Linked Lists in Action This chapter introduces linked
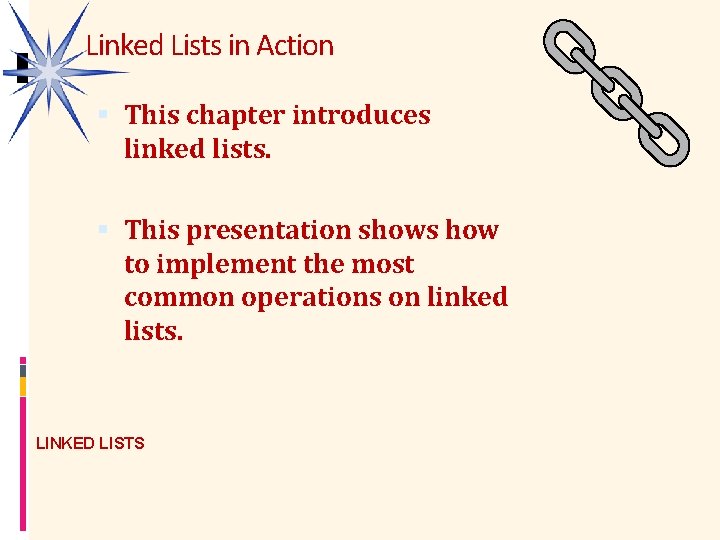
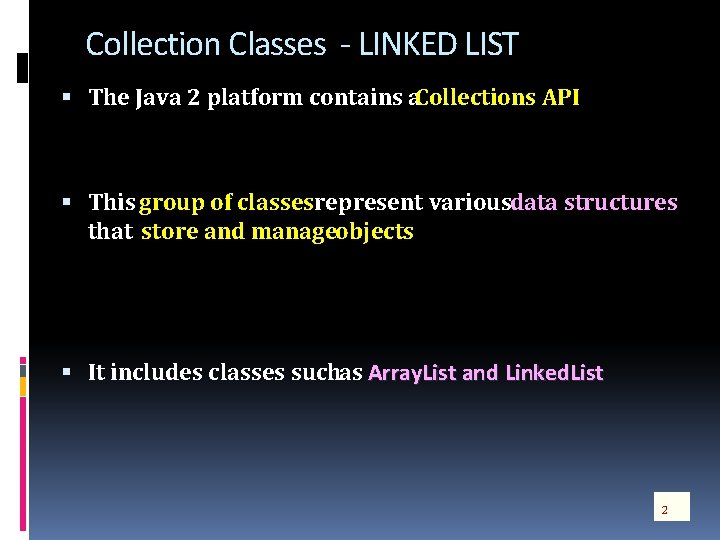
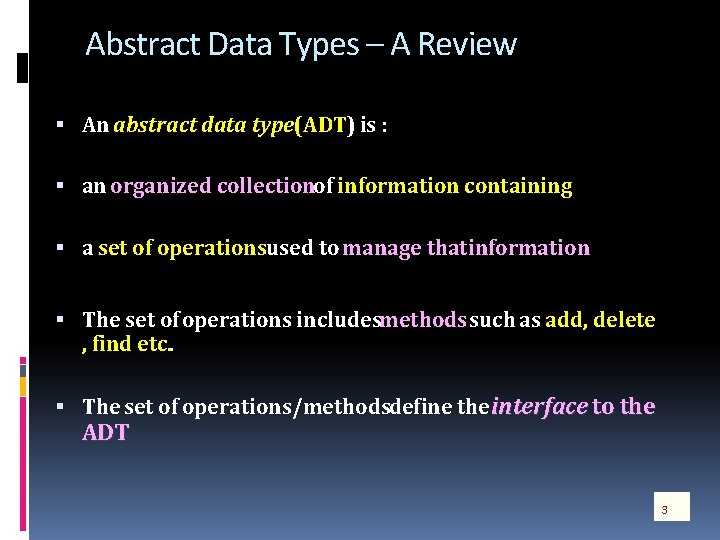
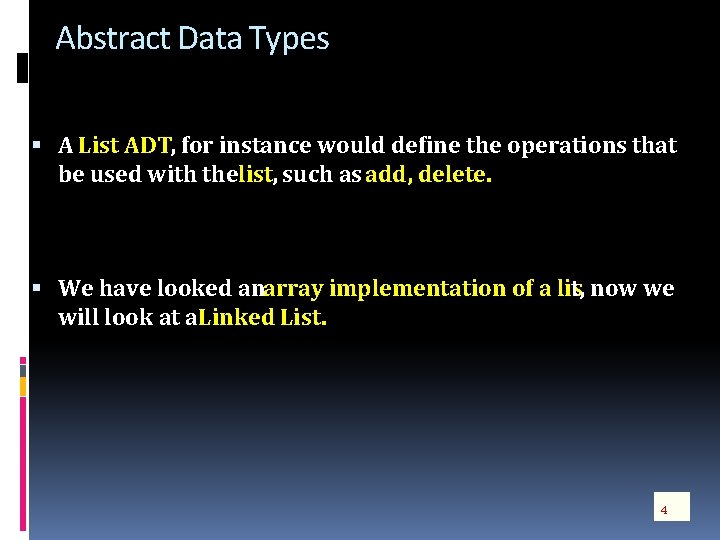
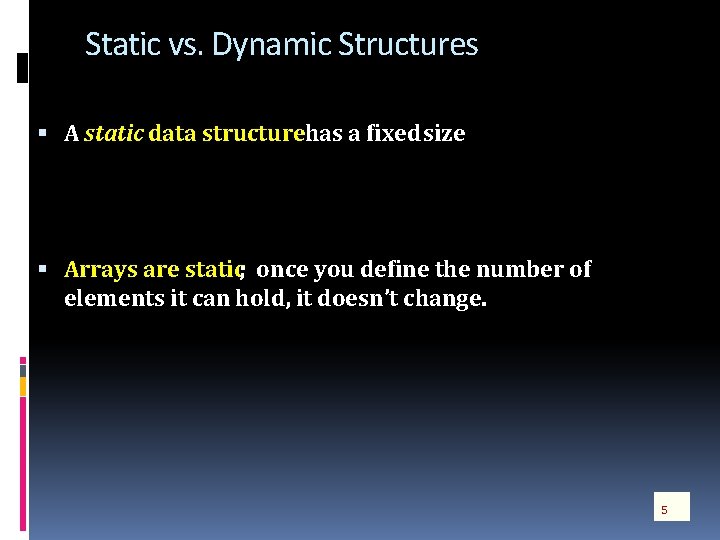
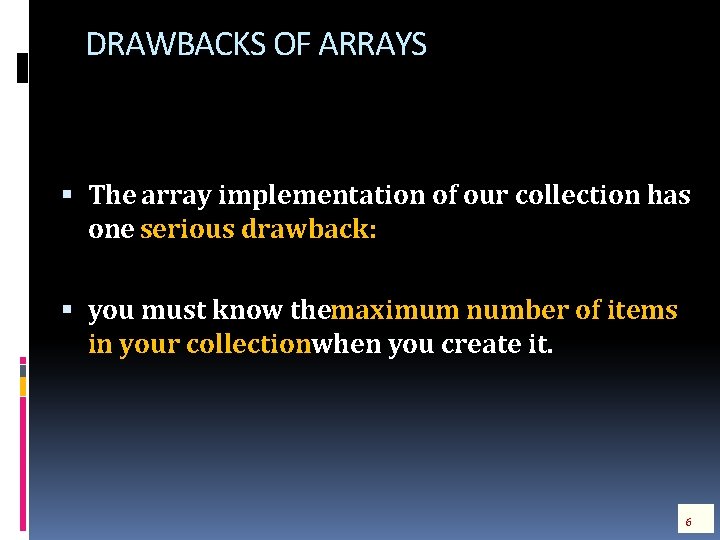
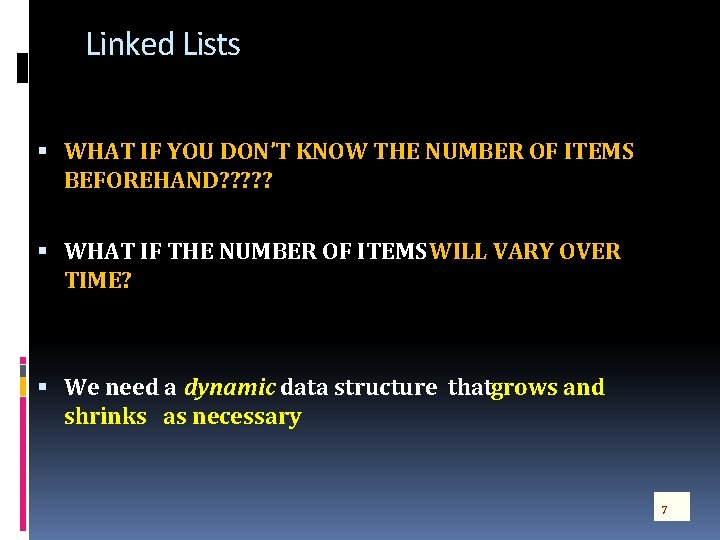
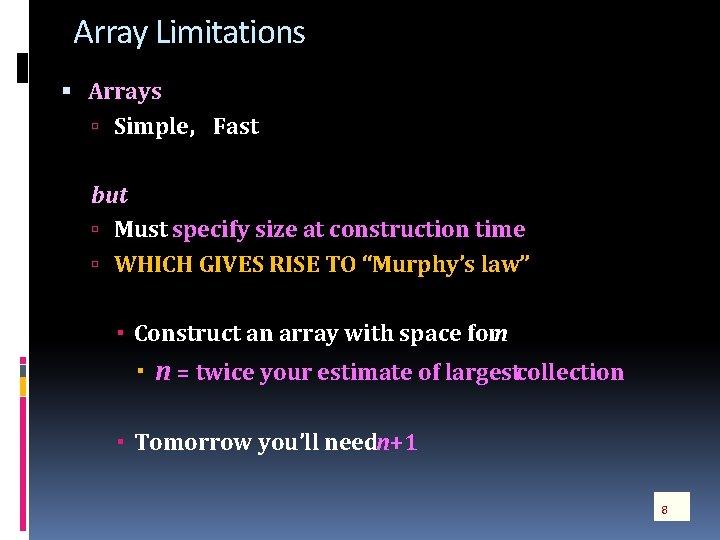
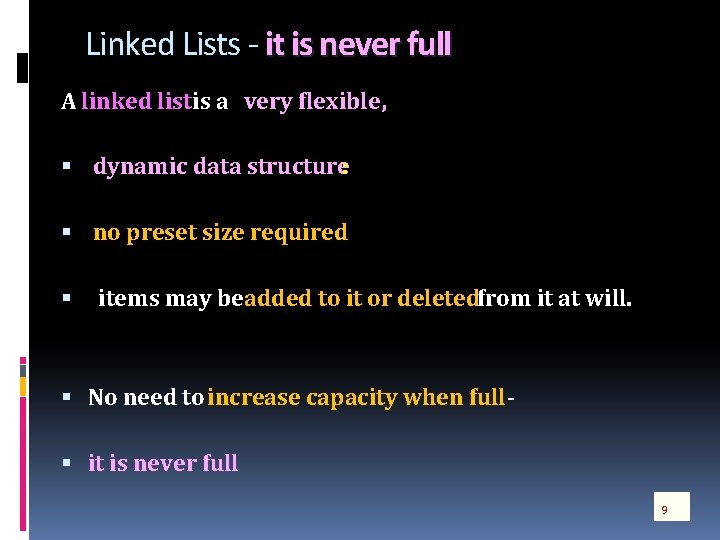
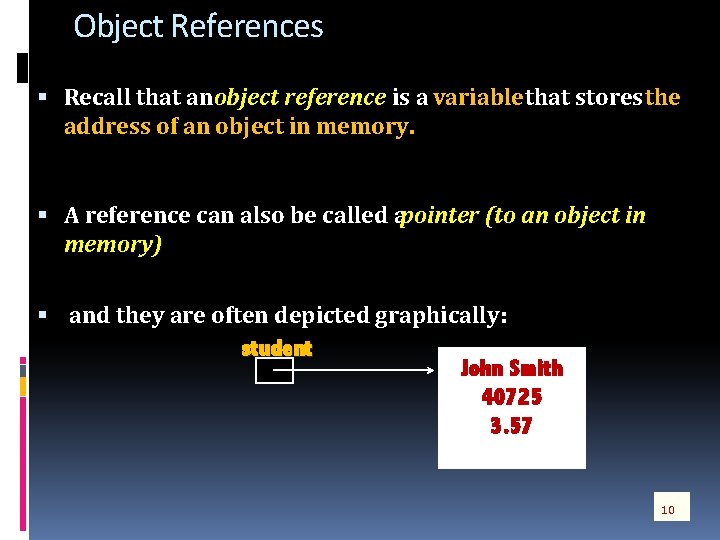
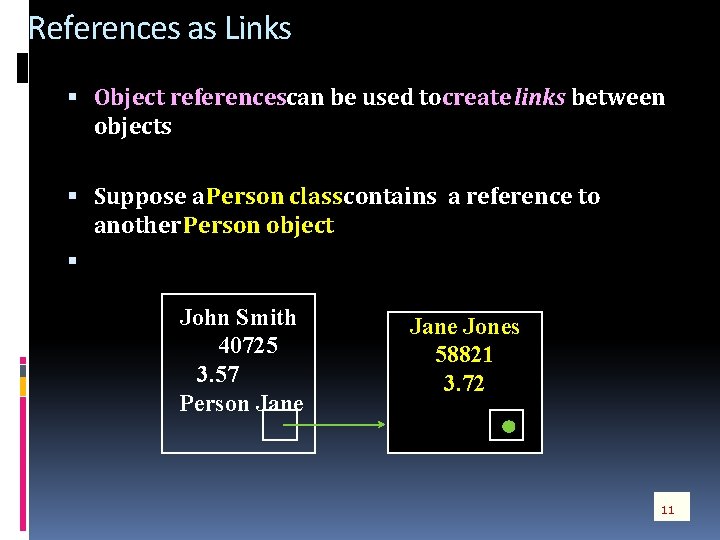
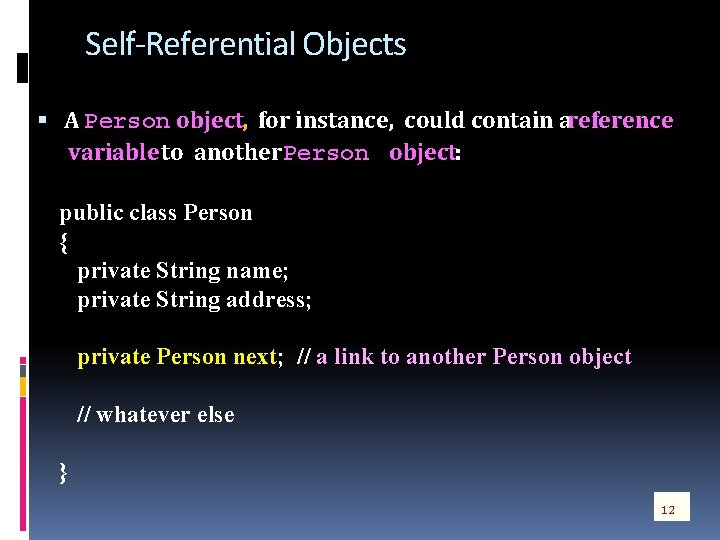
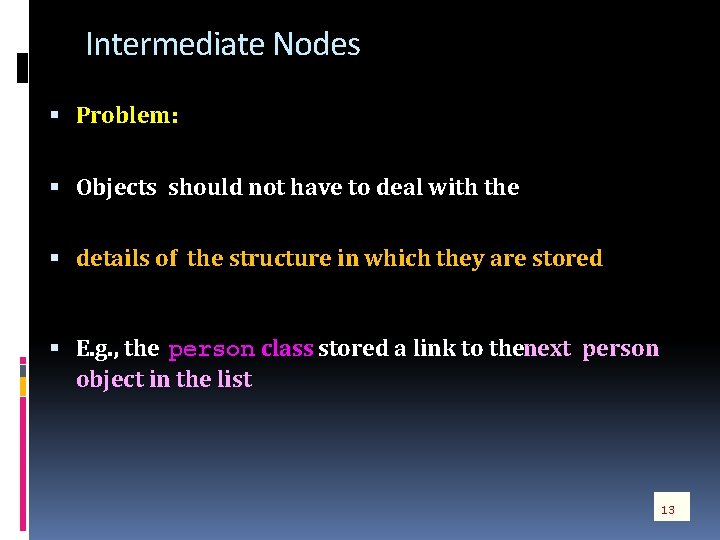
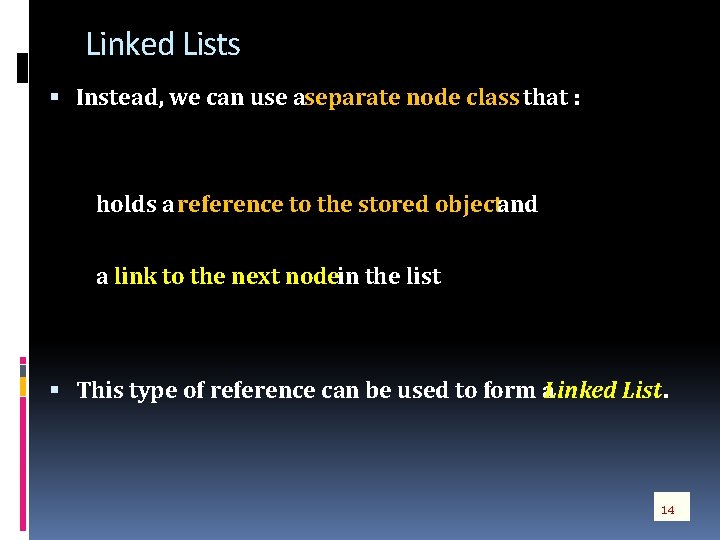
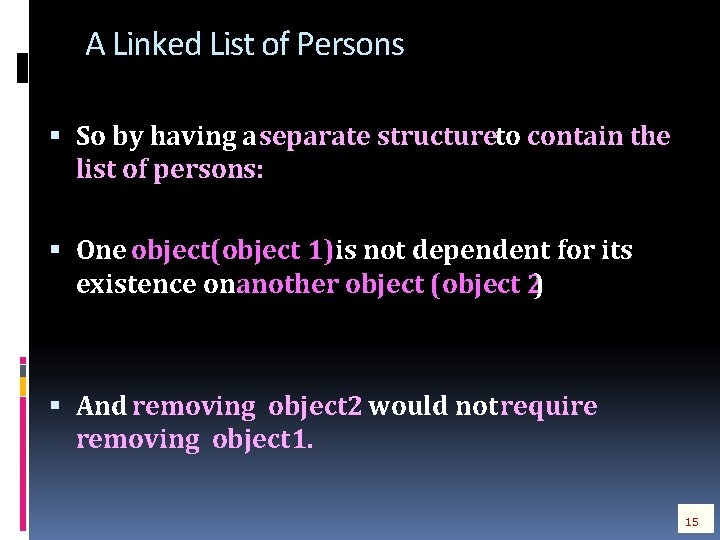
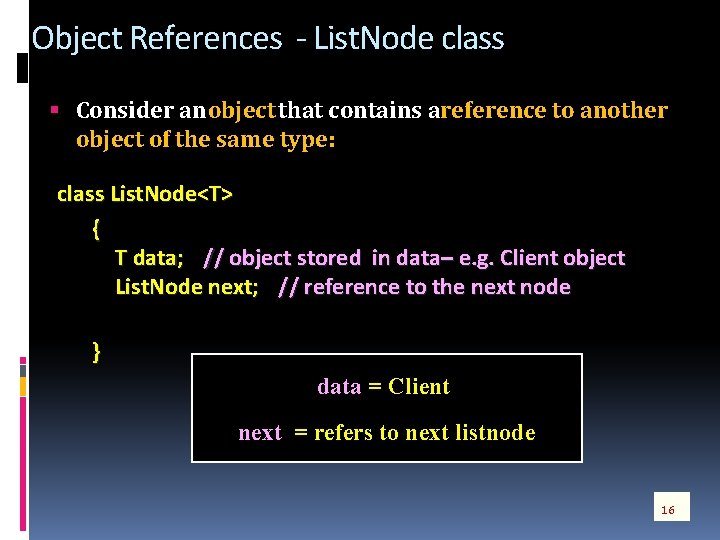
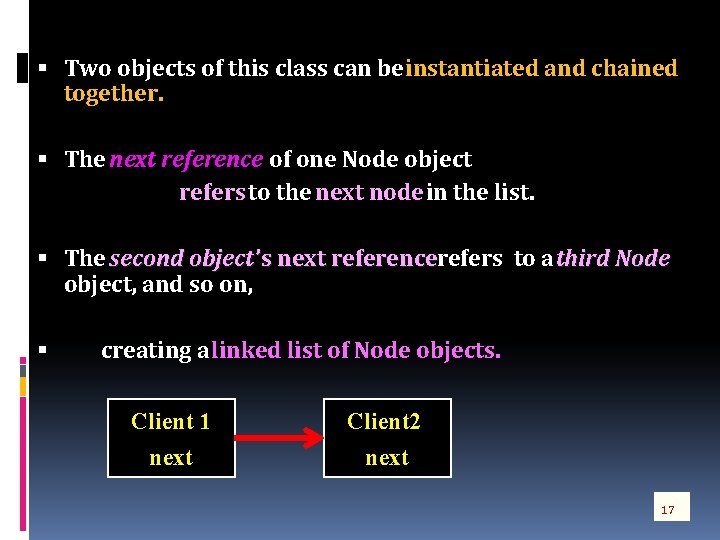
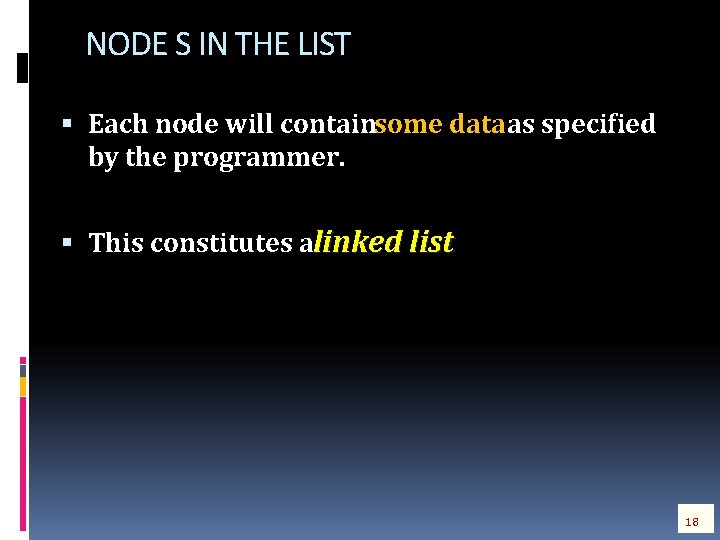
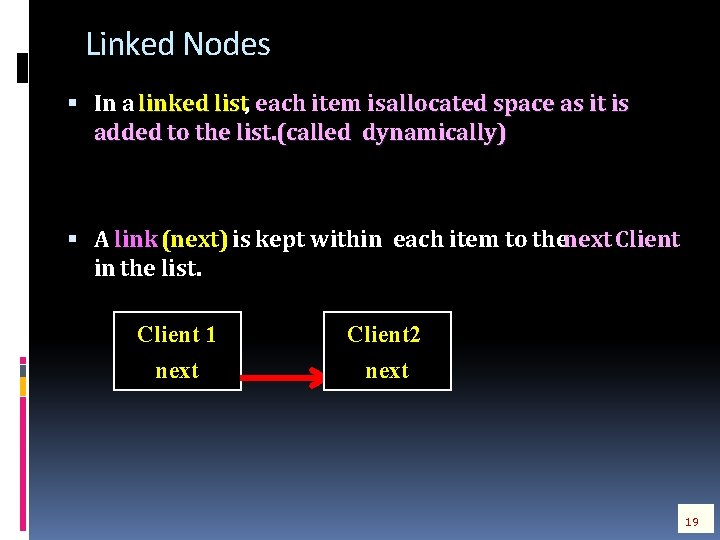
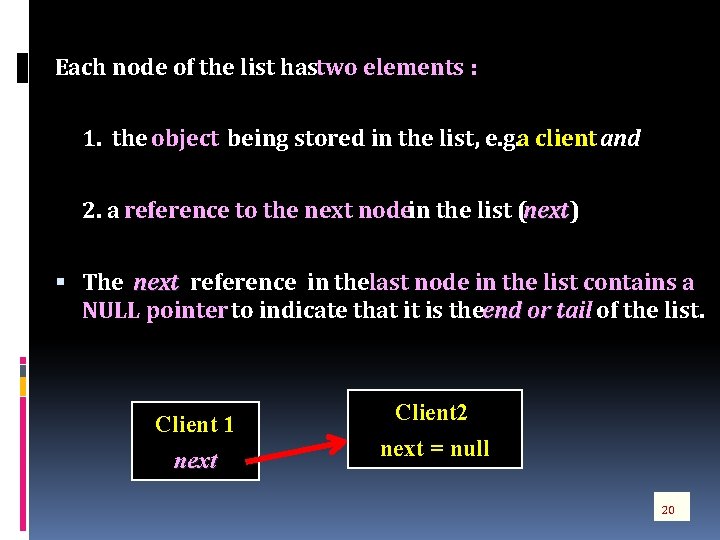
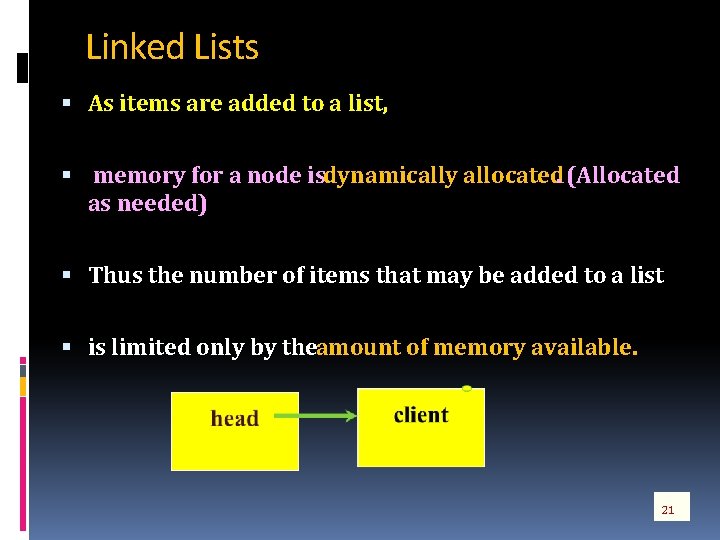
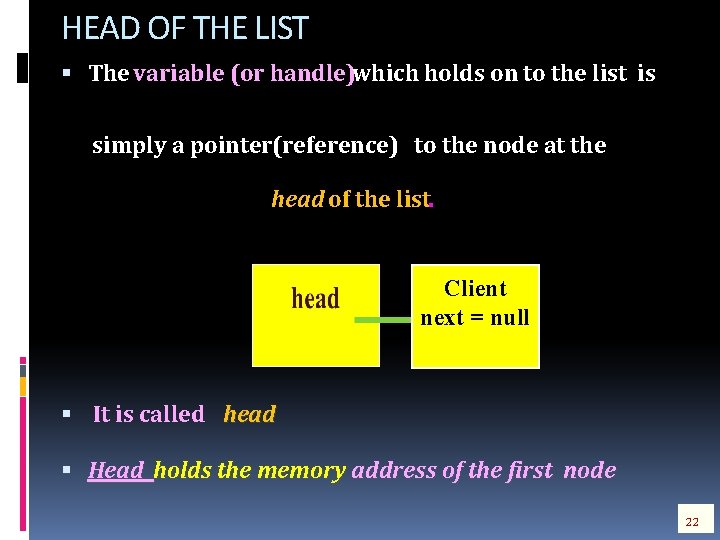
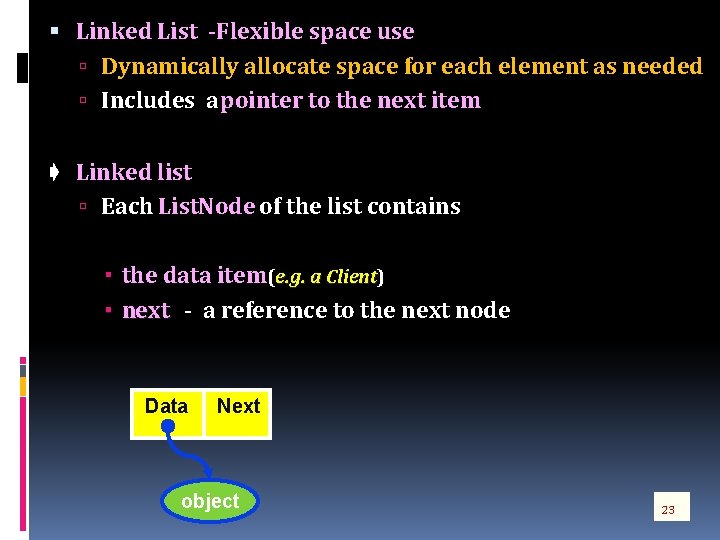
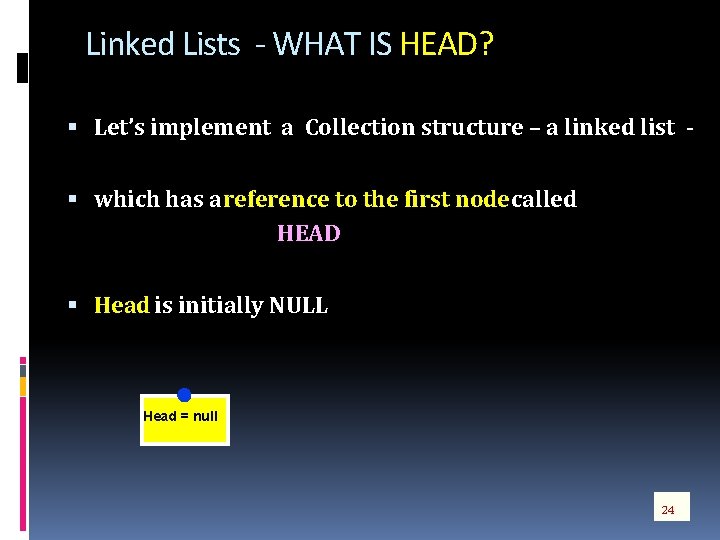
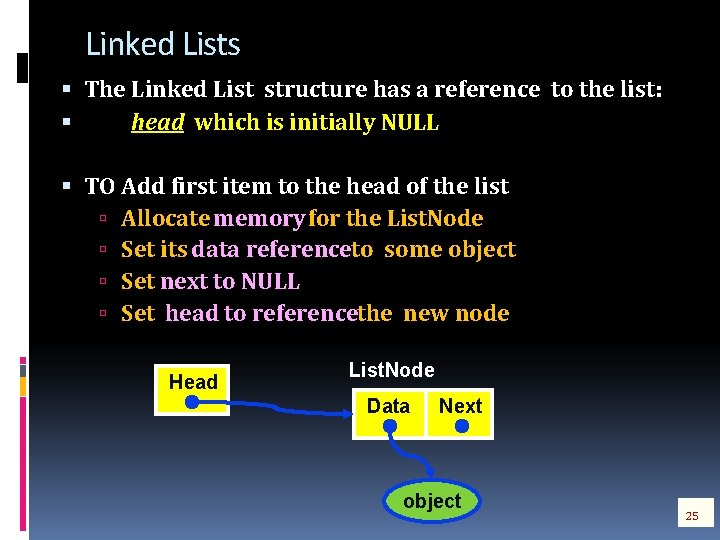
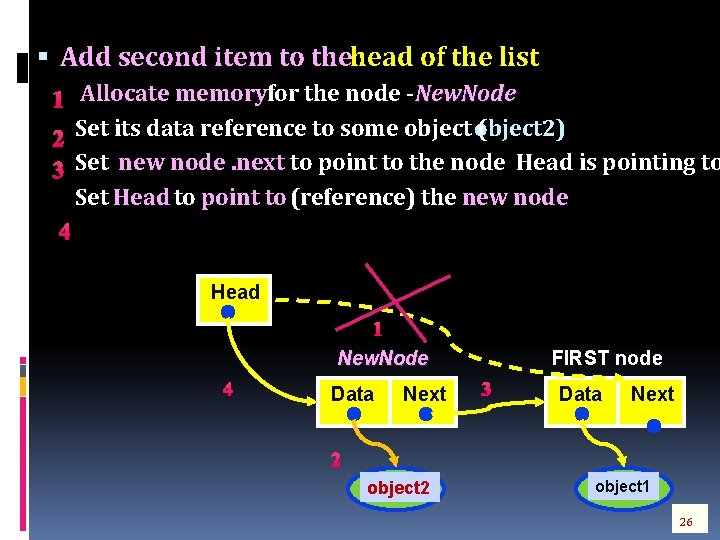
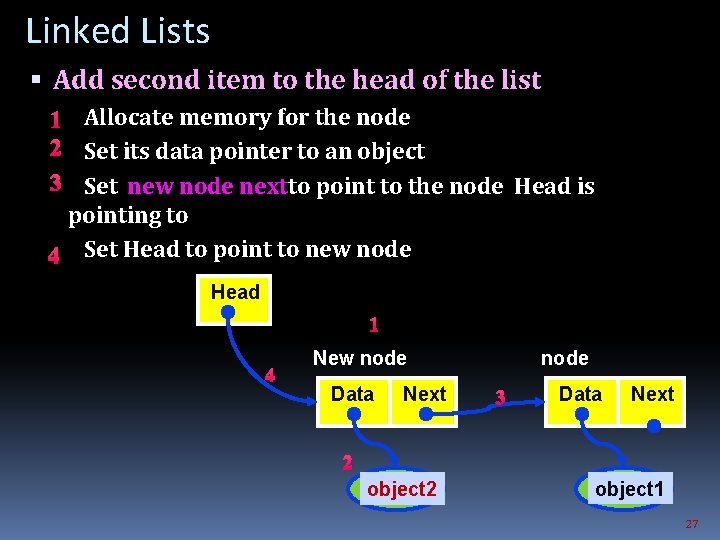
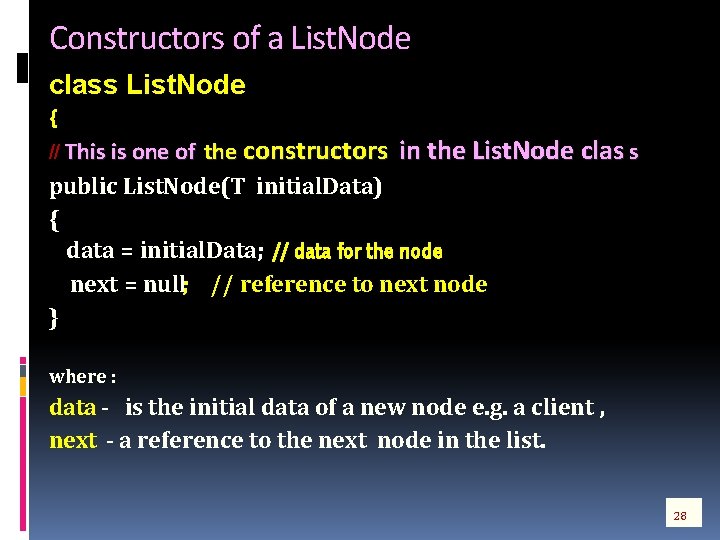
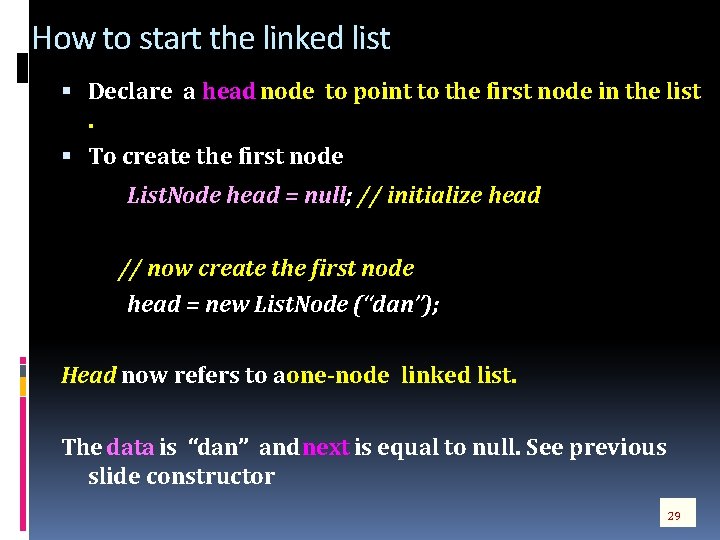
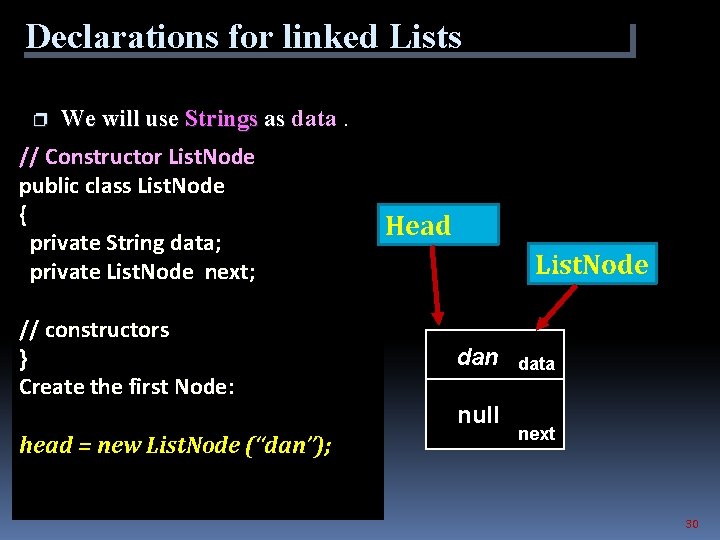
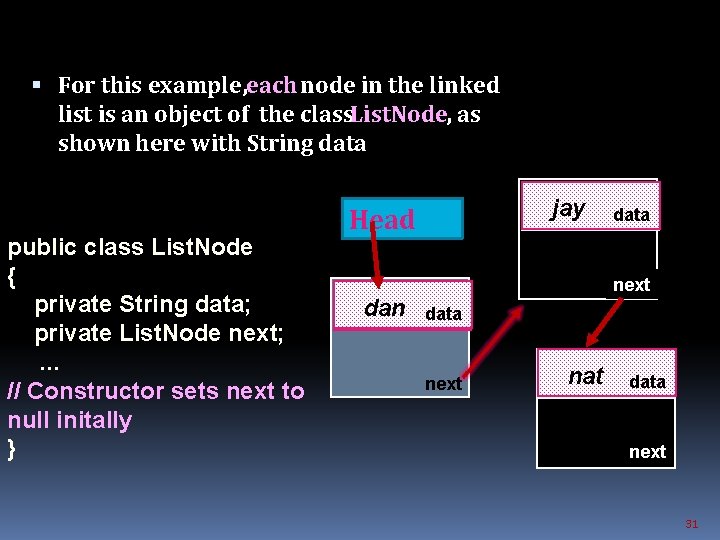
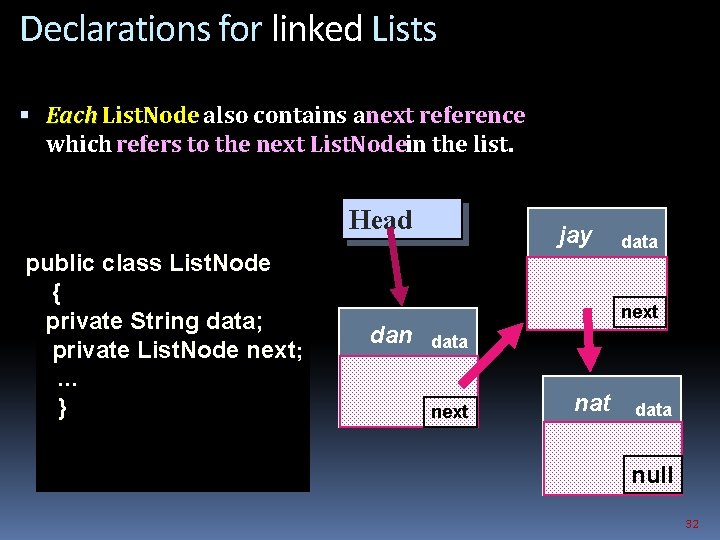
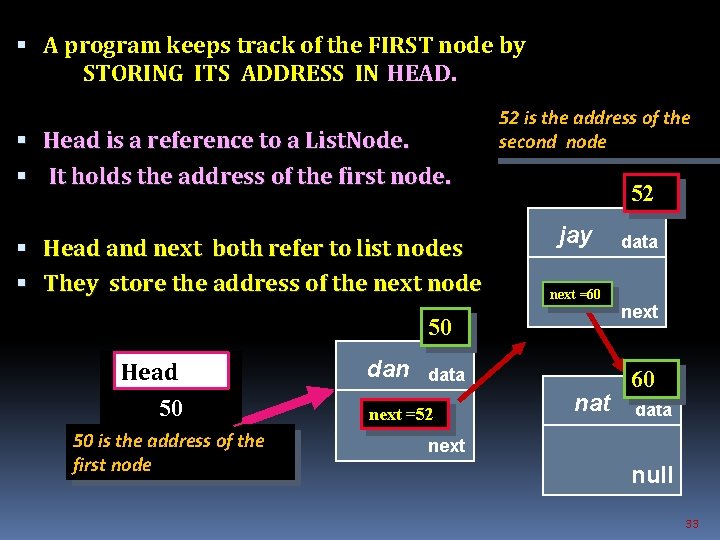
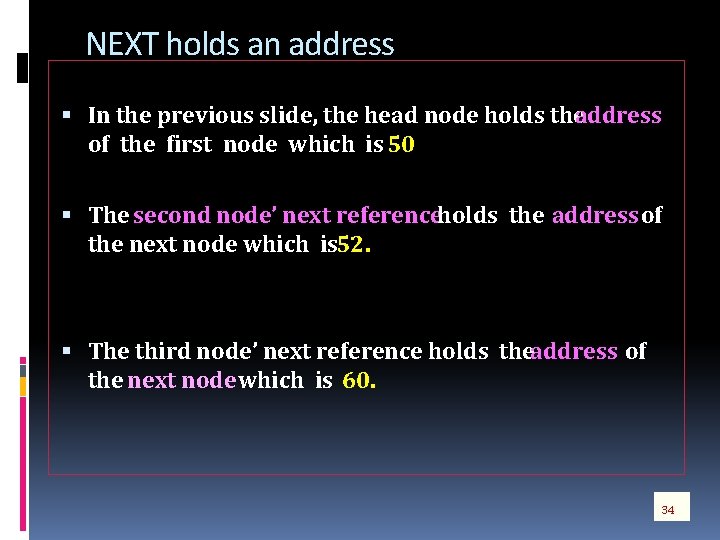
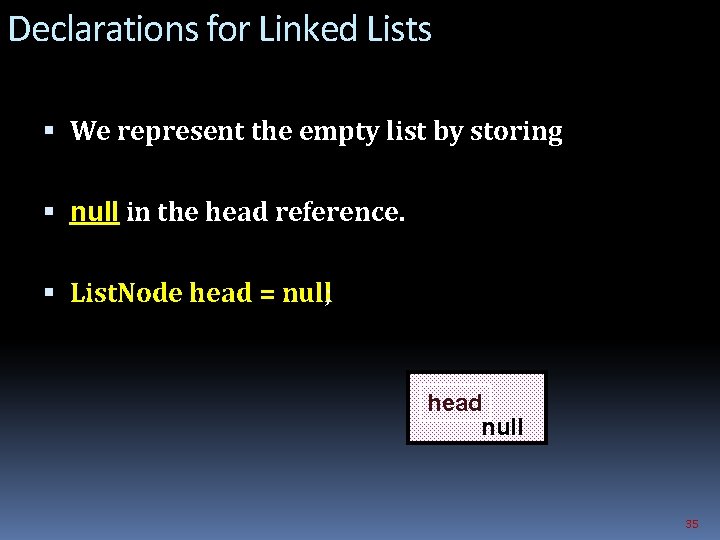
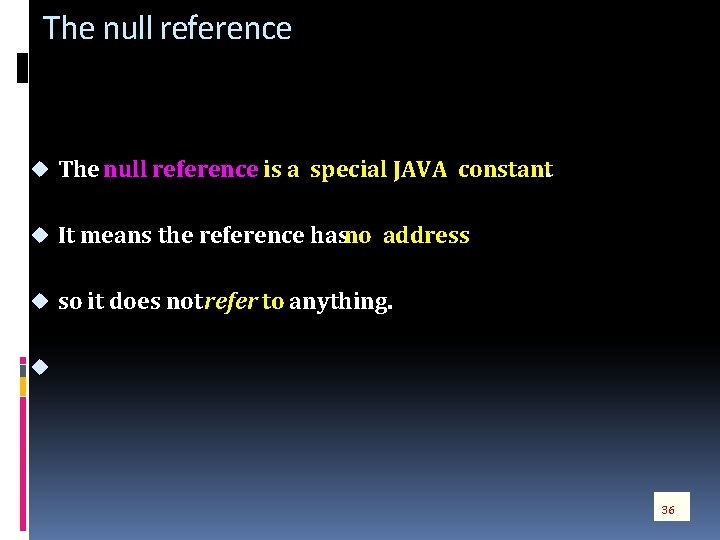
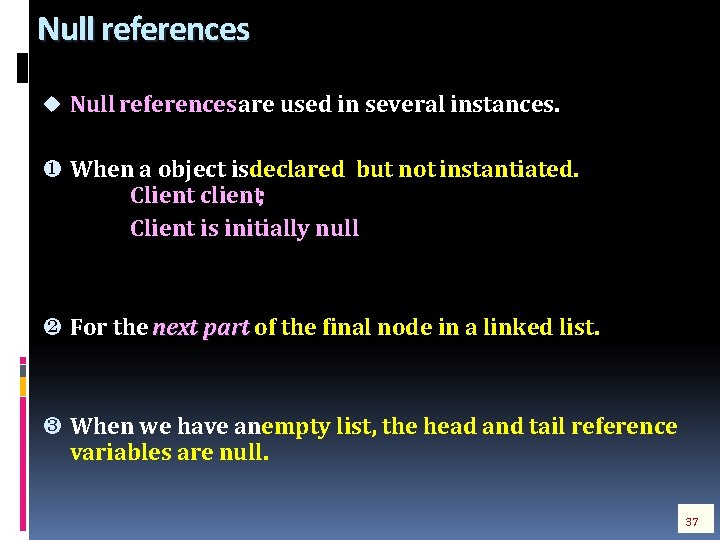
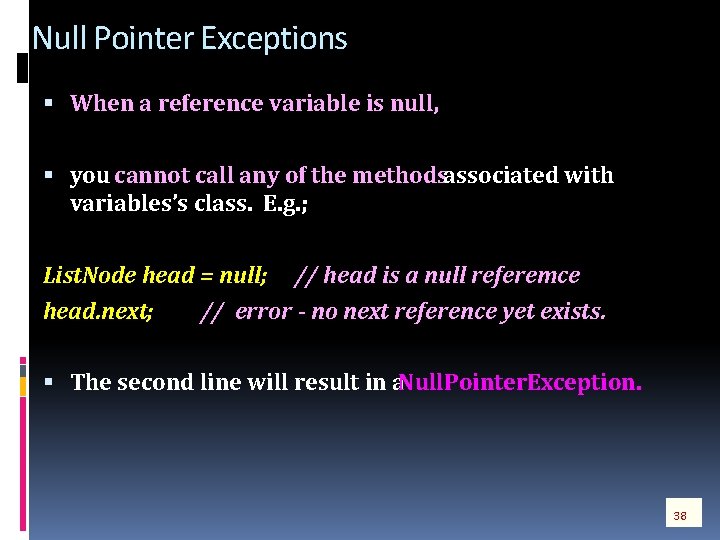
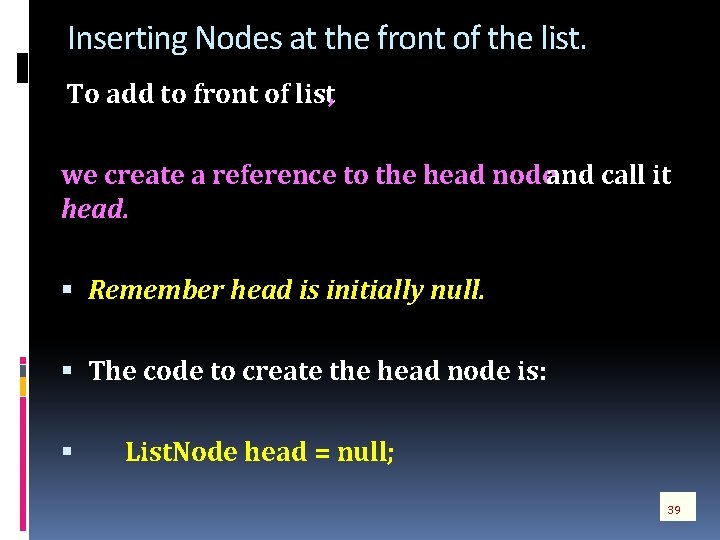
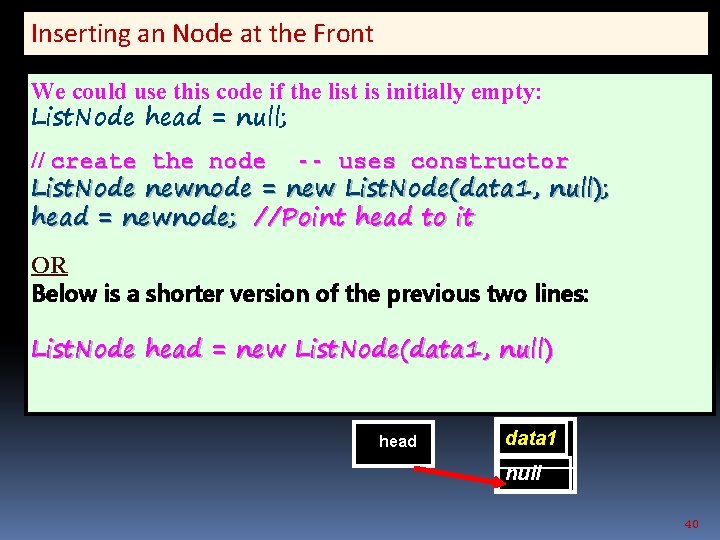
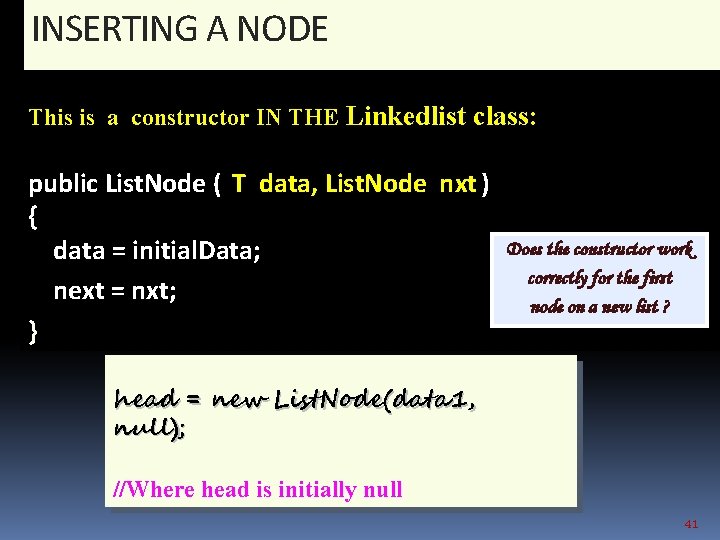
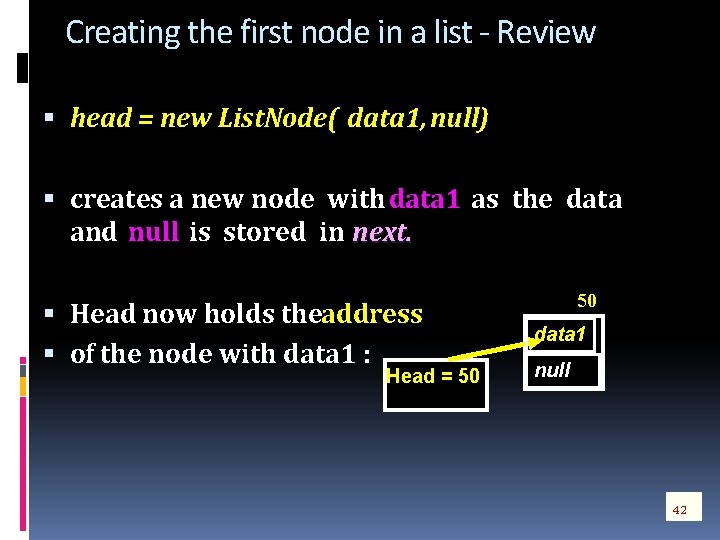
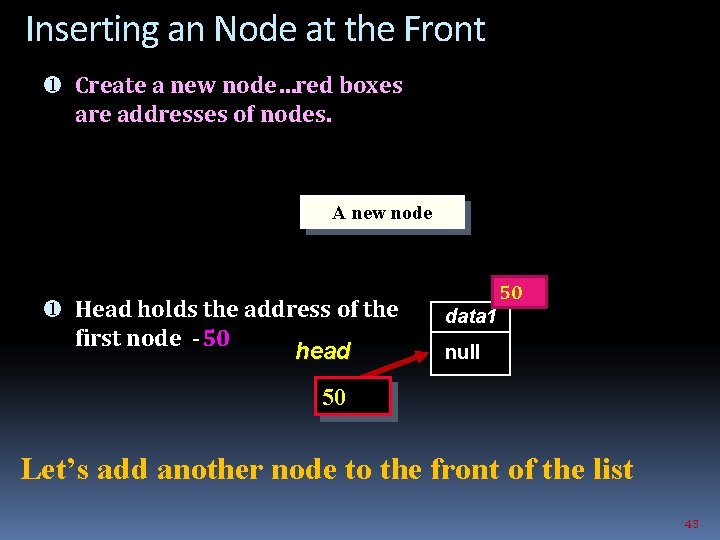
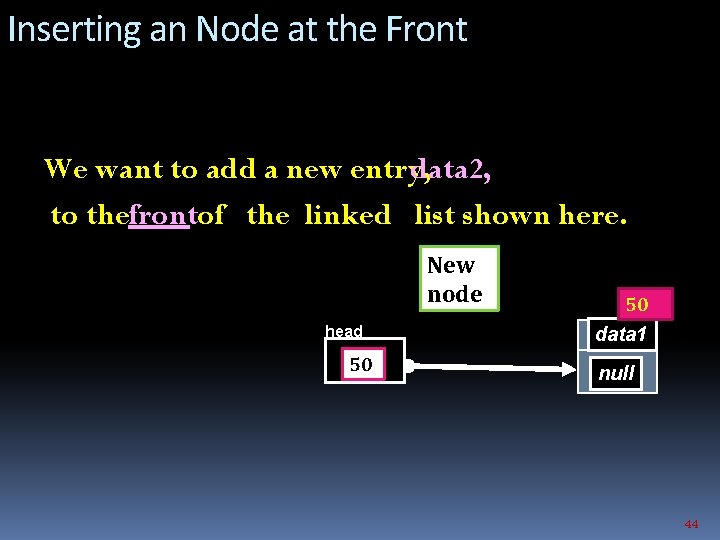
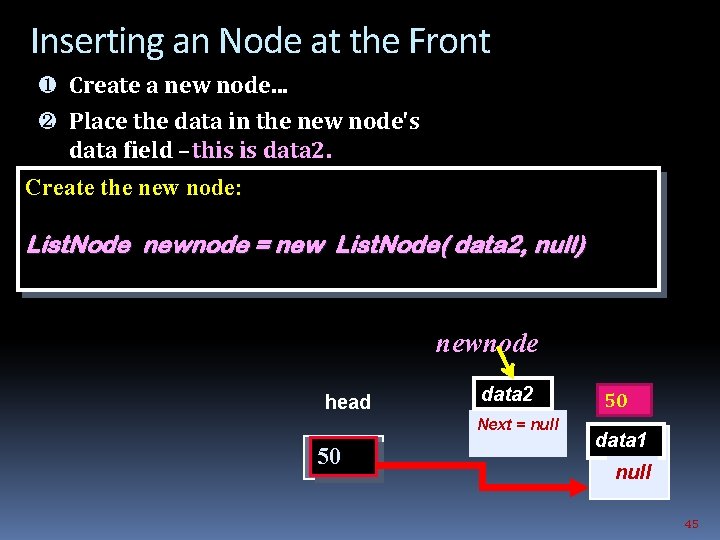
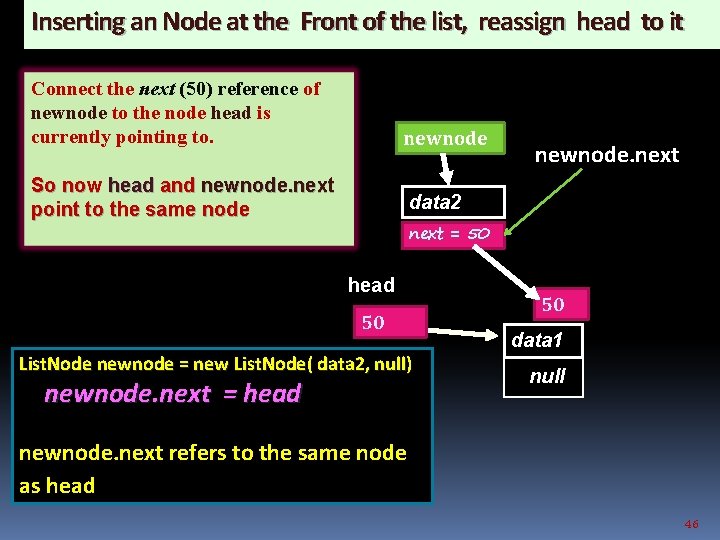
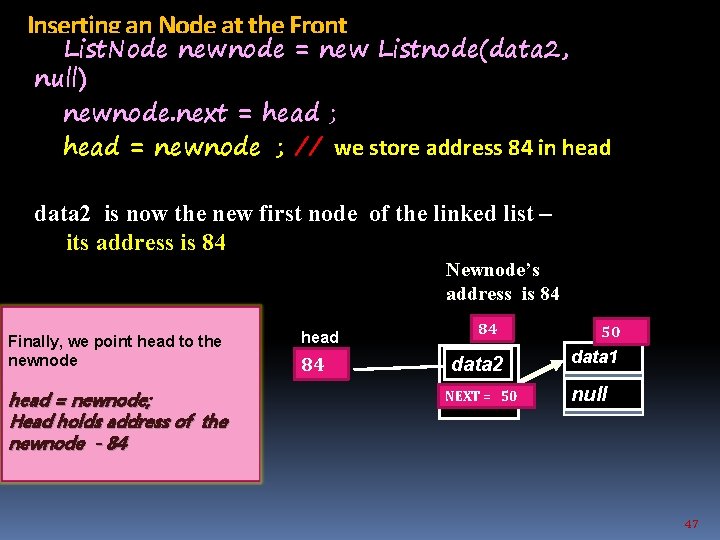
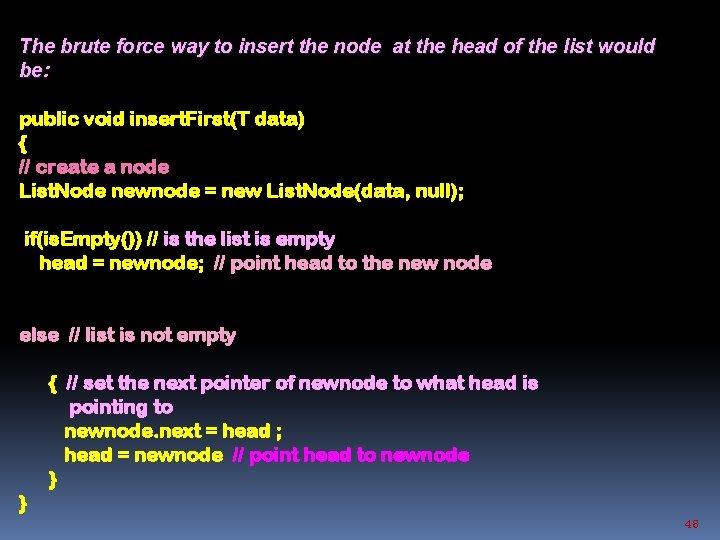
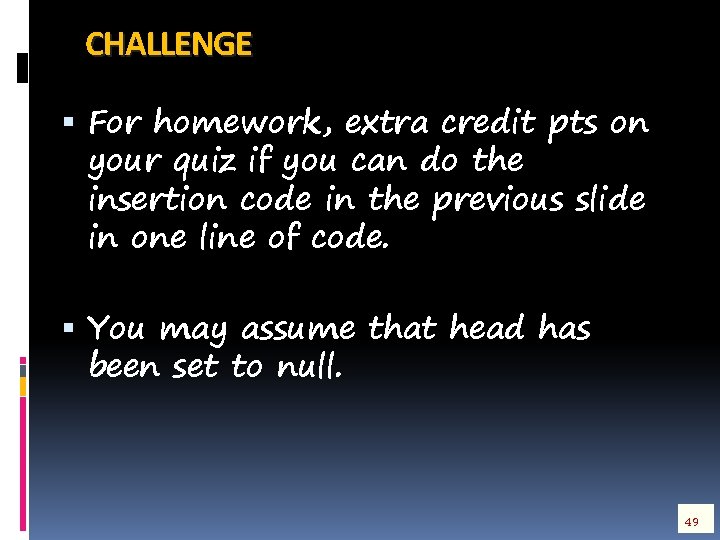
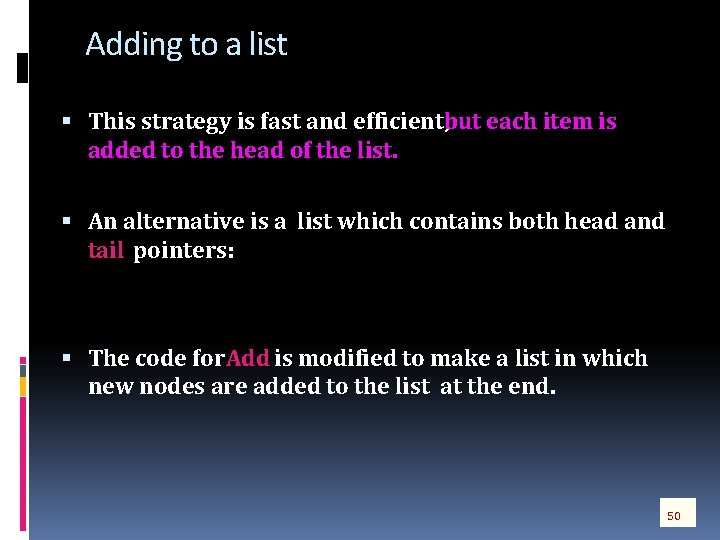
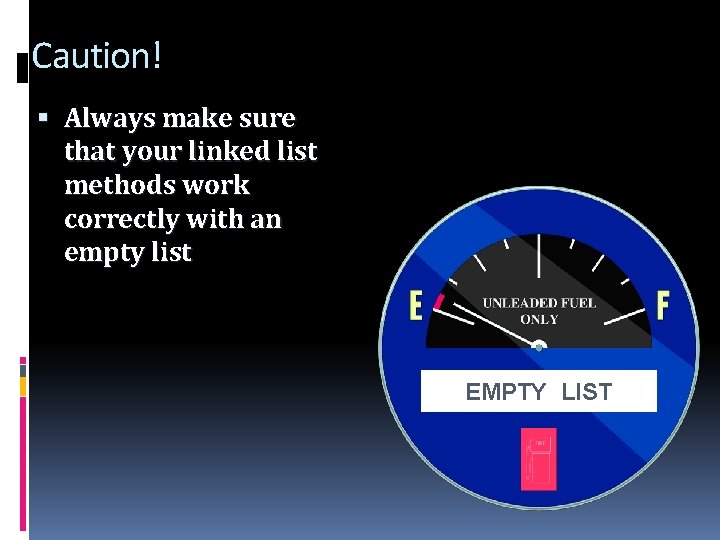
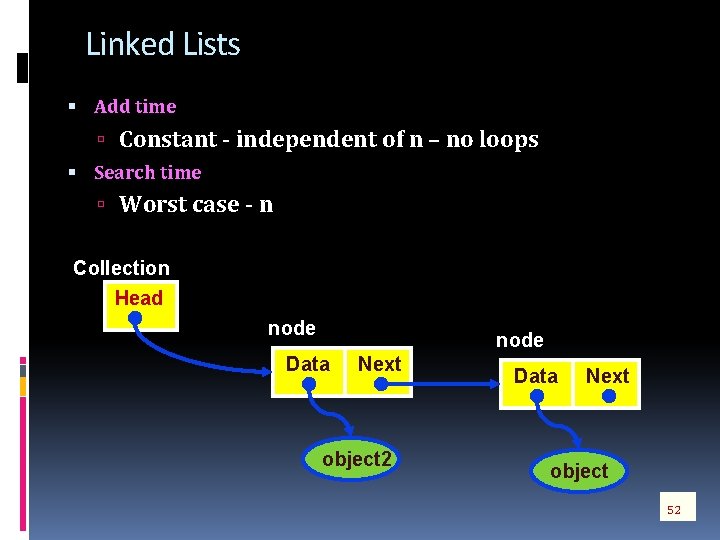
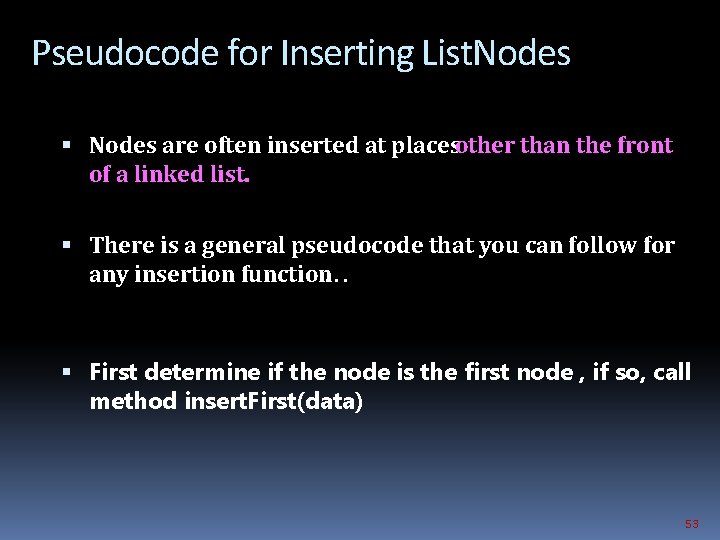
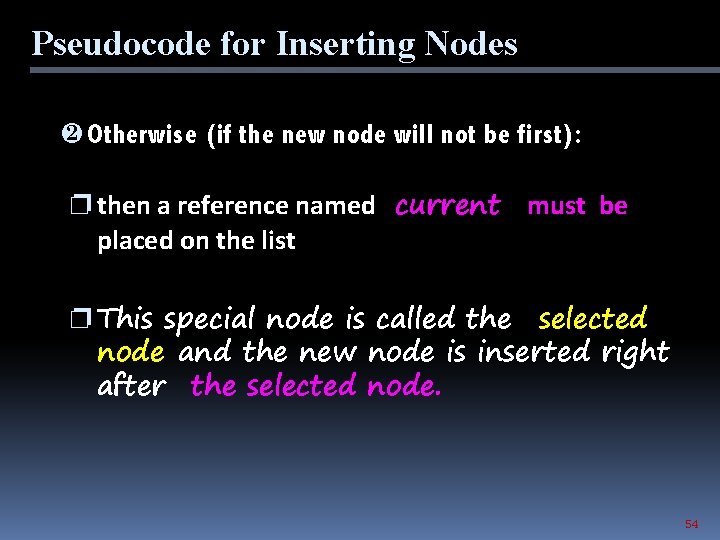
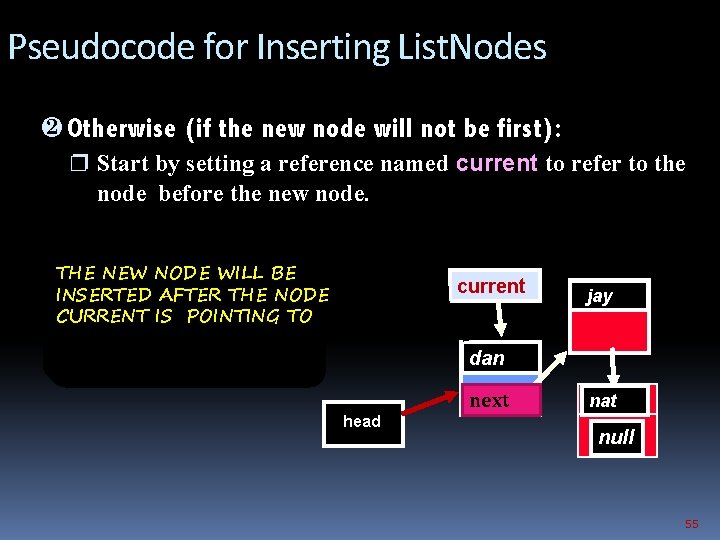
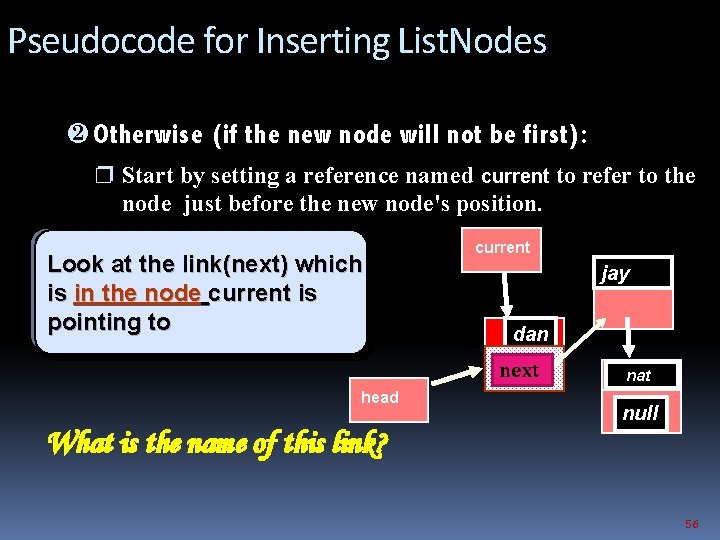
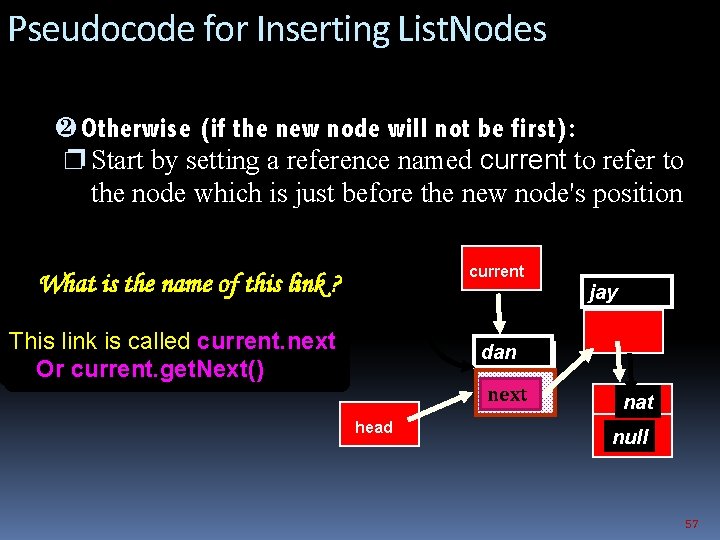
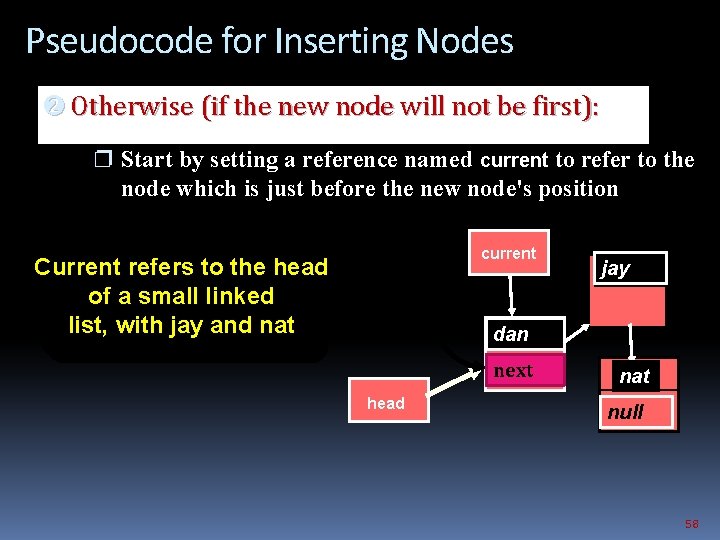
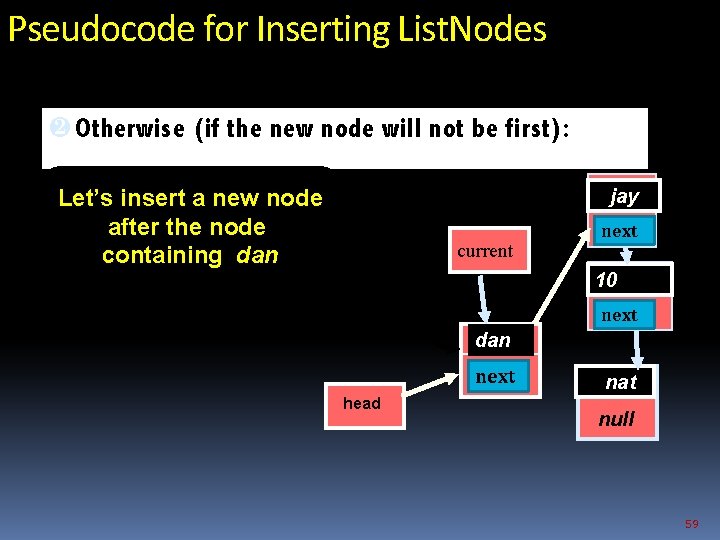
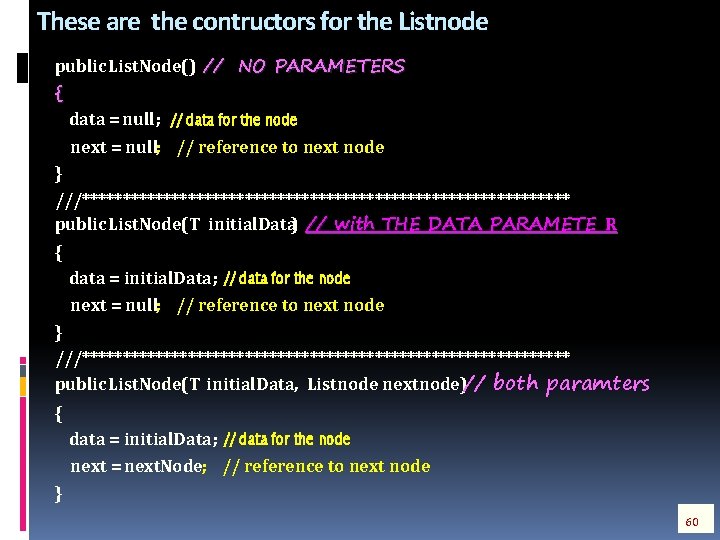
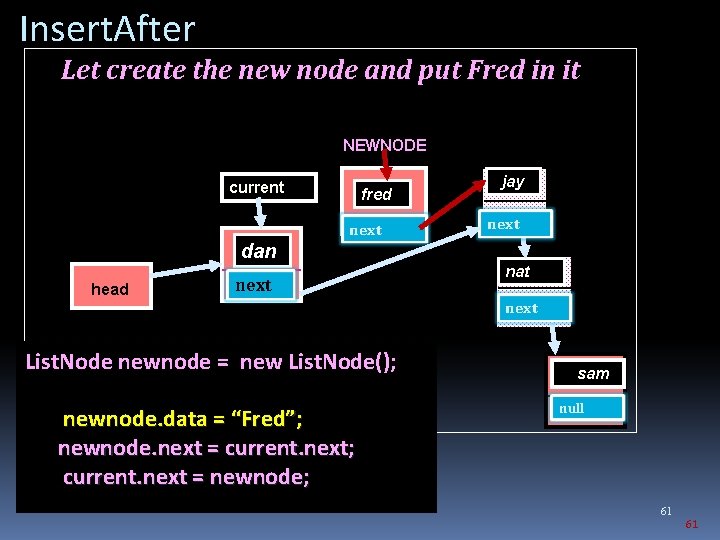
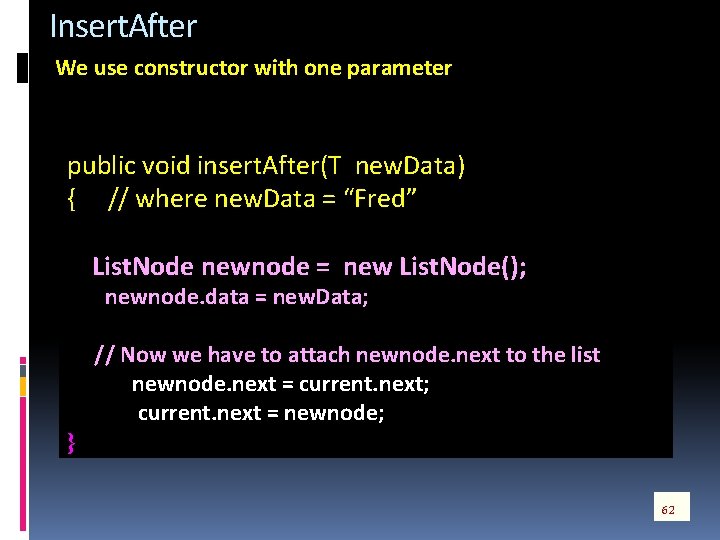
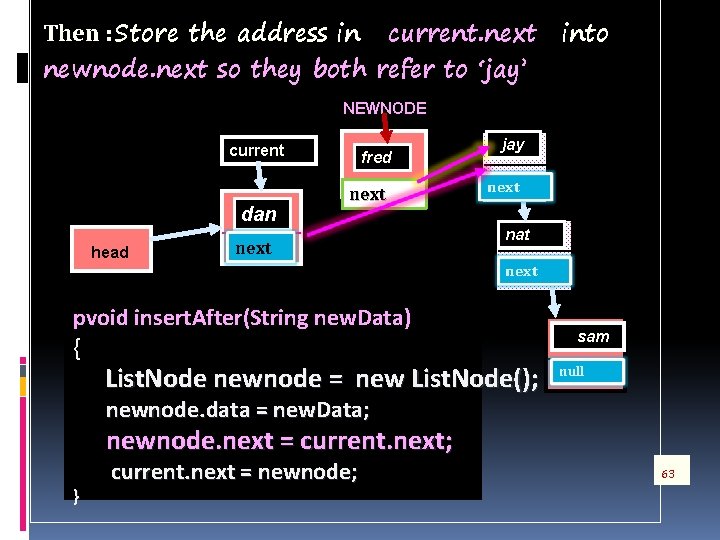
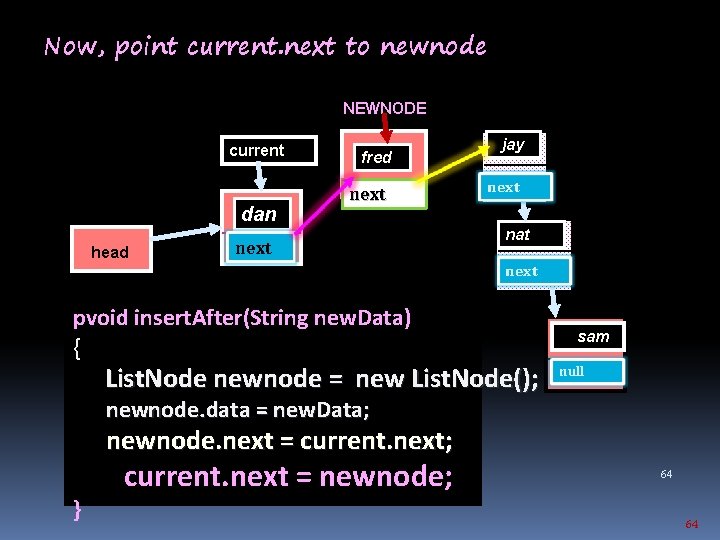
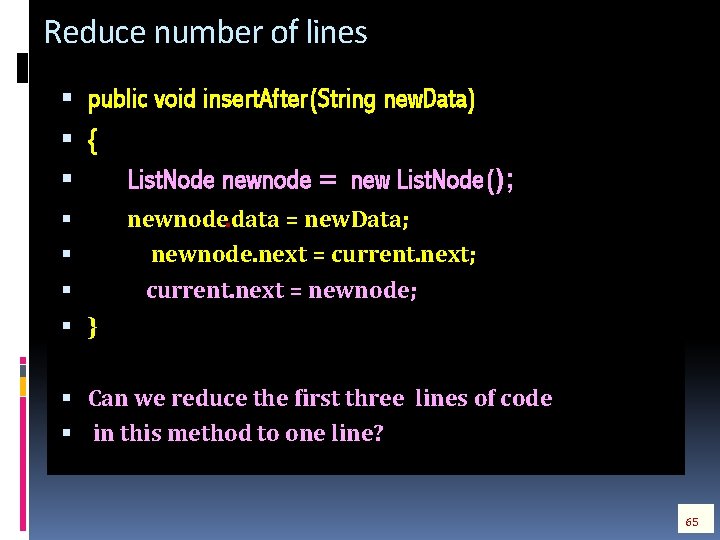
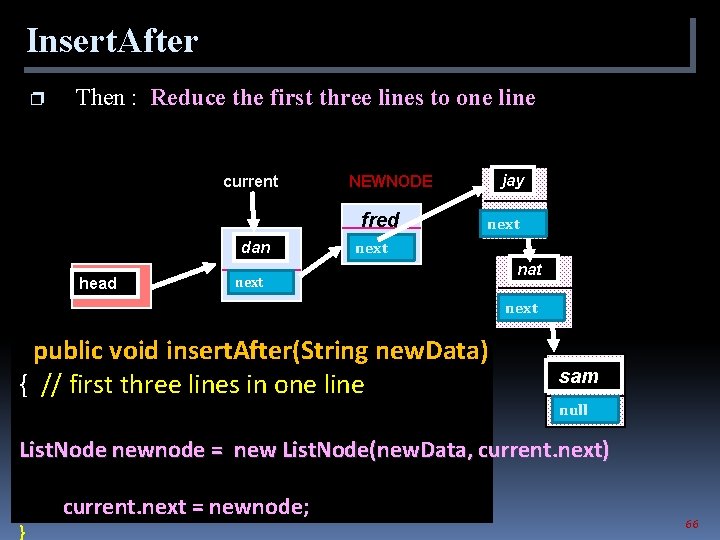
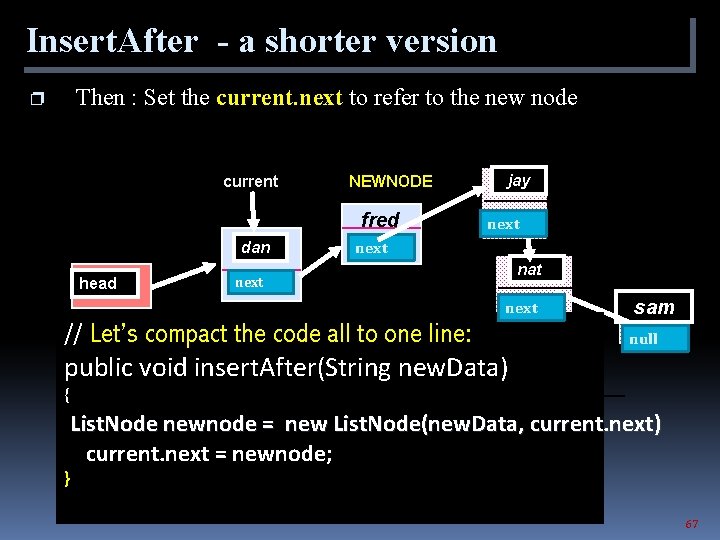
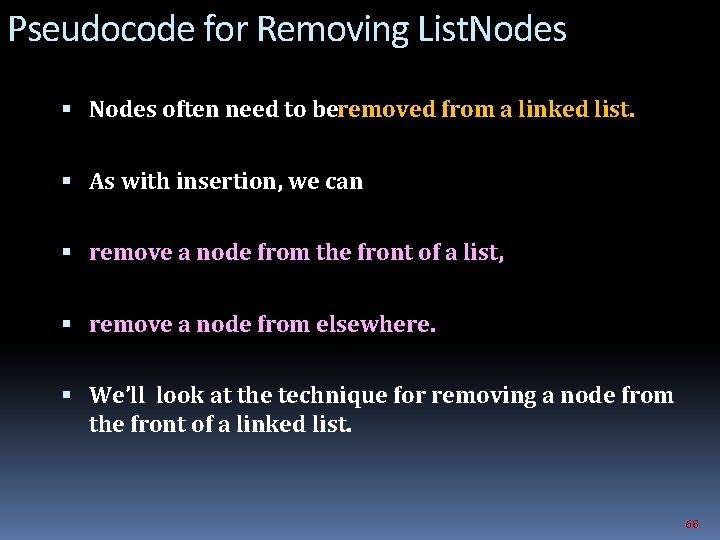
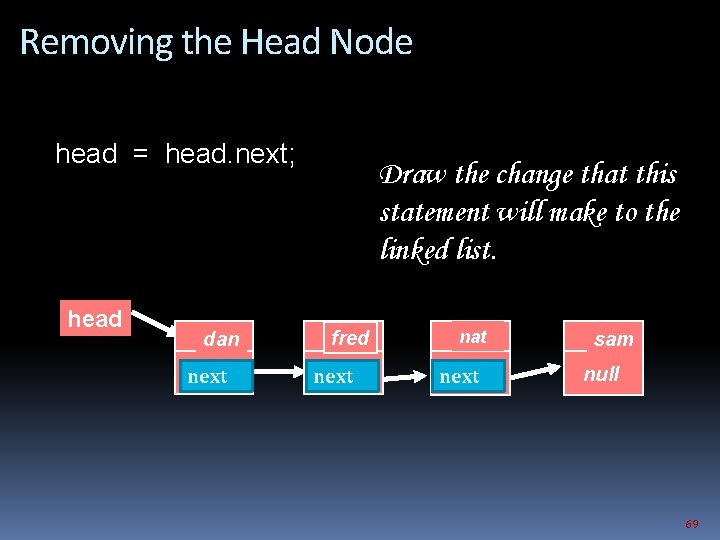
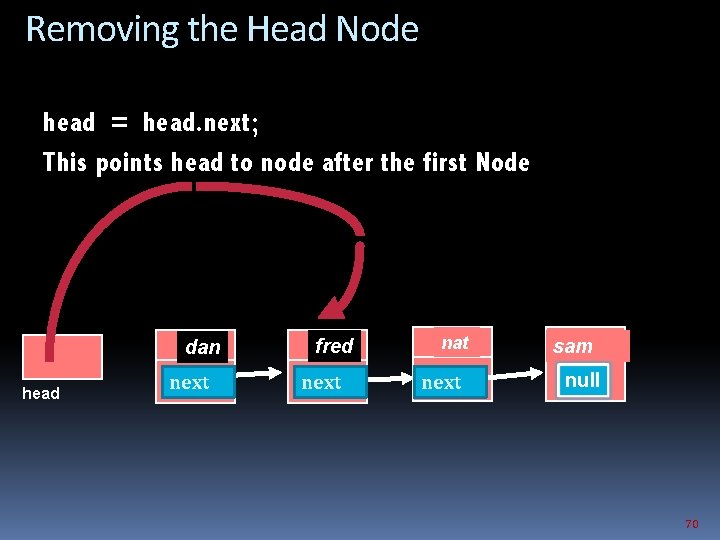
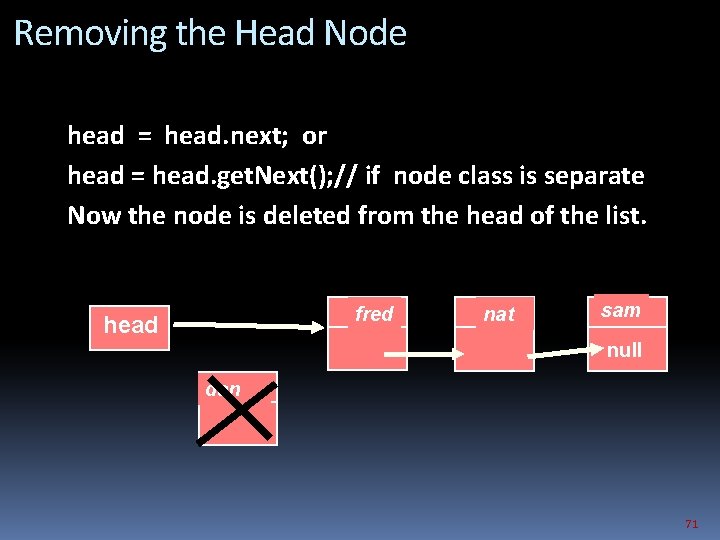
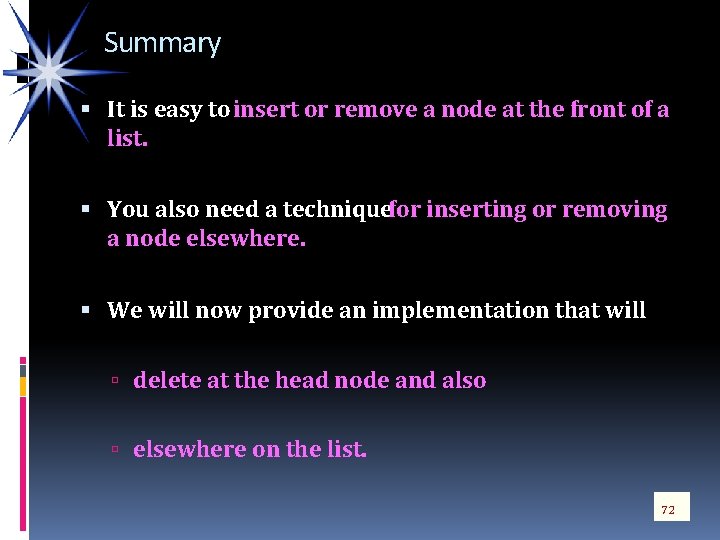
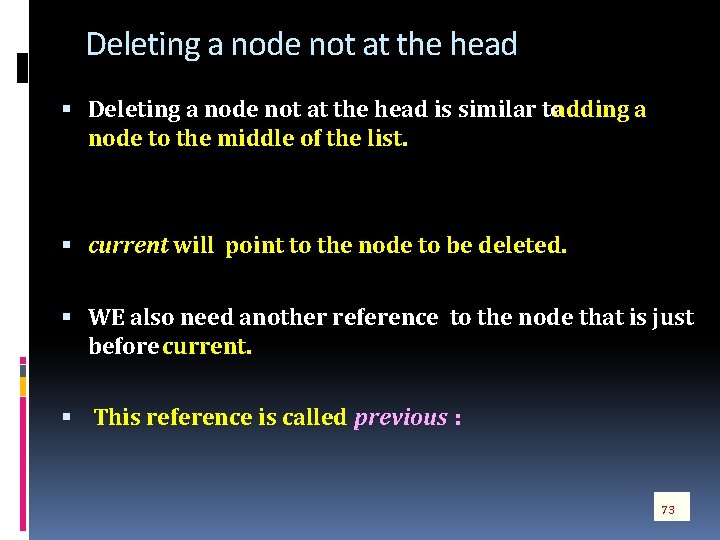
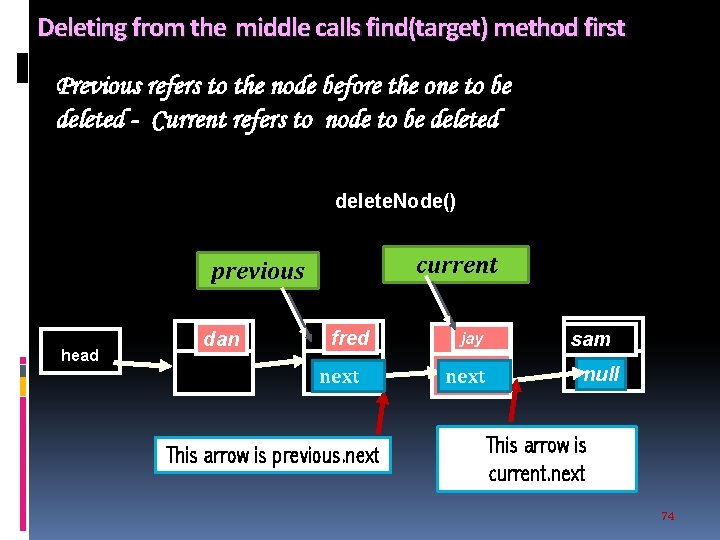
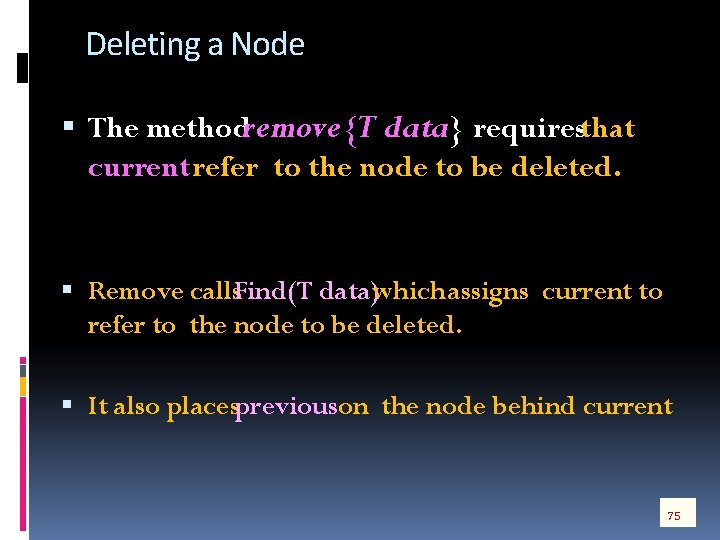
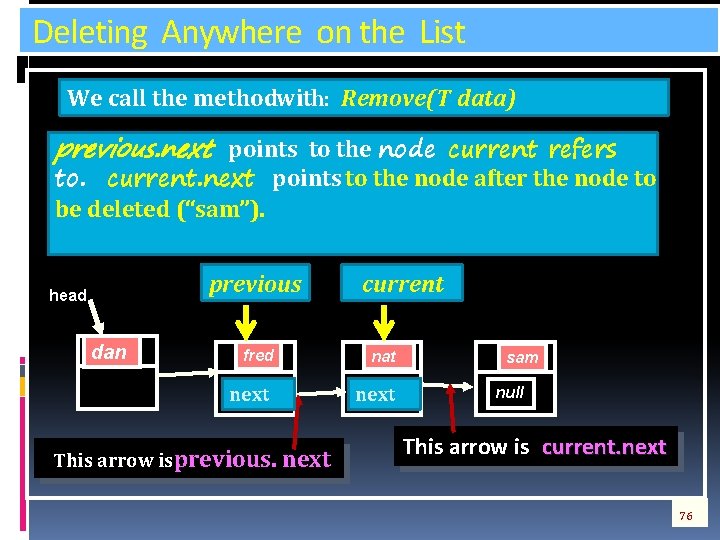
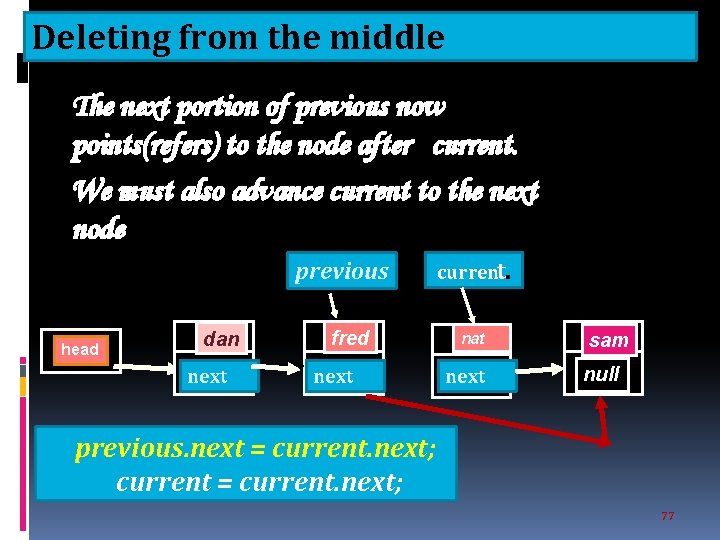
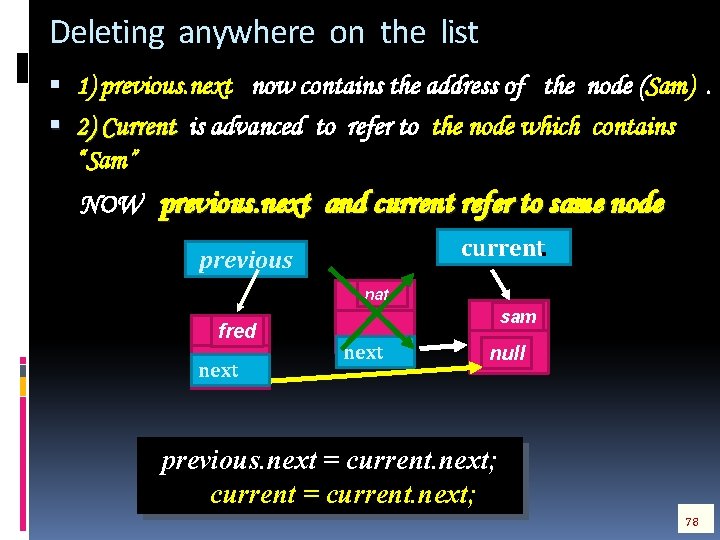
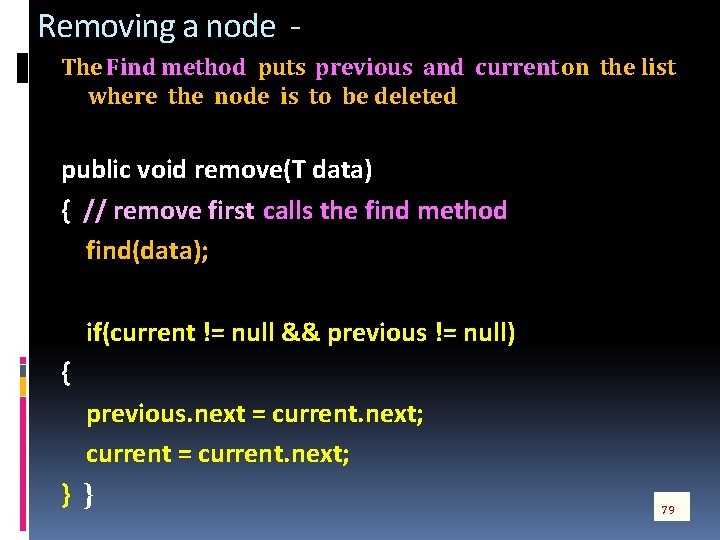
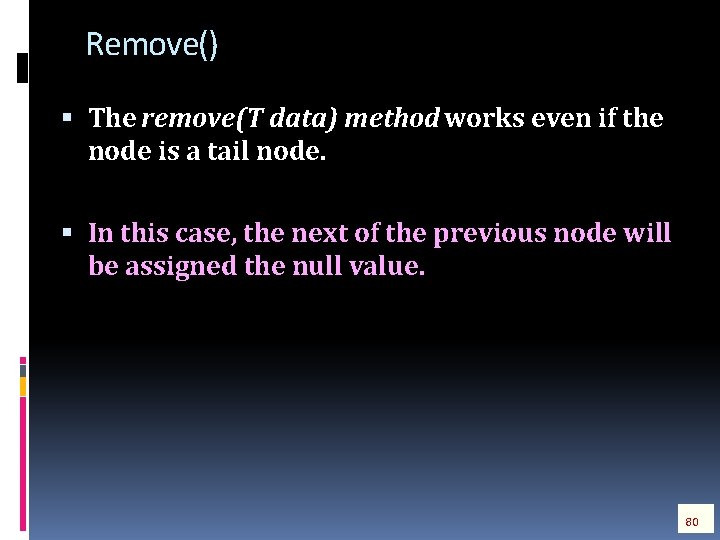
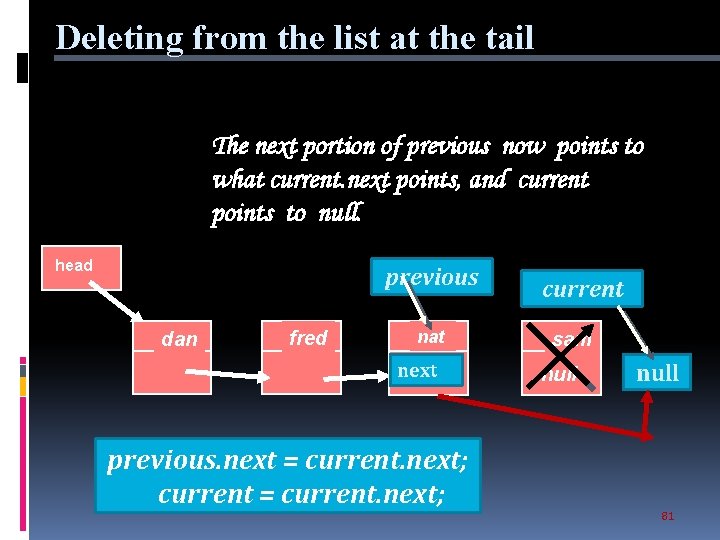
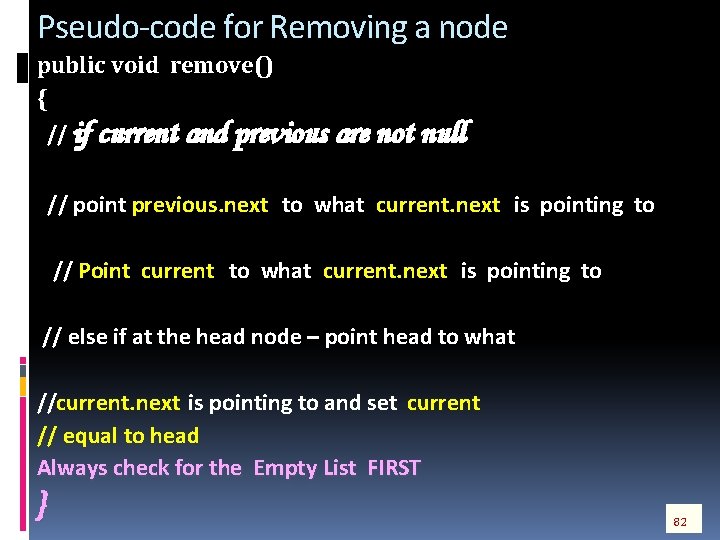
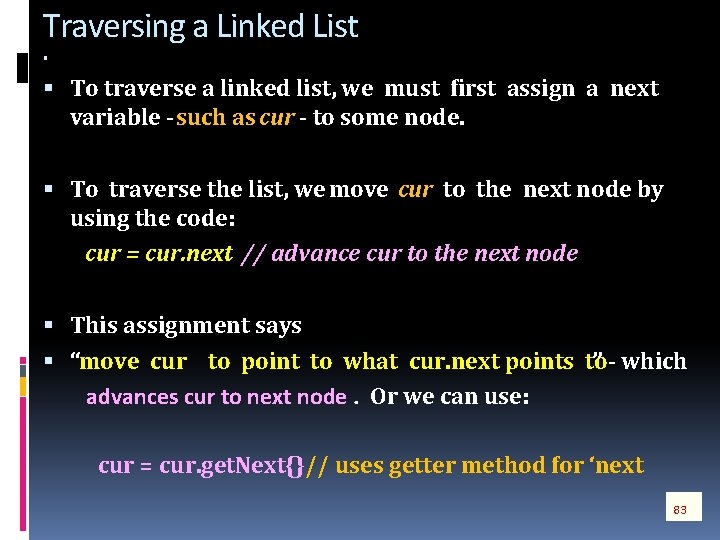
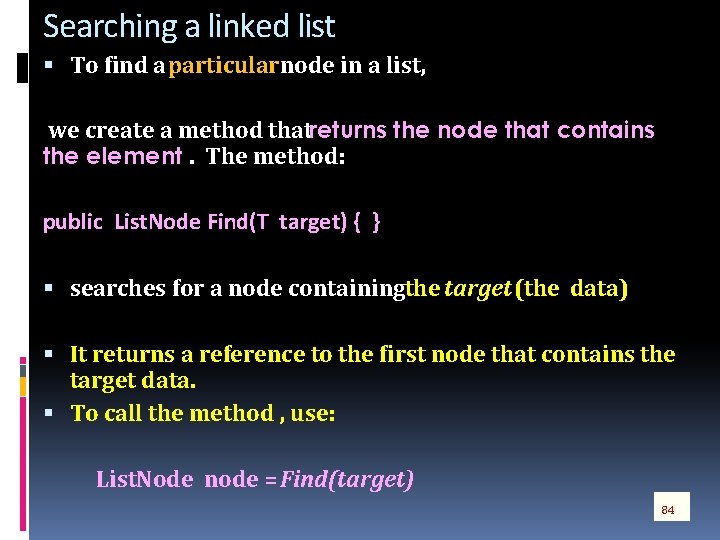
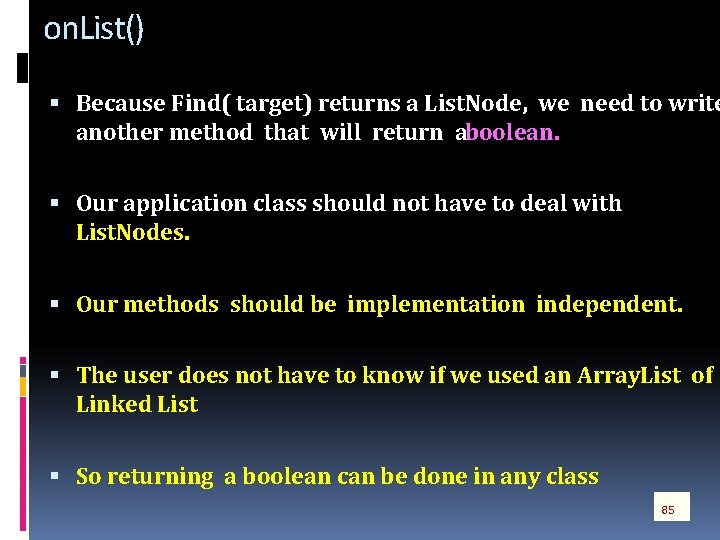
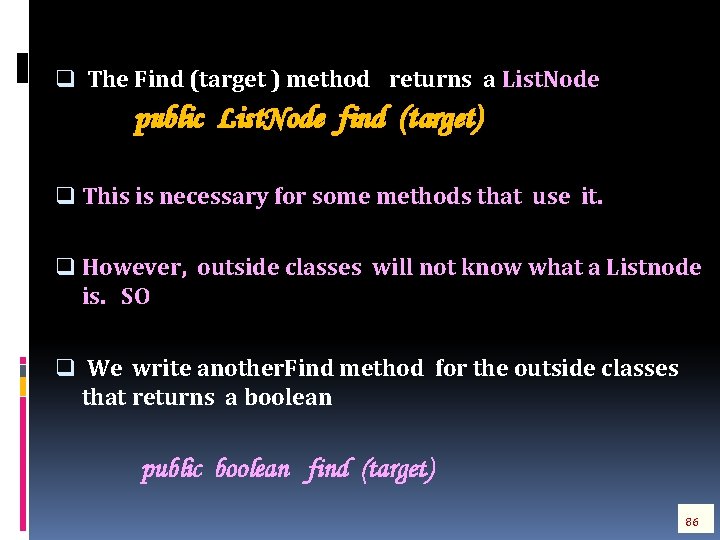
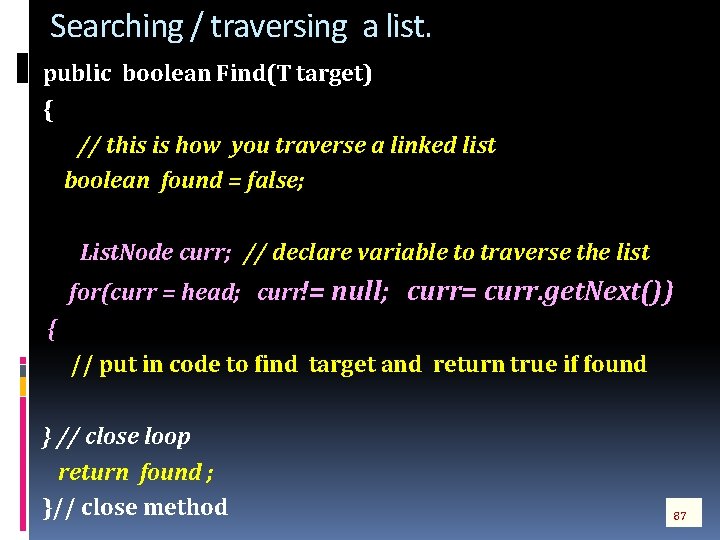
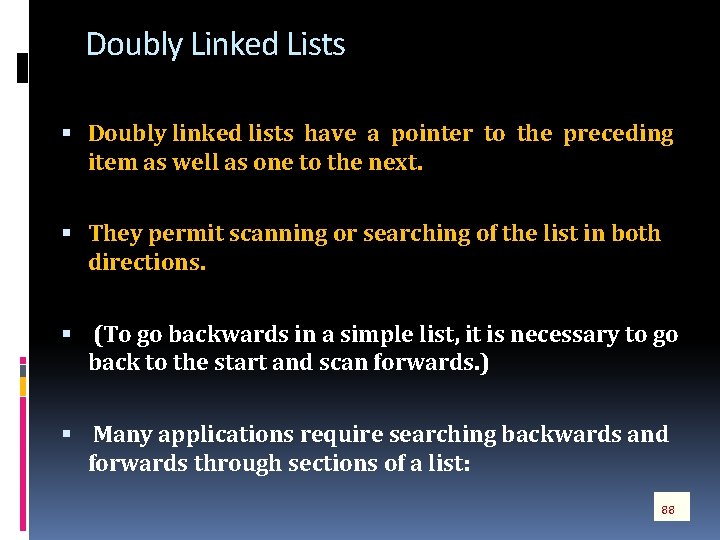
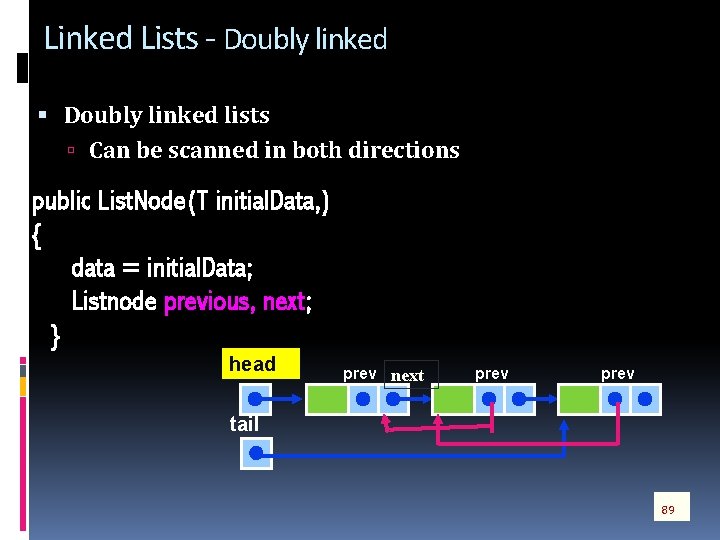
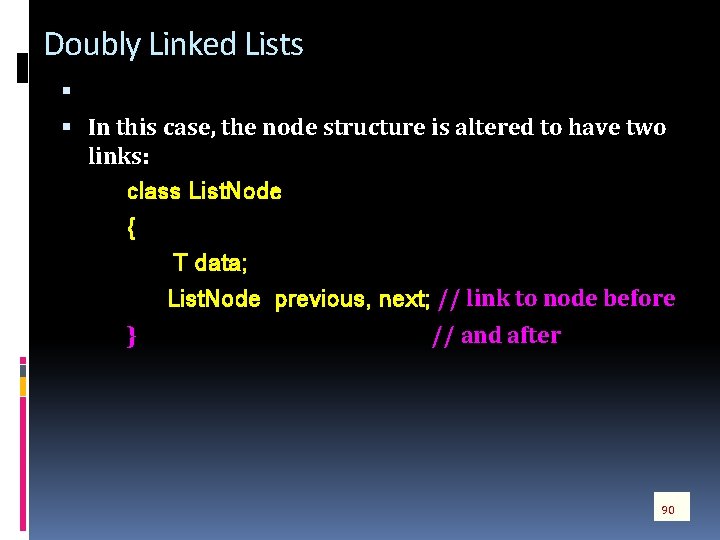
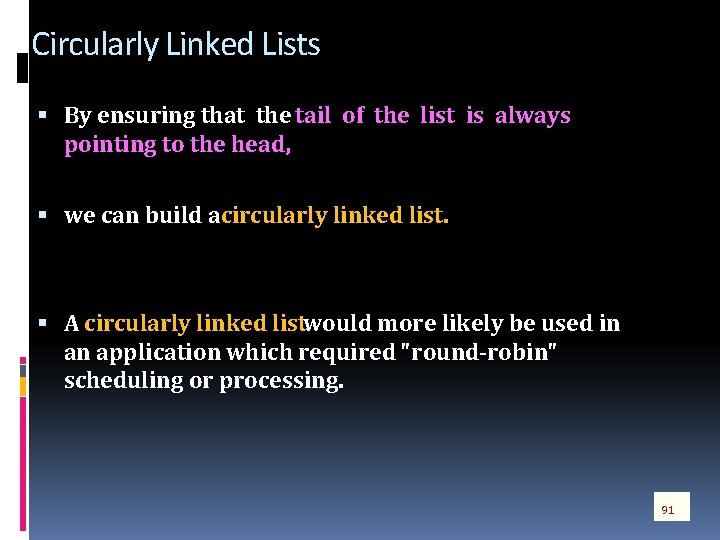
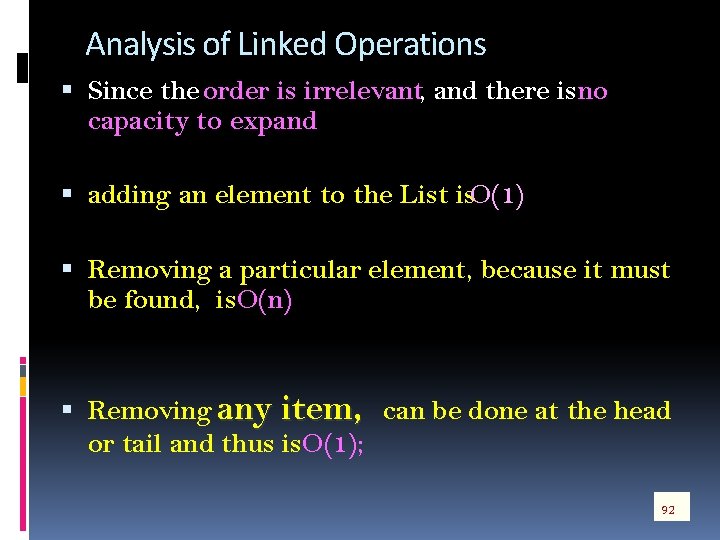
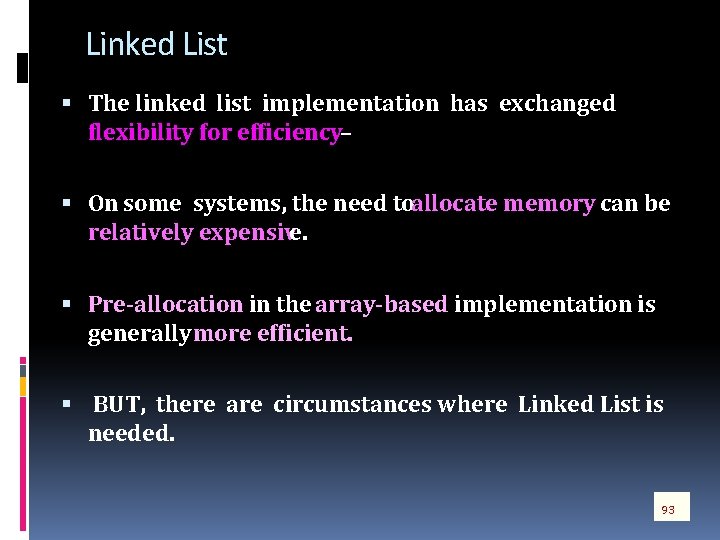
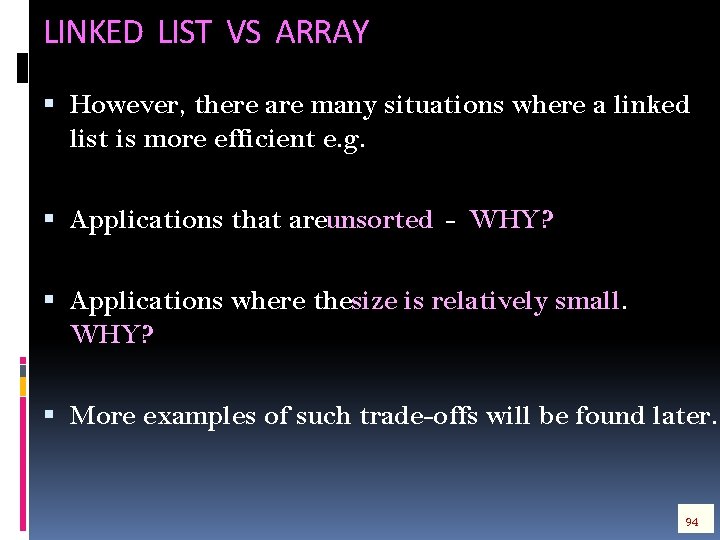
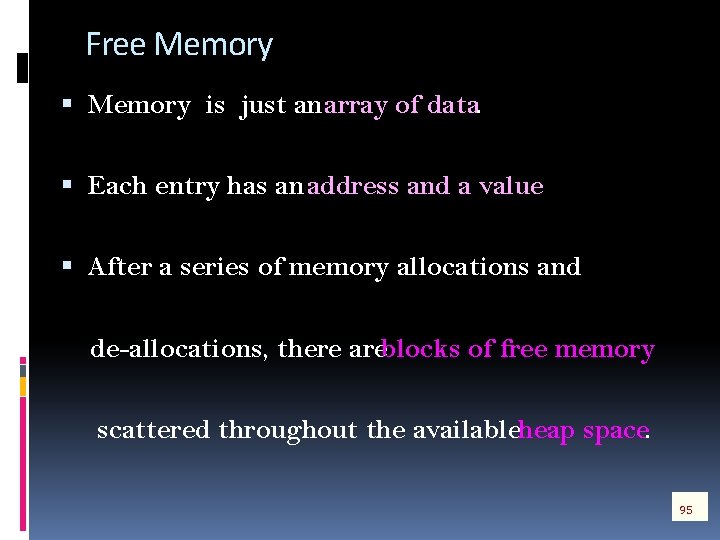
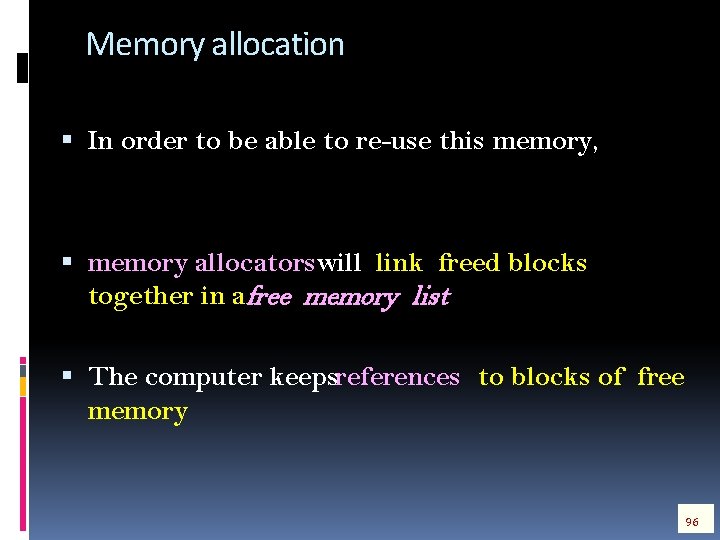
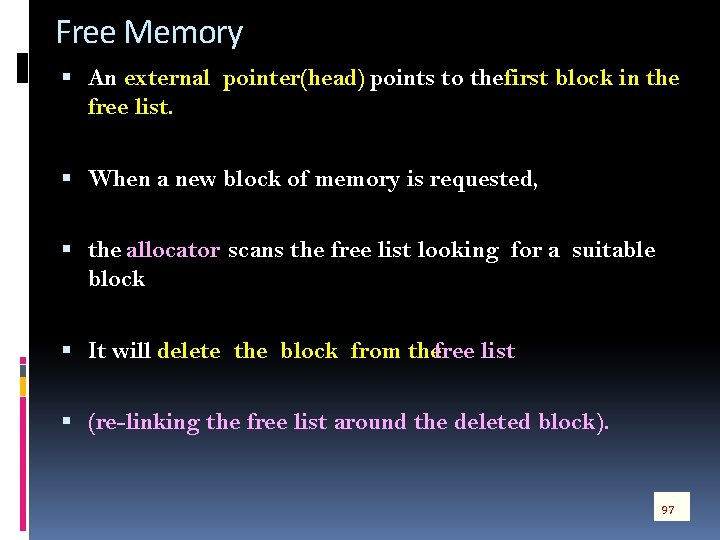
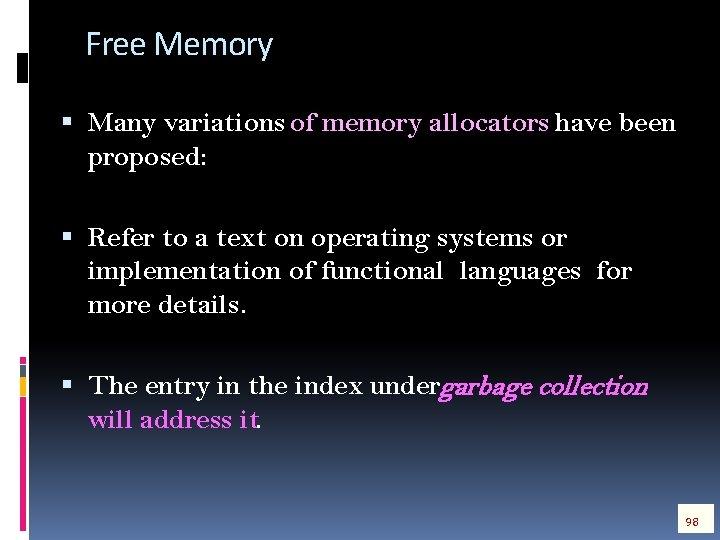
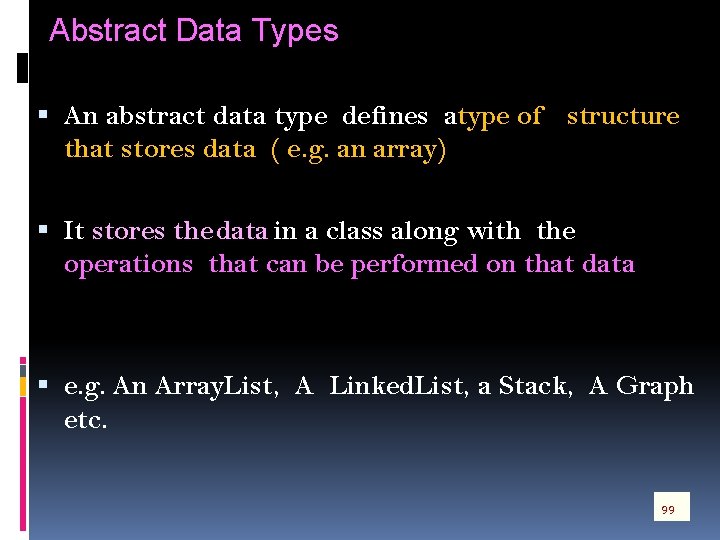
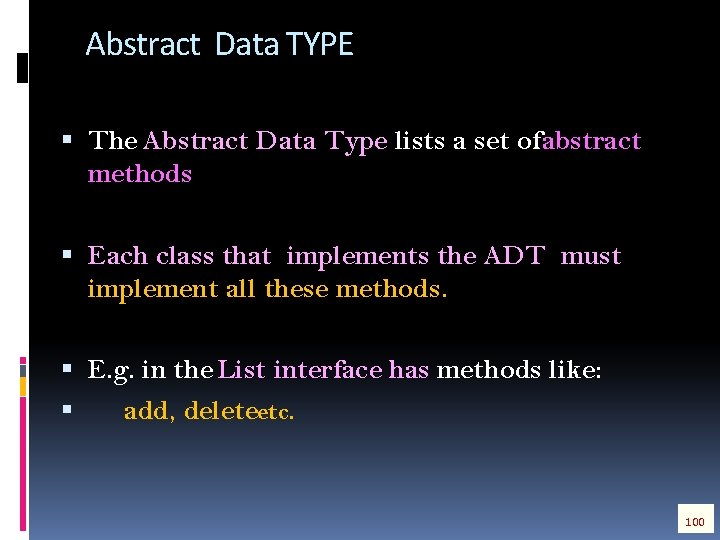
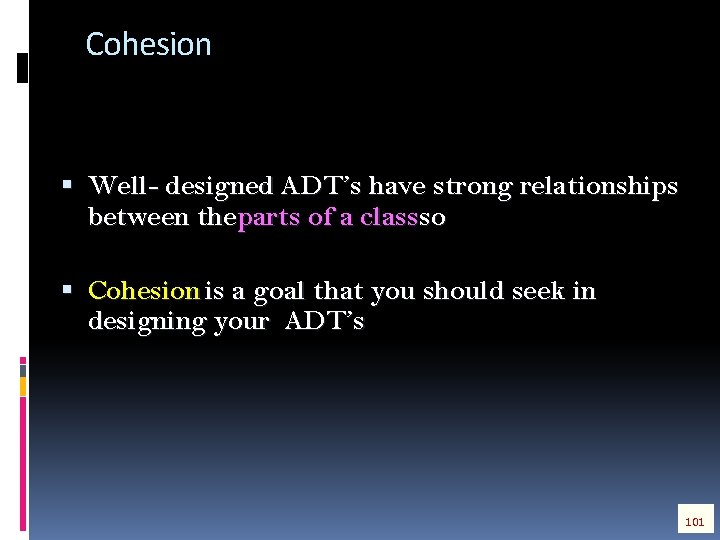
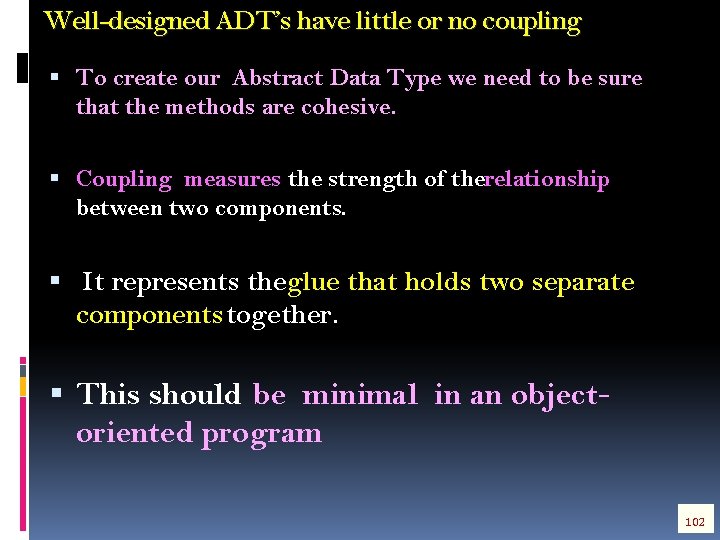
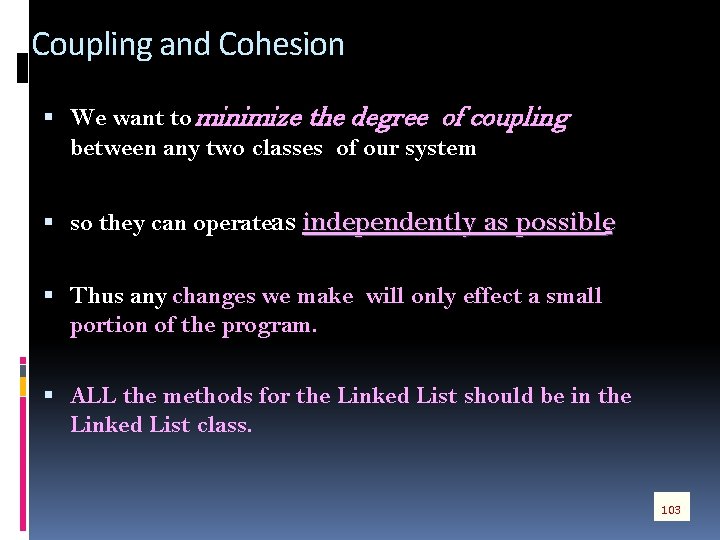
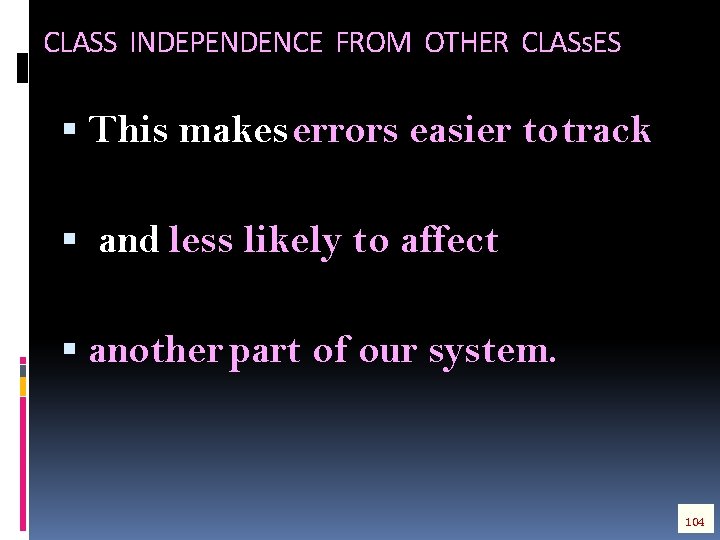
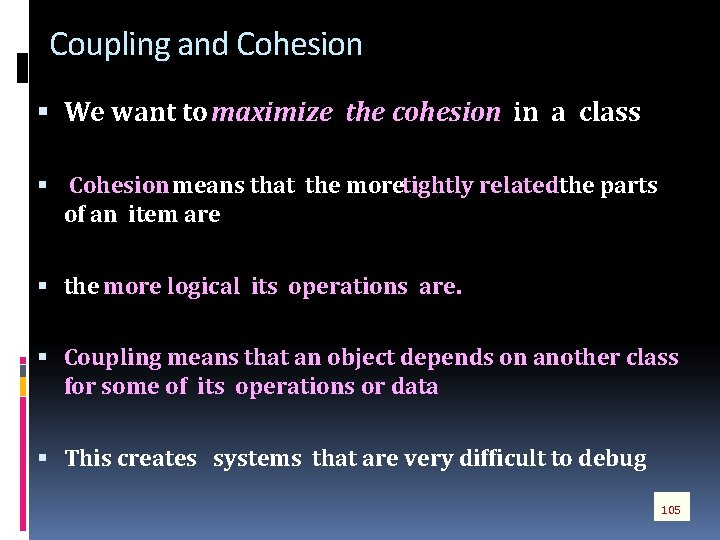
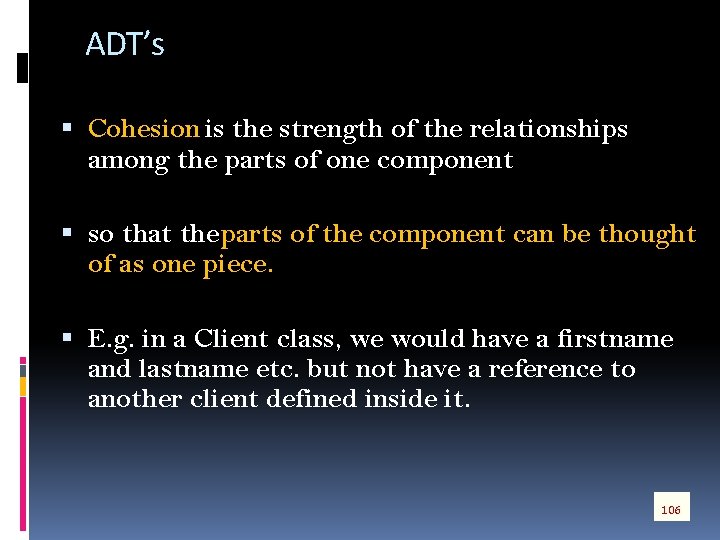
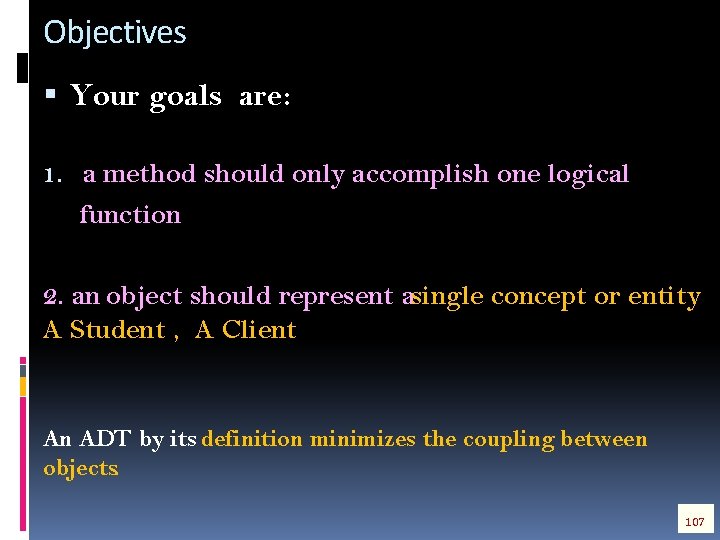
- Slides: 107
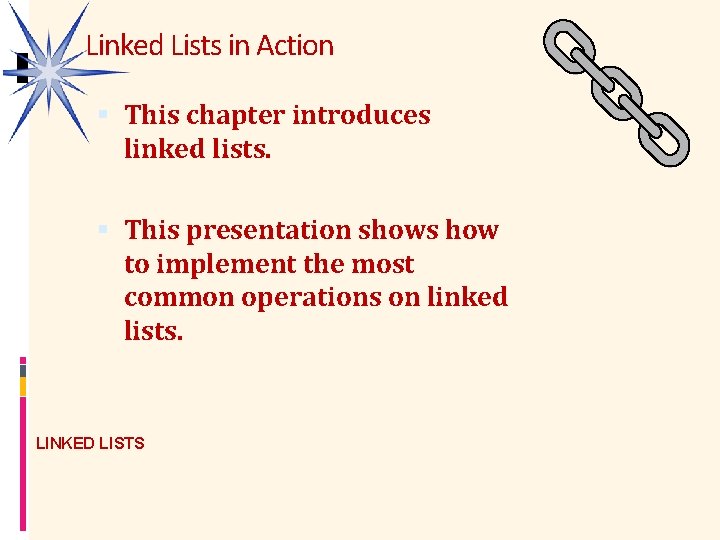
Linked Lists in Action This chapter introduces linked lists. This presentation shows how to implement the most common operations on linked lists. LINKED LISTS
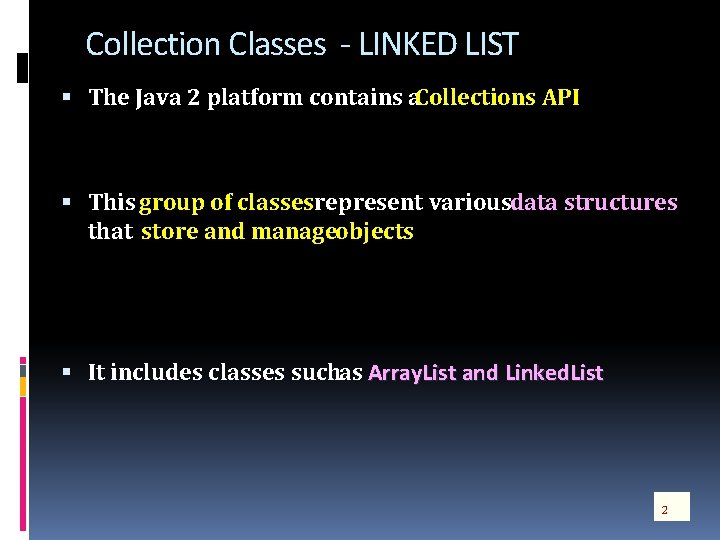
Collection Classes - LINKED LIST The Java 2 platform contains a Collections API This group of classes represent various data structures that store and manage objects It includes classes such as Array. List and Linked. List 2
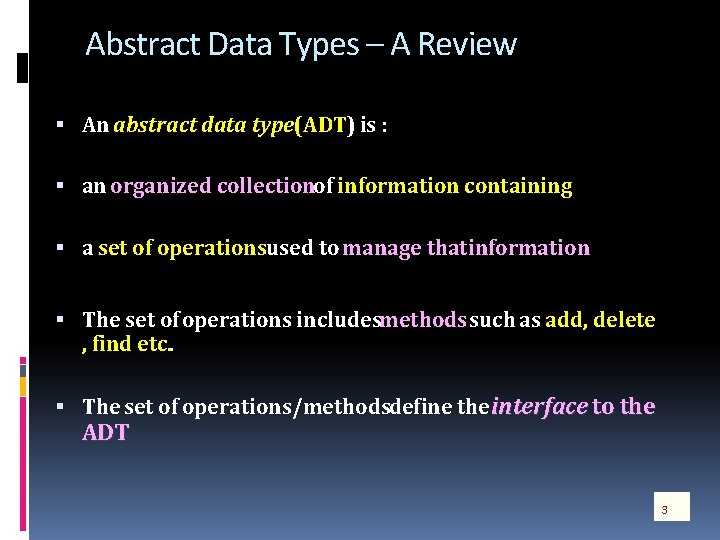
