Introduction to Information Security Python Python motivation Python
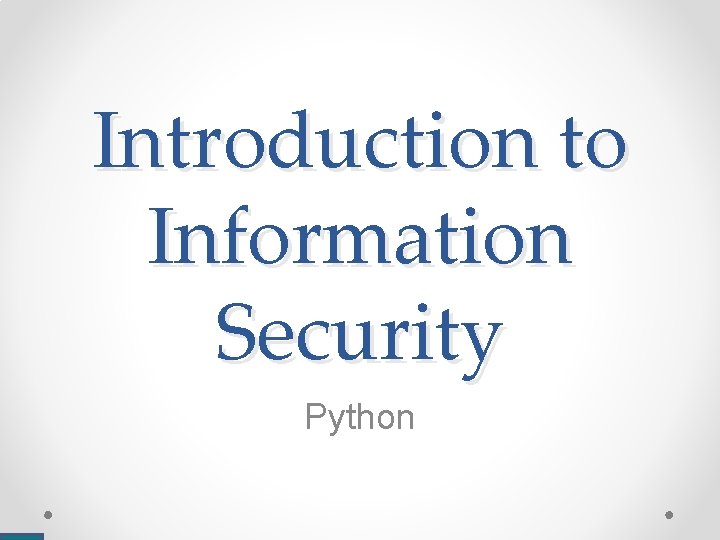
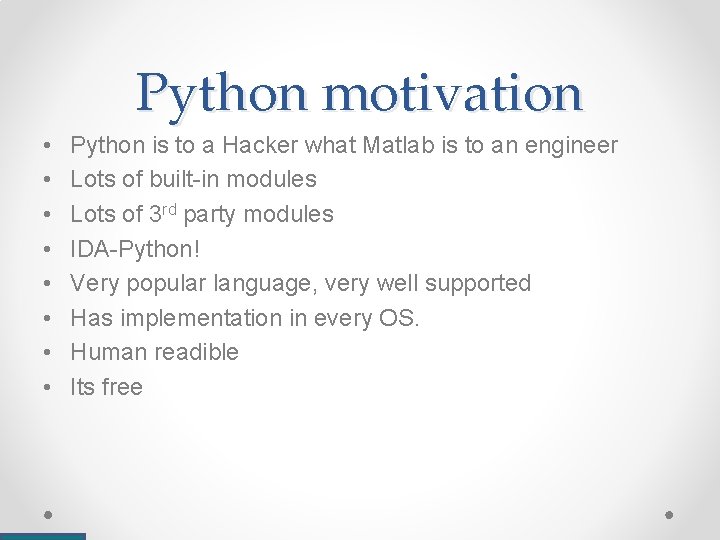
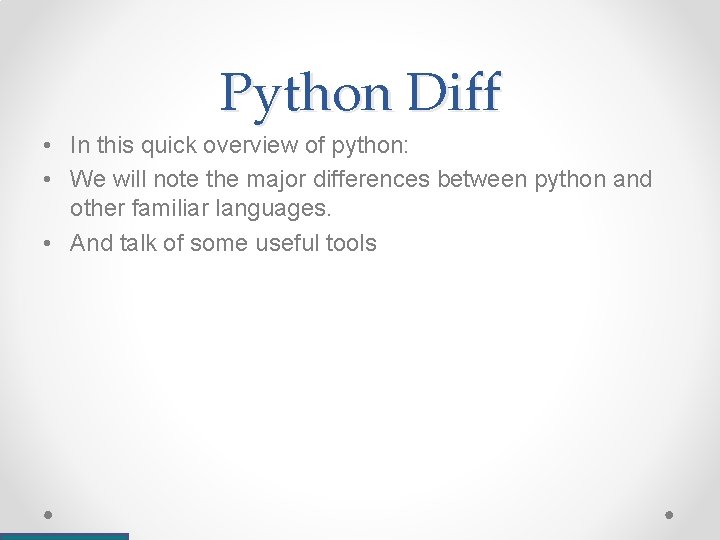
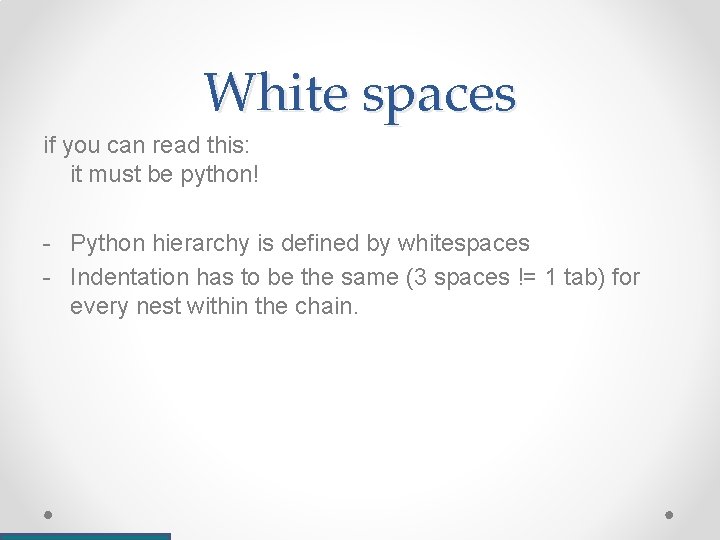
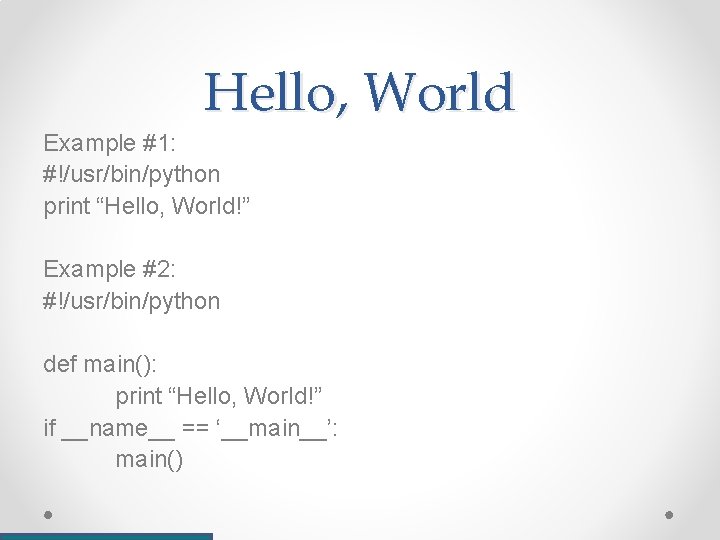
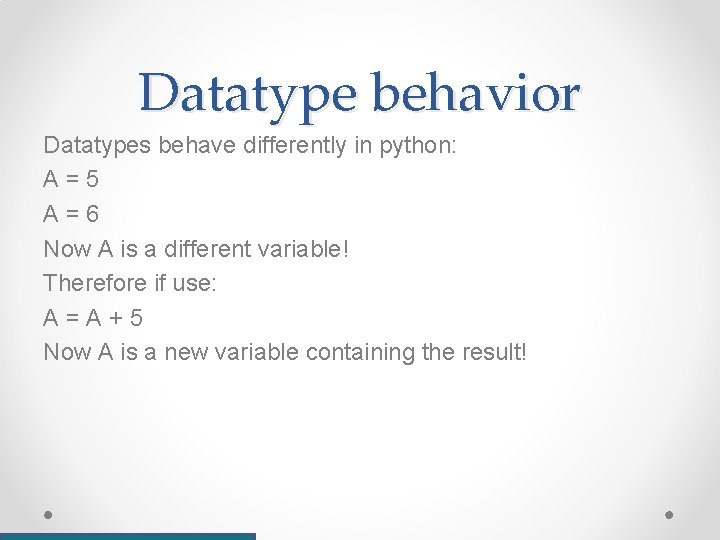
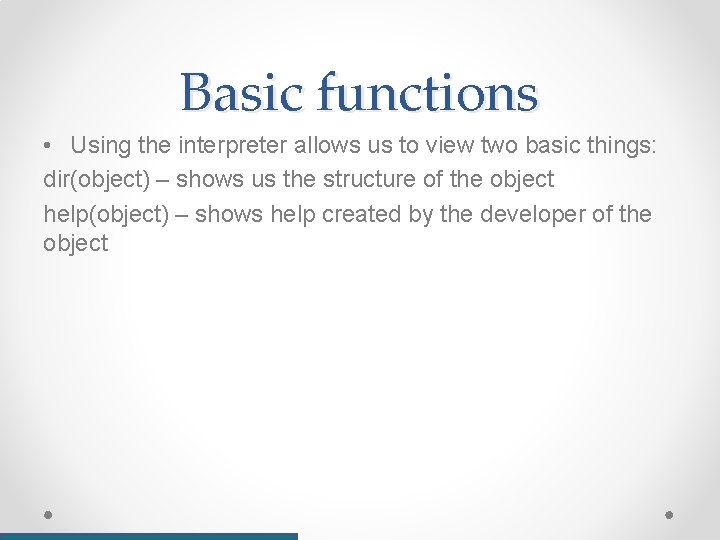
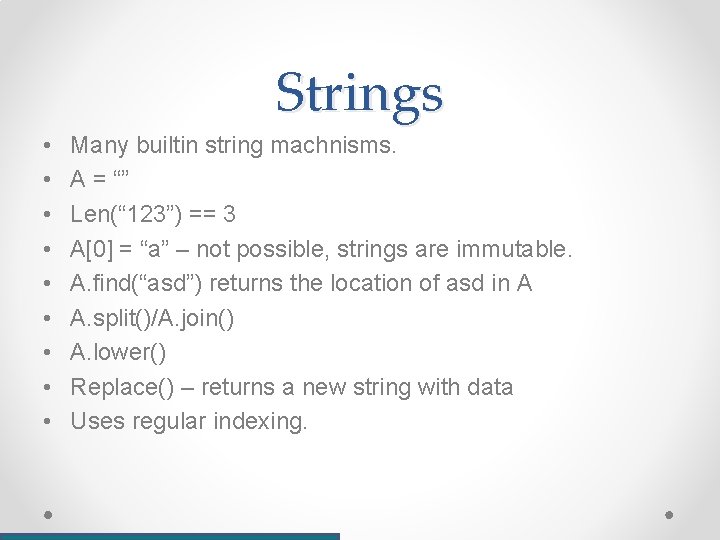
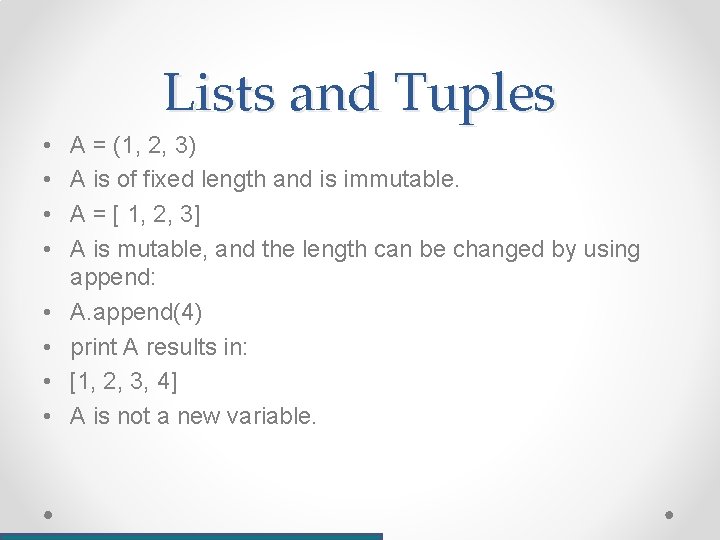
![Spans • Spans makes things very comfortable: A = “asd” A[0: ] == “asd” Spans • Spans makes things very