Systems Architecture ClientServer Systems 14 ClientServer CSC 407
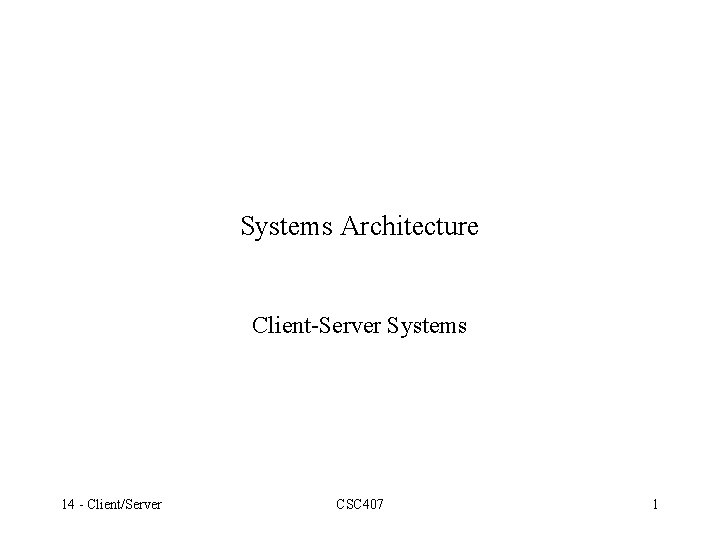
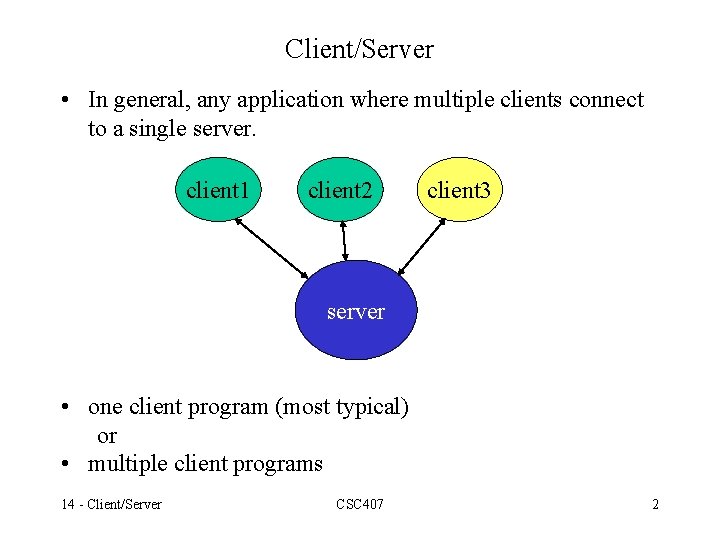
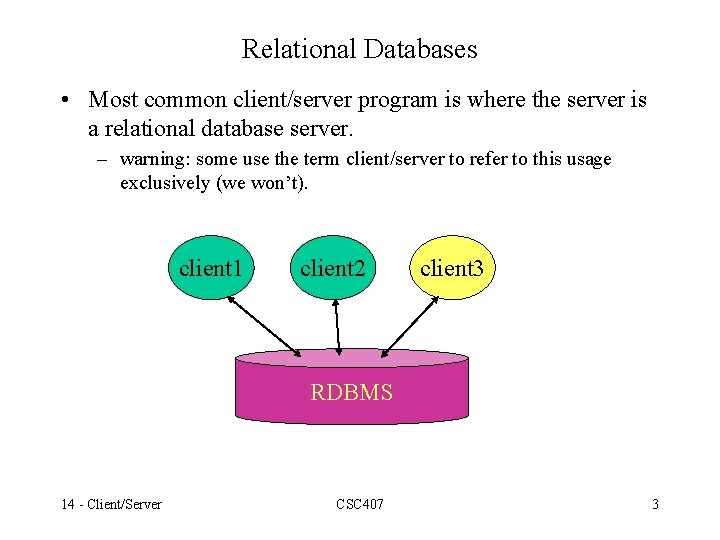
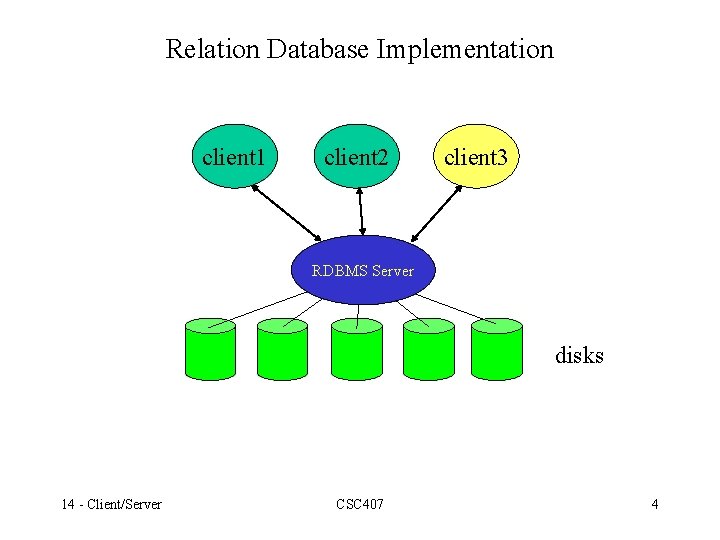
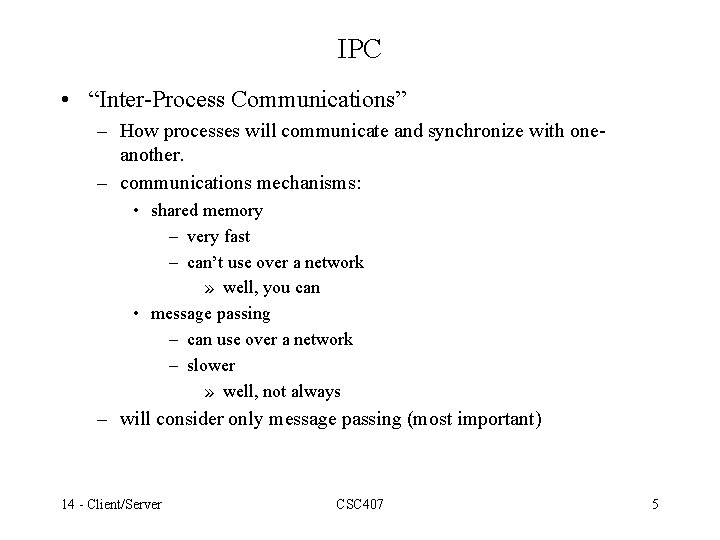
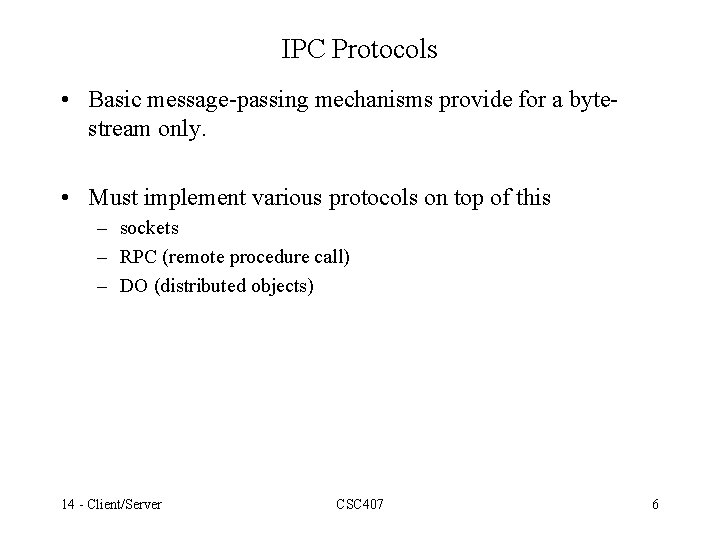
![Sockets code example public class Server { public static void main(String[] args) throws Exception Sockets code example public class Server { public static void main(String[] args) throws Exception](https://slidetodoc.com/presentation_image/f1fb74f5874f1b32885e5a8b3cef486f/image-7.jpg)
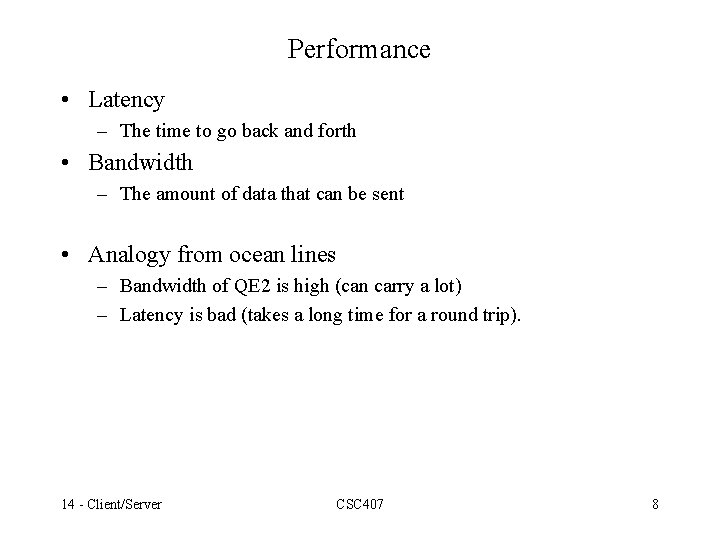
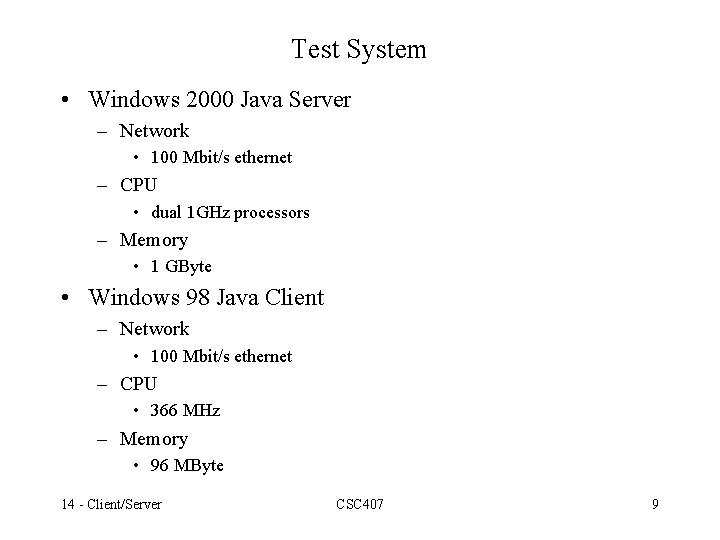
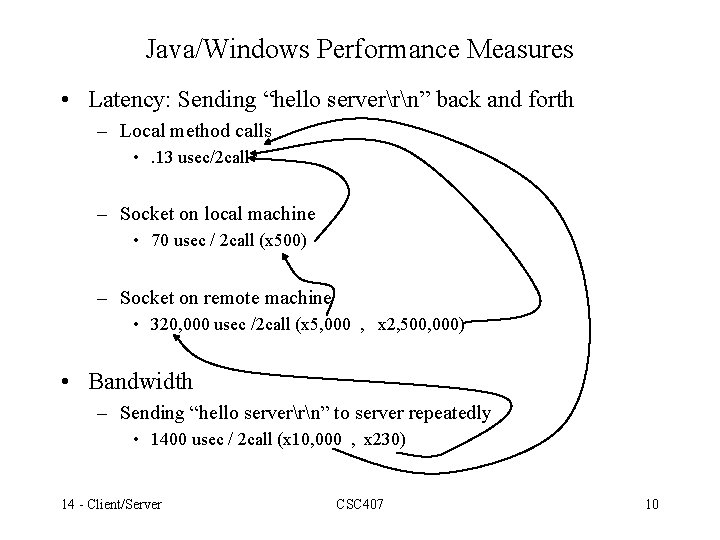
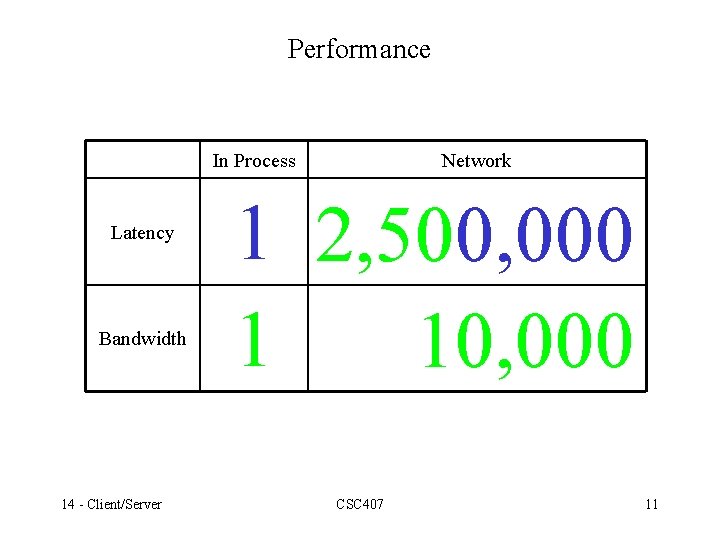
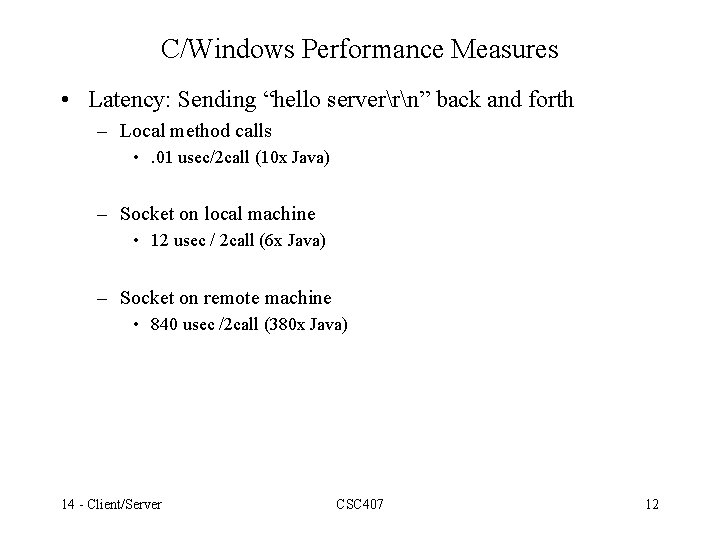
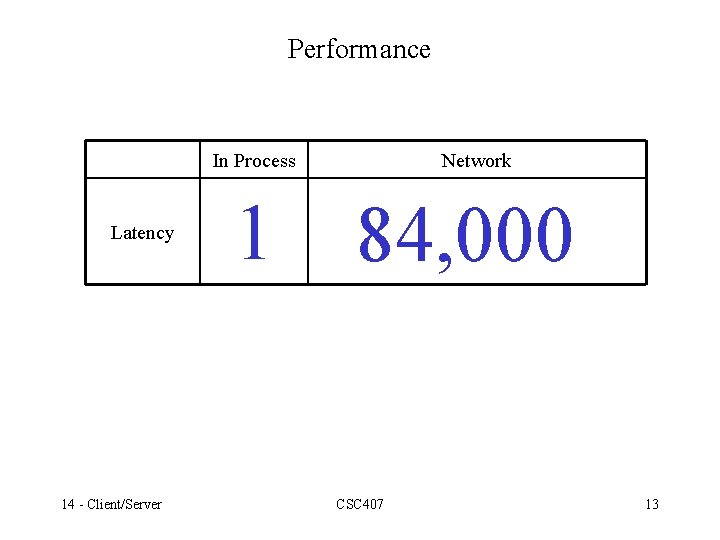
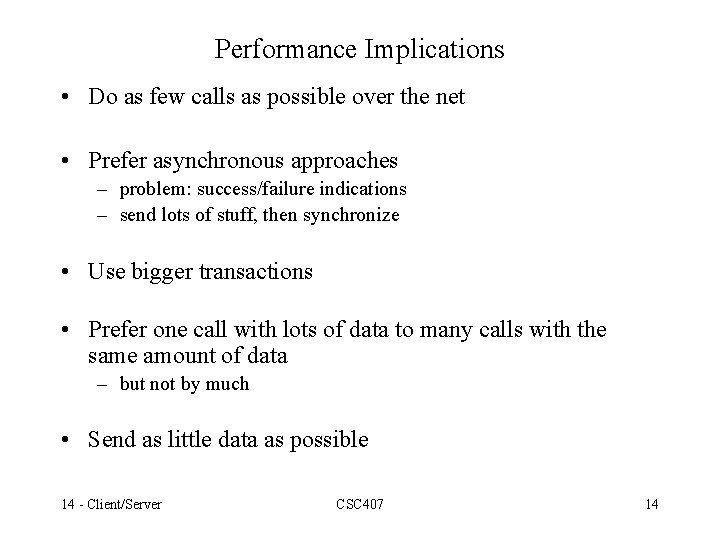
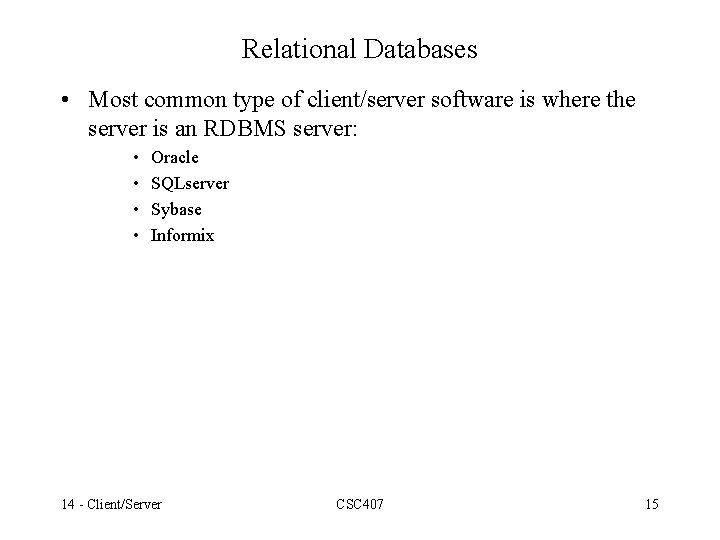
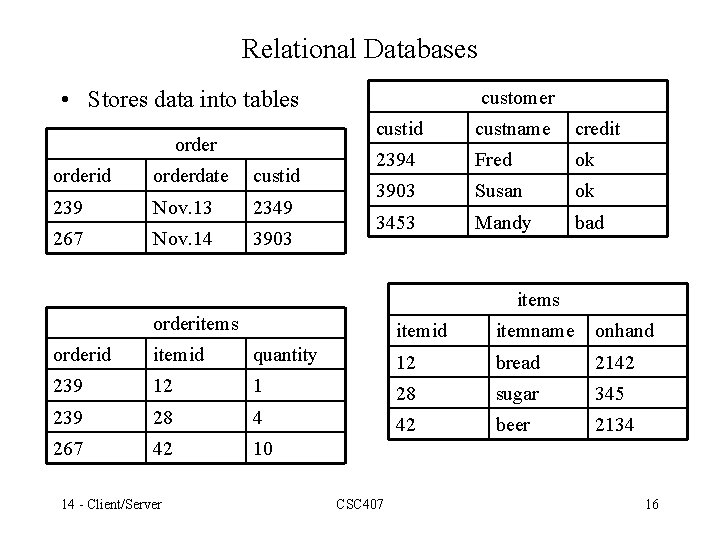
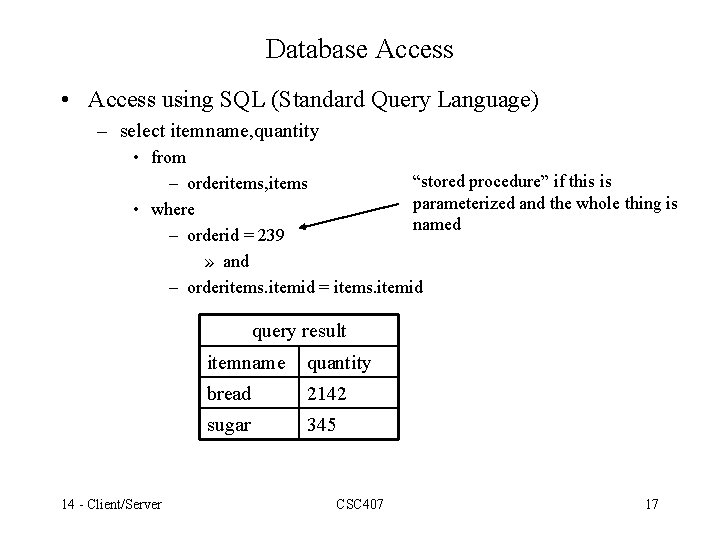
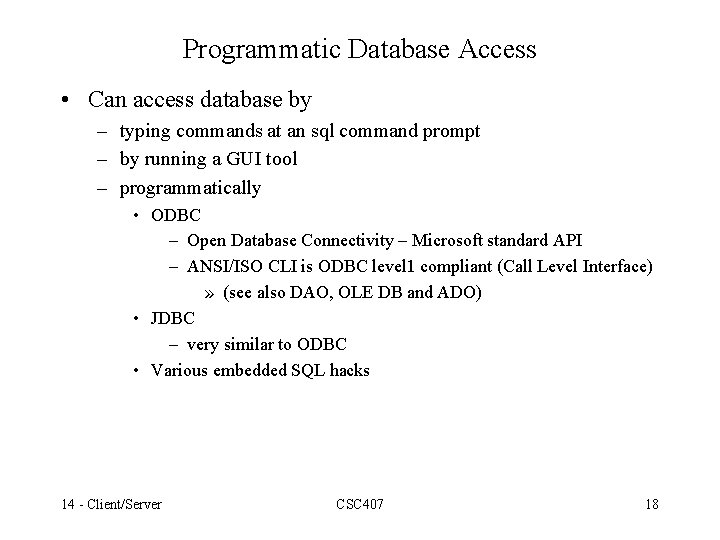
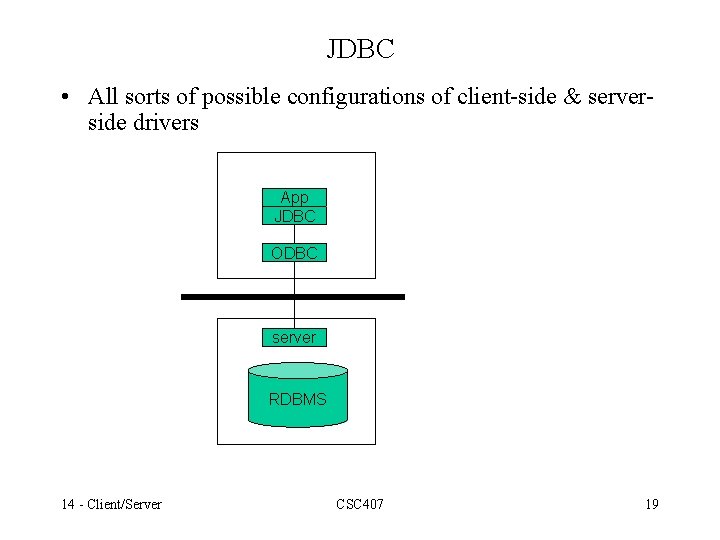
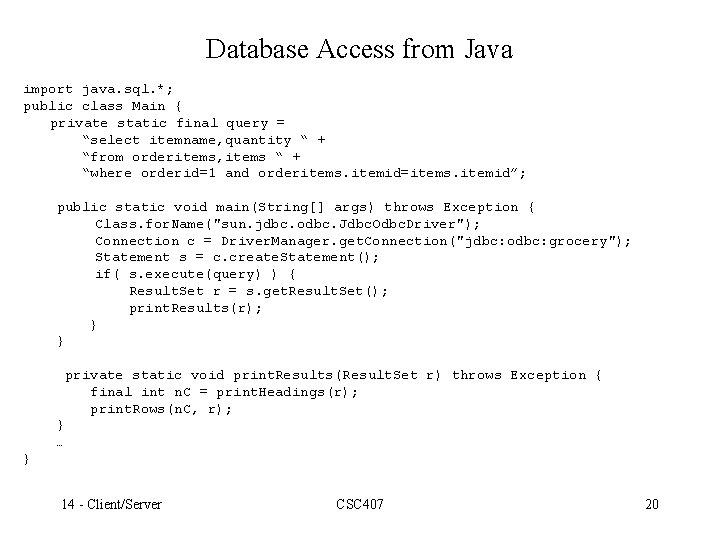
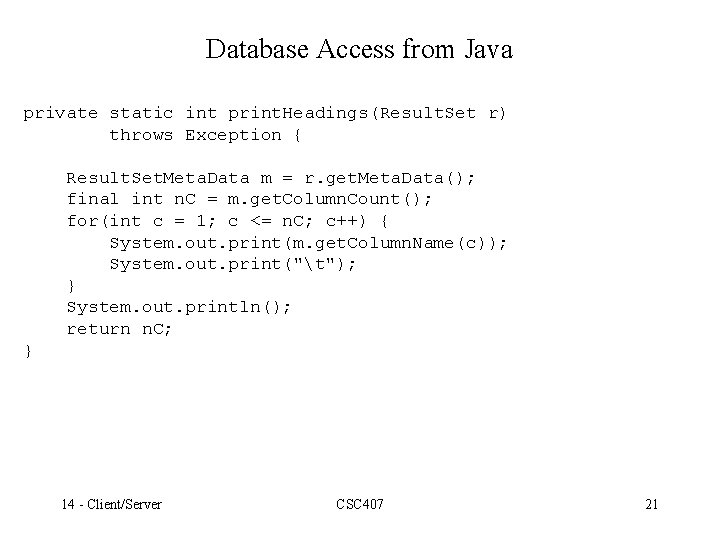
