Python ClientServer Shuai Zhao What is Python Python
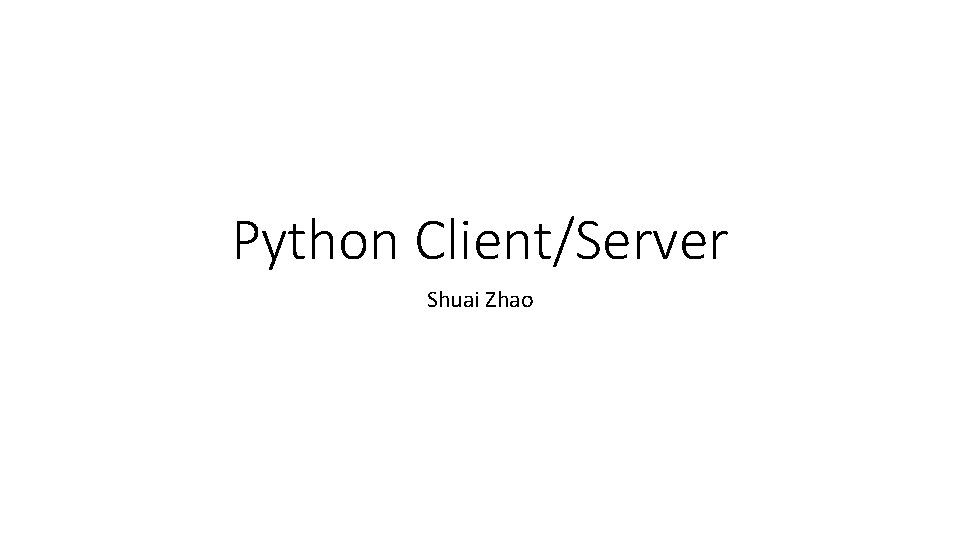
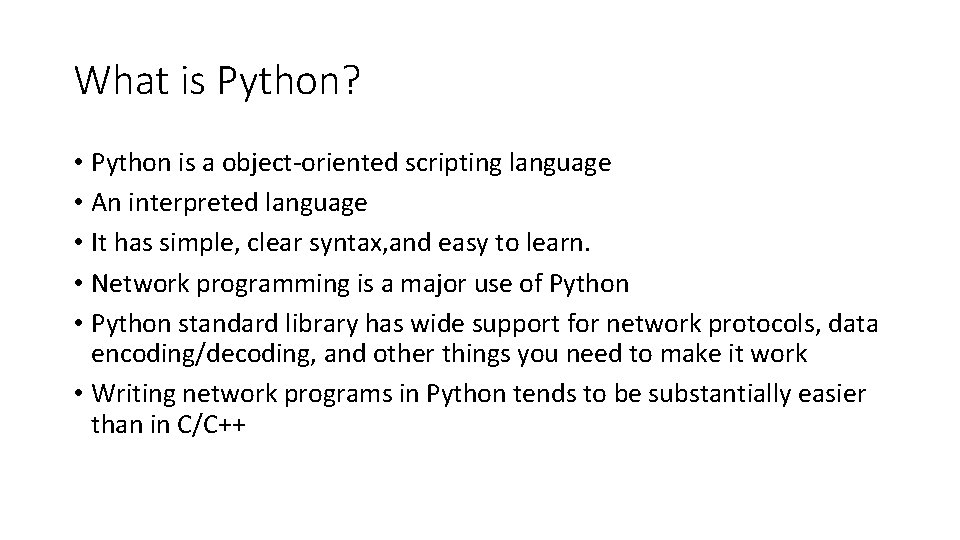
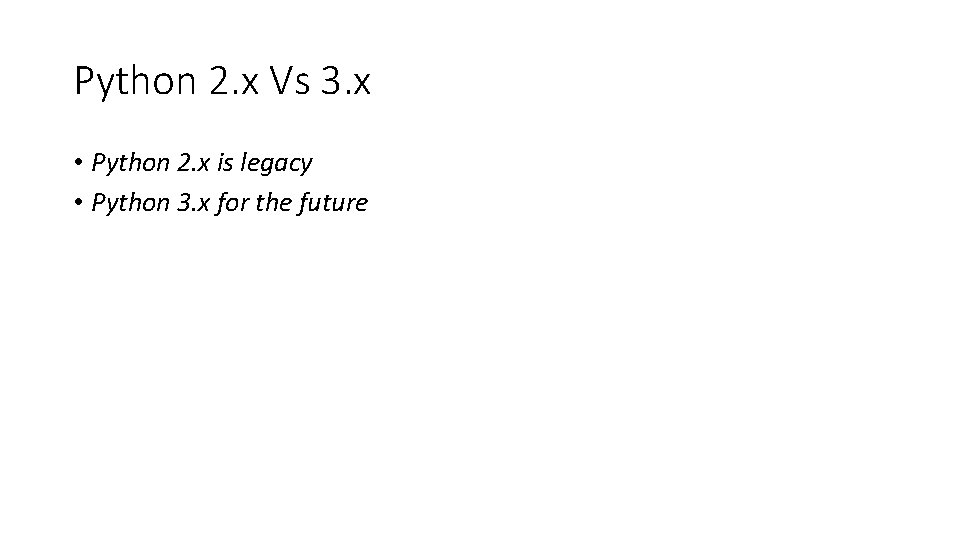
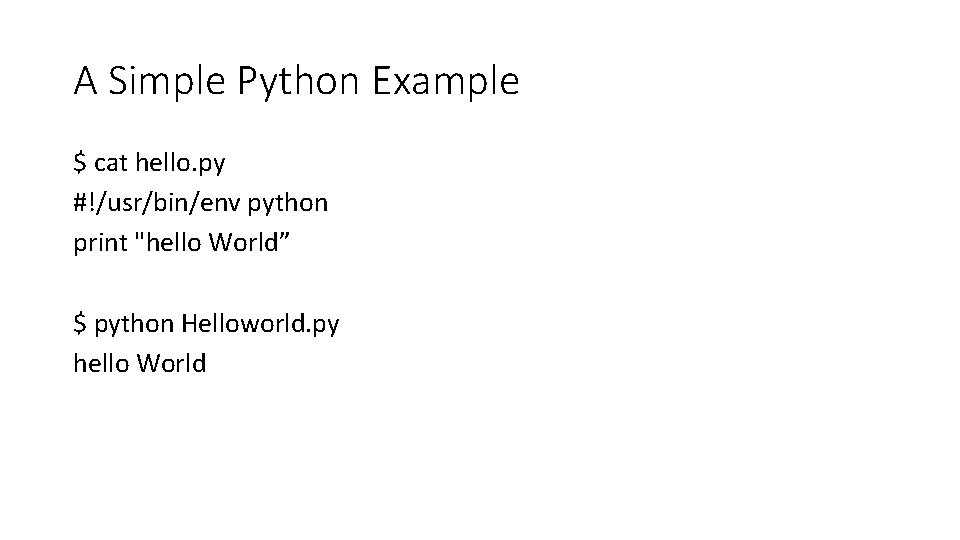
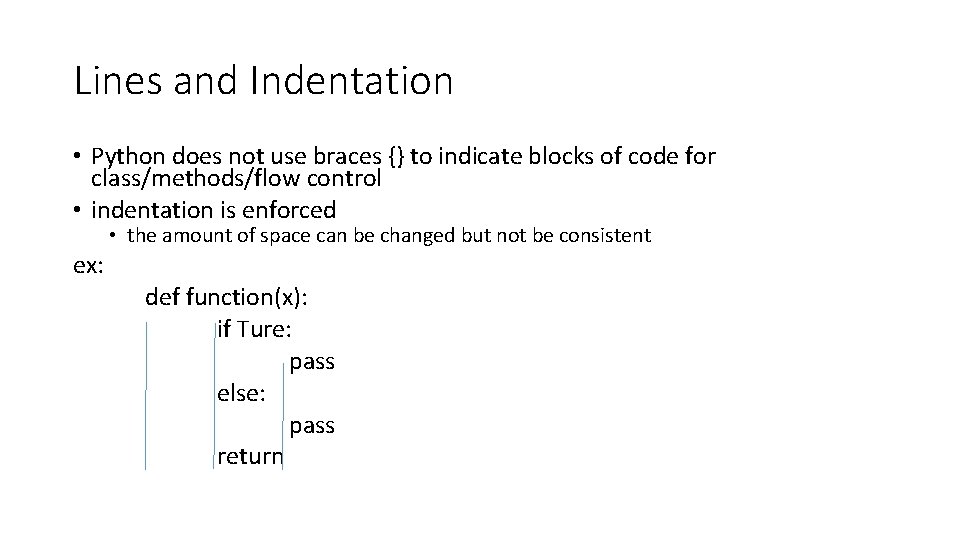
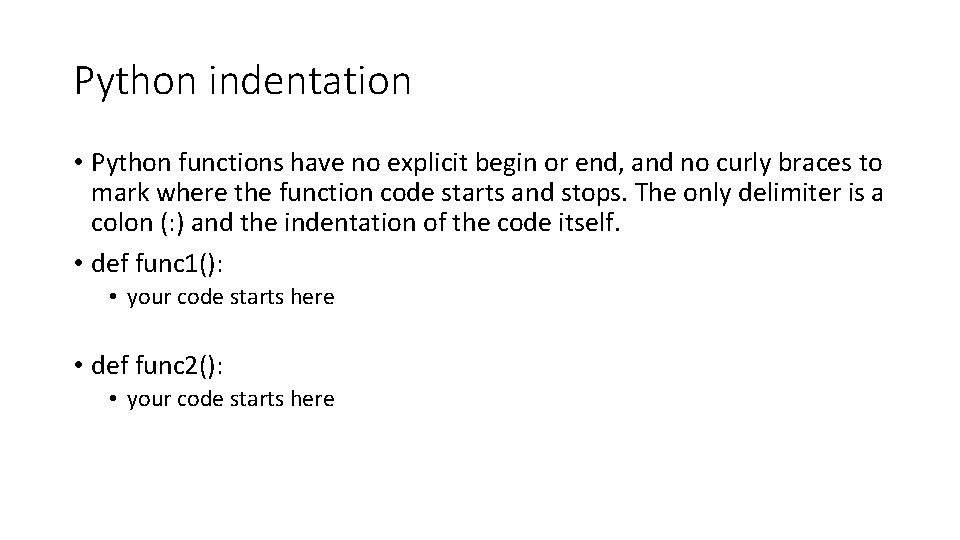
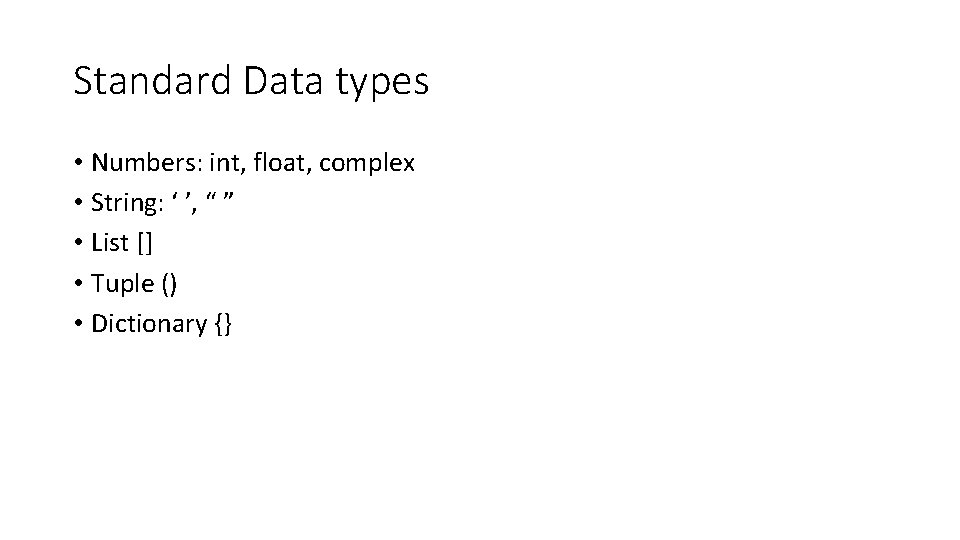
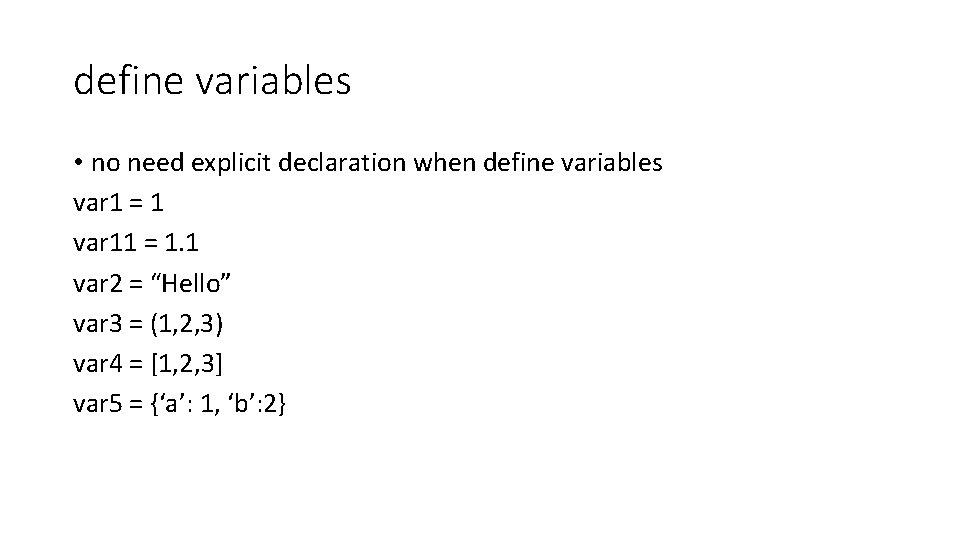
![Python containers • List [] • Tuple () • dictionary {key: value} Python containers • List [] • Tuple () • dictionary {key: value}](https://slidetodoc.com/presentation_image_h/ed769ba2f8d04bdce0256984e4bff273/image-9.jpg)
![Python List list 1 = [1, 2. 1, ’abcde’] print list 1[0] print list Python List list 1 = [1, 2. 1, ’abcde’] print list 1[0] print list](https://slidetodoc.com/presentation_image_h/ed769ba2f8d04bdce0256984e4bff273/image-10.jpg)
![Python dictionary • dict 1 = {‘Key’: Value} • dict 1['one'] = "This is Python dictionary • dict 1 = {‘Key’: Value} • dict 1['one'] = "This is](https://slidetodoc.com/presentation_image_h/ed769ba2f8d04bdce0256984e4bff273/image-11.jpg)
![python tuple 1 = (1, 2. 1, ’abcde’) print tuple 1[0] print tuple 1[1: python tuple 1 = (1, 2. 1, ’abcde’) print tuple 1[0] print tuple 1[1:](https://slidetodoc.com/presentation_image_h/ed769ba2f8d04bdce0256984e4bff273/image-12.jpg)