Abstract Data Types – A Review An abstract data type (ADT) is : an organized collection of information containing a set of operations used to manage that information The set of operations includes methods such as add, delete , find etc. . The set of operations/methods define the interface to the ADT 3
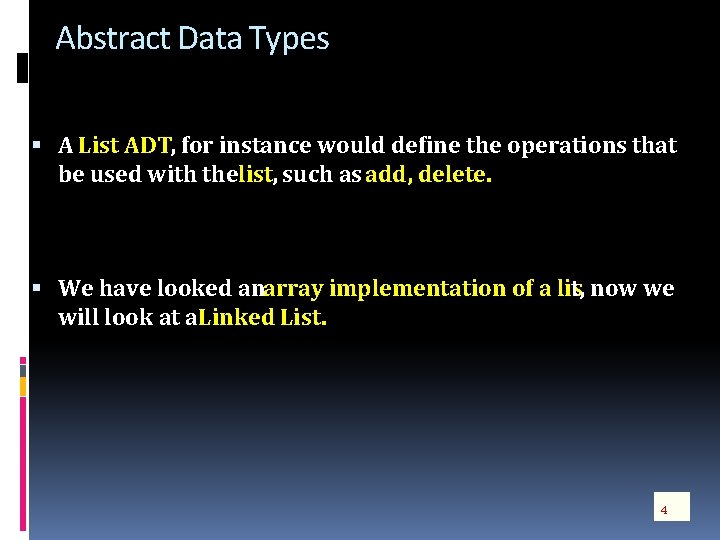
Abstract Data Types A List ADT, for instance would define the operations that be used with the list, such as add, delete. We have looked an array implementation of a lis t, now we will look at a Linked List. 4
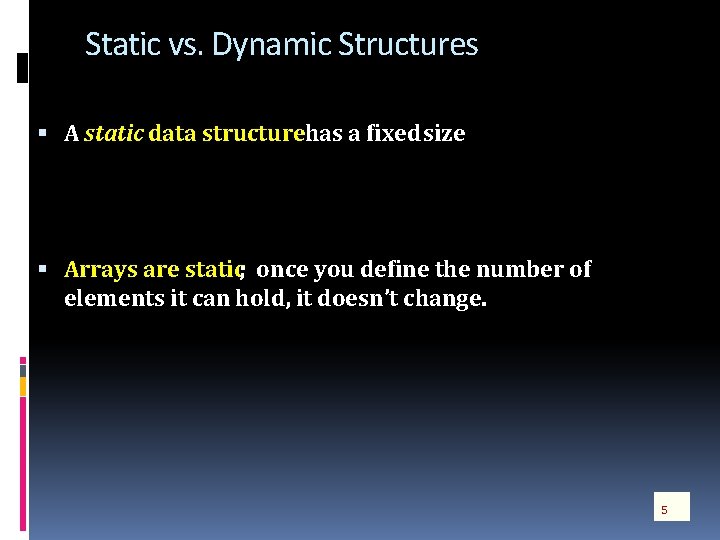
Static vs. Dynamic Structures A static data structure has a fixed size Arrays are static; once you define the number of elements it can hold, it doesn’t change. 5
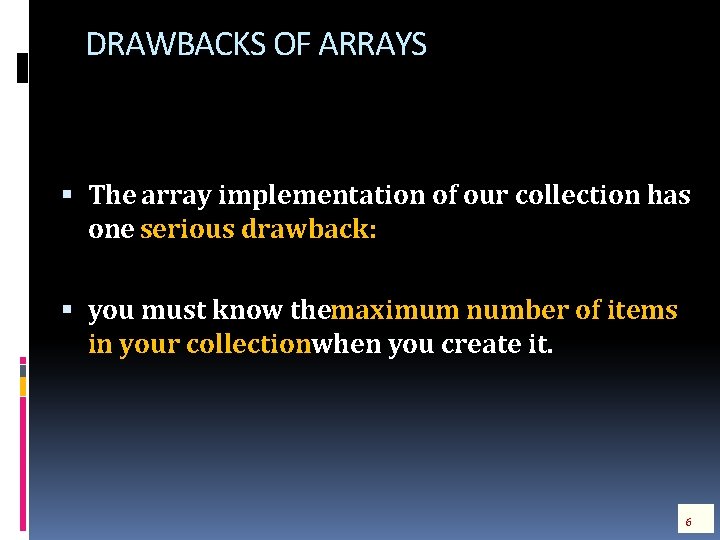
DRAWBACKS OF ARRAYS The array implementation of our collection has one serious drawback: you must know the maximum number of items in your collection when you create it. 6
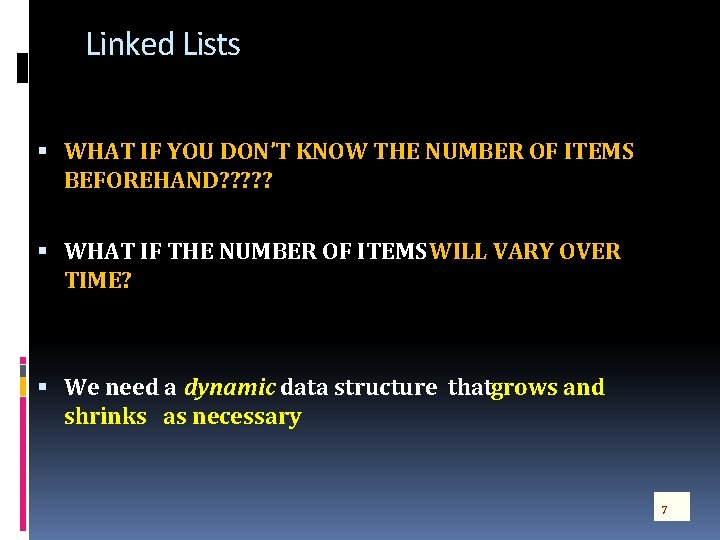
Linked Lists WHAT IF YOU DON’T KNOW THE NUMBER OF ITEMS BEFOREHAND? ? ? WHAT IF THE NUMBER OF ITEMS WILL VARY OVER TIME? We need a dynamic data structure that grows and shrinks as necessary 7
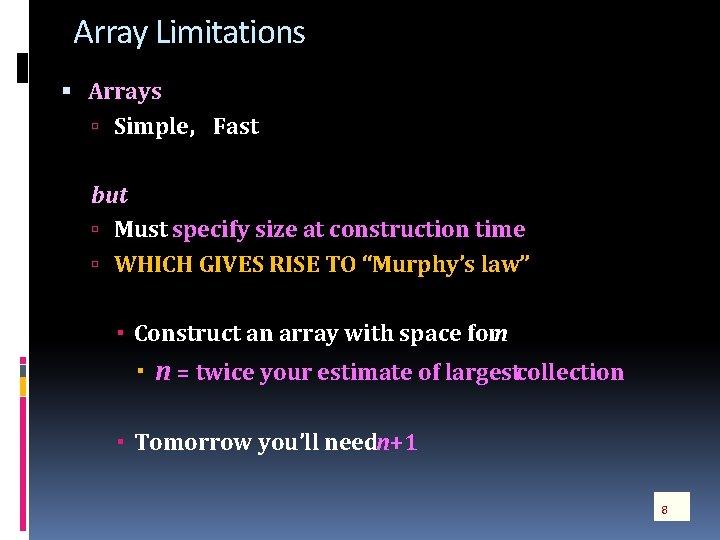
Array Limitations Arrays Simple, Fast but Must specify size at construction time WHICH GIVES RISE TO “Murphy’s law” Construct an array with space for n n = twice your estimate of largest collection Tomorrow you’ll need n+1 8
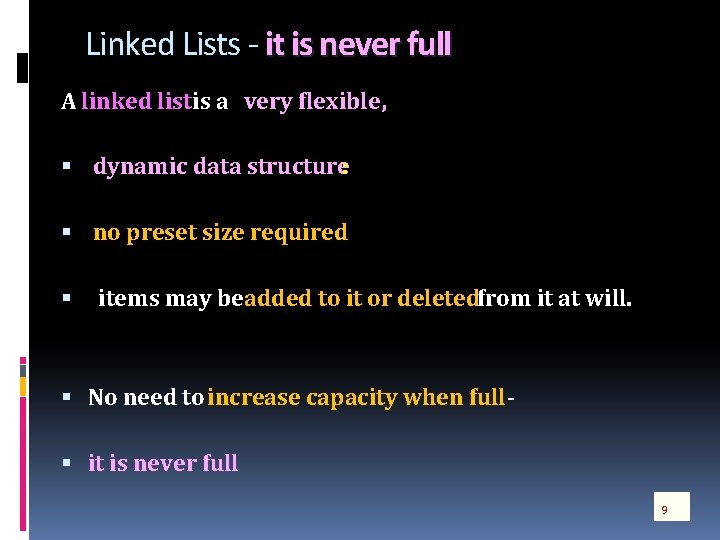
Linked Lists - it is never full A linked list is a very flexible, dynamic data structure: no preset size required items may be added to it or deleted from it at will. No need to increase capacity when full - it is never full 9
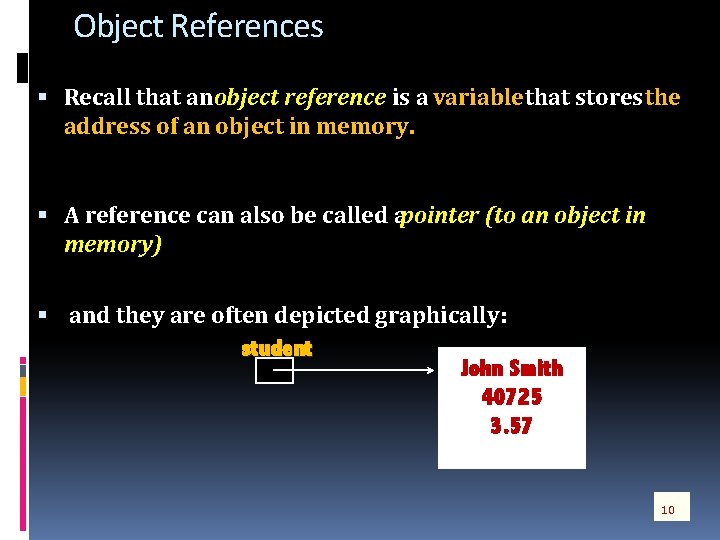
Object References Recall that an object reference is a variable that stores the address of an object in memory. A reference can also be called a pointer (to an object in memory) and they are often depicted graphically: student John Smith 40725 3. 57 10
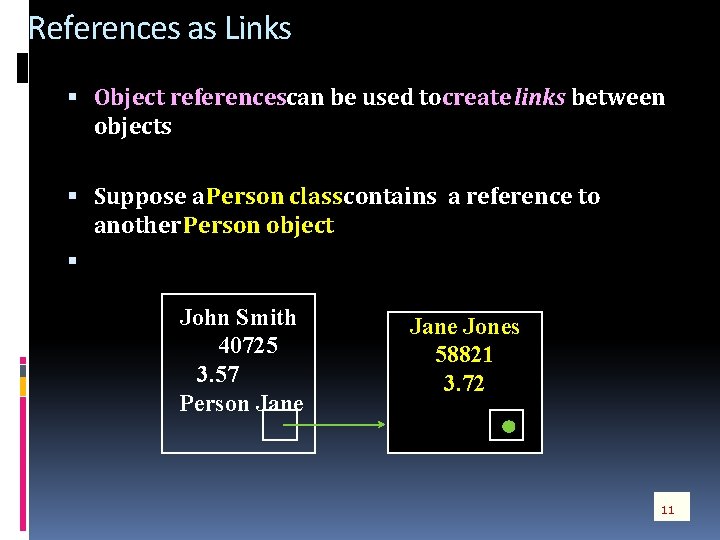
References as Links Object references can be used to create links between objects Suppose a Person class contains a reference to another Person object John Smith 40725 3. 57 Person Jane Jones 58821 3. 72 11
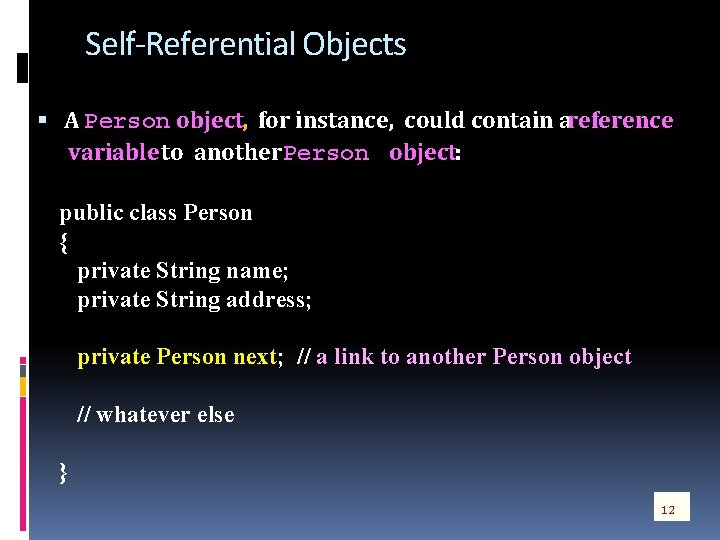
Self-Referential Objects A Person object, for instance, could contain a reference variable to another Person object: public class Person { private String name; private String address; private Person next; // a link to another Person object // whatever else } 12
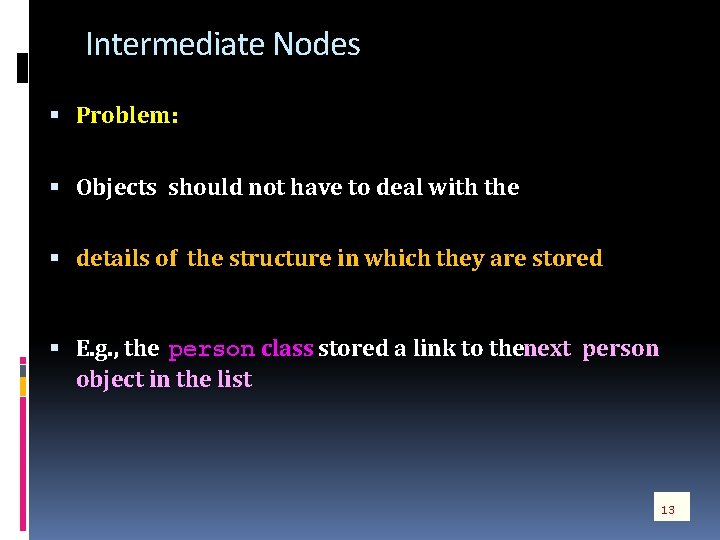
Intermediate Nodes Problem: Objects should not have to deal with the details of the structure in which they are stored E. g. , the person class stored a link to the next person object in the list 13
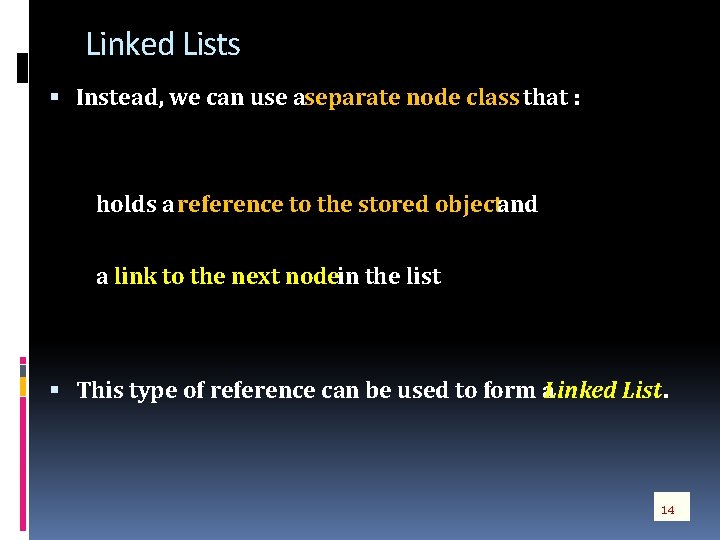
Linked Lists Instead, we can use a separate node class that : holds a reference to the stored object and a link to the next node in the list This type of reference can be used to form a Linked List. 14
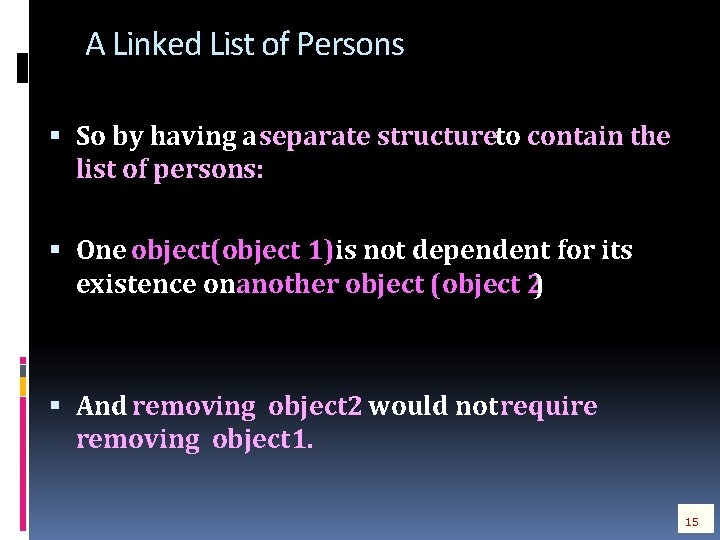
A Linked List of Persons So by having a separate structure to contain the list of persons: One object(object 1) is not dependent for its existence on another object (object 2) And removing object 2 would not require removing object 1. 15
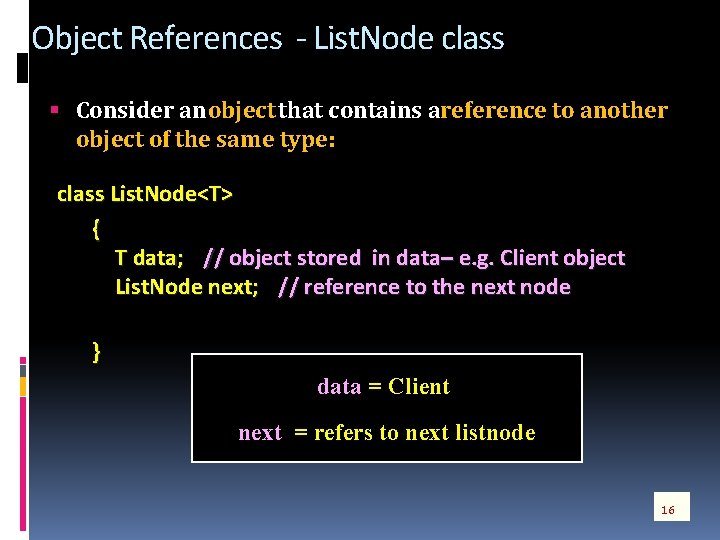
Object References - List. Node class Consider an object that contains a reference to another object of the same type: class List. Node<T> { T data; // object stored in data– e. g. Client object List. Node next; // reference to the next node } data = Client next = refers to next listnode 16
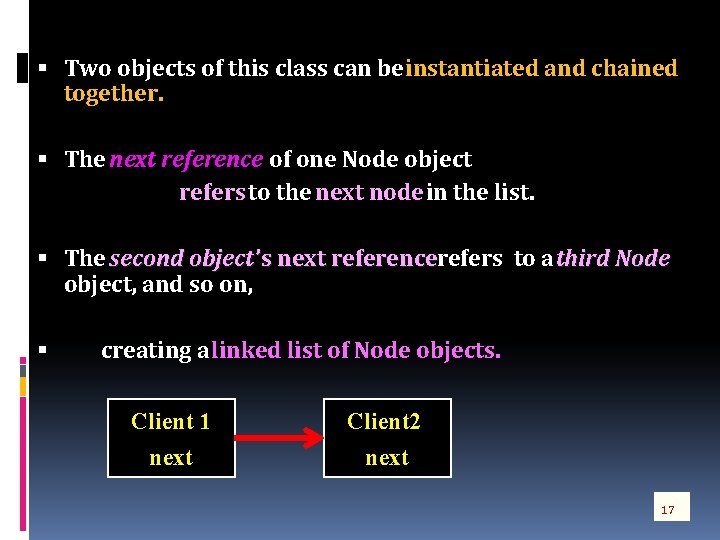
Two objects of this class can be instantiated and chained together. The next reference of one Node object refers to the next node in the list. The second object ’s next reference refers to a third Node object, and so on, creating a linked list of Node objects. Client 1 Client 2 next 17
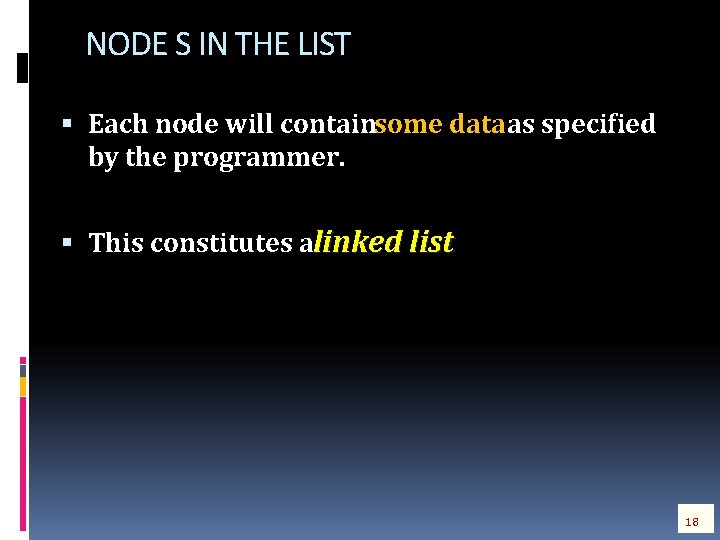
NODE S IN THE LIST Each node will contain some data as specified by the programmer. This constitutes a linked list 18
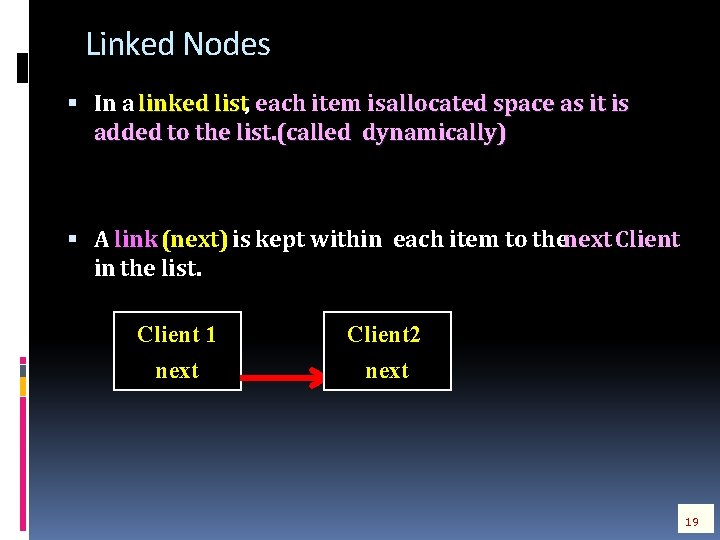
Linked Nodes In a linked list, each item is allocated space as it is linked list allocated space as it is added to the list. (called dynamically) A link (next) is kept within each item to the next Client in the list. Client 1 Client 2 next 19
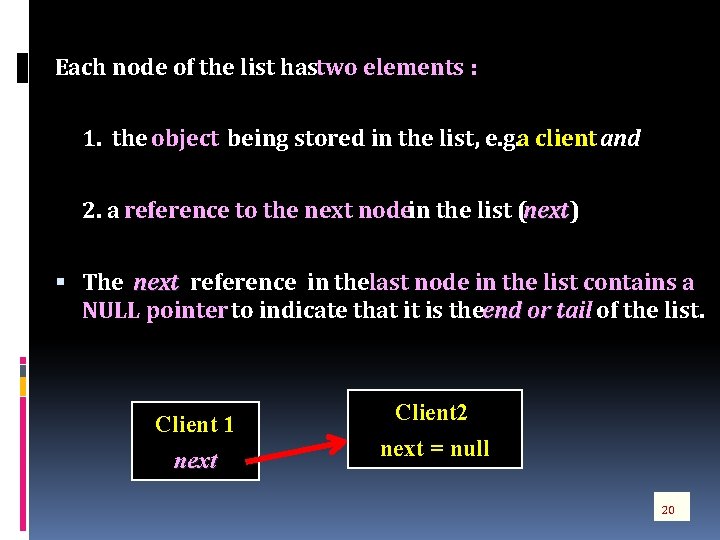
Each node of the list has two elements : 1. the object being stored in the list, e. g. a client and 2. a reference to the next node in the list (next) next The next reference in the last node in the list contains a next NULL pointer to indicate that it is the end or tail of the list. t Client 1 next Client 2 next = null 20
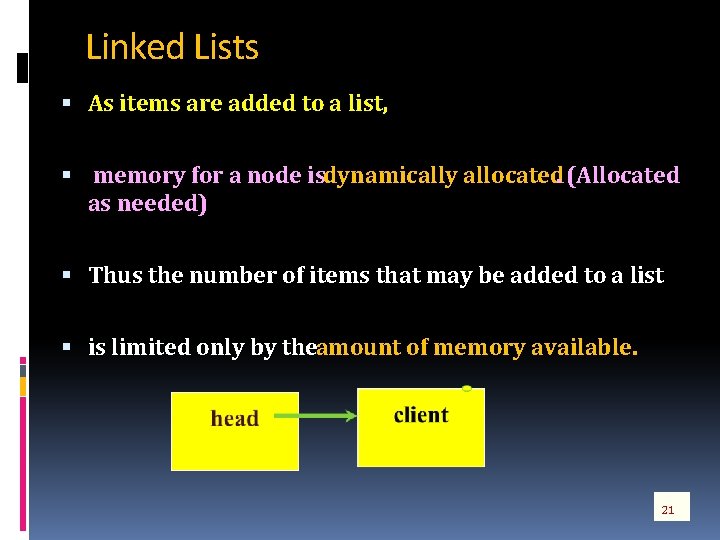