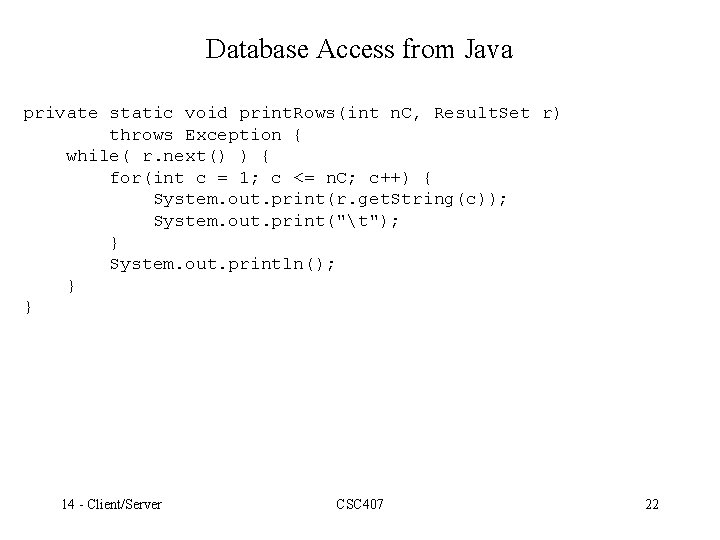
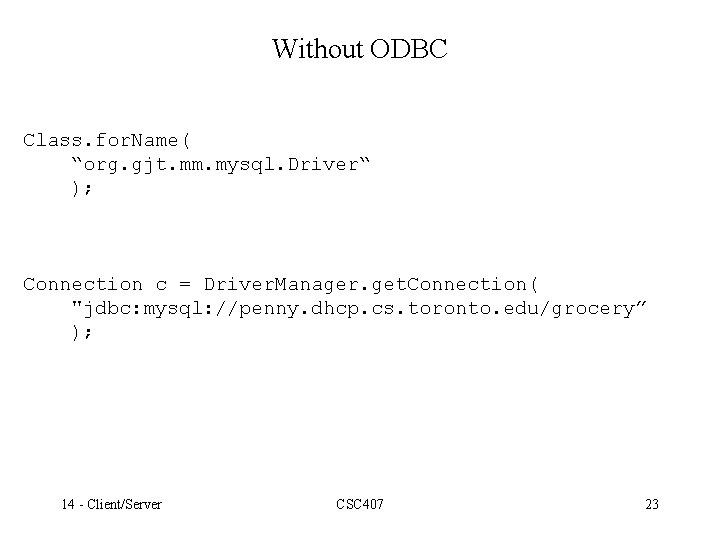
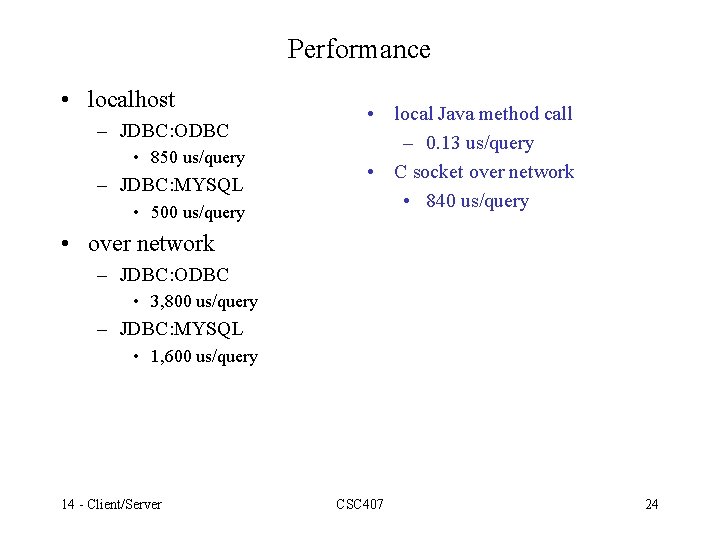
- Slides: 24
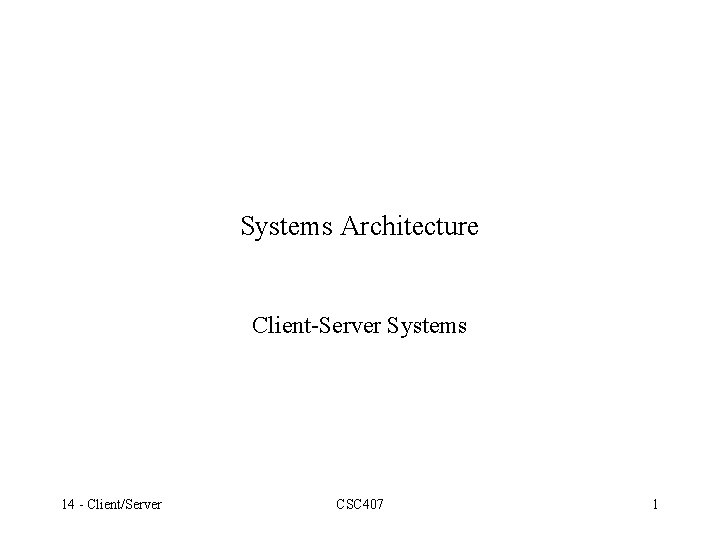
Systems Architecture Client-Server Systems 14 - Client/Server CSC 407 1
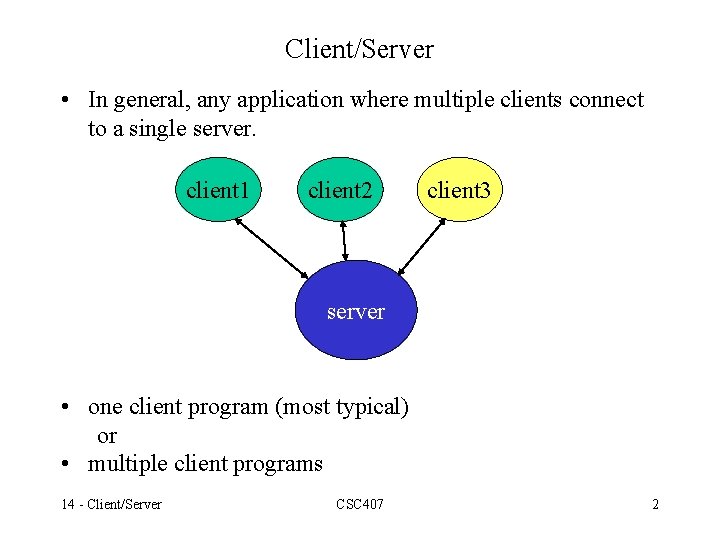
Client/Server • In general, any application where multiple clients connect to a single server. client 1 client 2 client 3 server • one client program (most typical) or • multiple client programs 14 - Client/Server CSC 407 2
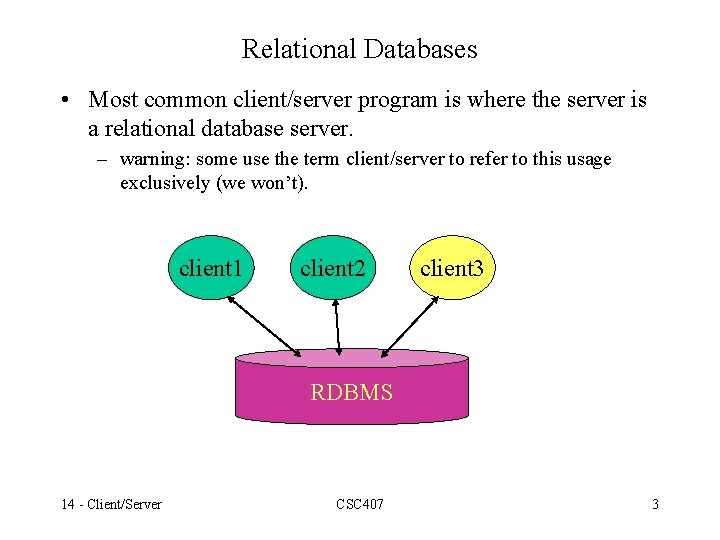
Relational Databases • Most common client/server program is where the server is a relational database server. – warning: some use the term client/server to refer to this usage exclusively (we won’t). client 1 client 2 client 3 RDBMS 14 - Client/Server CSC 407 3
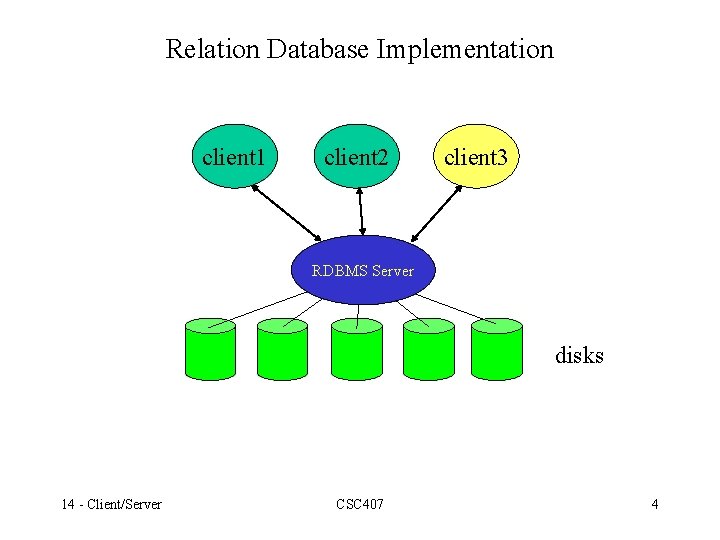
Relation Database Implementation client 1 client 2 client 3 RDBMS Server disks 14 - Client/Server CSC 407 4
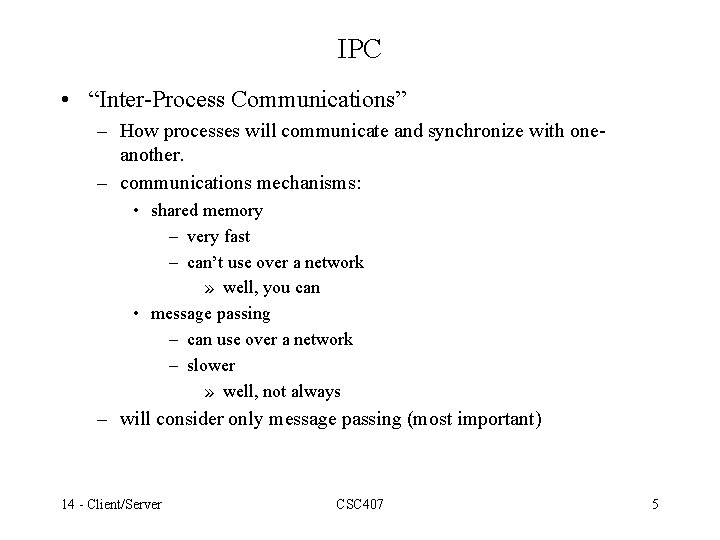