comfortable: A = “asd” A[0: ] == “asd”](https://slidetodoc.com/presentation_image_h/353306df764634d6f7c11acccc2f9a3b/image-10.jpg)
![dict()s >>> b = dict() >>> b["hello"] = "world" >>> b {'hello': 'world'} • dict()s >>> b = dict() >>> b["hello"] = "world" >>> b {'hello': 'world'} •](https://slidetodoc.com/presentation_image_h/353306df764634d6f7c11acccc2f9a3b/image-11.jpg)
![Mutable vs Immutable • Mutable A = [1, 2, 3, 4] B=A A. append(5) Mutable vs Immutable • Mutable A = [1, 2, 3, 4] B=A A. append(5)](https://slidetodoc.com/presentation_image_h/353306df764634d6f7c11acccc2f9a3b/image-12.jpg)
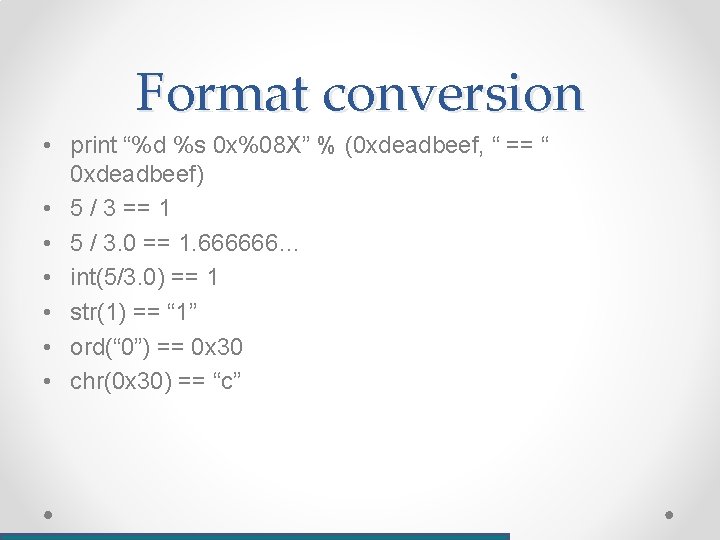
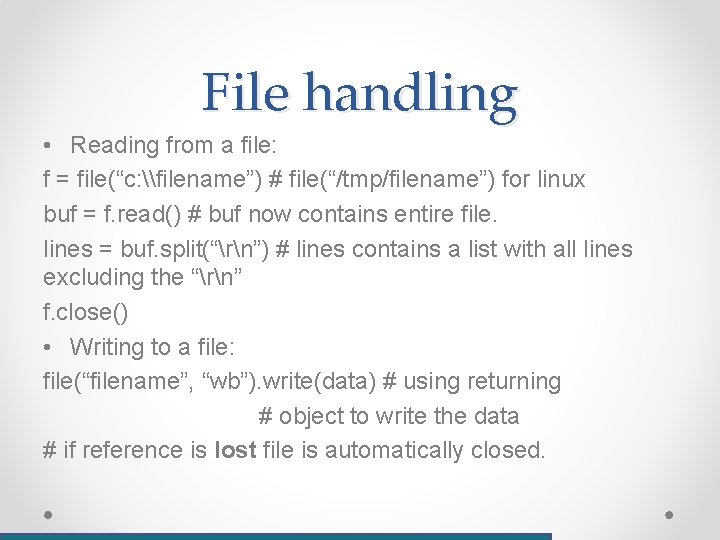
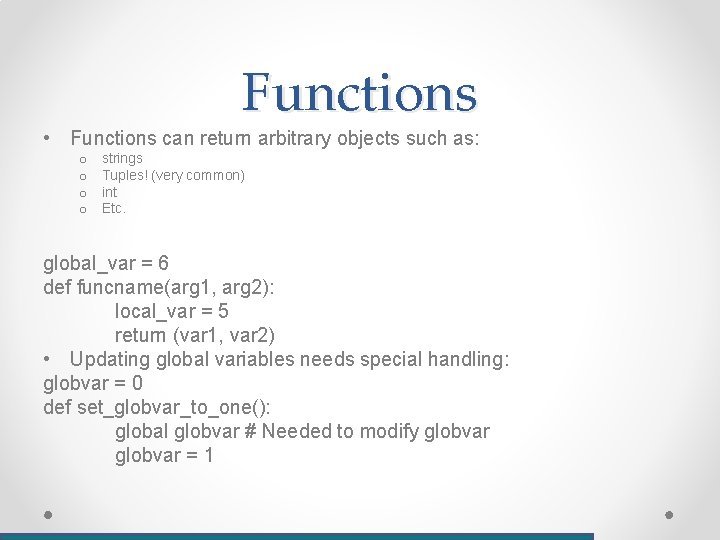
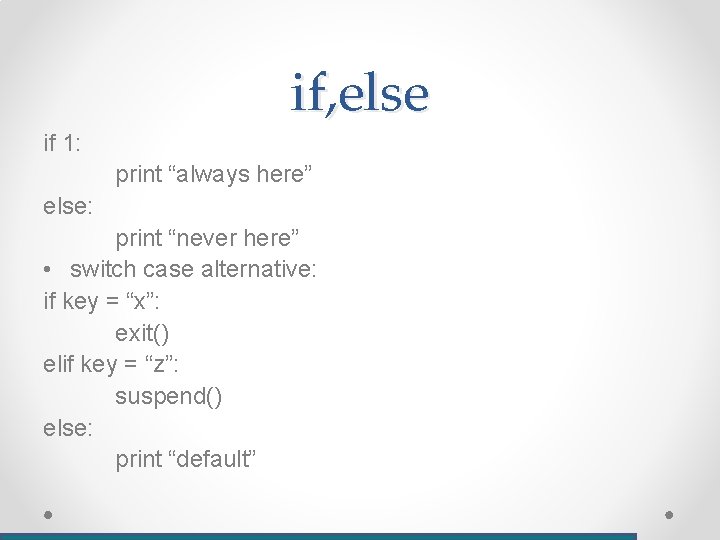
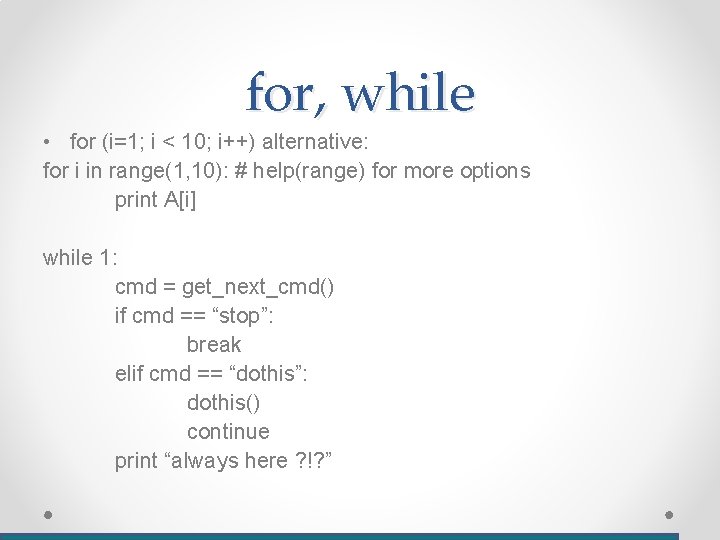
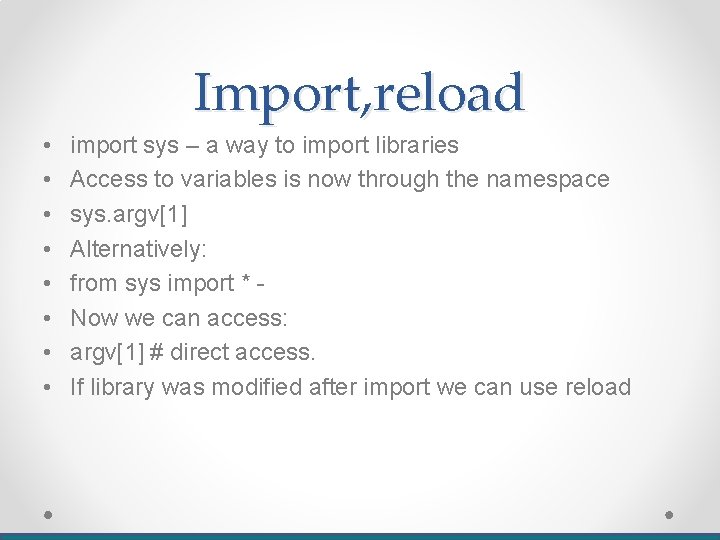
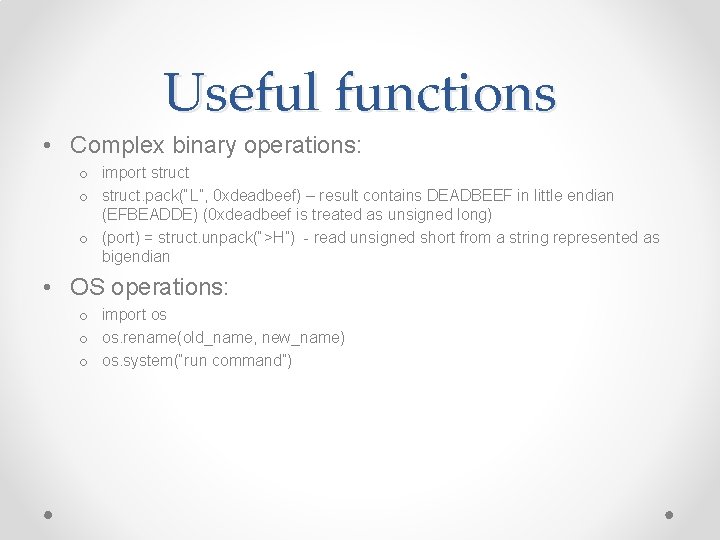
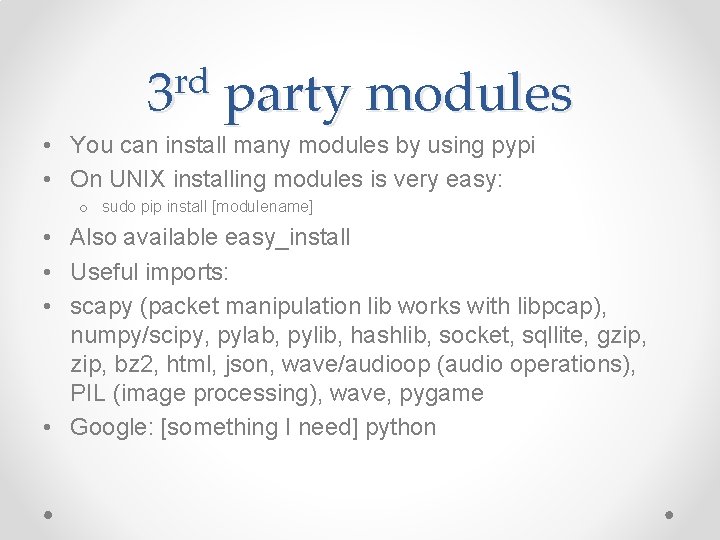
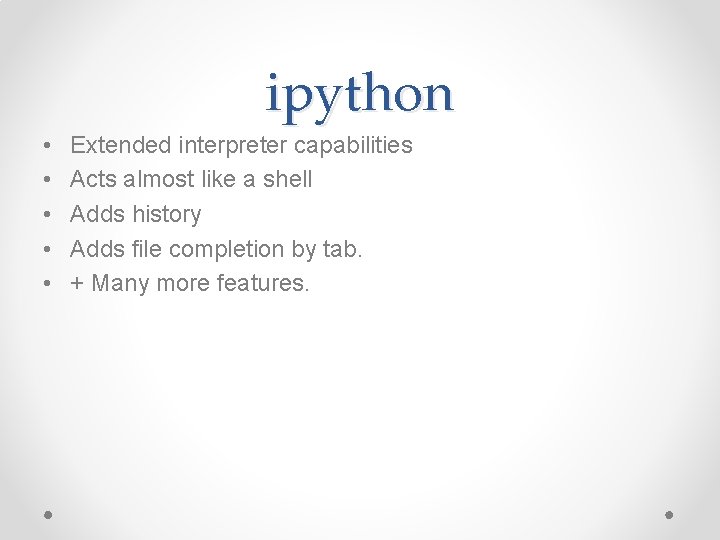
- Slides: 21
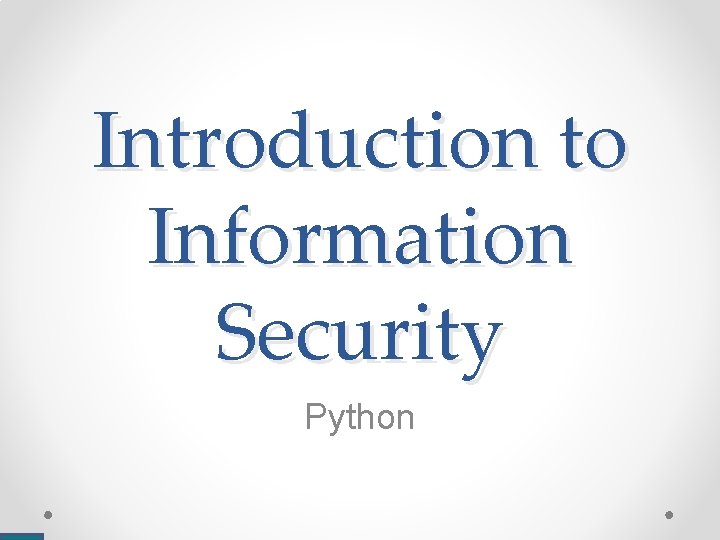
Introduction to Information Security Python
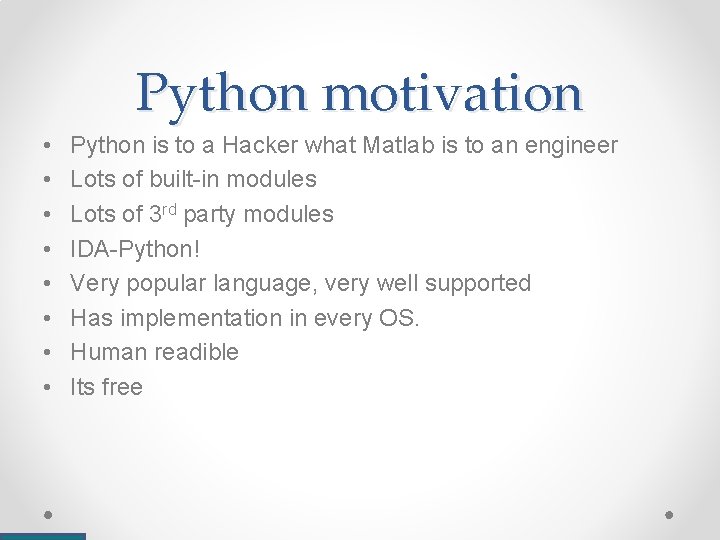