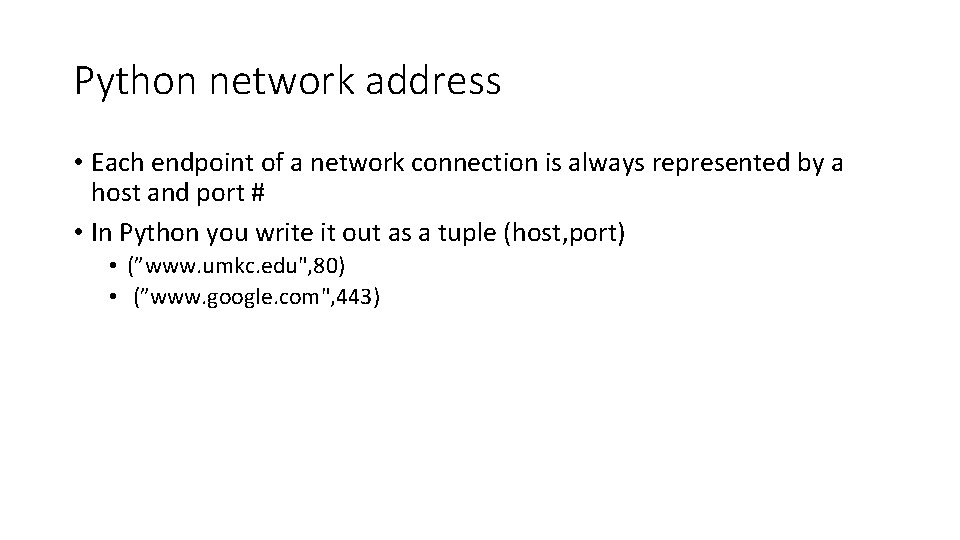
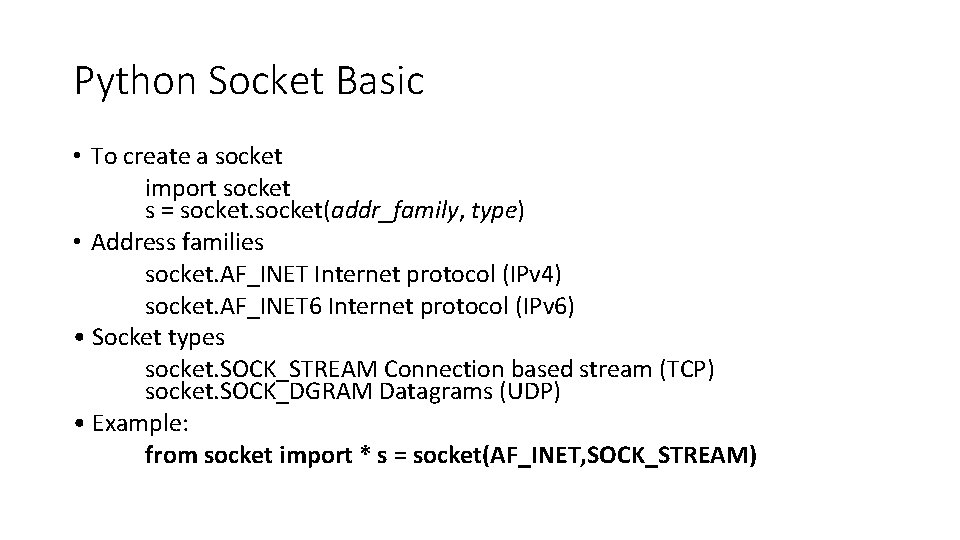
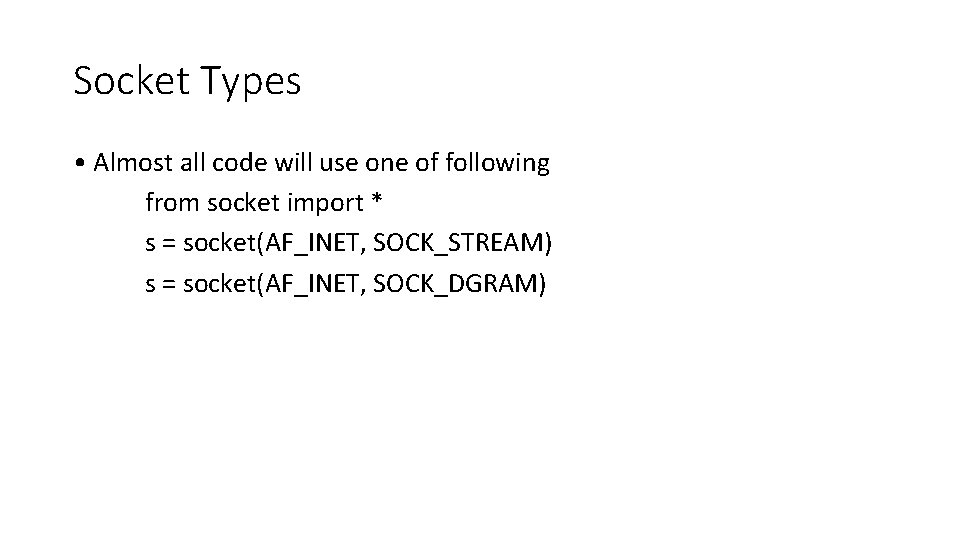
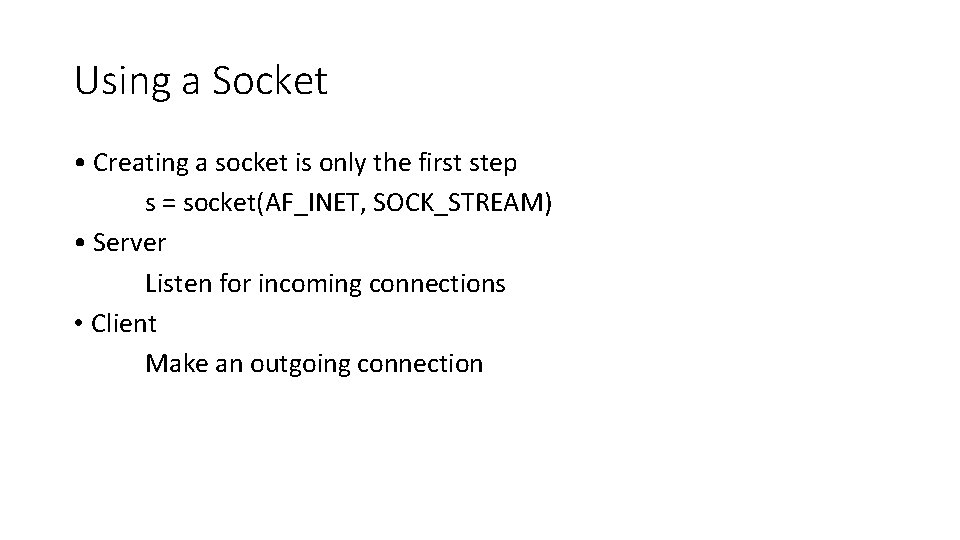
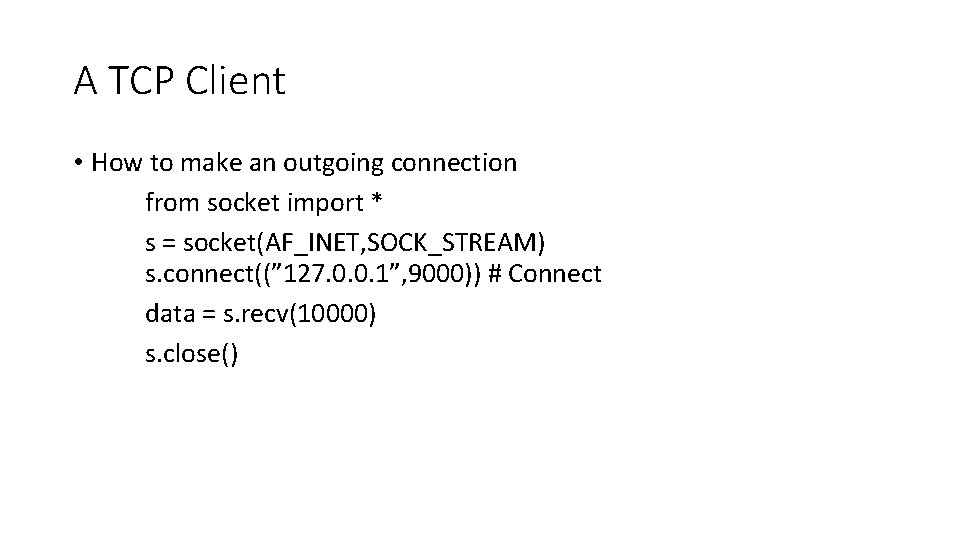
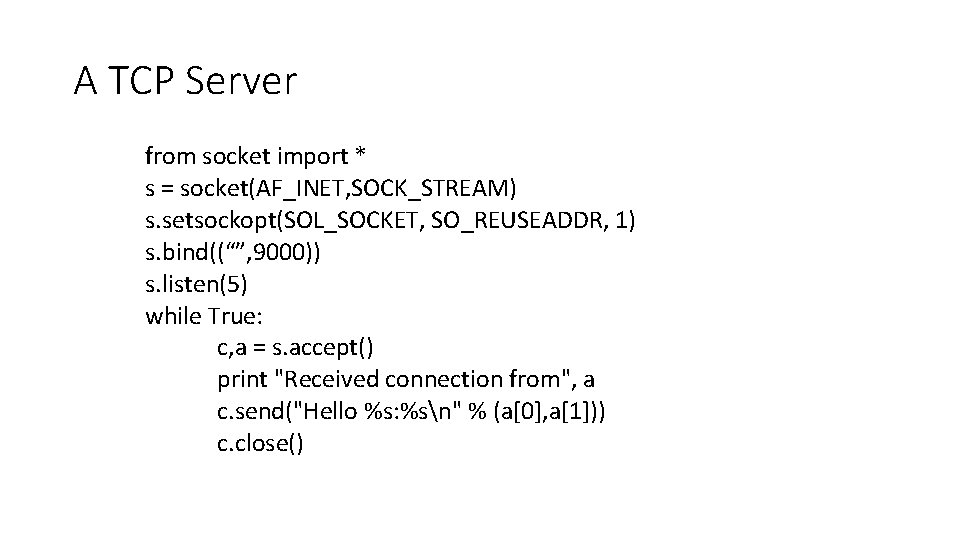
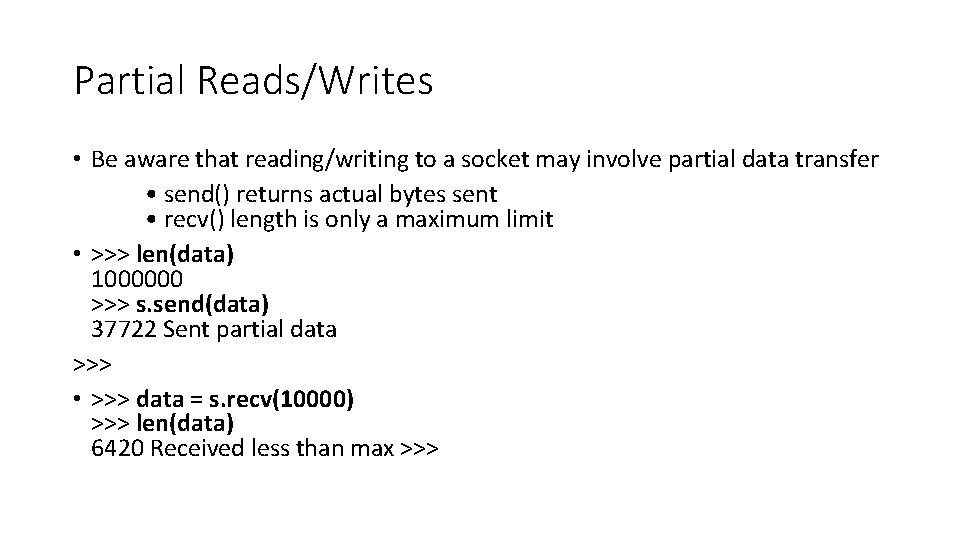
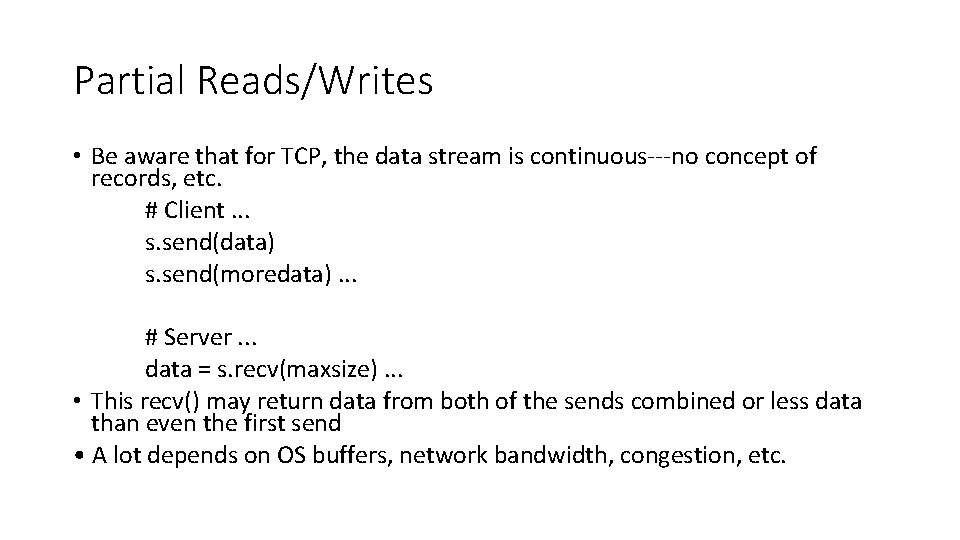
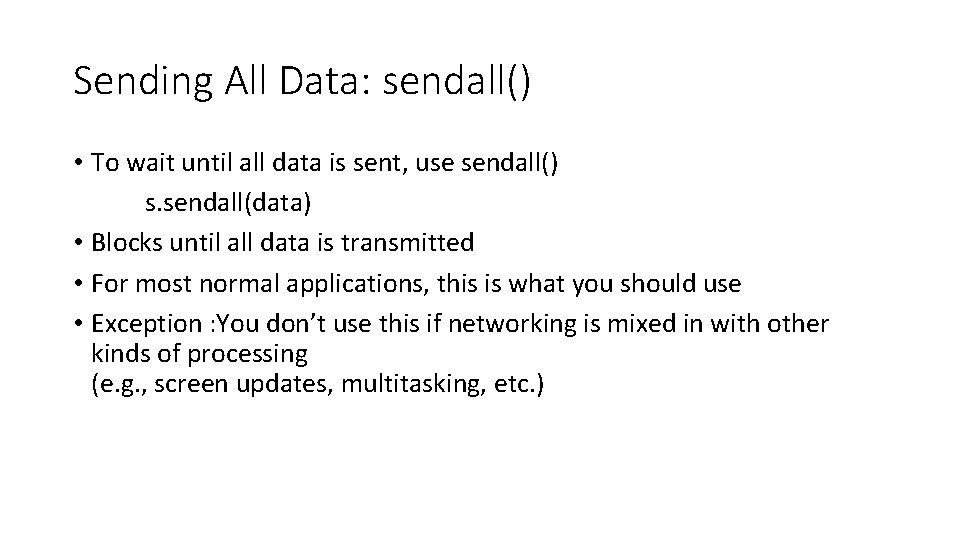
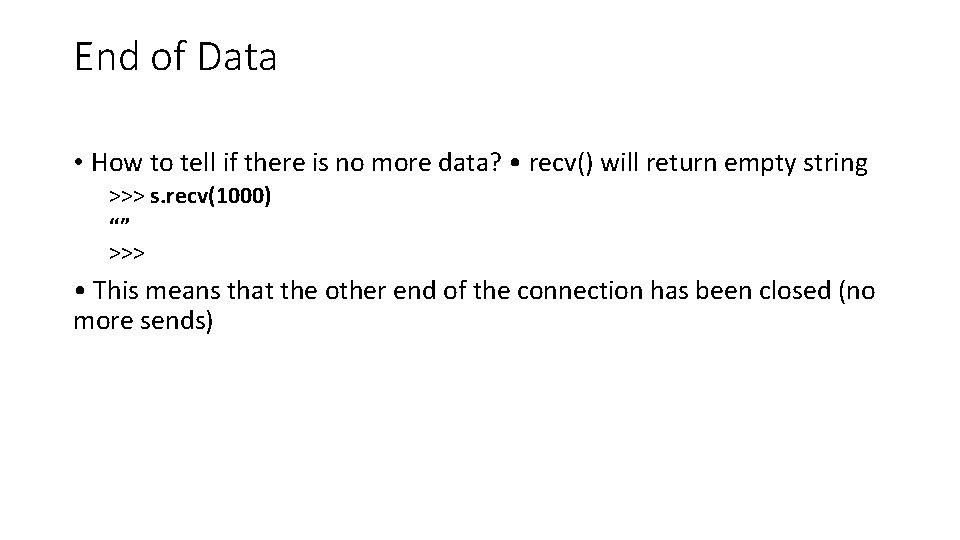
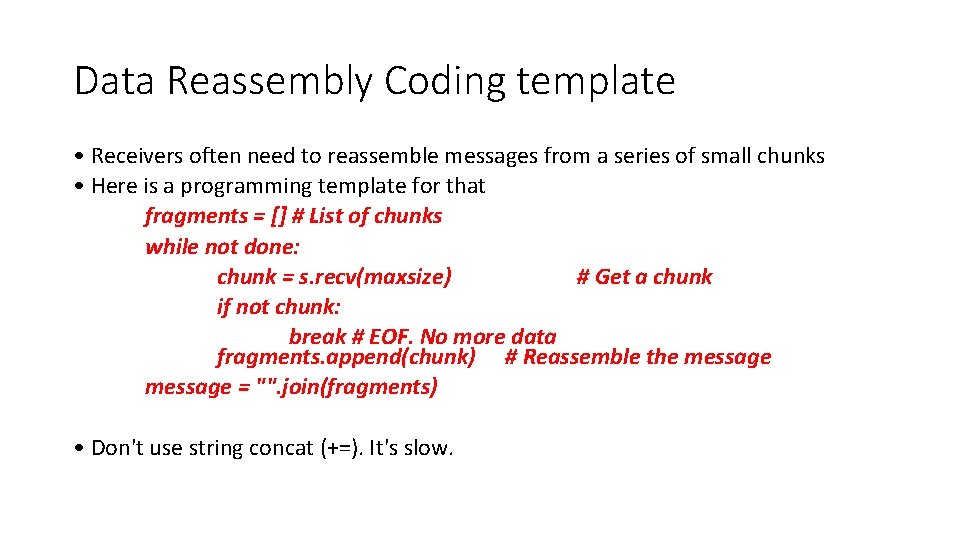
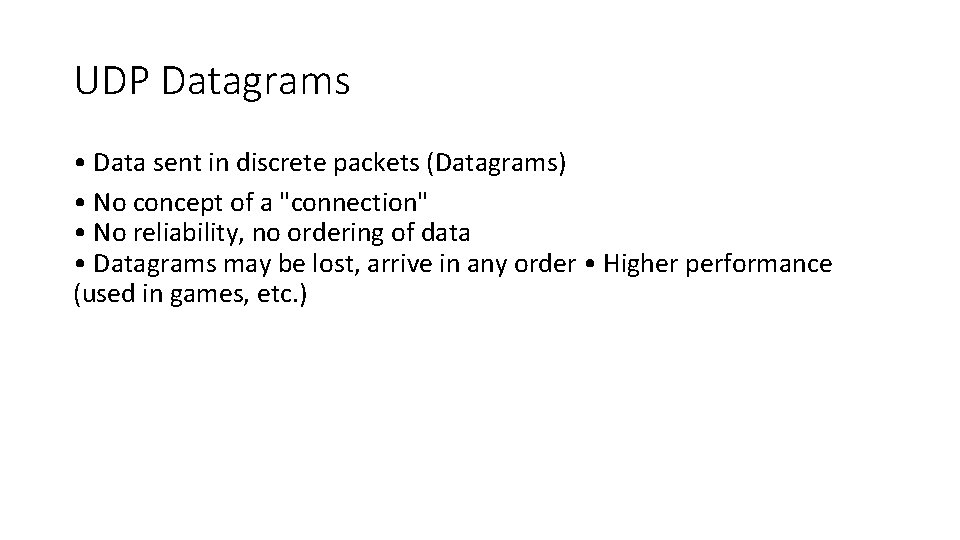
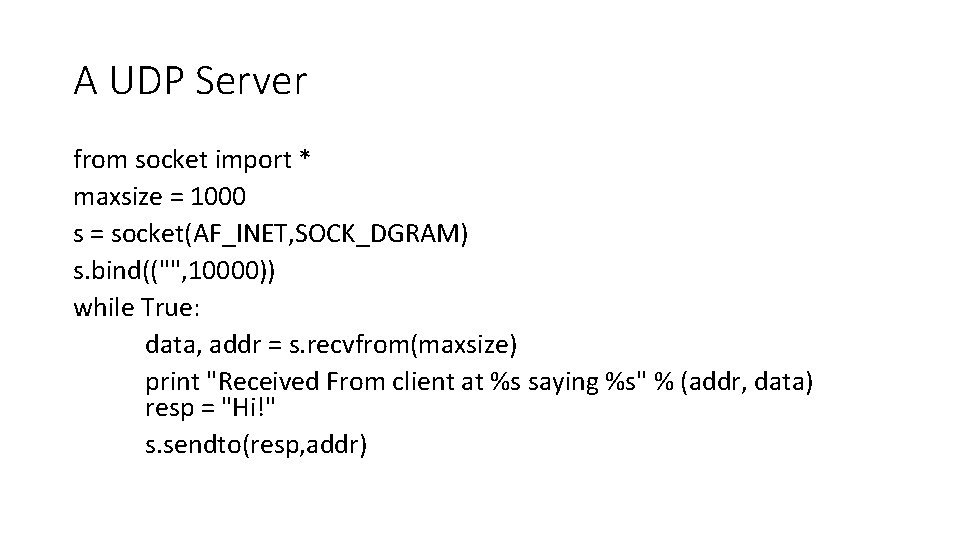
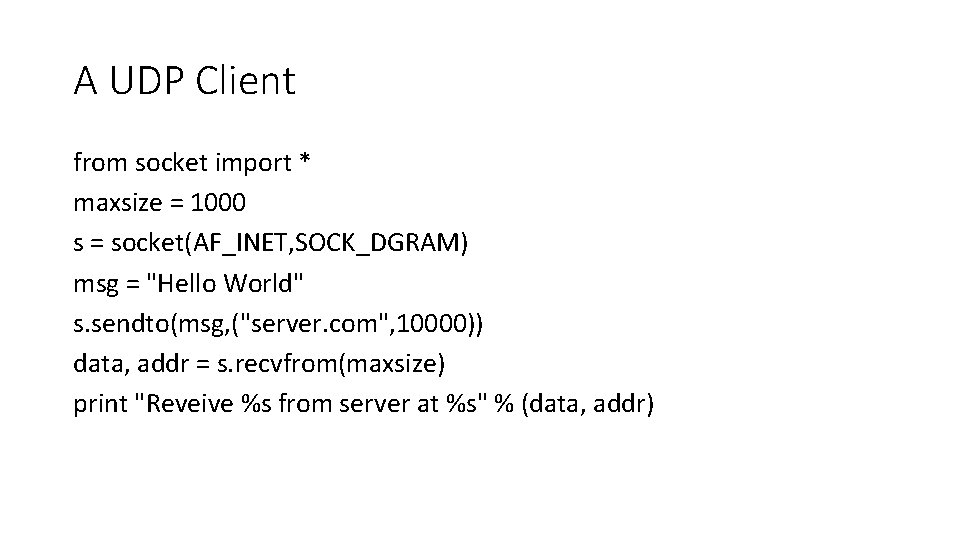
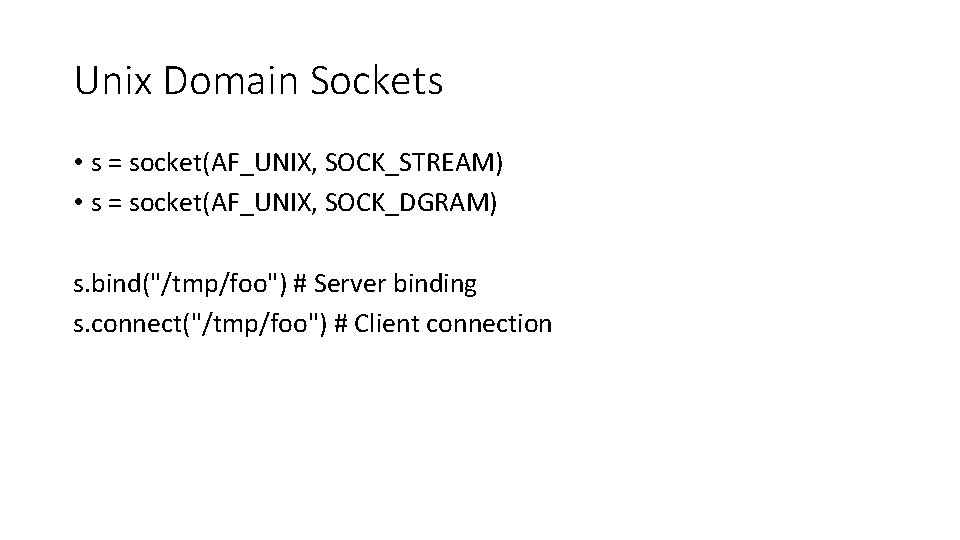
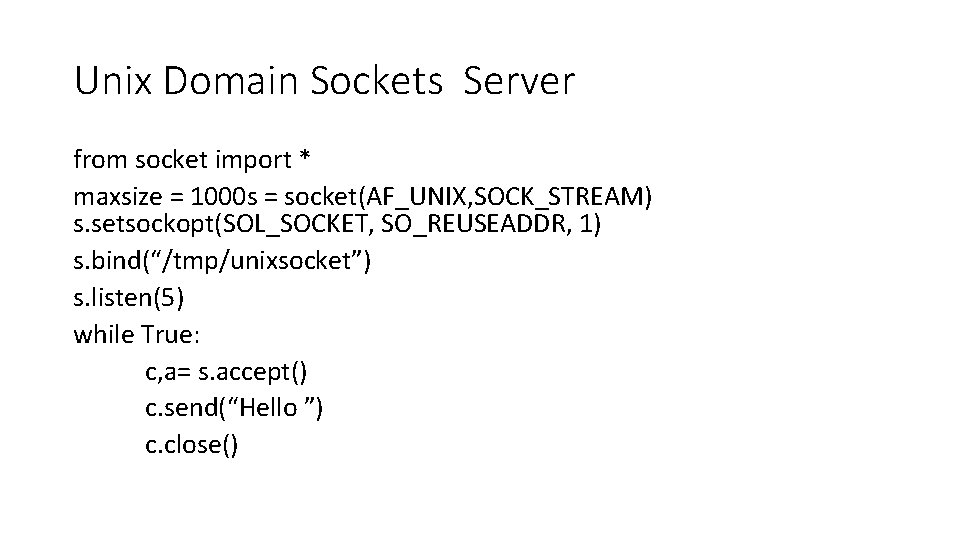
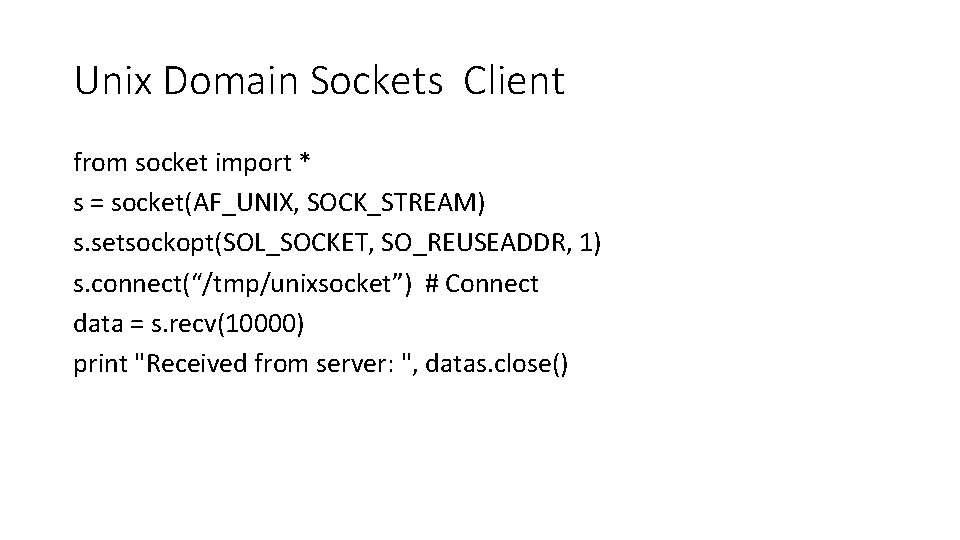
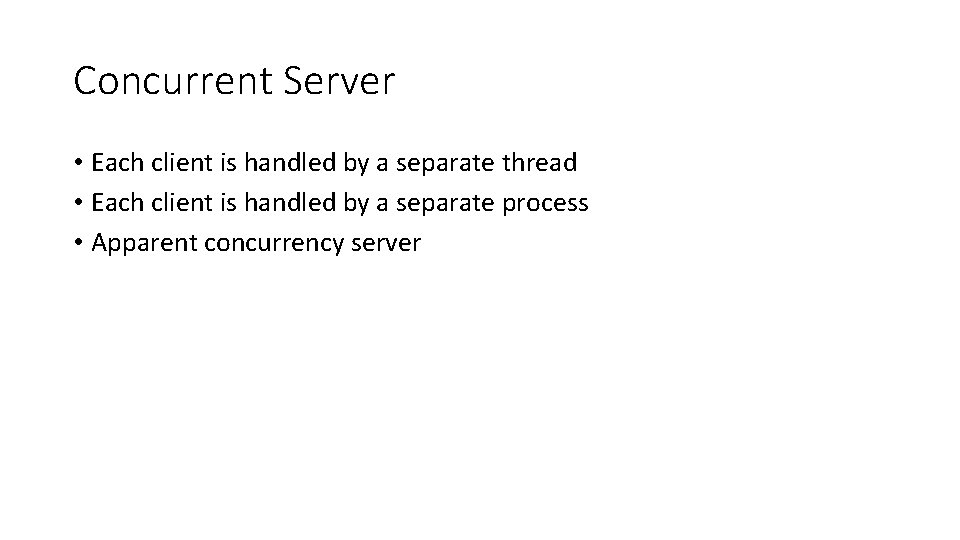
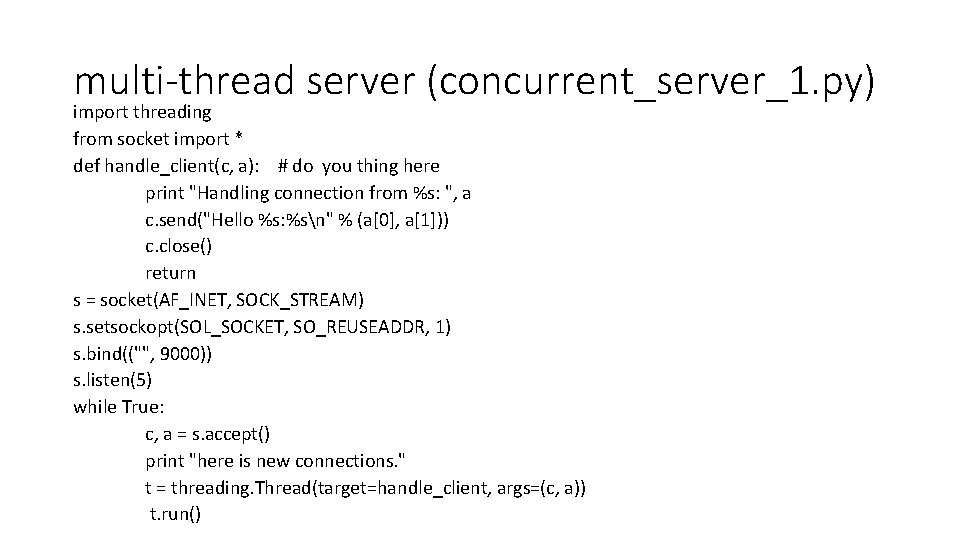
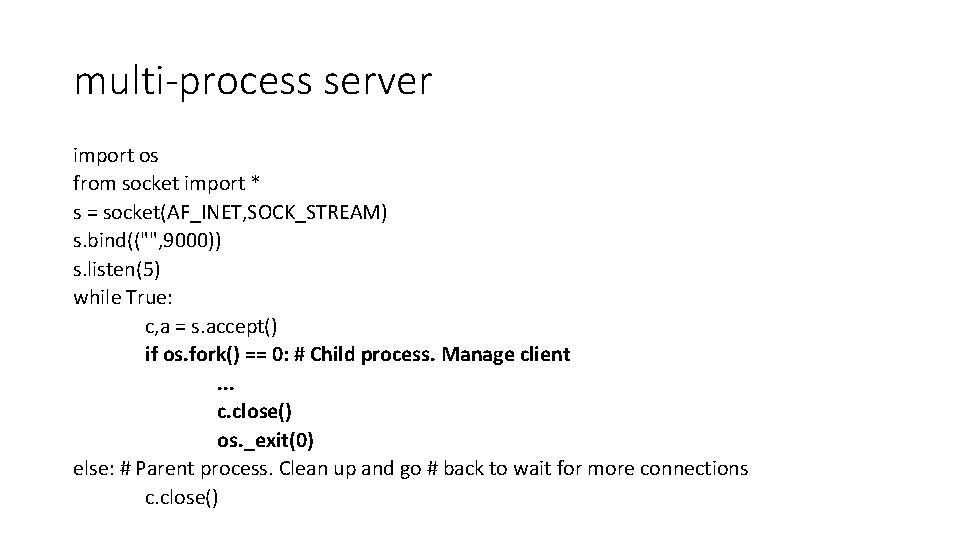
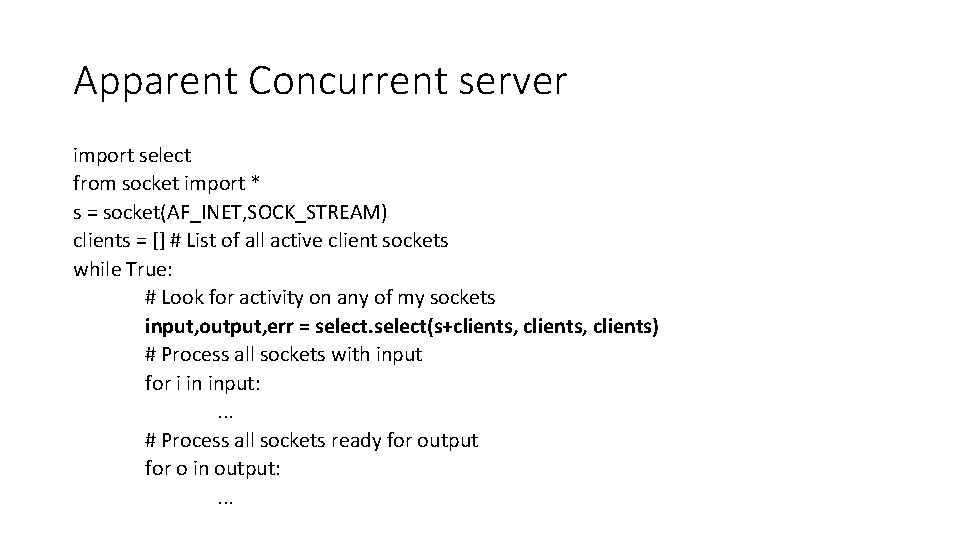
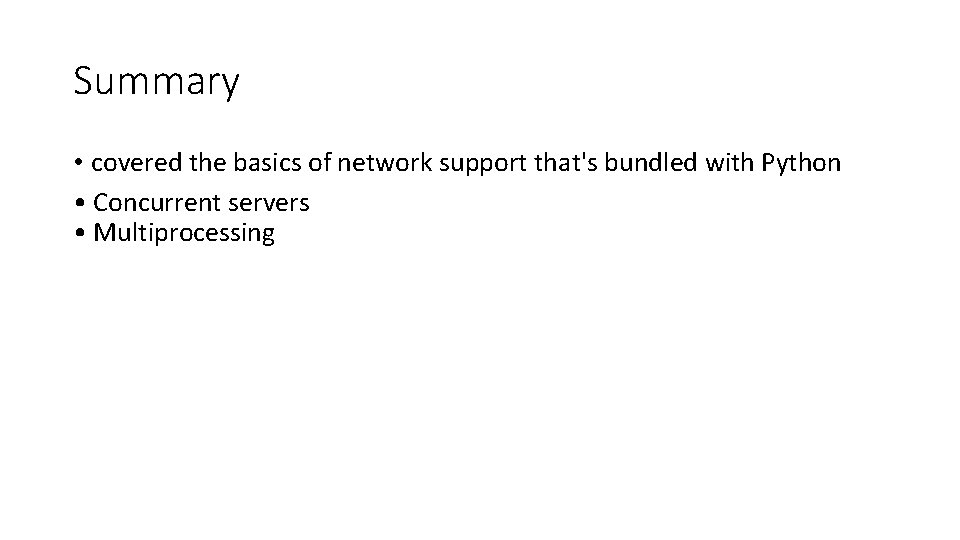
- Slides: 34
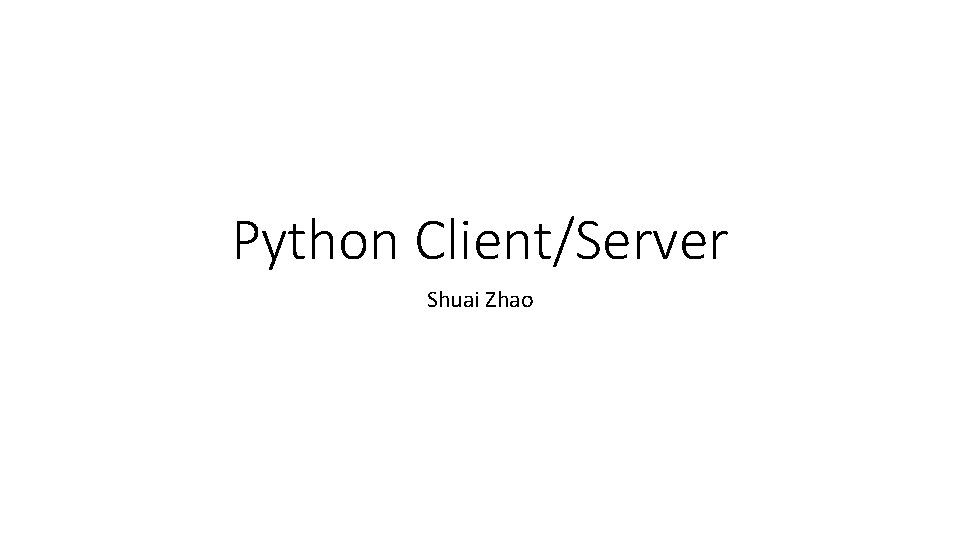
Python Client/Server Shuai Zhao
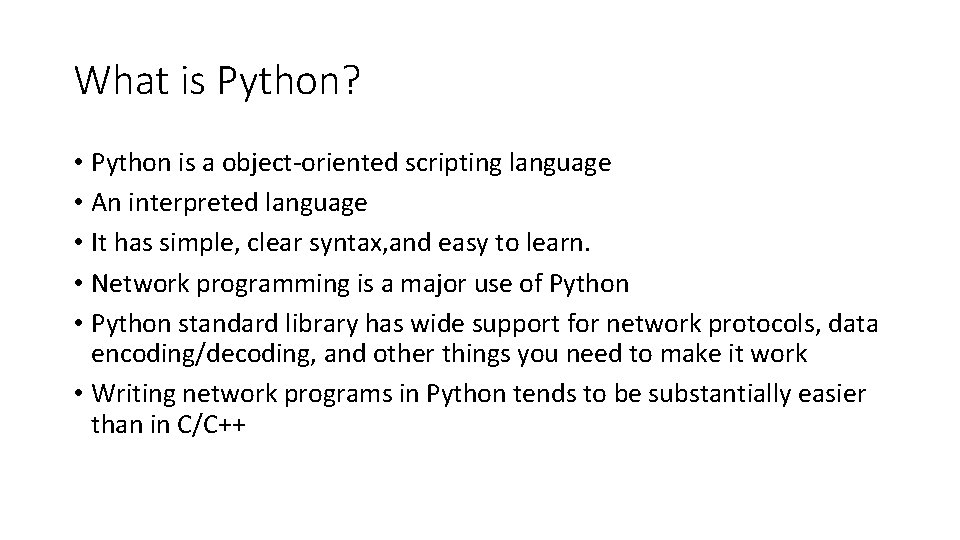
What is Python? • Python is a object-oriented scripting language • An interpreted language • It has simple, clear syntax, and easy to learn. • Network programming is a major use of Python • Python standard library has wide support for network protocols, data encoding/decoding, and other things you need to make it work • Writing network programs in Python tends to be substantially easier than in C/C++
