XNA Tutorial 1 For CS 134 Lecture Overview
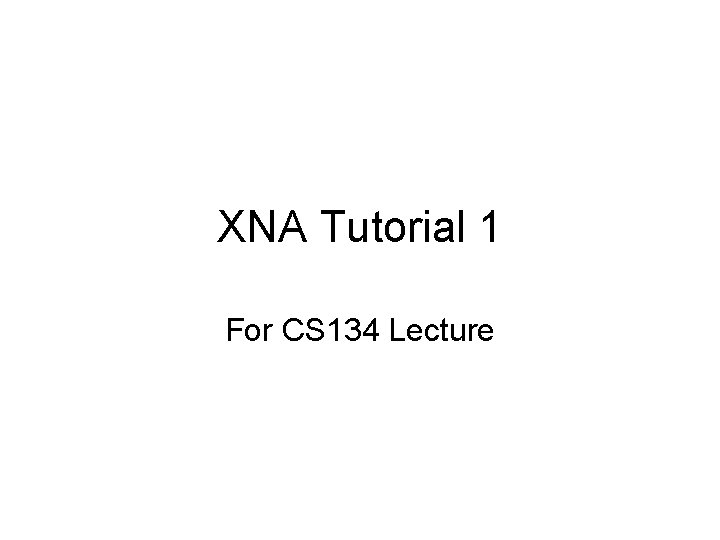
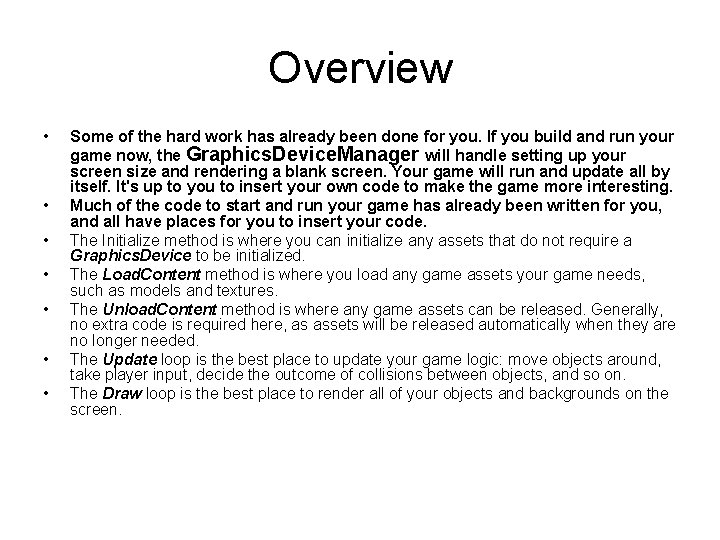
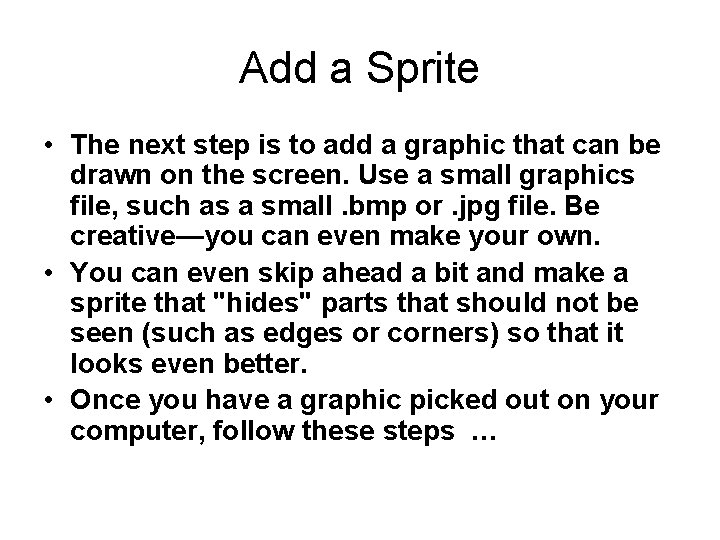
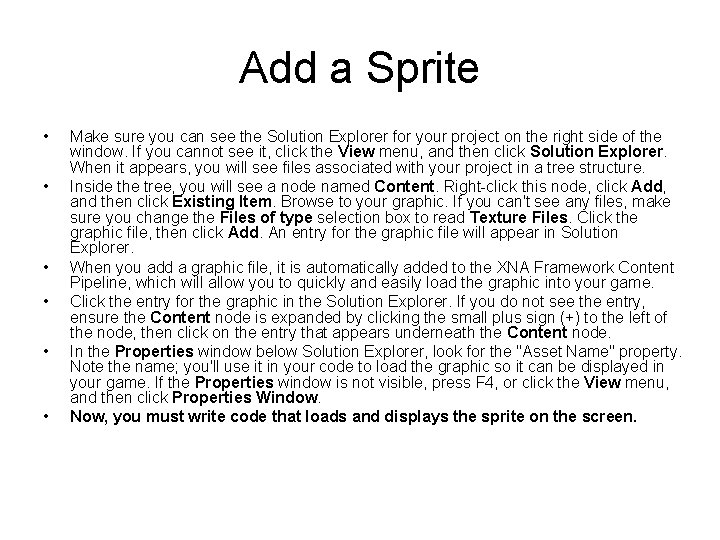
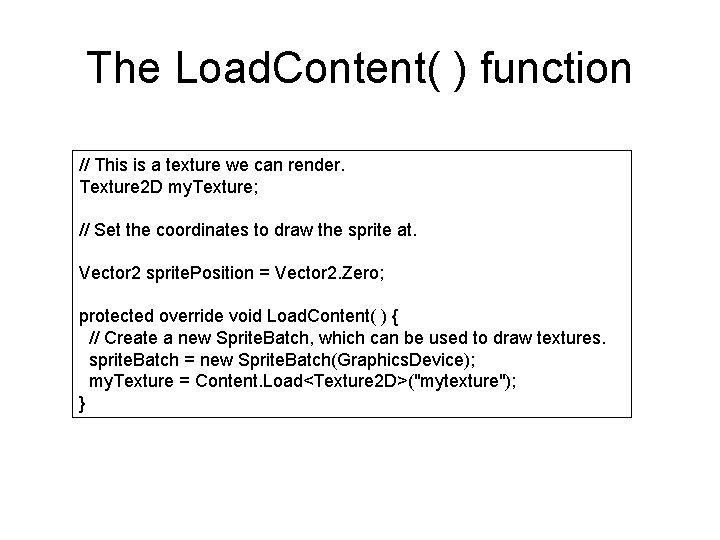
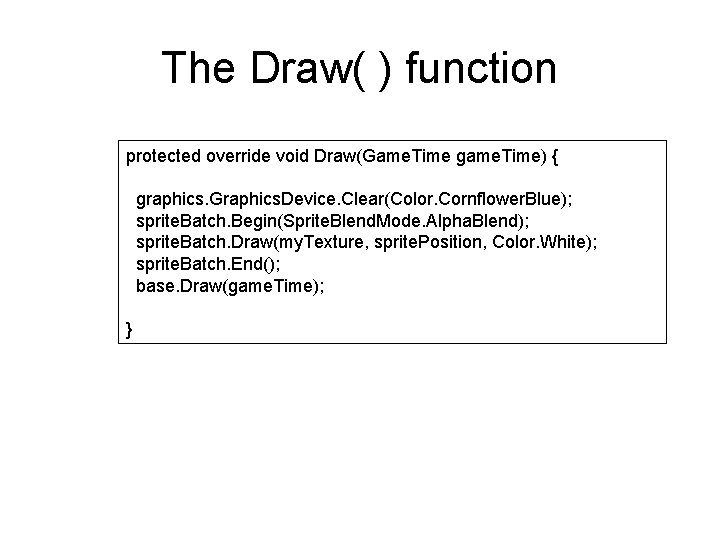
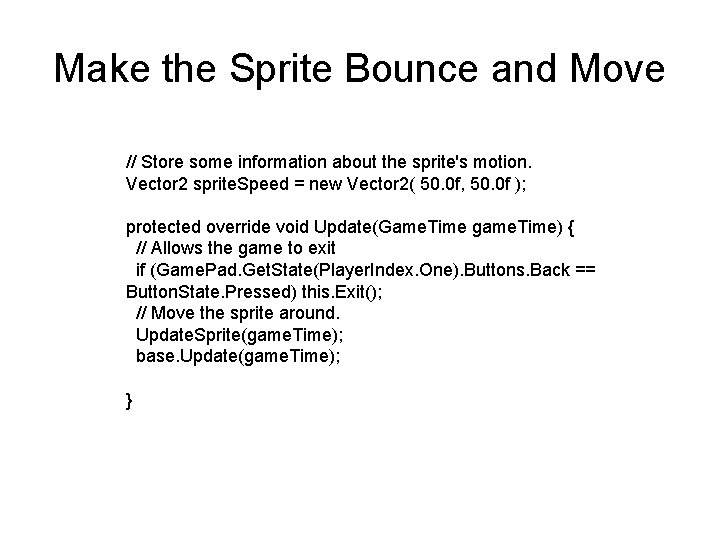
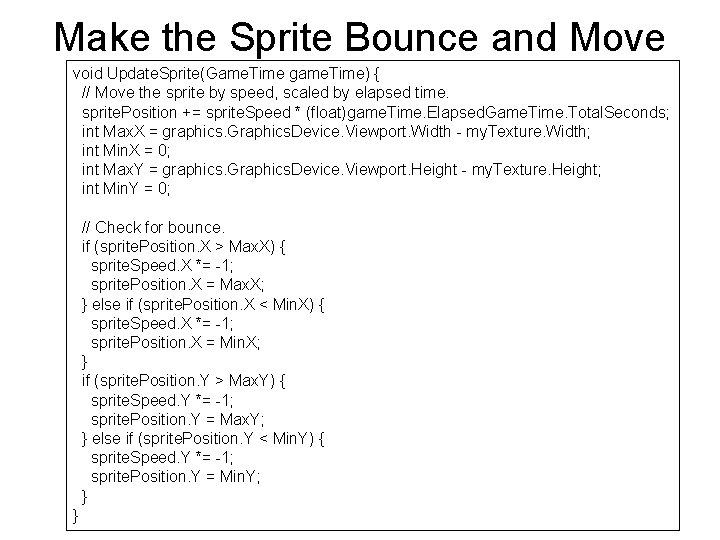
- Slides: 8
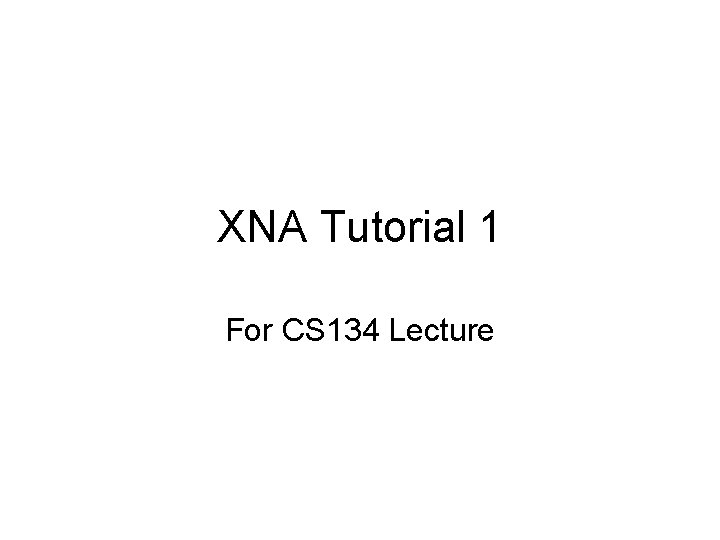
XNA Tutorial 1 For CS 134 Lecture
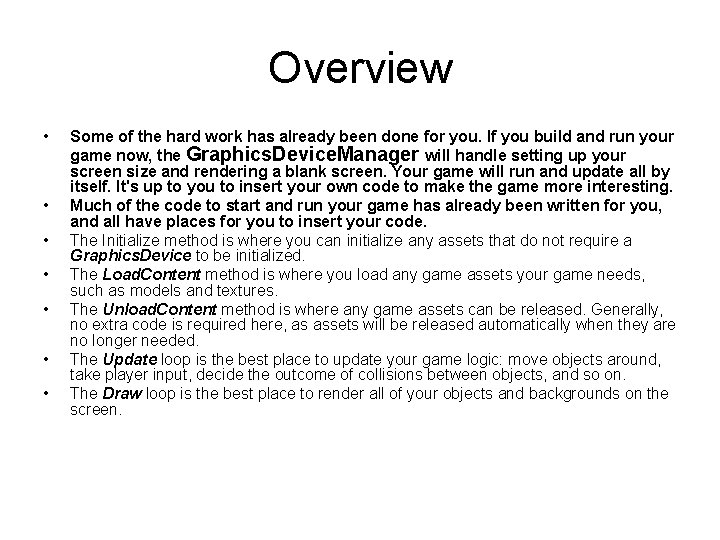
Overview • • Some of the hard work has already been done for you. If you build and run your game now, the Graphics. Device. Manager will handle setting up your screen size and rendering a blank screen. Your game will run and update all by itself. It's up to you to insert your own code to make the game more interesting. Much of the code to start and run your game has already been written for you, and all have places for you to insert your code. The Initialize method is where you can initialize any assets that do not require a Graphics. Device to be initialized. The Load. Content method is where you load any game assets your game needs, such as models and textures. The Unload. Content method is where any game assets can be released. Generally, no extra code is required here, as assets will be released automatically when they are no longer needed. The Update loop is the best place to update your game logic: move objects around, take player input, decide the outcome of collisions between objects, and so on. The Draw loop is the best place to render all of your objects and backgrounds on the screen.