Python motivation • • Python is to a Hacker what Matlab is to an engineer Lots of built-in modules Lots of 3 rd party modules IDA-Python! Very popular language, very well supported Has implementation in every OS. Human readible Its free
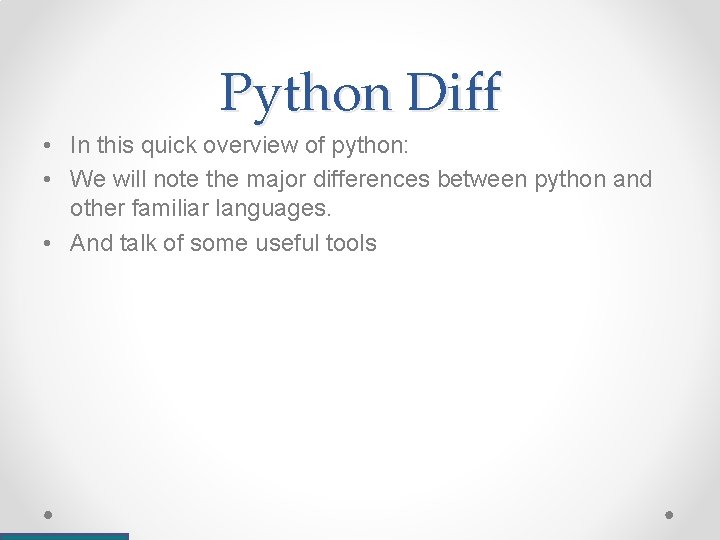
Python Diff • In this quick overview of python: • We will note the major differences between python and other familiar languages. • And talk of some useful tools
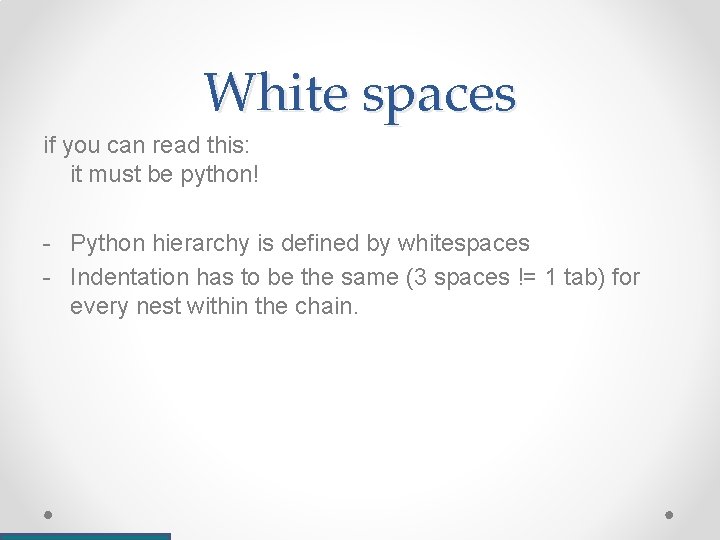