IPC • “Inter-Process Communications” – How processes will communicate and synchronize with oneanother. – communications mechanisms: • shared memory – very fast – can’t use over a network » well, you can • message passing – can use over a network – slower » well, not always – will consider only message passing (most important) 14 - Client/Server CSC 407 5
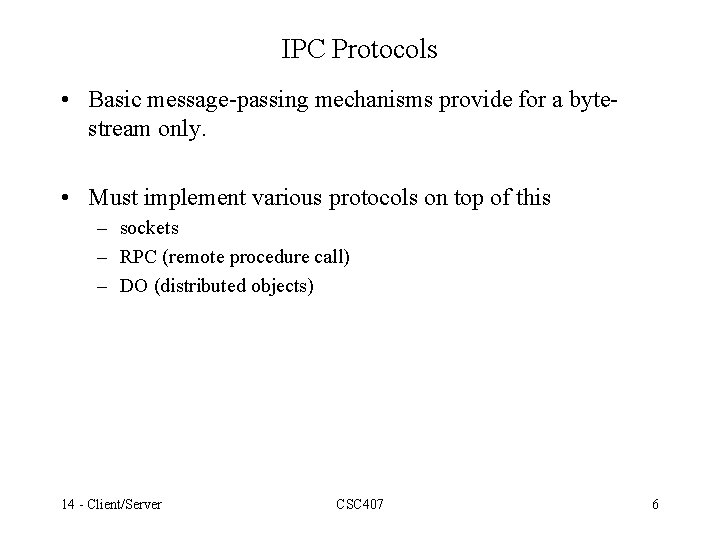
IPC Protocols • Basic message-passing mechanisms provide for a bytestream only. • Must implement various protocols on top of this – sockets – RPC (remote procedure call) – DO (distributed objects) 14 - Client/Server CSC 407 6
![Sockets code example public class Server public static void mainString args throws Exception Sockets code example public class Server { public static void main(String[] args) throws Exception](https://slidetodoc.com/presentation_image/f1fb74f5874f1b32885e5a8b3cef486f/image-7.jpg)
Sockets code example public class Server { public static void main(String[] args) throws Exception { Server. Socket server = new Server. Socket(1234); Socket client = server. accept(); Buffered. Reader from. Client = new Buffered. Reader( new Input. Stream. Reader(client. get. Input. Stream())); System. out. println(from. Client. read. Line()); } } public class Client { public static void main(String[] args) throws