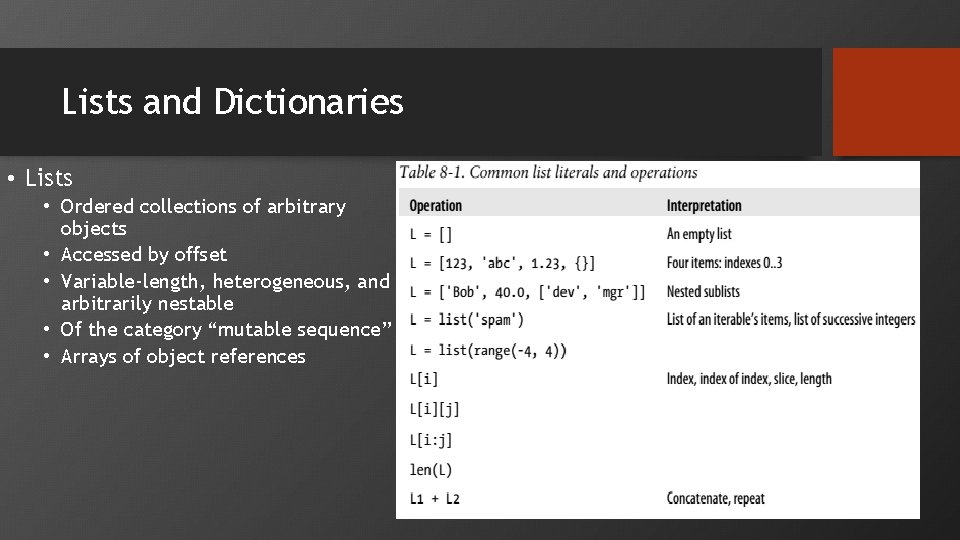
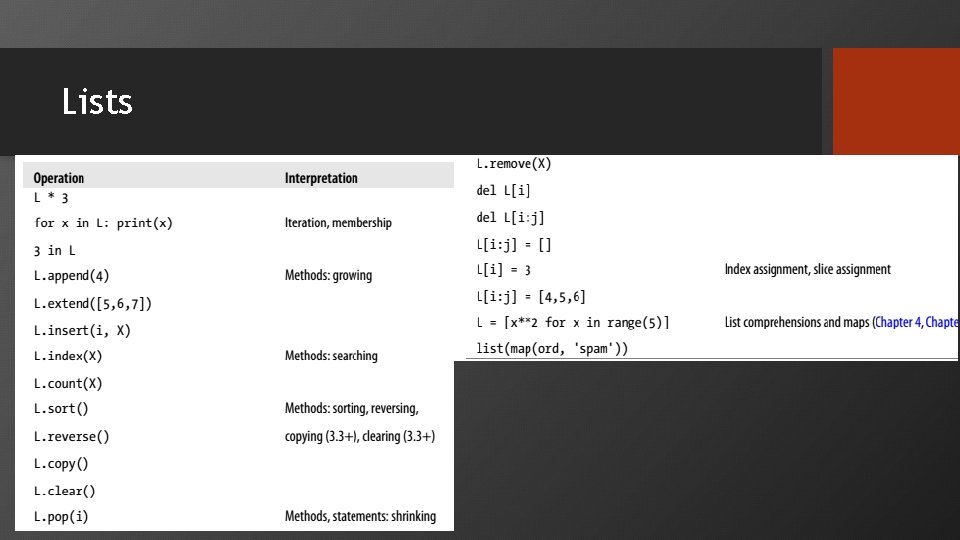
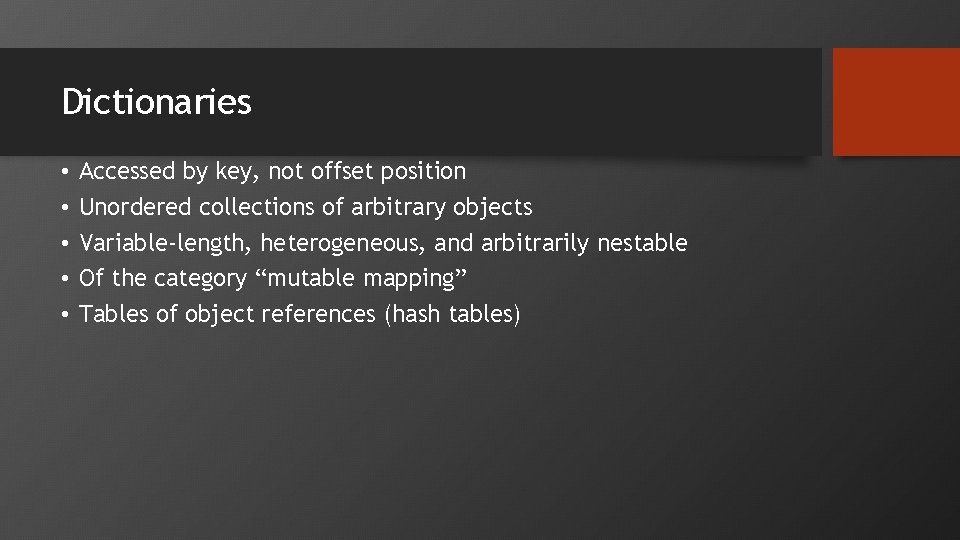
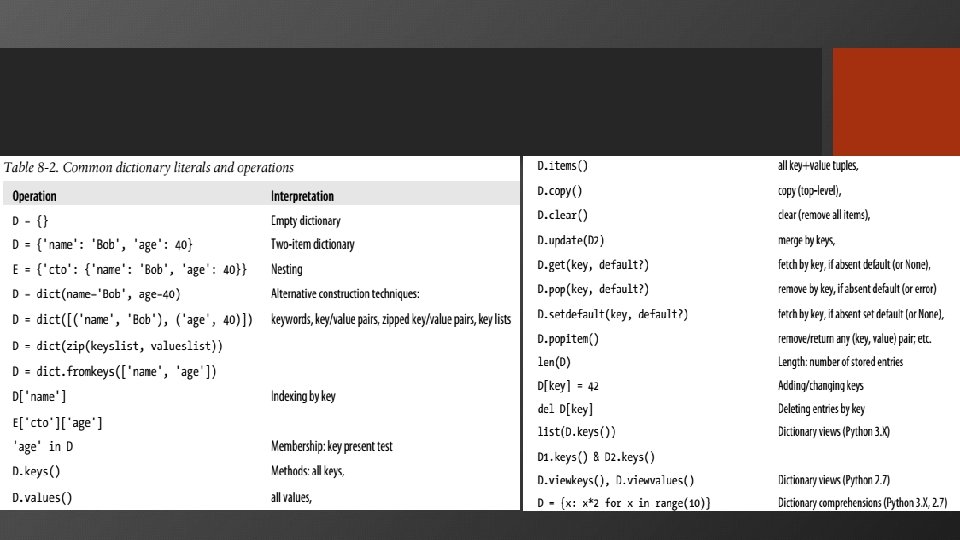
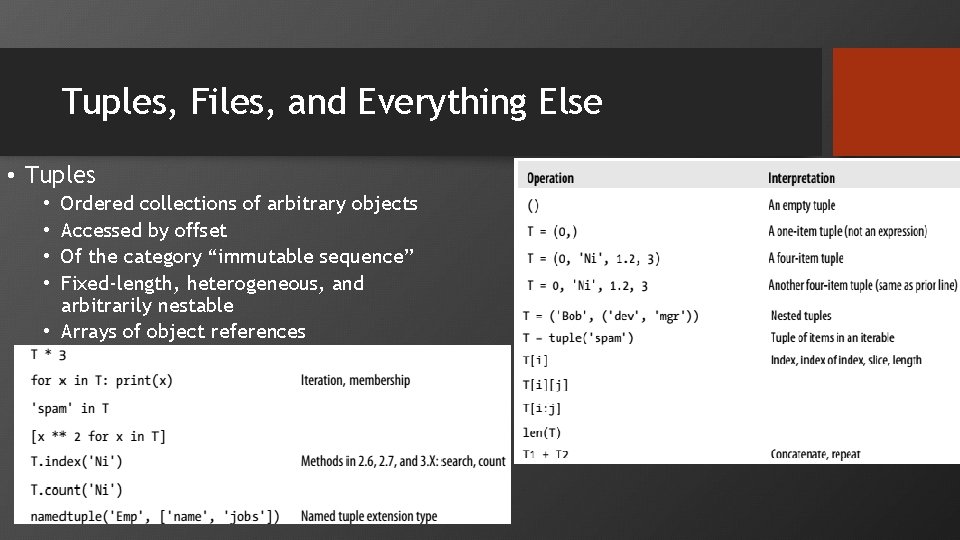
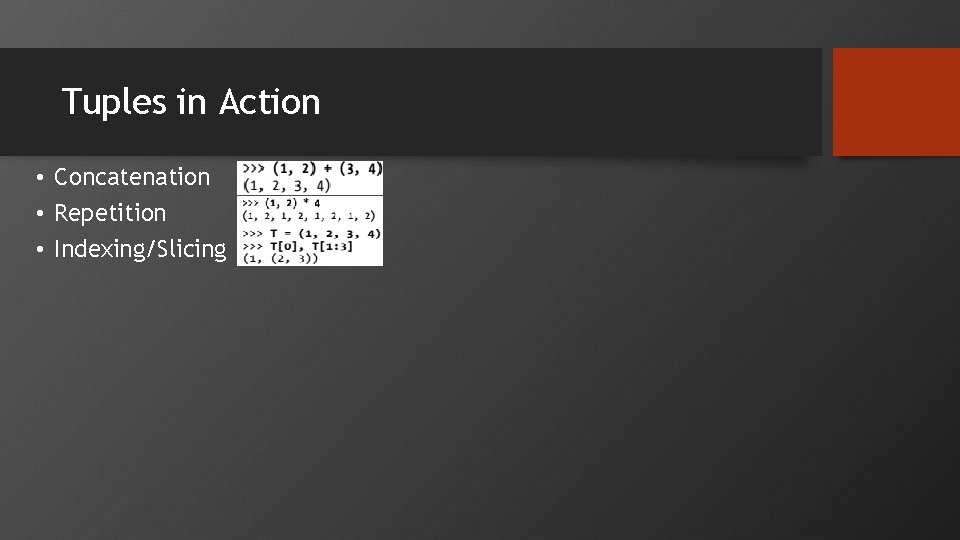
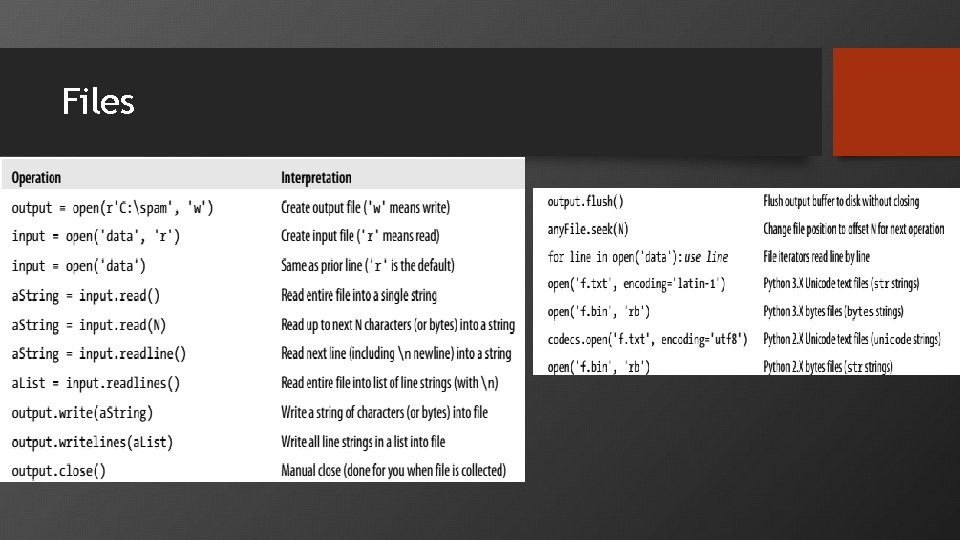
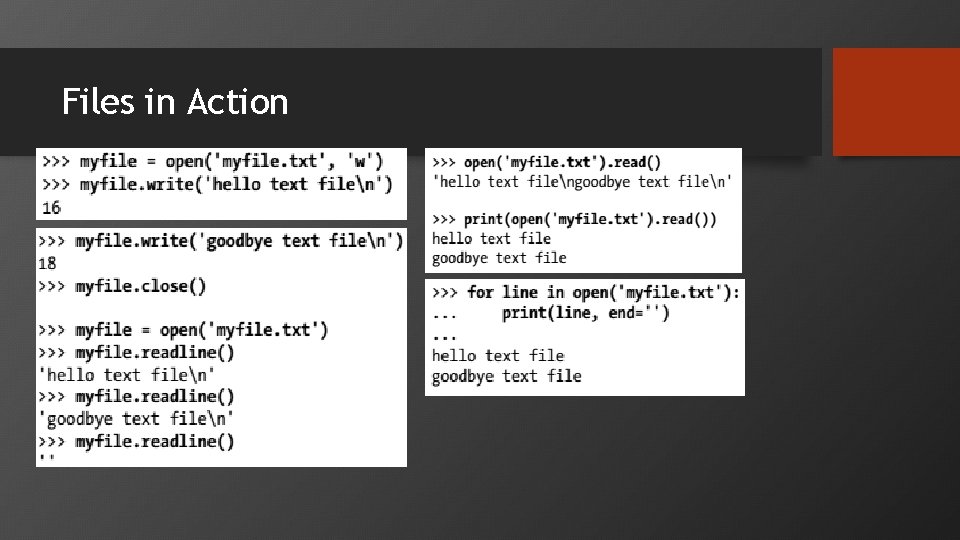
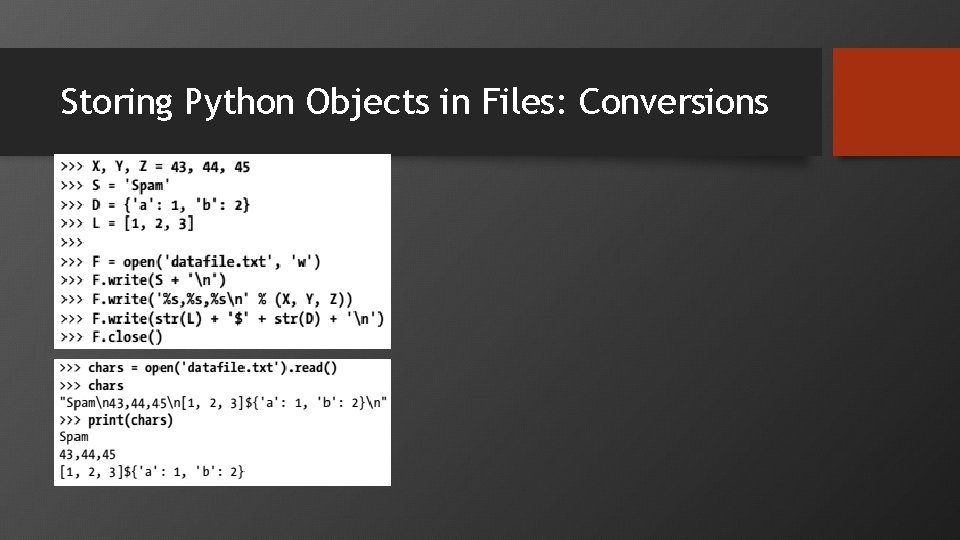
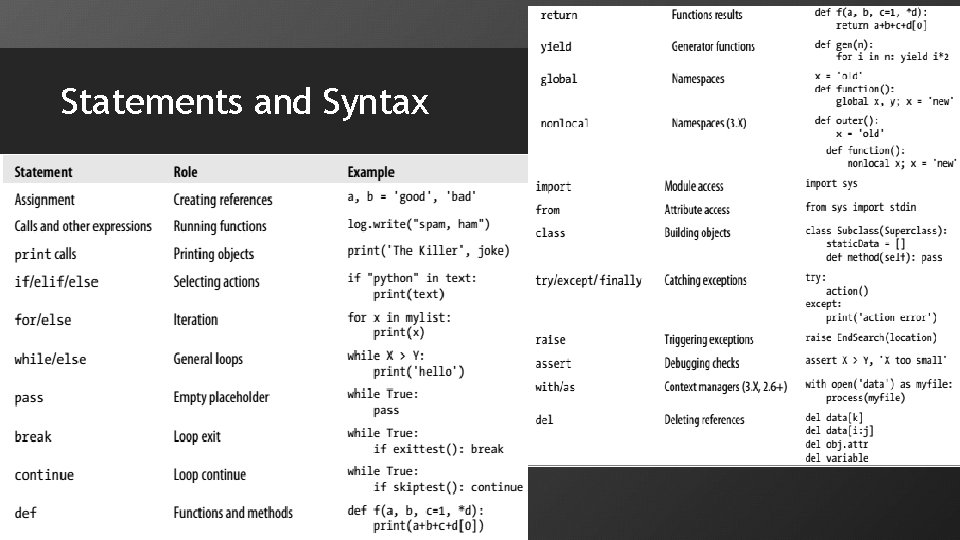
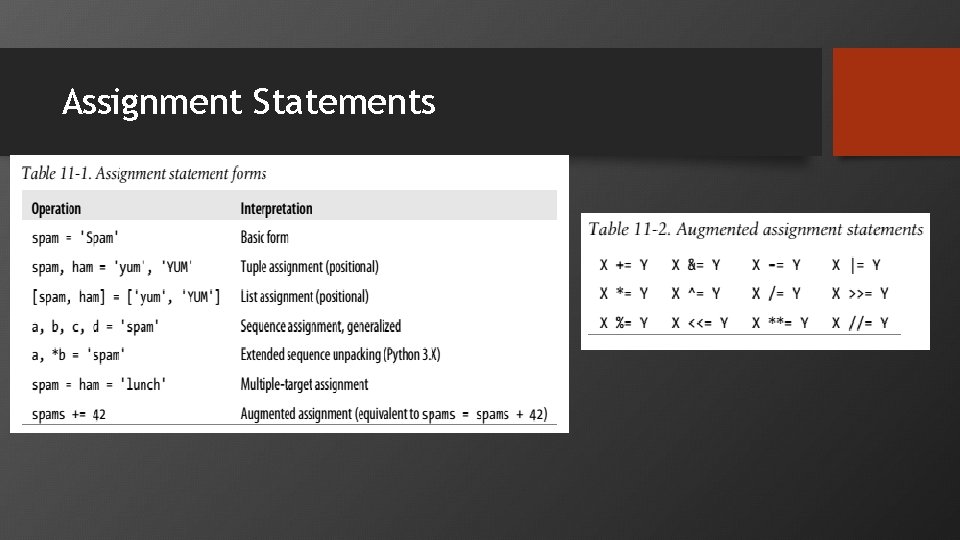
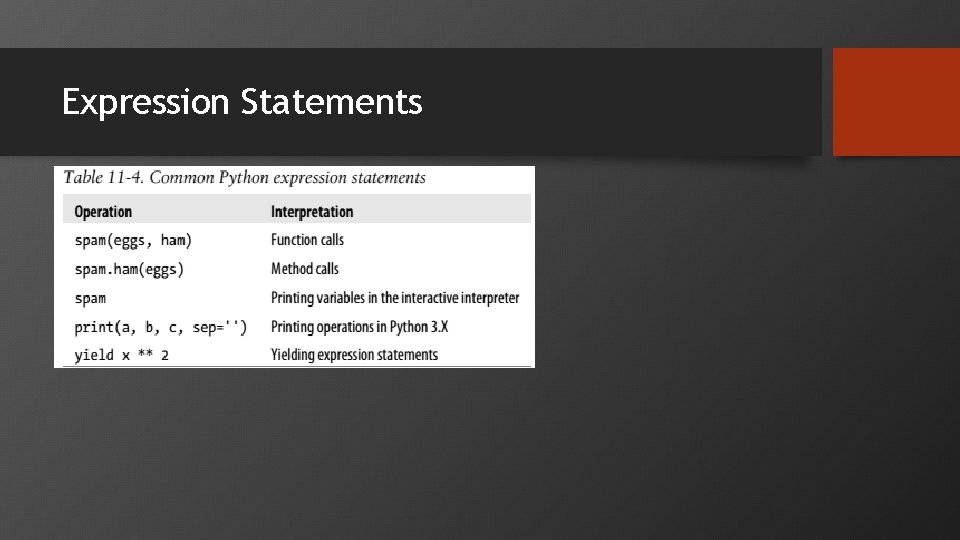
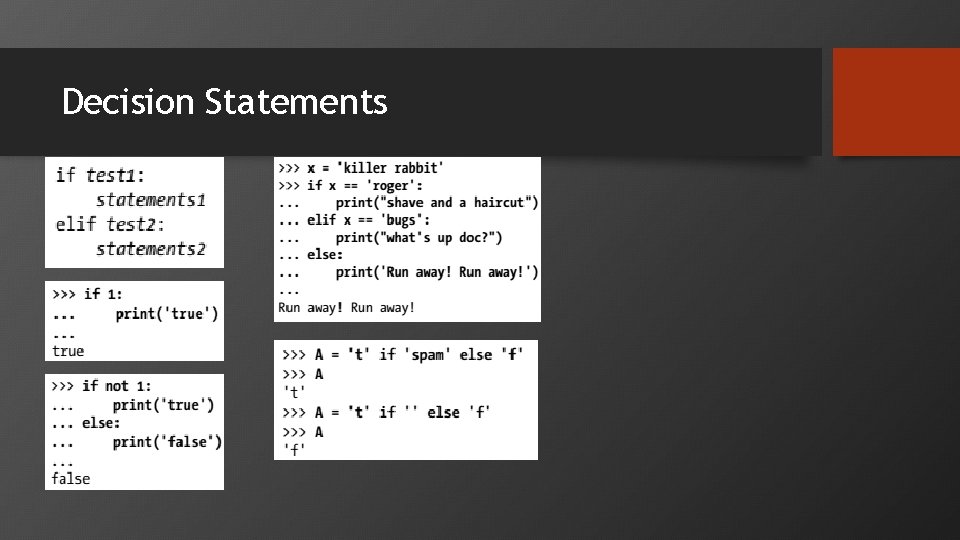
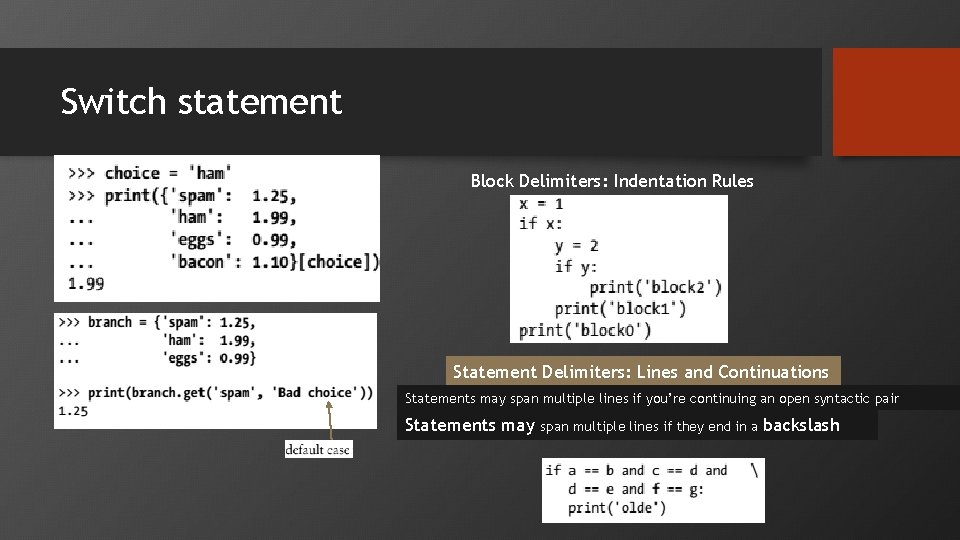
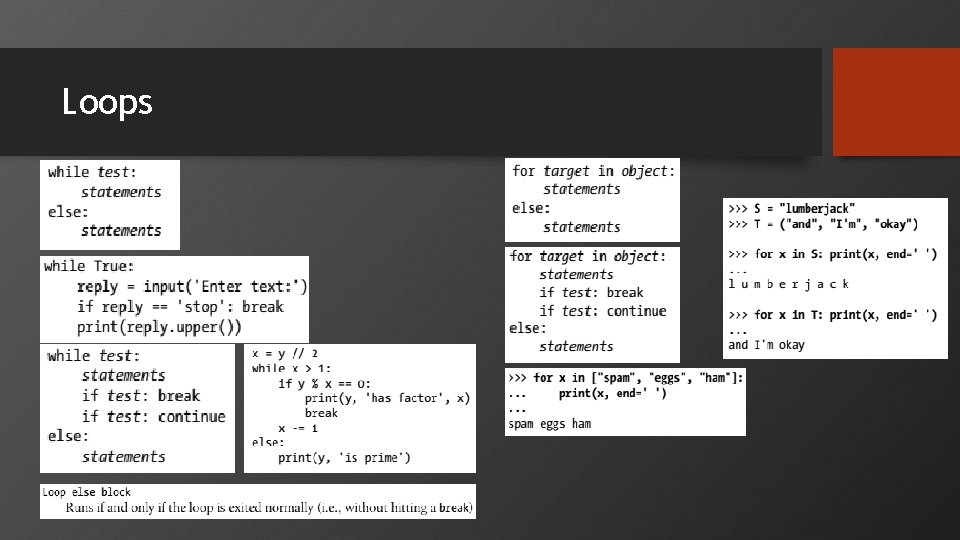
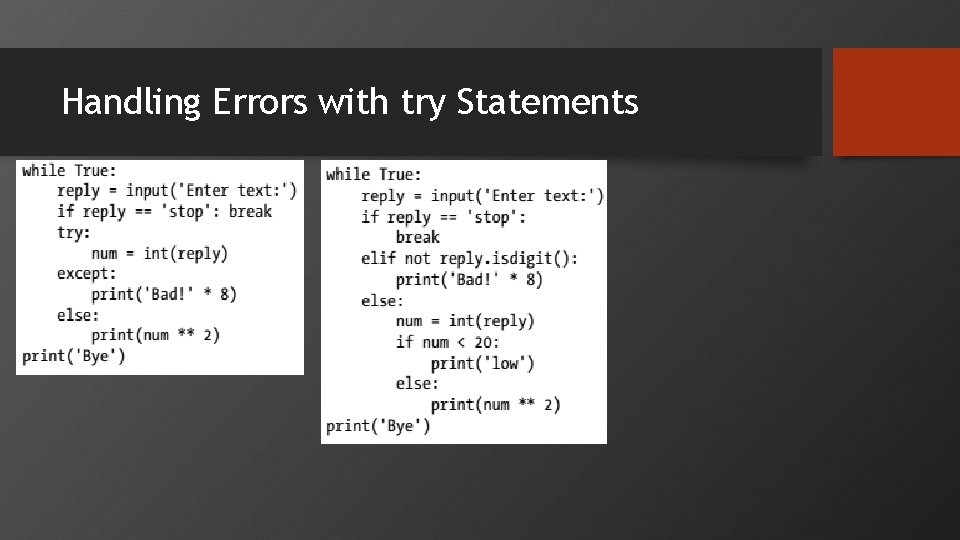
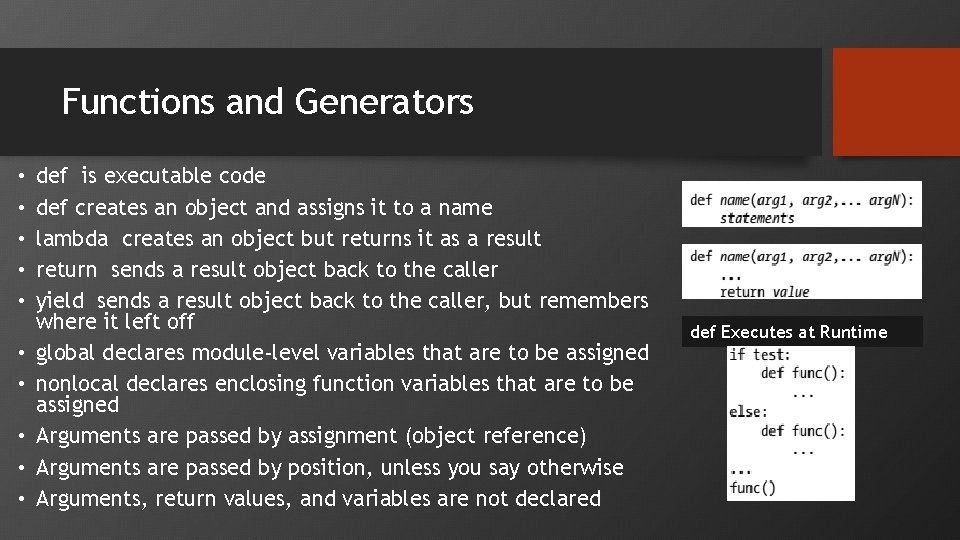
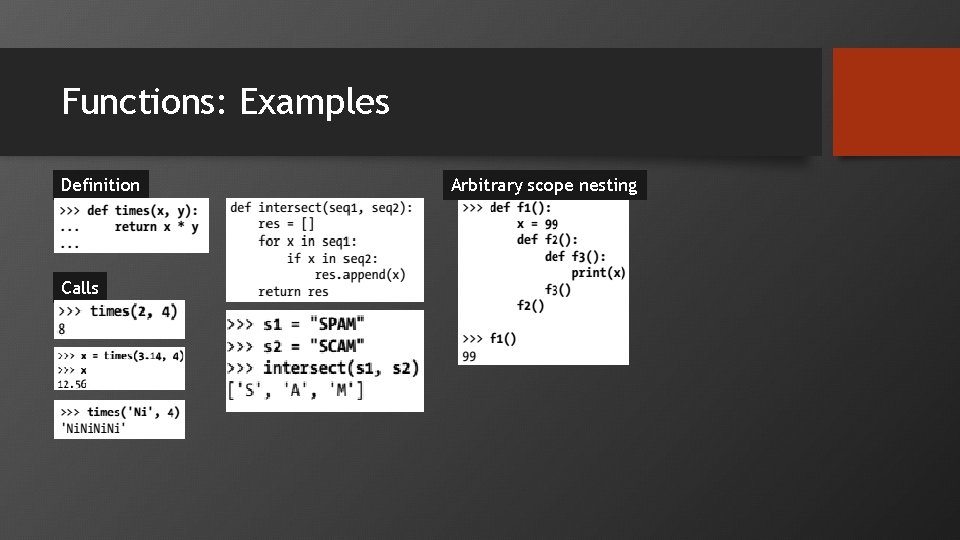
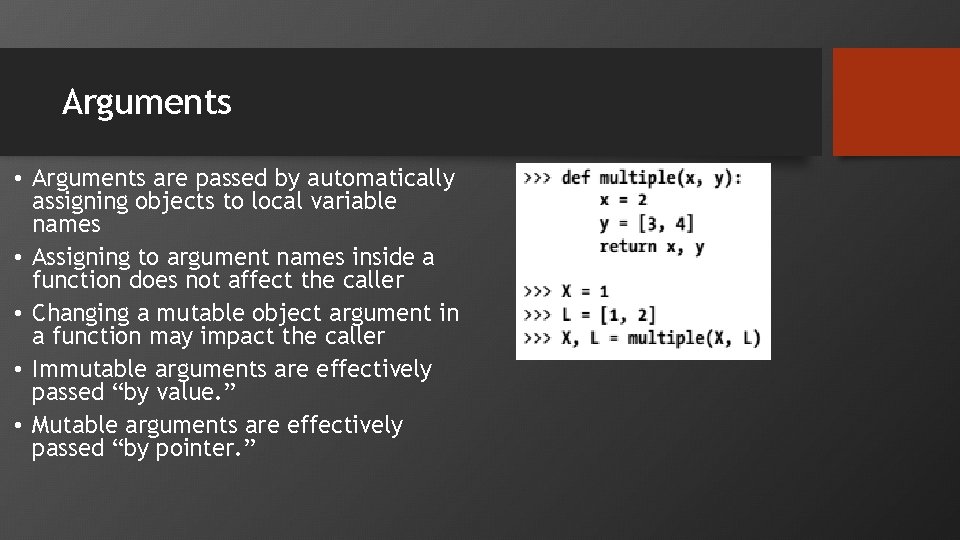