Linked Lists As items are added to a list, memory for a node is dynamically allocated. (Allocated as needed) Thus the number of items that may be added to a list is limited only by the amount of memory available. 21
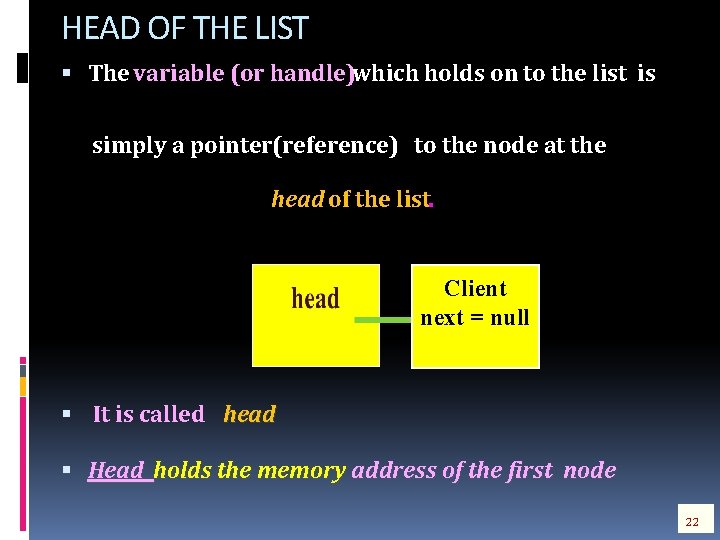
HEAD OF THE LIST The variable (or handle) which holds on to the list is simply a pointer(reference) to the node at the head of the list. Client next = null It is called head Head holds the memory address of the first node 22
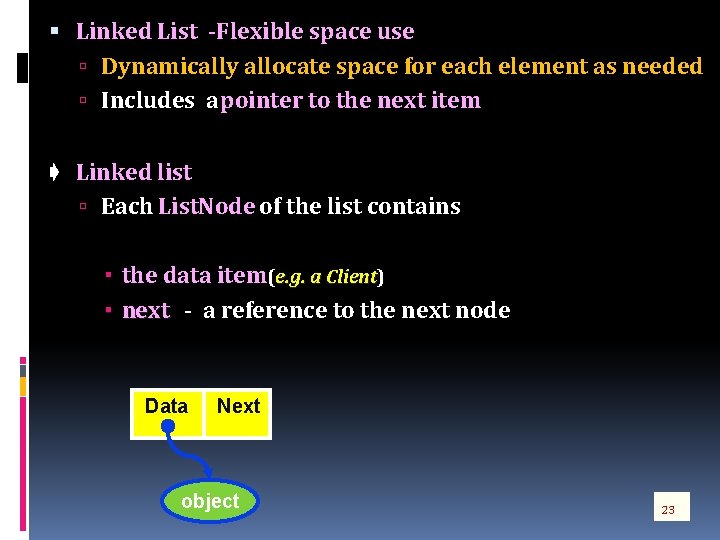
Linked List -Flexible space use Dynamically allocate space for each element as needed Includes a pointer to the next item ç Linked list Each List. Node of the list contains the data item (e. g. a Client) Client next - a reference to the next node ext Data Next object 23
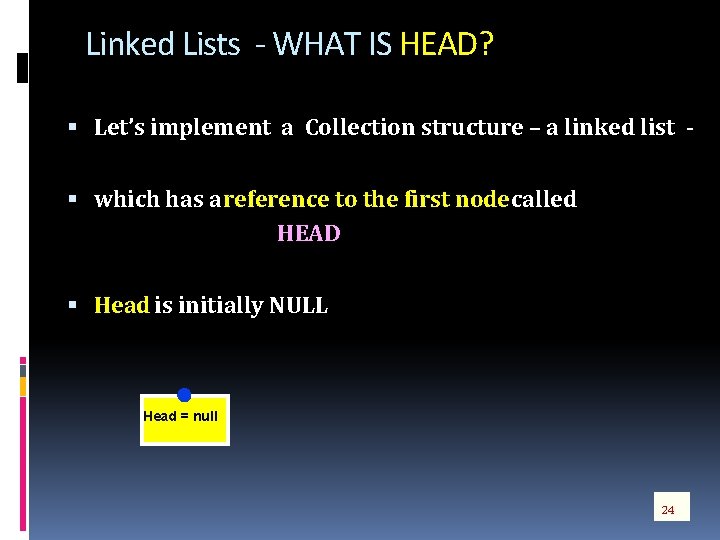
Linked Lists - WHAT IS HEAD? Let’s implement a Collection structure – a linked list which has a reference to the first node called HEAD Head is initially NULL Head = null 24
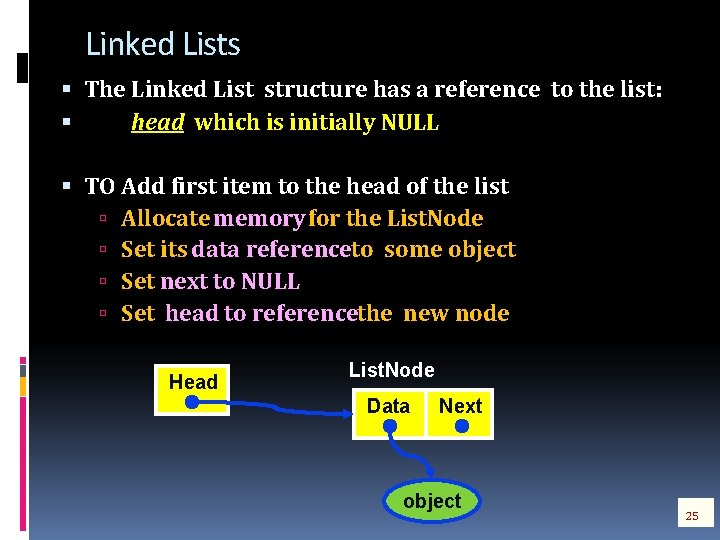
Linked Lists The Linked List structure has a reference to the list: head which is initially NULL TO Add first item to the head of the list Allocate memory for the List. Node Set its data reference to some object Set next to NULL Set head to reference the new node Head List. Node Data Next object 25
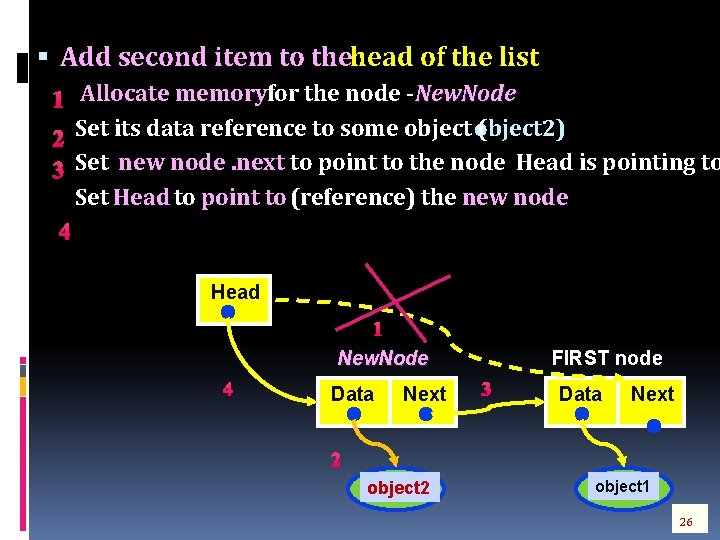
Add second item to the head of the list New. Node 1 Allocate memory for the node - New. Node object 2) 2 Set its data reference to some object ( 3 Set new node. next to point to the node Head is pointing to Set Head to point to (reference) the new node 4 Head 1 New. Node 4 Data Next FIRST node 3 Data Next 2 object 1 26
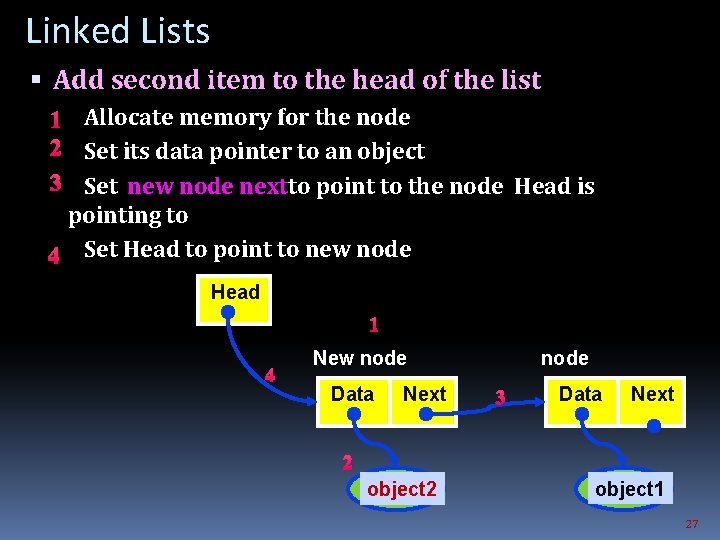
Linked Lists Add second item to the head of the list 1 Allocate memory for the node 2 Set its data pointer to an object 3 Set new node next to point to the node Head is pointing to 4 Set Head to point to new node Head 1 4 New node Data Next node 3 Data Next 2 object 1 27
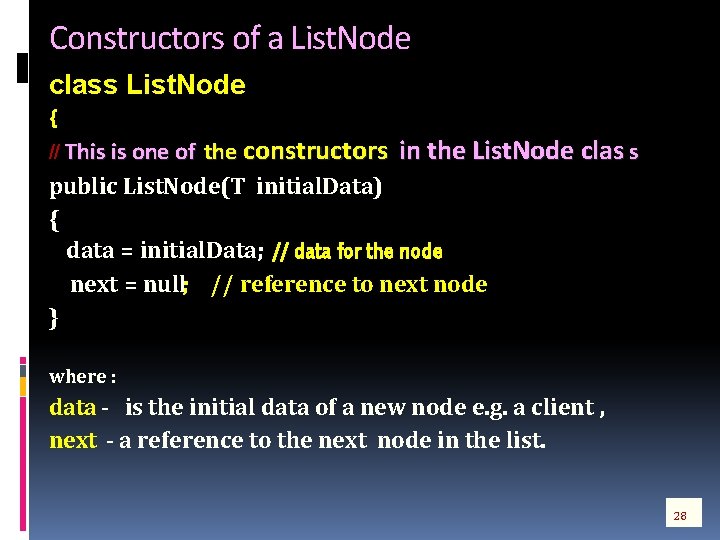
Constructors of a List. Node class List. Node {amine the constructor for the class. the constructors in the List. Node clas s public List. Node(T initial. Data) { data = initial. Data; // data for the node next = null; // reference to next node } // This is one of where : data - is the initial data of a new node e. g. a client , next - a reference to the next node in the list. 28
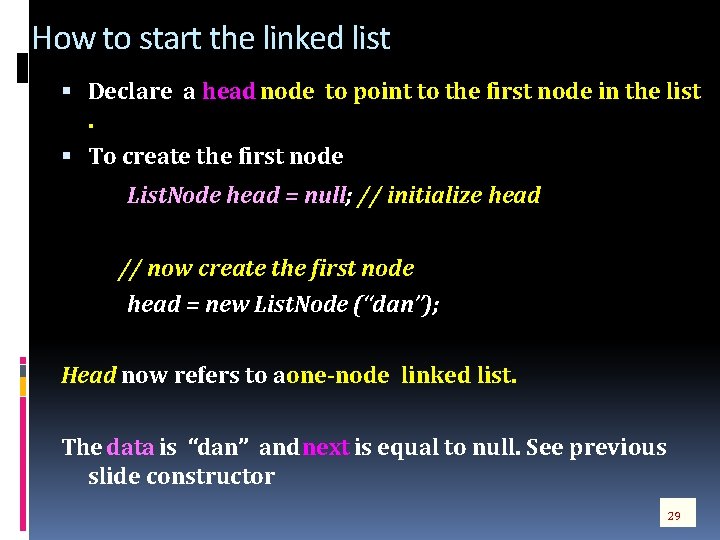
How to start the linked list Declare a head node to point to the first node in the list . To create the first node List. Node head = null; // initialize head // now create the first node head = new List. Node (“dan”); Head now refers to a one-node linked list. The data is “dan” and next is equal to null. See previous slide constructor 29
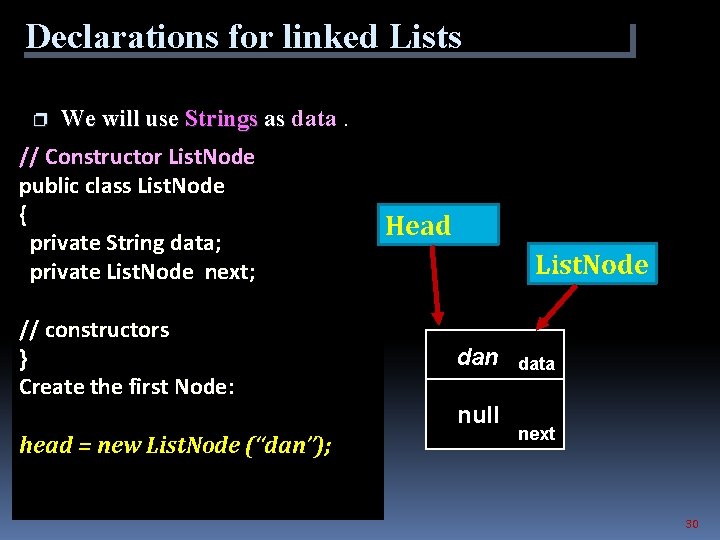
Declarations for linked Lists p We will use Strings as data. // Constructor List. Node public class List. Node { private String data; private List. Node next; // constructors } Create the first Node: Head List. Node dan null head = new List. Node (“dan”); data next 30
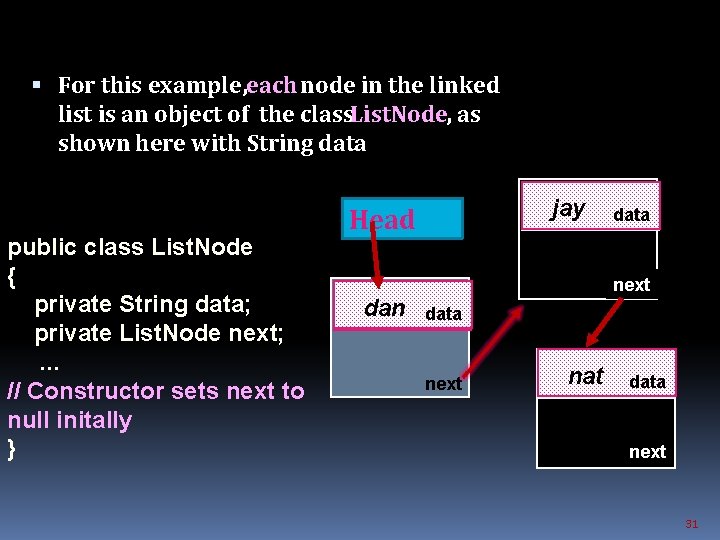
Declarations for linked Lists For this example, each node in the linked list is an object of the class List. Node, as shown here with String data public class List. Node { private String data; private List. Node next; . . . // Constructor sets next to null initally } jay Head dan data next nat data null next 31
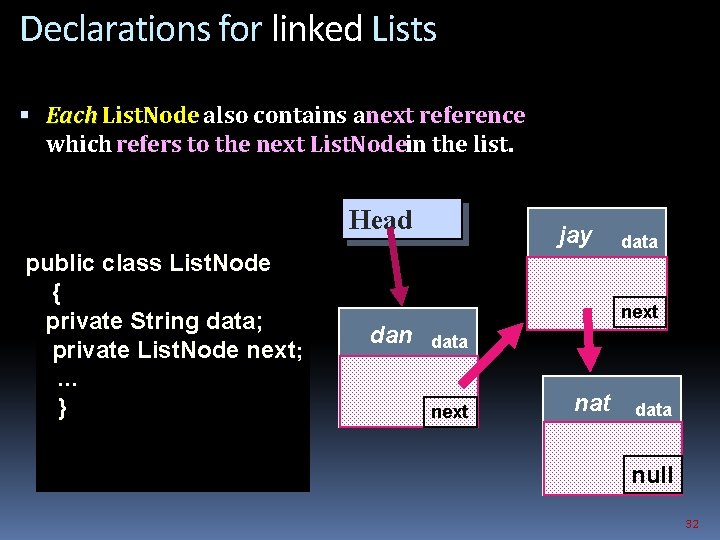
Declarations for linked Lists Each List. Node also contains a next reference which refers to the next List. Node in the list. Head public class List. Node { private String data; private List. Node next; . . . } dan jay data next nat data null 32
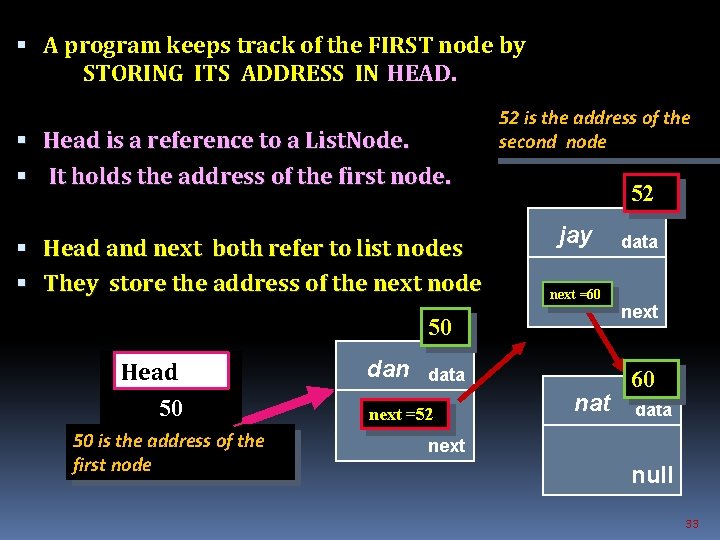
A program keeps track of the FIRST node by STORING ITS ADDRESS IN HEAD. Head is a reference to a List. Node. It holds the address of the first node. Head and next both refer to list nodes They store the address of the next node 52 is the address of the second node 52 jay next =60 50 Head head 50 50 is the address of the 50 first node dan data next =52 nat data next 60 data next null 33
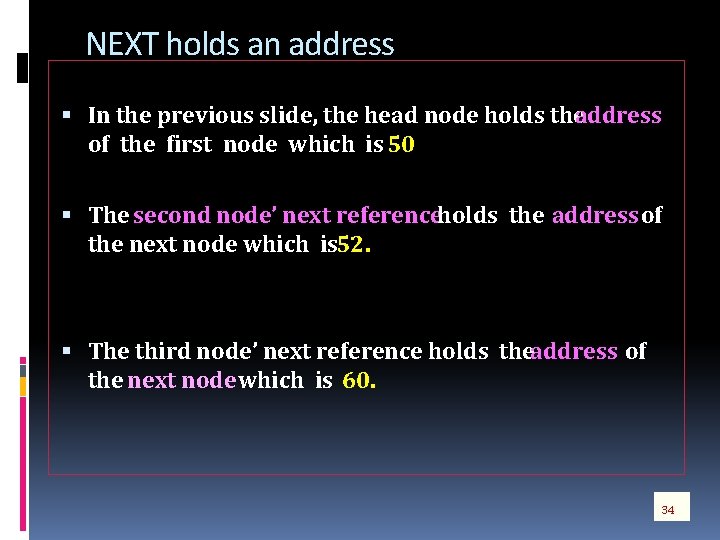
NEXT holds an address In the previous slide, the head node holds the address of the first node which is 50 The second node’ next reference holds the address of the next node which is 52. The third node’ next reference holds the address of the next node which is 60. 34
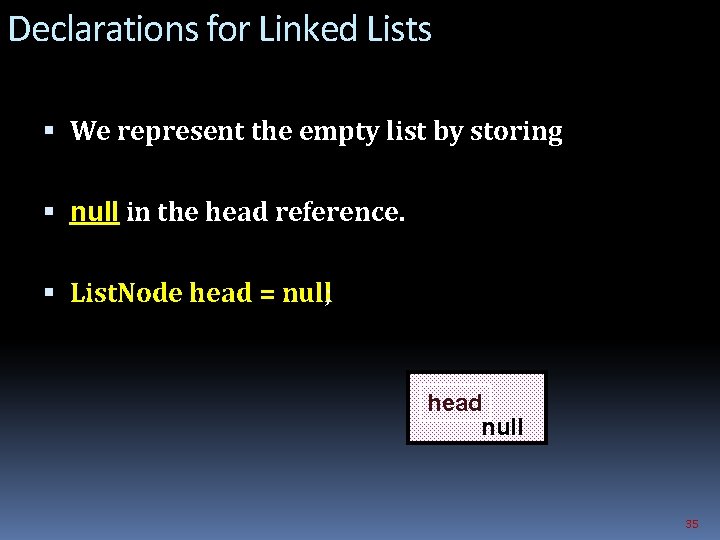
Declarations for Linked Lists We represent the empty list by storing null in the head reference. List. Node head = null; head null 35
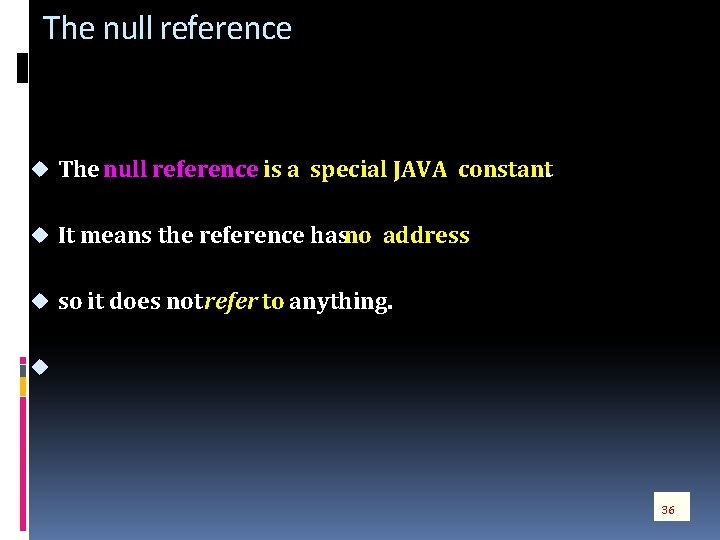
The null reference u The null reference is a special JAVA constant. u It means the reference has no address u so it does not refer to anything. u 36
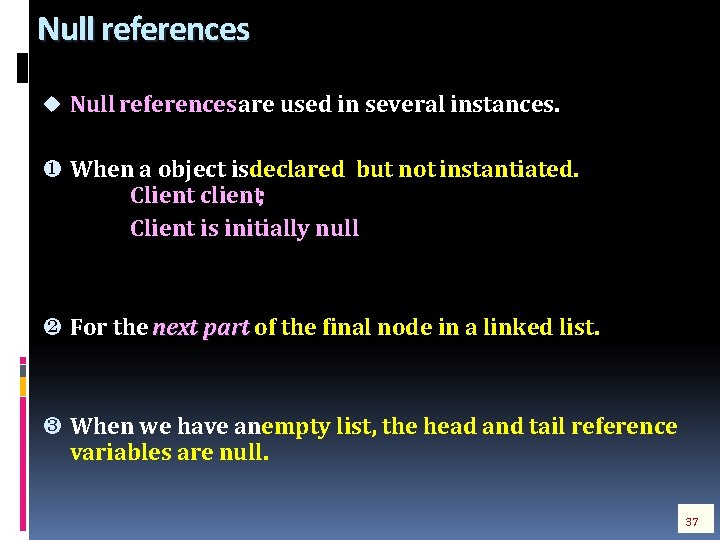