Exception { Socket server = new Socket(“penny", 1234); Data. Output. Stream to. Server = new Data. Output. Stream( server. get. Output. Stream()); to. Server. write. Bytes("hello server"); server. close(); } } 14 - Client/Server CSC 407 7
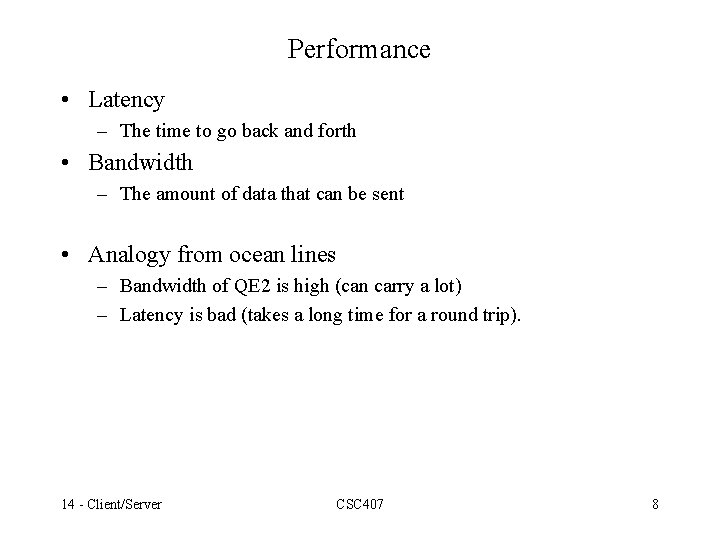
Performance • Latency – The time to go back and forth • Bandwidth – The amount of data that can be sent • Analogy from ocean lines – Bandwidth of QE 2 is high (can carry a lot) – Latency is bad (takes a long time for a round trip). 14 - Client/Server CSC 407 8
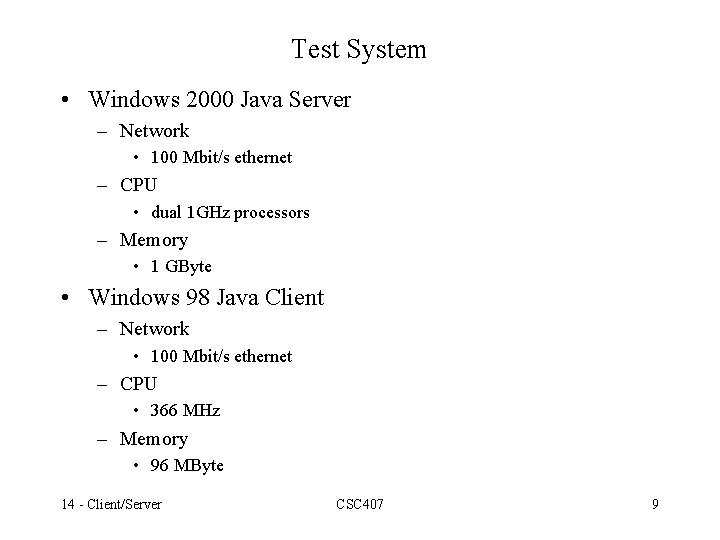
Test System • Windows 2000 Java Server – Network • 100 Mbit/s ethernet – CPU • dual 1 GHz processors – Memory • 1 GByte • Windows 98 Java Client – Network • 100 Mbit/s ethernet – CPU • 366 MHz – Memory • 96 MByte 14 - Client/Server CSC 407 9
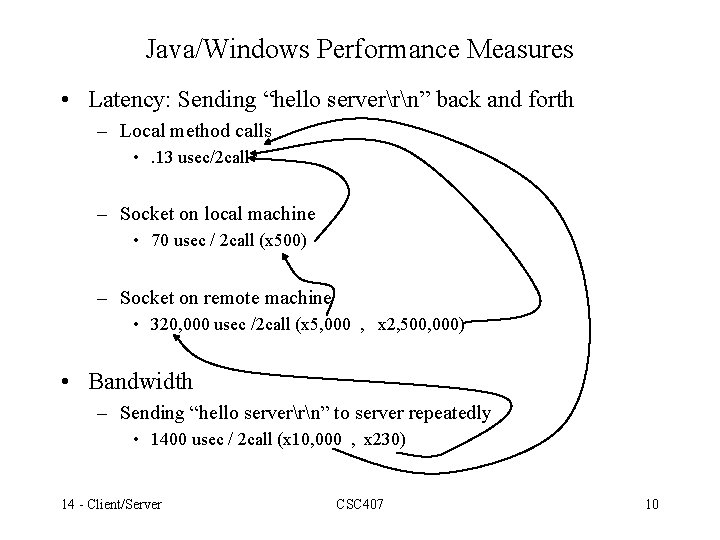
Java/Windows Performance Measures • Latency: Sending “hello serverrn” back and forth – Local method calls • . 13 usec/2 call – Socket on local machine • 70 usec / 2 call (x 500) – Socket on remote machine • 320, 000 usec /2 call (x 5, 000 , x 2, 500, 000) • Bandwidth – Sending “hello serverrn” to server repeatedly • 1400 usec / 2 call (x 10, 000 , x 230) 14 - Client/Server CSC 407 10