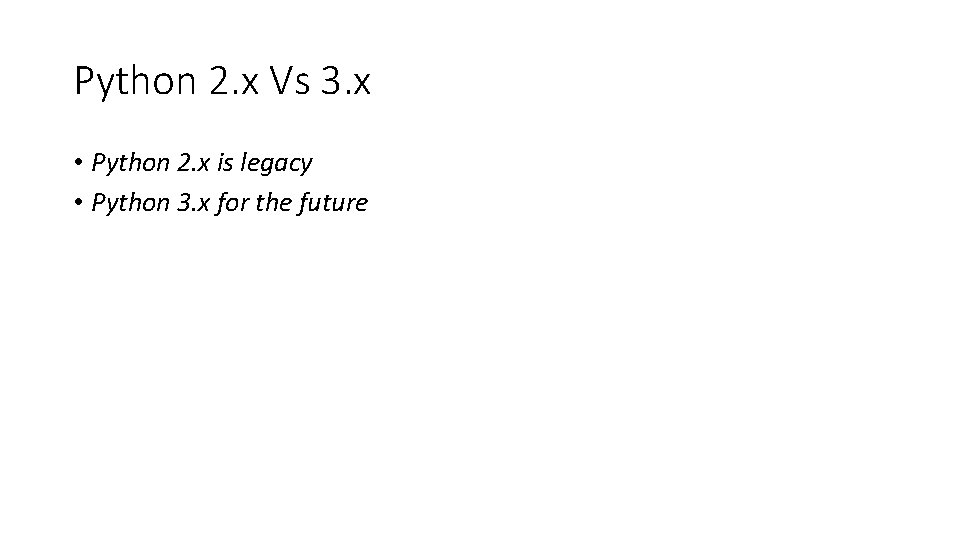
Python 2. x Vs 3. x • Python 2. x is legacy • Python 3. x for the future
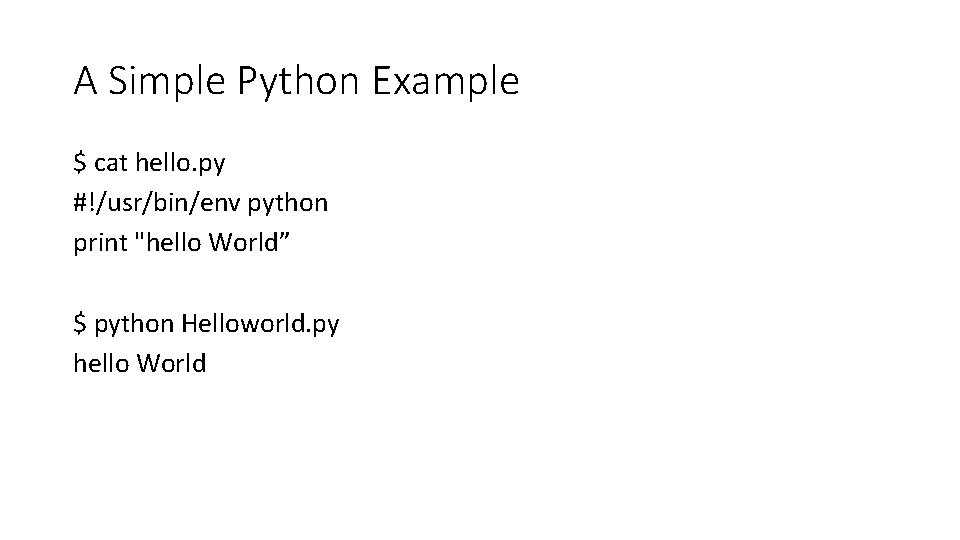
A Simple Python Example $ cat hello. py #!/usr/bin/env python print "hello World” $ python Helloworld. py hello World
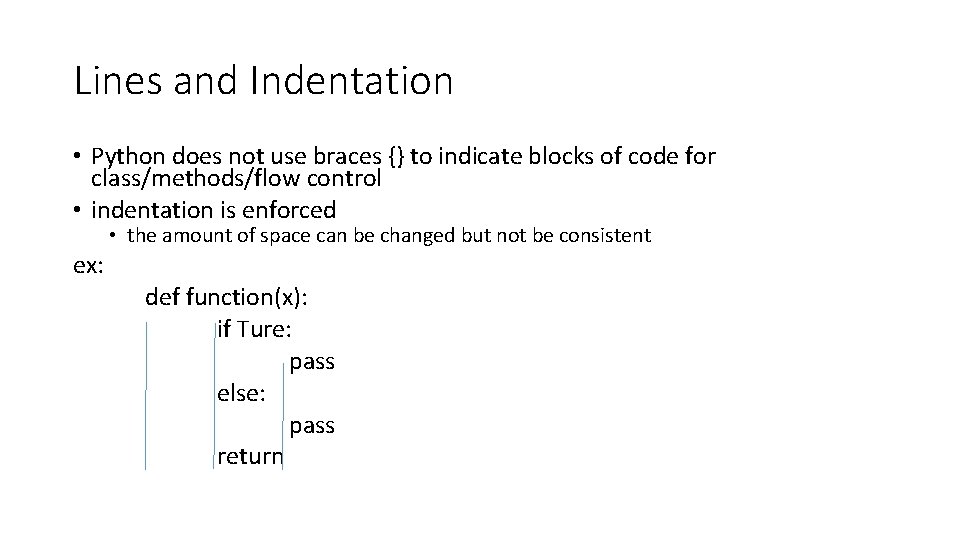
Lines and Indentation • Python does not use braces {} to indicate blocks of code for class/methods/flow control • indentation is enforced • the amount of space can be changed but not be consistent ex: def function(x): if Ture: pass else: pass return
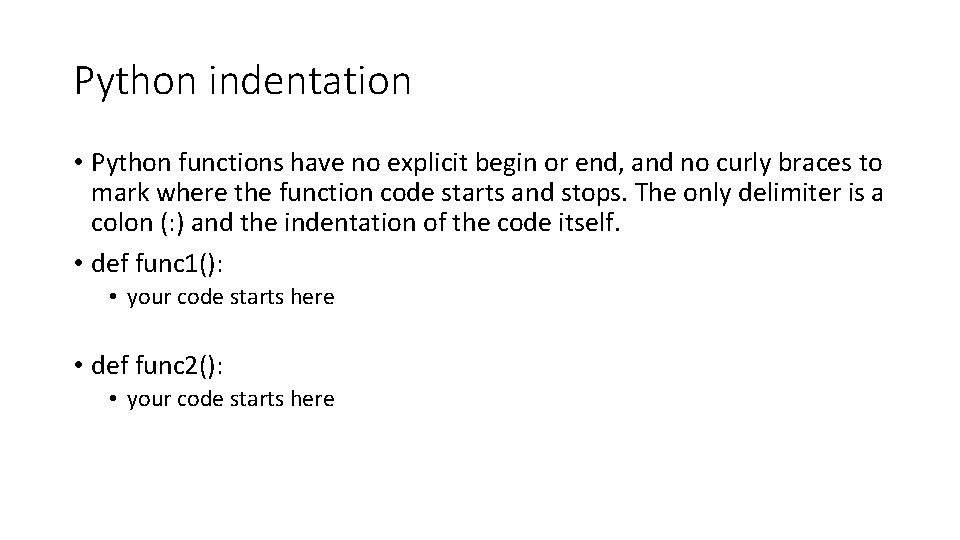
Python indentation • Python functions have no explicit begin or end, and no curly braces to mark where the function code starts and stops. The only delimiter is a colon (: ) and the indentation of the code itself. • def func 1(): • your code starts here • def func 2(): • your code starts here
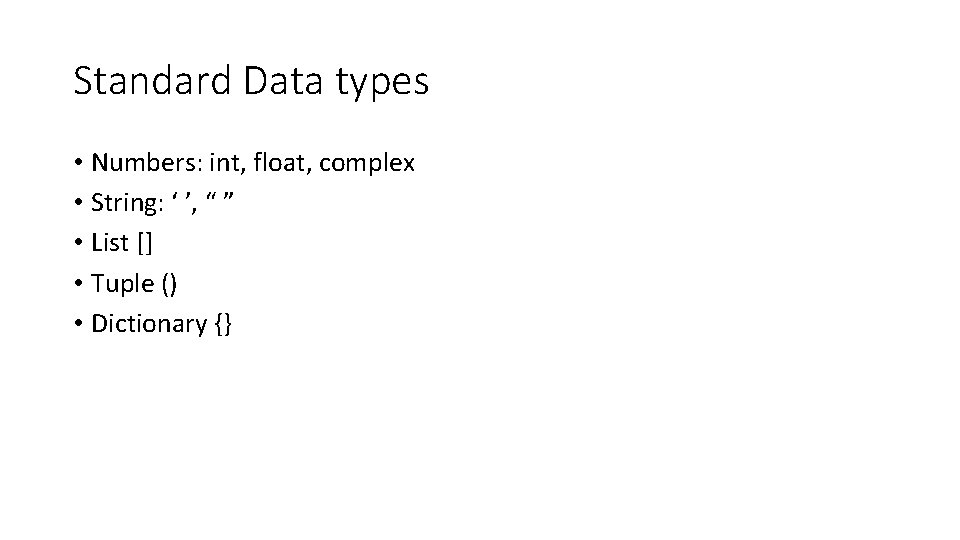
Standard Data types • Numbers: int, float, complex • String: ‘ ’, “ ” • List [] • Tuple () • Dictionary {}
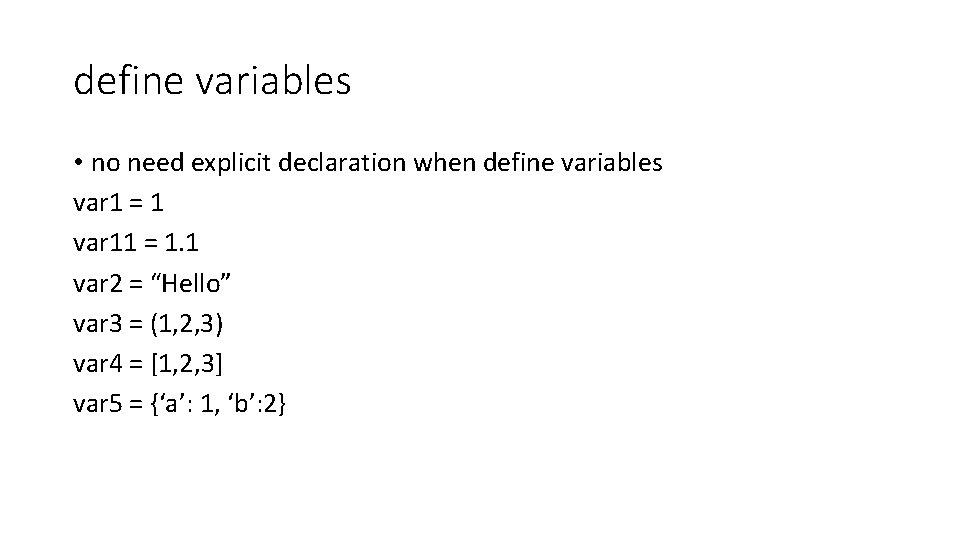
define variables • no need explicit declaration when define variables var 1 = 1 var 11 = 1. 1 var 2 = “Hello” var 3 = (1, 2, 3) var 4 = [1, 2, 3] var 5 = {‘a’: 1, ‘b’: 2}