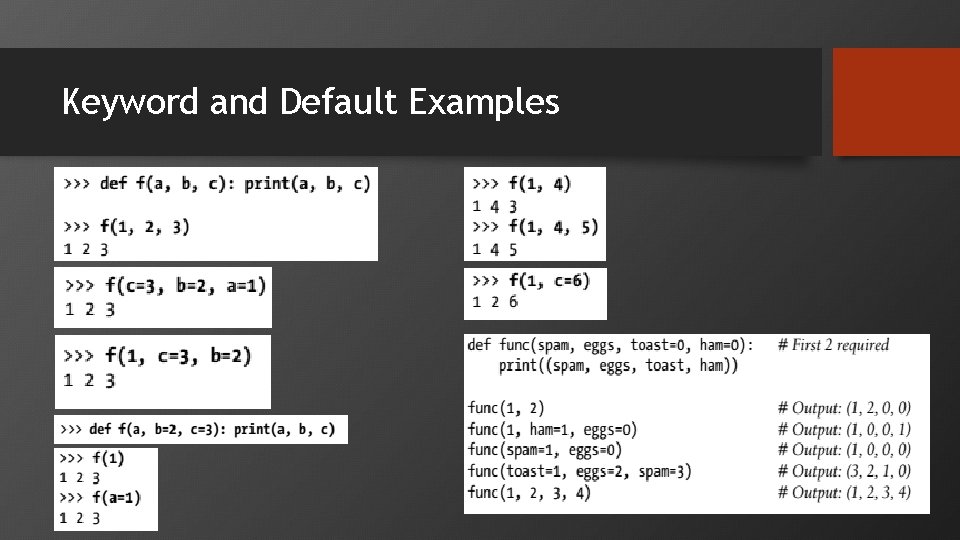
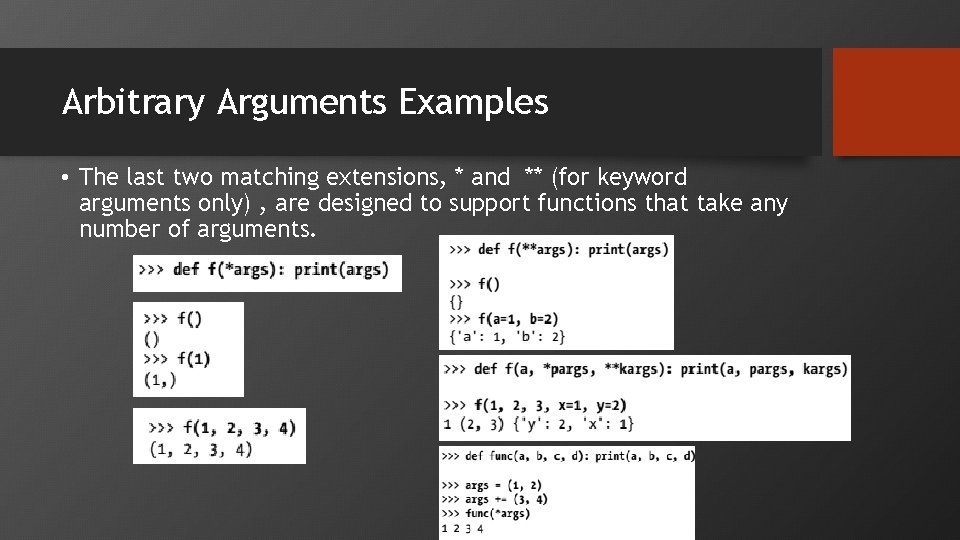
- Slides: 48
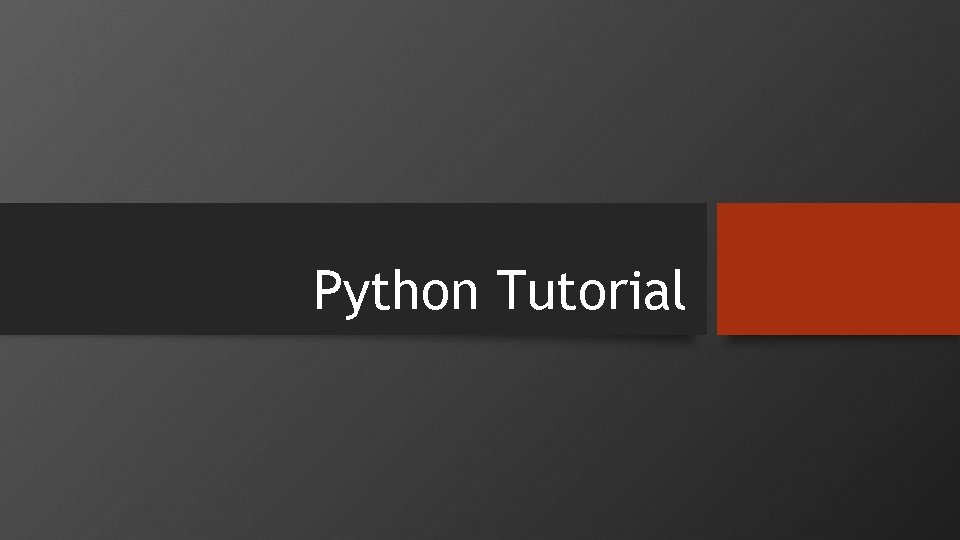
Python Tutorial
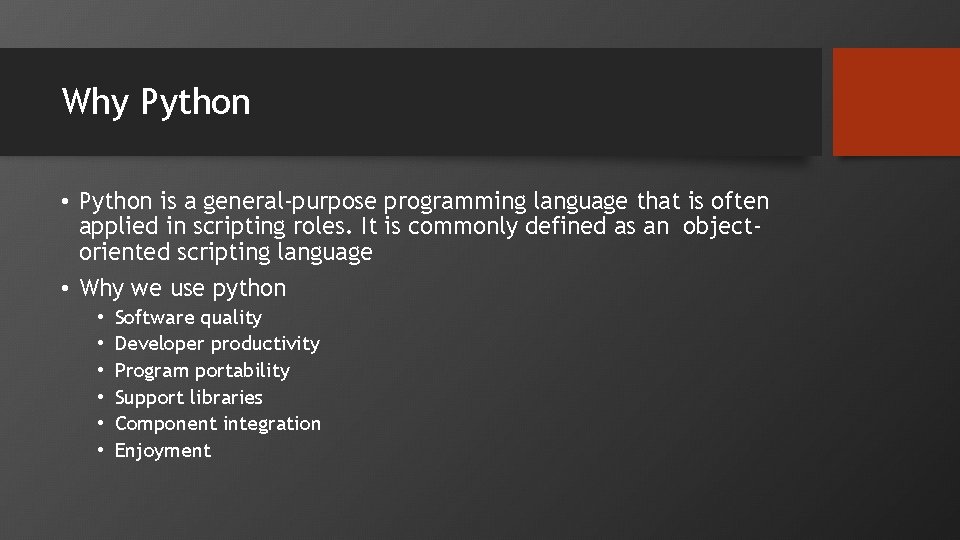
Why Python • Python is a general-purpose programming language that is often applied in scripting roles. It is commonly defined as an objectoriented scripting language • Why we use python • • • Software quality Developer productivity Program portability Support libraries Component integration Enjoyment
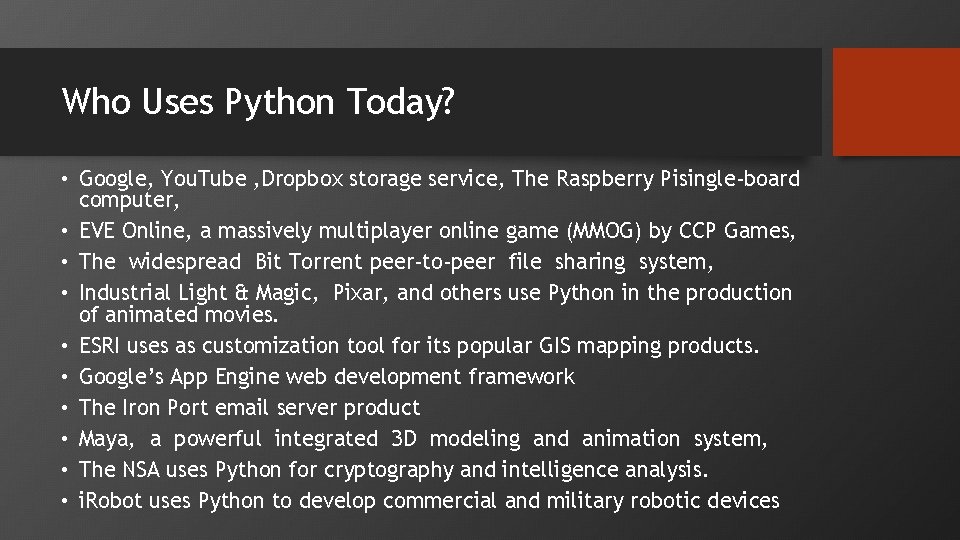
Who Uses Python Today? • Google, You. Tube , Dropbox storage service, The Raspberry Pisingle-board computer, • EVE Online, a massively multiplayer online game (MMOG) by CCP Games, • The widespread Bit Torrent