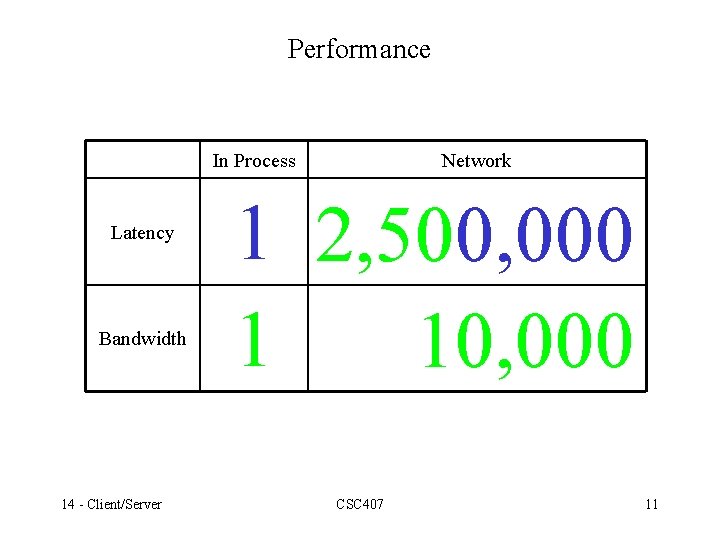
Performance In Process Latency Bandwidth 14 - Client/Server Network 1 2, 500, 000 1 10, 000 CSC 407 11
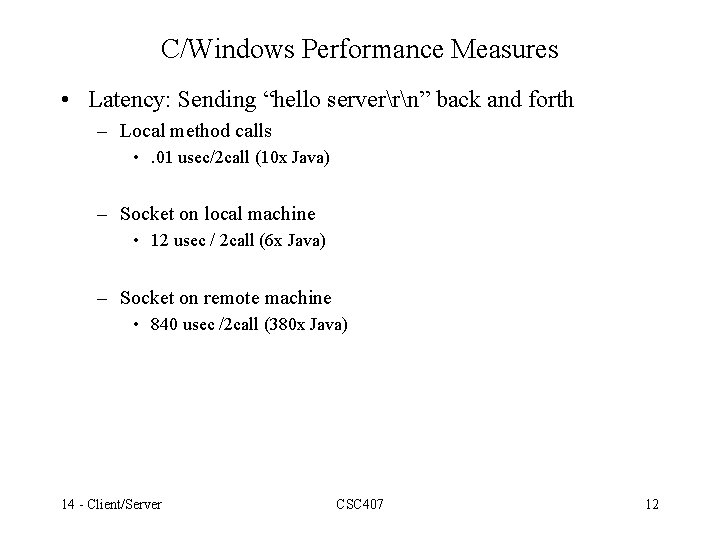
C/Windows Performance Measures • Latency: Sending “hello serverrn” back and forth – Local method calls • . 01 usec/2 call (10 x Java) – Socket on local machine • 12 usec / 2 call (6 x Java) – Socket on remote machine • 840 usec /2 call (380 x Java) 14 - Client/Server CSC 407 12
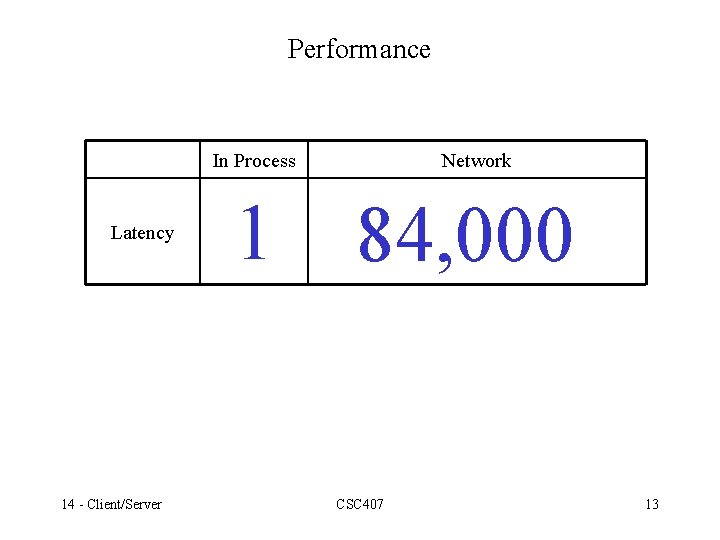
Performance In Process Latency 14 - Client/Server 1 Network 84, 000 CSC 407 13
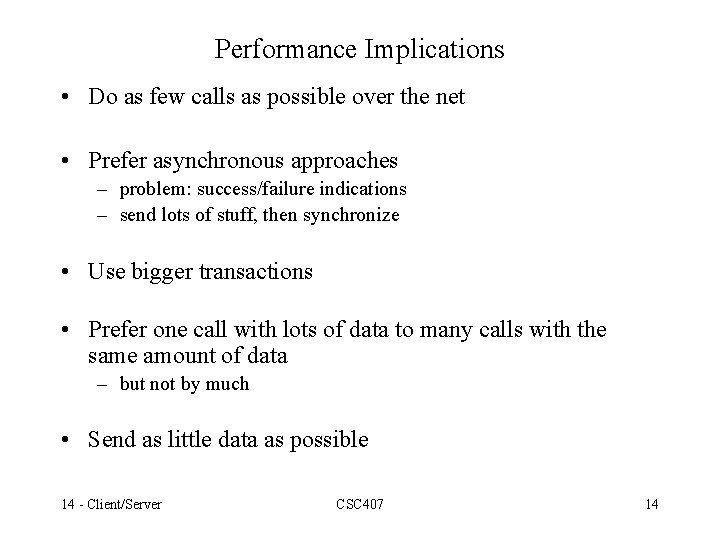
Performance Implications • Do as few calls as possible over the net • Prefer asynchronous approaches – problem: success/failure indications – send lots of stuff, then synchronize • Use bigger transactions • Prefer one call with lots of data to many calls with the same amount of data – but not by much • Send as little data as possible 14 - Client/Server CSC 407 14
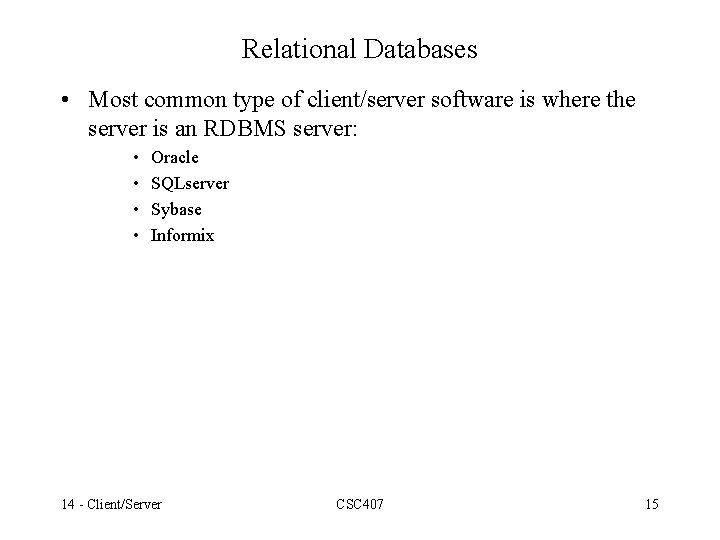