Null references u Null references are used in several instances. ¶ When a object is declared but not instantiated. Client client; Client is initially null · For the next part of the final node in a linked list. ¸ When we have an empty list, the head and tail reference variables are null. 37
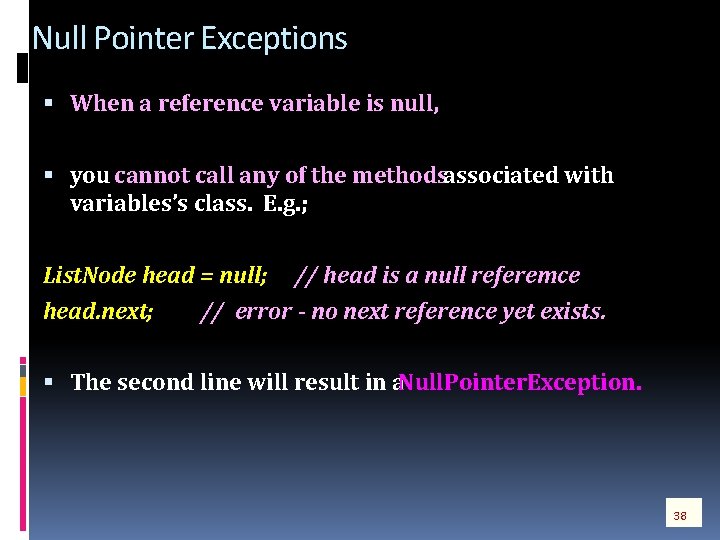
Null Pointer Exceptions When a reference variable is null, you cannot call any of the methods associated with variables’s class. E. g. ; List. Node head = null; // head is a null referemce head. next; // error - no next reference yet exists. The second line will result in a Null. Pointer. Exception. 38
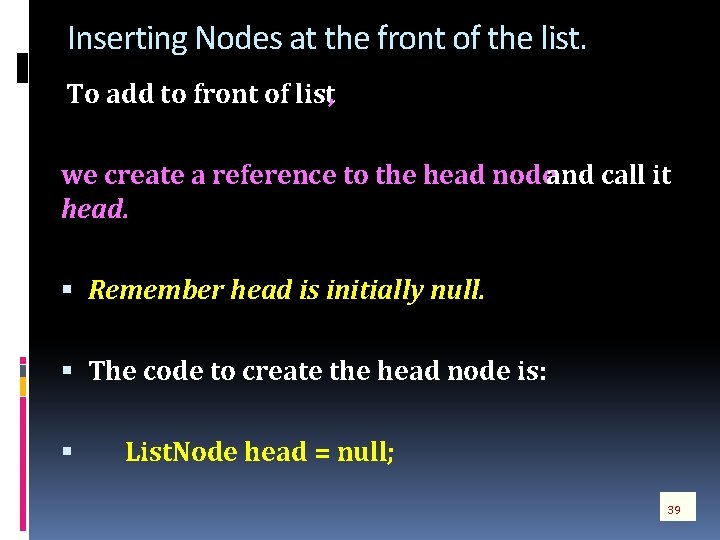
Inserting Nodes at the front of the list. To add to front of list, we create a reference to the head node and call it head. Remember head is initially null. The code to create the head node is: List. Node head = null; 39
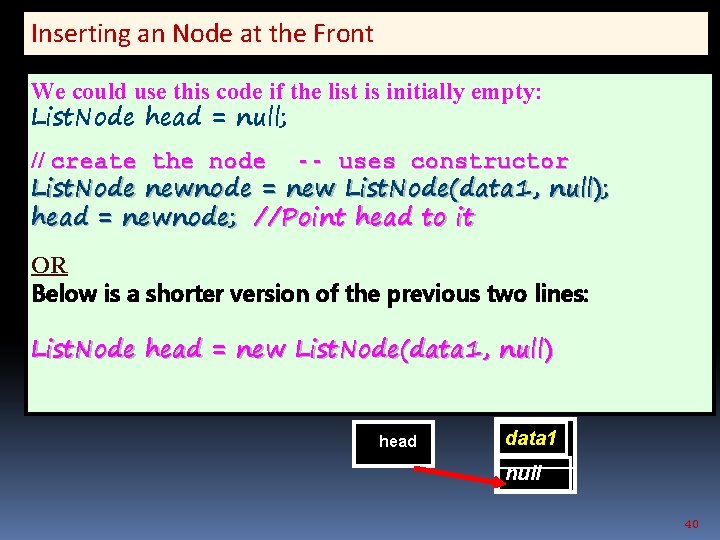
Inserting an Node at the Front We could use this code if the list is initially empty: List. Node head = null; // create the node -- uses constructor List. Node newnode = new List. Node(data 1, null); head = newnode; //Point head to it OR Below is a shorter version of the previous two lines: List. Node head = new List. Node(data 1, null) head data 1 null 40
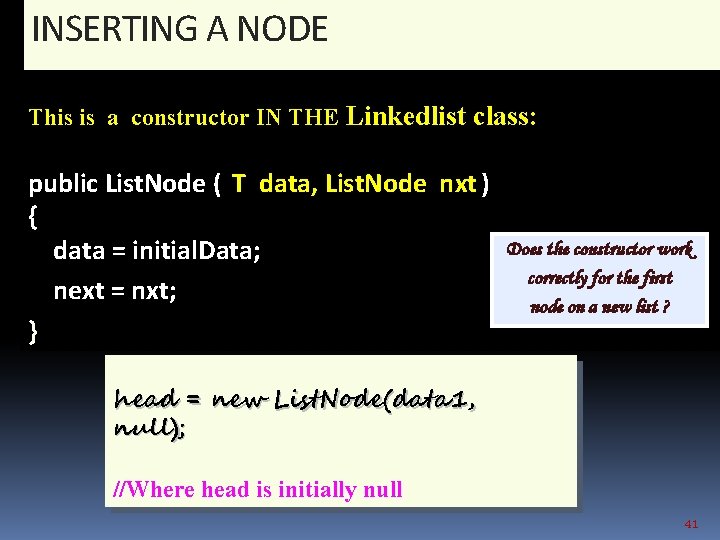
INSERTING A NODE This is a constructor IN THE Linkedlist class: public List. Node ( T data, List. Node nxt ) { data = initial. Data; next = nxt; } Does the constructor work correctly for the first node on a new list ? head = new List. Node(data 1, null); //Where head is initially null 41
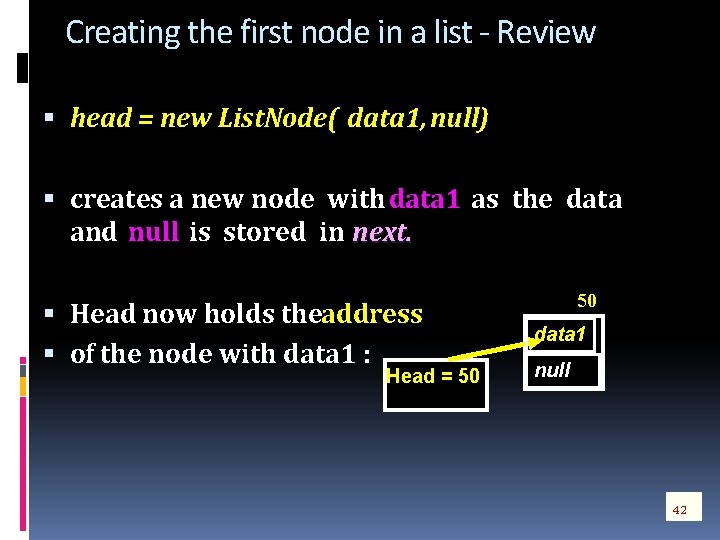
Creating the first node in a list - Review head = new List. Node( data 1, null) creates a new node with data 1 as the data and null is stored in next. Head now holds the address of the node with data 1 : Head = 50 50 data 1 null 42
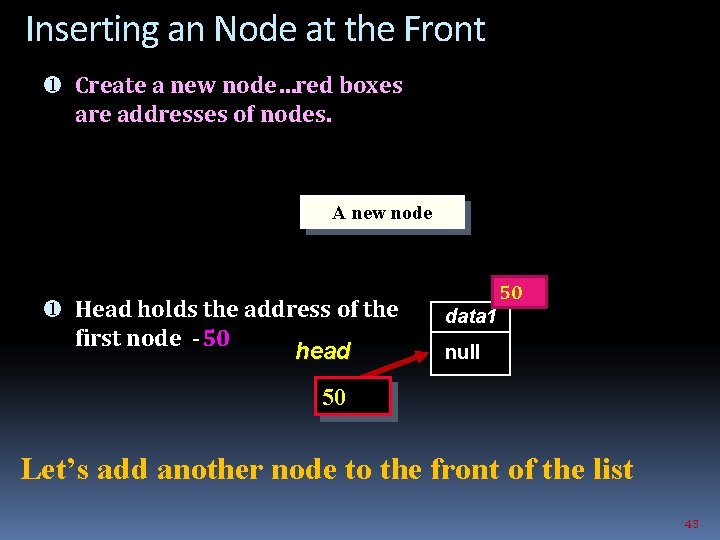
Inserting an Node at the Front ¶ Create a new node…red boxes are addresses of nodes. A new node ¶ Head holds the address of the first node - 50 head 50 data 1 null 50 Let’s add another node to the front of the list 43
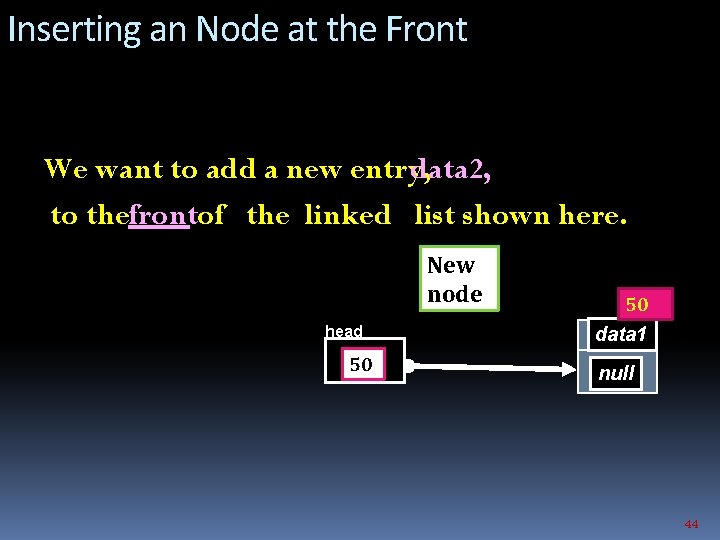
Inserting an Node at the Front We want to add a new entry, data 2, to thefrontof the linked list shown here. New node head 50 50 data 1 null 44
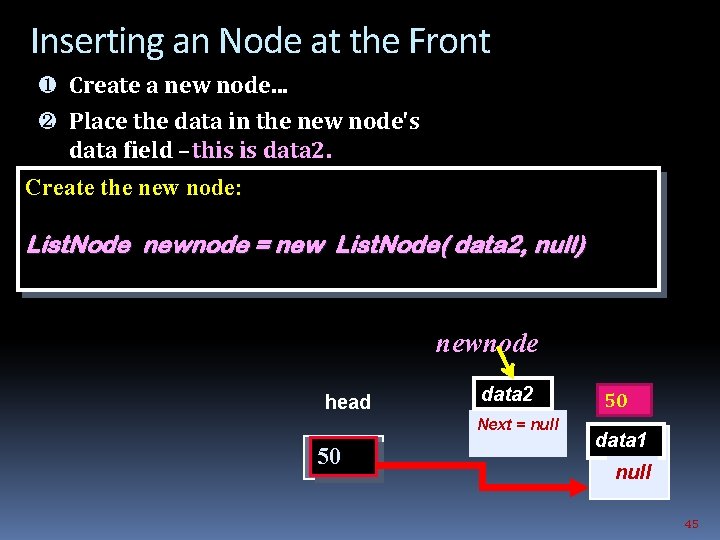
Inserting an Node at the Front ¶ Create a new node. . . · Place the data in the new node's data field – this is data 2. Create the new node: List. Node newnode = new List. Node( data 2, null) newnode head data 2 Next = null 50 50 data 1 null 45
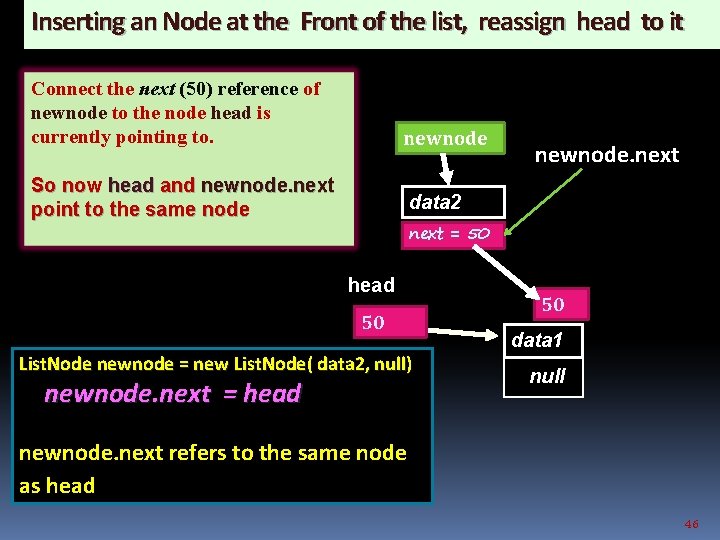
Inserting an Node at the Front of the list, reassign head to it Connect the next (50) reference of newnode to the node head is currently pointing to. newnode So now head and newnode. next point to the same node data 2 newnode. next = 50 head 50 List. Node newnode = new List. Node( data 2, null) newnode. next = head 50 data 1 null newnode. next refers to the same node as head 46
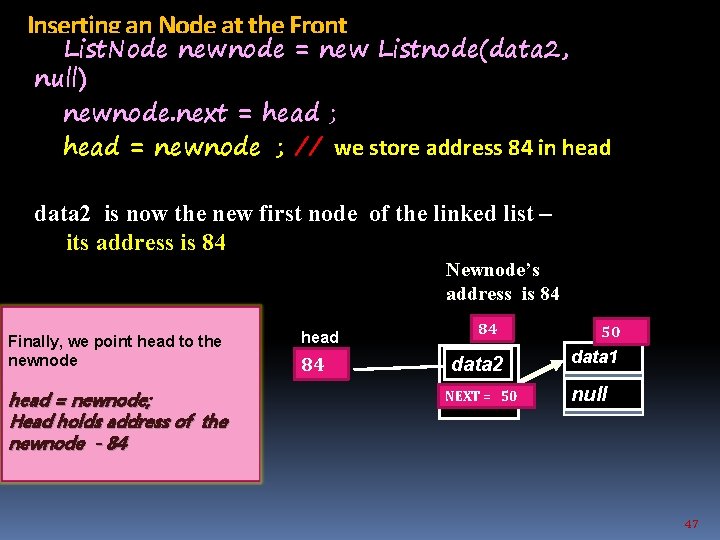
Inserting an Node at the Front List. Node newnode = new Listnode(data 2, null) newnode. next = head ; head = newnode ; // we store address 84 in head data 2 is now the new first node of the linked list – its address is 84 Newnode’s address is 84 Finally, we point head to the newnode head = newnode; Head holds address of the newnode - 84 head 84 84 data 2 NEXT = 50 50 data 1 null 47
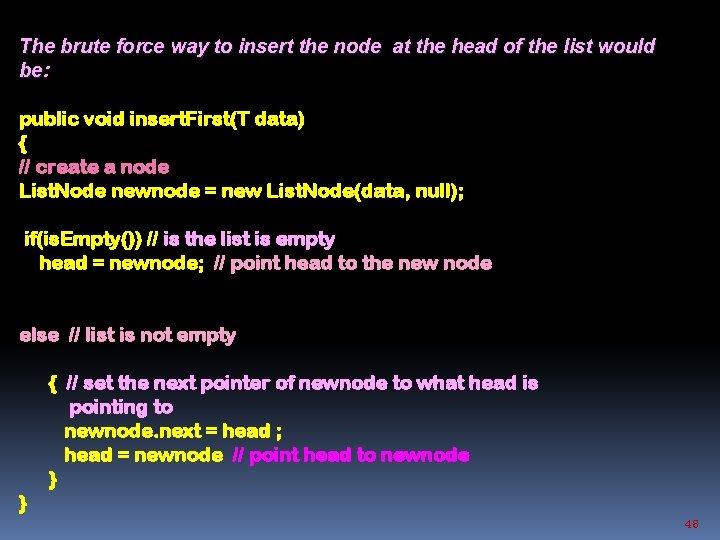
The brute force way to insert the node at the head of the list would be: public void insert. First(T data) { // create a node List. Node newnode = new List. Node(data, null); if(is. Empty()) // is the list is empty head = newnode; // point head to the new node else // list is not empty { // set the next pointer of newnode to what head is pointing to newnode. next = head ; head = newnode // point head to newnode } } 48
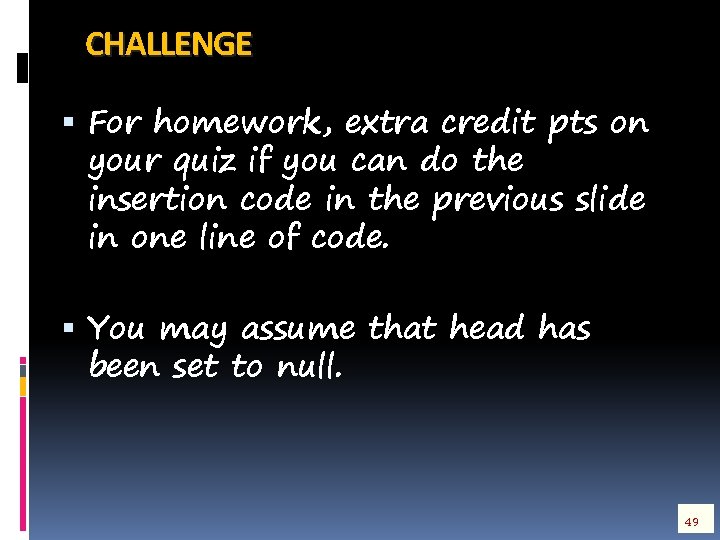
CHALLENGE For homework, extra credit pts on your quiz if you can do the insertion code in the previous slide in one line of code. You may assume that head has been set to null. 49
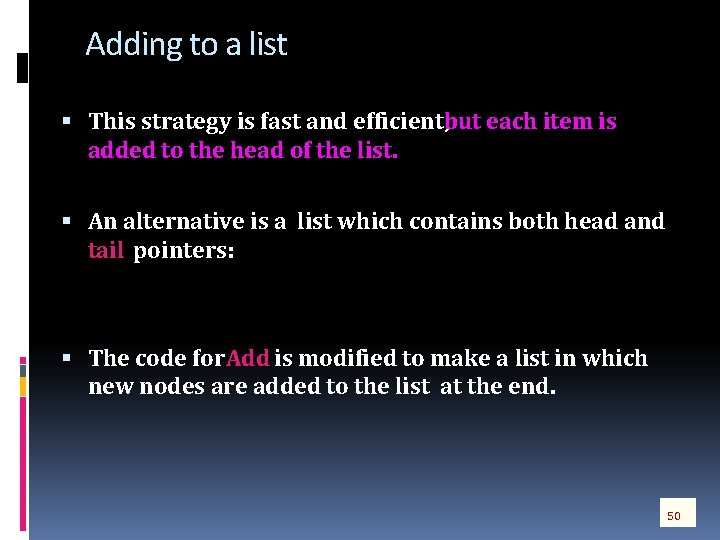
Adding to a list This strategy is fast and efficient, but each item is added to the head of the list. An alternative is a list which contains both head and tail pointers: The code for Add is modified to make a list in which new nodes are added to the list at the end. 50
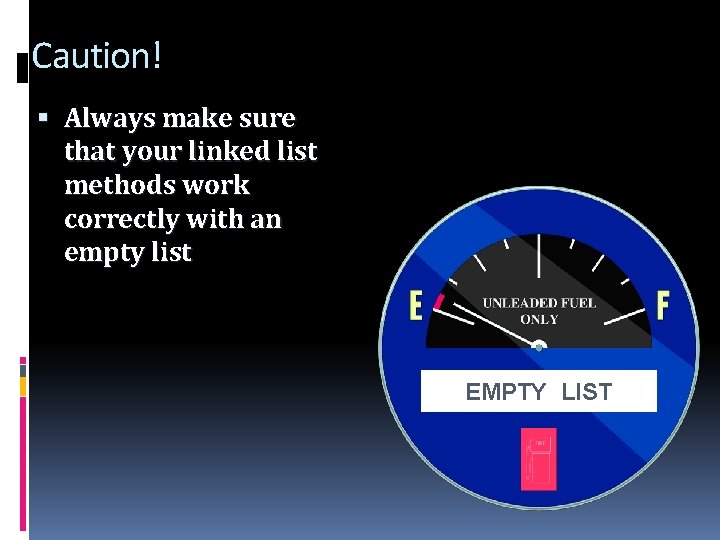
Caution! Always make sure that your linked list methods work correctly with an empty list. EMPTY LIST
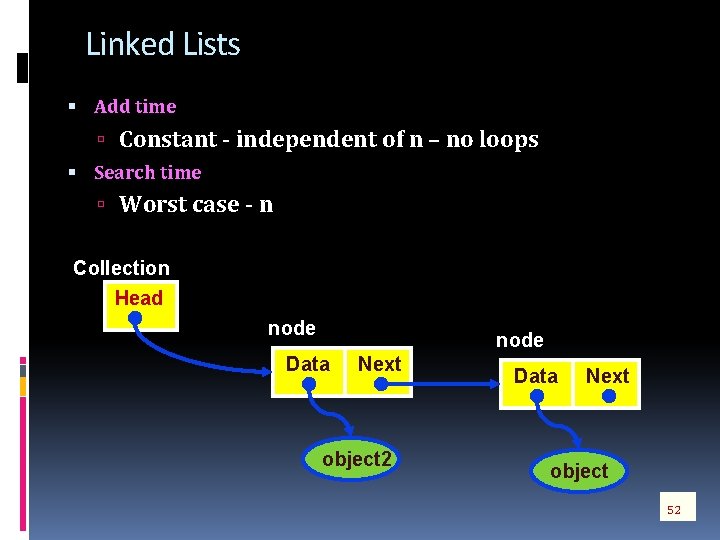
Linked Lists Add time Constant - independent of n – no loops Search time Worst case - n Collection Head node Data Next object 2 Data Next object 52
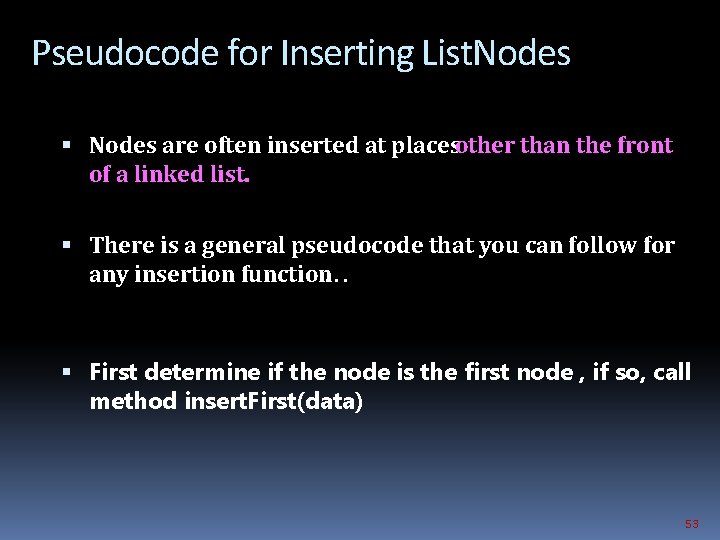
Pseudocode for Inserting List. Nodes are often inserted at places other than the front of a linked list. There is a general pseudocode that you can follow for any insertion function. . . First determine if the node is the first node , if so, call method insert. First(data) 53