peer-to-peer file sharing system, • Industrial Light & Magic, Pixar, and others use Python in the production of animated movies. • ESRI uses as customization tool for its popular GIS mapping products. • Google’s App Engine web development framework • The Iron Port email server product • Maya, a powerful integrated 3 D modeling and animation system, • The NSA uses Python for cryptography and intelligence analysis. • i. Robot uses Python to develop commercial and military robotic devices
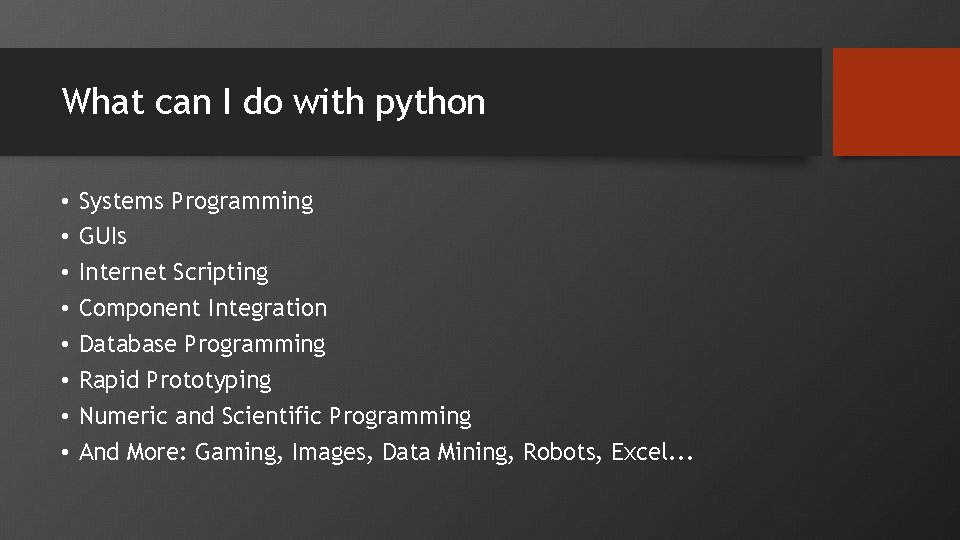
What can I do with python • • Systems Programming GUIs Internet Scripting Component Integration Database Programming Rapid Prototyping Numeric and Scientific Programming And More: Gaming, Images, Data Mining, Robots, Excel. . .