Relational Databases • Most common type of client/server software is where the server is an RDBMS server: • • Oracle SQLserver Sybase Informix 14 - Client/Server CSC 407 15
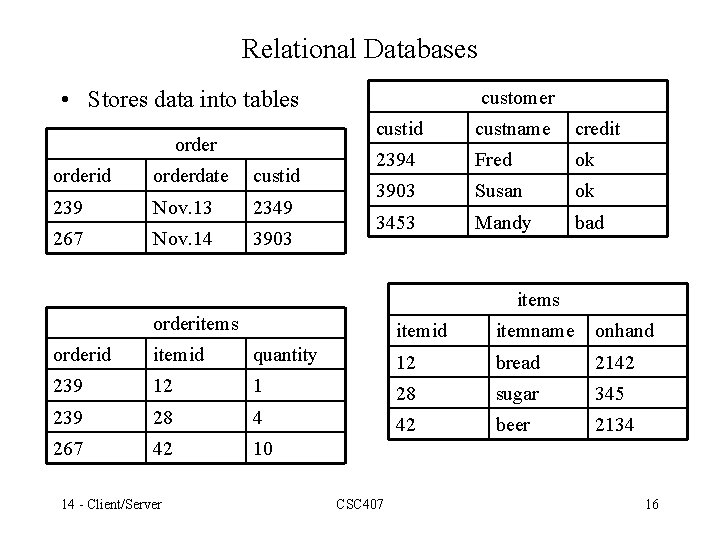
Relational Databases • Stores data into tables orderid orderdate custid 239 Nov. 13 2349 267 Nov. 14 3903 customer custid custname credit 2394 Fred ok 3903 Susan ok 3453 Mandy bad items orderitems itemid itemname onhand orderid itemid quantity 12 bread 2142 239 12 1 28 sugar 345 239 28 4 42 beer 2134 267 42 10 14 - Client/Server CSC 407 16
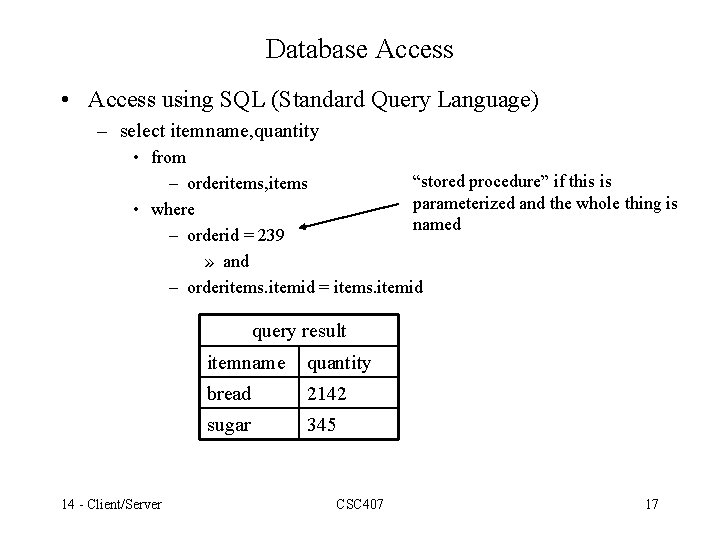
Database Access • Access using SQL (Standard Query Language) – select itemname, quantity • from “stored procedure” if this is – orderitems, items parameterized and the whole thing is • where named – orderid = 239 » and – orderitems. itemid = items. itemid query result 14 - Client/Server itemname quantity bread 2142 sugar 345 CSC 407 17
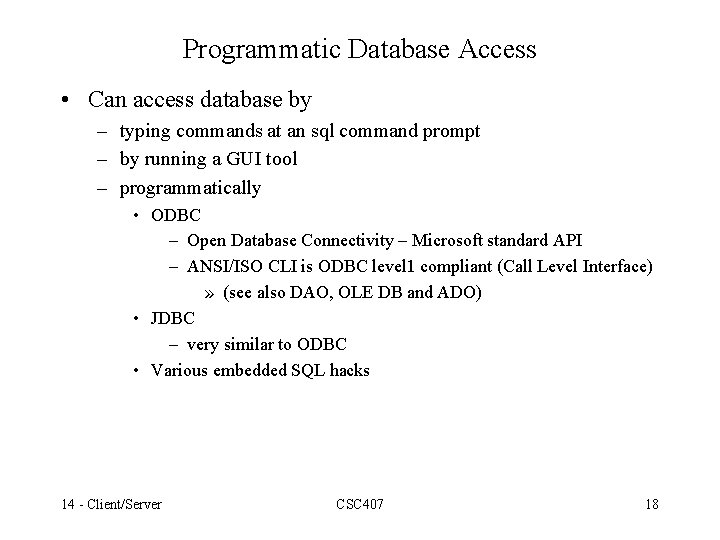
Programmatic Database Access • Can access database by – typing commands at an sql command prompt – by running a GUI tool – programmatically • ODBC – Open Database Connectivity – Microsoft standard API – ANSI/ISO CLI is ODBC level 1 compliant (Call Level Interface) » (see also DAO, OLE DB and ADO) • JDBC – very similar to ODBC • Various embedded SQL hacks 14 - Client/Server CSC 407 18