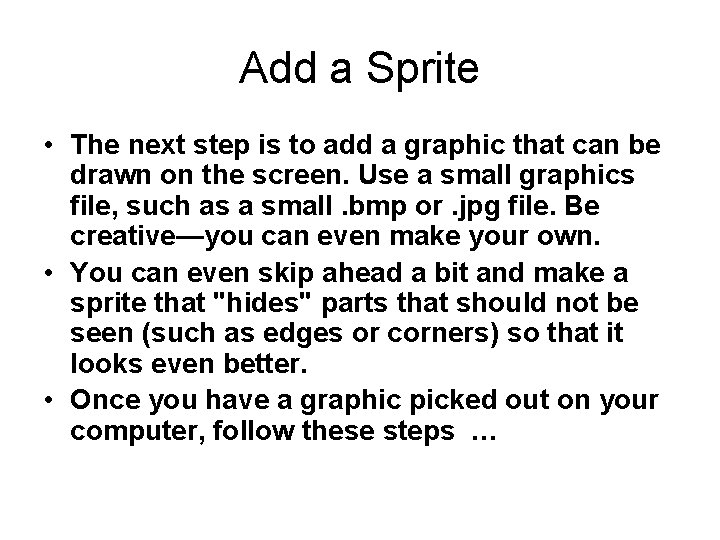
Add a Sprite • The next step is to add a graphic that can be drawn on the screen. Use a small graphics file, such as a small. bmp or. jpg file. Be creative—you can even make your own. • You can even skip ahead a bit and make a sprite that "hides" parts that should not be seen (such as edges or corners) so that it looks even better. • Once you have a graphic picked out on your computer, follow these steps …
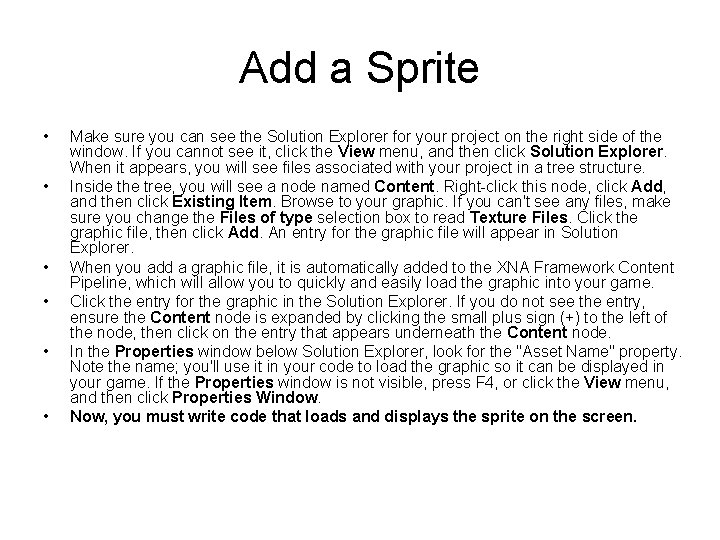
Add a Sprite • • • Make sure you can see the Solution Explorer for your project on the right side of the window. If you cannot see it, click the View menu, and then click Solution Explorer. When it appears, you will see files associated with your project in a tree structure. Inside the tree, you will see a node named Content. Right-click this node, click Add, and then click Existing Item. Browse to your graphic. If you can't see any files, make sure you change the Files of type selection box to read Texture Files. Click the graphic file, then click Add. An entry for the graphic file will appear in Solution Explorer. When you add a graphic file, it is automatically added to the XNA Framework Content Pipeline, which will allow you to quickly and easily load the graphic into your game. Click the entry for the graphic in the Solution Explorer. If you do not see the entry, ensure the Content node is expanded by clicking the small plus sign (+) to the left of the node, then click on the entry that appears underneath the Content node. In the Properties window below Solution Explorer, look for the "Asset Name" property. Note the name; you'll use it in your code to load the graphic so it can be displayed in your game. If the Properties window is not visible, press F 4, or click the View menu, and then click Properties Window. Now, you must write code that loads and displays the sprite on the screen.