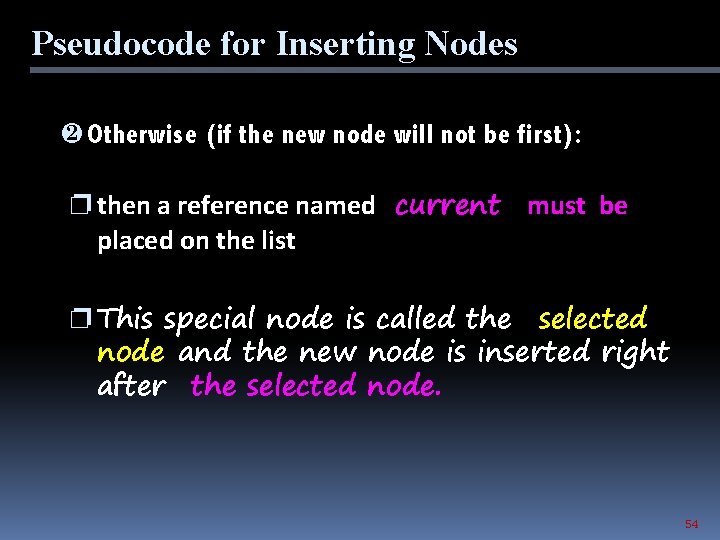
Pseudocode for Inserting Nodes · Otherwise (if the new node will not be first): p then a reference named current must be placed on the list p This special node is called the selected node and the new node is inserted right after the selected node. 54
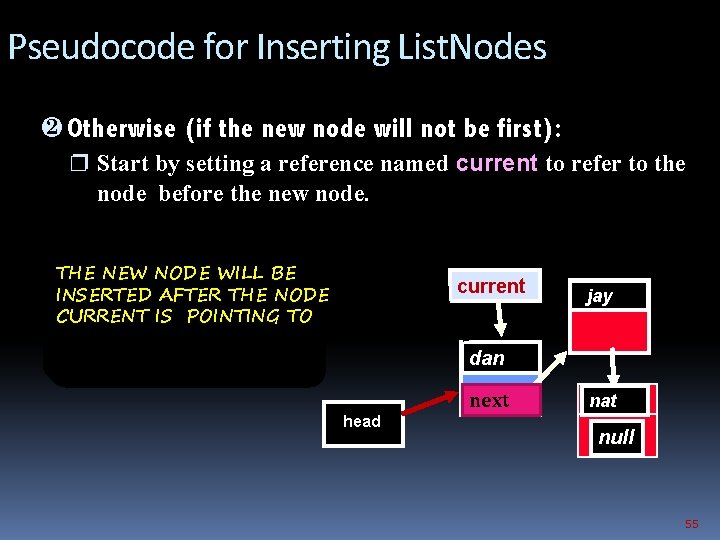
Pseudocode for Inserting List. Nodes · Otherwise (if the new node will not be first): p Start by setting a reference named current to refer to the node before the new node. THE NEW NODE WILL BE INSERTED AFTER THE NODE CURRENT IS POINTING TO current jay dan next head nat null 55
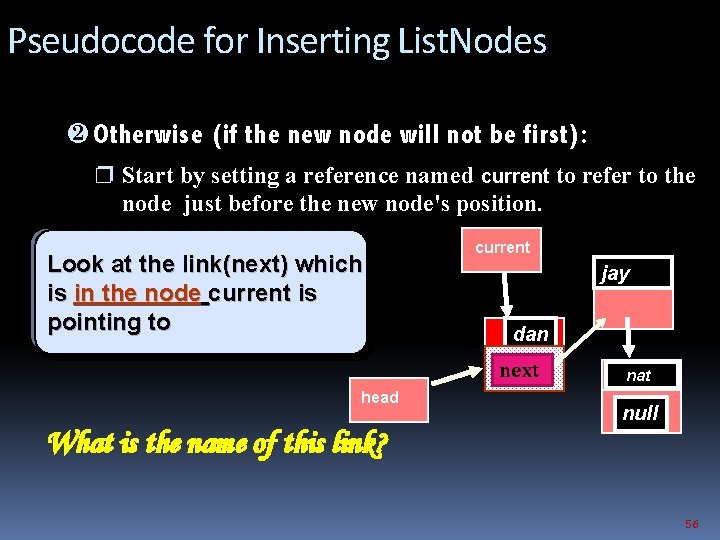
Pseudocode for Inserting List. Nodes · Otherwise (if the new node will not be first): p Start by setting a reference named current to refer to the node just before the new node's position. atlink(next) the link which Look at the in the node is is which in the is node current pointingprevious to current jay dan next head nat null What is the name of this link? 56
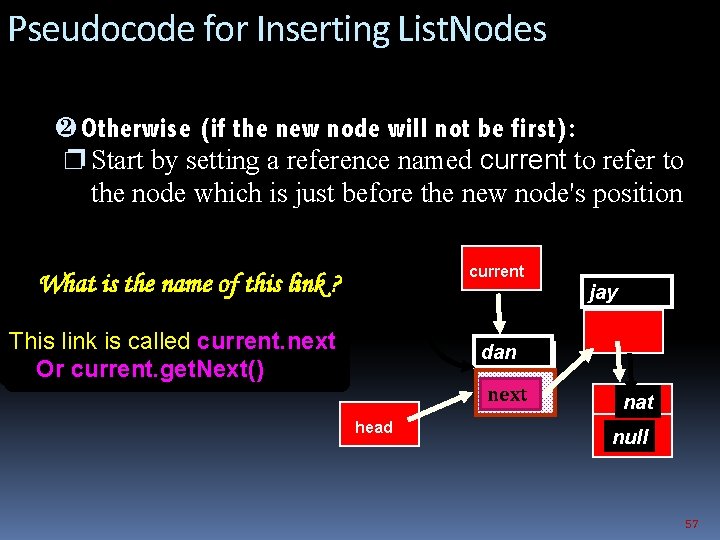
Pseudocode for Inserting List. Nodes · Otherwise (if the new node will not be first): p Start by setting a reference named current to refer to the node which is just before the new node's position current What is the name of this link ? jay This link is called current. next Or current. get. Next() dan next head nat null 57
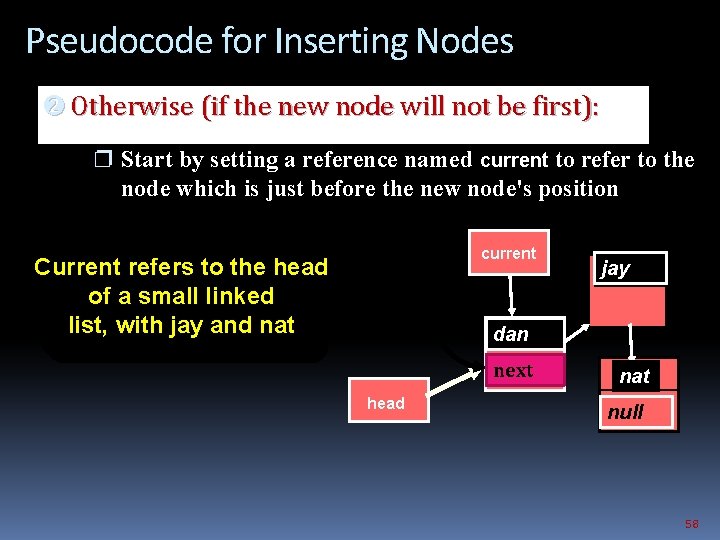
Pseudocode for Inserting Nodes Ë Otherwise (if the new node will not be first): p Start by setting a reference named current to refer to the node which is just before the new node's position current Current refers to the head of a small linked list, with jay and nat jay dan next head nat null 58
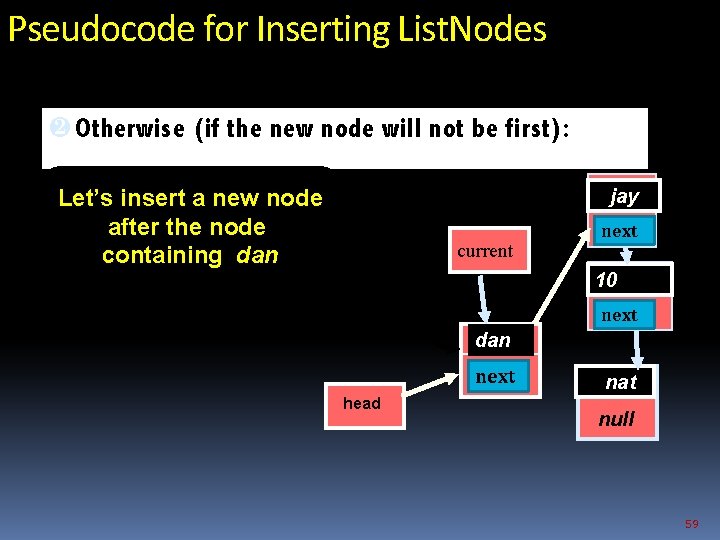
Pseudocode for Inserting List. Nodes · Otherwise (if the new node will not be first): Let’s insert a new node after the node containing dan jay current next 10 next dan next head nat null 59
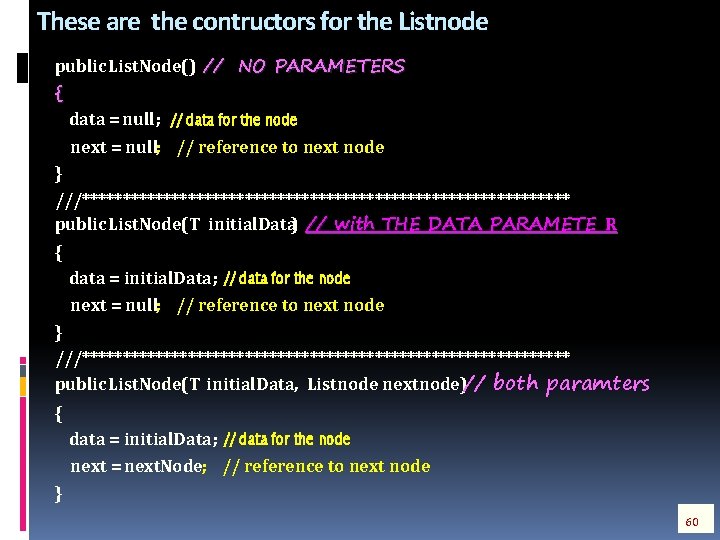
These are the contructors for the Listnode public List. Node() // NO PARAMETERS { data = null; // data for the node next = null; // reference to next node } ///****************************** public List. Node(T initial. Data) // with THE DATA PARAMETE R { data = initial. Data; // data for the node next = null; // reference to next node } ///****************************** public List. Node(T initial. Data, Listnode nextnode) // both paramters { data = initial. Data; // data for the node next = next. Node; // reference to next node } 60
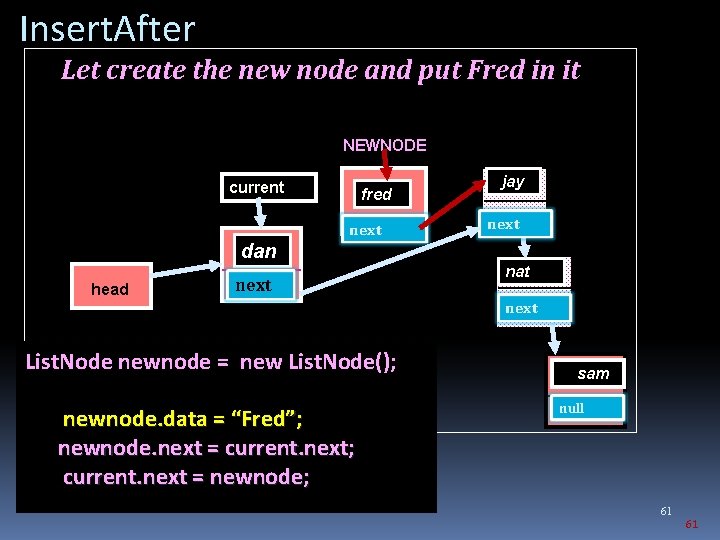
Insert. After Let create the new node and put Fred in it NEWNODE current fred next jay next dan head next List. Node newnode = new List. Node(); newnode. data = “Fred”; newnode. next = current. next; current. next = newnode; nat next sam null 61 61
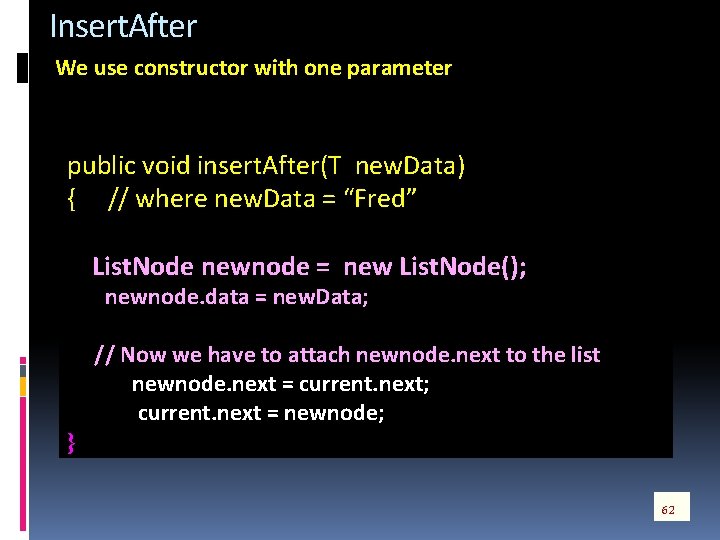
Insert. After We use constructor with one parameter public void insert. After(T new. Data) { // where new. Data = “Fred” List. Node newnode = new List. Node(); newnode. data = new. Data; // Now we have to attach newnode. next to the list newnode. next = current. next; current. next = newnode; } 62
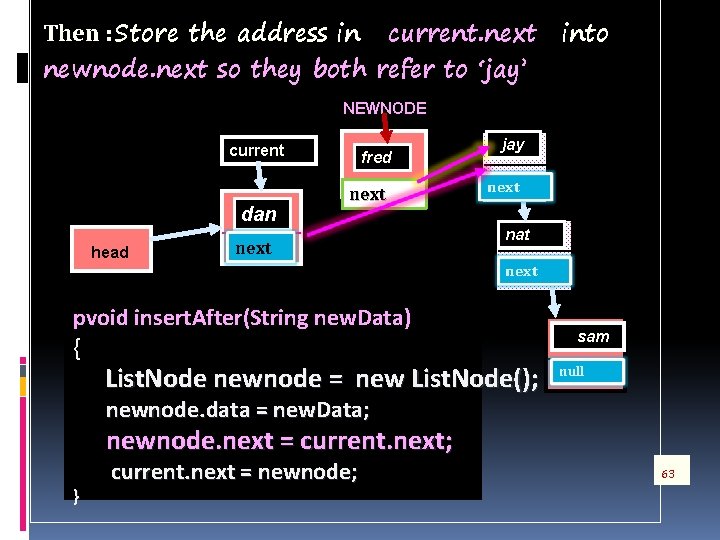
Then : Store the address in current. next newnode. next so they both refer to ‘jay’ into NEWNODE current dan head fred next pvoid insert. After(String new. Data) { jay next nat next List. Node newnode = new List. Node(); sam null newnode. data = new. Data; newnode. next = current. next; } current. next = newnode; 63
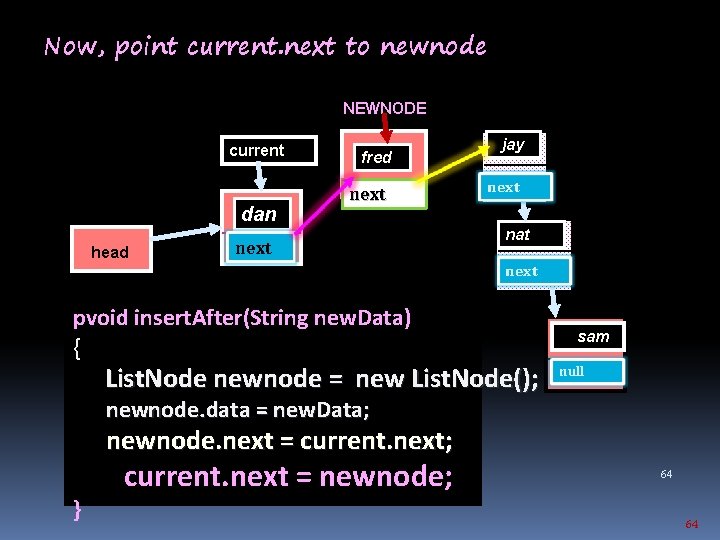
Now, point current. next to newnode NEWNODE current dan head fred next pvoid insert. After(String new. Data) { jay next nat next List. Node newnode = new List. Node(); sam null newnode. data = new. Data; newnode. next = current. next; } current. next = newnode; 64 64
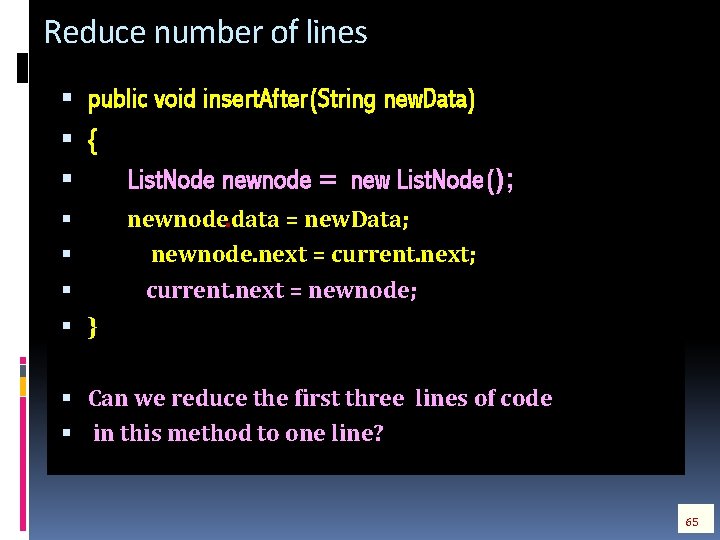
Reduce number of lines public void insert. After(String new. Data) { List. Node newnode = new List. Node(); newnode. data = new. Data; newnode. next = current. next; current. next = newnode; } Can we reduce the first three lines of code in this method to one line? 65
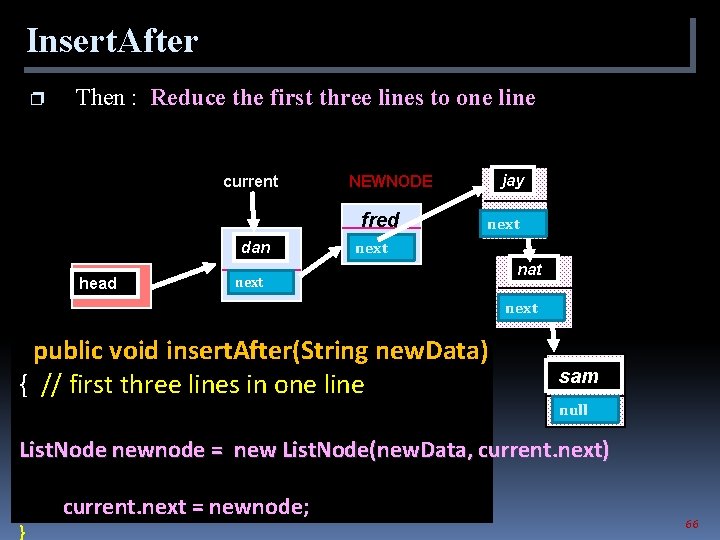
Insert. After p Then : Reduce the first three lines to one line current dan head jay NEWNODE fred next nat next 20 public void insert. After(String new. Data) { // first three lines in one line sam null List. Node newnode = new List. Node(new. Data, current. next) } current. next = newnode; 66
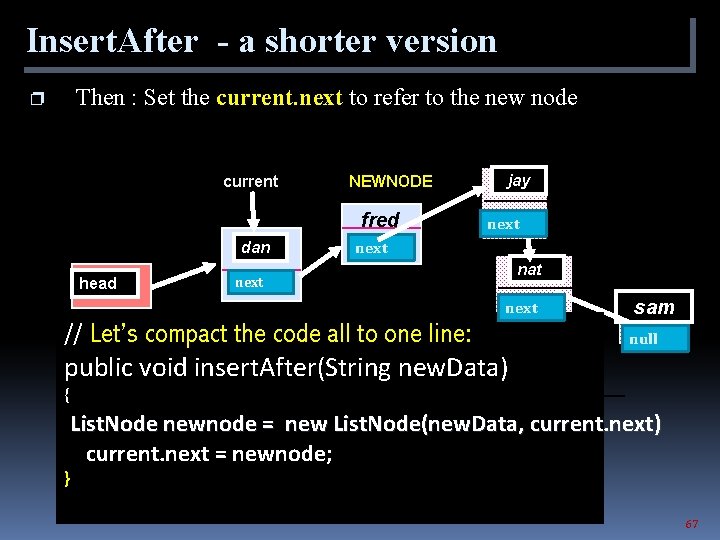
Insert. After - a shorter version Then : Set the current. next to refer to the new node p current dan head NEWNODE fred jay next nat next // Let’s compact the code all to one line: public void insert. After(String new. Data) sam null { } List. Node newnode = new List. Node(new. Data, current. next) current. next = newnode; 67
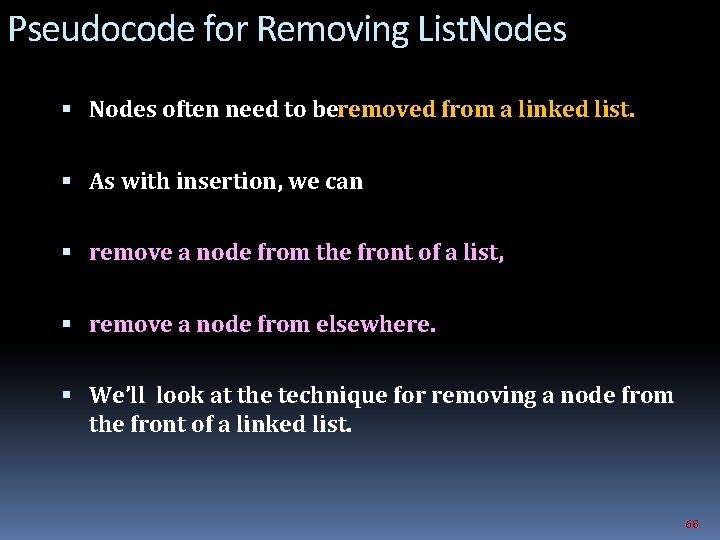