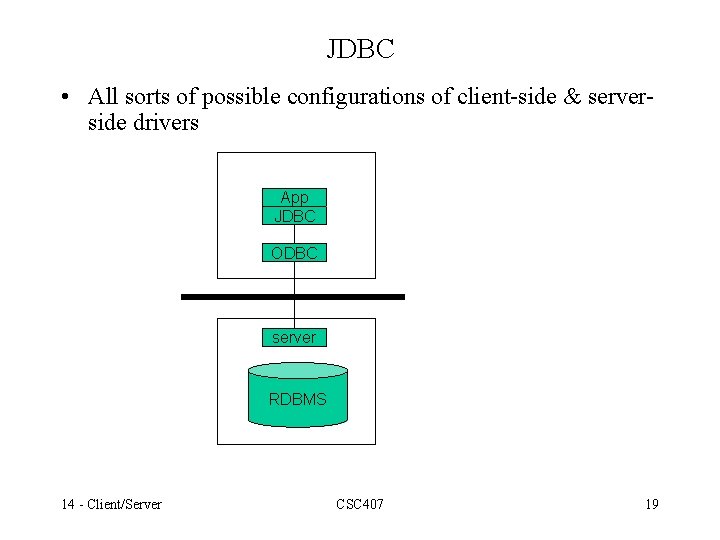
JDBC • All sorts of possible configurations of client-side & serverside drivers App JDBC ODBC server RDBMS 14 - Client/Server CSC 407 19
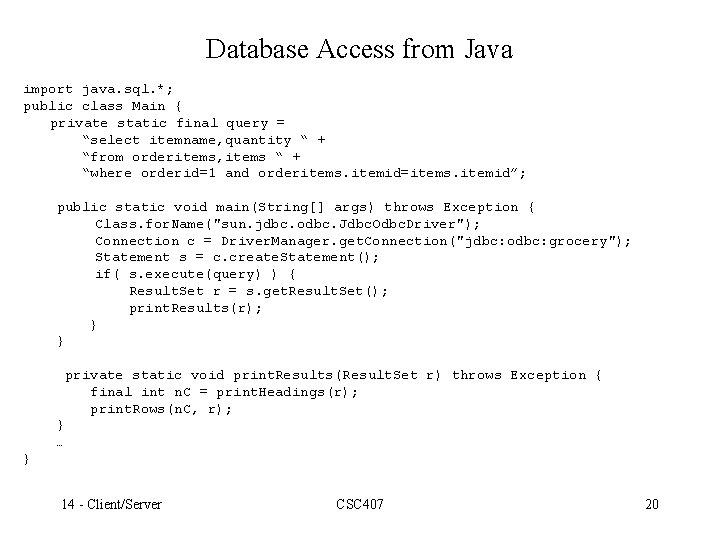
Database Access from Java import java. sql. *; public class Main { private static final query = “select itemname, quantity “ + “from orderitems, items “ + “where orderid=1 and orderitems. itemid=items. itemid”; public static void main(String[] args) throws Exception { Class. for. Name("sun. jdbc. odbc. Jdbc. Odbc. Driver"); Connection c = Driver. Manager. get. Connection("jdbc: odbc: grocery"); Statement s = c. create. Statement(); if( s. execute(query) ) { Result. Set r = s. get. Result. Set(); print. Results(r); } } private static void print. Results(Result. Set r) throws Exception { final int n. C = print. Headings(r); print. Rows(n. C, r); } … } 14 - Client/Server CSC 407 20
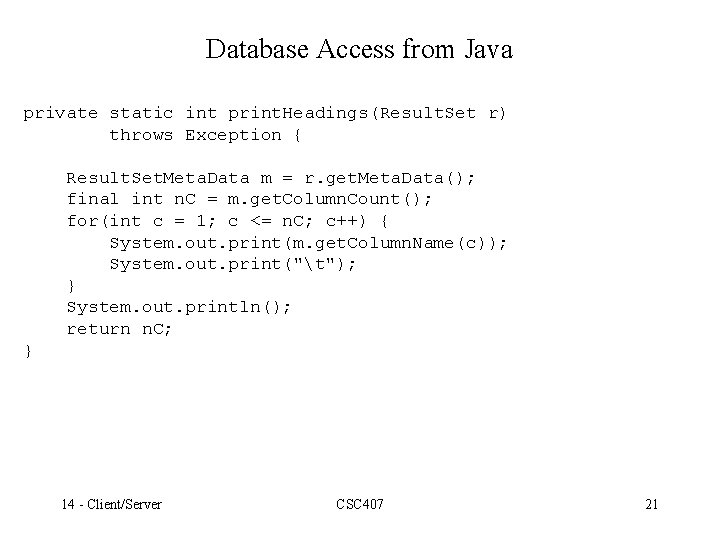