White spaces if you can read this: it must be python! - Python hierarchy is defined by whitespaces - Indentation has to be the same (3 spaces != 1 tab) for every nest within the chain.
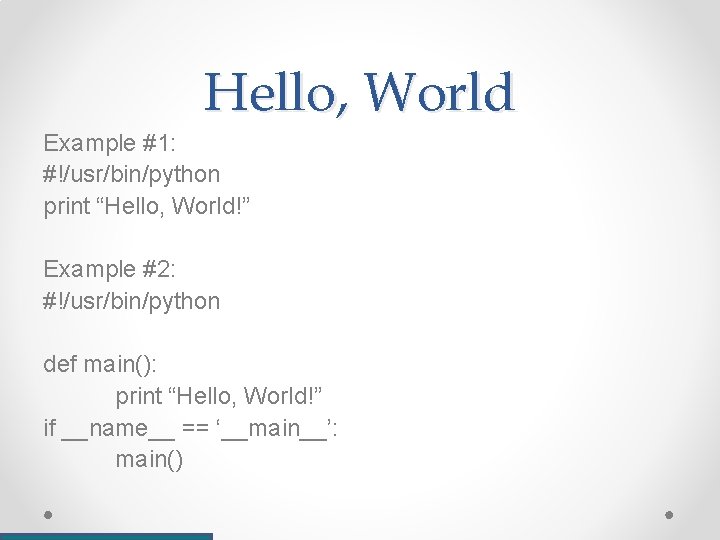
Hello, World Example #1: #!/usr/bin/python print “Hello, World!” Example #2: #!/usr/bin/python def main(): print “Hello, World!” if __name__ == ‘__main__’: main()
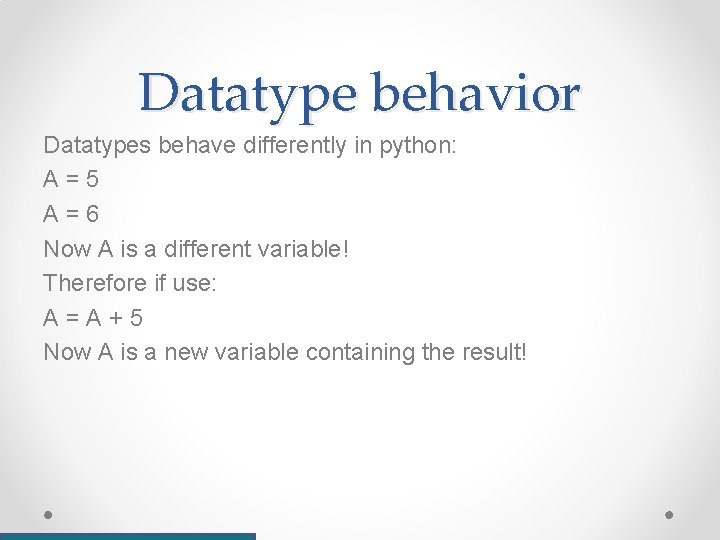
Datatype behavior Datatypes behave differently in python: A=5 A=6 Now A is a different variable! Therefore if use: A=A+5 Now A is a new variable containing the result!