Pseudocode for Removing List. Nodes often need to be removed from a linked list. As with insertion, we can remove a node from the front of a list, remove a node from elsewhere. We’ll look at the technique for removing a node from the front of a linked list. 68
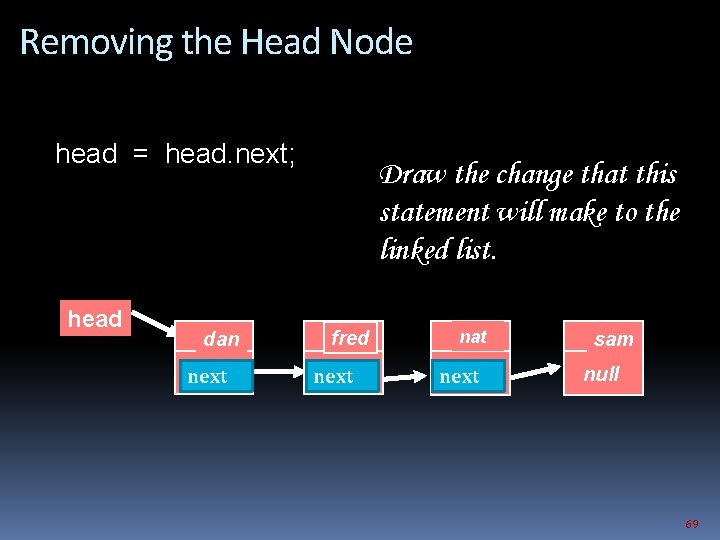
Removing the Head Node head = head. next; head dan next Draw the change that this statement will make to the linked list. fred next nat next sam null 69
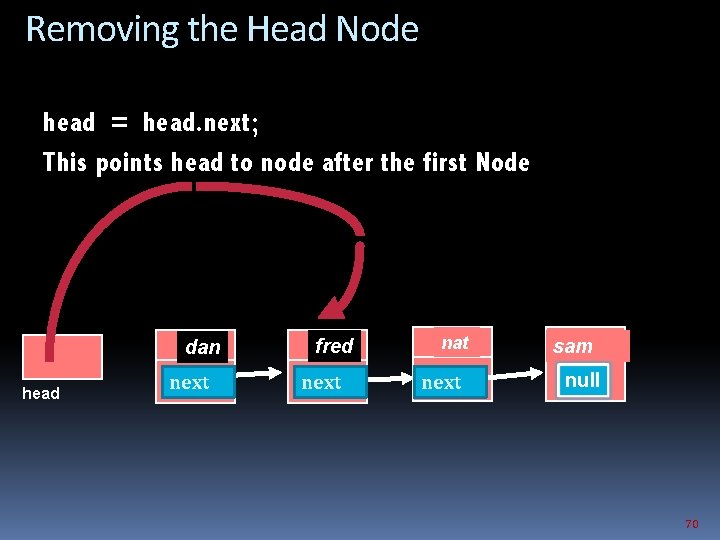
Removing the Head Node head = head. next; This points head to node after the first Node dan head next fred next nat next sam null 70
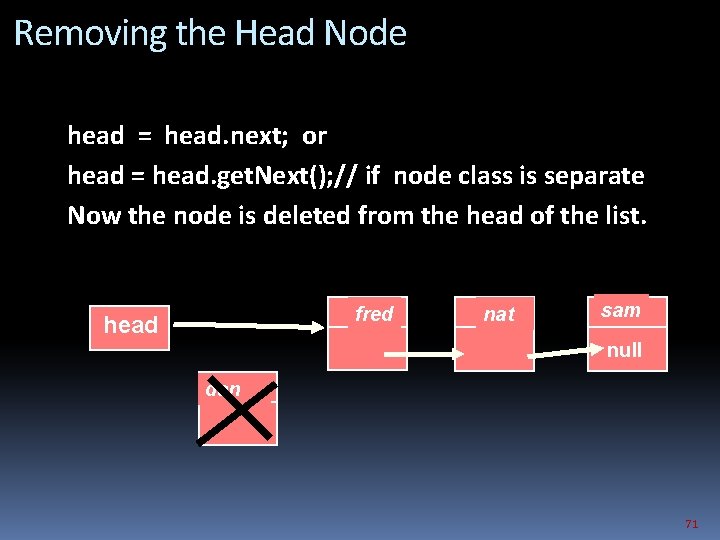
Removing the Head Node head = head. next; or head = head. get. Next(); // if node class is separate Now the node is deleted from the head of the list. fred head nat sam null dan 71
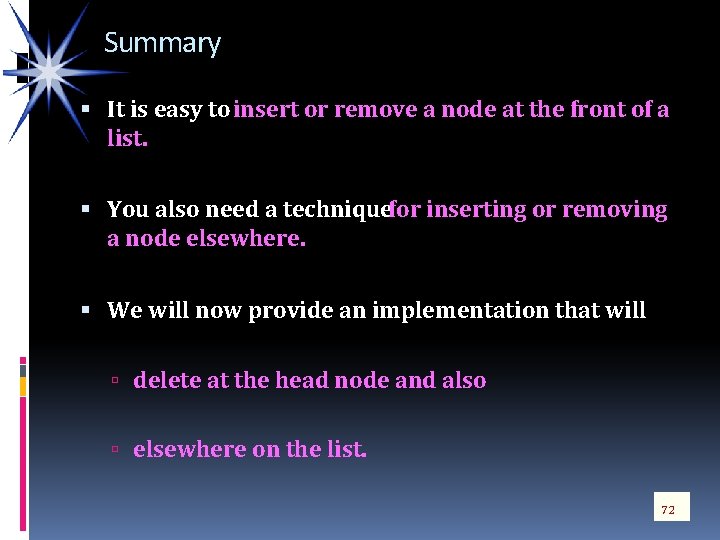
Summary It is easy to insert or remove a node at the front of a list. You also need a technique for inserting or removing a node elsewhere. We will now provide an implementation that will delete at the head node and also elsewhere on the list. 72
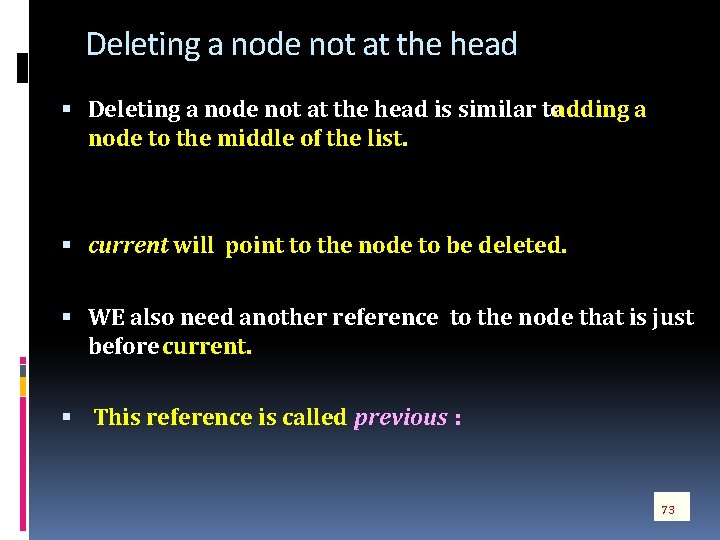
Deleting a node not at the head is similar to adding a node to the middle of the list. current will point to the node to be deleted. WE also need another reference to the node that is just before current. This reference is called previous : 73
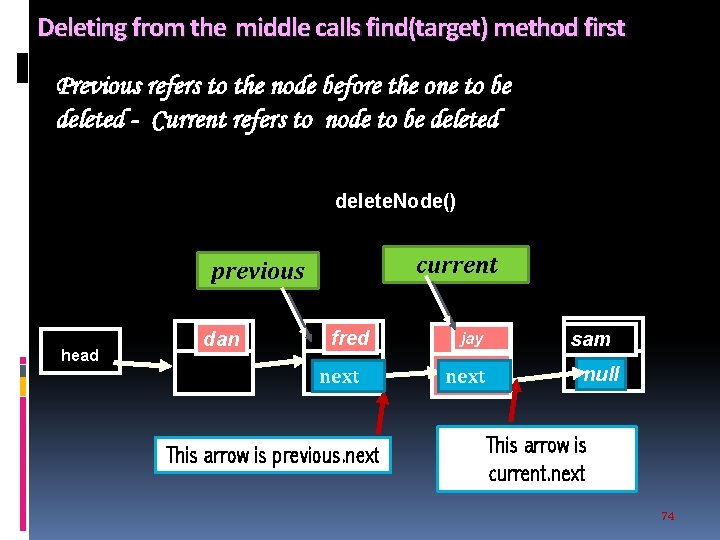
Deleting from the middle calls find(target) method first Previous refers to the node before the one to be deleted - Current refers to node to be deleted delete. Node() current previous head dan fred next This arrow is previous. next jay next sam null This arrow is current. next 74
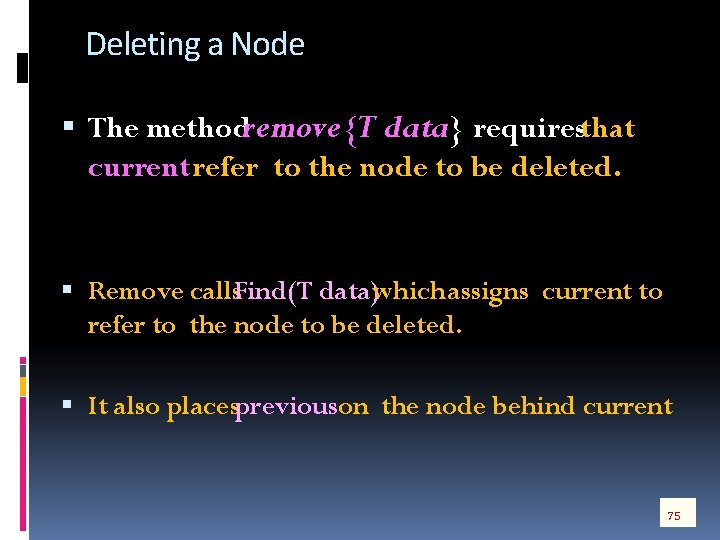
Deleting a Node The methodremove{T data} requiresthat current refer to the node to be deleted. Remove calls. Find(T data)which assigns current to refer to the node to be deleted. It also placespreviouson the node behind current 75
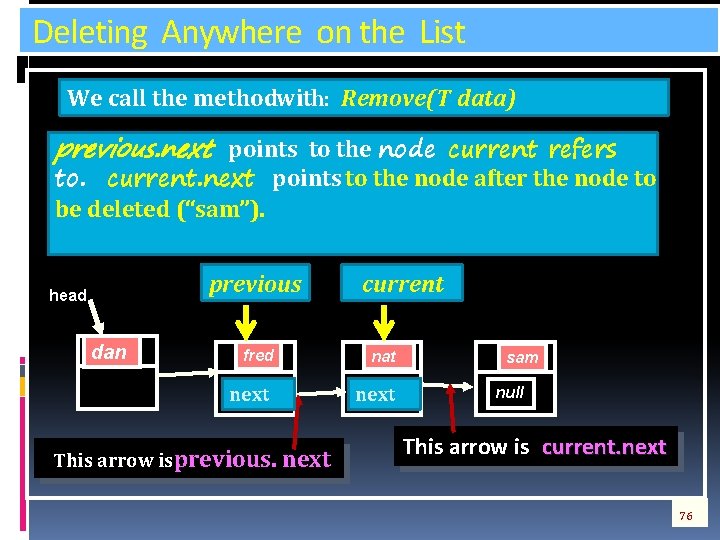
Deleting Anywhere on the List We call the method with: Remove(T data) previous. next points to the node current refers to. current. next points to the node after the node to be deleted (“sam”). previous head dan current fred nat next This arrow is previous. next sam null This arrow is current. next 76
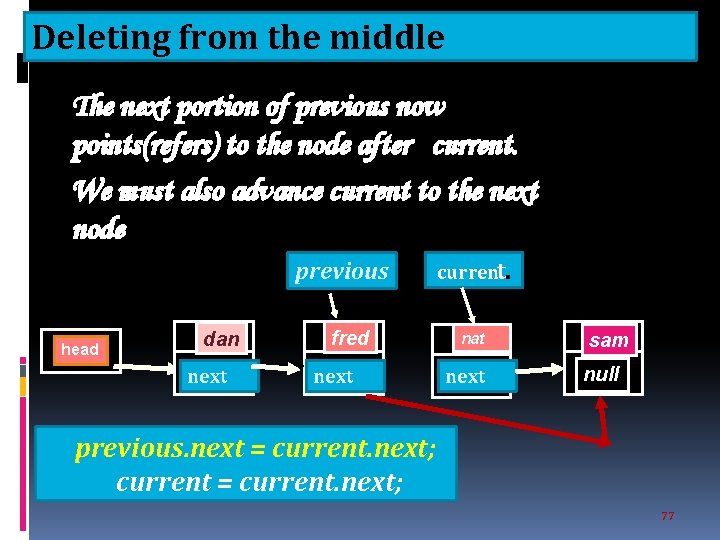
Deleting from the middle The next portion of previous now points(refers) to the node after current. We must also advance current to the next node previous head dan next fred next current. nat next sam null previous. next = current. next; current = current. next; 77
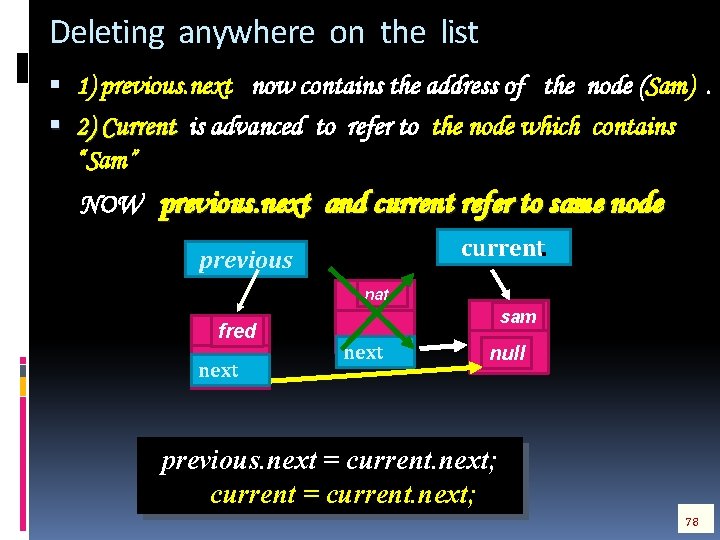
Deleting anywhere on the list 1) previous. next now contains the address of the node (Sam). 2) Current is advanced to refer to the node which contains “Sam” NOW previous. next and current refer to same node current. previous nat fred next sam next null previous. next = current. next; current = current. next; 78
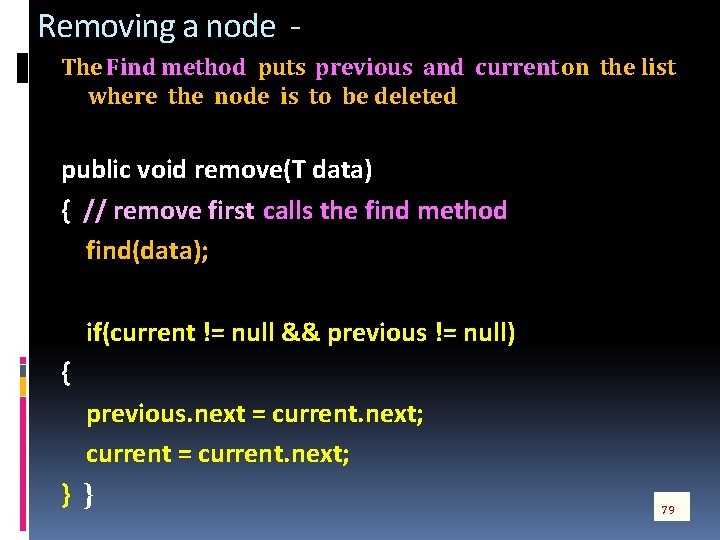
Removing a node The Find method puts previous and current on the list where the node is to be deleted public void remove(T data) { // remove first calls the find method find(data); if(current != null && previous != null) { previous. next = current. next; current = current. next; } } 79
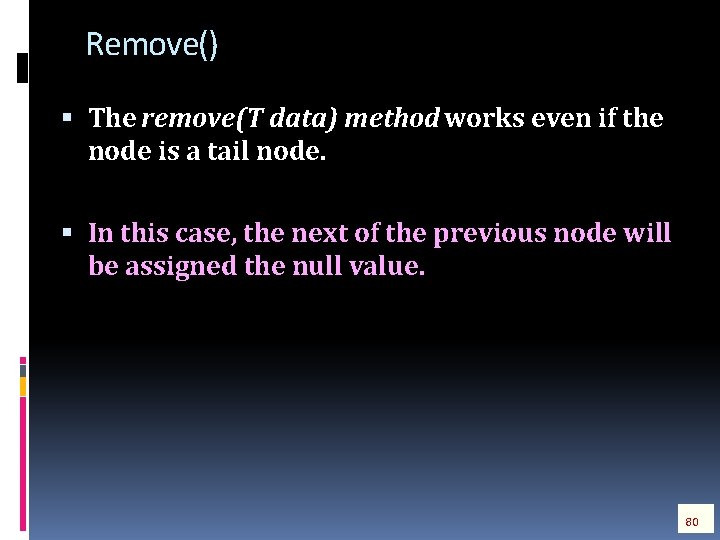
Remove() The remove(T data) method works even if the node is a tail node. In this case, the next of the previous node will be assigned the null value. 80
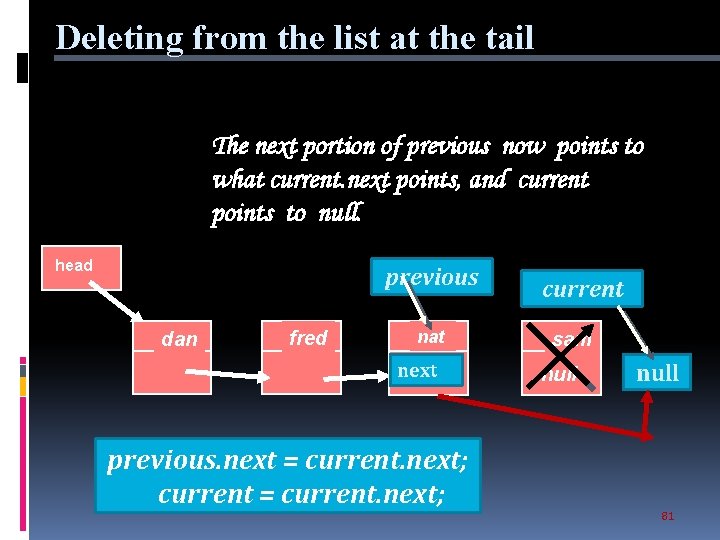
Deleting from the list at the tail The next portion of previous now points to what current. next points, and current points to null. head previous dan fred nat next previous. next = current. next; current = current. next; current sam null 81
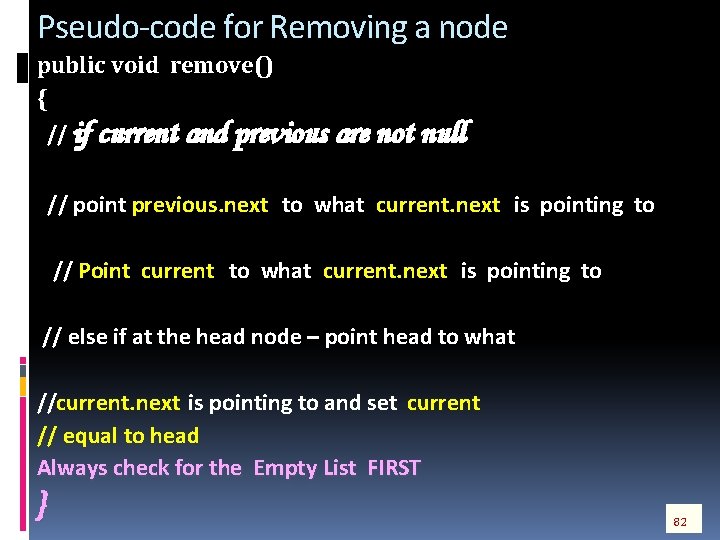
Pseudo-code for Removing a node public void remove() { // if current and previous are not null // point previous. next to what current. next is pointing to // Point current to what current. next is pointing to // else if at the head node – point head to what //current. next is pointing to and set current // equal to head Always check for the Empty List FIRST } 82
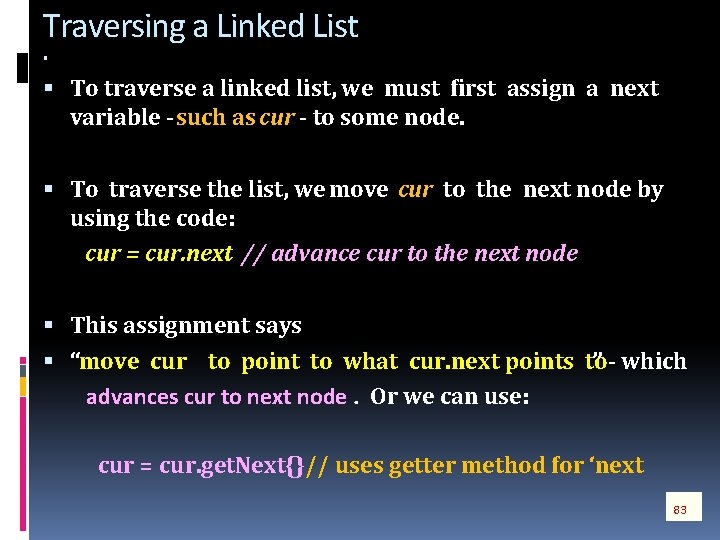
Traversing a Linked List To traverse a linked list, we must first assign a next variable - such as cur - to some node. To traverse the list, we move cur to the next node by using the code: cur = cur. next // advance cur to the next node This assignment says “move cur to point to what cur. next points to ” - which advances cur to next node. Or we can use: cur = cur. get. Next{} // uses getter method for ‘next’ 83
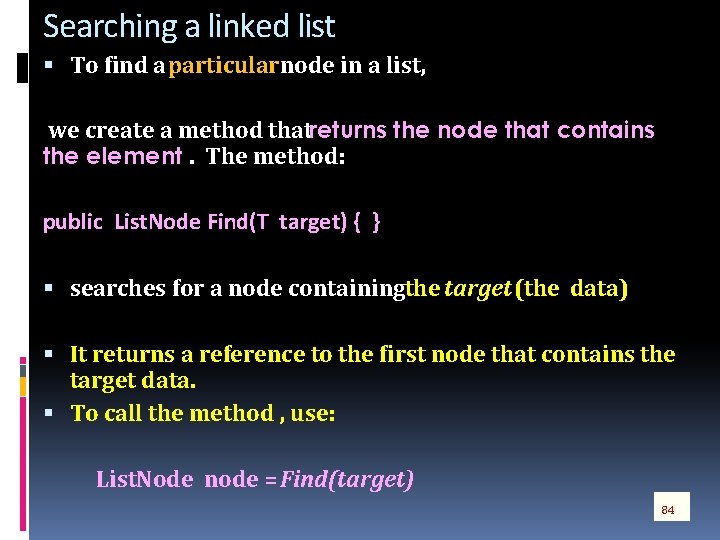
Searching a linked list To find a particular node in a list, we create a method that returns the node that contains the element. The method: public List. Node Find(T target) { } searches for a node containing the target (the data) It returns a reference to the first node that contains the target data. To call the method , use: List. Node node = Find(target) 84