![Python containers List Tuple dictionary key value Python containers • List [] • Tuple () • dictionary {key: value}](https://slidetodoc.com/presentation_image_h/ed769ba2f8d04bdce0256984e4bff273/image-9.jpg)
Python containers • List [] • Tuple () • dictionary {key: value}
![Python List list 1 1 2 1 abcde print list 10 print list Python List list 1 = [1, 2. 1, ’abcde’] print list 1[0] print list](https://slidetodoc.com/presentation_image_h/ed769ba2f8d04bdce0256984e4bff273/image-10.jpg)
Python List list 1 = [1, 2. 1, ’abcde’] print list 1[0] print list 1[1: 2] list 2 = [‘ 1’, ’ 100 x’] print list 1 + list 2
![Python dictionary dict 1 Key Value dict 1one This is Python dictionary • dict 1 = {‘Key’: Value} • dict 1['one'] = "This is](https://slidetodoc.com/presentation_image_h/ed769ba2f8d04bdce0256984e4bff273/image-11.jpg)
Python dictionary • dict 1 = {‘Key’: Value} • dict 1['one'] = "This is one" • dict 1[2] = "This is two" • another. Dict = {'name': 'john', 'code': 6734, 'dept': 'sales'} • • • print dict['one’]1 print dict[2] print another. Dict. keys() print another. Dict. values() # Prints value for 'one' key # Prints value for 2 key # Prints complete dictionary # Prints all the keys # Prints all the values