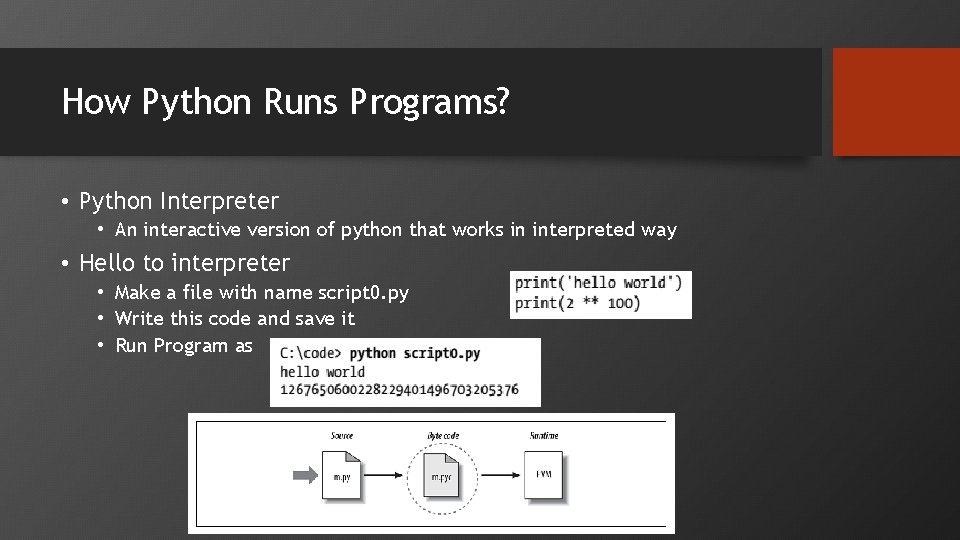
How Python Runs Programs? • Python Interpreter • An interactive version of python that works in interpreted way • Hello to interpreter • Make a file with name script 0. py • Write this code and save it • Run Program as
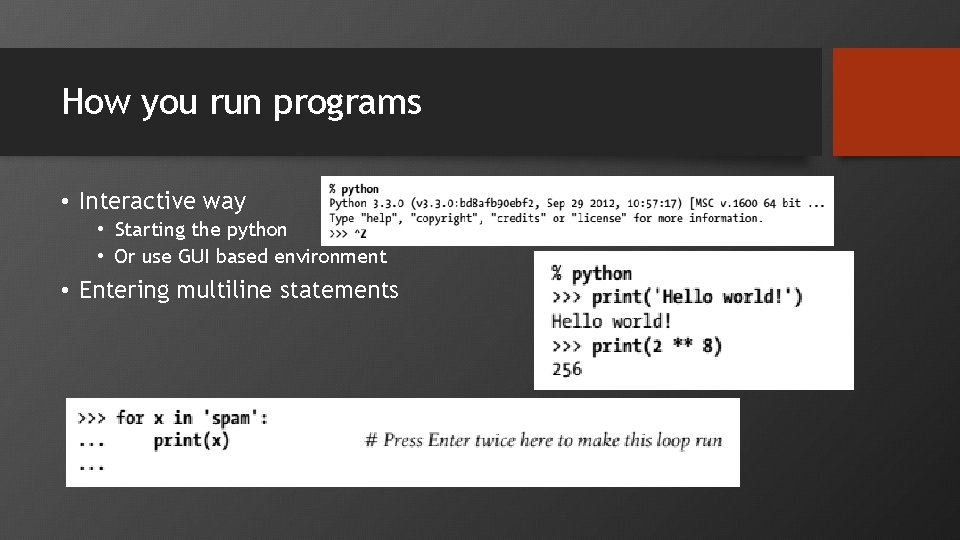
How you run programs • Interactive way • Starting the python • Or use GUI based environment • Entering multiline statements
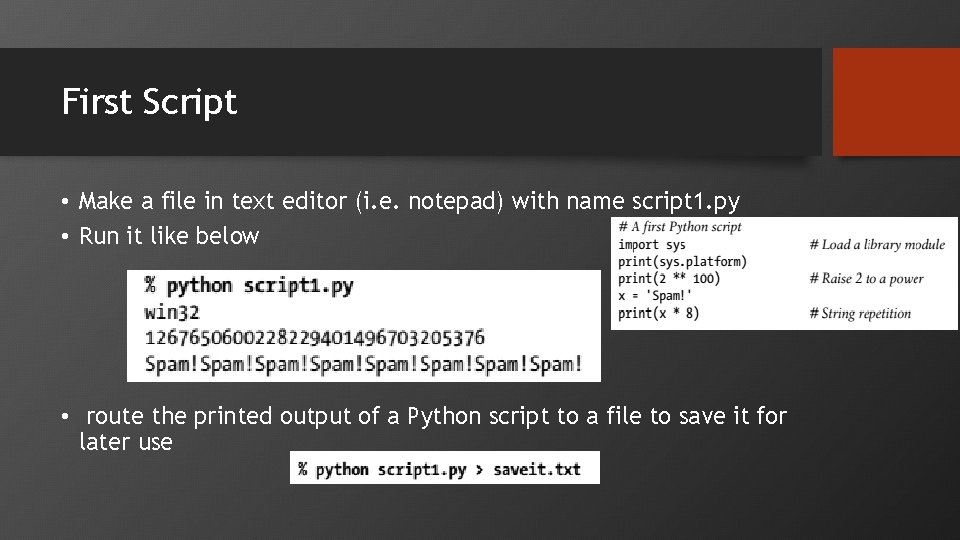
First Script • Make a file in text editor (i. e. notepad) with name script 1. py • Run it like below • route the printed output of a Python script to a file to save it for later use
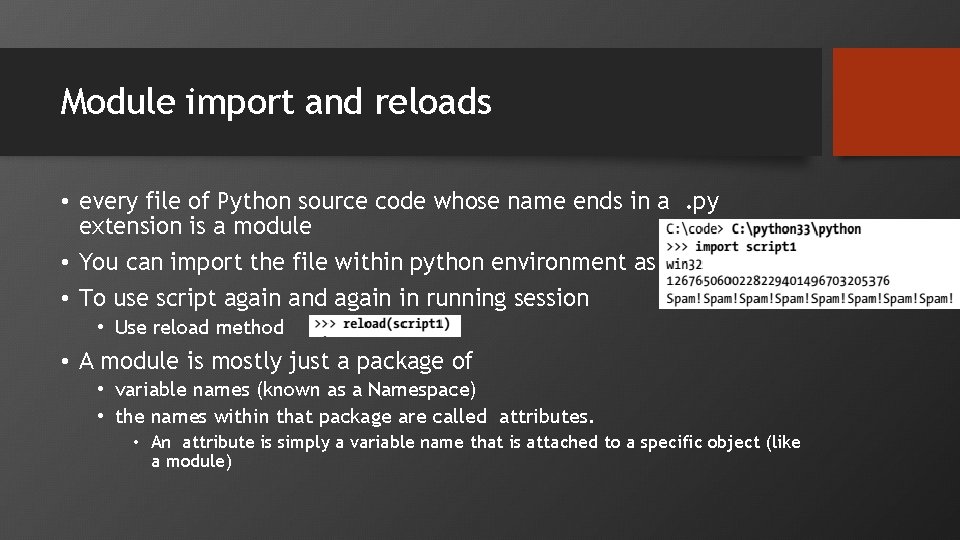
Module import and reloads • every file of Python source code whose name ends in a. py extension is a module • You can import the file within python environment as • To use script again and again in running session • Use reload method • A module is mostly just a package of • variable names (known as a Namespace) • the names within that package are called attributes. • An attribute is simply a variable name that is attached to a specific object (like a module)
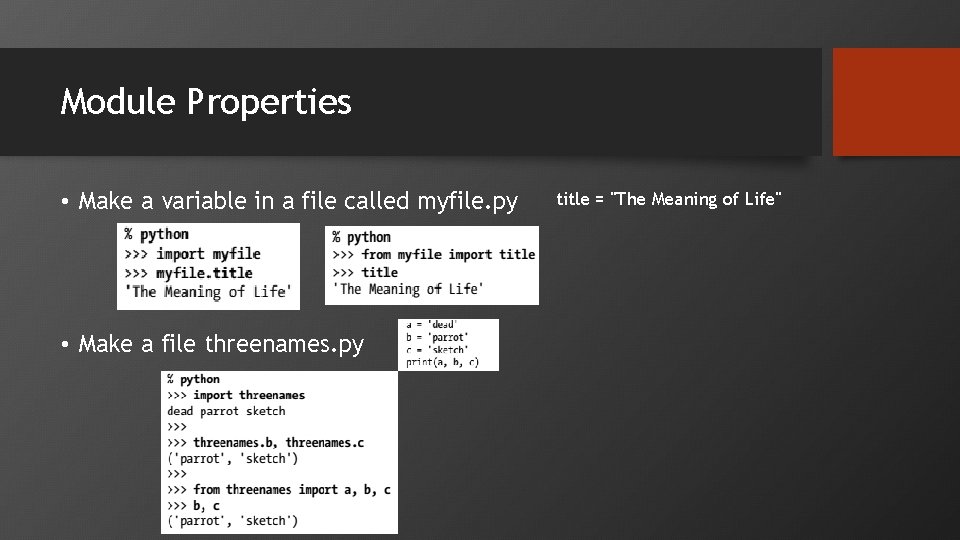
Module Properties • Make a variable in a file called myfile. py • Make a file threenames. py title = "The Meaning of Life"
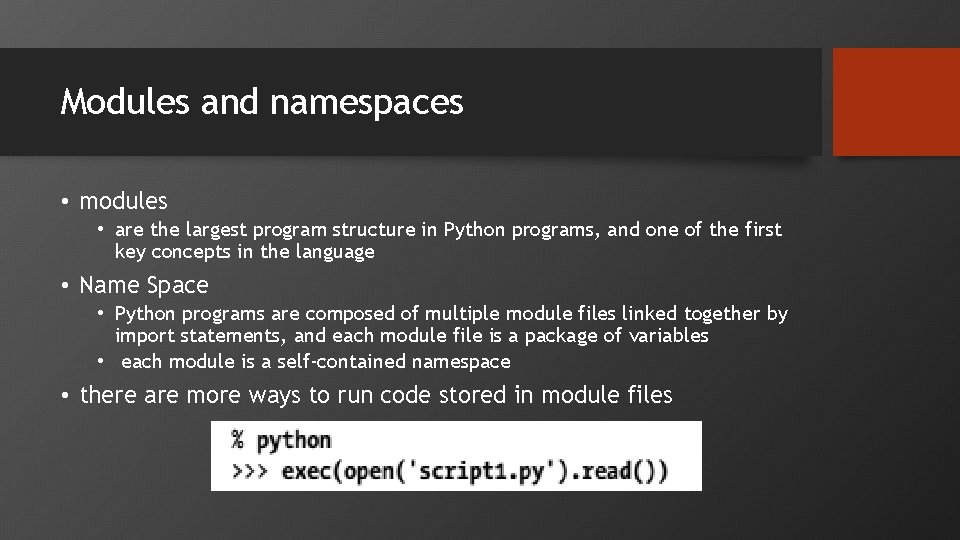
Modules and namespaces • modules • are the largest program structure in Python programs, and one of the first key concepts in the language • Name Space • Python programs are composed of multiple module files linked together by import statements, and each module file is a package of variables • each module is a self-contained namespace • there are more ways to run code stored in module files
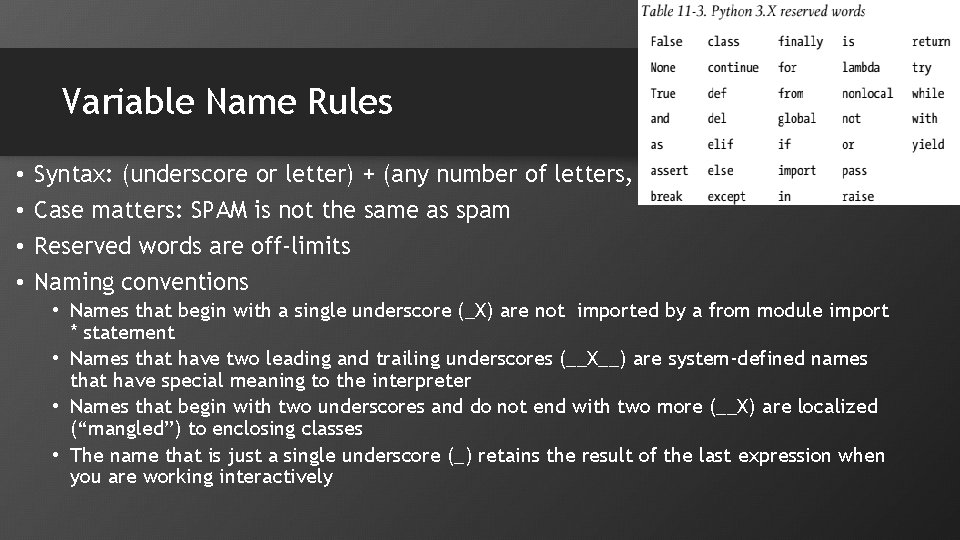
Variable Name Rules • • Syntax: (underscore or letter) + (any number of letters, digits, or underscores) Case matters: SPAM is not the same as spam Reserved words are off-limits Naming conventions • Names that begin with a single underscore (_X) are not imported by a from module import * statement • Names that have two leading and trailing underscores (__X__) are system-defined names that have special meaning to the interpreter • Names that begin with two underscores and do not end with two more (__X) are localized (“mangled”) to enclosing classes • The name that is just a single underscore (_) retains the result of the last expression when you are working interactively
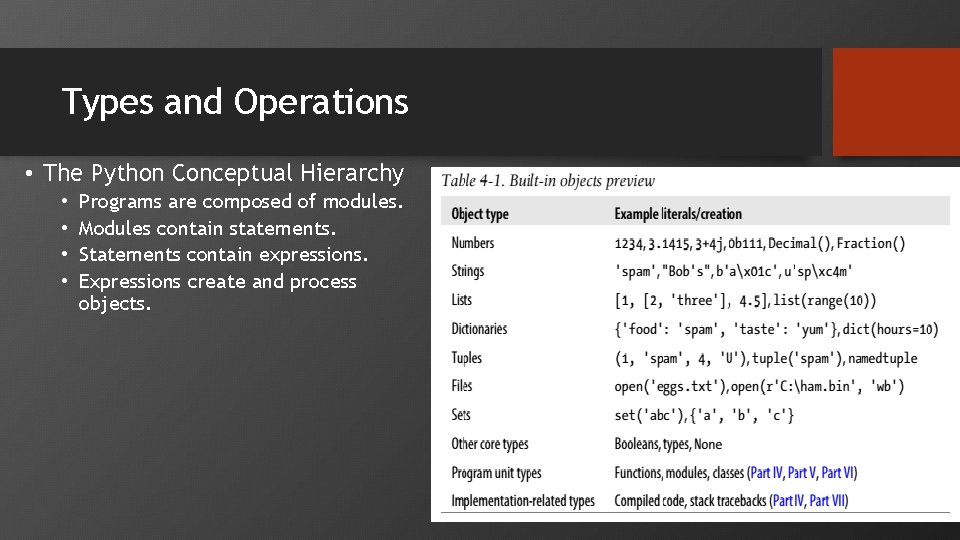
Types and Operations • The Python Conceptual Hierarchy • • Programs are composed of modules. Modules contain statements. Statements contain expressions. Expressions create and process objects.