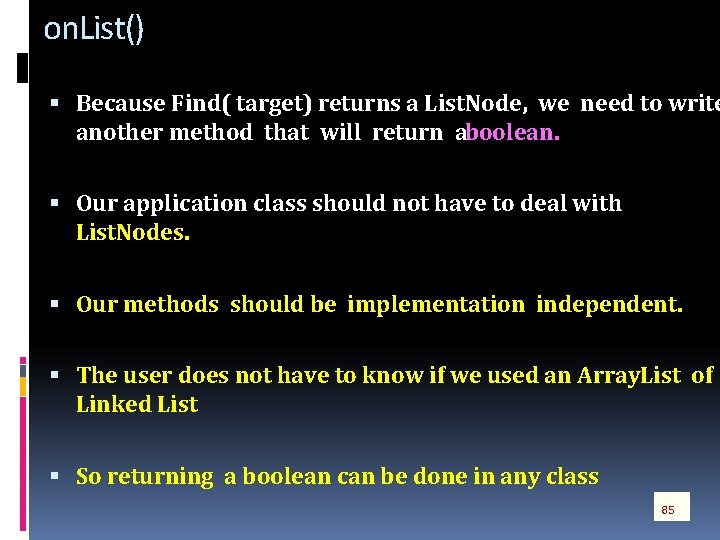
on. List() Because Find( target) returns a List. Node, we need to write another method that will return a boolean. Our application class should not have to deal with List. Nodes. Our methods should be implementation independent. The user does not have to know if we used an Array. List of Linked List So returning a boolean can be done in any class 85
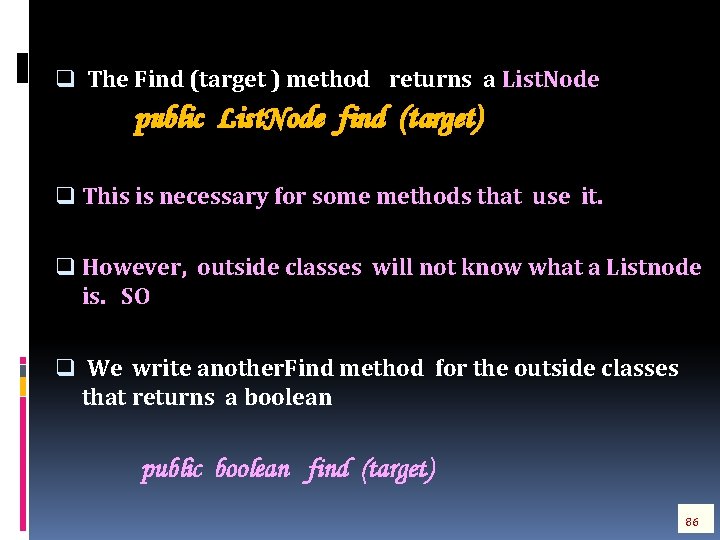
q The Find (target ) method returns a List. Node public List. Node find (target) q This is necessary for some methods that use it. q However, outside classes will not know what a Listnode is. SO q We write another Find method for the outside classes that returns a boolean public boolean find (target) 86
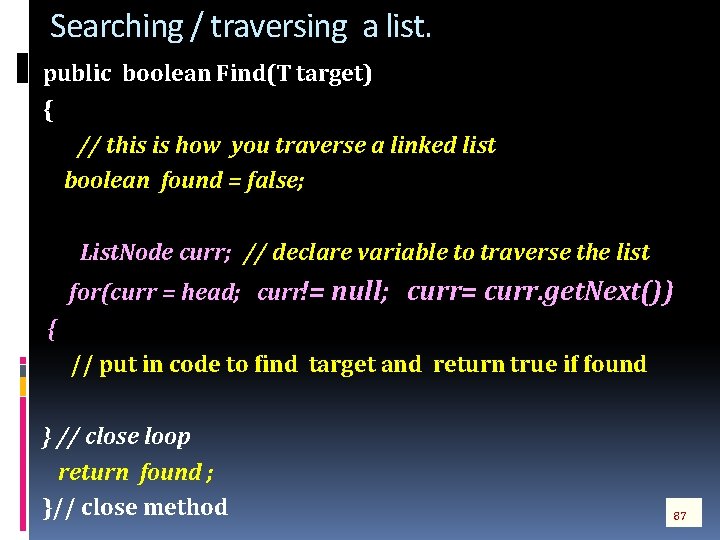
Searching / traversing a list. public boolean Find(T target) { // this is how you traverse a linked list boolean found = false; List. Node curr; // declare variable to traverse the list for(curr = head; curr!= null; curr= curr. get. Next()) { // put in code to find target and return true if found } // close loop return found ; }// close method 87
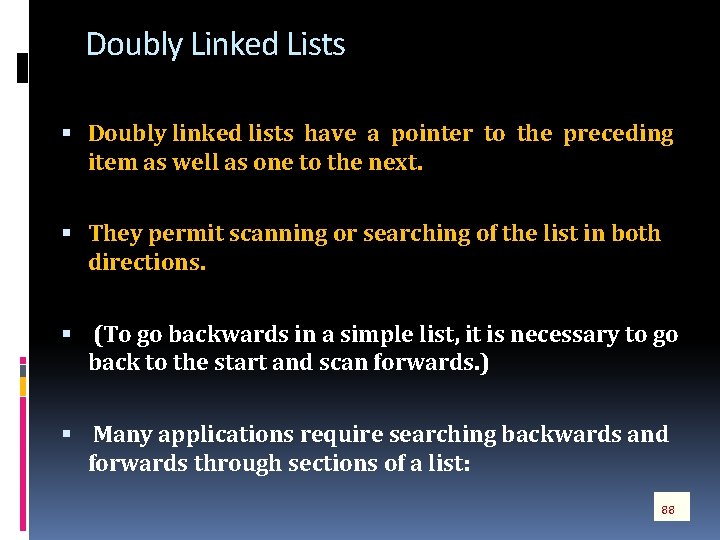
Doubly Linked Lists Doubly linked lists have a pointer to the preceding item as well as one to the next. They permit scanning or searching of the list in both directions. (To go backwards in a simple list, it is necessary to go back to the start and scan forwards. ) Many applications require searching backwards and forwards through sections of a list: 88
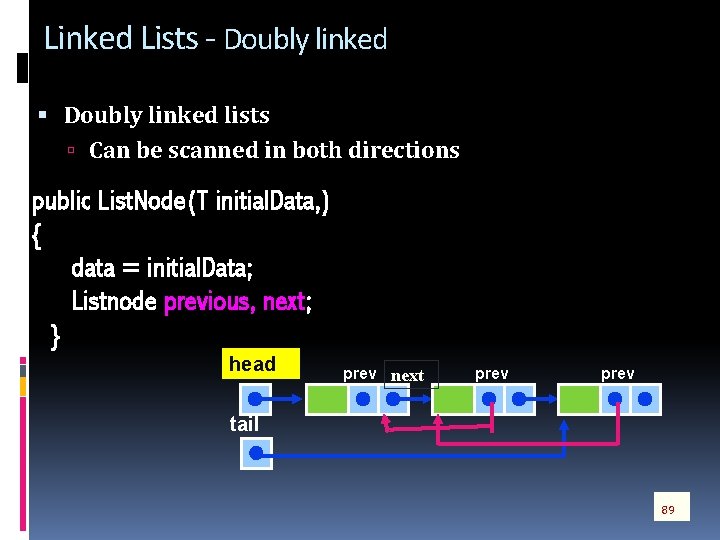
Linked Lists - Doubly linked lists Can be scanned in both directions public List. Node(T initial. Data, ) { data = initial. Data; Listnode previous, next; } head prev next prev tail 89
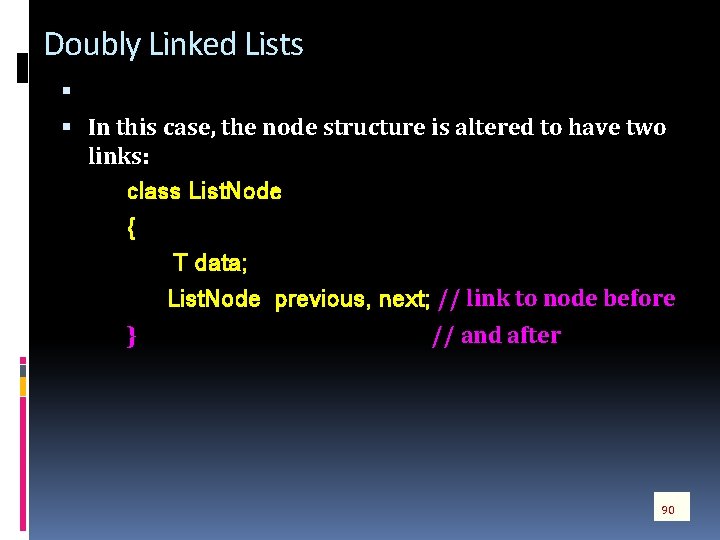
Doubly Linked Lists In this case, the node structure is altered to have two links: class List. Node { T data; List. Node previous, next; // link to node before } // and after 90
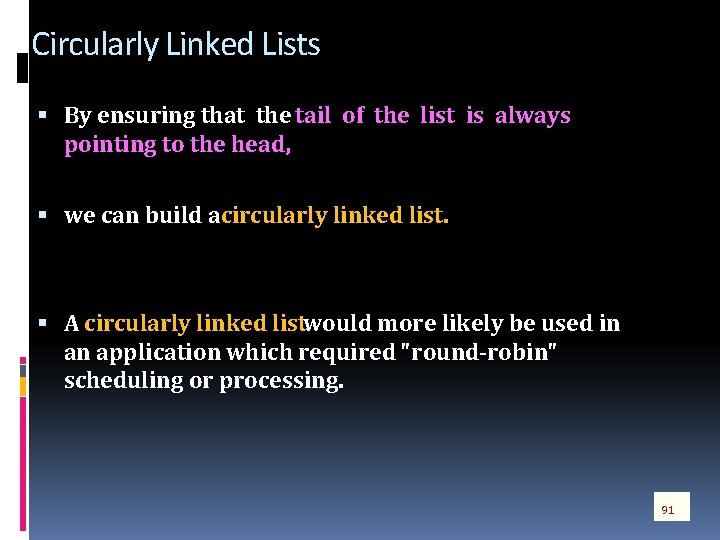
Circularly Linked Lists By ensuring that the tail of the list is always pointing to the head, we can build a circularly linked list. A circularly linked list would more likely be used in an application which required "round-robin" scheduling or processing. 91
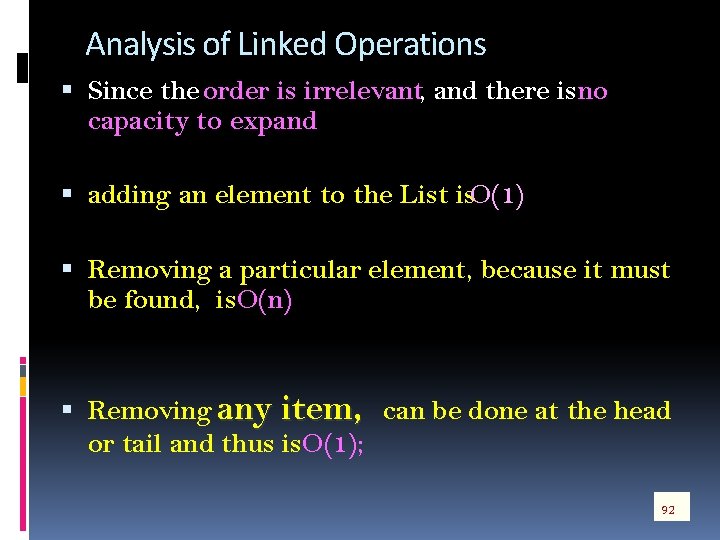
Analysis of Linked Operations Since the order is irrelevant, and there is no capacity to expand adding an element to the List is. O(1) Removing a particular element, because it must be found, is. O(n) Removing any item, can be done at the head or tail and thus is. O(1); 92
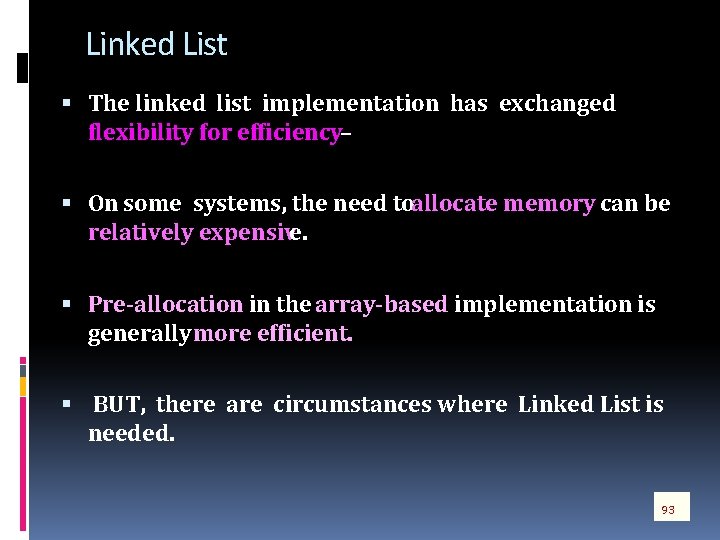
Linked List The linked list implementation has exchanged flexibility for efficiency – On some systems, the need to allocate memory can be relatively expensive. Pre-allocation in the array-based implementation is generally more efficient. BUT, there are circumstances where Linked List is needed. 93
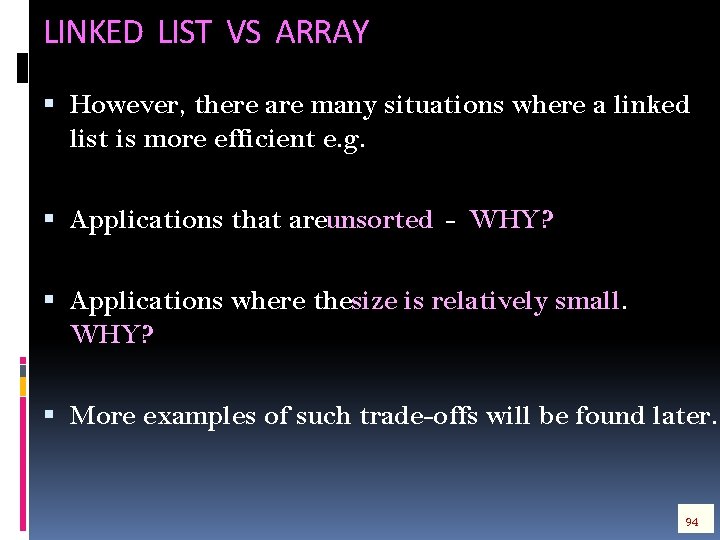
LINKED LIST VS ARRAY However, there are many situations where a linked list is more efficient e. g. Applications that areunsorted - WHY? Applications where thesize is relatively small. WHY? More examples of such trade-offs will be found later. 94
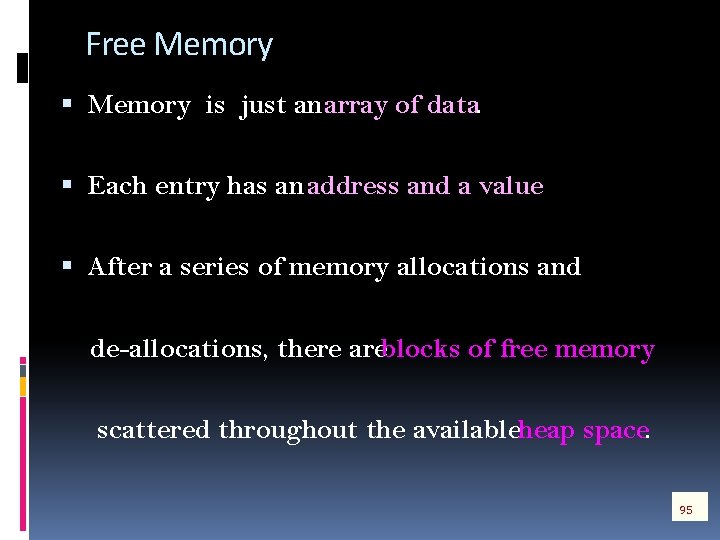
Free Memory is just an array of data. Each entry has an address and a value After a series of memory allocations and de-allocations, there areblocks of free memory scattered throughout the availableheap space. 95
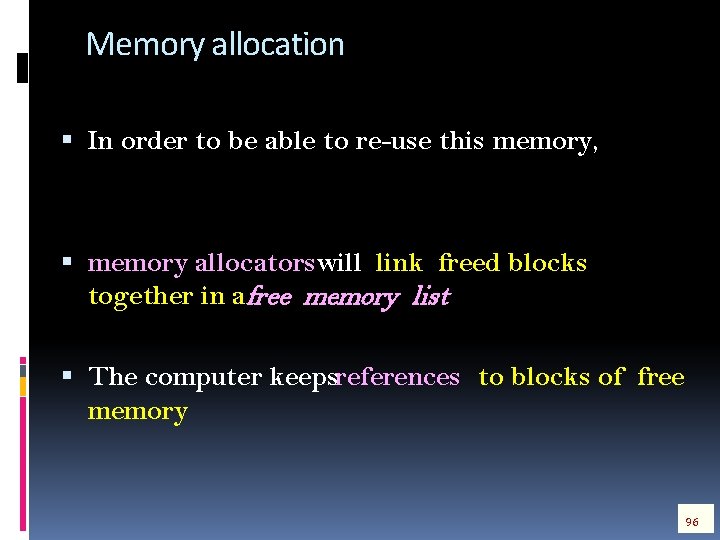
Memory allocation In order to be able to re-use this memory, memory allocatorswill link freed blocks together in a free memory list The computer keepsreferences to blocks of free memory 96
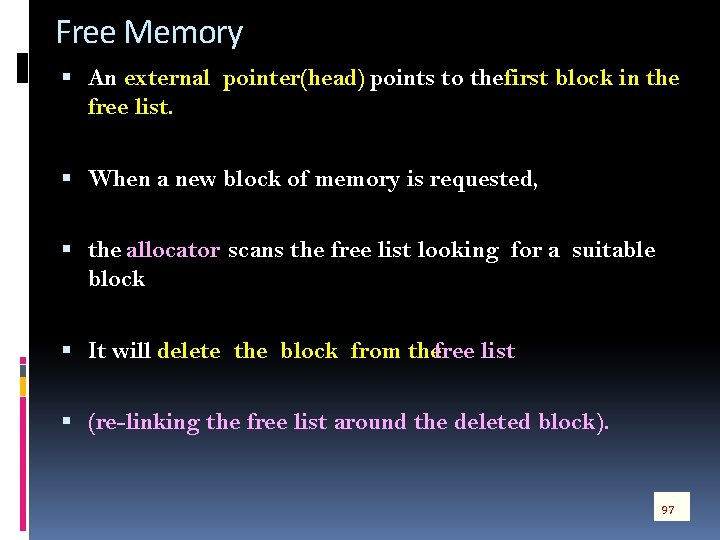
Free Memory An external pointer(head) points to thefirst block in the free list. When a new block of memory is requested, the allocator scans the free list looking for a suitable block It will delete the block from thefree list (re-linking the free list around the deleted block). 97
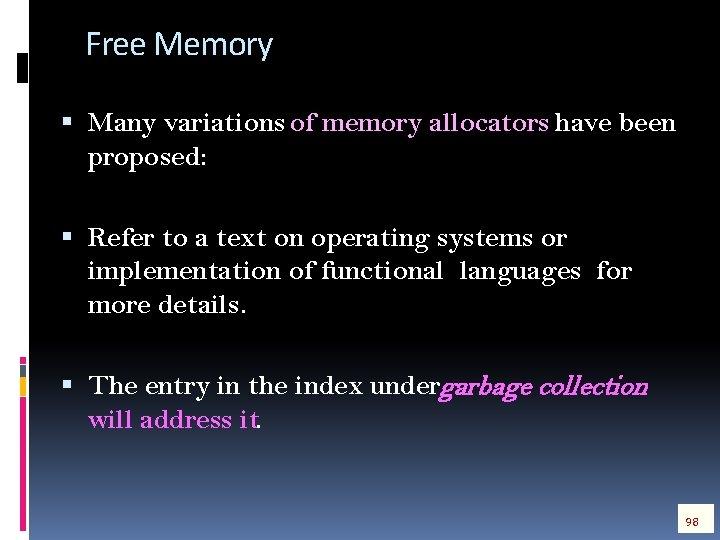
Free Memory Many variations of memory allocators have been proposed: Refer to a text on operating systems or implementation of functional languages for more details. The entry in the index undergarbage collection will address it. 98
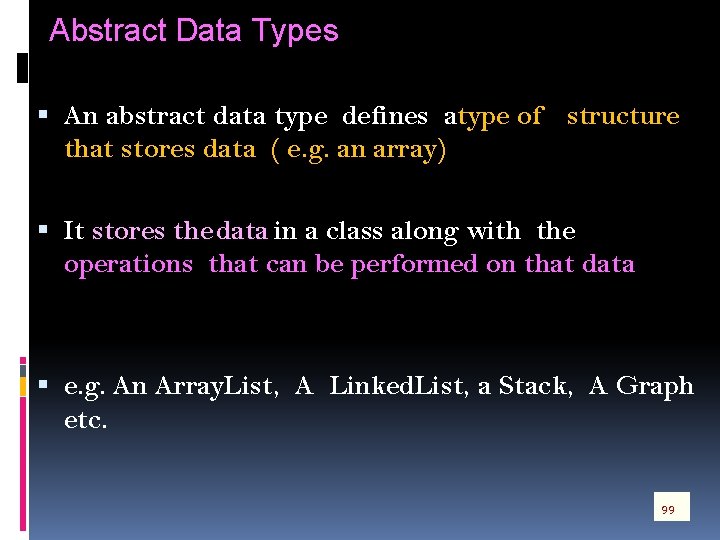
Abstract Data Types An abstract data type defines atype of structure that stores data ( e. g. an array) It stores the data in a class along with the operations that can be performed on that data e. g. An Array. List, A Linked. List, a Stack, A Graph etc. 99
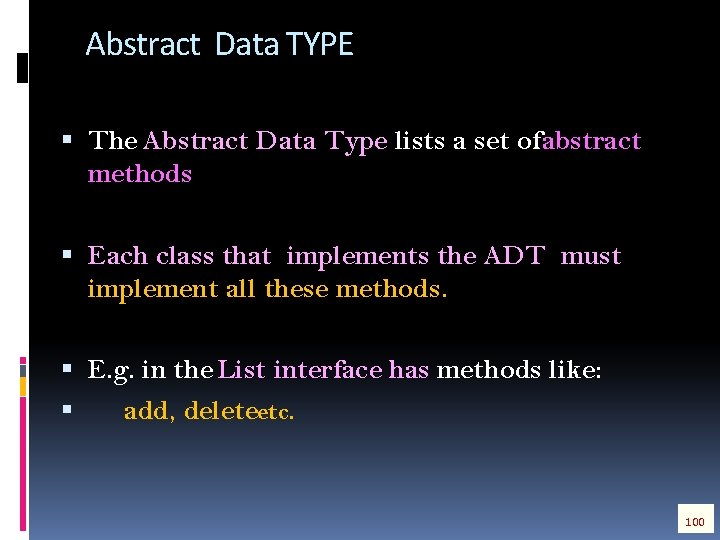
Abstract Data TYPE The Abstract Data Type lists a set ofabstract methods Each class that implements the ADT must implement all these methods. E. g. in the List interface has methods like: add, deleteetc. 100