![python tuple 1 1 2 1 abcde print tuple 10 print tuple 11 python tuple 1 = (1, 2. 1, ’abcde’) print tuple 1[0] print tuple 1[1:](https://slidetodoc.com/presentation_image_h/ed769ba2f8d04bdce0256984e4bff273/image-12.jpg)
python tuple 1 = (1, 2. 1, ’abcde’) print tuple 1[0] print tuple 1[1: 2] tuple 2 = [‘ 1’, ’ 100 x’] print tuple 1 + tuple 2
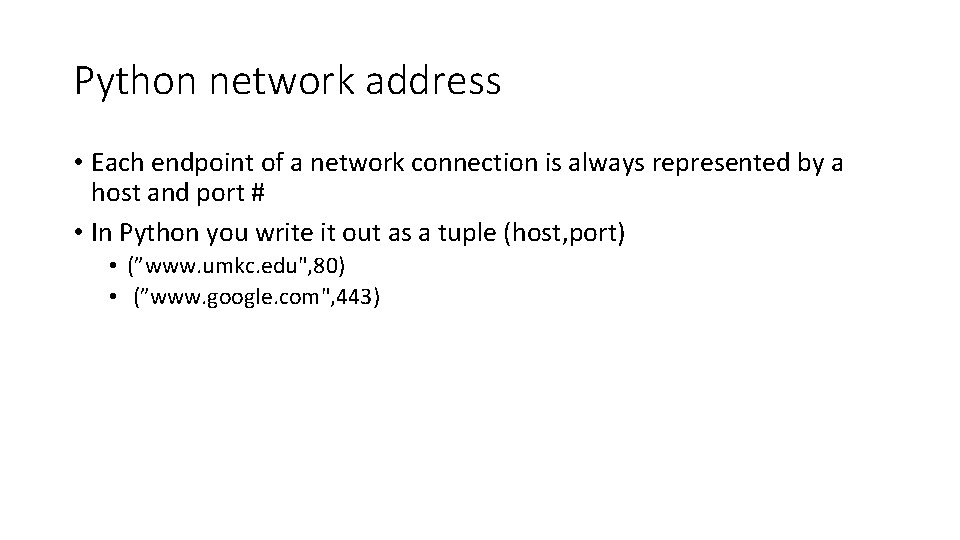
Python network address • Each endpoint of a network connection is always represented by a host and port # • In Python you write it out as a tuple (host, port) • (”www. umkc. edu", 80) • (”www. google. com", 443)
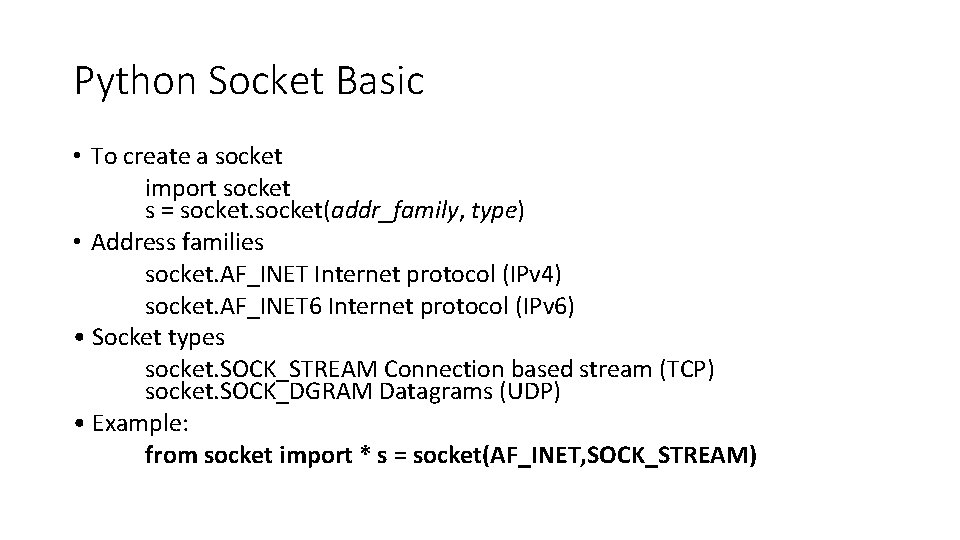
Python Socket Basic • To create a socket import socket s = socket(addr_family, type) • Address families socket. AF_INET Internet protocol (IPv 4) socket. AF_INET 6 Internet protocol (IPv 6) • Socket types socket. SOCK_STREAM Connection based stream (TCP) socket. SOCK_DGRAM Datagrams (UDP) • Example: from socket import * s = socket(AF_INET, SOCK_STREAM)
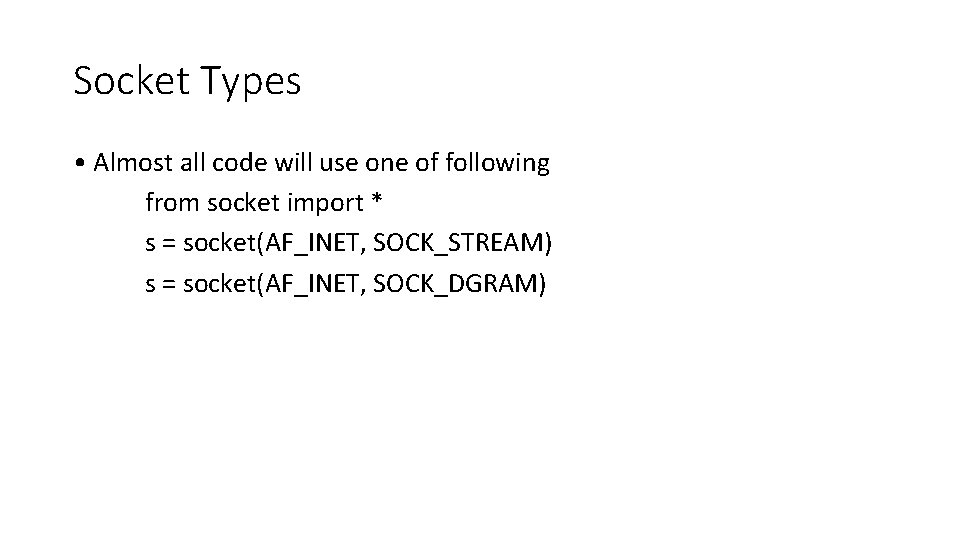
Socket Types • Almost all code will use one of following from socket import * s = socket(AF_INET, SOCK_STREAM) s = socket(AF_INET, SOCK_DGRAM)
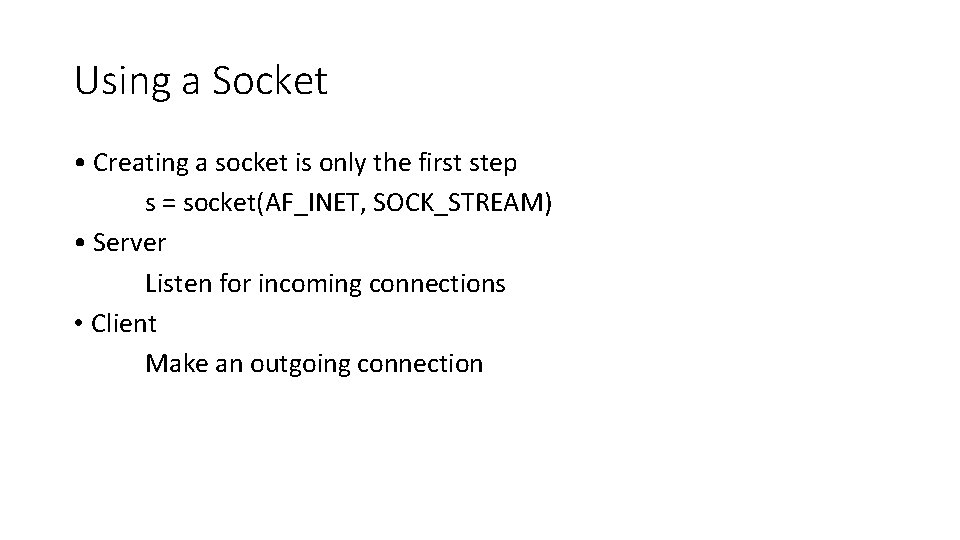
Using a Socket • Creating a socket is only the first step s = socket(AF_INET, SOCK_STREAM) • Server Listen for incoming connections • Client Make an outgoing connection