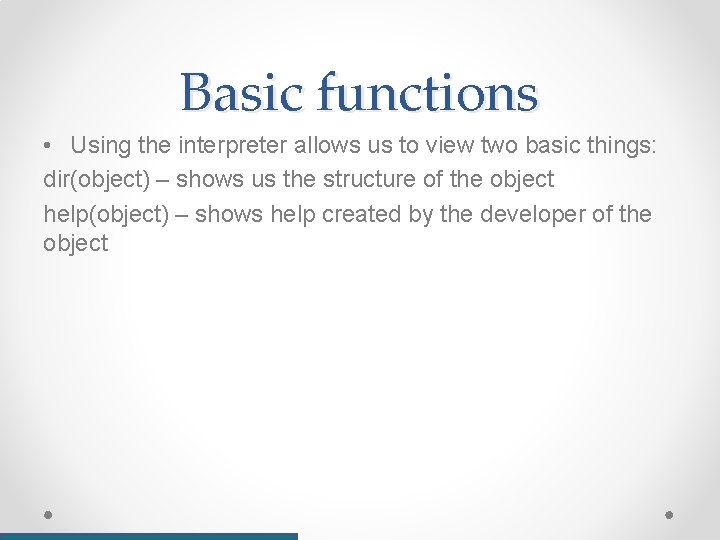
Basic functions • Using the interpreter allows us to view two basic things: dir(object) – shows us the structure of the object help(object) – shows help created by the developer of the object
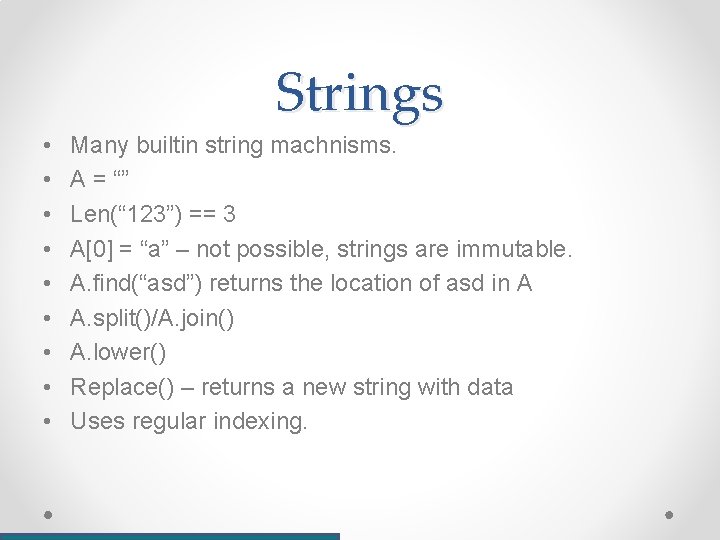
Strings • • • Many builtin string machnisms. A = “” Len(“ 123”) == 3 A[0] = “a” – not possible, strings are immutable. A. find(“asd”) returns the location of asd in A A. split()/A. join() A. lower() Replace() – returns a new string with data Uses regular indexing.
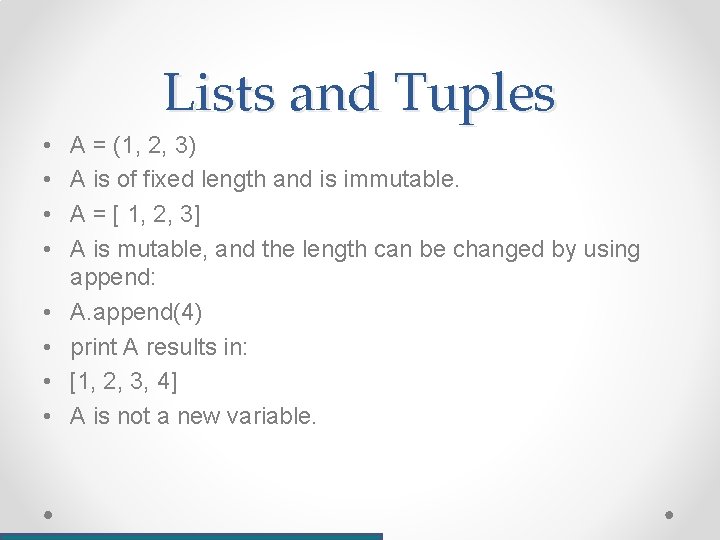
Lists and Tuples • • A = (1, 2, 3) A is of fixed length and is immutable. A = [ 1, 2, 3] A is mutable, and the length can be changed by using append: A. append(4) print A results in: [1, 2, 3, 4] A is not a new variable.
![Spans Spans makes things very comfortable A asd A0 asd Spans • Spans makes things very comfortable: A = “asd” A[0: ] == “asd”](https://slidetodoc.com/presentation_image_h/353306df764634d6f7c11acccc2f9a3b/image-10.jpg)
Spans • Spans makes things very comfortable: A = “asd” A[0: ] == “asd” A[1: ] == “sd” A[0: -1] == “as” A[0: 1] == “a” A[0: -2] == “a” A[: 2] == “as” A[1: 2] == “s” • Works on tuples, and lists!!