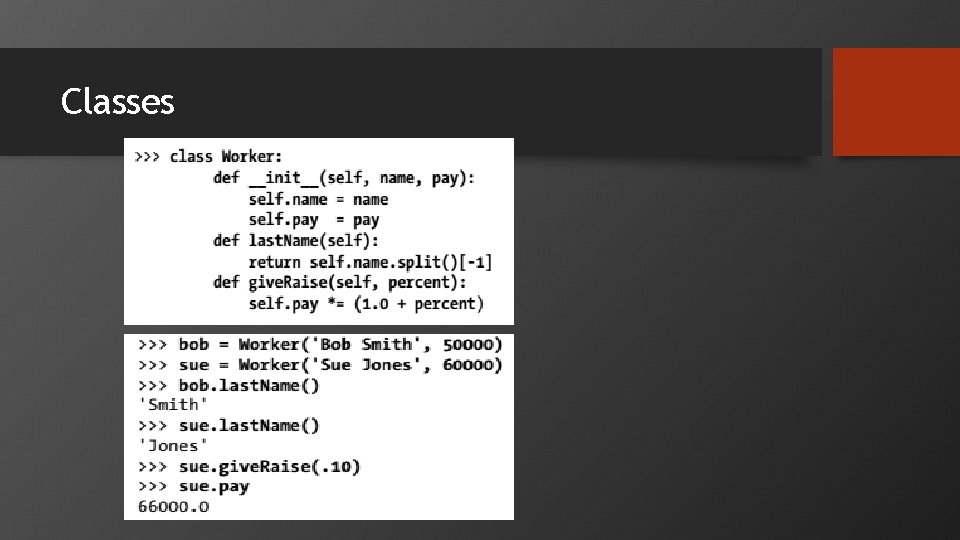
Classes
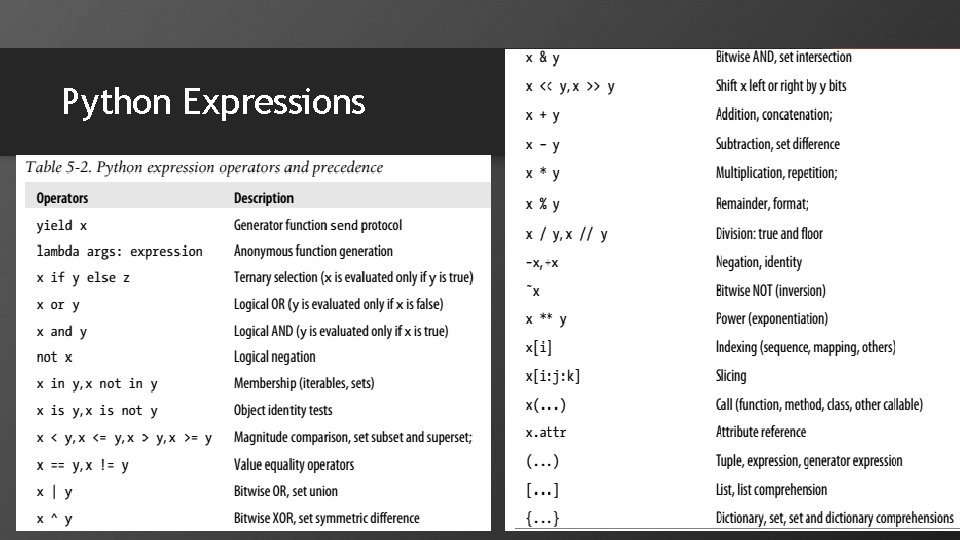
Python Expressions
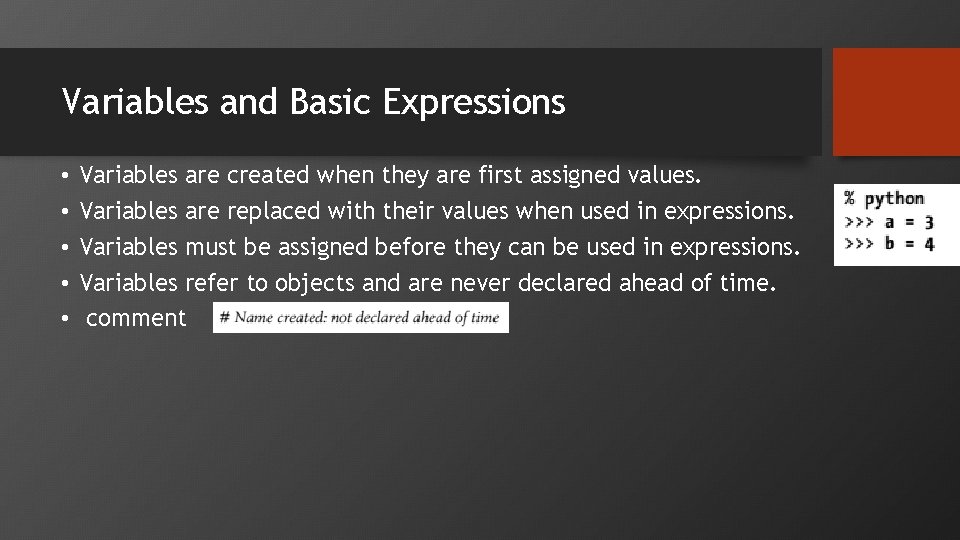
Variables and Basic Expressions • • • Variables are created when they are first assigned values. Variables are replaced with their values when used in expressions. Variables must be assigned before they can be used in expressions. Variables refer to objects and are never declared ahead of time. comment
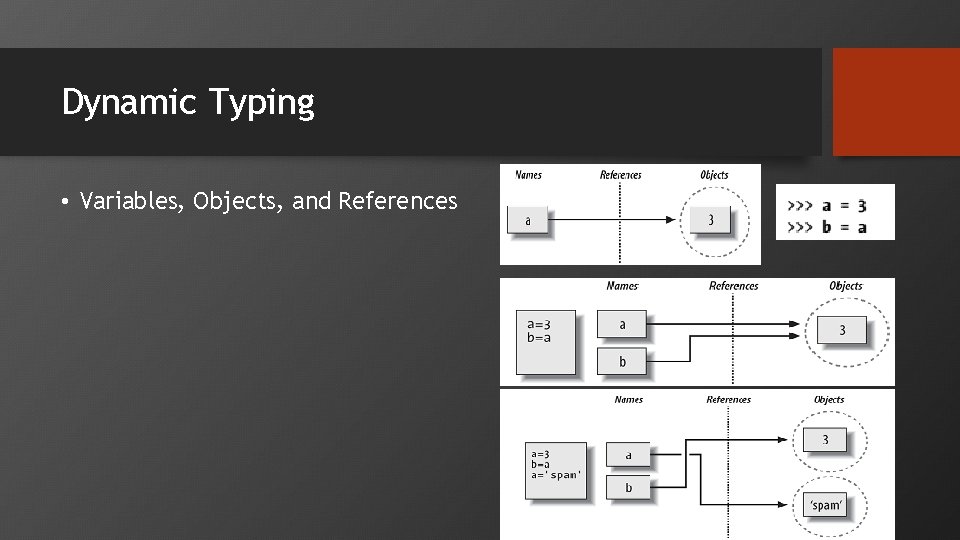
Dynamic Typing • Variables, Objects, and References
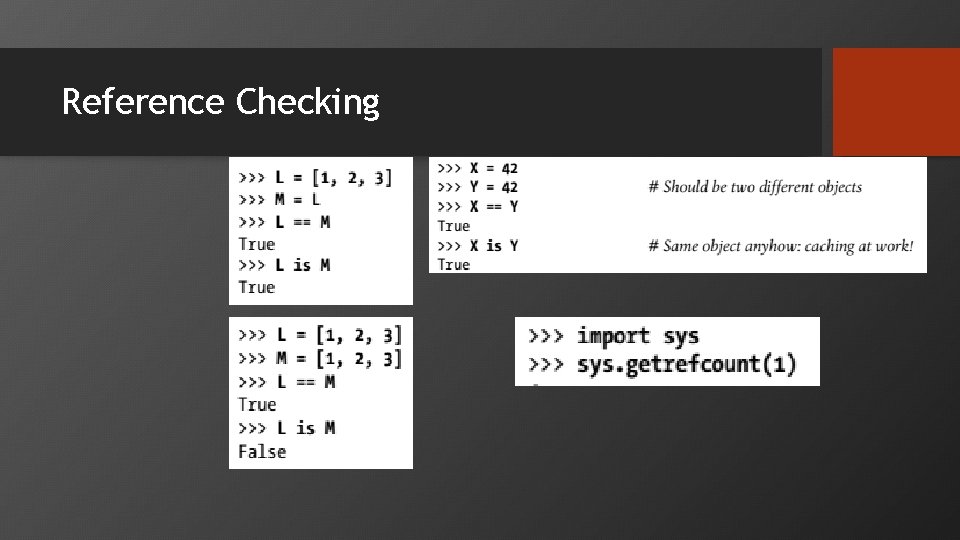
Reference Checking
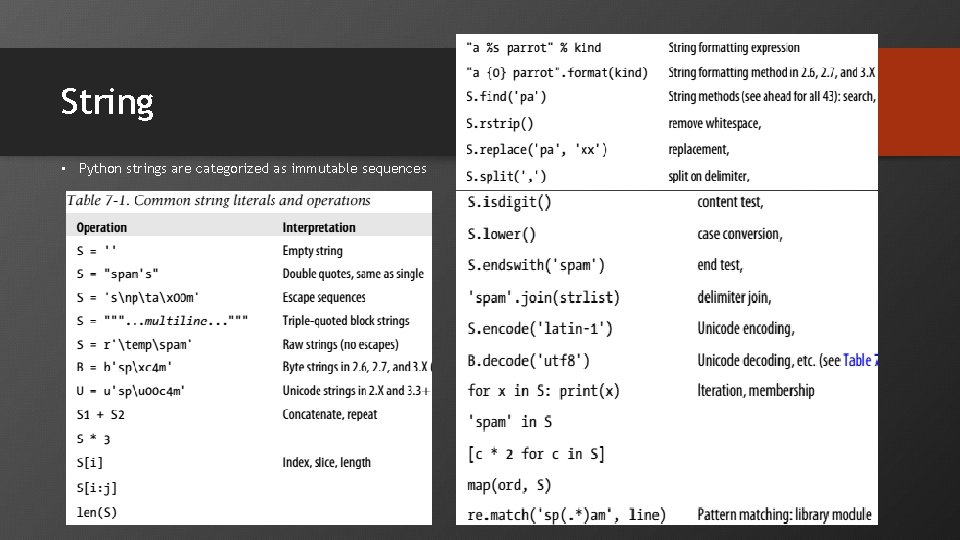
String • Python strings are categorized as immutable sequences
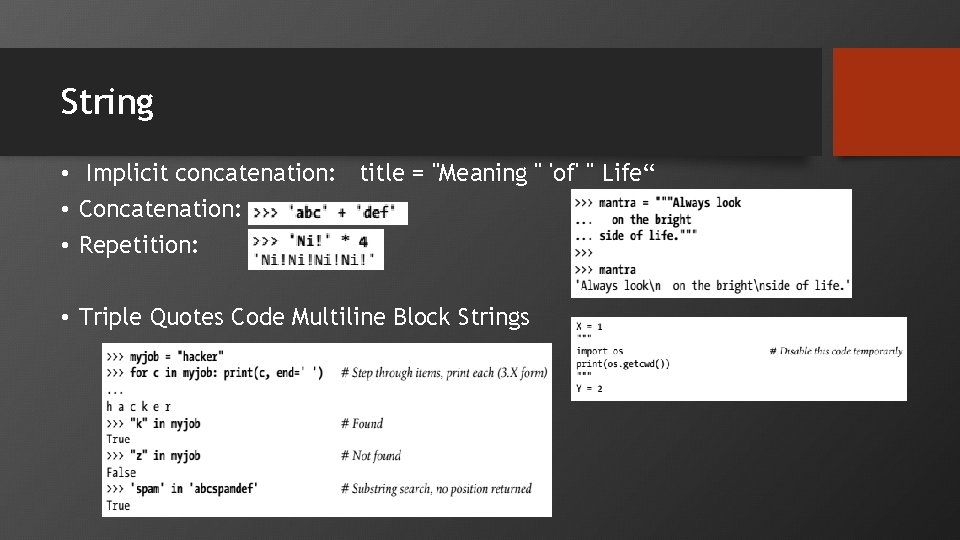
String • Implicit concatenation: title = "Meaning " 'of' " Life“ • Concatenation: • Repetition: • Triple Quotes Code Multiline Block Strings
![String Indexing and Slicing X1 10 2 will fetch every other item String Indexing and Slicing • X[1: 10: 2] • will fetch every other item](https://slidetodoc.com/presentation_image_h2/b83870a8ed120330c0c40eeb3521b05c/image-20.jpg)
String Indexing and Slicing • X[1: 10: 2] • will fetch every other item in X from offsets 1– 9 • Character code conversions
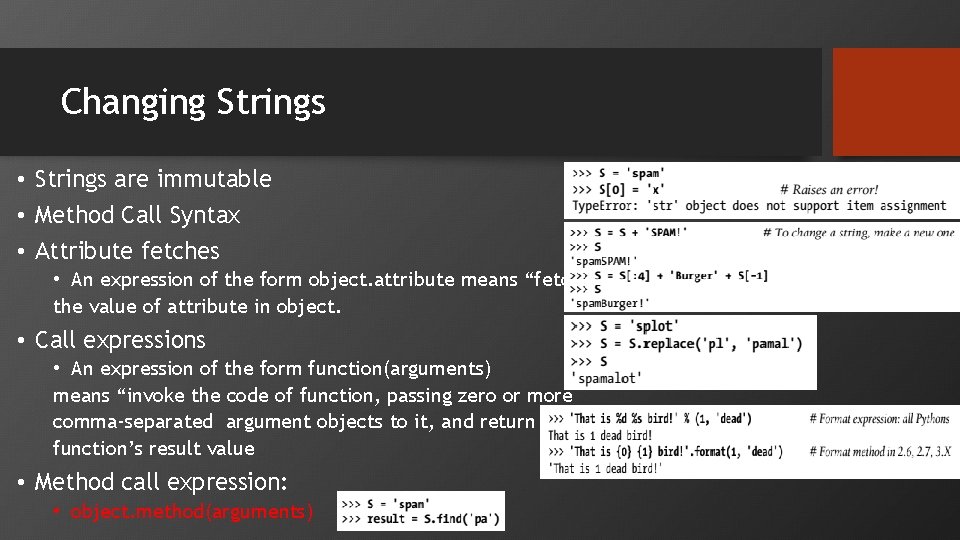
Changing Strings • Strings are immutable • Method Call Syntax • Attribute fetches • An expression of the form object. attribute means “fetch the value of attribute in object. • Call expressions • An expression of the form function(arguments) means “invoke the code of function, passing zero or more comma-separated argument objects to it, and return function’s result value • Method call expression: • object. method(arguments)