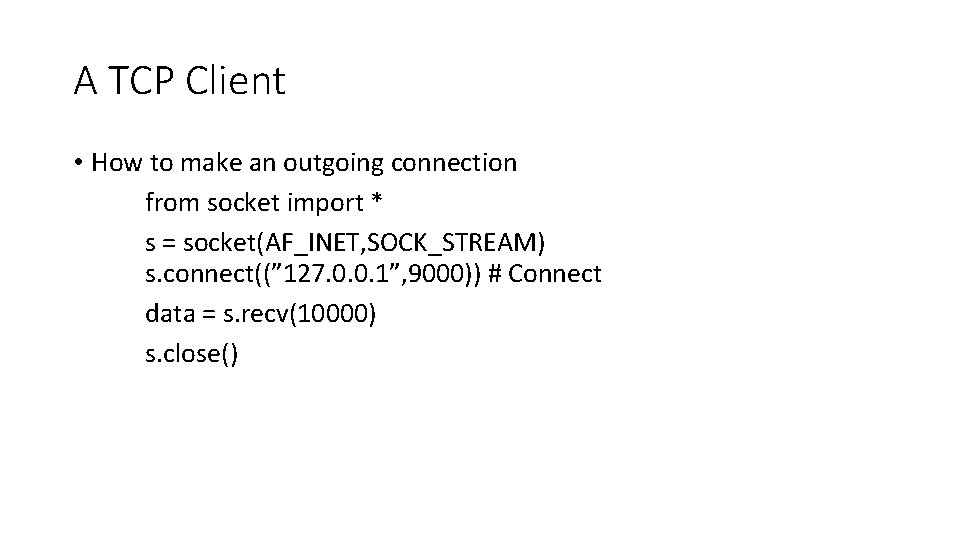
A TCP Client • How to make an outgoing connection from socket import * s = socket(AF_INET, SOCK_STREAM) s. connect((” 127. 0. 0. 1”, 9000)) # Connect data = s. recv(10000) s. close()
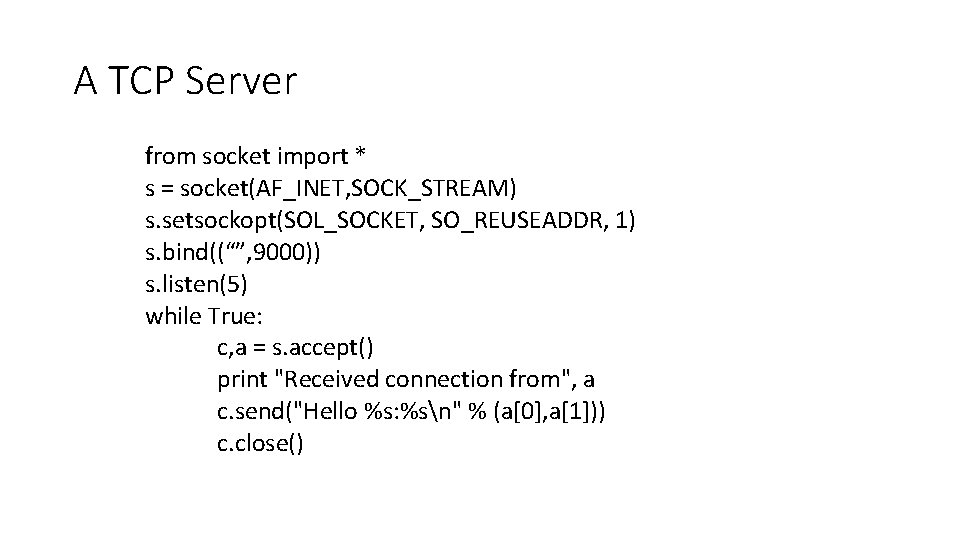
A TCP Server from socket import * s = socket(AF_INET, SOCK_STREAM) s. setsockopt(SOL_SOCKET, SO_REUSEADDR, 1) s. bind((“”, 9000)) s. listen(5) while True: c, a = s. accept() print "Received connection from", a c. send("Hello %s: %sn" % (a[0], a[1])) c. close()
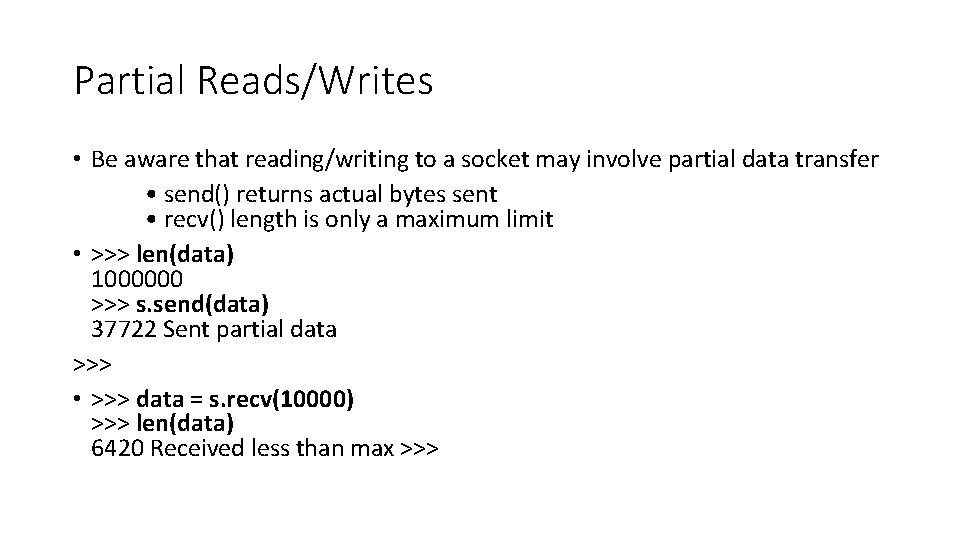
Partial Reads/Writes • Be aware that reading/writing to a socket may involve partial data transfer • send() returns actual bytes sent • recv() length is only a maximum limit • >>> len(data) 1000000 >>> s. send(data) 37722 Sent partial data >>> • >>> data = s. recv(10000) >>> len(data) 6420 Received less than max >>>
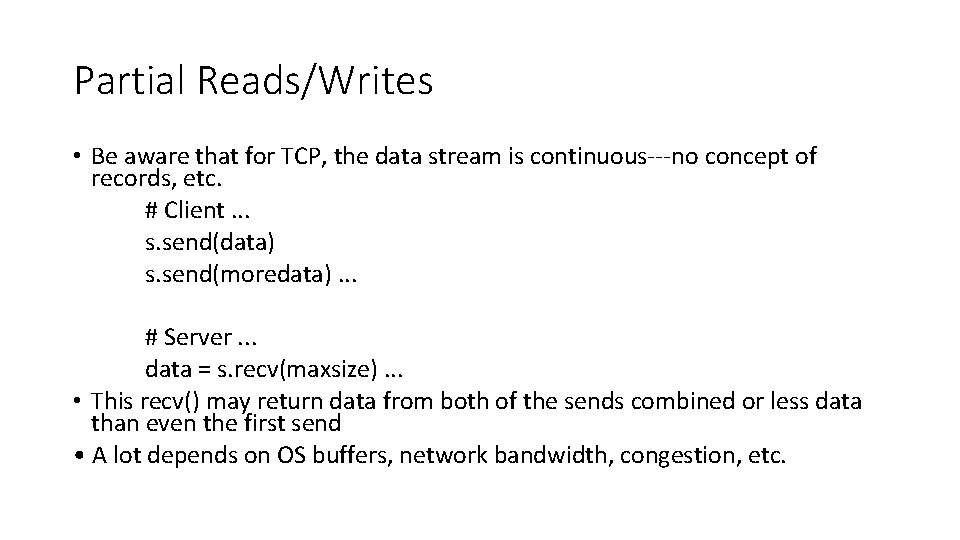
Partial Reads/Writes • Be aware that for TCP, the data stream is continuous---no concept of records, etc. # Client. . . s. send(data) s. send(moredata). . . # Server. . . data = s. recv(maxsize). . . • This recv() may return data from both of the sends combined or less data than even the first send • A lot depends on OS buffers, network bandwidth, congestion, etc.