![dicts b dict bhello world b hello world dict()s >>> b = dict() >>> b["hello"] = "world" >>> b {'hello': 'world'} •](https://slidetodoc.com/presentation_image_h/353306df764634d6f7c11acccc2f9a3b/image-11.jpg)
dict()s >>> b = dict() >>> b["hello"] = "world" >>> b {'hello': 'world'} • [Demo dicts]
![Mutable vs Immutable Mutable A 1 2 3 4 BA A append5 Mutable vs Immutable • Mutable A = [1, 2, 3, 4] B=A A. append(5)](https://slidetodoc.com/presentation_image_h/353306df764634d6f7c11acccc2f9a3b/image-12.jpg)
Mutable vs Immutable • Mutable A = [1, 2, 3, 4] B=A A. append(5) print B [1, 2, 3, 4, 5] • Immutable A = “foo” B=A A = A + “bar” print B “foo”
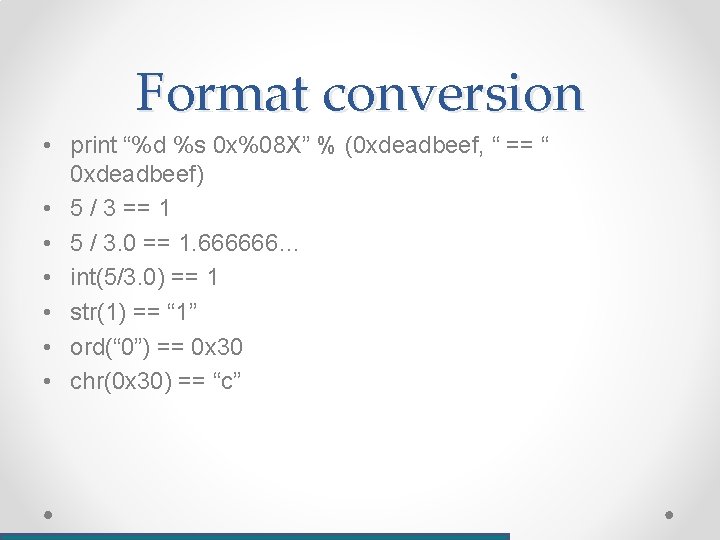
Format conversion • print “%d %s 0 x%08 X” % (0 xdeadbeef, “ == “ 0 xdeadbeef) • 5 / 3 == 1 • 5 / 3. 0 == 1. 666666… • int(5/3. 0) == 1 • str(1) == “ 1” • ord(“ 0”) == 0 x 30 • chr(0 x 30) == “c”
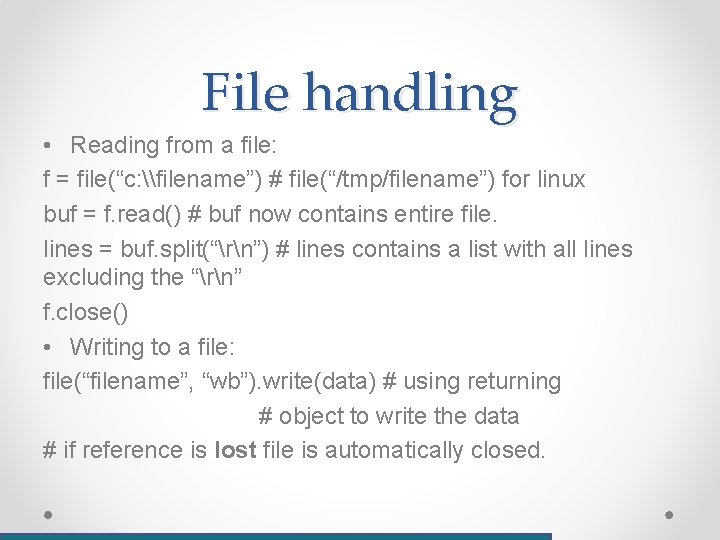
File handling • Reading from a file: f = file(“c: \filename”) # file(“/tmp/filename”) for linux buf = f. read() # buf now contains entire file. lines = buf. split(“rn”) # lines contains a list with all lines excluding the “rn” f. close() • Writing to a file: file(“filename”, “wb”). write(data) # using returning # object to write the data # if reference is lost file is automatically closed.
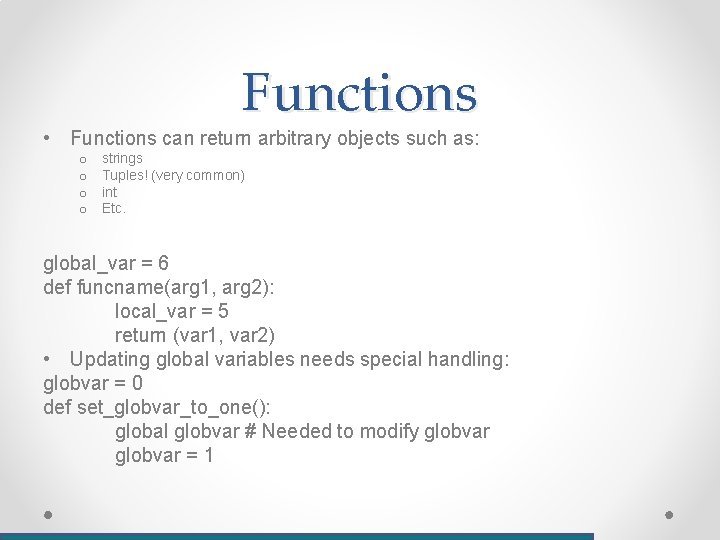
Functions • Functions can return arbitrary objects such as: o o strings Tuples! (very common) int Etc. global_var = 6 def funcname(arg 1, arg 2): local_var = 5 return (var 1, var 2) • Updating global variables needs special handling: globvar = 0 def set_globvar_to_one(): global globvar # Needed to modify globvar = 1
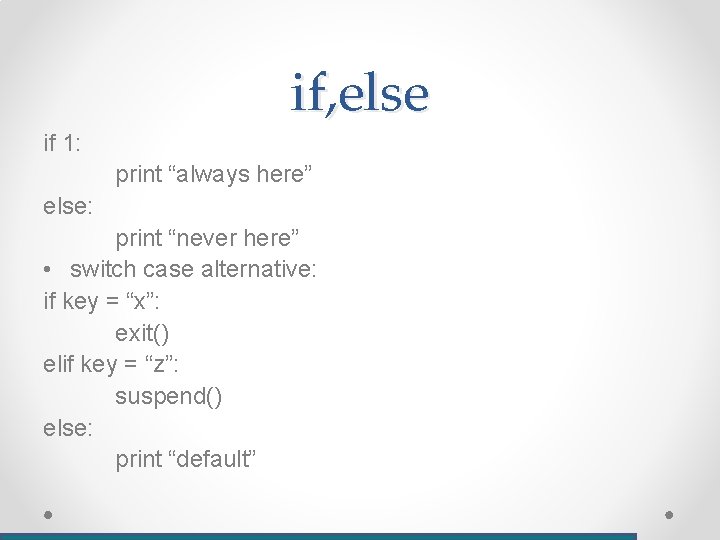