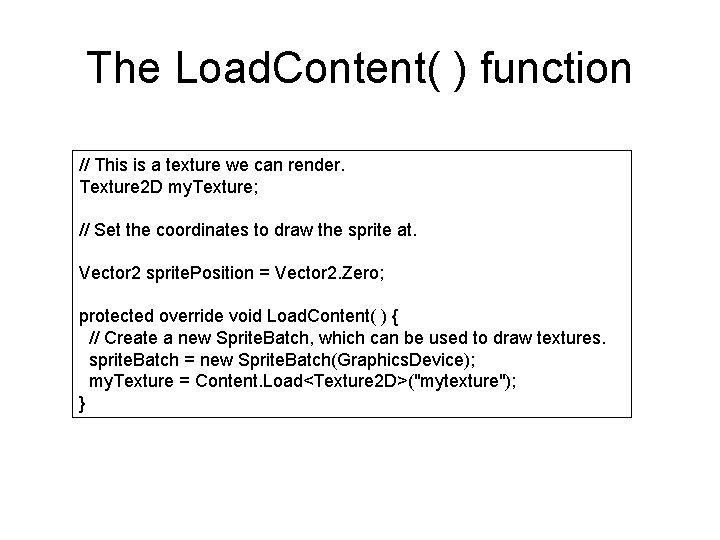
The Load. Content( ) function // This is a texture we can render. Texture 2 D my. Texture; // Set the coordinates to draw the sprite at. Vector 2 sprite. Position = Vector 2. Zero; protected override void Load. Content( ) { // Create a new Sprite. Batch, which can be used to draw textures. sprite. Batch = new Sprite. Batch(Graphics. Device); my. Texture = Content. Load<Texture 2 D>("mytexture"); }
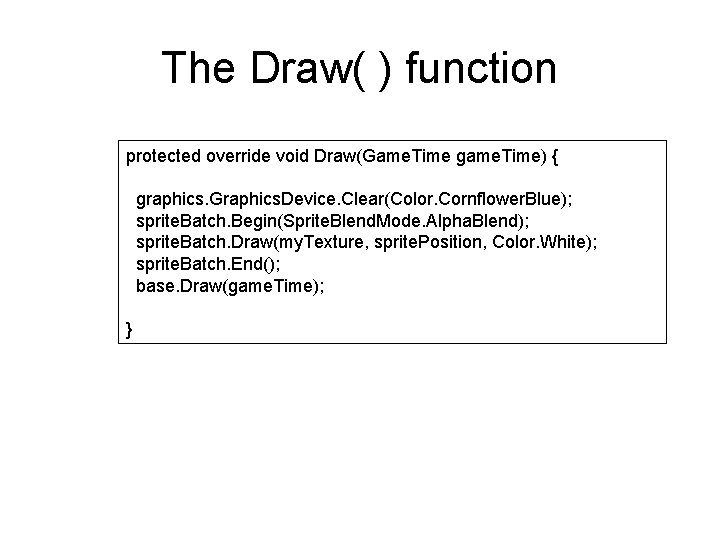
The Draw( ) function protected override void Draw(Game. Time game. Time) { graphics. Graphics. Device. Clear(Color. Cornflower. Blue); sprite. Batch. Begin(Sprite. Blend. Mode. Alpha. Blend); sprite. Batch. Draw(my. Texture, sprite. Position, Color. White); sprite. Batch. End(); base. Draw(game. Time); }
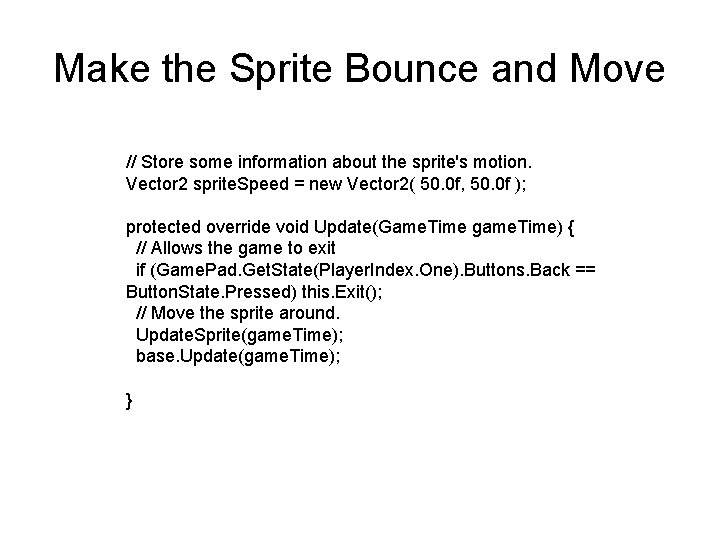
Make the Sprite Bounce and Move // Store some information about the sprite's motion. Vector 2 sprite. Speed = new Vector 2( 50. 0 f, 50. 