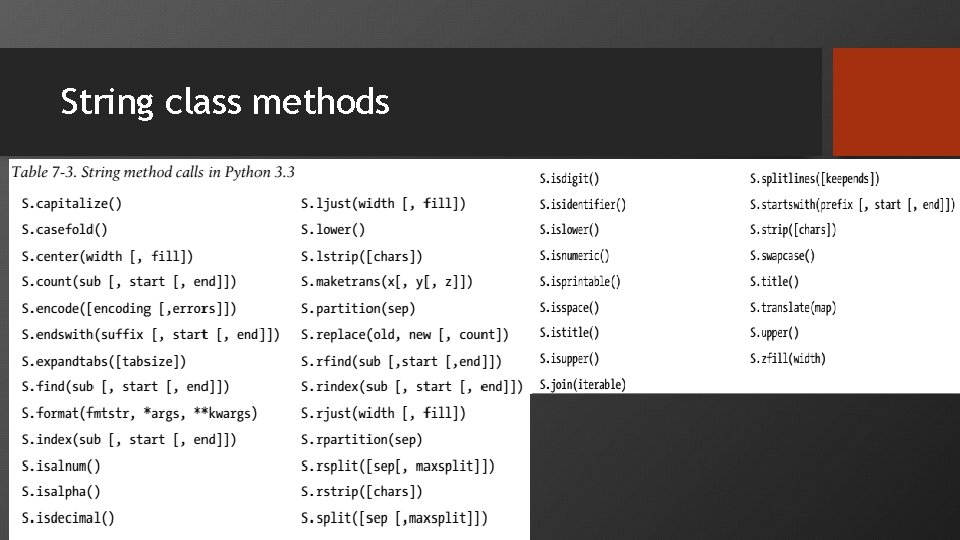
String class methods
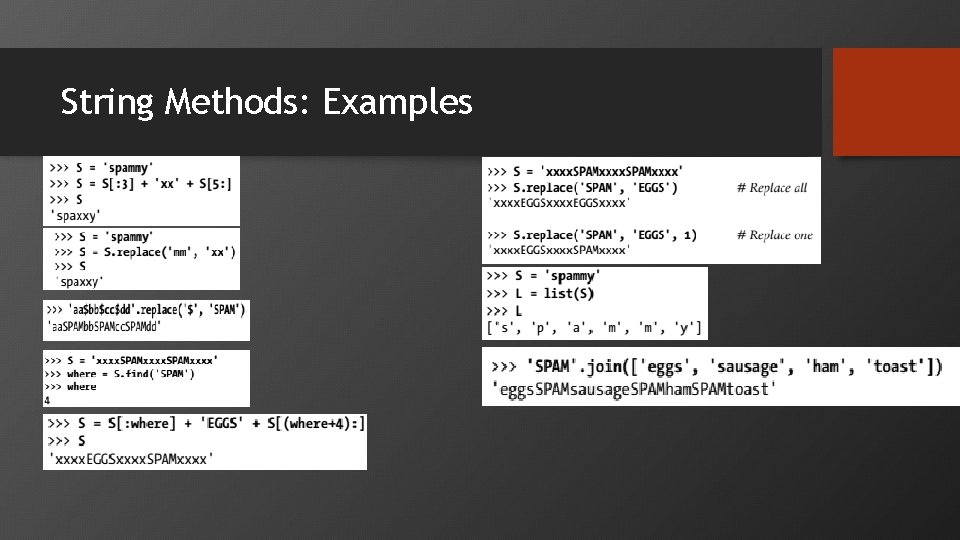
String Methods: Examples
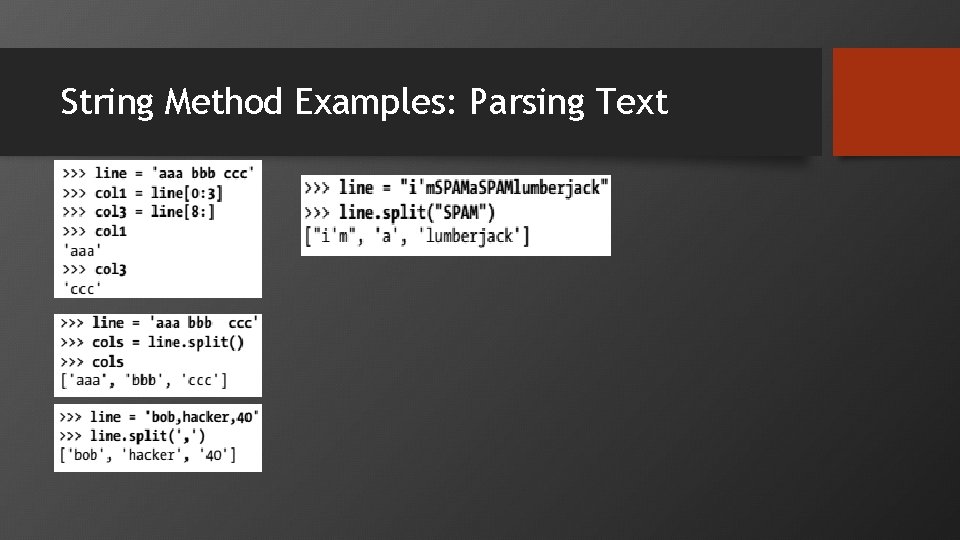
String Method Examples: Parsing Text
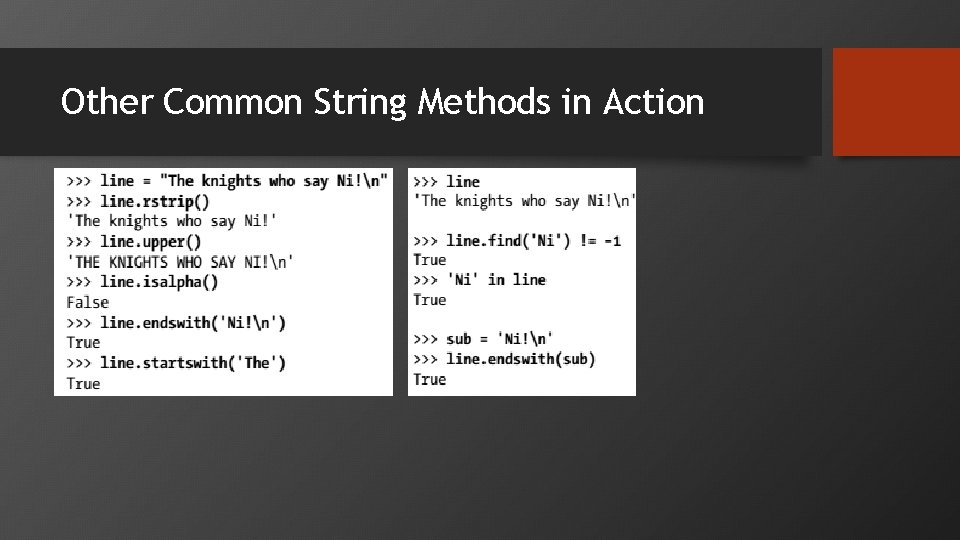
Other Common String Methods in Action
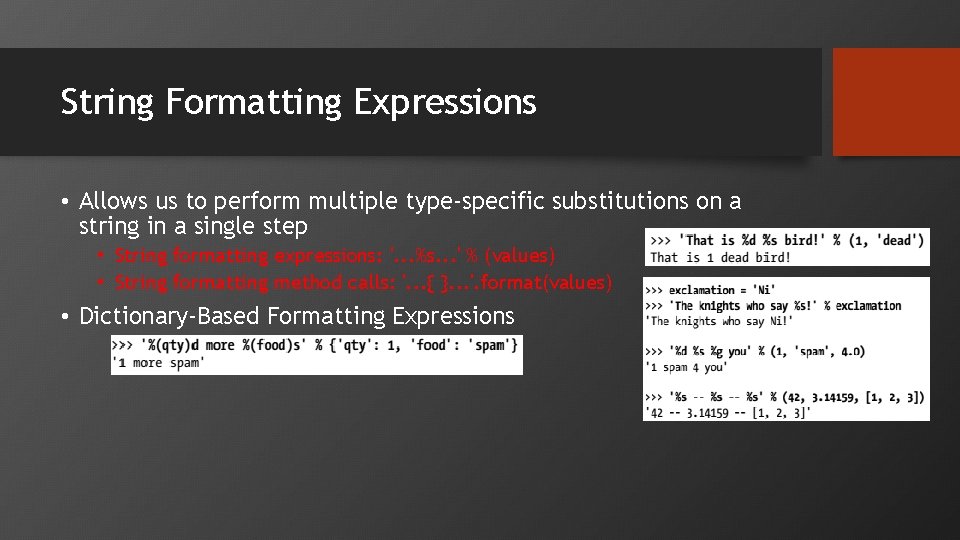
String Formatting Expressions • Allows us to perform multiple type-specific substitutions on a string in a single step • String formatting expressions: '. . . %s. . . ' % (values) • String formatting method calls: '. . . { }. . . '. format(values) • Dictionary-Based Formatting Expressions
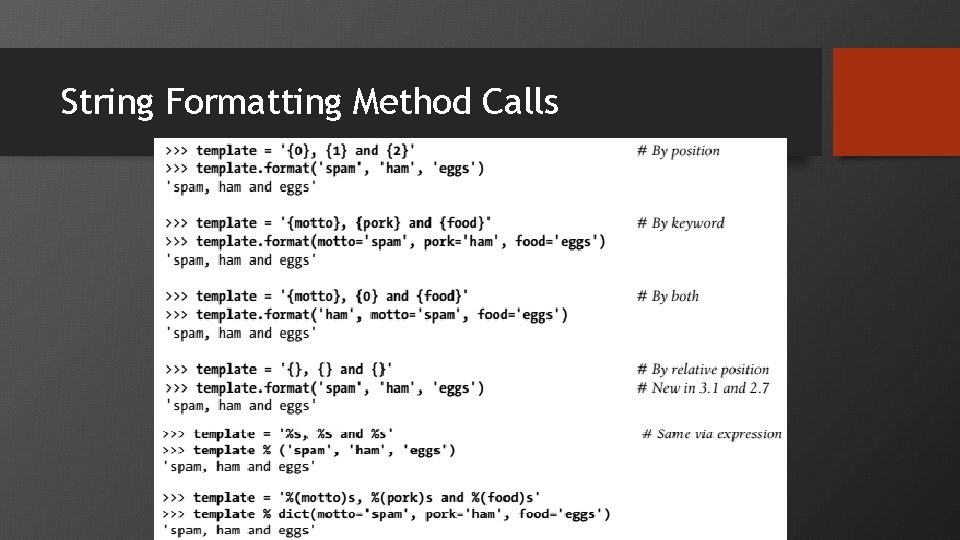
String Formatting Method Calls
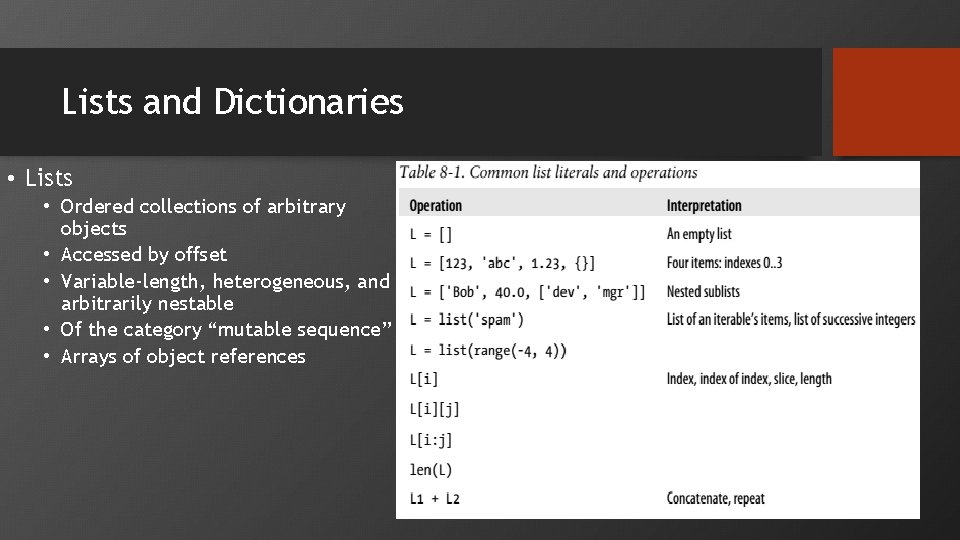
Lists and Dictionaries • Lists • Ordered collections of arbitrary objects • Accessed by offset • Variable-length, heterogeneous, and arbitrarily nestable • Of the category “mutable sequence” • Arrays of object references
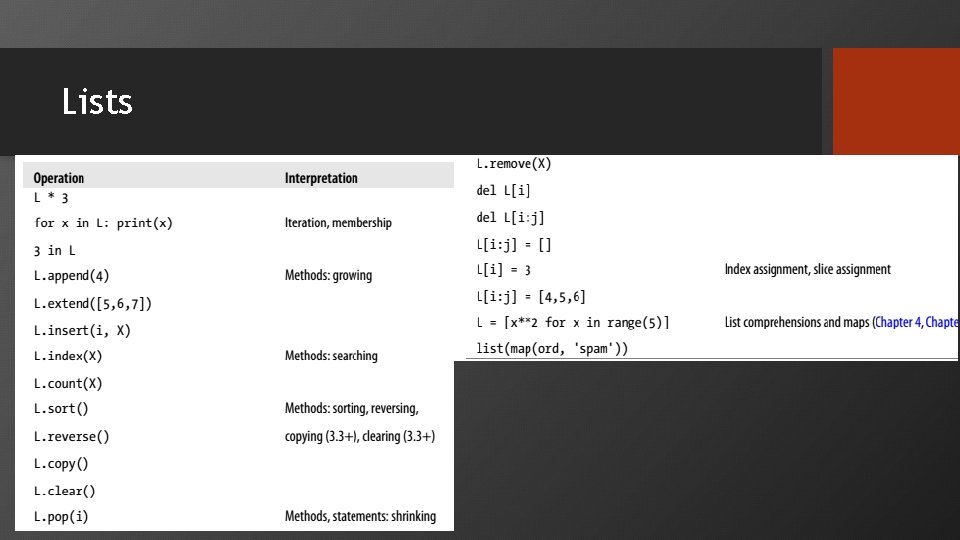
Lists
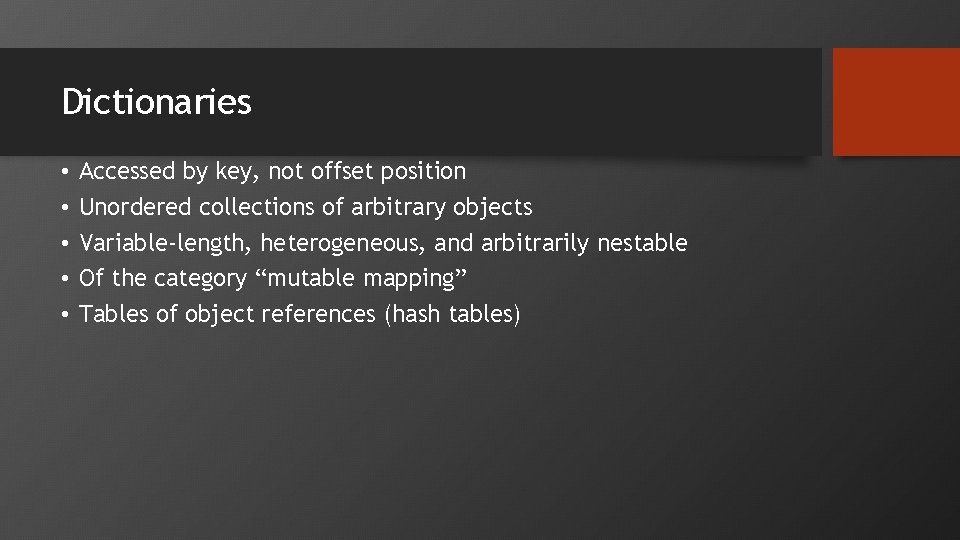
Dictionaries • • • Accessed by key, not offset position Unordered collections of arbitrary objects Variable-length, heterogeneous, and arbitrarily nestable Of the category “mutable mapping” Tables of object references (hash tables)
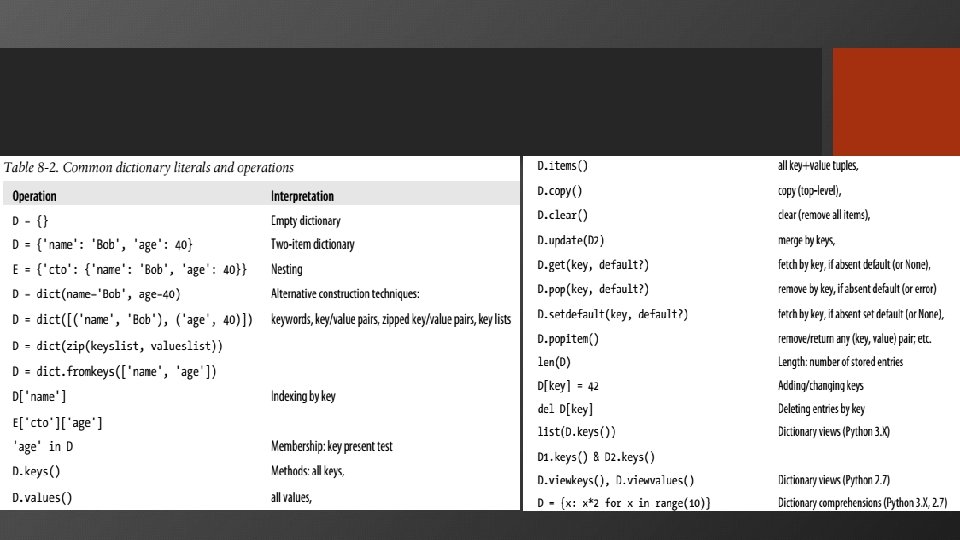
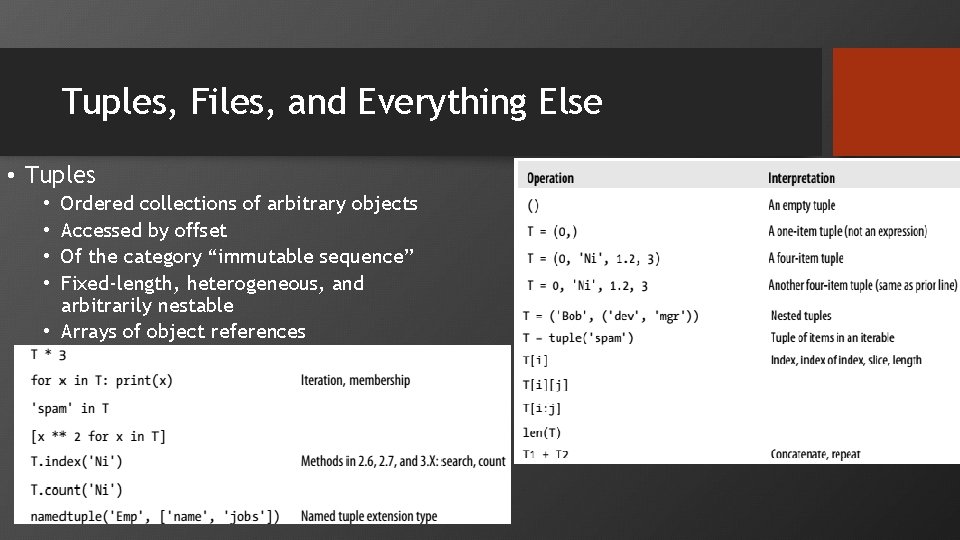
Tuples, Files, and Everything Else • Tuples Ordered collections of arbitrary objects Accessed by offset Of the category “immutable sequence” Fixed-length, heterogeneous, and arbitrarily nestable • Arrays of object references • •
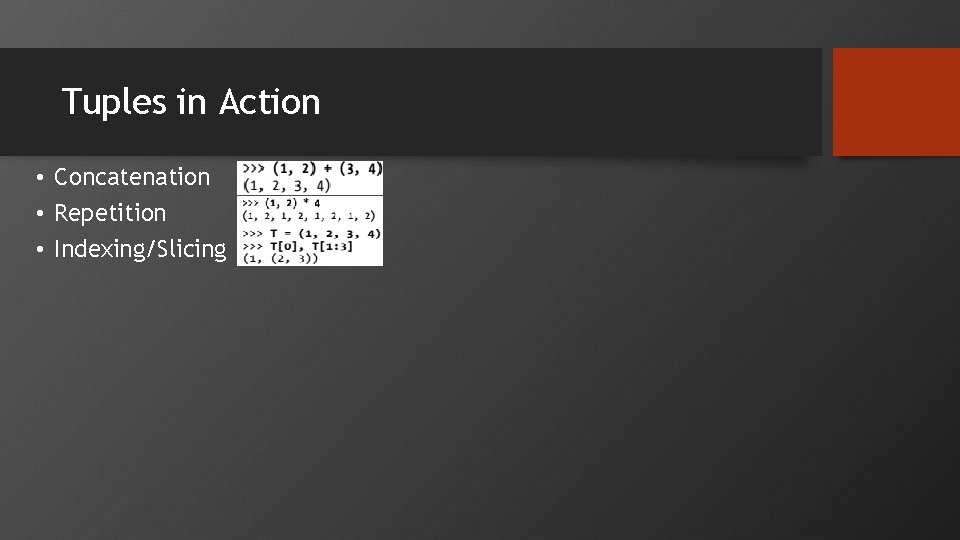
Tuples in Action • Concatenation • Repetition • Indexing/Slicing