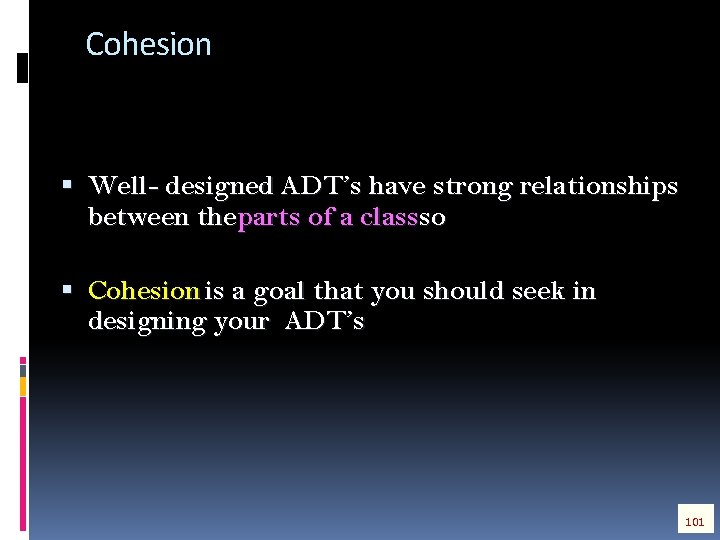
Cohesion Well- designed ADT’s have strong relationships between the parts of a classso Cohesion is a goal that you should seek in designing your ADT’s 101
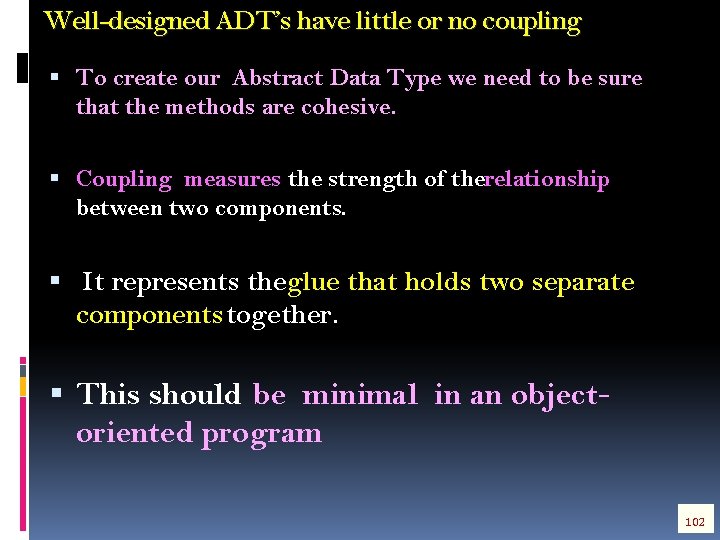
Well-designed ADT’s have little or no coupling To create our Abstract Data Type we need to be sure that the methods are cohesive. Coupling measures the strength of therelationship between two components. It represents theglue that holds two separate components together. This should be minimal in an objectoriented program 102
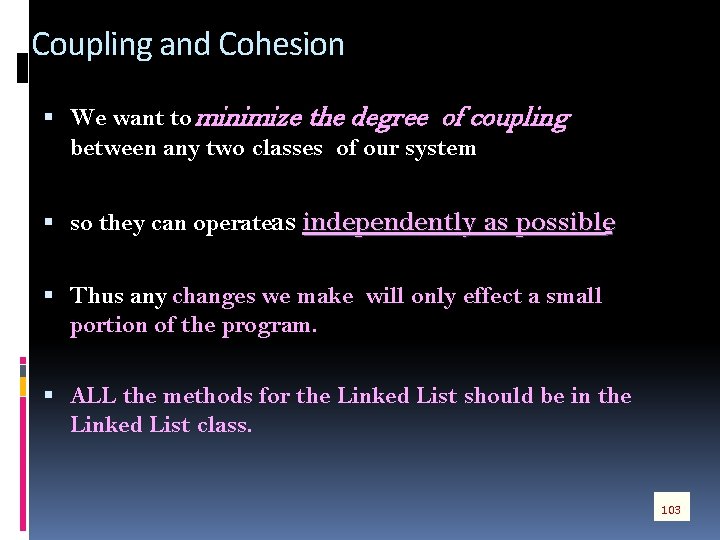
Coupling and Cohesion We want to minimize the degree of coupling between any two classes of our system so they can operateas independently as possible. Thus any changes we make will only effect a small portion of the program. ALL the methods for the Linked List should be in the Linked List class. 103
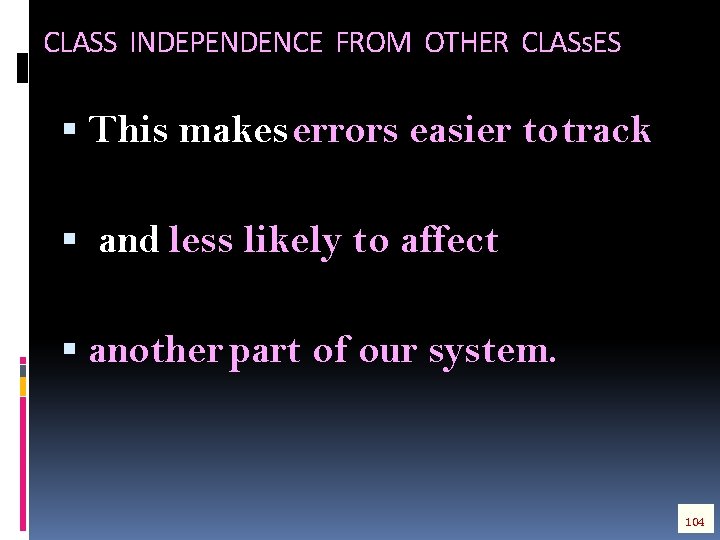
CLASS INDEPENDENCE FROM OTHER CLASs. ES This makes errors easier to track and less likely to affect another part of our system. 104
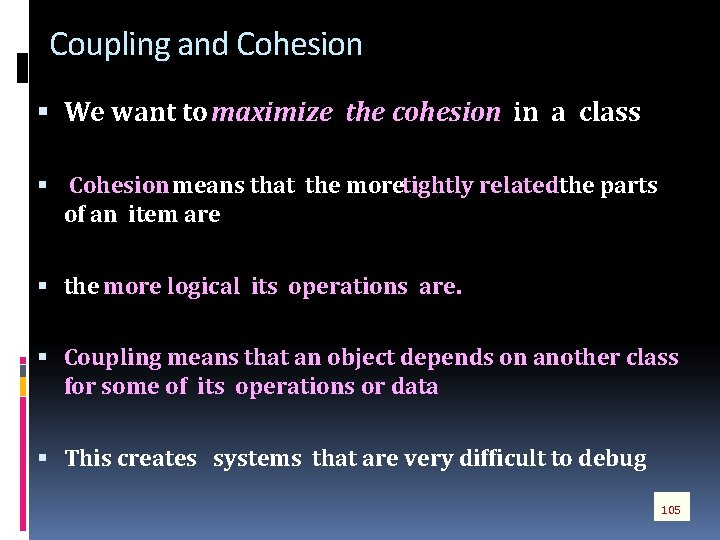
Coupling and Cohesion We want to maximize the cohesion in a class Cohesion means that the more tightly related the parts of an item are the more logical its operations are. Coupling means that an object depends on another class for some of its operations or data This creates systems that are very difficult to debug 105
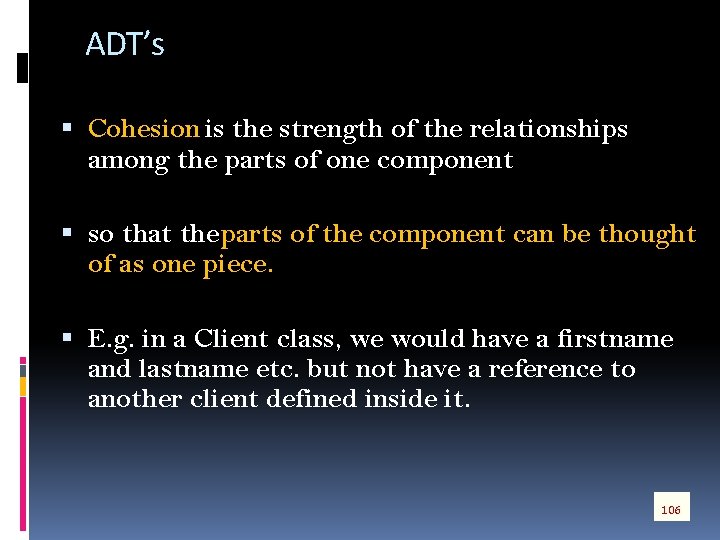
ADT’s Cohesion is the strength of the relationships among the parts of one component so that the parts of the component can be thought of as one piece. E. g. in a Client class, we would have a firstname and lastname etc. but not have a reference to another client defined inside it. 106
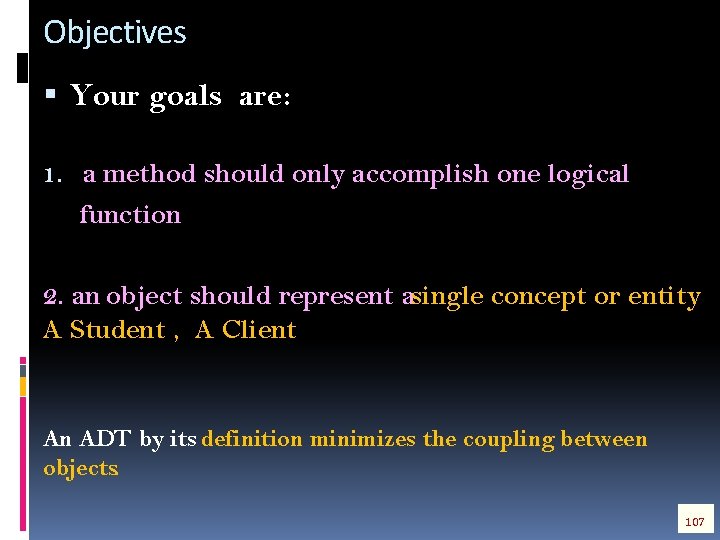
Objectives Your goals are: 1. a method should only accomplish one logical function 2. an object should represent asingle concept or entity. A Student , A Client An ADT by its definition minimizes the coupling between objects. 107
Singly vs doubly linked list
Introduction to linked list
Single linked list adalah yang paling dari semua varian
Exposition story elements
It introduces the characters and the setting
Morgan ts
The designer express the ideas in terms related to the
Pipeline interlock introduces a stall or bubble
Jeep/eagle introduces a new product
What is semicolon
Lesson 3: lists practice
Ow sound
Swst spelling test
Soft g words
Edinburgh resource lists
Python plot list of lists
Lists of tuples python
Lisp lists
Java types of lists
Words their way assessment
Read wrote inc
Prolog list of lists
Parallel structure in lists
List methods in python
Mesa verde ap world history
Wish lists year
Political lists new
Qri word lists
Words without vowels
Wish lists year
"new bookmarking lists 2018"
Https://www.diigo.com
Political wish lists
What is the spelling of gases
Ucl library reading lists
Swot analysis customer service example
Blockly lists
Medias res
5 stages of plot diagram
Short story with exposition
Suit the action to the word the word to the action meaning
Chapter 22 genetics and genetically linked diseases
Research action plan example
President appointment power
Basic accounting chapter 1
Example of assets liabilities and owner's equity
Accounting in action
Chapter 14 section 1 the growth of presidential power
X-linked dominant
Whats sex linked
Sex linked pedigree
Generic linked list java
Jenis linked list
Singly vs doubly linked list
X linked dominant punnett square
Is breast cancer autosomal or sexlinked
What are sexlinked traits
Sex linked chromosome punnett square
Whats sex linked
Linked genes
X linked punnett square
Sex limited traits example
Punnett square blood type
Aa x aa
Polynomial manipulation in data structure
Book bands linked to phonic phases
Is methemoglobinemia sex linked or autosomal
How to identify sex-linked traits from pedigree
X linked dominant pedigree
Sexlinked vs autosomal
Sex chromosome
Simple method cfd
Autosomal vs sex linked
X linked dominant punnett square
Market basket analysis
Xor linked list c++
C++ list
Linked list in mips
Definition of linked list
Linked list uml
Disadvantages of doubly linked list
Language
Apa itu circular linked list
Linked genes
Autosomal vs sex linked
Concurrent linked list
James lange theory of emotion vs schachter singer
What is a sex linked disorder
Linked data platform
Y linked disease
Linked genes and unlinked genes
Y linked disease
Fs club
Double linked list circular
List adt
Difference between linked list and queue
Obbligazioni strutturate credit linked
Circular linked list advantages
Linked list sentinel
Algorithm of linked list
Singly linked list in data structure
Priority queue linked list python
In fruit flies white eyes is a sex linked recessive trait
Public static void main
Multiple linked list
Linked list representation of disjoint sets
Polygenic inheritance
Assignment operator doubly linked list c++