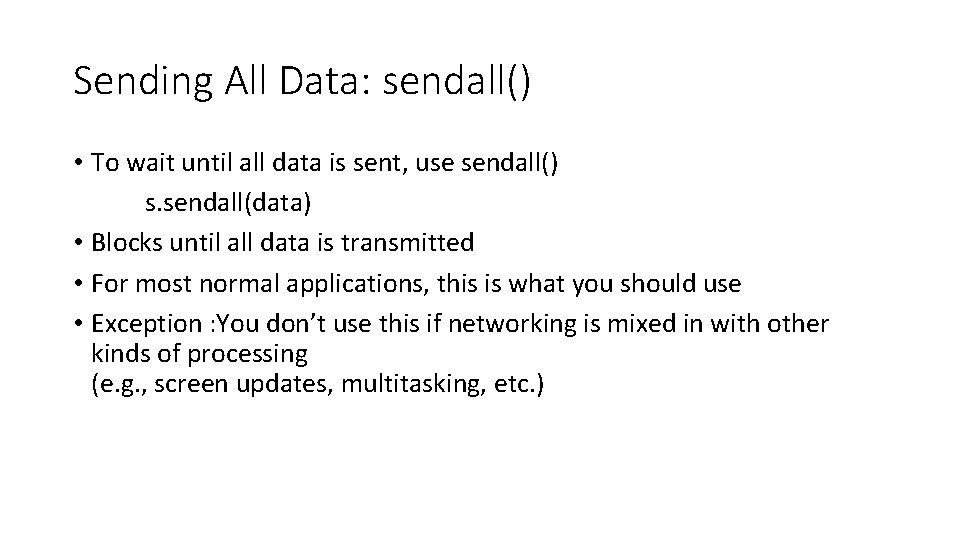
Sending All Data: sendall() • To wait until all data is sent, use sendall() s. sendall(data) • Blocks until all data is transmitted • For most normal applications, this is what you should use • Exception : You don’t use this if networking is mixed in with other kinds of processing (e. g. , screen updates, multitasking, etc. )
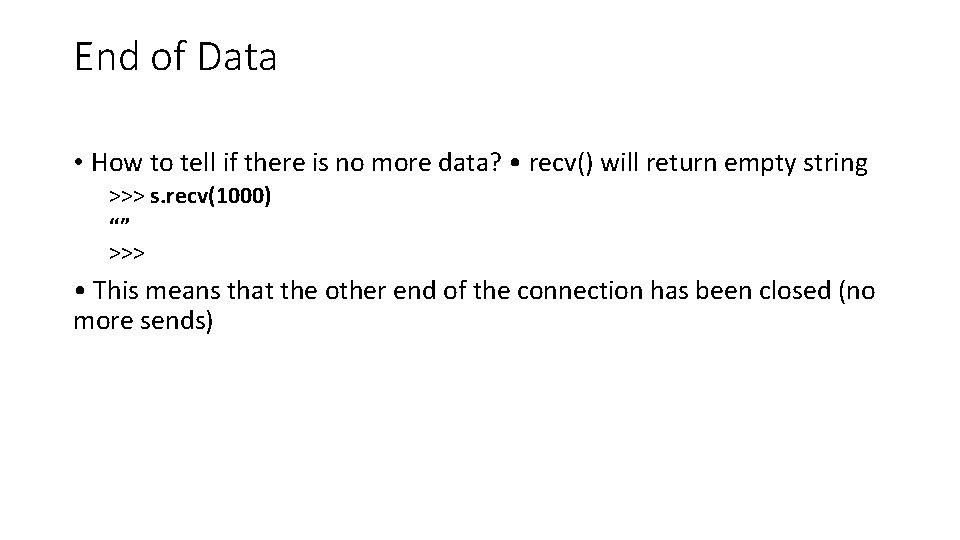
End of Data • How to tell if there is no more data? • recv() will return empty string >>> s. recv(1000) “” >>> • This means that the other end of the connection has been closed (no more sends)
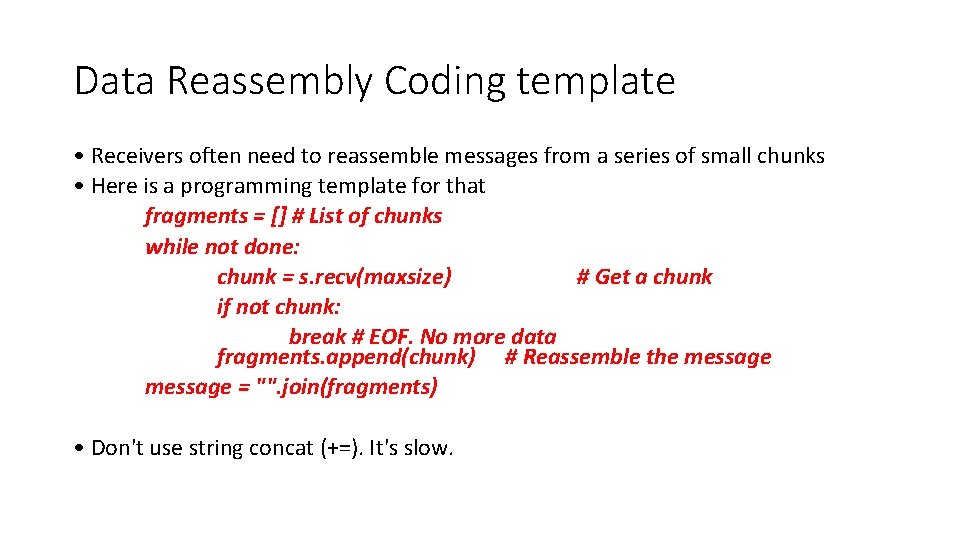
Data Reassembly Coding template • Receivers often need to reassemble messages from a series of small chunks • Here is a programming template for that fragments = [] # List of chunks while not done: chunk = s. recv(maxsize) # Get a chunk if not chunk: break # EOF. No more data fragments. append(chunk) # Reassemble the message = "". join(fragments) • Don't use string concat (+=). It's slow.
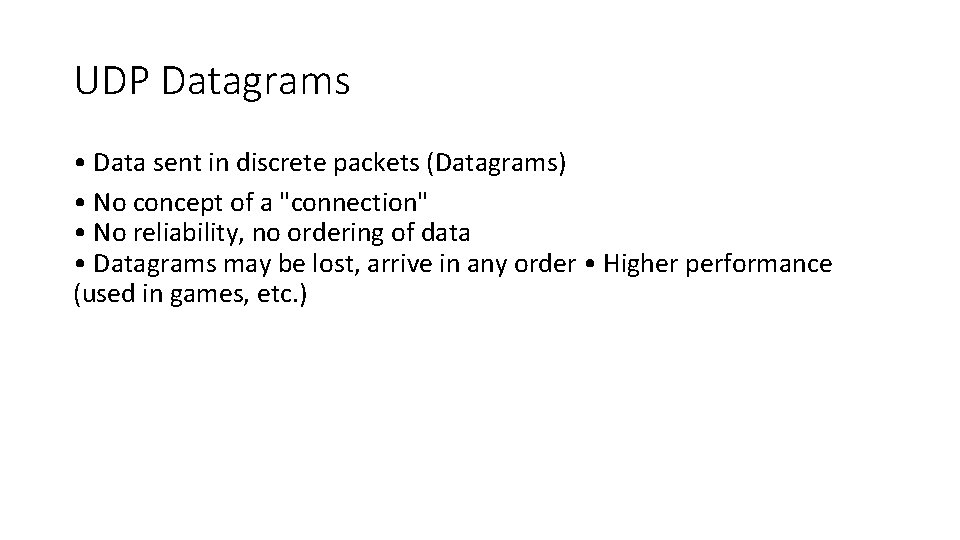
UDP Datagrams • Data sent in discrete packets (Datagrams) • No concept of a "connection" • No reliability, no ordering of data • Datagrams may be lost, arrive in any order • Higher performance (used in games, etc. )
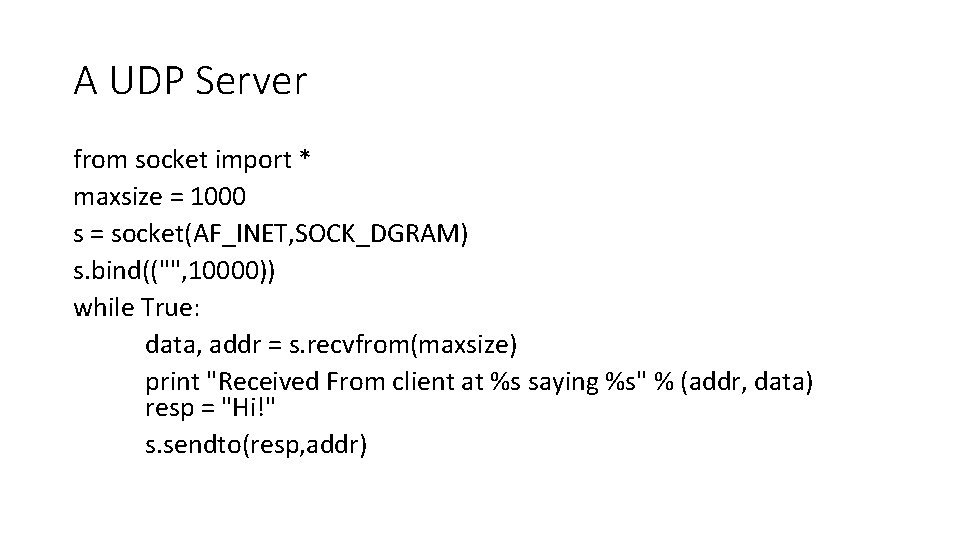
A UDP Server from socket import * maxsize = 1000 s = socket(AF_INET, SOCK_DGRAM) s. bind(("", 10000)) while True: data, addr = s. recvfrom(maxsize) print "Received From client at %s saying %s" % (addr, data) resp = "Hi!" s. sendto(resp, addr)
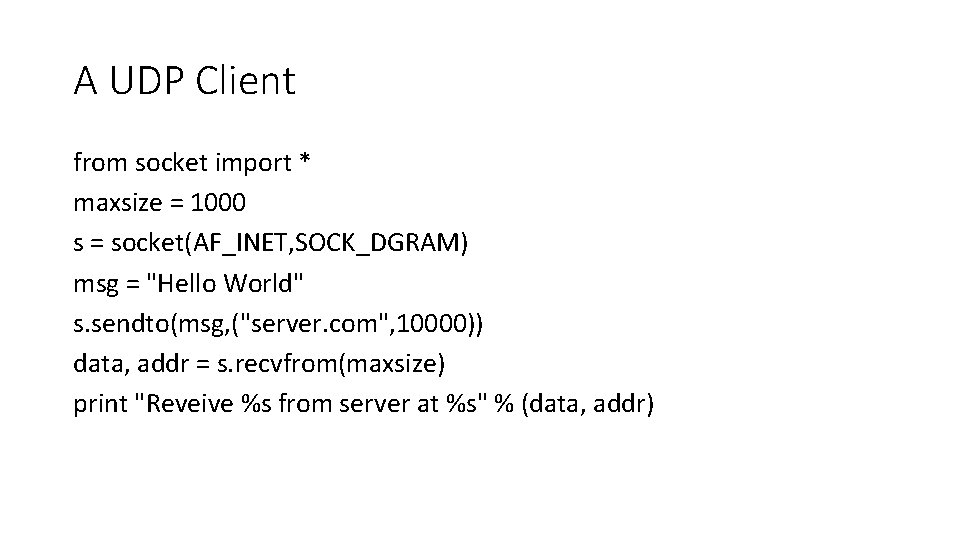
A UDP Client from socket import * maxsize = 1000 s = socket(AF_INET, SOCK_DGRAM) msg = "Hello World" s. sendto(msg, ("server. com", 10000)) data, addr = s. recvfrom(maxsize) print "Reveive %s from server at %s" % (data, addr)
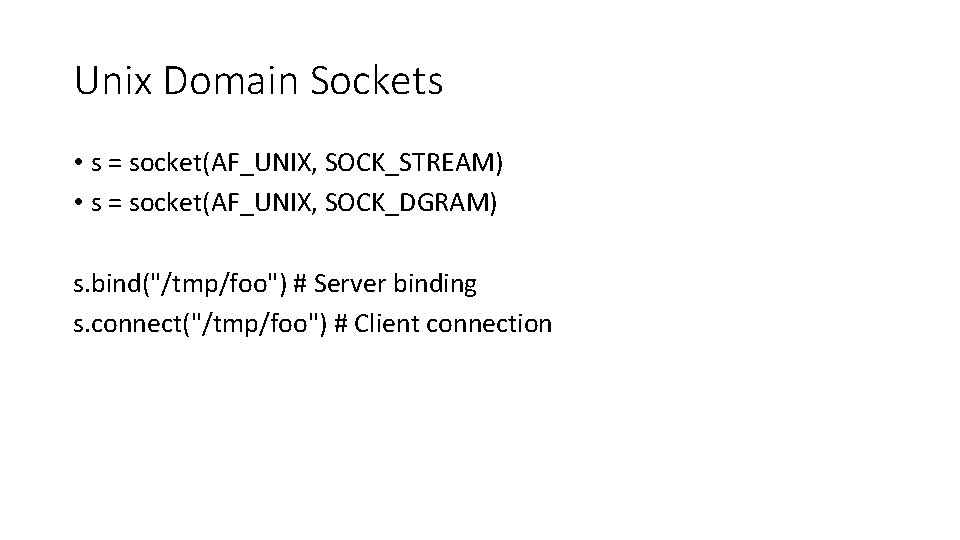
Unix Domain Sockets • s = socket(AF_UNIX, SOCK_STREAM) • s = socket(AF_UNIX, SOCK_DGRAM) s. bind("/tmp/foo") # Server binding s. connect("/tmp/foo") # Client connection
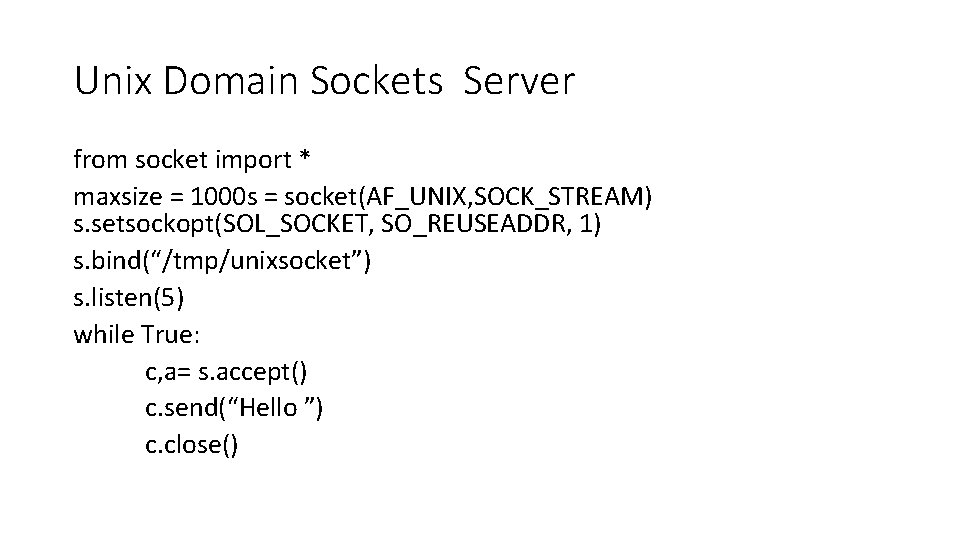
Unix Domain Sockets Server from socket import * maxsize = 1000 s = socket(AF_UNIX, SOCK_STREAM) s. setsockopt(SOL_SOCKET, SO_REUSEADDR, 1) s. bind(“/tmp/unixsocket”) s. listen(5) while True: c, a= s. accept() c. send(“Hello ”) c. close()
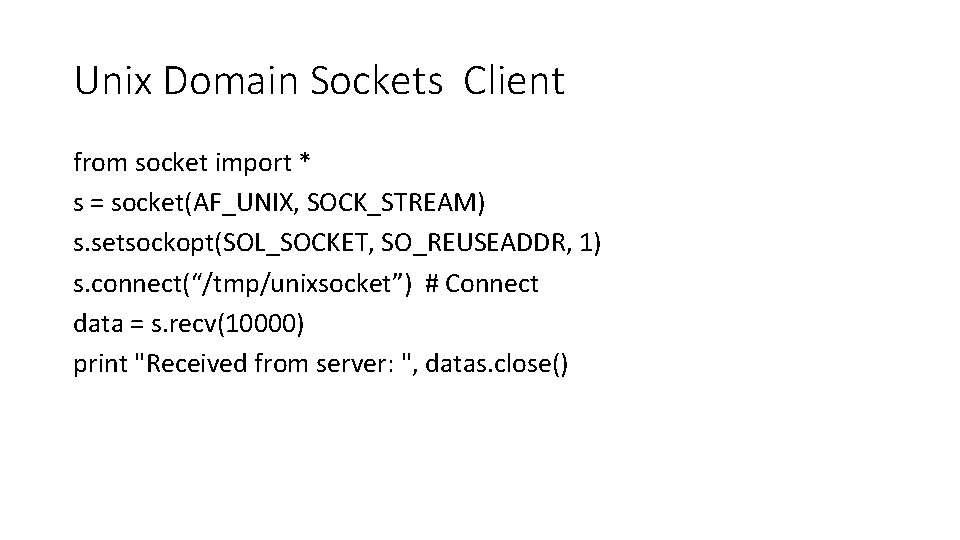