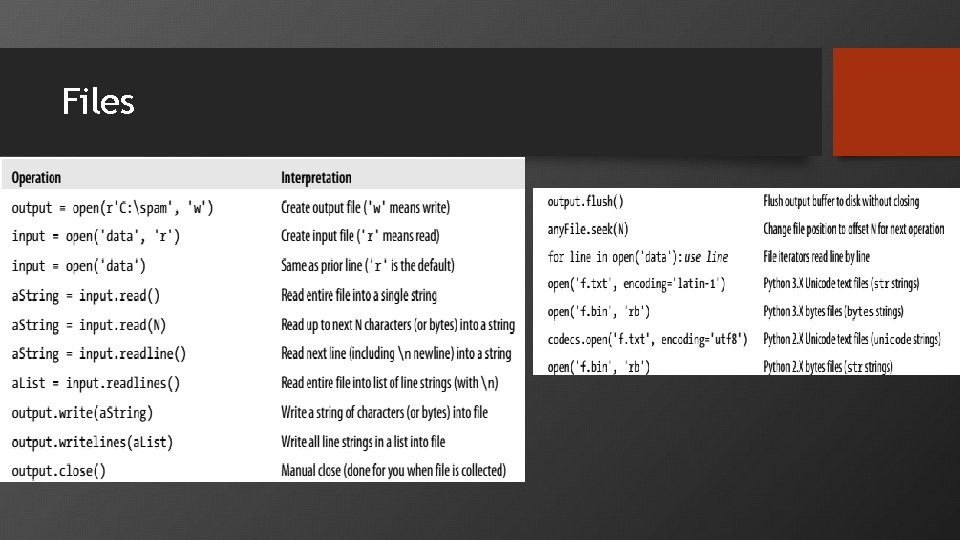
Files
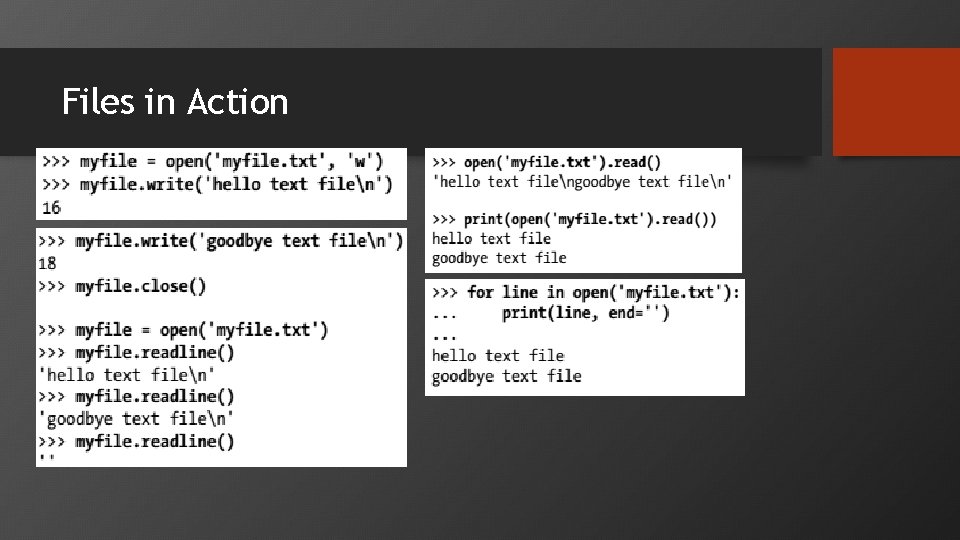
Files in Action
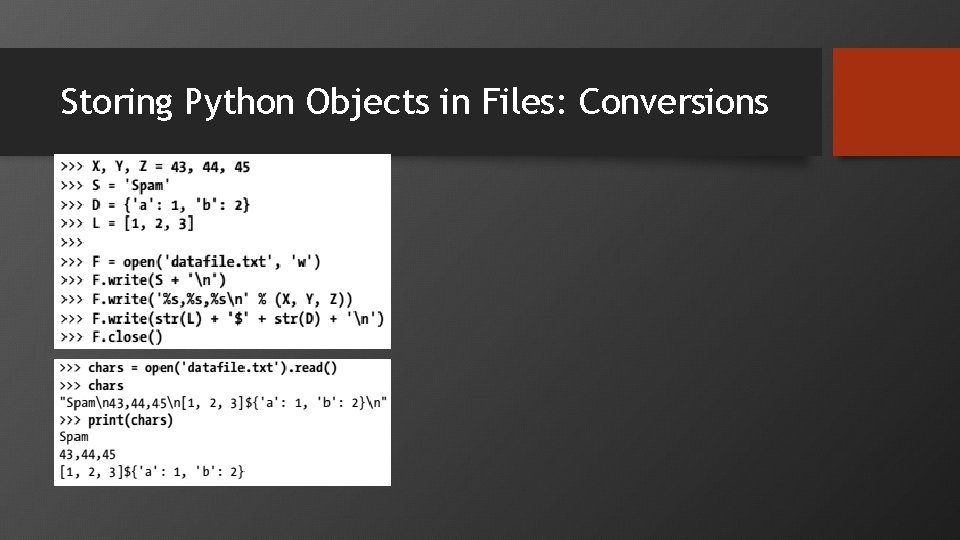
Storing Python Objects in Files: Conversions
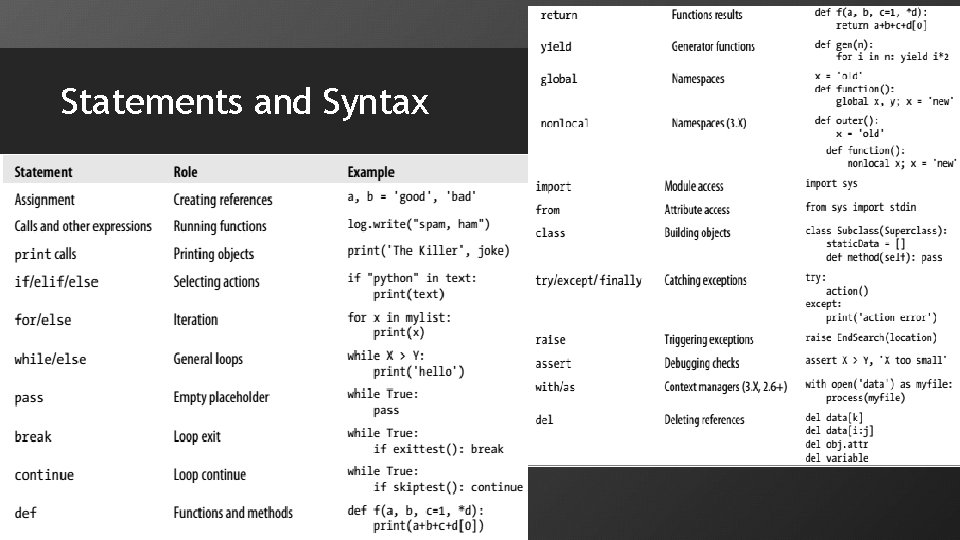
Statements and Syntax
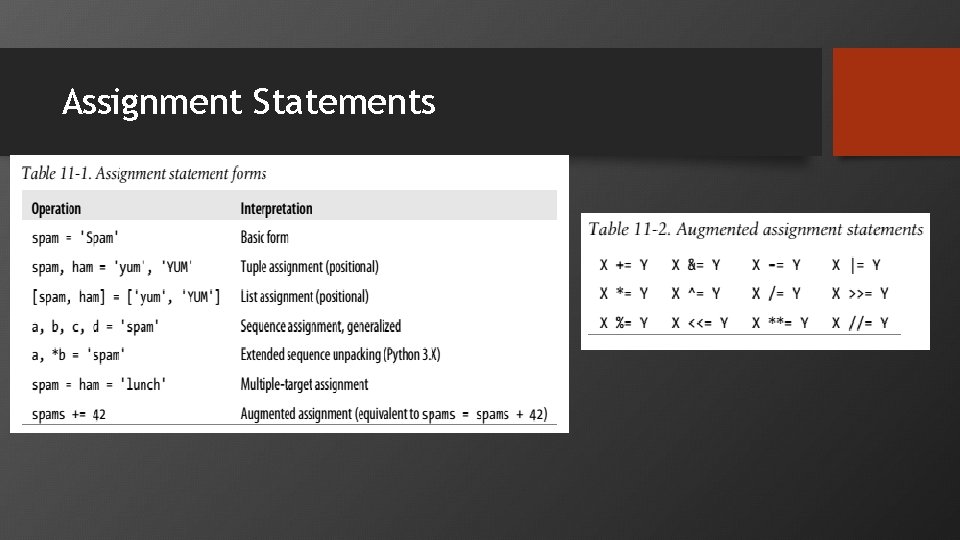
Assignment Statements
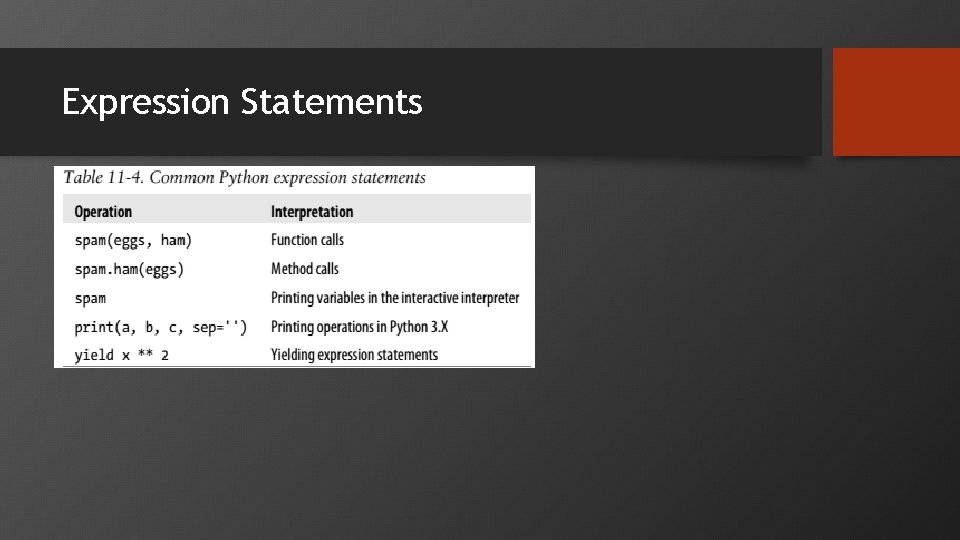
Expression Statements
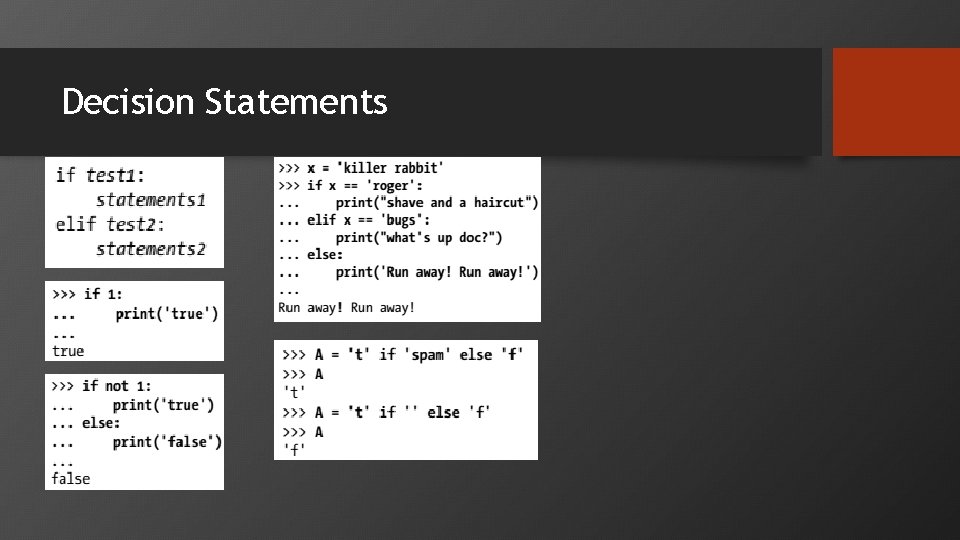
Decision Statements
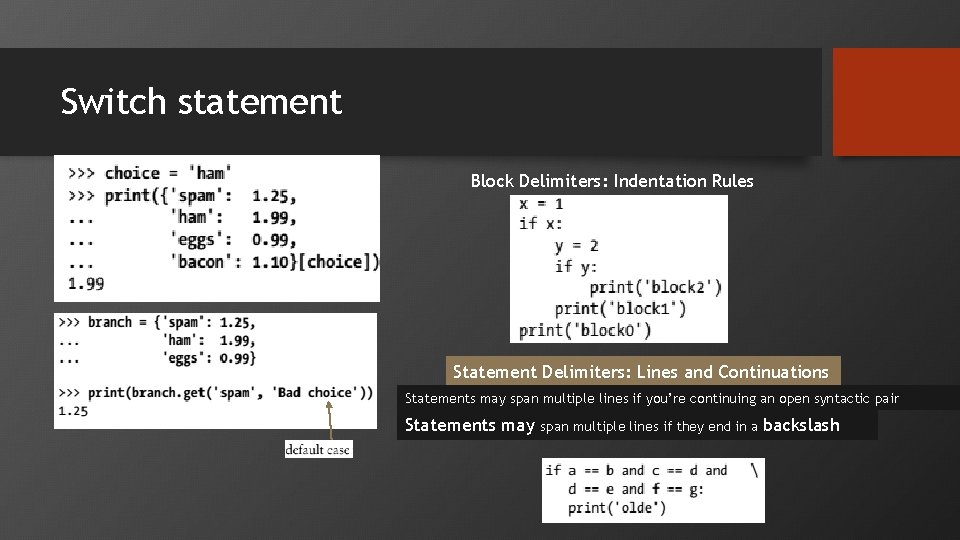
Switch statement Block Delimiters: Indentation Rules Statement Delimiters: Lines and Continuations Statements may span multiple lines if you’re continuing an open syntactic pair Statements may span multiple lines if they end in a backslash
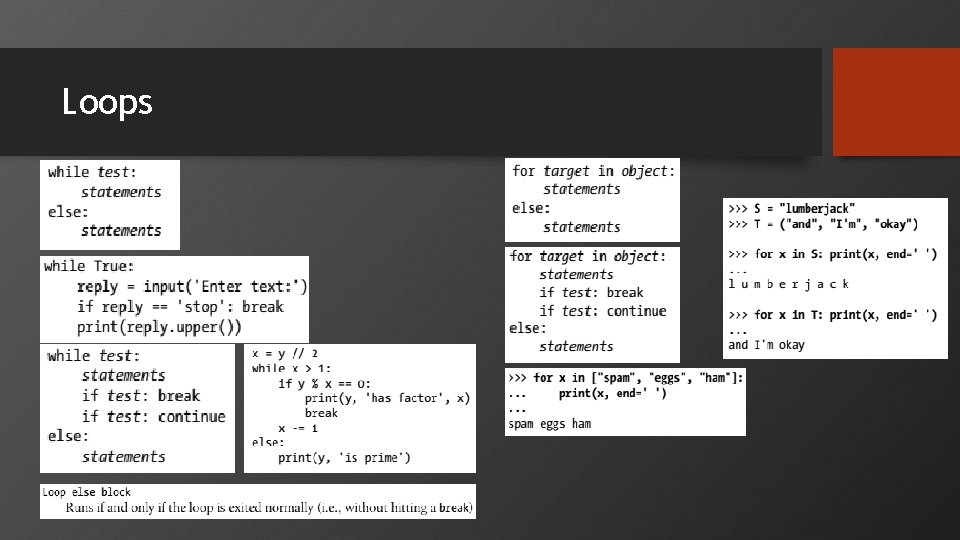
Loops
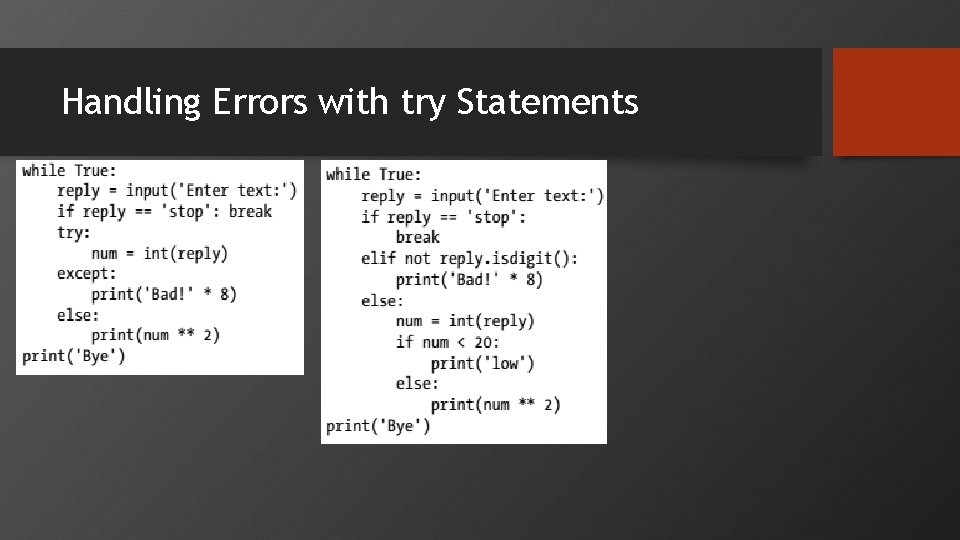
Handling Errors with try Statements
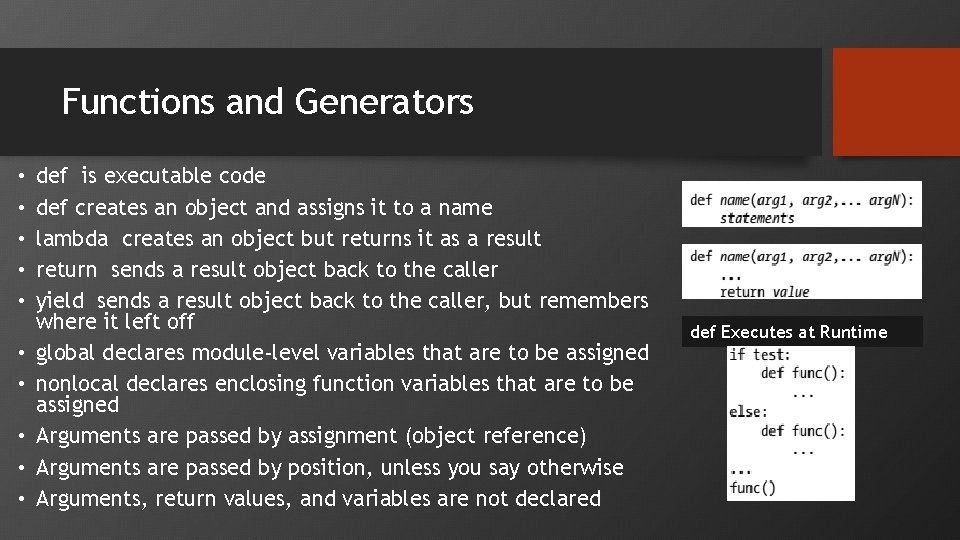
Functions and Generators • • • def is executable code def creates an object and assigns it to a name lambda creates an object but returns it as a result return sends a result object back to the caller yield sends a result object back to the caller, but remembers where it left off global declares module-level variables that are to be assigned nonlocal declares enclosing function variables that are to be assigned Arguments are passed by assignment (object reference) Arguments are passed by position, unless you say otherwise Arguments, return values, and variables are not declared def Executes at Runtime
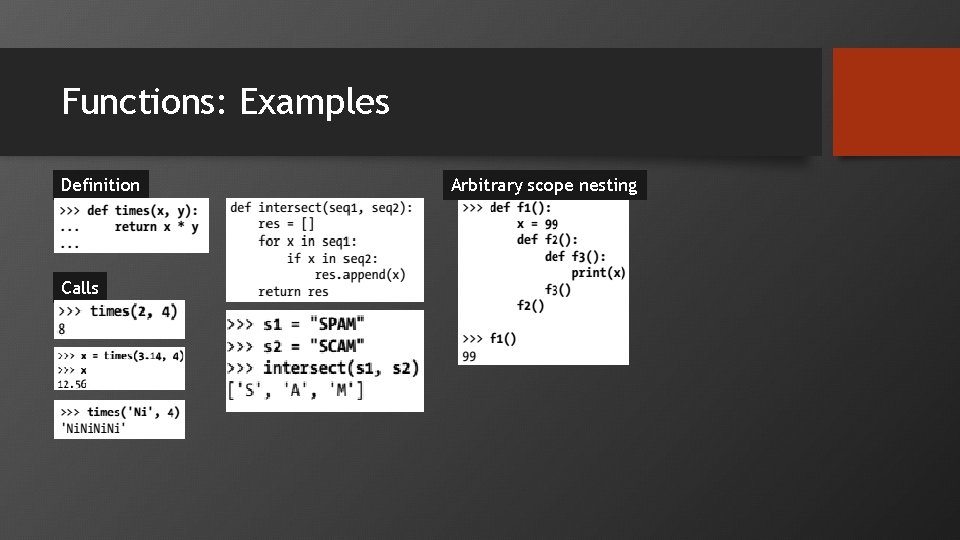
Functions: Examples Definition Calls Arbitrary scope nesting
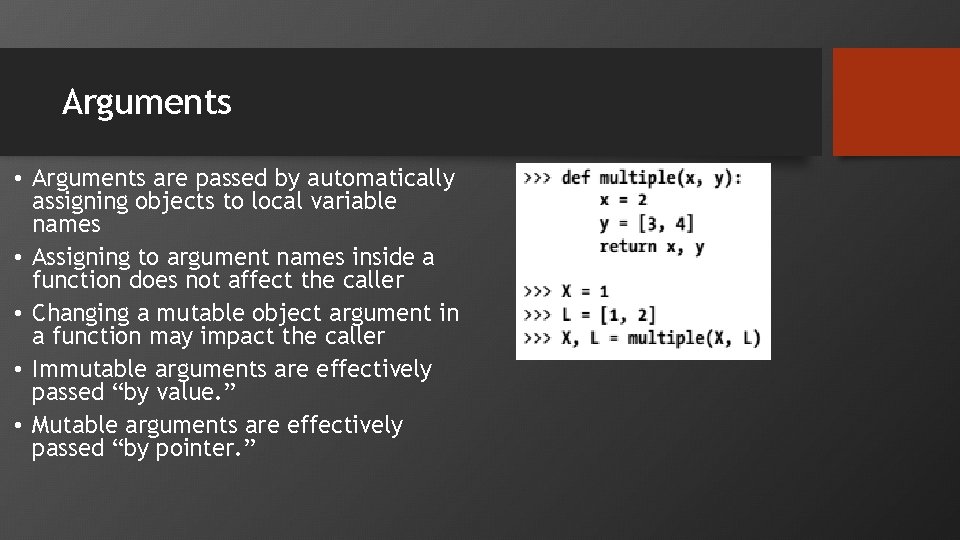