if, else if 1: print “always here” else: print “never here” • switch case alternative: if key = “x”: exit() elif key = “z”: suspend() else: print “default”
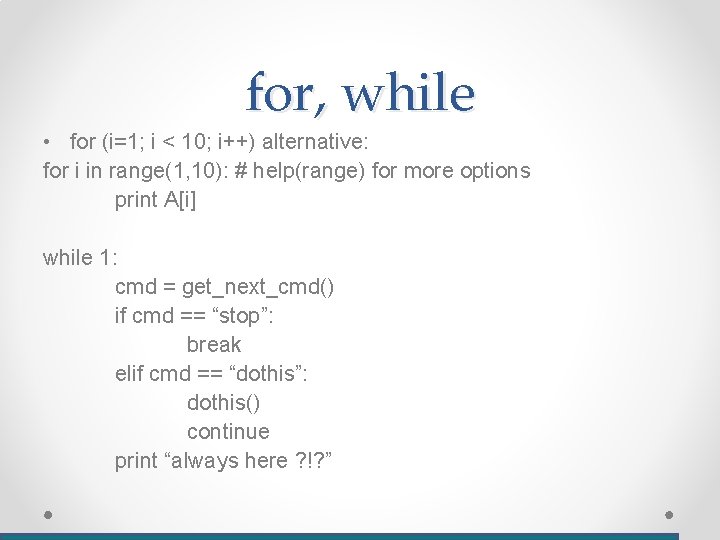
for, while • for (i=1; i < 10; i++) alternative: for i in range(1, 10): # help(range) for more options print A[i] while 1: cmd = get_next_cmd() if cmd == “stop”: break elif cmd == “dothis”: dothis() continue print “always here ? !? ”
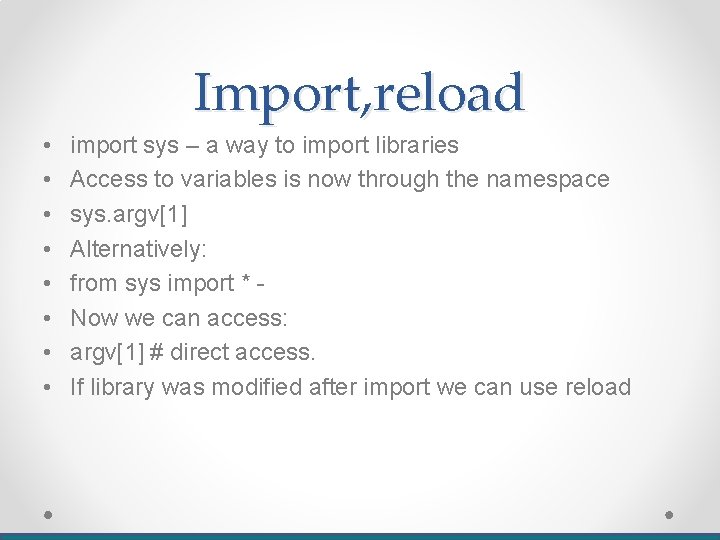
Import, reload • • import sys – a way to import libraries Access to variables is now through the namespace sys. argv[1] Alternatively: from sys import * Now we can access: argv[1] # direct access. If library was modified after import we can use reload
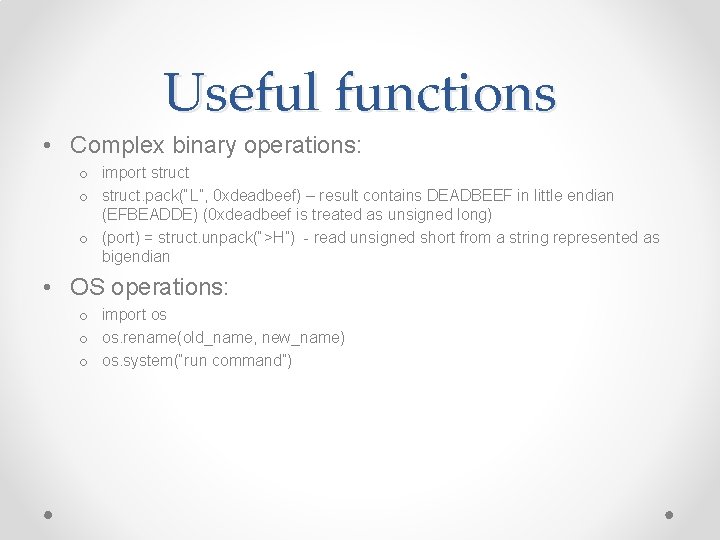
Useful functions • Complex binary operations: o import struct o struct. pack(“L”, 0 xdeadbeef) – result contains DEADBEEF in little endian (EFBEADDE) (0 xdeadbeef is treated as unsigned long) o (port) = struct. unpack(“>H”) - read unsigned short from a string represented as bigendian • OS operations: o import os o os. rename(old_name, new_name) o os. system(“run command”)
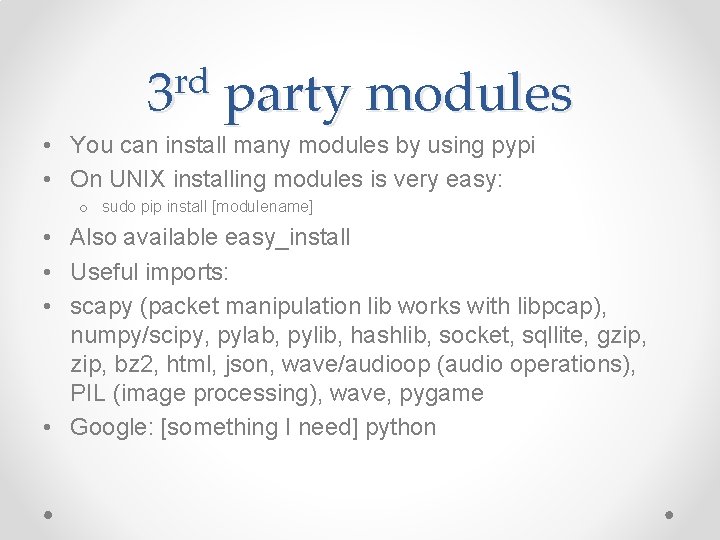
rd 3 party modules • You can install many modules by using pypi • On UNIX installing modules is very easy: o sudo pip install [modulename] • Also available easy_install • Useful imports: • scapy (packet manipulation lib works with libpcap), numpy/scipy, pylab, pylib, hashlib, socket, sqllite, gzip, bz 2, html, json, wave/audioop (audio operations), PIL (image processing), wave, pygame • Google: [something I need] python
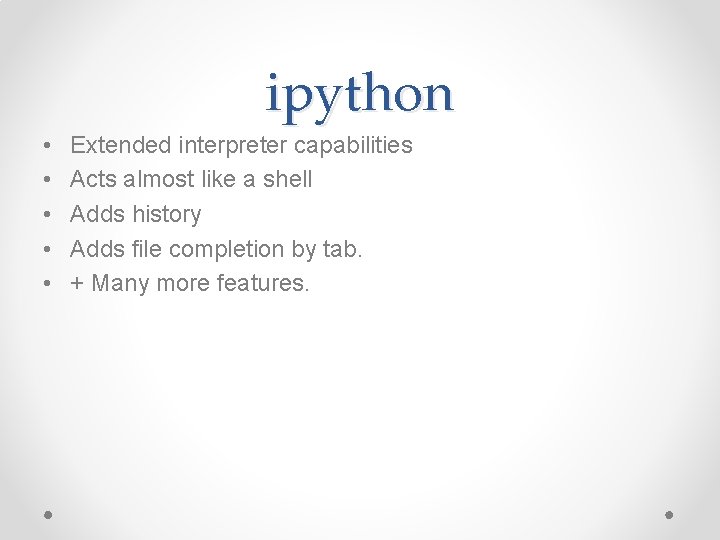
ipython • • • Extended interpreter capabilities Acts almost like a shell Adds history Adds file completion by tab. + Many more features.