Unix Domain Sockets Client from socket import * s = socket(AF_UNIX, SOCK_STREAM) s. setsockopt(SOL_SOCKET, SO_REUSEADDR, 1) s. connect(“/tmp/unixsocket”) # Connect data = s. recv(10000) print "Received from server: ", datas. close()
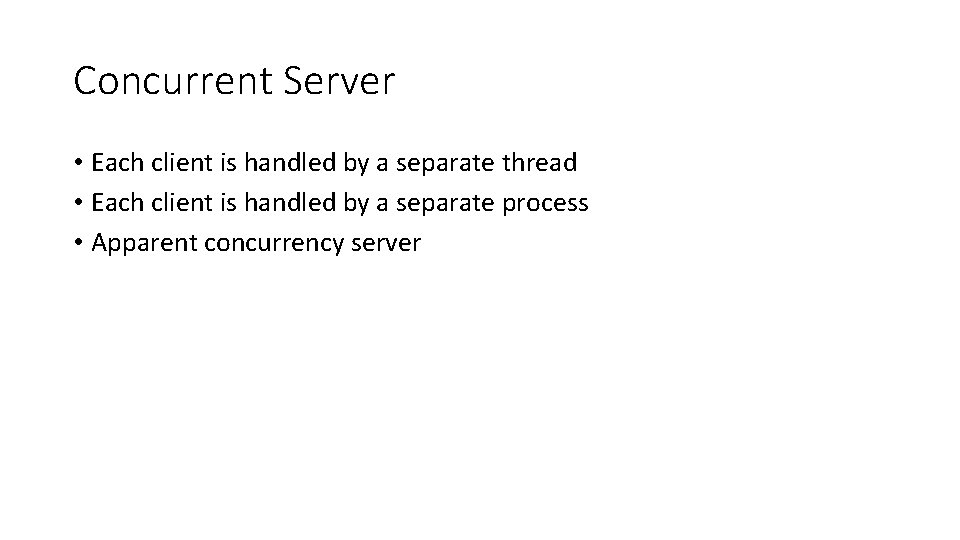
Concurrent Server • Each client is handled by a separate thread • Each client is handled by a separate process • Apparent concurrency server
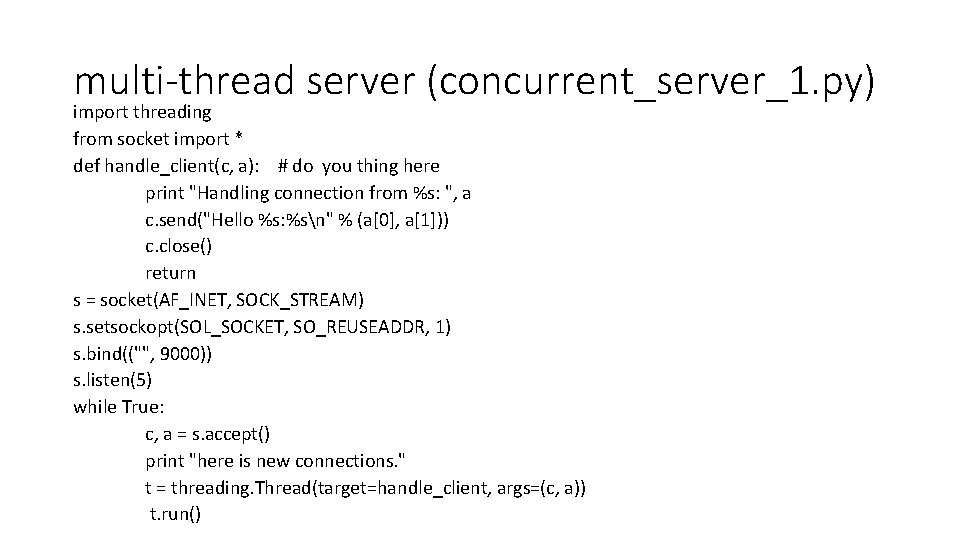
multi-thread server (concurrent_server_1. py) import threading from socket import * def handle_client(c, a): # do you thing here print "Handling connection from %s: ", a c. send("Hello %s: %sn" % (a[0], a[1])) c. close() return s = socket(AF_INET, SOCK_STREAM) s. setsockopt(SOL_SOCKET, SO_REUSEADDR, 1) s. bind(("", 9000)) s. listen(5) while True: c, a = s. accept() print "here is new connections. " t = threading. Thread(target=handle_client, args=(c, a)) t. run()
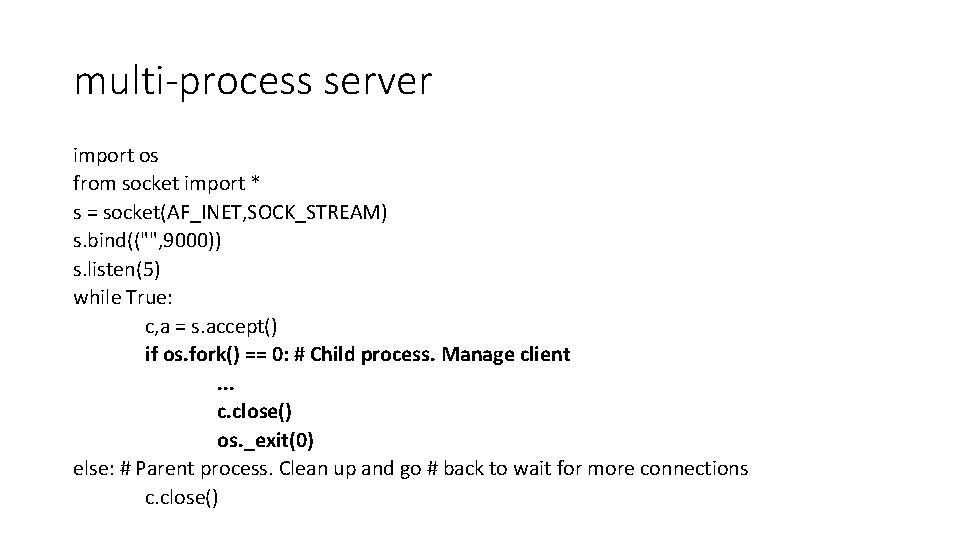
multi-process server import os from socket import * s = socket(AF_INET, SOCK_STREAM) s. bind(("", 9000)) s. listen(5) while True: c, a = s. accept() if os. fork() == 0: # Child process. Manage client. . . c. close() os. _exit(0) else: # Parent process. Clean up and go # back to wait for more connections c. close()
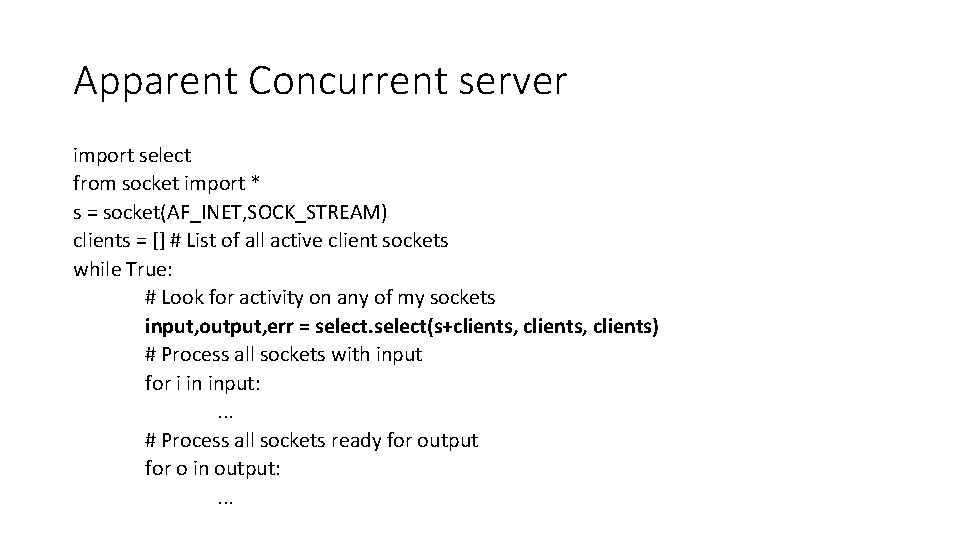
Apparent Concurrent server import select from socket import * s = socket(AF_INET, SOCK_STREAM) clients = [] # List of all active client sockets while True: # Look for activity on any of my sockets input, output, err = select(s+clients, clients) # Process all sockets with input for i in input: . . . # Process all sockets ready for output for o in output: . . .
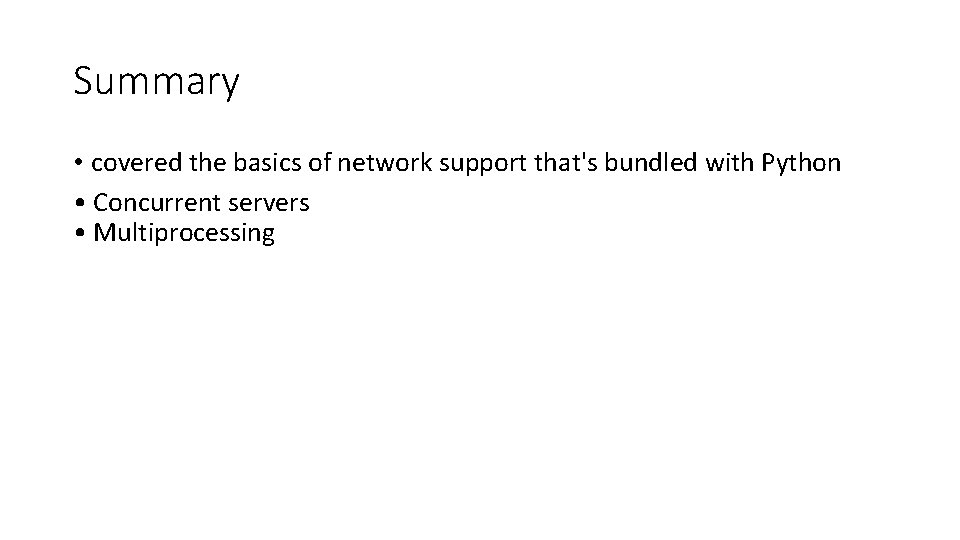
Summary • covered the basics of network support that's bundled with Python • Concurrent servers • Multiprocessing
Clientserver network
Clientserver model
Clientserver architecture
Clientserver model
System.net.peertopeer
Zhao dao
Amanda zhao
Hui zhao unlv
Shangqing zhao
Elise brown oakland university
Zhao yuqi
Wenbing zhao
Xin zhao challenge
David zhao wake forest
Jennifer wissink cornell
Amanda zhao
Mingda zhao
Ben y zhao
Irene zhao
Zhao cuiwei
David zhao md
Random sampling over joins revisited
Dr yi chen zhao
Zhengji zhao
Princess zhao of pingyang
Jeremy zhao
"tcm"
Zhao qian malaysia
Mingmin zhao mit
Zhao chun
Wenbing zhao
Zhao tingyang
Methods python list
How was python created
P2var