Arguments • Arguments are passed by automatically assigning objects to local variable names • Assigning to argument names inside a function does not affect the caller • Changing a mutable object argument in a function may impact the caller • Immutable arguments are effectively passed “by value. ” • Mutable arguments are effectively passed “by pointer. ”
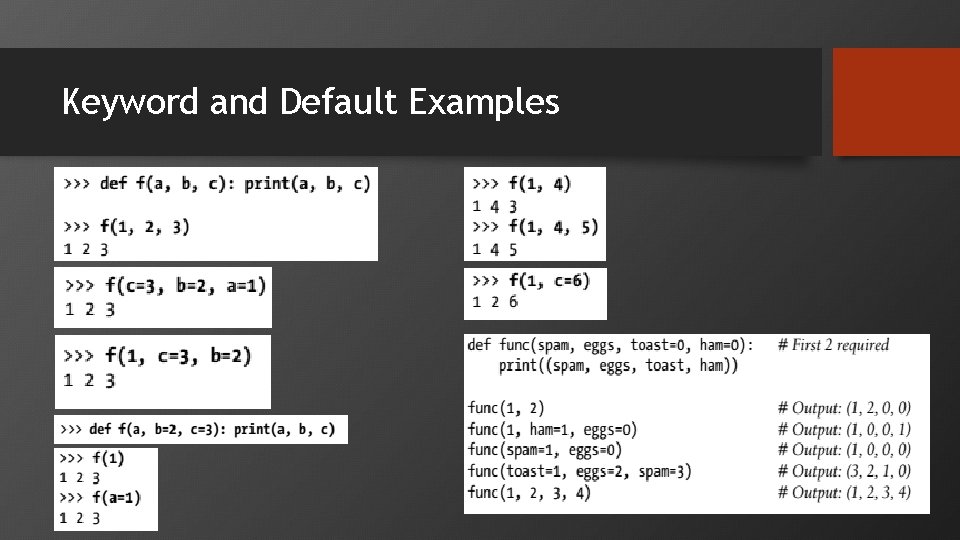
Keyword and Default Examples
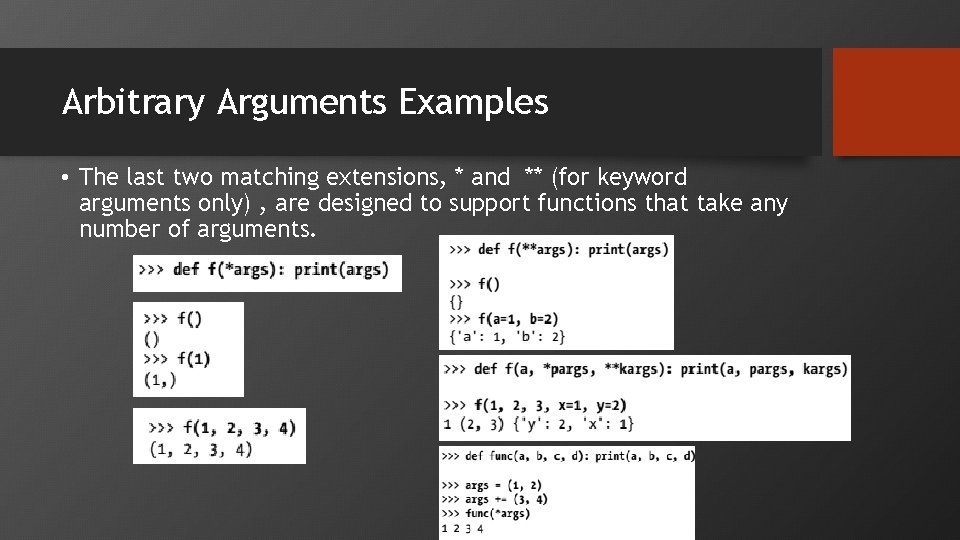
Arbitrary Arguments Examples • The last two matching extensions, * and ** (for keyword arguments only) , are designed to support functions that take any number of arguments.
Why why why why
Don't ask why why why
Pip install pyfcm
Python cgi tutorial
Shodan tutorial
Data mining python tutorial
Spark tutorial python
Itk python tutorial
Caffe tutorial
Why python is high level language
Why-why analysis
Why do you cry willie
Does the table represent a function why or why not
What does a table represent
Why or why not
Why why analysis
Windows xp tutorial
Tutorial word 2003
Xna tutorial
Textml server
Clucene tutorial
Wrf online tutorial
What is birt
Gpgpu sim tutorial
Biucache
Valgrind tutorial
Bagaimana cara mengakses layanan tutorial online
Xcode 10 tutorial for beginners
Mfix to
Expression web 4 tutorial
Use case diagram tutorial
Unified modeling language tutorial
Innsbruck uni
Class and object diagram in uml
Xcms tutorial
Duproc
Coquille tribe
Performance engineering tutorial
Visual c express 2010
Green's function tutorial
Construction of cathode ray oscilloscope
Supercell tutorial
Netconf/yang tutorial
Virtual breadboard tutorial
Xml in java
Equation editor tutorial
Symbaloo registrarse
Tutorial gantt project
Epipolar geometry tutorial