0 f ); protected override void Update(Game. Time game. Time) { // Allows the game to exit if (Game. Pad. Get. State(Player. Index. One). Buttons. Back == Button. State. Pressed) this. Exit(); // Move the sprite around. Update. Sprite(game. Time); base. Update(game. Time); }
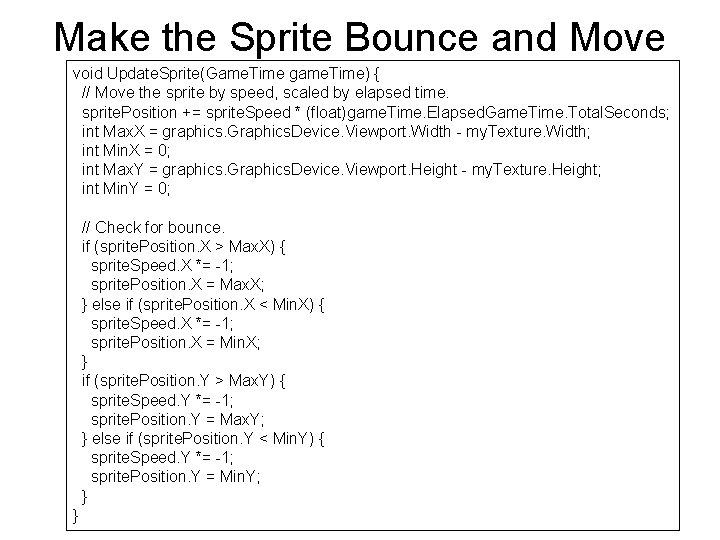
Make the Sprite Bounce and Move void Update. Sprite(Game. Time game. Time) { // Move the sprite by speed, scaled by elapsed time. sprite. Position += sprite. Speed * (float)game. Time. Elapsed. Game. Time. Total. Seconds; int Max. X = graphics. Graphics. Device. Viewport. Width - my. Texture. Width; int Min. X = 0; int Max. Y = graphics. Graphics. Device. Viewport. Height - my. Texture. Height; int Min. Y = 0; // Check for bounce. if (sprite. Position. X > Max. X) { sprite. Speed. X *= -1; sprite. Position. X = Max. X; } else if (sprite. Position. X < Min. X) { sprite. Speed. X *= -1; sprite. Position. X = Min. X; } if (sprite. Position. Y > Max. Y) { sprite. Speed. Y *= -1; sprite. Position. Y = Max. Y; } else if (sprite. Position. Y < Min. Y) { sprite. Speed. Y *= -1; sprite. Position. Y = Min. Y; } }