Database Access from Java private static int print. Headings(Result. Set r) throws Exception { Result. Set. Meta. Data m = r. get. Meta. Data(); final int n. C = m. get. Column. Count(); for(int c = 1; c <= n. C; c++) { System. out. print(m. get. Column. Name(c)); System. out. print("t"); } System. out. println(); return n. C; } 14 - Client/Server CSC 407 21
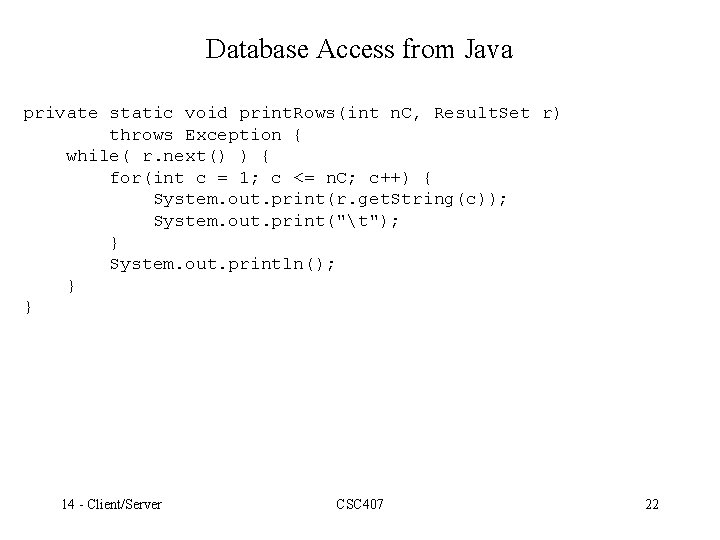
Database Access from Java private static void print. Rows(int n. C, Result. Set r) throws Exception { while( r. next() ) { for(int c = 1; c <= n. C; c++) { System. out. print(r. get. String(c)); System. out. print("t"); } System. out. println(); } } 14 - Client/Server CSC 407 22
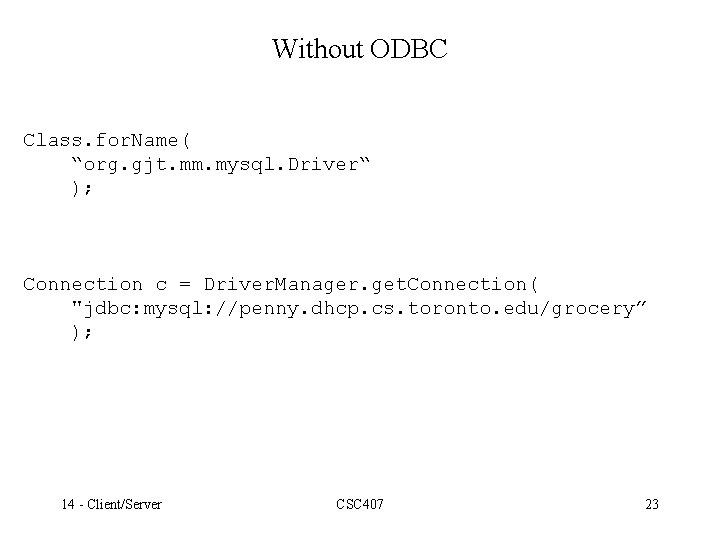
Without ODBC Class. for. Name( “org. gjt. mm. mysql. Driver“ ); Connection c = Driver. Manager. get. Connection( "jdbc: mysql: //penny. dhcp. cs. toronto. edu/grocery” ); 14 - Client/Server CSC 407 23
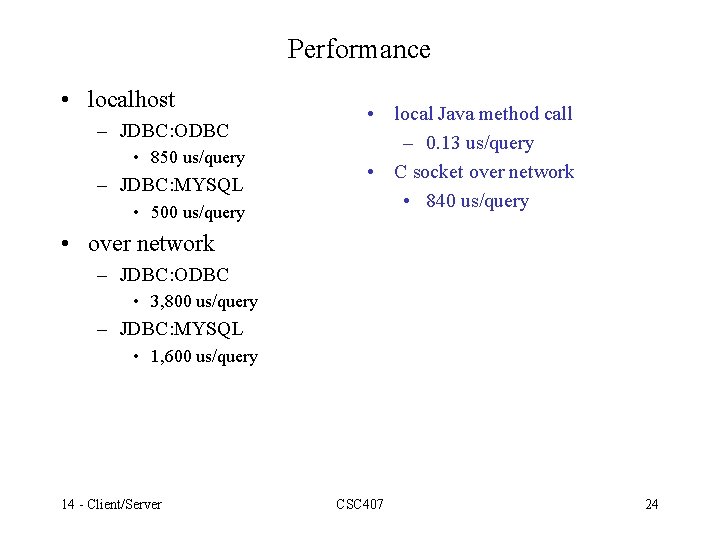
Performance • localhost – JDBC: ODBC • 850 us/query – JDBC: MYSQL • 500 us/query • local Java method call – 0. 13 us/query • C socket over network • 840 us/query • over network – JDBC: ODBC • 3, 800 us/query – JDBC: MYSQL • 1, 600 us/query 14 - Client/Server CSC 407 24